Three Design Patterns Adapter Faade Flyweight C Sc
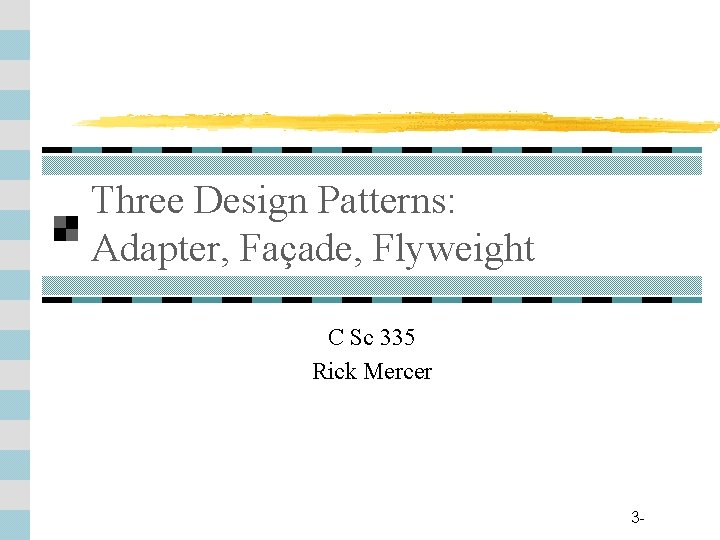
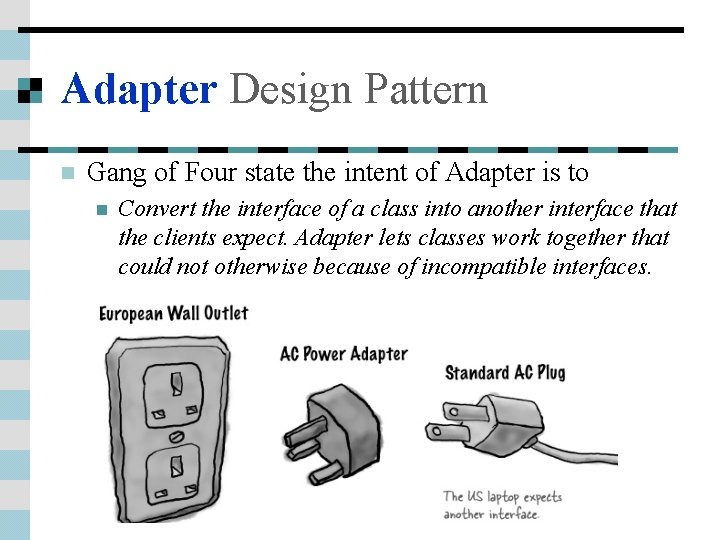
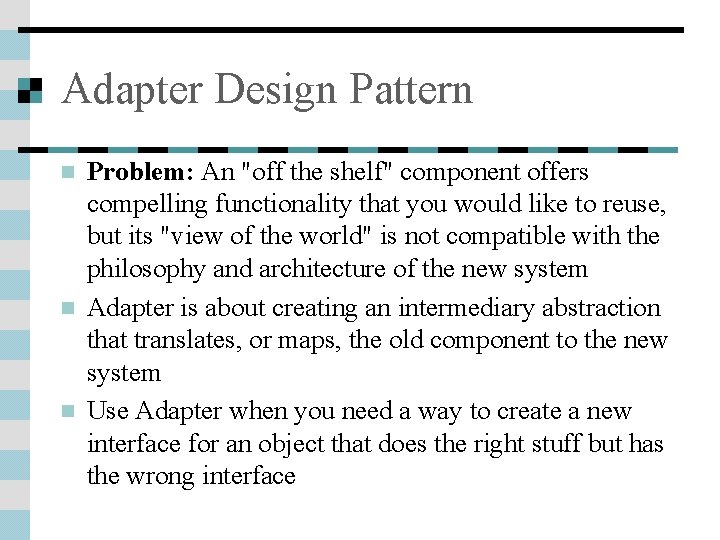
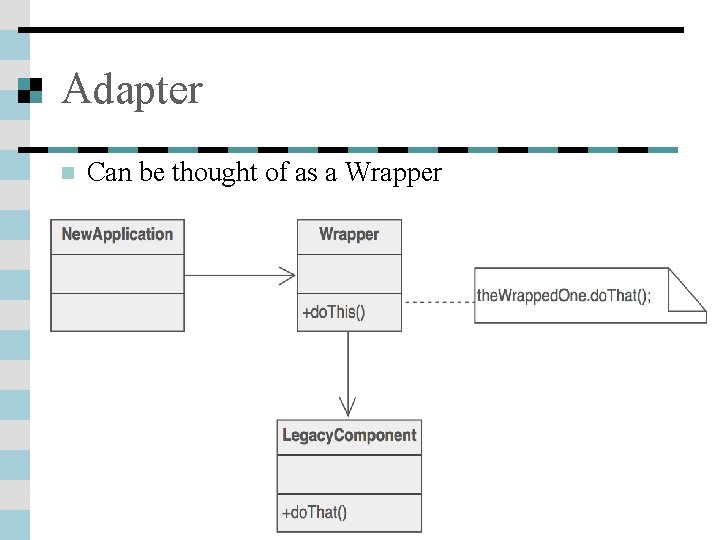
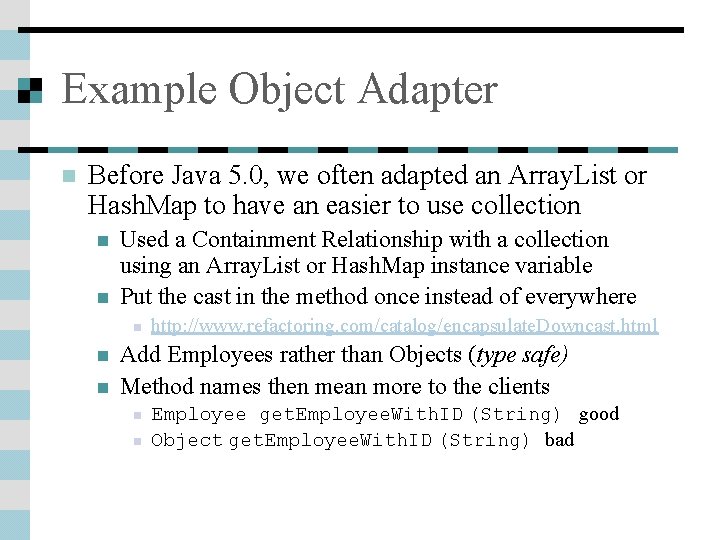
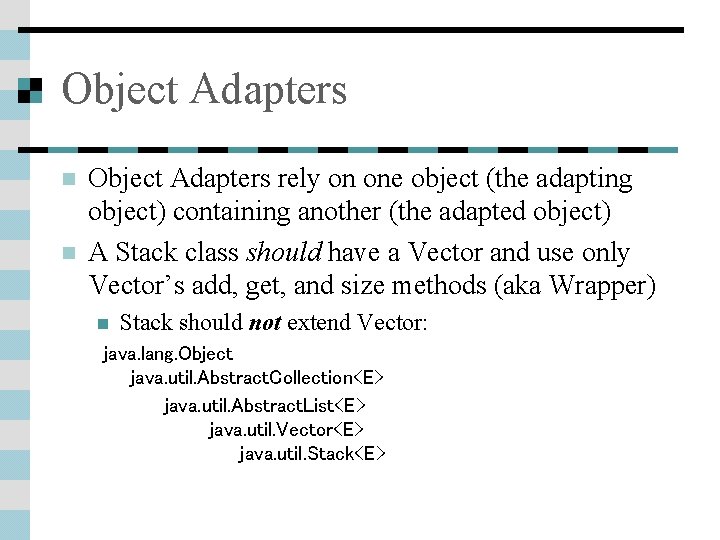
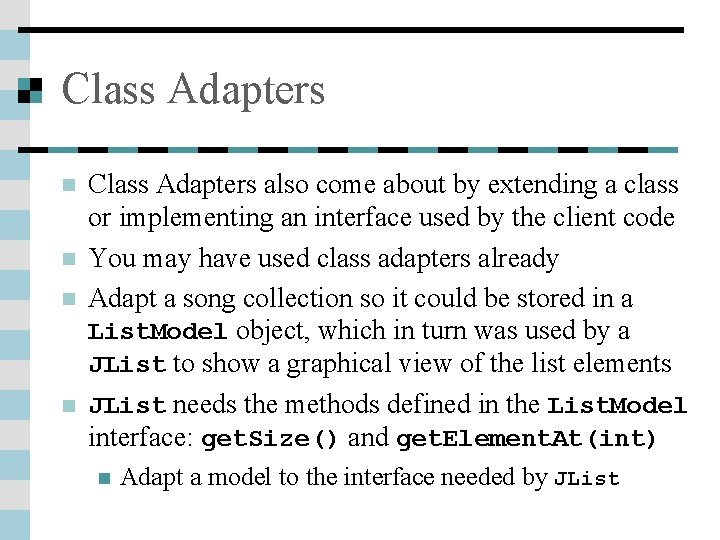
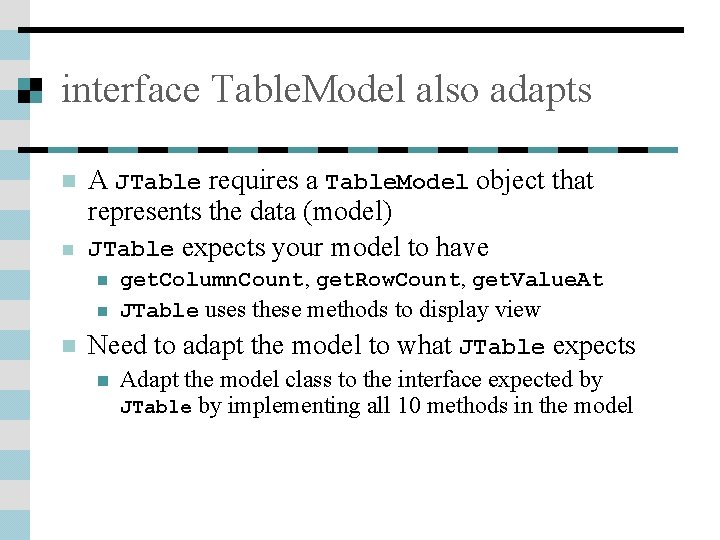
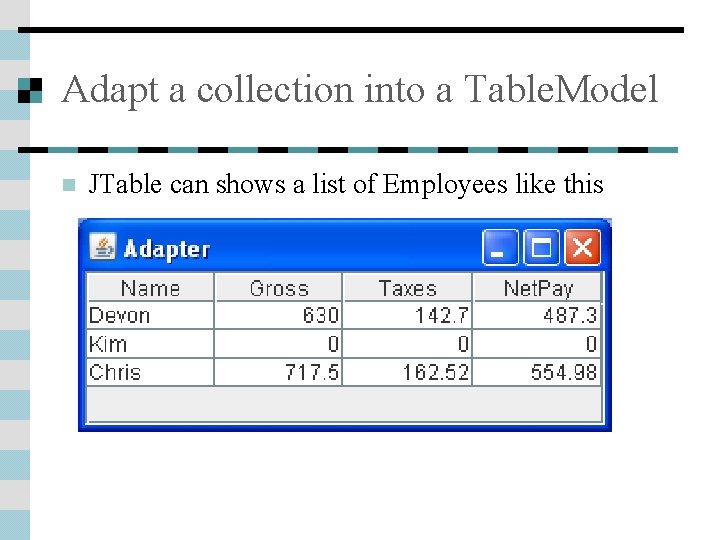
