The University of North Carolina at Chapel Hill
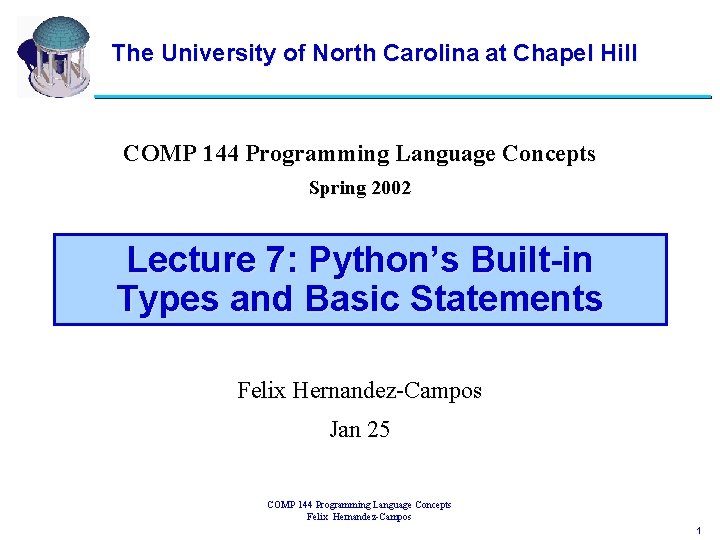
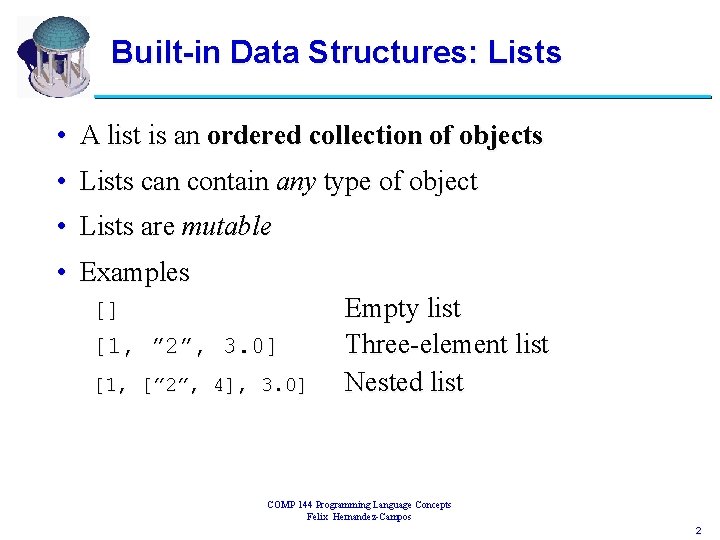
![Lists: Accessing Items • Syntax: list[index] – Indexing from the left starts at 0 Lists: Accessing Items • Syntax: list[index] – Indexing from the left starts at 0](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-3.jpg)
![Lists: Accessing Items • Syntax: list[-index] – Indexing from the right starts at -1 Lists: Accessing Items • Syntax: list[-index] – Indexing from the right starts at -1](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-4.jpg)
![Lists: Deleting Items • Syntax: del list[index] – E. g. >>> l = [1, Lists: Deleting Items • Syntax: del list[index] – E. g. >>> l = [1,](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-5.jpg)
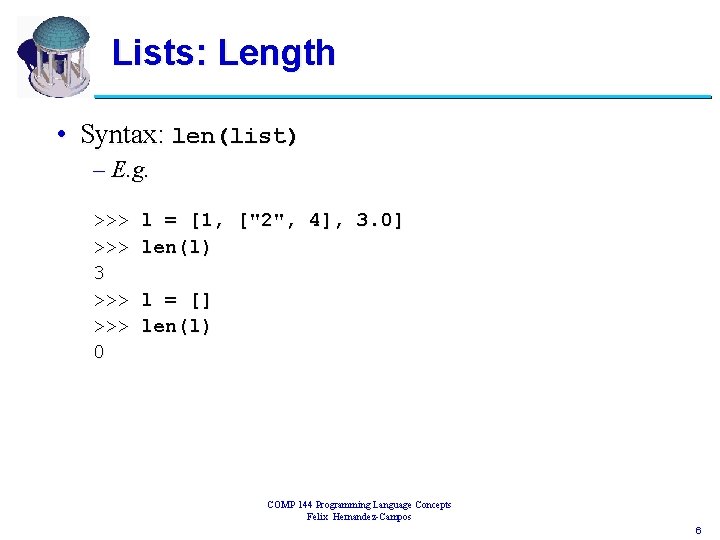
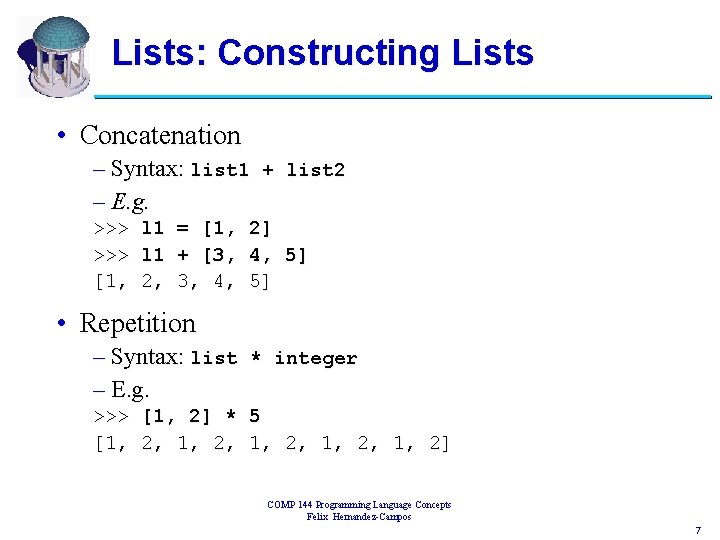
![Lists: Constructing Lists • Slicing – Syntax: list[i: j] – E. g. >>> l Lists: Constructing Lists • Slicing – Syntax: list[i: j] – E. g. >>> l](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-8.jpg)
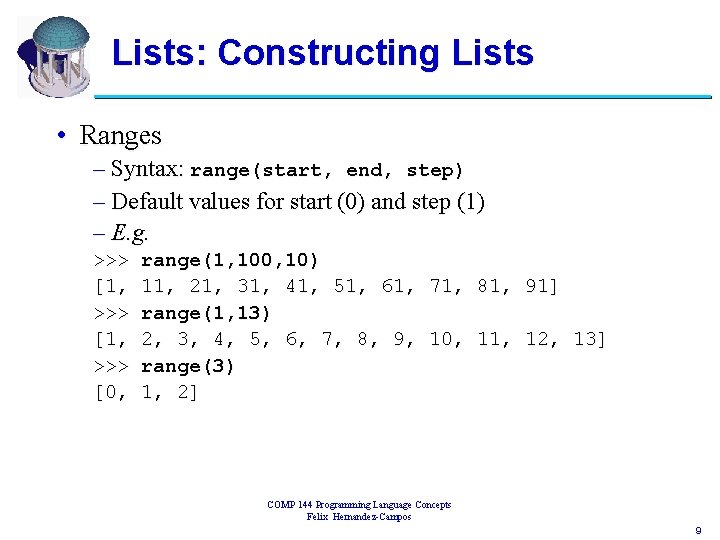
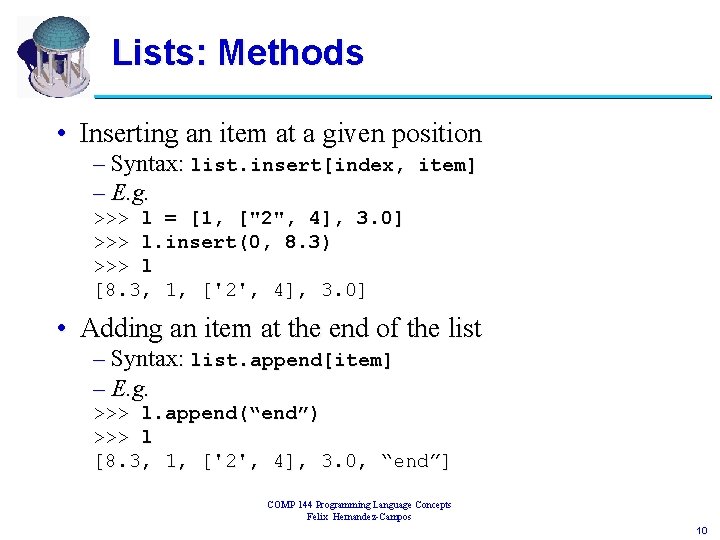
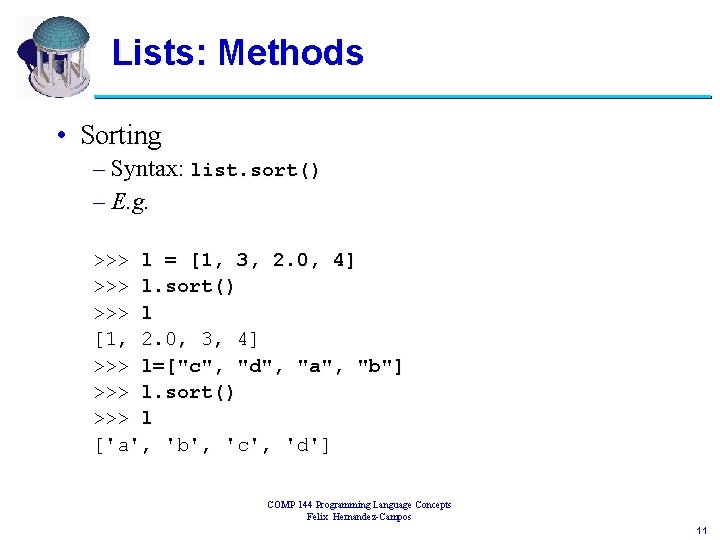
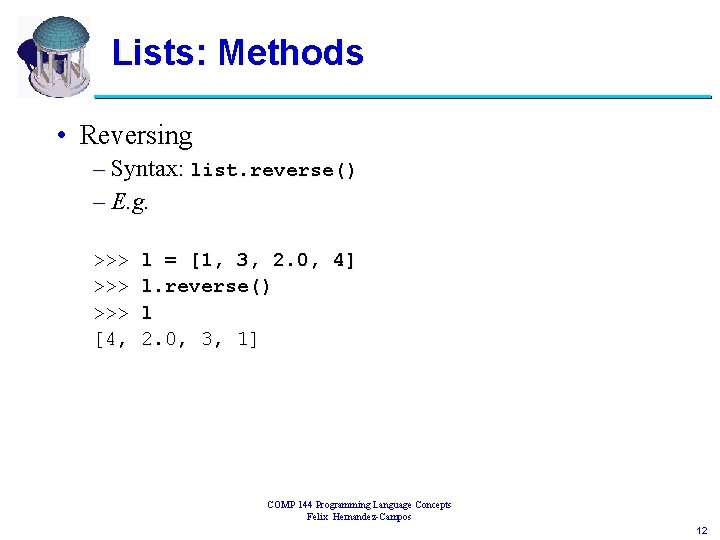
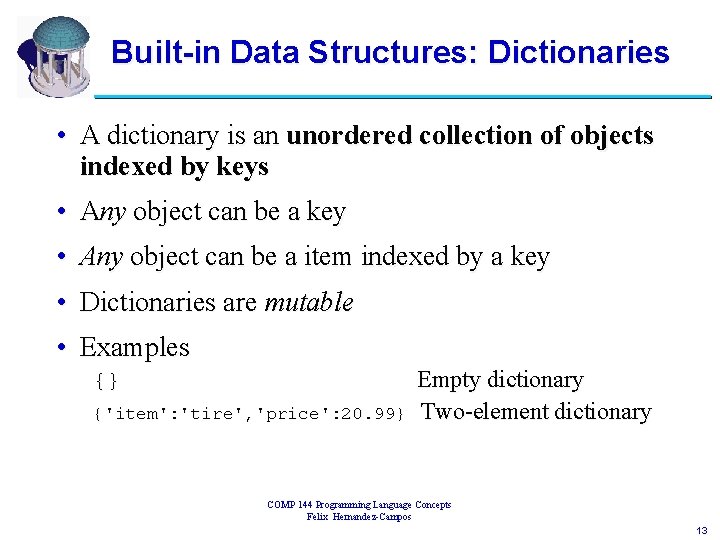
![Dictionaries: Accessing items • Syntax: list[key] – E. g. >>> d = {'item': 'tire', Dictionaries: Accessing items • Syntax: list[key] – E. g. >>> d = {'item': 'tire',](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-14.jpg)
![Dictionaries: Deleting items • Syntax: del list[key] – E. g. >>> d = {'item': Dictionaries: Deleting items • Syntax: del list[key] – E. g. >>> d = {'item':](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-15.jpg)
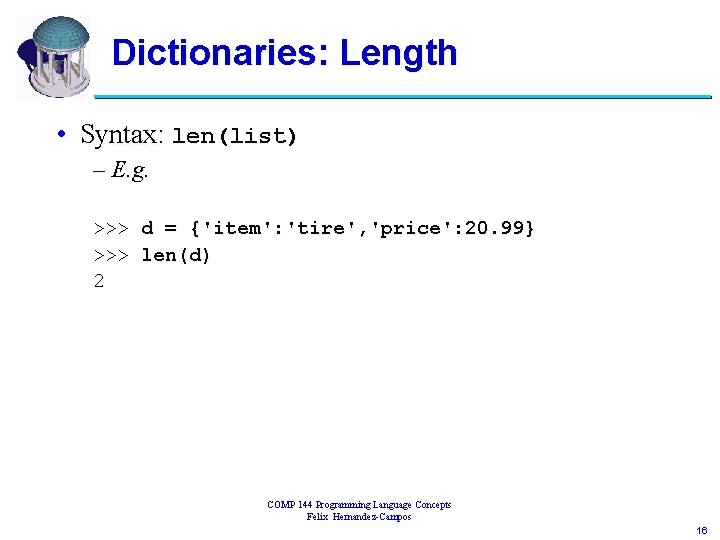
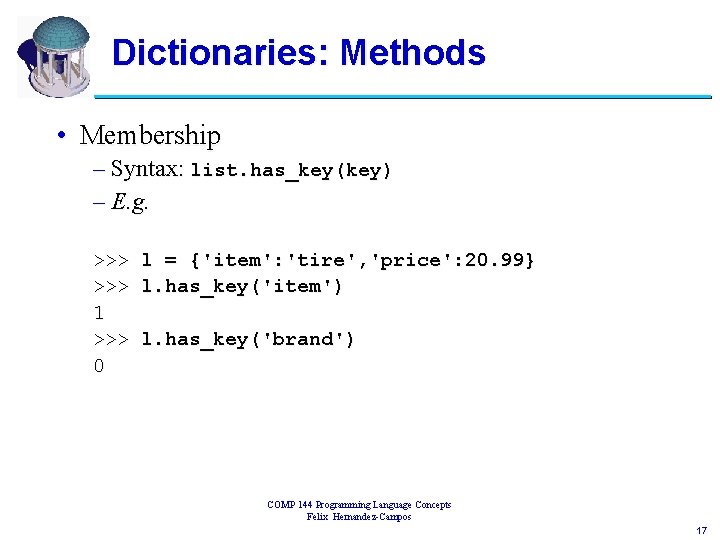
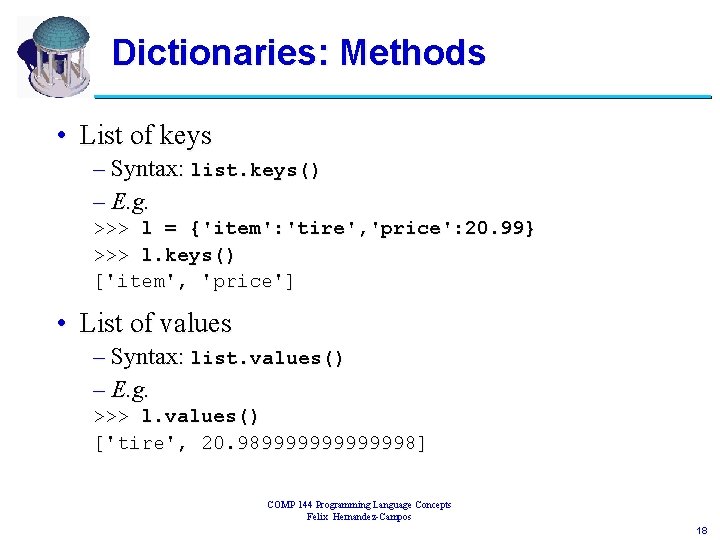
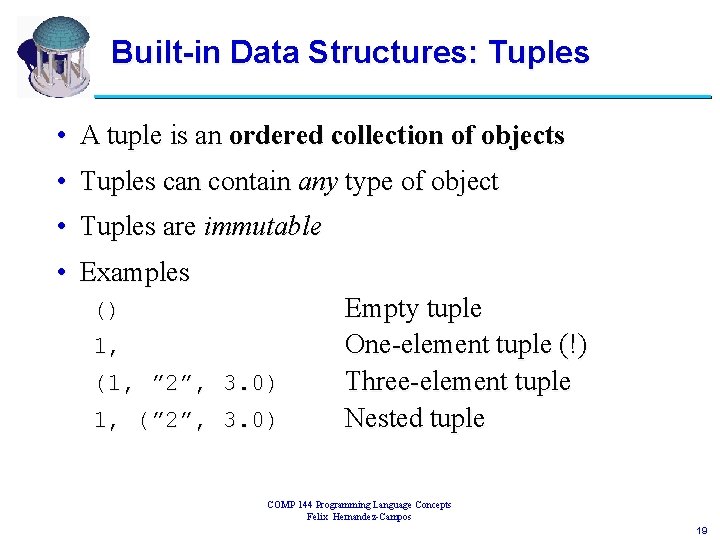
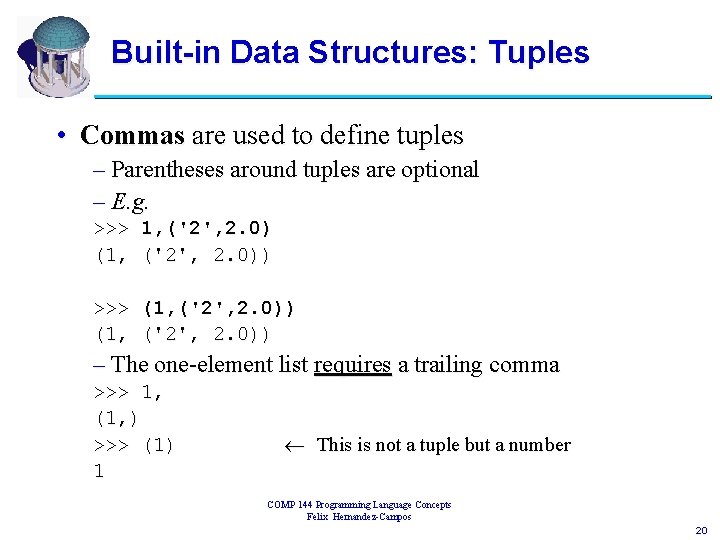
![Tuples: Accessing Items • Syntax: tuple[index] – E. g. >>> t = (1, 2, Tuples: Accessing Items • Syntax: tuple[index] – E. g. >>> t = (1, 2,](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-21.jpg)
