Unit 1 I Polymorphism Inheritance Lecture 3 Inheritance
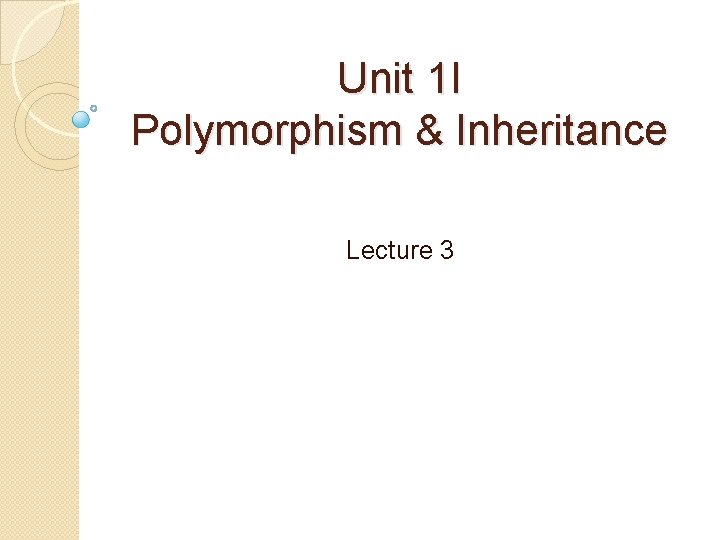
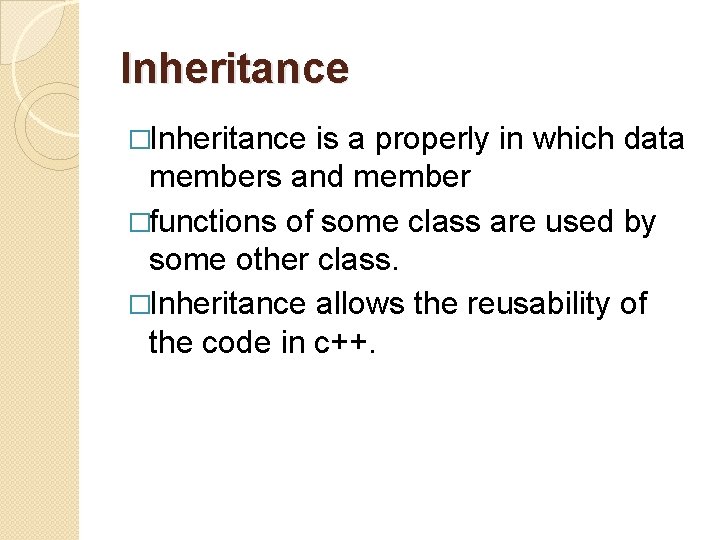
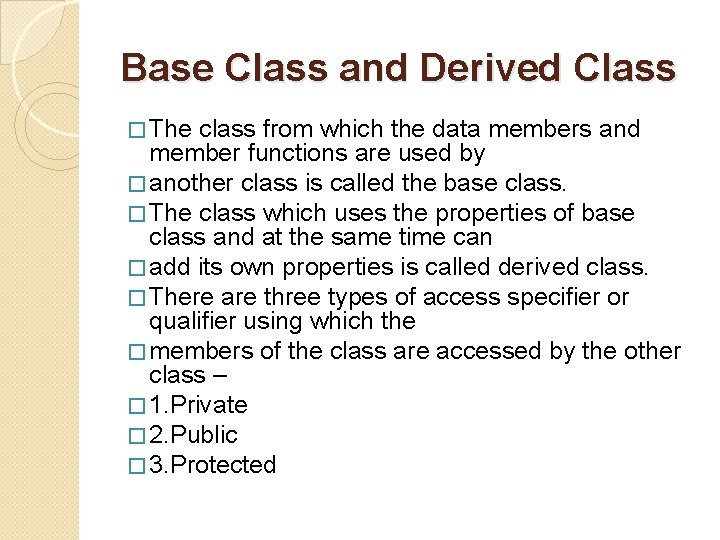
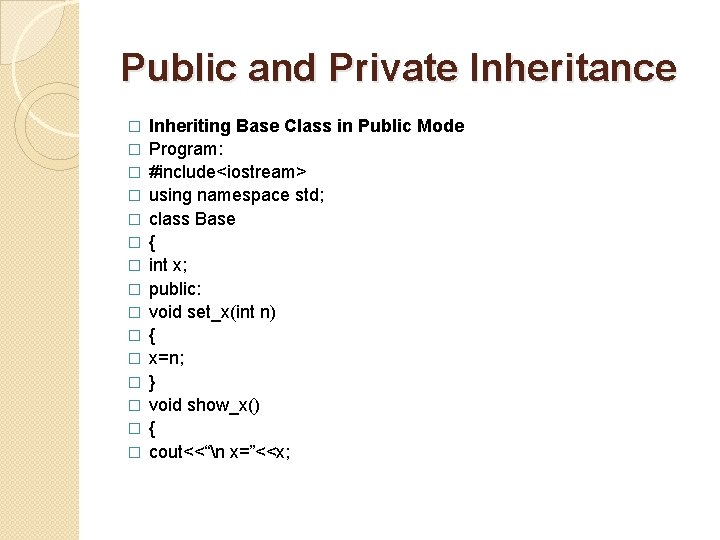
