Tree What is a TREE Node Edge Path
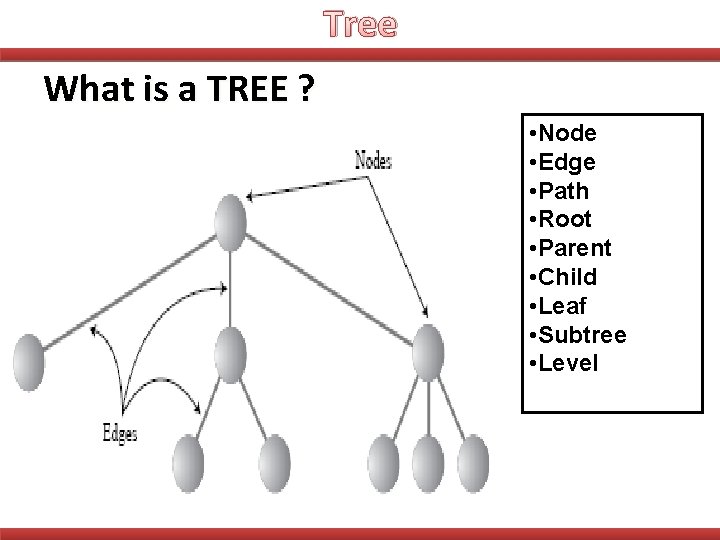
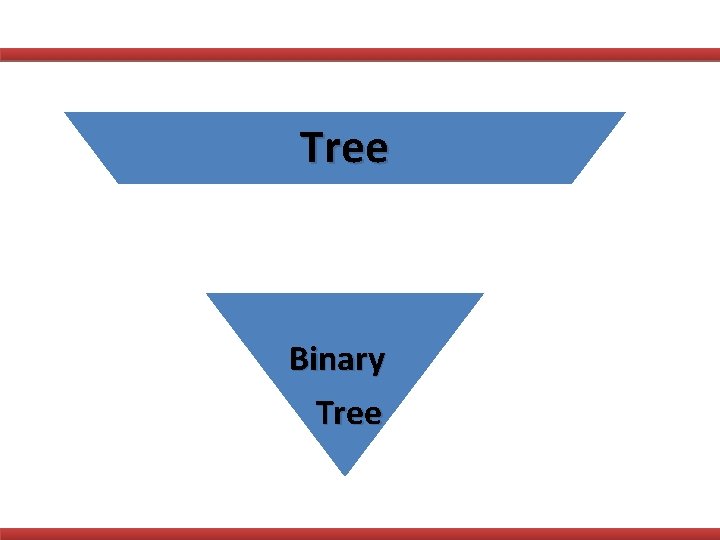
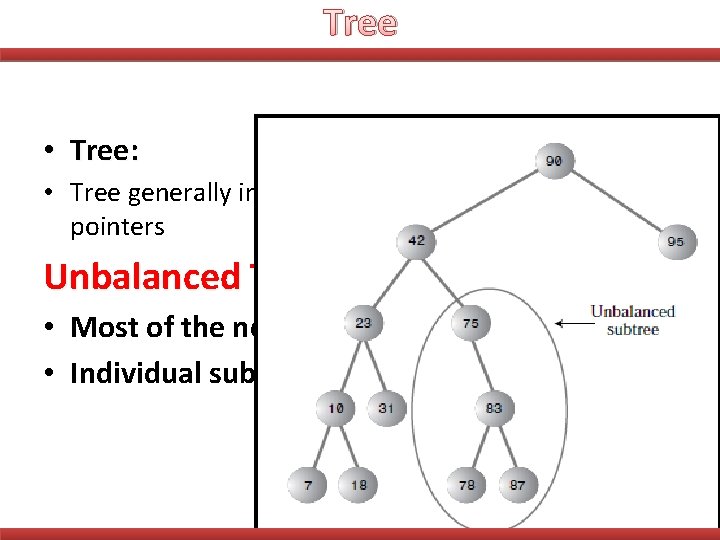
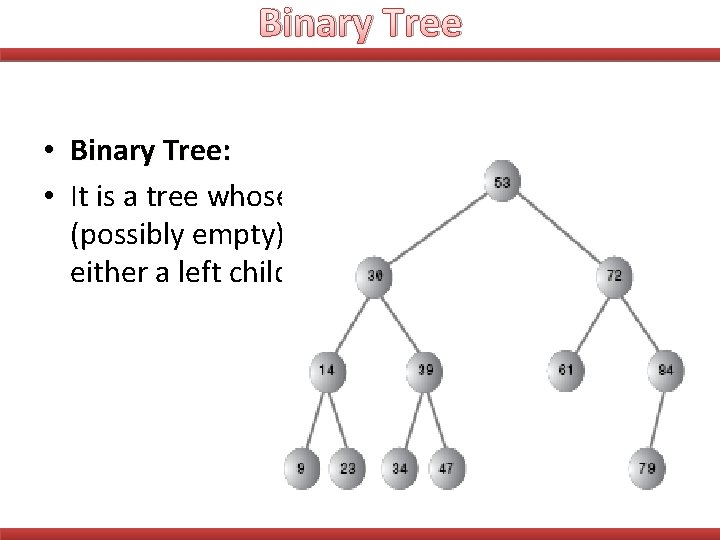
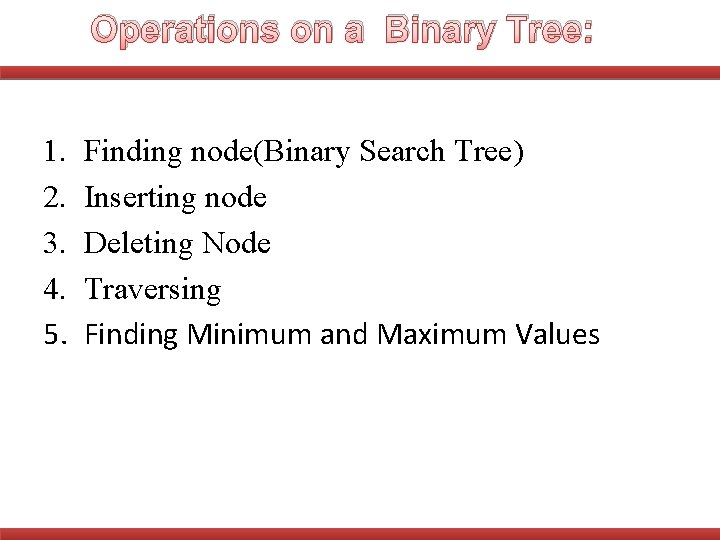
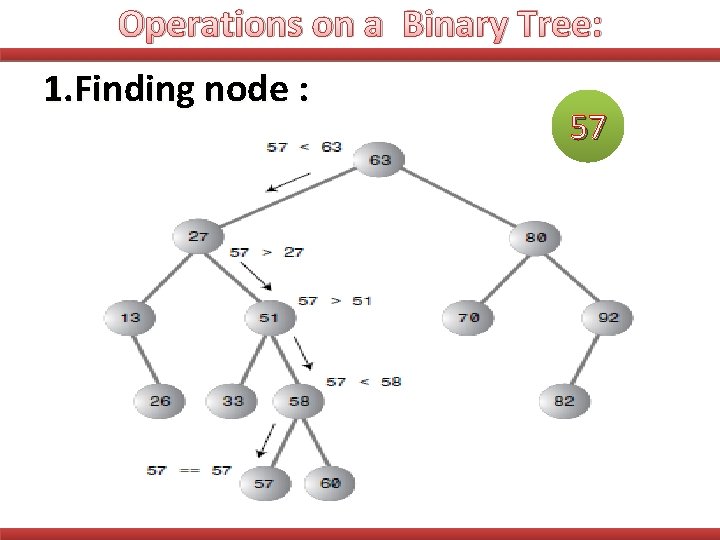
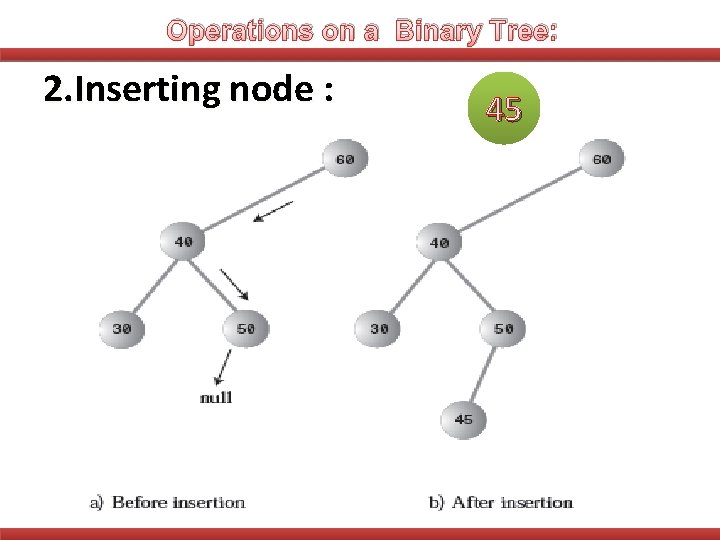
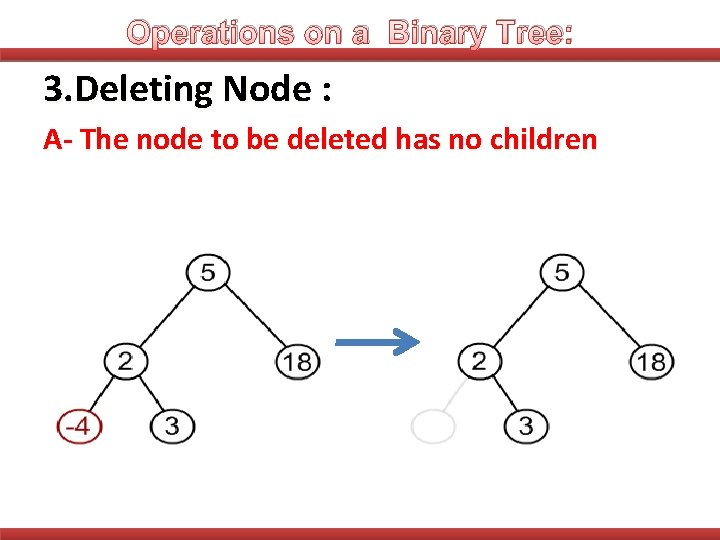
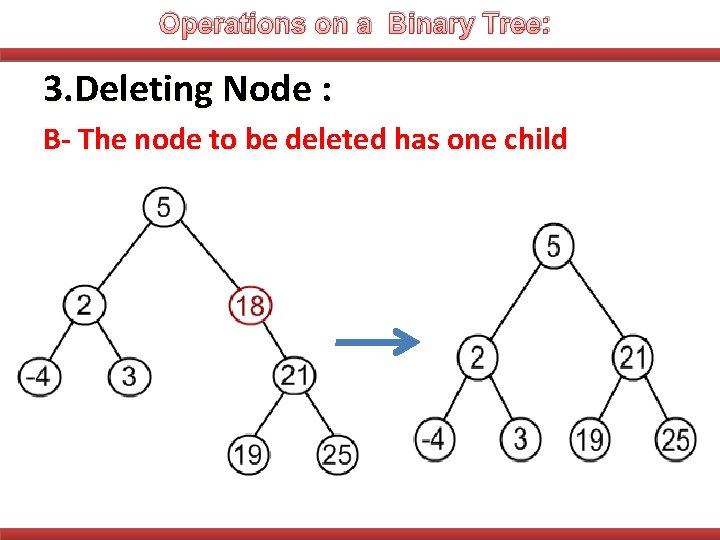
