template class Elem Type 25 Seq ListElem Type
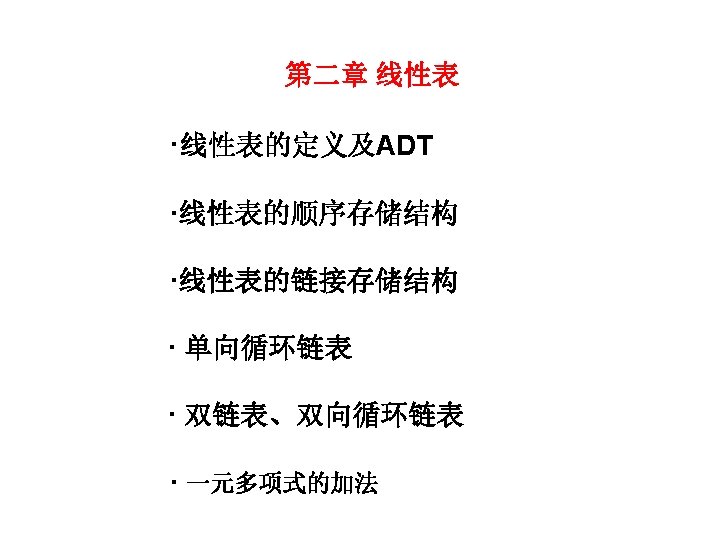
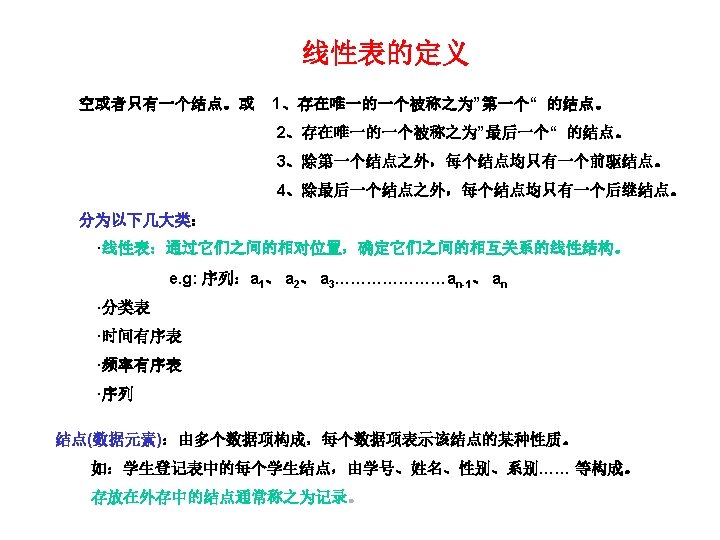
