Unit Testing with JUnit Dan Fleck Fall 2007
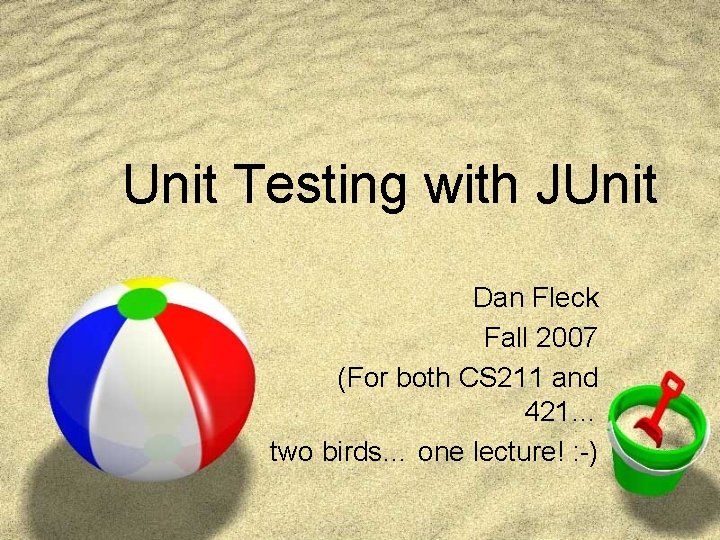
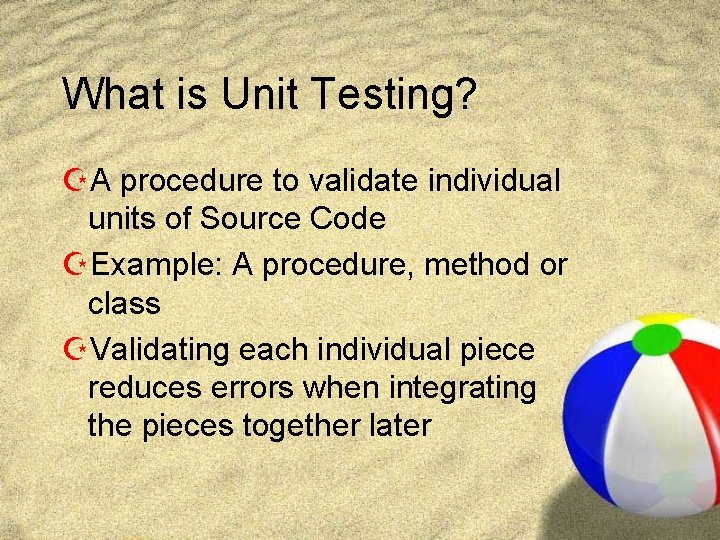
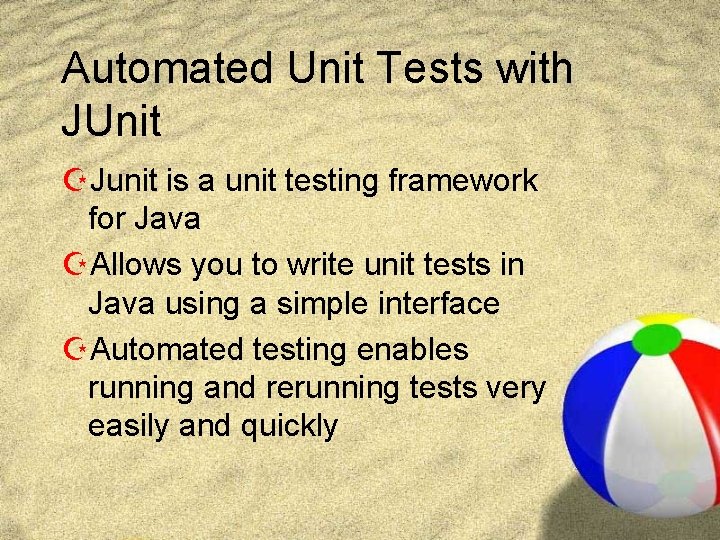
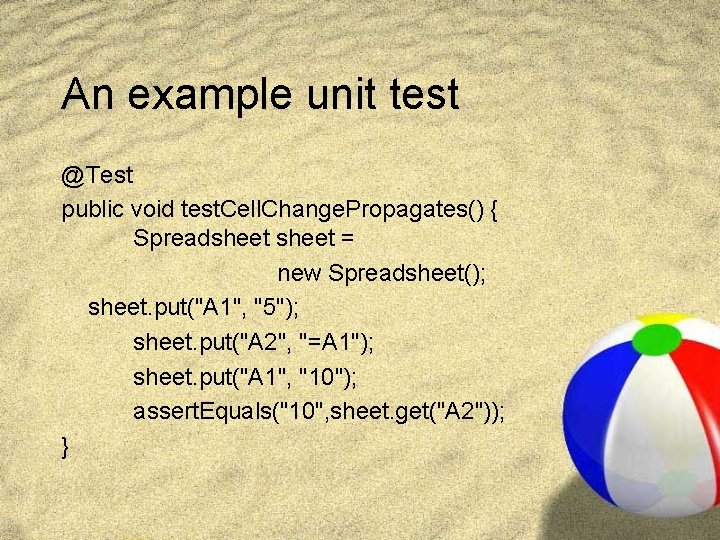
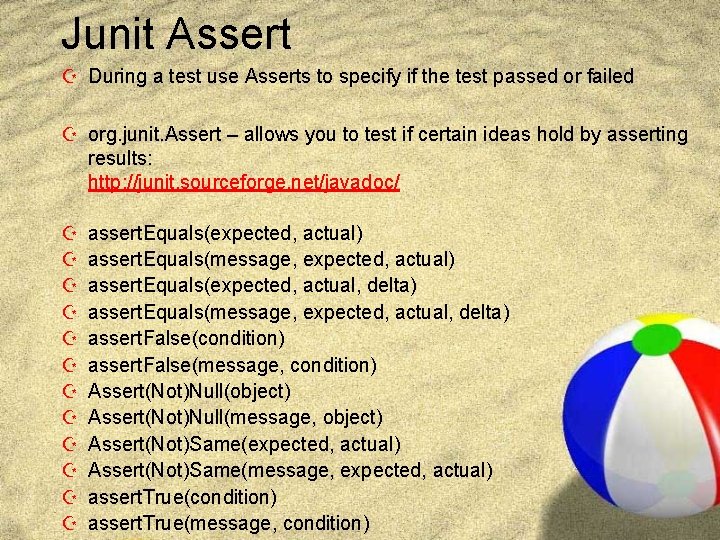
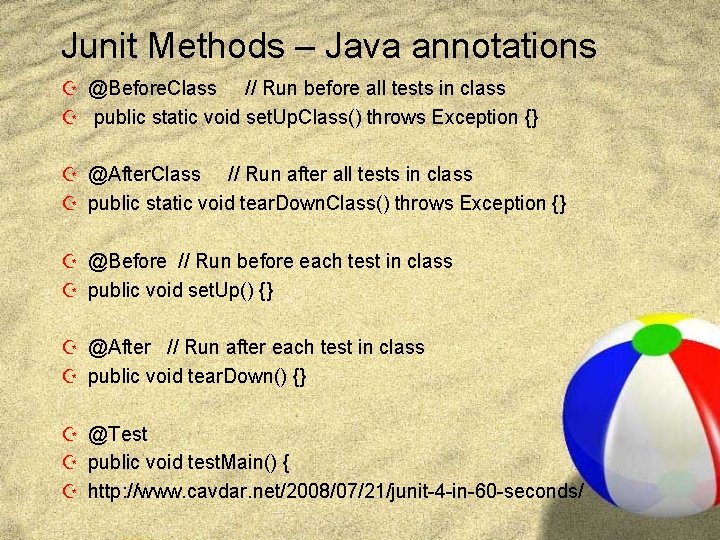
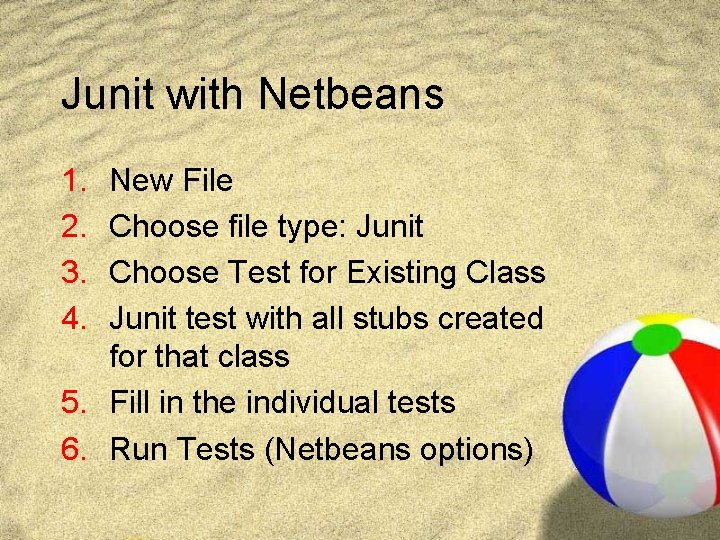
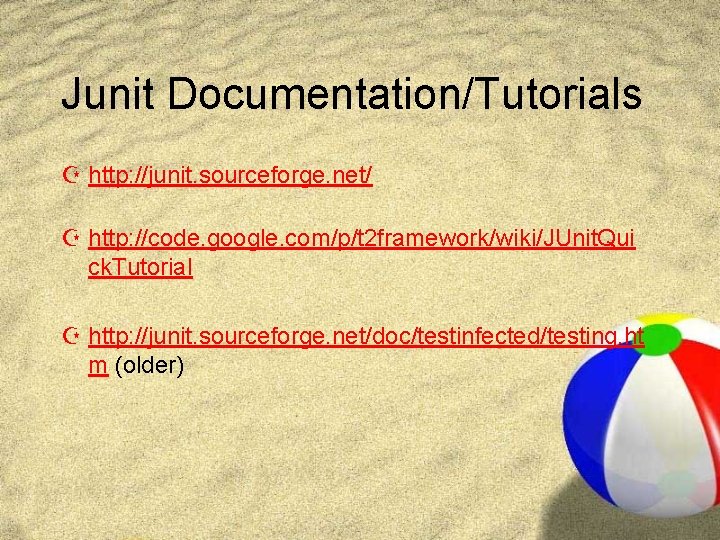
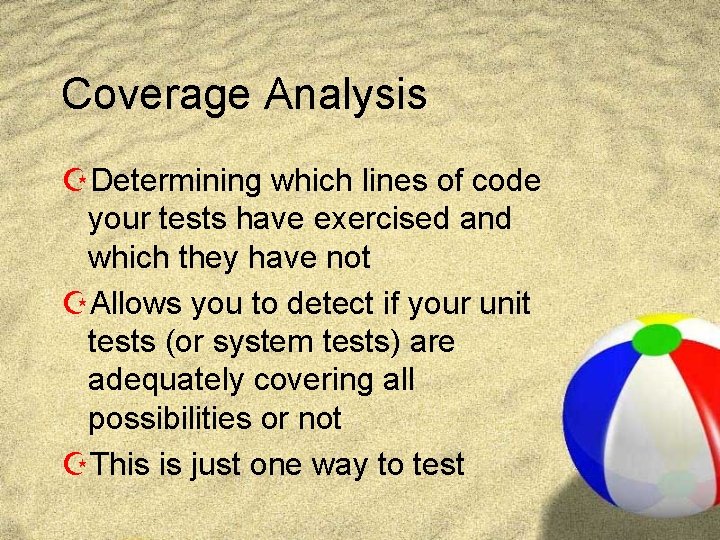
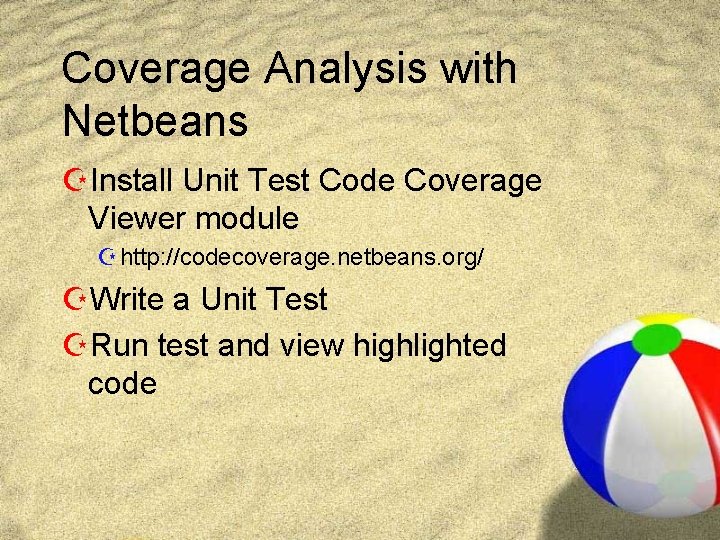
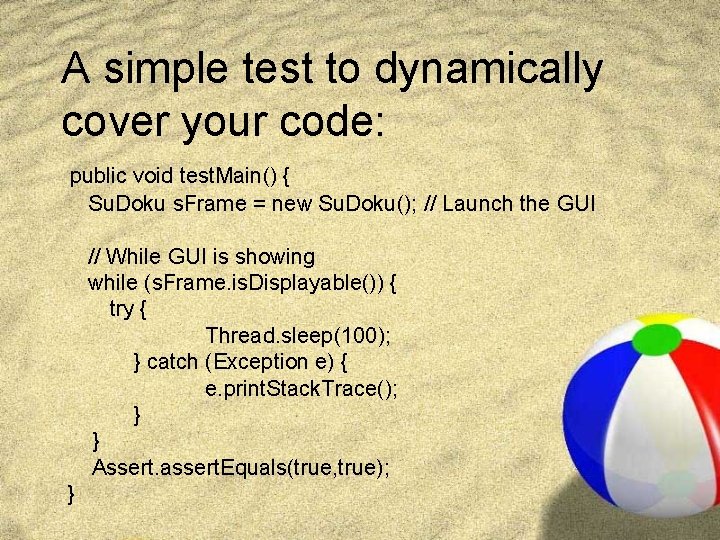
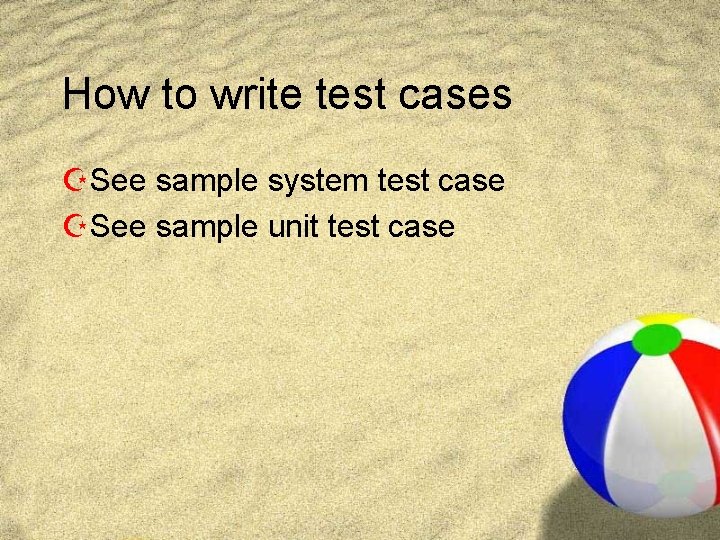
