Three types of computer languages l Machine language
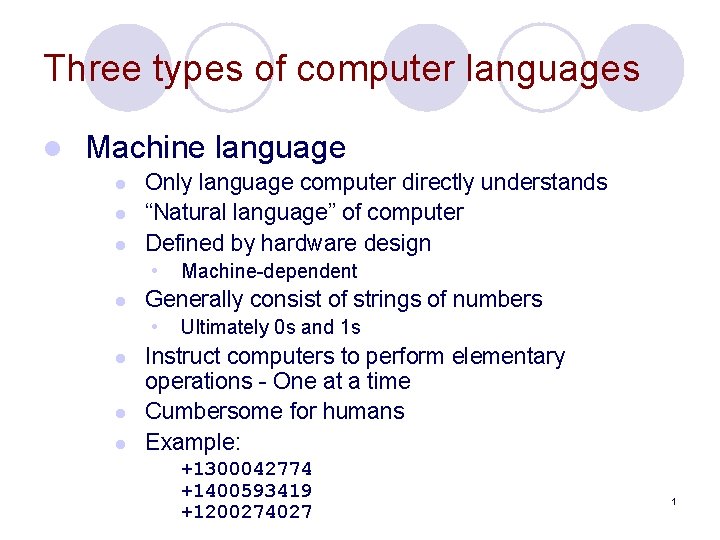
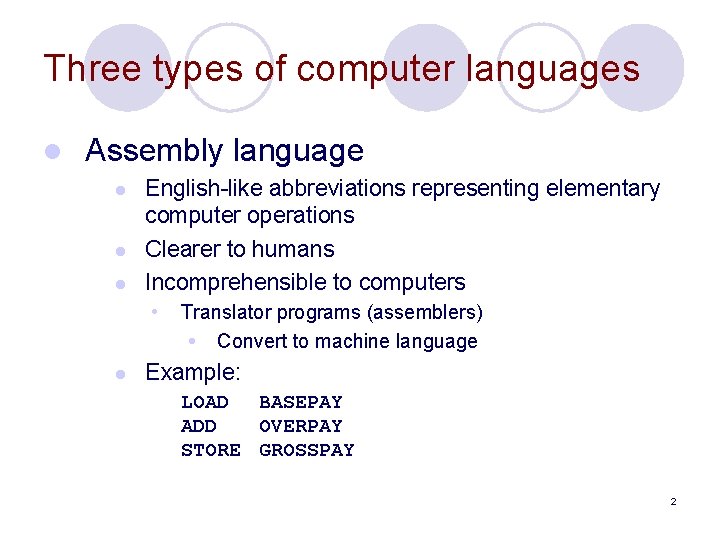
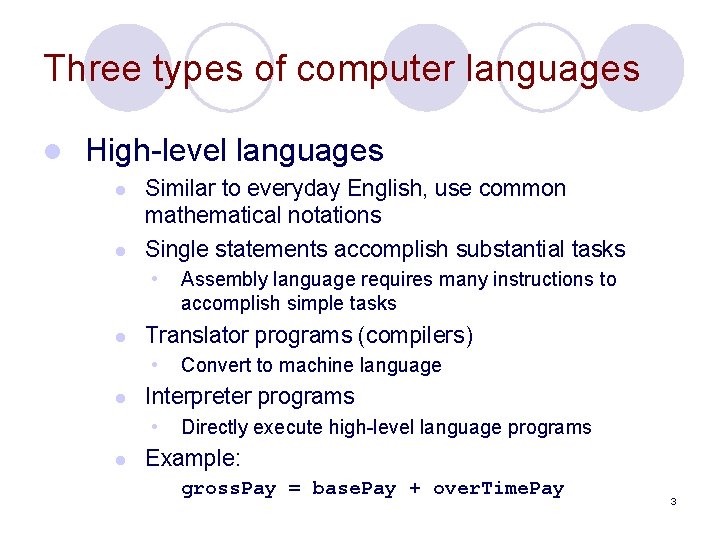
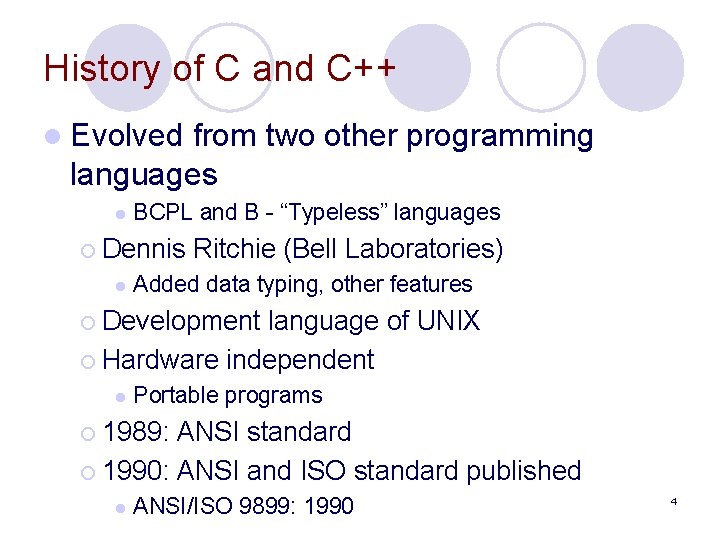
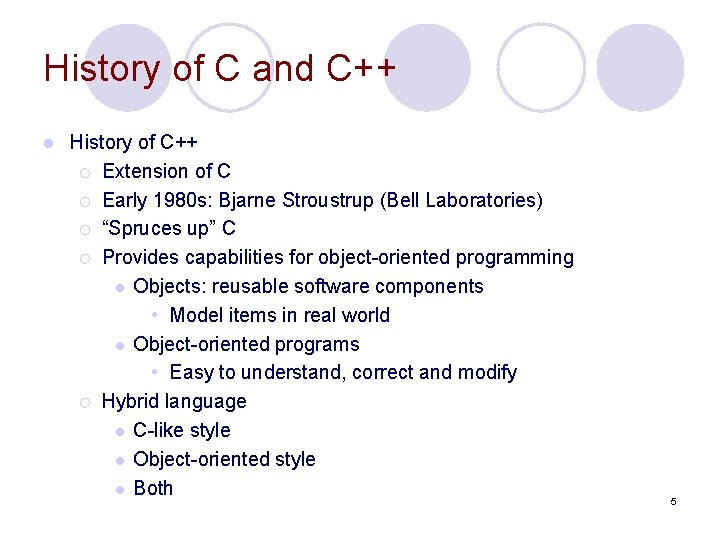
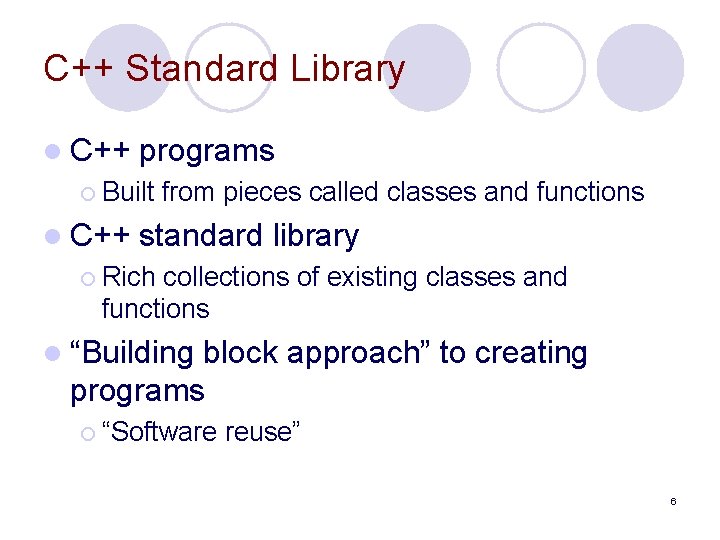
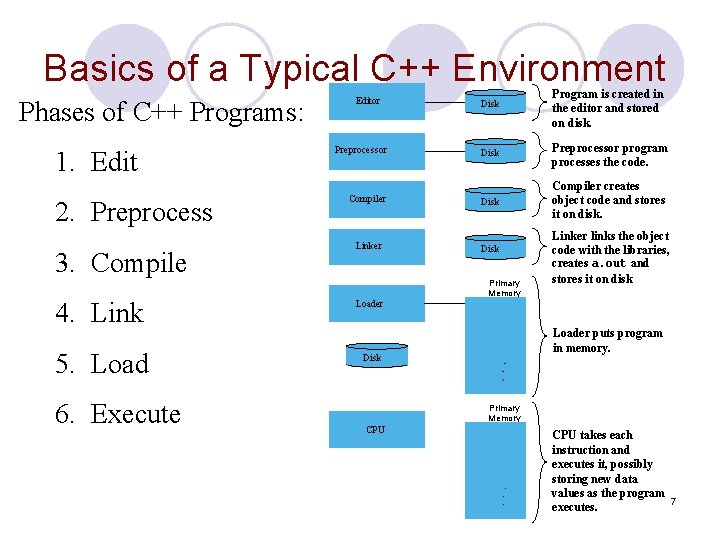
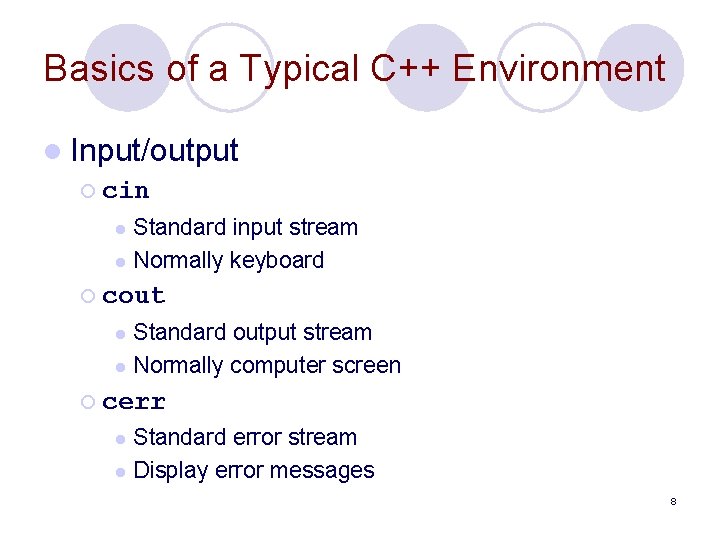
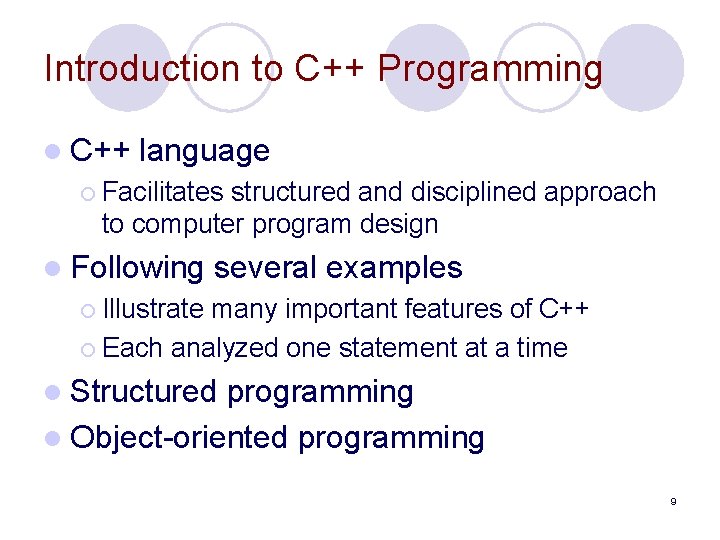
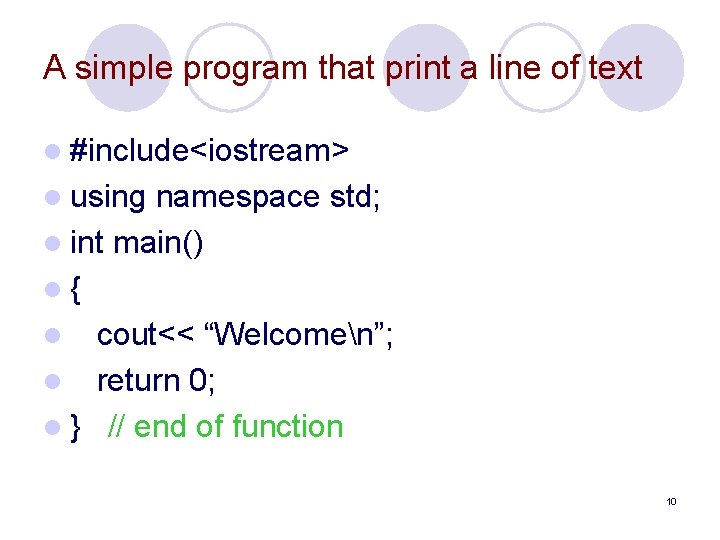
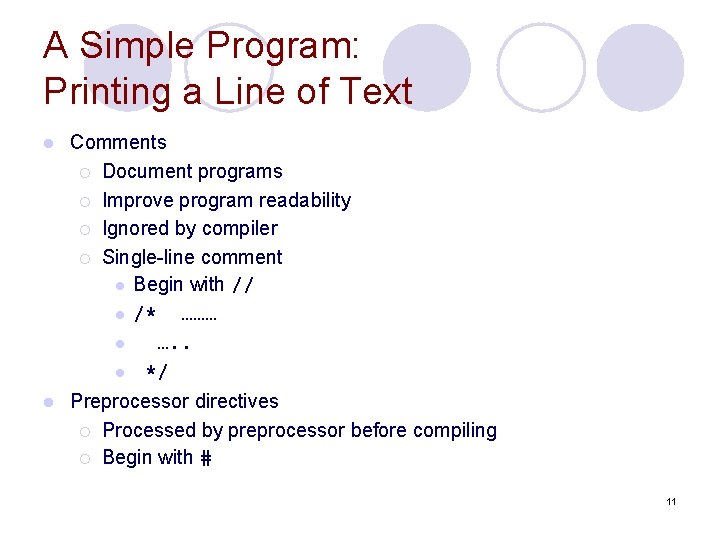
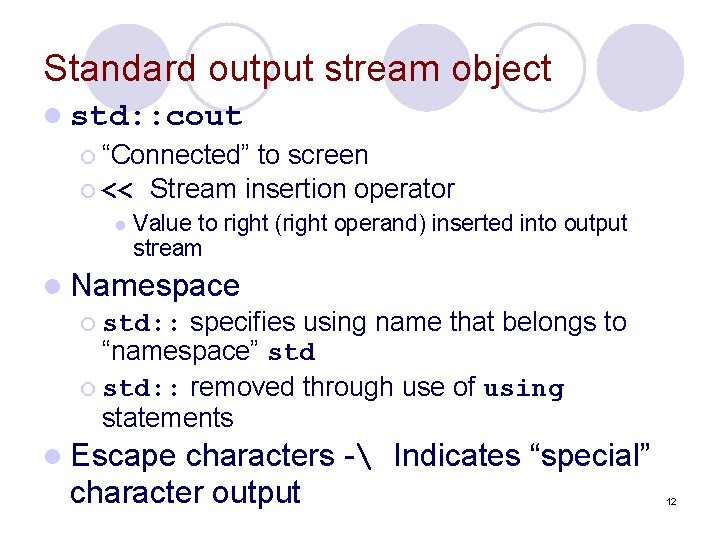
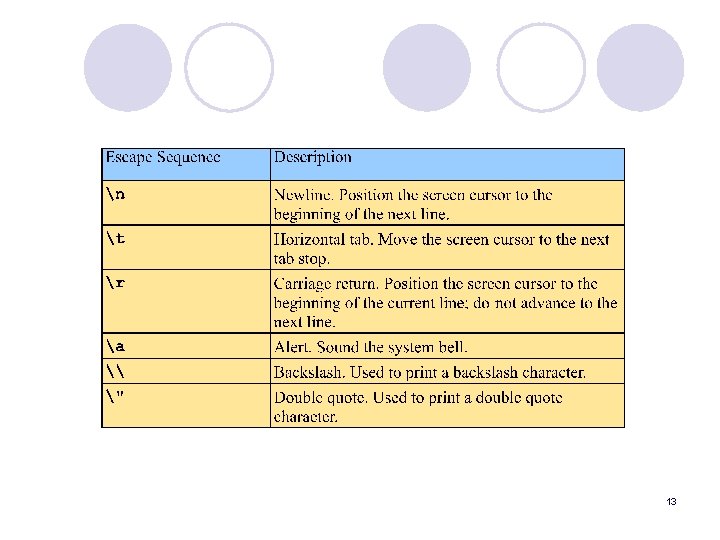
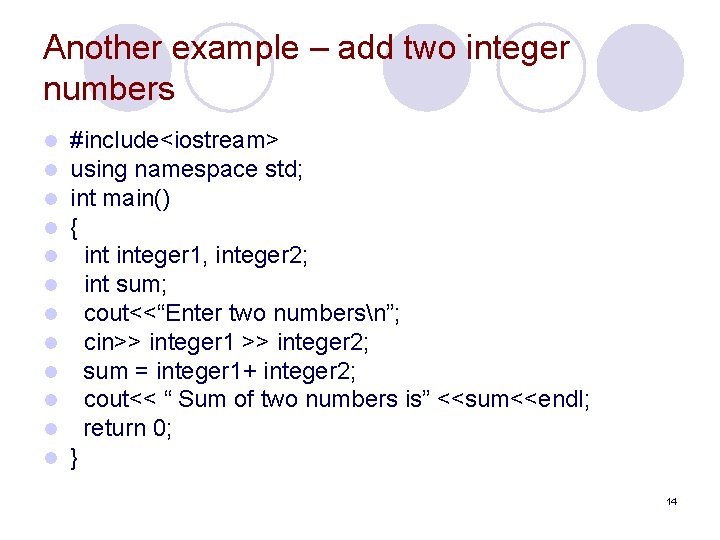
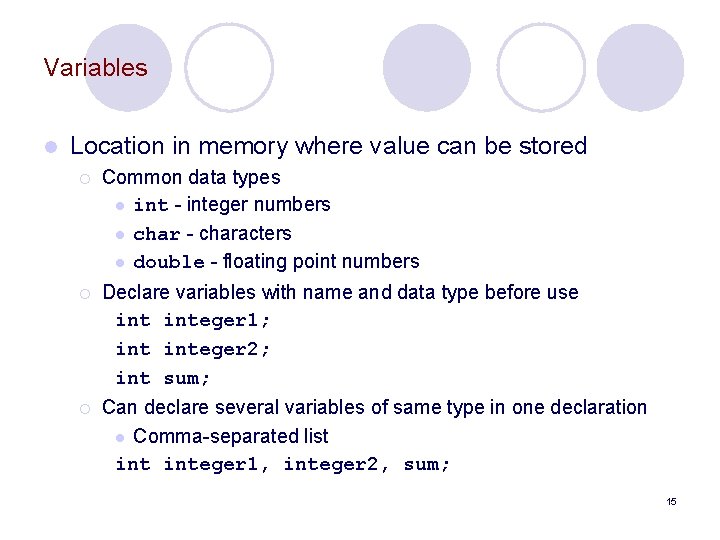
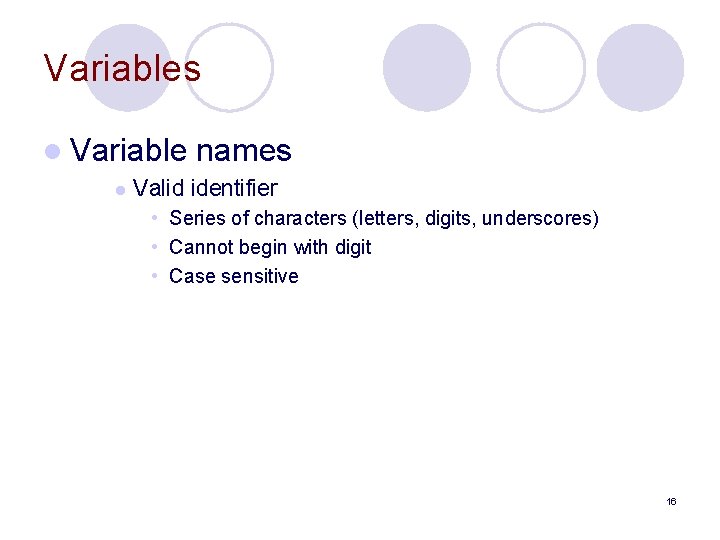
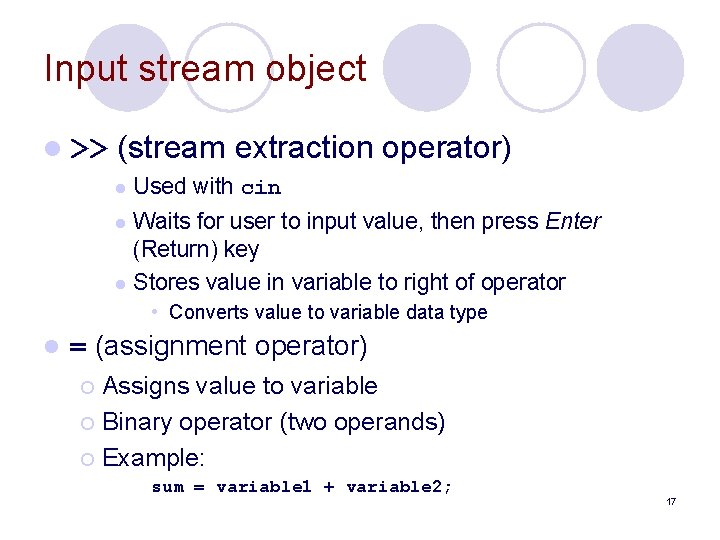
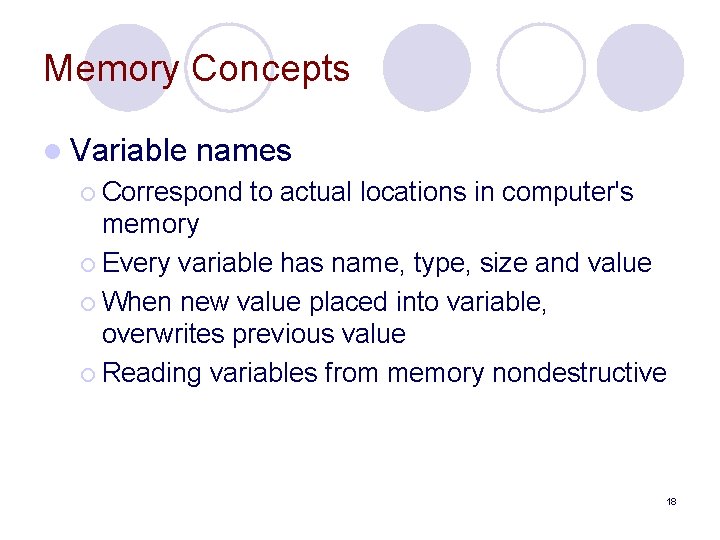
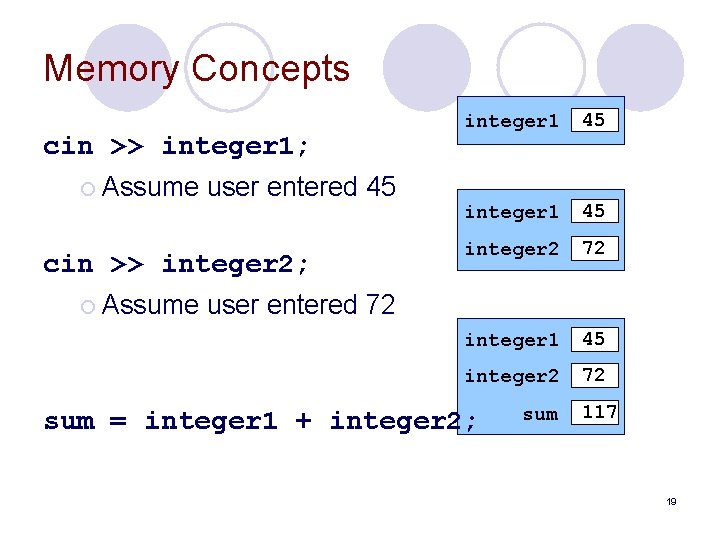
- Slides: 19
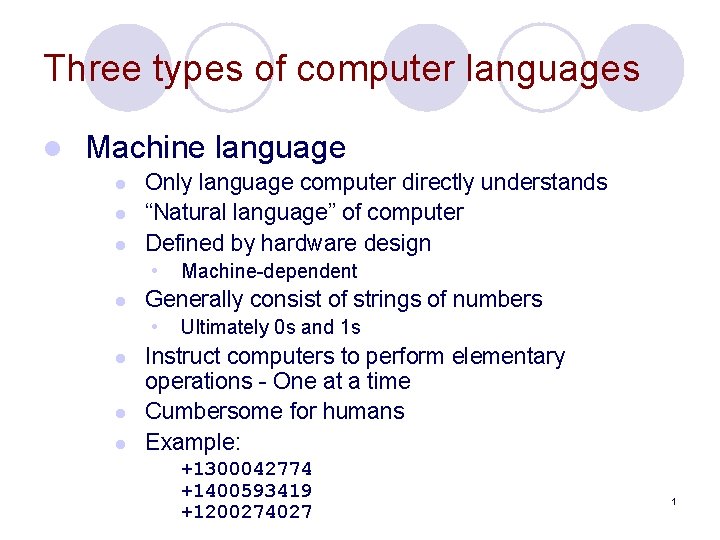
