Threads CSIT 402 Data Structures II Multithreading models
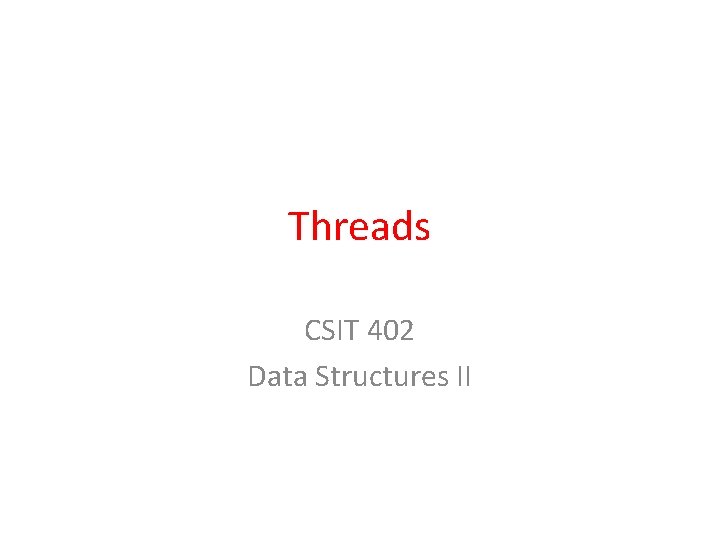
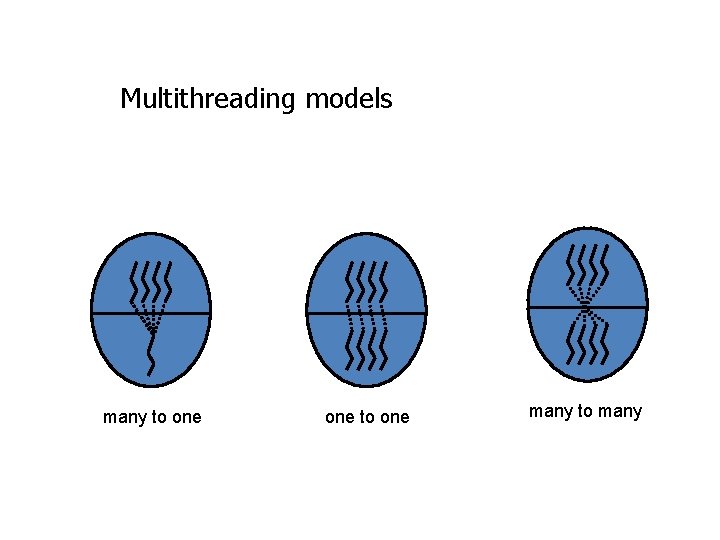
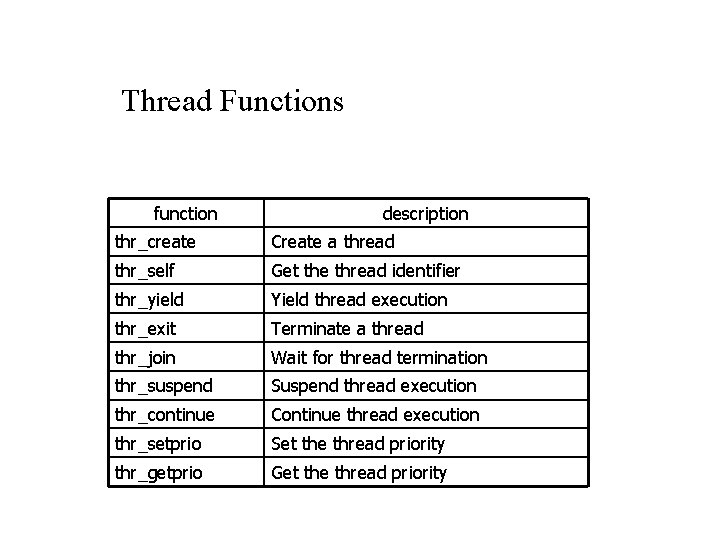
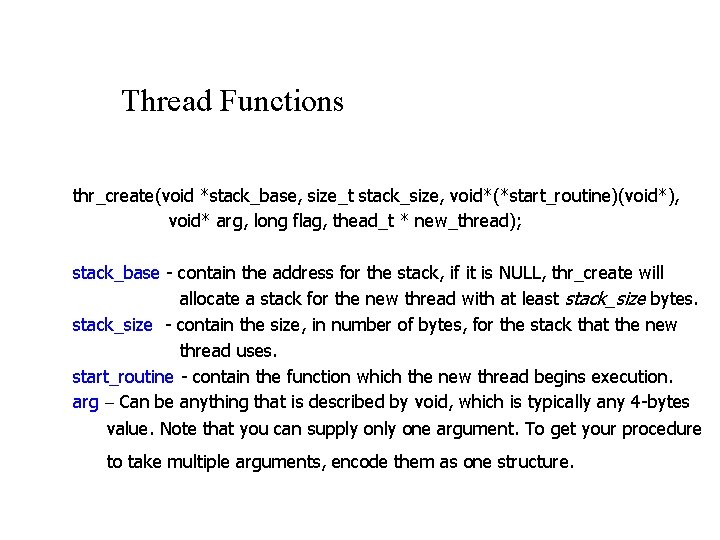
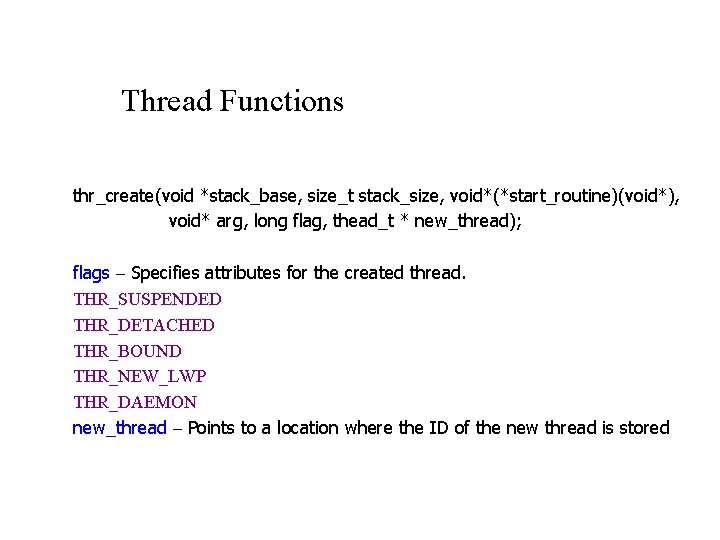
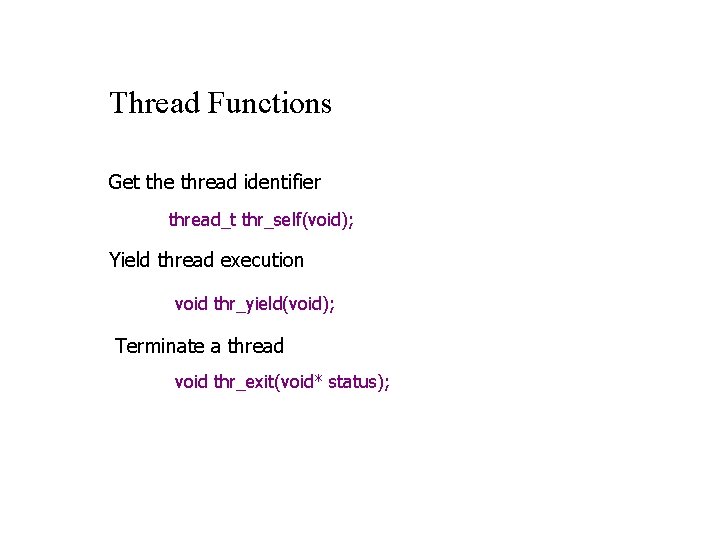
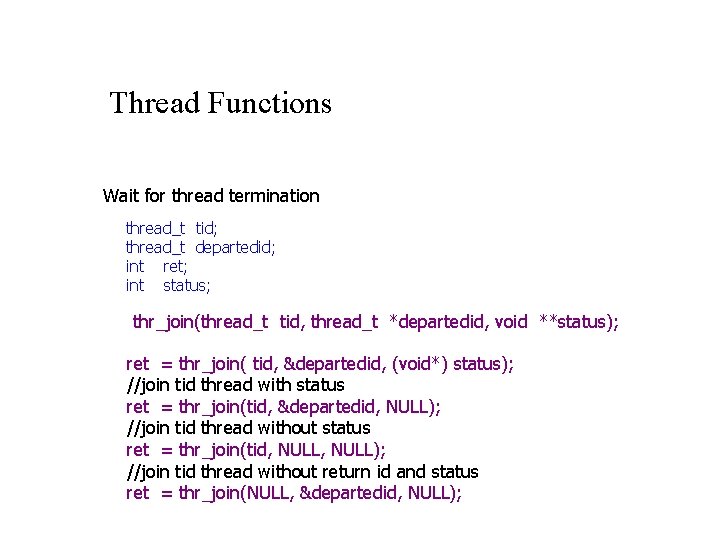
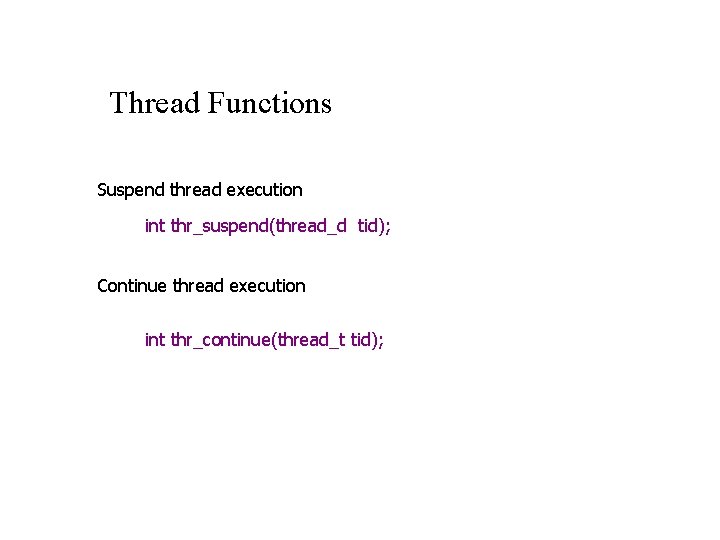
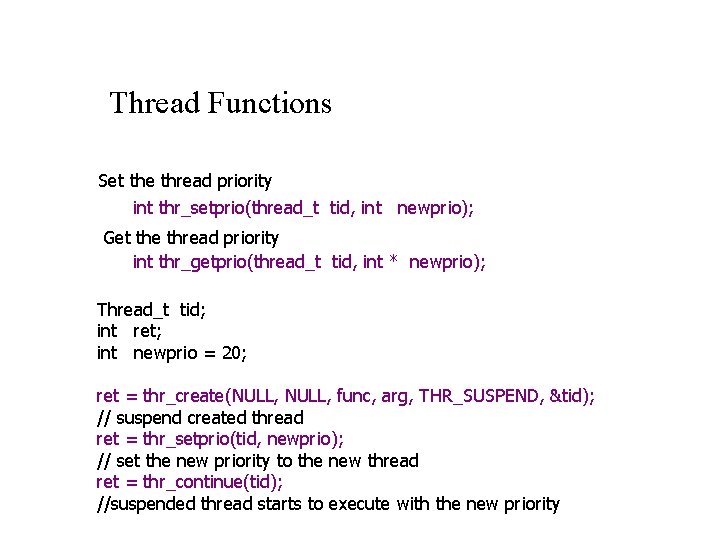
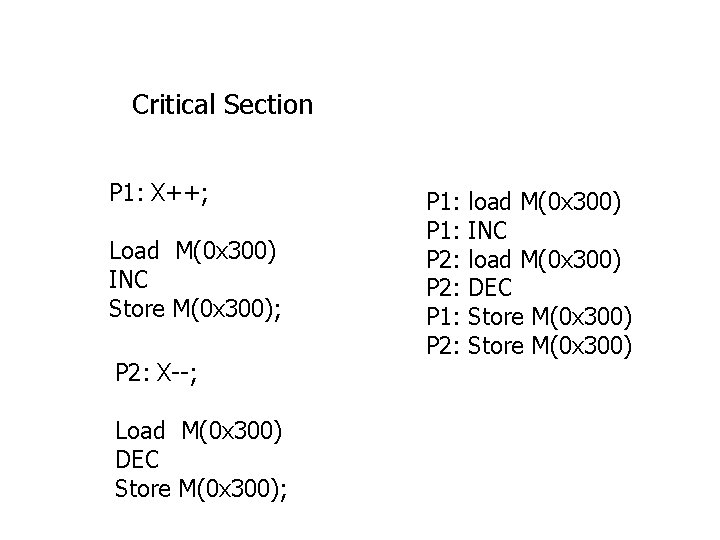
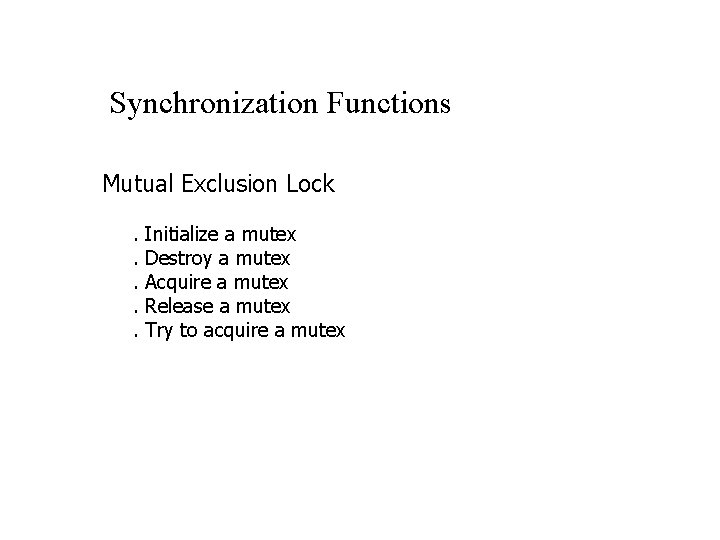
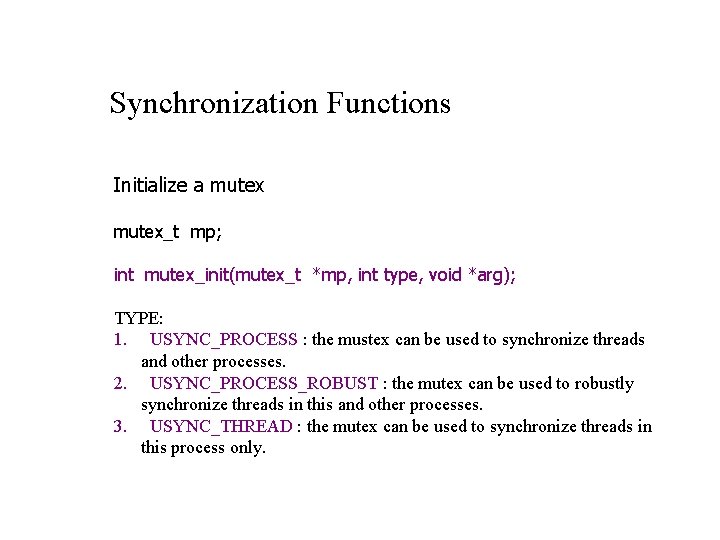
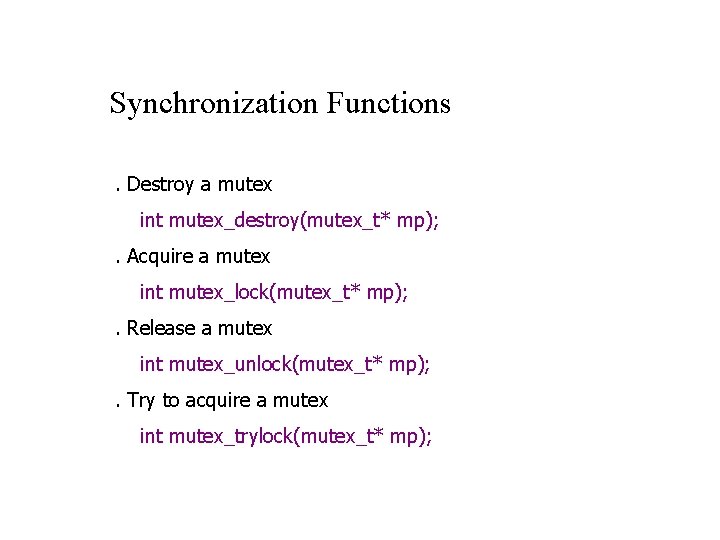
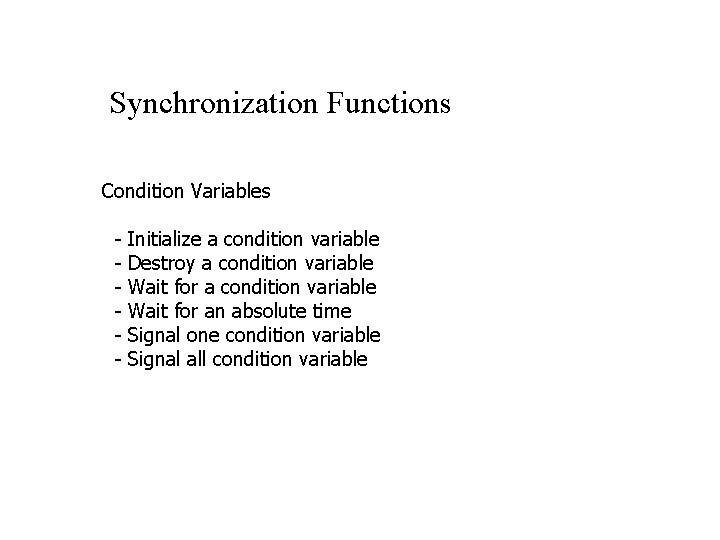
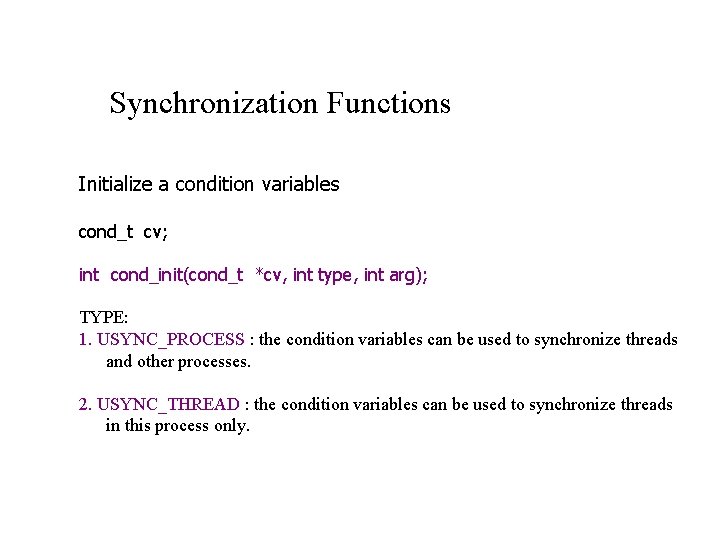
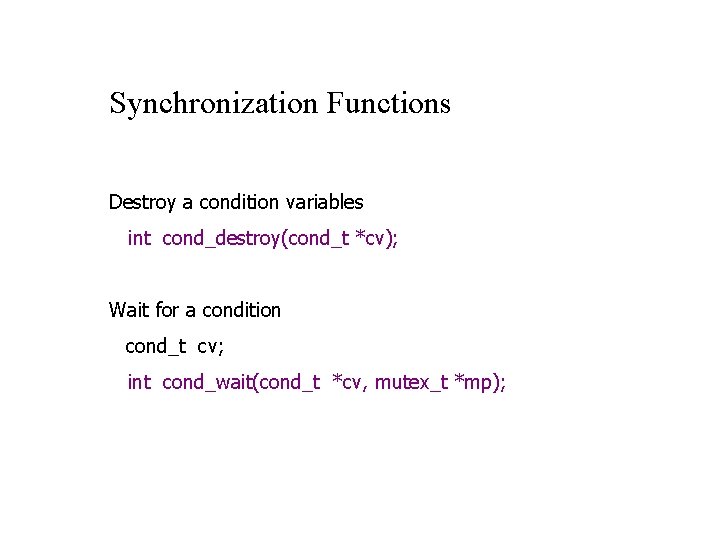
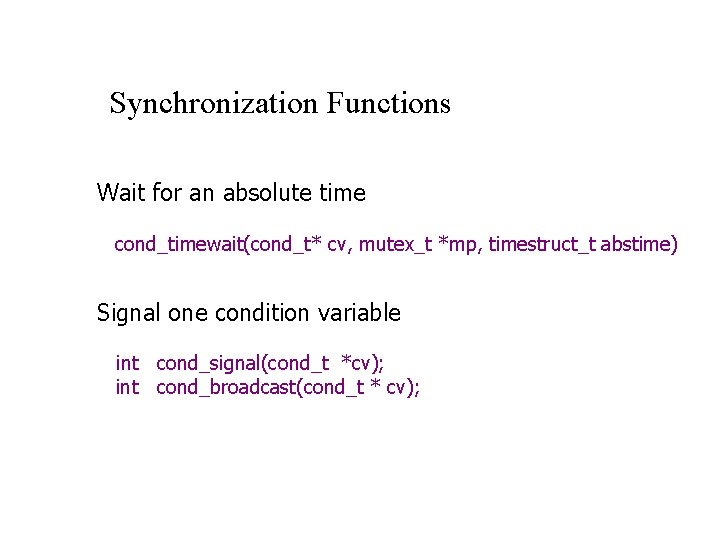
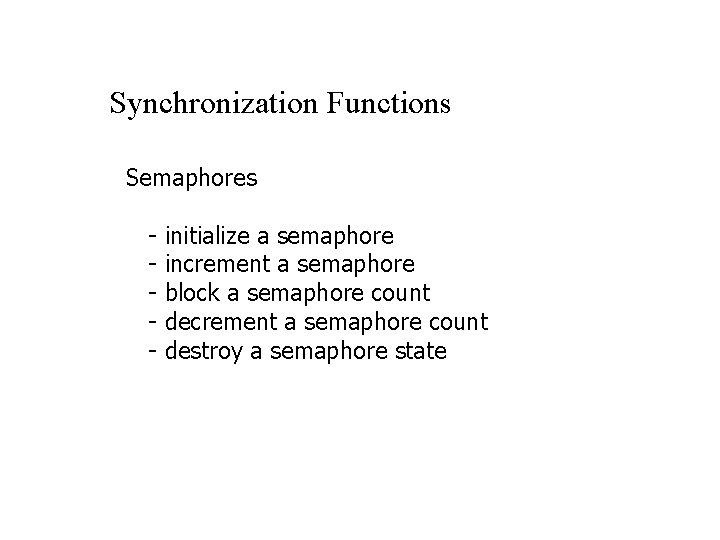