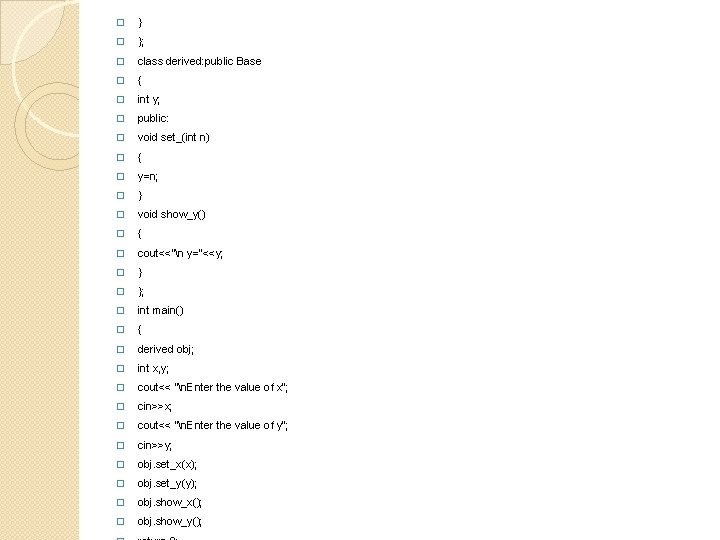
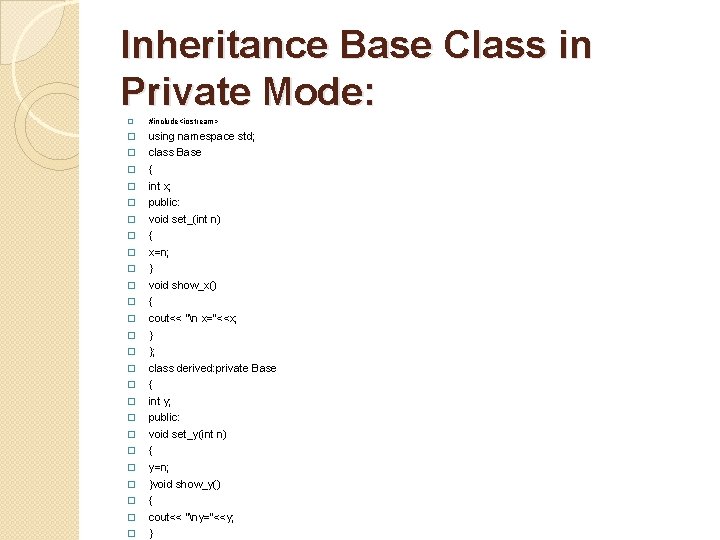
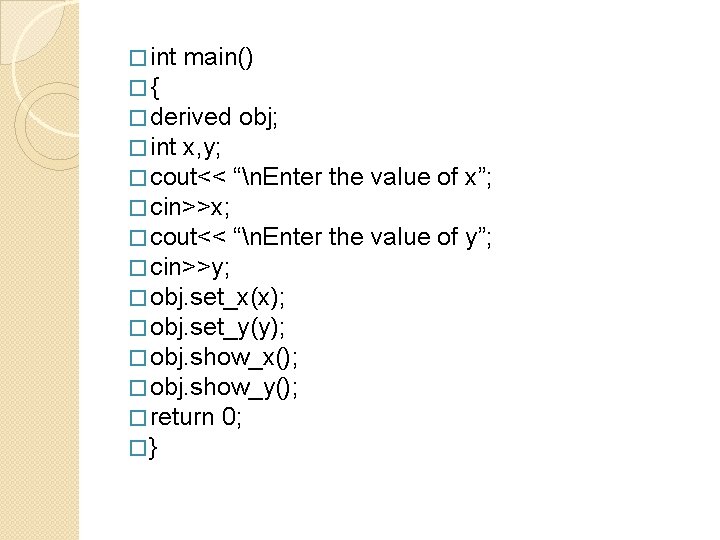
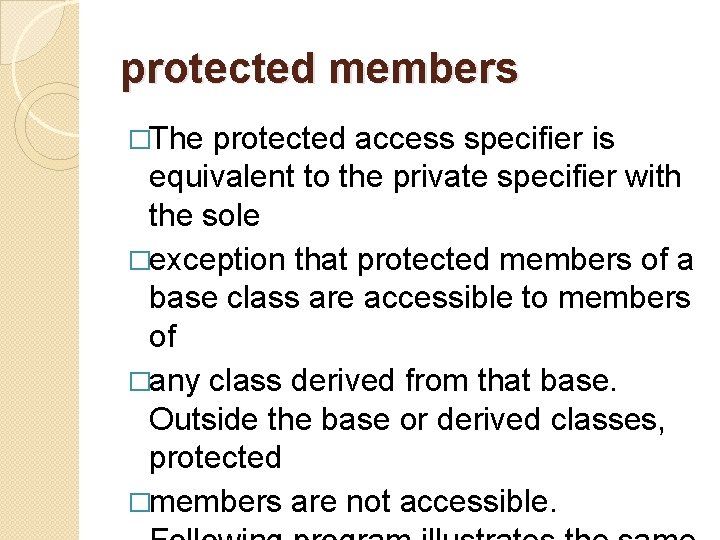
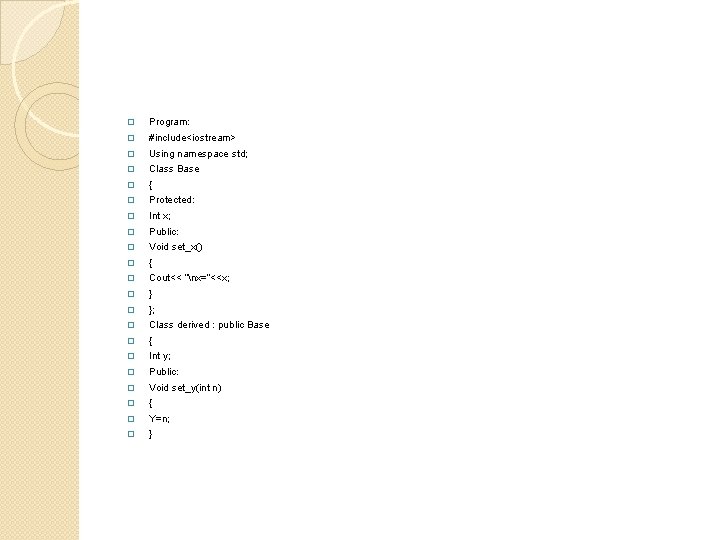