Three types of computer languages l Machine language l l l Only language computer directly understands “Natural language” of computer Defined by hardware design • l Generally consist of strings of numbers • l l l Machine-dependent Ultimately 0 s and 1 s Instruct computers to perform elementary operations - One at a time Cumbersome for humans Example: +1300042774 +1400593419 +1200274027 1
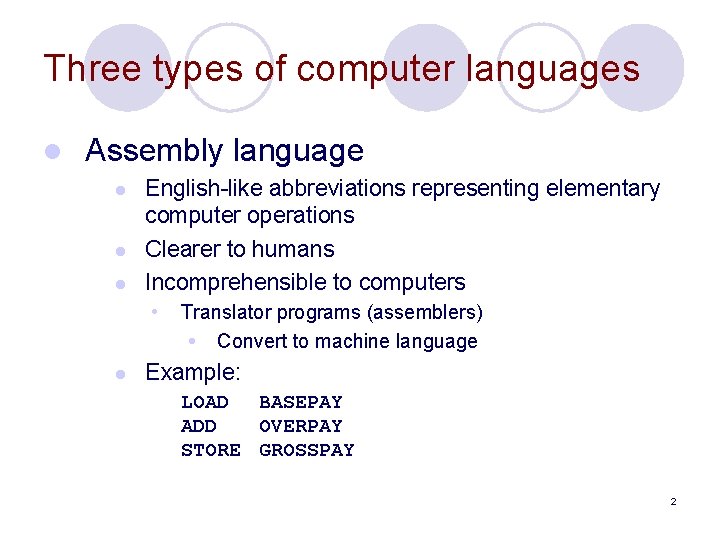
Three types of computer languages l Assembly language l l l English-like abbreviations representing elementary computer operations Clearer to humans Incomprehensible to computers • l Translator programs (assemblers) Convert to machine language Example: LOAD BASEPAY ADD OVERPAY STORE GROSSPAY 2
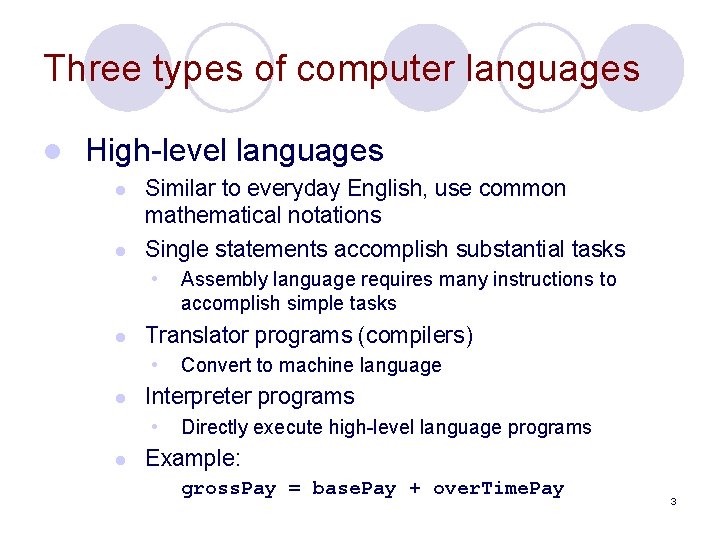
