Unit III Linked Lists Introduction Definitions Lists and
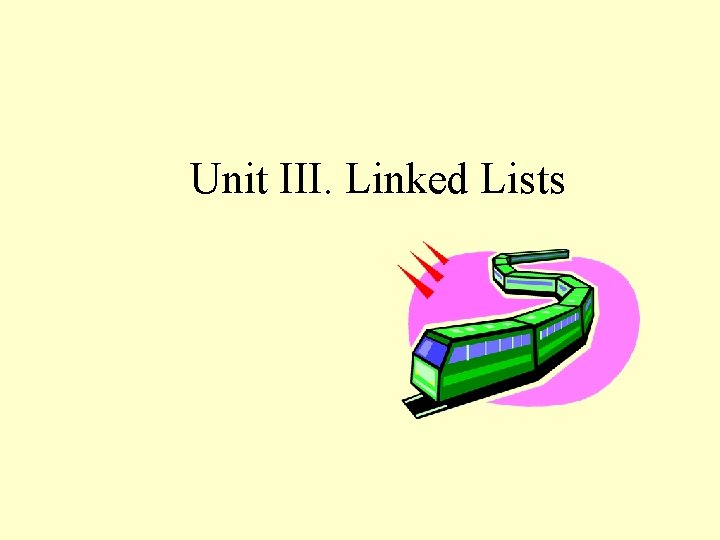
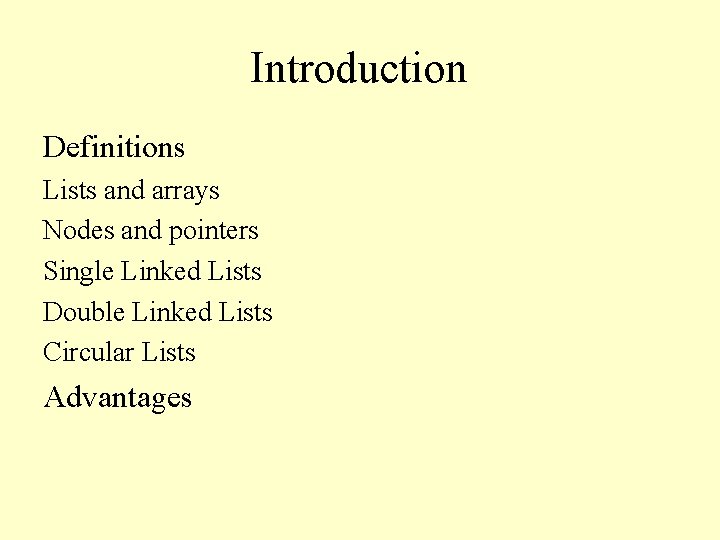
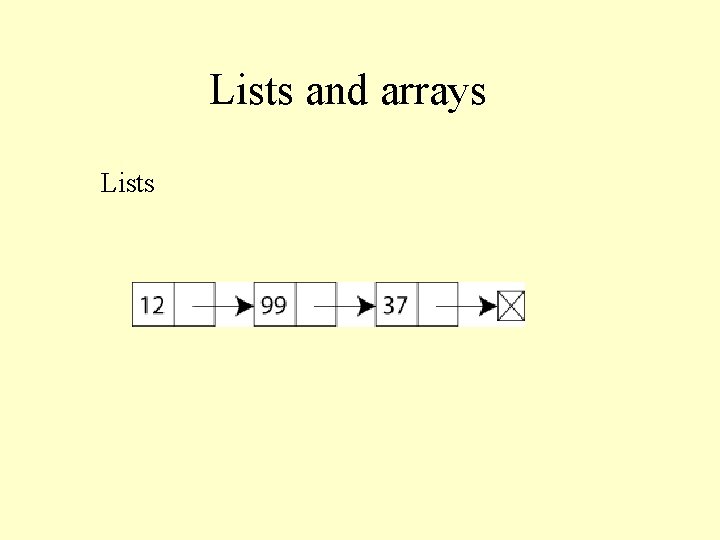
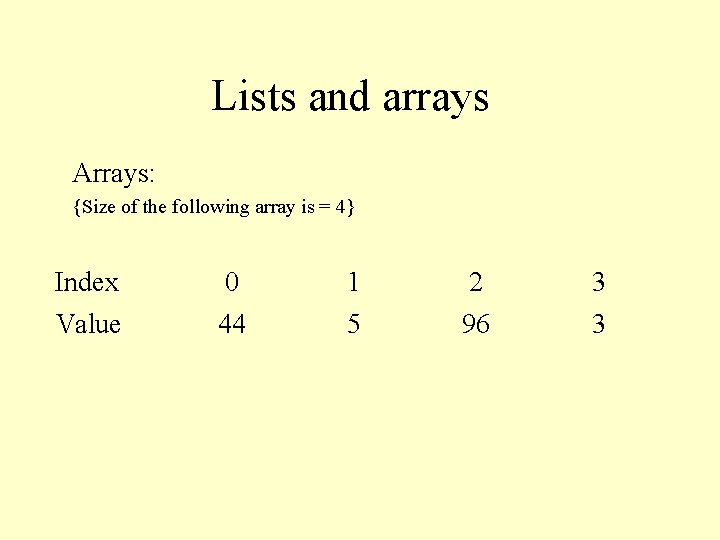
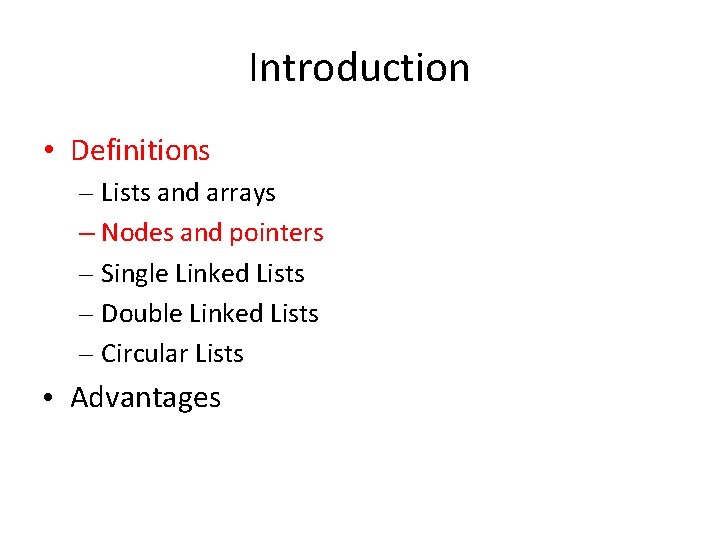
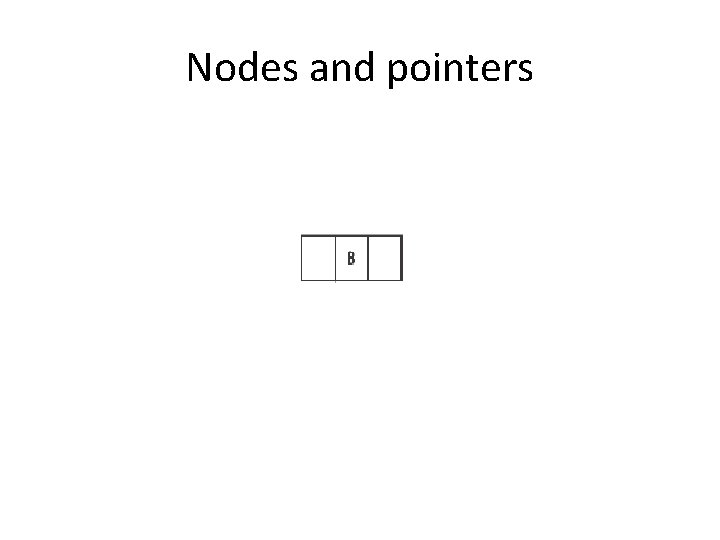
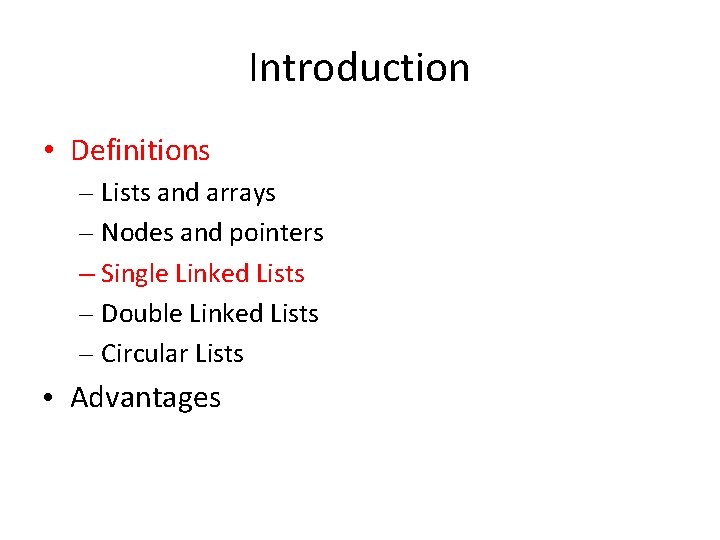
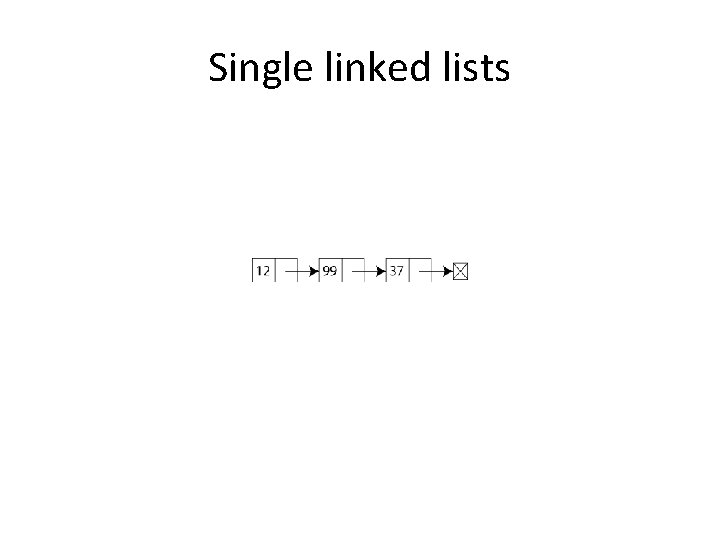
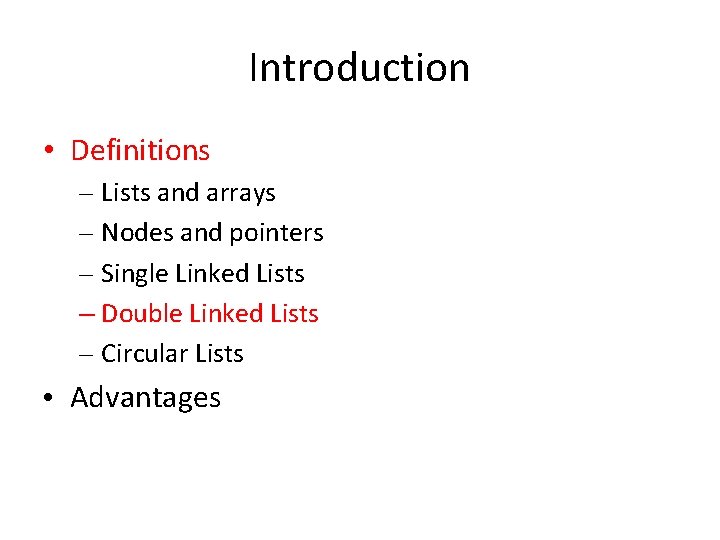
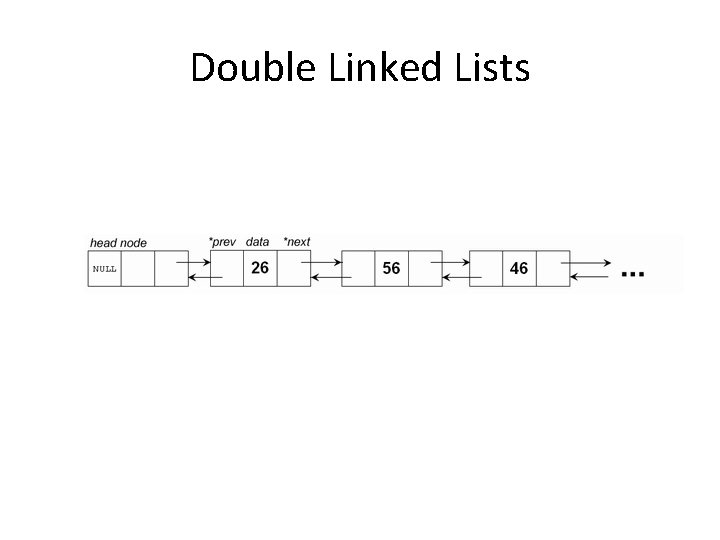
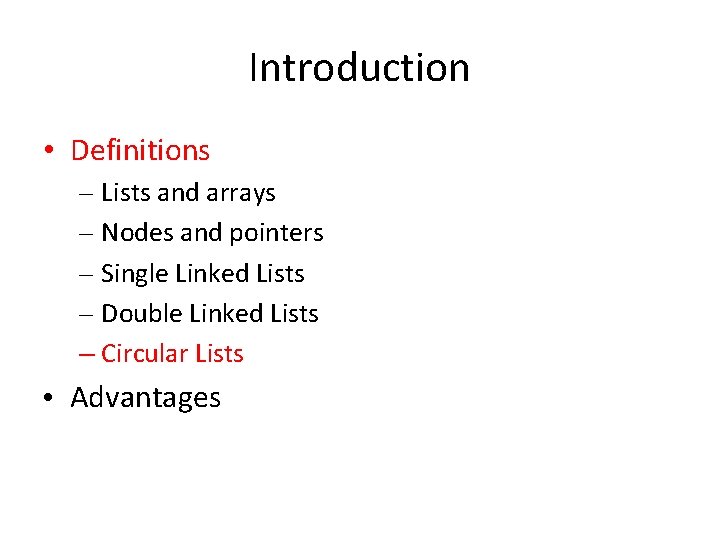
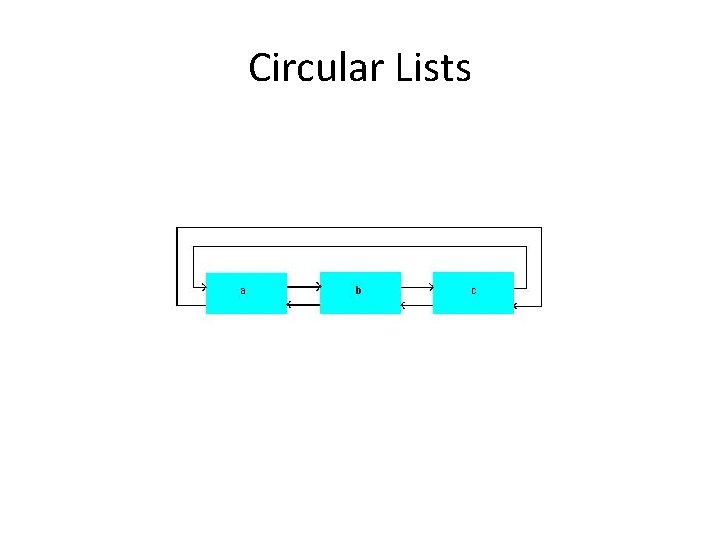
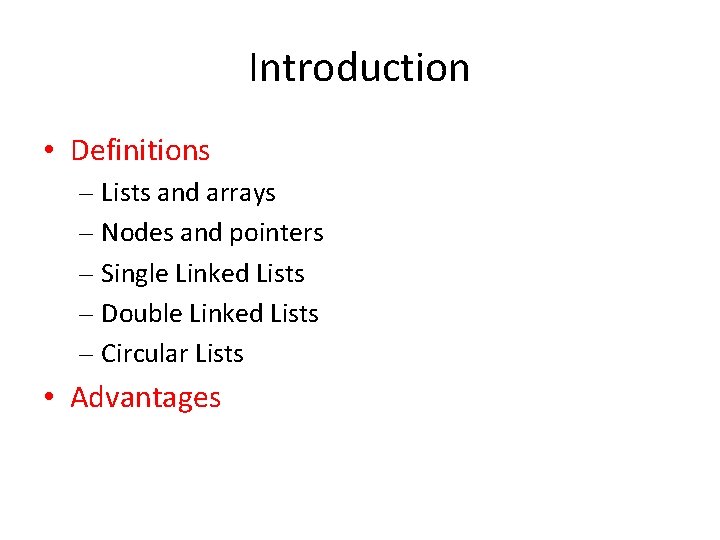
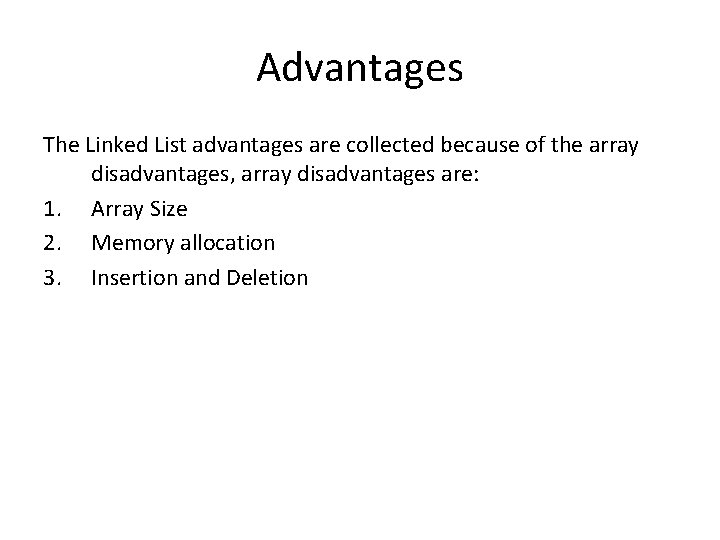
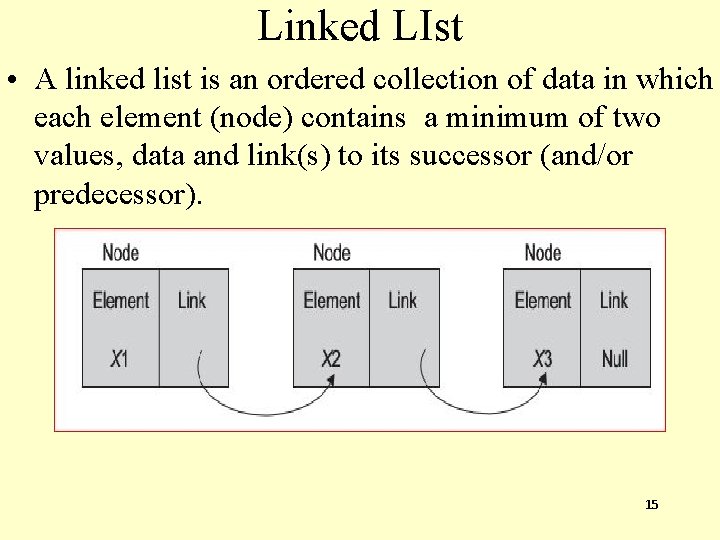
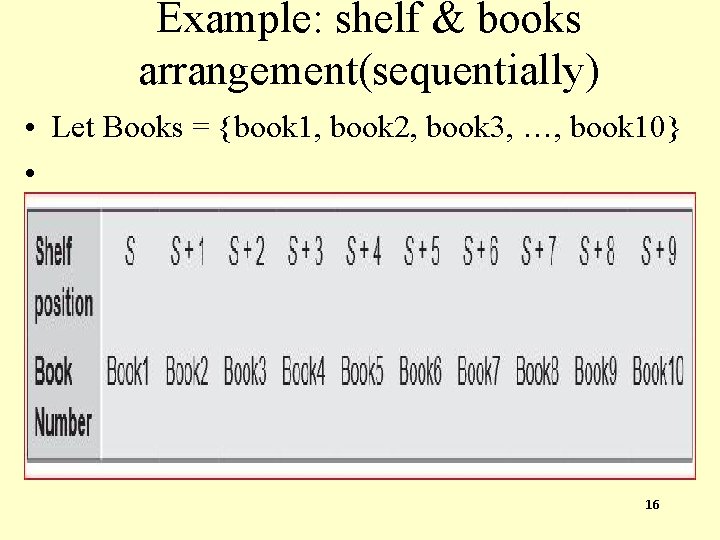
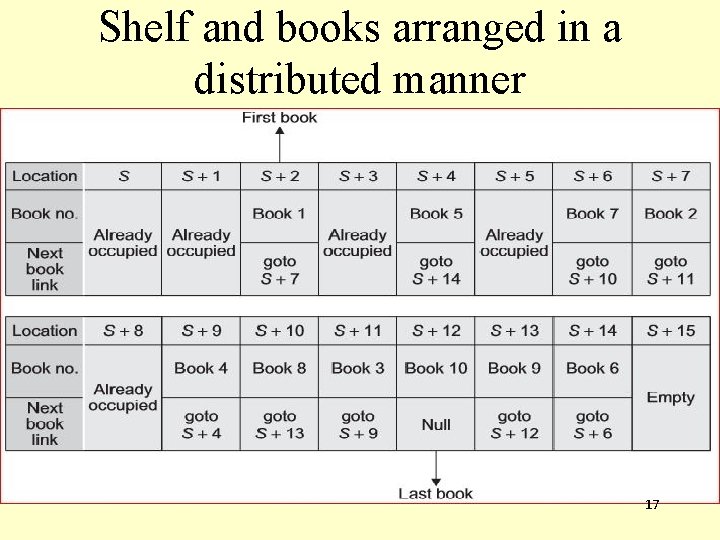
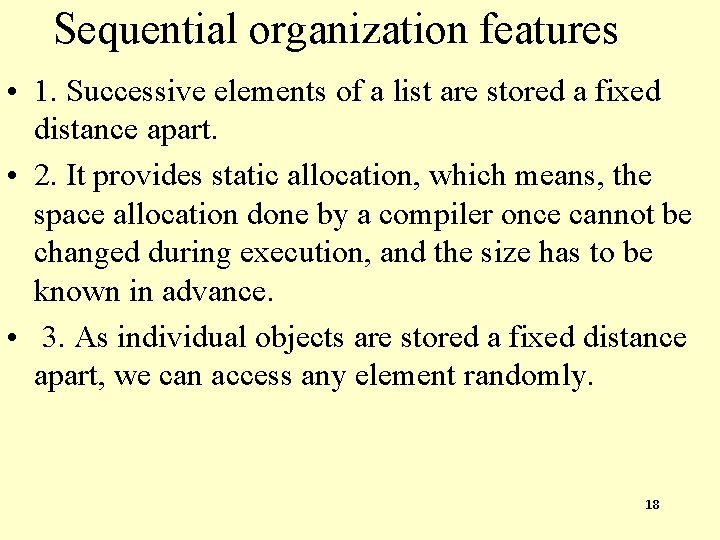
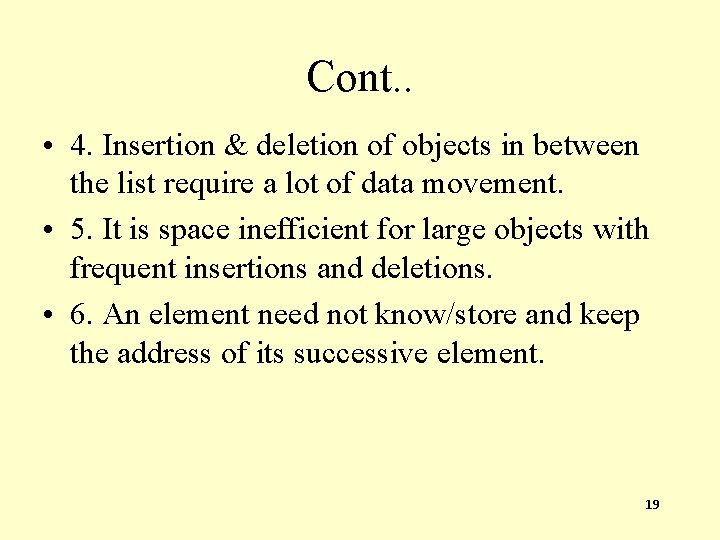
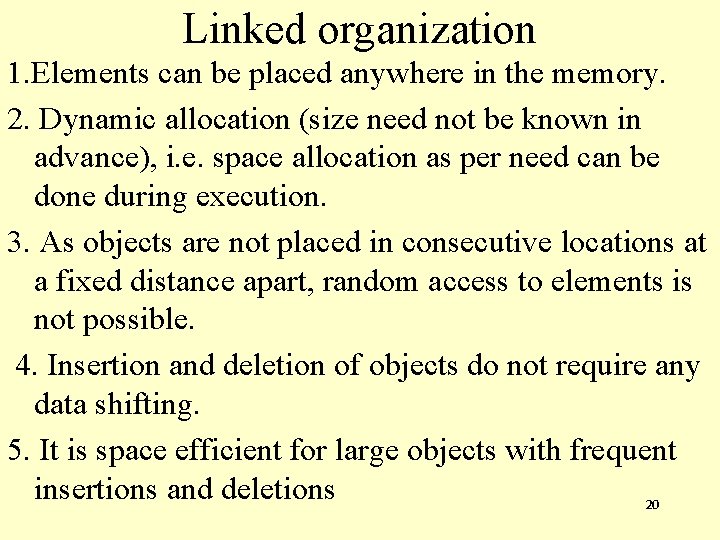
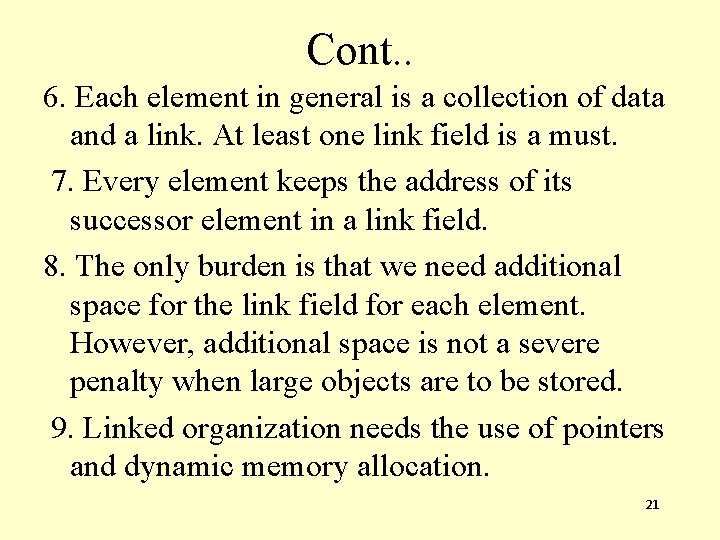
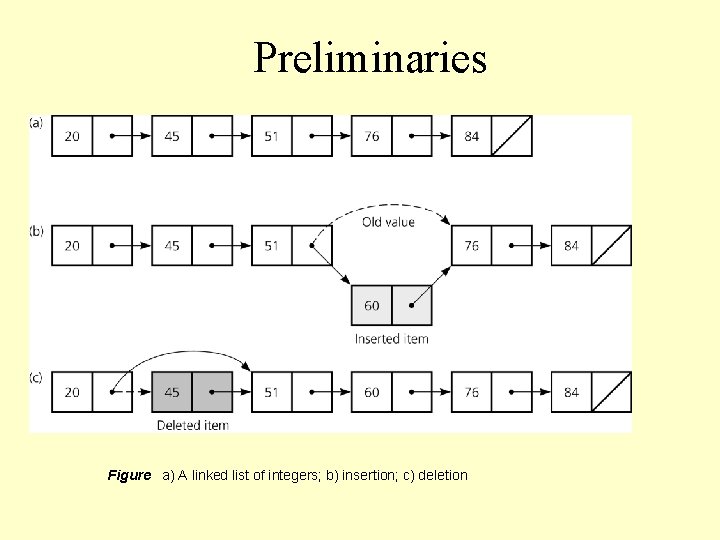
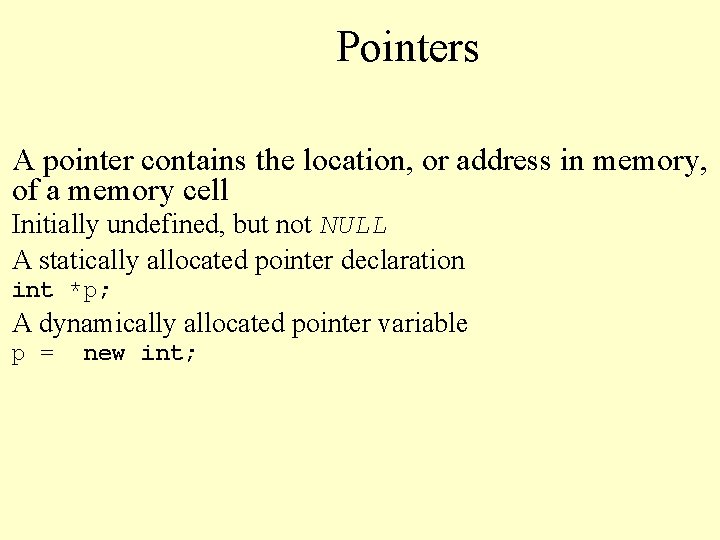
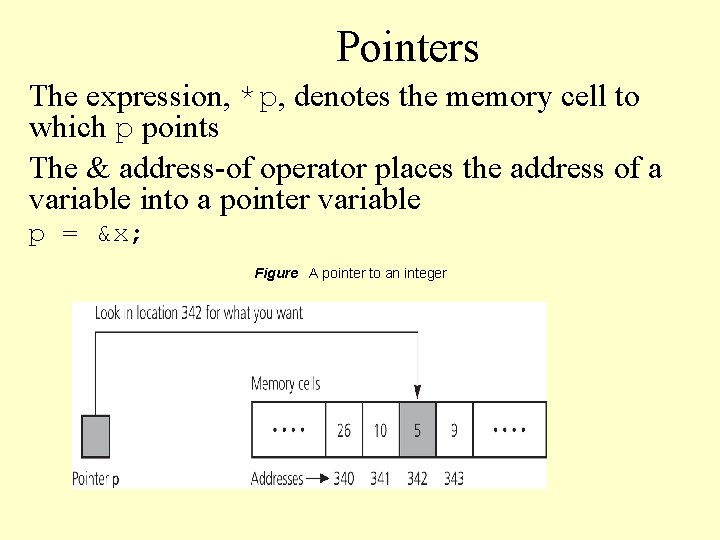
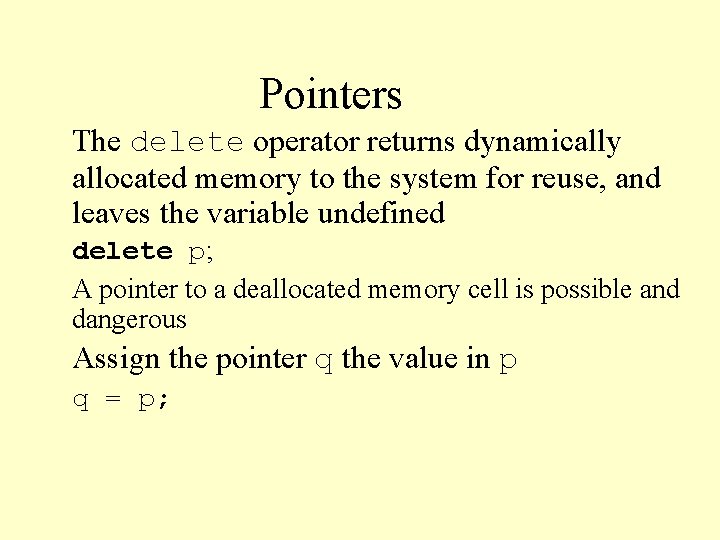
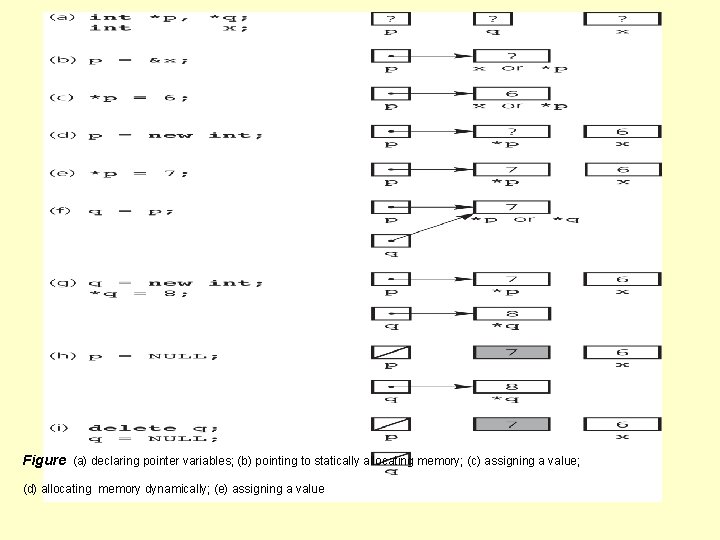
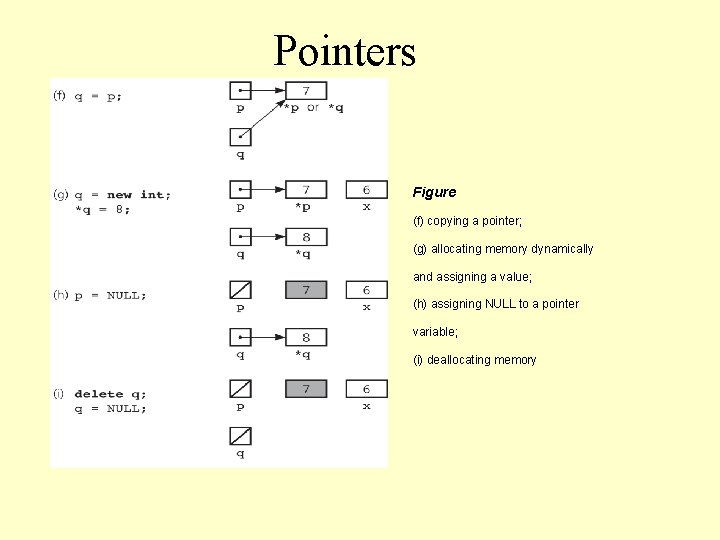
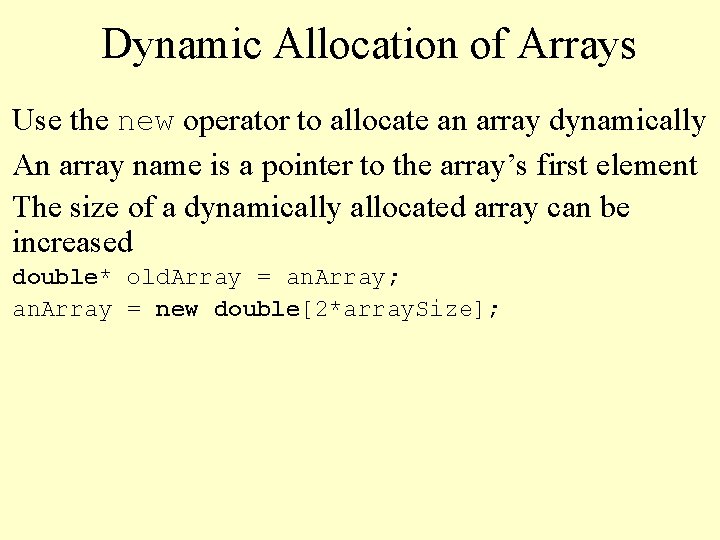
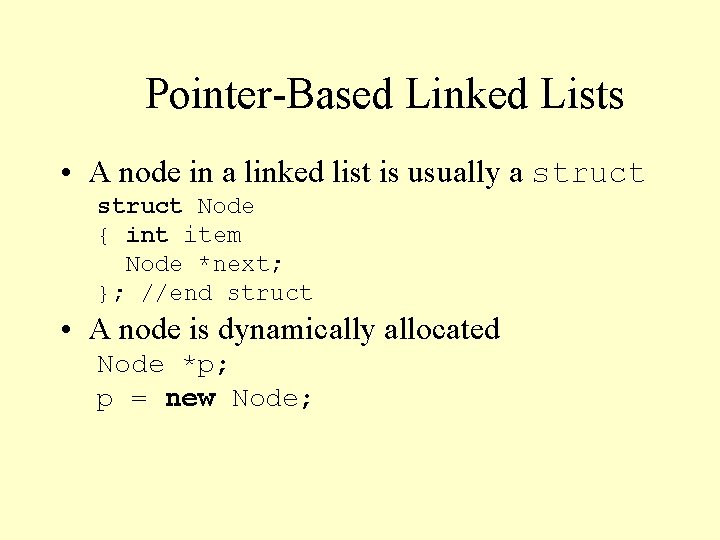
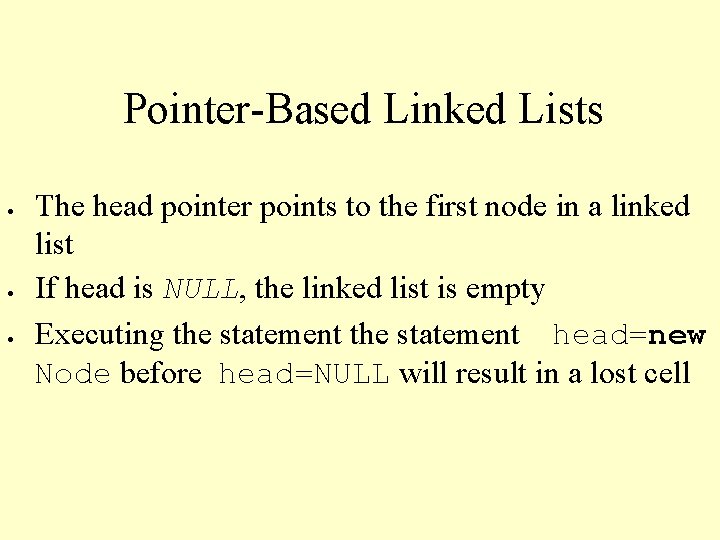
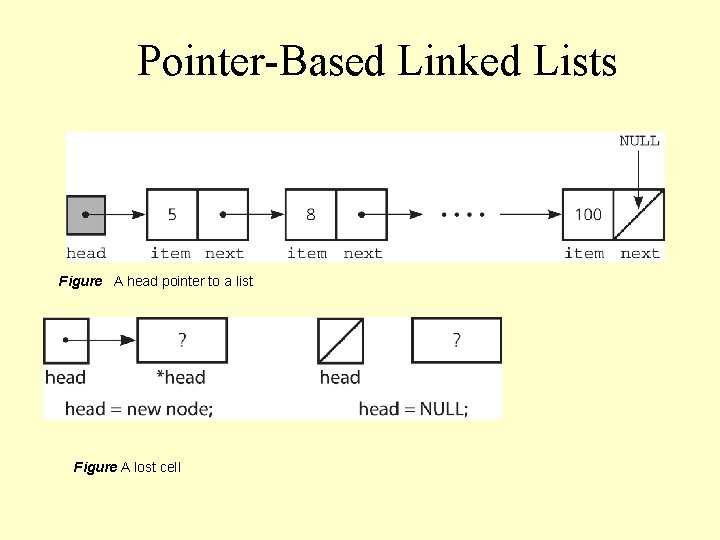
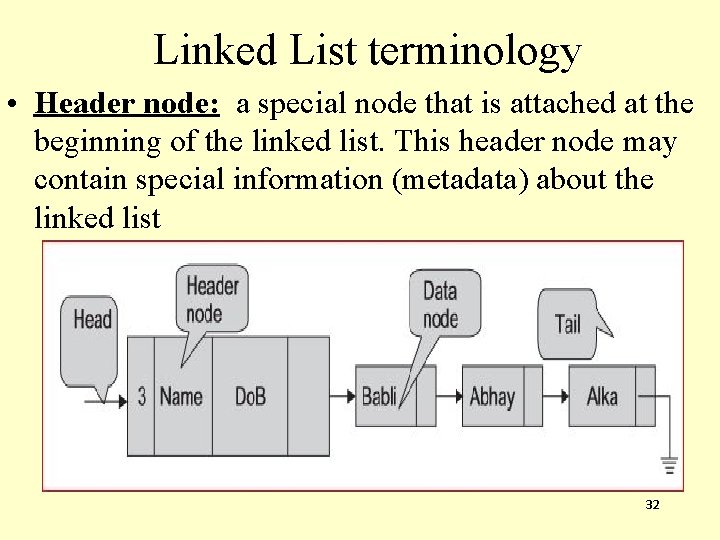
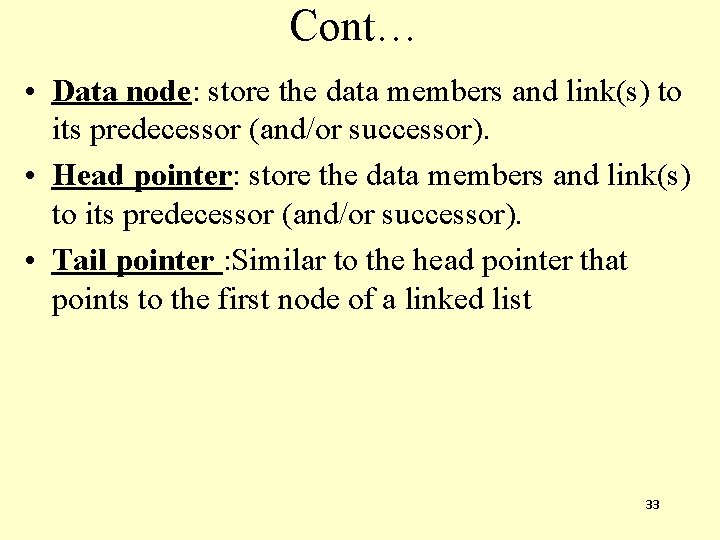
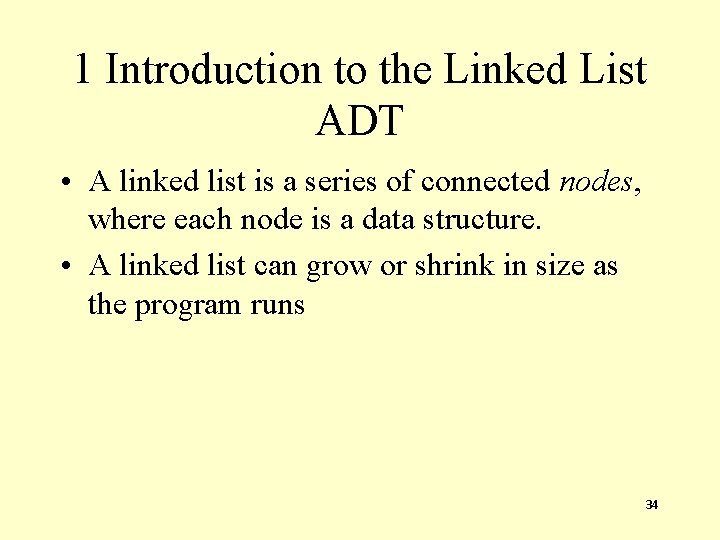
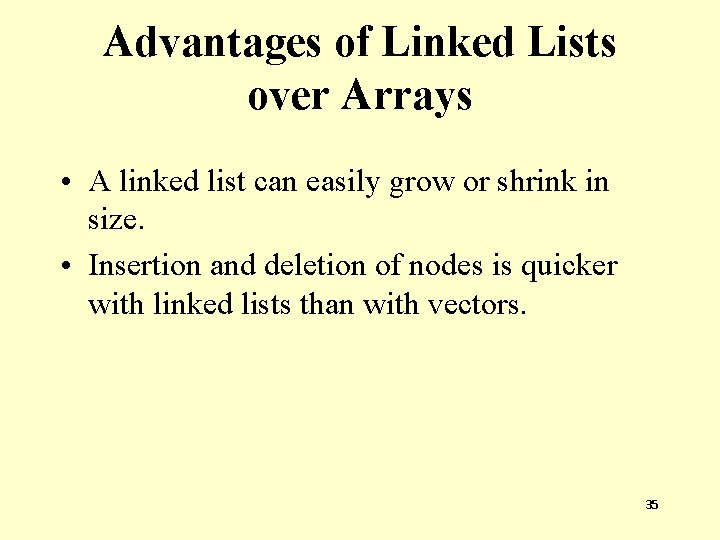
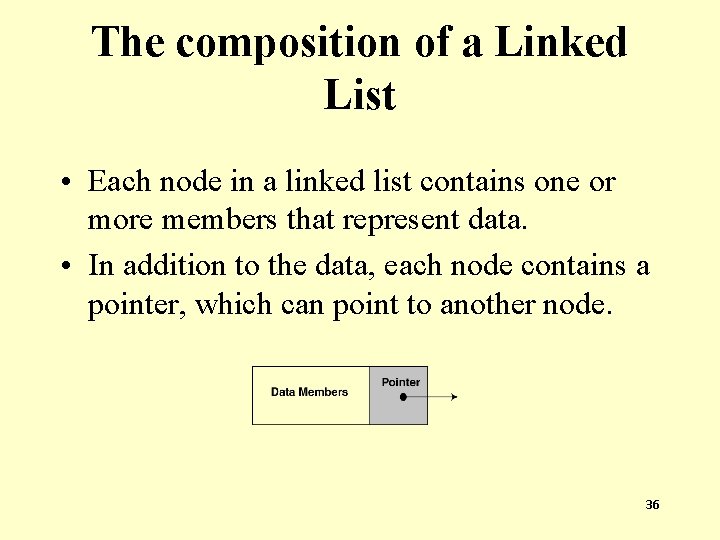
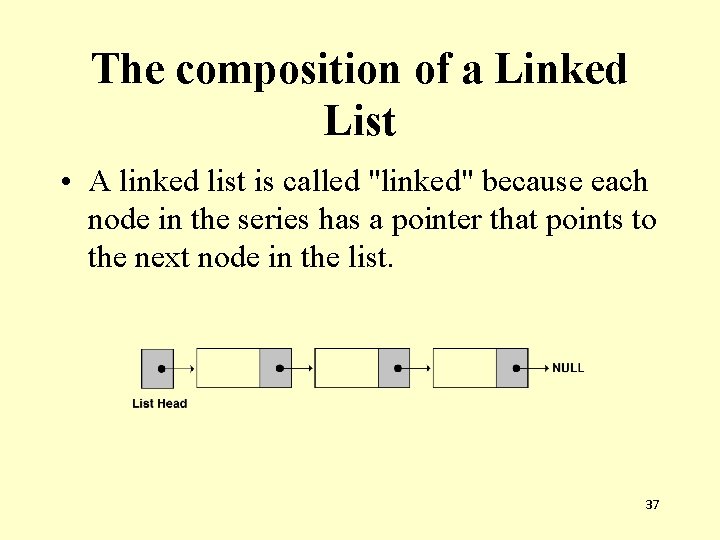
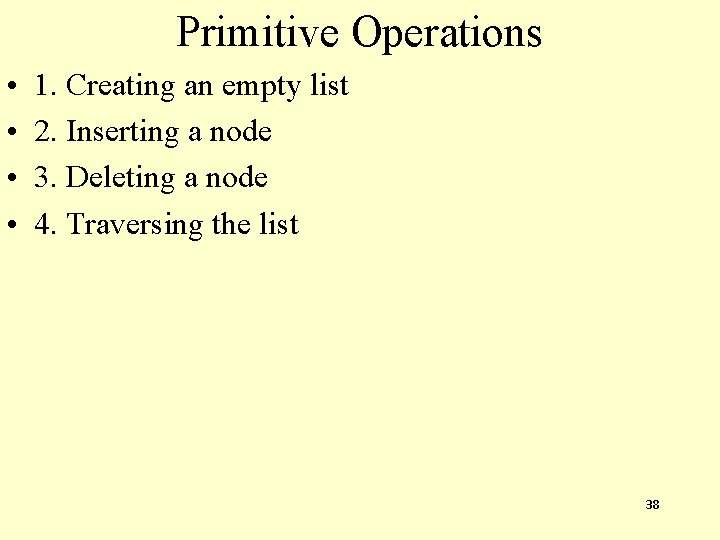
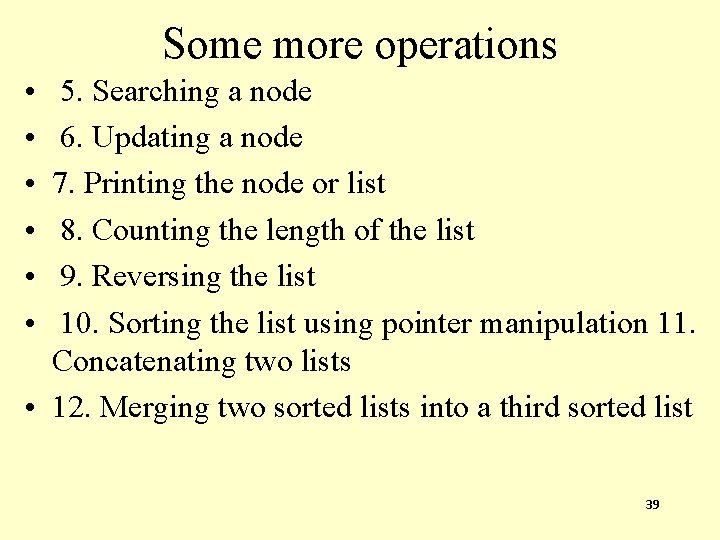
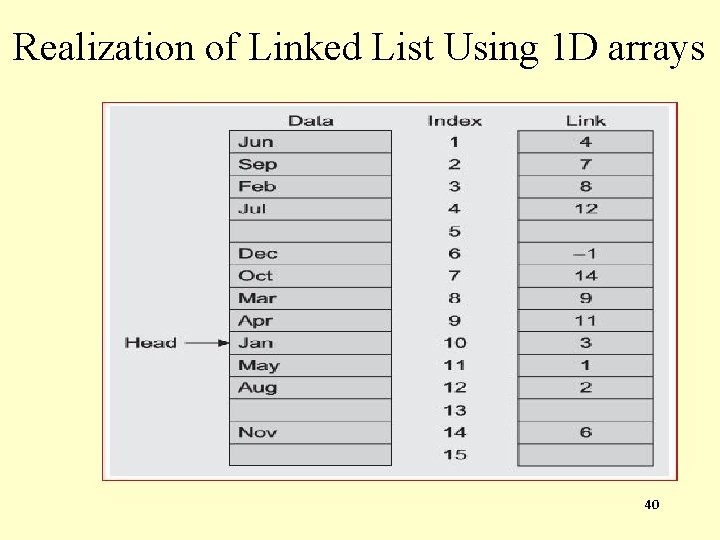
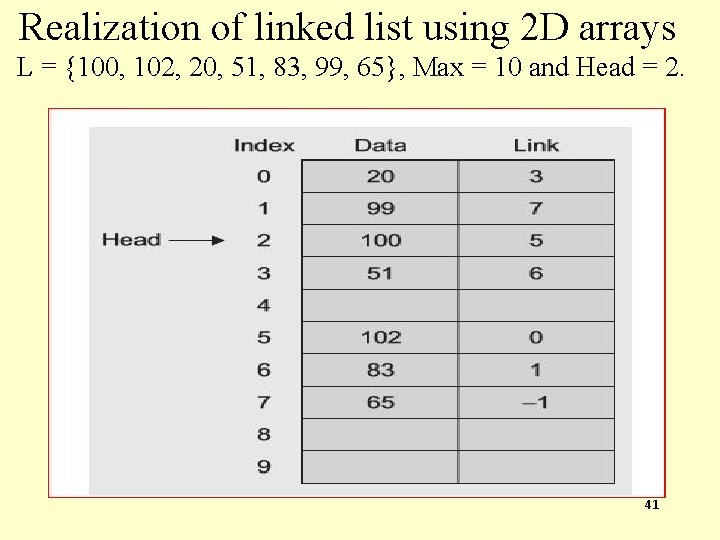
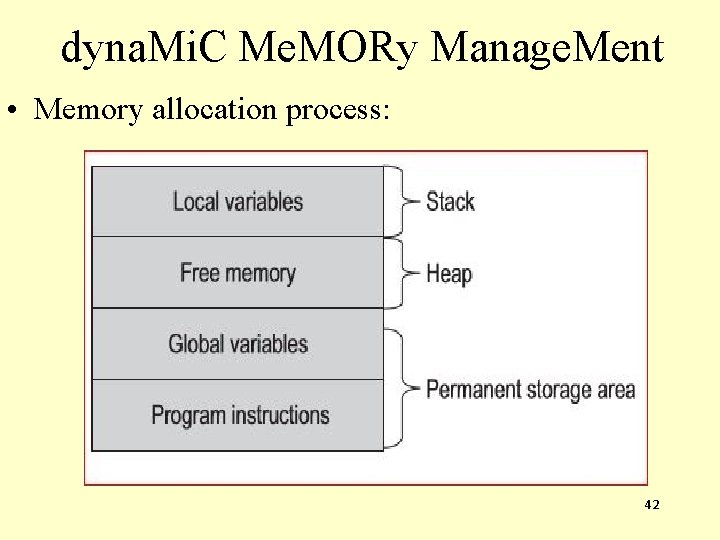
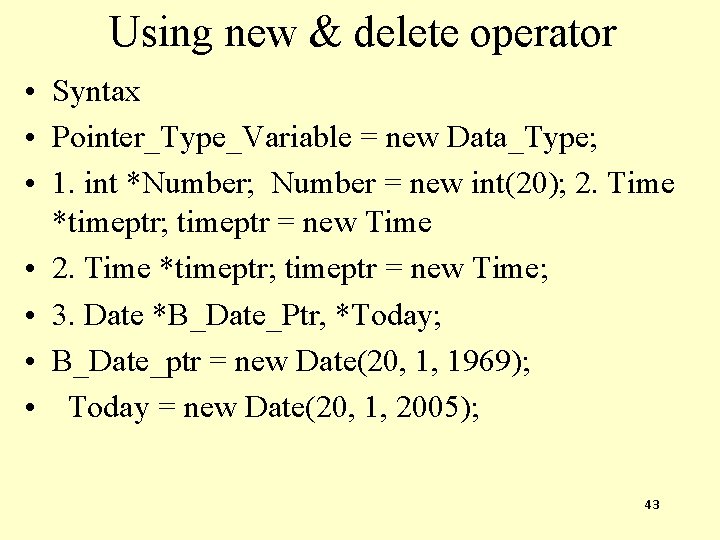
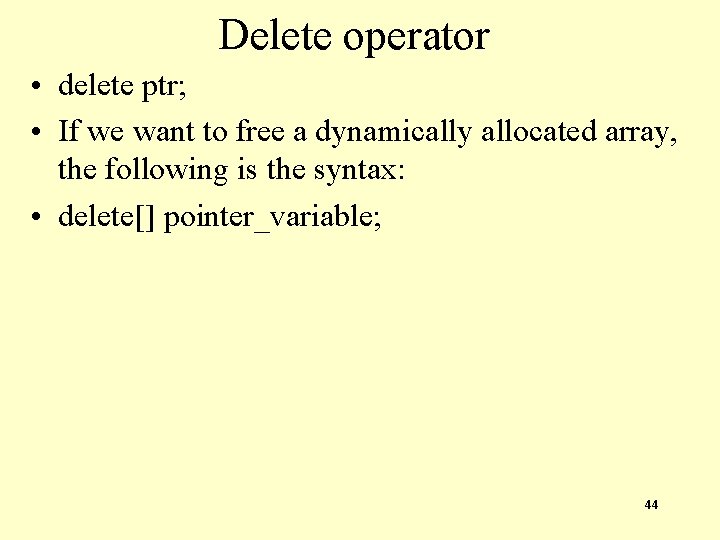
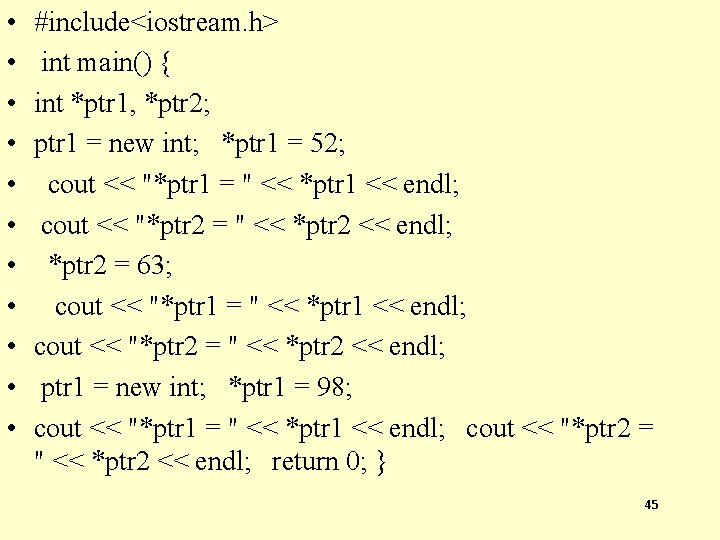
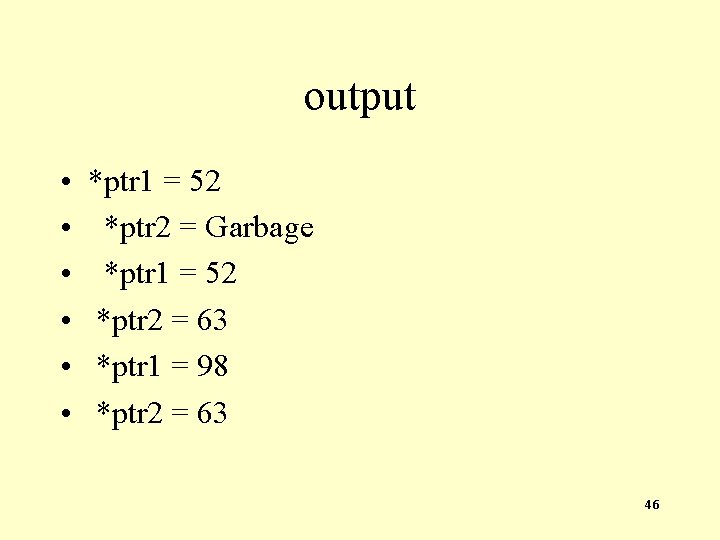
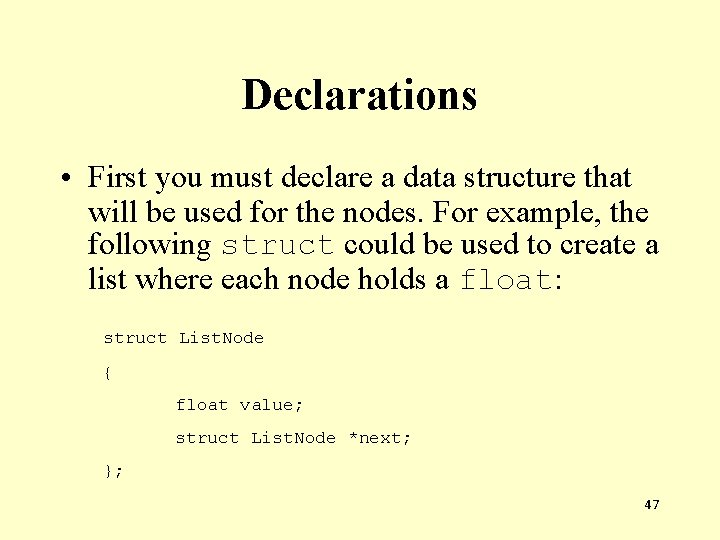
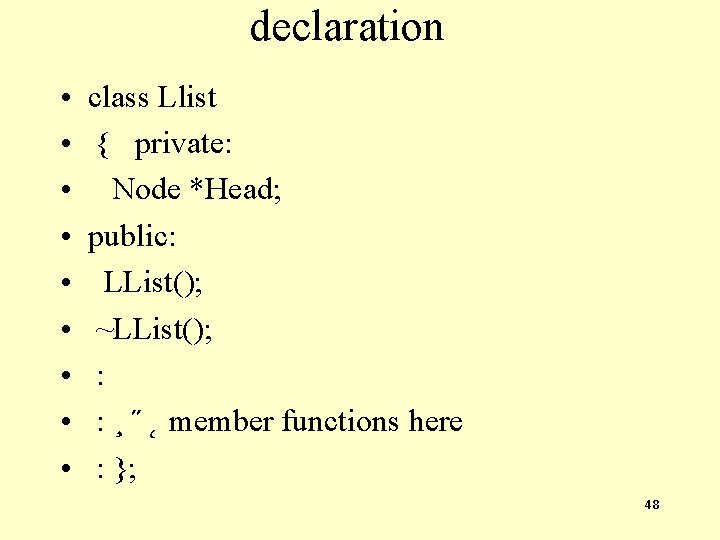
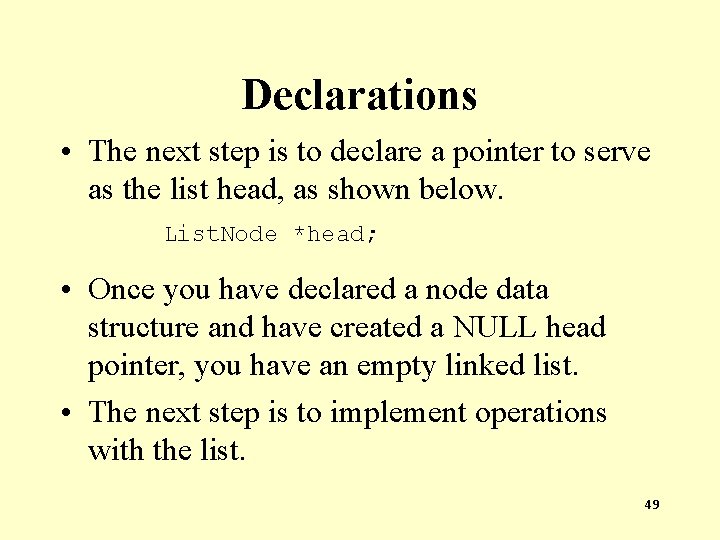
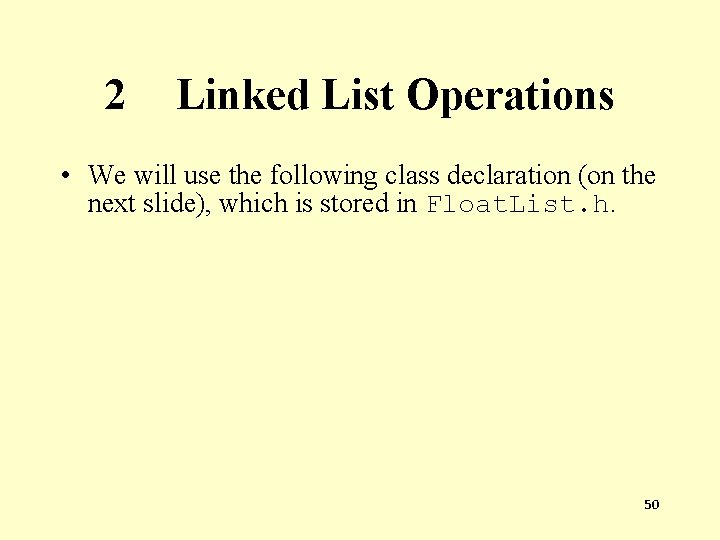
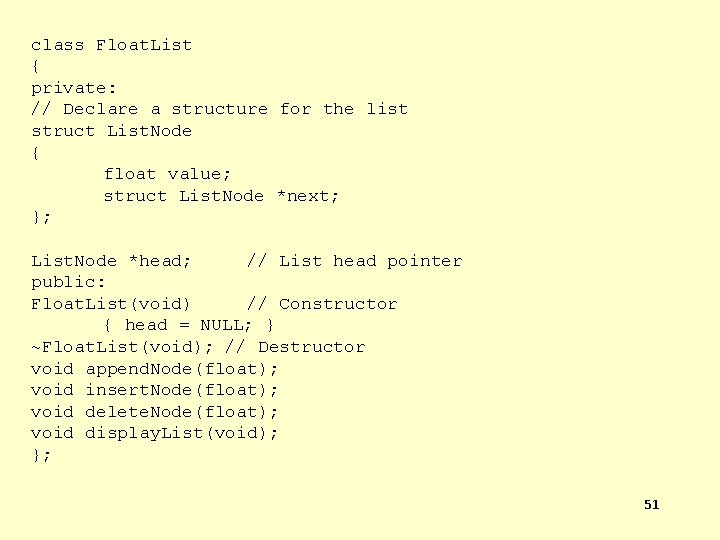
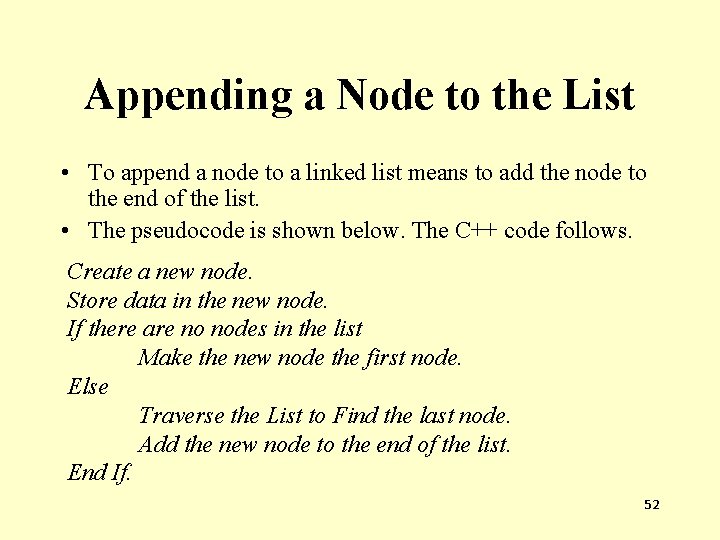
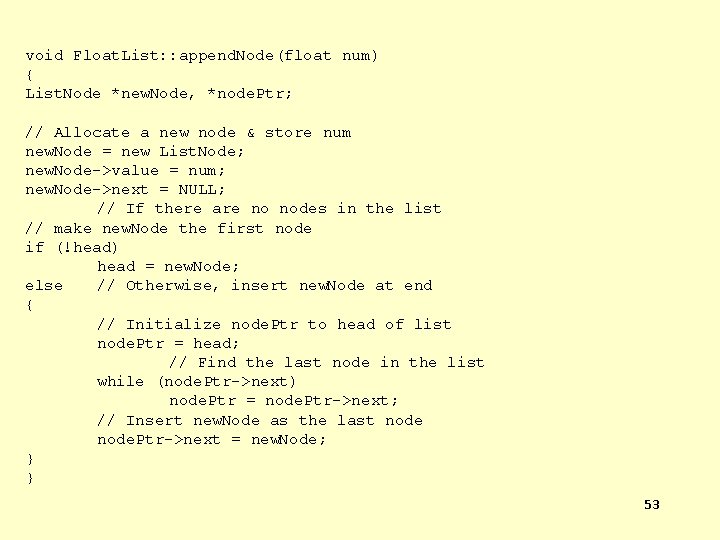
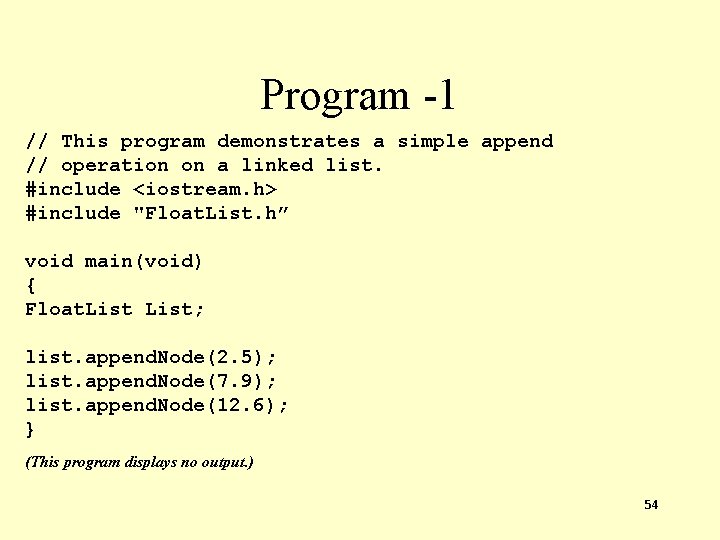
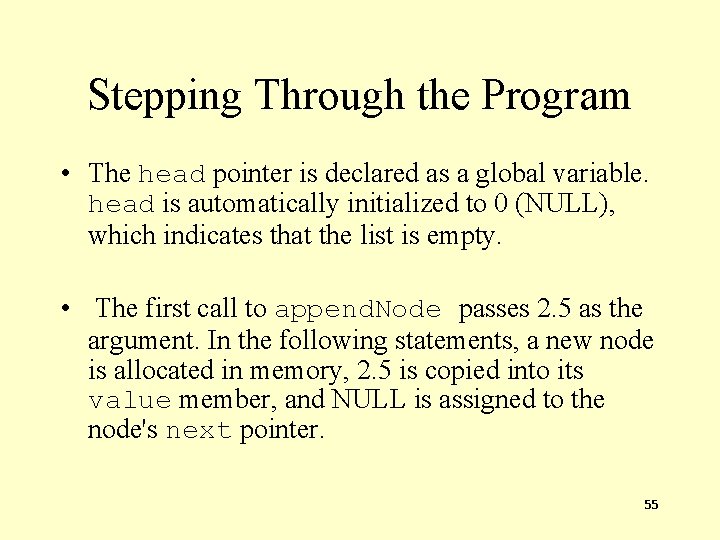
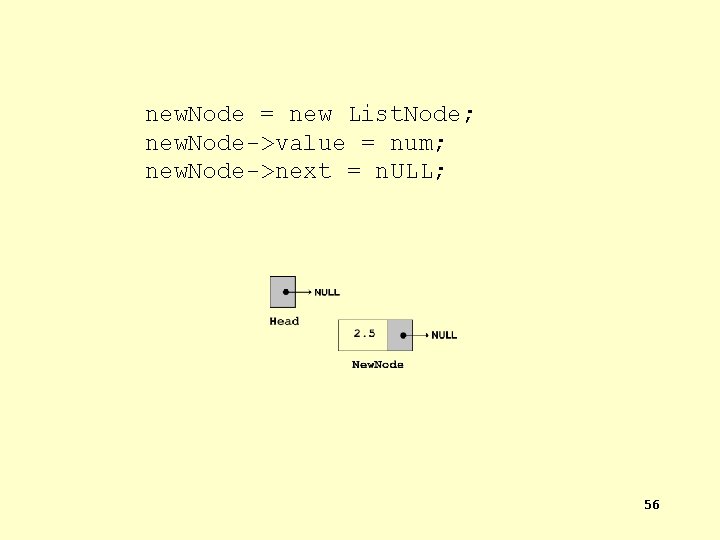
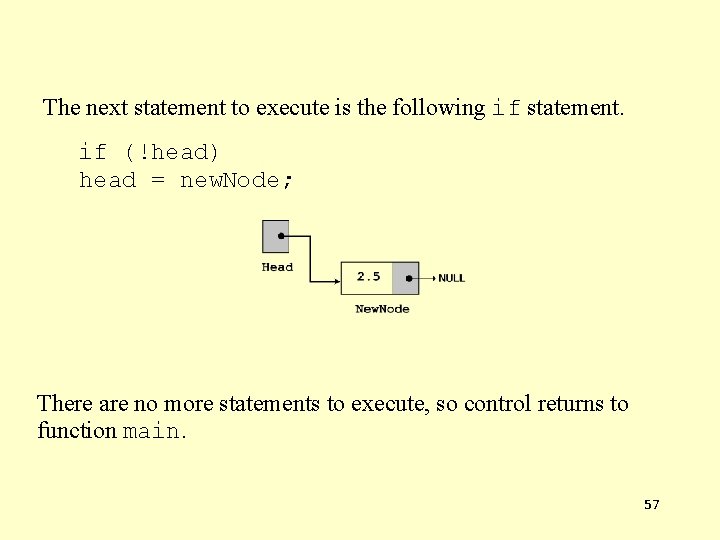
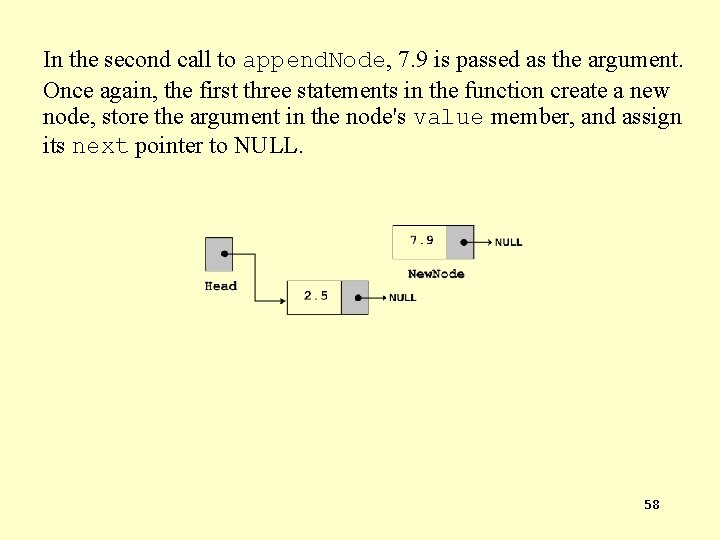
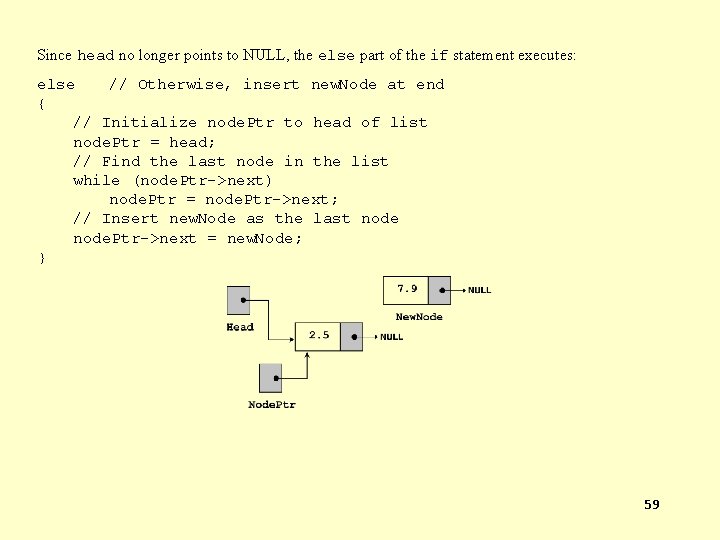
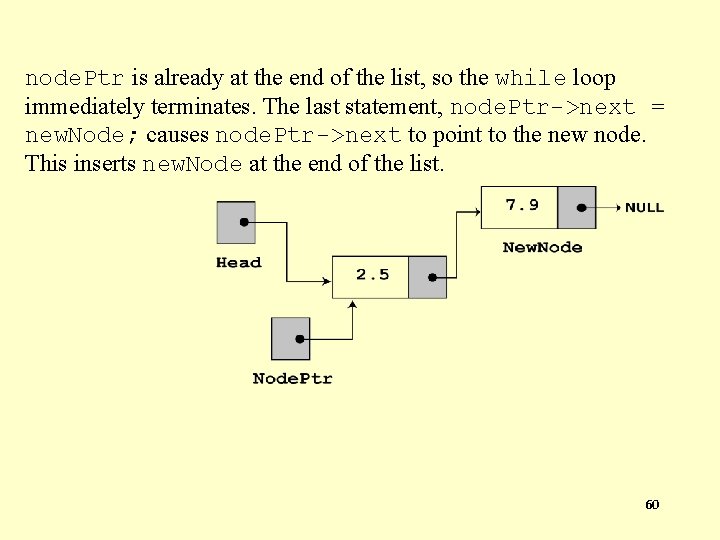
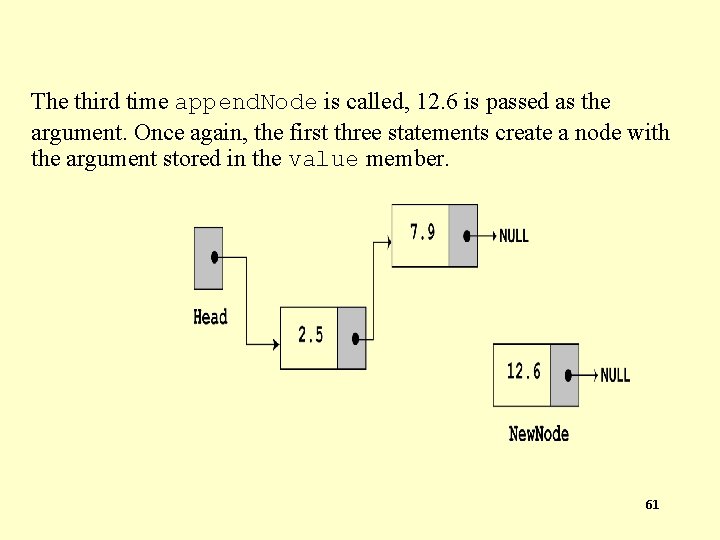
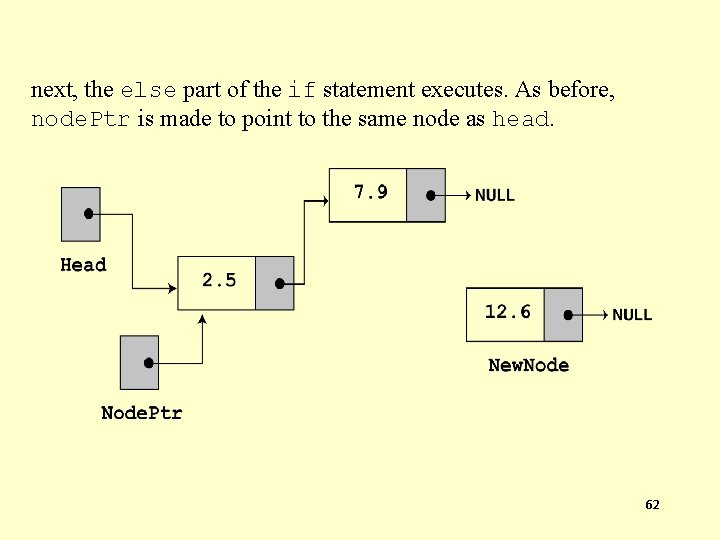
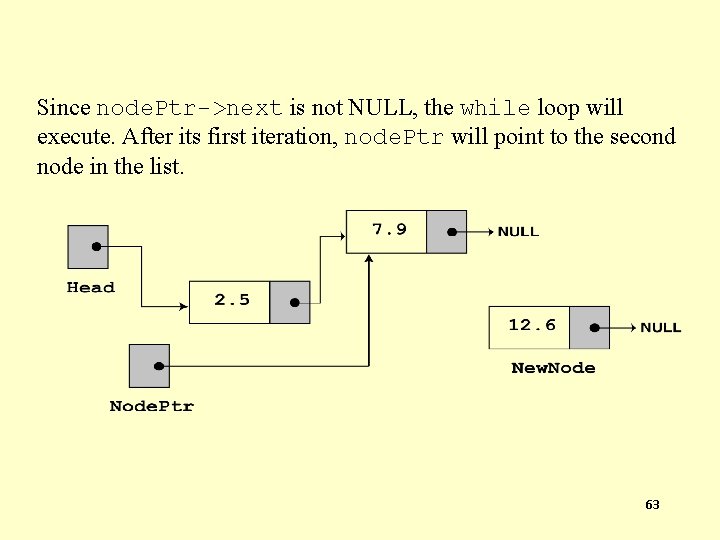
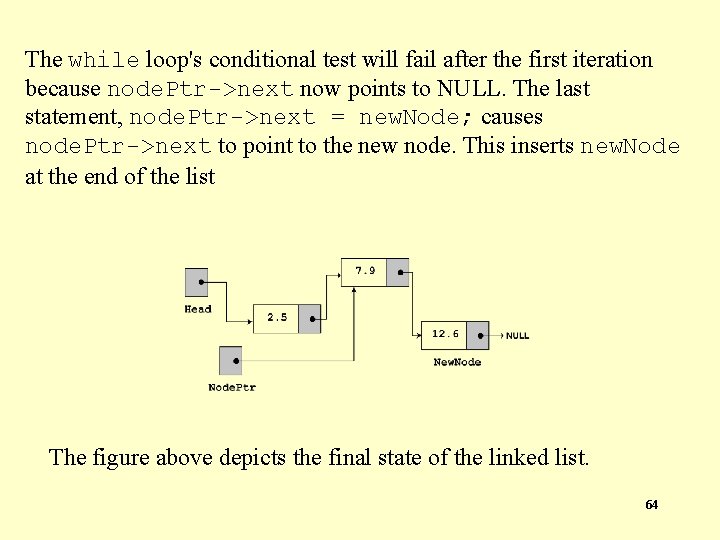
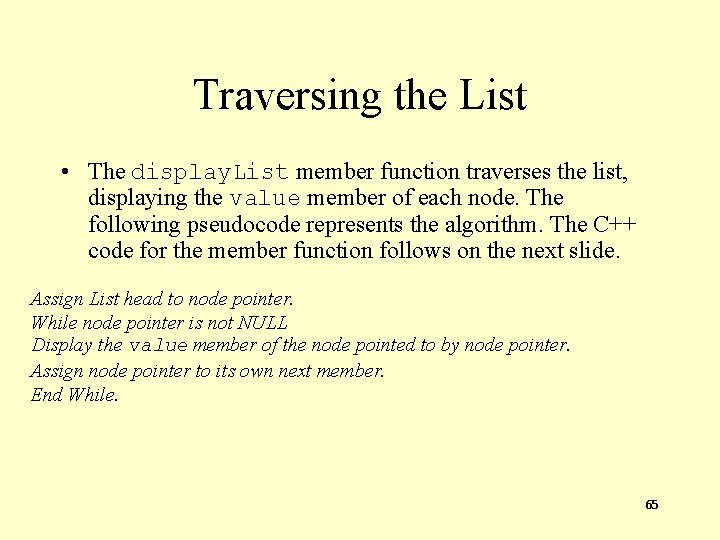
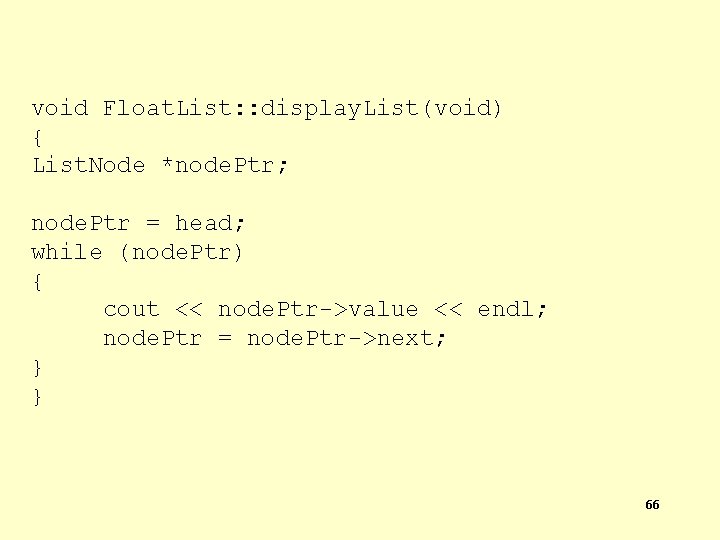
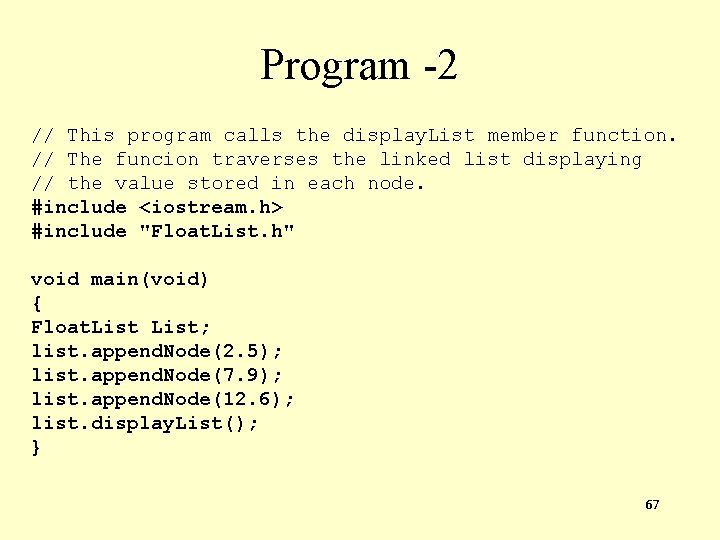
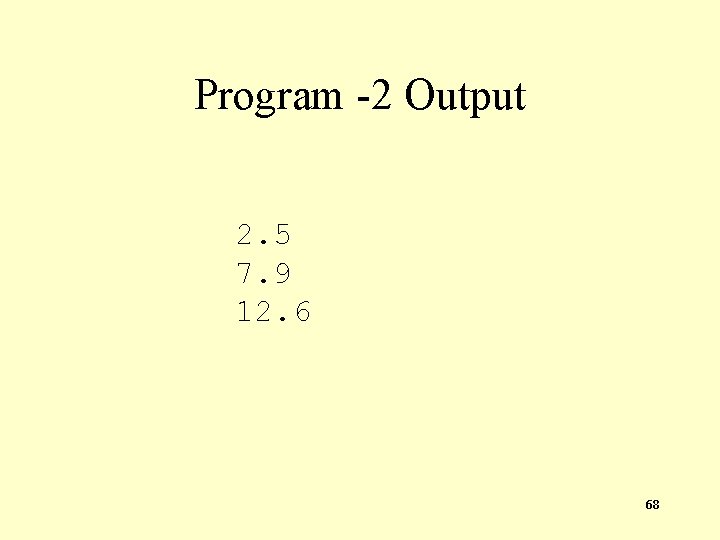
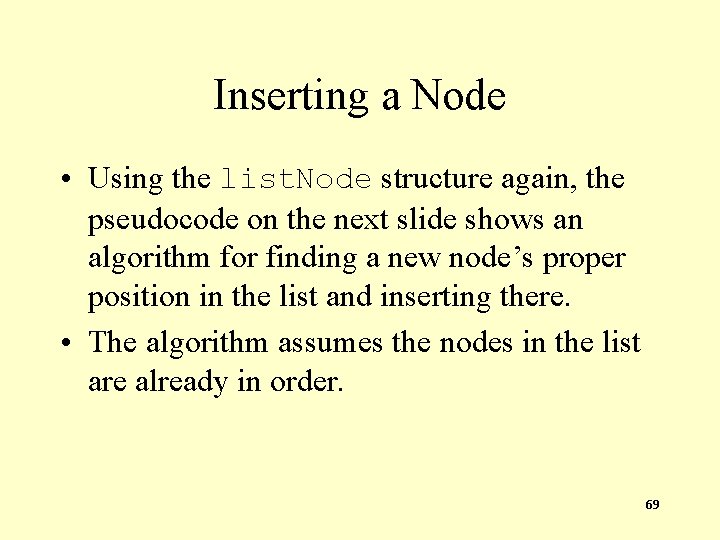
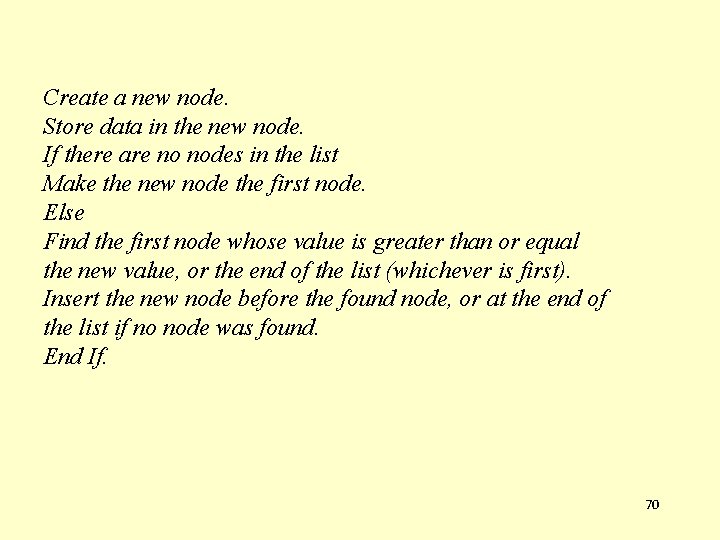
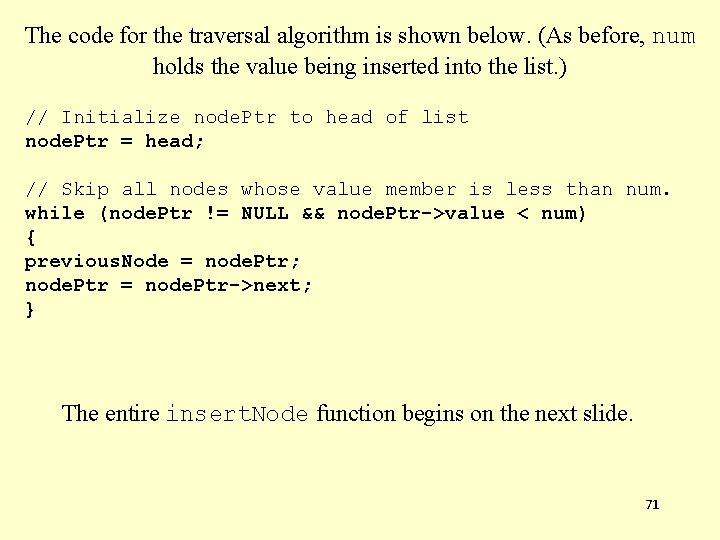
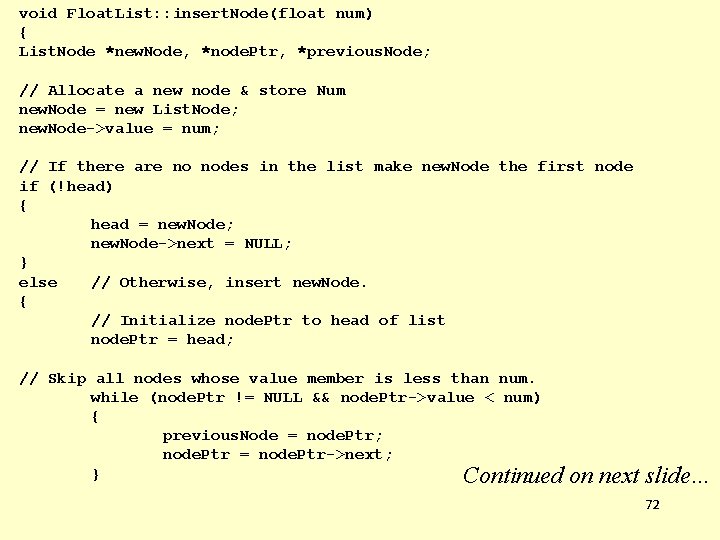
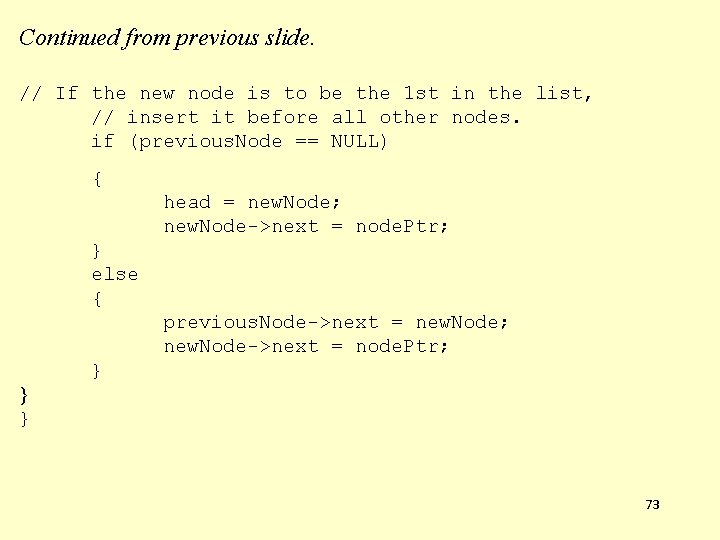
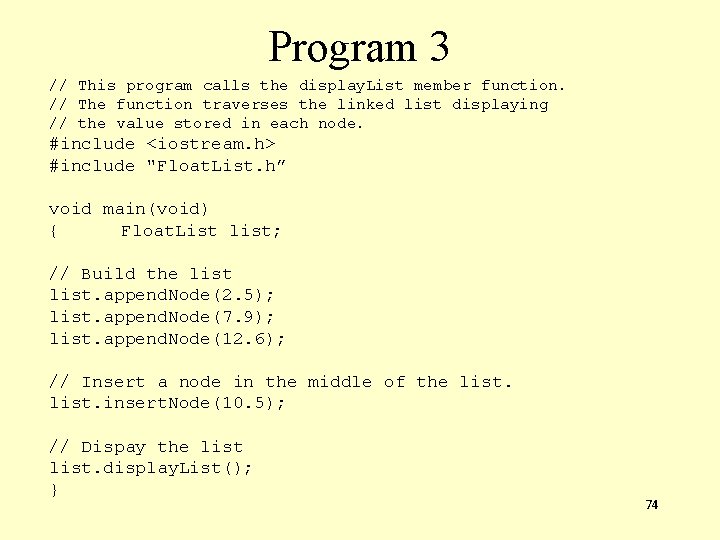
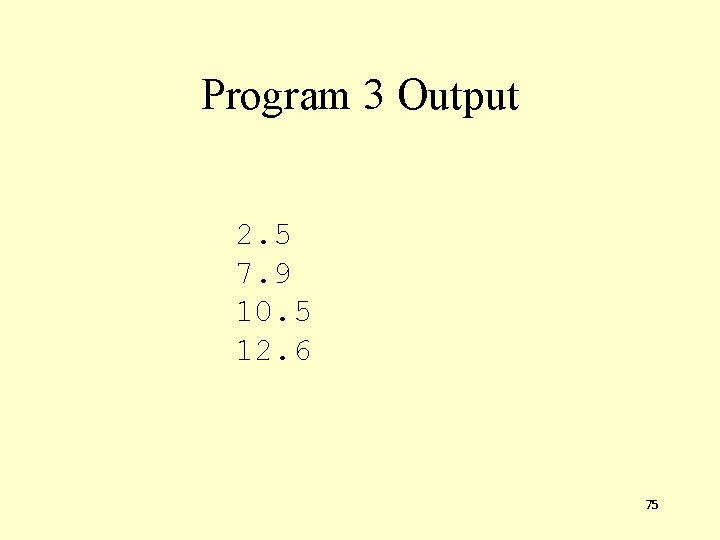
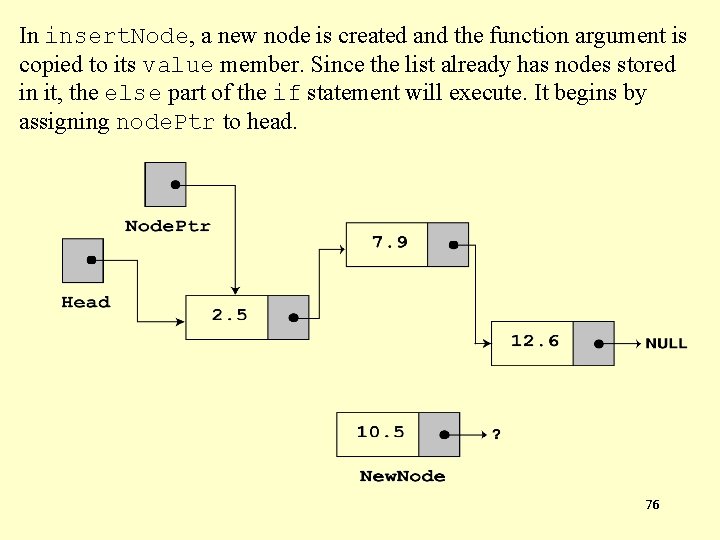
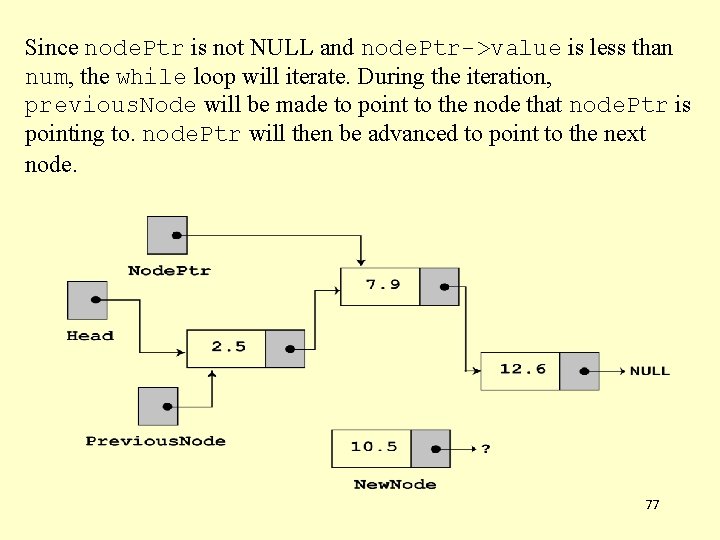
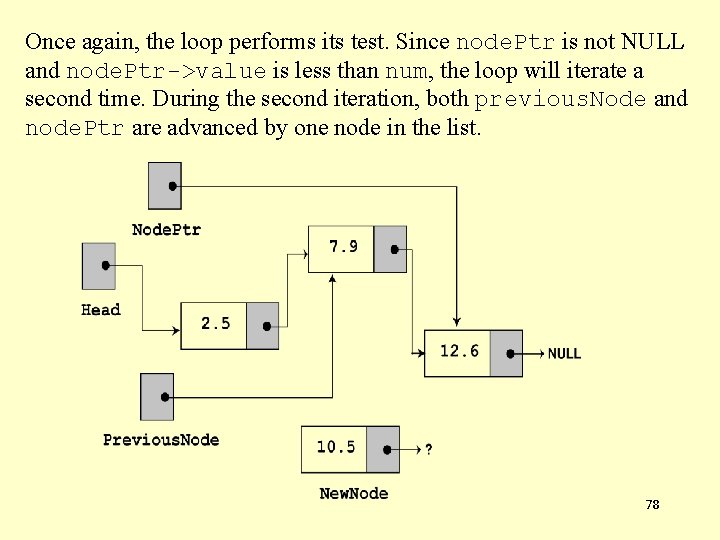
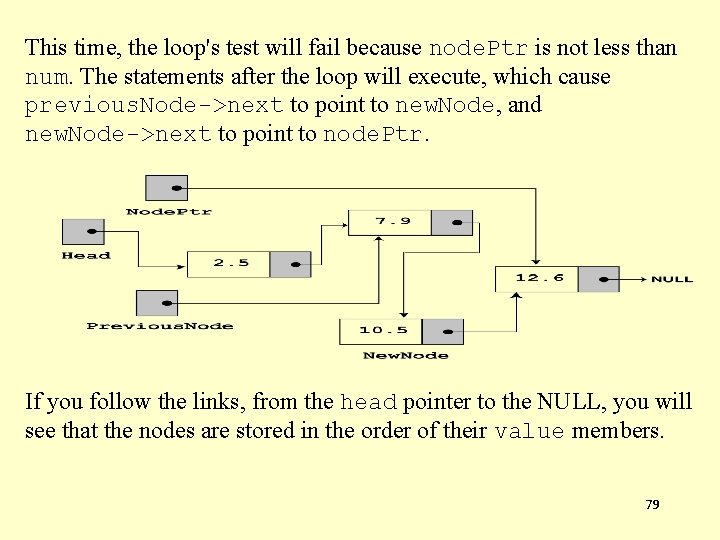
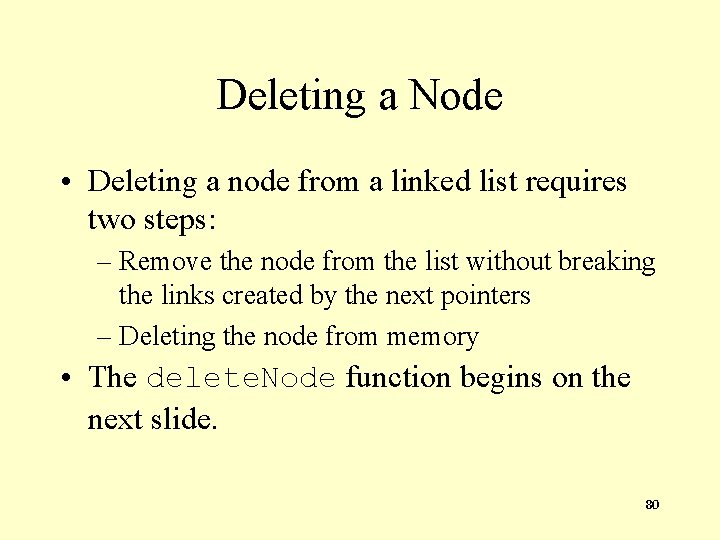
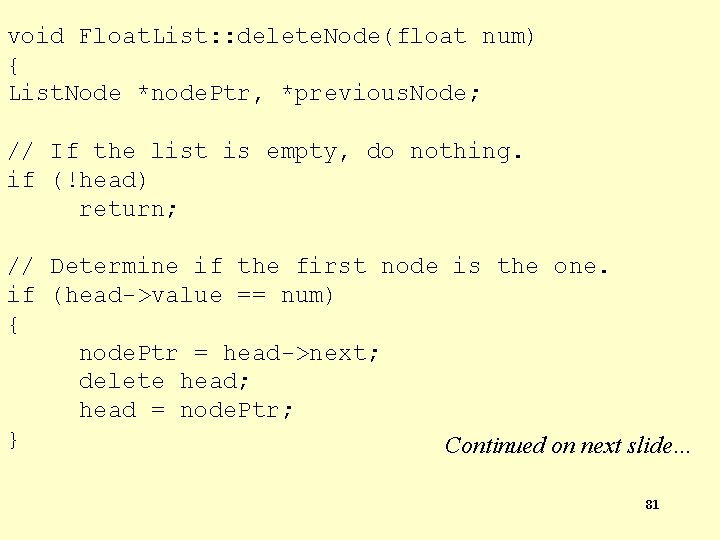
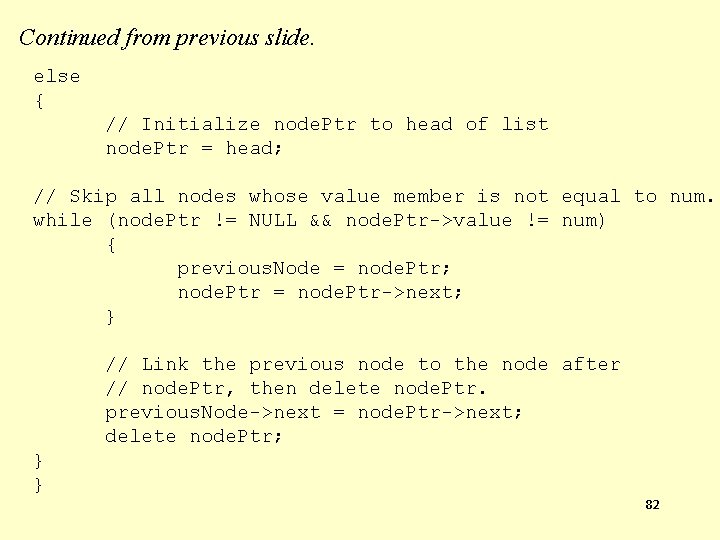
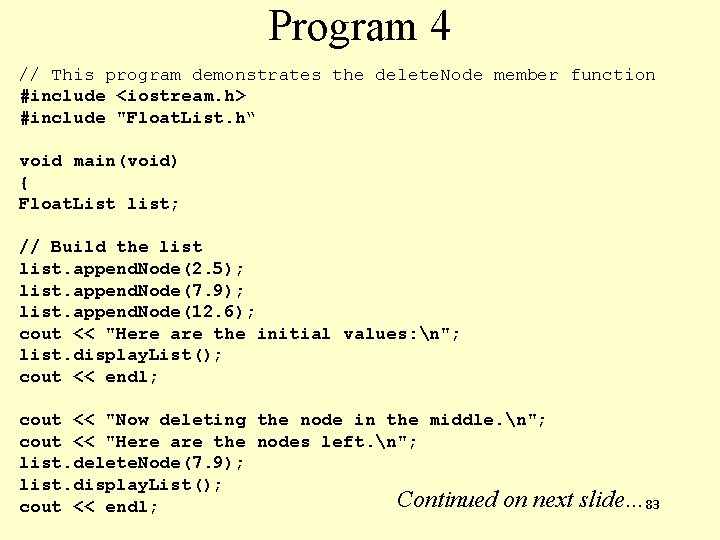
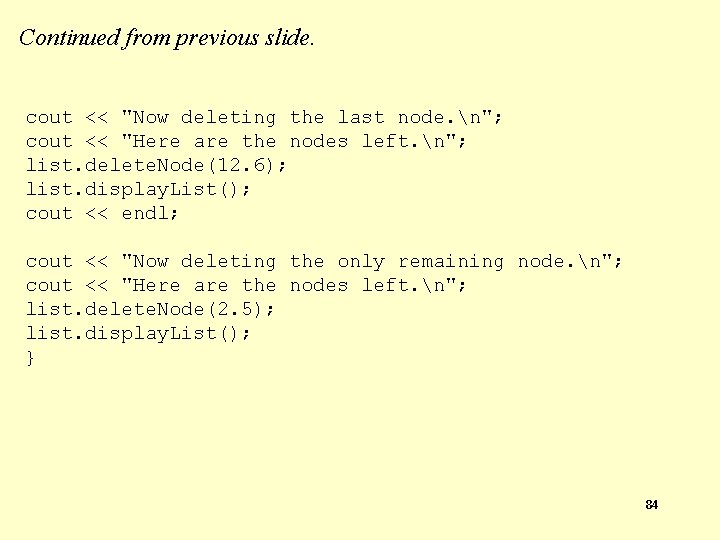
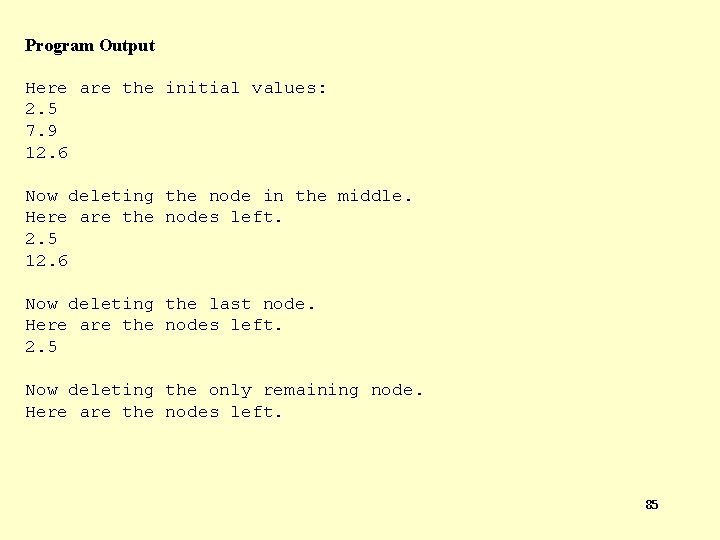
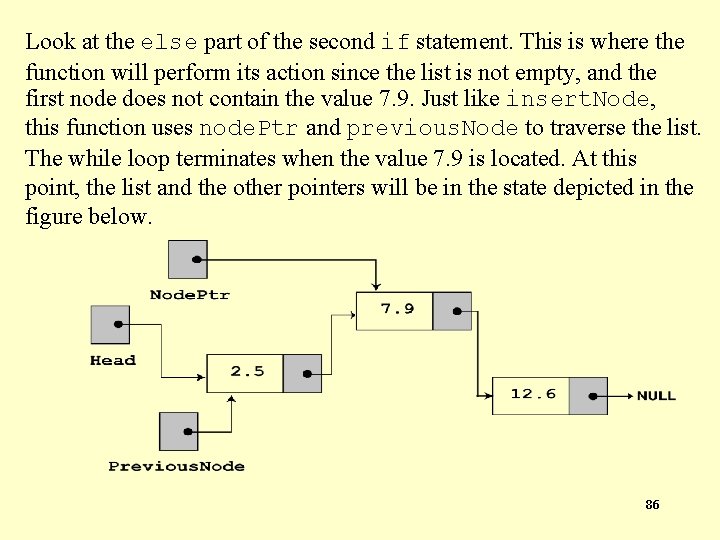
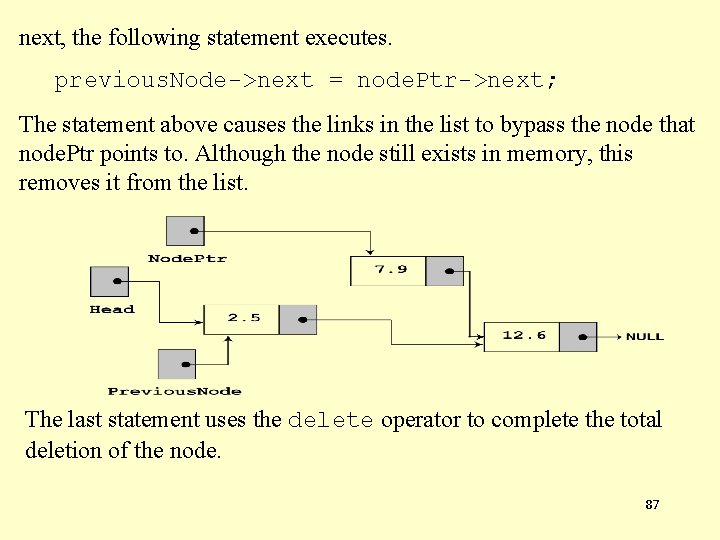
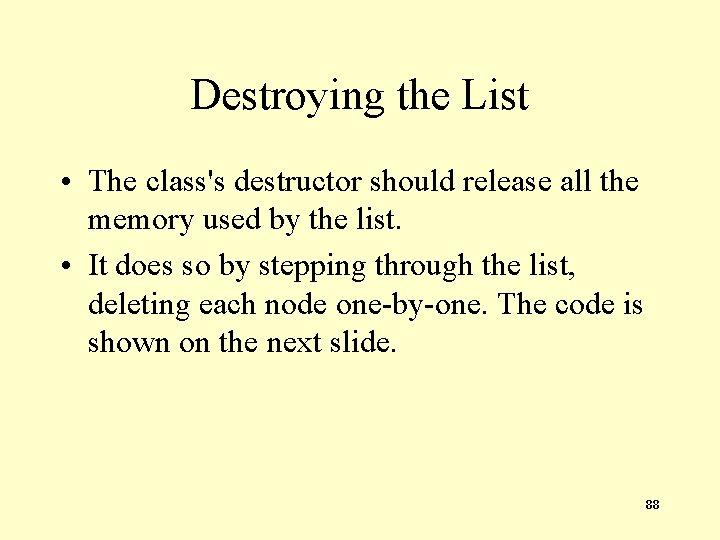
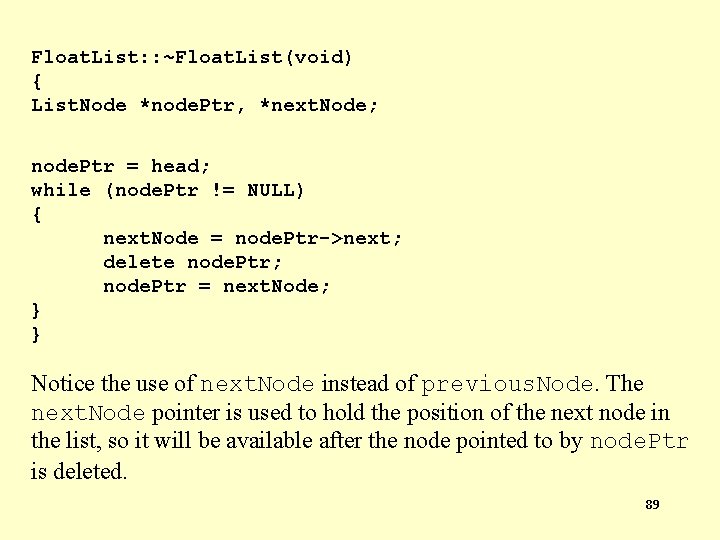
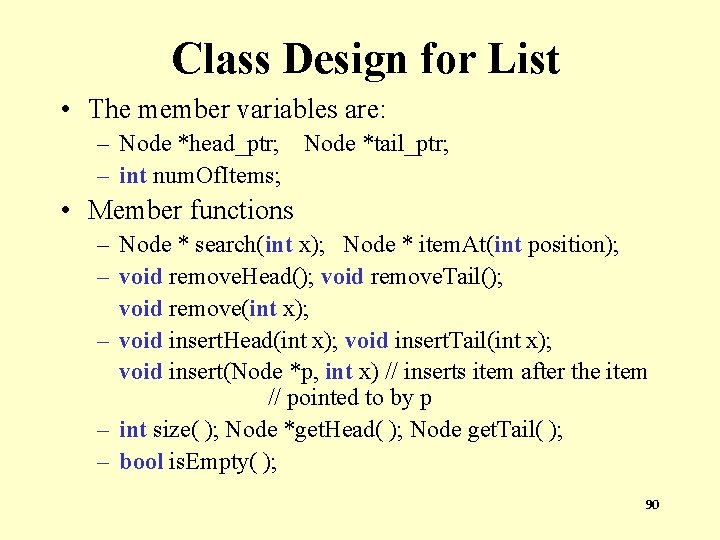
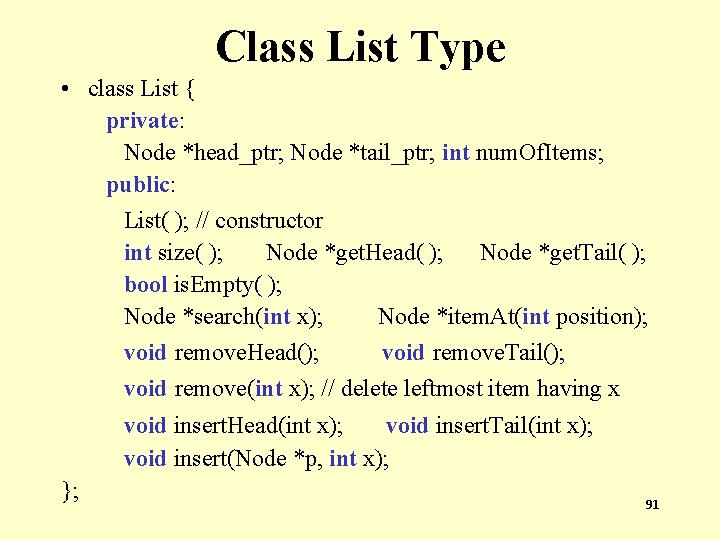
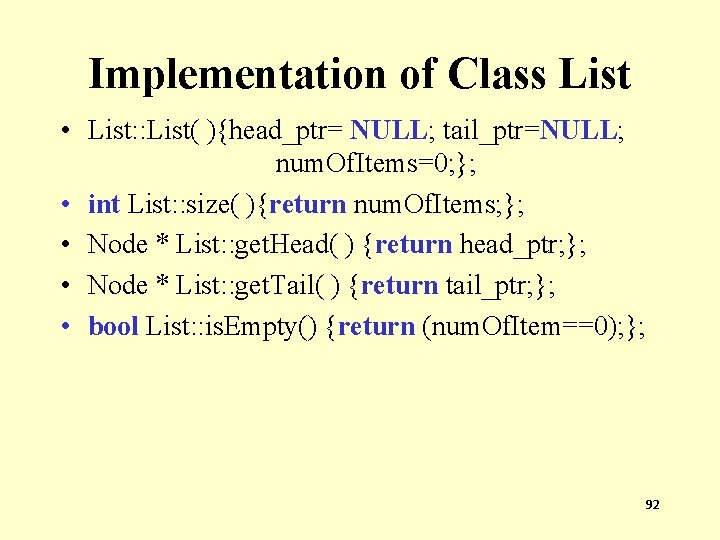
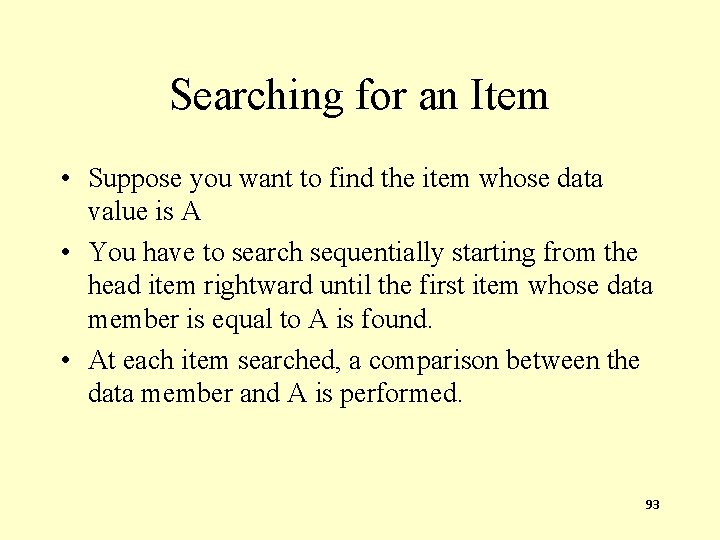
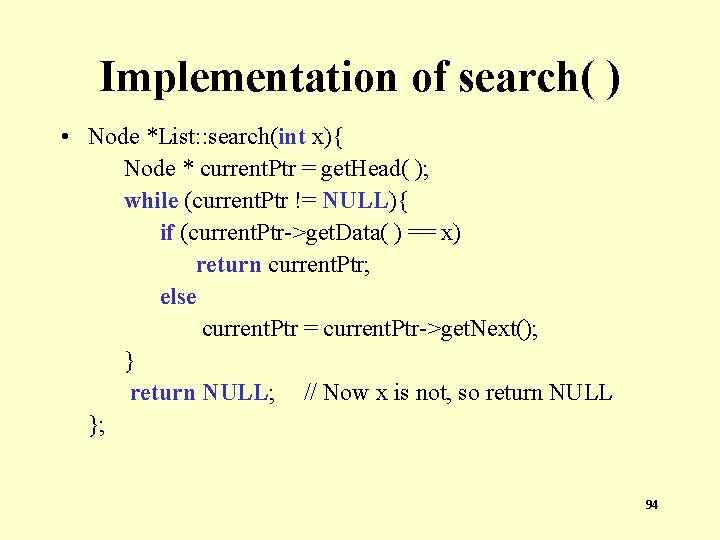
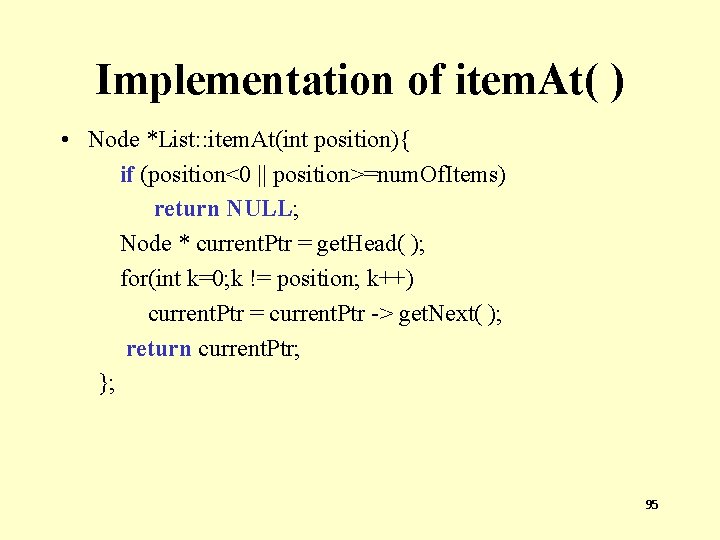
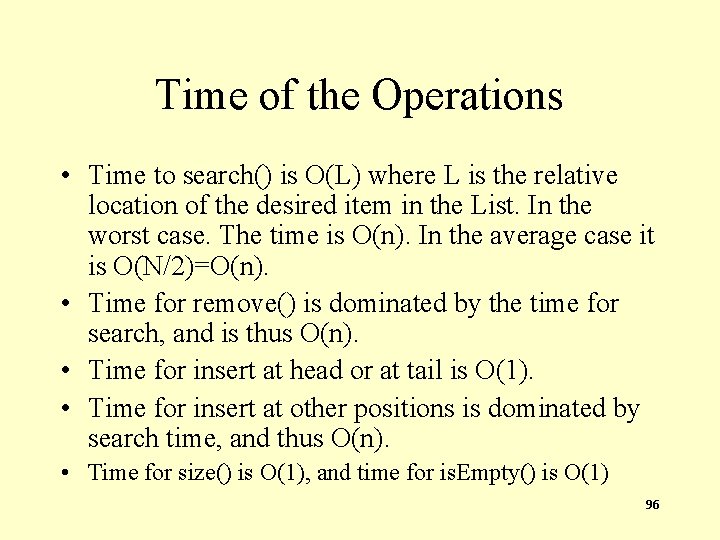
