Topics q String Constructors q String Methods Strings
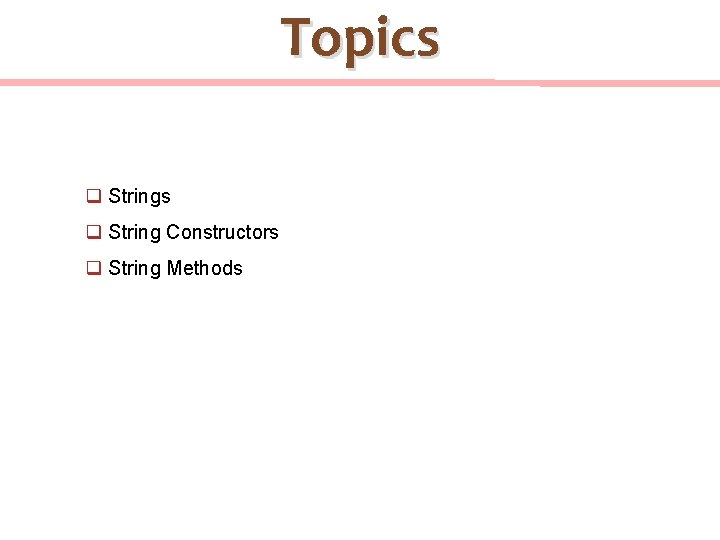
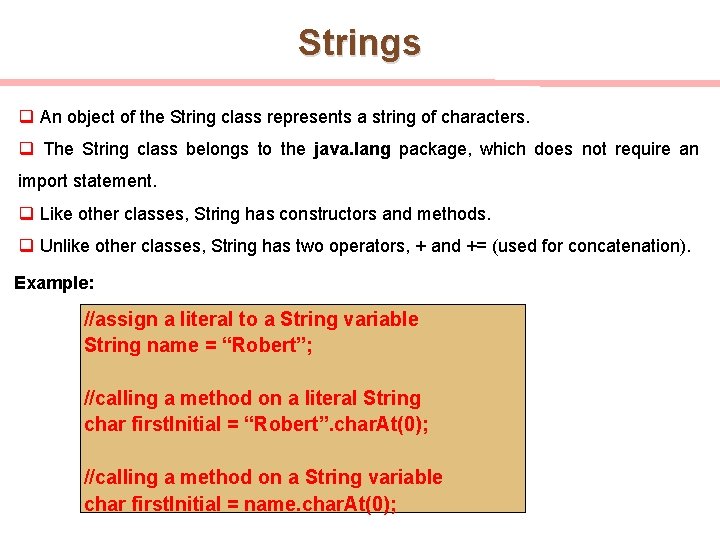
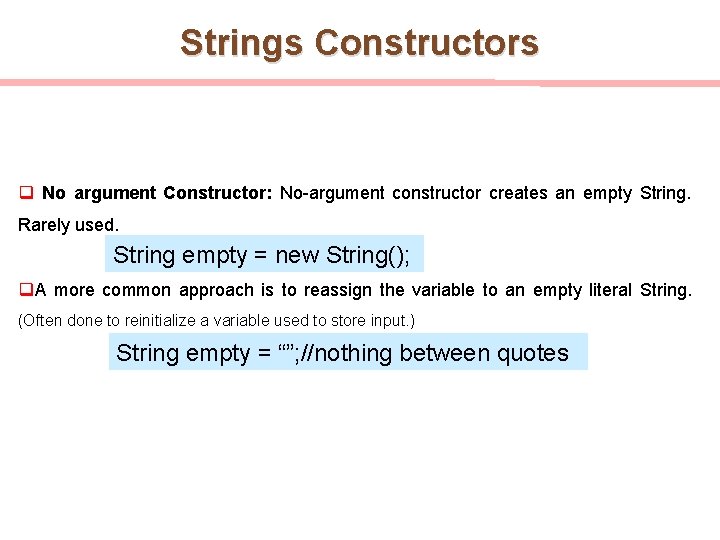
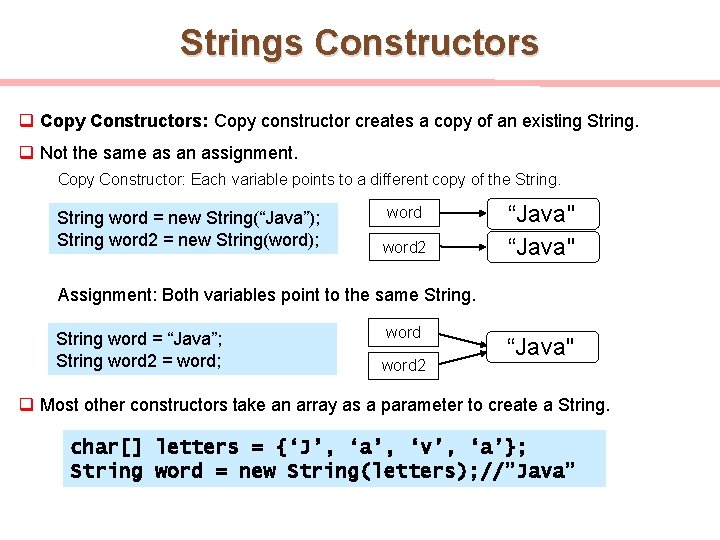
![Strings Constructors q For Example: char[ ] char. Array = {‘b’, ‘i’, ‘r’, ‘t’, Strings Constructors q For Example: char[ ] char. Array = {‘b’, ‘i’, ‘r’, ‘t’,](https://slidetodoc.com/presentation_image/37d46827bc57494a05dfd51adbd2464b/image-5.jpg)
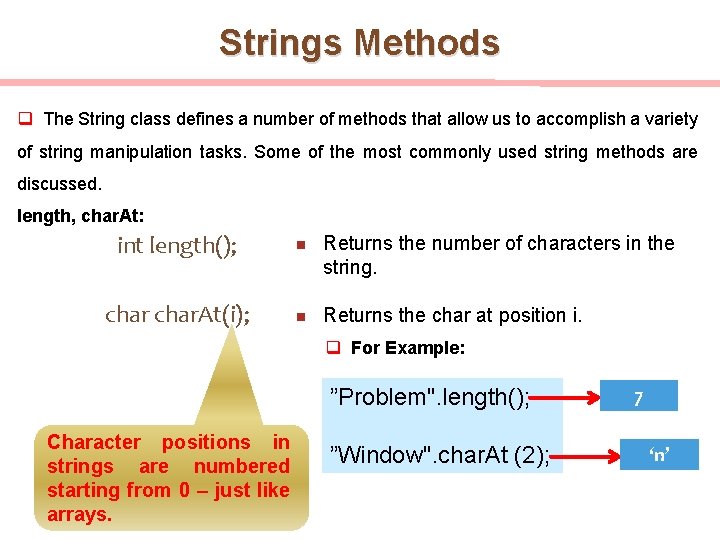
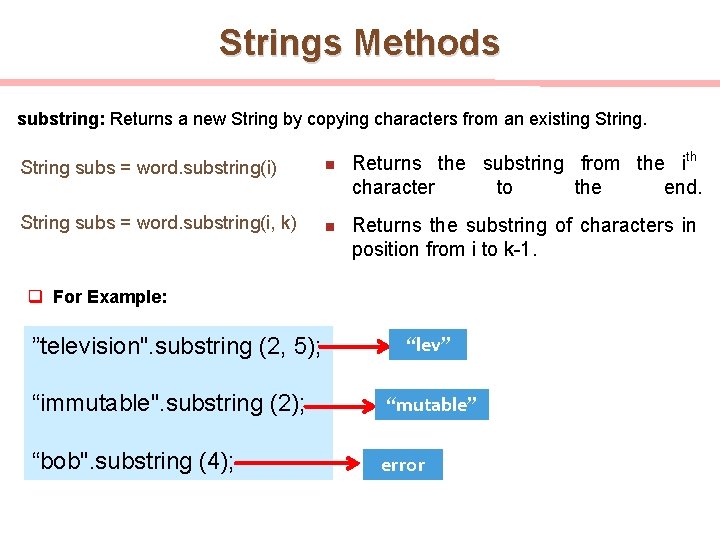
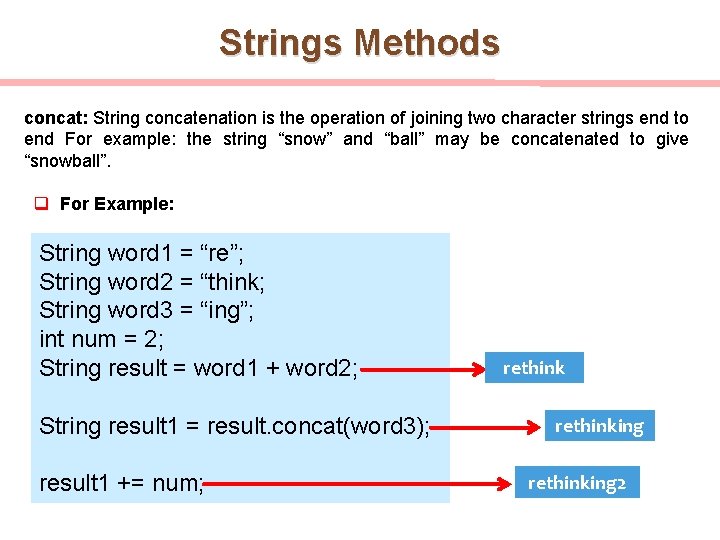
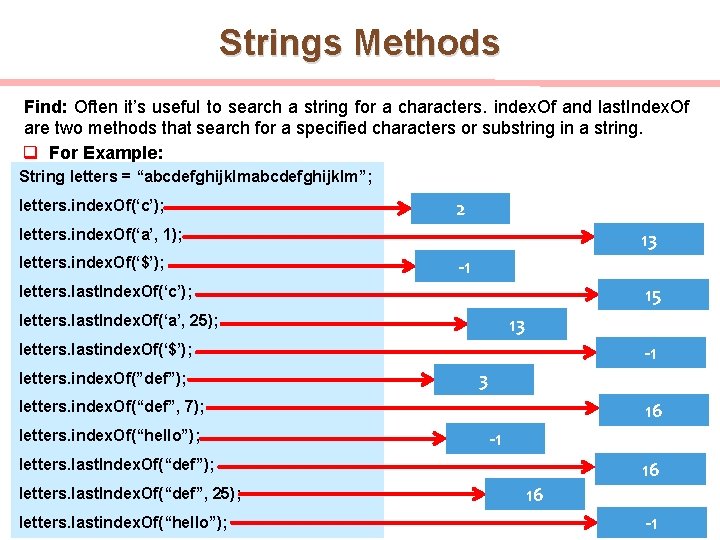
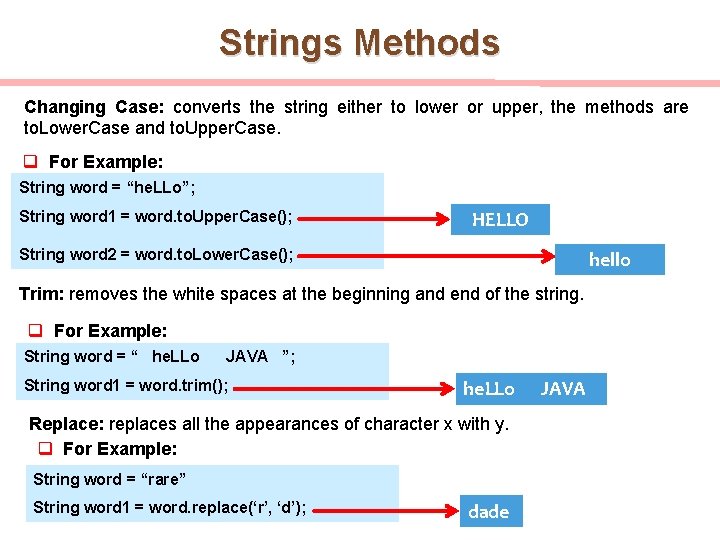
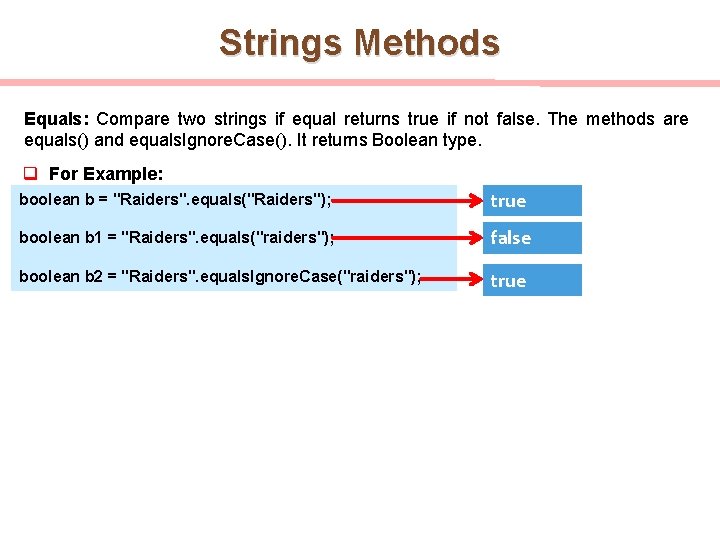
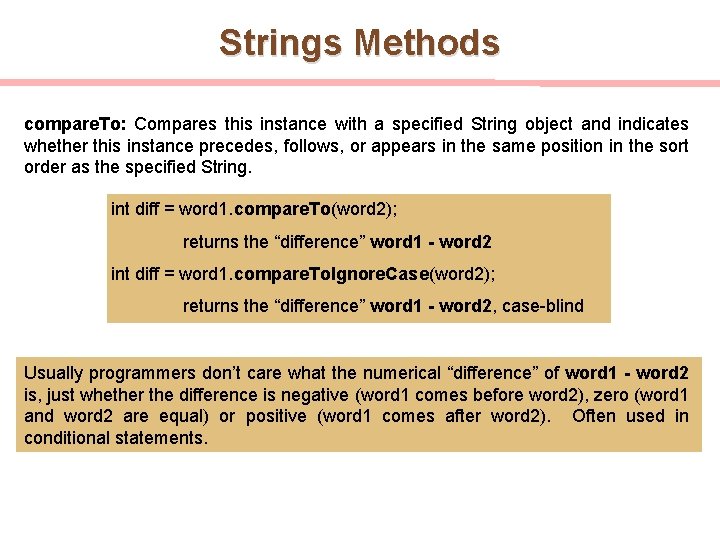
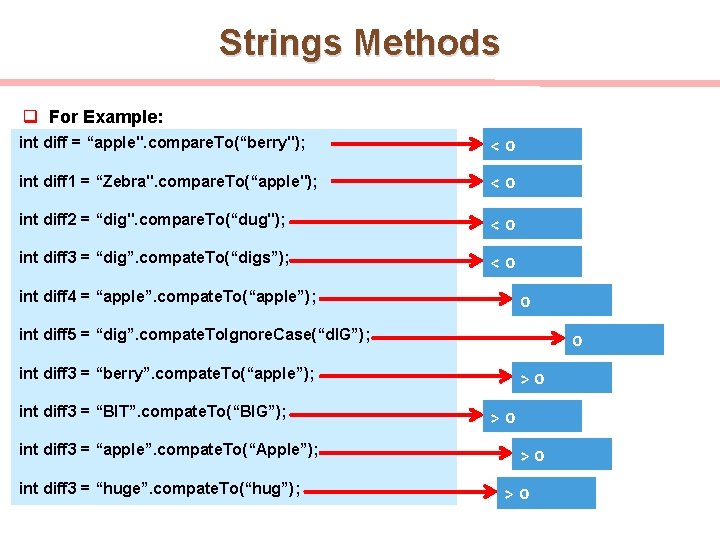
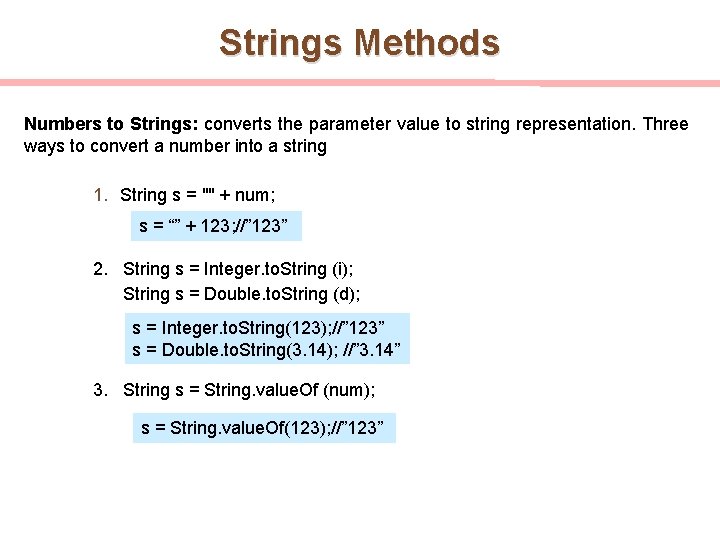
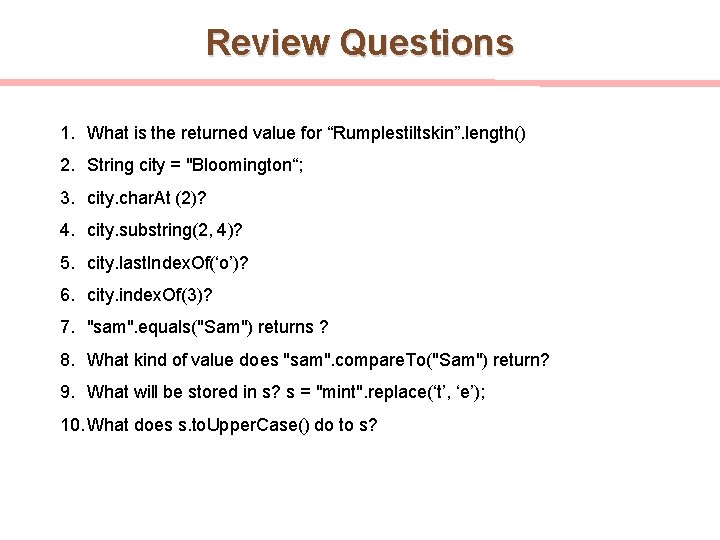
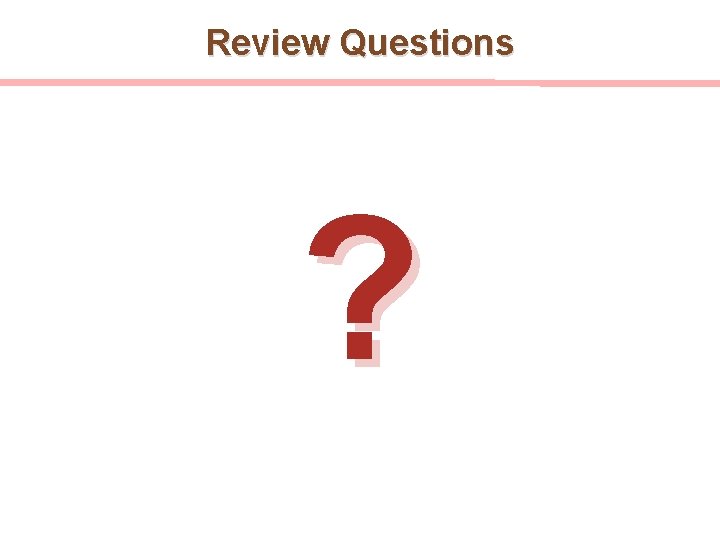
- Slides: 16
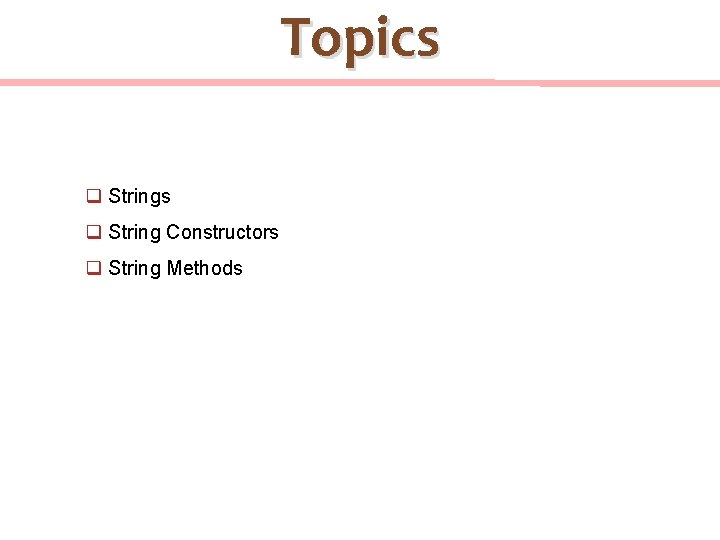