Three types of computer languages l High-level languages l l Similar to everyday English, use common mathematical notations Single statements accomplish substantial tasks • l Translator programs (compilers) • l Convert to machine language Interpreter programs • l Assembly language requires many instructions to accomplish simple tasks Directly execute high-level language programs Example: gross. Pay = base. Pay + over. Time. Pay 3
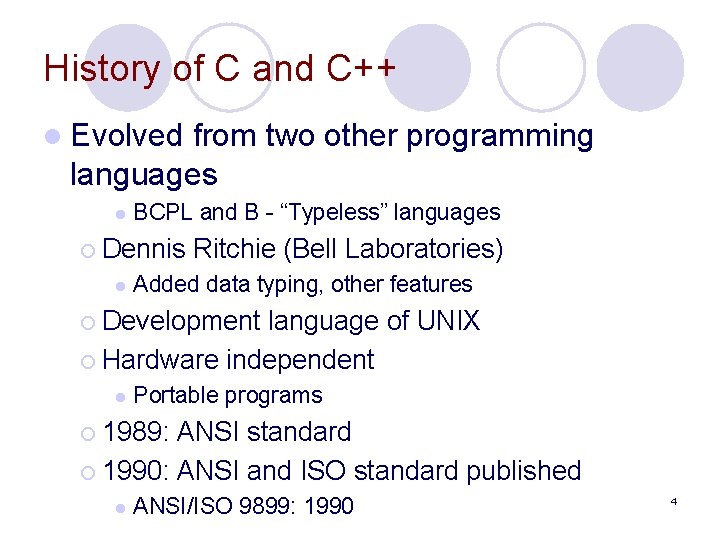
History of C and C++ l Evolved from two other programming languages l BCPL and B - “Typeless” languages ¡ Dennis l Ritchie (Bell Laboratories) Added data typing, other features ¡ Development language of UNIX ¡ Hardware independent l Portable programs ¡ 1989: ANSI standard ¡ 1990: ANSI and ISO standard published l ANSI/ISO 9899: 1990 4
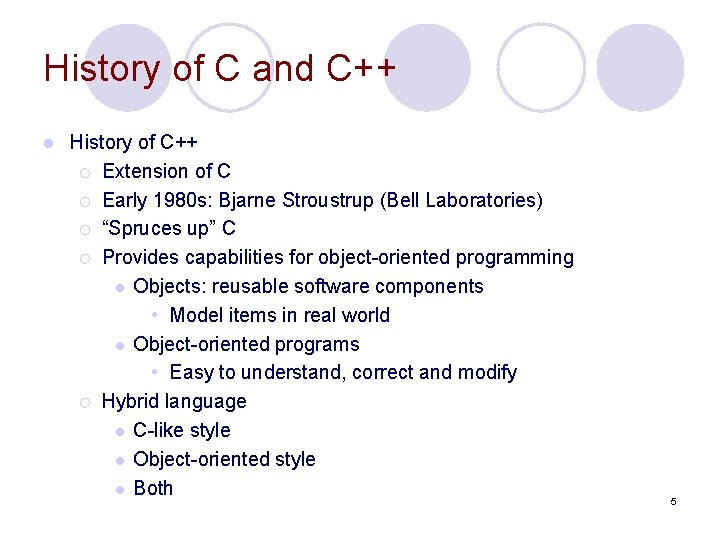
History of C and C++ l History of C++ ¡ Extension of C ¡ Early 1980 s: Bjarne Stroustrup (Bell Laboratories) ¡ “Spruces up” C ¡ Provides capabilities for object-oriented