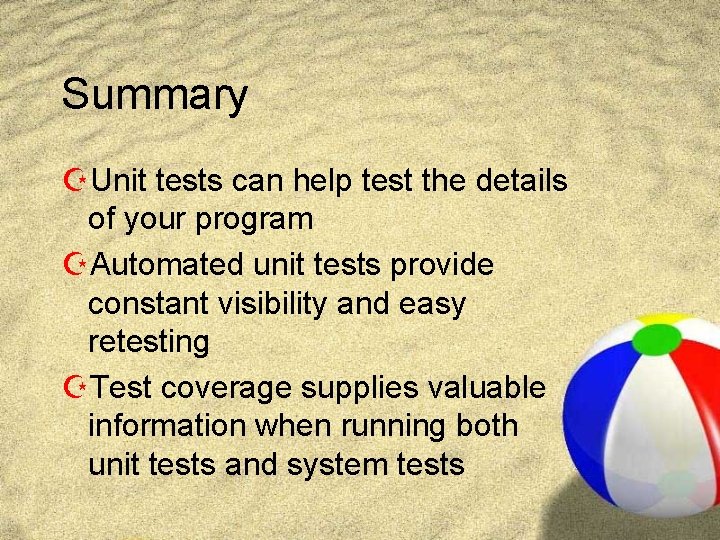
- Slides: 13
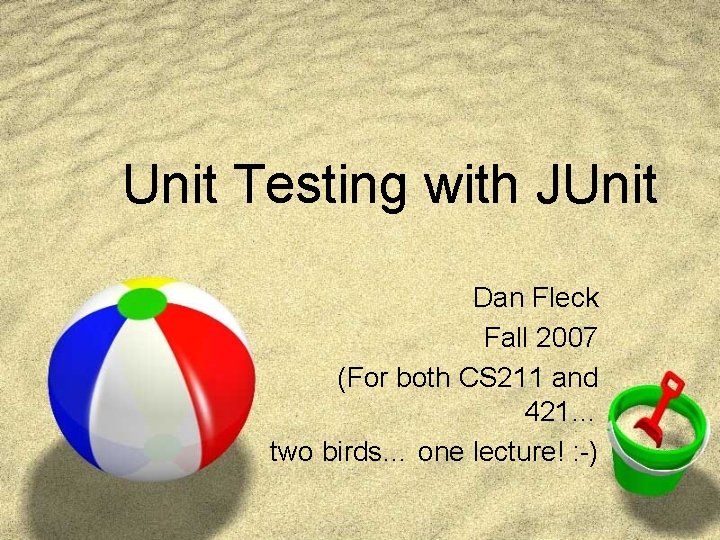
Unit Testing with JUnit Dan Fleck Fall 2007 (For both CS 211 and 421… two birds… one lecture! : -)
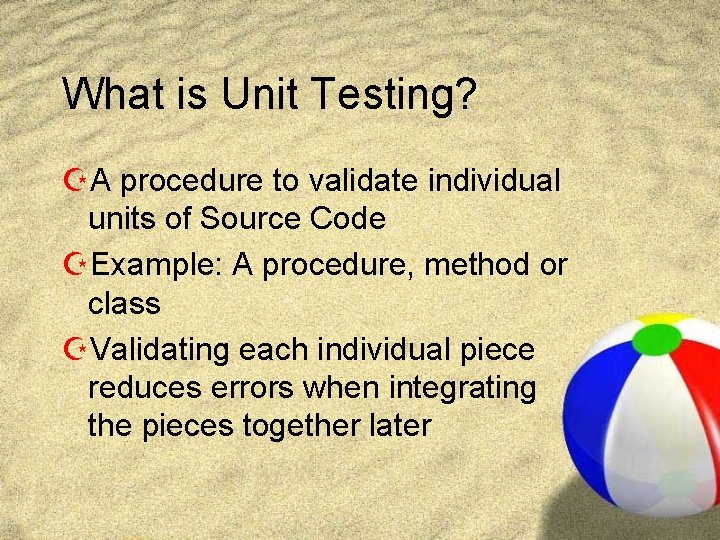
What is Unit Testing? ZA procedure to validate individual units of Source Code ZExample: A procedure, method or class ZValidating each individual piece reduces errors when integrating the pieces together later
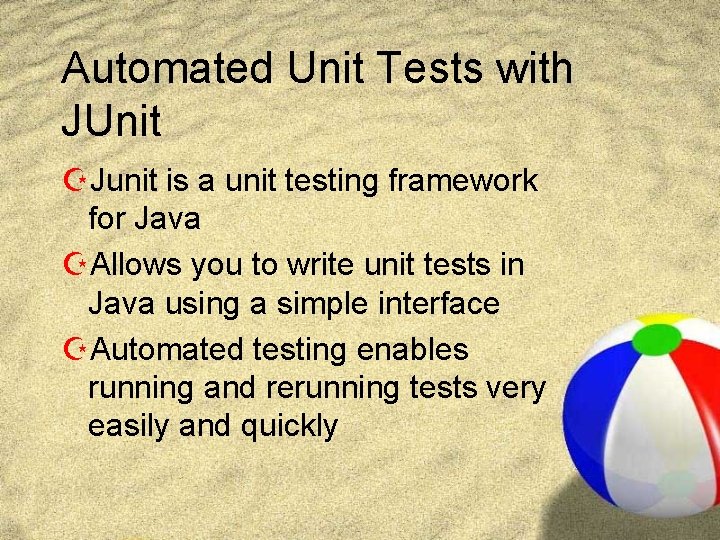
Automated Unit Tests with JUnit ZJunit is a unit testing framework for Java ZAllows you