Unit II Unit II Total Marks 13 Total
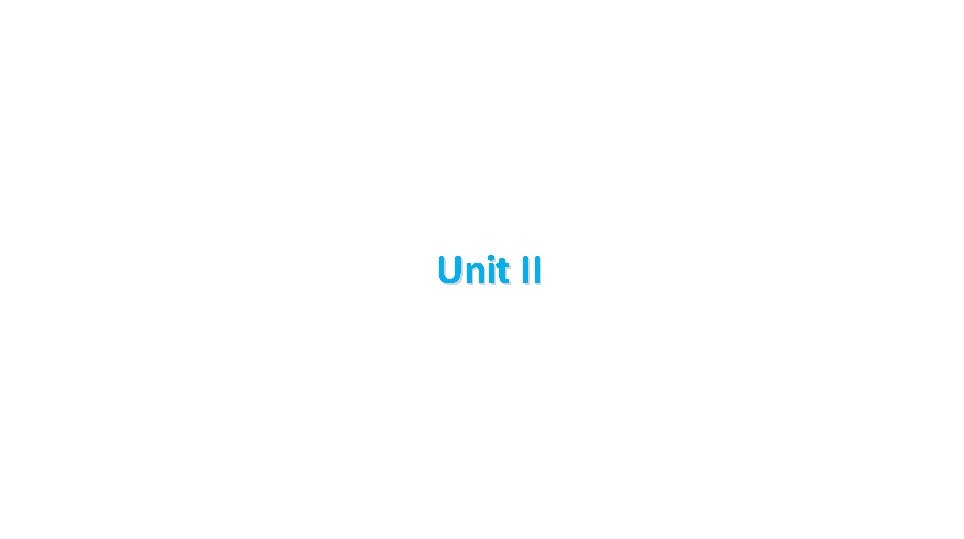
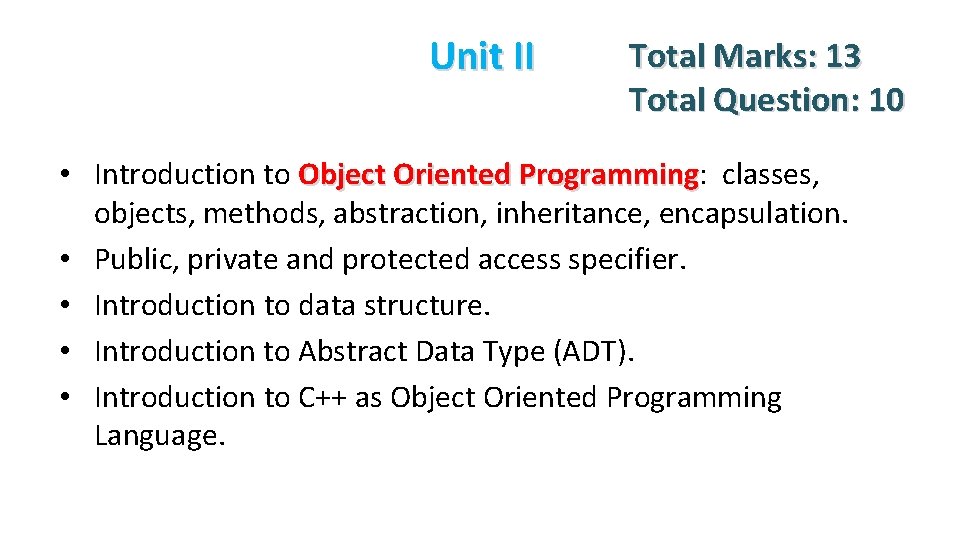
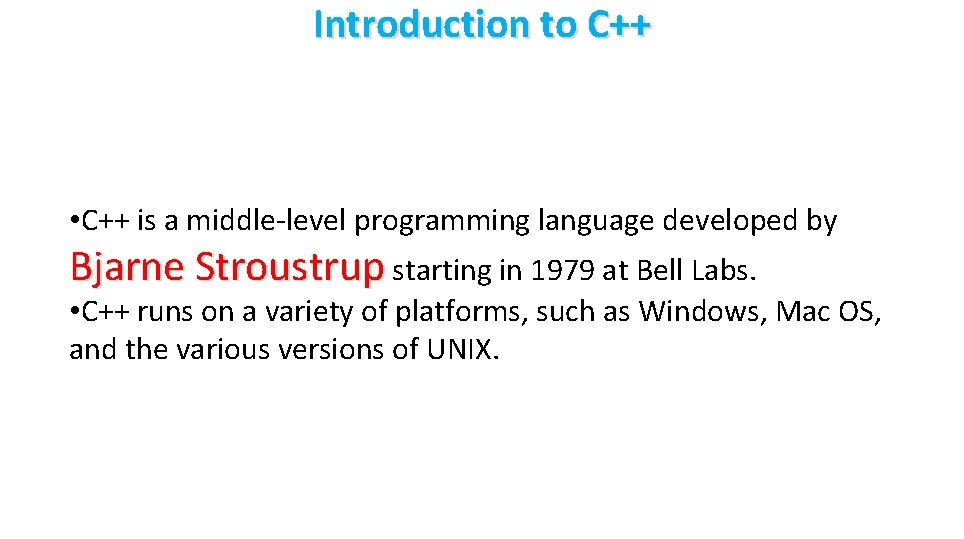
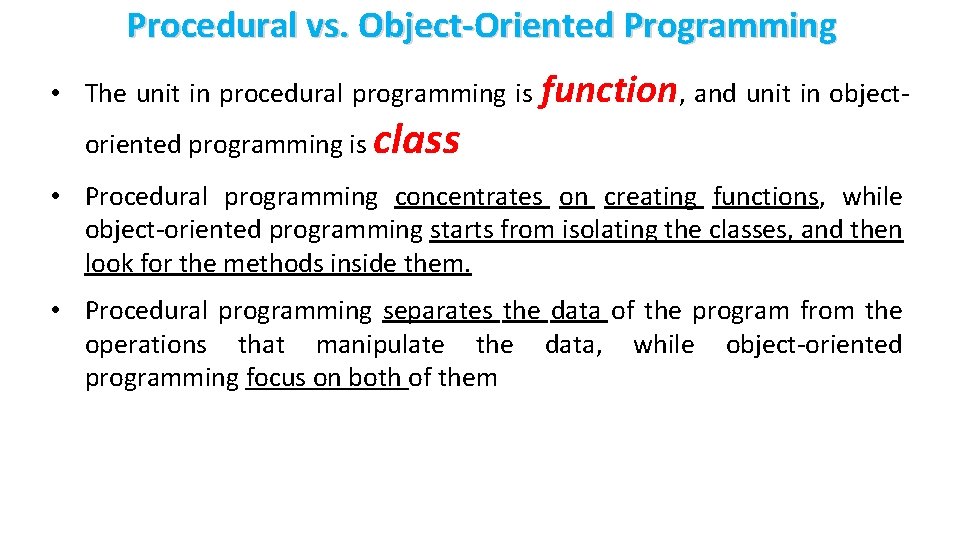
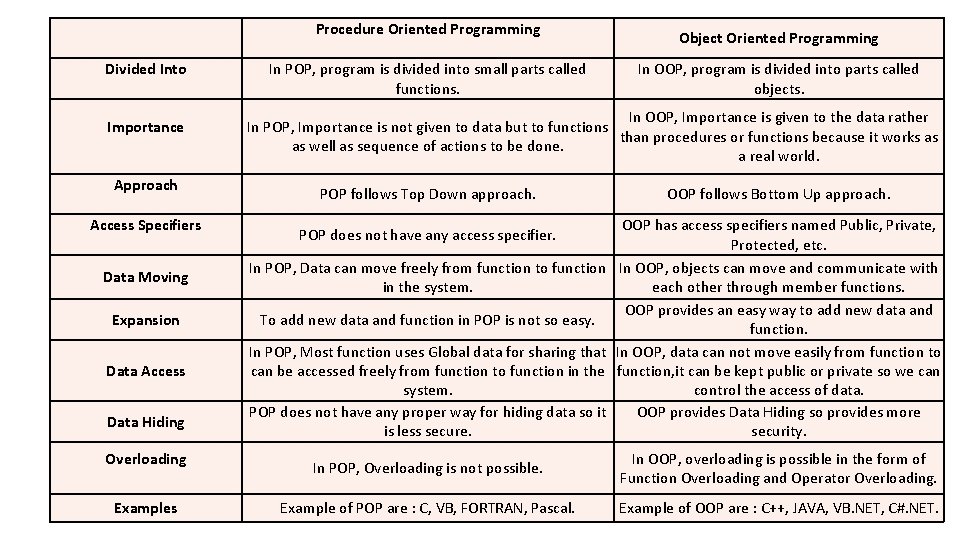
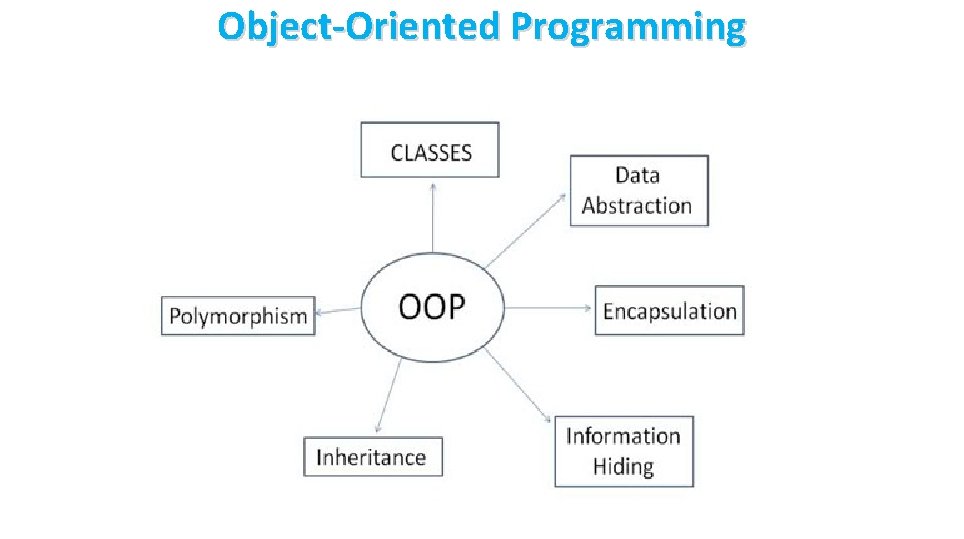
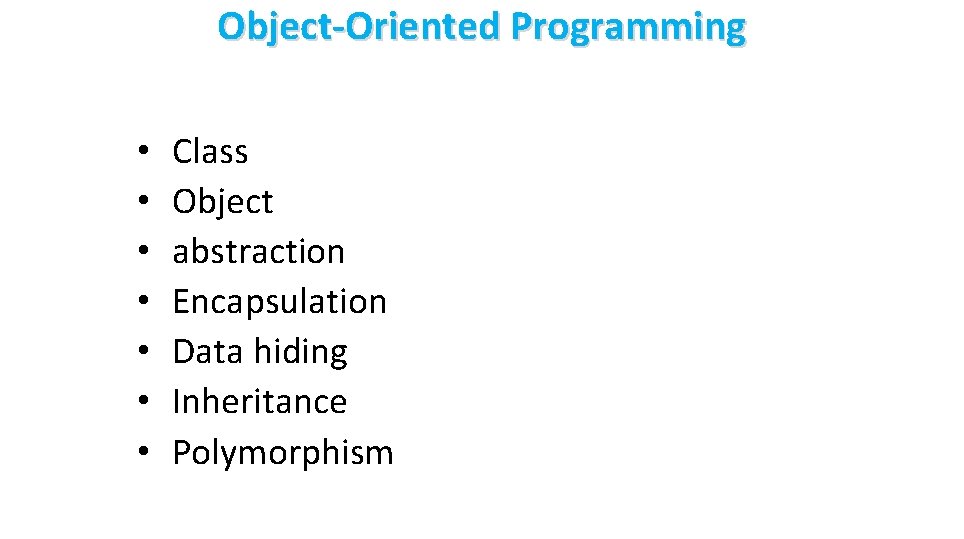
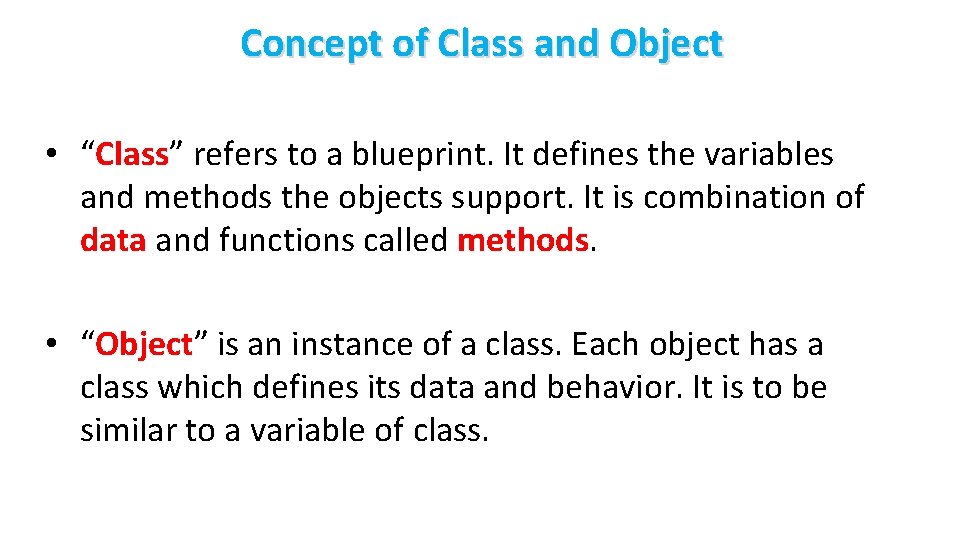
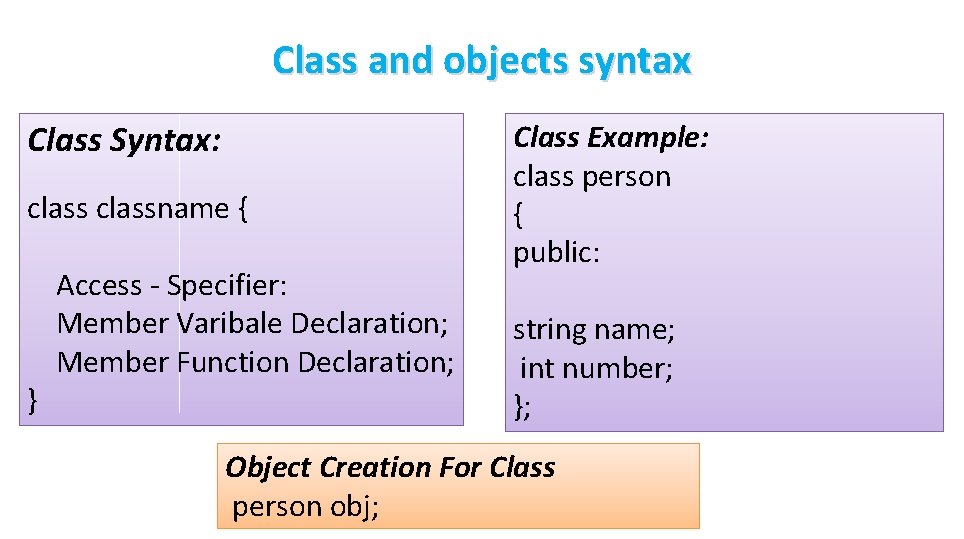
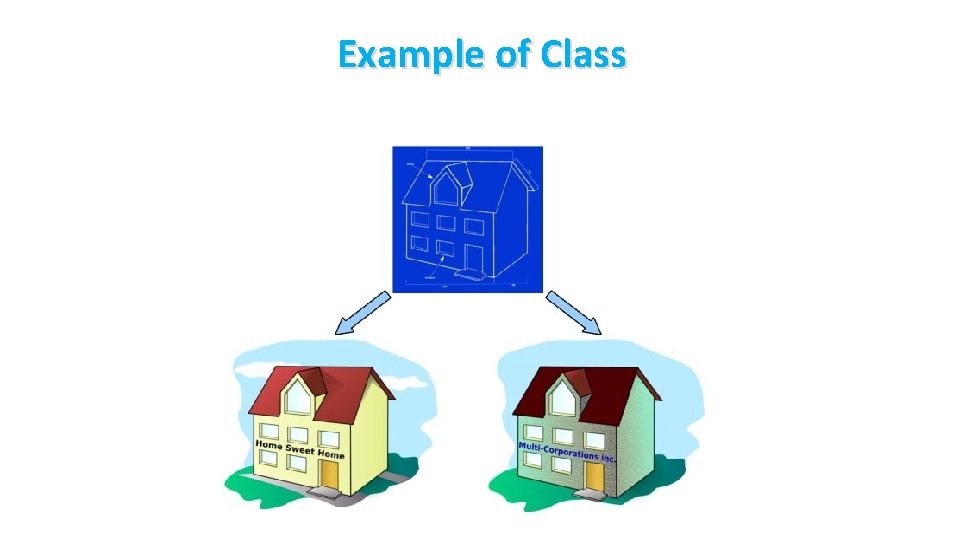
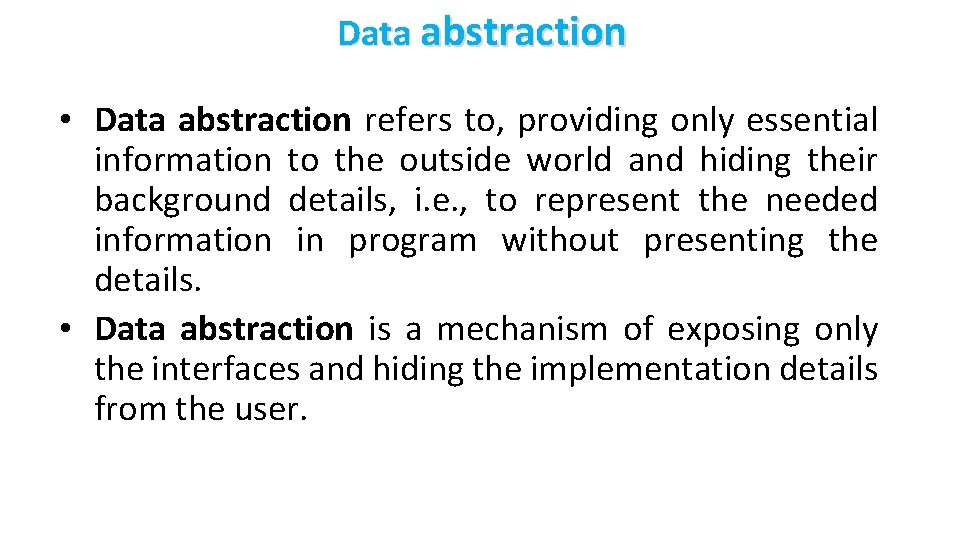
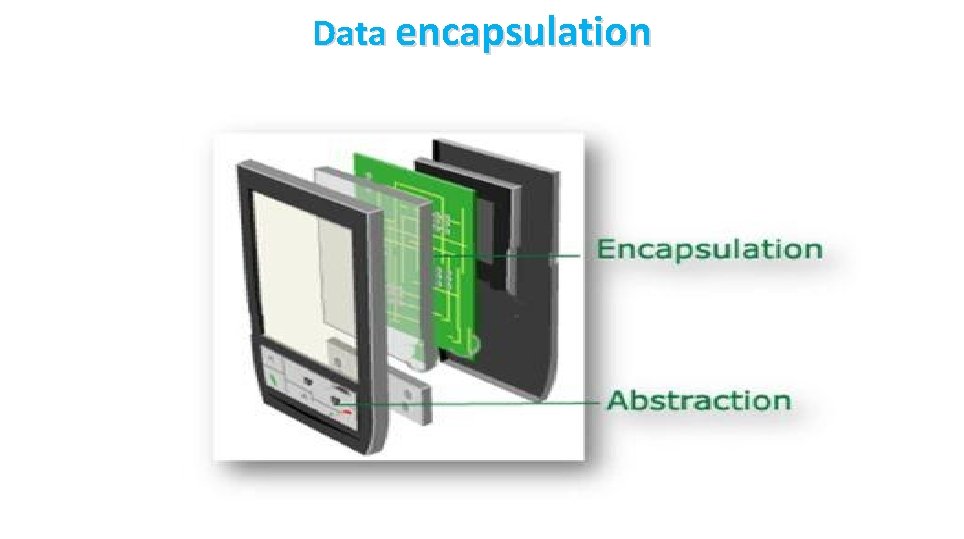
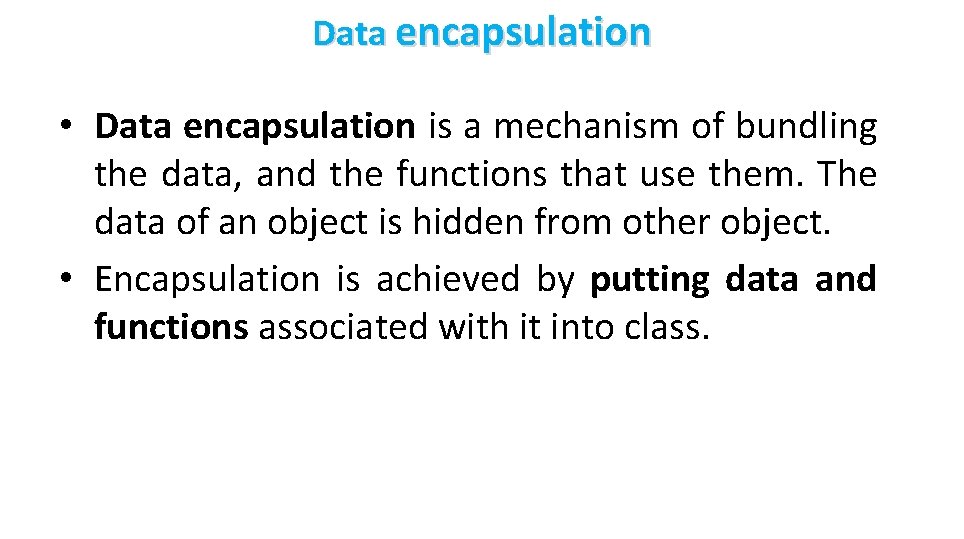
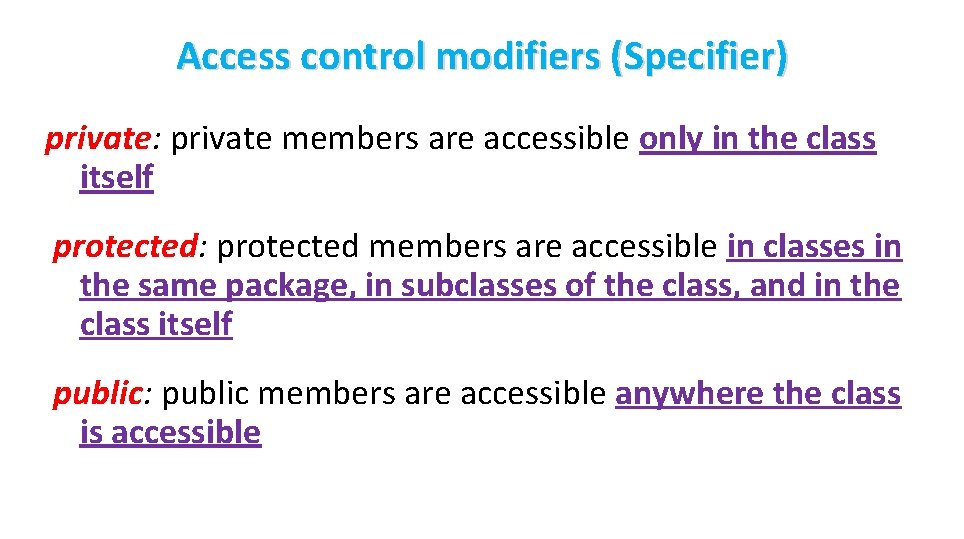
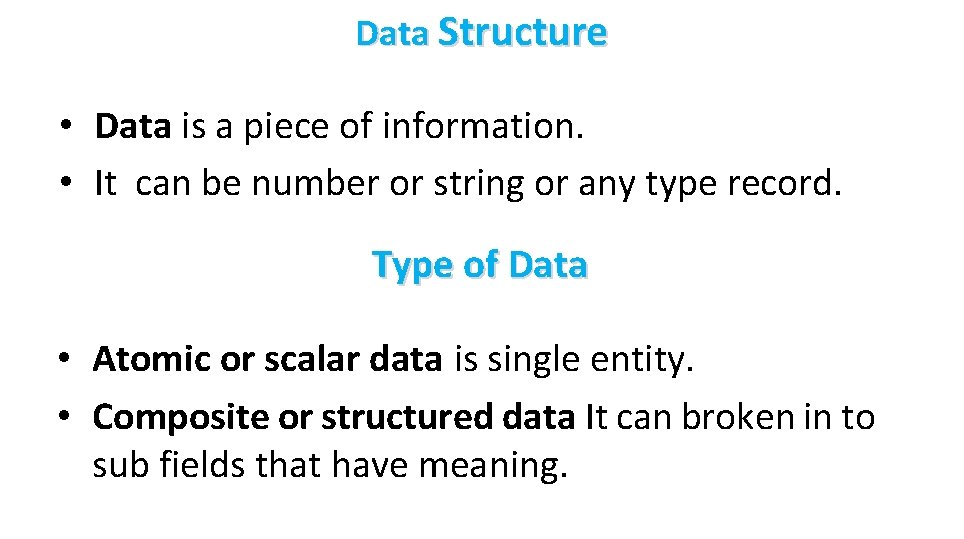
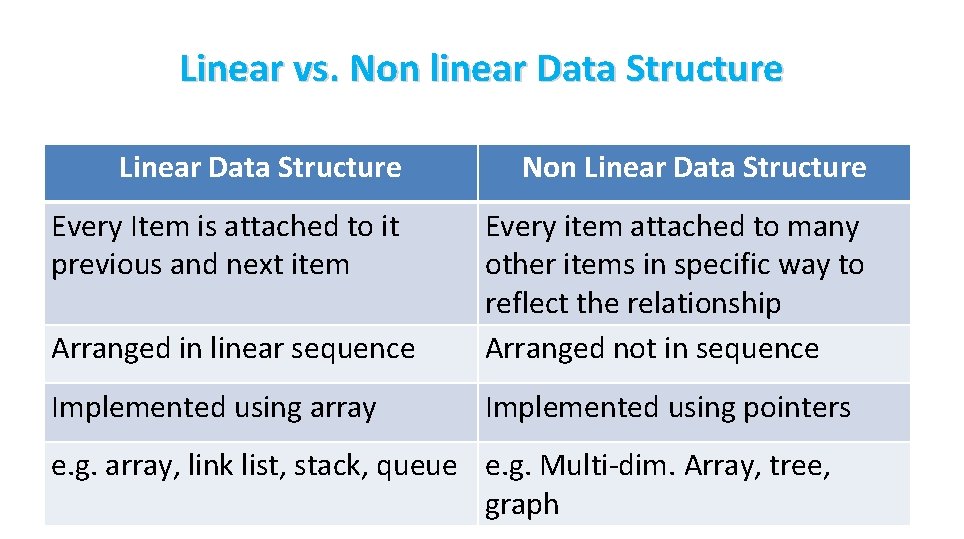
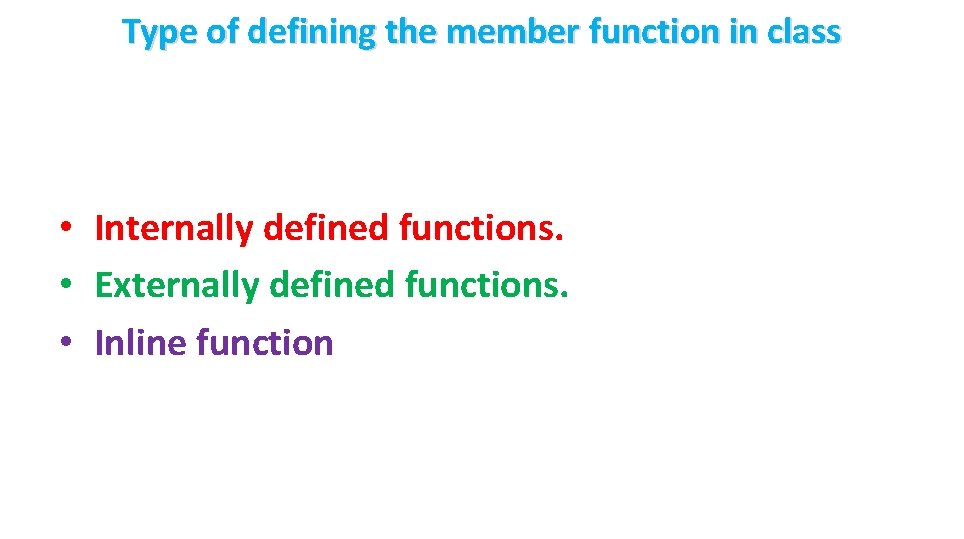
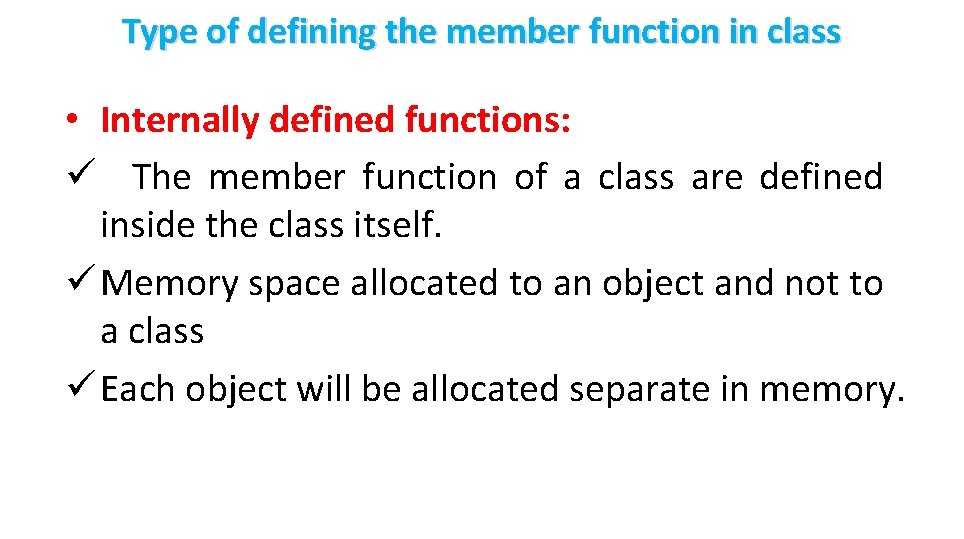
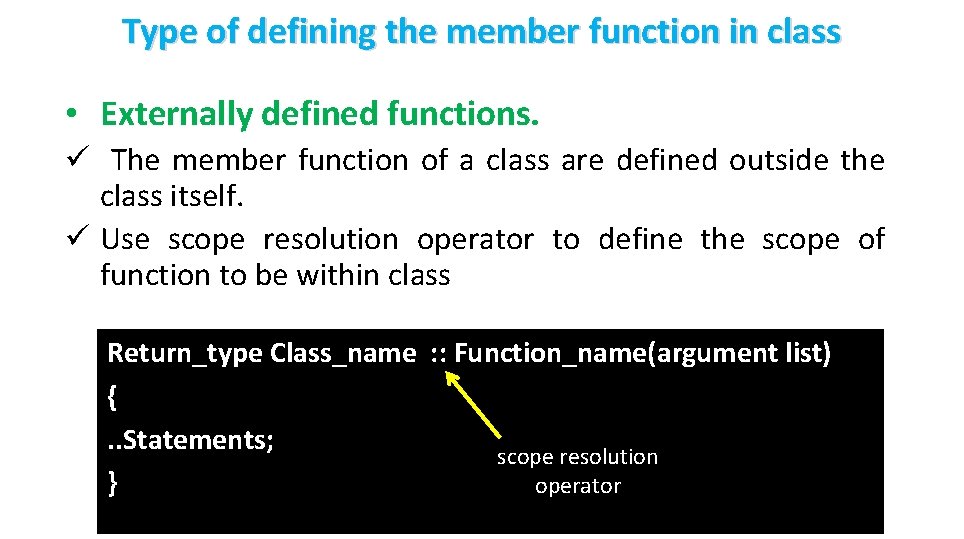
