Type Systems What is a type system System
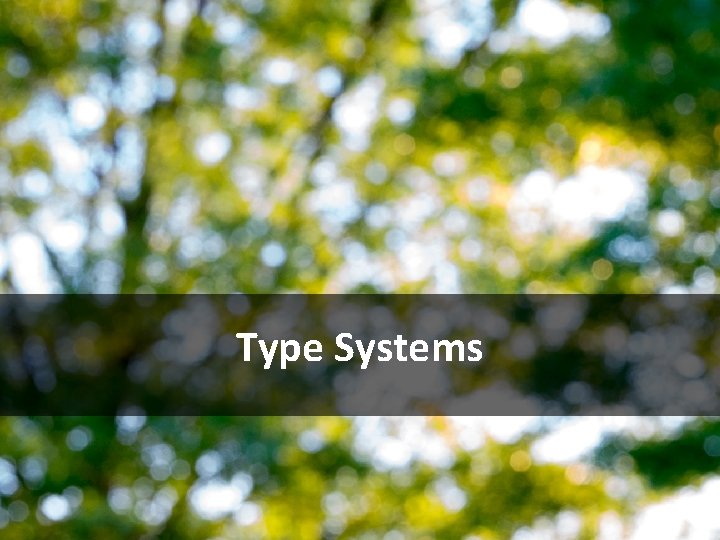
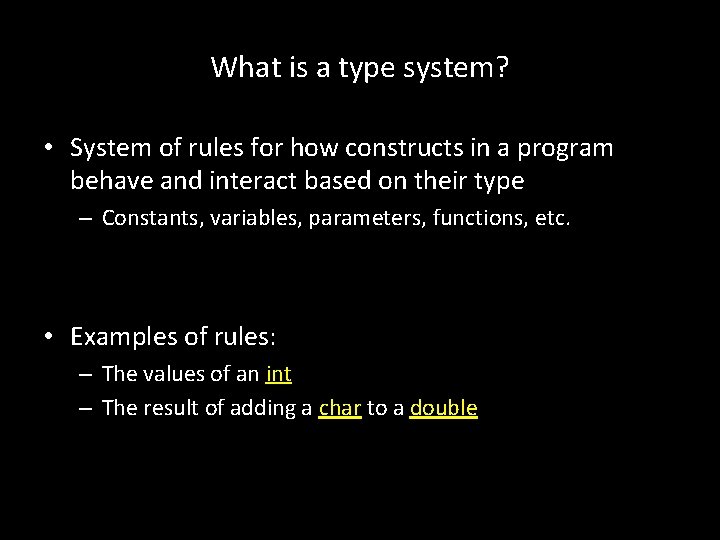
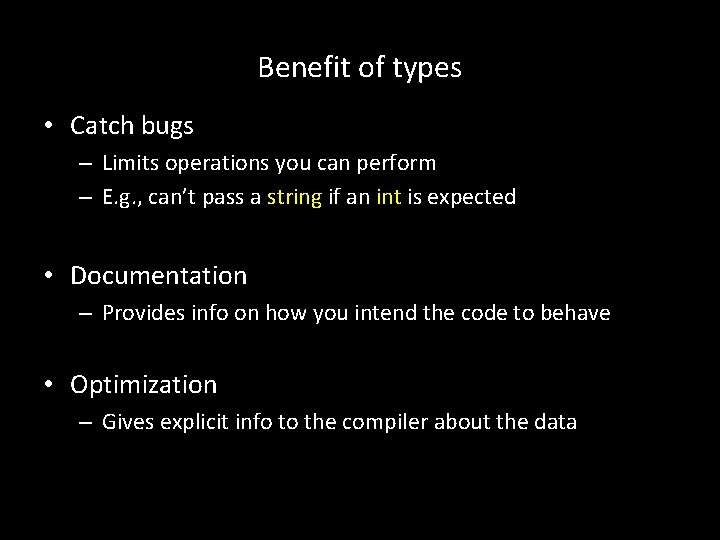
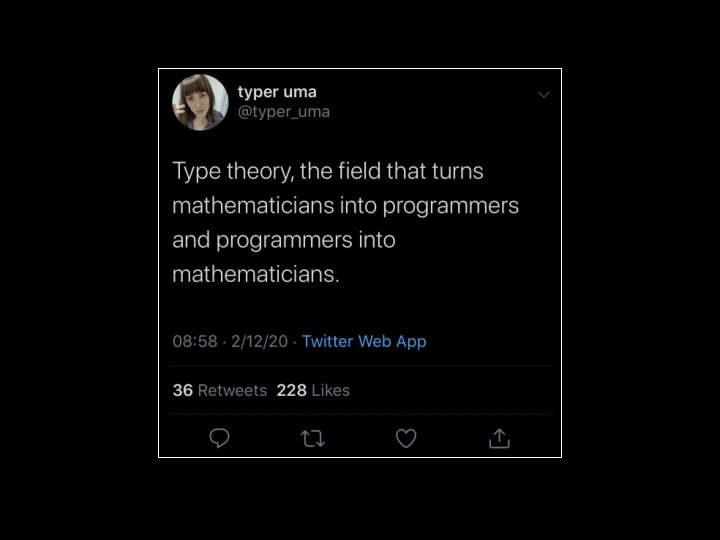
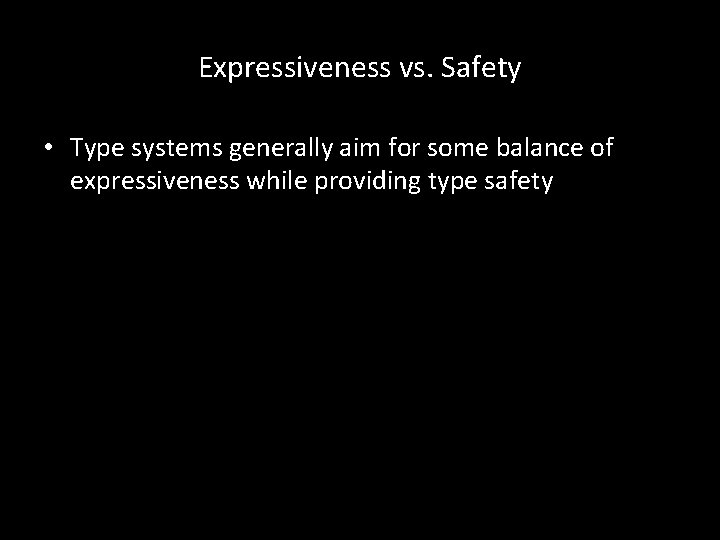
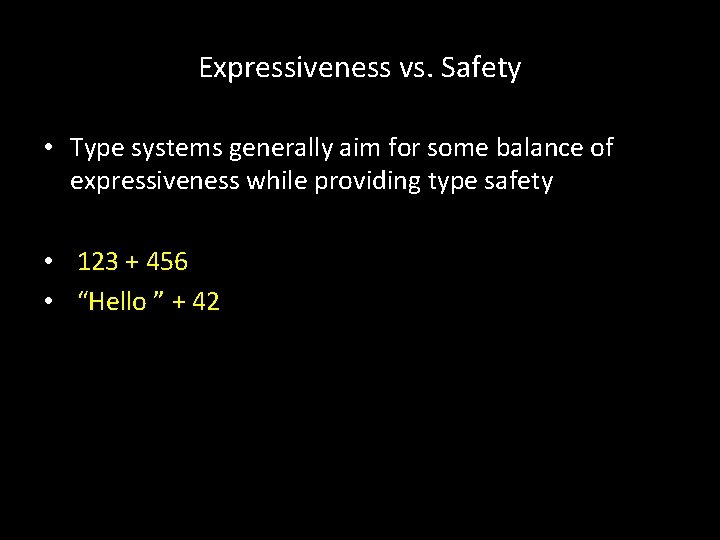
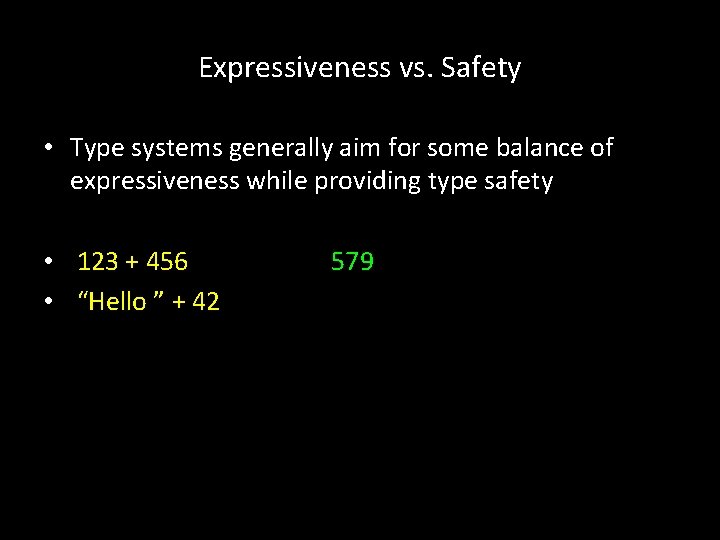
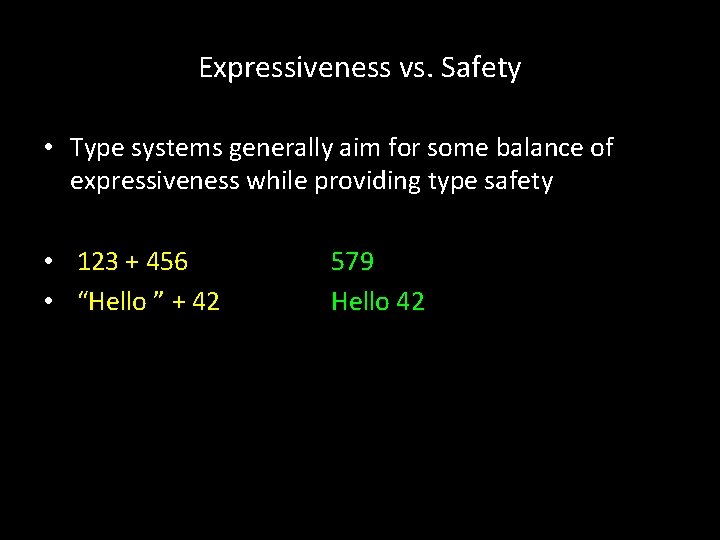
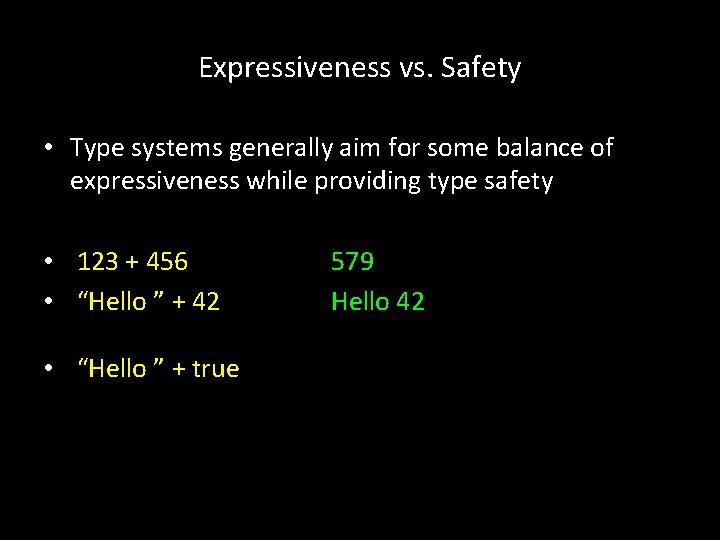
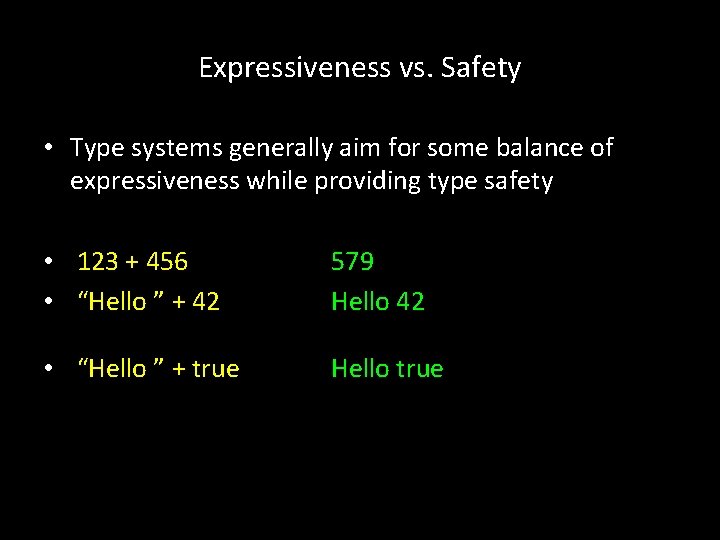
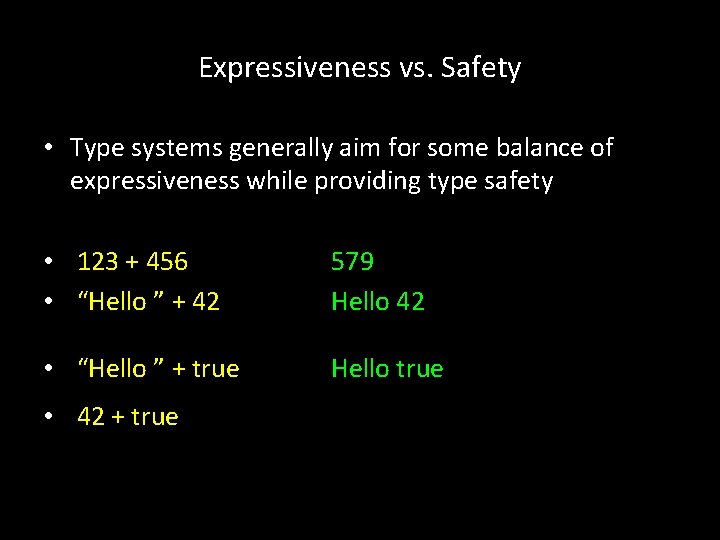
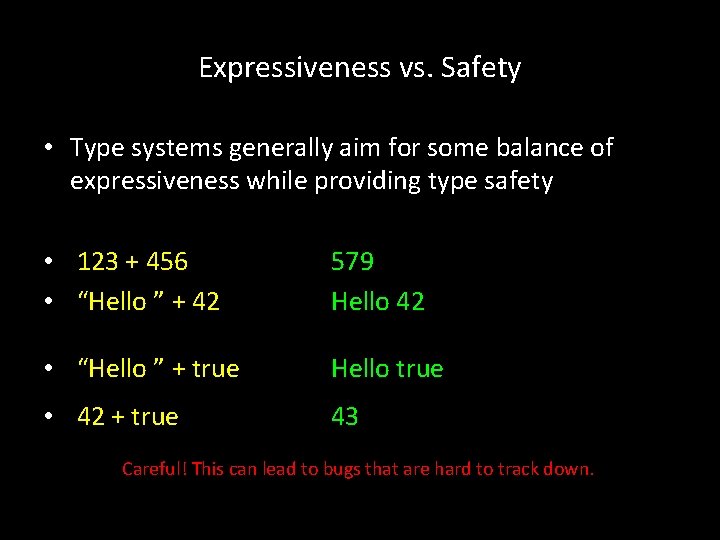
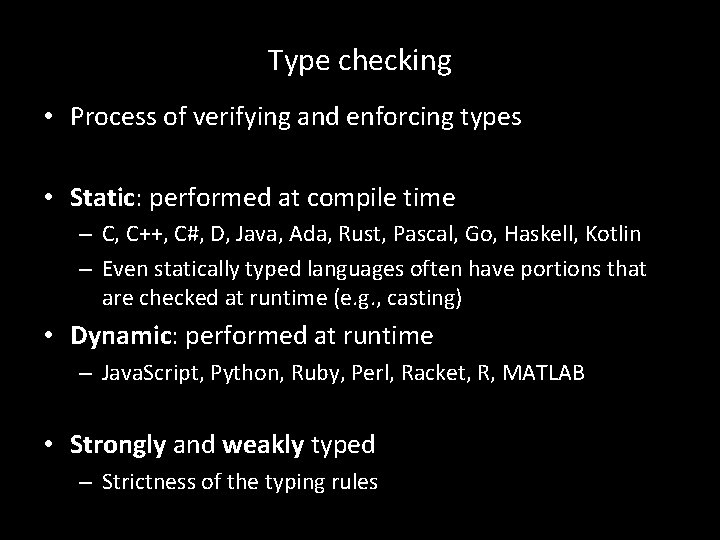
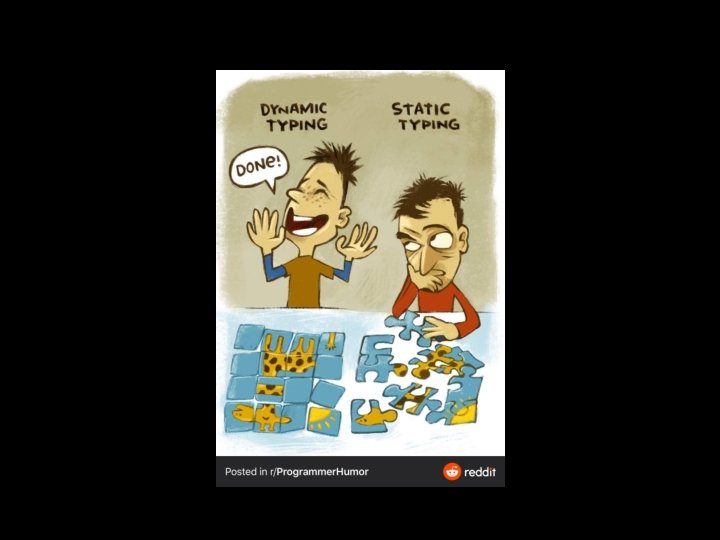
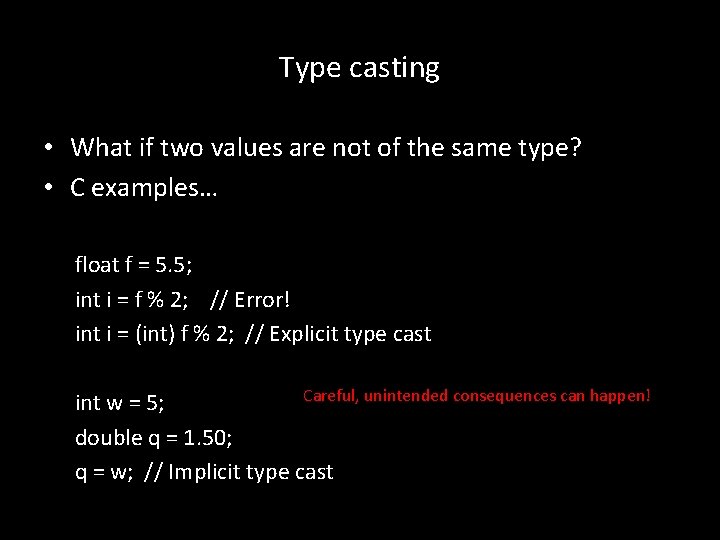