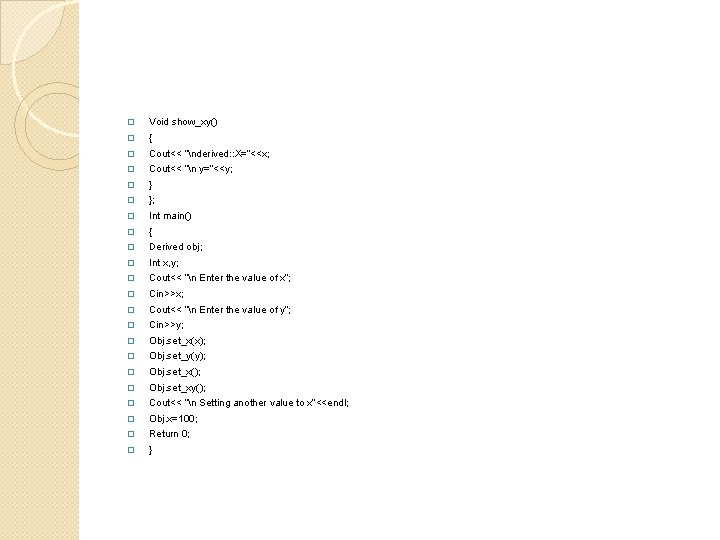
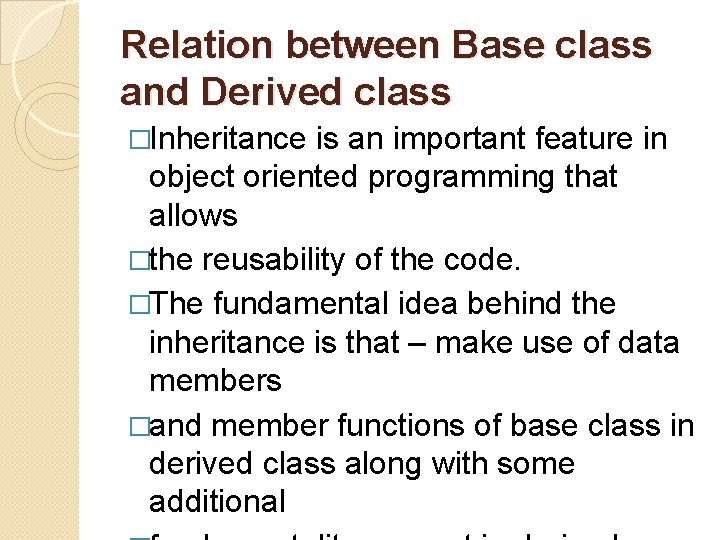
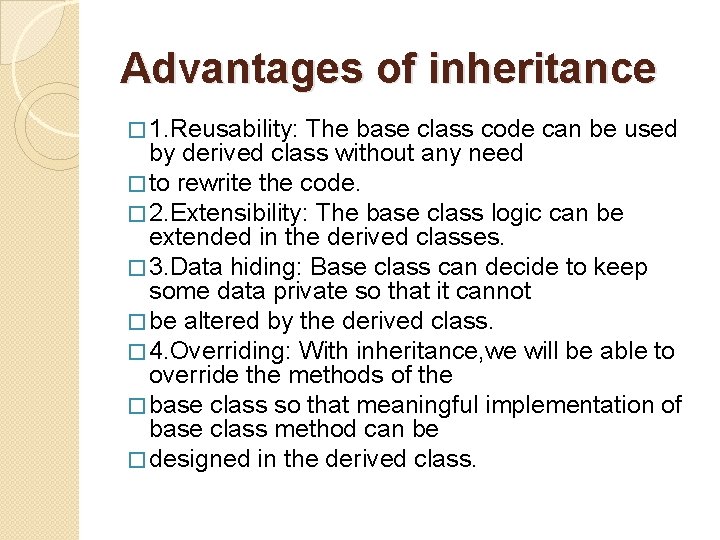
- Slides: 12
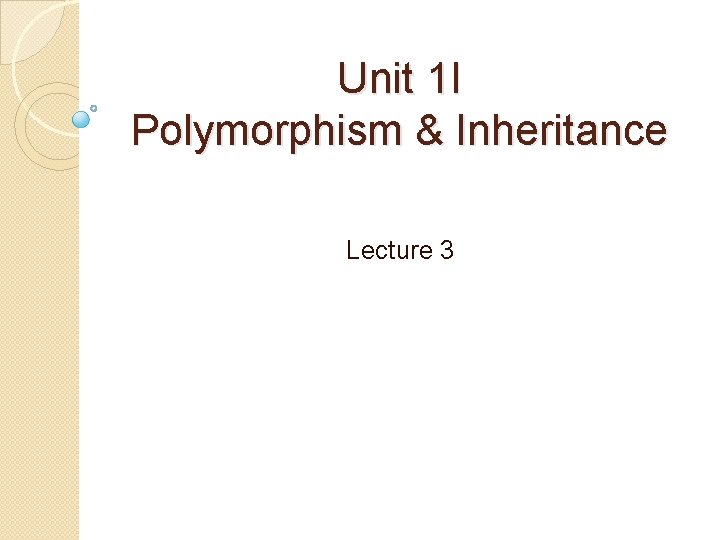
Unit 1 I Polymorphism & Inheritance Lecture 3