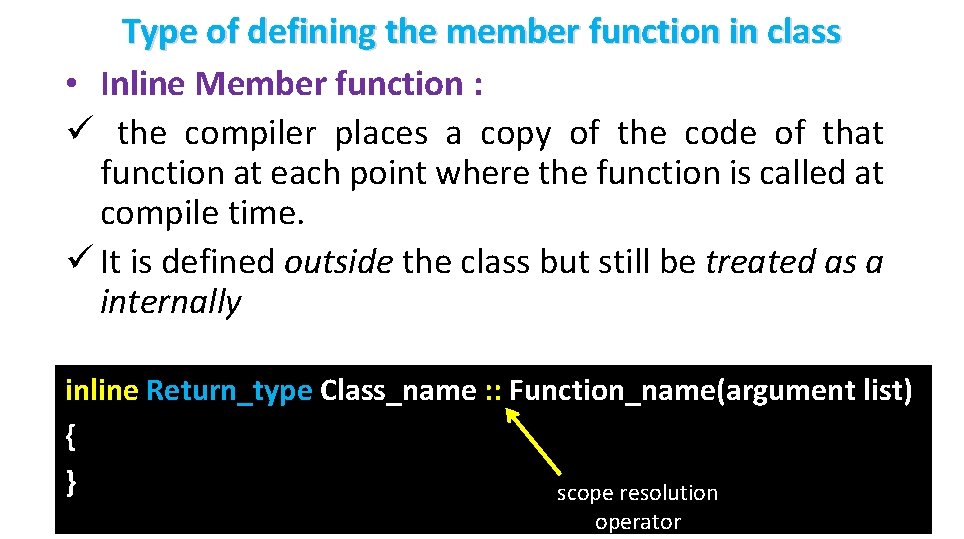
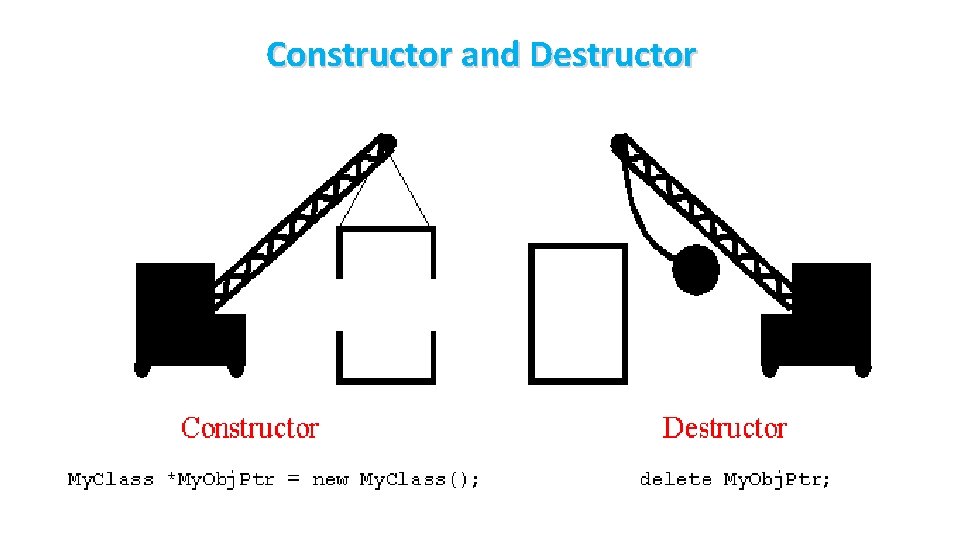
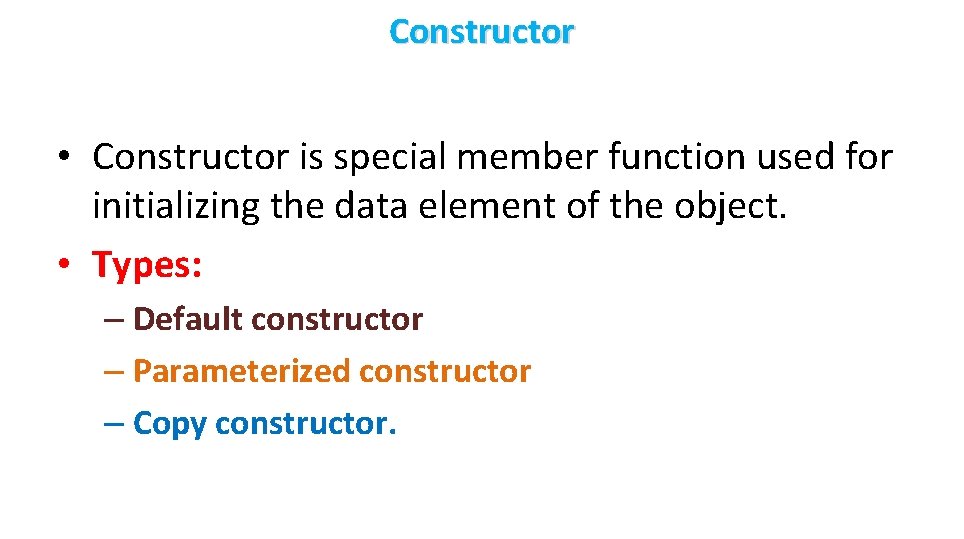
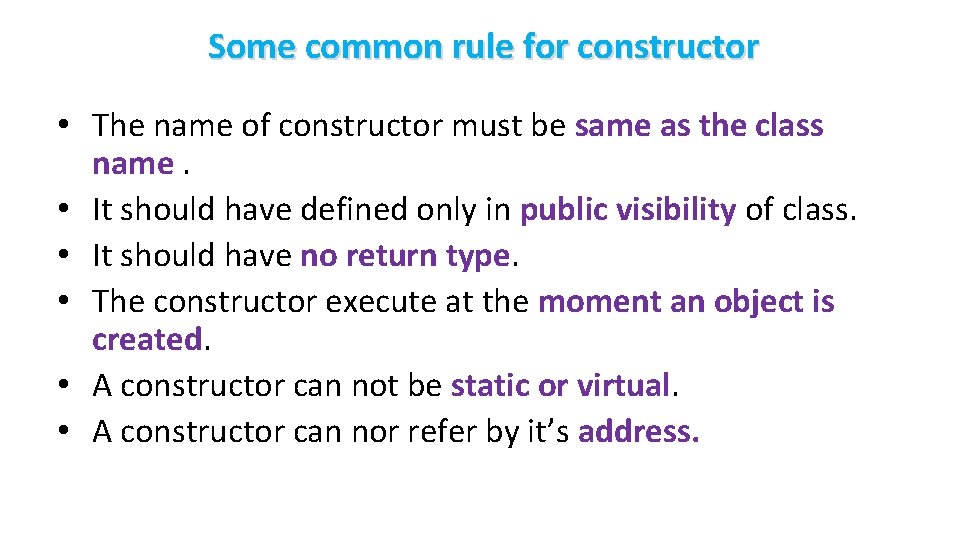
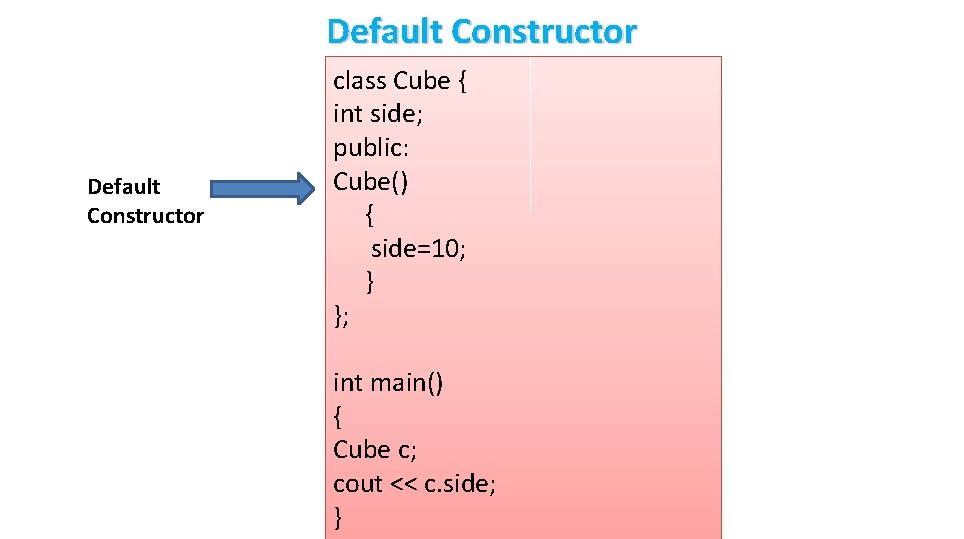
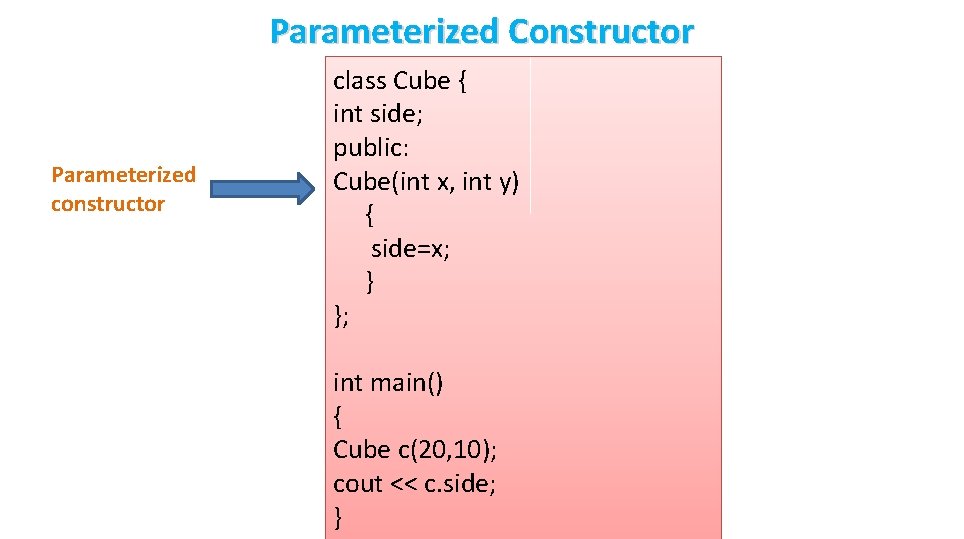
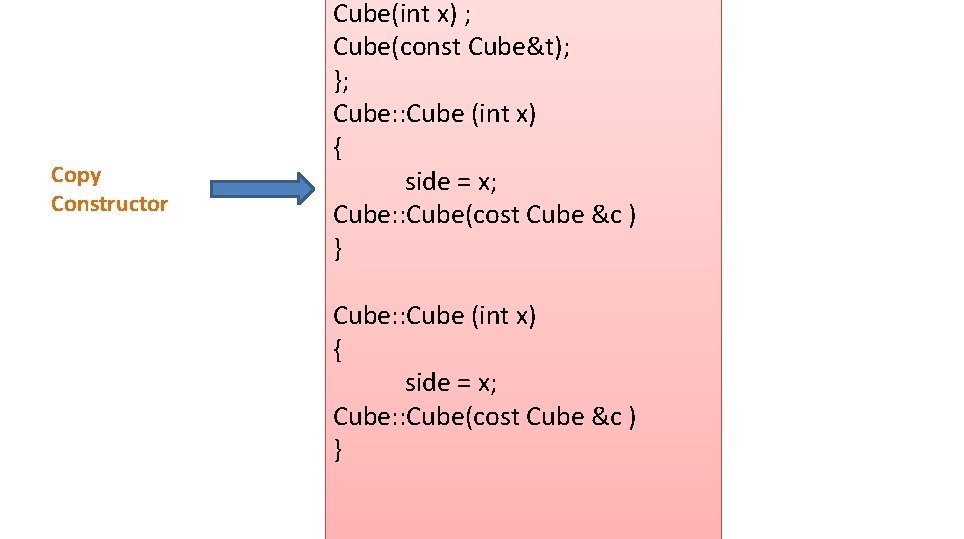
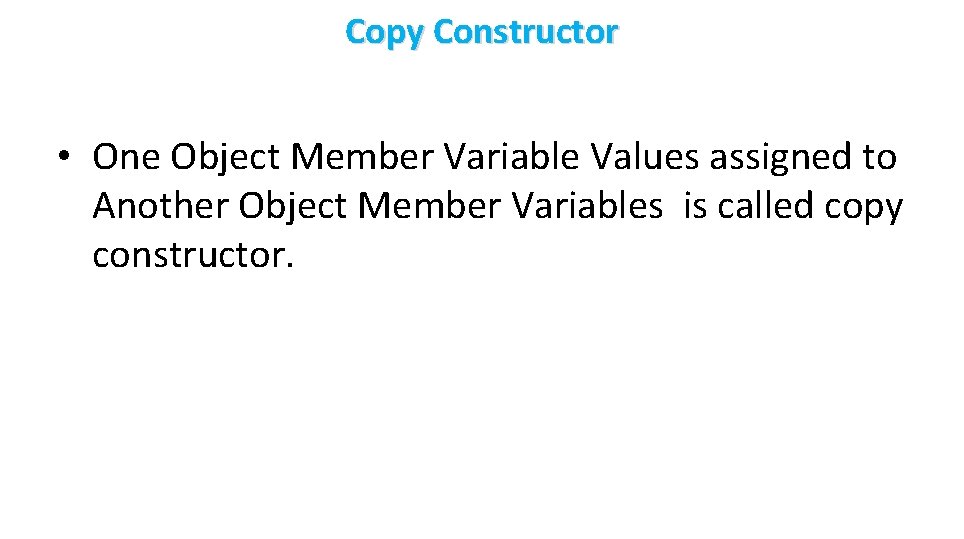
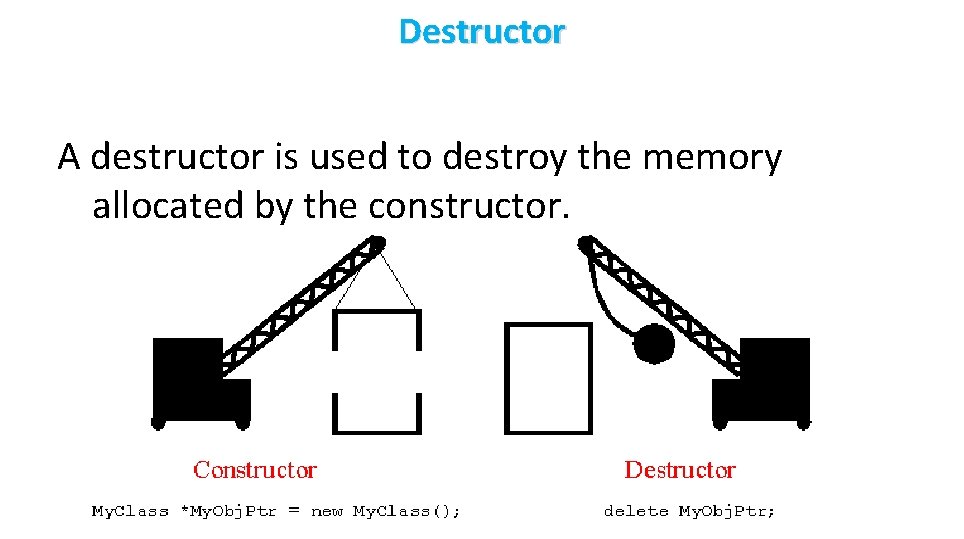
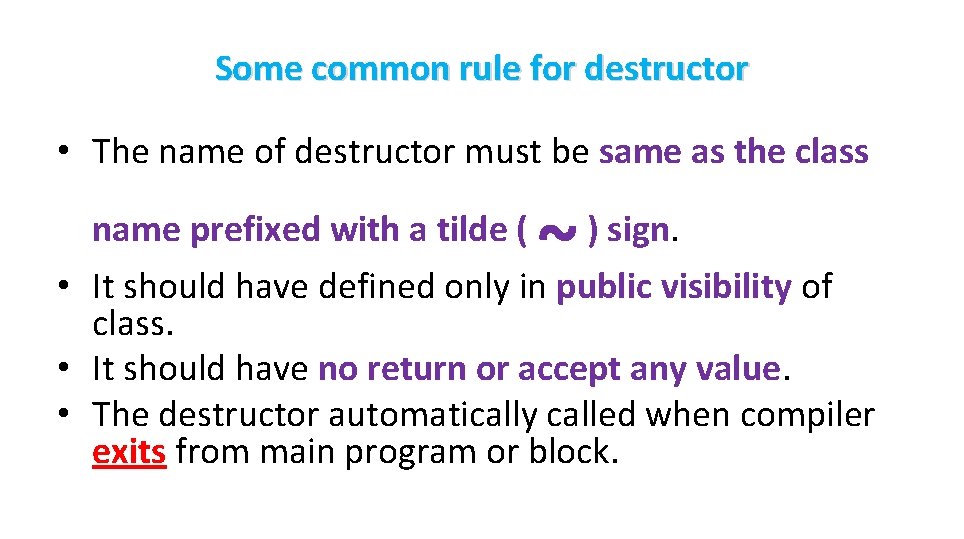
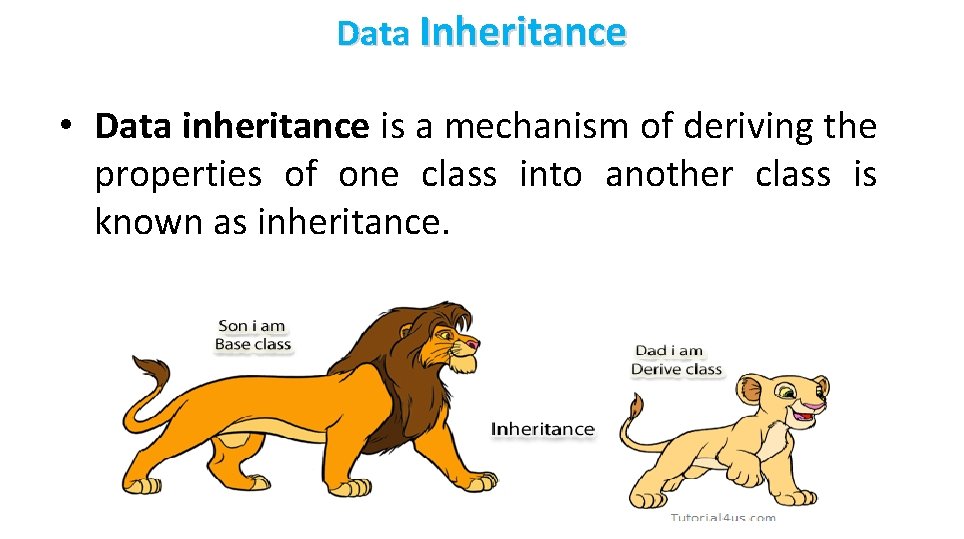
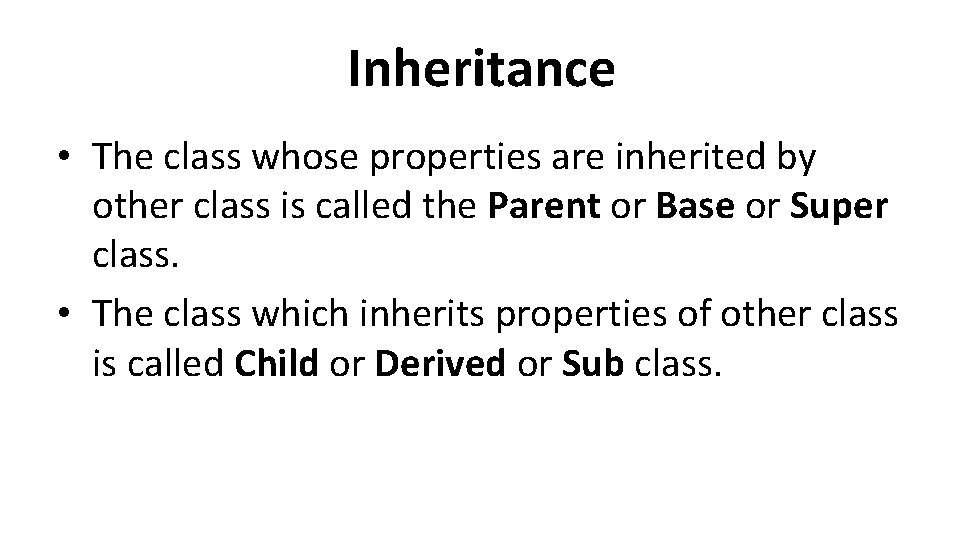
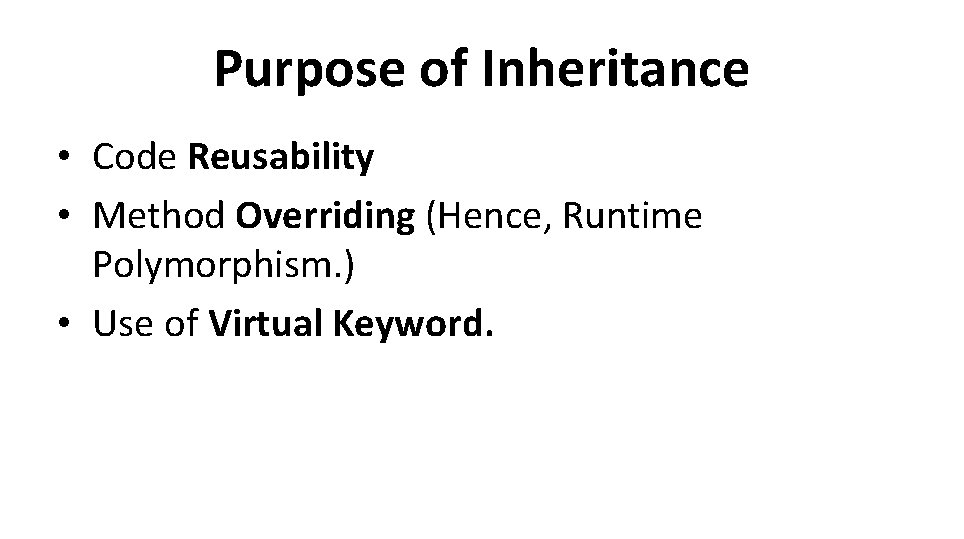
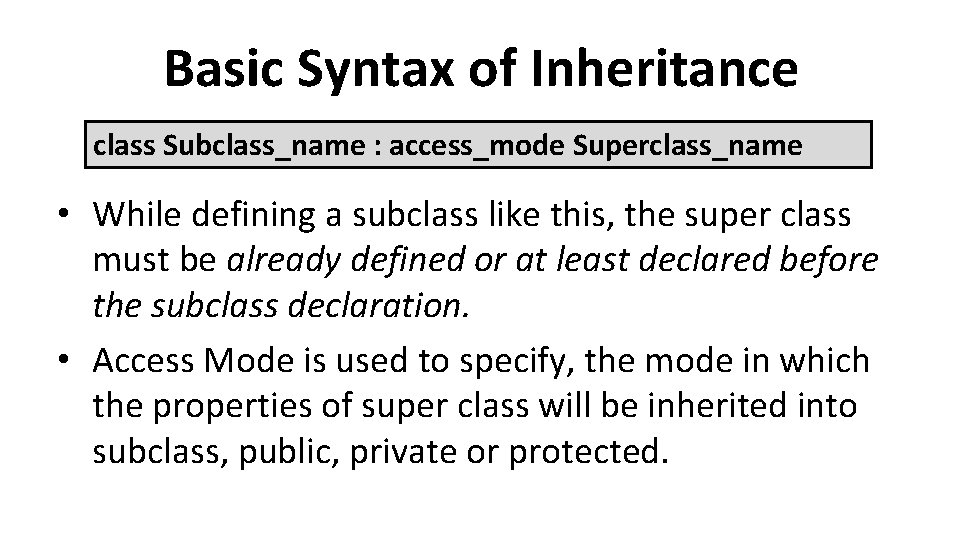
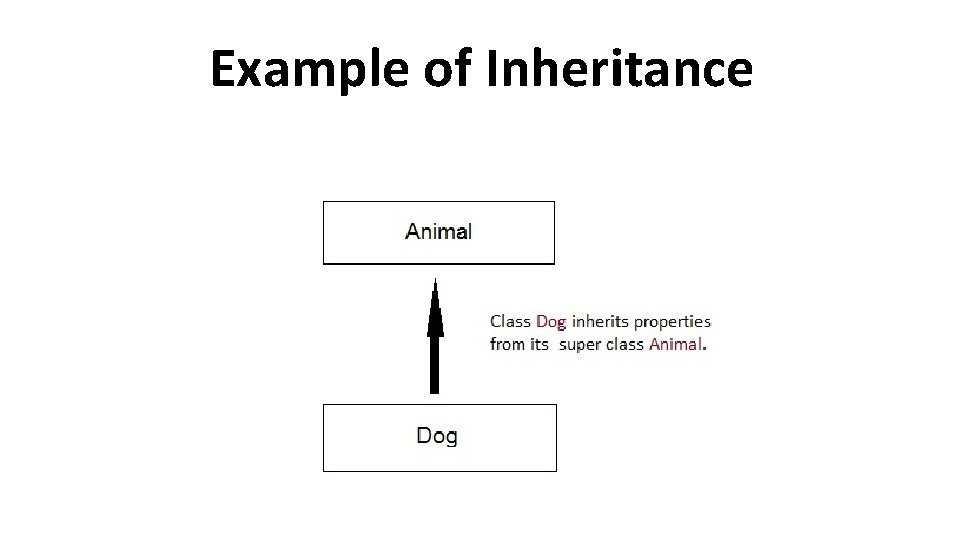
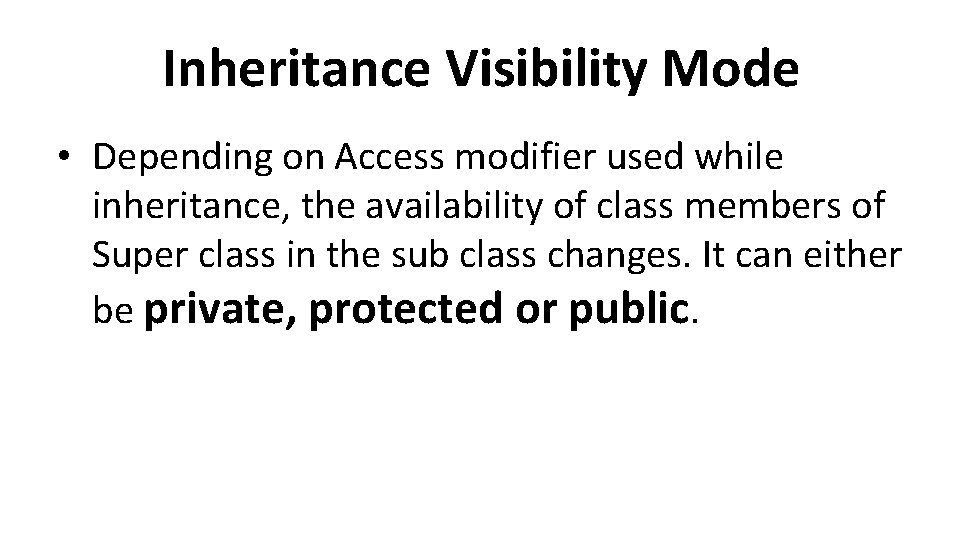
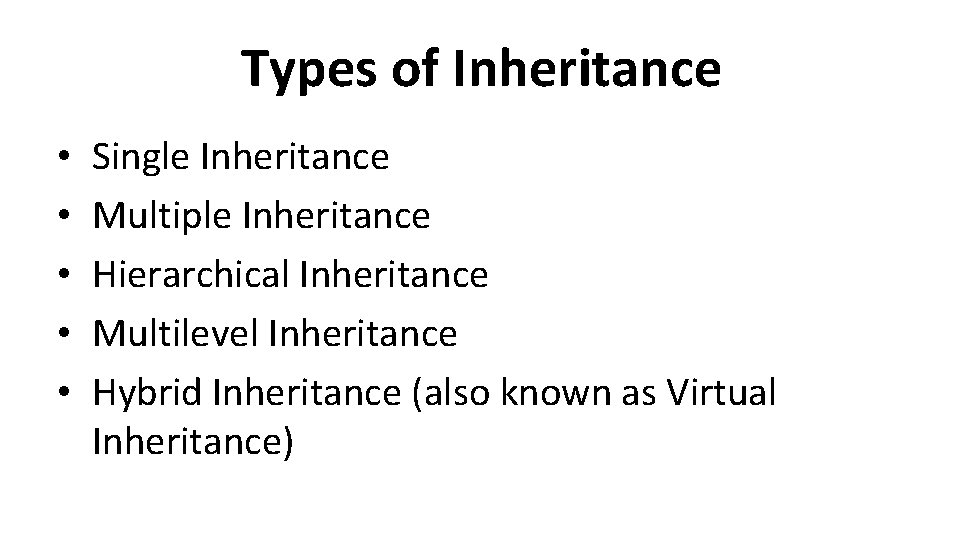
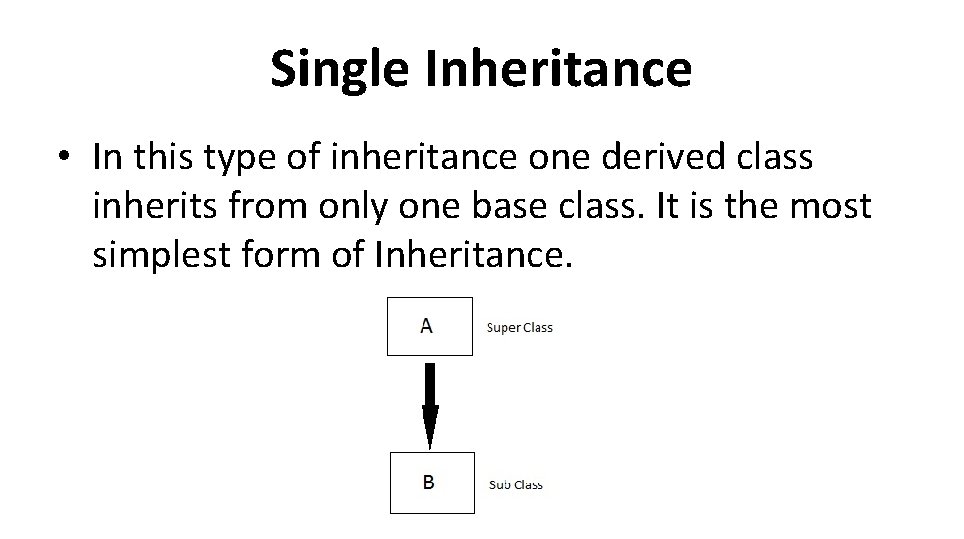
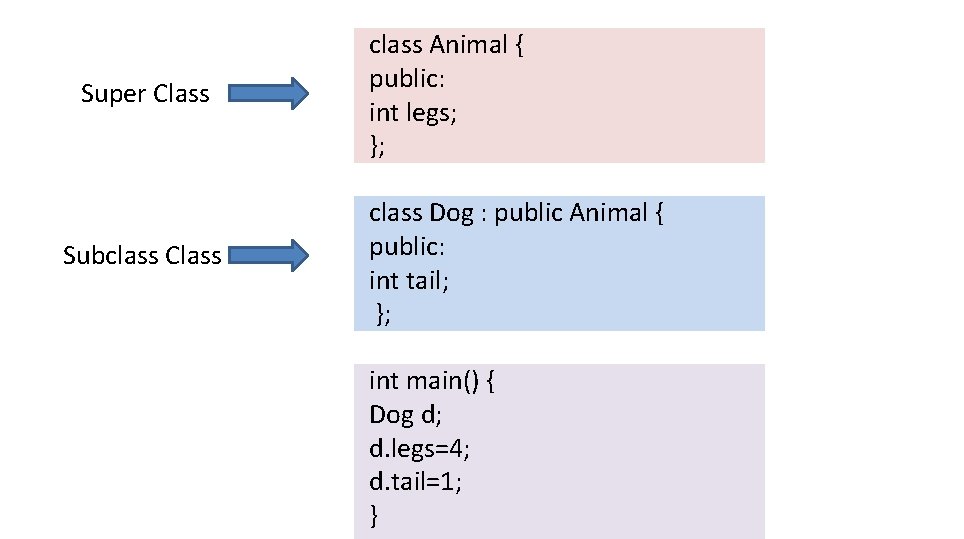
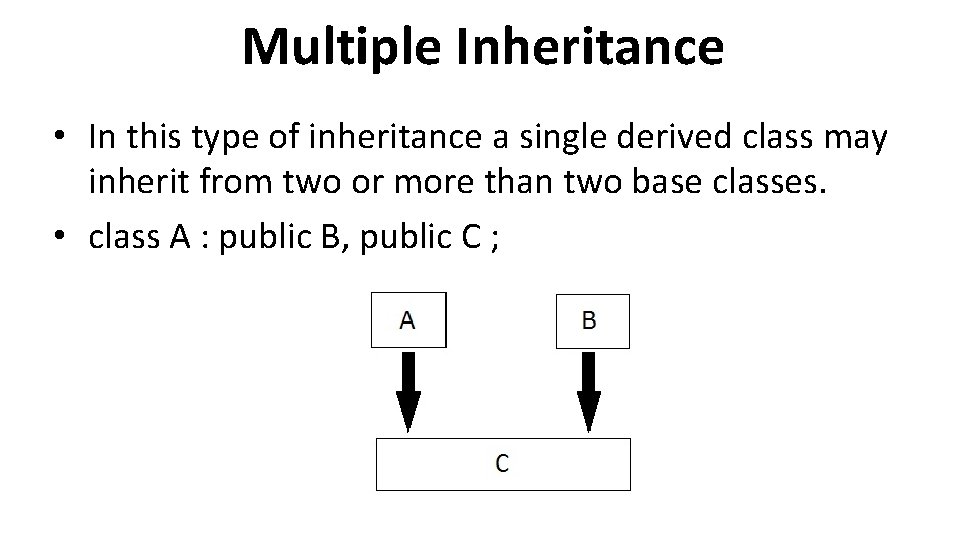