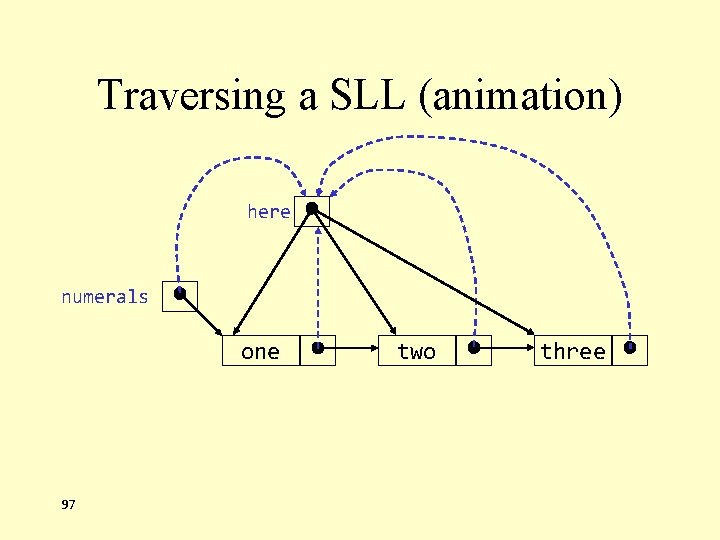
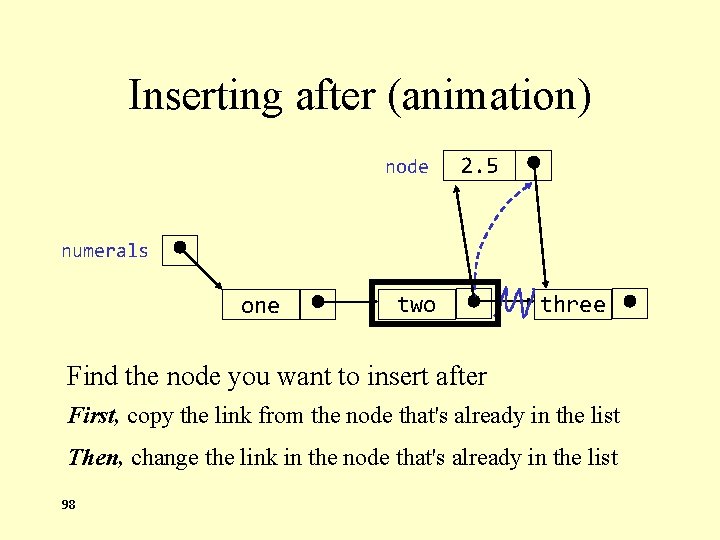
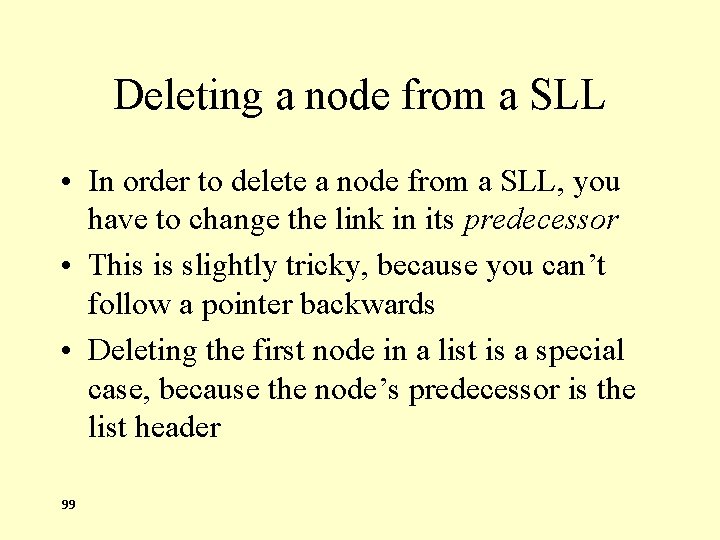
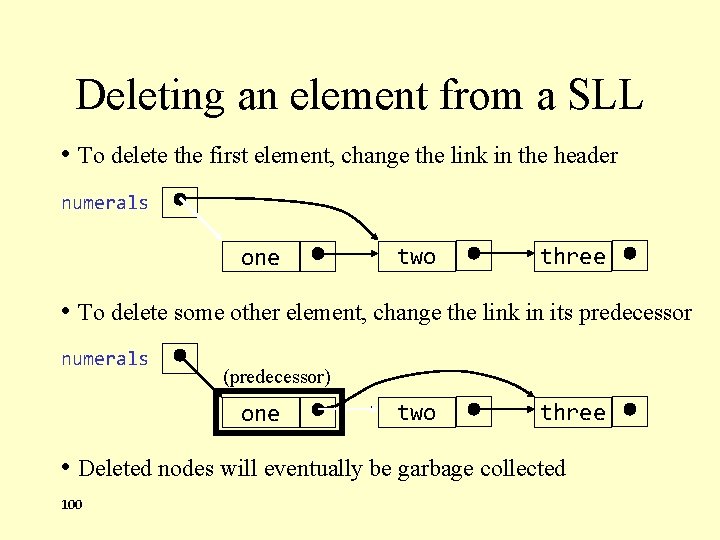
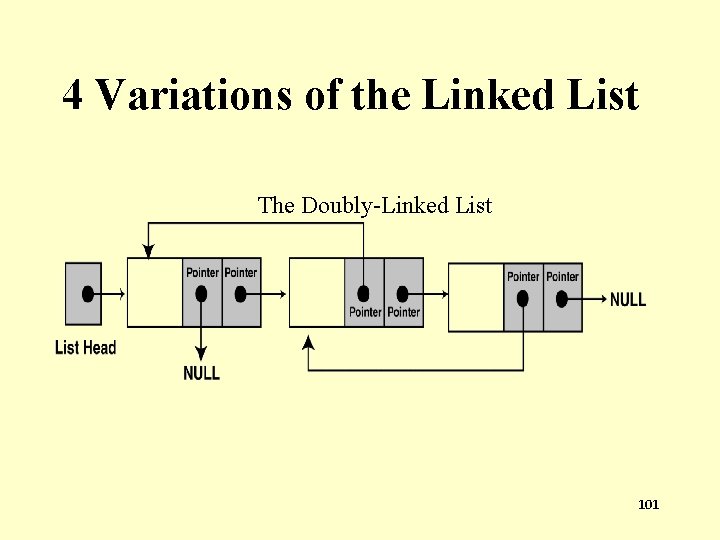
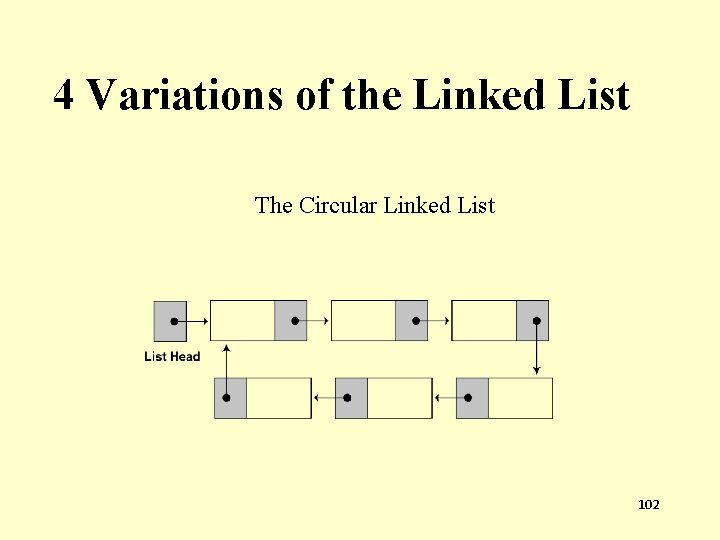
- Slides: 102
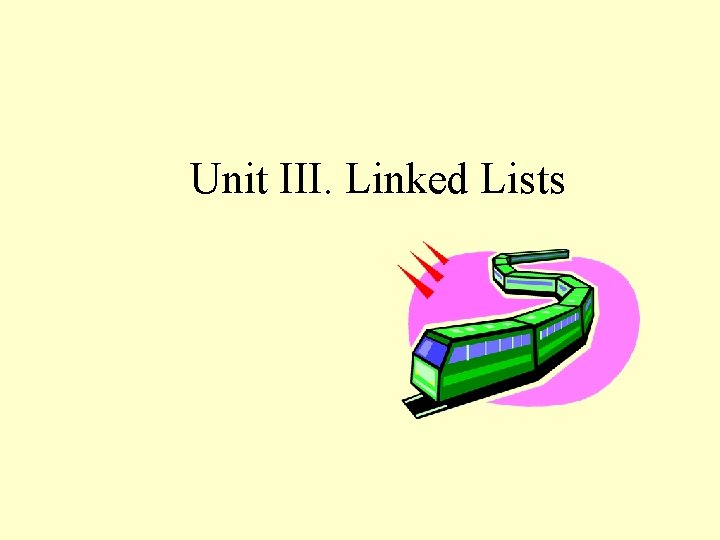
Unit III. Linked Lists
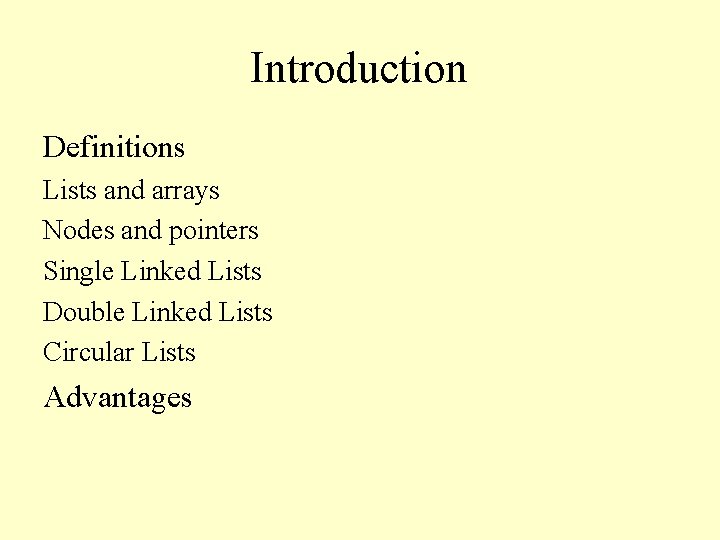
Introduction Definitions Lists and arrays Nodes and pointers Single Linked Lists Double Linked Lists Circular Lists Advantages
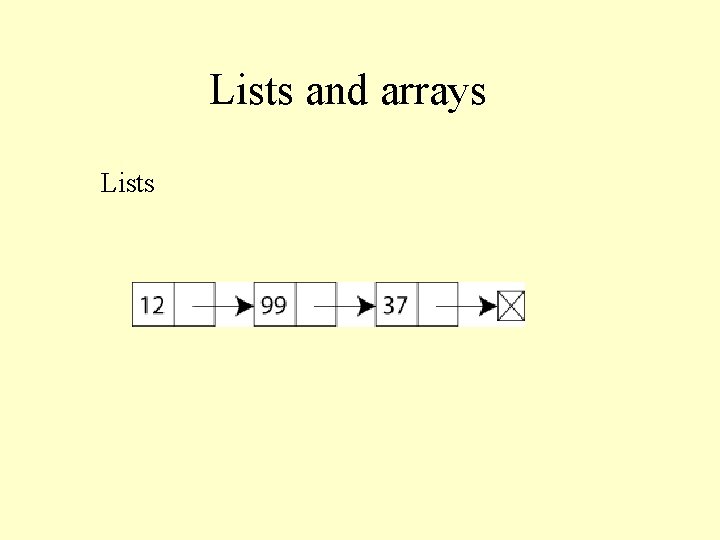
Lists and arrays Lists
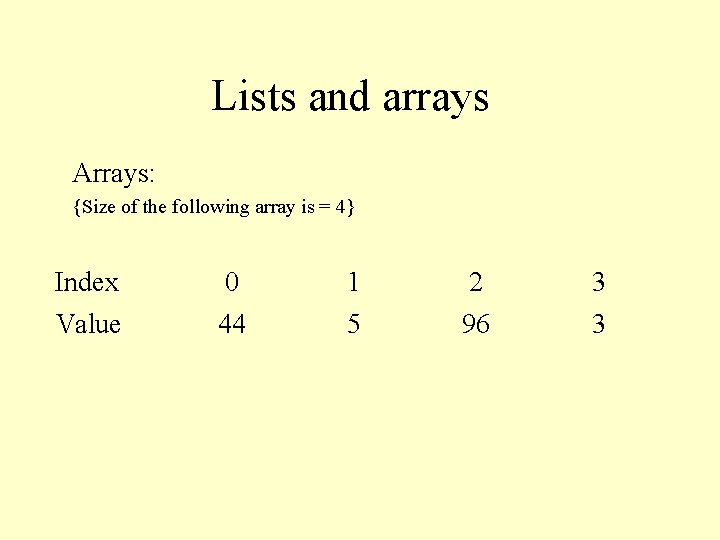
Lists and arrays Arrays: {Size of the following array is = 4} Index 0 1 2 3 Value 44 5 96 3
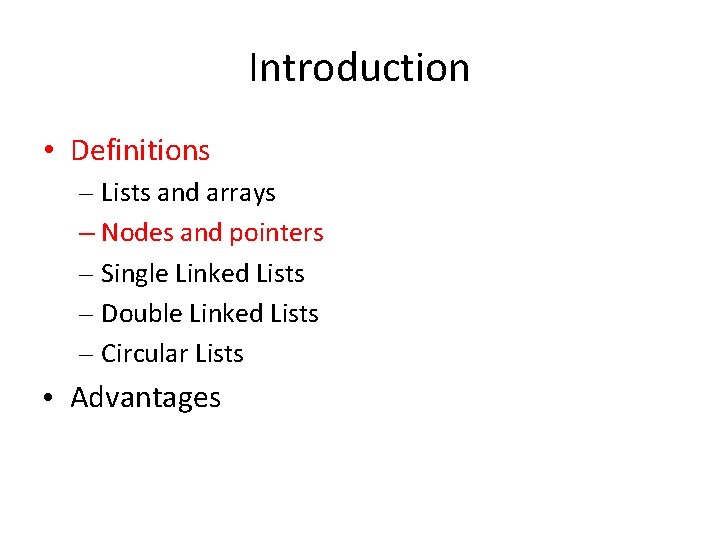
Introduction • Definitions – Lists and arrays – Nodes and pointers – Single Linked Lists – Double Linked Lists – Circular Lists • Advantages
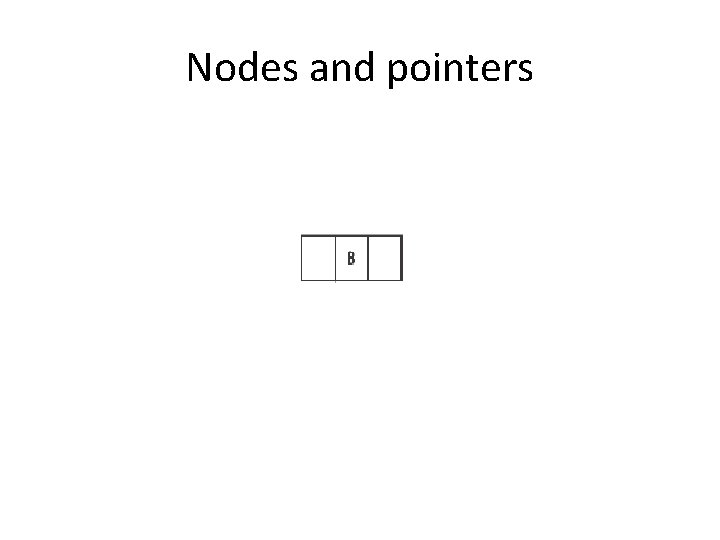
Nodes and pointers
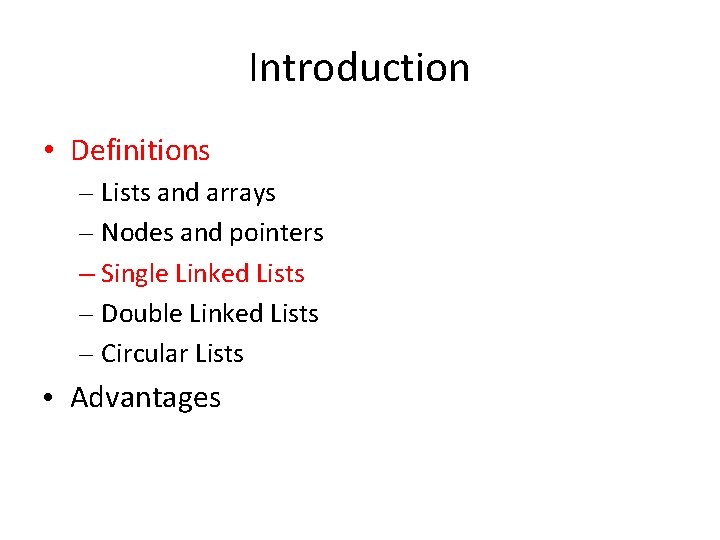
Introduction • Definitions – Lists and arrays – Nodes and pointers – Single Linked Lists – Double Linked Lists – Circular Lists • Advantages
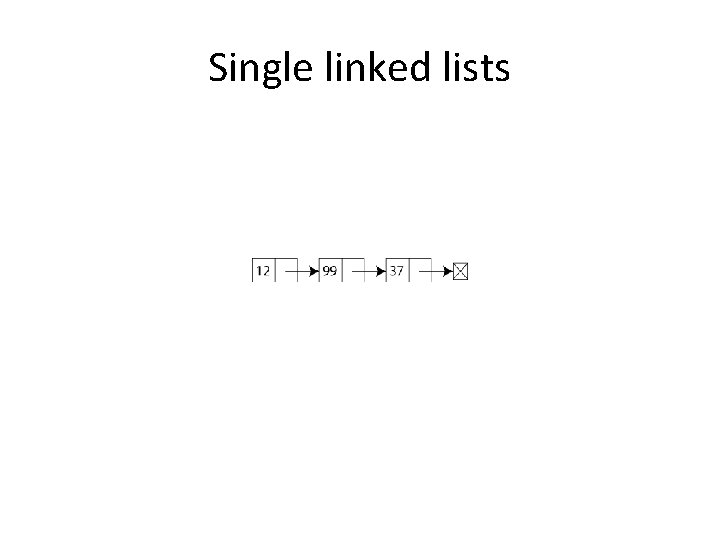
Single linked lists
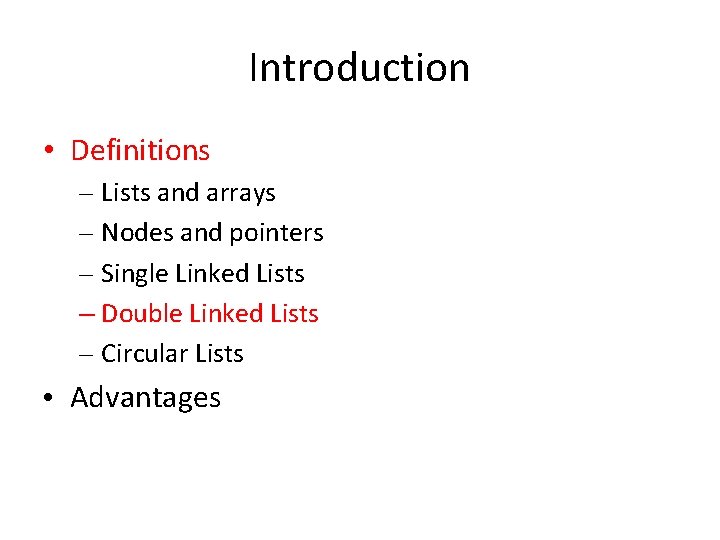
Introduction • Definitions – Lists and arrays – Nodes and pointers – Single Linked Lists – Double Linked Lists – Circular Lists • Advantages
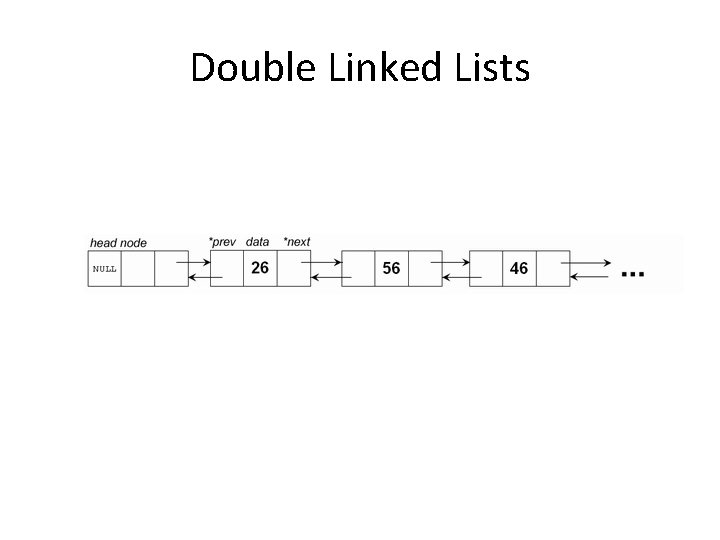
Double Linked Lists
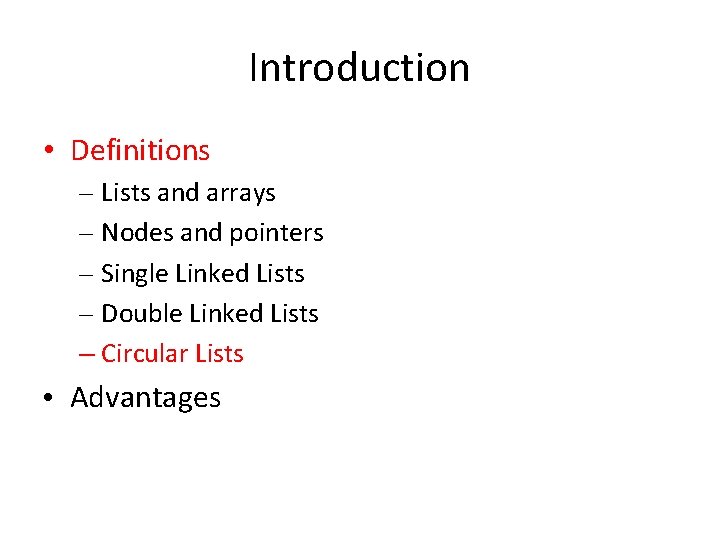
Introduction • Definitions – Lists and arrays – Nodes and pointers – Single Linked Lists – Double Linked Lists – Circular Lists • Advantages
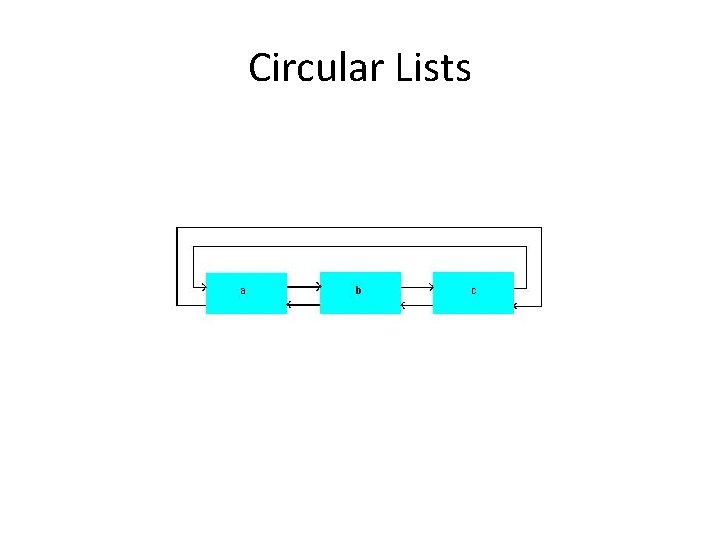
Circular Lists
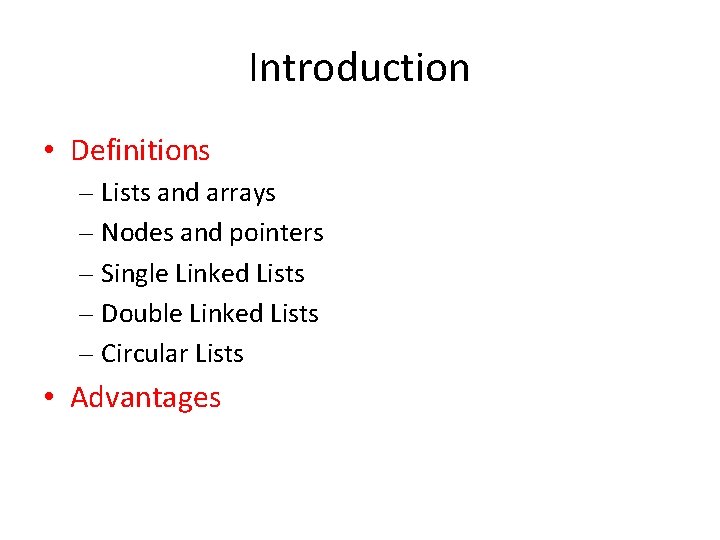
Introduction • Definitions – Lists and arrays – Nodes and pointers – Single Linked Lists – Double Linked Lists – Circular Lists • Advantages
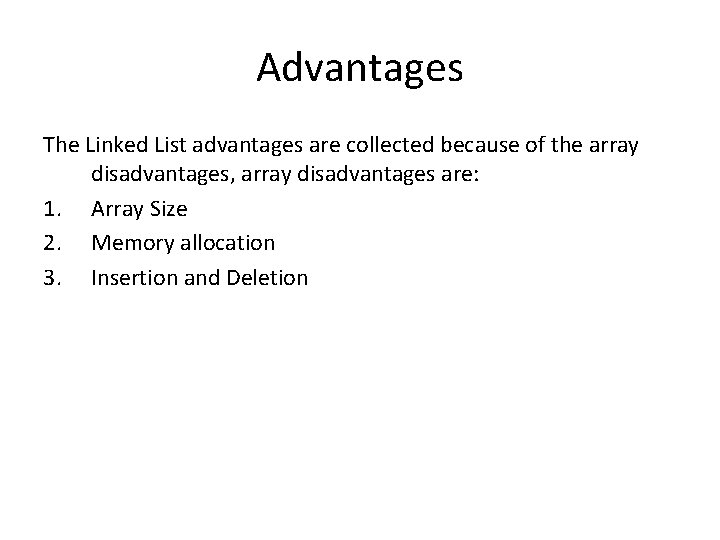
Advantages The Linked List advantages are collected because of the array disadvantages, array disadvantages are: 1. Array Size 2. Memory allocation 3. Insertion and Deletion
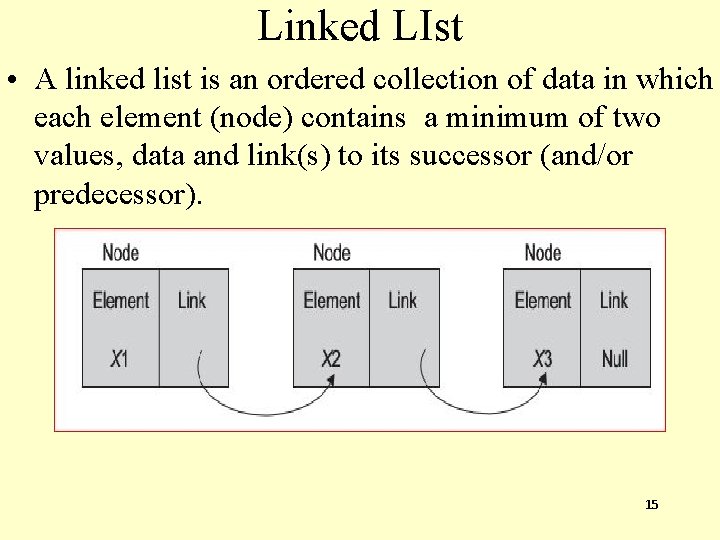
Linked LIst • A linked list is an ordered collection of data in which each element (node) contains a minimum of two values, data and link(s) to its successor (and/or predecessor). 15
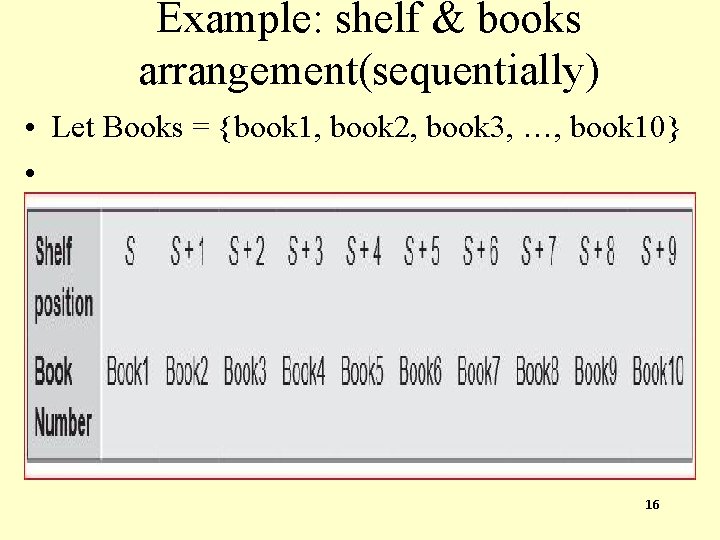
Example: shelf & books arrangement(sequentially) • Let Books = {book 1, book 2, book 3, …, book 10} • 16
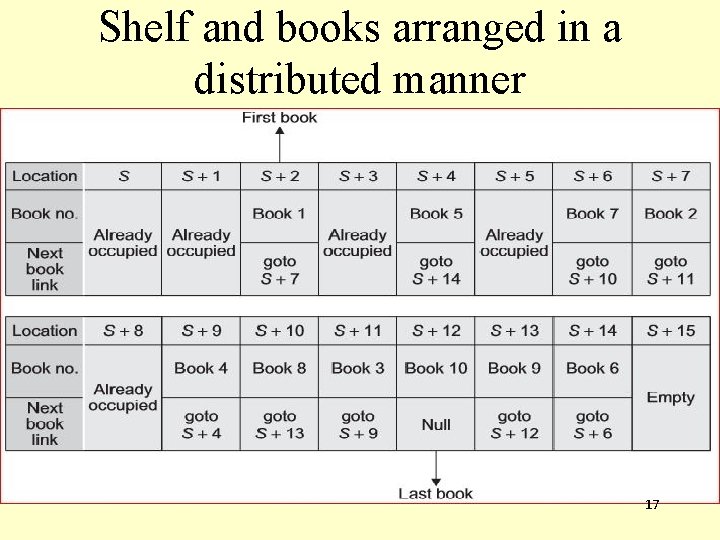
Shelf and books arranged in a distributed manner 17
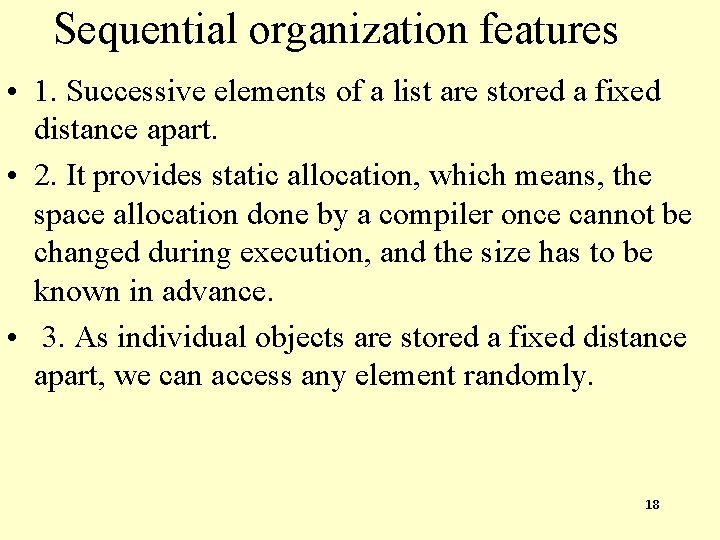
Sequential organization features • 1. Successive elements of a list are stored a fixed distance apart. • 2. It provides static allocation, which means, the space allocation done by a compiler once cannot be changed during execution, and the size has to be known in advance. • 3. As individual objects are stored a fixed distance apart, we can access any element randomly. 18
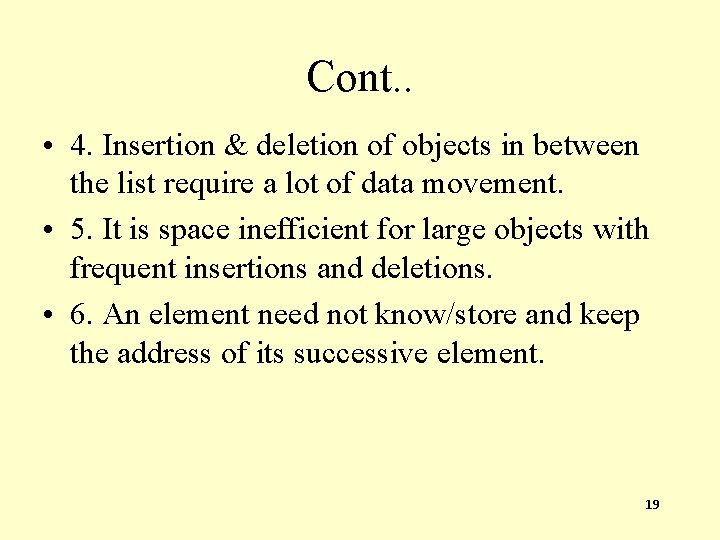
Cont. . • 4. Insertion & deletion of objects in between the list require a lot of data movement. • 5. It is space inefficient for large objects with frequent insertions and deletions. • 6. An element need not know/store and keep the address of its successive element. 19
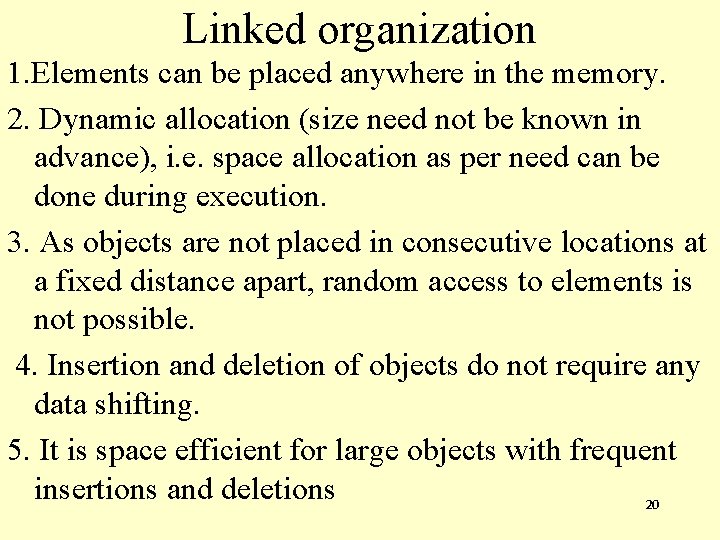
Linked organization 1. Elements can be placed anywhere in the memory. 2. Dynamic allocation (size need not be known in advance), i. e. space allocation as per need can be done during execution. 3. As objects are not placed in consecutive locations at a fixed distance apart, random access to elements is not possible. 4. Insertion and deletion of objects do not require any data shifting. 5. It is space efficient for large objects with frequent insertions and deletions 20
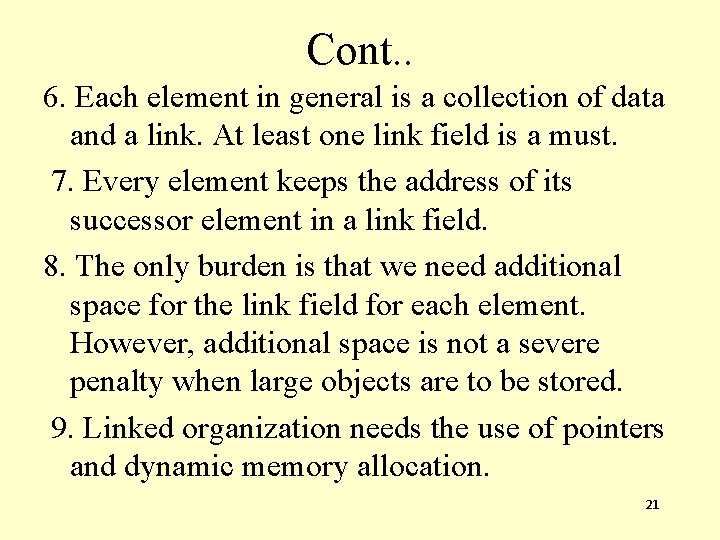