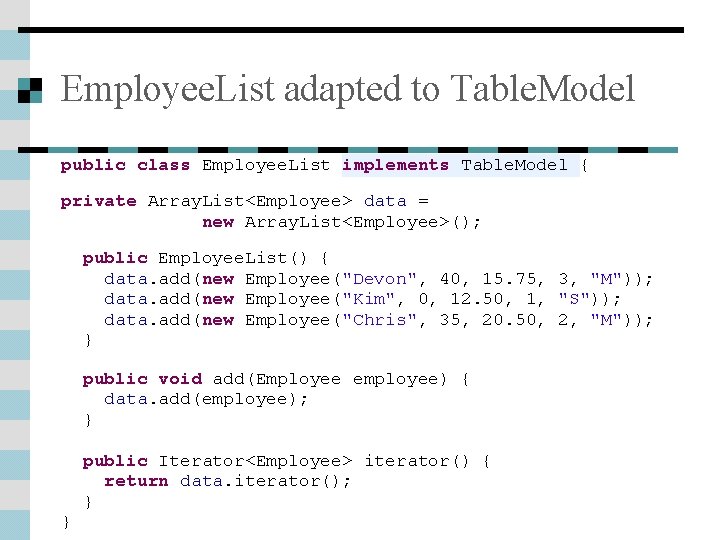
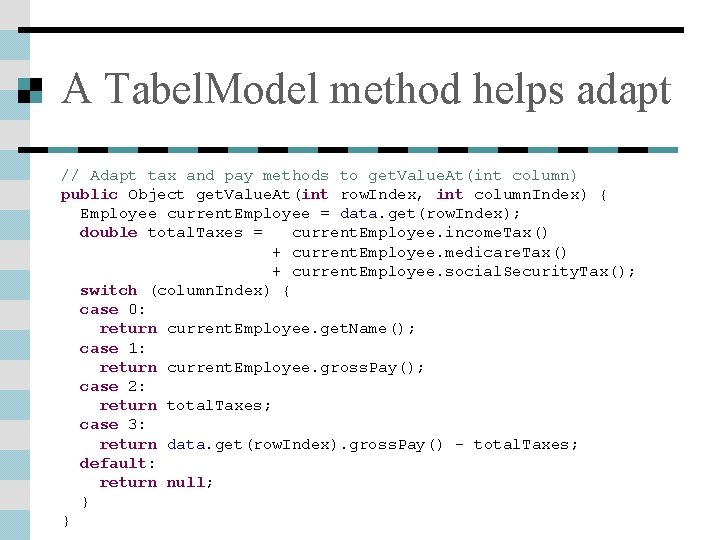
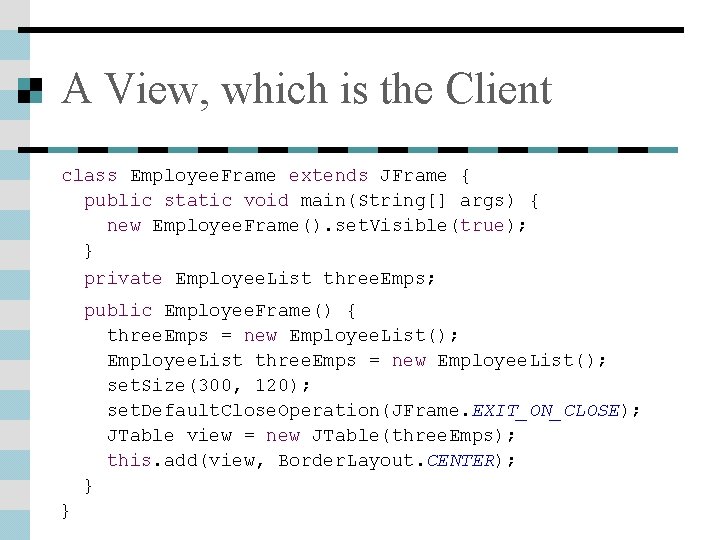
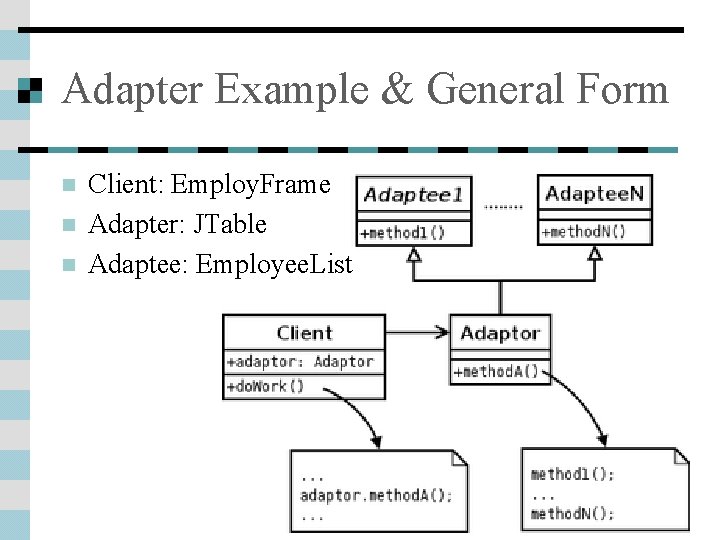
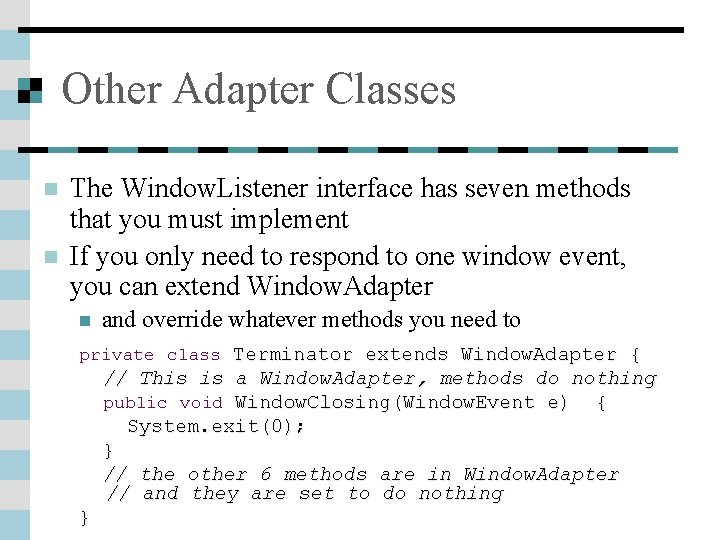
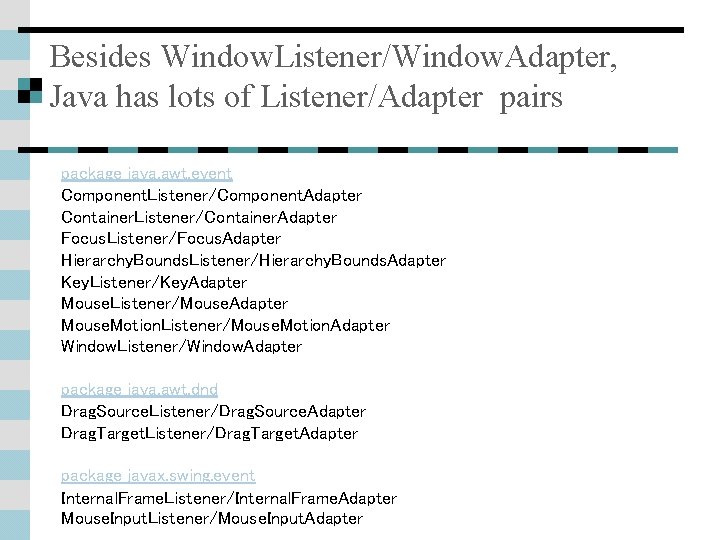
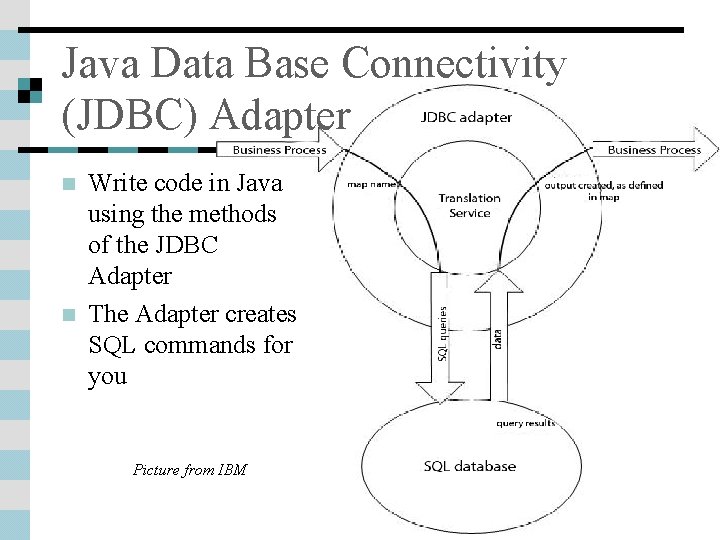
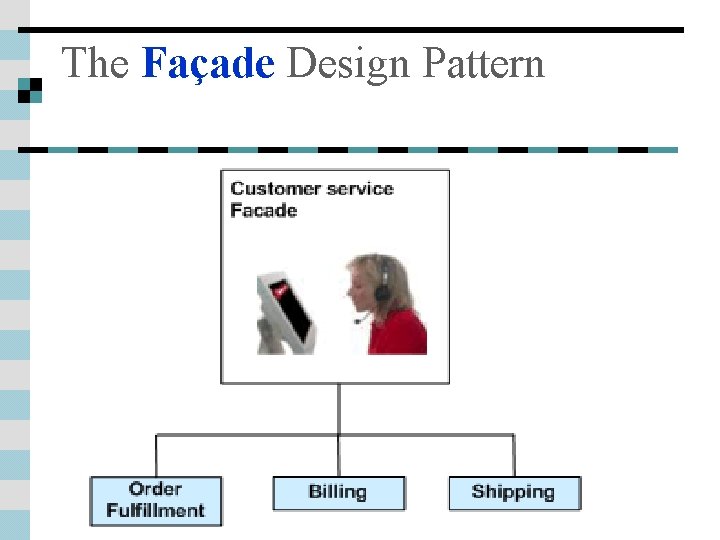
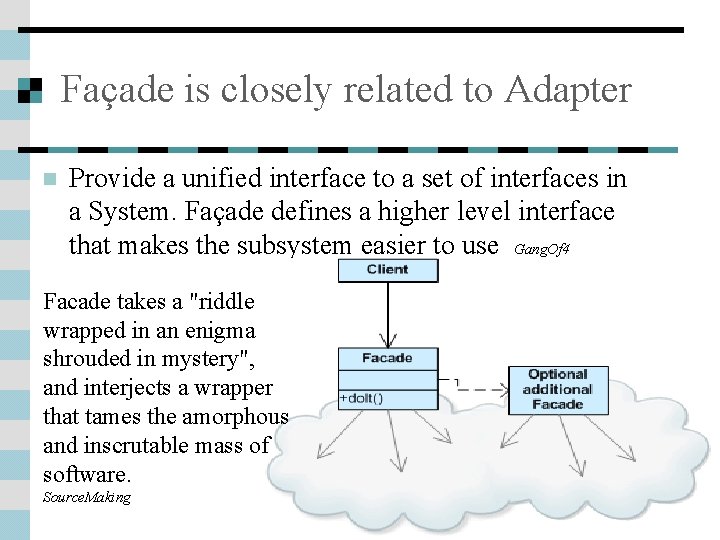
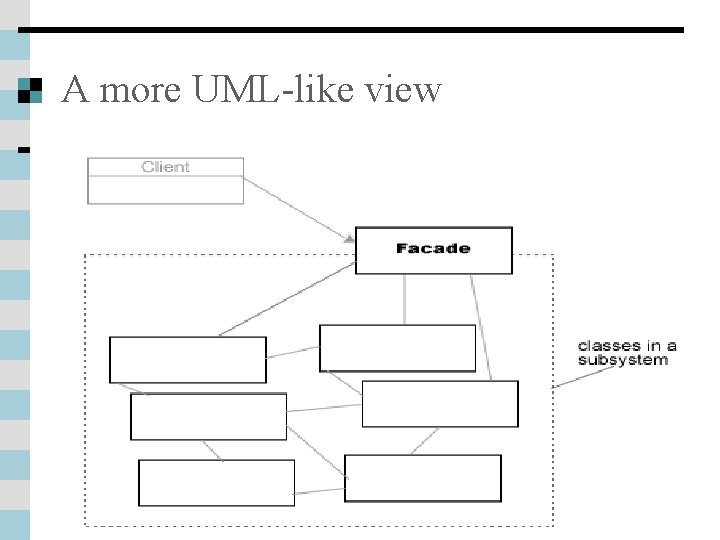
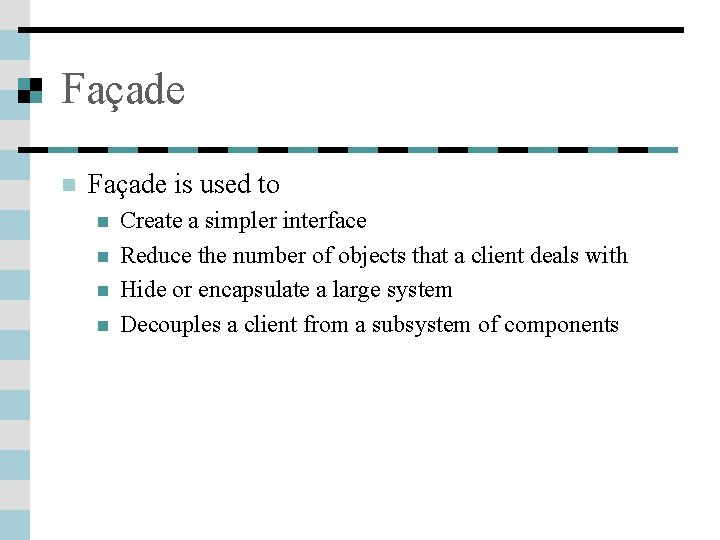