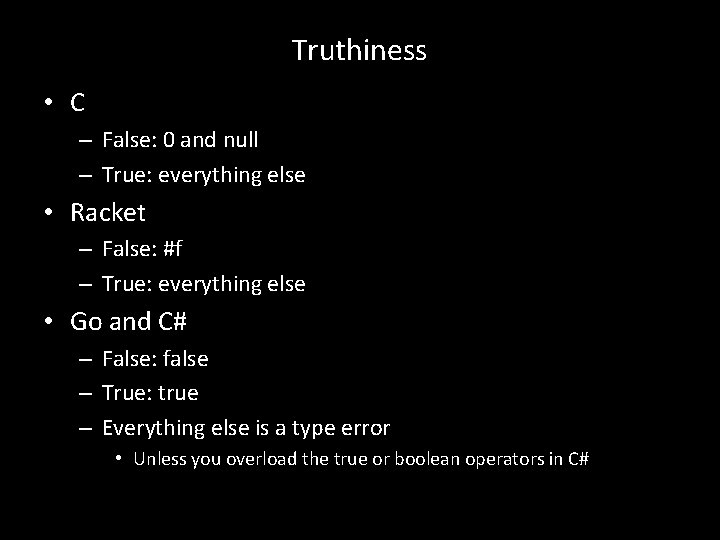
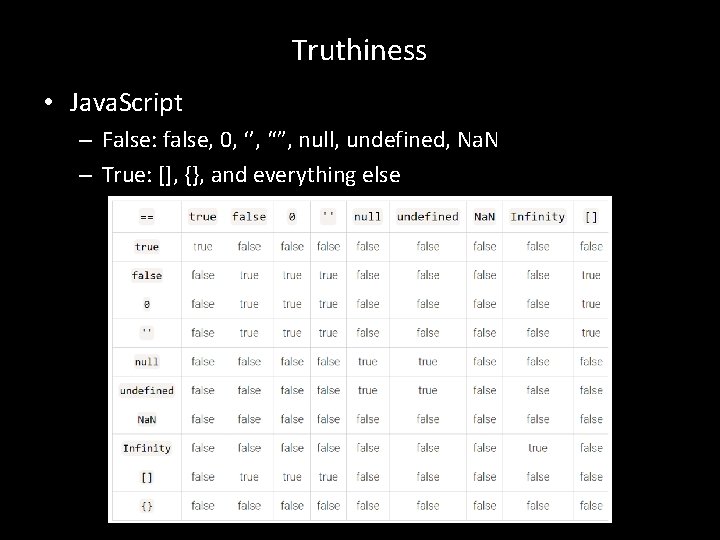
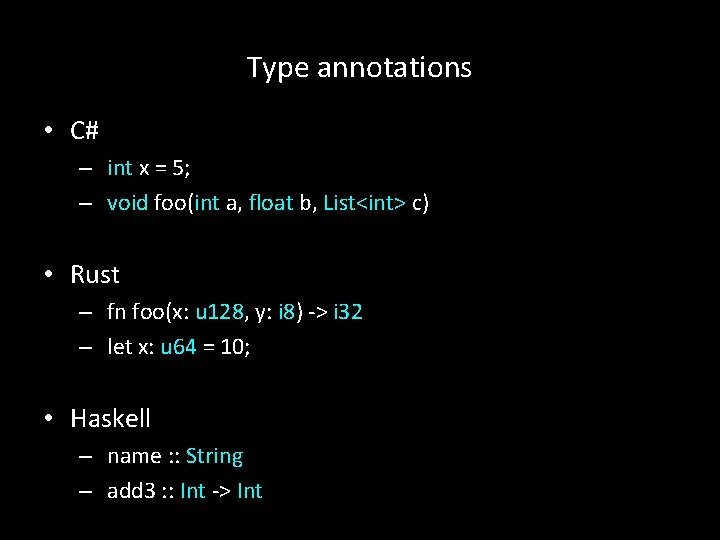
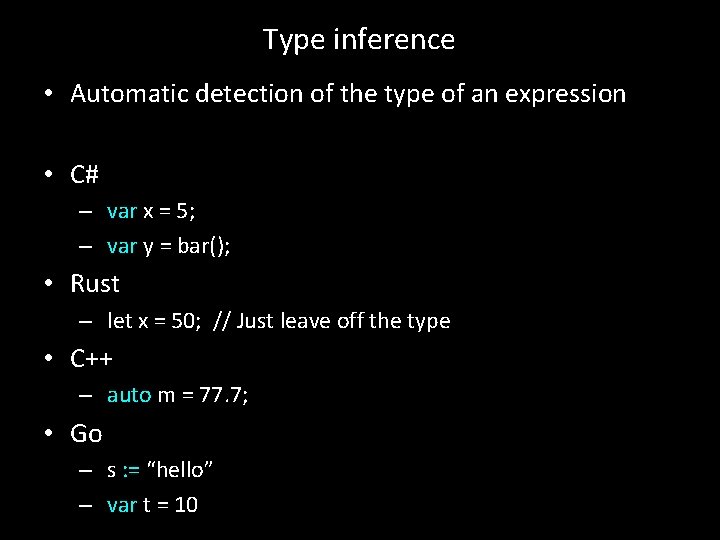
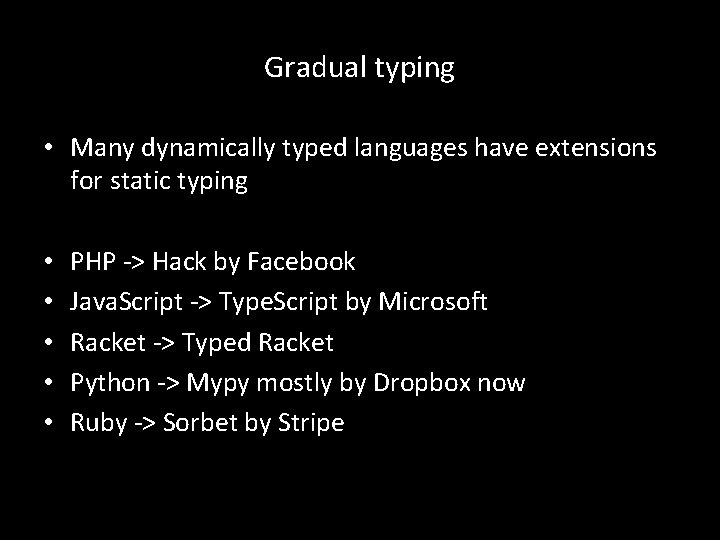
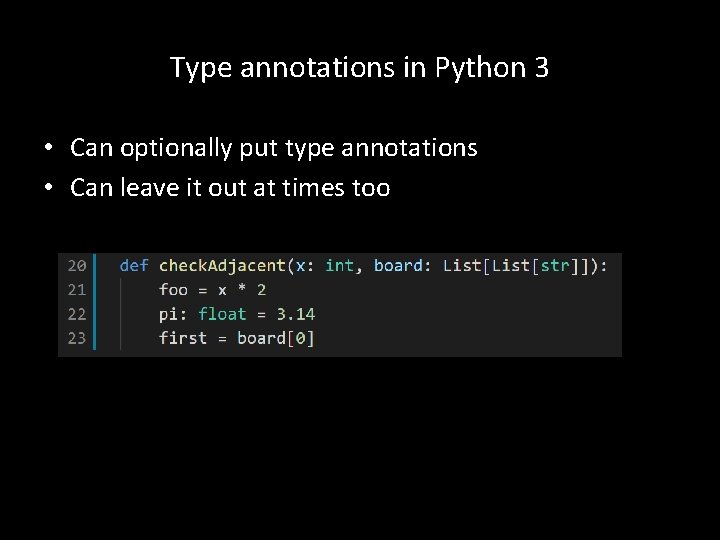
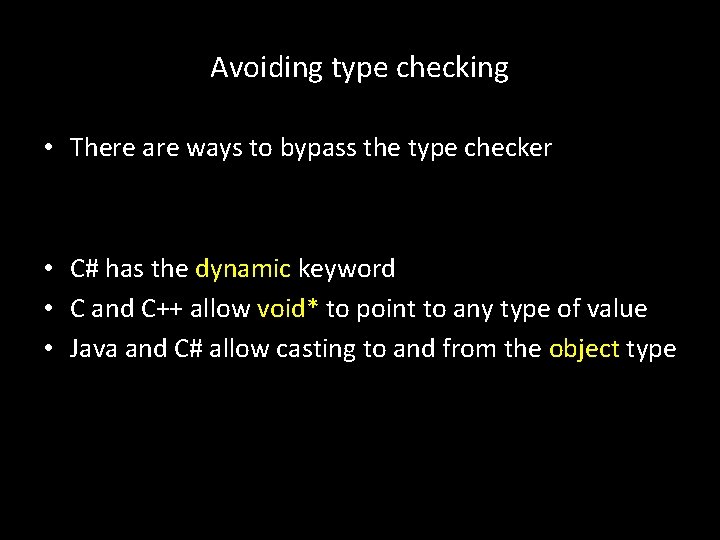
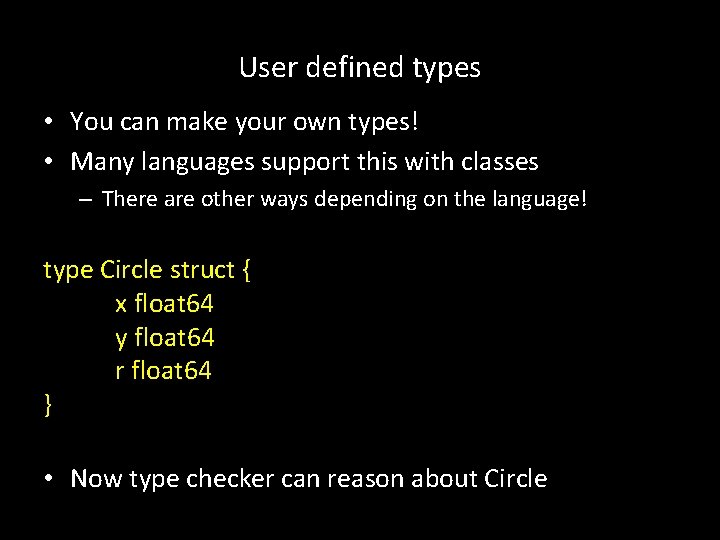
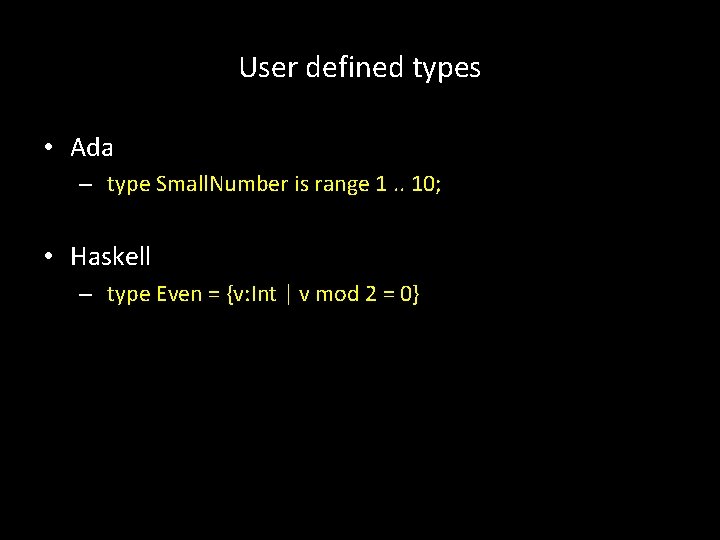
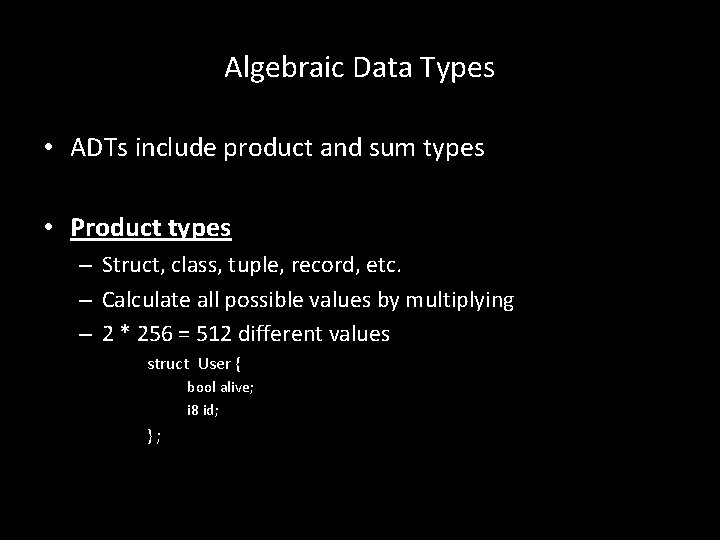
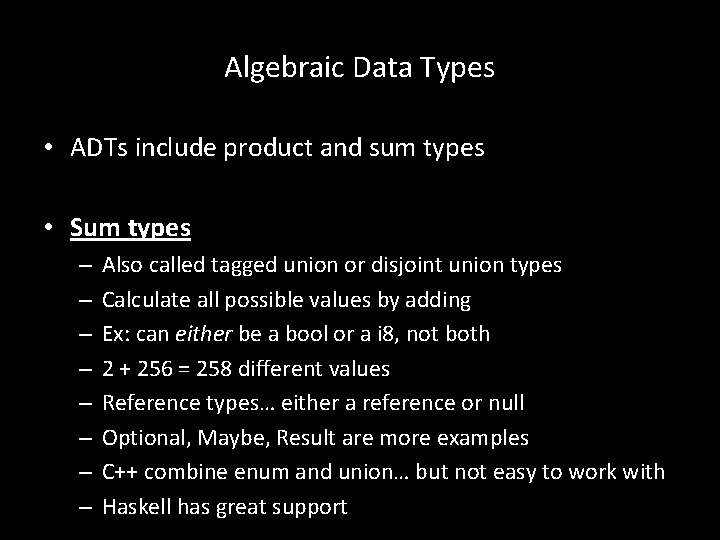
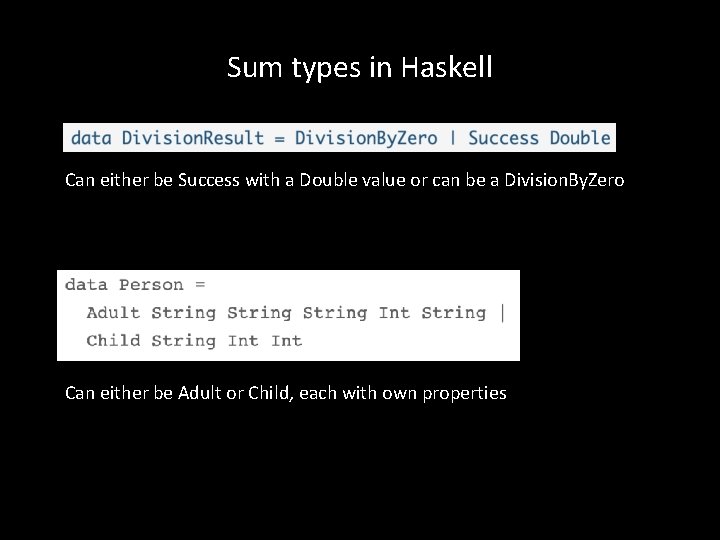
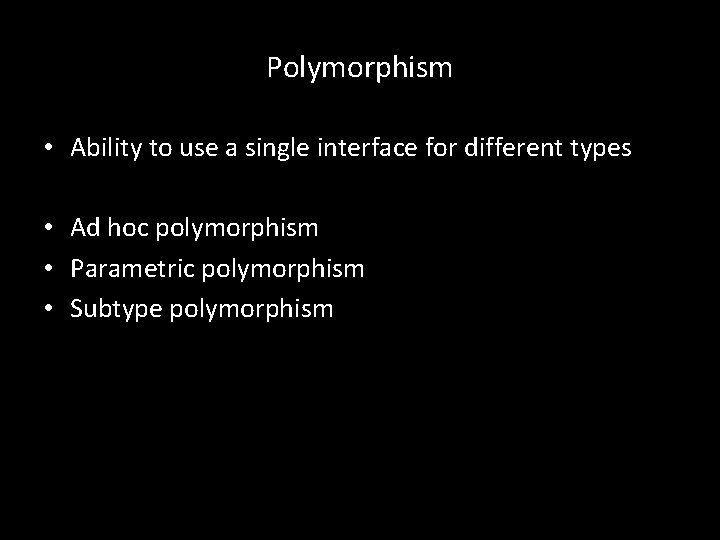
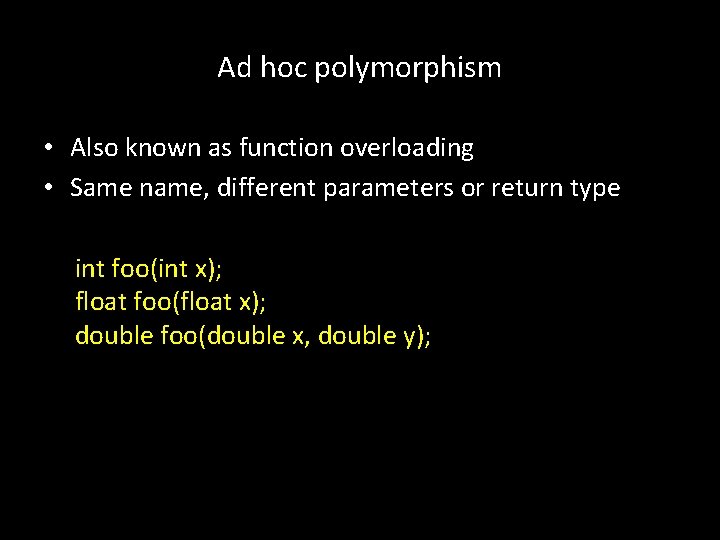
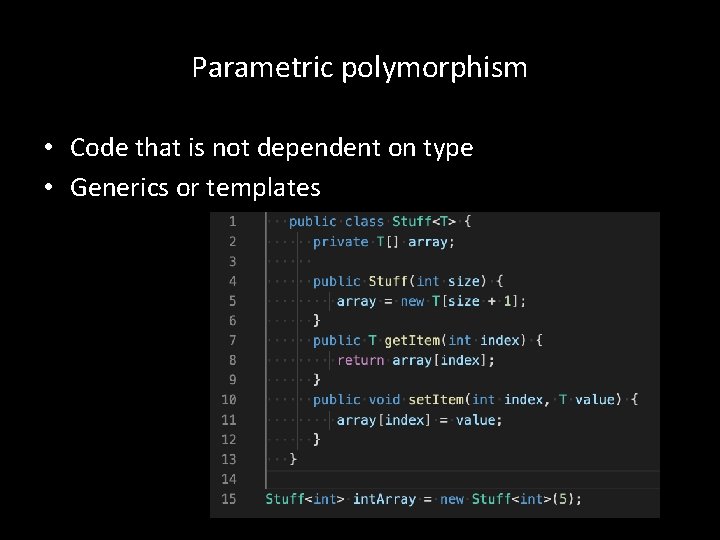