Cont. . 6. Each element in general is a collection of data and a link. At least one link field is a must. 7. Every element keeps the address of its successor element in a link field. 8. The only burden is that we need additional space for the link field for each element. However, additional space is not a severe penalty when large objects are to be stored. 9. Linked organization needs the use of pointers and dynamic memory allocation. 21
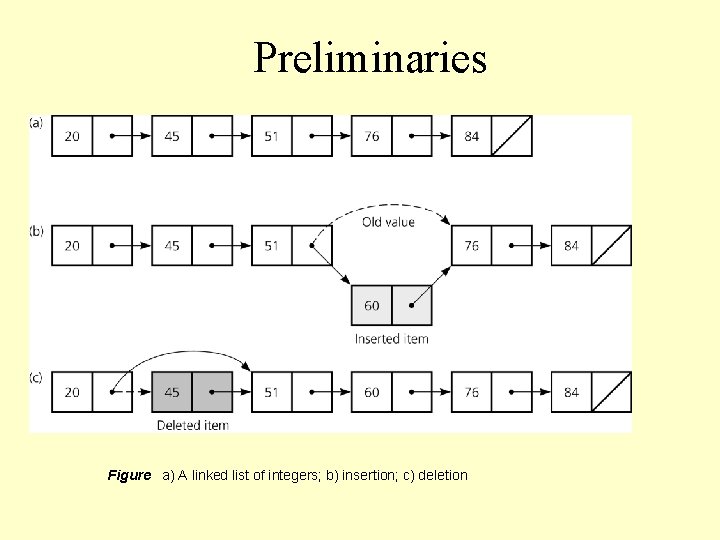
Preliminaries Figure a) A linked list of integers; b) insertion; c) deletion
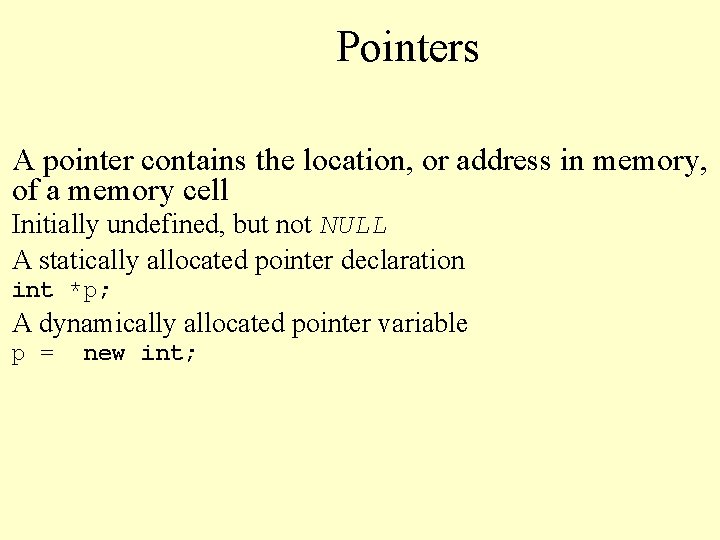
Pointers A pointer contains the location, or address in memory, of a memory cell Initially undefined, but not NULL A statically allocated pointer declaration int *p; A dynamically allocated pointer variable p = new int;
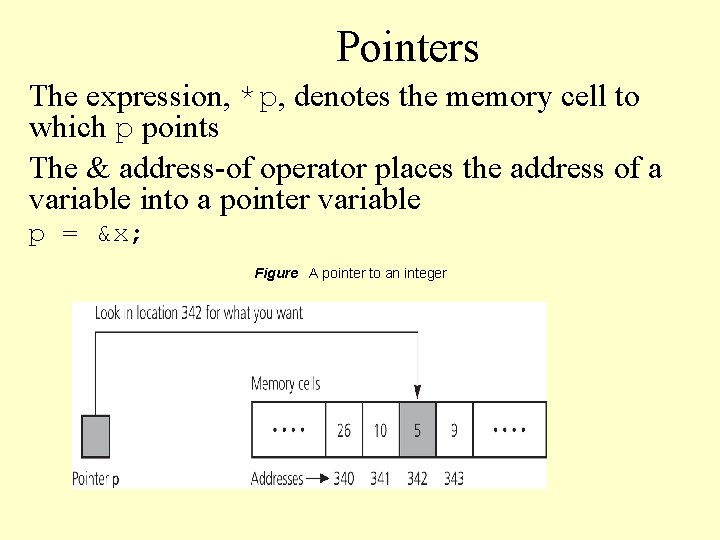
Pointers The expression, *p, denotes the memory cell to which p points The & address-of operator places the address of a variable into a pointer variable p = &x; Figure A pointer to an integer
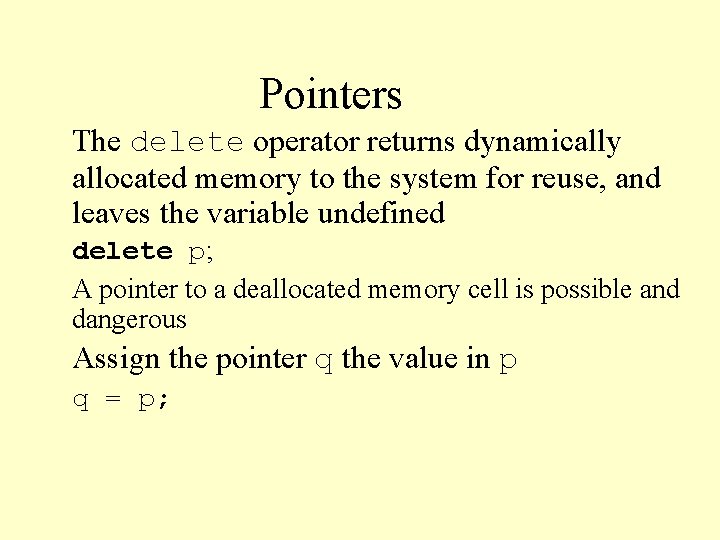
Pointers The delete operator returns dynamically allocated memory to the system for reuse, and leaves the variable undefined delete p; A pointer to a deallocated memory cell is possible and dangerous Assign the pointer q the value in p q = p;
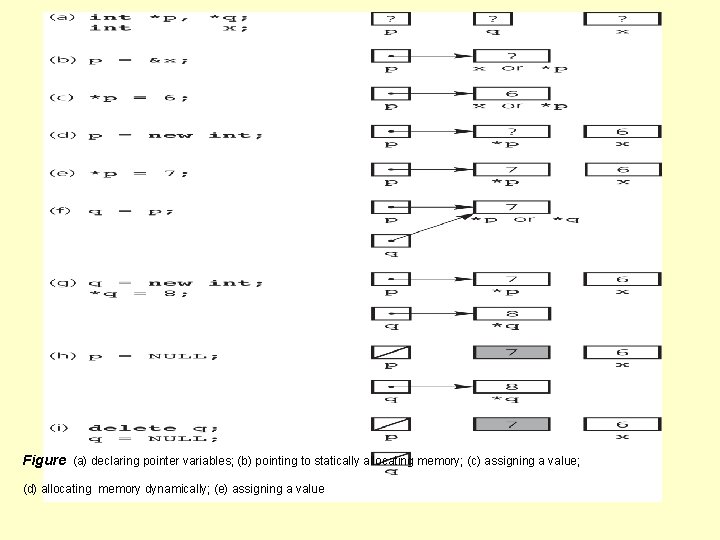
Pointers Figure (a) declaring pointer variables; (b) pointing to statically allocating memory; (c) assigning a value; (d) allocating memory dynamically; (e) assigning a value
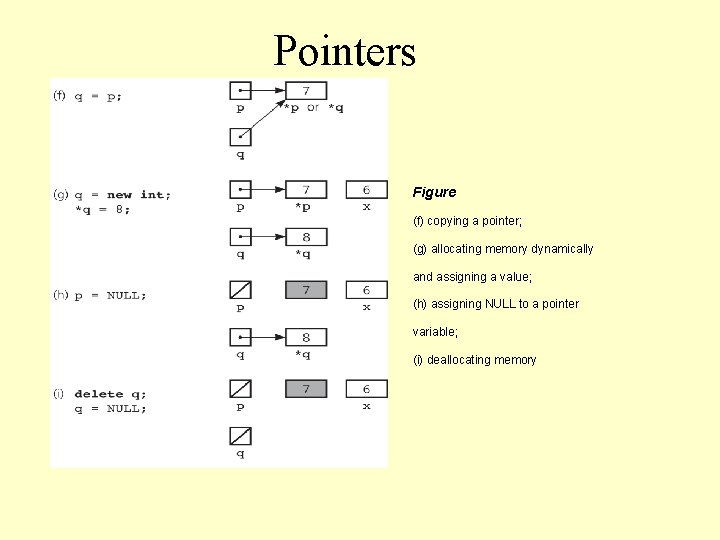
Pointers Figure (f) copying a pointer; (g) allocating memory dynamically and assigning a value; (h) assigning NULL to a pointer variable; (i) deallocating memory
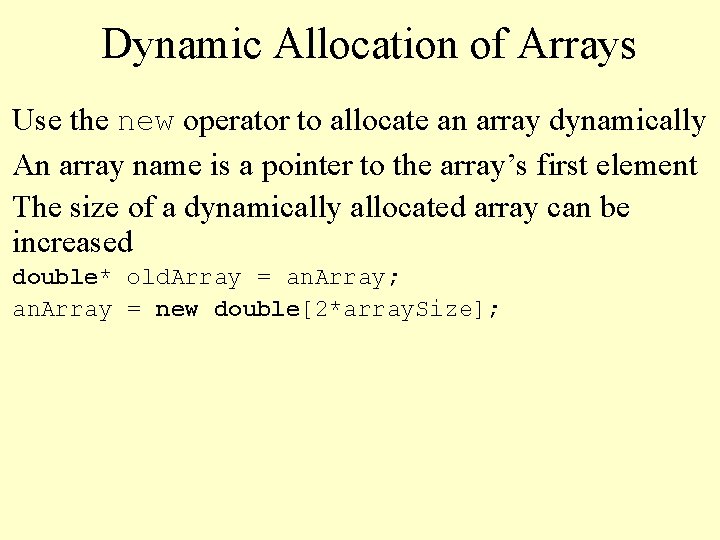
Dynamic Allocation of Arrays Use the new operator to allocate an array dynamically An array name is a pointer to the array’s first element The size of a dynamically allocated array can be increased double* old. Array = an. Array; an. Array = new double[2*array. Size];
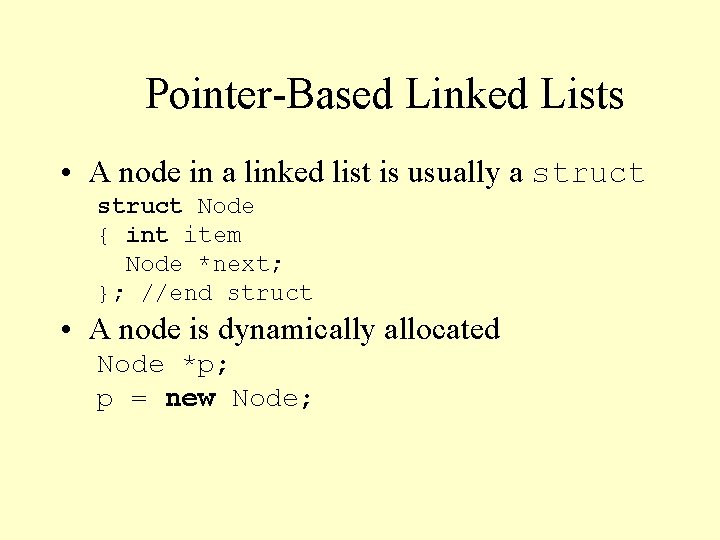
Pointer-Based Linked Lists • A node in a linked list is usually a struct Node { int item Node *next; }; //end struct • A node is dynamically allocated Node *p; p = new Node;
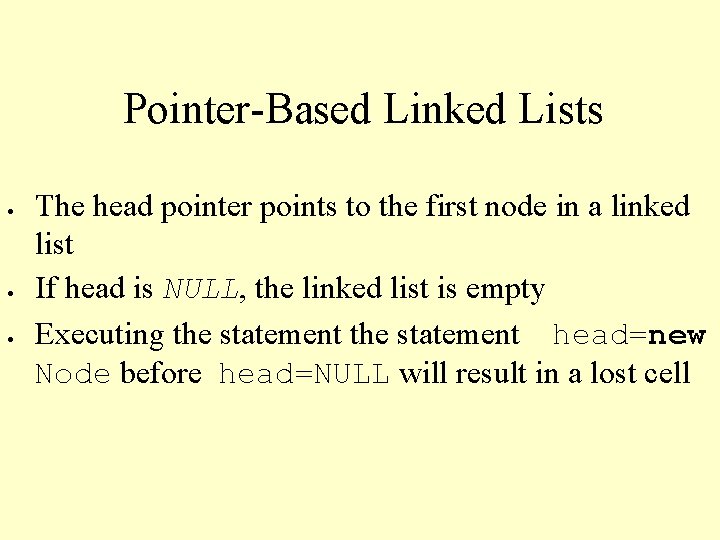
Pointer-Based Linked Lists The head pointer points to the first node in a linked list If head is NULL, the linked list is empty Executing the statement head=new Node before head=NULL will result in a lost cell
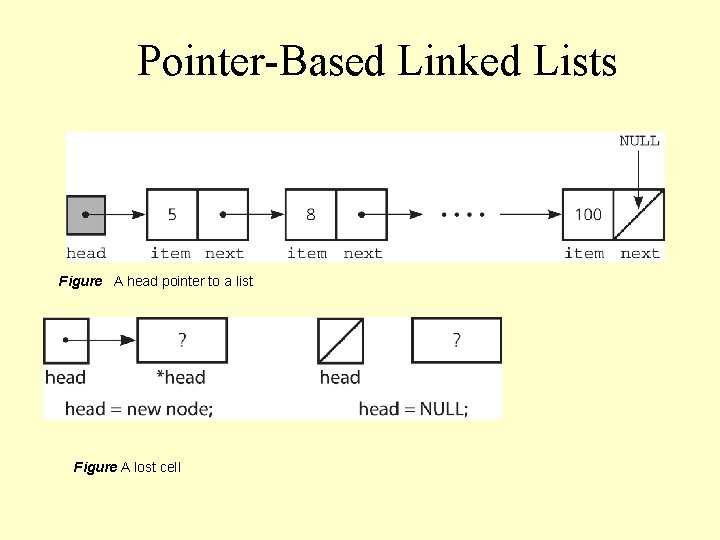
Pointer-Based Linked Lists Figure A head pointer to a list Figure A lost cell
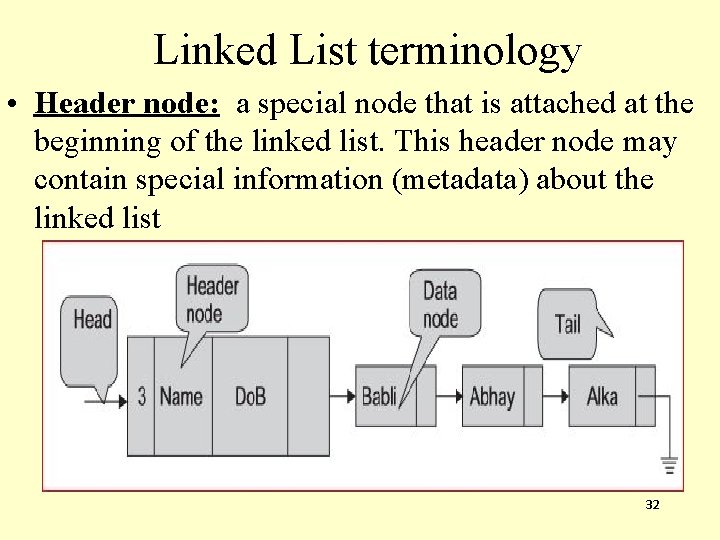
Linked List terminology • Header node: a special node that is attached at the beginning of the linked list. This header node may contain special information (metadata) about the linked list 32
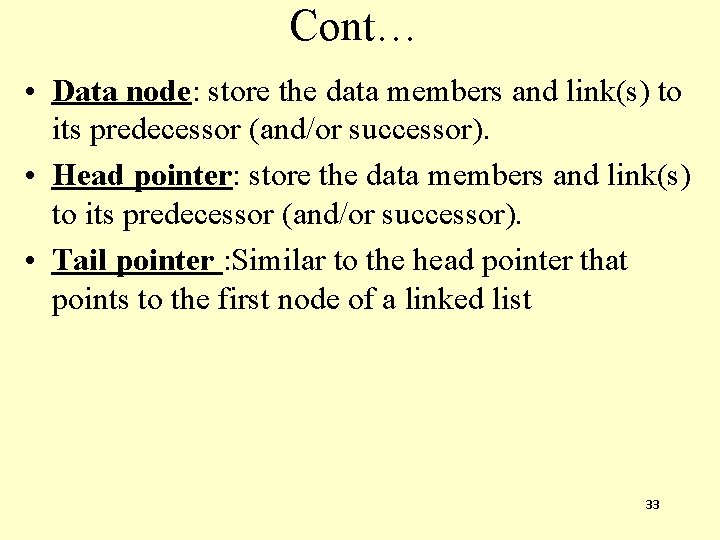
Cont… • Data node: store the data members and link(s) to its predecessor (and/or successor). • Head pointer: store the data members and link(s) to its predecessor (and/or successor). • Tail pointer : Similar to the head pointer that points to the first node of a linked list 33
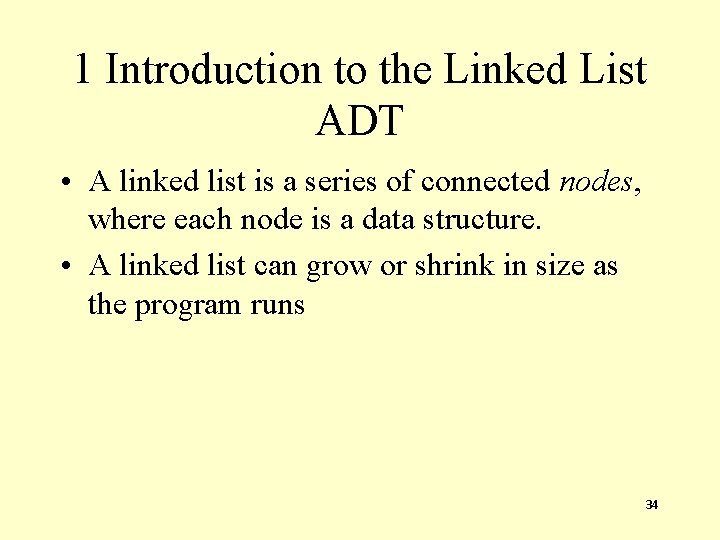
1 Introduction to the Linked List ADT • A linked list is a series of connected nodes, where each node is a data structure. • A linked list can grow or shrink in size as the program runs 34
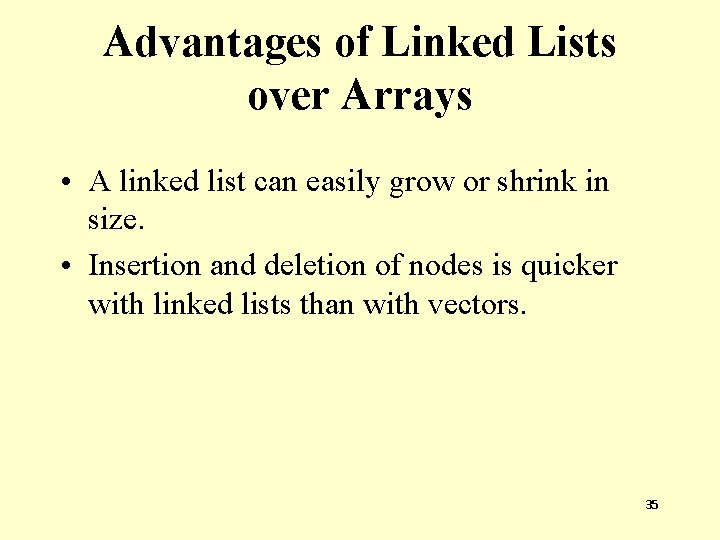
Advantages of Linked Lists over Arrays • A linked list can easily grow or shrink in size. • Insertion and deletion of nodes is quicker with linked lists than with vectors. 35
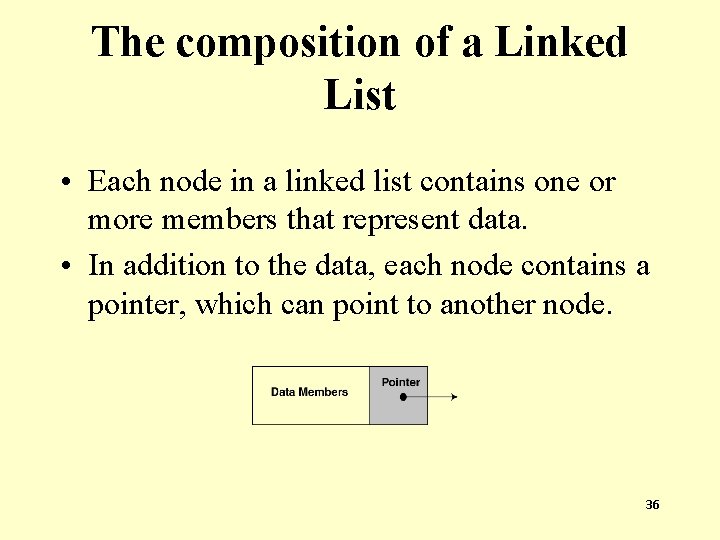
The composition of a Linked List • Each node in a linked list contains one or more members that represent data. • In addition to the data, each node contains a pointer, which can point to another node. 36
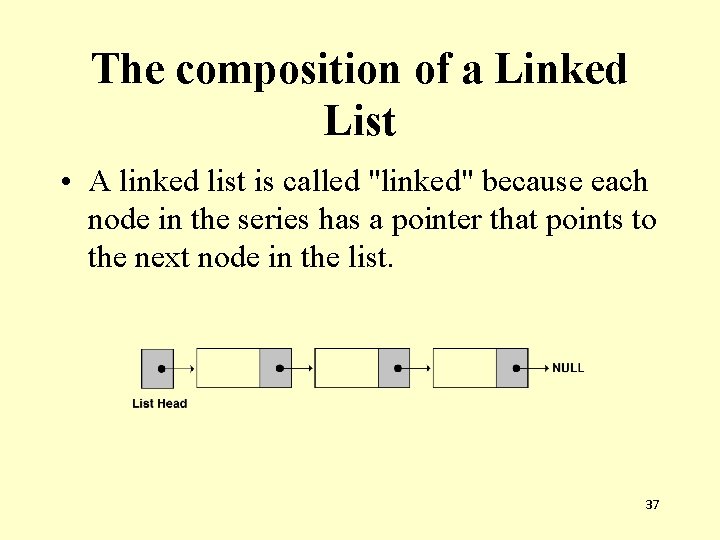
The composition of a Linked List • A linked list is called "linked" because each node in the series has a pointer that points to the next node in the list. 37
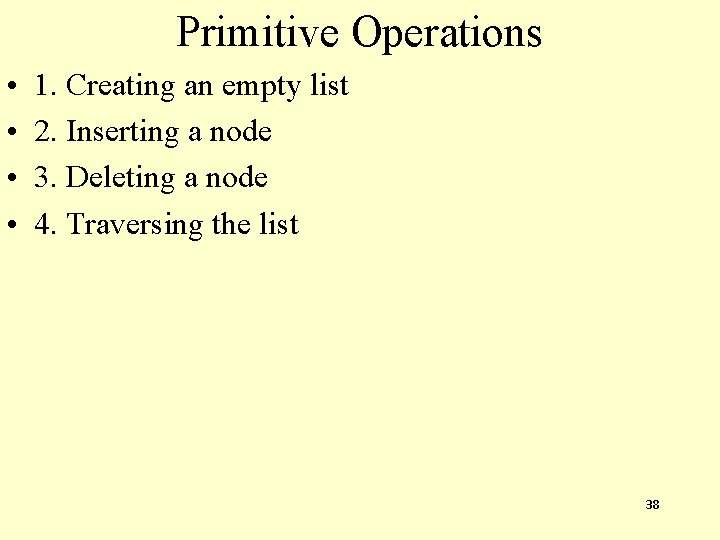
Primitive Operations • • 1. Creating an empty list 2. Inserting a node 3. Deleting a node 4. Traversing the list 38