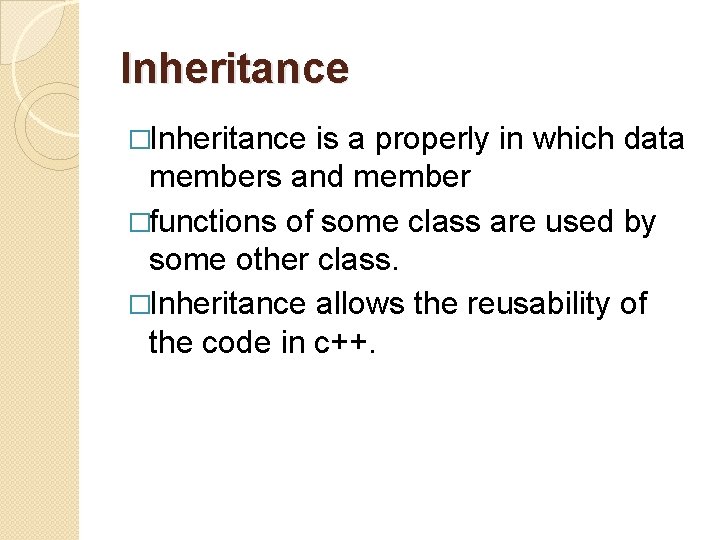
Inheritance �Inheritance is a properly in which data members and member �functions of some class are used by some other class. �Inheritance allows the reusability of the code in c++.