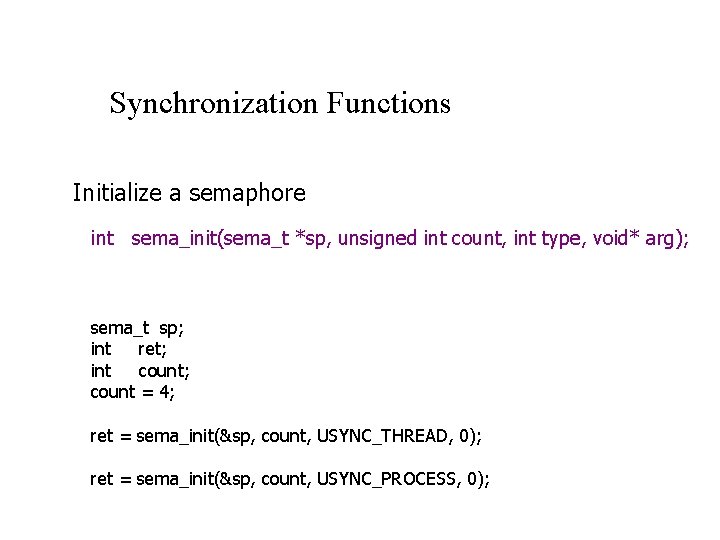
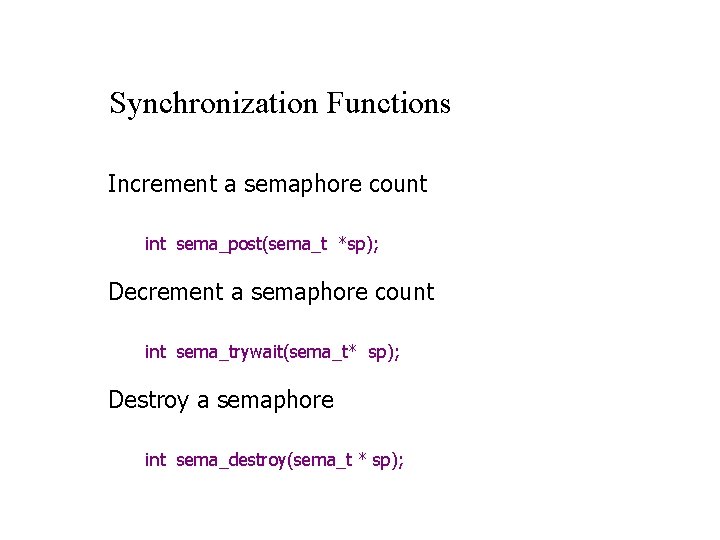
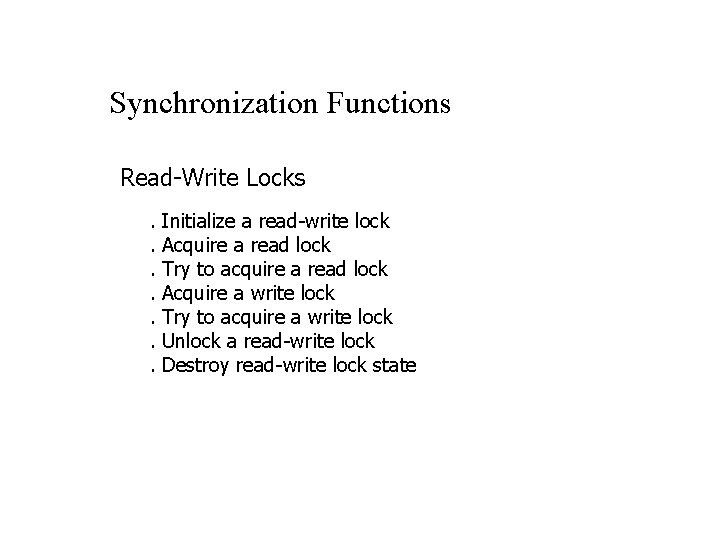
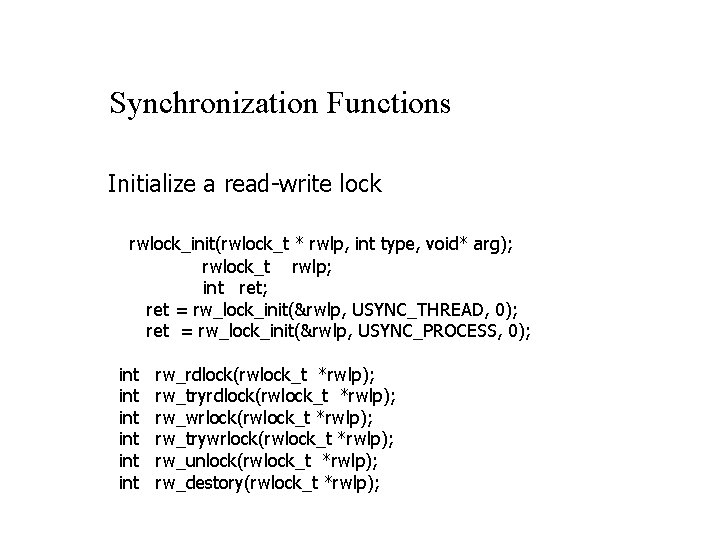
- Slides: 22
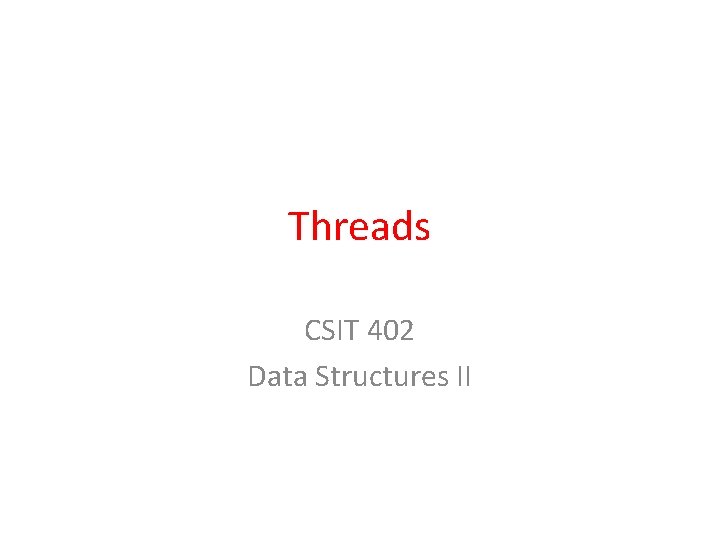
Threads CSIT 402 Data Structures II
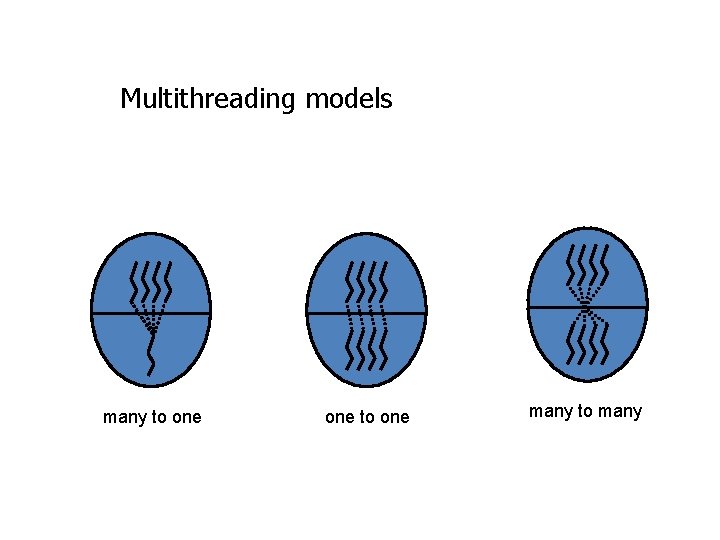
Multithreading models many to one many to many
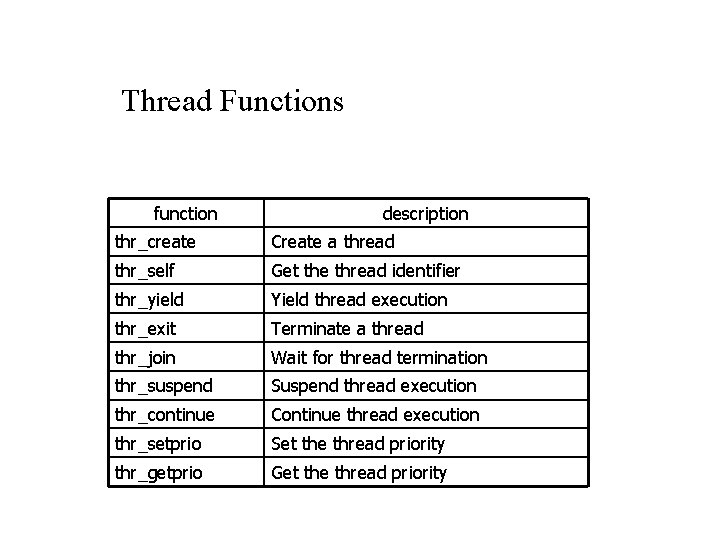
Thread Functions function description thr_create Create a thread thr_self Get the thread identifier thr_yield Yield thread execution thr_exit Terminate a thread thr_join Wait for thread termination thr_suspend Suspend thread execution thr_continue Continue thread execution thr_setprio Set the thread priority thr_getprio Get the thread priority
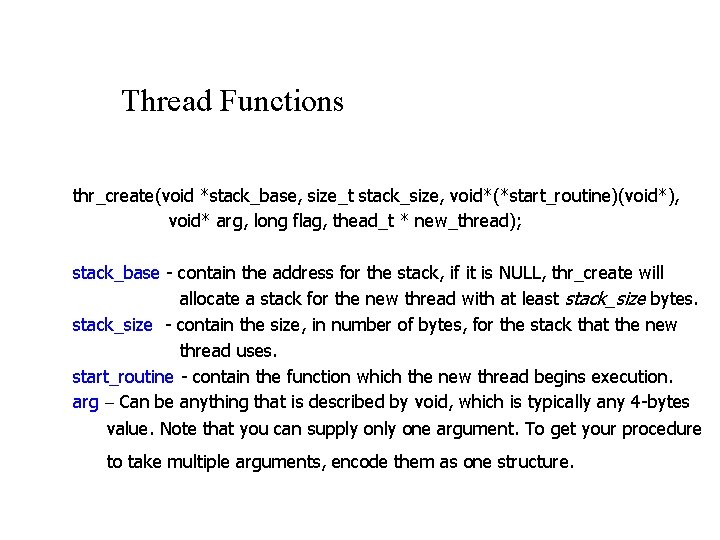