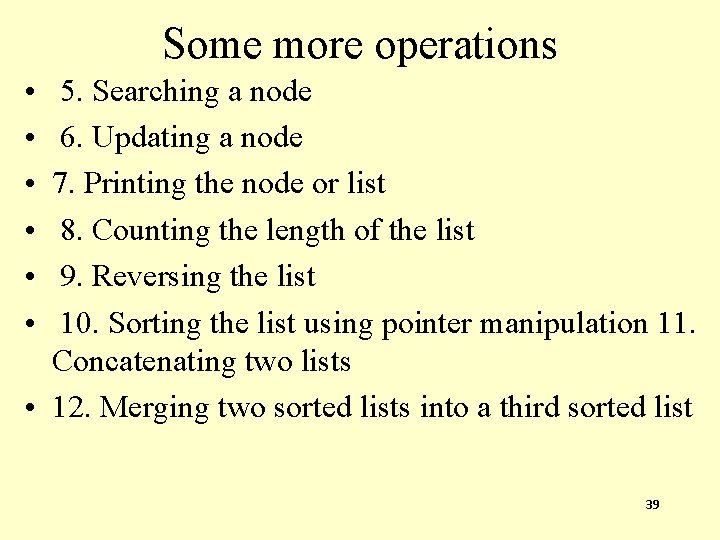
Some more operations • • • 5. Searching a node 6. Updating a node 7. Printing the node or list 8. Counting the length of the list 9. Reversing the list 10. Sorting the list using pointer manipulation 11. Concatenating two lists • 12. Merging two sorted lists into a third sorted list 39
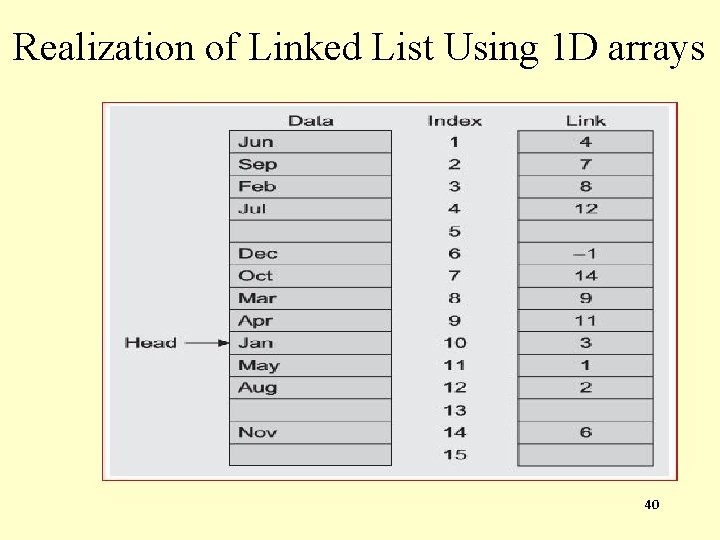
Realization of Linked List Using 1 D arrays 40
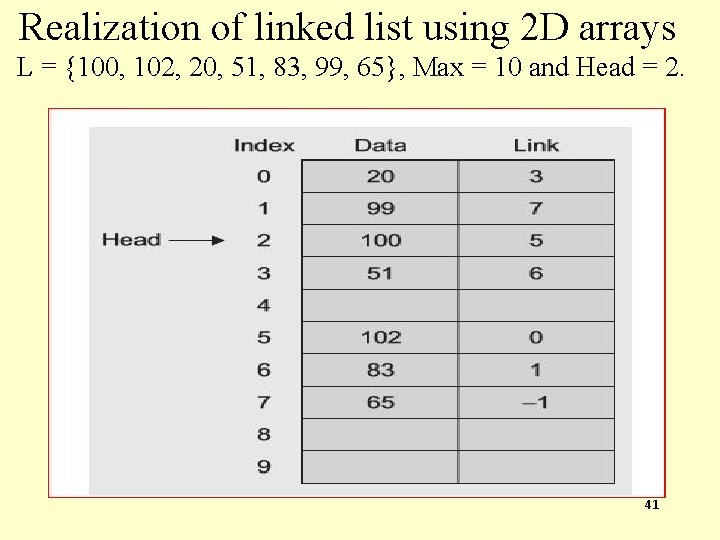
Realization of linked list using 2 D arrays L = {100, 102, 20, 51, 83, 99, 65}, Max = 10 and Head = 2. 41
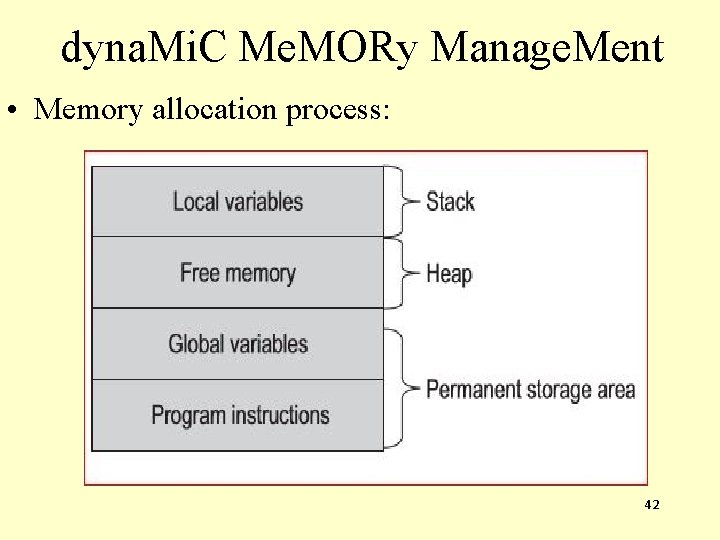
dyna. Mi. C Me. MORy Manage. Ment • Memory allocation process: 42
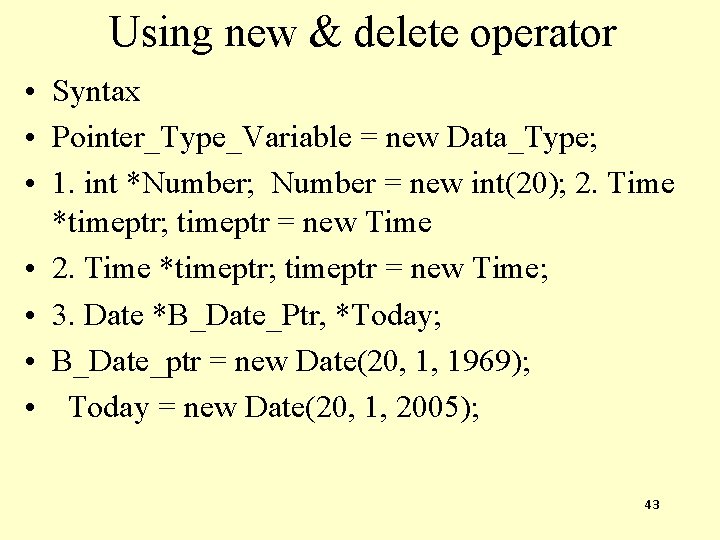
Using new & delete operator • Syntax • Pointer_Type_Variable = new Data_Type; • 1. int *Number; Number = new int(20); 2. Time *timeptr; timeptr = new Time • 2. Time *timeptr; timeptr = new Time; • 3. Date *B_Date_Ptr, *Today; • B_Date_ptr = new Date(20, 1, 1969); • Today = new Date(20, 1, 2005); 43
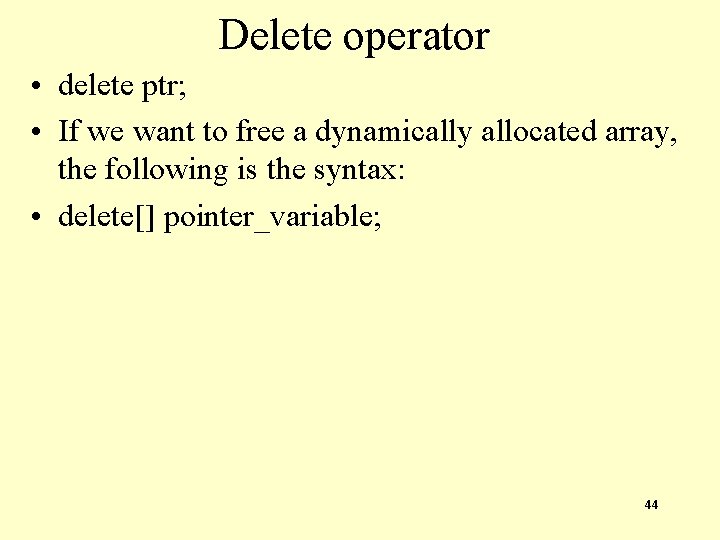
Delete operator • delete ptr; • If we want to free a dynamically allocated array, the following is the syntax: • delete[] pointer_variable; 44
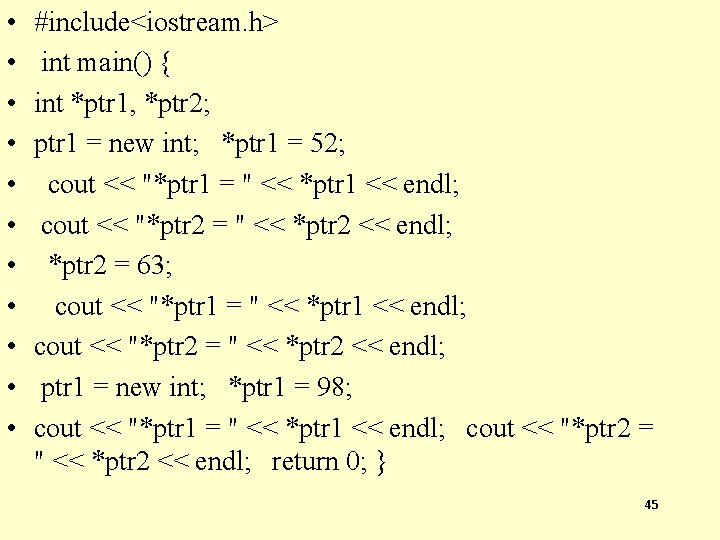
• • • #include<iostream. h> int main() { int *ptr 1, *ptr 2; ptr 1 = new int; *ptr 1 = 52; cout << "*ptr 1 = " << *ptr 1 << endl; cout << "*ptr 2 = " << *ptr 2 << endl; *ptr 2 = 63; cout << "*ptr 1 = " << *ptr 1 << endl; cout << "*ptr 2 = " << *ptr 2 << endl; ptr 1 = new int; *ptr 1 = 98; cout << "*ptr 1 = " << *ptr 1 << endl; cout << "*ptr 2 = " << *ptr 2 << endl; return 0; } 45
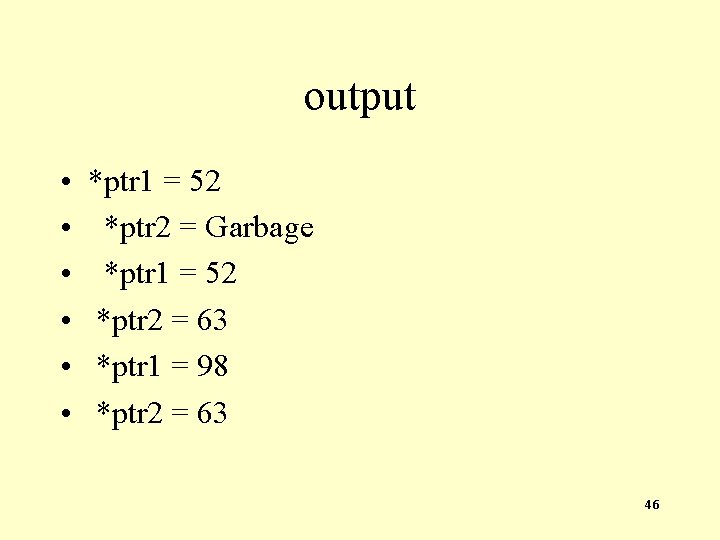
output • • • *ptr 1 = 52 *ptr 2 = Garbage *ptr 1 = 52 *ptr 2 = 63 *ptr 1 = 98 *ptr 2 = 63 46
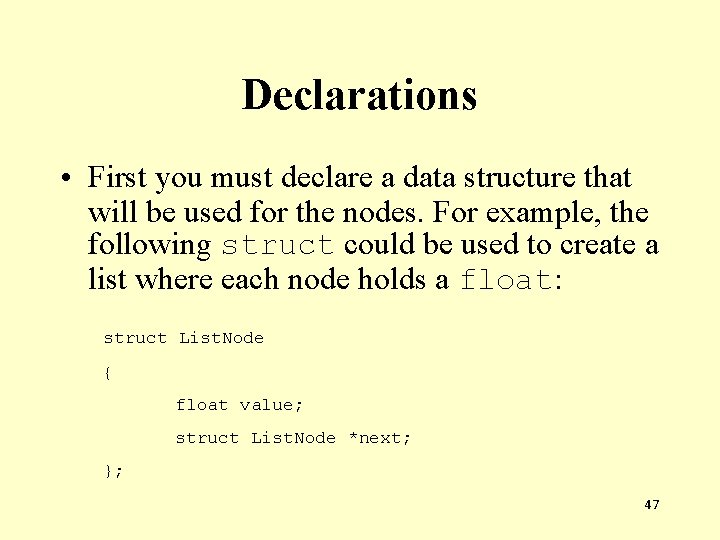
Declarations • First you must declare a data structure that will be used for the nodes. For example, the following struct could be used to create a list where each node holds a float: struct List. Node { float value; struct List. Node *next; }; 47
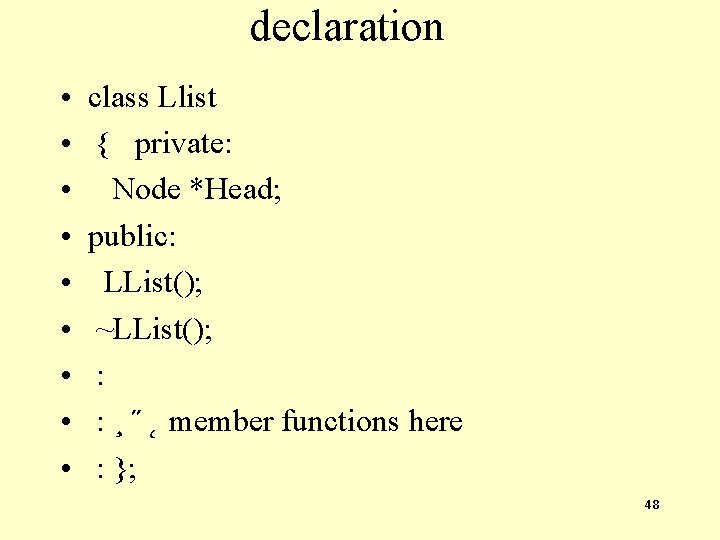
declaration • • • class Llist { private: Node *Head; public: LList(); ~LList(); : : ¸ ˝ ˛ member functions here : }; 48
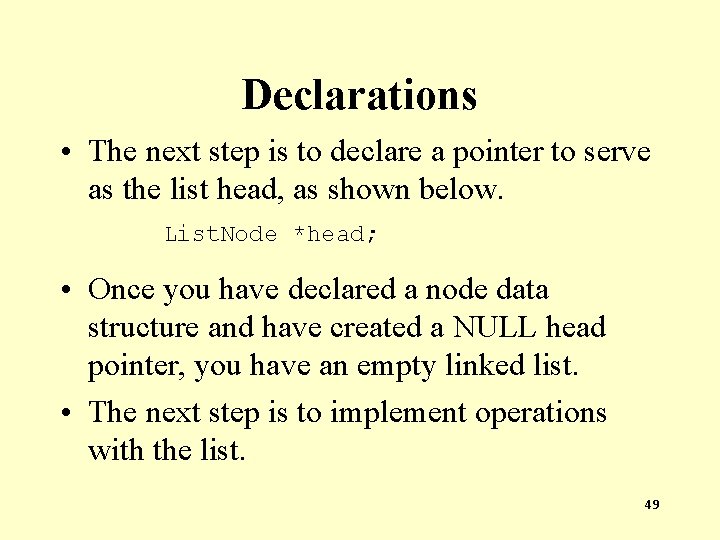
Declarations • The next step is to declare a pointer to serve as the list head, as shown below. List. Node *head; • Once you have declared a node data structure and have created a NULL head pointer, you have an empty linked list. • The next step is to implement operations with the list. 49
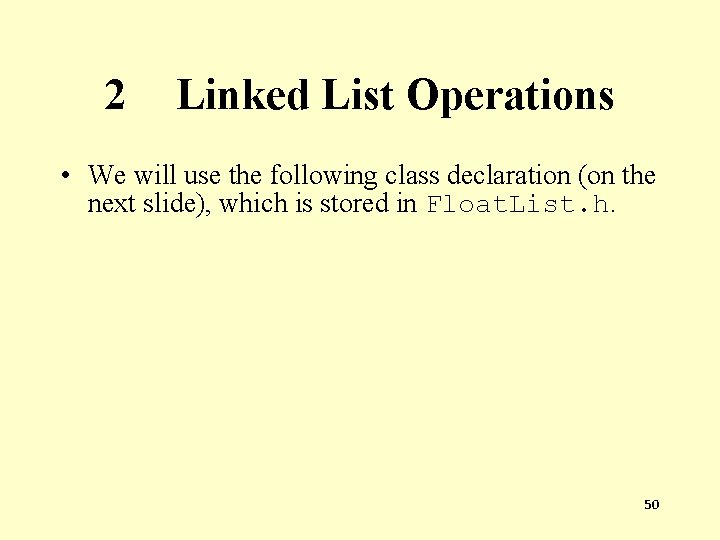
2 Linked List Operations • We will use the following class declaration (on the next slide), which is stored in Float. List. h. 50
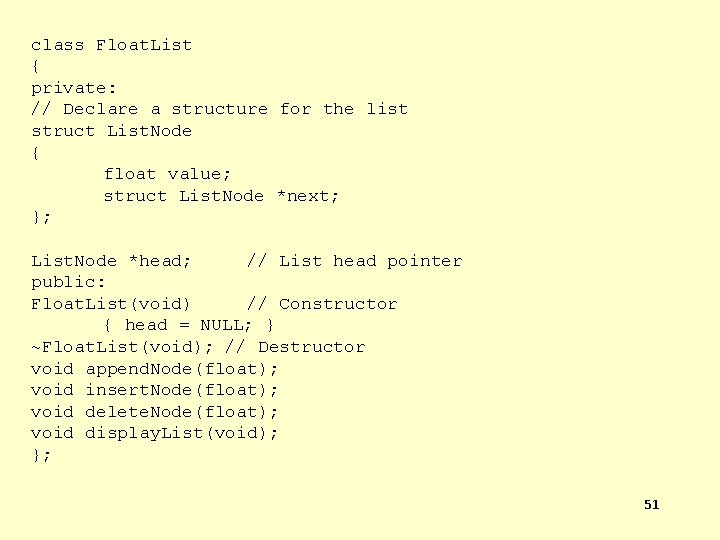
class Float. List { private: // Declare a structure for the list struct List. Node { float value; struct List. Node *next; }; List. Node *head; // List head pointer public: Float. List(void) // Constructor { head = NULL; } ~Float. List(void); // Destructor void append. Node(float); void insert. Node(float); void delete. Node(float); void display. List(void); }; 51
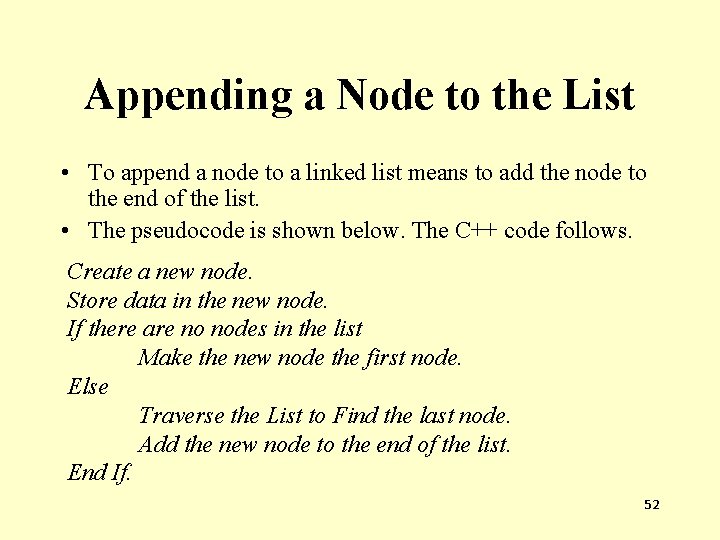
Appending a Node to the List • To append a node to a linked list means to add the node to the end of the list. • The pseudocode is shown below. The C++ code follows. Create a new node. Store data in the new node. If there are no nodes in the list Make the new node the first node. Else Traverse the List to Find the last node. Add the new node to the end of the list. End If. 52
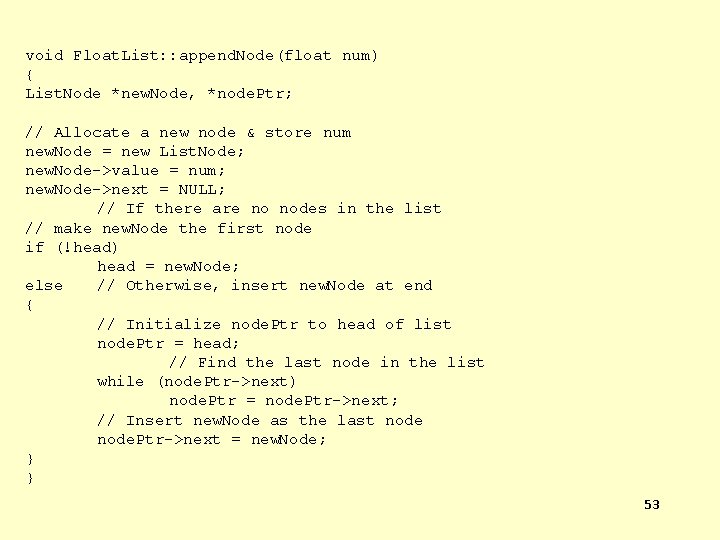
void Float. List: : append. Node(float num) { List. Node *new. Node, *node. Ptr; // Allocate a new node & store num new. Node = new List. Node; new. Node->value = num; new. Node->next = NULL; // If there are no nodes in the list // make new. Node the first node if (!head) head = new. Node; else // Otherwise, insert new. Node at end { // Initialize node. Ptr to head of list node. Ptr = head; // Find the last node in the list while (node. Ptr->next) node. Ptr = node. Ptr->next; // Insert new. Node as the last node. Ptr->next = new. Node; } } 53