Topics q String Constructors q String Methods
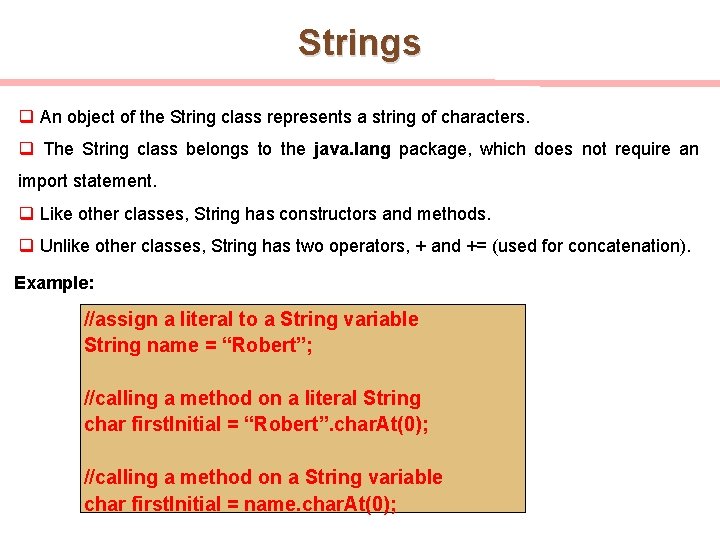
Strings q An object of the String class represents a string of characters. q The String class belongs to the java. lang package, which does not require an import statement. q Like other classes, String has constructors and methods. q Unlike other classes, String has two operators, + and += (used for concatenation). Example: //assign a literal to a String variable String name = “Robert”; //calling a method on a literal String char first. Initial = “Robert”. char. At(0); //calling a method on a String variable char first. Initial = name. char. At(0);
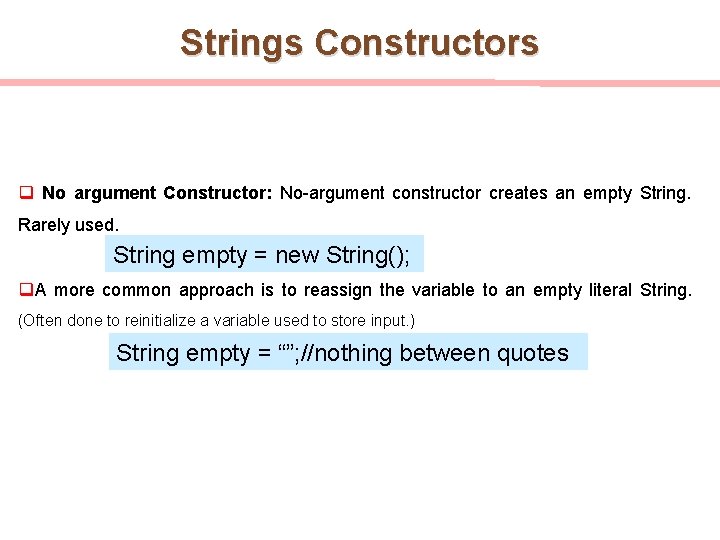
Strings Constructors q No argument Constructor: No-argument constructor creates an empty String. Rarely used. String empty = new String(); q. A more common approach is to reassign the variable to an empty literal String. (Often done to reinitialize a variable used to store input. ) String empty = “”; //nothing between quotes