programming l Objects: reusable software components • Model items in real world l Object-oriented programs • Easy to understand, correct and modify ¡ Hybrid language l C-like style l Object-oriented style l Both 5
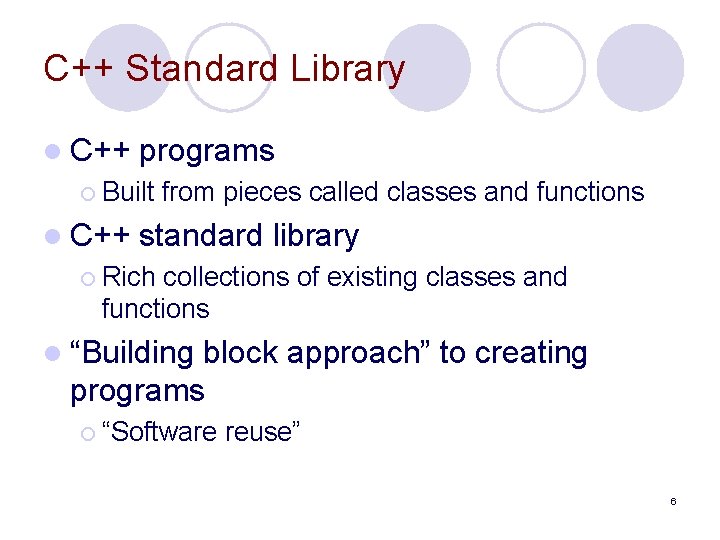
C++ Standard Library l C++ programs ¡ Built l C++ from pieces called classes and functions standard library ¡ Rich collections of existing classes and functions l “Building block approach” to creating programs ¡ “Software reuse” 6
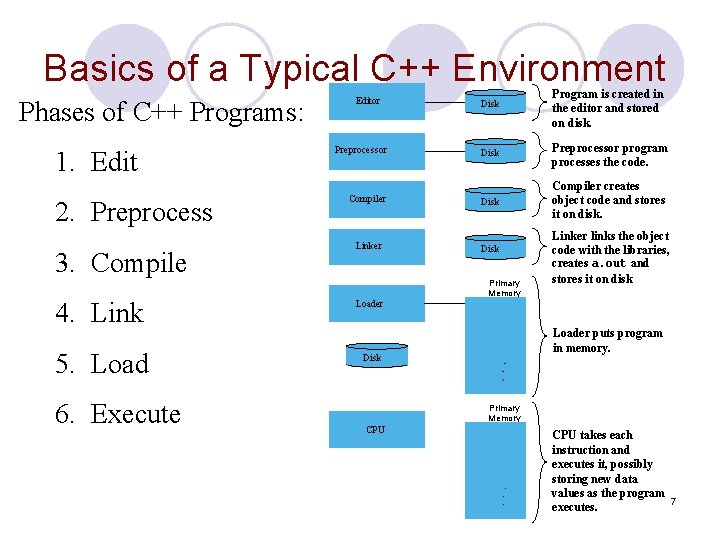
Basics of a Typical C++ Environment Phases of C++ Programs: 1. Edit 2. Preprocess 3. Compile Editor Preprocessor Compiler Linker 4. Link Loader 5. Load Disk 6. Execute Disk Program is created in the editor and stored on disk. Disk Preprocessor program processes the code. Disk Compiler creates object code and stores it on disk. Disk Primary Memory Linker links the