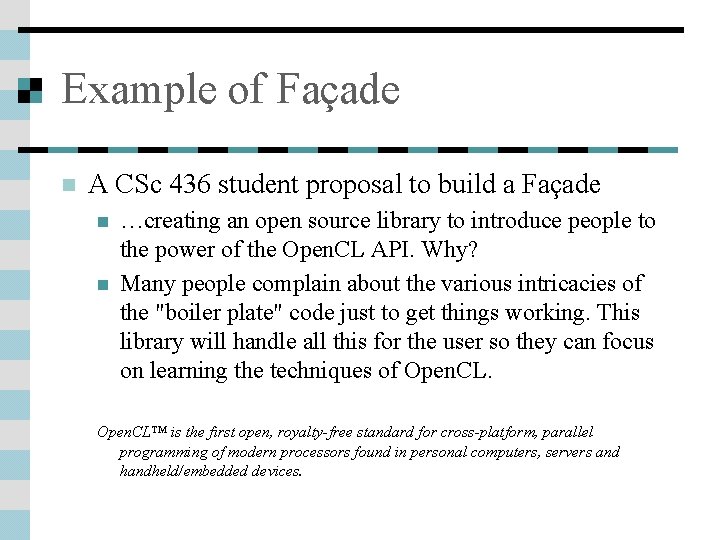
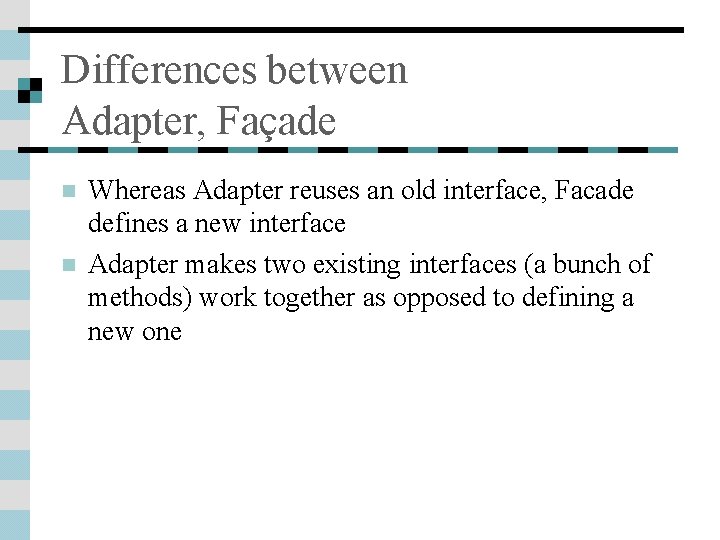
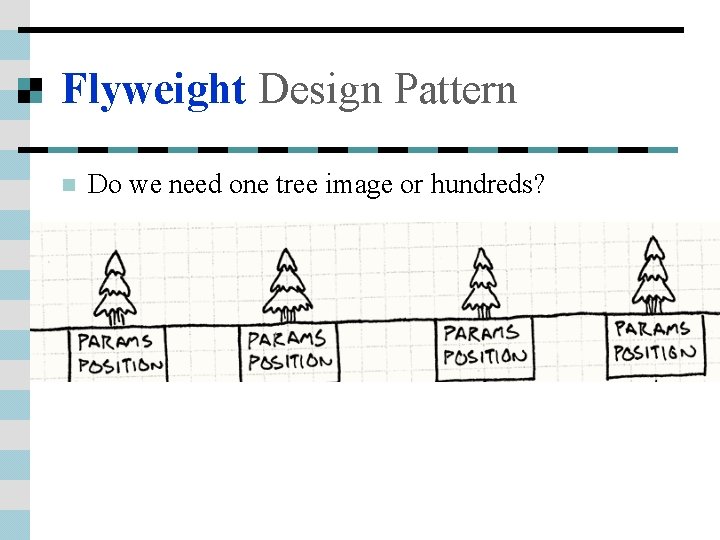
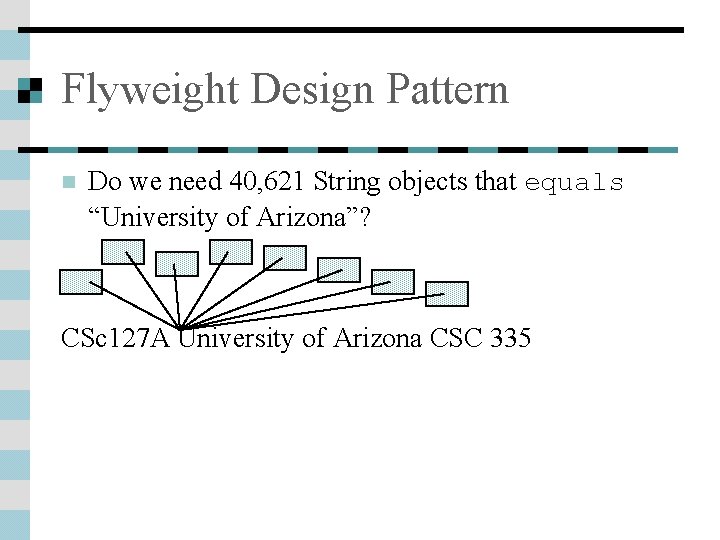
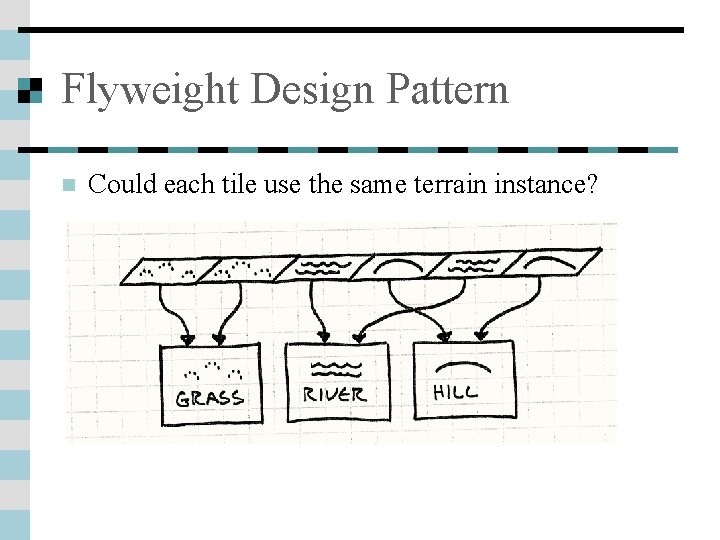
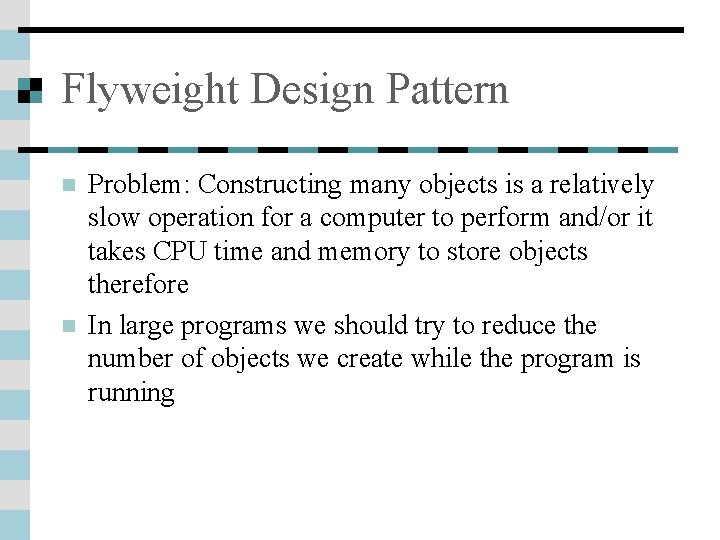
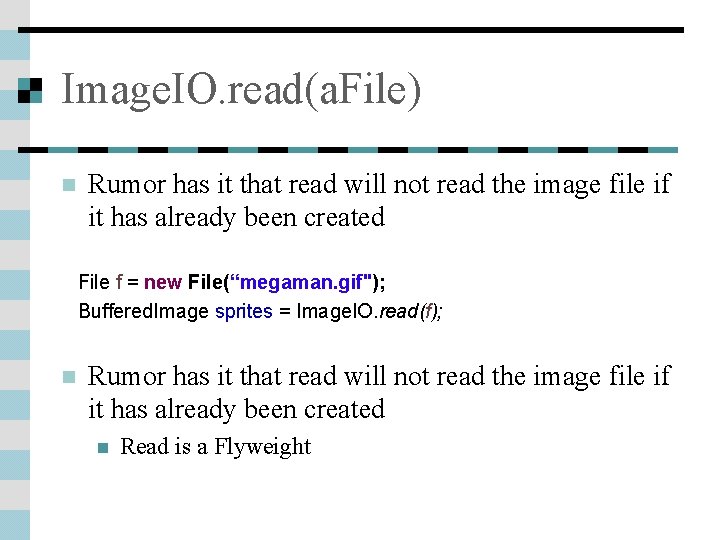
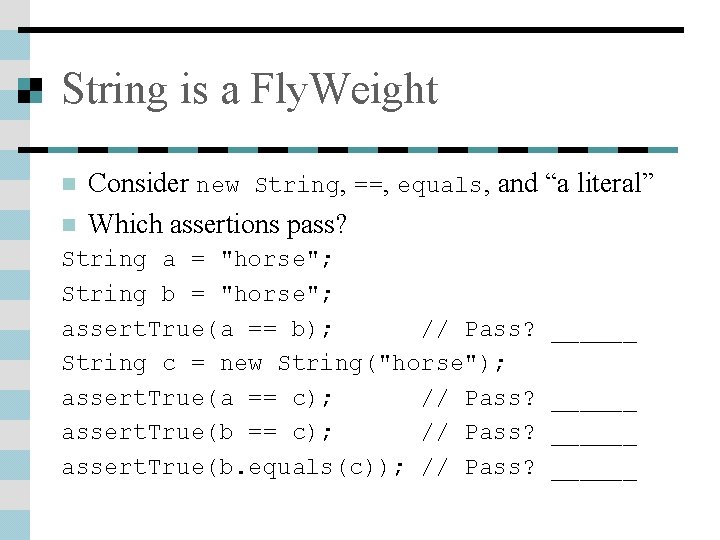
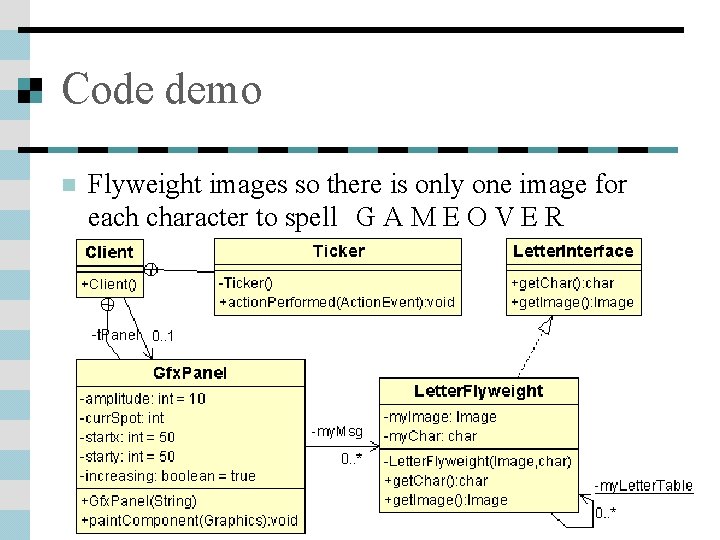
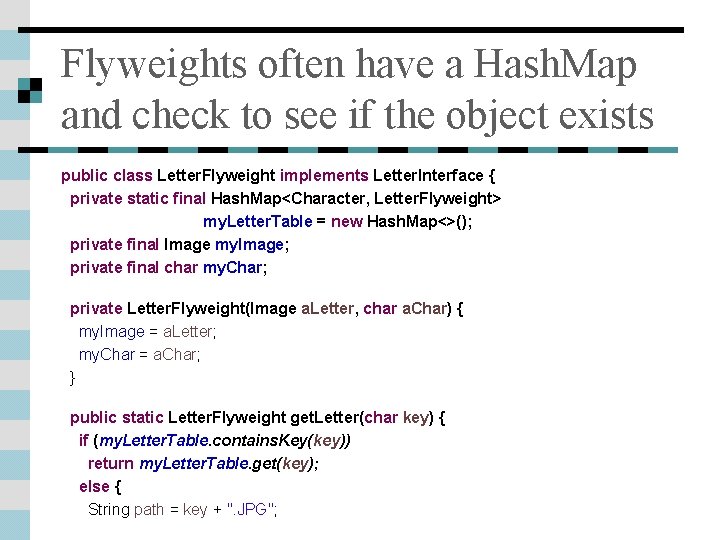