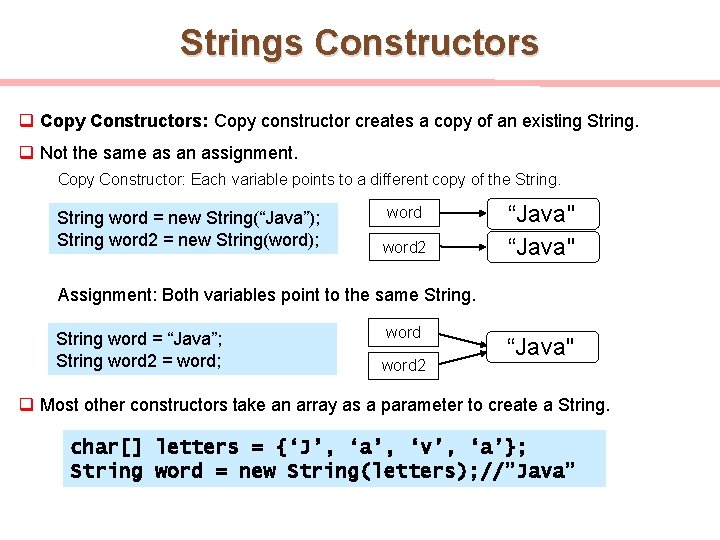
Strings Constructors q Copy Constructors: Copy constructor creates a copy of an existing String. q Not the same as an assignment. Copy Constructor: Each variable points to a different copy of the String word = new String(“Java”); String word 2 = new String(word); word 2 “Java" Assignment: Both variables point to the same String word = “Java”; String word 2 = word; word 2 “Java" q Most other constructors take an array as a parameter to create a String. char[] letters = {‘J’, ‘a’, ‘v’, ‘a’}; String word = new String(letters); //”Java”
![Strings Constructors q For Example char char Array b i r t Strings Constructors q For Example: char[ ] char. Array = {‘b’, ‘i’, ‘r’, ‘t’,](https://slidetodoc.com/presentation_image/37d46827bc57494a05dfd51adbd2464b/image-5.jpg)