object code with the libraries, creates a. out and stores it on disk Loader puts program in memory. . . . Primary Memory CPU . . . CPU takes each instruction and executes it, possibly storing new data values as the program 7 executes.
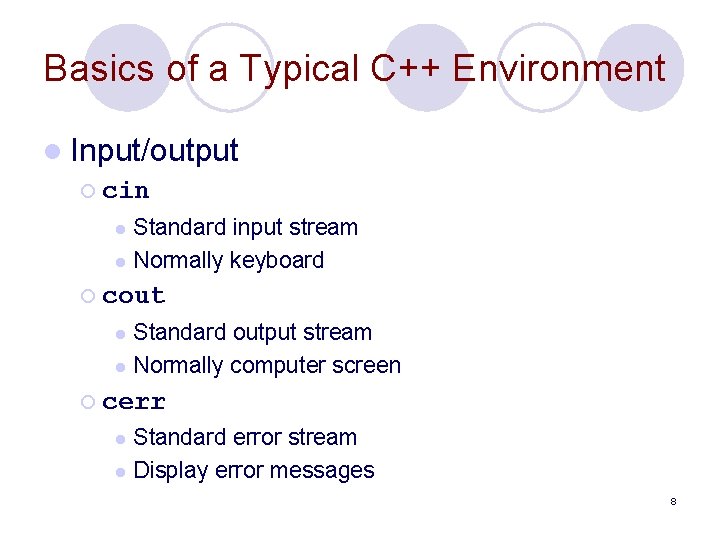
Basics of a Typical C++ Environment l Input/output ¡ cin Standard input stream l Normally keyboard l ¡ cout Standard output stream l Normally computer screen l ¡ cerr Standard error stream l Display error messages l 8
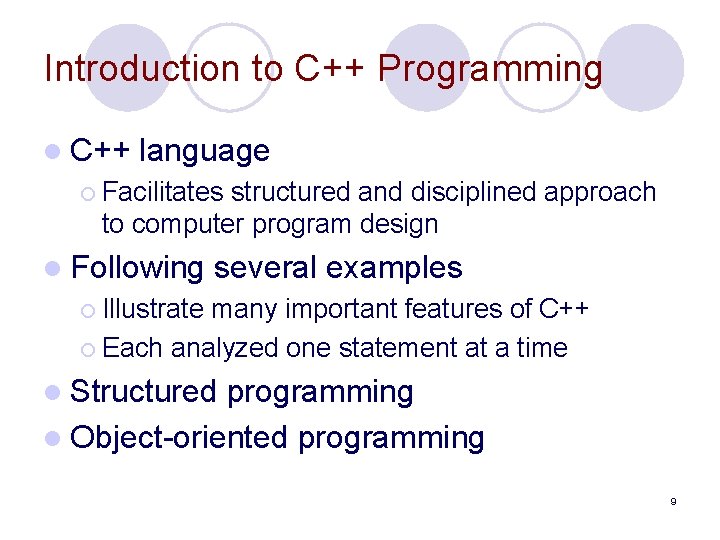
Introduction to C++ Programming l C++ language ¡ Facilitates structured and disciplined approach to computer program design l Following several examples ¡ Illustrate many important features of C++ ¡ Each analyzed one statement at a time l Structured programming l Object-oriented programming 9
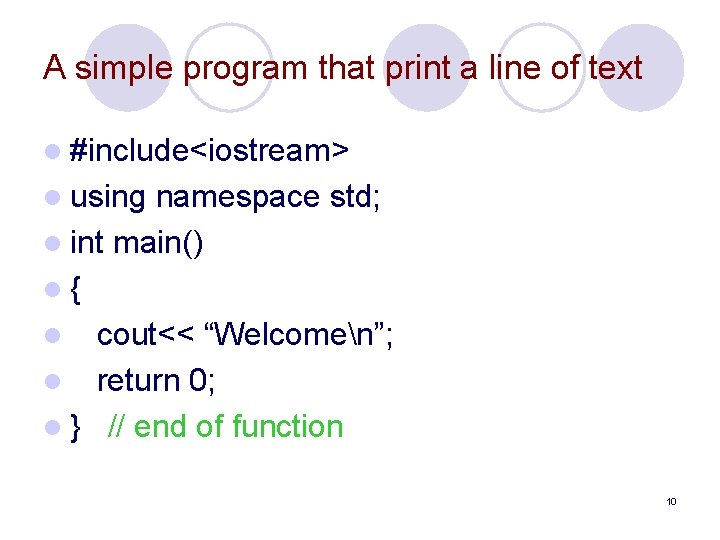