Thread Functions thr_create(void *stack_base, size_t stack_size, void*(*start_routine)(void*), void* arg, long flag, thead_t * new_thread); stack_base - contain the address for the stack, if it is NULL, thr_create will allocate a stack for the new thread with at least stack_size bytes. stack_size - contain the size, in number of bytes, for the stack that the new thread uses. start_routine - contain the function which the new thread begins execution. arg – Can be anything that is described by void, which is typically any 4 -bytes value. Note that you can supply one argument. To get your procedure to take multiple arguments, encode them as one structure.
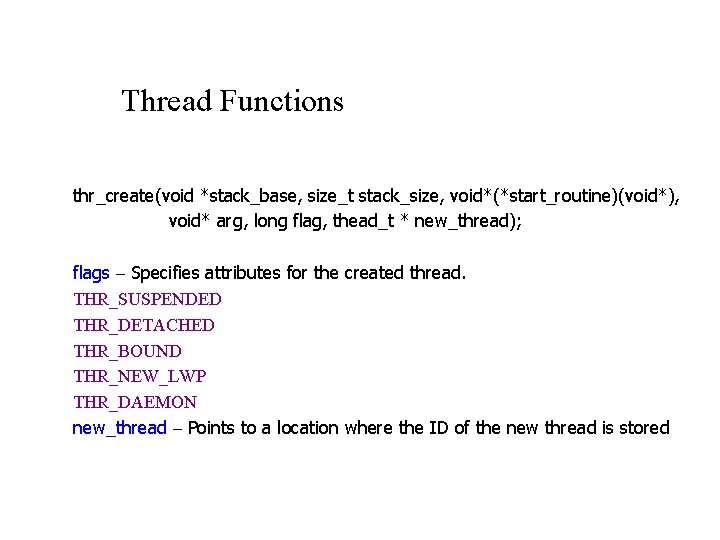