to write unit tests in Java using a simple interface ZAutomated testing enables running and rerunning tests very easily and quickly
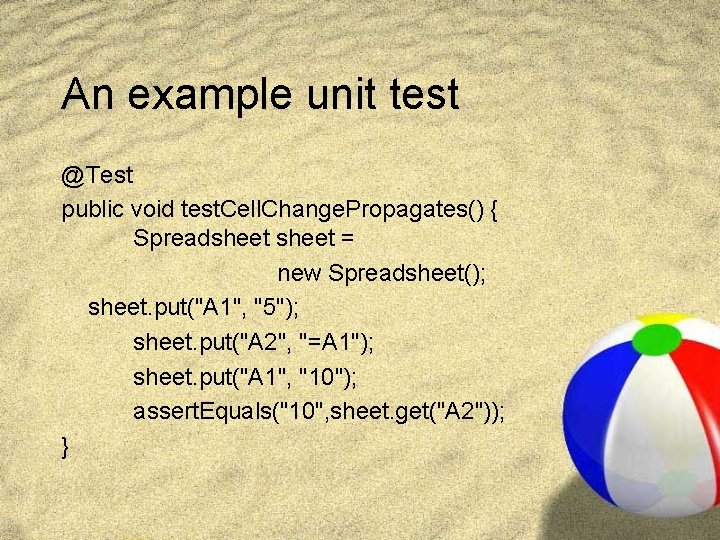
An example unit test @Test public void test. Cell. Change. Propagates() { Spreadsheet = new Spreadsheet(); sheet. put("A 1", "5"); sheet. put("A 2", "=A 1"); sheet. put("A 1", "10"); assert. Equals("10", sheet. get("A 2")); }
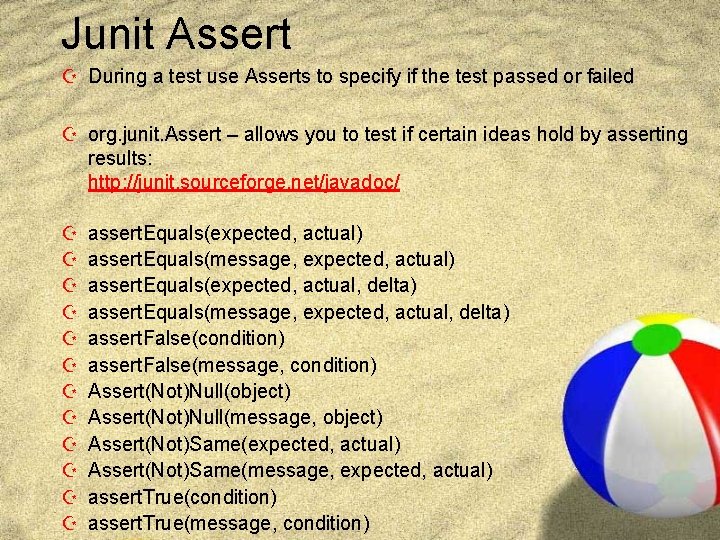
Junit Assert Z During a test use Asserts to specify if the test passed or failed Z org. junit. Assert – allows you