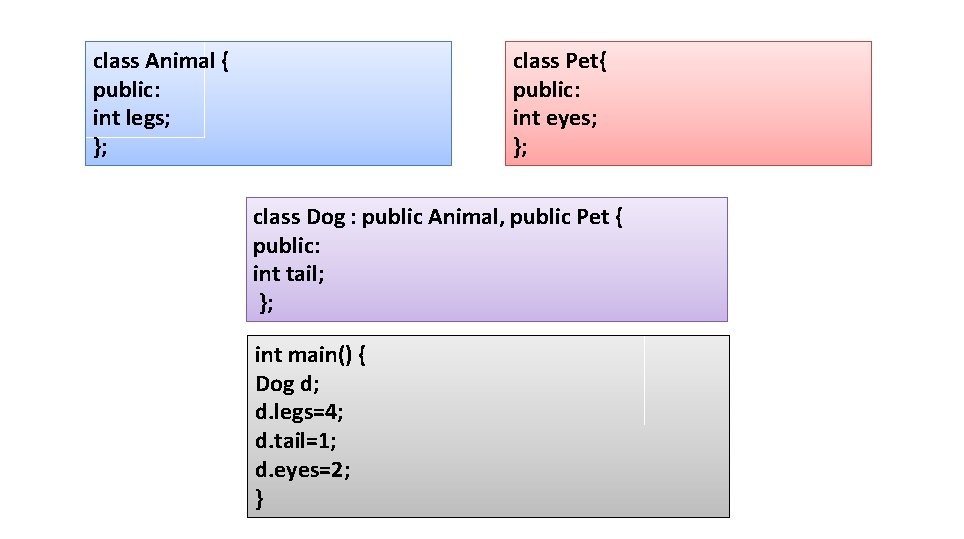
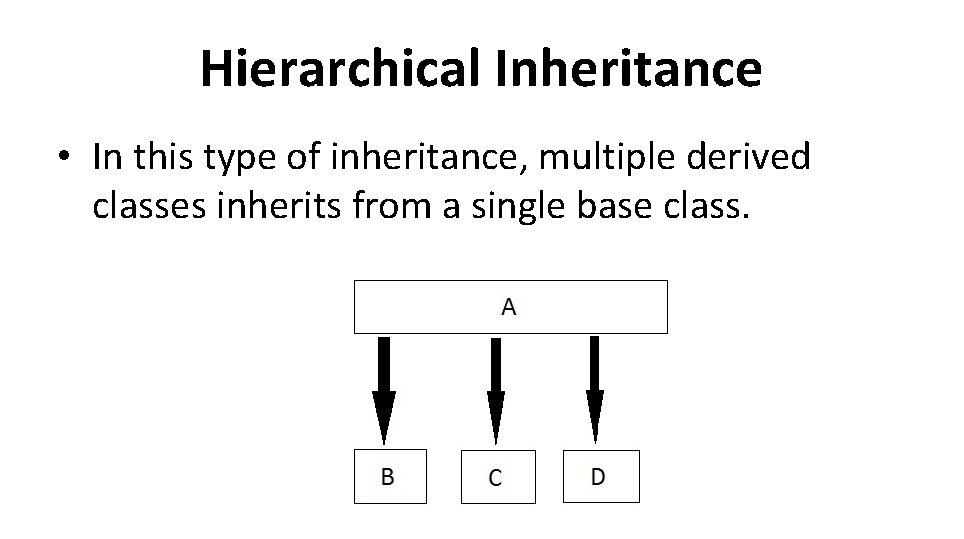
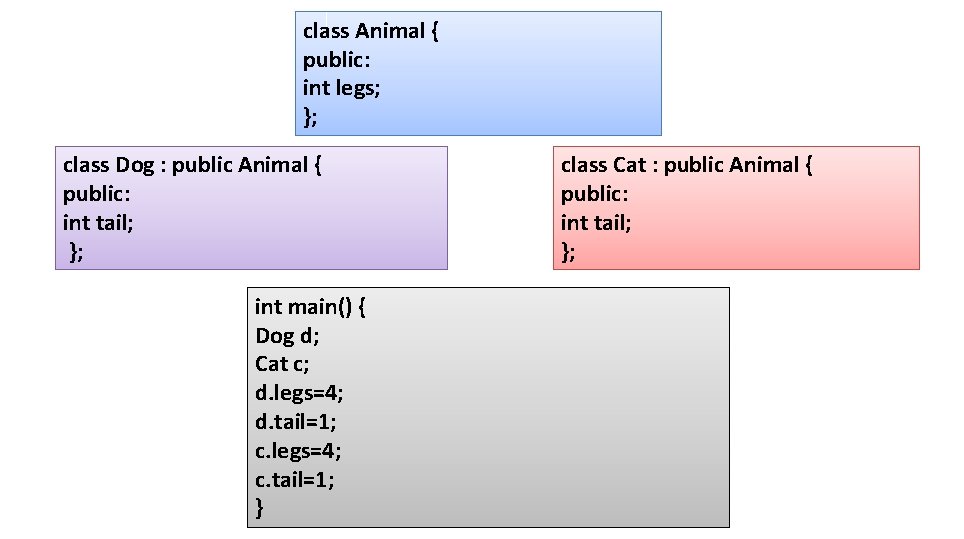
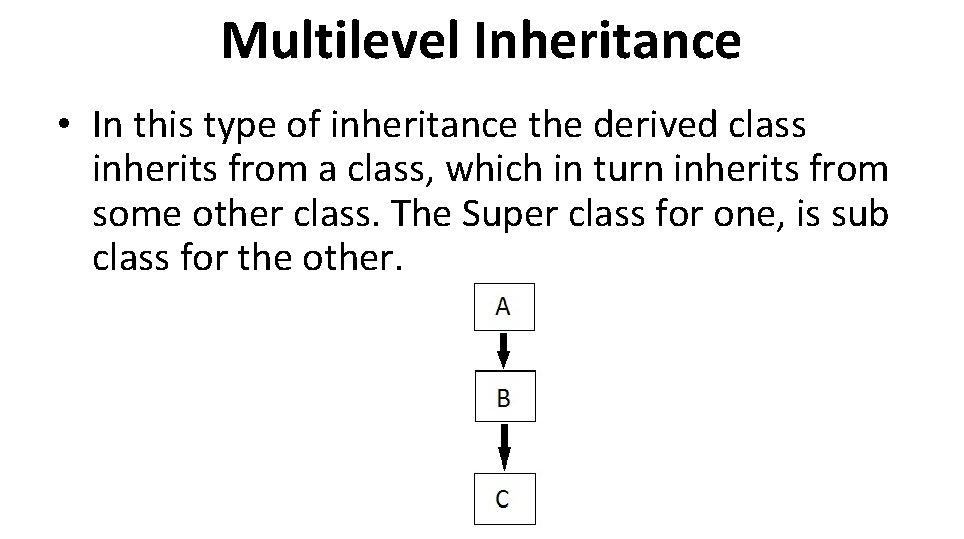
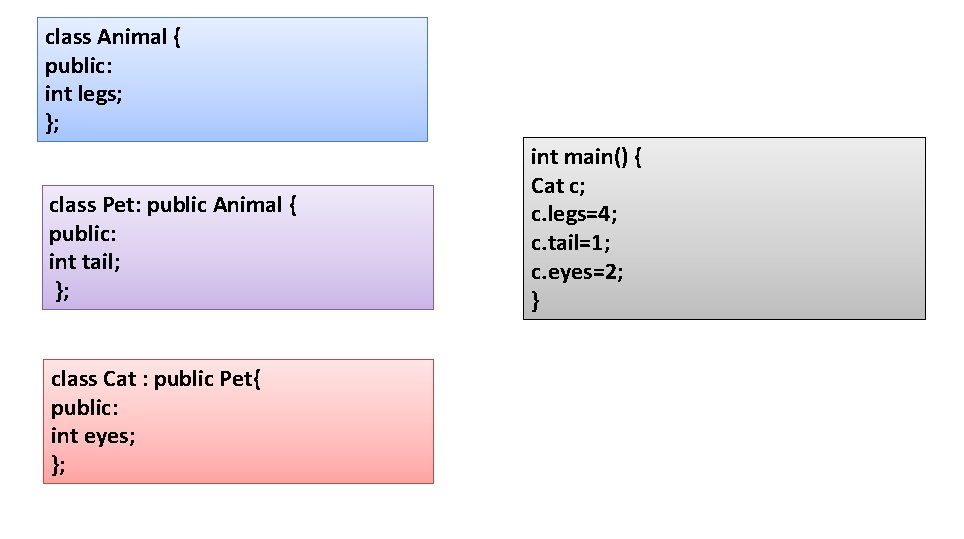
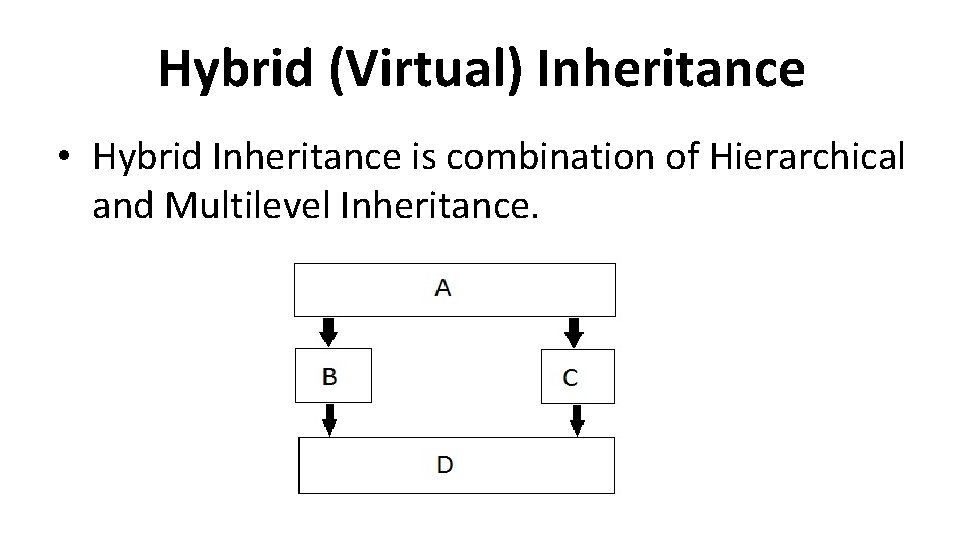
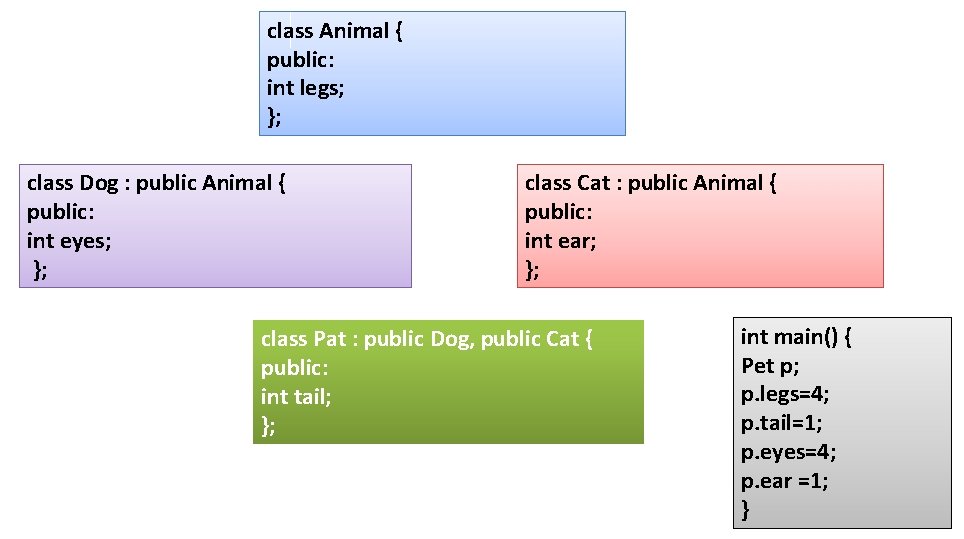
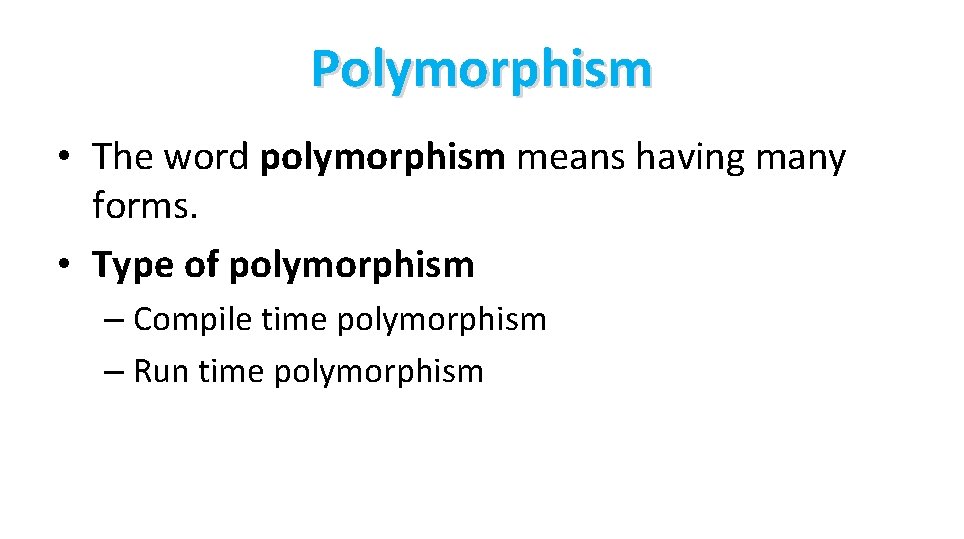
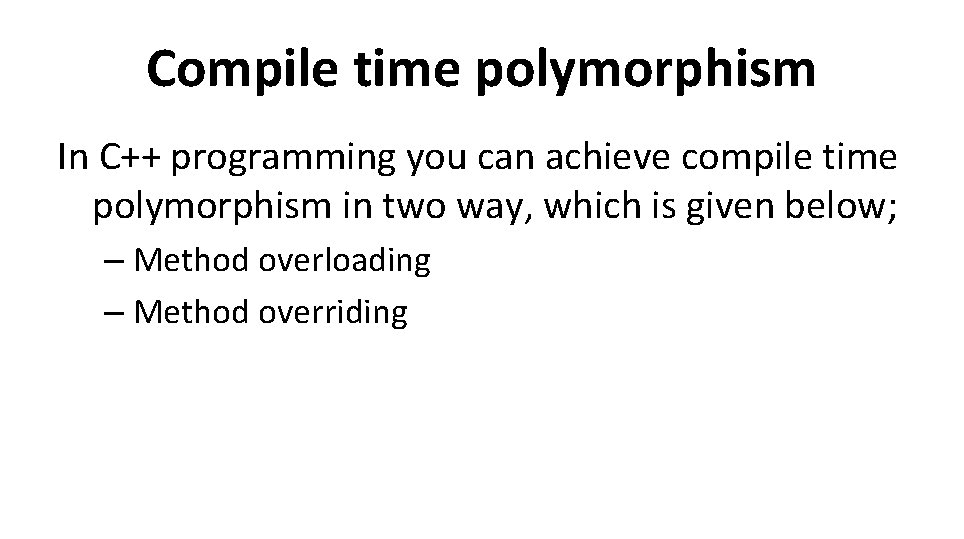
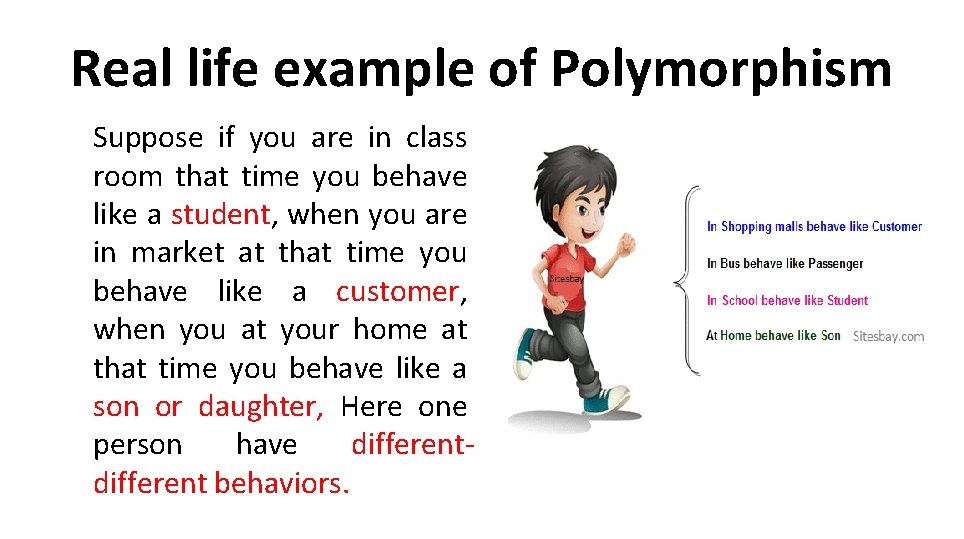
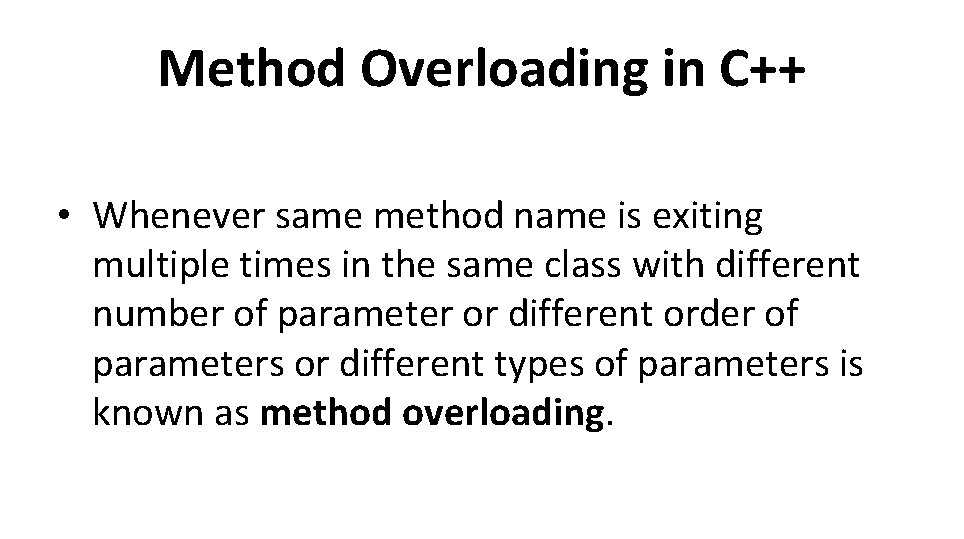
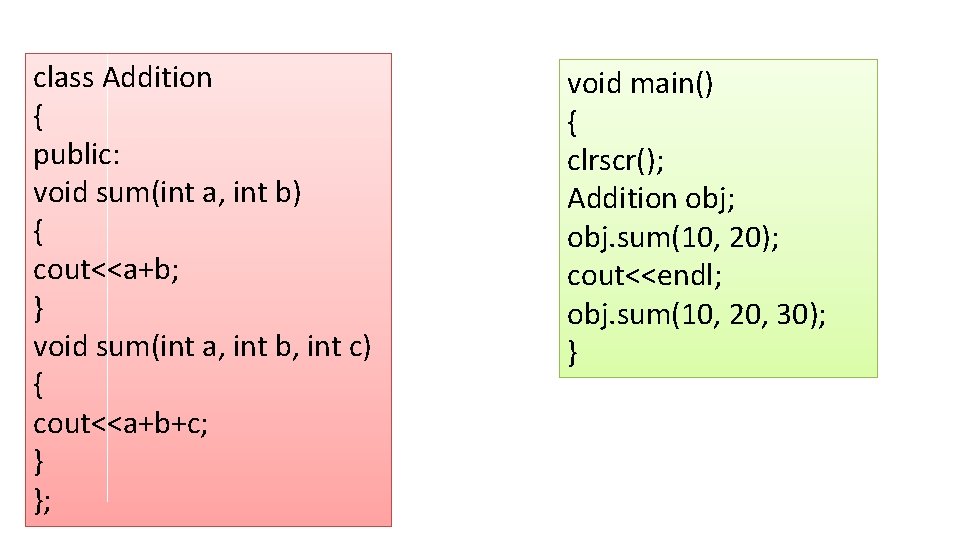
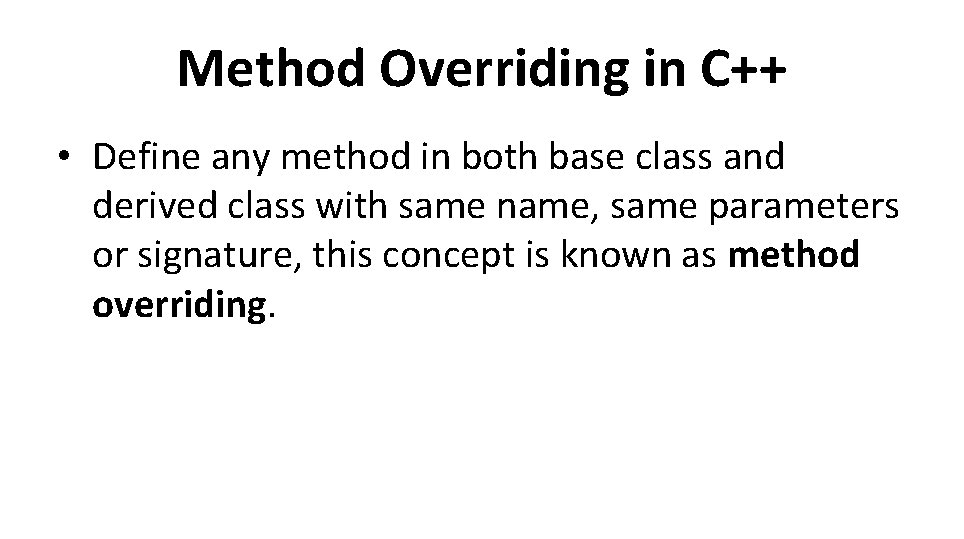
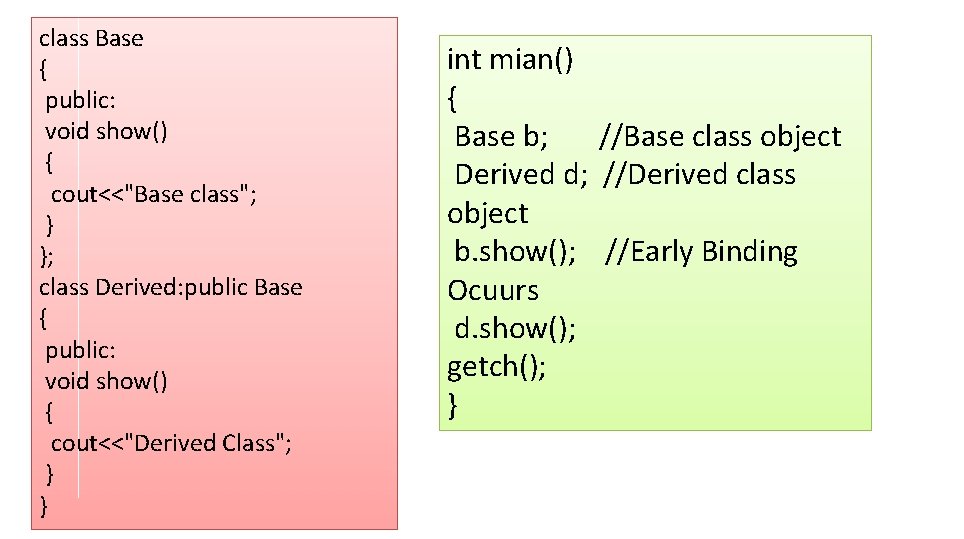
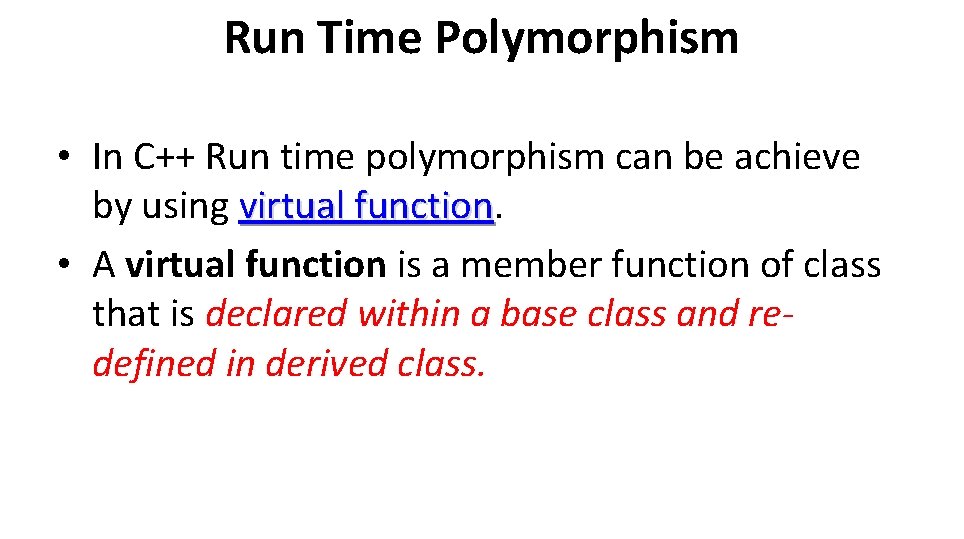
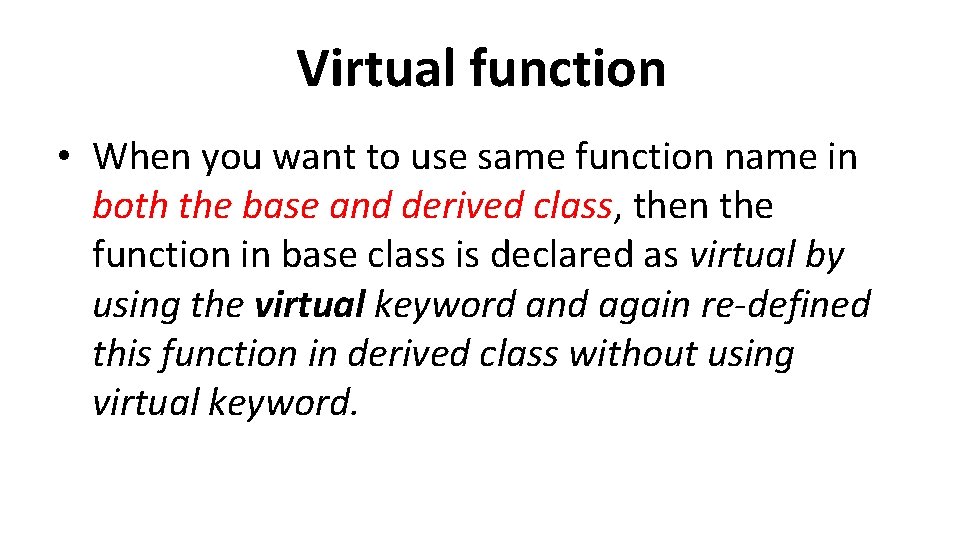
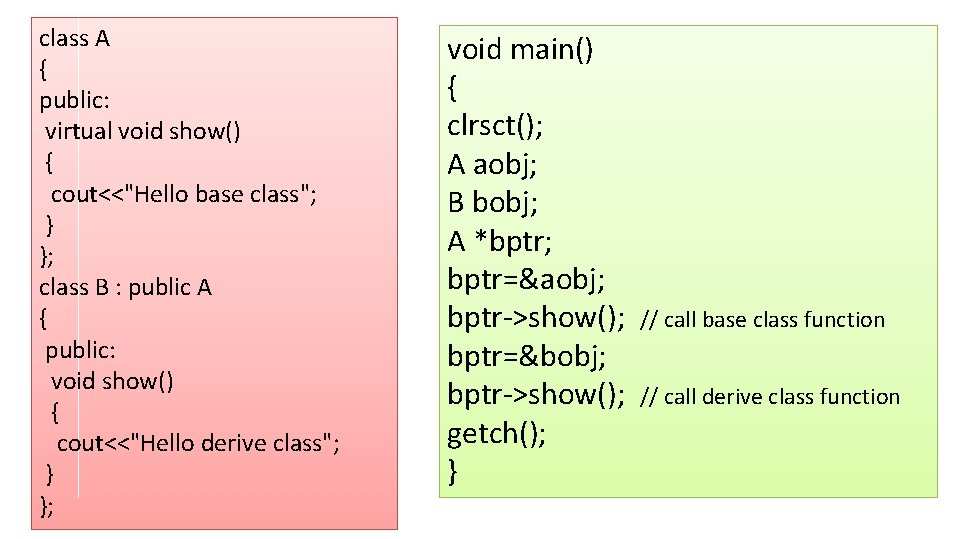
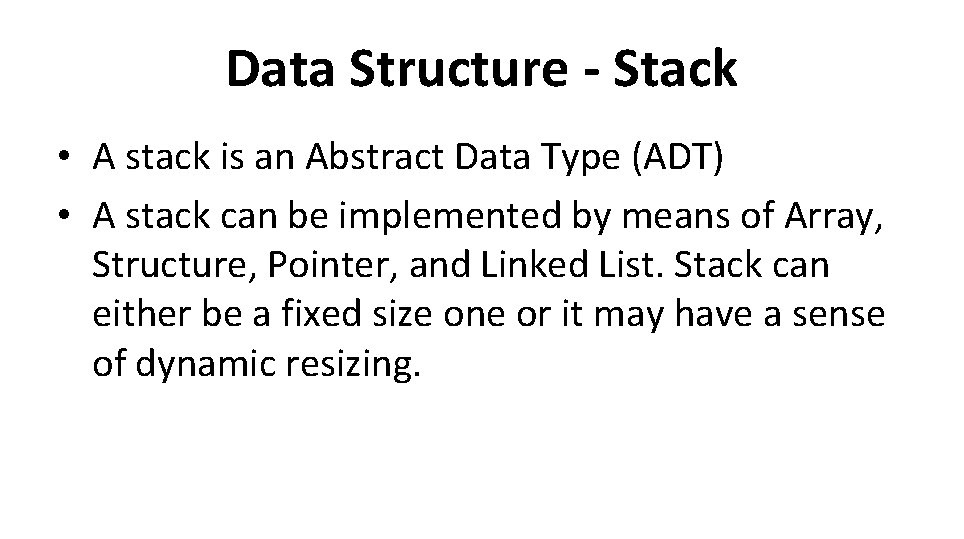
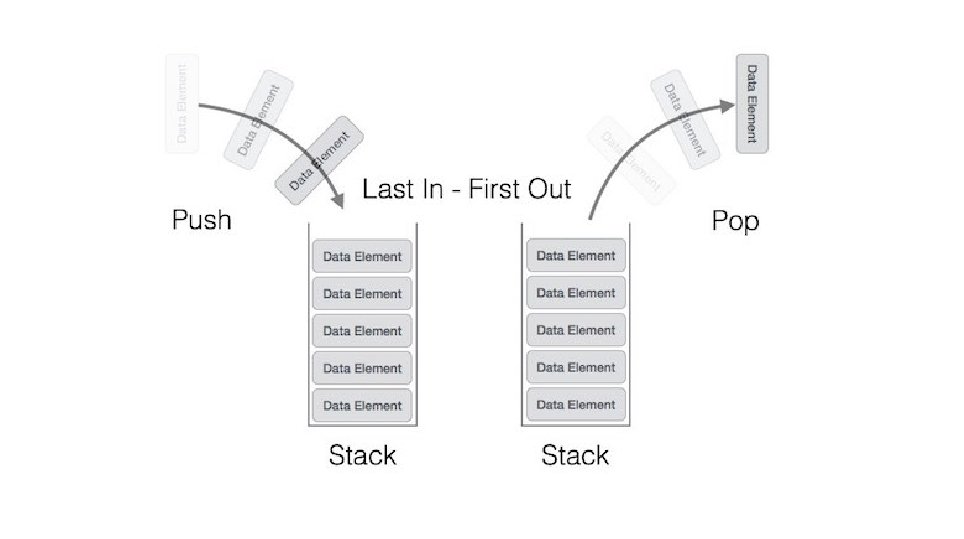
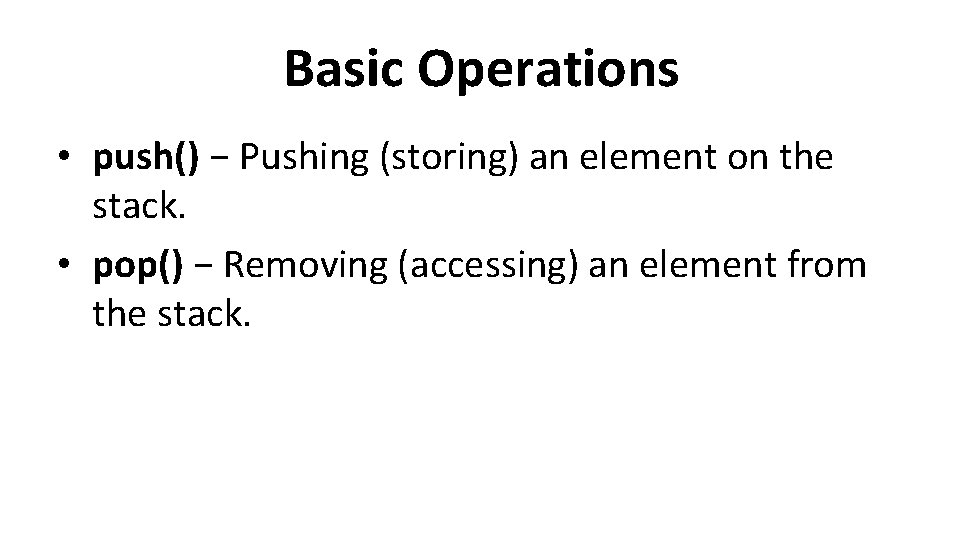