Thread Functions thr_create(void *stack_base, size_t stack_size, void*(*start_routine)(void*), void* arg, long flag, thead_t * new_thread); flags – Specifies attributes for the created thread. THR_SUSPENDED THR_DETACHED THR_BOUND THR_NEW_LWP THR_DAEMON new_thread – Points to a location where the ID of the new thread is stored
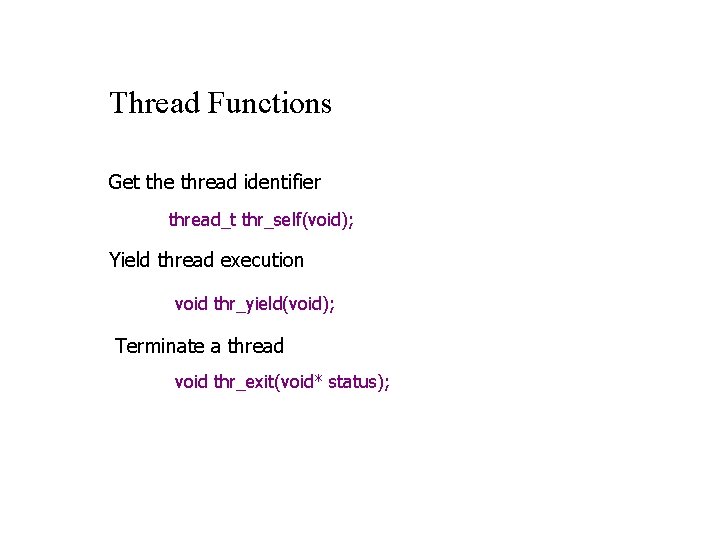
Thread Functions Get the thread identifier thread_t thr_self(void); Yield thread execution void thr_yield(void); Terminate a thread void thr_exit(void* status);
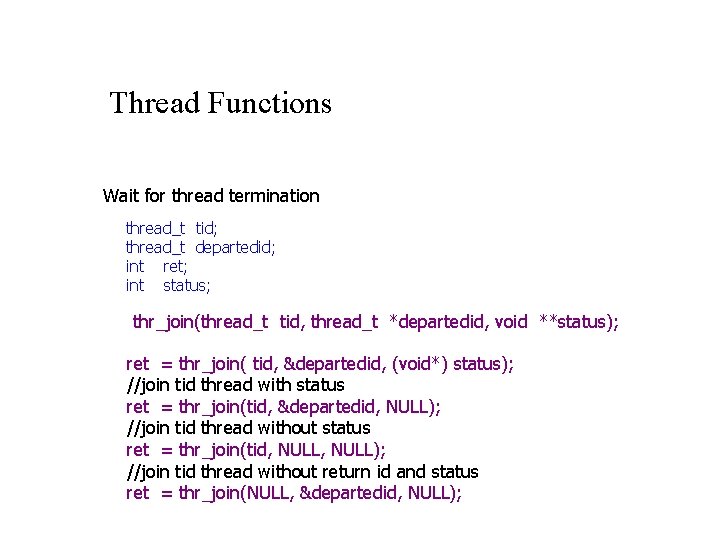
Thread Functions Wait for thread termination thread_t tid; thread_t departedid; int ret; int status; thr_join(thread_t tid, thread_t *departedid, void **status); ret = thr_join( tid, &departedid, (void*) status); //join tid thread with status ret = thr_join(tid, &departedid, NULL); //join tid thread without status ret = thr_join(tid, NULL); //join tid thread without return id and status ret = thr_join(NULL, &departedid, NULL);