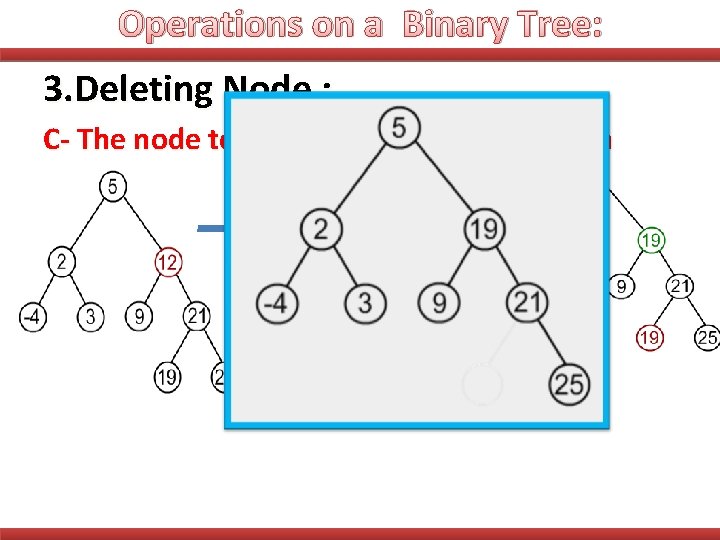
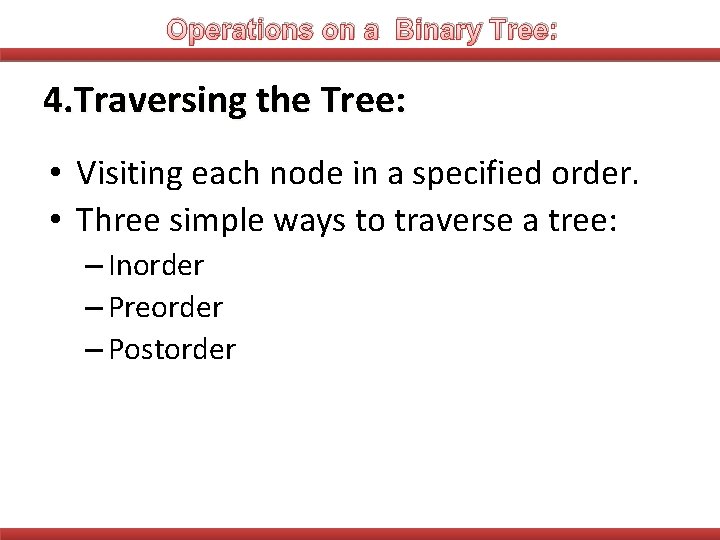
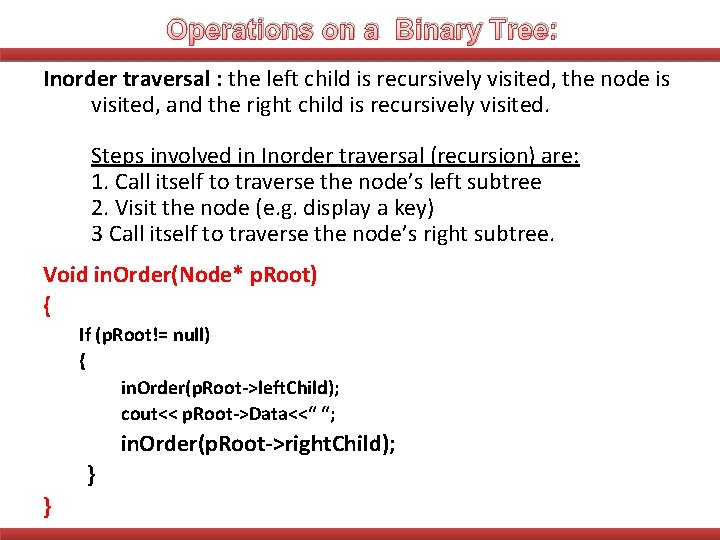
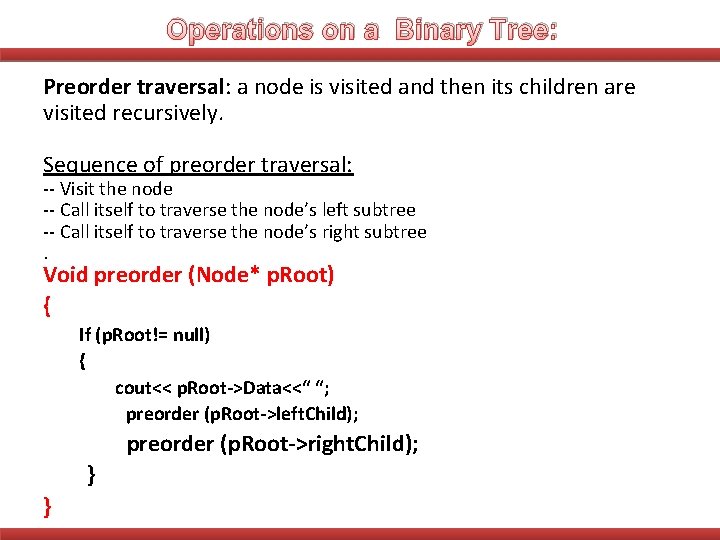
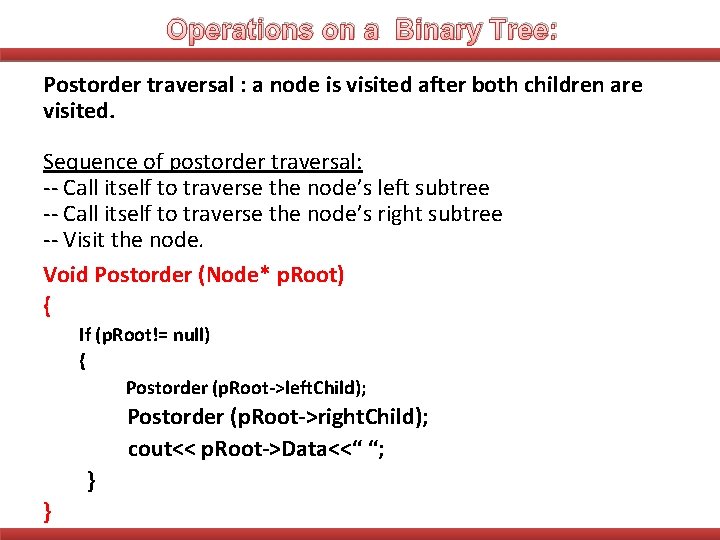
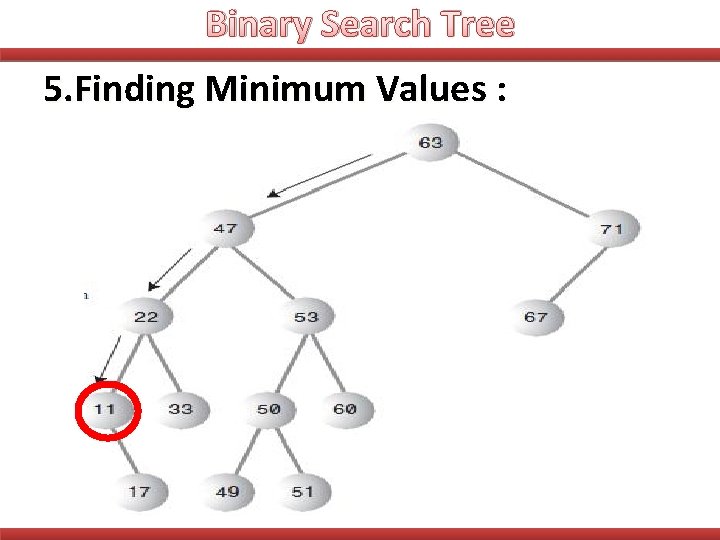
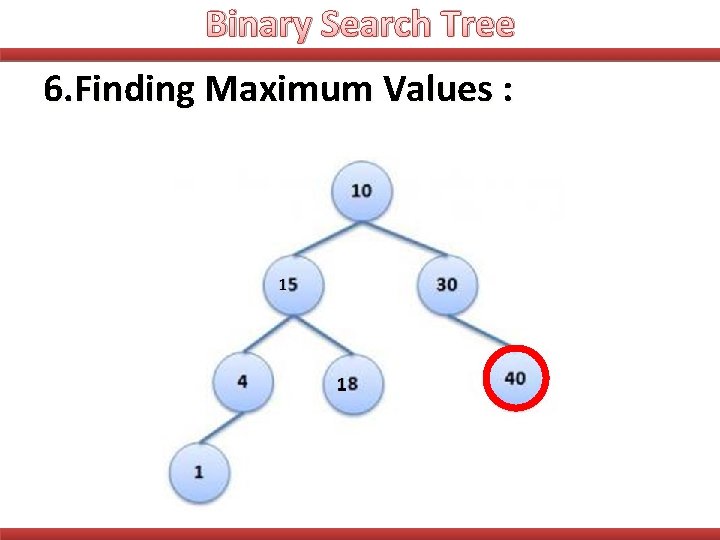
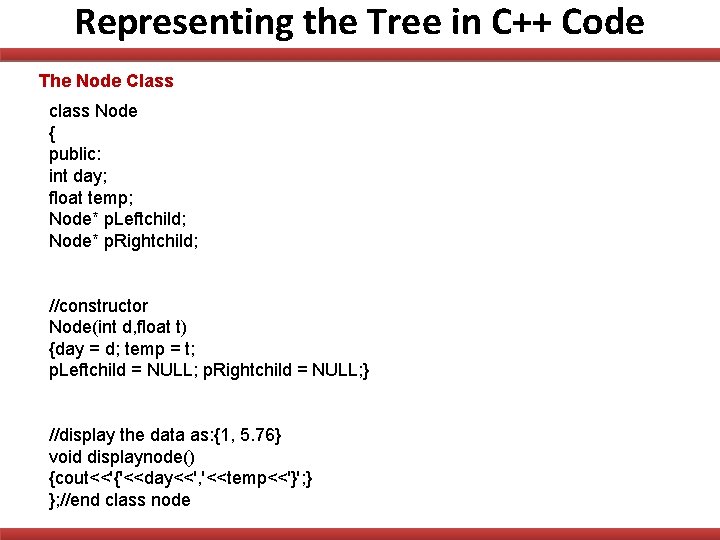
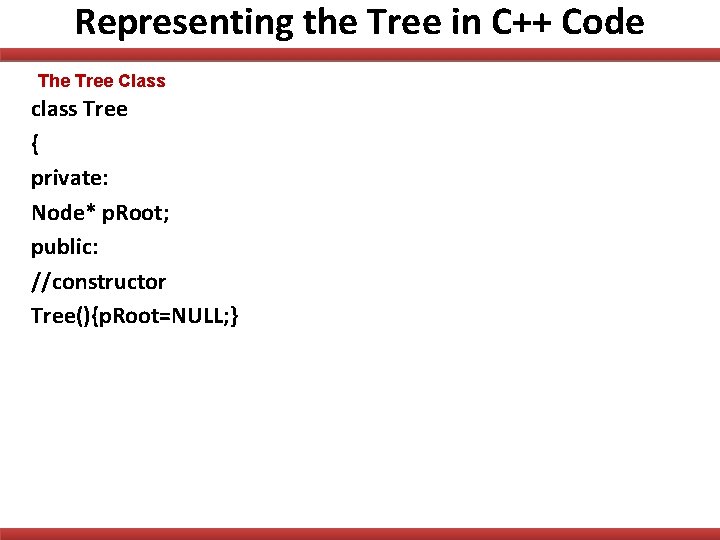