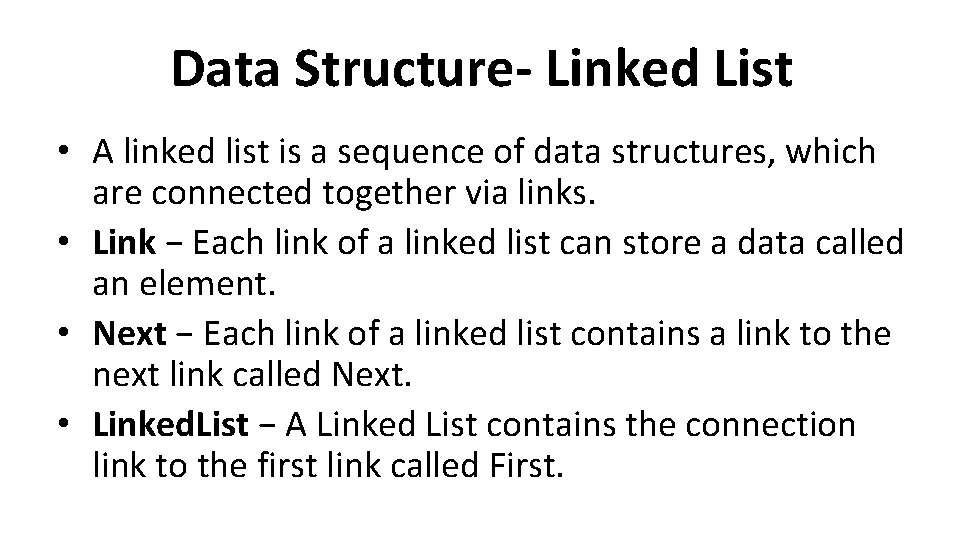
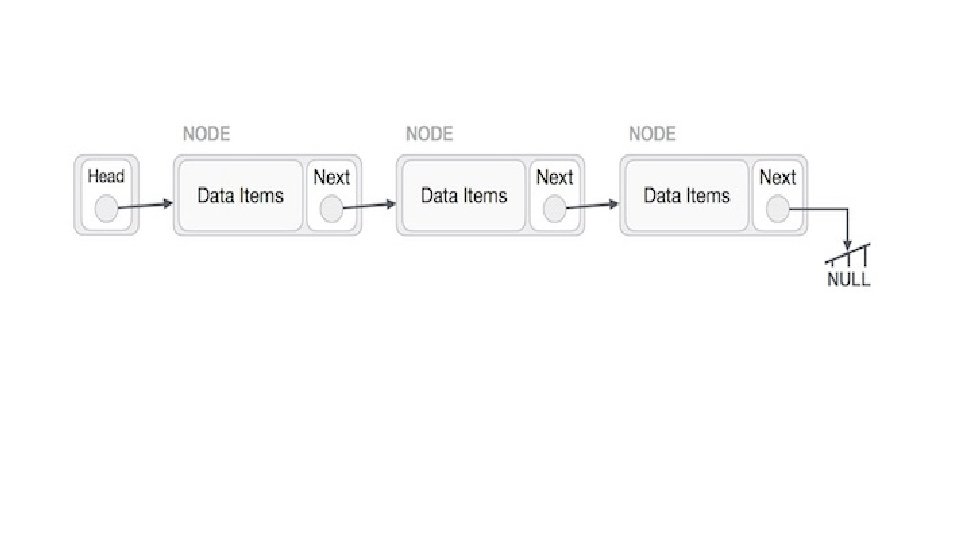
- Slides: 61
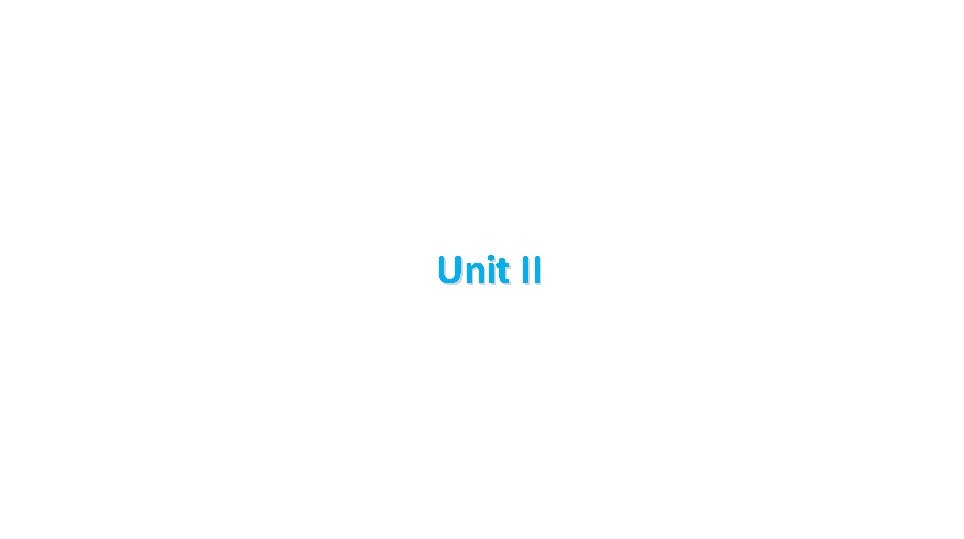
Unit II
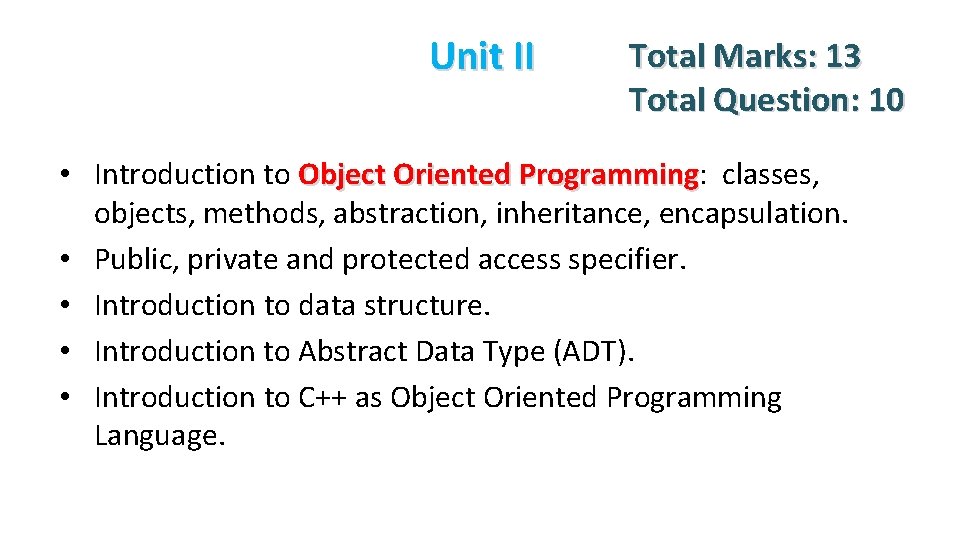
Unit II Total Marks: 13 Total Question: 10 • Introduction to Object Oriented Programming: Programming classes, objects, methods, abstraction, inheritance, encapsulation. • Public, private and protected access specifier. • Introduction to data structure. • Introduction to Abstract Data Type (ADT). • Introduction to C++ as Object Oriented Programming Language.
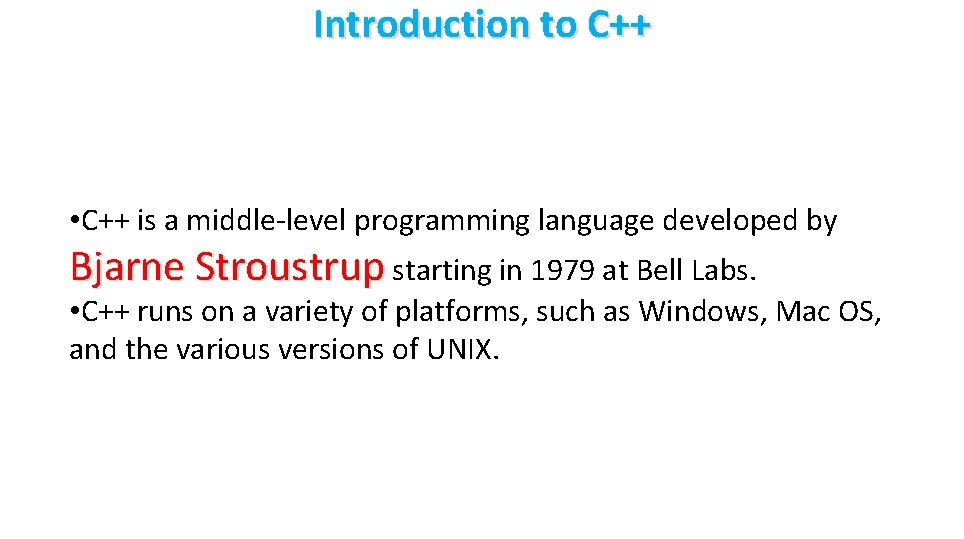
Introduction to C++ • C++ is a middle-level programming language developed by Bjarne Stroustrup starting in 1979 at Bell Labs. • C++ runs on a variety of platforms, such as Windows, Mac OS, and the various versions of UNIX.