Strings Constructors q For Example: char[ ] char. Array = {‘b’, ‘i’, ‘r’, ‘t’, ‘h’, ‘ ‘, ‘d’, ‘a’, ‘y’}; String s = new String(“Hello”); String s 1 = new String(); String s 2 = new String(s); Empty Hello String s 3 = new String (char. Array); String s 4 = new String(char. Array, 3, 6); birth day
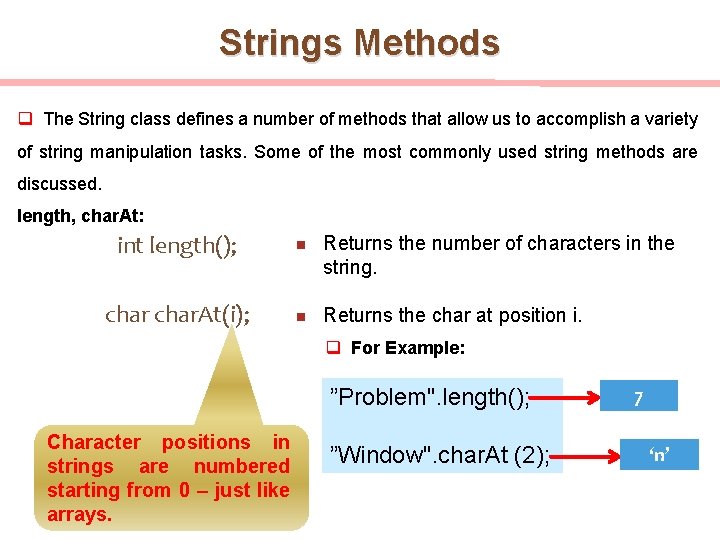
Strings Methods q The String class defines a number of methods that allow us to accomplish a variety of string manipulation tasks. Some of the most commonly used string methods are discussed. length, char. At: int length(); n Returns the number of characters in the string. char. At(i); n Returns the char at position i. q For Example: ”Problem". length(); Character positions in strings are numbered starting from 0 – just like arrays. ”Window". char. At (2); 7 ‘n’
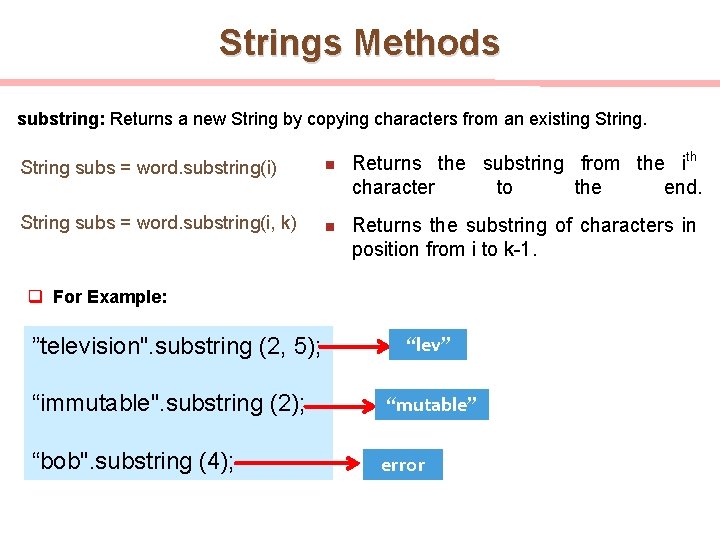
Strings Methods substring: Returns a new String by copying characters from an existing String subs = word. substring(i) n String subs = word. substring(i, k) n Returns the substring from the ith character to the end. Returns the substring of characters in position from i to k-1. q For Example: ”television". substring (2, 5); “lev” “immutable". substring (2); “mutable” “bob". substring (4); error