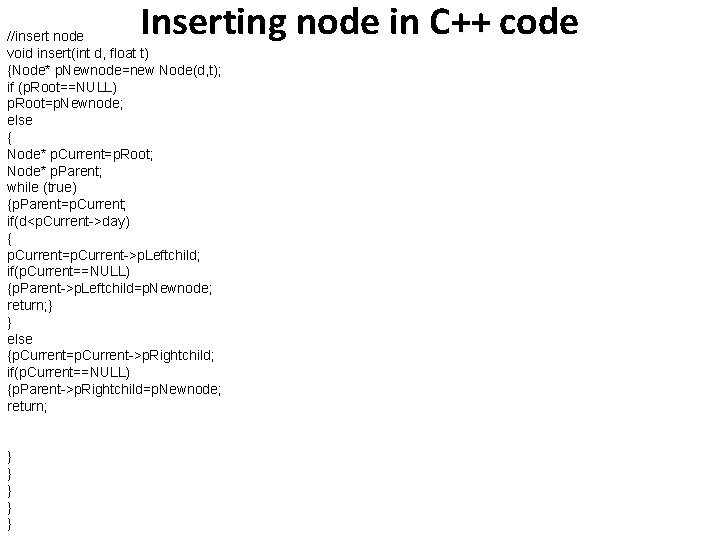
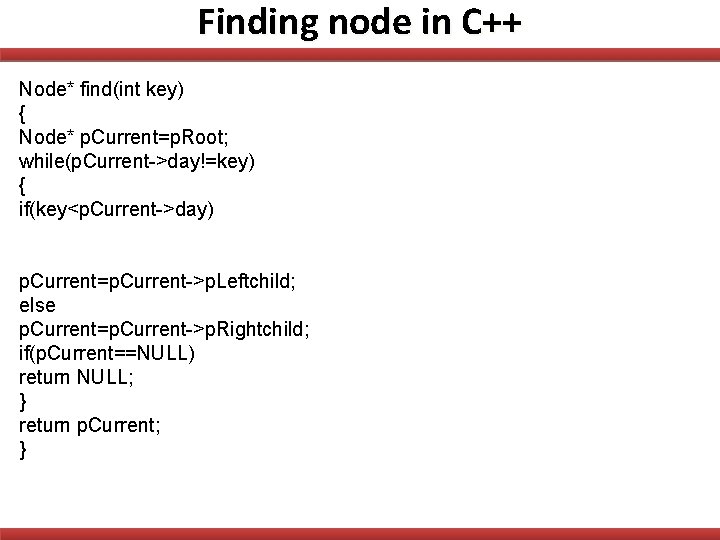
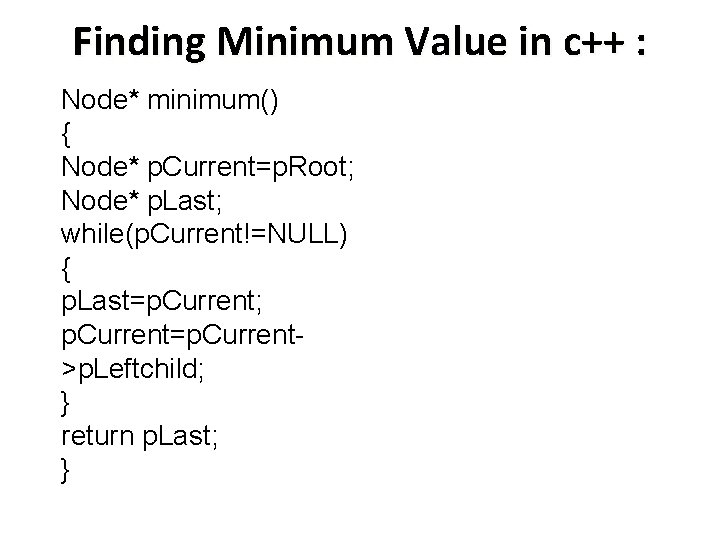
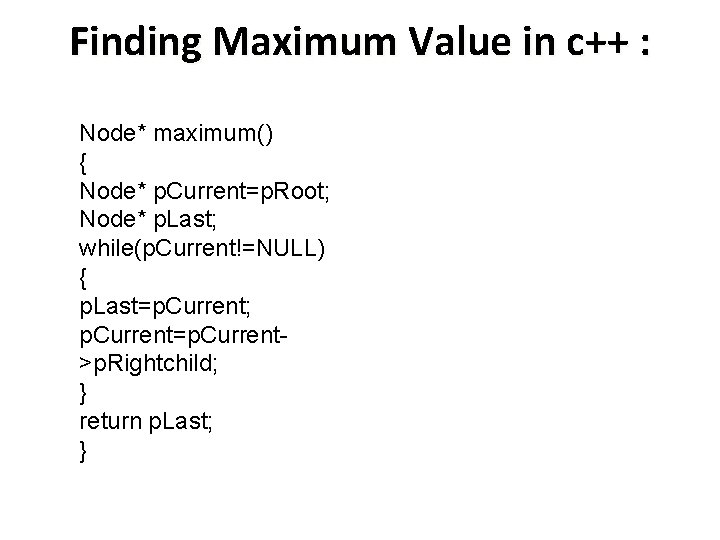
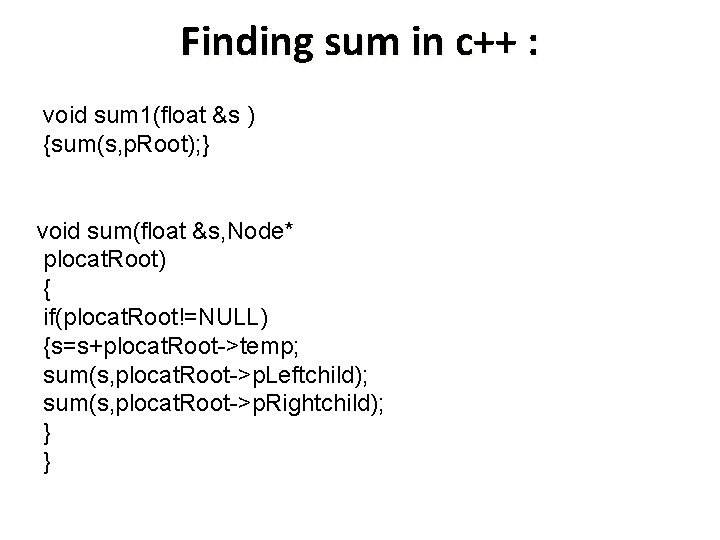
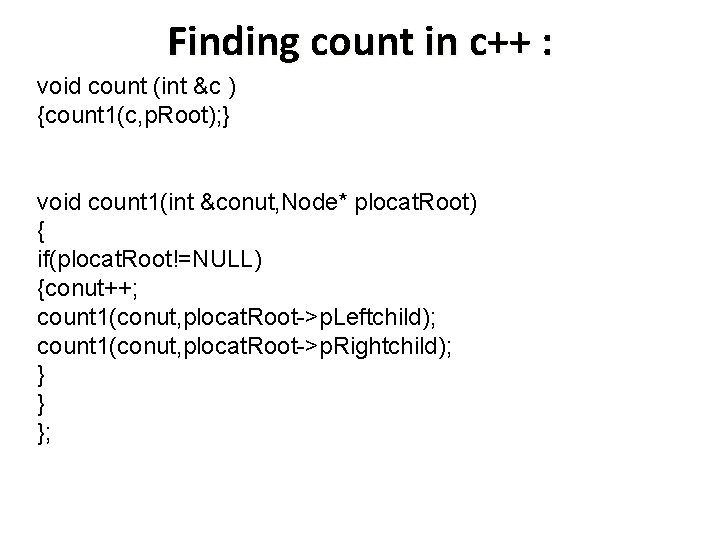
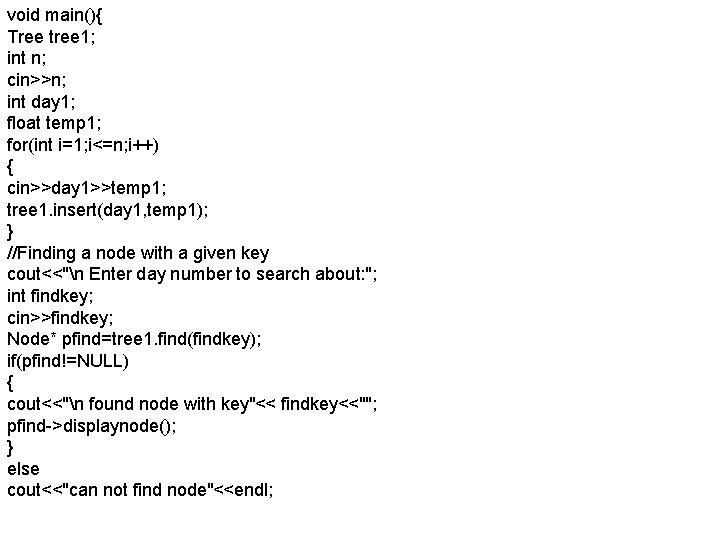
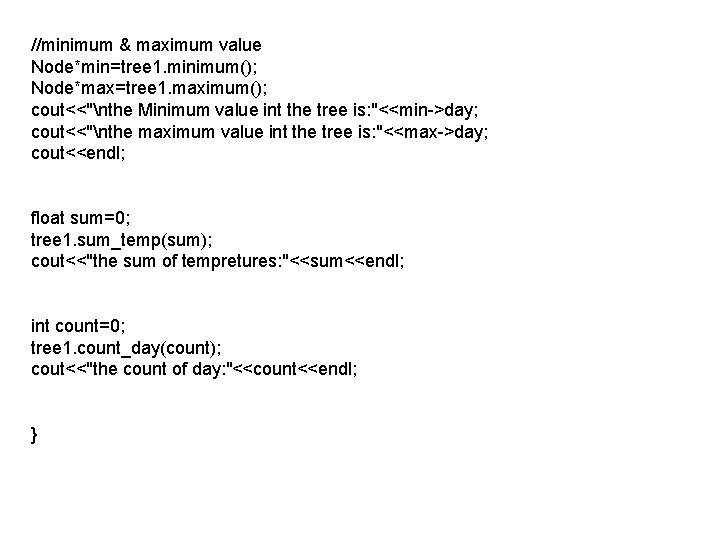
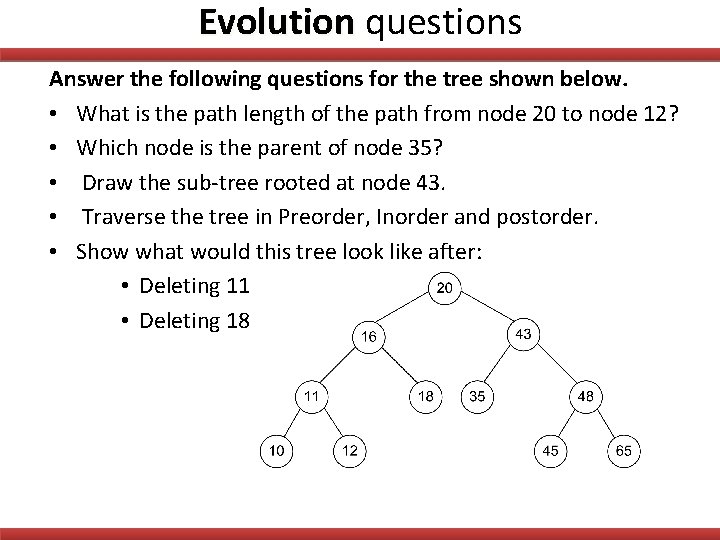
- Slides: 27
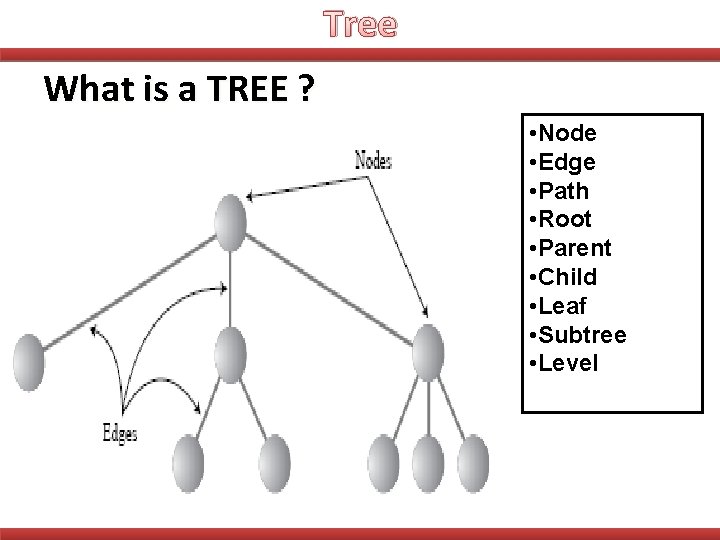