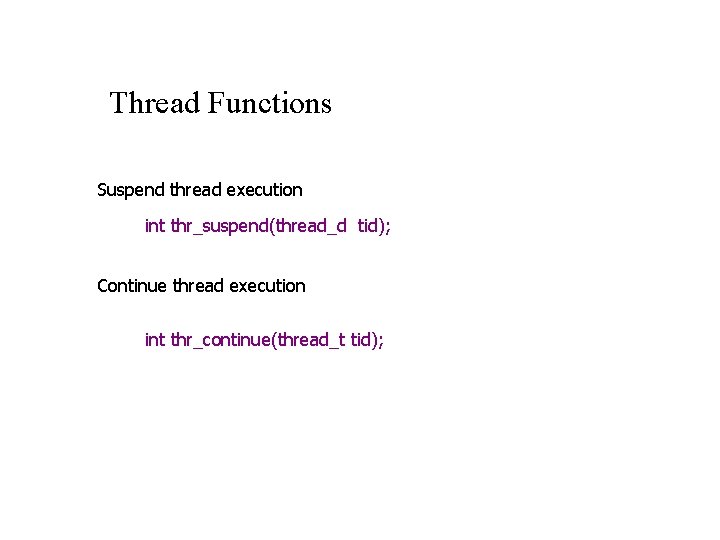
Thread Functions Suspend thread execution int thr_suspend(thread_d tid); Continue thread execution int thr_continue(thread_t tid);
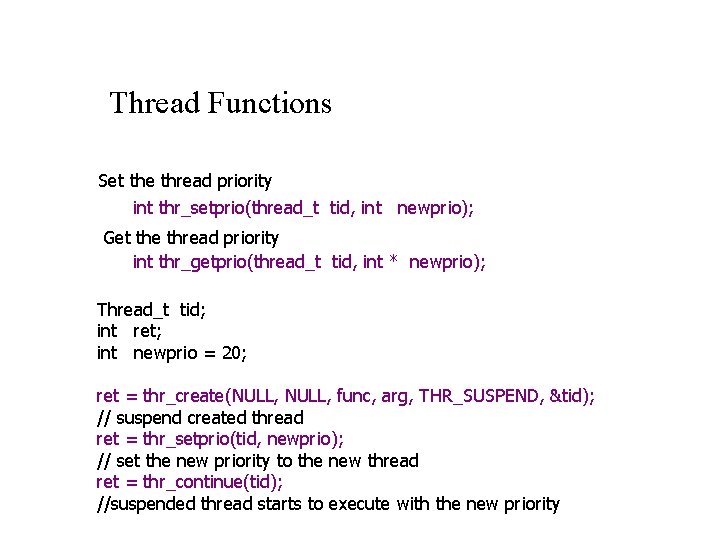
Thread Functions Set the thread priority int thr_setprio(thread_t tid, int newprio); Get the thread priority int thr_getprio(thread_t tid, int * newprio); Thread_t tid; int ret; int newprio = 20; ret = thr_create(NULL, func, arg, THR_SUSPEND, &tid); // suspend created thread ret = thr_setprio(tid, newprio); // set the new priority to the new thread ret = thr_continue(tid); //suspended thread starts to execute with the new priority
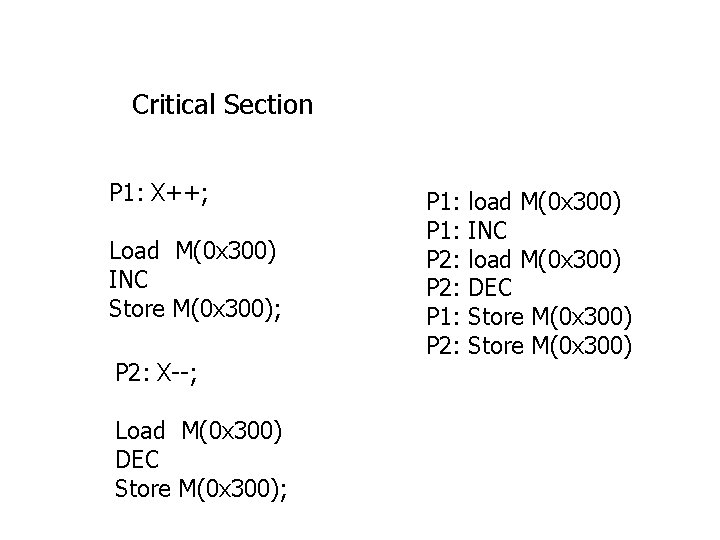