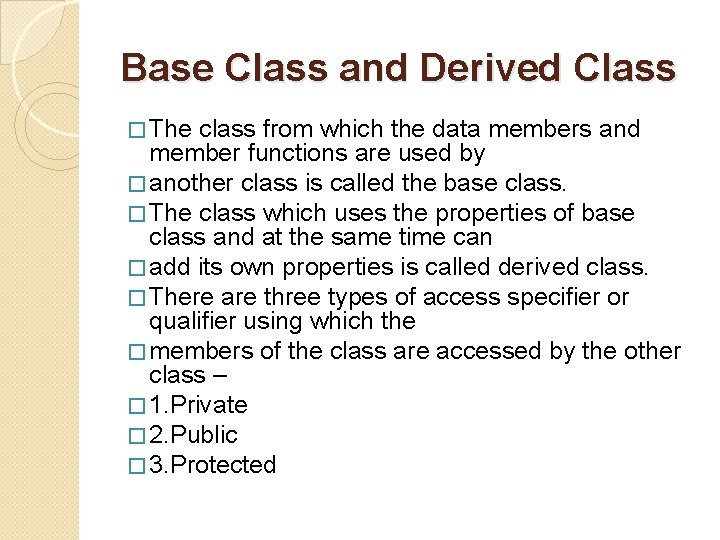
Base Class and Derived Class � The class from which the data members and member functions are used by � another class is called the base class. � The class which uses the properties of base class and at the same time can � add its own properties is called derived class. � There are three types of access specifier or qualifier using which the � members of the class are accessed by the other class – � 1. Private � 2. Public � 3. Protected
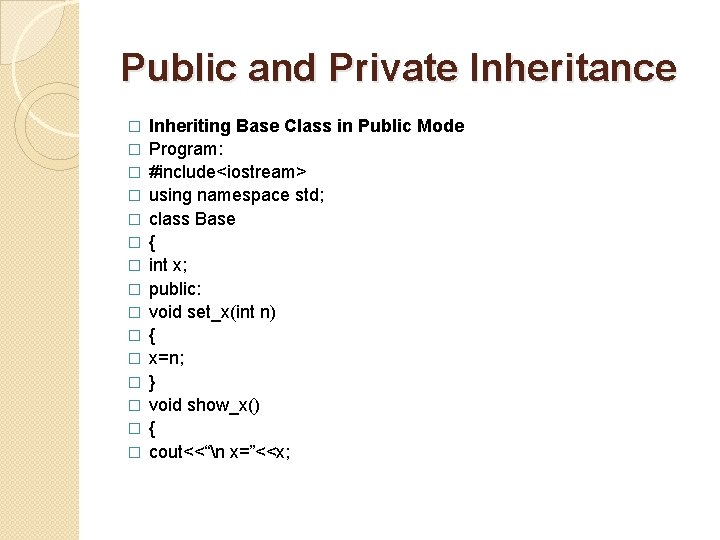
Public and Private Inheritance � � � � Inheriting Base Class in Public Mode Program: #include<iostream> using namespace std; class Base { int x; public: void set_x(int n) { x=n; } void show_x() { cout<<“n x=”<<x;
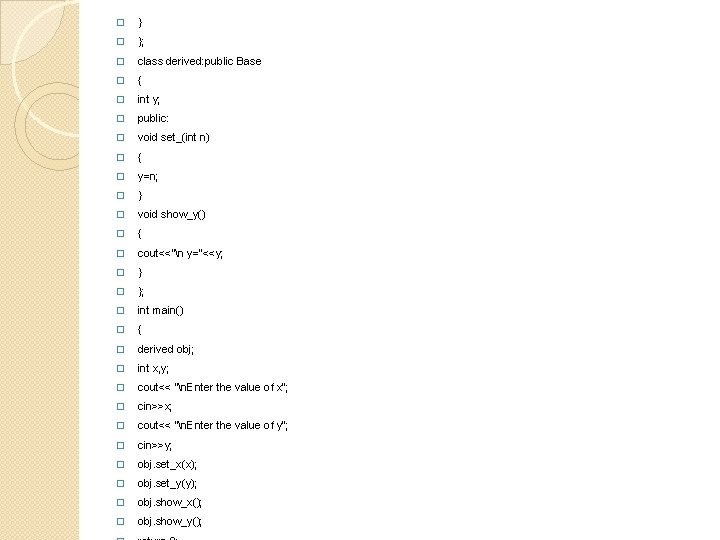
� } � }; � class derived: public Base � { � int y; � public: � void set_(int n) � { � y=n; � } � void show_y() � { � cout<<“n y=”<<y; � }; � int main() � { � derived obj; � int x, y; � cout<< “n. Enter the value of x”; � cin>>x; � cout<< “n. Enter the value of y”; � cin>>y; � obj. set_x(x); � obj. set_y(y); � obj. show_x(); � obj. show_y();