Tree What is a TREE ? • Node • Edge • Path • Root • Parent • Child • Leaf • Subtree • Level
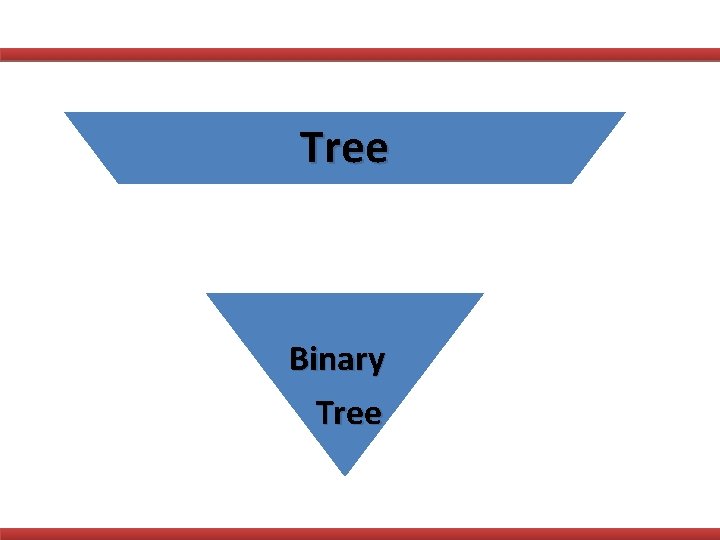
Tree Binary Tree
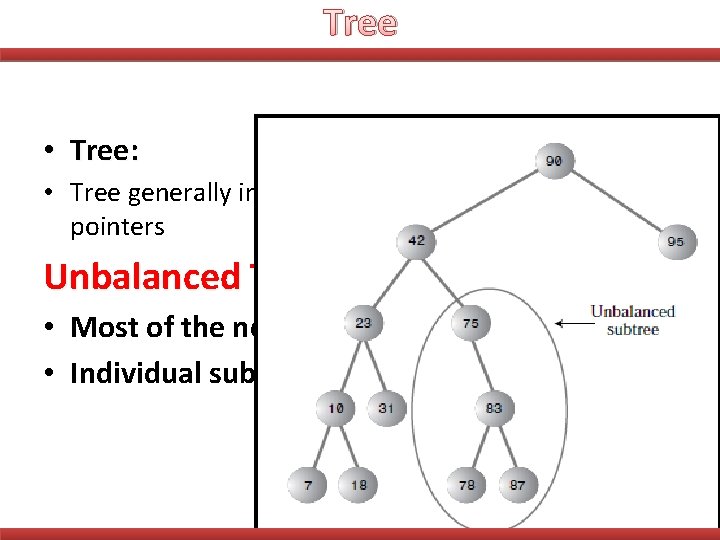
Tree • Tree: • Tree generally implemented in the computer using pointers Unbalanced Trees means that : • Most of the nodes are on one side of the root. • Individual subtrees may also be unbalanced.