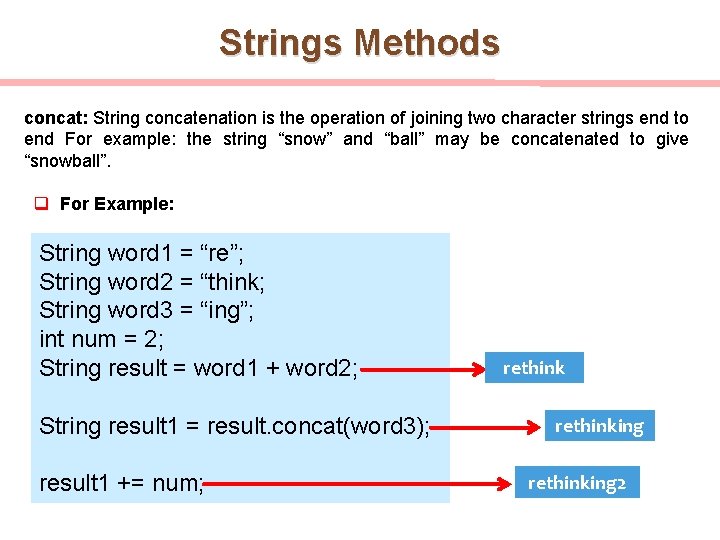
Strings Methods concat: String concatenation is the operation of joining two character strings end to end For example: the string “snow” and “ball” may be concatenated to give “snowball”. q For Example: String word 1 = “re”; String word 2 = “think; String word 3 = “ing”; int num = 2; String result = word 1 + word 2; String result 1 = result. concat(word 3); result 1 += num; rethinking 2
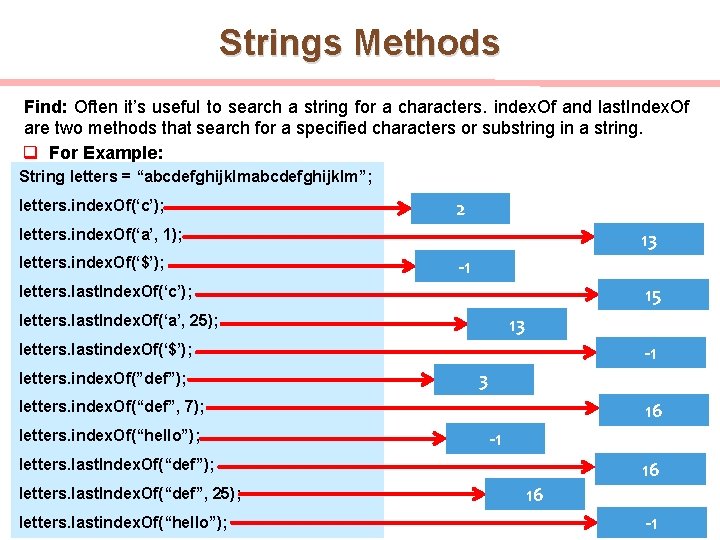
Strings Methods Find: Often it’s useful to search a string for a characters. index. Of and last. Index. Of are two methods that search for a specified characters or substring in a string. q For Example: String letters = “abcdefghijklm”; letters. index. Of(‘c’); 2 letters. index. Of(‘a’, 1); letters. index. Of(‘$’); 13 -1 15 letters. last. Index. Of(‘c’); letters. last. Index. Of(‘a’, 