- Slides: 30
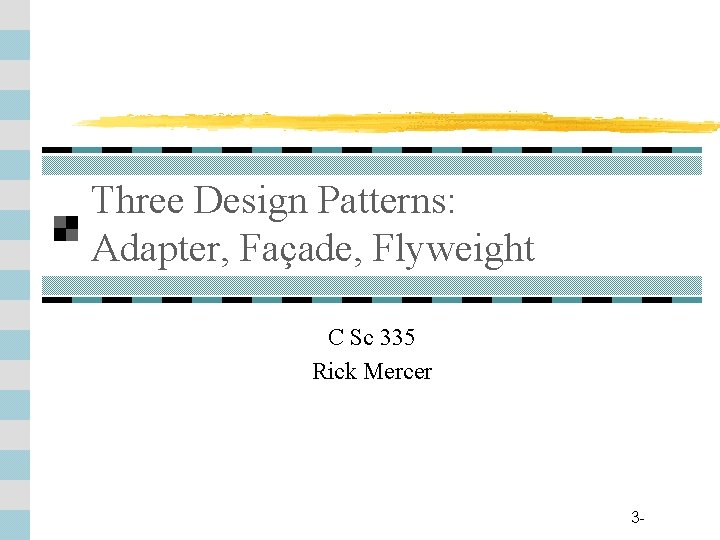
Three Design Patterns: Adapter, Façade, Flyweight C Sc 335 Rick Mercer 3 -
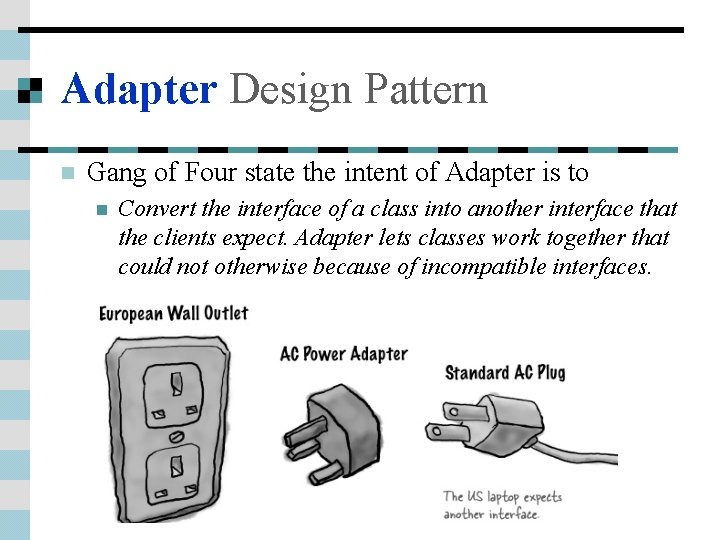
Adapter Design Pattern n Gang of Four state the intent of Adapter is to n Convert the interface of a class into another interface that the clients expect. Adapter lets classes work together that could not otherwise because of incompatible interfaces.