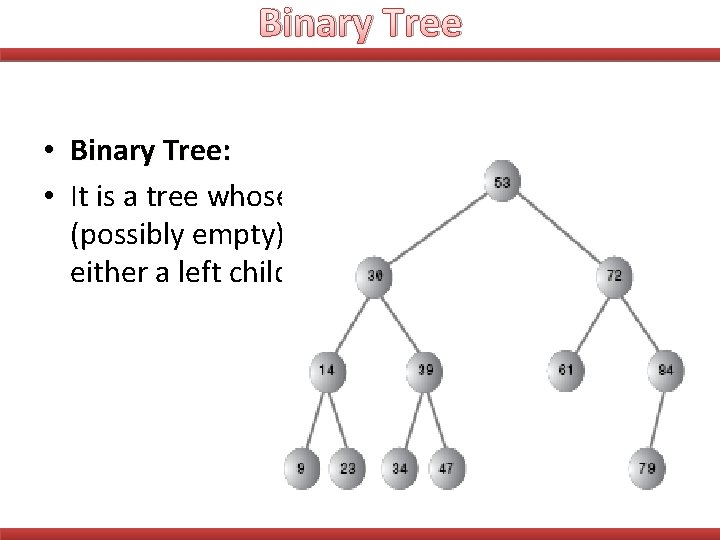
Binary Tree • Binary Tree: • It is a tree whose nodes have two children (possibly empty), and each child is designed as either a left child or a right child.
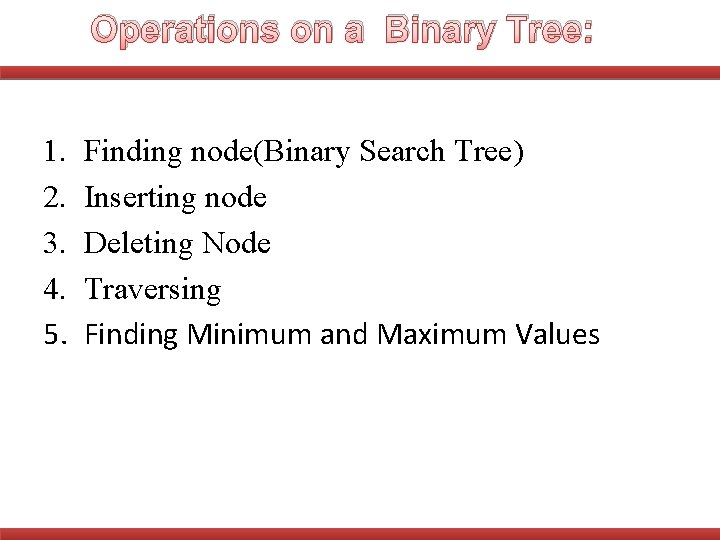
Operations on a Binary Tree: 1. 2. 3. 4. 5. Finding node(Binary Search Tree) Inserting node Deleting Node Traversing Finding Minimum and Maximum Values
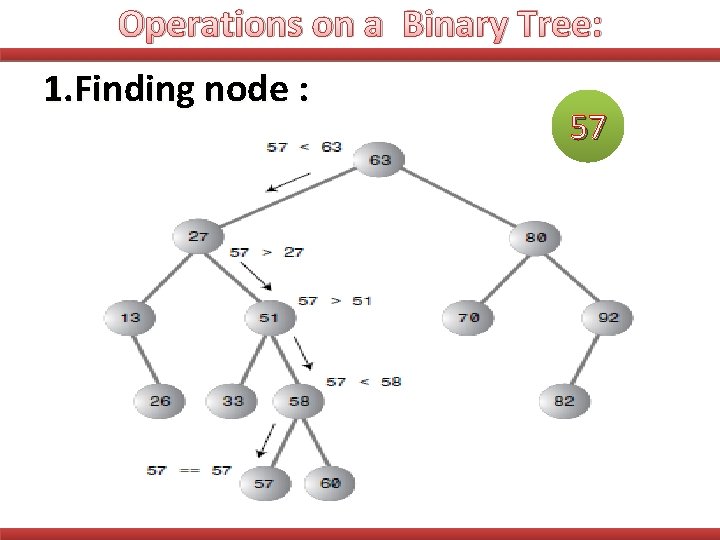
Operations on a Binary Tree: 1. Finding node : 57
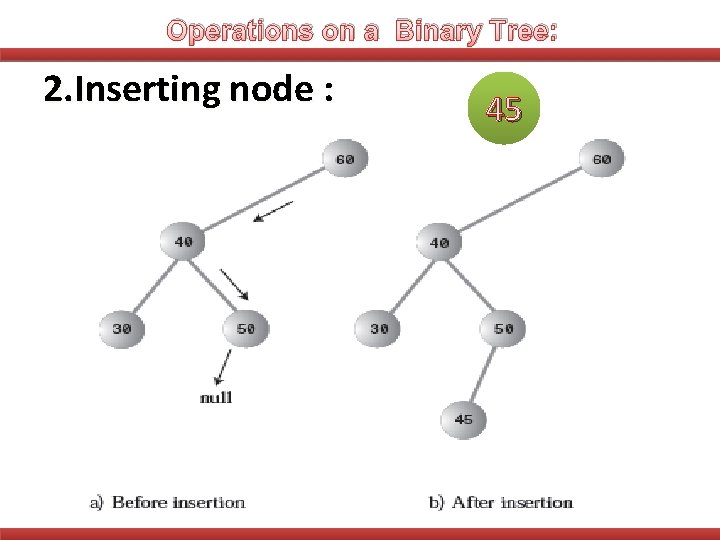
Operations on a Binary Tree: 2. Inserting node : 45
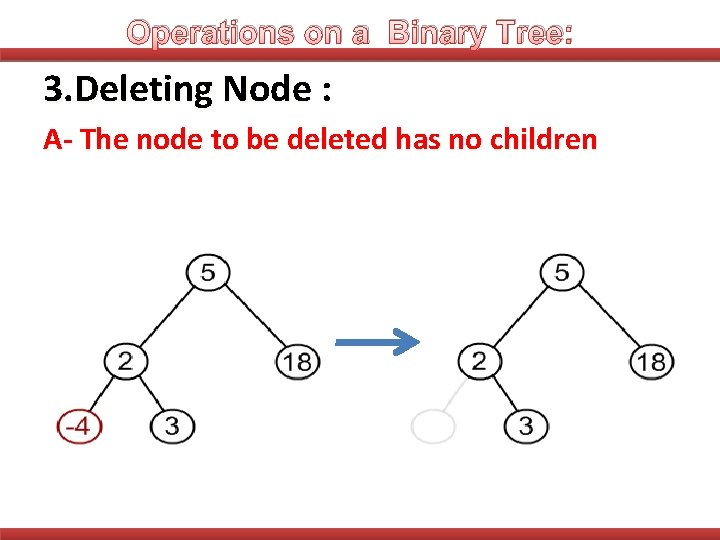
Operations on a Binary Tree: 3. Deleting Node : A- The node to be deleted has no children
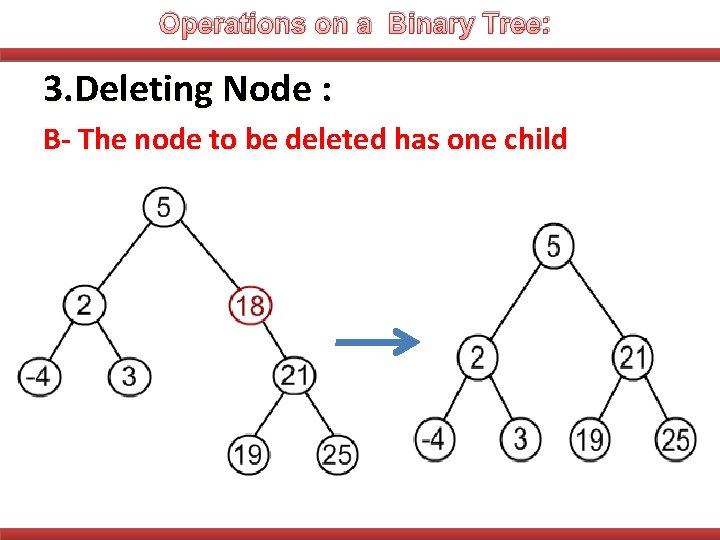
Operations on a Binary Tree: 3. Deleting Node : B- The node to be deleted has one child
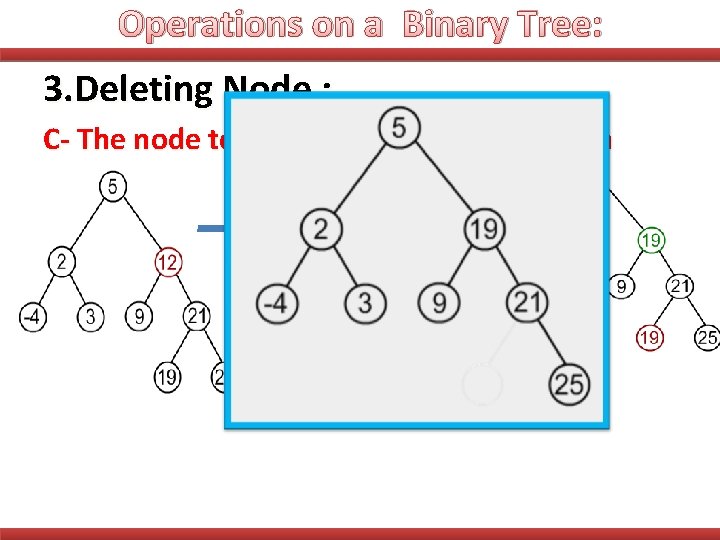
Operations on a Binary Tree: 3. Deleting Node : C- The node to be deleted has two children
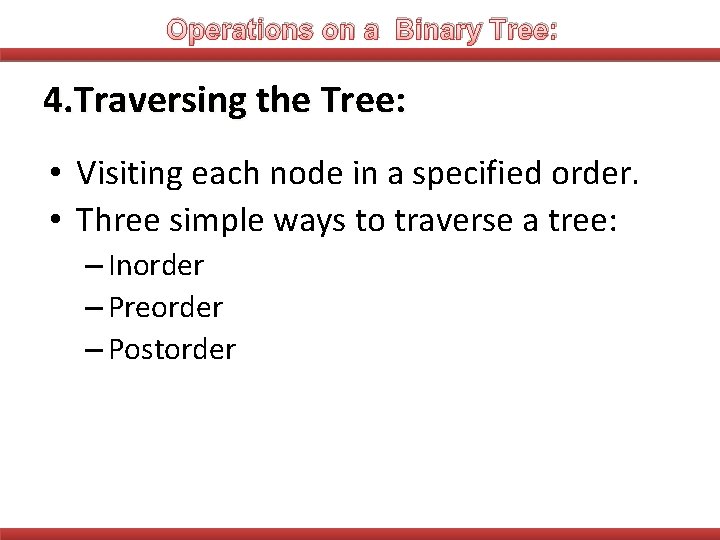
Operations on a Binary Tree: 4. Traversing the Tree: • Visiting each node in a specified order. • Three simple ways to traverse a tree: – Inorder – Preorder – Postorder
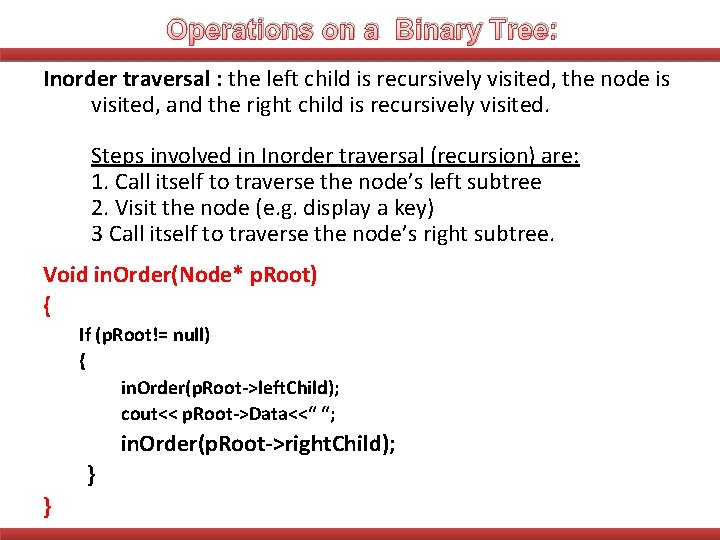
Operations on a Binary Tree: Inorder traversal : the left child is recursively visited, the node is visited, and the right child is recursively visited. Steps involved in Inorder traversal (recursion) are: 1. Call itself to traverse the node’s left subtree 2. Visit the node (e. g. display a key) 3 Call itself to traverse the node’s right subtree. Void in. Order(Node* p. Root) { If (p. Root!= null) { in. Order(p. Root->left. Child); cout<< p. Root->Data<<“ “; in. Order(p. Root->right. Child); } }
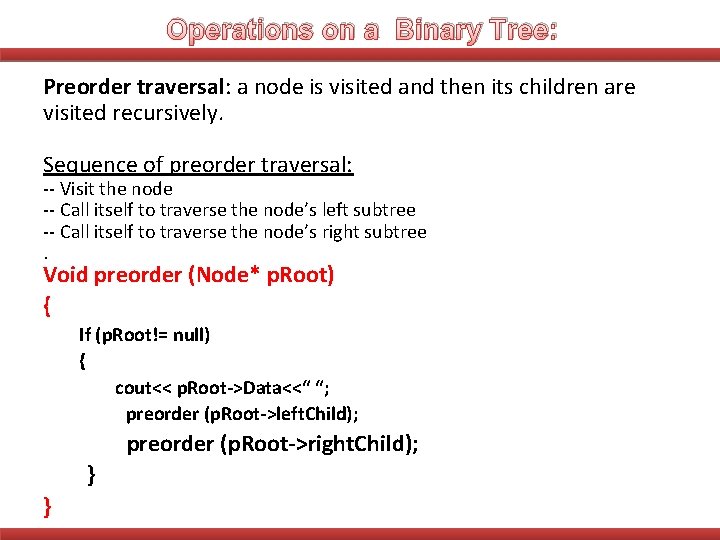