to test if certain ideas hold by asserting results: http: //junit. sourceforge. net/javadoc/ Z Z Z assert. Equals(expected, actual) assert. Equals(message, expected, actual) assert. Equals(expected, actual, delta) assert. Equals(message, expected, actual, delta) assert. False(condition) assert. False(message, condition) Assert(Not)Null(object) Assert(Not)Null(message, object) Assert(Not)Same(expected, actual) Assert(Not)Same(message, expected, actual) assert. True(condition) assert. True(message, condition)
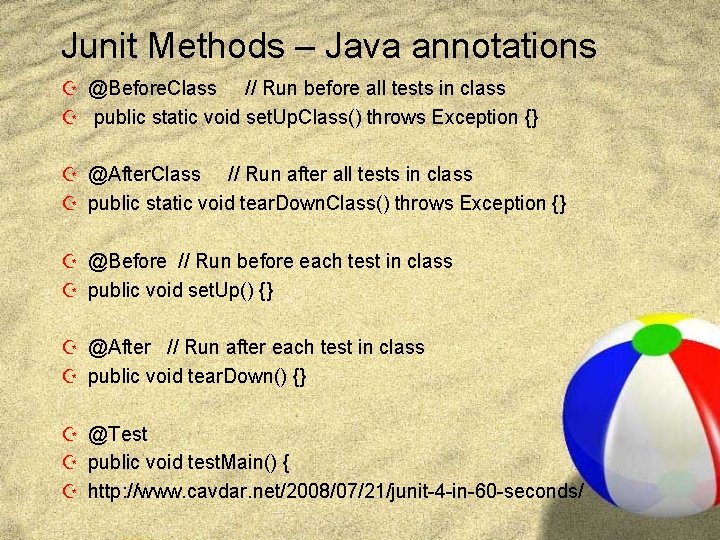