A simple program that print a line of text l #include<iostream> l using namespace std; l int main() l{ l cout<< “Welcomen”; l return 0; l } // end of function 10
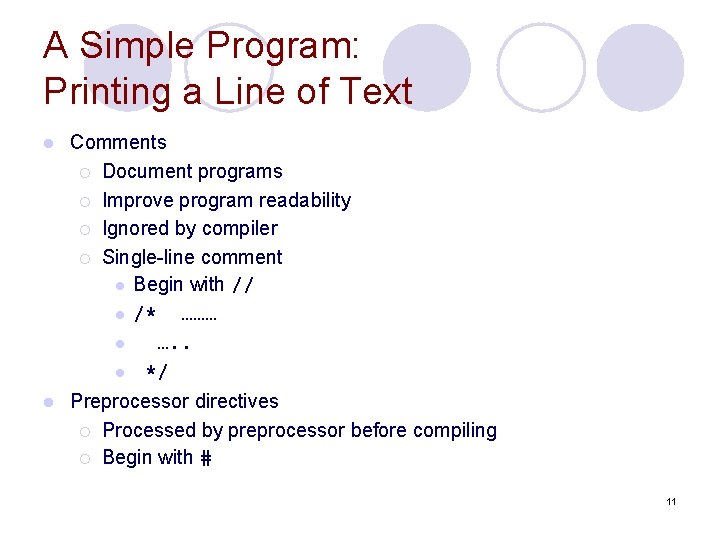
A Simple Program: Printing a Line of Text l Comments ¡ Document programs ¡ Improve program readability ¡ Ignored by compiler ¡ Single-line comment l Begin with // l /* ……… l …. . l */ l Preprocessor directives ¡ Processed by preprocessor before compiling ¡ Begin with # 11
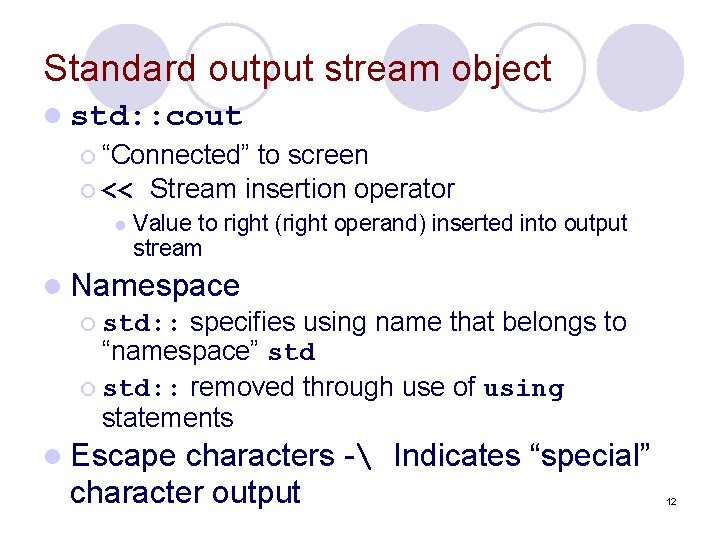
Standard output stream object l std: : cout ¡ “Connected” to screen ¡ << Stream insertion operator l Value to right (right operand) inserted into output stream l Namespace ¡ std: : specifies using name that belongs to “namespace” std ¡ std: : removed through use of using statements l Escape characters - Indicates “special” character output 12
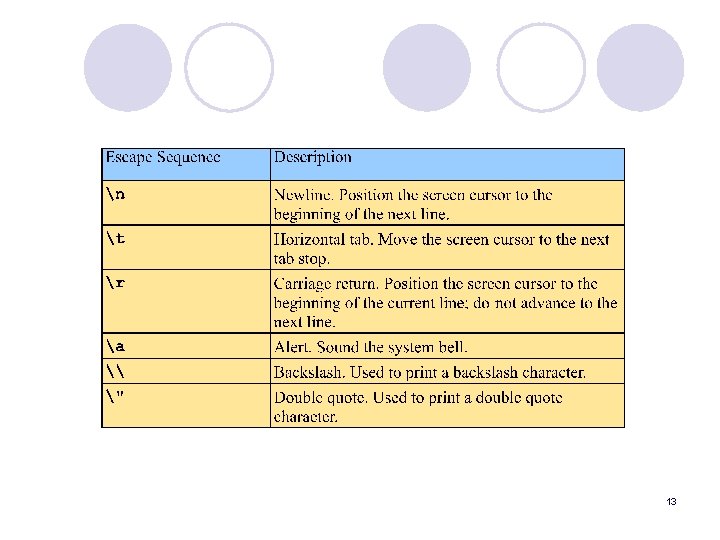
13