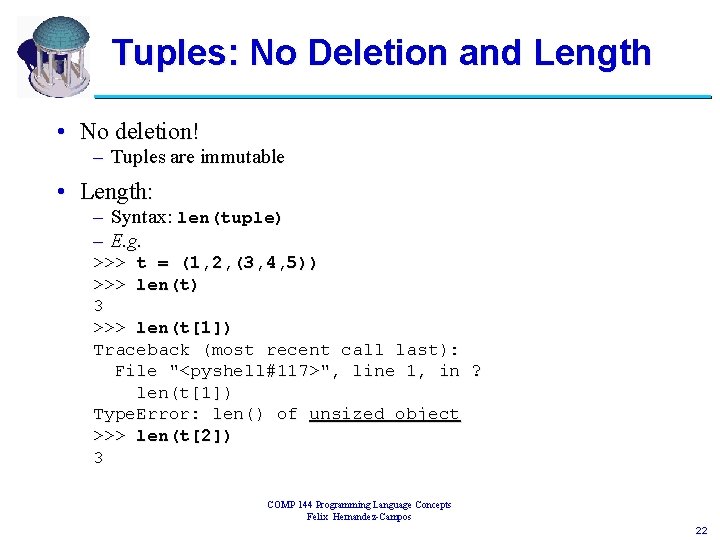
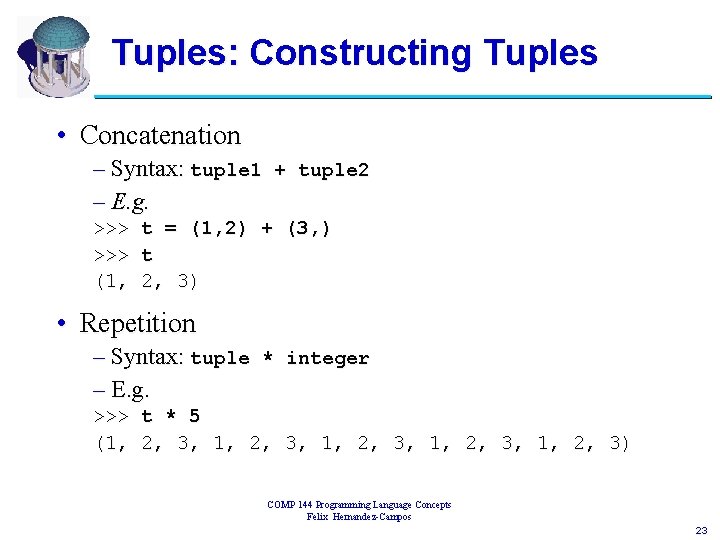
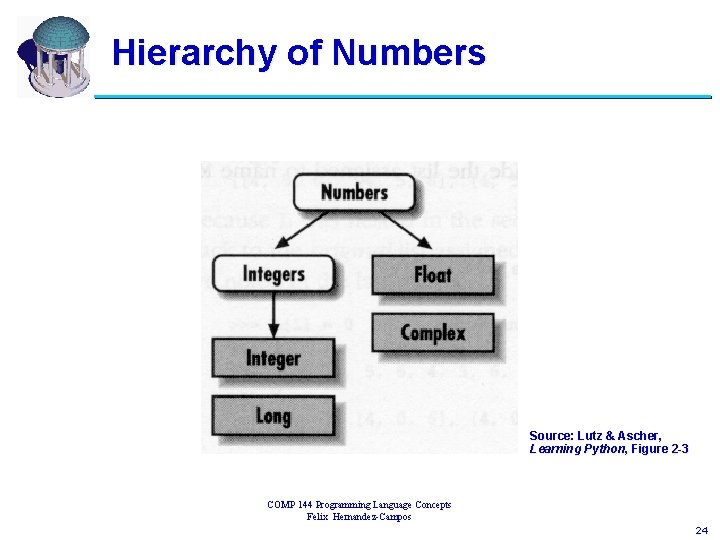
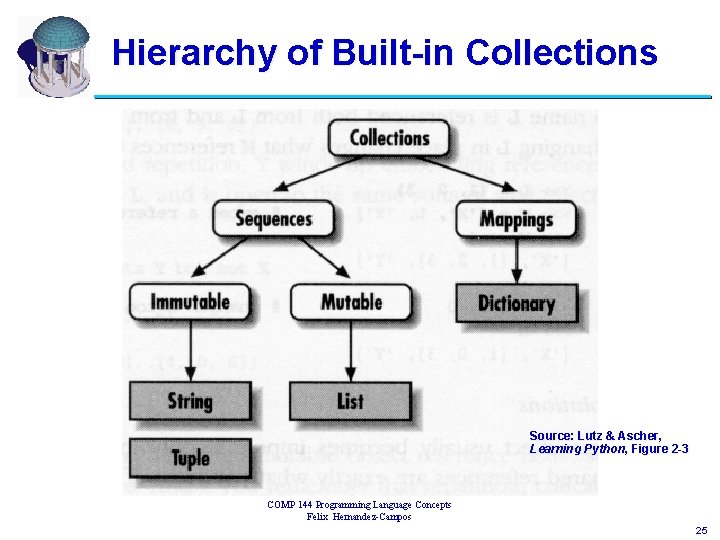
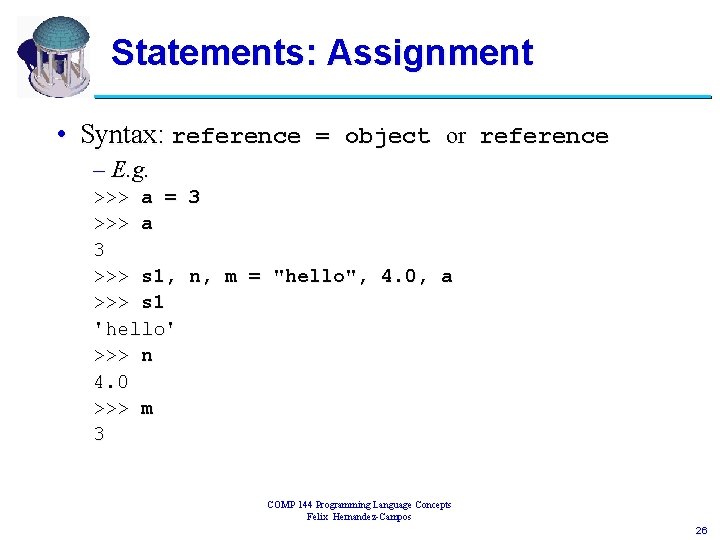
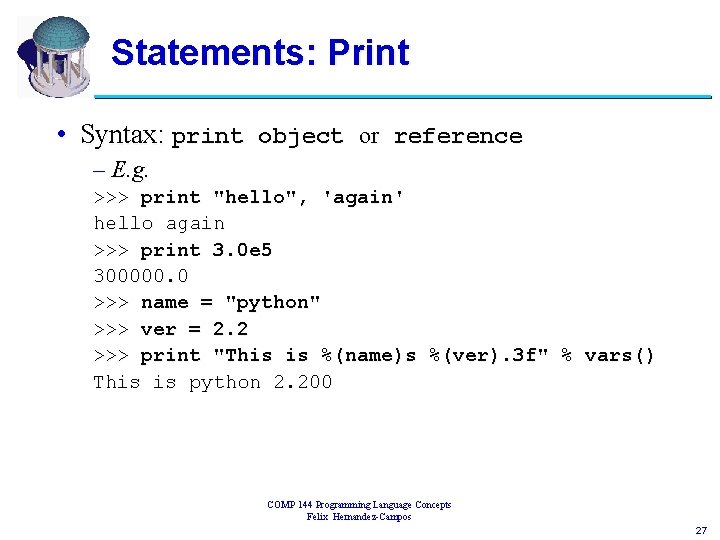
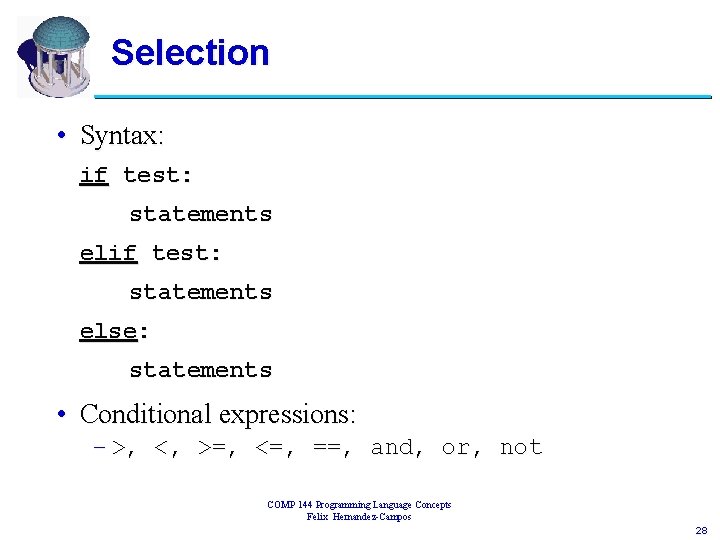
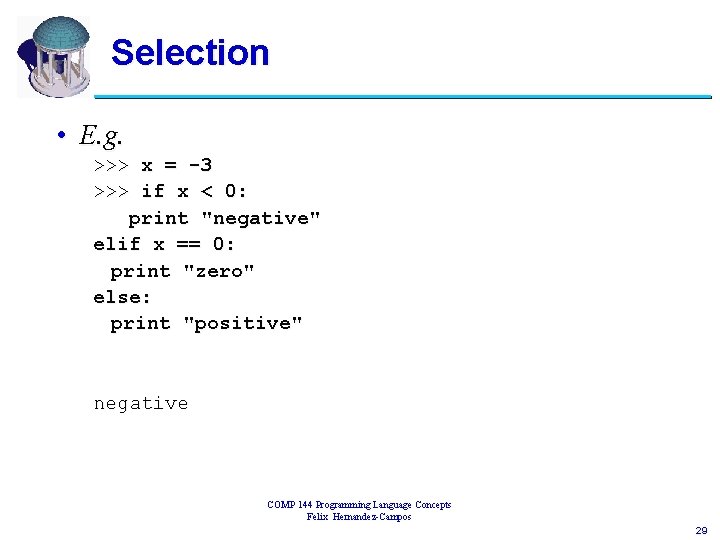
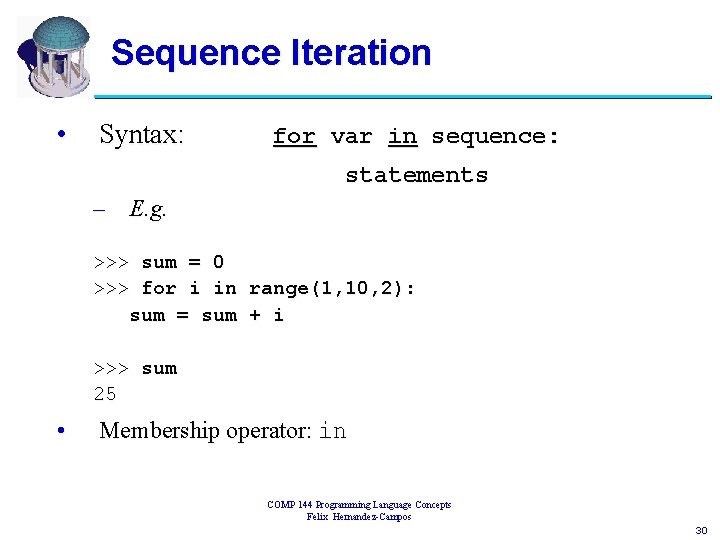
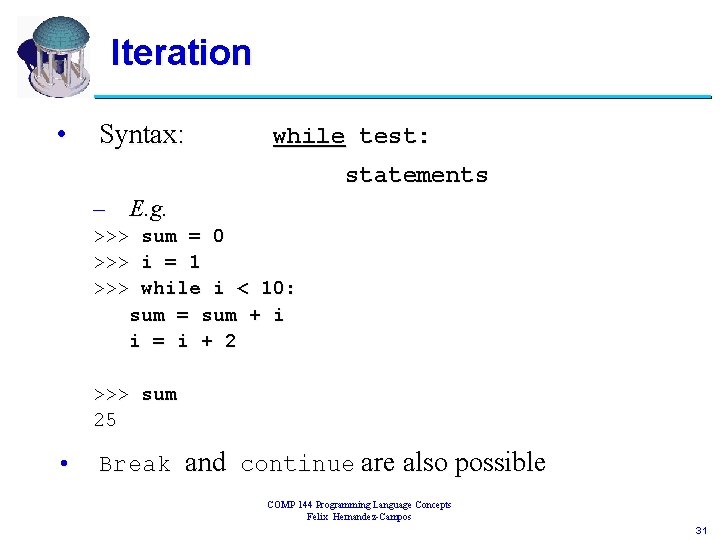
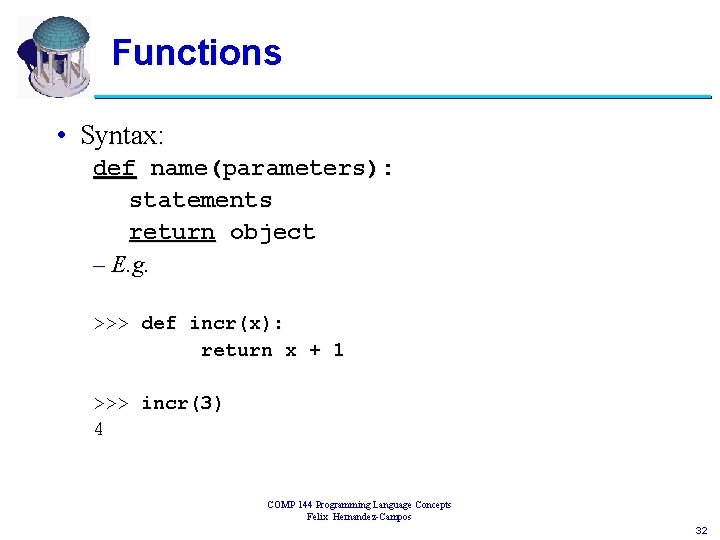
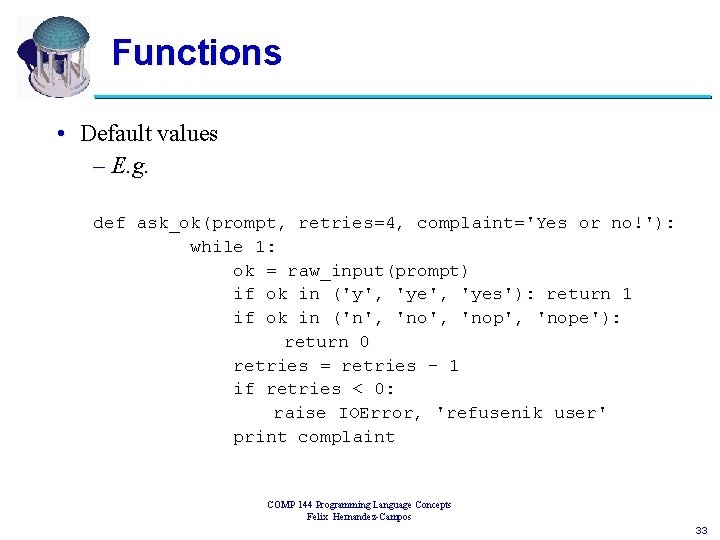
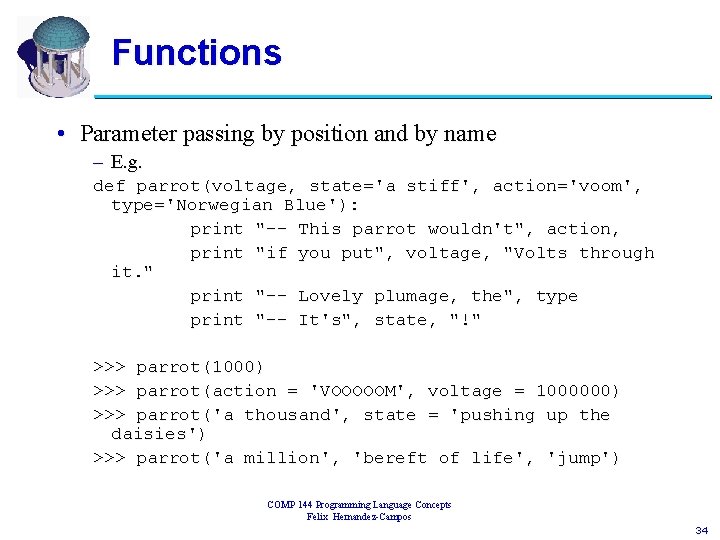
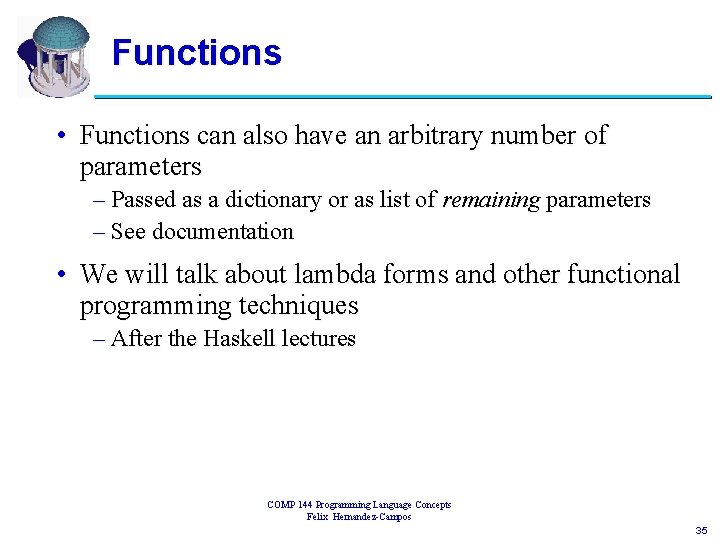
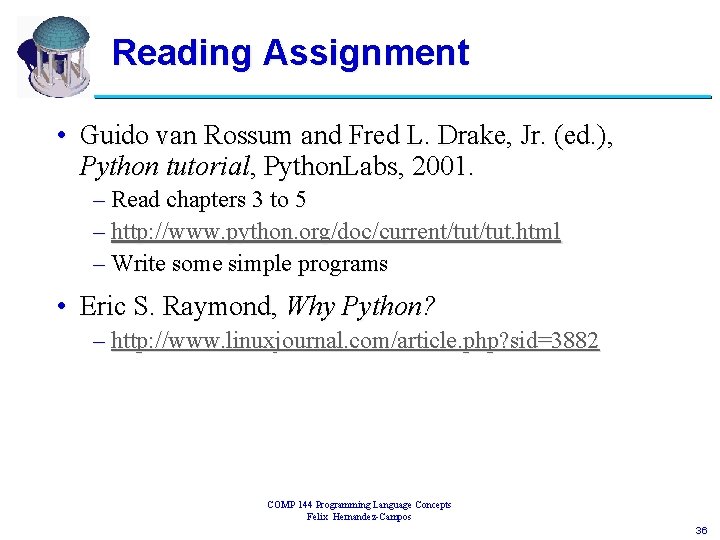
- Slides: 36
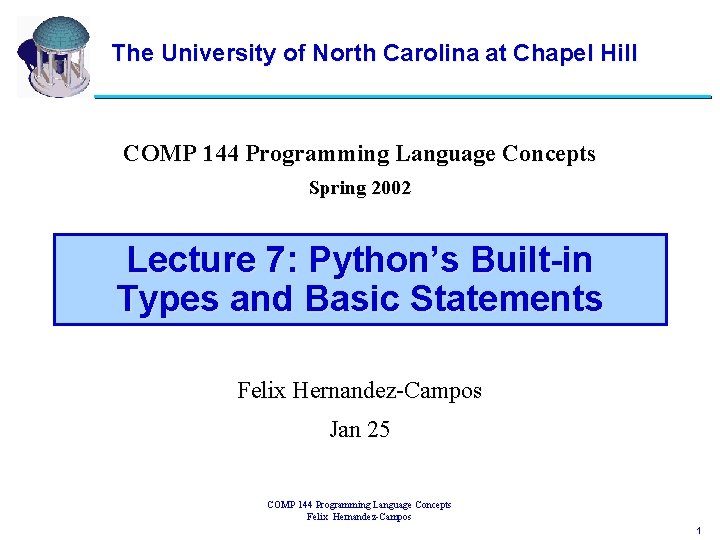
The University of North Carolina at Chapel Hill COMP 144 Programming Language Concepts Spring 2002 Lecture 7: Python’s Built-in Types and Basic Statements Felix Hernandez-Campos Jan 25 COMP 144 Programming Language Concepts Felix Hernandez-Campos 1
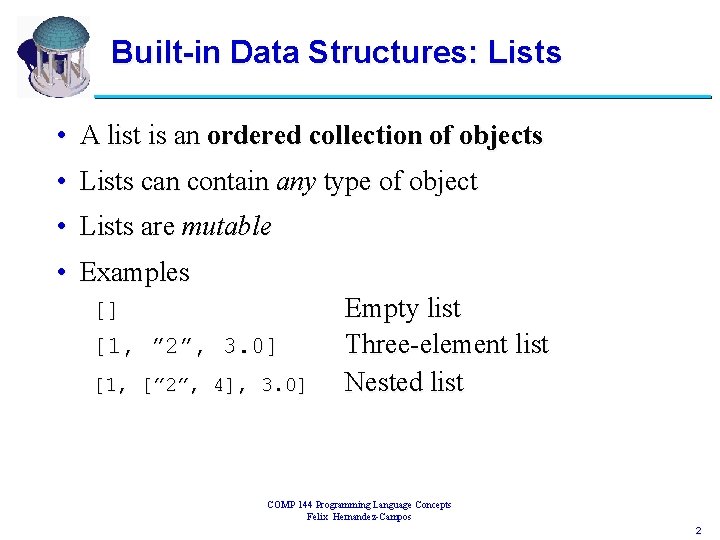
Built-in Data Structures: Lists • A list is an ordered collection of objects • Lists can contain any type of object • Lists are mutable • Examples [] [1, ” 2”, 3. 0] [1, [” 2”, 4], 3. 0] Empty list Three-element list Nested list COMP 144 Programming Language Concepts Felix Hernandez-Campos 2
![Lists Accessing Items Syntax listindex Indexing from the left starts at 0 Lists: Accessing Items • Syntax: list[index] – Indexing from the left starts at 0](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-3.jpg)
Lists: Accessing Items • Syntax: list[index] – Indexing from the left starts at 0 – E. g. >>> l = [1, ["2", 4], 3. 0] >>> l[0] 1 >>> l[2] 3. 0 >>> l[1] ['2', 4] >>> l[3] = 4 Traceback (most recent call File "<pyshell#17>", line l[3] = 4 Index. Error: list assignment last): 1, in ? index out of range COMP 144 Programming Language Concepts Felix Hernandez-Campos 3
![Lists Accessing Items Syntax listindex Indexing from the right starts at 1 Lists: Accessing Items • Syntax: list[-index] – Indexing from the right starts at -1](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-4.jpg)