25); 13 -1 letters. lastindex. Of(‘$’); letters. index. Of(”def”); 3 16 letters. index. Of(“def”, 7); letters. index. Of(“hello”); -1 letters. last. Index. Of(“def”); letters. last. Index. Of(“def”, 25); letters. lastindex. Of(“hello”); 16 16 -1
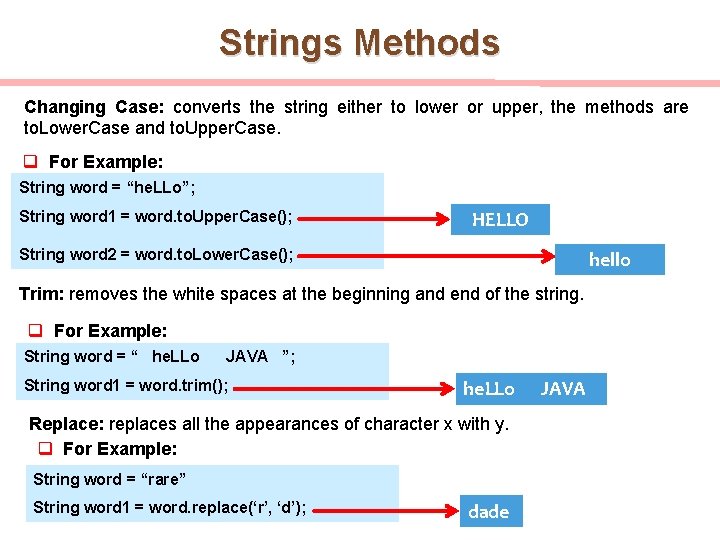
Strings Methods Changing Case: converts the string either to lower or upper, the methods are to. Lower. Case and to. Upper. Case. q For Example: String word = “he. LLo”; String word 1 = word. to. Upper. Case(); HELLO String word 2 = word. to. Lower. Case(); hello Trim: removes the white spaces at the beginning and end of the string. q For Example: String word = “ he. LLo JAVA ”; String word 1 = word. trim(); he. LLo Replace: replaces all the appearances of character x with y. q For Example: String word = “rare” String word 1 = word. replace(‘r’, ‘d’); dade JAVA
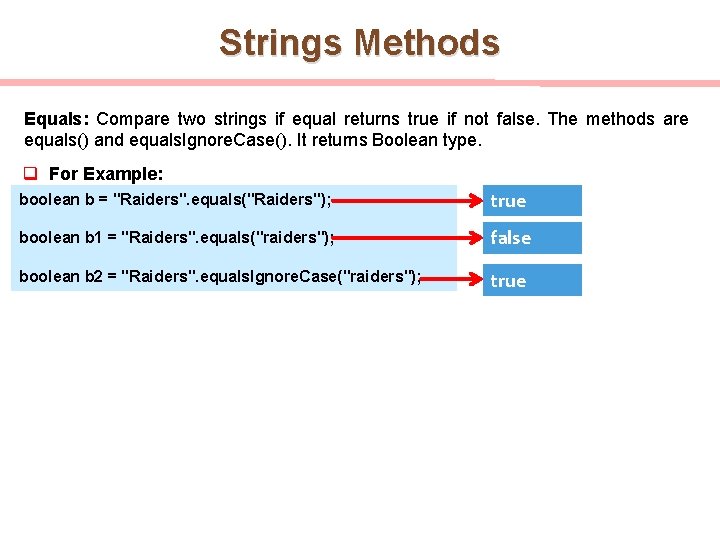