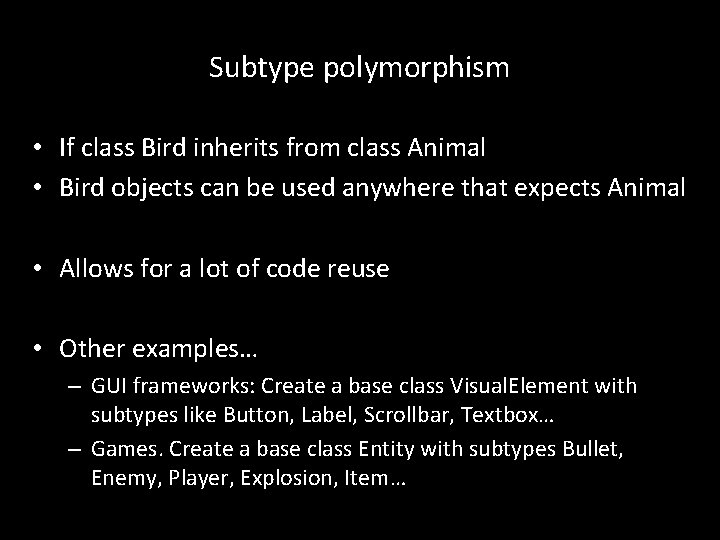
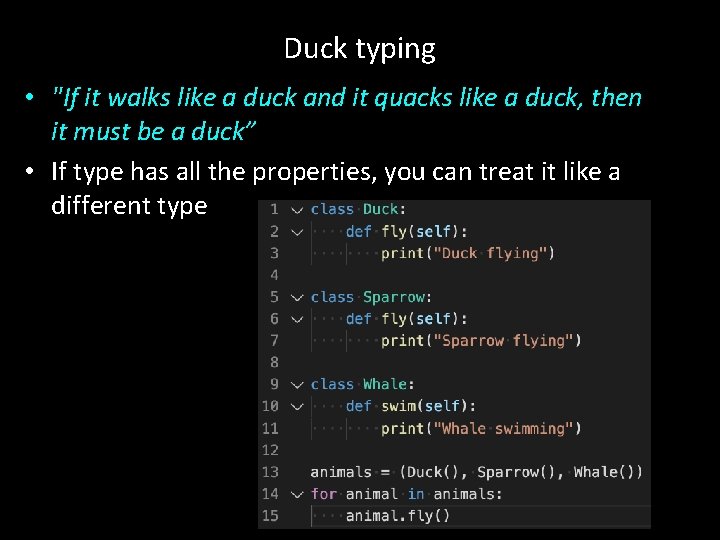
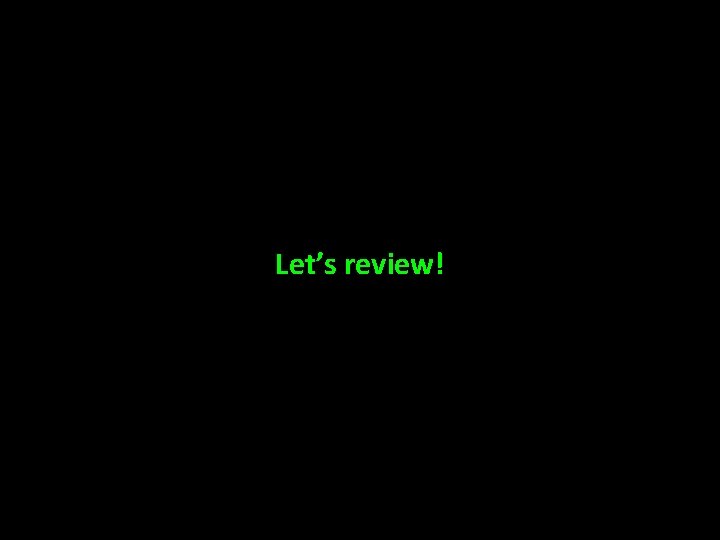
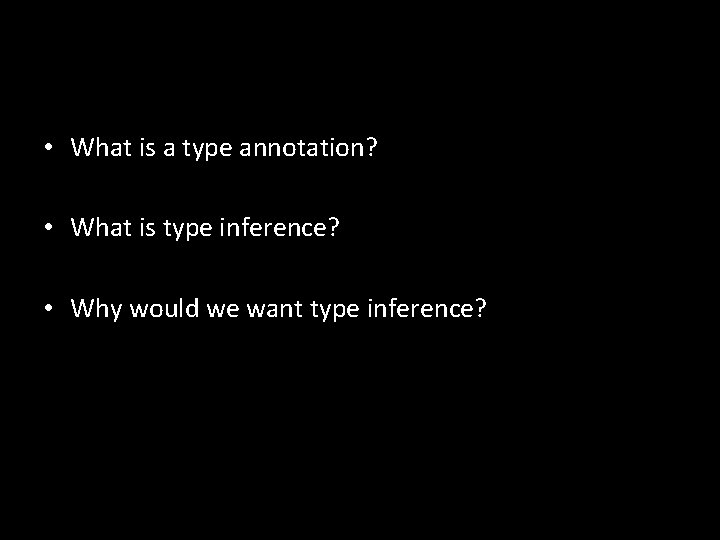
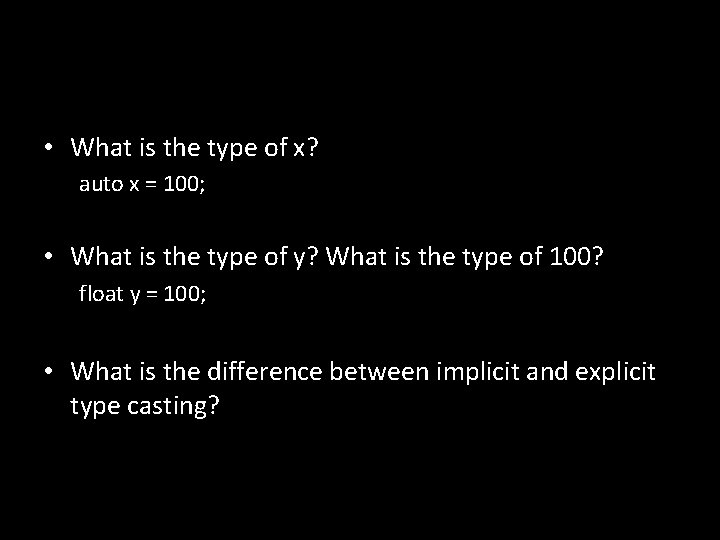
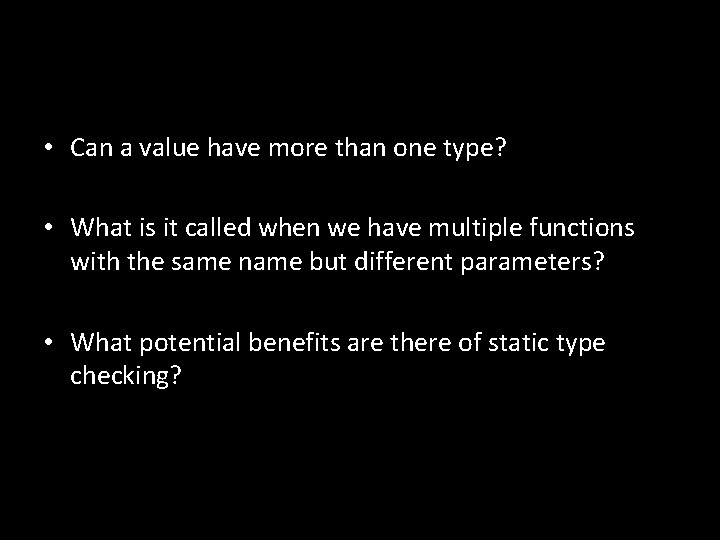
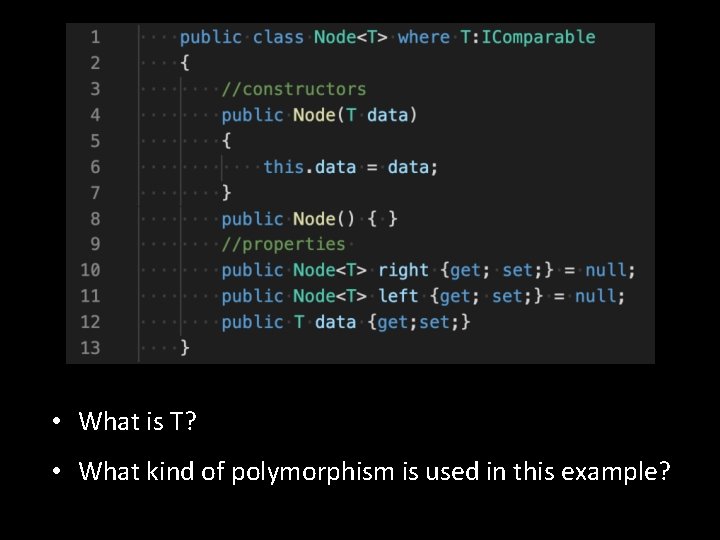
- Slides: 37
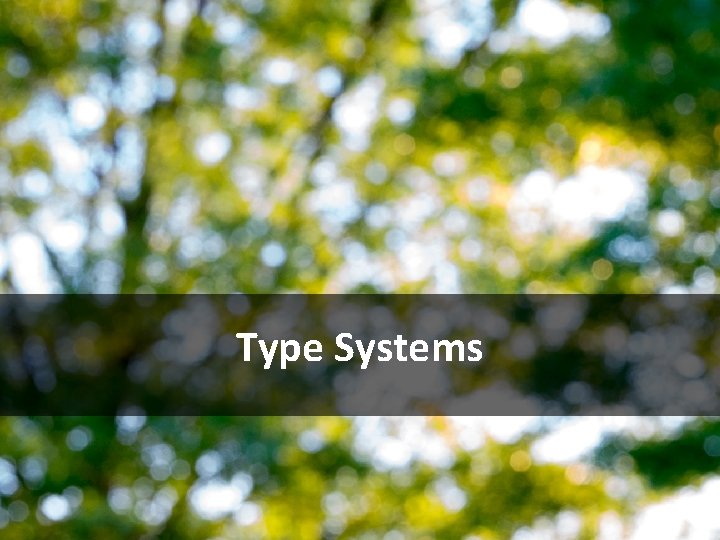
Type Systems
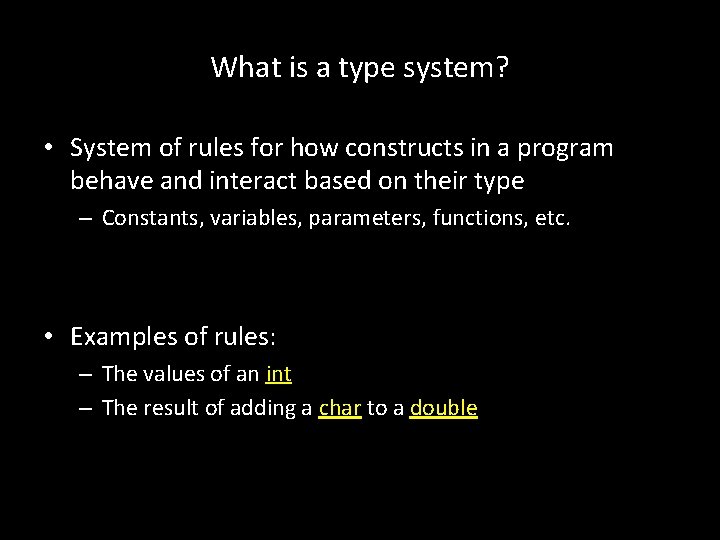
What is a type system? • System of rules for how constructs in a program behave and interact based on their type – Constants, variables, parameters, functions, etc. • Examples of rules: – The values of an int – The result of adding a char to a double