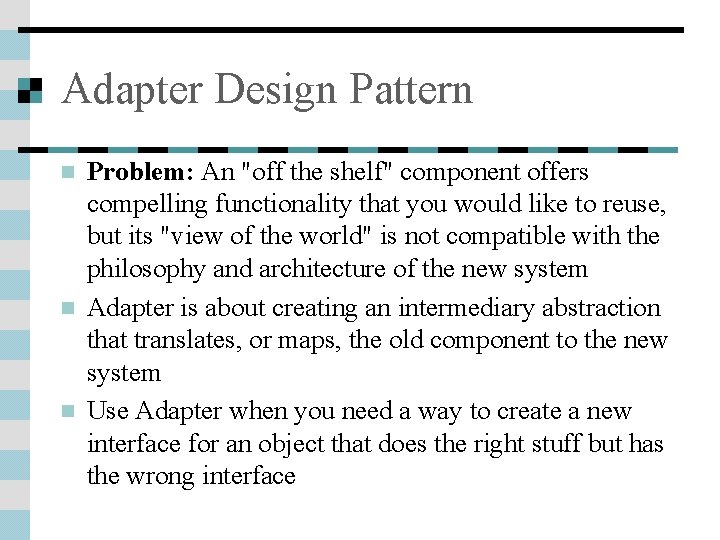
Adapter Design Pattern n Problem: An "off the shelf" component offers compelling functionality that you would like to reuse, but its "view of the world" is not compatible with the philosophy and architecture of the new system Adapter is about creating an intermediary abstraction that translates, or maps, the old component to the new system Use Adapter when you need a way to create a new interface for an object that does the right stuff but has the wrong interface
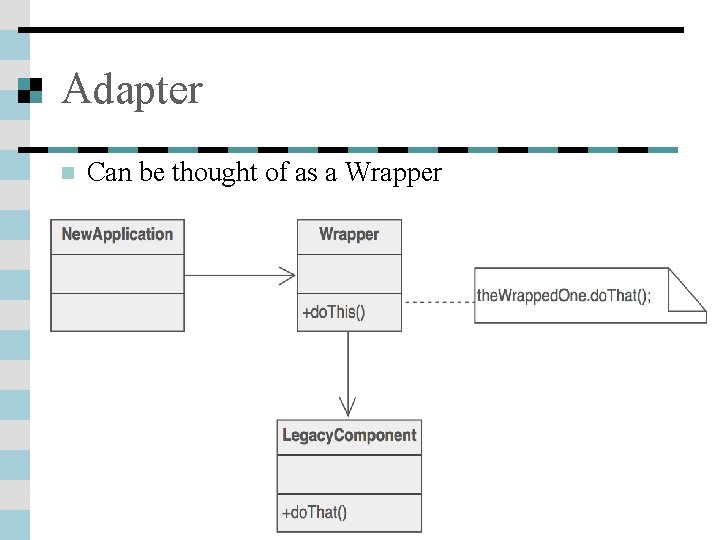
Adapter n Can be thought of as a Wrapper
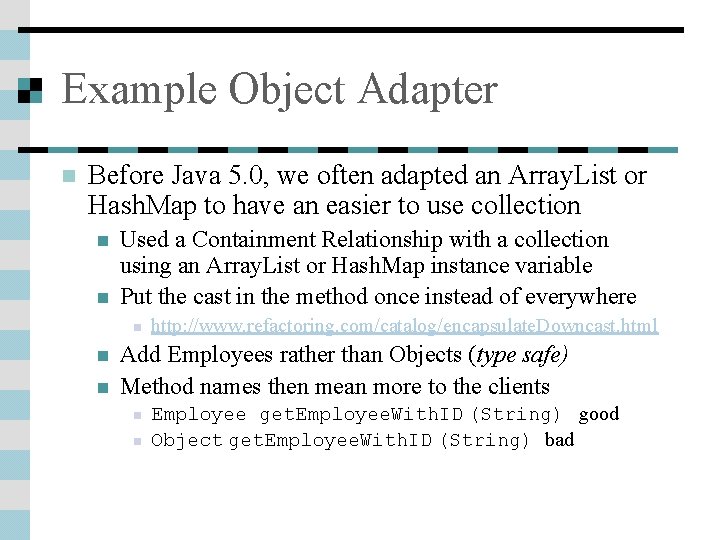
Example Object Adapter n Before Java 5. 0, we often adapted an Array. List or Hash. Map to have an easier to use collection n n Used a Containment Relationship with a collection using an Array. List or Hash. Map instance variable Put the cast in the method once instead of everywhere n n n http: //www. refactoring. com/catalog/encapsulate. Downcast. html Add Employees rather than Objects (type safe) Method names then mean more to the clients n n Employee get. Employee. With. ID (String) good Object get. Employee. With. ID (String) bad
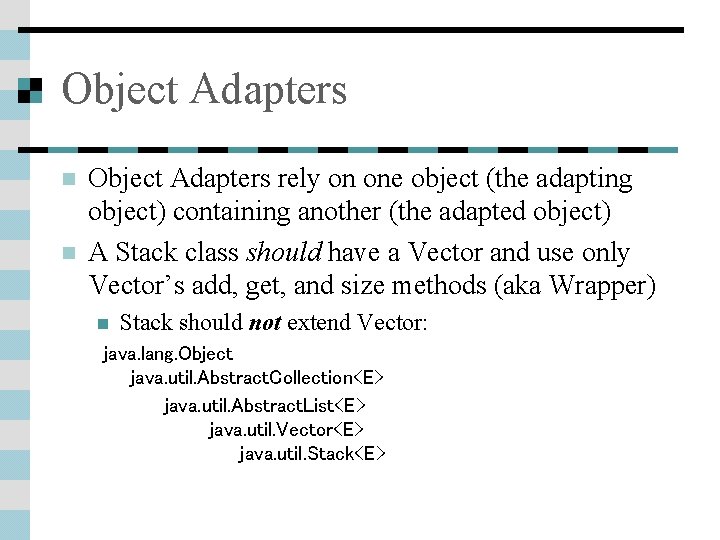
Object Adapters n n Object Adapters rely on one object (the adapting object) containing another (the adapted object) A Stack class should have a Vector and use only Vector’s add, get, and size methods (aka Wrapper) n Stack should not extend Vector: java. lang. Object java. util. Abstract. Collection<E> java. util. Abstract. List<E> java. util. Vector<E> java. util. Stack<E>
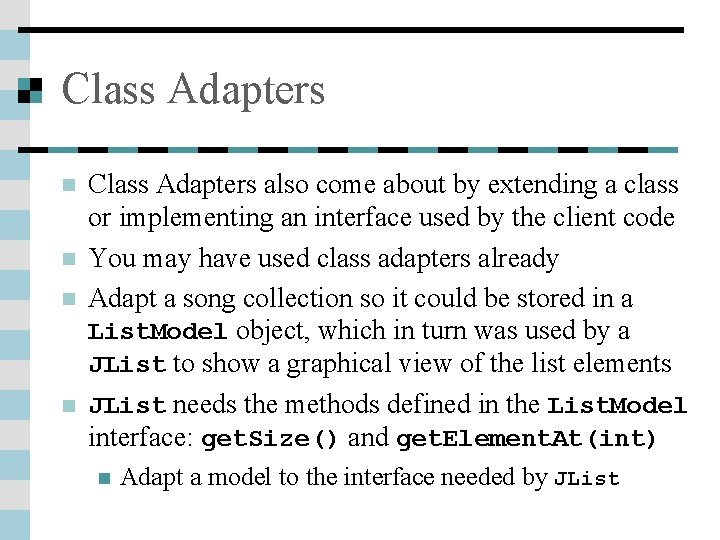
Class Adapters n n Class Adapters also come about by extending a class or implementing an interface used by the client code You may have used class adapters already Adapt a song collection so it could be stored in a List. Model object, which in turn was used by a JList to show a graphical view of the list elements JList needs the methods defined in the List. Model interface: get. Size() and get. Element. At(int) n Adapt a model to the interface needed by JList
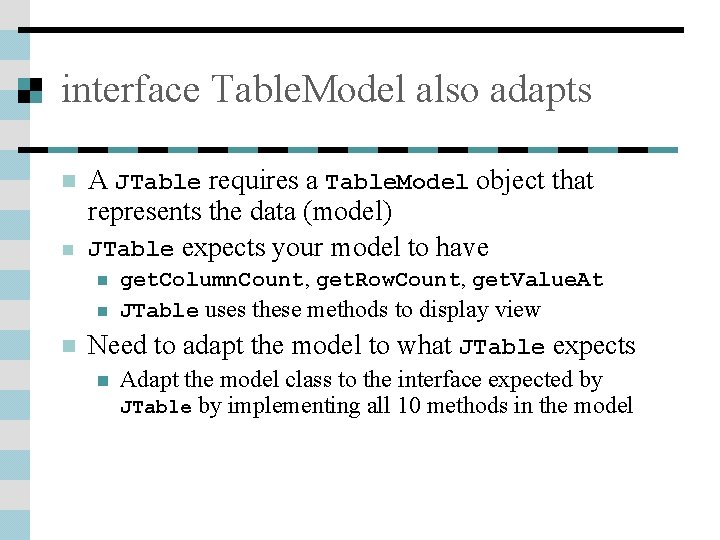
interface Table. Model also adapts n n A JTable requires a Table. Model object that represents the data (model) JTable expects your model to have n n n get. Column. Count, get. Row. Count, get. Value. At JTable uses these methods to display view Need to adapt the model to what JTable expects n Adapt the model class to the interface expected by JTable by implementing all 10 methods in the model