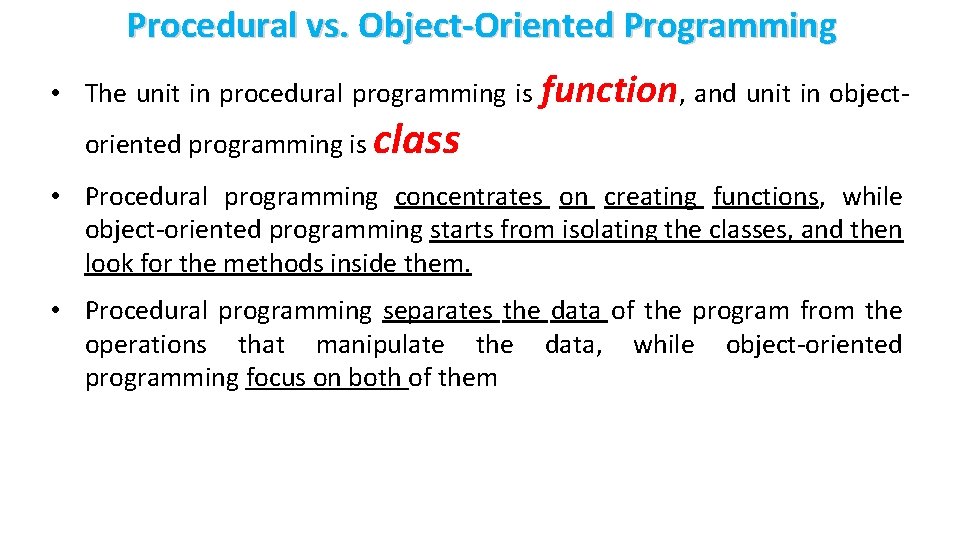
Procedural vs. Object-Oriented Programming • The unit in procedural programming is oriented programming is class function, and unit in object- • Procedural programming concentrates on creating functions, while object-oriented programming starts from isolating the classes, and then look for the methods inside them. • Procedural programming separates the data of the program from the operations that manipulate the data, while object-oriented programming focus on both of them
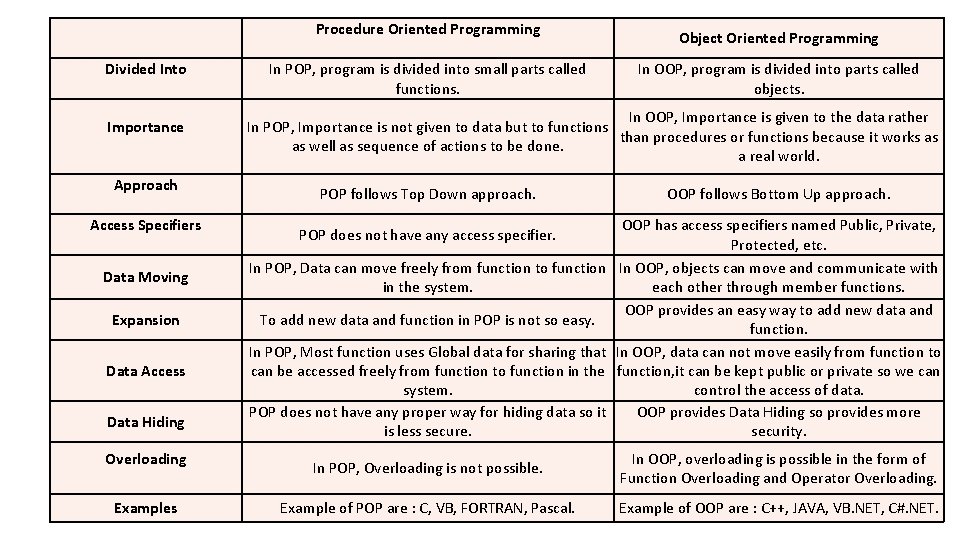
Procedure Oriented Programming Divided Into Importance Approach Access Specifiers In POP, program is divided into small parts called functions. POP follows Top Down approach. POP does not have any access specifier. In POP, Data can move freely from function to function in the system. Expansion To add new data and function in POP is not so easy. Data Hiding Overloading Examples In OOP, program is divided into parts called objects. In OOP, Importance is given to the data rather In POP, Importance is not given to data but to functions than procedures or functions because it works as as well as sequence of actions to be done. a real world. Data Moving Data Access Object Oriented Programming In POP, Most function uses Global data for sharing that can be accessed freely from function to function in the system. POP does not have any proper way for hiding data so it is less secure. OOP follows Bottom Up approach. OOP has access specifiers named Public, Private, Protected, etc. In OOP, objects can move and communicate with each other through member functions. OOP provides an easy way to add new data and function. In OOP, data can not move easily from function to function, it can be kept public or private so we can control the access of data. OOP provides Data Hiding so provides more security. In POP, Overloading is not possible. In OOP, overloading is possible in the form of Function Overloading and Operator Overloading. Example of POP are : C, VB, FORTRAN, Pascal. Example of OOP are : C++, JAVA, VB. NET, C#. NET.
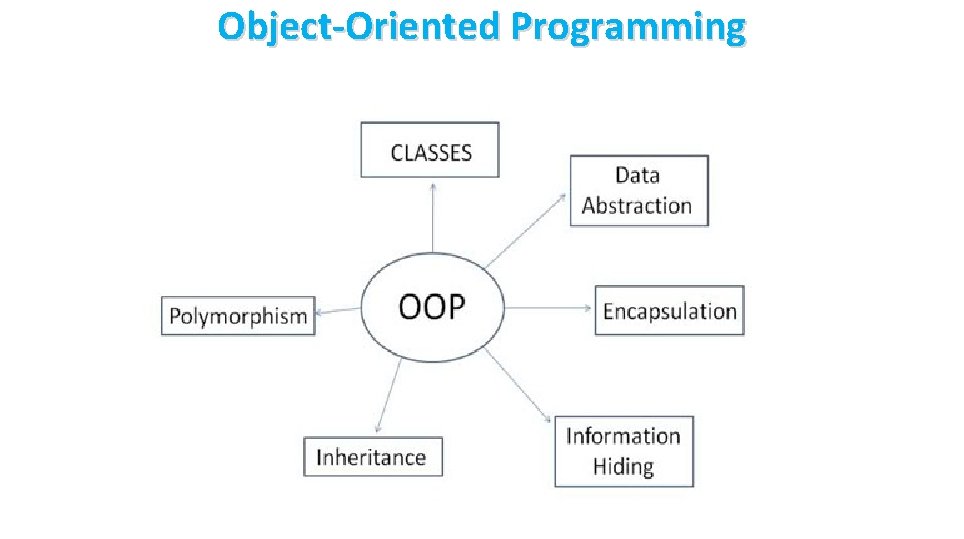
Object-Oriented Programming
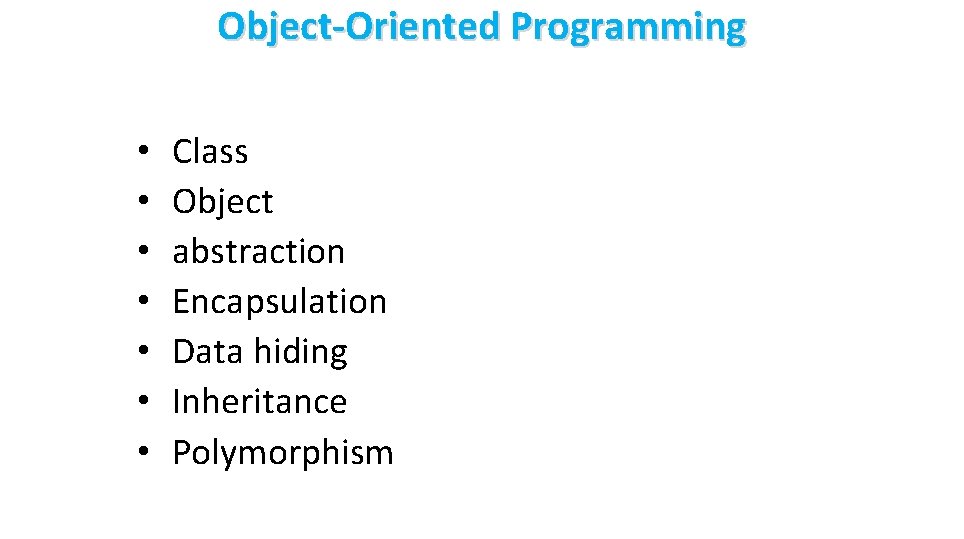
Object-Oriented Programming • • Class Object abstraction Encapsulation Data hiding Inheritance Polymorphism
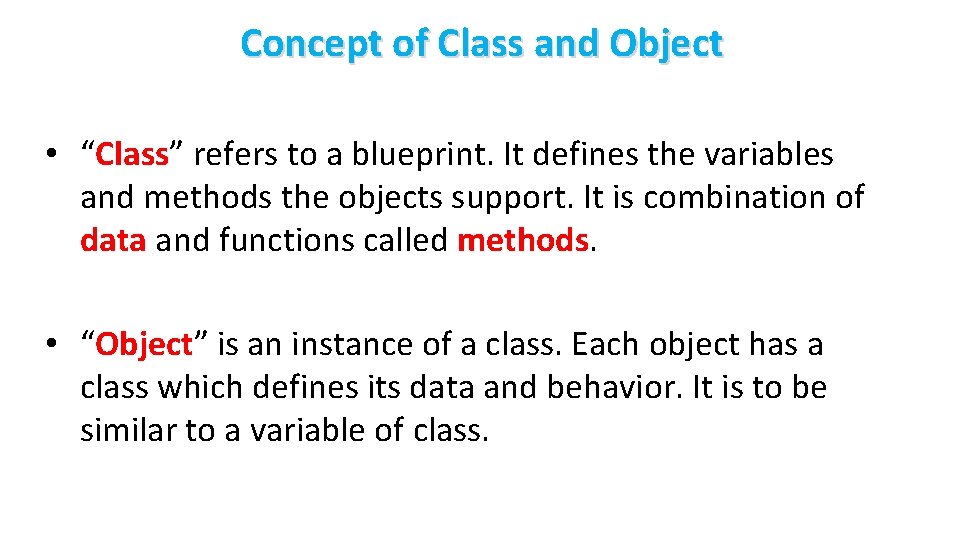
Concept of Class and Object • “Class” refers to a blueprint. It defines the variables and methods the objects support. It is combination of data and functions called methods. • “Object” is an instance of a class. Each object has a class which defines its data and behavior. It is to be similar to a variable of class.
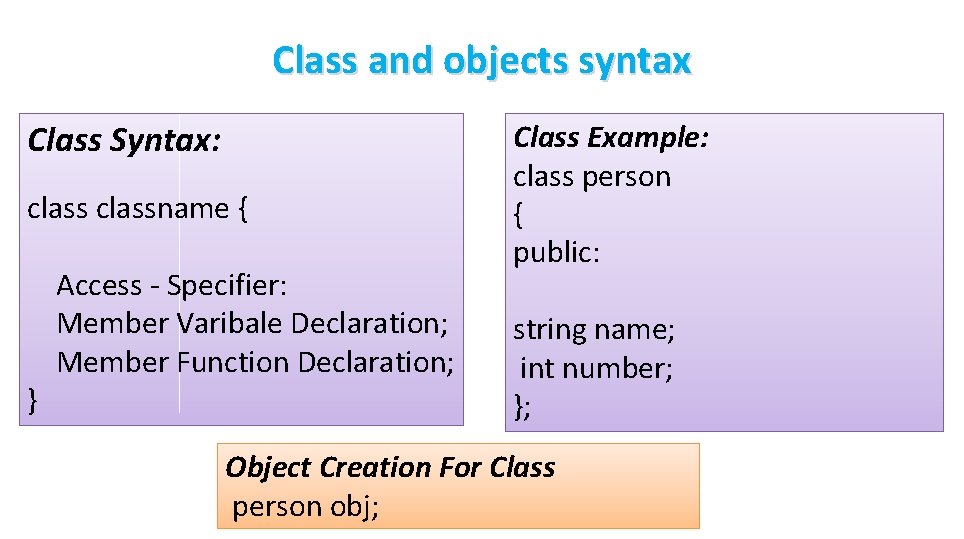
Class and objects syntax Class Syntax: classname { } Access - Specifier: Member Varibale Declaration; Member Function Declaration; Class Example: class person { public: string name; int number; }; Object Creation For Class person obj;
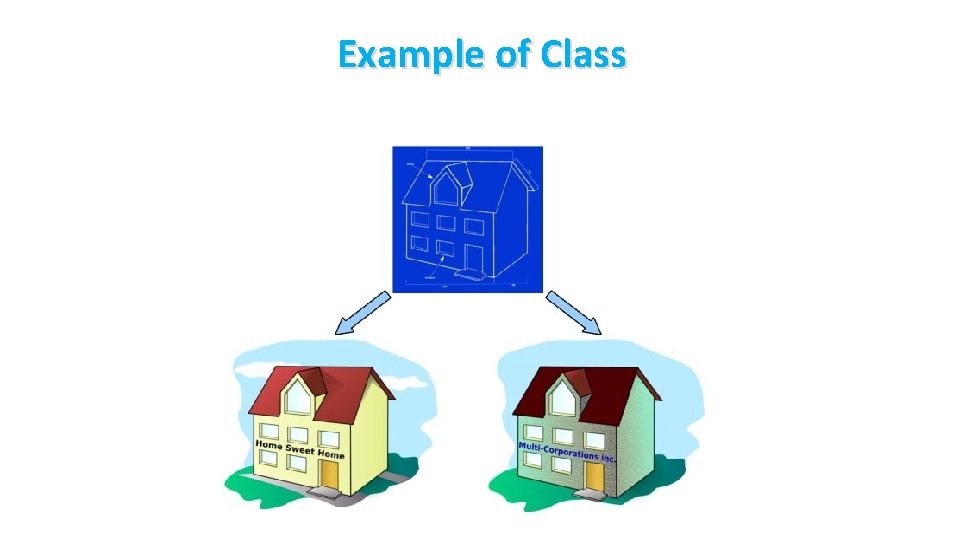
Example of Class
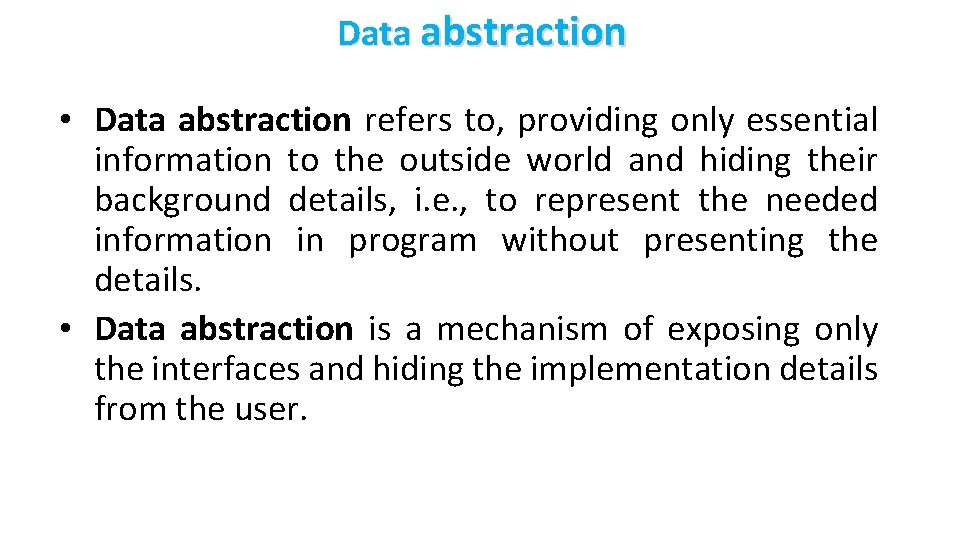
Data abstraction • Data abstraction refers to, providing only essential information to the outside world and hiding their background details, i. e. , to represent the needed information in program without presenting the details. • Data abstraction is a mechanism of exposing only the interfaces and hiding the implementation details from the user.
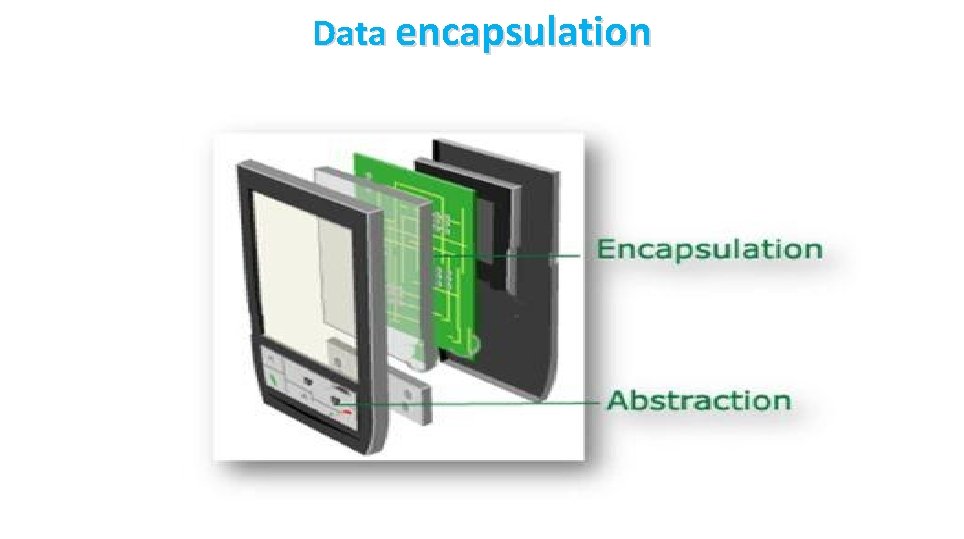
Data encapsulation
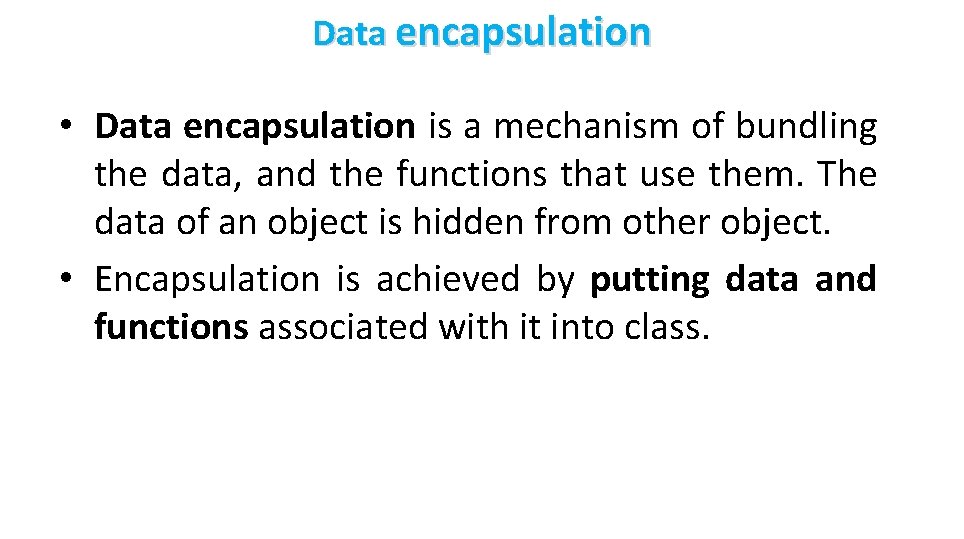
Data encapsulation • Data encapsulation is a mechanism of bundling the data, and the functions that use them. The data of an object is hidden from other object. • Encapsulation is achieved by putting data and functions associated with it into class.
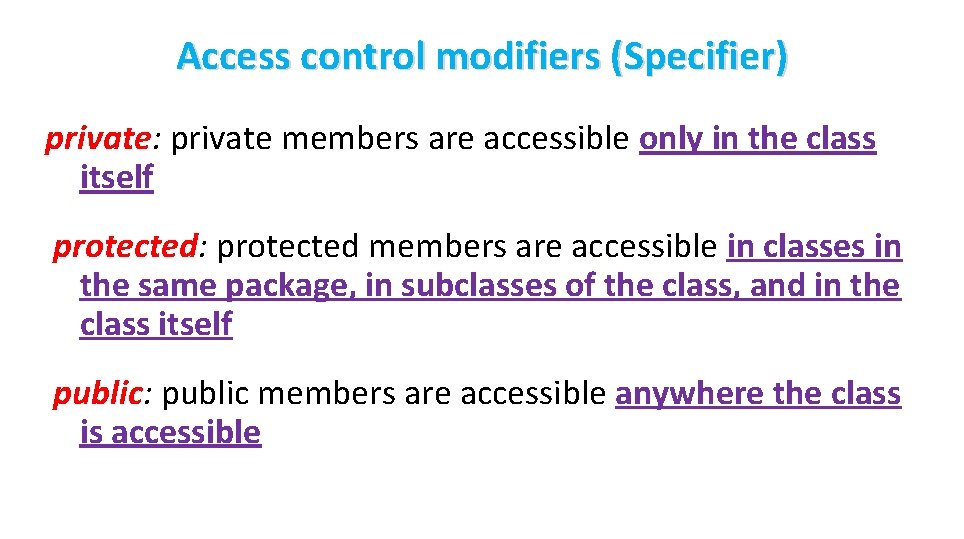
Access control modifiers (Specifier) private: private members are accessible only in the class itself protected: protected members are accessible in classes in the same package, in subclasses of the class, and in the class itself public: public members are accessible anywhere the class is accessible
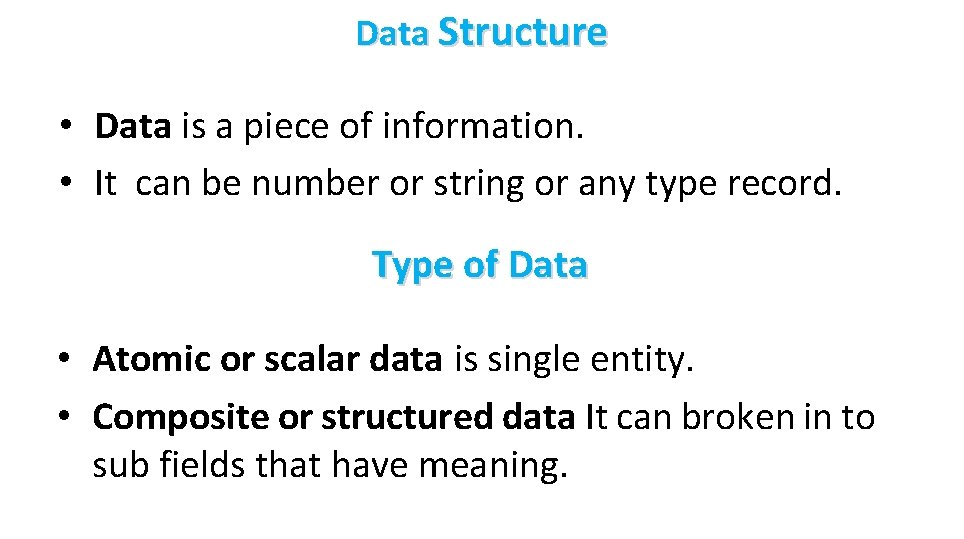
Data Structure • Data is a piece of information. • It can be number or string or any type record. Type of Data • Atomic or scalar data is single entity. • Composite or structured data It can broken in to sub fields that have meaning.
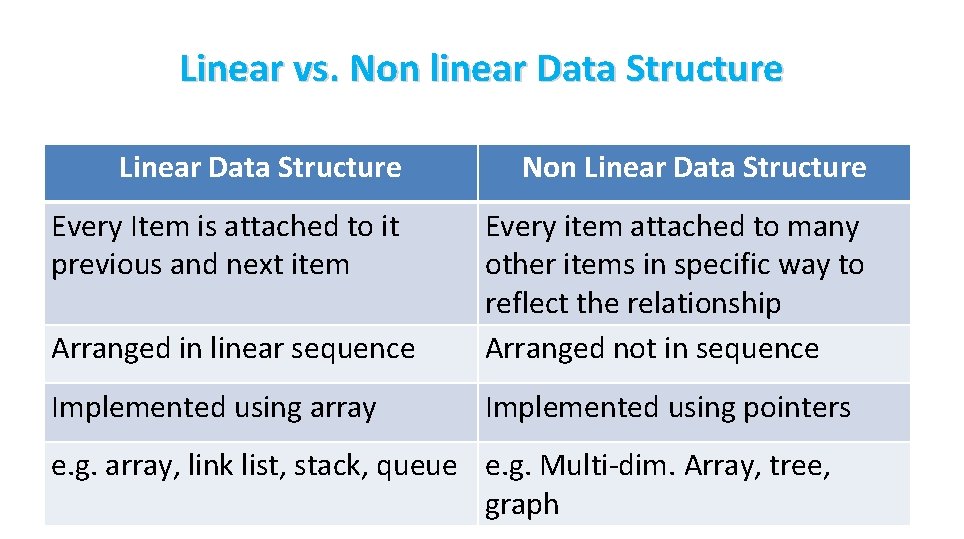
Linear vs. Non linear Data Structure Linear Data Structure Non Linear Data Structure Every Item is attached to it previous and next item Arranged in linear sequence Every item attached to many other items in specific way to reflect the relationship Arranged not in sequence Implemented using array Implemented using pointers e. g. array, link list, stack, queue e. g. Multi-dim. Array, tree, graph
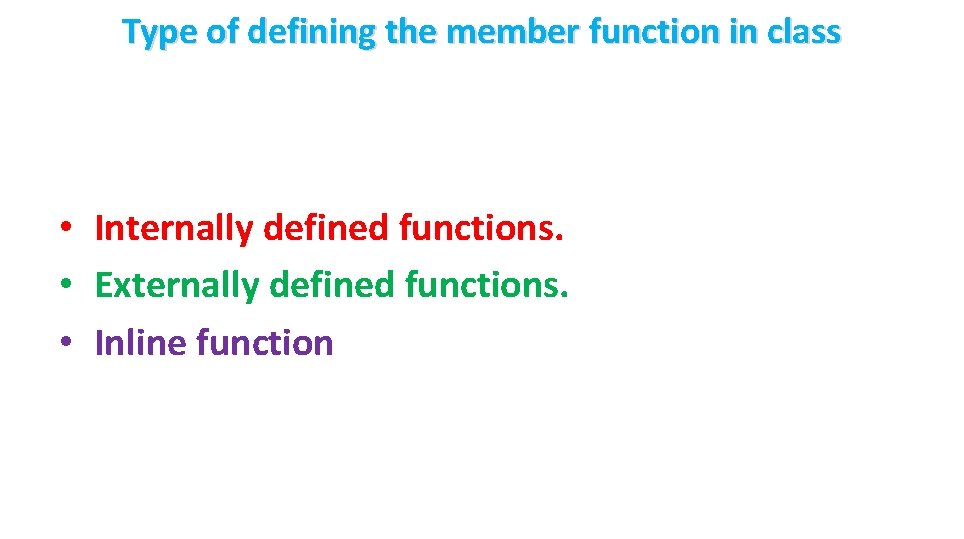
Type of defining the member function in class • Internally defined functions. • Externally defined functions. • Inline function
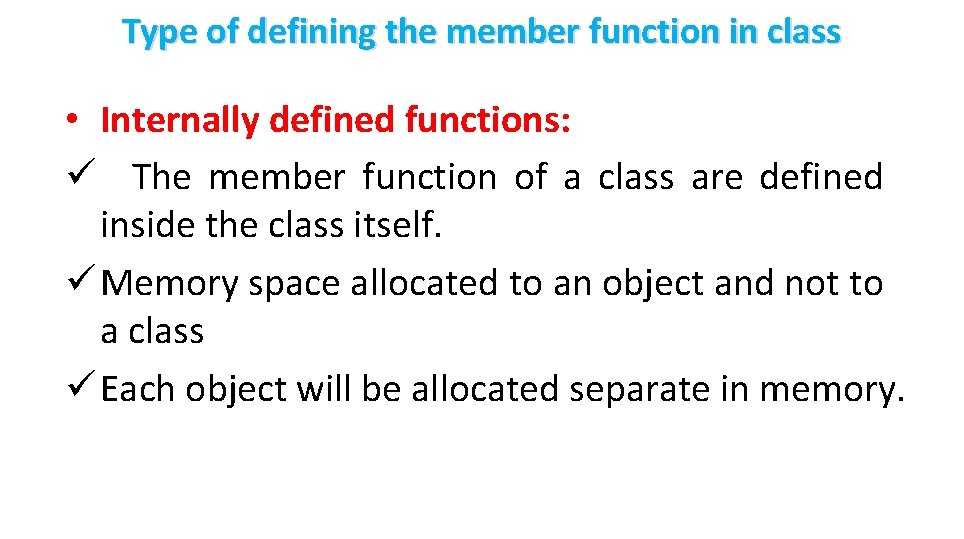
Type of defining the member function in class • Internally defined functions: ü The member function of a class are defined inside the class itself. ü Memory space allocated to an object and not to a class ü Each object will be allocated separate in memory.