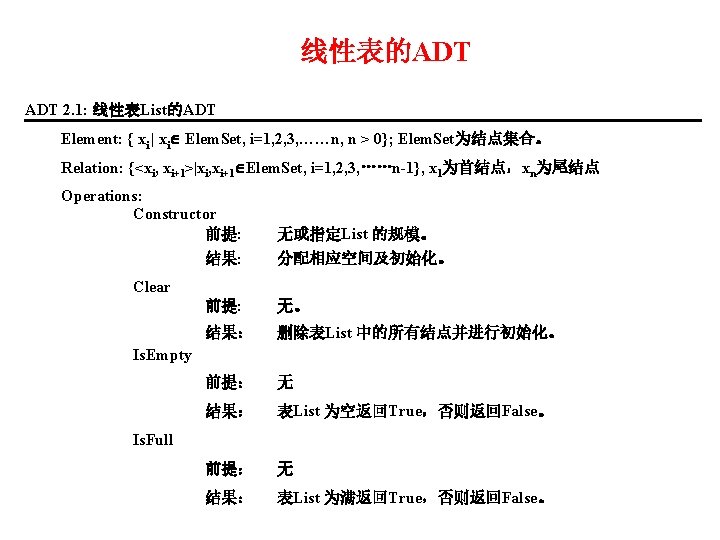
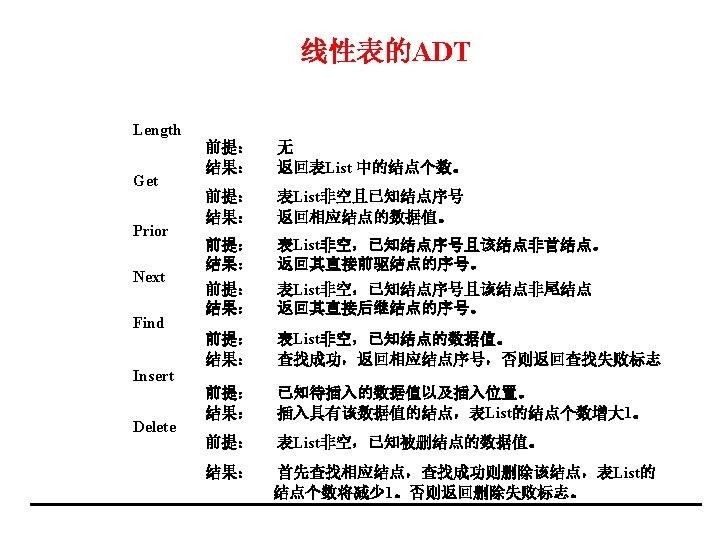
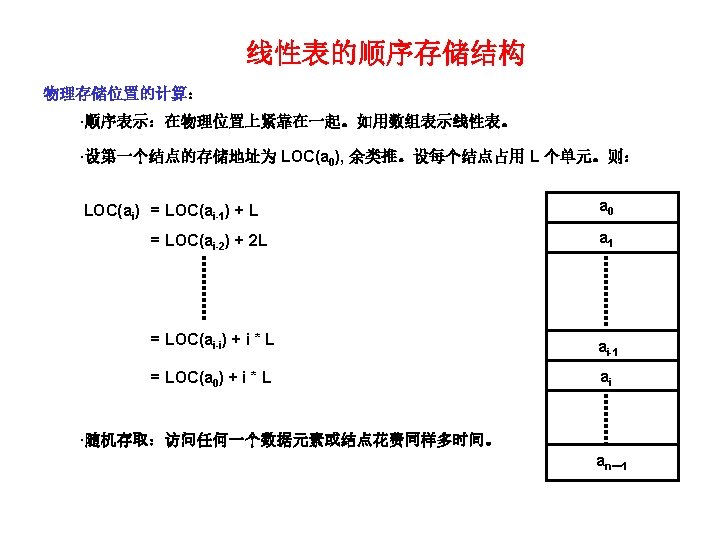
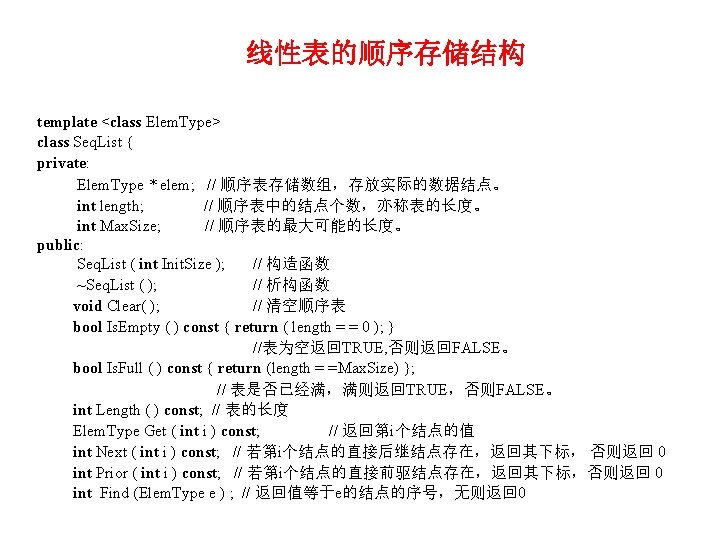