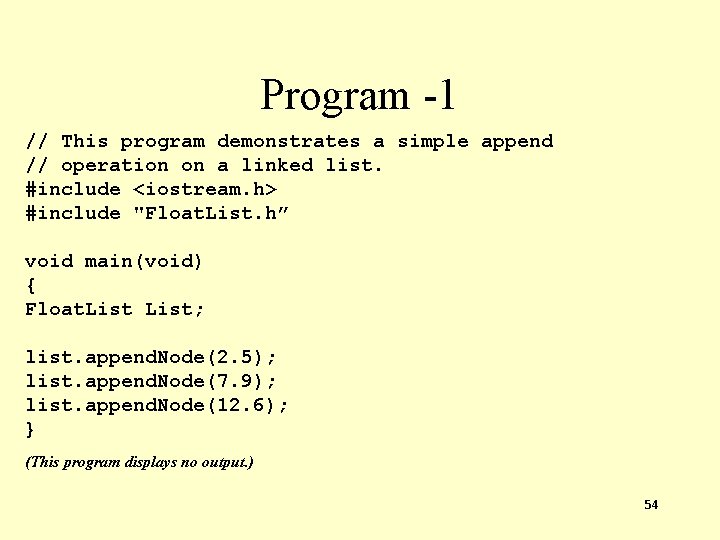
Program -1 // This program demonstrates a simple append // operation on a linked list. #include <iostream. h> #include "Float. List. h” void main(void) { Float. List; list. append. Node(2. 5); list. append. Node(7. 9); list. append. Node(12. 6); } (This program displays no output. ) 54
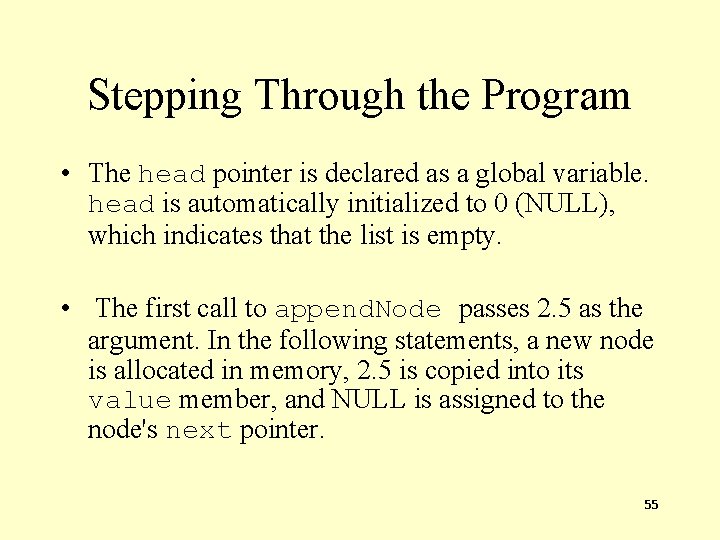
Stepping Through the Program • The head pointer is declared as a global variable. head is automatically initialized to 0 (NULL), which indicates that the list is empty. • The first call to append. Node passes 2. 5 as the argument. In the following statements, a new node is allocated in memory, 2. 5 is copied into its value member, and NULL is assigned to the node's next pointer. 55
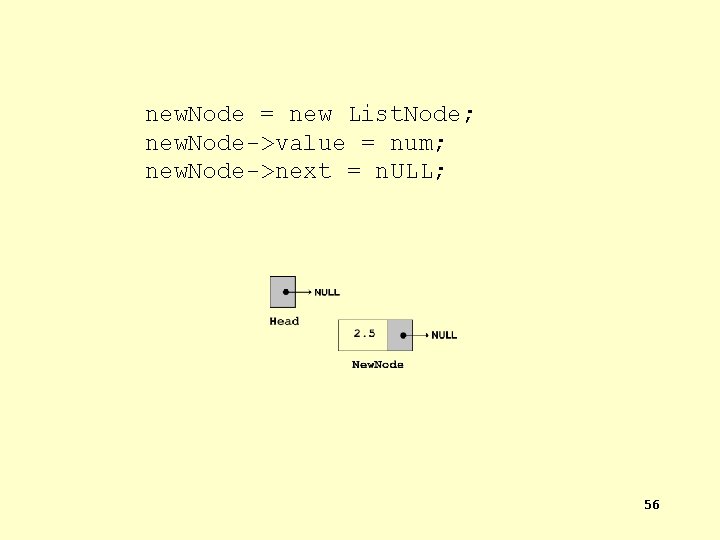
new. Node = new List. Node; new. Node->value = num; new. Node->next = n. ULL; 56
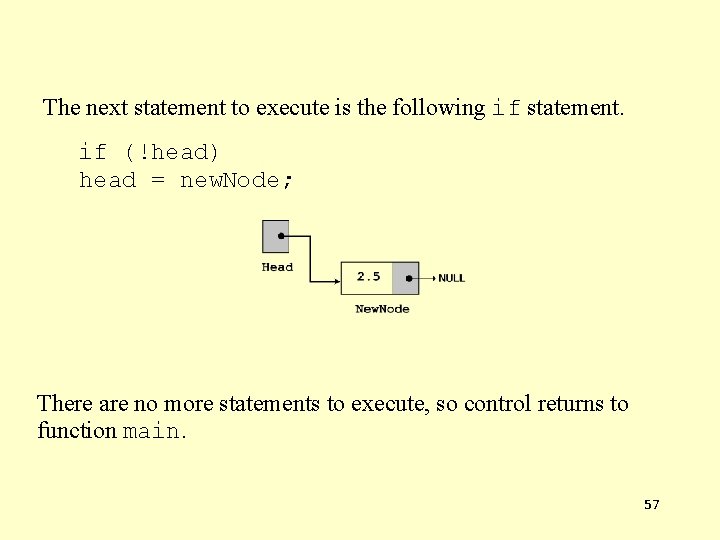
The next statement to execute is the following if statement. if (!head) head = new. Node; There are no more statements to execute, so control returns to function main. 57
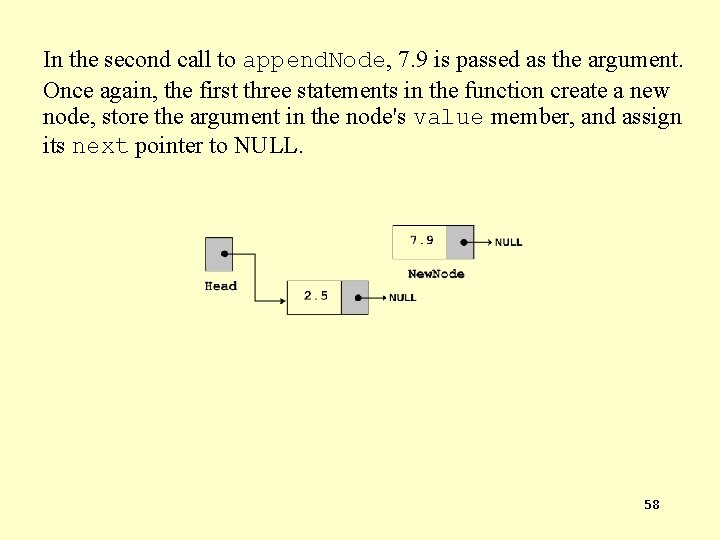
In the second call to append. Node, 7. 9 is passed as the argument. Once again, the first three statements in the function create a new node, store the argument in the node's value member, and assign its next pointer to NULL. 58
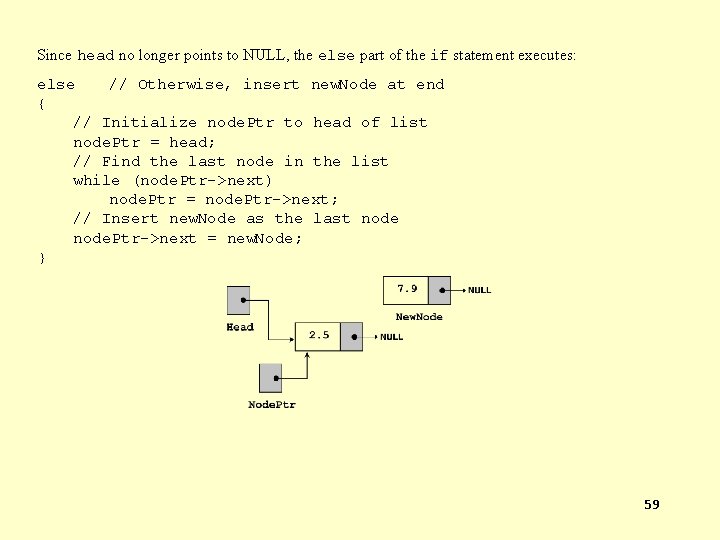
Since head no longer points to NULL, the else part of the if statement executes: else // Otherwise, insert new. Node at end { // Initialize node. Ptr to head of list node. Ptr = head; // Find the last node in the list while (node. Ptr->next) node. Ptr = node. Ptr->next; // Insert new. Node as the last node. Ptr->next = new. Node; } 59
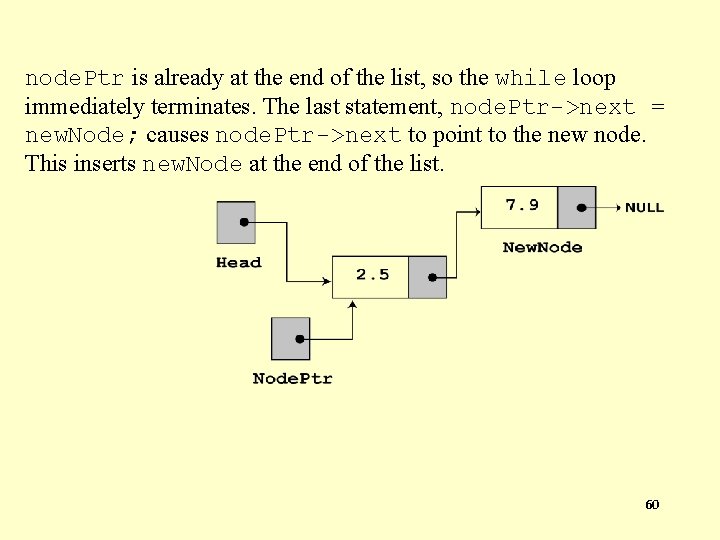
node. Ptr is already at the end of the list, so the while loop immediately terminates. The last statement, node. Ptr->next = new. Node; causes node. Ptr->next to point to the new node. This inserts new. Node at the end of the list. 60
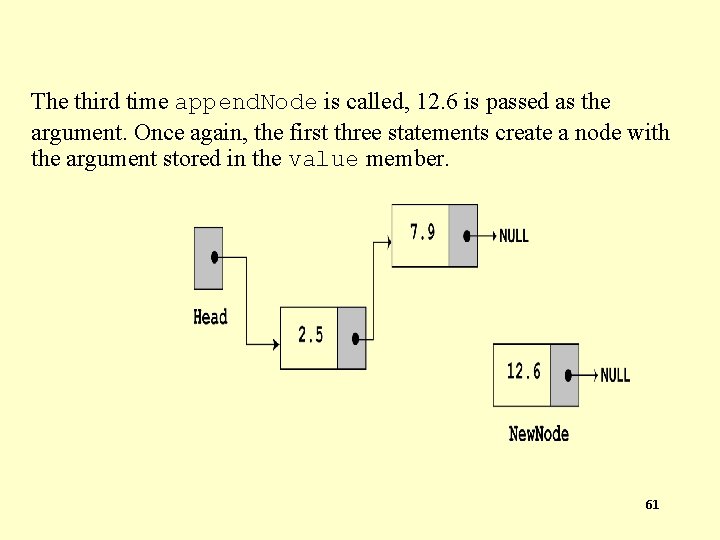
The third time append. Node is called, 12. 6 is passed as the argument. Once again, the first three statements create a node with the argument stored in the value member. 61
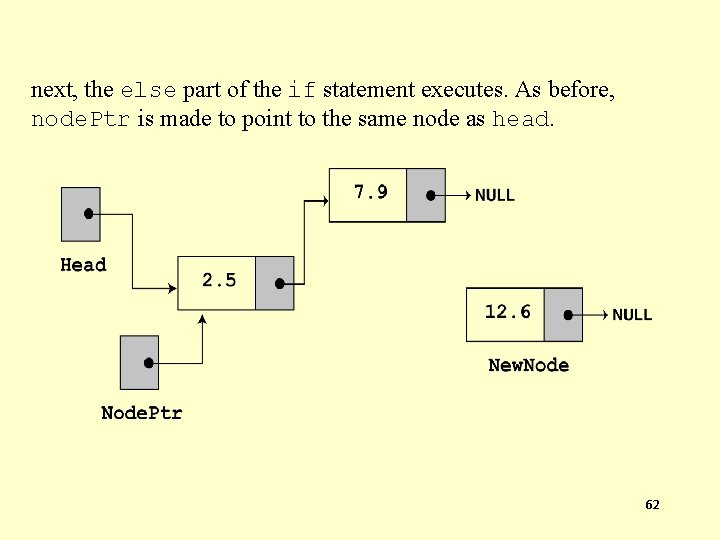
next, the else part of the if statement executes. As before, node. Ptr is made to point to the same node as head. 62
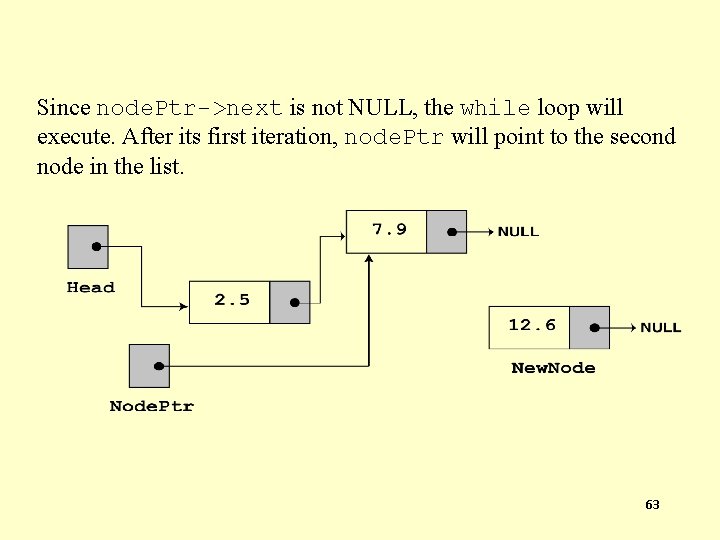
Since node. Ptr->next is not NULL, the while loop will execute. After its first iteration, node. Ptr will point to the second node in the list. 63
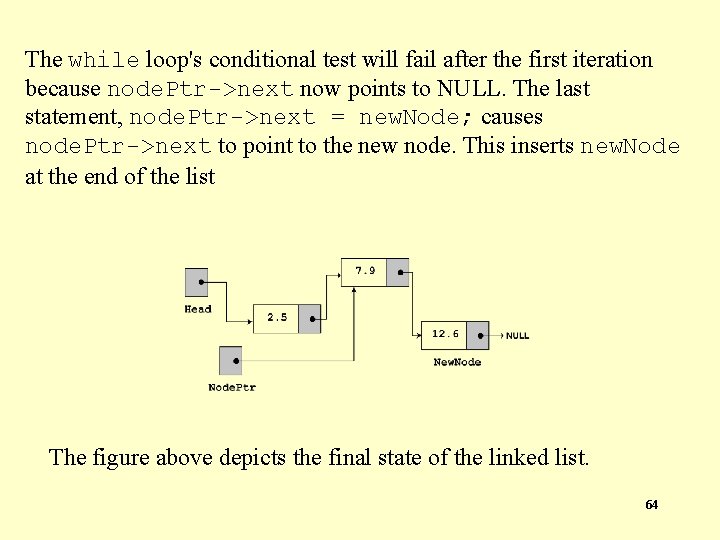
The while loop's conditional test will fail after the first iteration because node. Ptr->next now points to NULL. The last statement, node. Ptr->next = new. Node; causes node. Ptr->next to point to the new node. This inserts new. Node at the end of the list The figure above depicts the final state of the linked list. 64
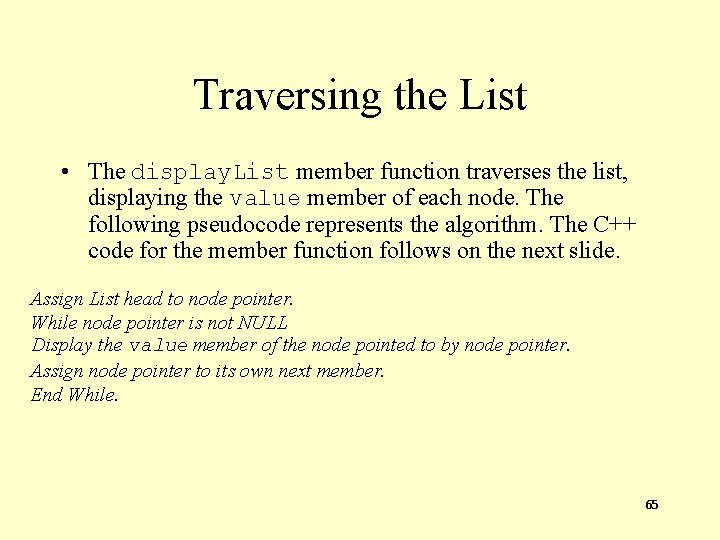
Traversing the List • The display. List member function traverses the list, displaying the value member of each node. The following pseudocode represents the algorithm. The C++ code for the member function follows on the next slide. Assign List head to node pointer. While node pointer is not NULL Display the value member of the node pointed to by node pointer. Assign node pointer to its own next member. End While. 65
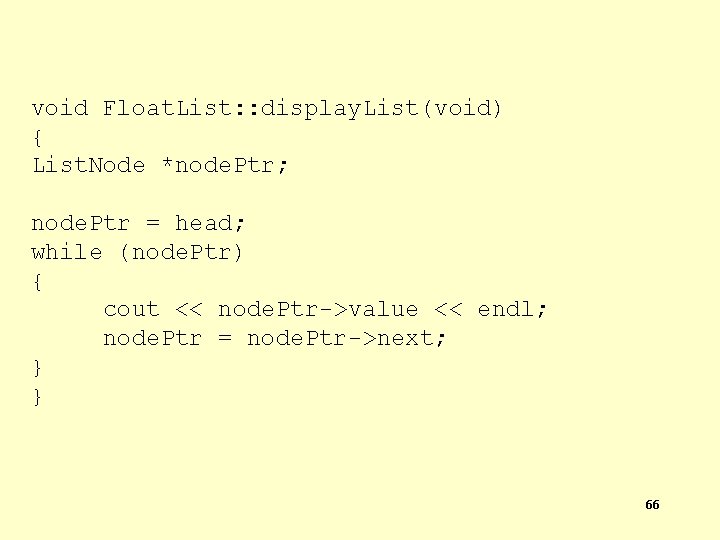
void Float. List: : display. List(void) { List. Node *node. Ptr; node. Ptr = head; while (node. Ptr) { cout << node. Ptr->value << endl; node. Ptr = node. Ptr->next; } } 66
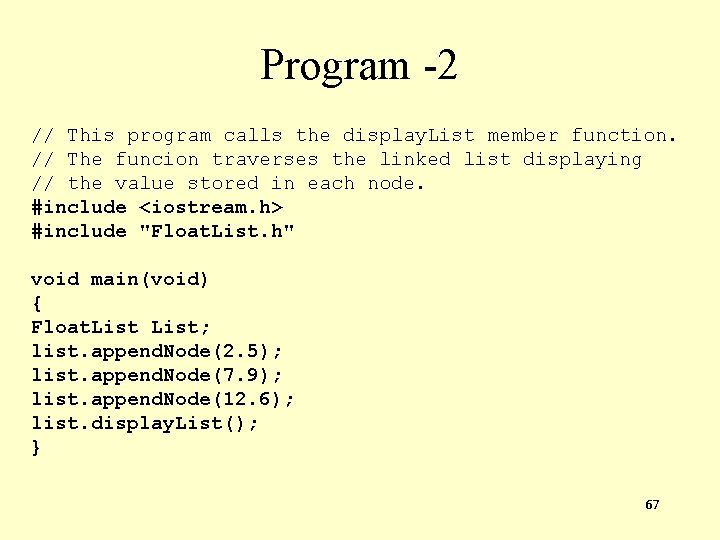
Program -2 // This program calls the display. List member function. // The funcion traverses the linked list displaying // the value stored in each node. #include <iostream. h> #include "Float. List. h" void main(void) { Float. List; list. append. Node(2. 5); list. append. Node(7. 9); list. append. Node(12. 6); list. display. List(); } 67