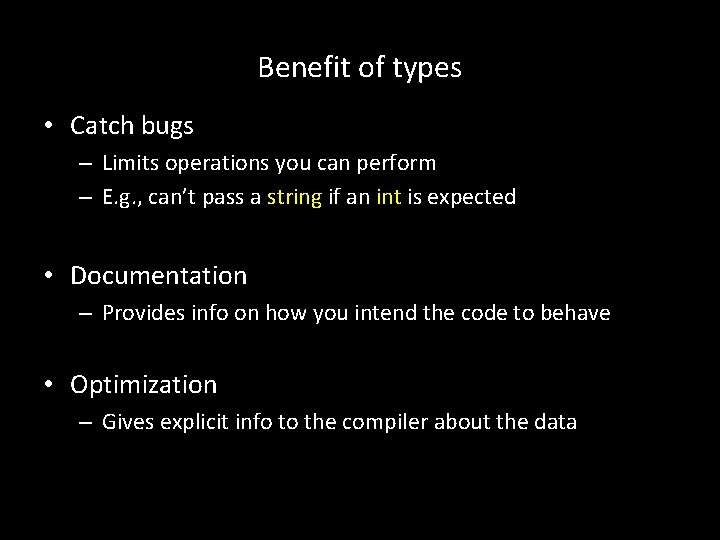
Benefit of types • Catch bugs – Limits operations you can perform – E. g. , can’t pass a string if an int is expected • Documentation – Provides info on how you intend the code to behave • Optimization – Gives explicit info to the compiler about the data
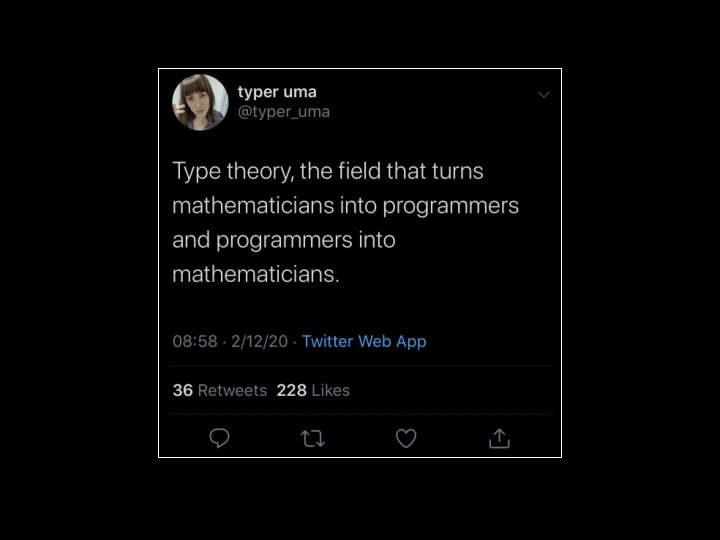
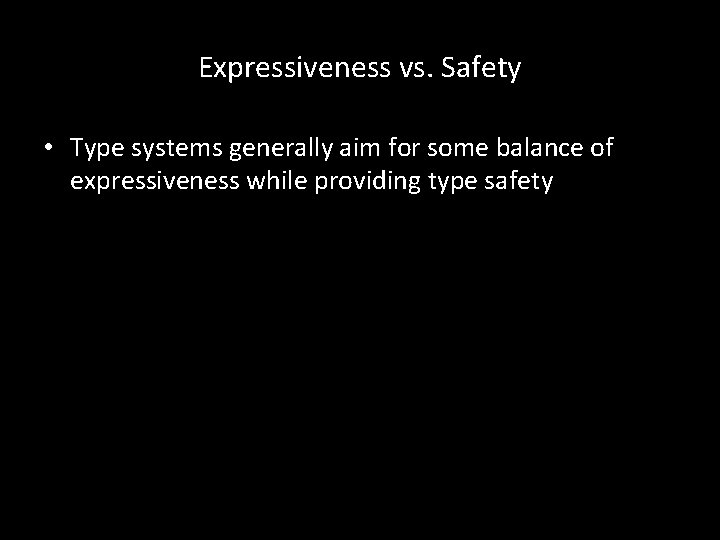
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
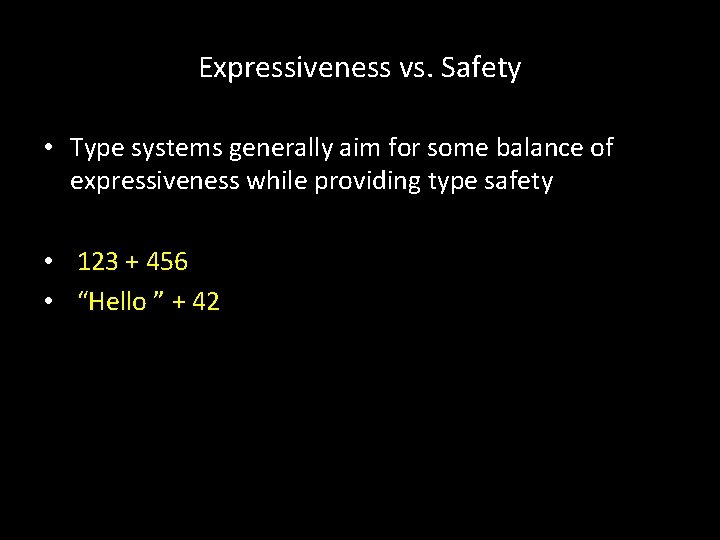
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
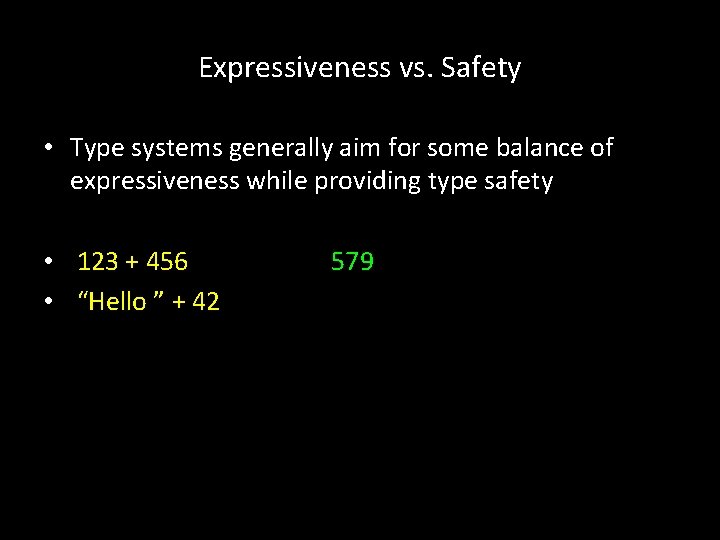
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
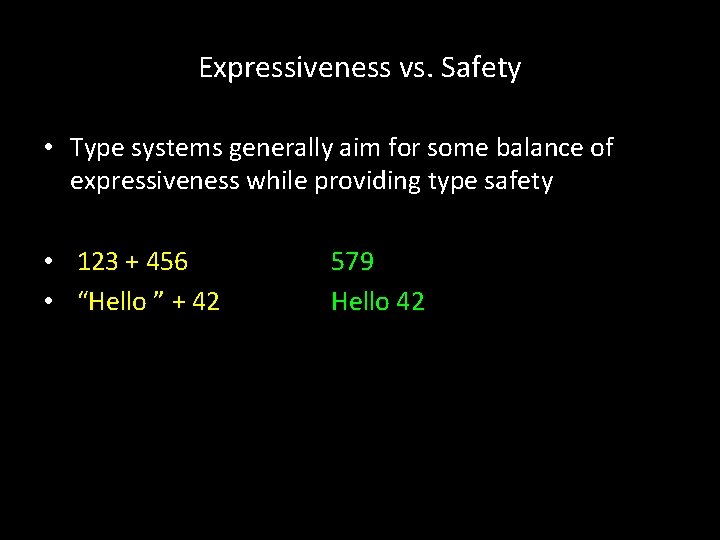