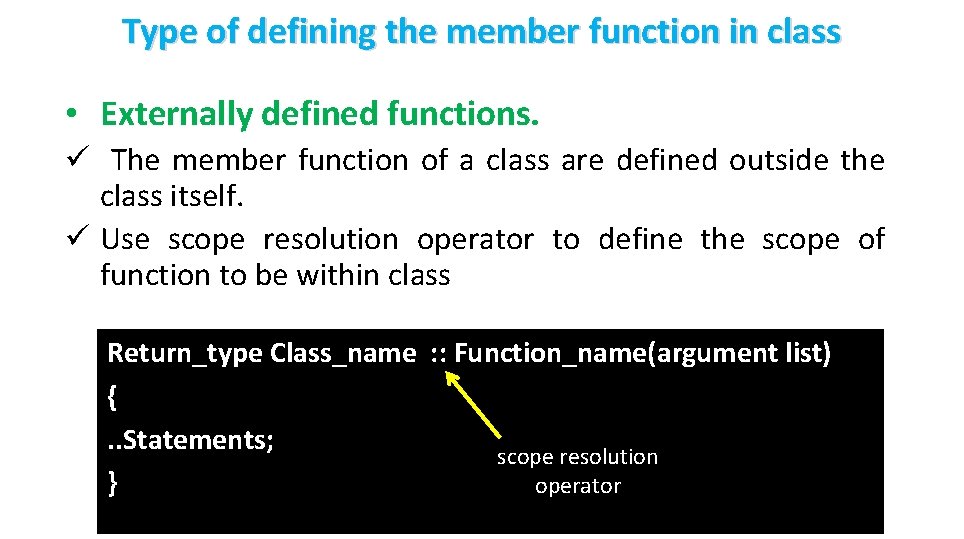
Type of defining the member function in class • Externally defined functions. ü The member function of a class are defined outside the class itself. ü Use scope resolution operator to define the scope of function to be within class Return_type Class_name : : Function_name(argument list) {. . Statements; scope resolution } operator
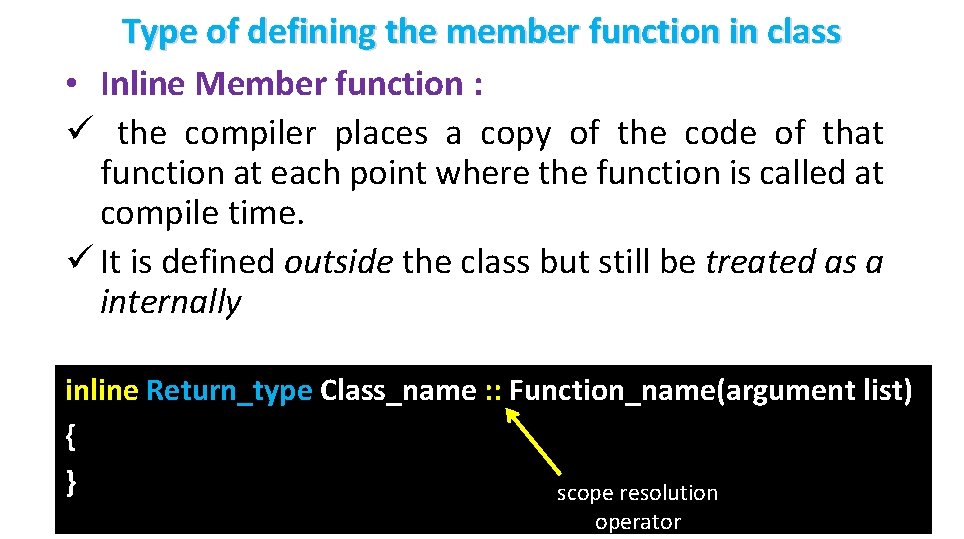
Type of defining the member function in class • Inline Member function : ü the compiler places a copy of the code of that function at each point where the function is called at compile time. ü It is defined outside the class but still be treated as a internally inline Return_type Class_name : : Function_name(argument list) { } scope resolution operator
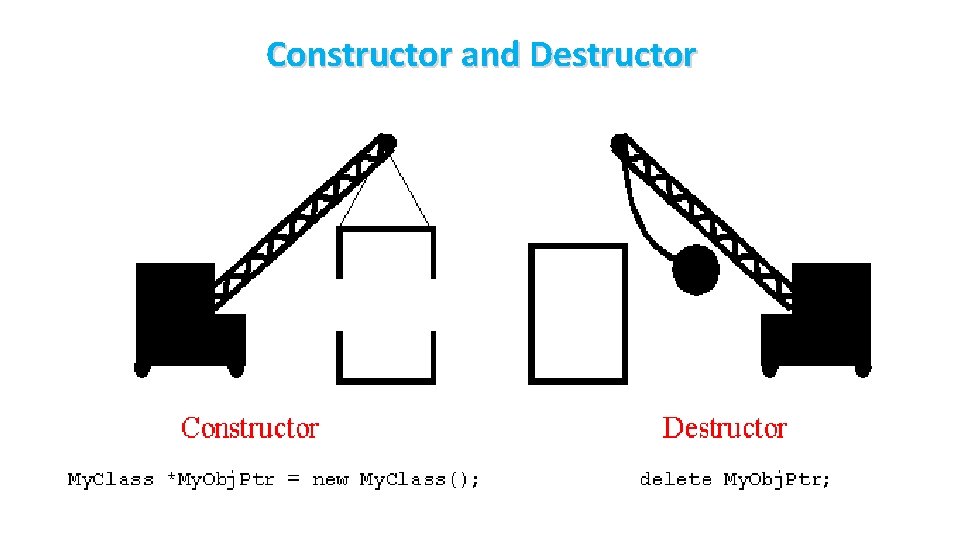
Constructor and Destructor
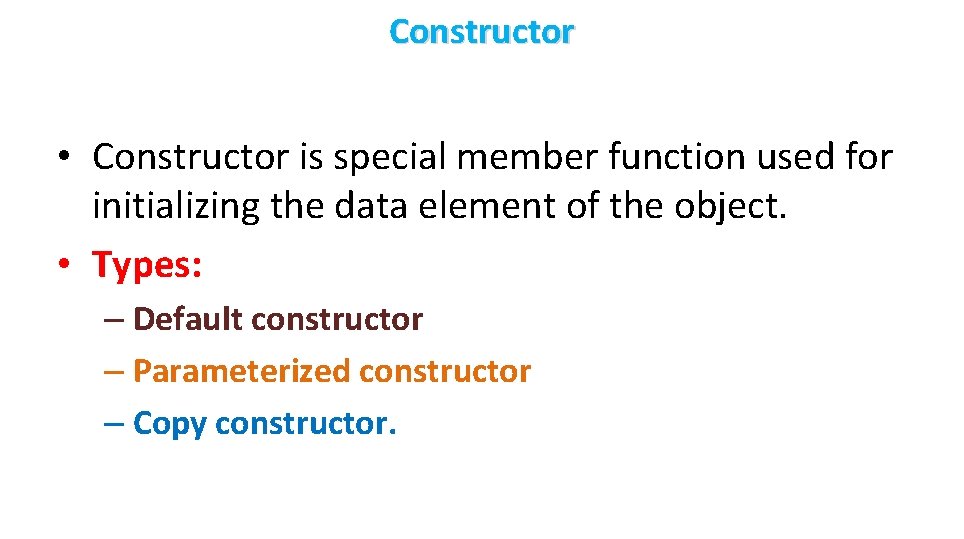
Constructor • Constructor is special member function used for initializing the data element of the object. • Types: – Default constructor – Parameterized constructor – Copy constructor.
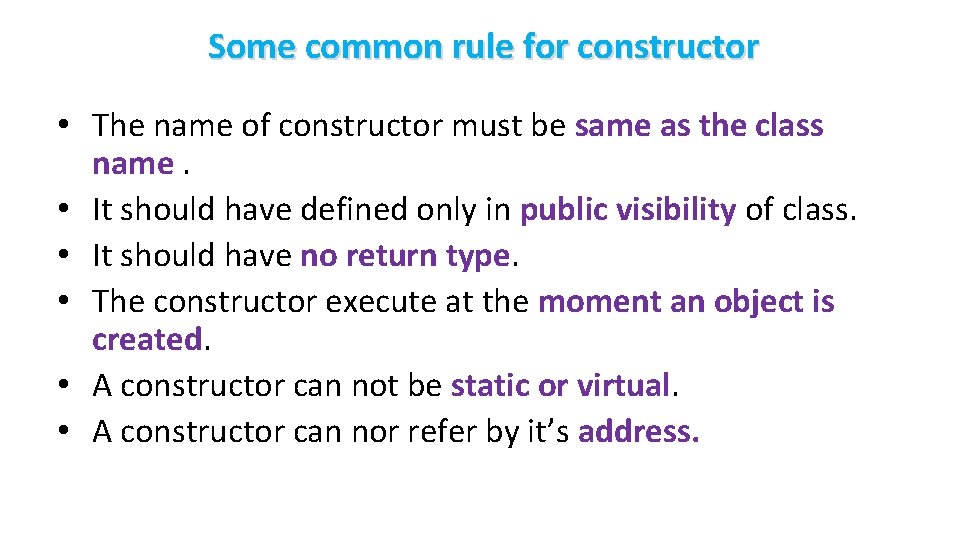
Some common rule for constructor • The name of constructor must be same as the class name. • It should have defined only in public visibility of class. • It should have no return type. • The constructor execute at the moment an object is created. • A constructor can not be static or virtual. • A constructor can nor refer by it’s address.
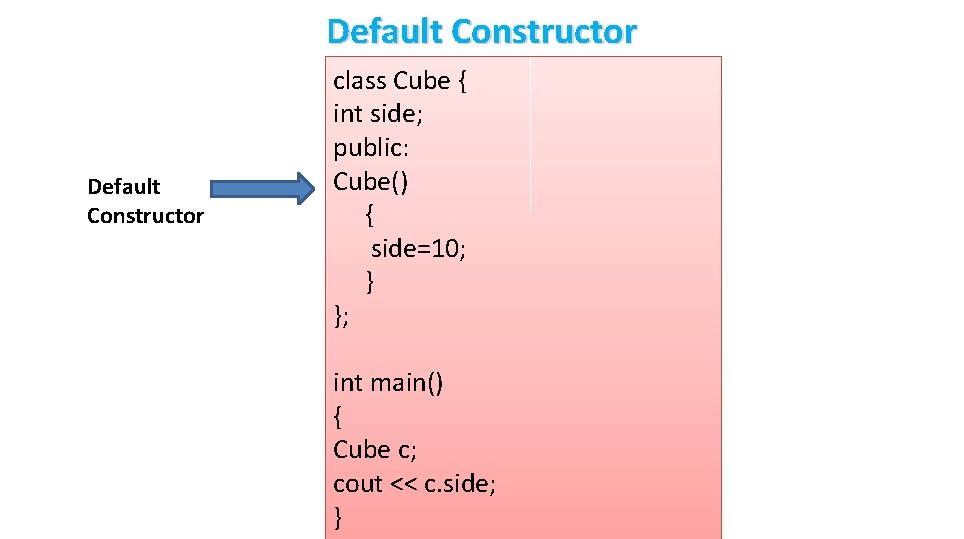
Default Constructor class Cube { int side; public: Cube() { side=10; } }; int main() { Cube c; cout << c. side; }
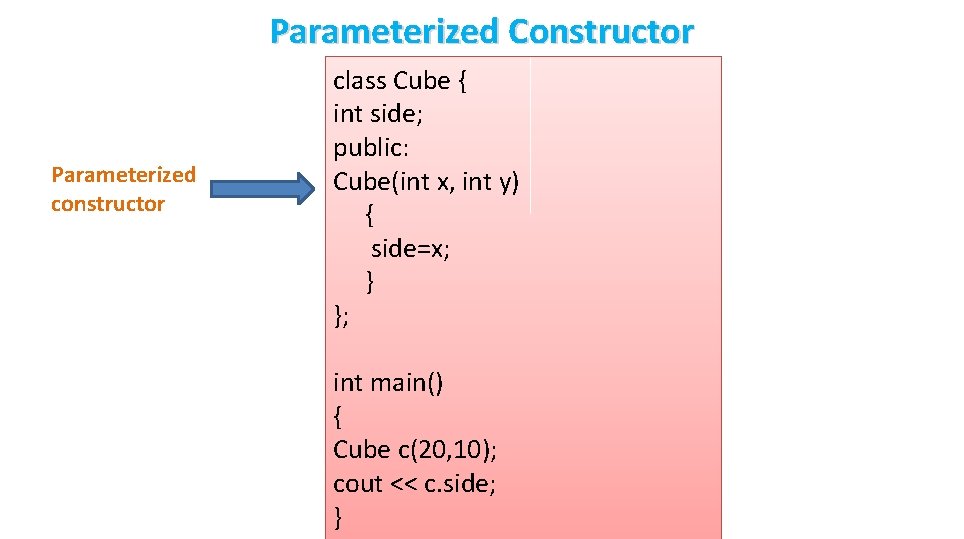
Parameterized Constructor Parameterized constructor class Cube { int side; public: Cube(int x, int y) { side=x; } }; int main() { Cube c(20, 10); cout << c. side; }
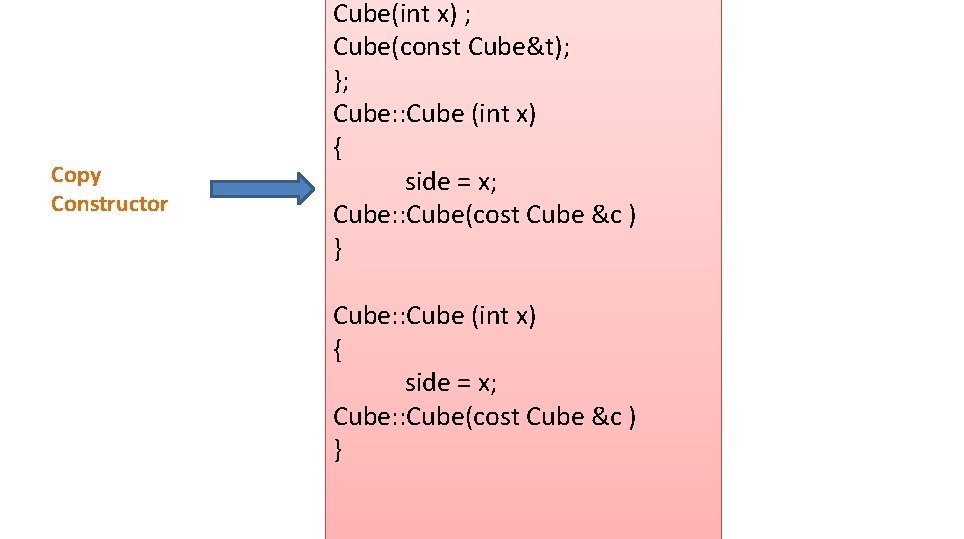
Copy Constructor Cube(int x) ; Copy Constructor Cube(const Cube&t); }; Cube: : Cube (int x) { side = x; Cube: : Cube(cost Cube &c ) }
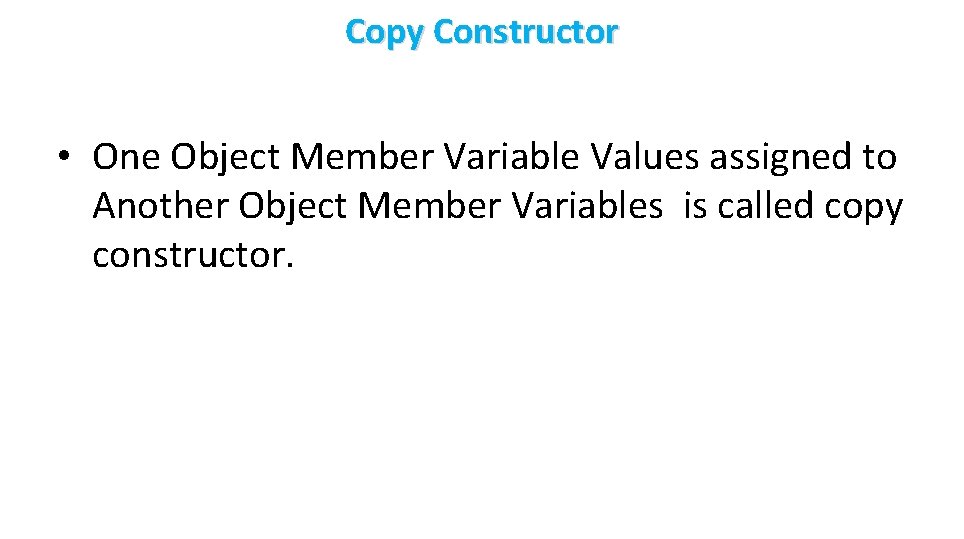
Copy Constructor • One Object Member Variable Values assigned to Another Object Member Variables is called copy constructor.
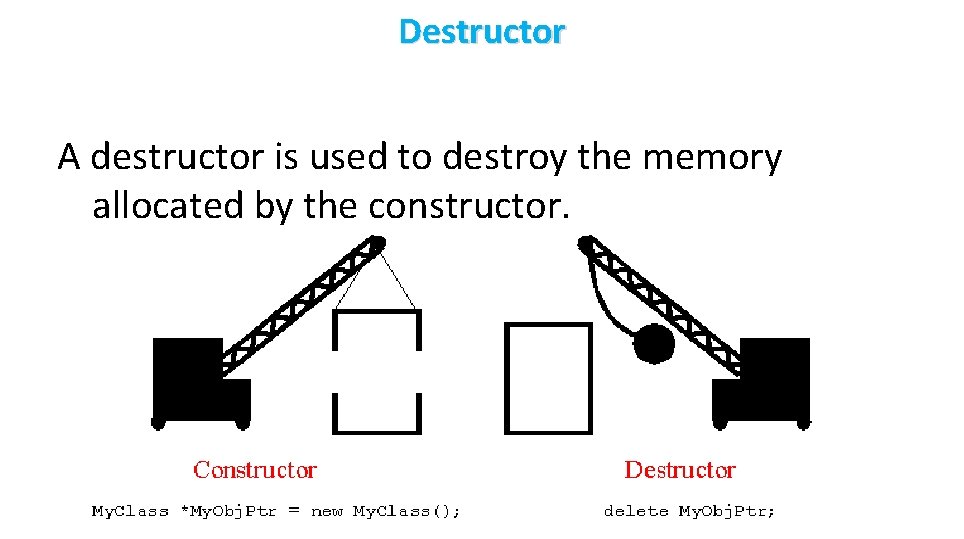
Destructor A destructor is used to destroy the memory allocated by the constructor.
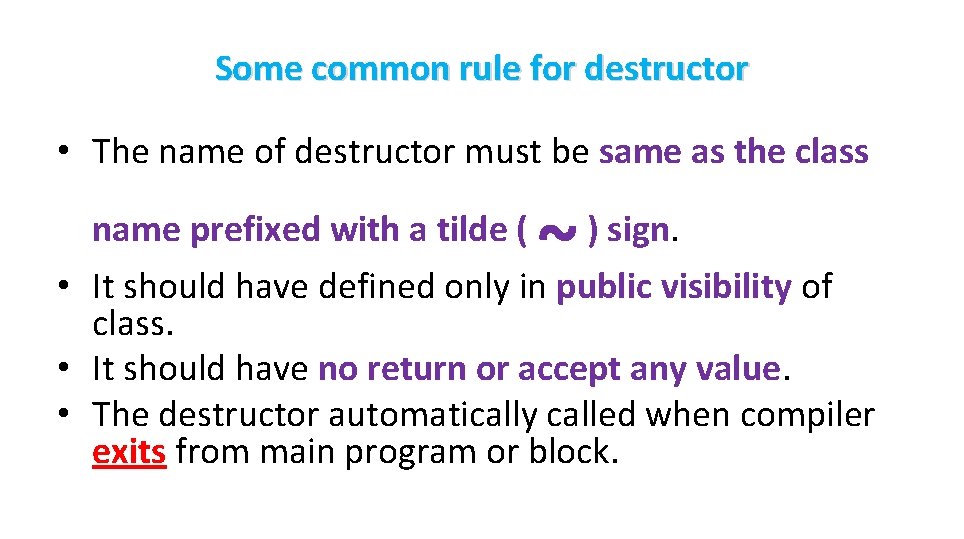
Some common rule for destructor • The name of destructor must be same as the class ~ • It should have defined only in public visibility of name prefixed with a tilde ( ) sign. class. • It should have no return or accept any value. • The destructor automatically called when compiler exits from main program or block.
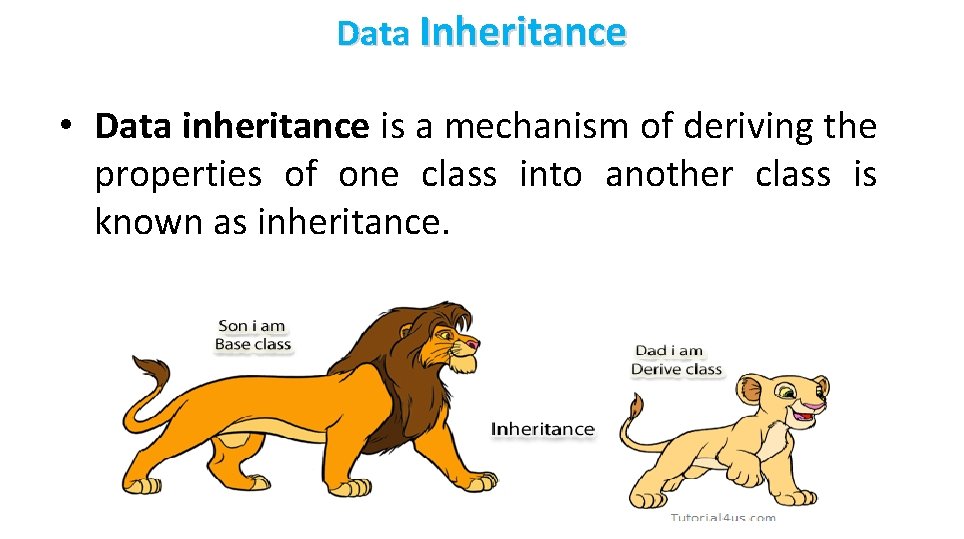
Data Inheritance • Data inheritance is a mechanism of deriving the properties of one class into another class is known as inheritance.
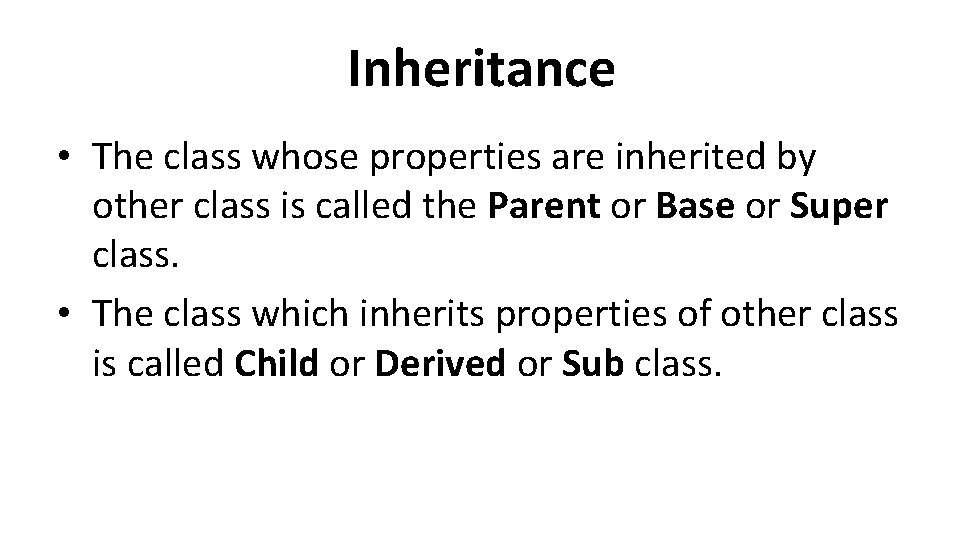
Inheritance • The class whose properties are inherited by other class is called the Parent or Base or Super class. • The class which inherits properties of other class is called Child or Derived or Sub class.
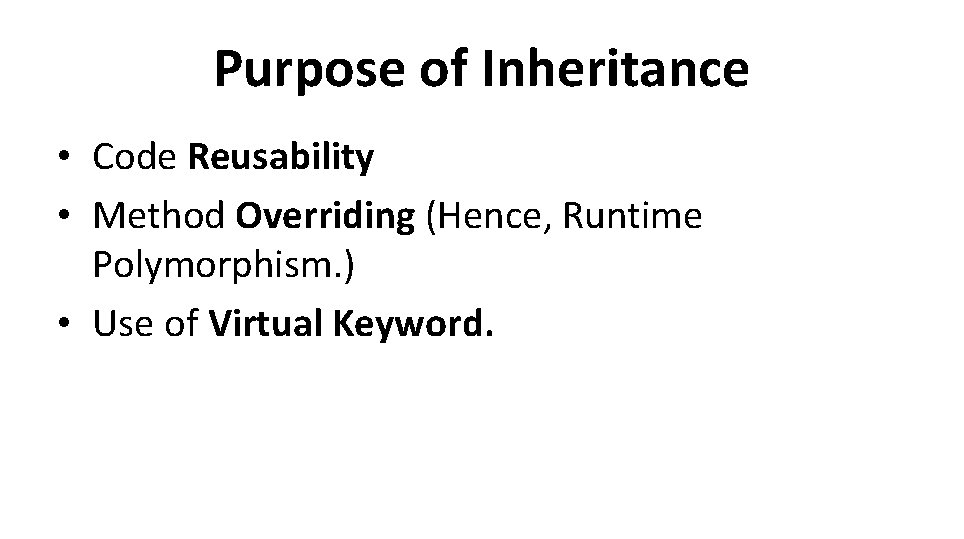
Purpose of Inheritance • Code Reusability • Method Overriding (Hence, Runtime Polymorphism. ) • Use of Virtual Keyword.