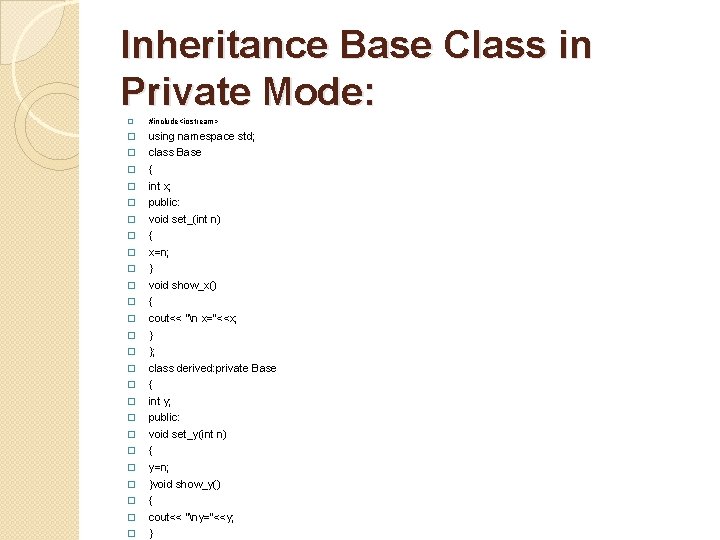
Inheritance Base Class in Private Mode: � #include<iostream> � using namespace std; � class Base � { � int x; � public: � void set_(int n) � { � x=n; � } � void show_x() � { � cout<< “n x=”<<x; � }; � class derived: private Base � { � int y; � public: � void set_y(int n) � { � y=n; � }void show_y() � { � cout<< “ny=”<<y; � }
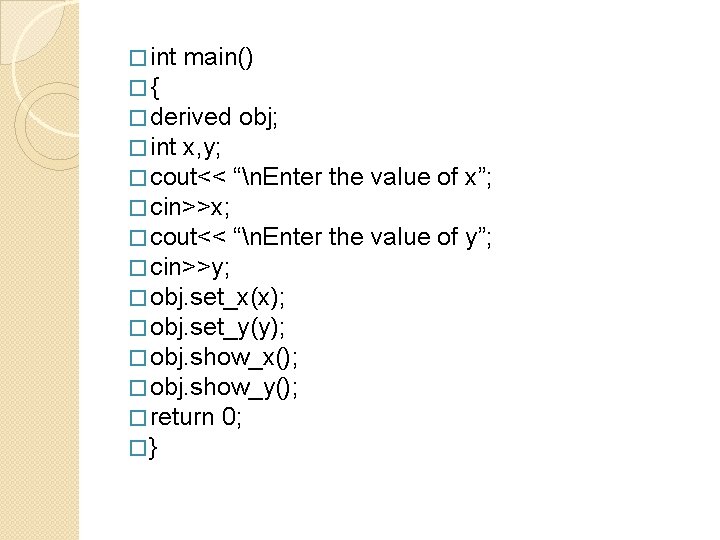
� int main() �{ � derived obj; � int x, y; � cout<< “n. Enter � cin>>x; � cout<< “n. Enter � cin>>y; � obj. set_x(x); � obj. set_y(y); � obj. show_x(); � obj. show_y(); � return 0; �} the value of x”; the value of y”;
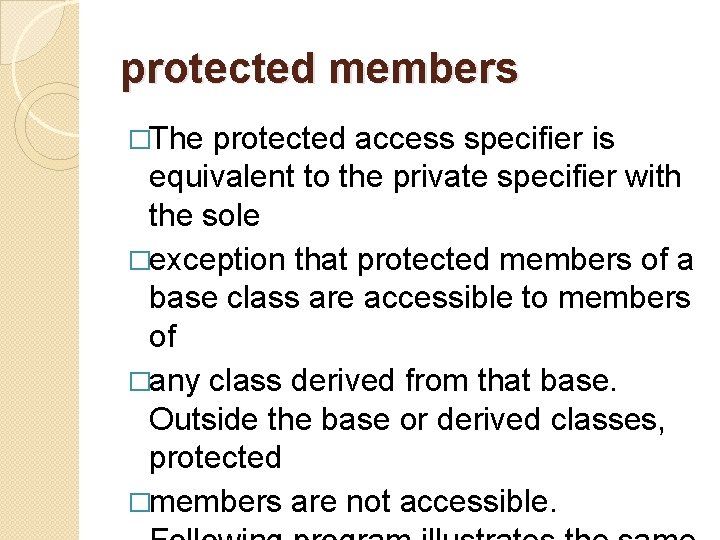
protected members �The protected access specifier is equivalent to the private specifier with the sole �exception that protected members of a base class are accessible to members of �any class derived from that base. Outside the base or derived classes, protected �members are not accessible.