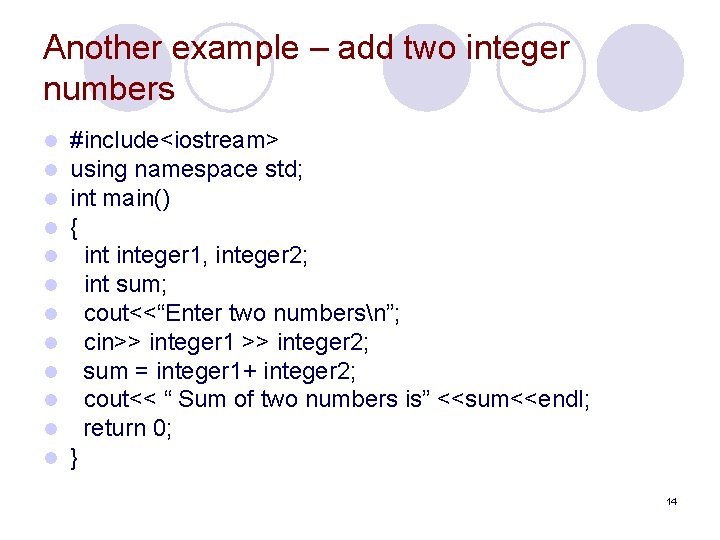
Another example – add two integer numbers l l l #include<iostream> using namespace std; int main() { integer 1, integer 2; int sum; cout<<“Enter two numbersn”; cin>> integer 1 >> integer 2; sum = integer 1+ integer 2; cout<< “ Sum of two numbers is” <<sum<<endl; return 0; } 14
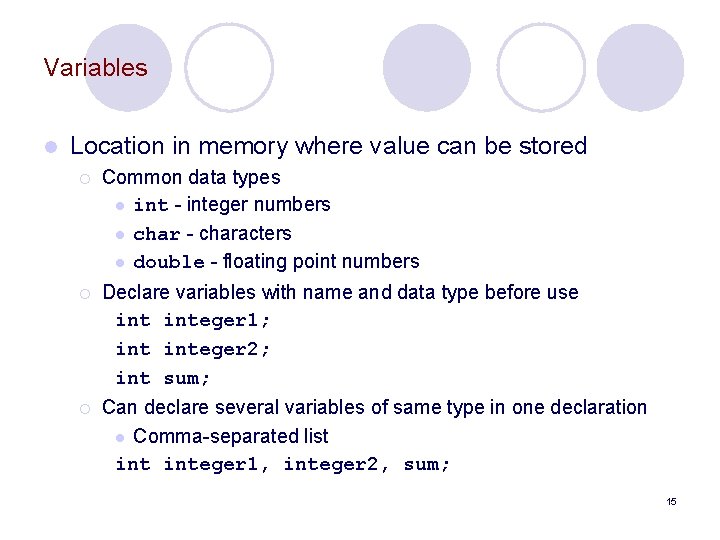
Variables l Location in memory where value can be stored ¡ Common data types l int - integer numbers l char - characters l double - floating point numbers ¡ Declare variables with name and data type before use integer 1; integer 2; int sum; ¡ Can declare several variables of same type in one declaration l Comma-separated list integer 1, integer 2, sum; 15
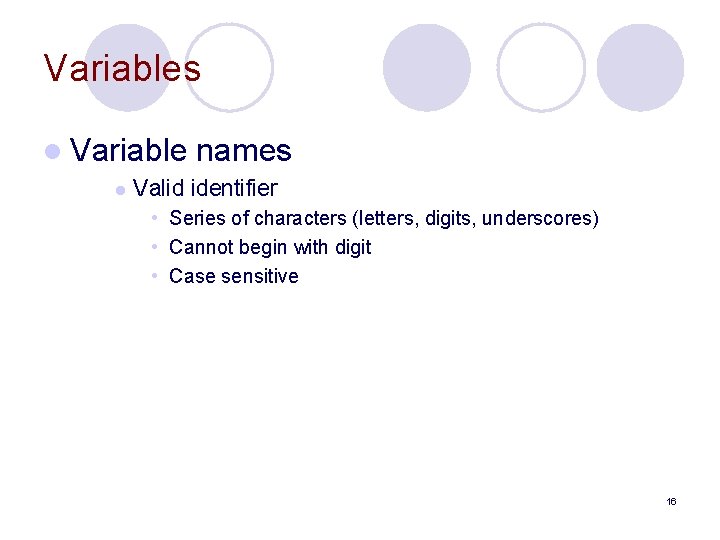
Variables l Variable l names Valid identifier • Series of characters (letters, digits, underscores) • Cannot begin with digit • Case sensitive 16