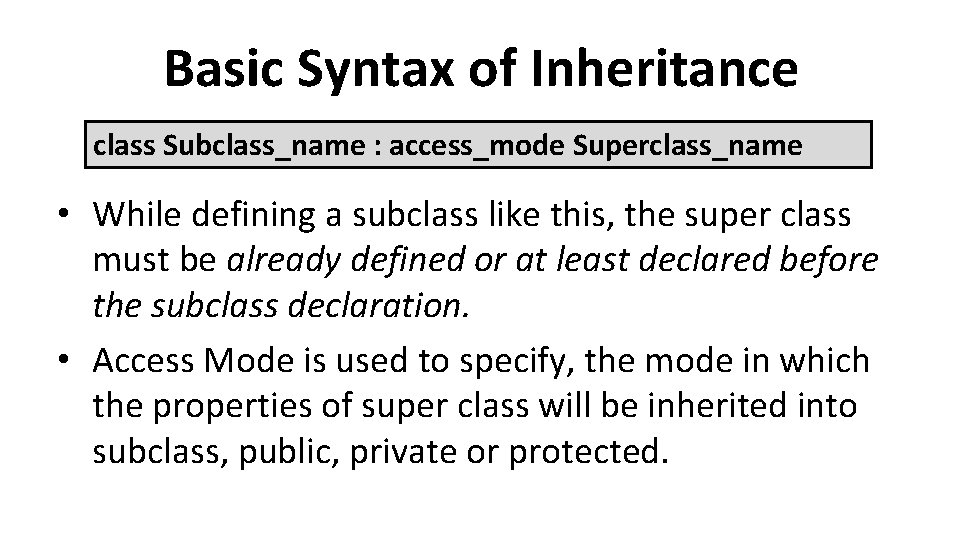
Basic Syntax of Inheritance class Subclass_name : access_mode Superclass_name • While defining a subclass like this, the super class must be already defined or at least declared before the subclass declaration. • Access Mode is used to specify, the mode in which the properties of super class will be inherited into subclass, public, private or protected.
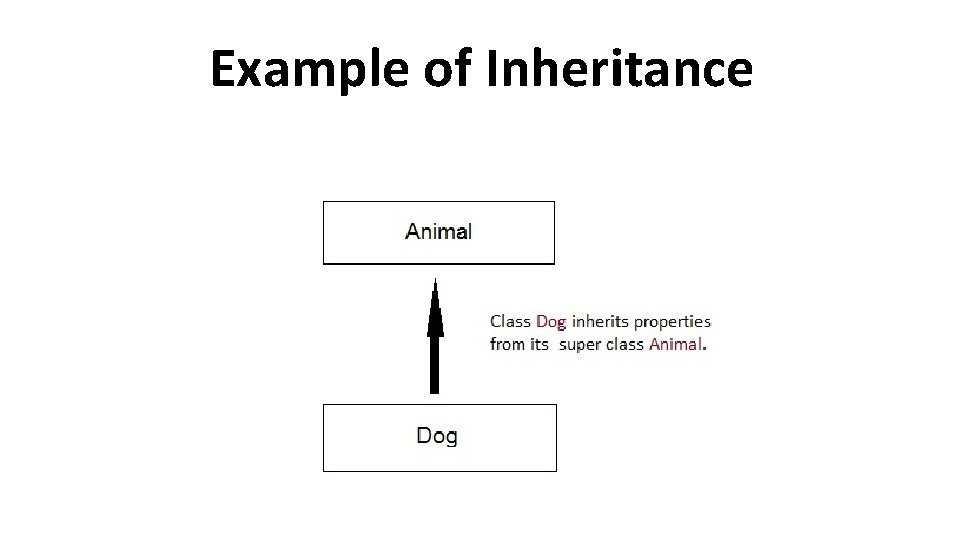
Example of Inheritance
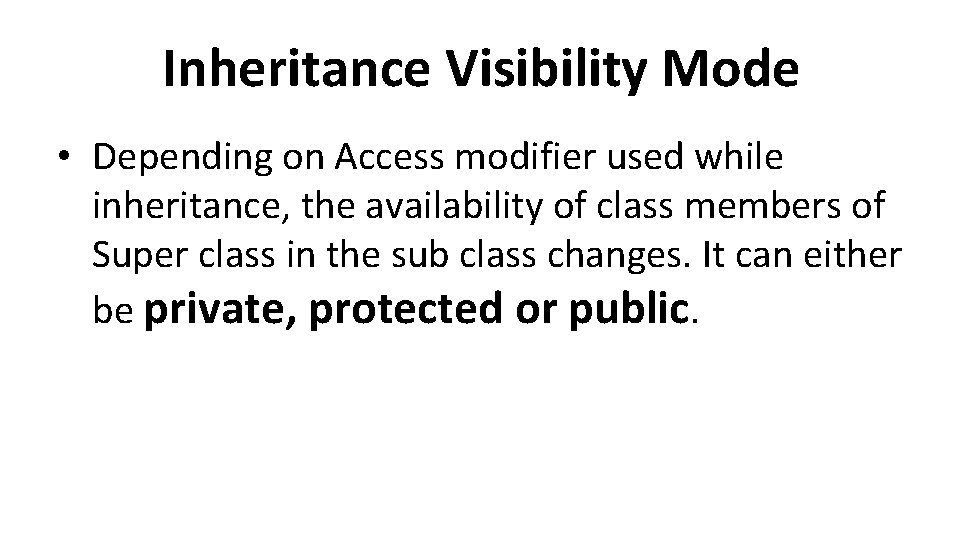
Inheritance Visibility Mode • Depending on Access modifier used while inheritance, the availability of class members of Super class in the sub class changes. It can either be private, protected or public.
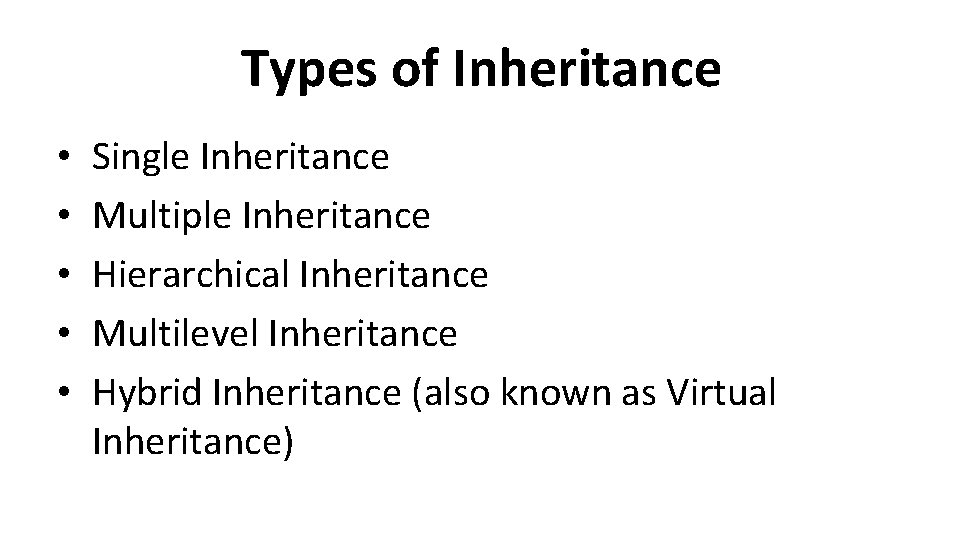
Types of Inheritance • • • Single Inheritance Multiple Inheritance Hierarchical Inheritance Multilevel Inheritance Hybrid Inheritance (also known as Virtual Inheritance)
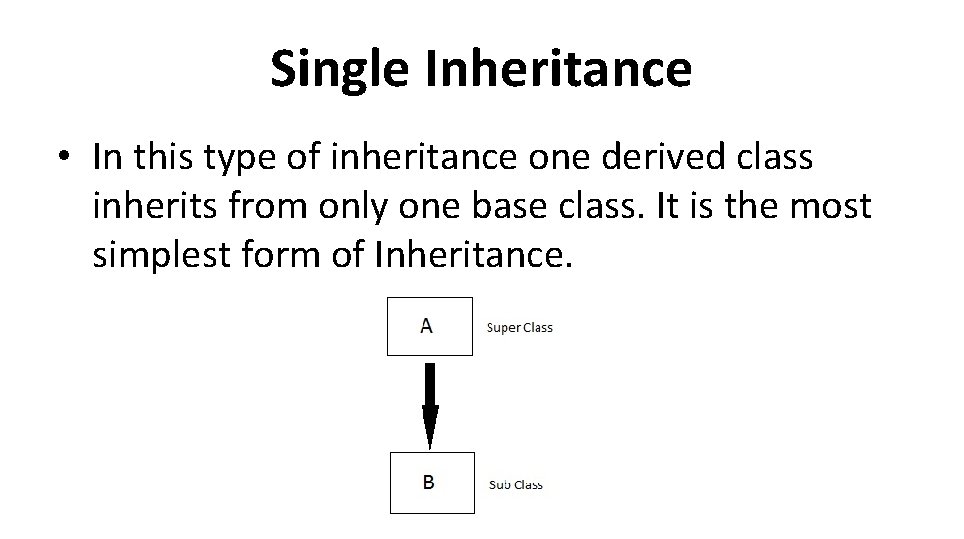
Single Inheritance • In this type of inheritance one derived class inherits from only one base class. It is the most simplest form of Inheritance.
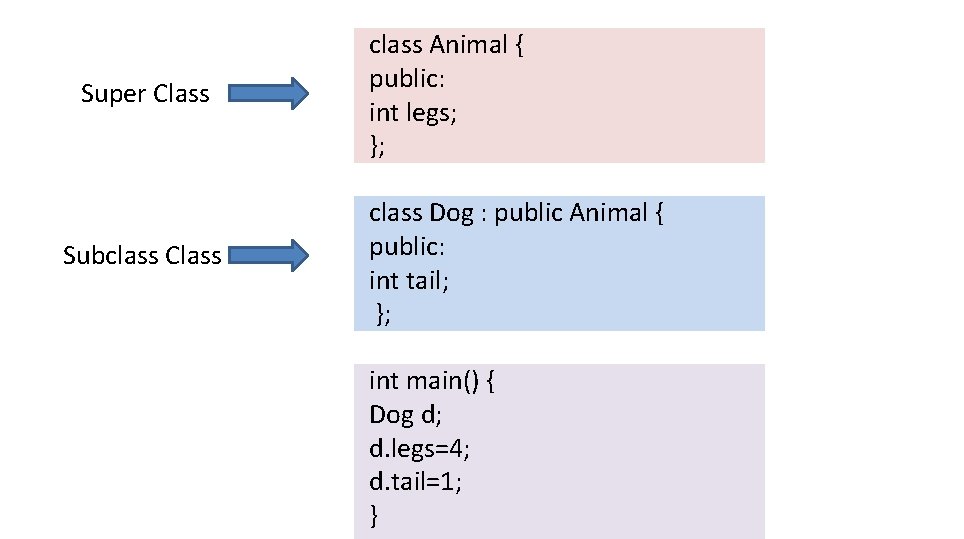
Super Class Subclass Class class Animal { public: int legs; }; class Dog : public Animal { public: int tail; }; int main() { Dog d; d. legs=4; d. tail=1; }
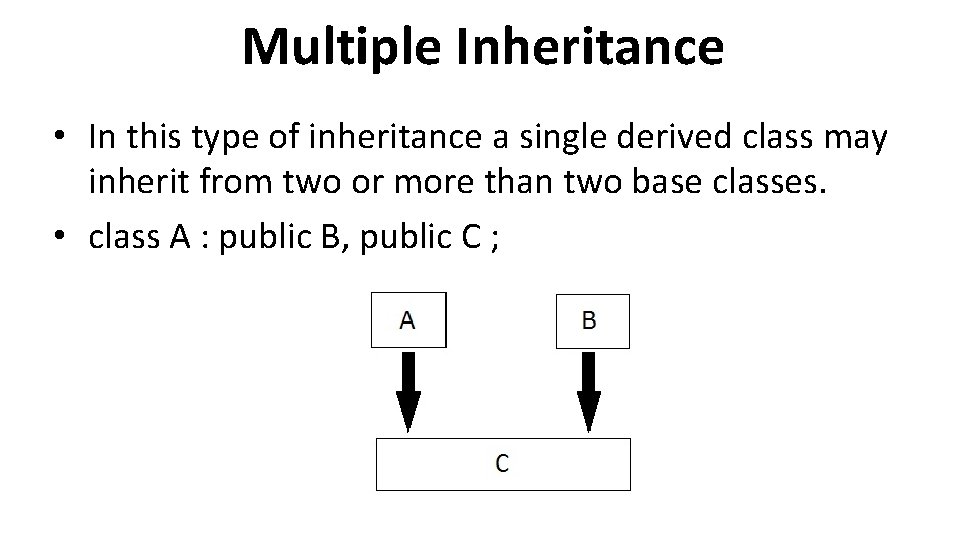
Multiple Inheritance • In this type of inheritance a single derived class may inherit from two or more than two base classes. • class A : public B, public C ;
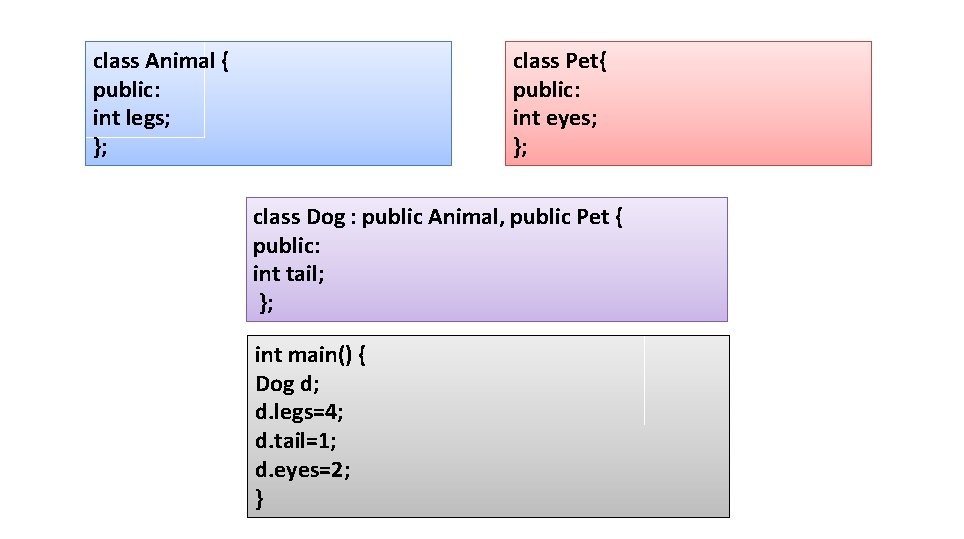
class Animal { public: int legs; }; class Pet{ public: int eyes; }; class Dog : public Animal, public Pet { public: int tail; }; int main() { Dog d; d. legs=4; d. tail=1; d. eyes=2; }
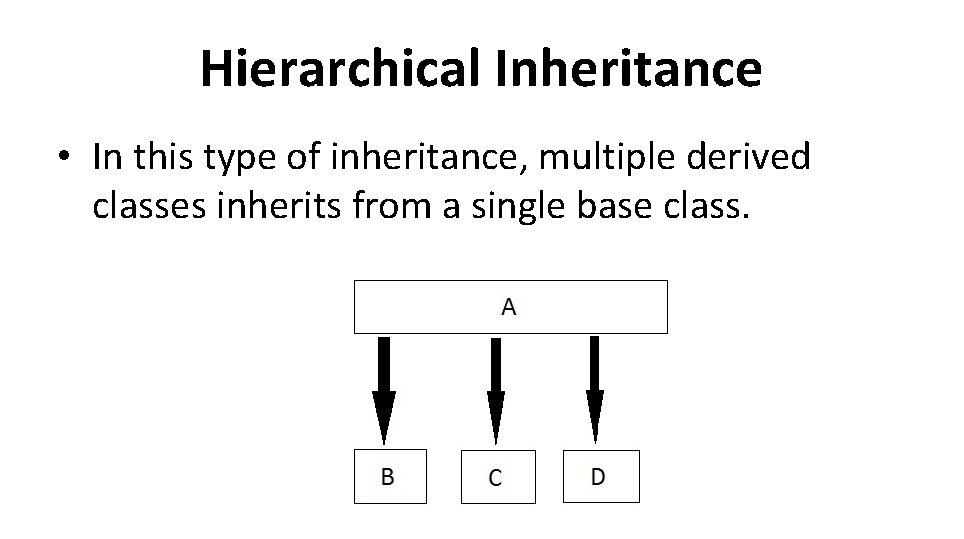
Hierarchical Inheritance • In this type of inheritance, multiple derived classes inherits from a single base class.
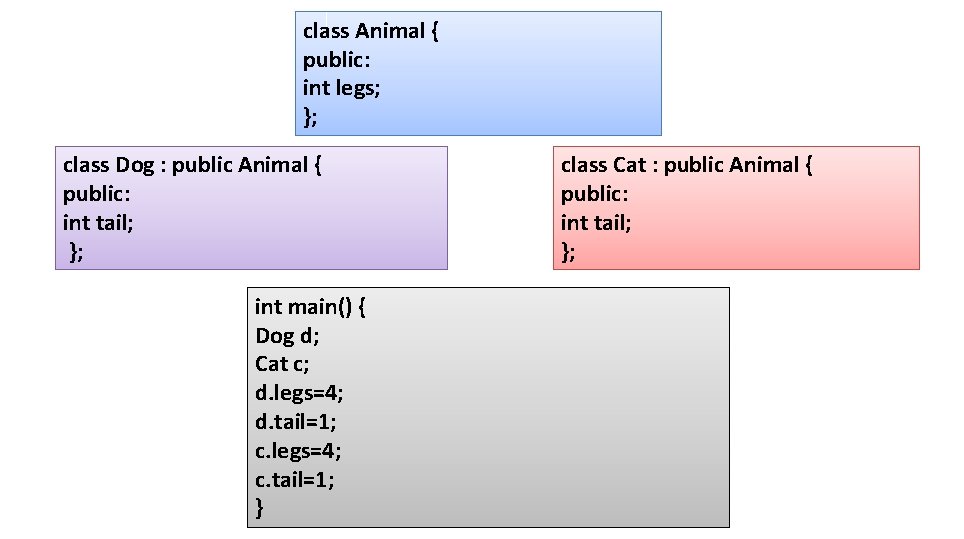
class Animal { public: int legs; }; class Dog : public Animal { public: int tail; }; int main() { Dog d; Cat c; d. legs=4; d. tail=1; c. legs=4; c. tail=1; } class Cat : public Animal { public: int tail; };
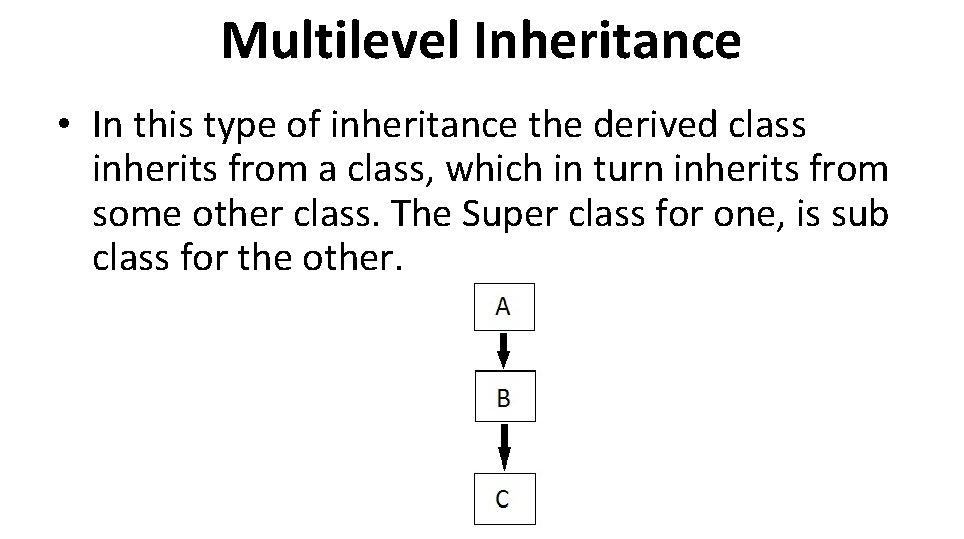
Multilevel Inheritance • In this type of inheritance the derived class inherits from a class, which in turn inherits from some other class. The Super class for one, is sub class for the other.
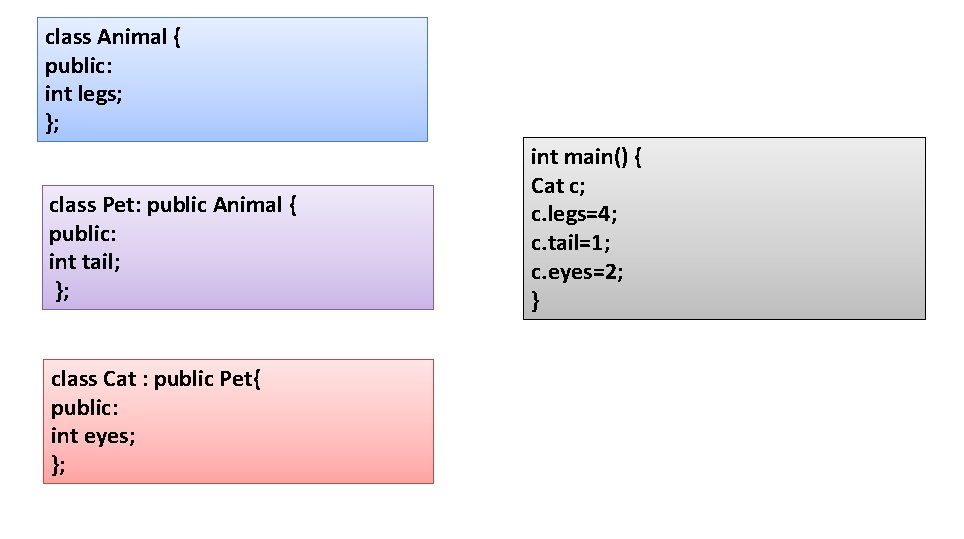
class Animal { public: int legs; }; class Pet: public Animal { public: int tail; }; class Cat : public Pet{ public: int eyes; }; int main() { Cat c; c. legs=4; c. tail=1; c. eyes=2; }
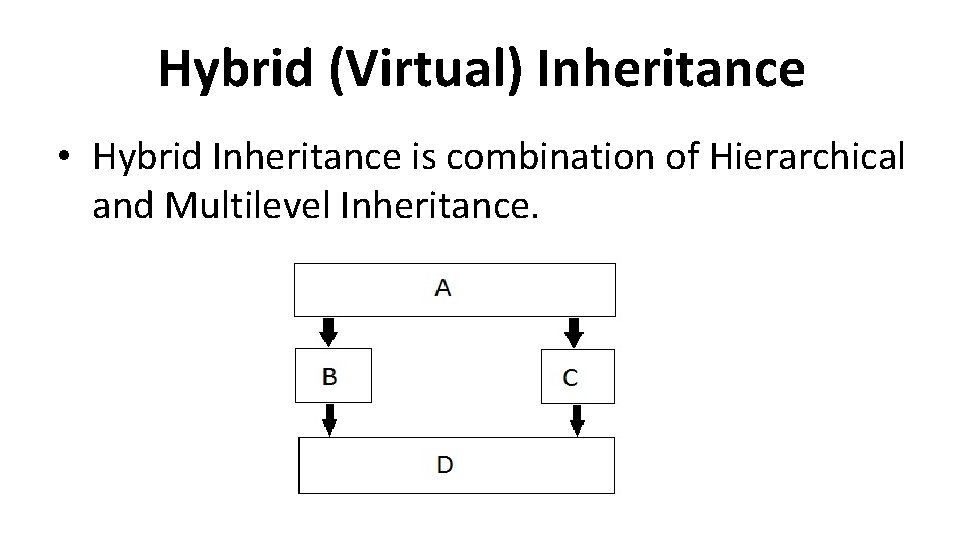
Hybrid (Virtual) Inheritance • Hybrid Inheritance is combination of Hierarchical and Multilevel Inheritance.