Critical Section P 1: X++; Load M(0 x 300) INC Store M(0 x 300); P 2: X--; Load M(0 x 300) DEC Store M(0 x 300); P 1: P 2: load M(0 x 300) INC load M(0 x 300) DEC Store M(0 x 300)
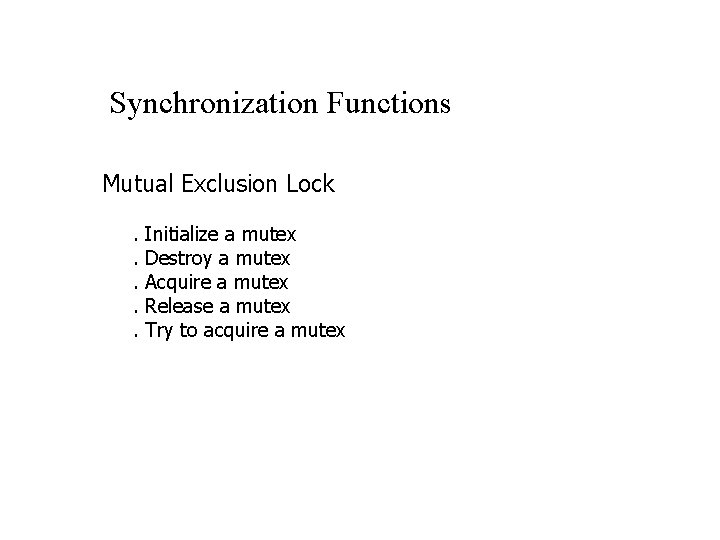
Synchronization Functions Mutual Exclusion Lock. . . Initialize a mutex Destroy a mutex Acquire a mutex Release a mutex Try to acquire a mutex
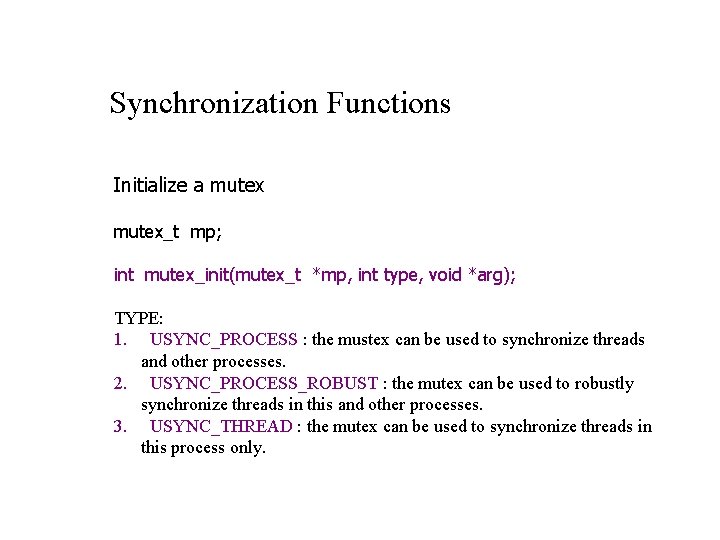
Synchronization Functions Initialize a mutex_t mp; int mutex_init(mutex_t *mp, int type, void *arg); TYPE: 1. USYNC_PROCESS : the mustex can be used to synchronize threads and other processes. 2. USYNC_PROCESS_ROBUST : the mutex can be used to robustly synchronize threads in this and other processes. 3. USYNC_THREAD : the mutex can be used to synchronize threads in this process only.