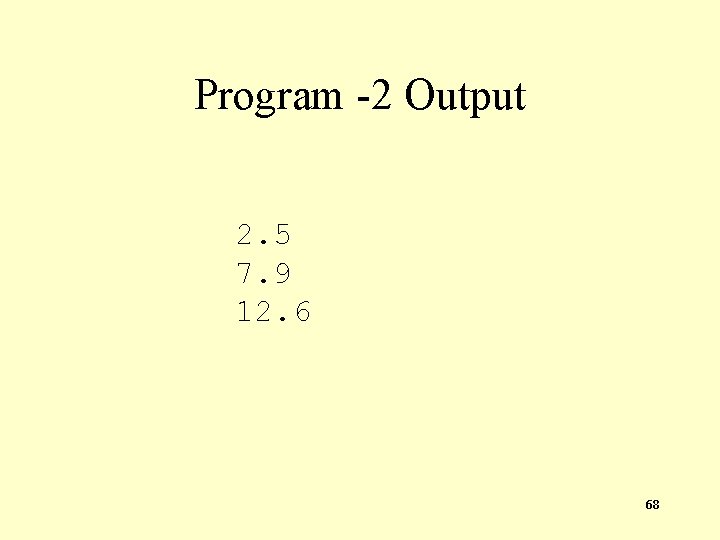
Program -2 Output 2. 5 7. 9 12. 6 68
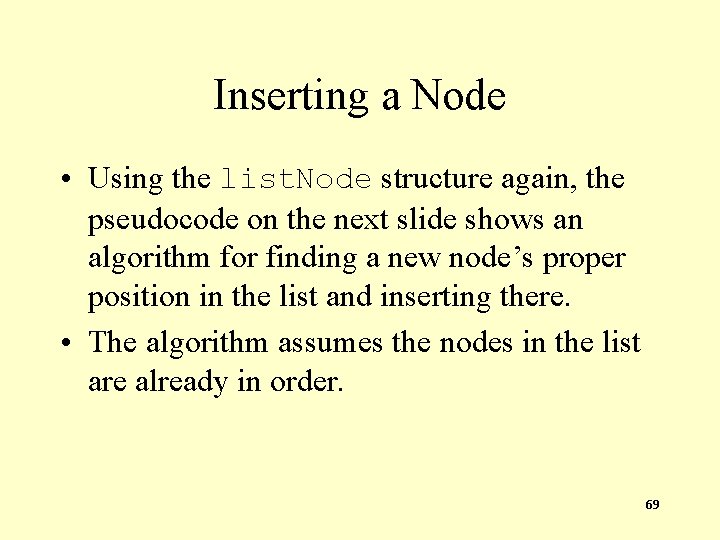
Inserting a Node • Using the list. Node structure again, the pseudocode on the next slide shows an algorithm for finding a new node’s proper position in the list and inserting there. • The algorithm assumes the nodes in the list are already in order. 69
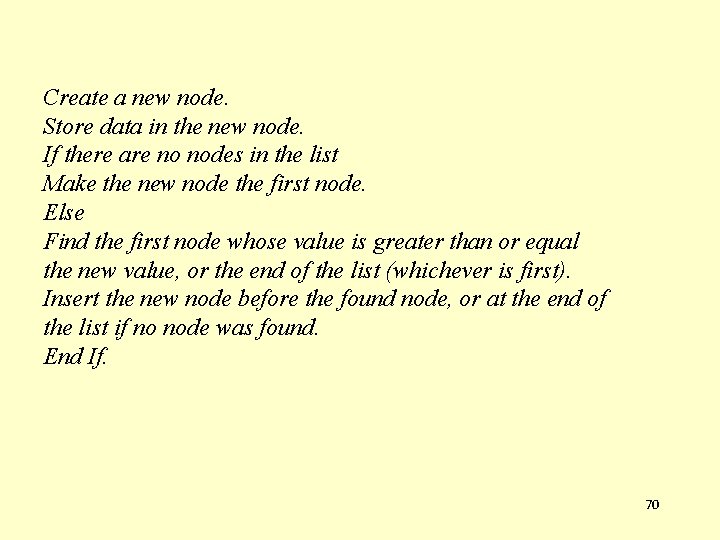
Create a new node. Store data in the new node. If there are no nodes in the list Make the new node the first node. Else Find the first node whose value is greater than or equal the new value, or the end of the list (whichever is first). Insert the new node before the found node, or at the end of the list if no node was found. End If. 70
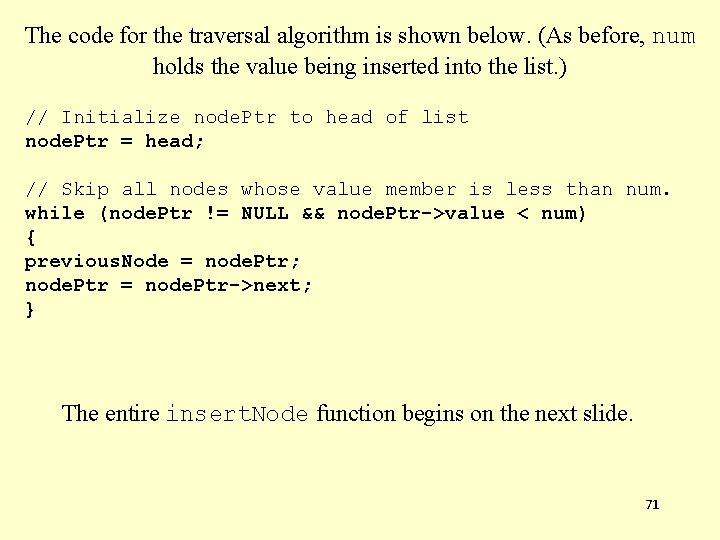
The code for the traversal algorithm is shown below. (As before, num holds the value being inserted into the list. ) // Initialize node. Ptr to head of list node. Ptr = head; // Skip all nodes whose value member is less than num. while (node. Ptr != NULL && node. Ptr->value < num) { previous. Node = node. Ptr; node. Ptr = node. Ptr->next; } The entire insert. Node function begins on the next slide. 71
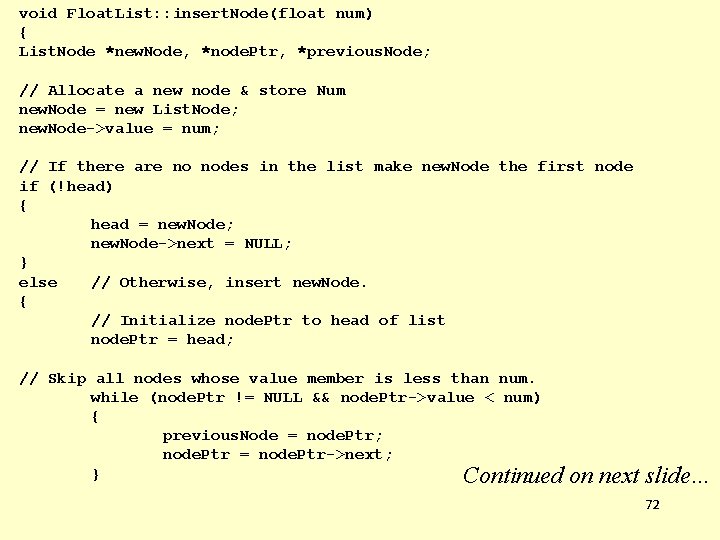
void Float. List: : insert. Node(float num) { List. Node *new. Node, *node. Ptr, *previous. Node; // Allocate a new node & store Num new. Node = new List. Node; new. Node->value = num; // If there are no nodes in the list make new. Node the first node if (!head) { head = new. Node; new. Node->next = NULL; } else // Otherwise, insert new. Node. { // Initialize node. Ptr to head of list node. Ptr = head; // Skip all nodes whose value member is less than num. while (node. Ptr != NULL && node. Ptr->value < num) { previous. Node = node. Ptr; node. Ptr = node. Ptr->next; } Continued on next slide… 72
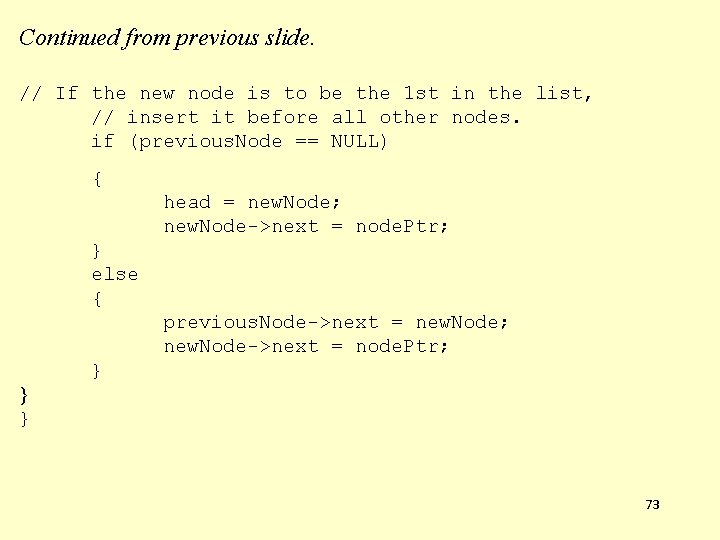
Continued from previous slide. // If the new node is to be the 1 st in the list, // insert it before all other nodes. if (previous. Node == NULL) { head = new. Node; new. Node->next = node. Ptr; } else { previous. Node->next = new. Node; new. Node->next = node. Ptr; } } } 73
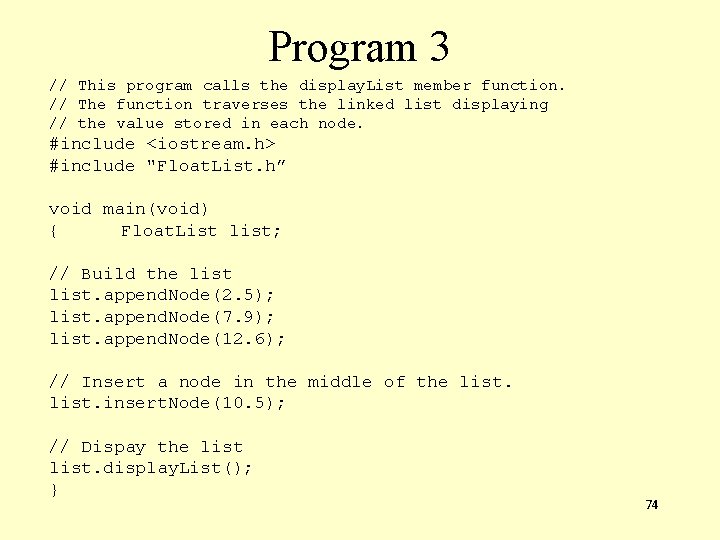
Program 3 // This program calls the display. List member function. // The function traverses the linked list displaying // the value stored in each node. #include <iostream. h> #include "Float. List. h” void main(void) { Float. List list; // Build the list. append. Node(2. 5); list. append. Node(7. 9); list. append. Node(12. 6); // Insert a node in the middle of the list. insert. Node(10. 5); // Dispay the list. display. List(); } 74
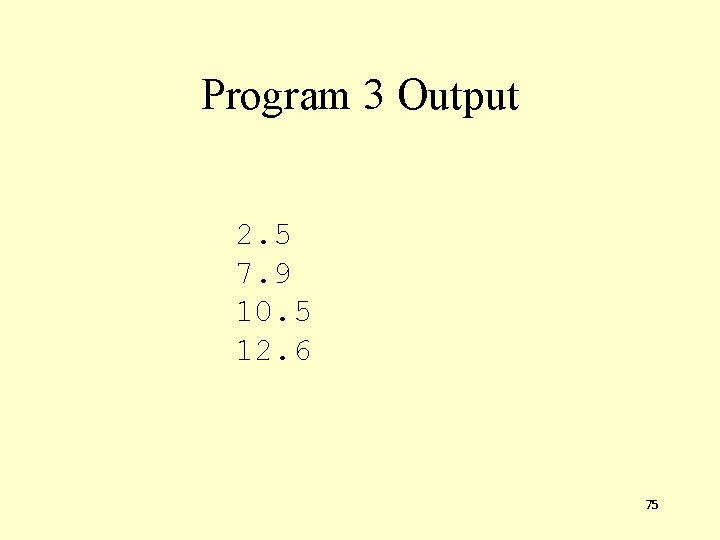
Program 3 Output 2. 5 7. 9 10. 5 12. 6 75
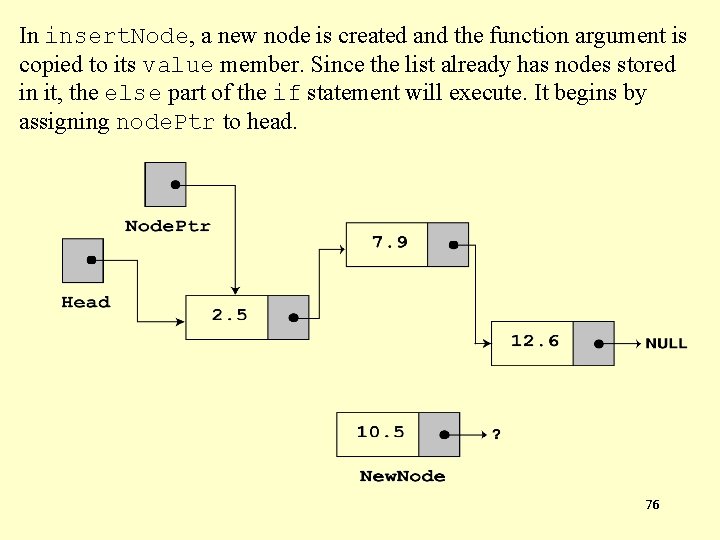
In insert. Node, a new node is created and the function argument is copied to its value member. Since the list already has nodes stored in it, the else part of the if statement will execute. It begins by assigning node. Ptr to head. 76
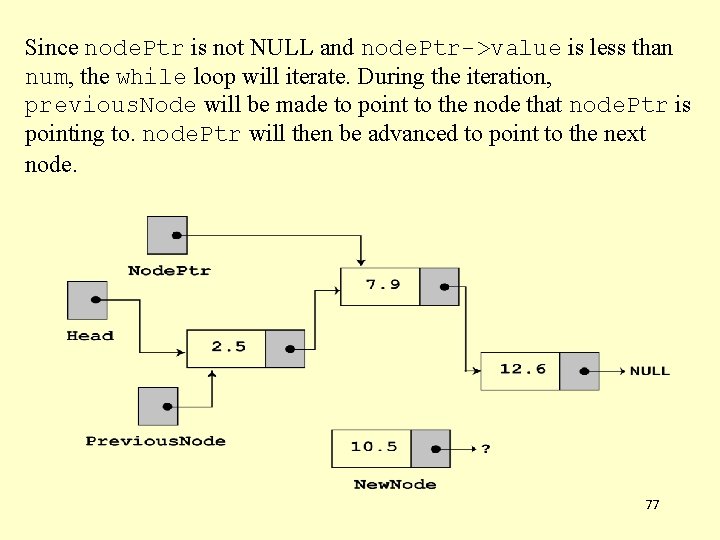
Since node. Ptr is not NULL and node. Ptr->value is less than num, the while loop will iterate. During the iteration, previous. Node will be made to point to the node that node. Ptr is pointing to. node. Ptr will then be advanced to point to the next node. 77
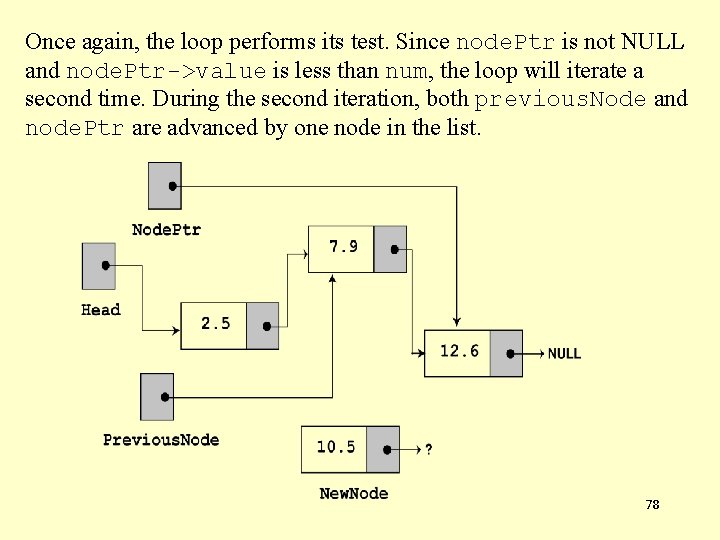
Once again, the loop performs its test. Since node. Ptr is not NULL and node. Ptr->value is less than num, the loop will iterate a second time. During the second iteration, both previous. Node and node. Ptr are advanced by one node in the list. 78
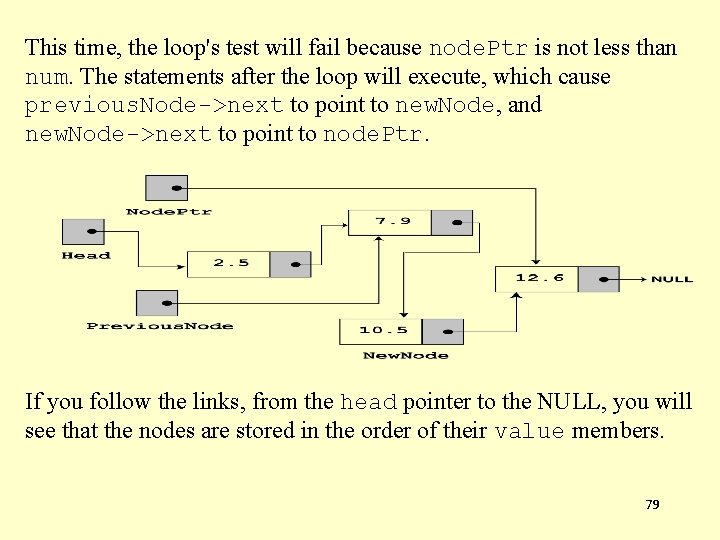
This time, the loop's test will fail because node. Ptr is not less than num. The statements after the loop will execute, which cause previous. Node->next to point to new. Node, and new. Node->next to point to node. Ptr. If you follow the links, from the head pointer to the NULL, you will see that the nodes are stored in the order of their value members. 79