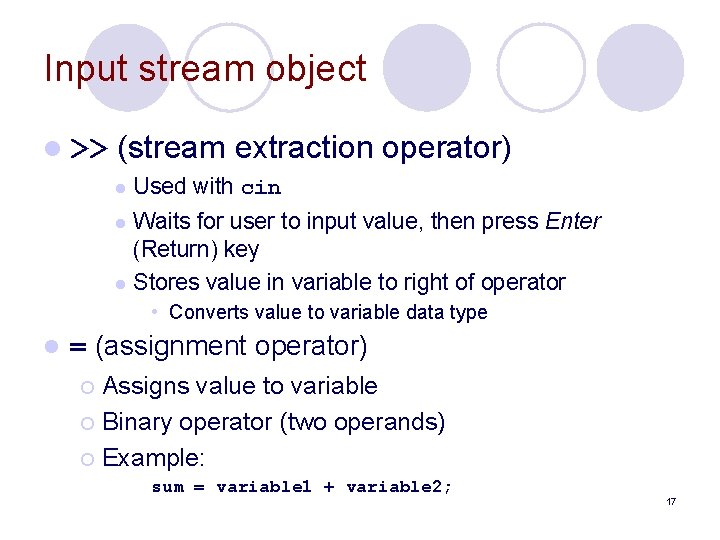
Input stream object l >> (stream extraction operator) l Used with cin Waits for user to input value, then press Enter (Return) key l Stores value in variable to right of operator l • Converts value to variable data type l = (assignment operator) Assigns value to variable ¡ Binary operator (two operands) ¡ Example: ¡ sum = variable 1 + variable 2; 17
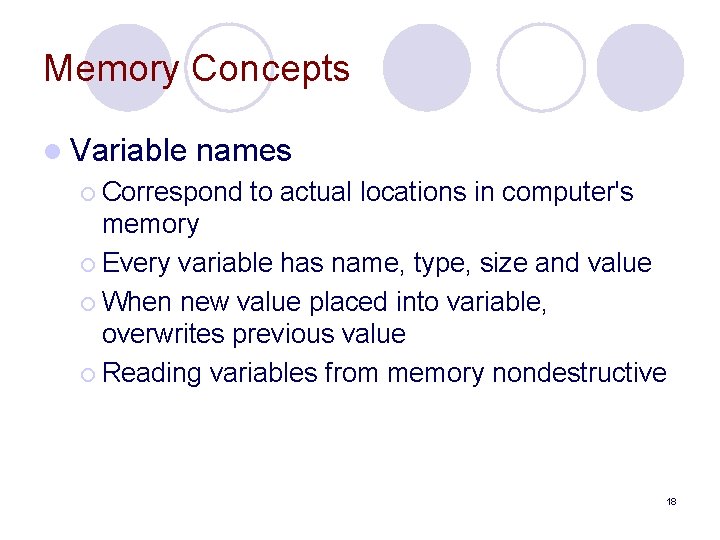
Memory Concepts l Variable names ¡ Correspond to actual locations in computer's memory ¡ Every variable has name, type, size and value ¡ When new value placed into variable, overwrites previous value ¡ Reading variables from memory nondestructive 18
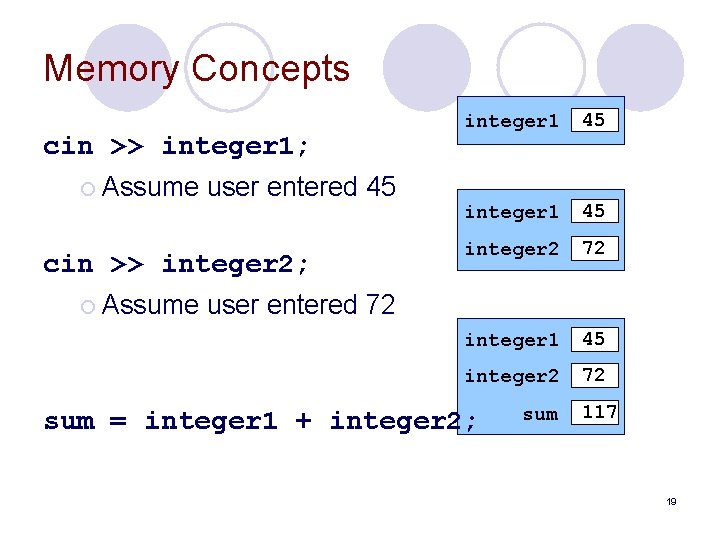
Memory Concepts cin >> integer 1; ¡ Assume user entered 45 cin >> integer 2; ¡ Assume user entered 72 integer 1 45 integer 2 72 sum = integer 1 + integer 2; sum 117 19