Junit Methods – Java annotations Z @Before. Class // Run before all tests in class Z public static void set. Up. Class() throws Exception {} Z @After. Class // Run after all tests in class Z public static void tear. Down. Class() throws Exception {} Z @Before // Run before each test in class Z public void set. Up() {} Z @After // Run after each test in class Z public void tear. Down() {} Z @Test Z public void test. Main() { Z http: //www. cavdar. net/2008/07/21/junit-4 -in-60 -seconds/
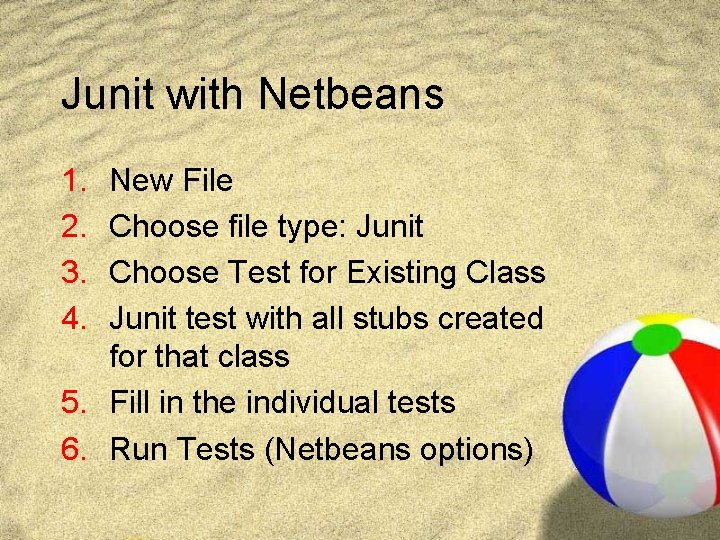