Lists: Accessing Items • Syntax: list[-index] – Indexing from the right starts at -1 – E. g. >>> l = [1, ["2", 4], 3. 0] >>> l[-1] 3. 0 >>> l[-3] 1 >>> l[-4] Traceback (most recent call last): File "<pyshell#29>", line 1, in ? l[-4] Index. Error: list index out of range COMP 144 Programming Language Concepts Felix Hernandez-Campos 4
![Lists Deleting Items Syntax del listindex E g l 1 Lists: Deleting Items • Syntax: del list[index] – E. g. >>> l = [1,](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-5.jpg)
Lists: Deleting Items • Syntax: del list[index] – E. g. >>> l = [1, ["2", 4], 3. 0] >>> del l[2] >>> l [1, ['2', 4]] >>> del l[2] Traceback (most recent call last): File "<pyshell#16>", line 1, in ? del l[2] Index. Error: list assignment index out of range COMP 144 Programming Language Concepts Felix Hernandez-Campos 5
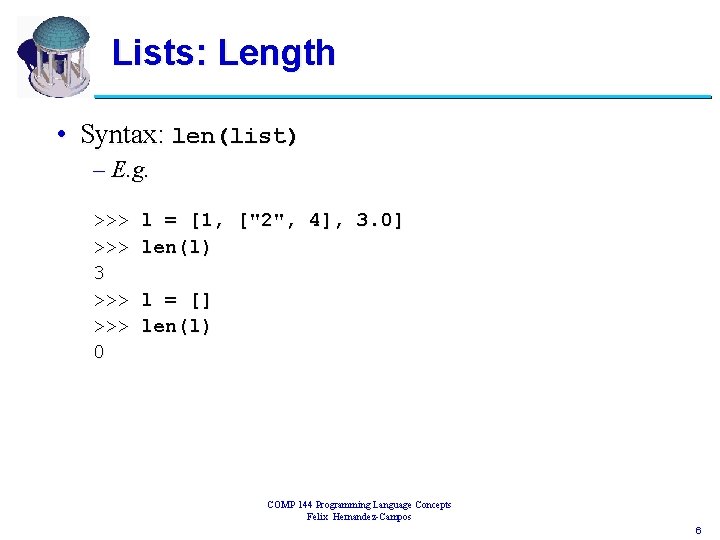
Lists: Length • Syntax: len(list) – E. g. >>> 3 >>> 0 l = [1, ["2", 4], 3. 0] len(l) l = [] len(l) COMP 144 Programming Language Concepts Felix Hernandez-Campos 6
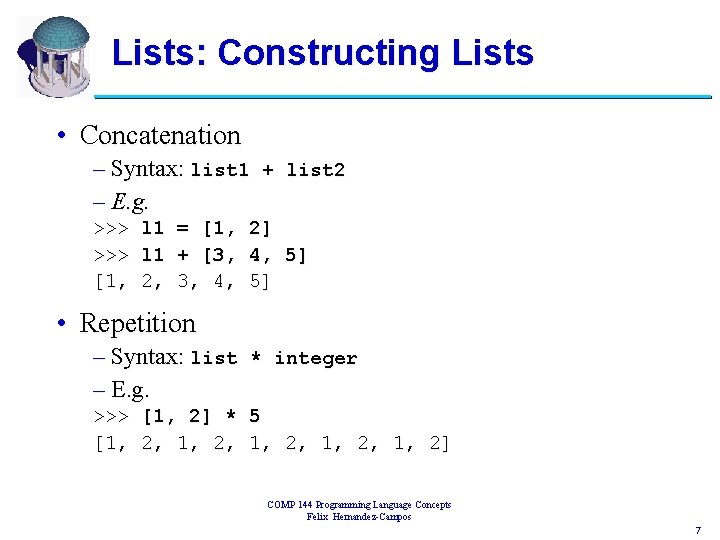
Lists: Constructing Lists • Concatenation – Syntax: list 1 + list 2 – E. g. >>> [1, l 1 2, = [1, + [3, 3, 4, 2] 4, 5] 5] • Repetition – Syntax: list * integer – E. g. >>> [1, 2] * 5 [1, 2, 1, 2] COMP 144 Programming Language Concepts Felix Hernandez-Campos 7
![Lists Constructing Lists Slicing Syntax listi j E g l Lists: Constructing Lists • Slicing – Syntax: list[i: j] – E. g. >>> l](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-8.jpg)
Lists: Constructing Lists • Slicing – Syntax: list[i: j] – E. g. >>> l = [1, ["2", 4], 3. 0] >>> l[1: 2] [['2', 4]] >>> l[0: -2] [1] >>> l[1: -2] [] >>> l[1: -3] [] >>> l[1: 3] = [2, 3] >>> l [1, 2, 3] COMP 144 Programming Language Concepts Felix Hernandez-Campos 8
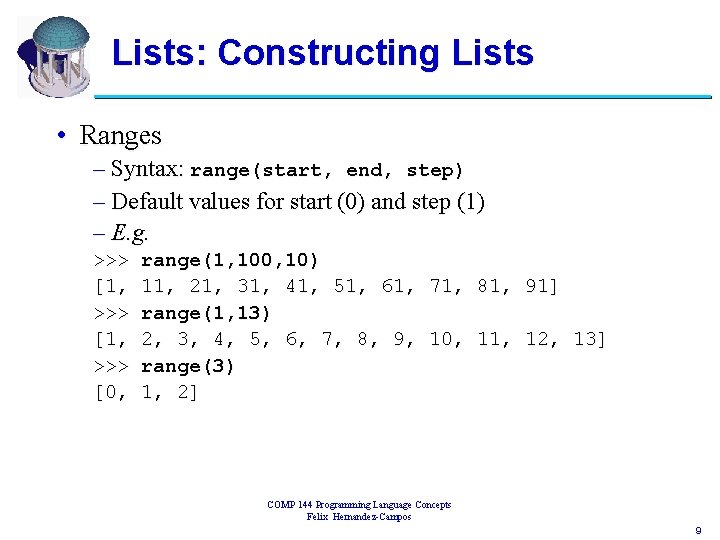
Lists: Constructing Lists • Ranges – Syntax: range(start, end, step) – Default values for start (0) and step (1) – E. g. >>> [1, >>> [0, range(1, 100, 10) 11, 21, 31, 41, 51, 61, 71, 81, 91] range(1, 13) 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13] range(3) 1, 2] COMP 144 Programming Language Concepts Felix Hernandez-Campos 9