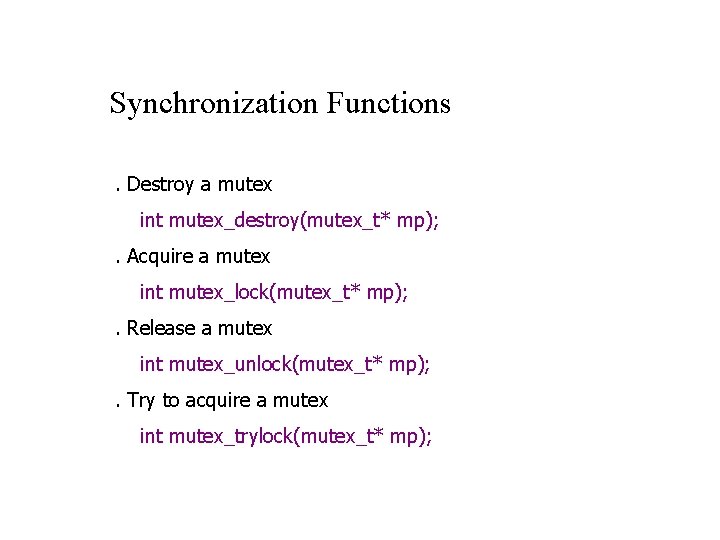
Synchronization Functions. Destroy a mutex int mutex_destroy(mutex_t* mp); . Acquire a mutex int mutex_lock(mutex_t* mp); . Release a mutex int mutex_unlock(mutex_t* mp); . Try to acquire a mutex int mutex_trylock(mutex_t* mp);
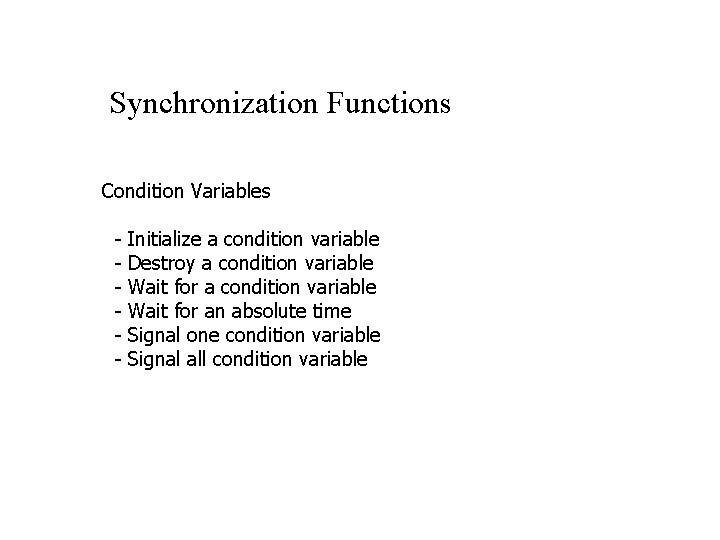
Synchronization Functions Condition Variables - Initialize a condition variable Destroy a condition variable Wait for an absolute time Signal one condition variable Signal all condition variable
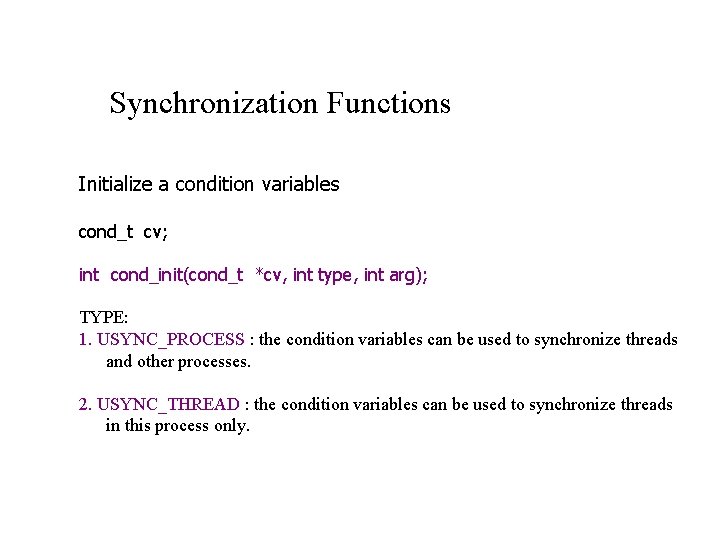
Synchronization Functions Initialize a condition variables cond_t cv; int cond_init(cond_t *cv, int type, int arg); TYPE: 1. USYNC_PROCESS : the condition variables can be used to synchronize threads and other processes. 2. USYNC_THREAD : the condition variables can be used to synchronize threads in this process only.
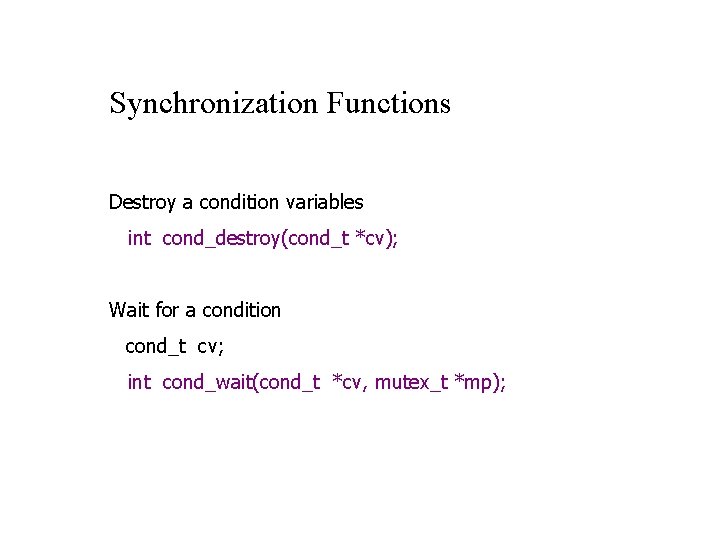
Synchronization Functions Destroy a condition variables int cond_destroy(cond_t *cv); Wait for a condition cond_t cv; int cond_wait(cond_t *cv, mutex_t *mp);
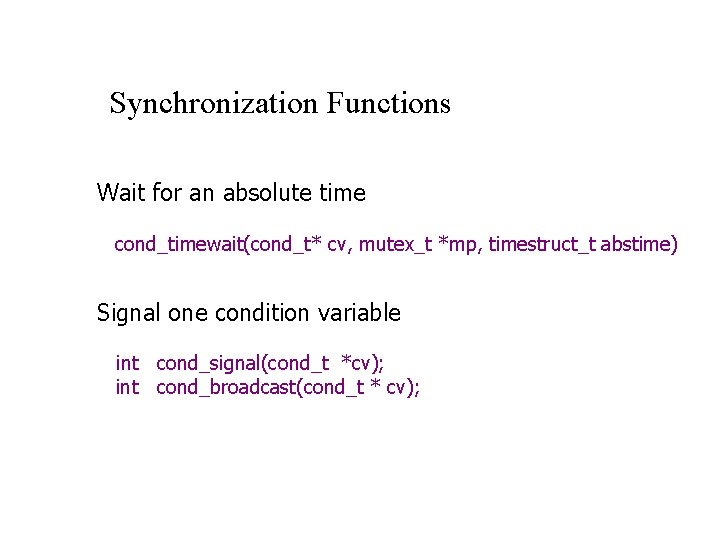
Synchronization Functions Wait for an absolute time cond_timewait(cond_t* cv, mutex_t *mp, timestruct_t abstime) Signal one condition variable int cond_signal(cond_t *cv); int cond_broadcast(cond_t * cv);
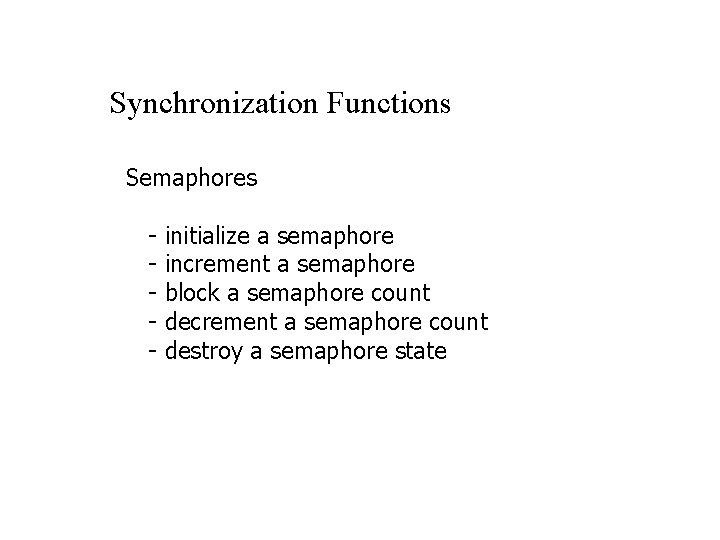