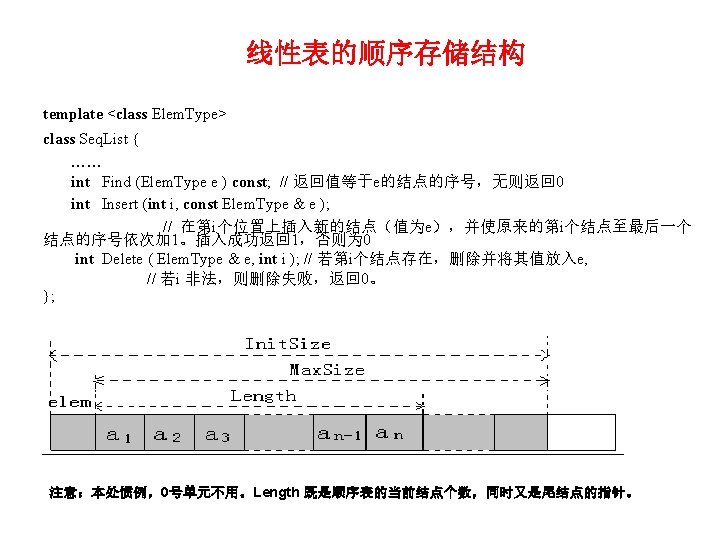
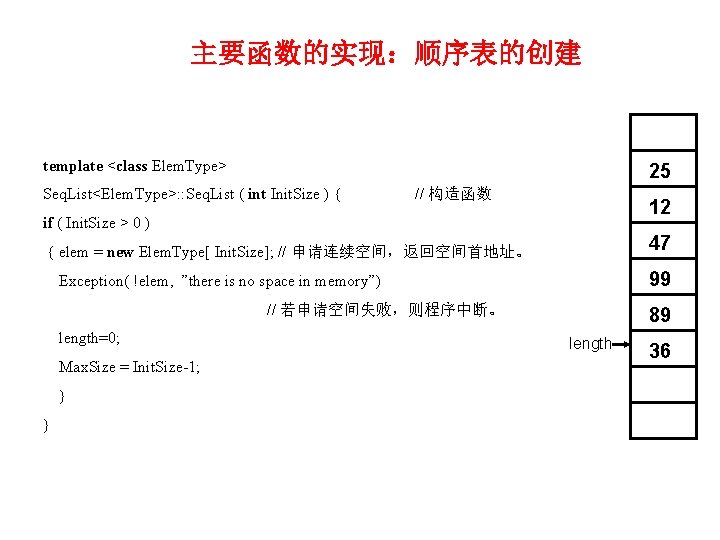
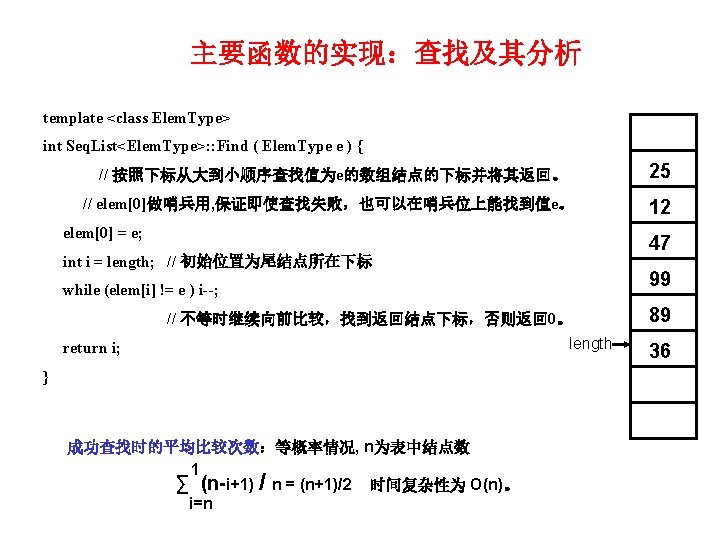