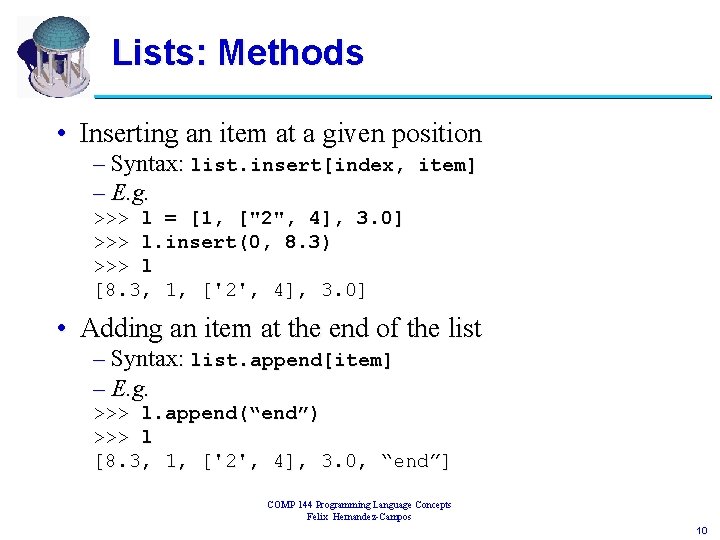
Lists: Methods • Inserting an item at a given position – Syntax: list. insert[index, item] – E. g. >>> l = [1, ["2", 4], 3. 0] >>> l. insert(0, 8. 3) >>> l [8. 3, 1, ['2', 4], 3. 0] • Adding an item at the end of the list – Syntax: list. append[item] – E. g. >>> l. append(“end”) >>> l [8. 3, 1, ['2', 4], 3. 0, “end”] COMP 144 Programming Language Concepts Felix Hernandez-Campos 10
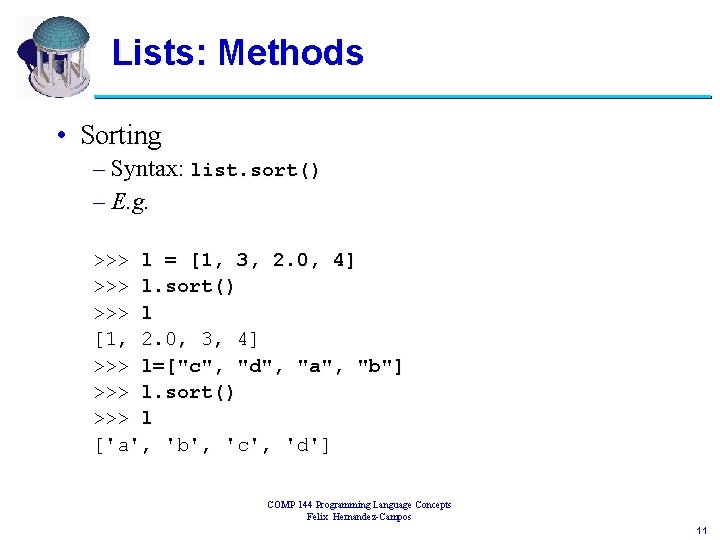
Lists: Methods • Sorting – Syntax: list. sort() – E. g. >>> l = [1, 3, 2. 0, 4] >>> l. sort() >>> l [1, 2. 0, 3, 4] >>> l=["c", "d", "a", "b"] >>> l. sort() >>> l ['a', 'b', 'c', 'd'] COMP 144 Programming Language Concepts Felix Hernandez-Campos 11
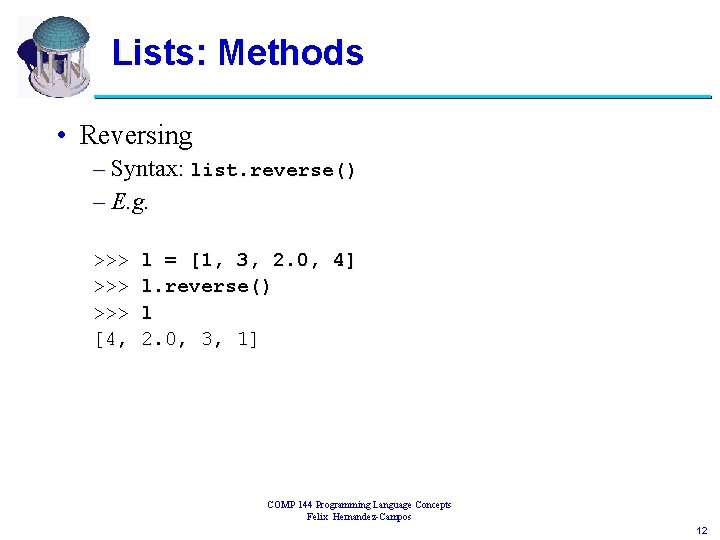
Lists: Methods • Reversing – Syntax: list. reverse() – E. g. >>> >>> [4, l = [1, 3, 2. 0, 4] l. reverse() l 2. 0, 3, 1] COMP 144 Programming Language Concepts Felix Hernandez-Campos 12
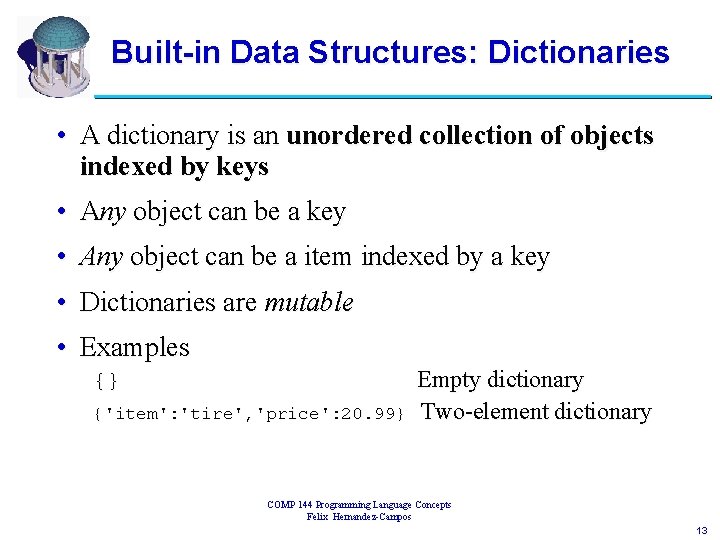
Built-in Data Structures: Dictionaries • A dictionary is an unordered collection of objects indexed by keys • Any object can be a key • Any object can be a item indexed by a key • Dictionaries are mutable • Examples {} {'item': 'tire', 'price': 20. 99} Empty dictionary Two-element dictionary COMP 144 Programming Language Concepts Felix Hernandez-Campos 13
![Dictionaries Accessing items Syntax listkey E g d item tire Dictionaries: Accessing items • Syntax: list[key] – E. g. >>> d = {'item': 'tire',](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-14.jpg)
Dictionaries: Accessing items • Syntax: list[key] – E. g. >>> d = {'item': 'tire', 'price': 20. 99} >>> d[‘price'] 20. 99 >>> d[item] Traceback (most recent File "<pyshell#88>", d[item] Name. Error: name 'item' >>> str = 'item' >>> d[str] 'tire' call last): line 1, in ? is not defined COMP 144 Programming Language Concepts Felix Hernandez-Campos 14