Strings Methods Equals: Compare two strings if equal returns true if not false. The methods are equals() and equals. Ignore. Case(). It returns Boolean type. q For Example: boolean b = "Raiders". equals("Raiders"); true boolean b 1 = "Raiders". equals("raiders"); false boolean b 2 = "Raiders". equals. Ignore. Case("raiders"); true
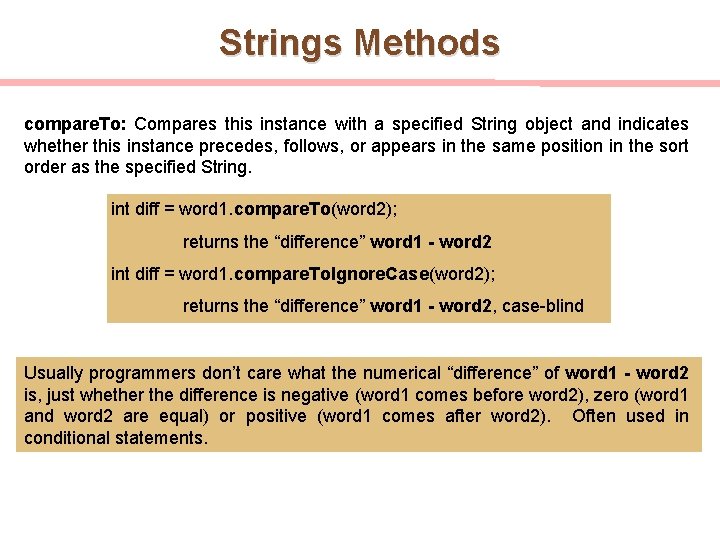
Strings Methods compare. To: Compares this instance with a specified String object and indicates whether this instance precedes, follows, or appears in the same position in the sort order as the specified String. int diff = word 1. compare. To(word 2); returns the “difference” word 1 - word 2 int diff = word 1. compare. To. Ignore. Case(word 2); returns the “difference” word 1 - word 2, case-blind Usually programmers don’t care what the numerical “difference” of word 1 - word 2 is, just whether the difference is negative (word 1 comes before word 2), zero (word 1 and word 2 are equal) or positive (word 1 comes after word 2). Often used in conditional statements.