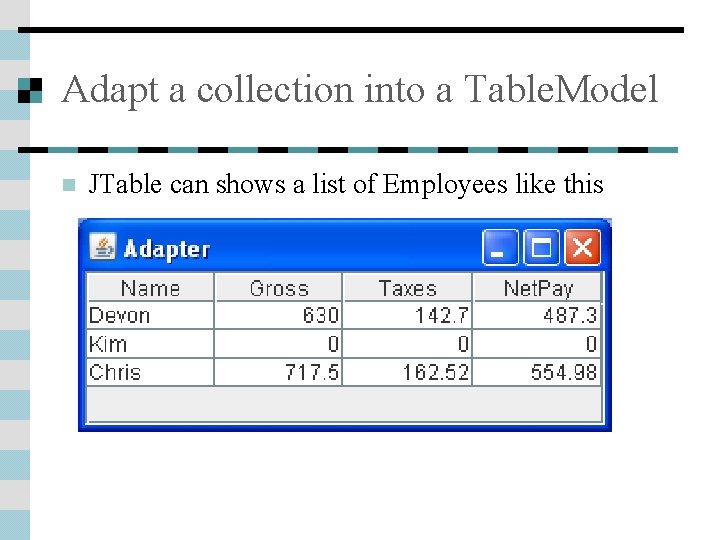
Adapt a collection into a Table. Model n JTable can shows a list of Employees like this
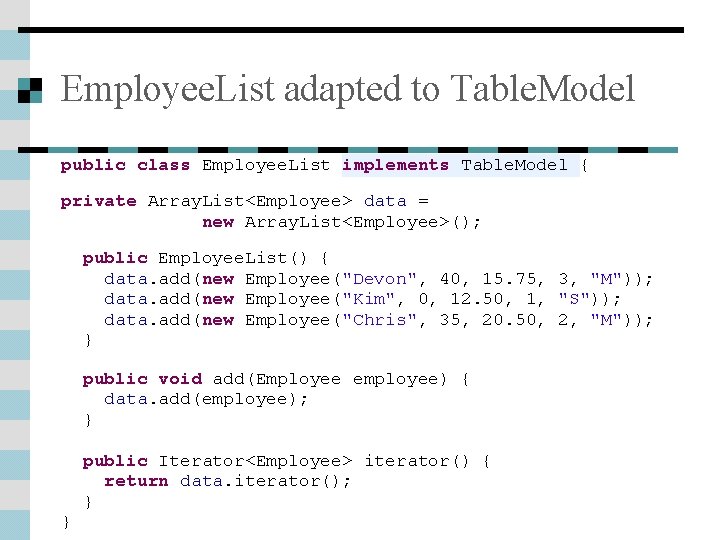
Employee. List adapted to Table. Model public class Employee. List implements Table. Model { private Array. List<Employee> data = new Array. List<Employee>(); public Employee. List() { data. add(new Employee("Devon", 40, 15. 75, 3, "M")); data. add(new Employee("Kim", 0, 12. 50, 1, "S")); data. add(new Employee("Chris", 35, 20. 50, 2, "M")); } public void add(Employee employee) { data. add(employee); } public Iterator<Employee> iterator() { return data. iterator(); } }
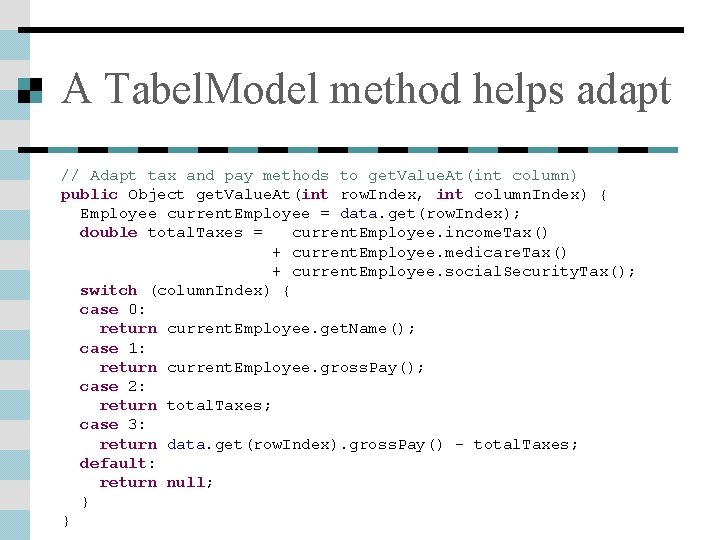
A Tabel. Model method helps adapt // Adapt tax and pay methods to get. Value. At(int column) public Object get. Value. At(int row. Index, int column. Index) { Employee current. Employee = data. get(row. Index); double total. Taxes = current. Employee. income. Tax() + current. Employee. medicare. Tax() + current. Employee. social. Security. Tax(); switch (column. Index) { case 0: return current. Employee. get. Name(); case 1: return current. Employee. gross. Pay(); case 2: return total. Taxes; case 3: return data. get(row. Index). gross. Pay() - total. Taxes; default: return null; } }
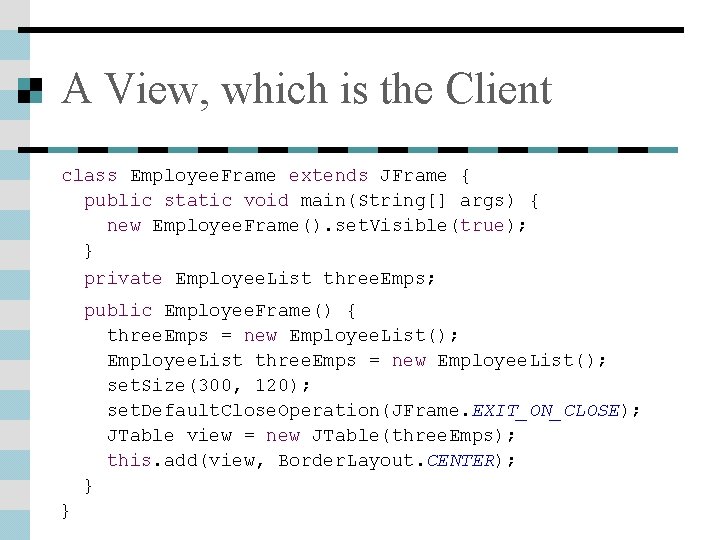
A View, which is the Client class Employee. Frame extends JFrame { public static void main(String[] args) { new Employee. Frame(). set. Visible(true); } private Employee. List three. Emps; public Employee. Frame() { three. Emps = new Employee. List(); Employee. List three. Emps = new Employee. List(); set. Size(300, 120); set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); JTable view = new JTable(three. Emps); this. add(view, Border. Layout. CENTER); } }
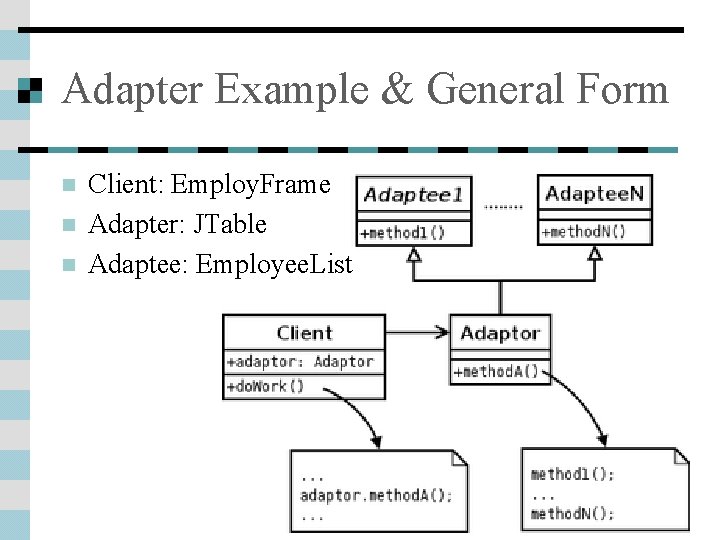
Adapter Example & General Form n n n Client: Employ. Frame Adapter: JTable Adaptee: Employee. List
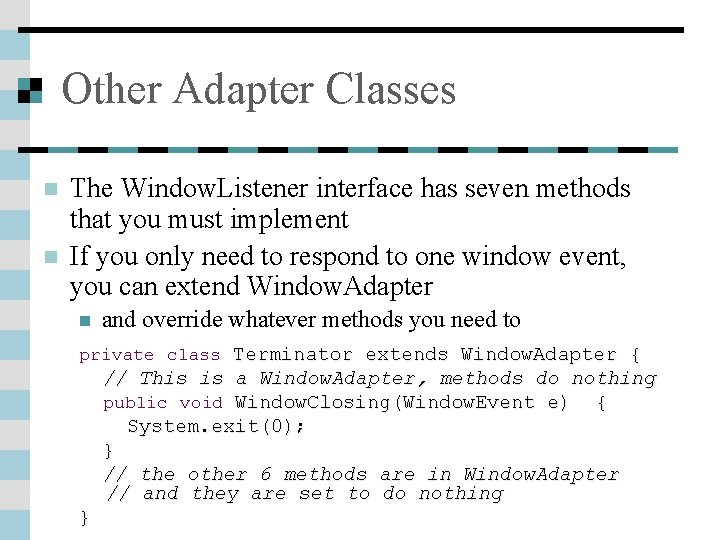
Other Adapter Classes n n The Window. Listener interface has seven methods that you must implement If you only need to respond to one window event, you can extend Window. Adapter n and override whatever methods you need to private class Terminator extends Window. Adapter { // This is a Window. Adapter, methods do nothing public void Window. Closing(Window. Event e) { System. exit(0); } // the other 6 methods are in Window. Adapter // and they are set to do nothing }
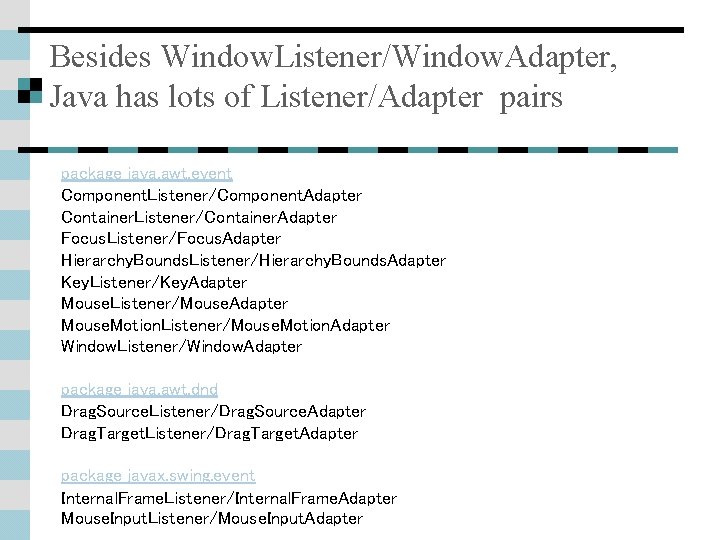