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
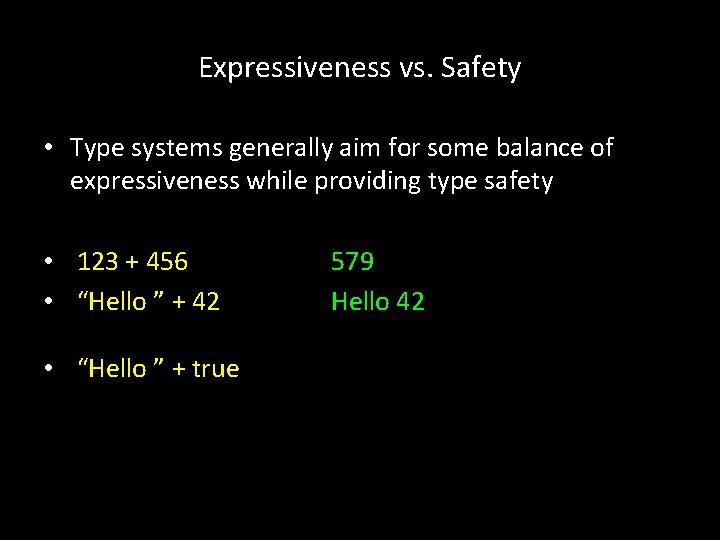
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
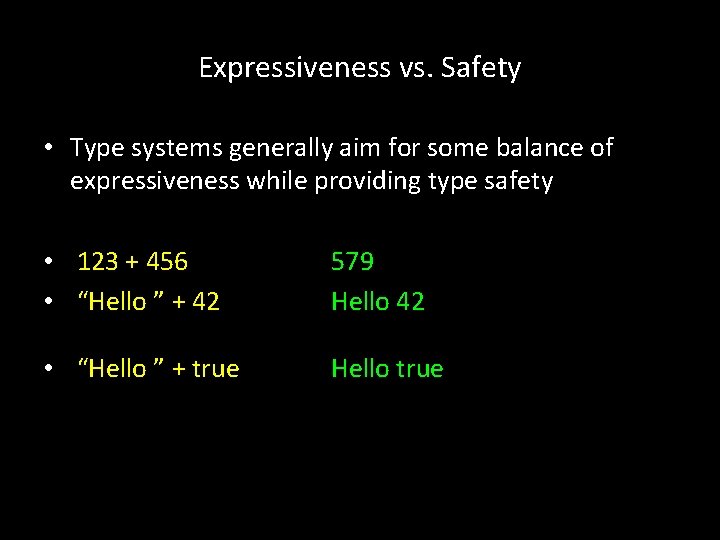
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
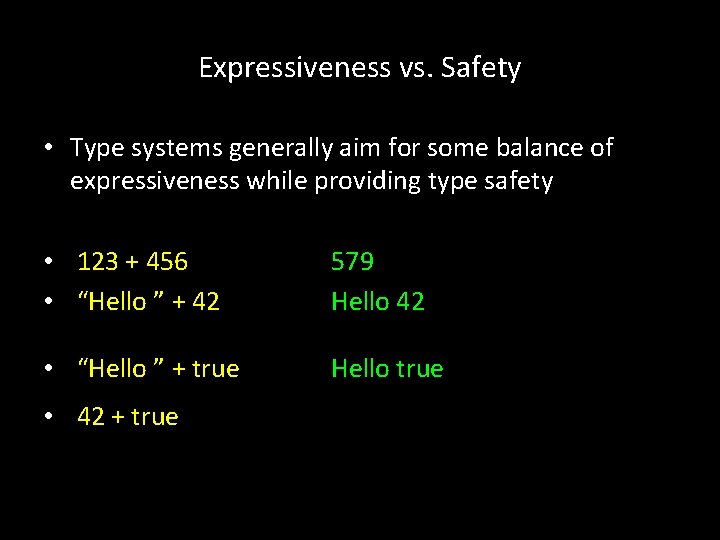
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43
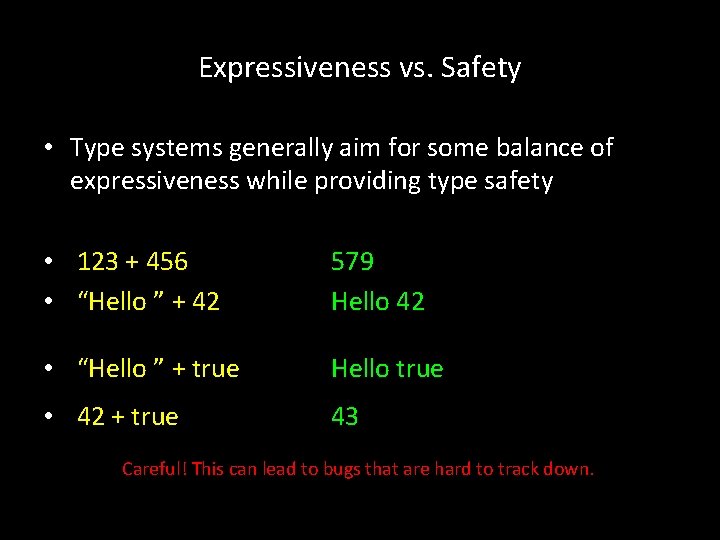
Expressiveness vs. Safety • Type systems generally aim for some balance of expressiveness while providing type safety • 123 + 456 • “Hello ” + 42 579 Hello 42 • “Hello ” + true Hello true • 42 + true 43 Careful! This can lead to bugs that are hard to track down.