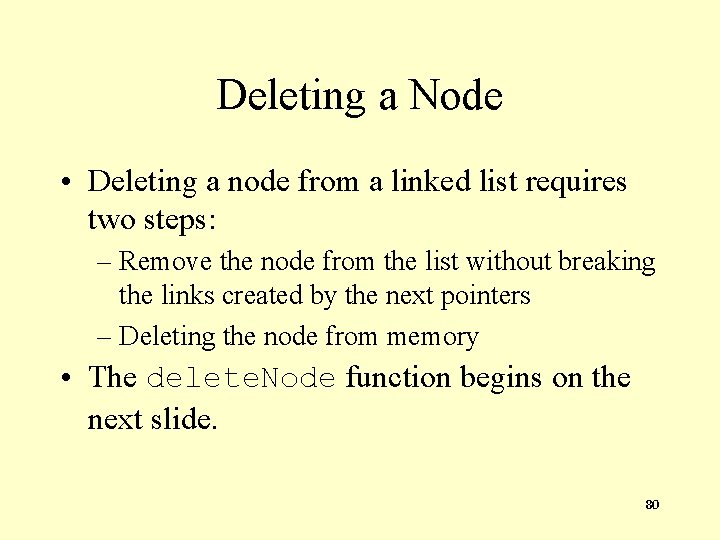
Deleting a Node • Deleting a node from a linked list requires two steps: – Remove the node from the list without breaking the links created by the next pointers – Deleting the node from memory • The delete. Node function begins on the next slide. 80
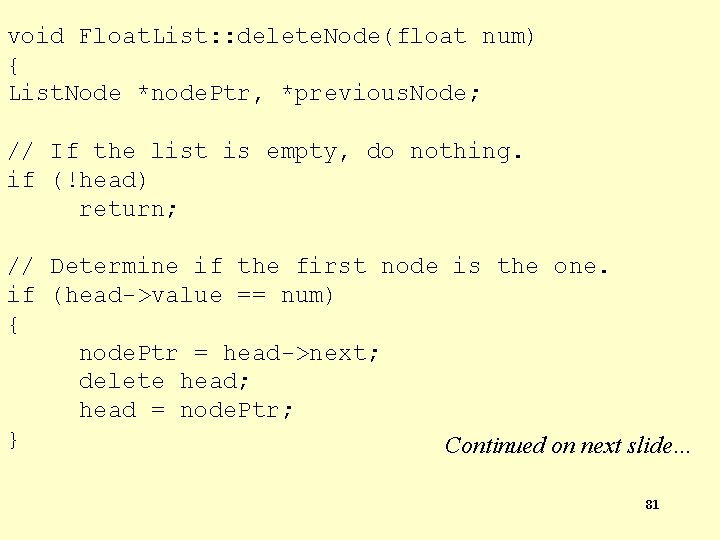
void Float. List: : delete. Node(float num) { List. Node *node. Ptr, *previous. Node; // If the list is empty, do nothing. if (!head) return; // Determine if the first node is the one. if (head->value == num) { node. Ptr = head->next; delete head; head = node. Ptr; } Continued on next slide… 81
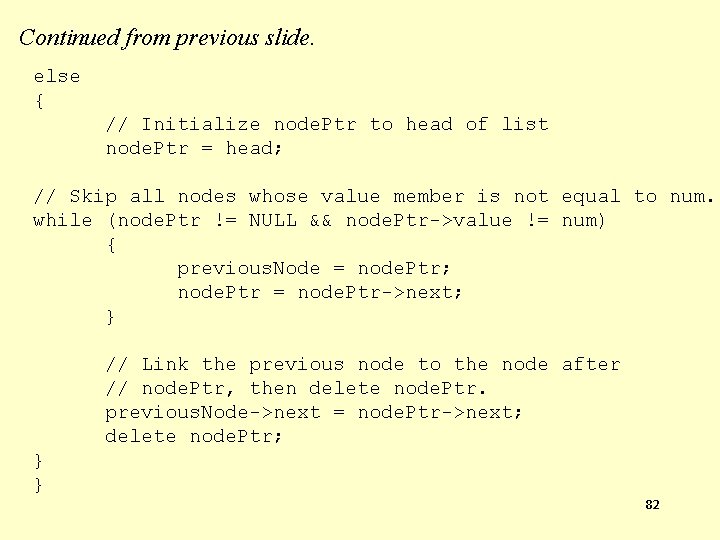
Continued from previous slide. else { // Initialize node. Ptr to head of list node. Ptr = head; // Skip all nodes whose value member is not equal to num. while (node. Ptr != NULL && node. Ptr->value != num) { previous. Node = node. Ptr; node. Ptr = node. Ptr->next; } // Link the previous node to the node after // node. Ptr, then delete node. Ptr. previous. Node->next = node. Ptr->next; delete node. Ptr; } } 82
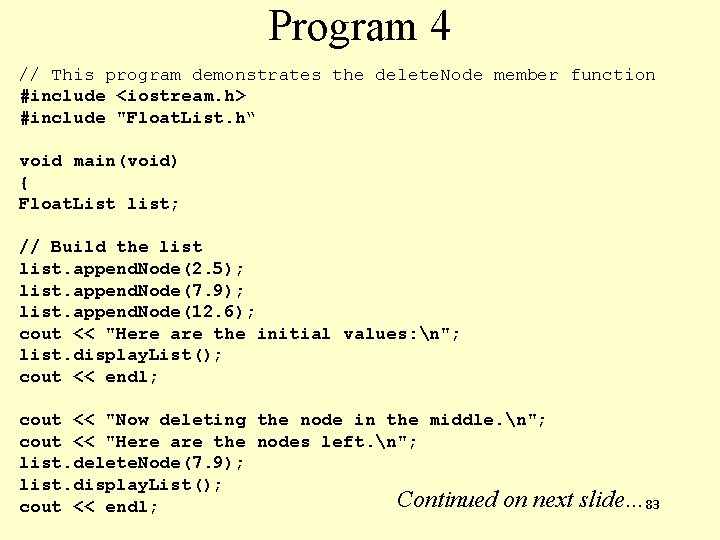
Program 4 // This program demonstrates the delete. Node member function #include <iostream. h> #include "Float. List. h“ void main(void) { Float. List list; // Build the list. append. Node(2. 5); list. append. Node(7. 9); list. append. Node(12. 6); cout << "Here are the initial values: n"; list. display. List(); cout << endl; cout << "Now deleting the node in the middle. n"; cout << "Here are the nodes left. n"; list. delete. Node(7. 9); list. display. List(); Continued on next cout << endl; slide… 83
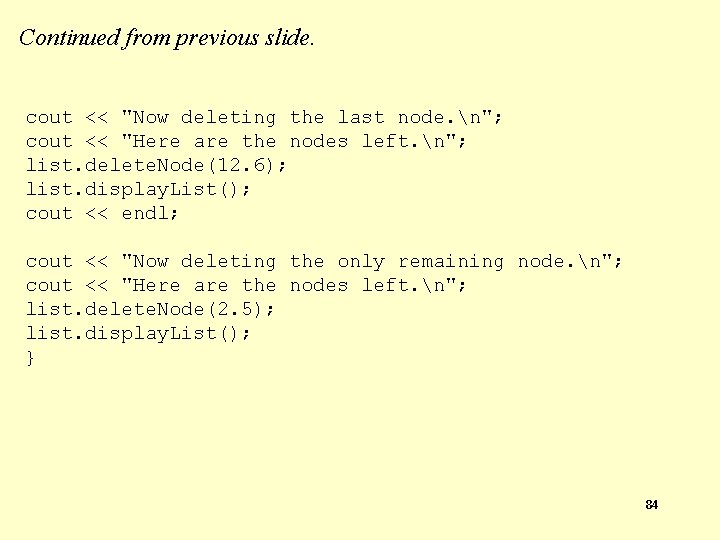
Continued from previous slide. cout << "Now deleting the last node. n"; cout << "Here are the nodes left. n"; list. delete. Node(12. 6); list. display. List(); cout << endl; cout << "Now deleting the only remaining node. n"; cout << "Here are the nodes left. n"; list. delete. Node(2. 5); list. display. List(); } 84
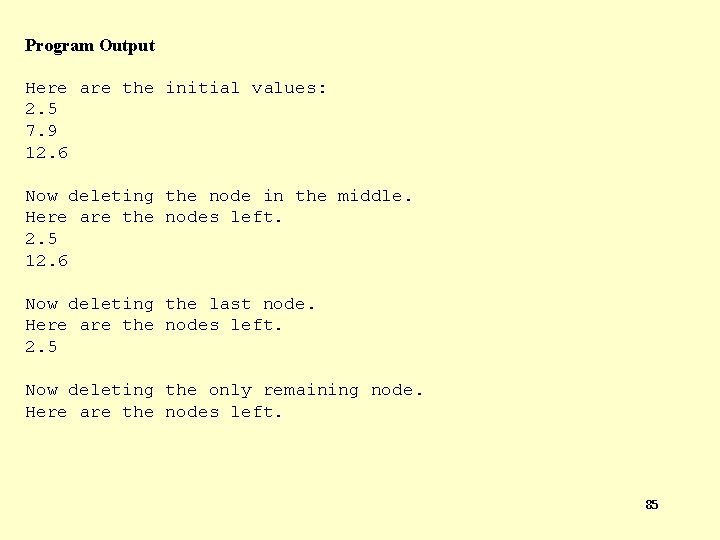
Program Output Here are the initial values: 2. 5 7. 9 12. 6 Now deleting the node in the middle. Here are the nodes left. 2. 5 12. 6 Now deleting the last node. Here are the nodes left. 2. 5 Now deleting the only remaining node. Here are the nodes left. 85
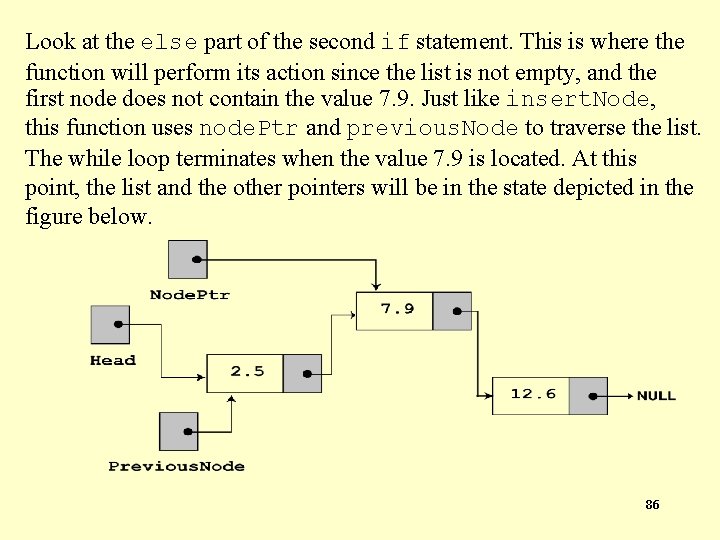
Look at the else part of the second if statement. This is where the function will perform its action since the list is not empty, and the first node does not contain the value 7. 9. Just like insert. Node, this function uses node. Ptr and previous. Node to traverse the list. The while loop terminates when the value 7. 9 is located. At this point, the list and the other pointers will be in the state depicted in the figure below. 86
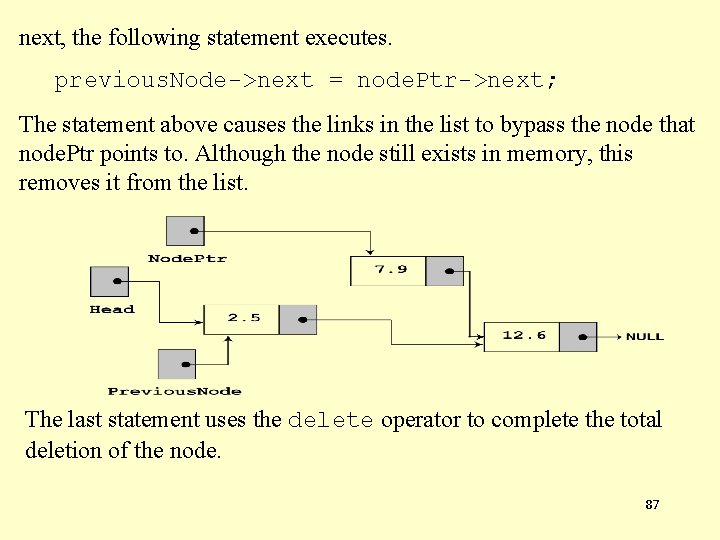
next, the following statement executes. previous. Node->next = node. Ptr->next; The statement above causes the links in the list to bypass the node that node. Ptr points to. Although the node still exists in memory, this removes it from the list. The last statement uses the delete operator to complete the total deletion of the node. 87
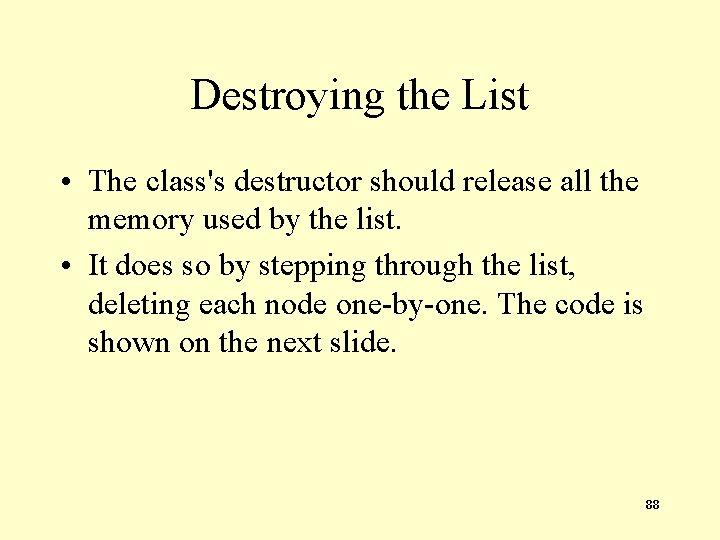
Destroying the List • The class's destructor should release all the memory used by the list. • It does so by stepping through the list, deleting each node one-by-one. The code is shown on the next slide. 88
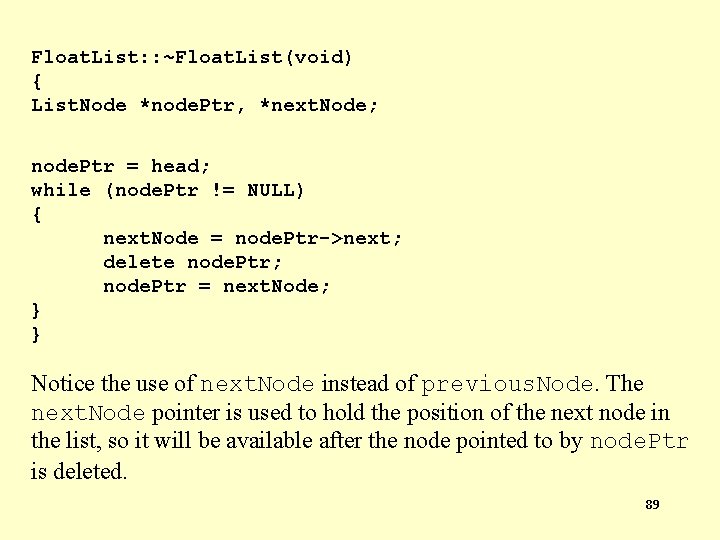
Float. List: : ~Float. List(void) { List. Node *node. Ptr, *next. Node; node. Ptr = head; while (node. Ptr != NULL) { next. Node = node. Ptr->next; delete node. Ptr; node. Ptr = next. Node; } } Notice the use of next. Node instead of previous. Node. The next. Node pointer is used to hold the position of the next node in the list, so it will be available after the node pointed to by node. Ptr is deleted. 89