Synchronization Functions Semaphores - initialize a semaphore increment a semaphore block a semaphore count decrement a semaphore count destroy a semaphore state
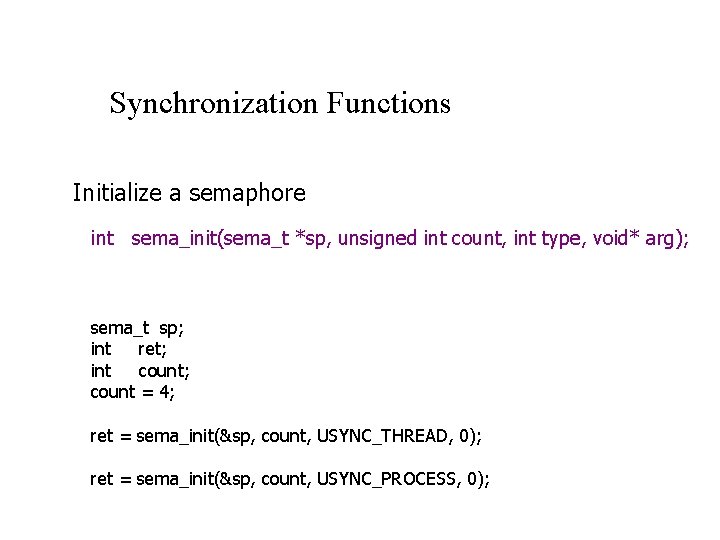
Synchronization Functions Initialize a semaphore int sema_init(sema_t *sp, unsigned int count, int type, void* arg); sema_t sp; int ret; int count; count = 4; ret = sema_init(&sp, count, USYNC_THREAD, 0); ret = sema_init(&sp, count, USYNC_PROCESS, 0);
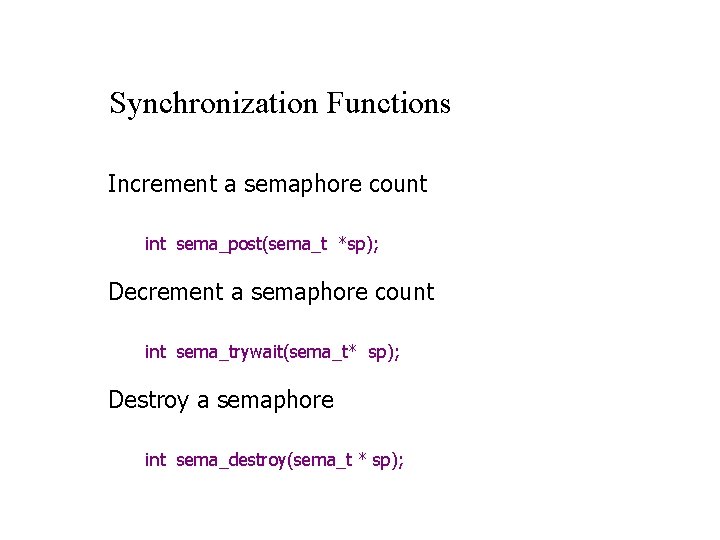
Synchronization Functions Increment a semaphore count int sema_post(sema_t *sp); Decrement a semaphore count int sema_trywait(sema_t* sp); Destroy a semaphore int sema_destroy(sema_t * sp);
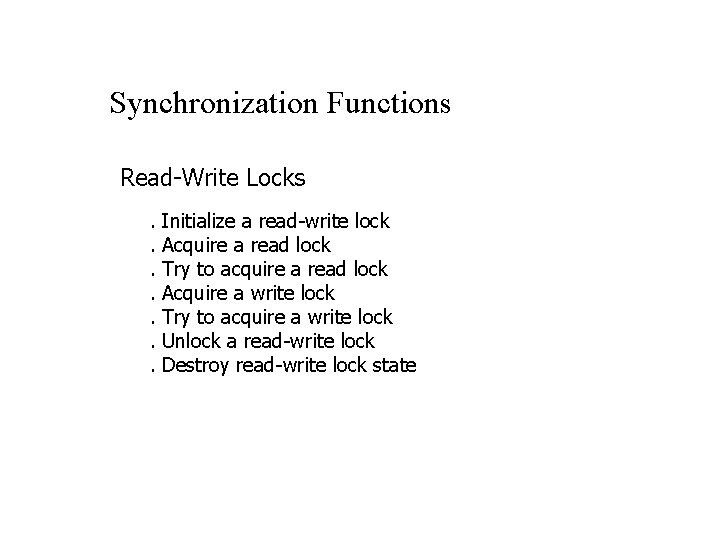
Synchronization Functions Read-Write Locks. . . . Initialize a read-write lock Acquire a read lock Try to acquire a read lock Acquire a write lock Try to acquire a write lock Unlock a read-write lock Destroy read-write lock state
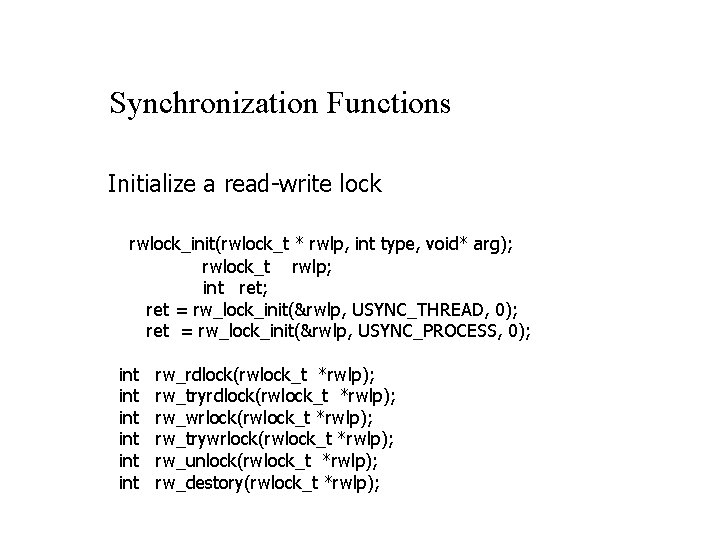
Synchronization Functions Initialize a read-write lock rwlock_init(rwlock_t * rwlp, int type, void* arg); rwlock_t rwlp; int ret; ret = rw_lock_init(&rwlp, USYNC_THREAD, 0); ret = rw_lock_init(&rwlp, USYNC_PROCESS, 0); int int int rw_rdlock(rwlock_t *rwlp); rw_tryrdlock(rwlock_t *rwlp); rw_wrlock(rwlock_t *rwlp); rw_trywrlock(rwlock_t *rwlp); rw_unlock(rwlock_t *rwlp); rw_destory(rwlock_t *rwlp);