Junit with Netbeans 1. 2. 3. 4. New File Choose file type: Junit Choose Test for Existing Class Junit test with all stubs created for that class 5. Fill in the individual tests 6. Run Tests (Netbeans options)
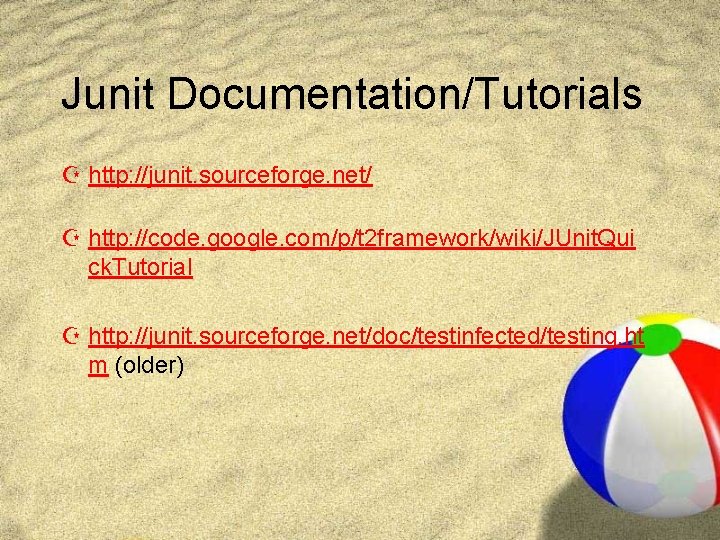
Junit Documentation/Tutorials Z http: //junit. sourceforge. net/ Z http: //code. google. com/p/t 2 framework/wiki/JUnit. Qui ck. Tutorial Z http: //junit. sourceforge. net/doc/testinfected/testing. ht m (older)
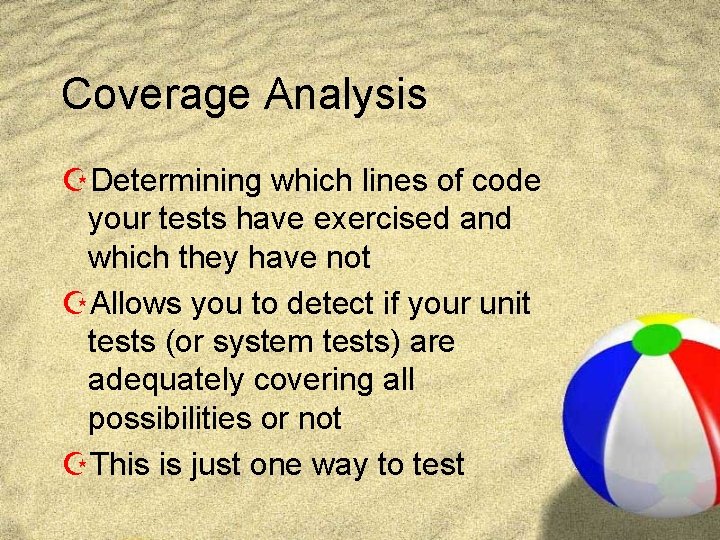