![Dictionaries Deleting items Syntax del listkey E g d item Dictionaries: Deleting items • Syntax: del list[key] – E. g. >>> d = {'item':](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-15.jpg)
Dictionaries: Deleting items • Syntax: del list[key] – E. g. >>> d = {'item': 'tire', 'price': 20. 99} >>> del d['item'] >>> d {'price': 20. 989999998} >>> del d['brand'] Traceback (most recent call last): File "<pyshell#95>", line 1, in ? del d['brand'] Key. Error: brand COMP 144 Programming Language Concepts Felix Hernandez-Campos 15
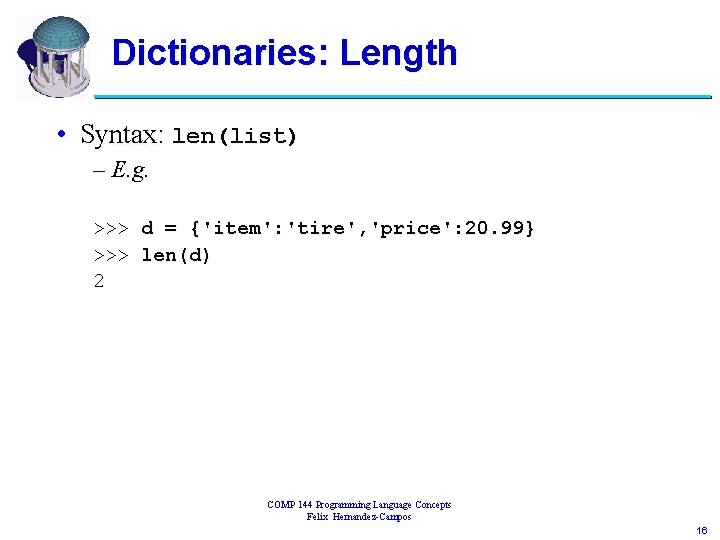
Dictionaries: Length • Syntax: len(list) – E. g. >>> d = {'item': 'tire', 'price': 20. 99} >>> len(d) 2 COMP 144 Programming Language Concepts Felix Hernandez-Campos 16
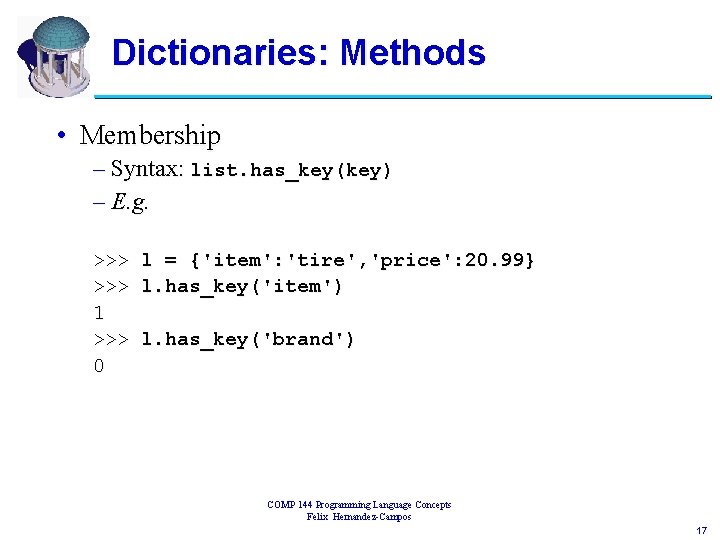
Dictionaries: Methods • Membership – Syntax: list. has_key(key) – E. g. >>> l = {'item': 'tire', 'price': 20. 99} >>> l. has_key('item') 1 >>> l. has_key('brand') 0 COMP 144 Programming Language Concepts Felix Hernandez-Campos 17
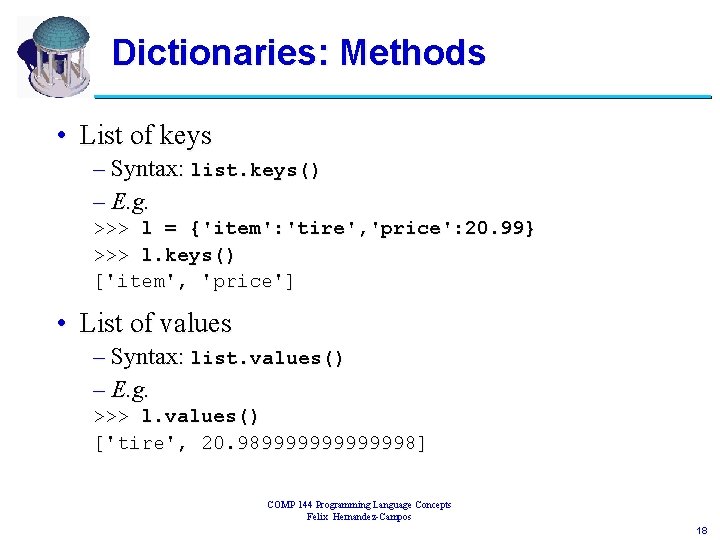
Dictionaries: Methods • List of keys – Syntax: list. keys() – E. g. >>> l = {'item': 'tire', 'price': 20. 99} >>> l. keys() ['item', 'price'] • List of values – Syntax: list. values() – E. g. >>> l. values() ['tire', 20. 989999998] COMP 144 Programming Language Concepts Felix Hernandez-Campos 18
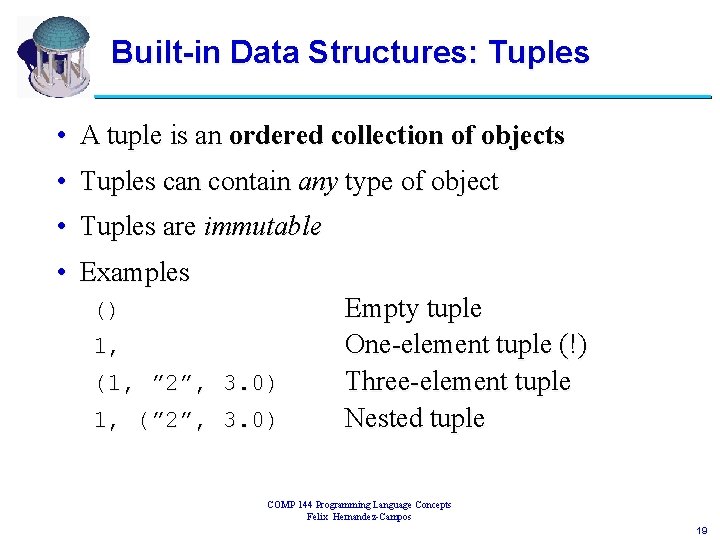
Built-in Data Structures: Tuples • A tuple is an ordered collection of objects • Tuples can contain any type of object • Tuples are immutable • Examples () 1, (1, ” 2”, 3. 0) 1, (” 2”, 3. 0) Empty tuple One-element tuple (!) Three-element tuple Nested tuple COMP 144 Programming Language Concepts Felix Hernandez-Campos 19
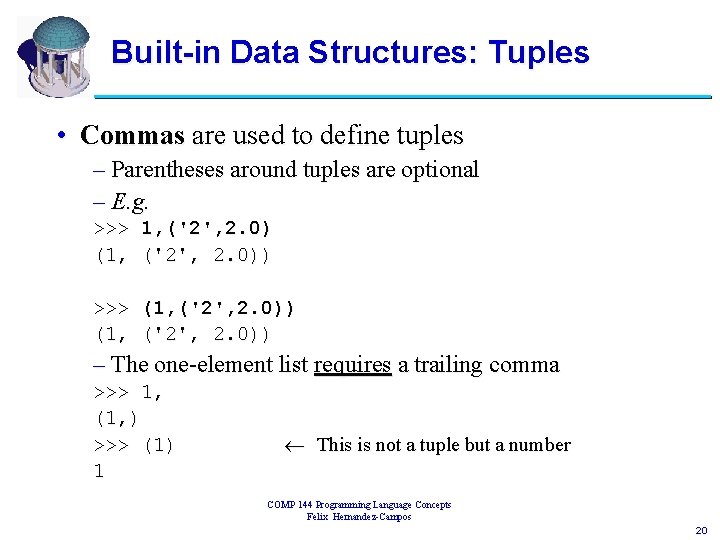
Built-in Data Structures: Tuples • Commas are used to define tuples – Parentheses around tuples are optional – E. g. >>> 1, ('2', 2. 0) (1, ('2', 2. 0)) >>> (1, ('2', 2. 0)) – The one-element list requires a trailing comma >>> 1, (1, ) >>> (1) 1 This is not a tuple but a number COMP 144 Programming Language Concepts Felix Hernandez-Campos 20