Operations on a Binary Tree: Preorder traversal: a node is visited and then its children are visited recursively. Sequence of preorder traversal: -- Visit the node -- Call itself to traverse the node’s left subtree -- Call itself to traverse the node’s right subtree. Void preorder (Node* p. Root) { If (p. Root!= null) { cout<< p. Root->Data<<“ “; preorder (p. Root->left. Child); preorder (p. Root->right. Child); } }
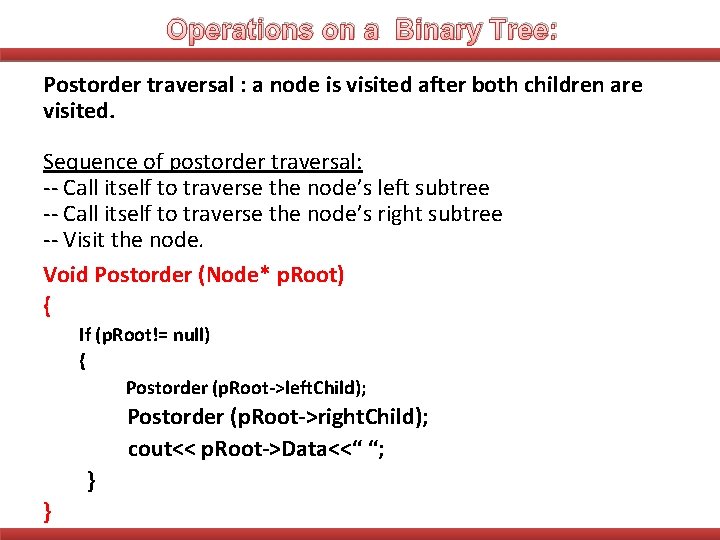
Operations on a Binary Tree: Postorder traversal : a node is visited after both children are visited. Sequence of postorder traversal: -- Call itself to traverse the node’s left subtree -- Call itself to traverse the node’s right subtree -- Visit the node. Void Postorder (Node* p. Root) { If (p. Root!= null) { Postorder (p. Root->left. Child); Postorder (p. Root->right. Child); cout<< p. Root->Data<<“ “; } }
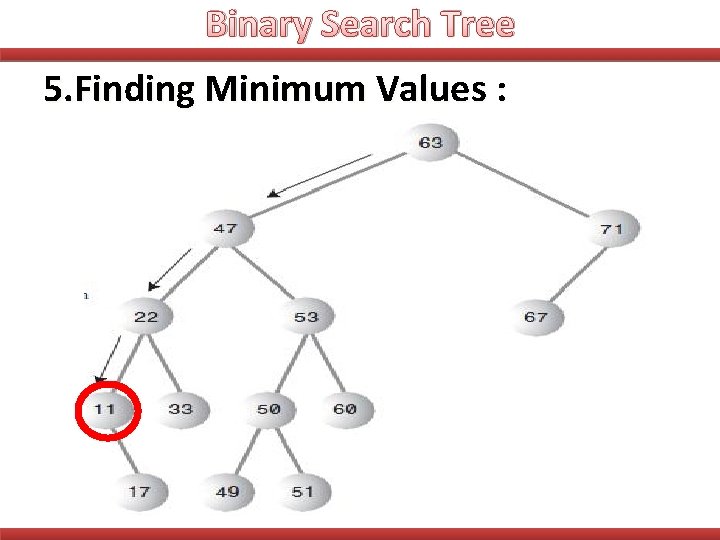
Binary Search Tree 5. Finding Minimum Values :
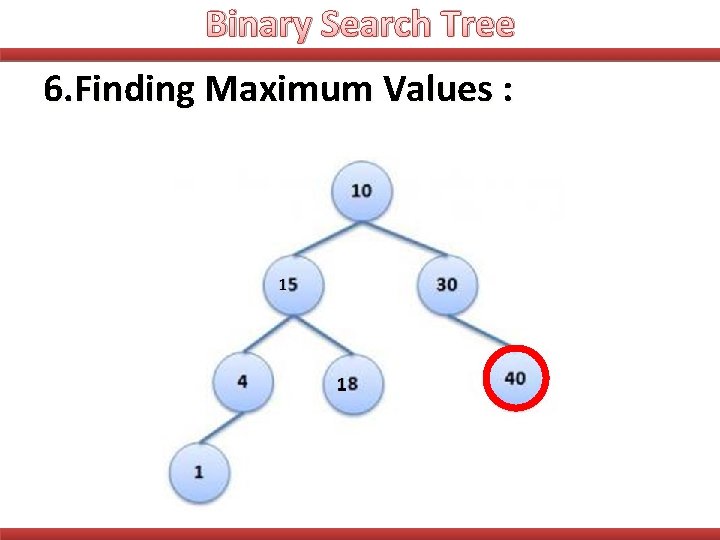
Binary Search Tree 6. Finding Maximum Values :
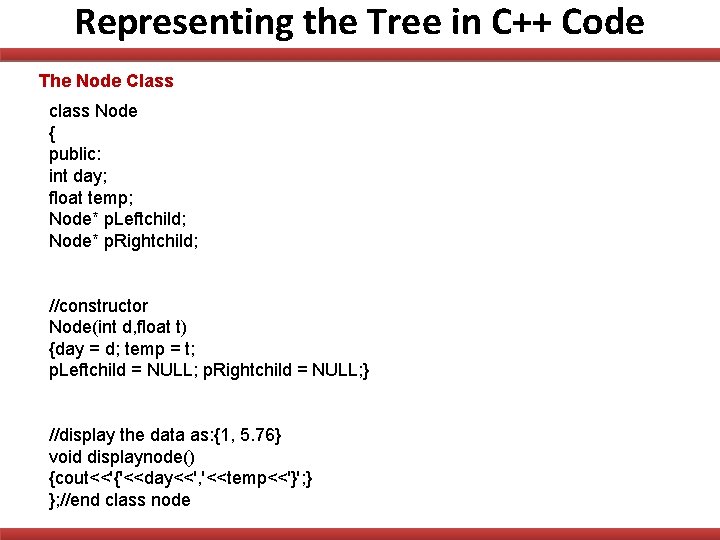
Representing the Tree in C++ Code The Node Class class Node { public: int day; float temp; Node* p. Leftchild; Node* p. Rightchild; //constructor Node(int d, float t) {day = d; temp = t; p. Leftchild = NULL; p. Rightchild = NULL; } //display the data as: {1, 5. 76} void displaynode() {cout<<'{'<<day<<', '<<temp<<'}'; } }; //end class node
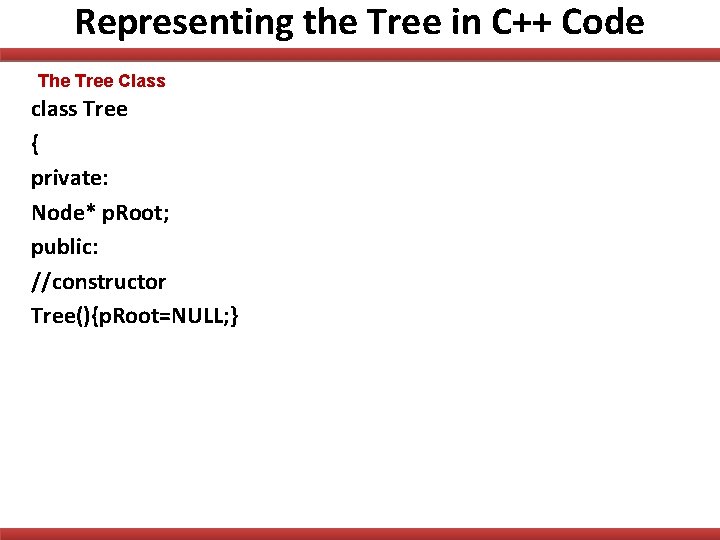
Representing the Tree in C++ Code The Tree Class class Tree { private: Node* p. Root; public: //constructor Tree(){p. Root=NULL; }
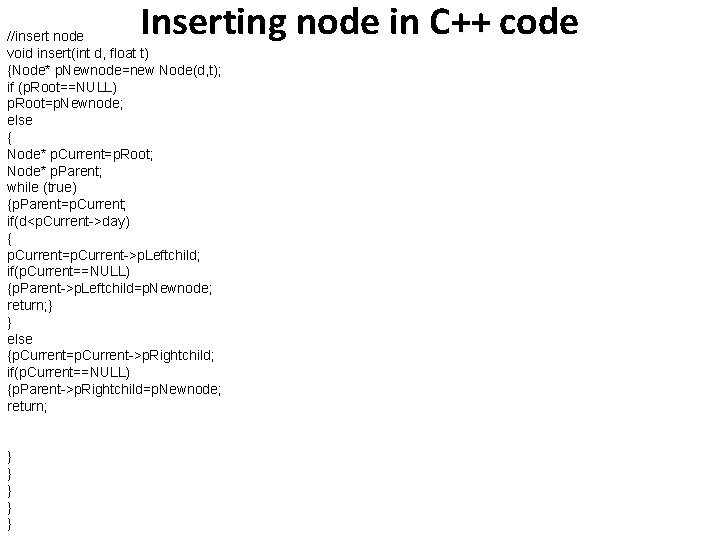