Coverage Analysis ZDetermining which lines of code your tests have exercised and which they have not ZAllows you to detect if your unit tests (or system tests) are adequately covering all possibilities or not ZThis is just one way to test
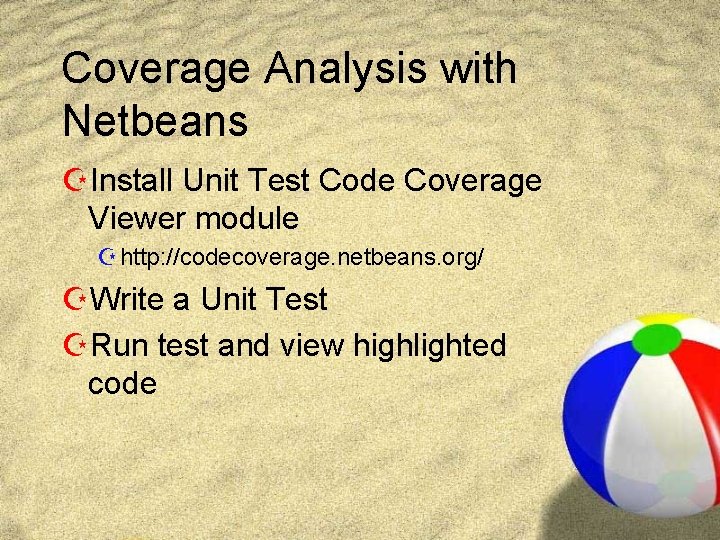
Coverage Analysis with Netbeans ZInstall Unit Test Code Coverage Viewer module Z http: //codecoverage. netbeans. org/ ZWrite a Unit Test ZRun test and view highlighted code
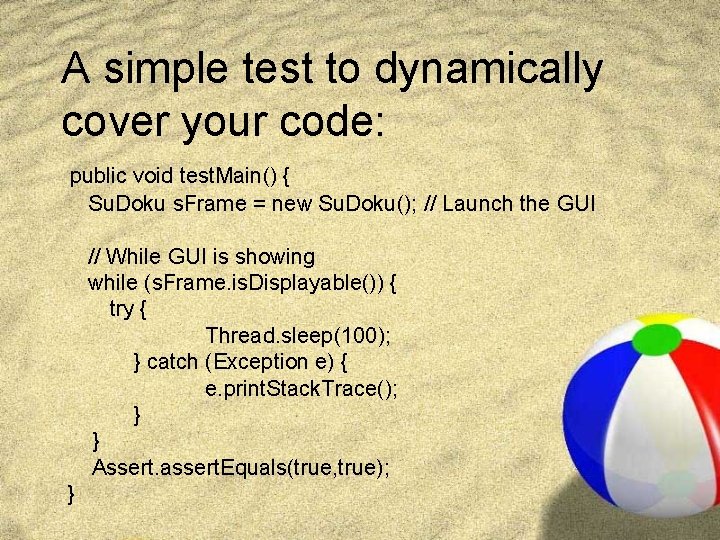
A simple test to dynamically cover your code: public void test. Main() { Su. Doku s. Frame = new Su. Doku(); // Launch the GUI // While