![Tuples Accessing Items Syntax tupleindex E g t 1 2 Tuples: Accessing Items • Syntax: tuple[index] – E. g. >>> t = (1, 2,](https://slidetodoc.com/presentation_image_h2/f2bf377e5c540b4ceca453789ff7d9d8/image-21.jpg)
Tuples: Accessing Items • Syntax: tuple[index] – E. g. >>> t = (1, 2, (3, 4, 5)) >>> t[1] 2 >>> t[-1] (3, 4, 5) >>> t[-1][1] 4 >>> t[3] Traceback (most recent call last): File "<pyshell#110>", line 1, in ? t[3] Index. Error: tuple index out of range COMP 144 Programming Language Concepts Felix Hernandez-Campos 21
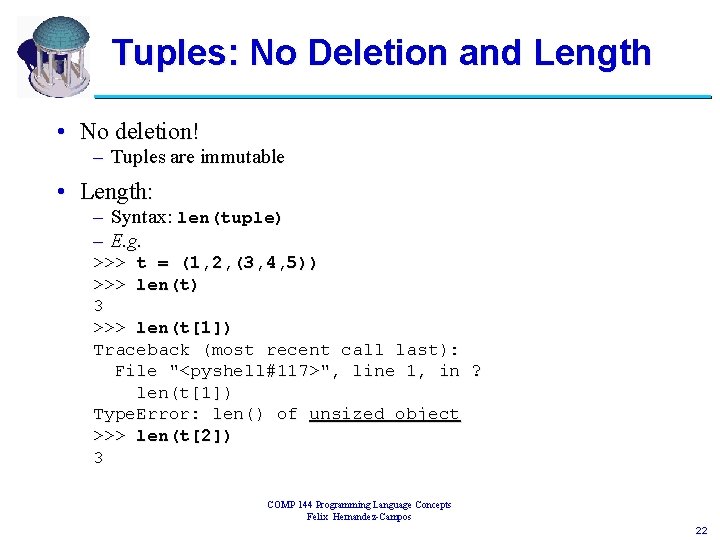
Tuples: No Deletion and Length • No deletion! – Tuples are immutable • Length: – Syntax: len(tuple) – E. g. >>> t = (1, 2, (3, 4, 5)) >>> len(t) 3 >>> len(t[1]) Traceback (most recent call last): File "<pyshell#117>", line 1, in ? len(t[1]) Type. Error: len() of unsized object >>> len(t[2]) 3 COMP 144 Programming Language Concepts Felix Hernandez-Campos 22
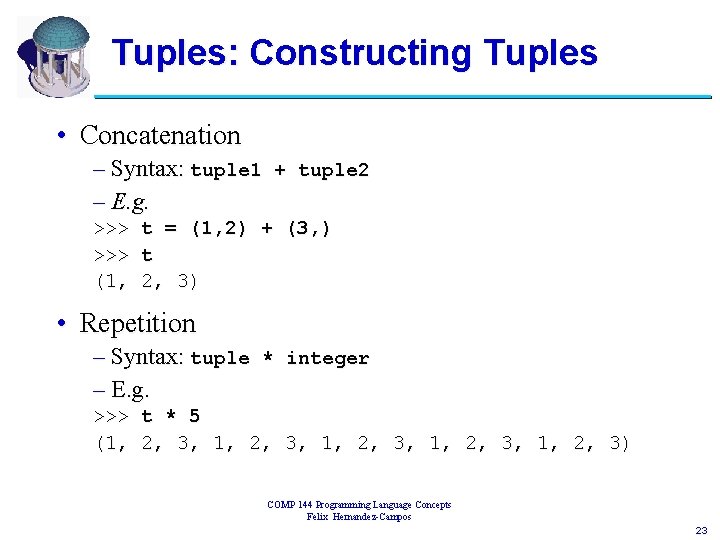
Tuples: Constructing Tuples • Concatenation – Syntax: tuple 1 + tuple 2 – E. g. >>> (1, t = (1, 2) + (3, ) t 2, 3) • Repetition – Syntax: tuple * integer – E. g. >>> t * 5 (1, 2, 3, 1, 2, 3) COMP 144 Programming Language Concepts Felix Hernandez-Campos 23
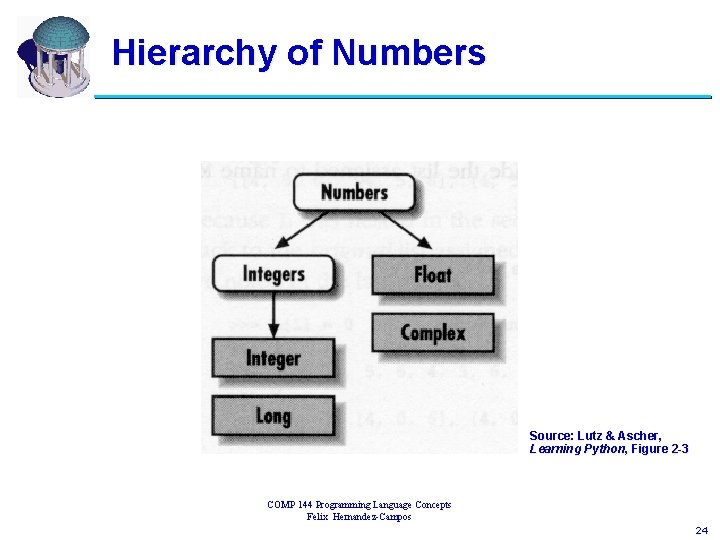
Hierarchy of Numbers Source: Lutz & Ascher, Learning Python, Figure 2 -3 COMP 144 Programming Language Concepts Felix Hernandez-Campos 24
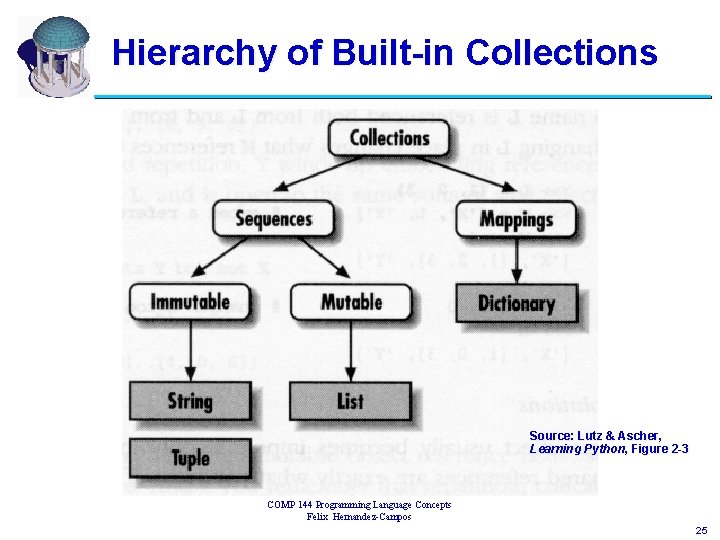
Hierarchy of Built-in Collections Source: Lutz & Ascher, Learning Python, Figure 2 -3 COMP 144 Programming Language Concepts Felix Hernandez-Campos 25
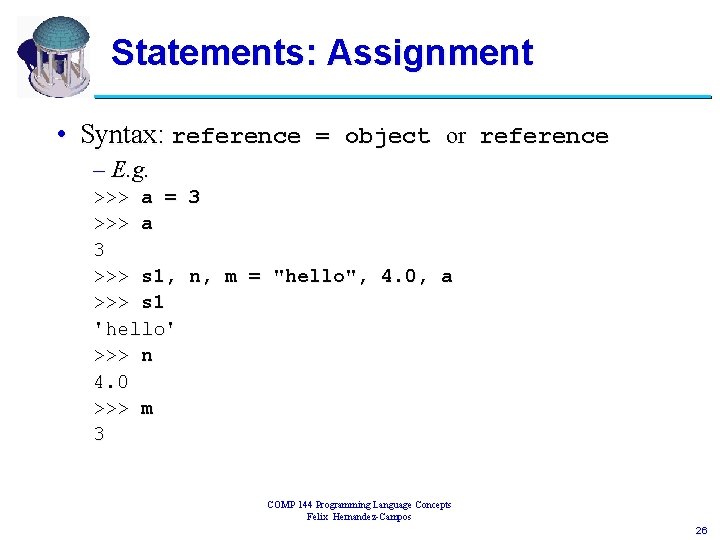
Statements: Assignment • Syntax: reference = object or reference – E. g. >>> a = 3 >>> a 3 >>> s 1, n, m = "hello", 4. 0, a >>> s 1 'hello' >>> n 4. 0 >>> m 3 COMP 144 Programming Language Concepts Felix Hernandez-Campos 26
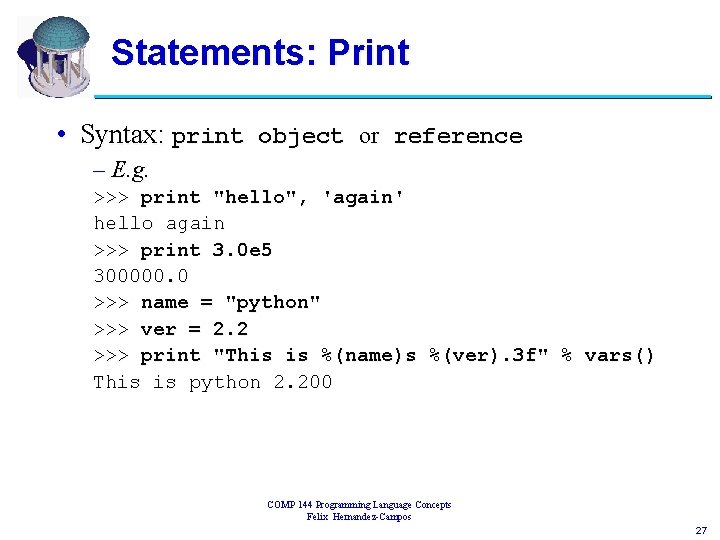
Statements: Print • Syntax: print object or reference – E. g. >>> print "hello", 'again' hello again >>> print 3. 0 e 5 300000. 0 >>> name = "python" >>> ver = 2. 2 >>> print "This is %(name)s %(ver). 3 f" % vars() This is python 2. 200 COMP 144 Programming Language Concepts Felix Hernandez-Campos 27