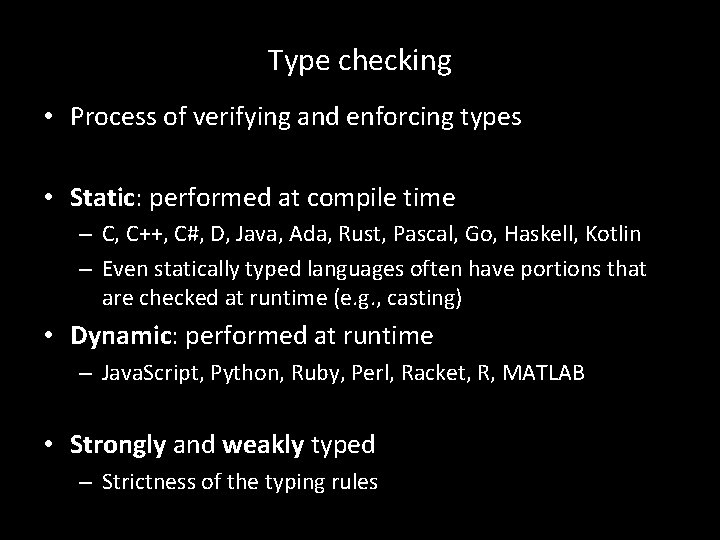
Type checking • Process of verifying and enforcing types • Static: performed at compile time – C, C++, C#, D, Java, Ada, Rust, Pascal, Go, Haskell, Kotlin – Even statically typed languages often have portions that are checked at runtime (e. g. , casting) • Dynamic: performed at runtime – Java. Script, Python, Ruby, Perl, Racket, R, MATLAB • Strongly and weakly typed – Strictness of the typing rules
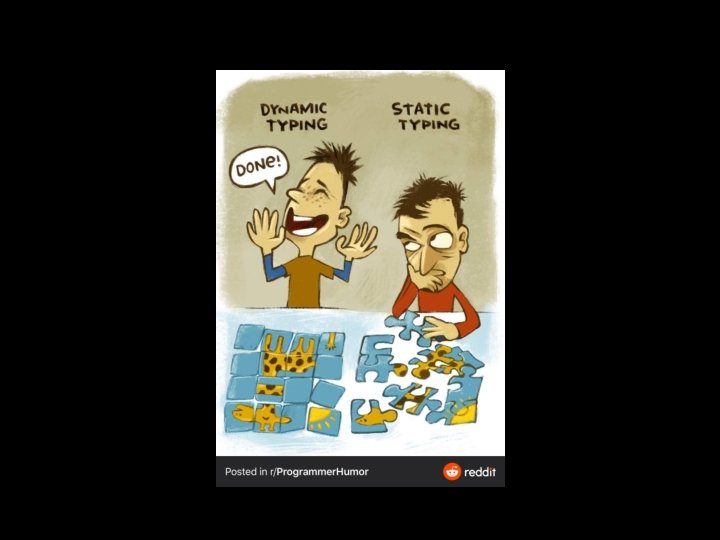
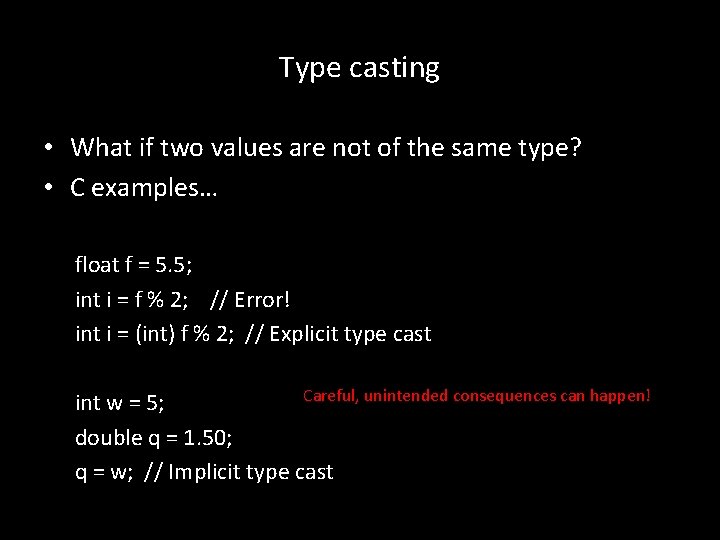
Type casting • What if two values are not of the same type? • C examples… float f = 5. 5; int i = f % 2; // Error! int i = (int) f % 2; // Explicit type cast Careful, unintended consequences can happen! int w = 5; double q = 1. 50; q = w; // Implicit type cast
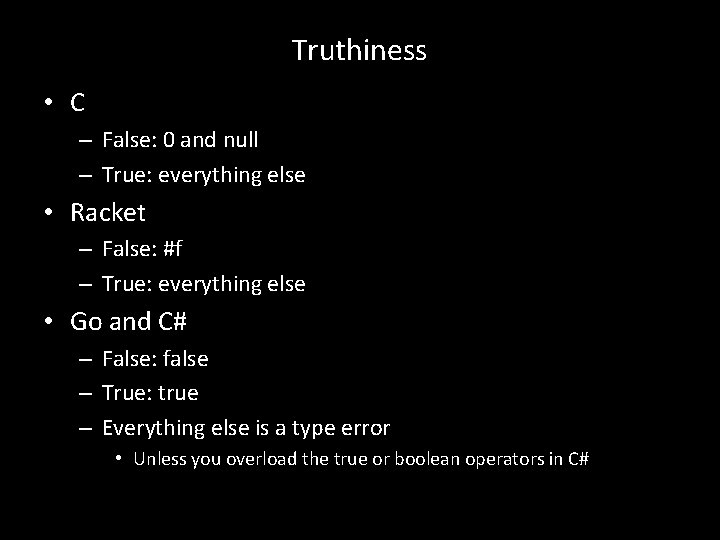
Truthiness • C – False: 0 and null – True: everything else • Racket – False: #f – True: everything else • Go and C# – False: false – True: true – Everything else is a type error • Unless you overload the true or boolean operators in C#
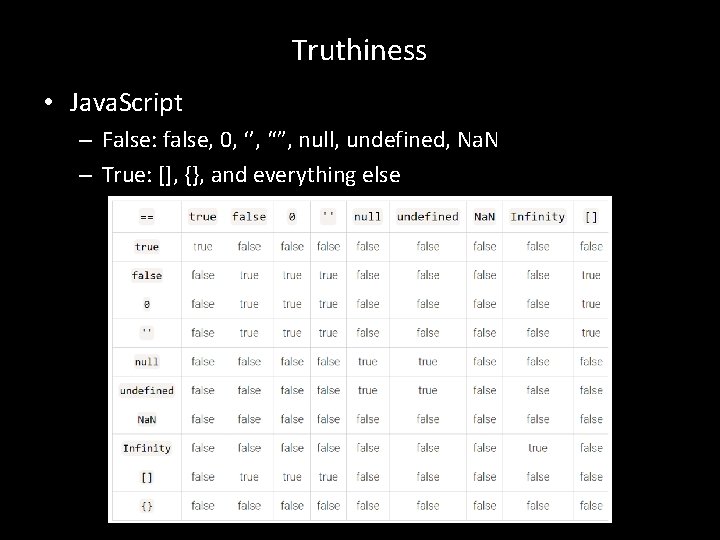
Truthiness • Java. Script – False: false, 0, ‘’, “”, null, undefined, Na. N – True: [], {}, and everything else
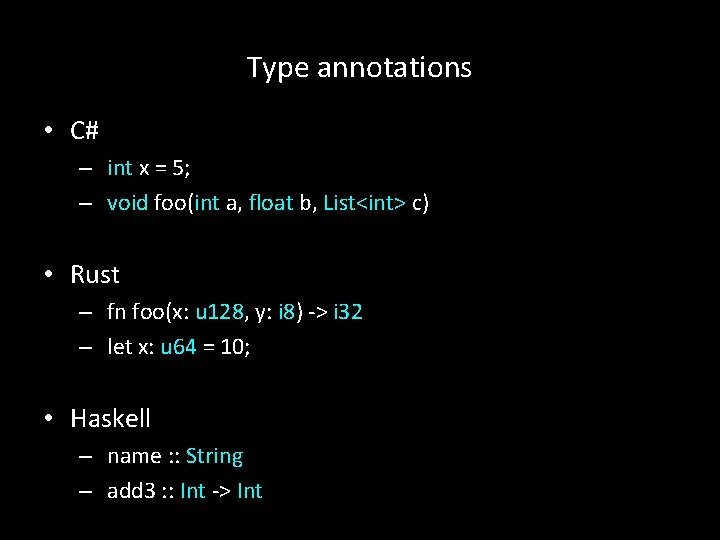
Type annotations • C# – int x = 5; – void foo(int a, float b, List<int> c) • Rust – fn foo(x: u 128, y: i 8) -> i 32 – let x: u 64 = 10; • Haskell – name : : String – add 3 : : Int -> Int
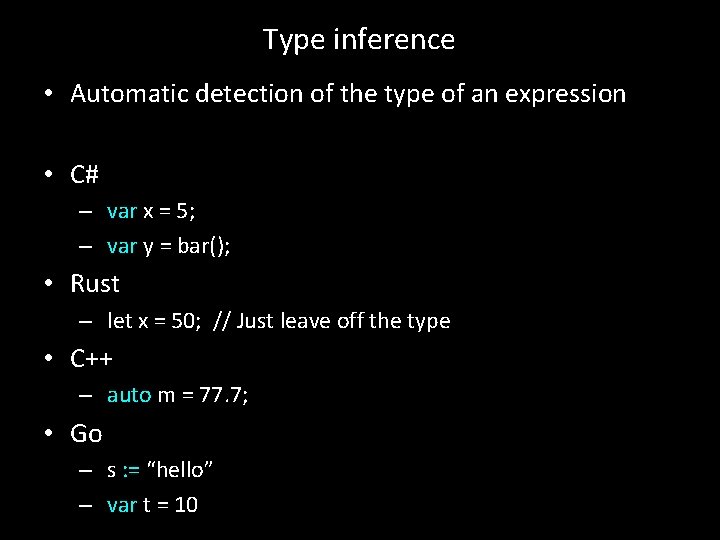
Type inference • Automatic detection of the type of an expression • C# – var x = 5; – var y = bar(); • Rust – let x = 50; // Just leave off the type • C++ – auto m = 77. 7; • Go – s : = “hello” – var t = 10
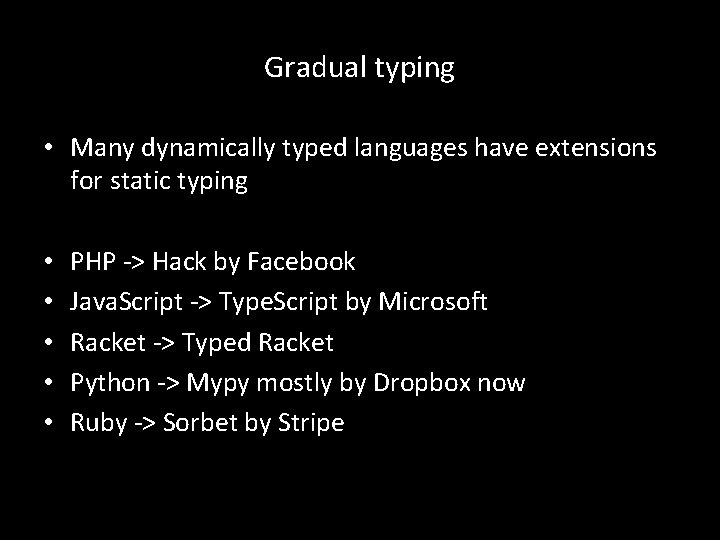
Gradual typing • Many dynamically typed languages have extensions for static typing • • • PHP -> Hack by Facebook Java. Script -> Type. Script by Microsoft Racket -> Typed Racket Python -> Mypy mostly by Dropbox now Ruby -> Sorbet by Stripe