GUI is showing while (s. Frame. is. Displayable()) { try { Thread. sleep(100); } catch (Exception e) { e. print. Stack. Trace(); } } Assert. assert. Equals(true, true); }
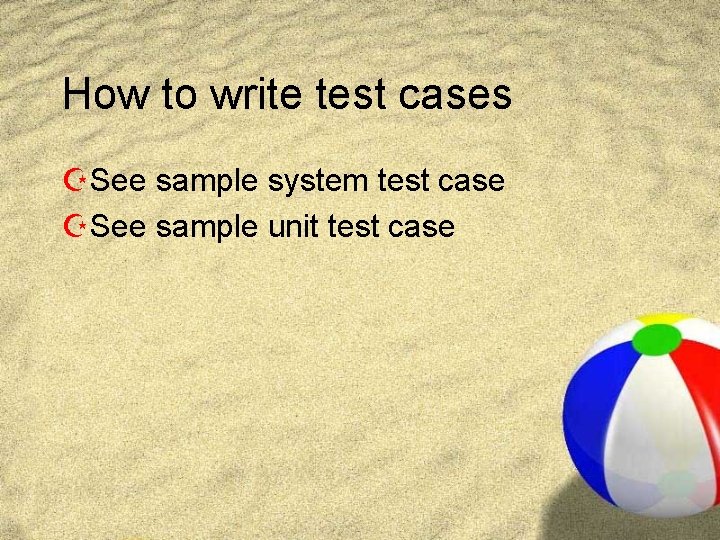
How to write test cases ZSee sample system test case ZSee sample unit test case
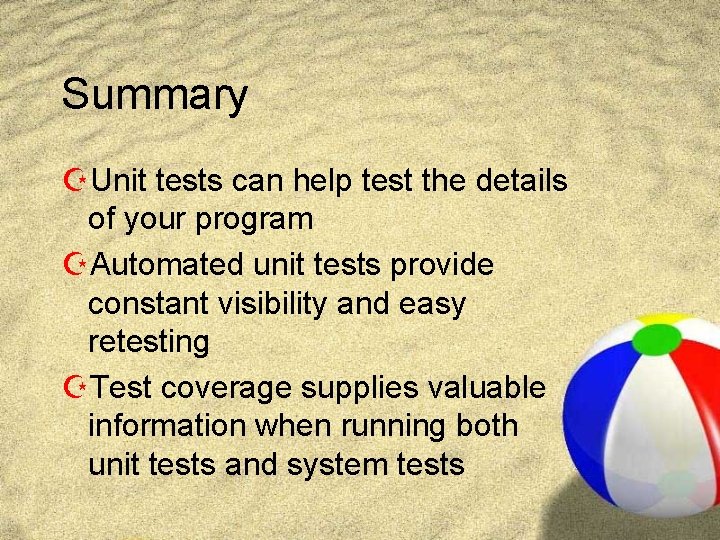
Summary ZUnit tests can help test the details of your program ZAutomated unit tests provide constant visibility and easy retesting ZTest coverage supplies valuable information when running both unit tests and system tests