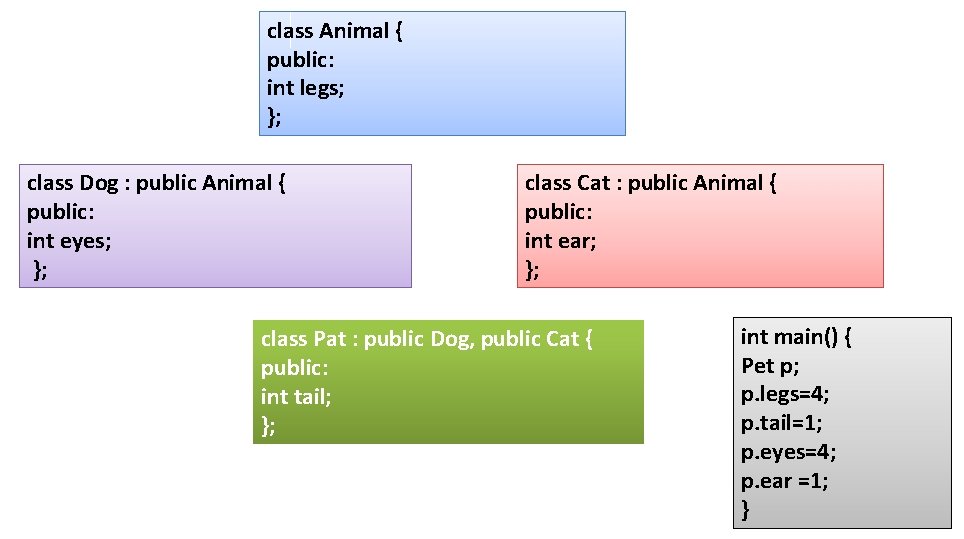
class Animal { public: int legs; }; class Dog : public Animal { public: int eyes; }; class Cat : public Animal { public: int ear; }; class Pat : public Dog, public Cat { public: int tail; }; int main() { Pet p; p. legs=4; p. tail=1; p. eyes=4; p. ear =1; }
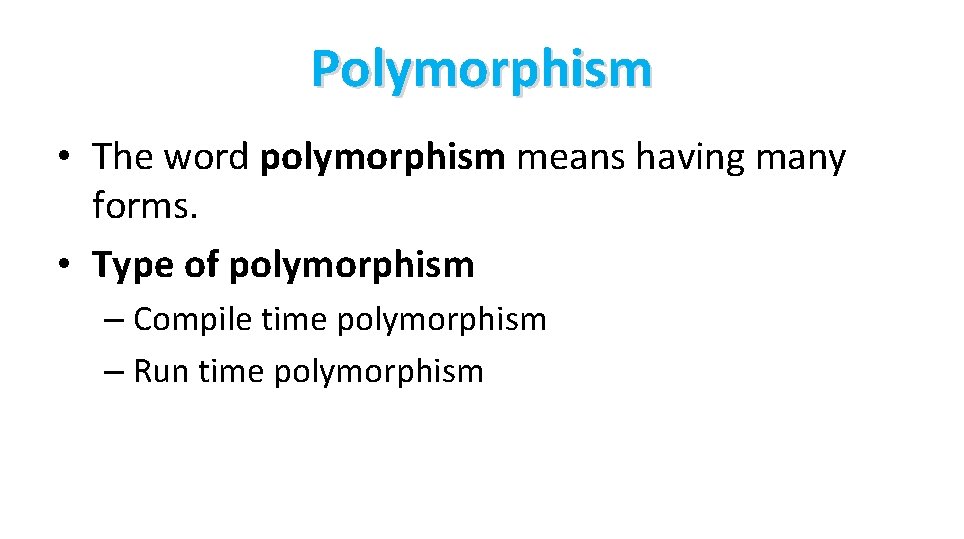
Polymorphism • The word polymorphism means having many forms. • Type of polymorphism – Compile time polymorphism – Run time polymorphism
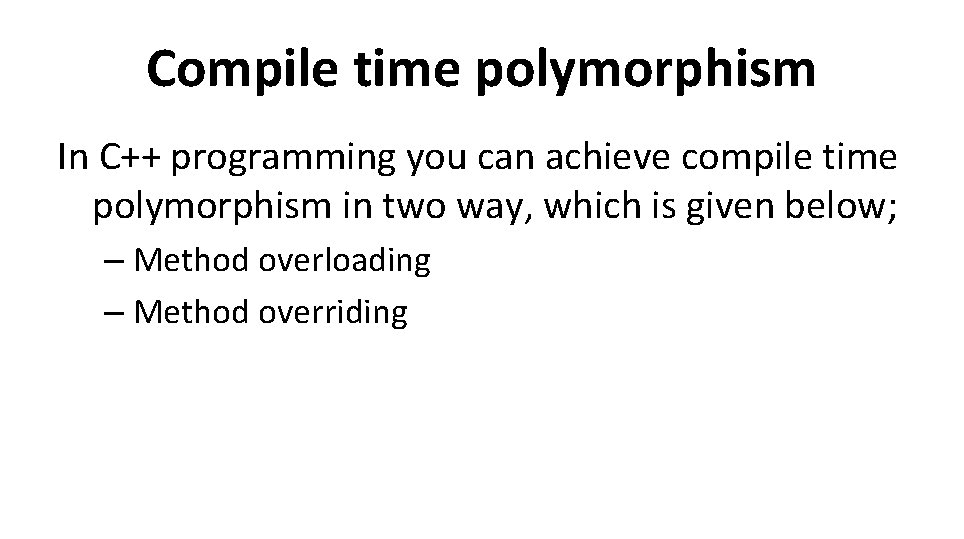
Compile time polymorphism In C++ programming you can achieve compile time polymorphism in two way, which is given below; – Method overloading – Method overriding
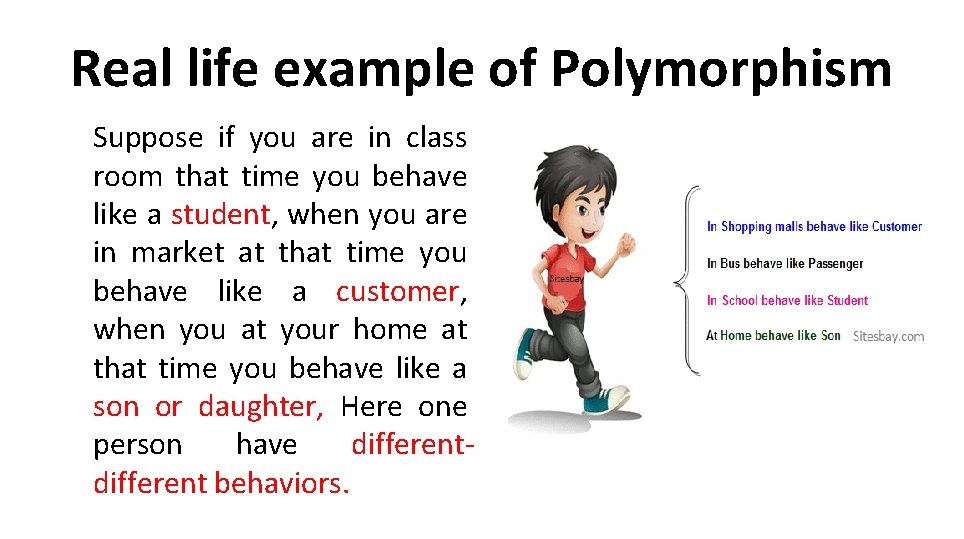
Real life example of Polymorphism Suppose if you are in class room that time you behave like a student, when you are in market at that time you behave like a customer, when you at your home at that time you behave like a son or daughter, Here one person have different behaviors.
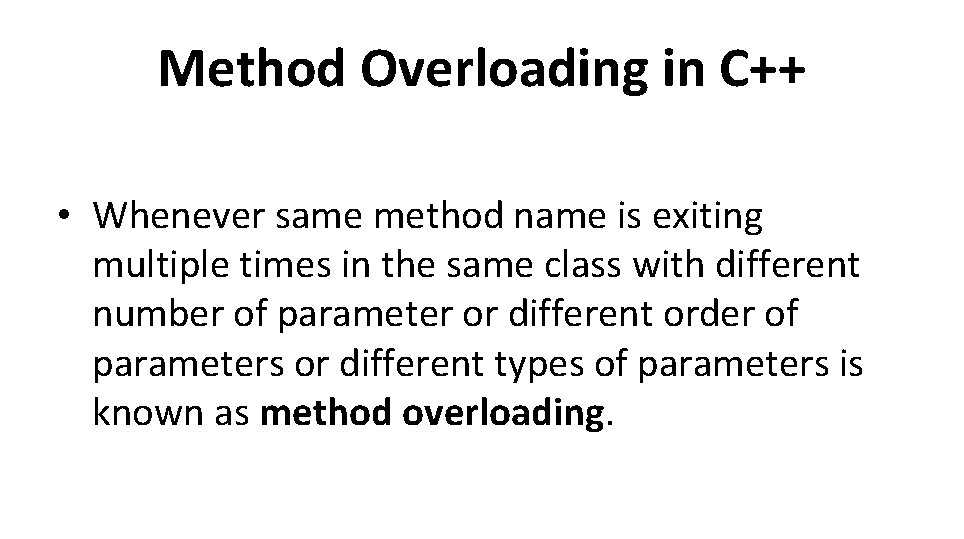
Method Overloading in C++ • Whenever same method name is exiting multiple times in the same class with different number of parameter or different order of parameters or different types of parameters is known as method overloading.
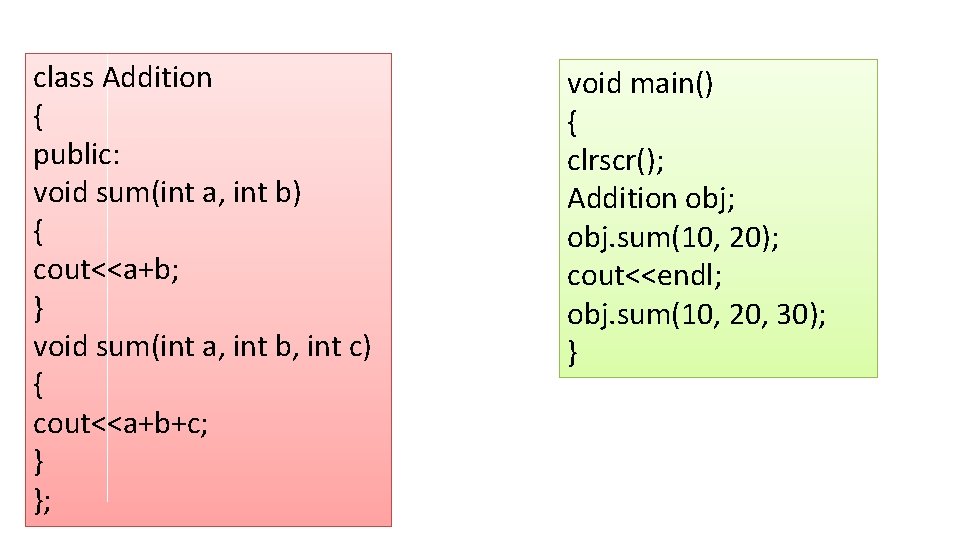
class Addition { public: void sum(int a, int b) { cout<<a+b; } void sum(int a, int b, int c) { cout<<a+b+c; } }; void main() { clrscr(); Addition obj; obj. sum(10, 20); cout<<endl; obj. sum(10, 20, 30); }
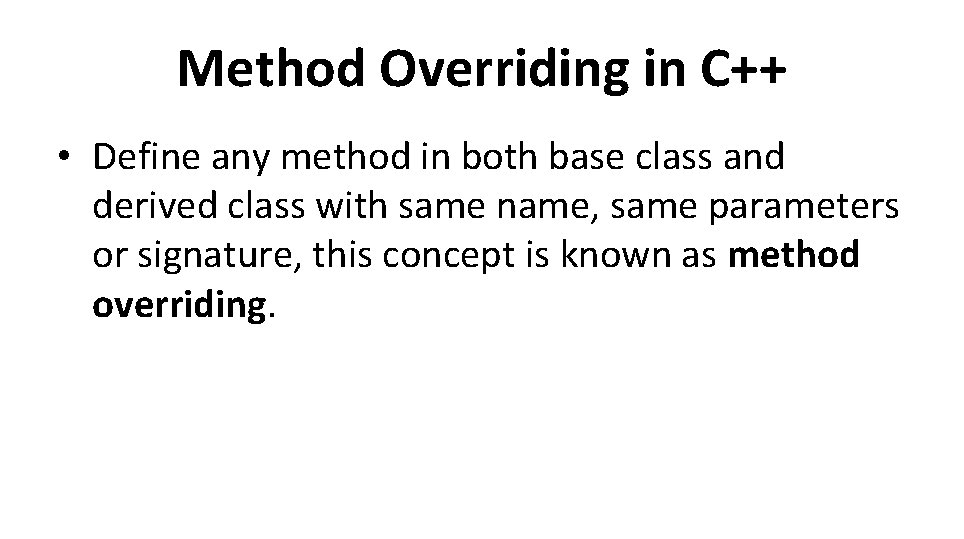
Method Overriding in C++ • Define any method in both base class and derived class with same name, same parameters or signature, this concept is known as method overriding.
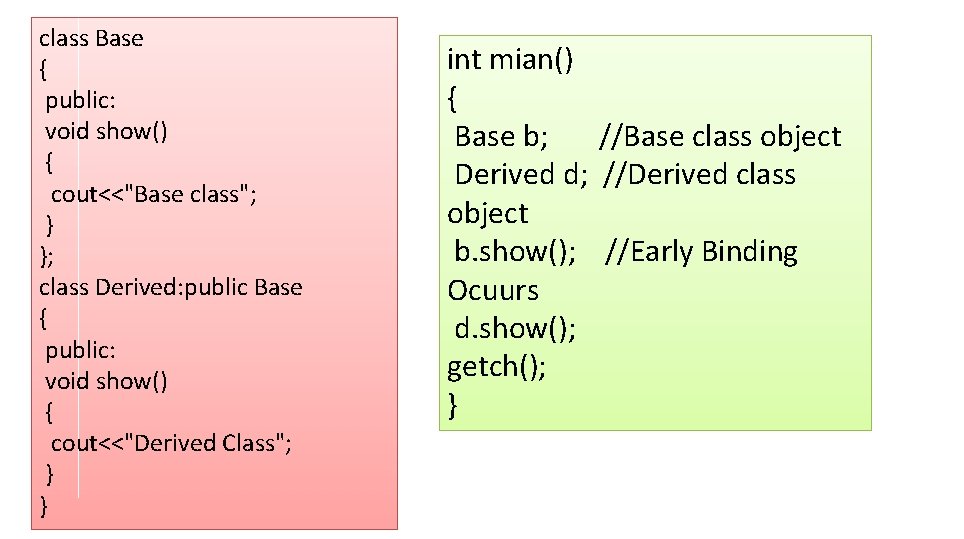
class Base { public: void show() { cout<<"Base class"; } }; class Derived: public Base { public: void show() { cout<<"Derived Class"; } } int mian() { Base b; //Base class object Derived d; //Derived class object b. show(); //Early Binding Ocuurs d. show(); getch(); }
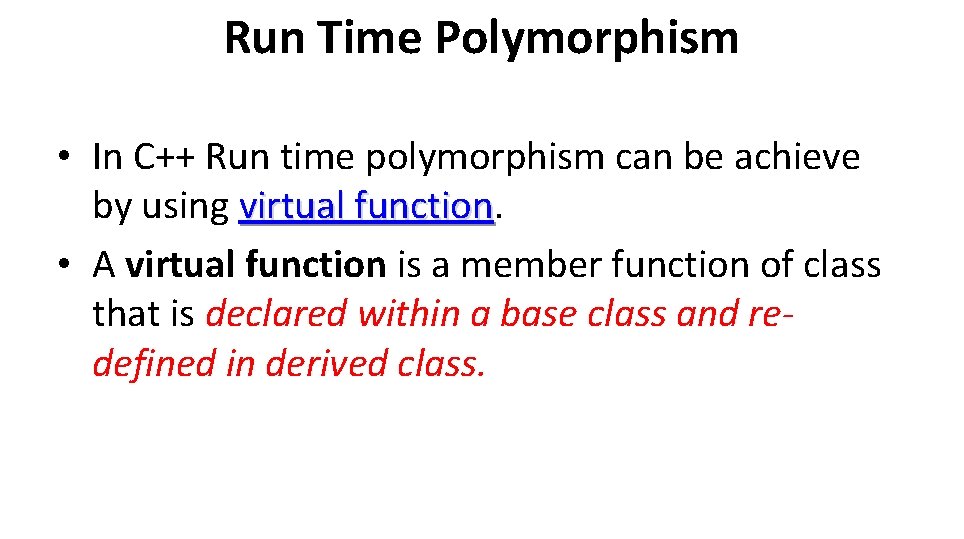
Run Time Polymorphism • In C++ Run time polymorphism can be achieve by using virtual function • A virtual function is a member function of class that is declared within a base class and redefined in derived class.
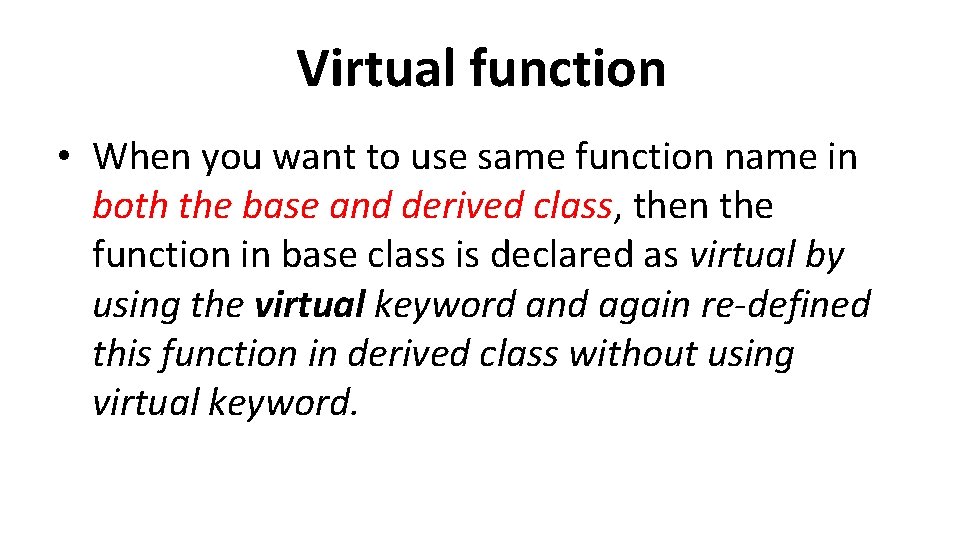
Virtual function • When you want to use same function name in both the base and derived class, then the function in base class is declared as virtual by using the virtual keyword and again re-defined this function in derived class without using virtual keyword.
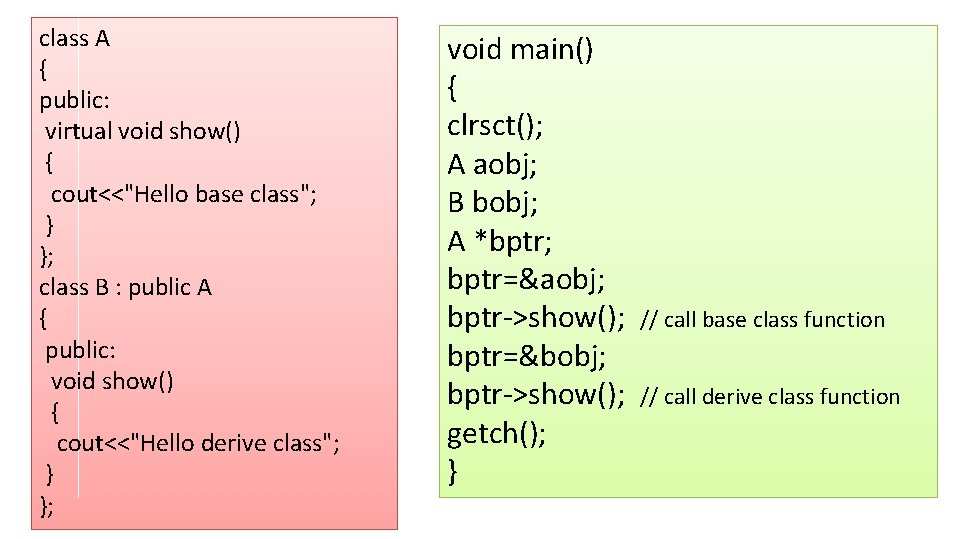
class A { public: virtual void show() { cout<<"Hello base class"; } }; class B : public A { public: void show() { cout<<"Hello derive class"; } }; void main() { clrsct(); A aobj; B bobj; A *bptr; bptr=&aobj; bptr->show(); bptr=&bobj; bptr->show(); getch(); } // call base class function // call derive class function
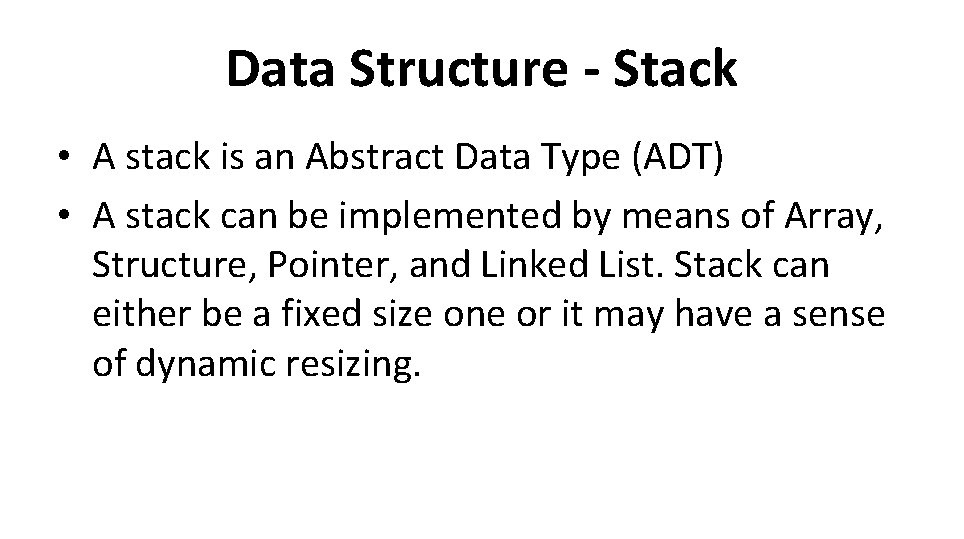
Data Structure - Stack • A stack is an Abstract Data Type (ADT) • A stack can be implemented by means of Array, Structure, Pointer, and Linked List. Stack can either be a fixed size one or it may have a sense of dynamic resizing.
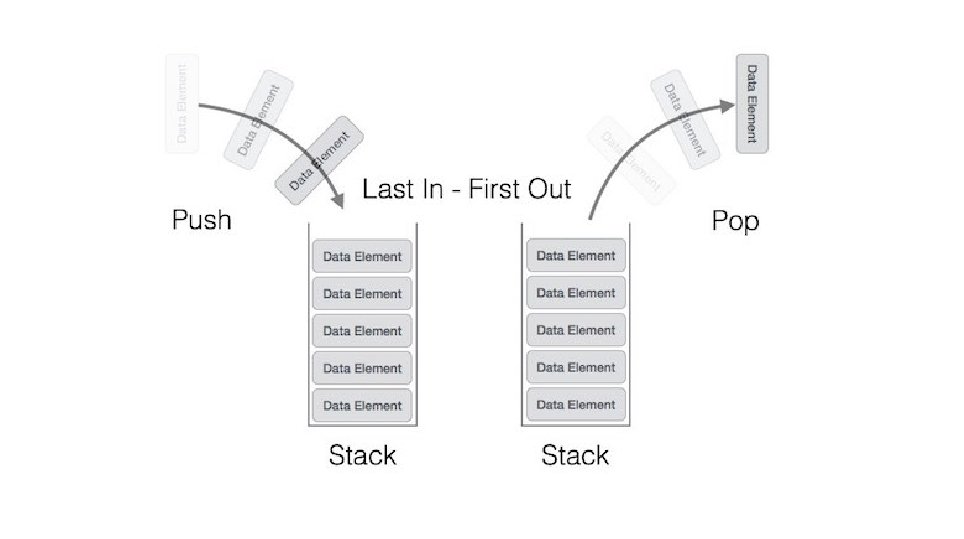
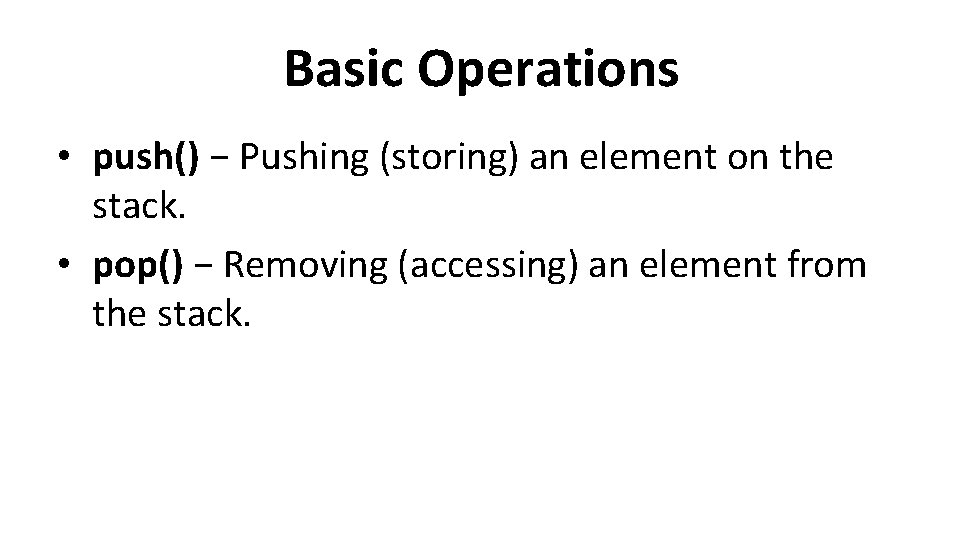
Basic Operations • push() − Pushing (storing) an element on the stack. • pop() − Removing (accessing) an element from the stack.
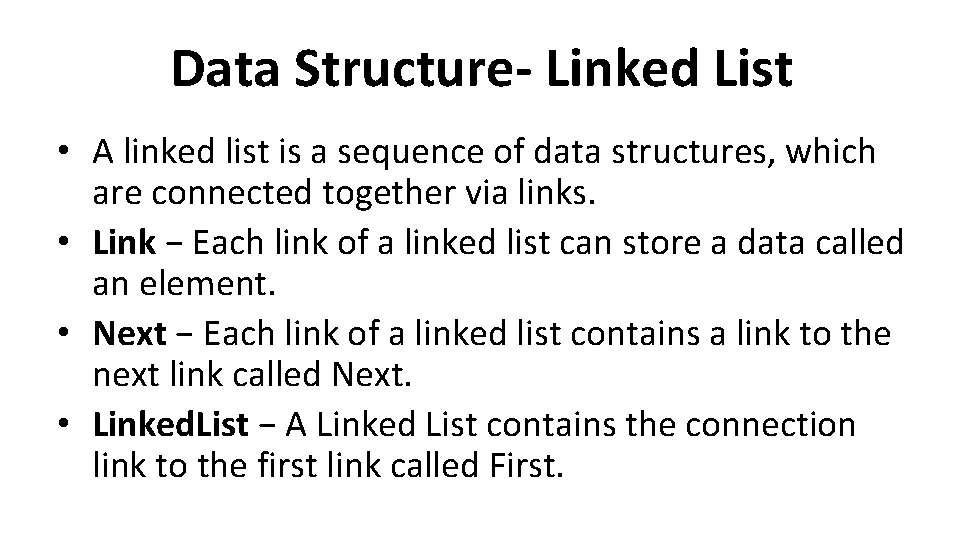
Data Structure- Linked List • A linked list is a sequence of data structures, which are connected together via links. • Link − Each link of a linked list can store a data called an element. • Next − Each link of a linked list contains a link to the next link called Next. • Linked. List − A Linked List contains the connection link to the first link called First.
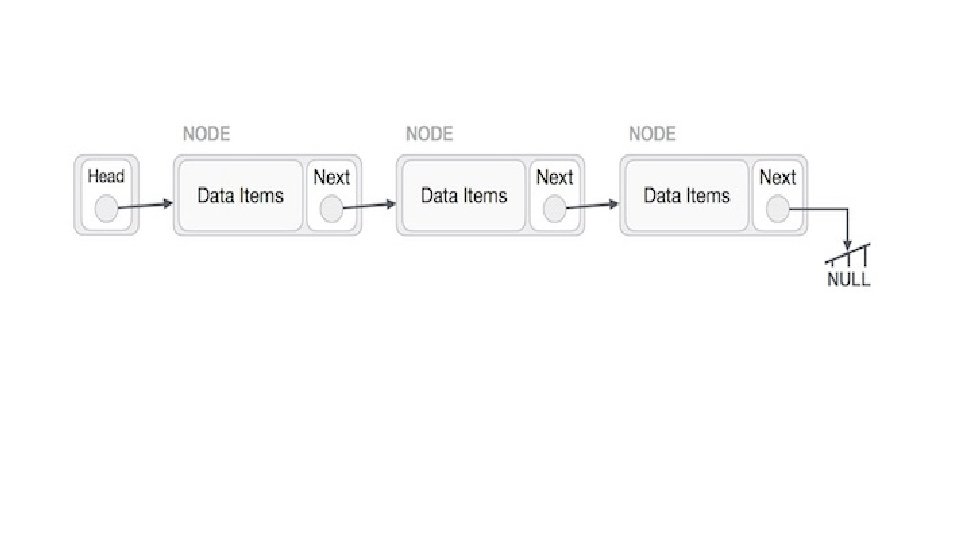