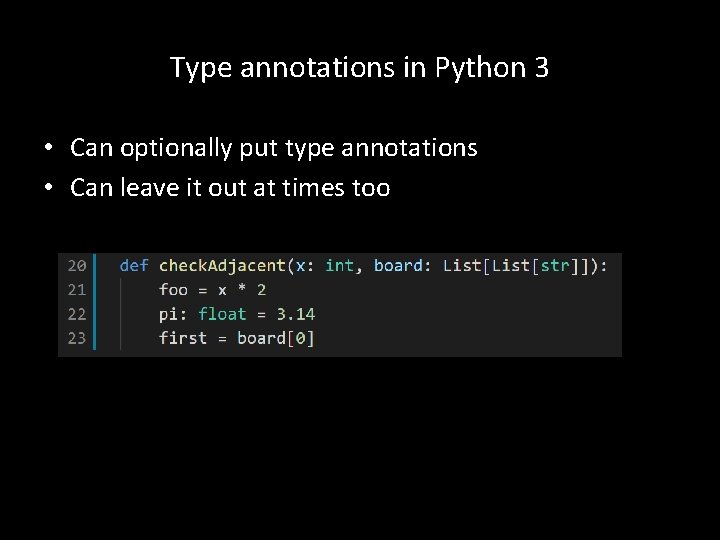
Type annotations in Python 3 • Can optionally put type annotations • Can leave it out at times too
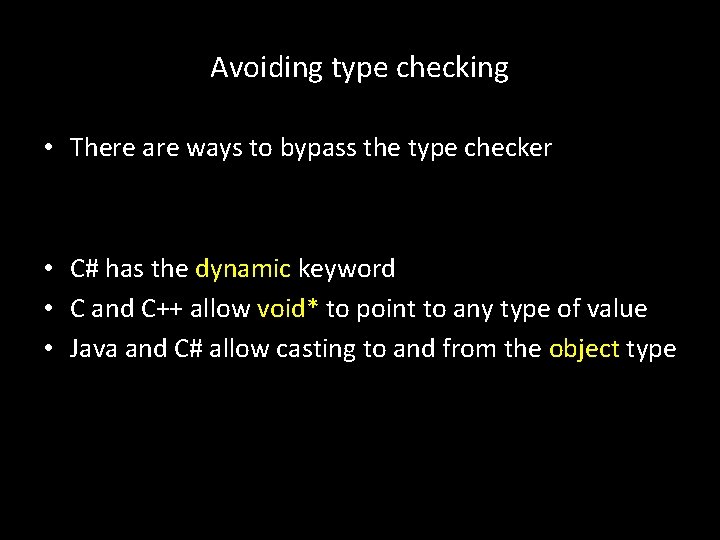
Avoiding type checking • There are ways to bypass the type checker • C# has the dynamic keyword • C and C++ allow void* to point to any type of value • Java and C# allow casting to and from the object type
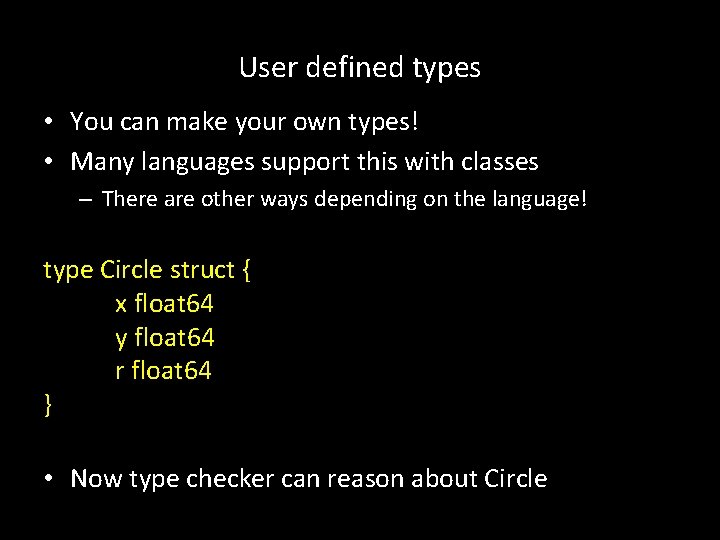
User defined types • You can make your own types! • Many languages support this with classes – There are other ways depending on the language! type Circle struct { x float 64 y float 64 r float 64 } • Now type checker can reason about Circle
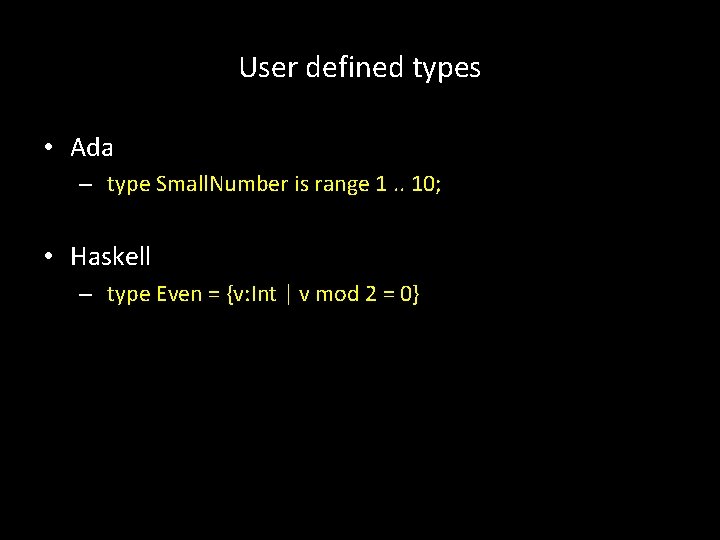
User defined types • Ada – type Small. Number is range 1. . 10; • Haskell – type Even = {v: Int | v mod 2 = 0}
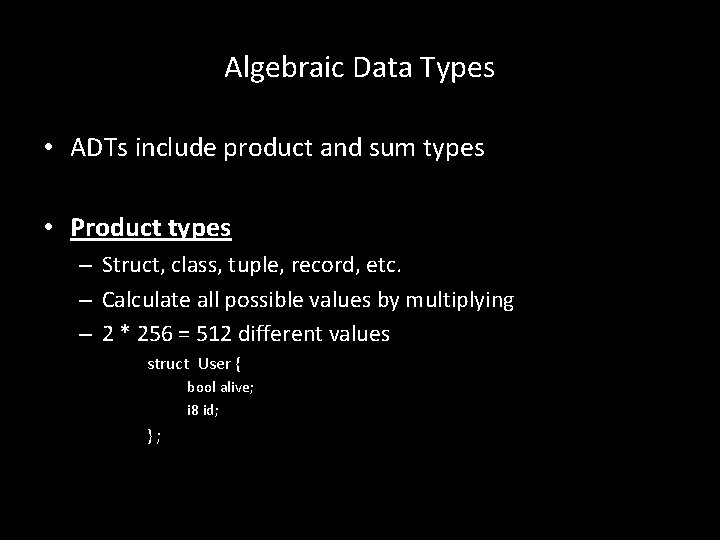
Algebraic Data Types • ADTs include product and sum types • Product types – Struct, class, tuple, record, etc. – Calculate all possible values by multiplying – 2 * 256 = 512 different values struct User { bool alive; i 8 id; } ;
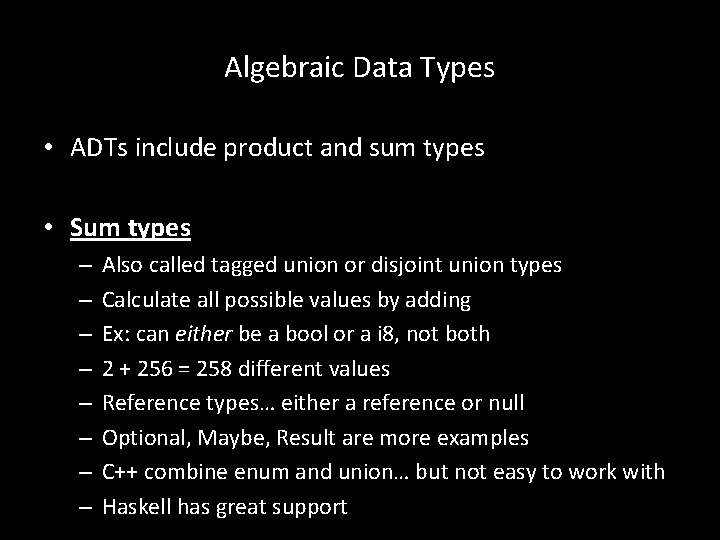
Algebraic Data Types • ADTs include product and sum types • Sum types – – – – Also called tagged union or disjoint union types Calculate all possible values by adding Ex: can either be a bool or a i 8, not both 2 + 256 = 258 different values Reference types… either a reference or null Optional, Maybe, Result are more examples C++ combine enum and union… but not easy to work with Haskell has great support
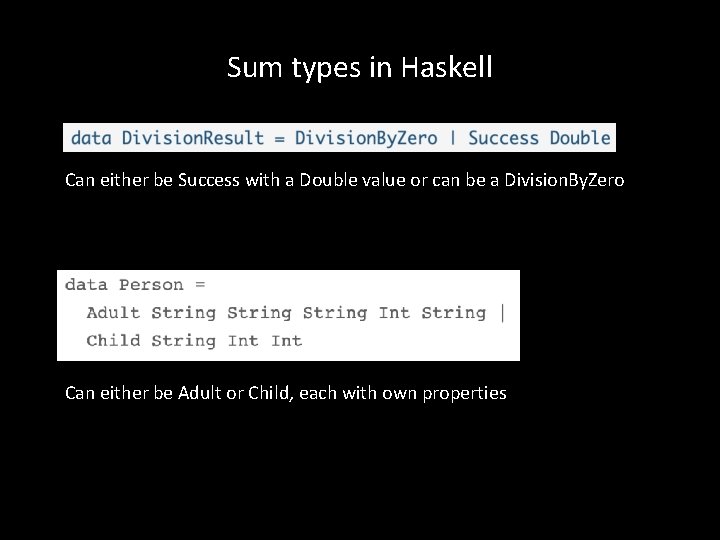
Sum types in Haskell Can either be Success with a Double value or can be a Division. By. Zero Can either be Adult or Child, each with own properties