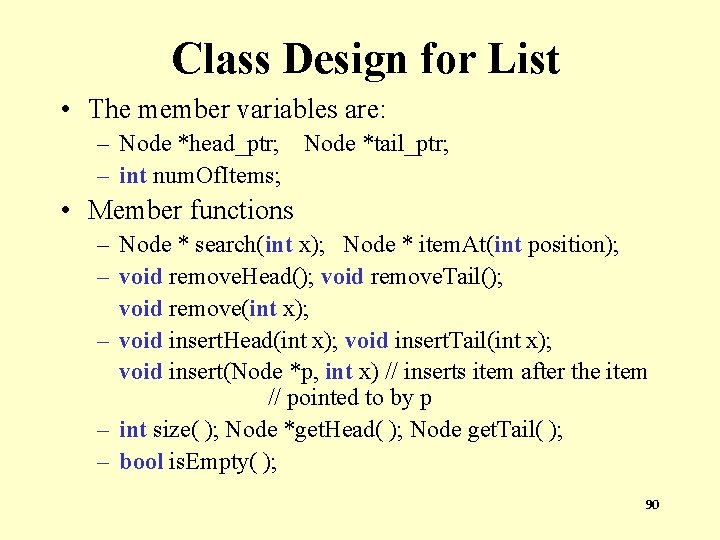
Class Design for List • The member variables are: – Node *head_ptr; Node *tail_ptr; – int num. Of. Items; • Member functions – Node * search(int x); Node * item. At(int position); – void remove. Head(); void remove. Tail(); void remove(int x); – void insert. Head(int x); void insert. Tail(int x); void insert(Node *p, int x) // inserts item after the item // pointed to by p – int size( ); Node *get. Head( ); Node get. Tail( ); – bool is. Empty( ); 90
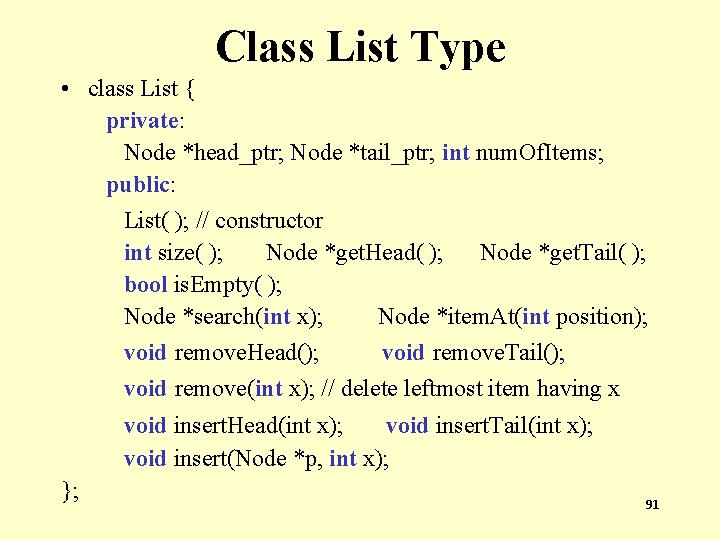
Class List Type • class List { private: Node *head_ptr; Node *tail_ptr; int num. Of. Items; public: List( ); // constructor int size( ); Node *get. Head( ); Node *get. Tail( ); bool is. Empty( ); Node *search(int x); Node *item. At(int position); void remove. Head(); void remove. Tail(); void remove(int x); // delete leftmost item having x void insert. Head(int x); void insert. Tail(int x); void insert(Node *p, int x); }; 91
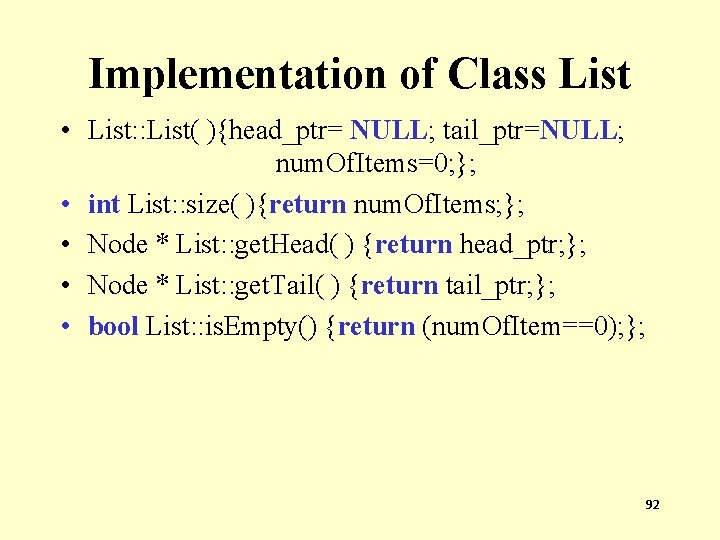
Implementation of Class List • List: : List( ){head_ptr= NULL; tail_ptr=NULL; num. Of. Items=0; }; • int List: : size( ){return num. Of. Items; }; • Node * List: : get. Head( ) {return head_ptr; }; • Node * List: : get. Tail( ) {return tail_ptr; }; • bool List: : is. Empty() {return (num. Of. Item==0); }; 92
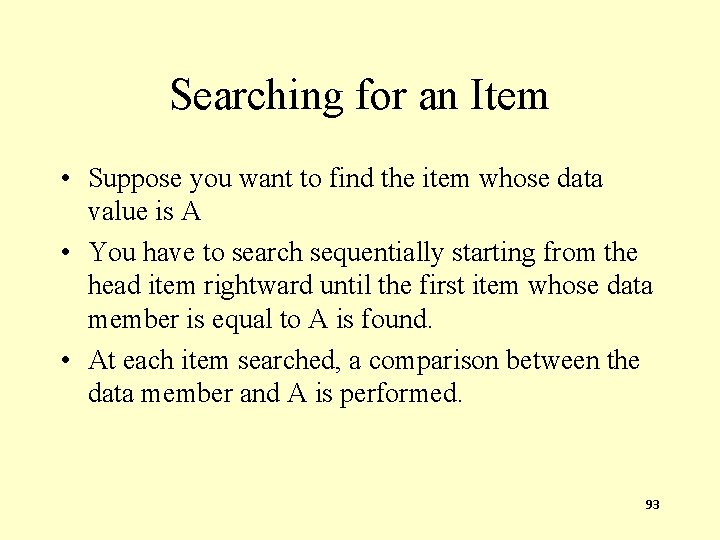
Searching for an Item • Suppose you want to find the item whose data value is A • You have to search sequentially starting from the head item rightward until the first item whose data member is equal to A is found. • At each item searched, a comparison between the data member and A is performed. 93
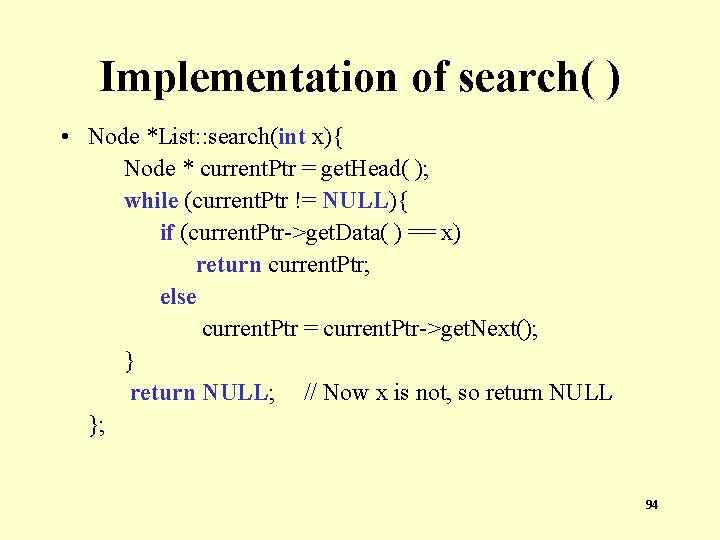
Implementation of search( ) • Node *List: : search(int x){ Node * current. Ptr = get. Head( ); while (current. Ptr != NULL){ if (current. Ptr->get. Data( ) == x) return current. Ptr; else current. Ptr = current. Ptr->get. Next(); } return NULL; // Now x is not, so return NULL }; 94
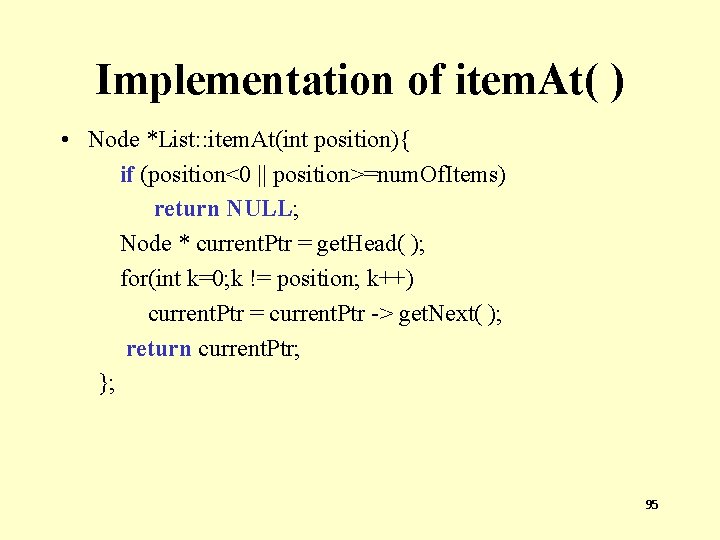
Implementation of item. At( ) • Node *List: : item. At(int position){ if (position<0 || position>=num. Of. Items) return NULL; Node * current. Ptr = get. Head( ); for(int k=0; k != position; k++) current. Ptr = current. Ptr -> get. Next( ); return current. Ptr; }; 95
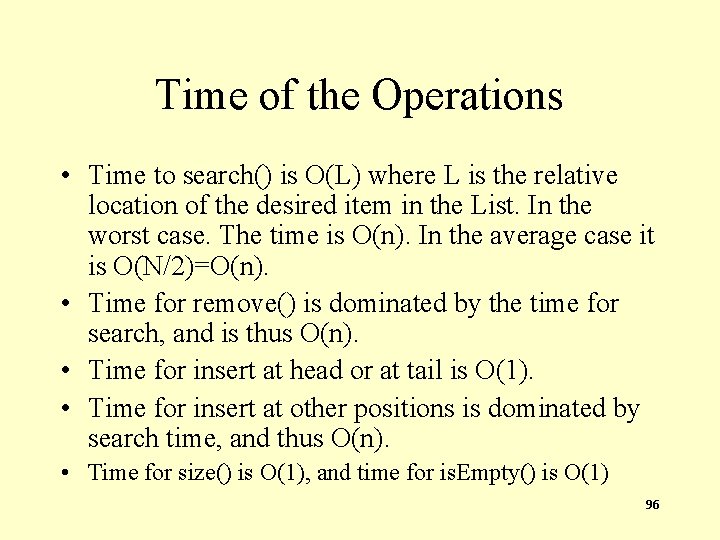
Time of the Operations • Time to search() is O(L) where L is the relative location of the desired item in the List. In the worst case. The time is O(n). In the average case it is O(N/2)=O(n). • Time for remove() is dominated by the time for search, and is thus O(n). • Time for insert at head or at tail is O(1). • Time for insert at other positions is dominated by search time, and thus O(n). • Time for size() is O(1), and time for is. Empty() is O(1) 96
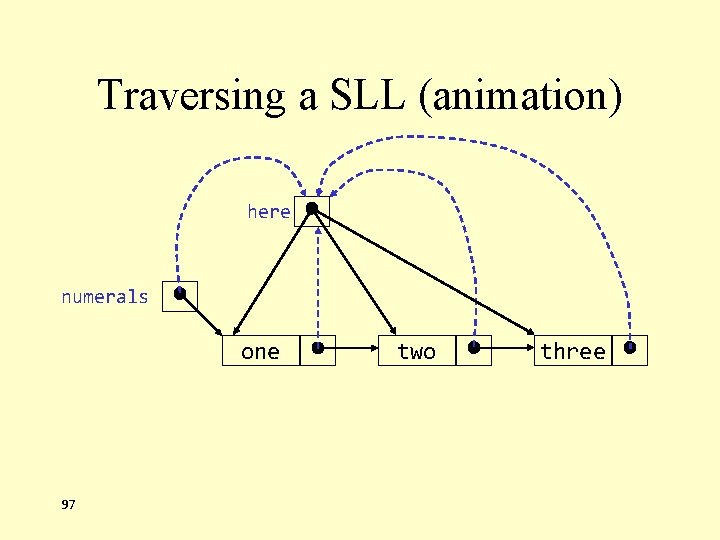
Traversing a SLL (animation) here numerals one 97 two three
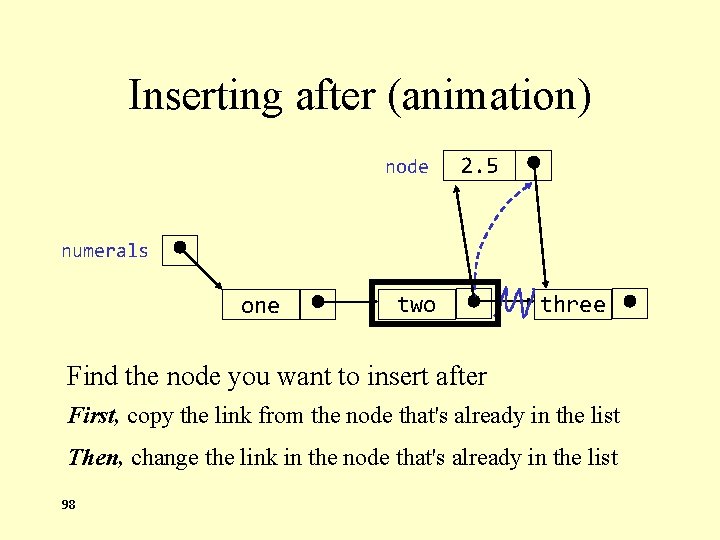
Inserting after (animation) node 2. 5 numerals one two three Find the node you want to insert after First, copy the link from the node that's already in the list Then, change the link in the node that's already in the list 98
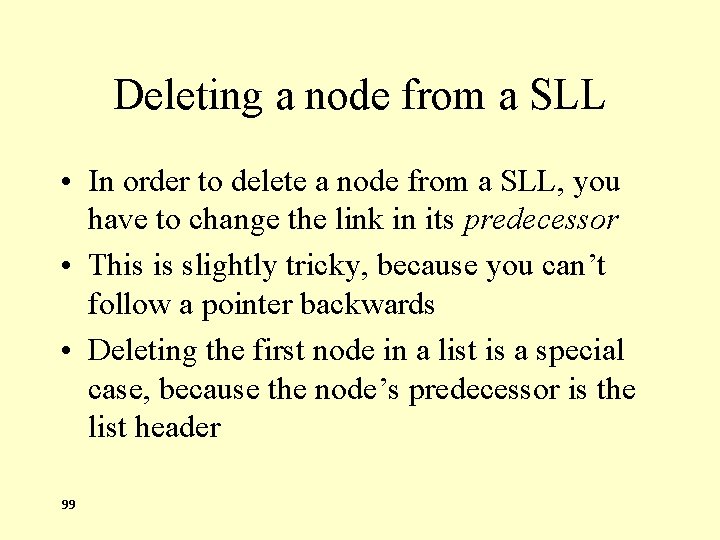
Deleting a node from a SLL • In order to delete a node from a SLL, you have to change the link in its predecessor • This is slightly tricky, because you can’t follow a pointer backwards • Deleting the first node in a list is a special case, because the node’s predecessor is the list header 99
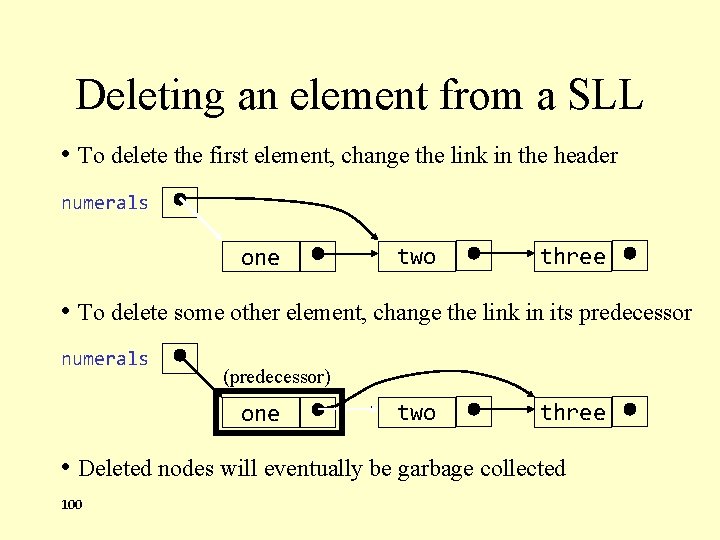
Deleting an element from a SLL • To delete the first element, change the link in the header numerals one two three • To delete some other element, change the link in its predecessor numerals (predecessor) one two three • Deleted nodes will eventually be garbage collected 100
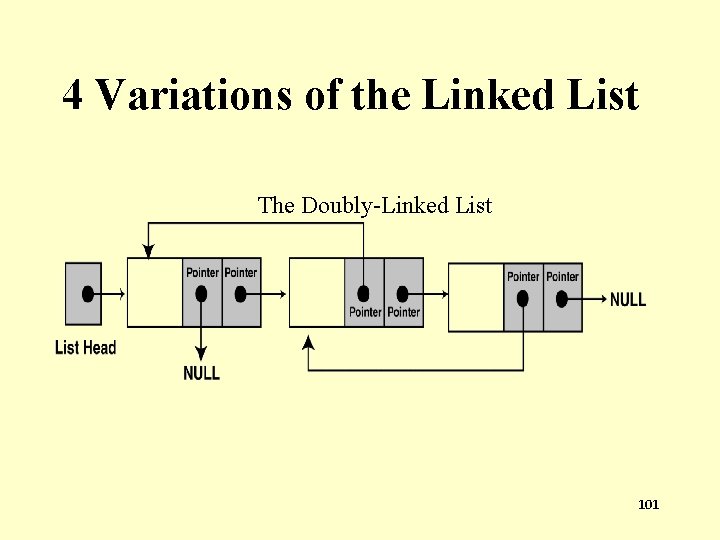
4 Variations of the Linked List The Doubly-Linked List 101
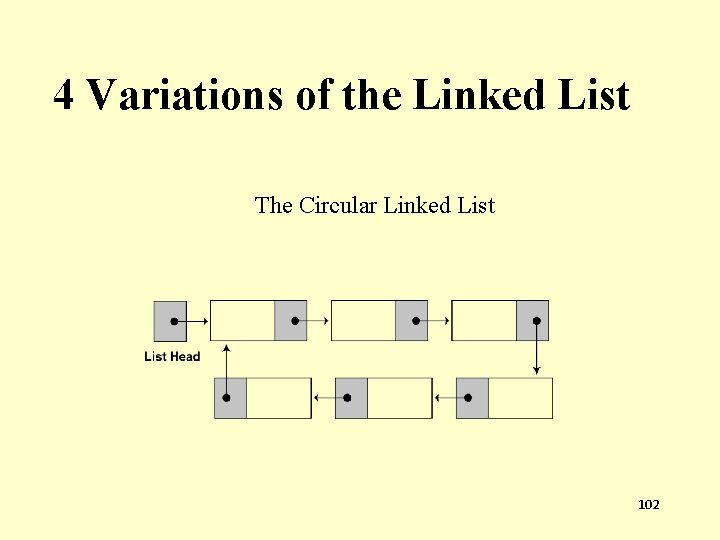
4 Variations of the Linked List The Circular Linked List 102