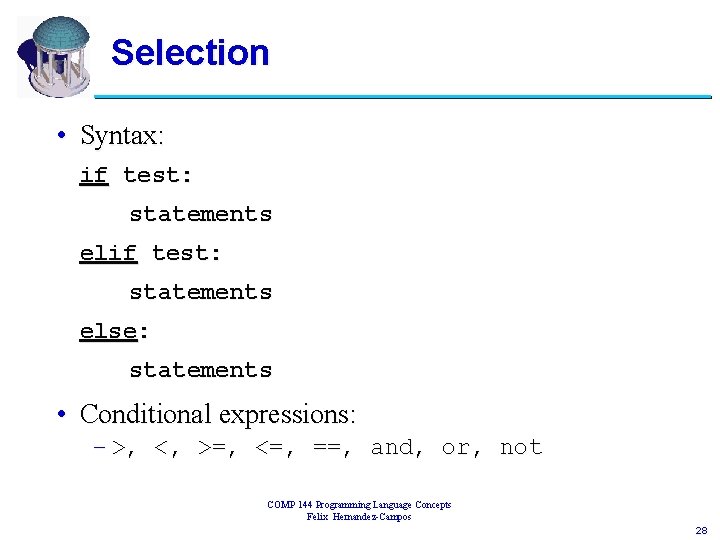
Selection • Syntax: if test: statements else: statements • Conditional expressions: – >, <, >=, <=, ==, and, or, not COMP 144 Programming Language Concepts Felix Hernandez-Campos 28
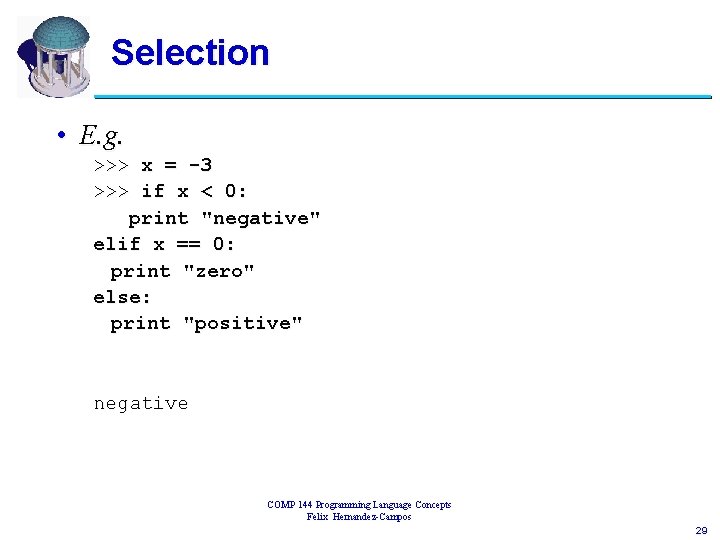
Selection • E. g. >>> x = -3 >>> if x < 0: print "negative" elif x == 0: print "zero" else: print "positive" negative COMP 144 Programming Language Concepts Felix Hernandez-Campos 29
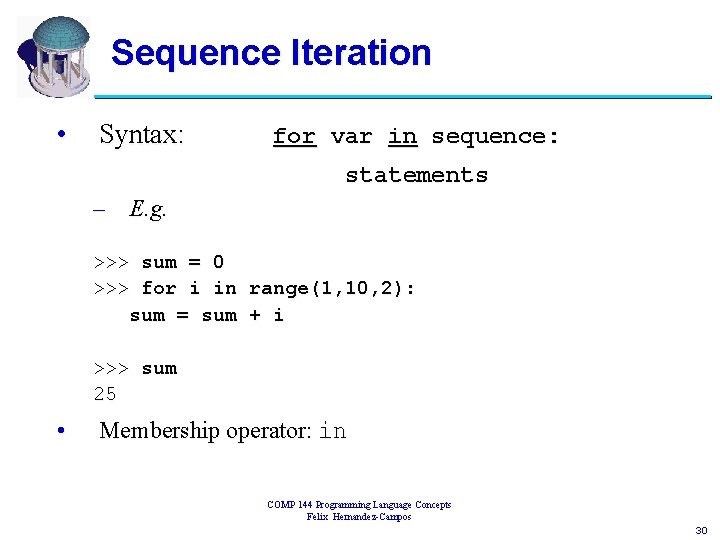
Sequence Iteration • Syntax: for var in sequence: statements – E. g. >>> sum = 0 >>> for i in range(1, 10, 2): sum = sum + i >>> sum 25 • Membership operator: in COMP 144 Programming Language Concepts Felix Hernandez-Campos 30
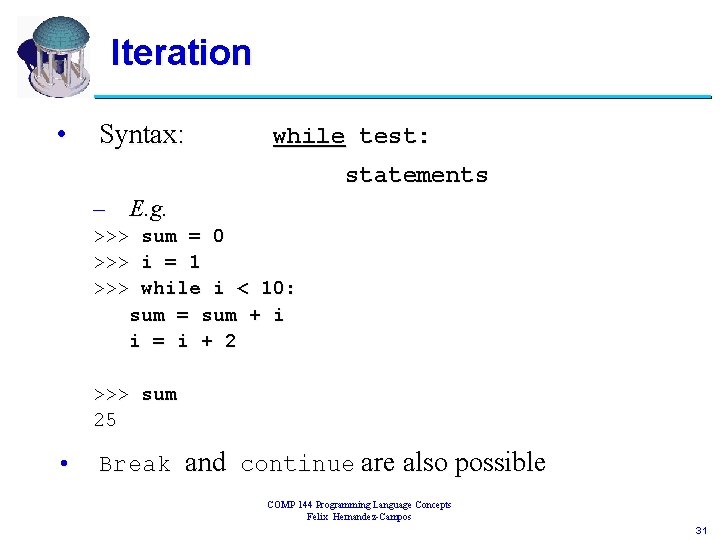
Iteration • Syntax: while test: statements – E. g. >>> sum = 0 >>> i = 1 >>> while i < 10: sum = sum + i i = i + 2 >>> sum 25 • Break and continue are also possible COMP 144 Programming Language Concepts Felix Hernandez-Campos 31
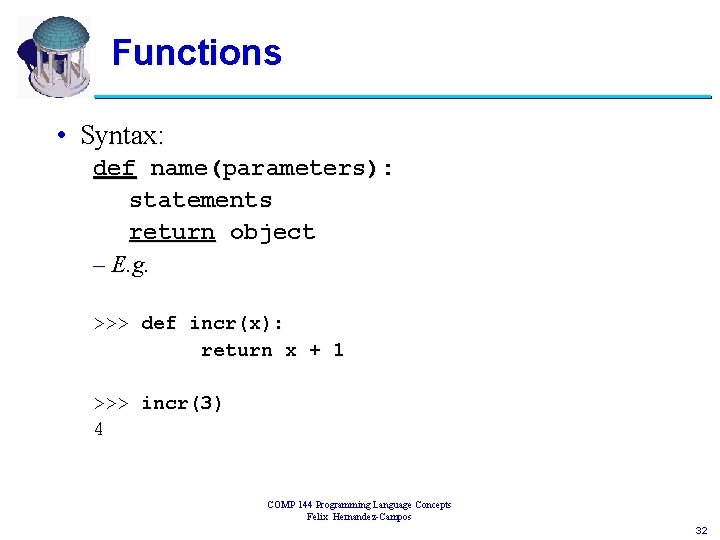
Functions • Syntax: def name(parameters): statements return object – E. g. >>> def incr(x): return x + 1 >>> incr(3) 4 COMP 144 Programming Language Concepts Felix Hernandez-Campos 32
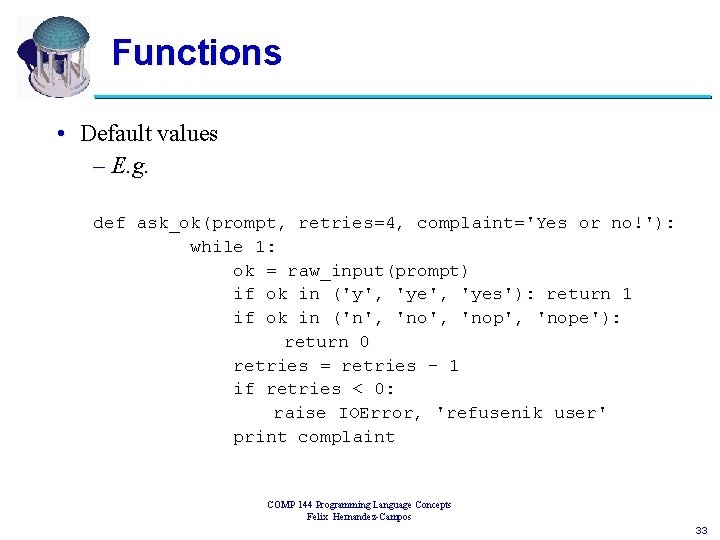
Functions • Default values – E. g. def ask_ok(prompt, retries=4, complaint='Yes or no!'): while 1: ok = raw_input(prompt) if ok in ('y', 'yes'): return 1 if ok in ('n', 'nop', 'nope'): return 0 retries = retries - 1 if retries < 0: raise IOError, 'refusenik user' print complaint COMP 144 Programming Language Concepts Felix Hernandez-Campos 33
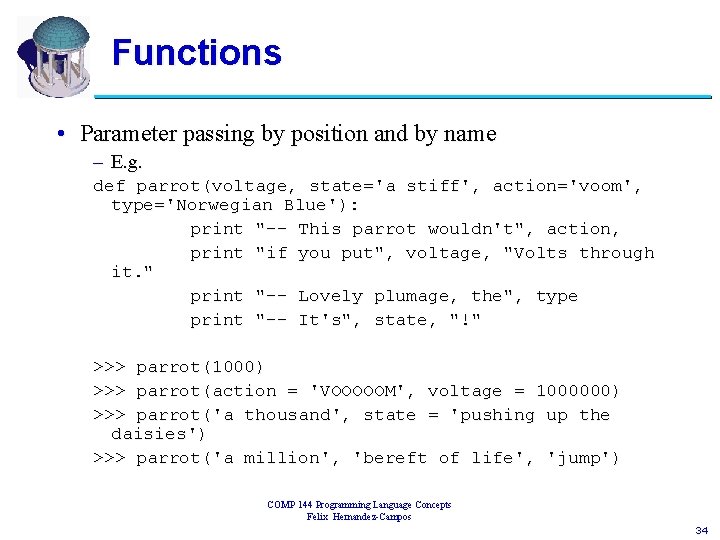
Functions • Parameter passing by position and by name – E. g. def parrot(voltage, state='a stiff', action='voom', type='Norwegian Blue'): print "-- This parrot wouldn't", action, print "if you put", voltage, "Volts through it. " print "-- Lovely plumage, the", type print "-- It's", state, "!" >>> parrot(1000) >>> parrot(action = 'VOOOOOM', voltage = 1000000) >>> parrot('a thousand', state = 'pushing up the daisies') >>> parrot('a million', 'bereft of life', 'jump') COMP 144 Programming Language Concepts Felix Hernandez-Campos 34