Inserting node in C++ code //insert node void insert(int d, float t) {Node* p. Newnode=new Node(d, t); if (p. Root==NULL) p. Root=p. Newnode; else { Node* p. Current=p. Root; Node* p. Parent; while (true) {p. Parent=p. Current; if(d<p. Current->day) { p. Current=p. Current->p. Leftchild; if(p. Current==NULL) {p. Parent->p. Leftchild=p. Newnode; return; } } else {p. Current=p. Current->p. Rightchild; if(p. Current==NULL) {p. Parent->p. Rightchild=p. Newnode; return; } } }
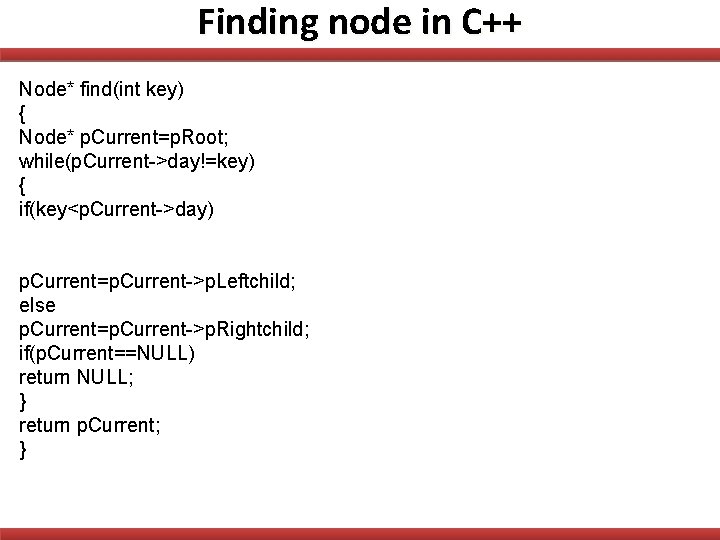
Finding node in C++ Node* find(int key) { Node* p. Current=p. Root; while(p. Current->day!=key) { if(key<p. Current->day) p. Current=p. Current->p. Leftchild; else p. Current=p. Current->p. Rightchild; if(p. Current==NULL) return NULL; } return p. Current; }
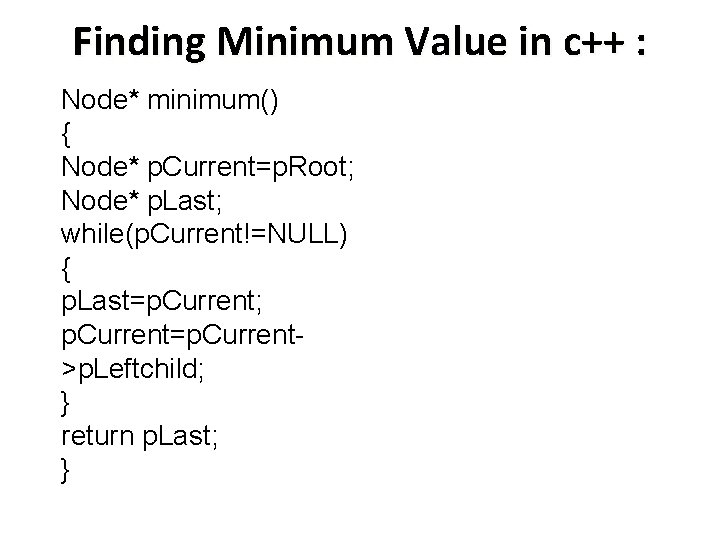
Finding Minimum Value in c++ : Node* minimum() { Node* p. Current=p. Root; Node* p. Last; while(p. Current!=NULL) { p. Last=p. Current; p. Current=p. Current>p. Leftchild; } return p. Last; }
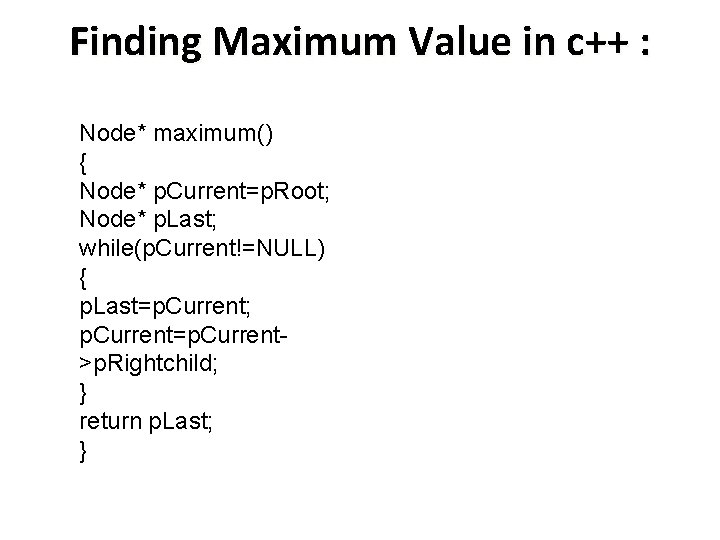
Finding Maximum Value in c++ : Node* maximum() { Node* p. Current=p. Root; Node* p. Last; while(p. Current!=NULL) { p. Last=p. Current; p. Current=p. Current>p. Rightchild; } return p. Last; }
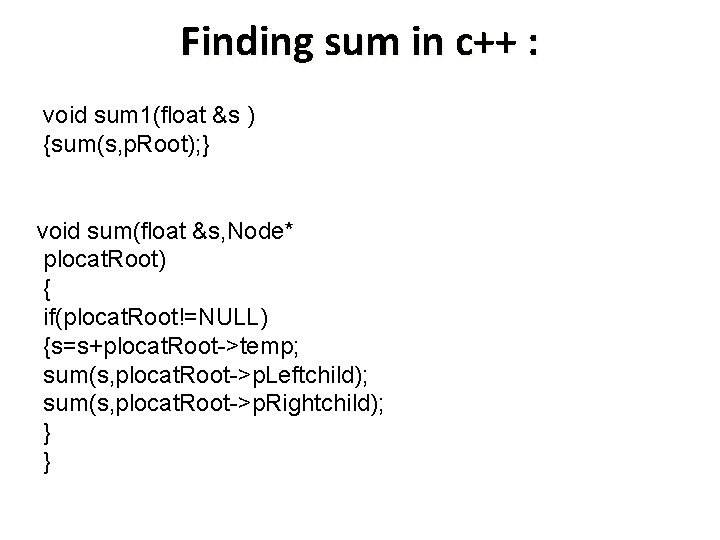
Finding sum in c++ : void sum 1(float &s ) {sum(s, p. Root); } void sum(float &s, Node* plocat. Root) { if(plocat. Root!=NULL) {s=s+plocat. Root->temp; sum(s, plocat. Root->p. Leftchild); sum(s, plocat. Root->p. Rightchild); } }
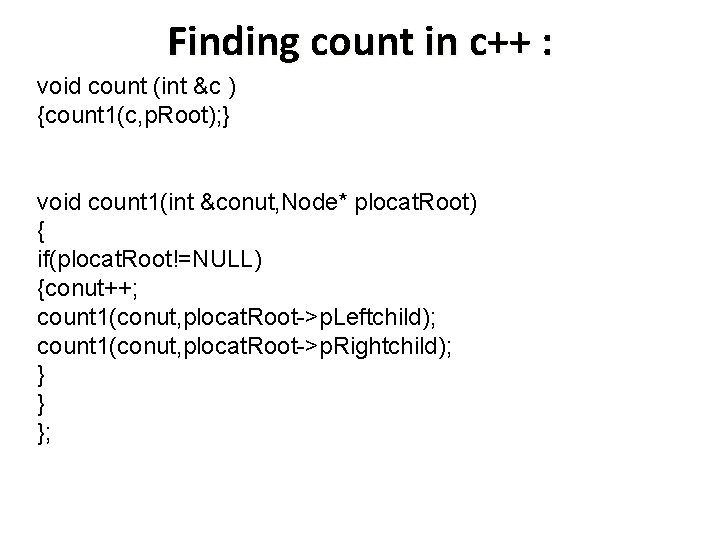
Finding count in c++ : void count (int &c ) {count 1(c, p. Root); } void count 1(int &conut, Node* plocat. Root) { if(plocat. Root!=NULL) {conut++; count 1(conut, plocat. Root->p. Leftchild); count 1(conut, plocat. Root->p. Rightchild); } } };
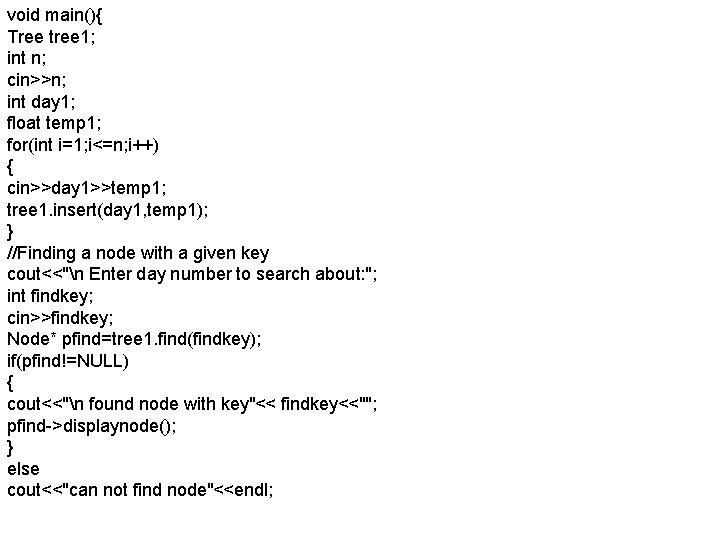