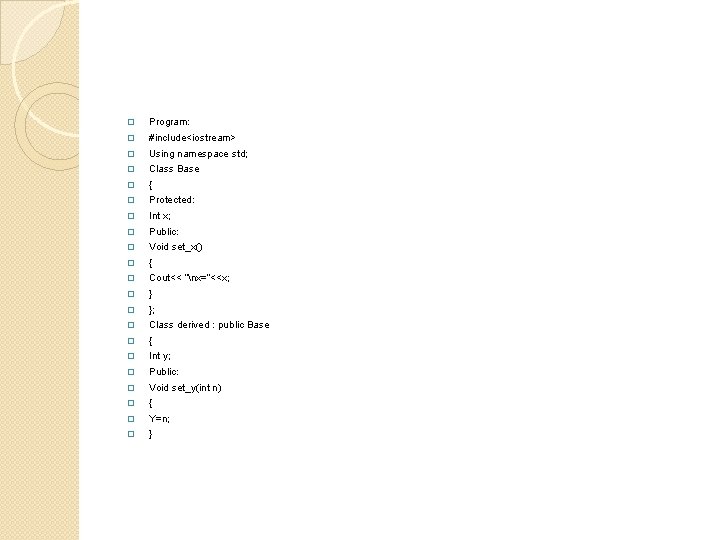
� Program: � #include<iostream> � Using namespace std; � Class Base � { � Protected: � Int x; � Public: � Void set_x() � { � Cout<< “nx=”<<x; � }; � Class derived : public Base � { � Int y; � Public: � Void set_y(int n) � { � Y=n; � }
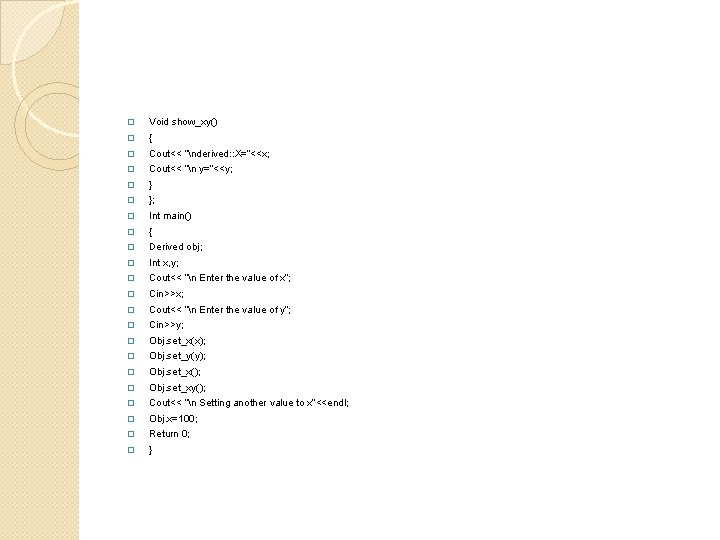
� Void show_xy() � { � Cout<< “nderived: : X=”<<x; � Cout<< “n y=”<<y; � }; � Int main() � { � Derived obj; � Int x, y; � Cout<< “n Enter the value of x”; � Cin>>x; � Cout<< “n Enter the value of y”; � Cin>>y; � Obj. set_x(x); � Obj. set_y(y); � Obj. set_x(); � Obj. set_xy(); � Cout<< “n Setting another value to x”<<endl; � Obj. x=100; � Return 0; � }
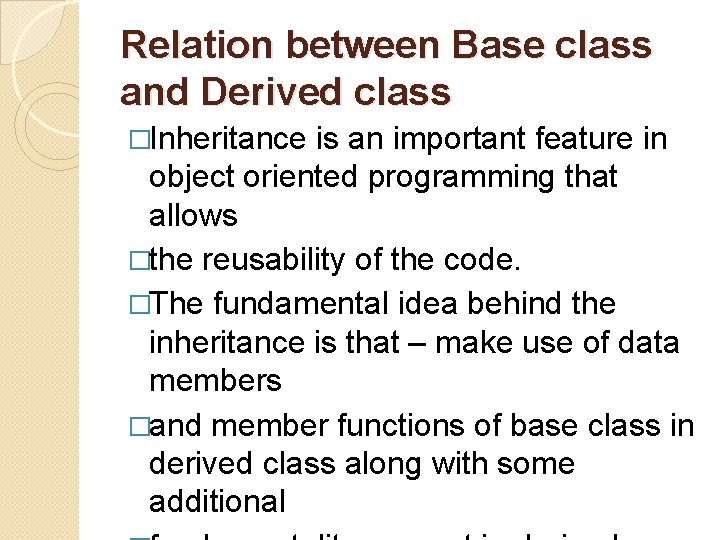
Relation between Base class and Derived class �Inheritance is an important feature in object oriented programming that allows �the reusability of the code. �The fundamental idea behind the inheritance is that – make use of data members �and member functions of base class in derived class along with some additional