Besides Window. Listener/Window. Adapter, Java has lots of Listener/Adapter pairs package java. awt. event Component. Listener/Component. Adapter Container. Listener/Container. Adapter Focus. Listener/Focus. Adapter Hierarchy. Bounds. Listener/Hierarchy. Bounds. Adapter Key. Listener/Key. Adapter Mouse. Listener/Mouse. Adapter Mouse. Motion. Listener/Mouse. Motion. Adapter Window. Listener/Window. Adapter package java. awt. dnd Drag. Source. Listener/Drag. Source. Adapter Drag. Target. Listener/Drag. Target. Adapter package javax. swing. event Internal. Frame. Listener/Internal. Frame. Adapter Mouse. Input. Listener/Mouse. Input. Adapter
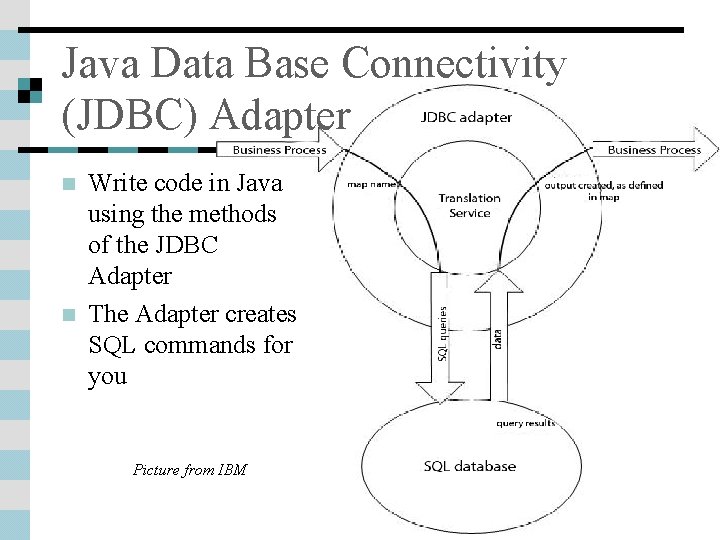
Java Data Base Connectivity (JDBC) Adapter n n Write code in Java using the methods of the JDBC Adapter The Adapter creates SQL commands for you Picture from IBM
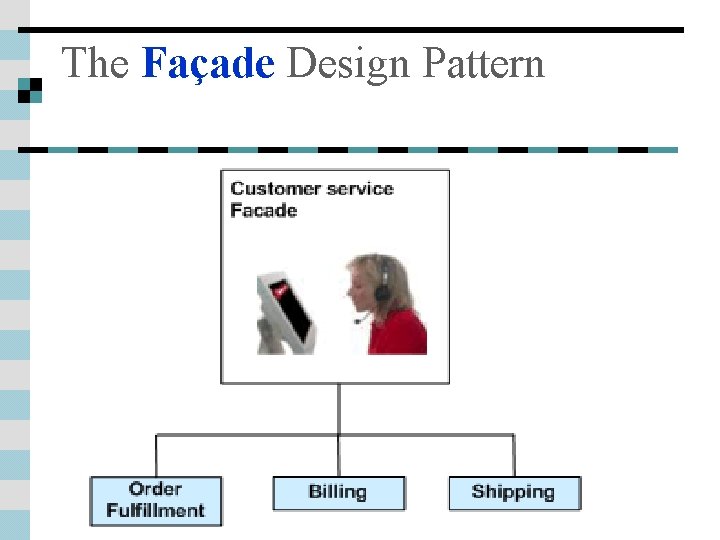
The Façade Design Pattern
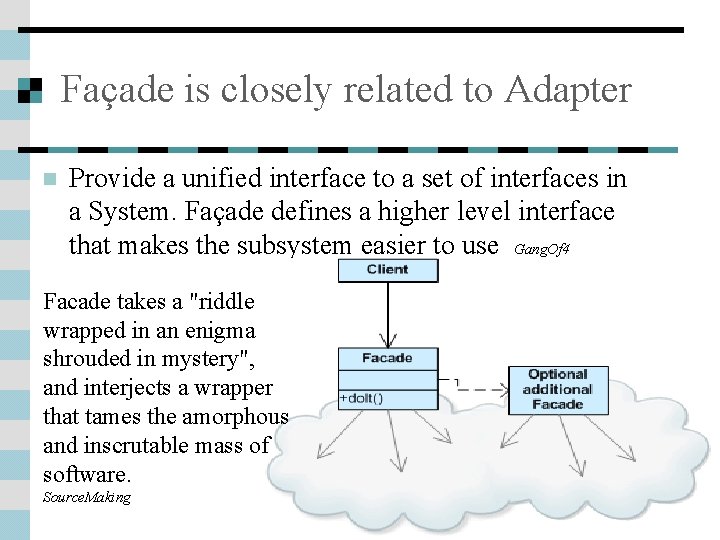
Façade is closely related to Adapter n Provide a unified interface to a set of interfaces in a System. Façade defines a higher level interface that makes the subsystem easier to use Gang. Of 4 Facade takes a "riddle wrapped in an enigma shrouded in mystery", and interjects a wrapper that tames the amorphous and inscrutable mass of software. Source. Making 18
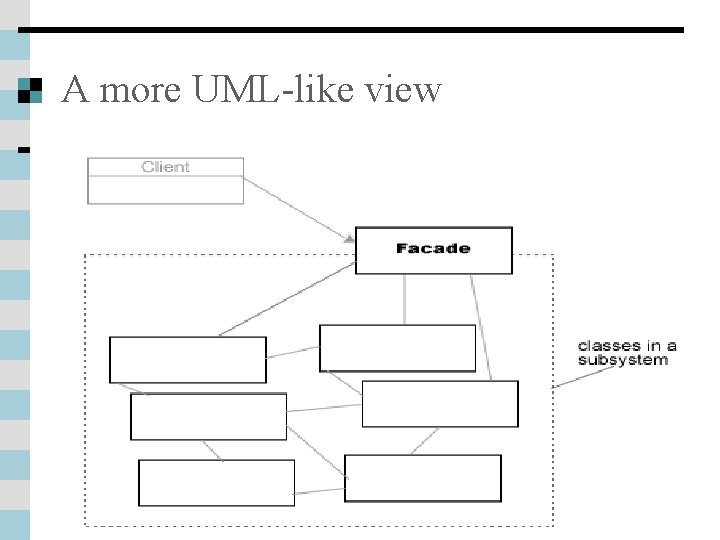
A more UML-like view
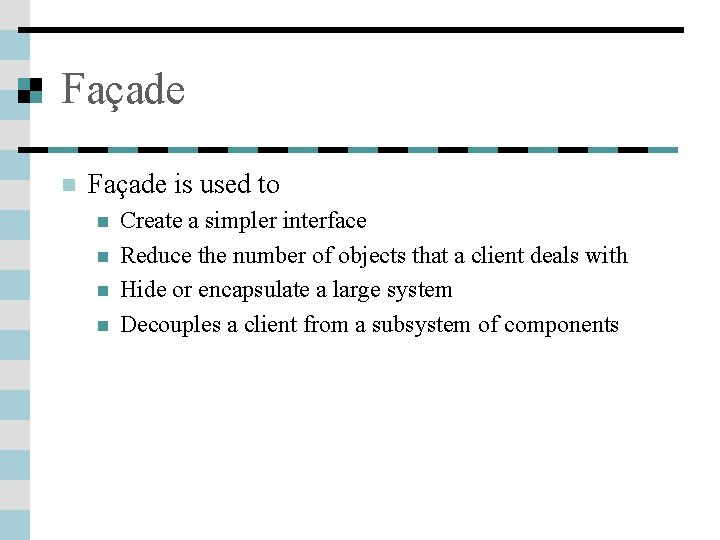
Façade n Façade is used to n n Create a simpler interface Reduce the number of objects that a client deals with Hide or encapsulate a large system Decouples a client from a subsystem of components
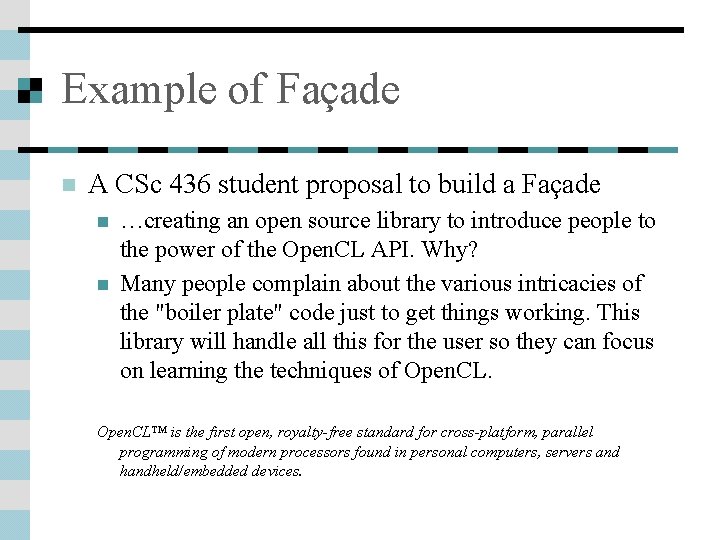
Example of Façade n A CSc 436 student proposal to build a Façade n n …creating an open source library to introduce people to the power of the Open. CL API. Why? Many people complain about the various intricacies of the "boiler plate" code just to get things working. This library will handle all this for the user so they can focus on learning the techniques of Open. CL™ is the first open, royalty-free standard for cross-platform, parallel programming of modern processors found in personal computers, servers and handheld/embedded devices.
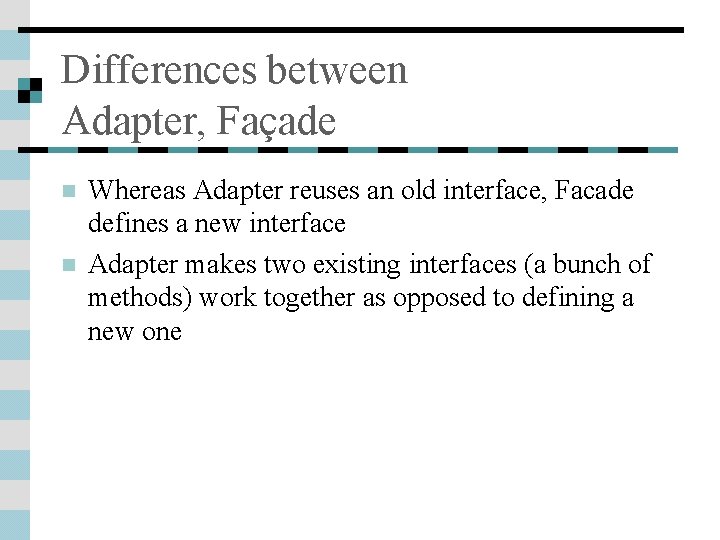
Differences between Adapter, Façade n n Whereas Adapter reuses an old interface, Facade defines a new interface Adapter makes two existing interfaces (a bunch of methods) work together as opposed to defining a new one
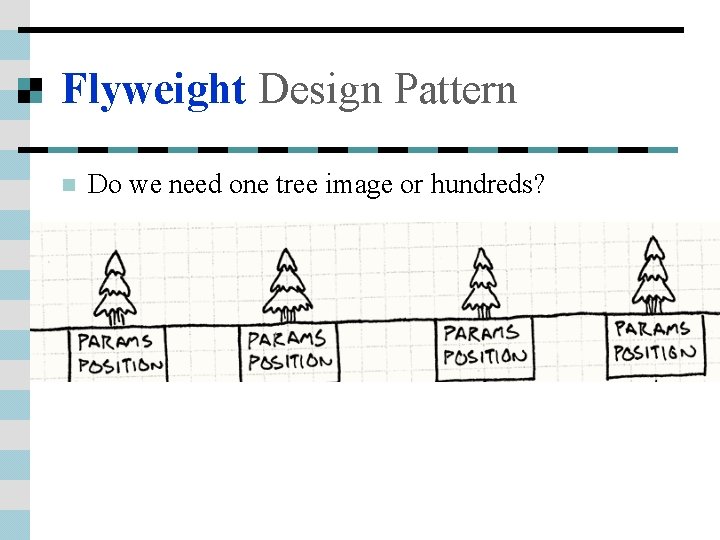
Flyweight Design Pattern n Do we need one tree image or hundreds?