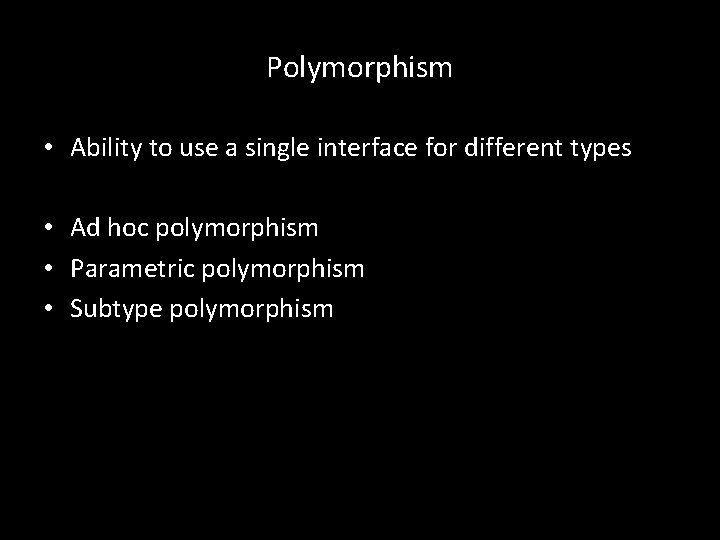
Polymorphism • Ability to use a single interface for different types • Ad hoc polymorphism • Parametric polymorphism • Subtype polymorphism
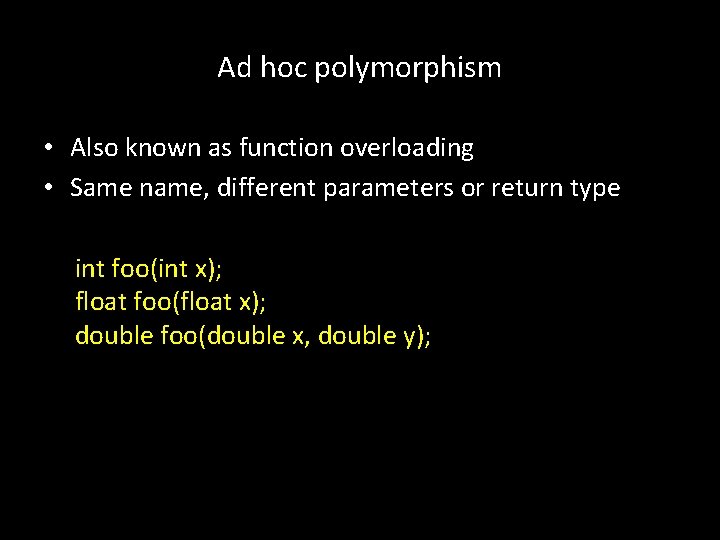
Ad hoc polymorphism • Also known as function overloading • Same name, different parameters or return type int foo(int x); float foo(float x); double foo(double x, double y);
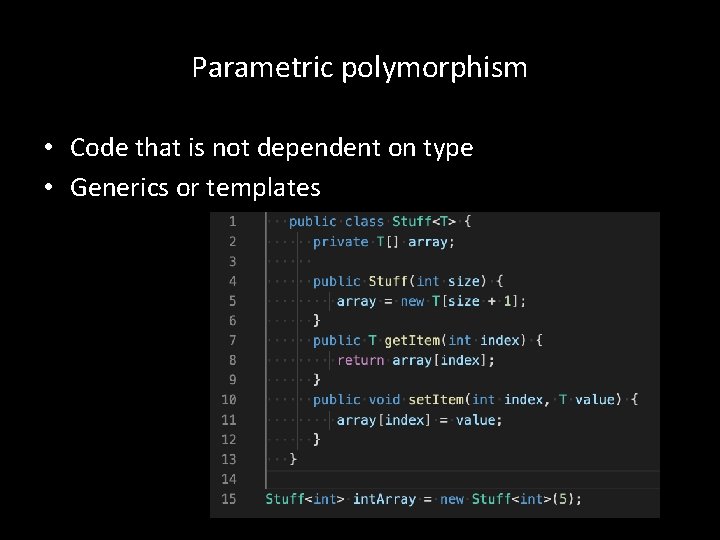
Parametric polymorphism • Code that is not dependent on type • Generics or templates
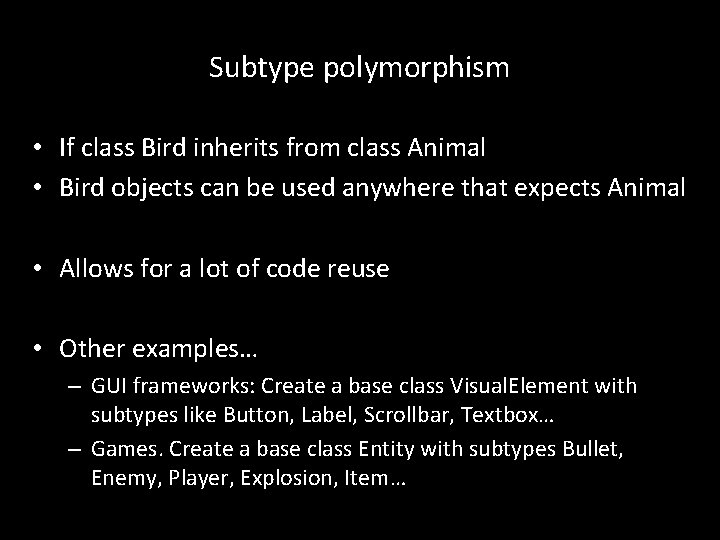
Subtype polymorphism • If class Bird inherits from class Animal • Bird objects can be used anywhere that expects Animal • Allows for a lot of code reuse • Other examples… – GUI frameworks: Create a base class Visual. Element with subtypes like Button, Label, Scrollbar, Textbox… – Games. Create a base class Entity with subtypes Bullet, Enemy, Player, Explosion, Item…
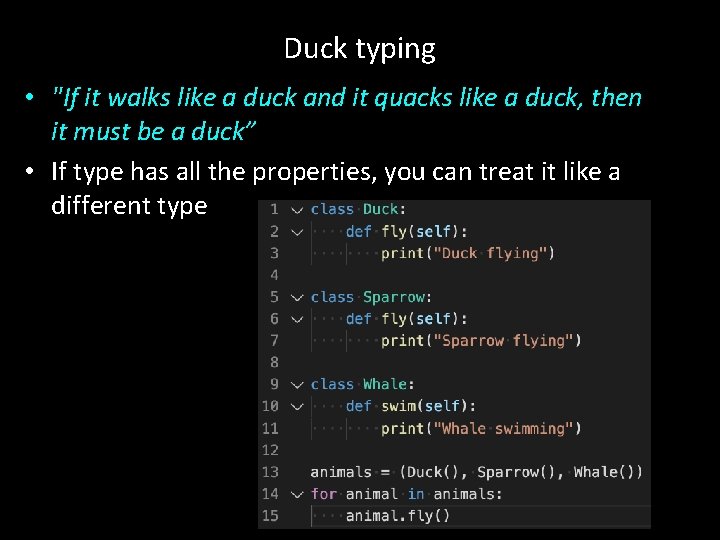
Duck typing • "If it walks like a duck and it quacks like a duck, then it must be a duck” • If type has all the properties, you can treat it like a different type
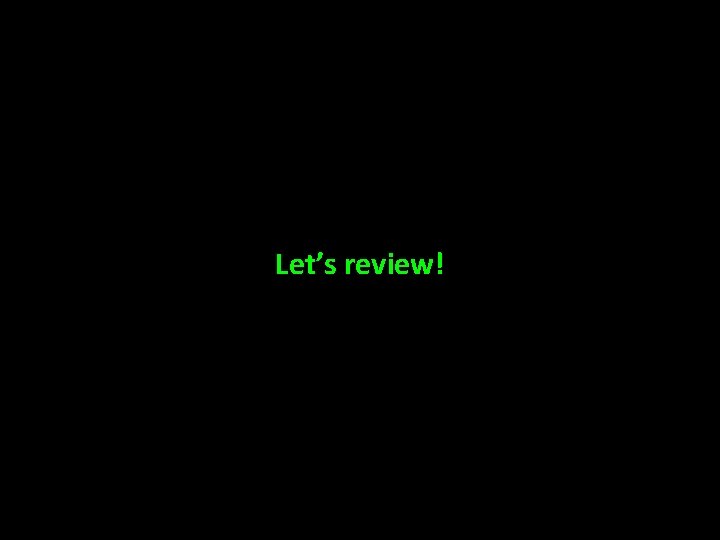
Let’s review!
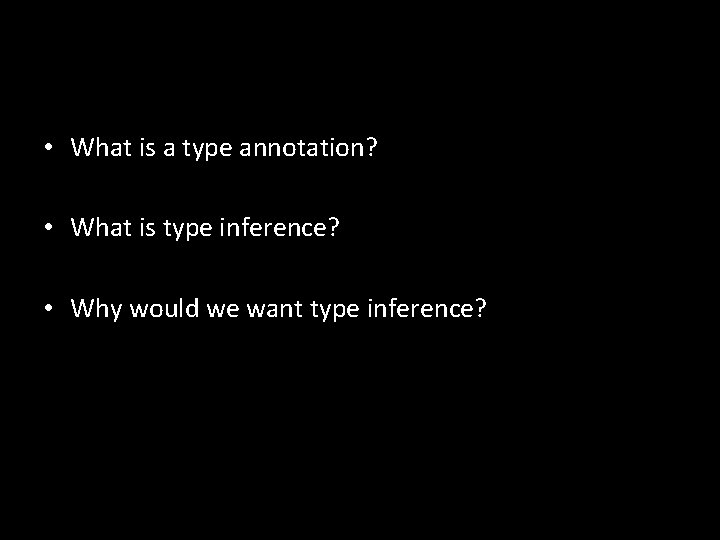
• What is a type annotation? • What is type inference? • Why would we want type inference?
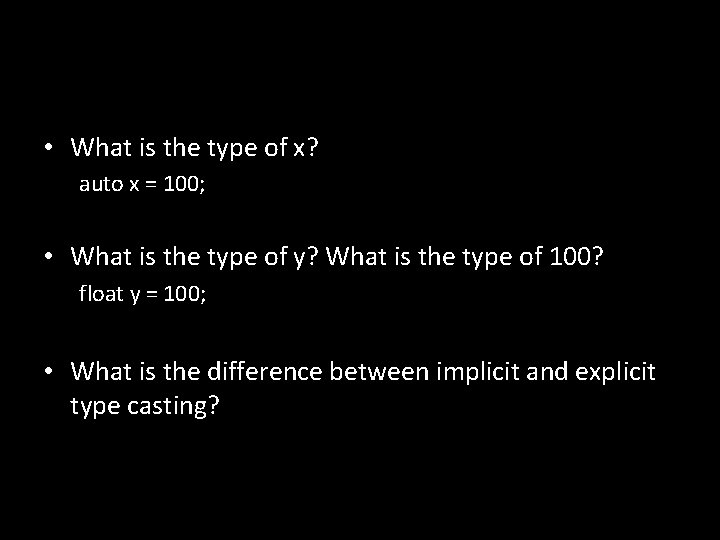
• What is the type of x? auto x = 100; • What is the type of y? What is the type of 100? float y = 100; • What is the difference between implicit and explicit type casting?
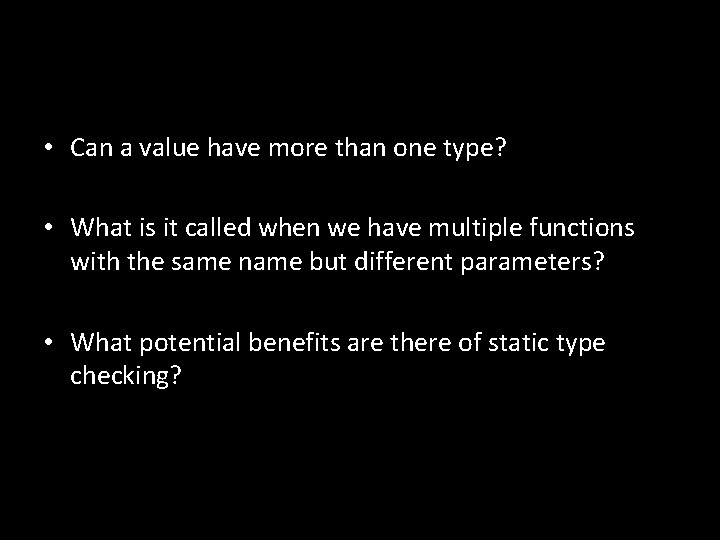
• Can a value have more than one type? • What is it called when we have multiple functions with the same name but different parameters? • What potential benefits are there of static type checking?
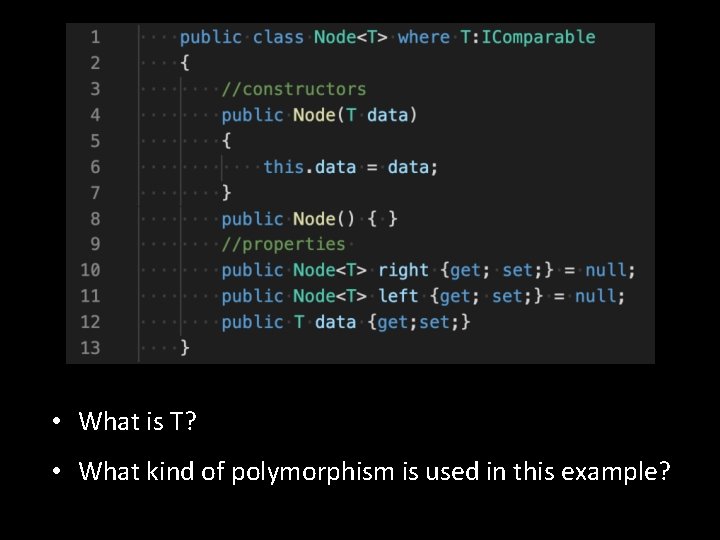
• What is T? • What kind of polymorphism is used in this example?