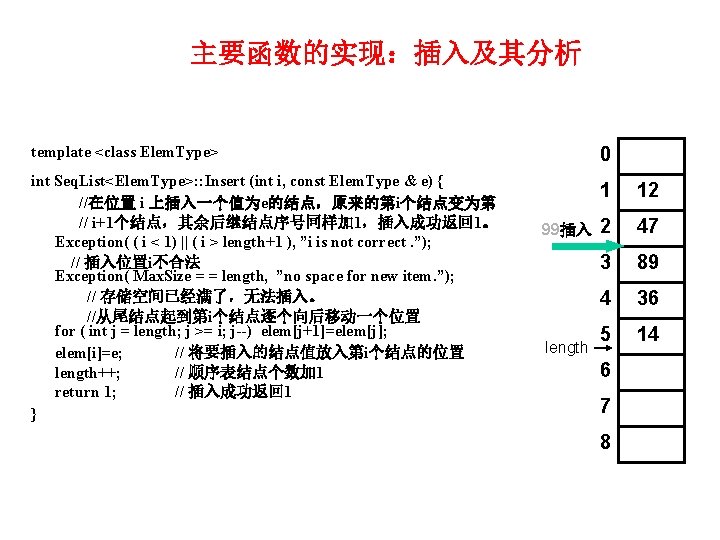
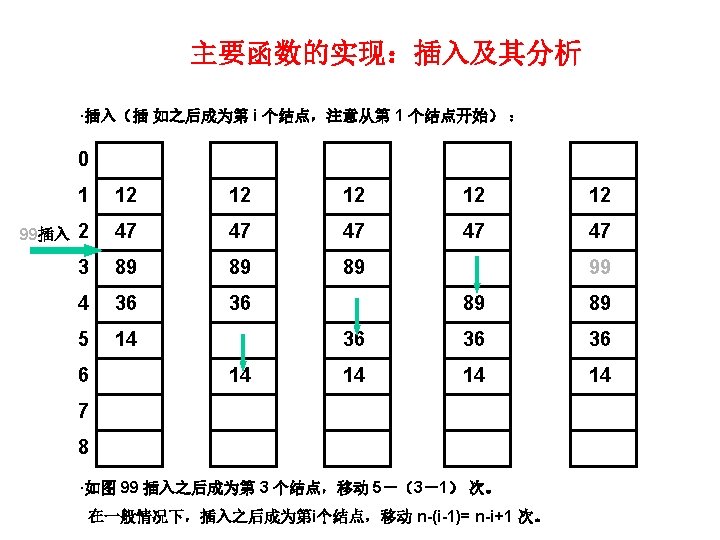
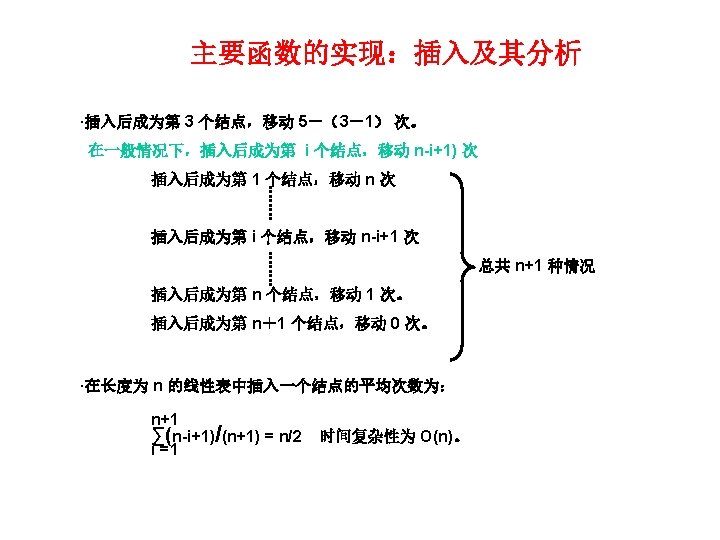