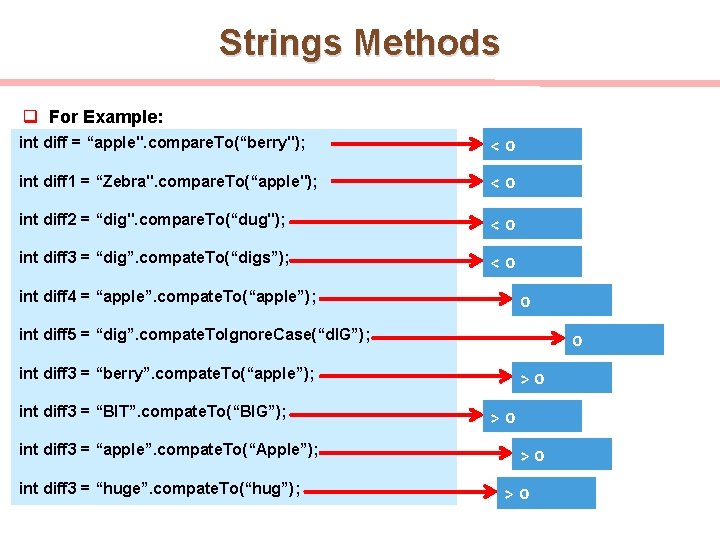
Strings Methods q For Example: int diff = “apple". compare. To(“berry"); <0 int diff 1 = “Zebra". compare. To(“apple"); <0 int diff 2 = “dig". compare. To(“dug"); <0 int diff 3 = “dig”. compate. To(“digs”); <0 int diff 4 = “apple”. compate. To(“apple”); 0 int diff 5 = “dig”. compate. To. Ignore. Case(“d. IG”); 0 int diff 3 = “berry”. compate. To(“apple”); int diff 3 = “BIT”. compate. To(“BIG”); int diff 3 = “apple”. compate. To(“Apple”); int diff 3 = “huge”. compate. To(“hug”); >0 >0
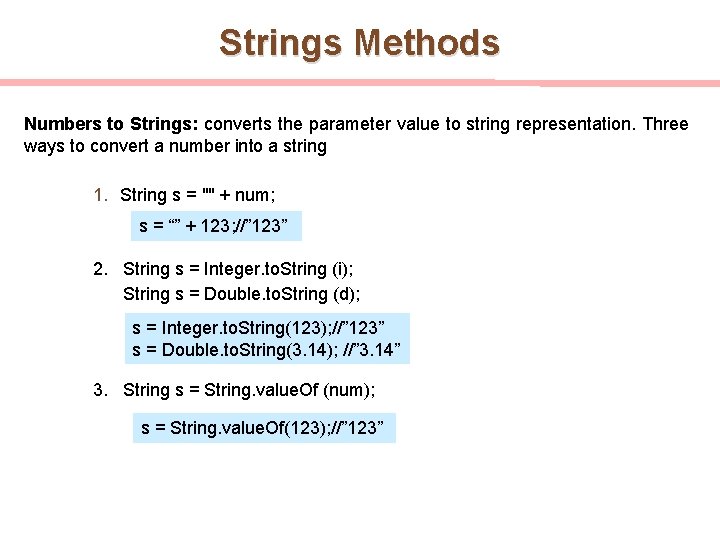
Strings Methods Numbers to Strings: converts the parameter value to string representation. Three ways to convert a number into a string 1. String s = "" + num; s = “” + 123; //” 123” 2. String s = Integer. to. String (i); String s = Double. to. String (d); s = Integer. to. String(123); //” 123” s = Double. to. String(3. 14); //” 3. 14” 3. String s = String. value. Of (num); s = String. value. Of(123); //” 123”
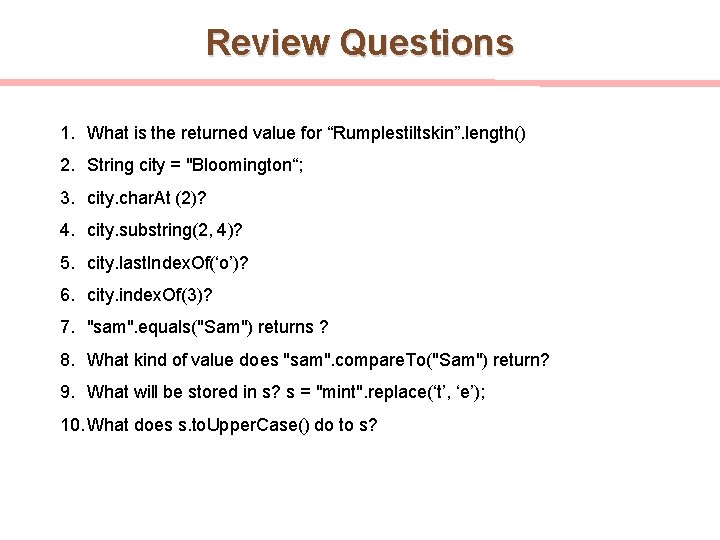
Review Questions 1. What is the returned value for “Rumplestiltskin”. length() 2. String city = "Bloomington“; 3. city. char. At (2)? 4. city. substring(2, 4)? 5. city. last. Index. Of(‘o’)? 6. city. index. Of(3)? 7. "sam". equals("Sam") returns ? 8. What kind of value does "sam". compare. To("Sam") return? 9. What will be stored in s? s = "mint". replace(‘t’, ‘e’); 10. What does s. to. Upper. Case() do to s?
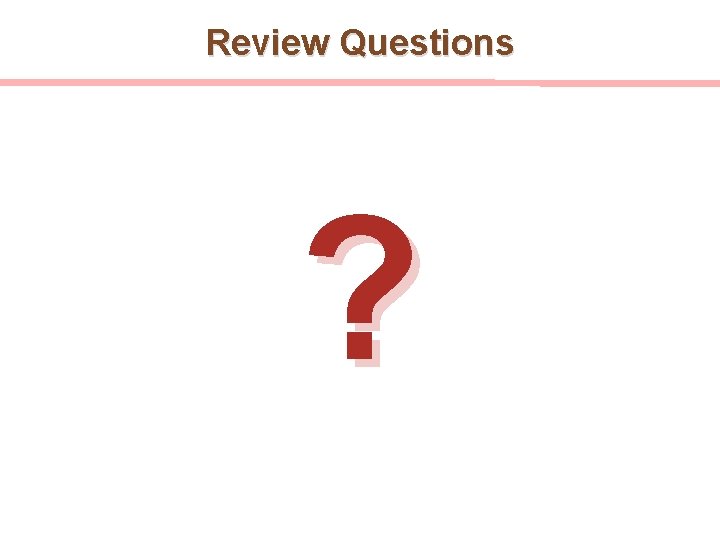
Review Questions ?