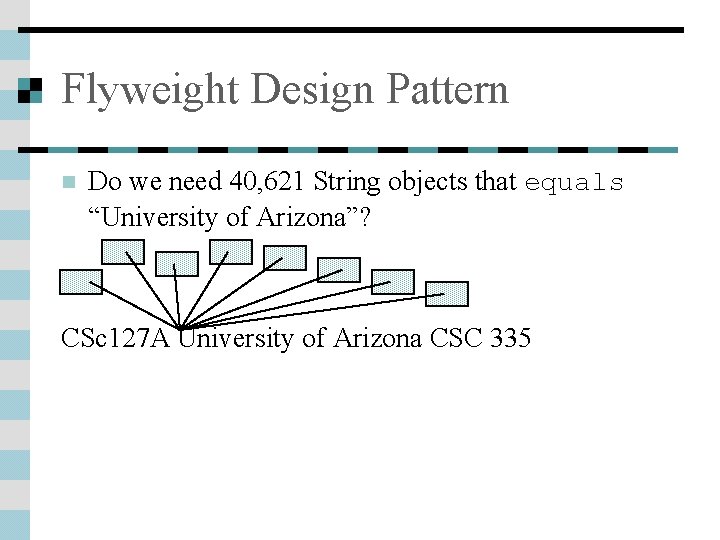
Flyweight Design Pattern n Do we need 40, 621 String objects that equals “University of Arizona”? CSc 127 A University of Arizona CSC 335
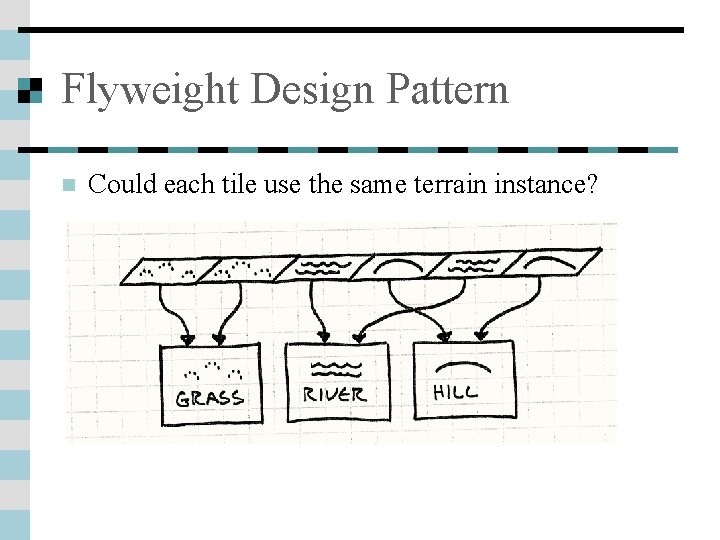
Flyweight Design Pattern n Could each tile use the same terrain instance?
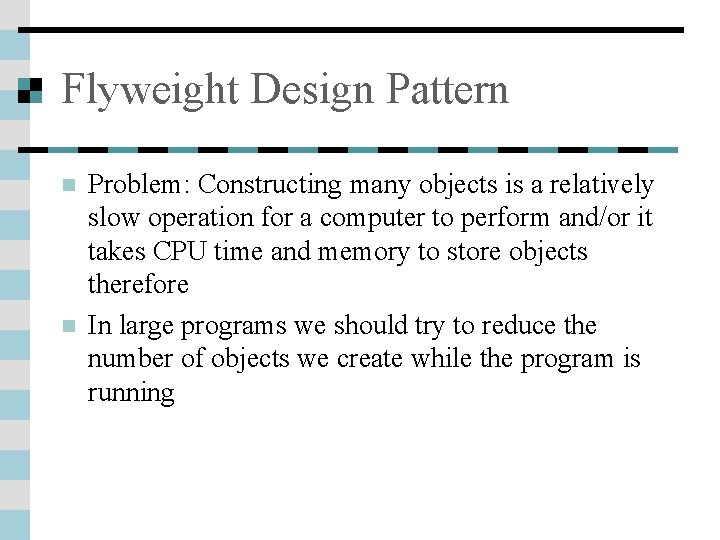
Flyweight Design Pattern n n Problem: Constructing many objects is a relatively slow operation for a computer to perform and/or it takes CPU time and memory to store objects therefore In large programs we should try to reduce the number of objects we create while the program is running
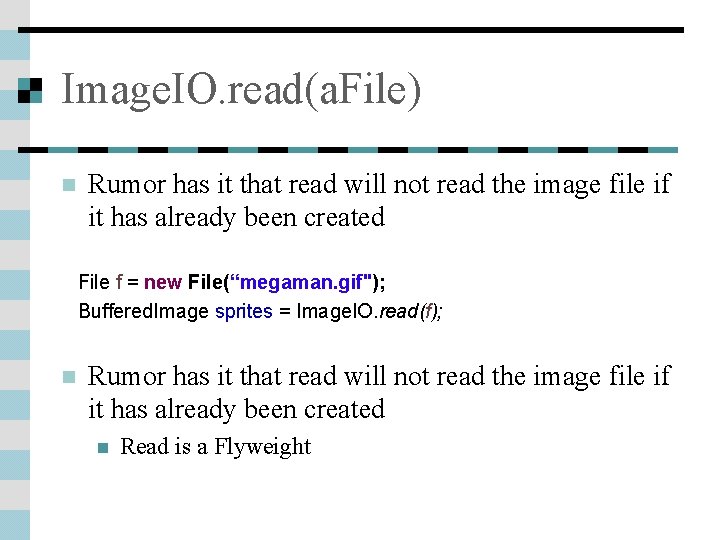
Image. IO. read(a. File) n Rumor has it that read will not read the image file if it has already been created File f = new File(“megaman. gif"); Buffered. Image sprites = Image. IO. read(f); n Rumor has it that read will not read the image file if it has already been created n Read is a Flyweight
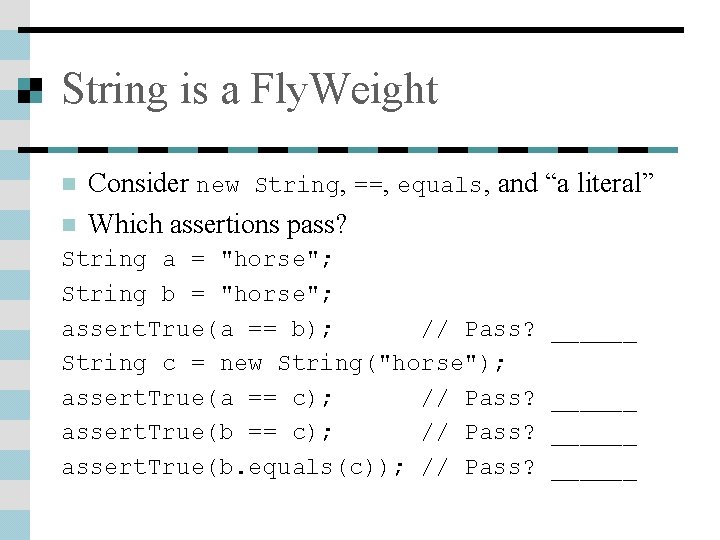
String is a Fly. Weight n n Consider new String, ==, equals, and “a literal” Which assertions pass? String a = "horse"; String b = "horse"; assert. True(a == b); // Pass? String c = new String("horse"); assert. True(a == c); // Pass? assert. True(b. equals(c)); // Pass? ______
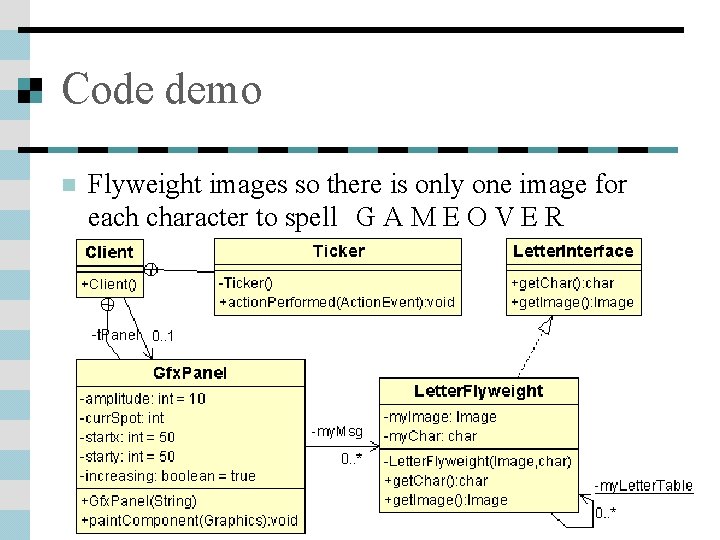
Code demo n Flyweight images so there is only one image for each character to spell G A M E O V E R
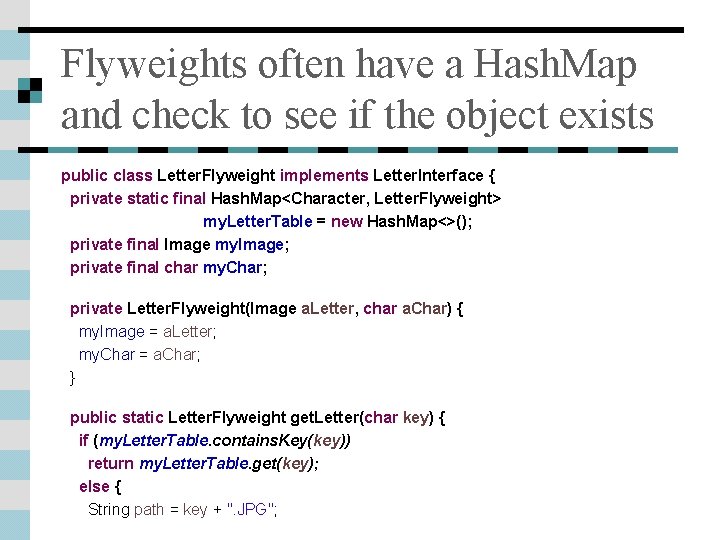
Flyweights often have a Hash. Map and check to see if the object exists public class Letter. Flyweight implements Letter. Interface { private static final Hash. Map<Character, Letter. Flyweight> my. Letter. Table = new Hash. Map<>(); private final Image my. Image; private final char my. Char; private Letter. Flyweight(Image a. Letter, char a. Char) { my. Image = a. Letter; my. Char = a. Char; } public static Letter. Flyweight get. Letter(char key) { if (my. Letter. Table. contains. Key(key)) return my. Letter. Table. get(key); else { String path = key + ". JPG";
Ex-link ( rs-232c ) samsung
Faade
Dot
Flyweight vs singleton
Flyweight example
Obj dating
X videos
Kentico sap adapter
Fujitsu wlan adapter
Moodle sis integration
Unsymmetriedämpfung
Entwurfsmuster adapter
Tests the basic functionality of computer ports
Adapter pattern c
Mouse adapter class in java
Planet usb wifi adapter driver
Shoretel workgroup real time monitor
Coaxial network adapter
Boston scientific latitude wireless internet adapter
Esb adapter
Kentico dynamics ax adapter
Eai adapter
Is samsung a cloner imitator or adapter
Mouse adapter java
Vga video graphics adapter
Adapter vs strategy pattern
Avaya cti adapter for salesforce
Cisco sta1520
Storage area network emc
A network adapter also called a ____
Sitecore dynamics gp adapter