void main(){ Tree tree 1; int n; cin>>n; int day 1; float temp 1; for(int i=1; i<=n; i++) { cin>>day 1>>temp 1; tree 1. insert(day 1, temp 1); } //Finding a node with a given key cout<<"n Enter day number to search about: "; int findkey; cin>>findkey; Node* pfind=tree 1. find(findkey); if(pfind!=NULL) { cout<<"n found node with key"<< findkey<<""; pfind->displaynode(); } else cout<<"can not find node"<<endl;
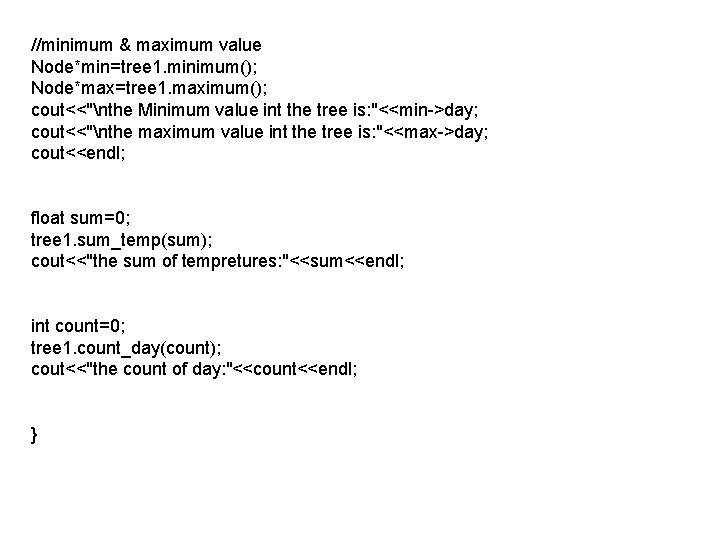
//minimum & maximum value Node*min=tree 1. minimum(); Node*max=tree 1. maximum(); cout<<"nthe Minimum value int the tree is: "<<min->day; cout<<"nthe maximum value int the tree is: "<<max->day; cout<<endl; float sum=0; tree 1. sum_temp(sum); cout<<"the sum of tempretures: "<<sum<<endl; int count=0; tree 1. count_day(count); cout<<"the count of day: "<<count<<endl; }
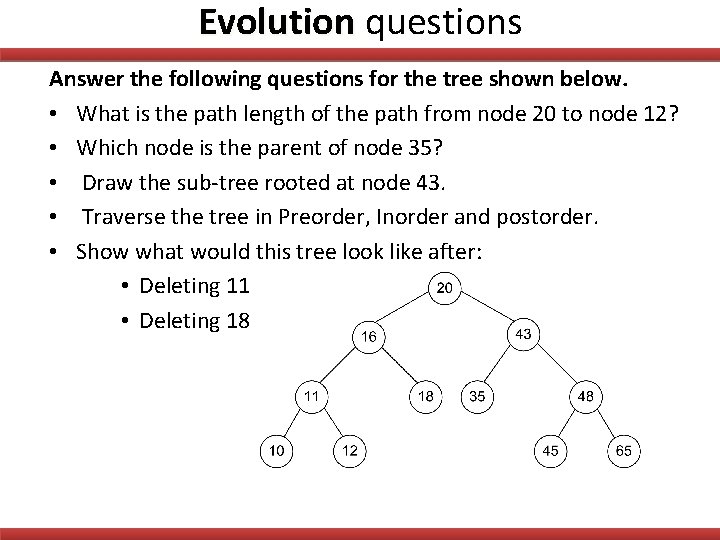
Evolution questions Answer the following questions for the tree shown below. • What is the path length of the path from node 20 to node 12? • Which node is the parent of node 35? • Draw the sub-tree rooted at node 43. • Traverse the tree in Preorder, Inorder and postorder. • Show what would this tree look like after: • Deleting 11 • Deleting 18
Typedef struct node
Reference node and non reference node
Parallel spin
Reference node and non reference node
Struct node int data struct node* next
Wavelength constructive interference
Rising edge and falling edge
Unity signalr
Minimum spanning tree shortest path
Typedef struct tree int info
Av node delay
Root node
Explicit architecture
Node lookup in peer to peer network
Internal node
Typedef struct tree int info
Example 3
Sentinel node
Node has no position networkx
Node of ranvier channels
Struct node int data
Node analysis
Nodered https
Lon mng
Number needed to harm
Document 意味
Local lymph node assay
Node xl