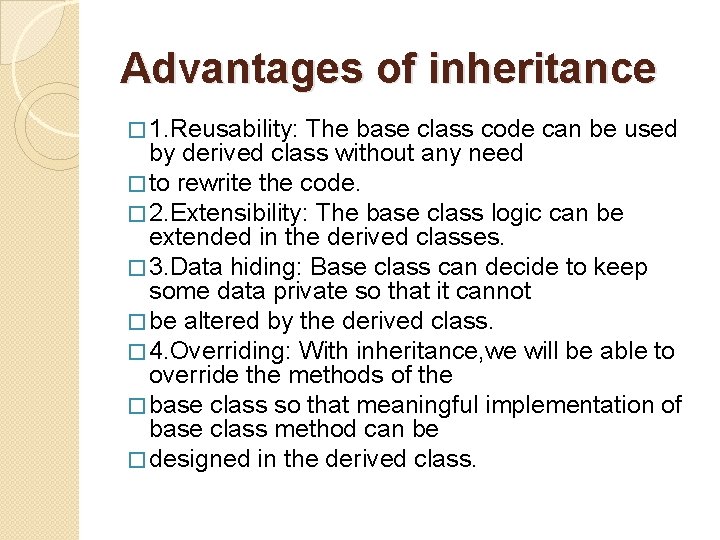
Advantages of inheritance � 1. Reusability: The base class code can be used by derived class without any need � to rewrite the code. � 2. Extensibility: The base class logic can be extended in the derived classes. � 3. Data hiding: Base class can decide to keep some data private so that it cannot � be altered by the derived class. � 4. Overriding: With inheritance, we will be able to override the methods of the � base class so that meaningful implementation of base class method can be � designed in the derived class.
Abstraction encapsulation inheritance polymorphism
Encapsulation inheritance and polymorphism
Encapsulation inheritance polymorphism
Encapsulation inheritance polymorphism
Abstraction encapsulation inheritance polymorphism
Polymorphism adalah
01:640:244 lecture notes - lecture 15: plat, idah, farad
Unit 10, unit 10 review tests, unit 10 general test
Unit root test lecture notes
Medusoid zooids are
Polymorphism in c
Ad hoc polymorphism c++
What is polymorphism in oop