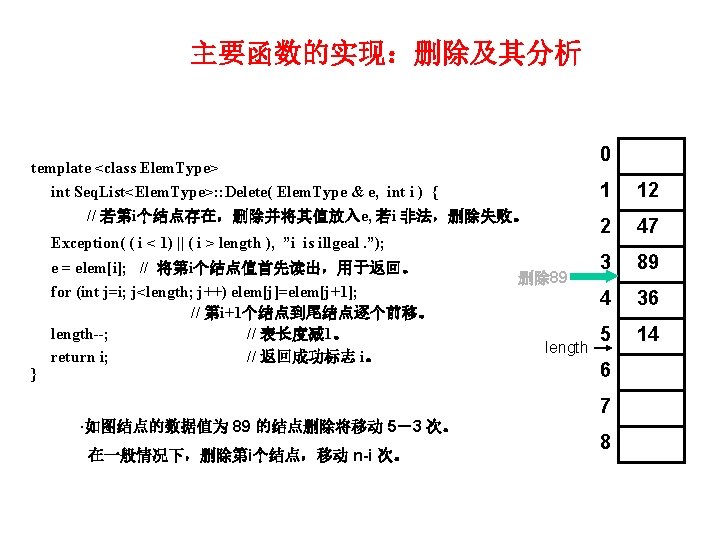
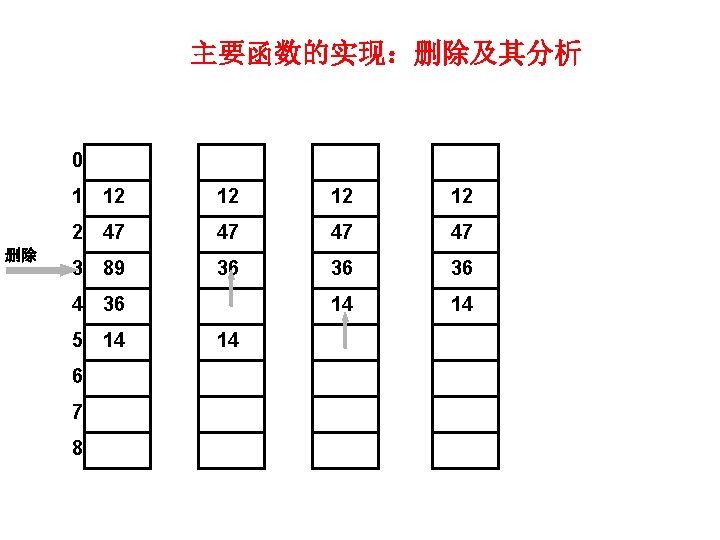
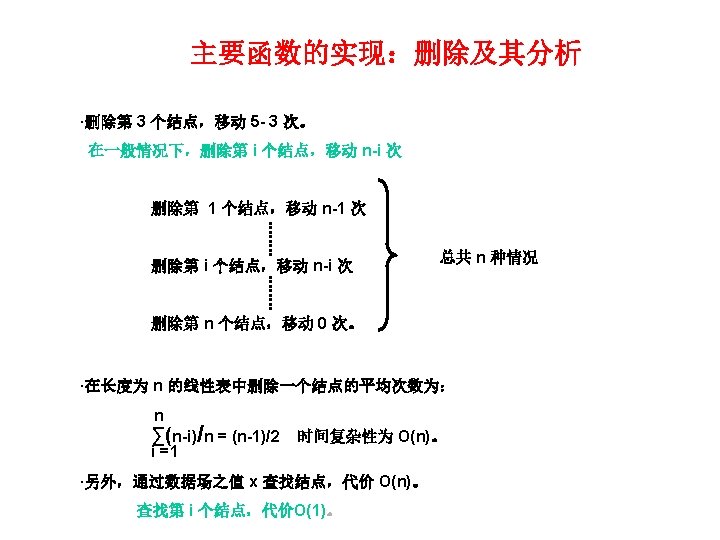
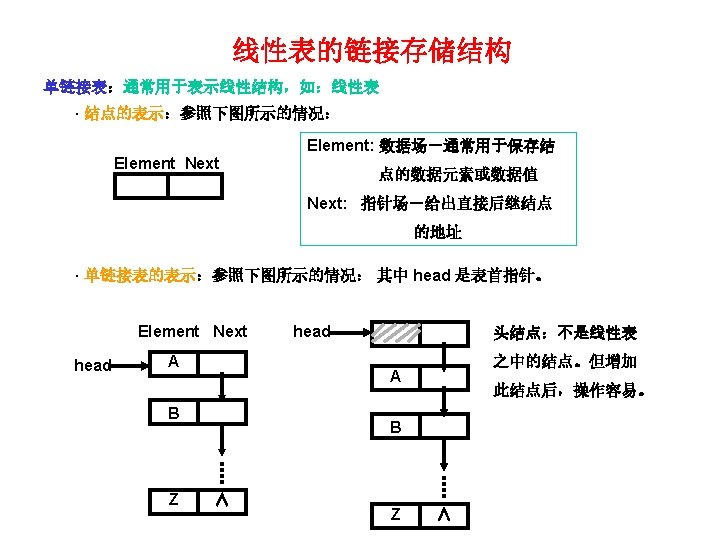