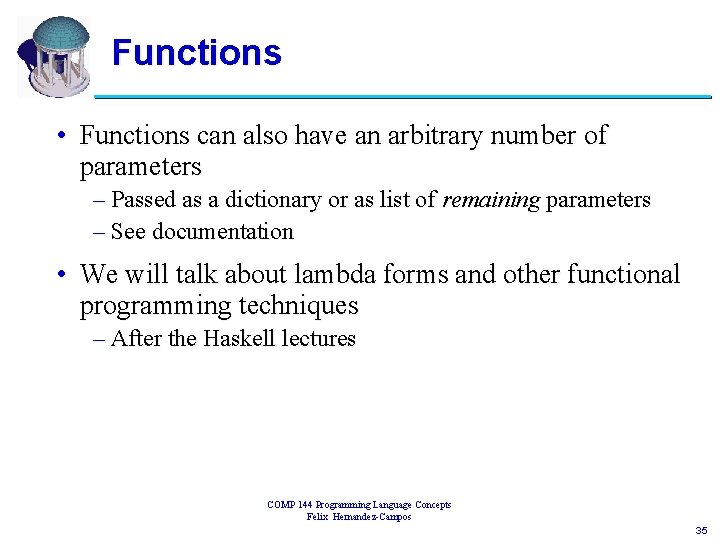
Functions • Functions can also have an arbitrary number of parameters – Passed as a dictionary or as list of remaining parameters – See documentation • We will talk about lambda forms and other functional programming techniques – After the Haskell lectures COMP 144 Programming Language Concepts Felix Hernandez-Campos 35
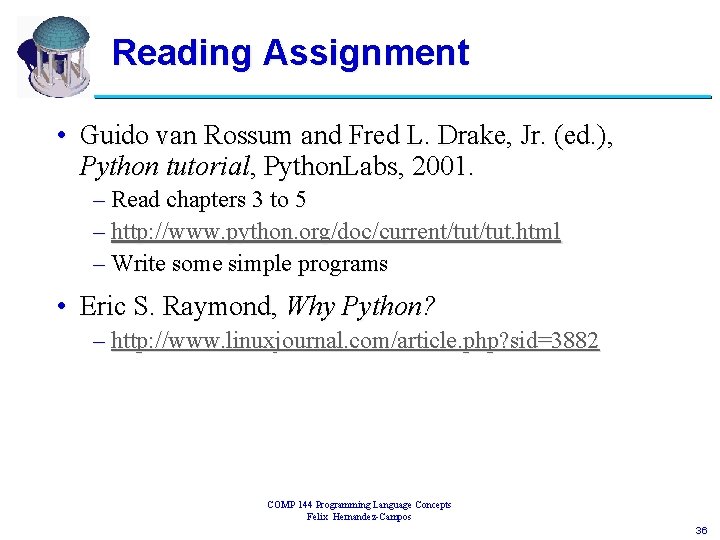
Reading Assignment • Guido van Rossum and Fred L. Drake, Jr. (ed. ), Python tutorial, Python. Labs, 2001. – Read chapters 3 to 5 – http: //www. python. org/doc/current/tut. html – Write some simple programs • Eric S. Raymond, Why Python? – http: //www. linuxjournal. com/article. php? sid=3882 COMP 144 Programming Language Concepts Felix Hernandez-Campos 36
Unc chapel hill computer science
Georgia, south carolina, north carolina, virginia, maryland
Map of north carolina and south carolina
Maryland virginia north carolina south carolina and georgia
Virginia, maryland, north carolina, south carolina, georgia
Unc hematology oncology fellows
Consenso de chapel hill
Enrolled agent chapel hill, nc
Dr ramona raya
Unc ohre
Unc chapel hill ib credit
Conect carolina
North carolina state university
Nc sunshine law
Boston state abbreviation
North carolina victim assistance network
Callie zipple
South carolinas state bird
North carolina presbyterian pilgrimage
What is the state shell of north carolina
Nchsaa board of directors
Nc famous landmarks
Face jugs north carolina
Craiglist escorts lykens pa
North carolina relative location
Exploring north carolina
North carolina paralegal degrees
North carolina council on developmental disabilities
Map of north carolina lighthouses
North carolina geographic regions
North carolina digital government summit
Arbiter referee login
Family support network of north carolina
Piedmont landform
How many river basins are in nc
North carolina teacher evaluation rubric indicators
North carolina medical examiner toxicology