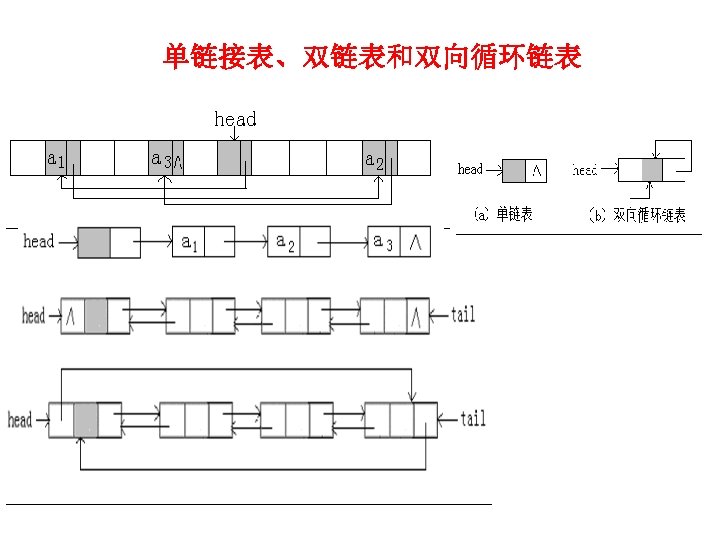
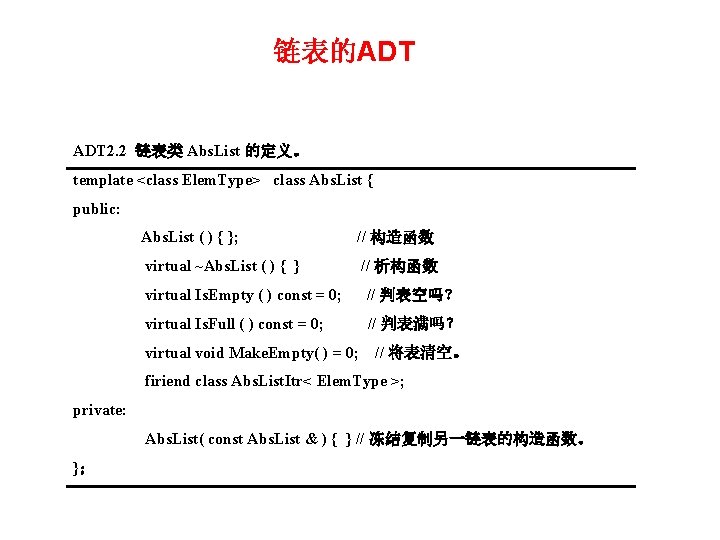
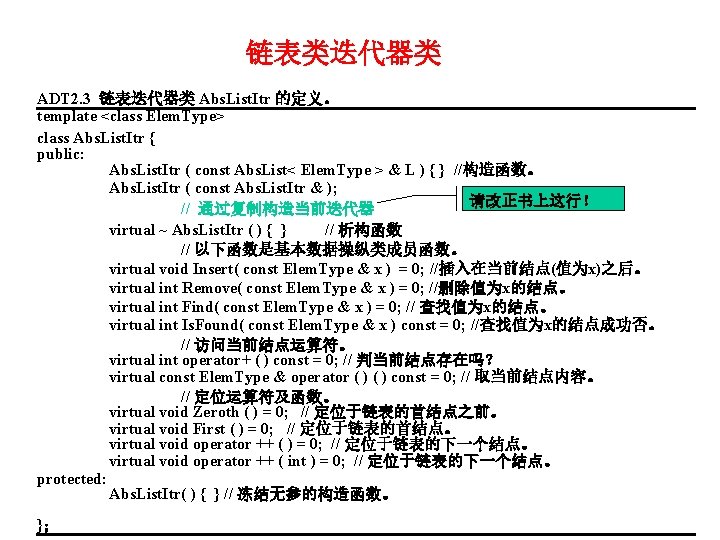
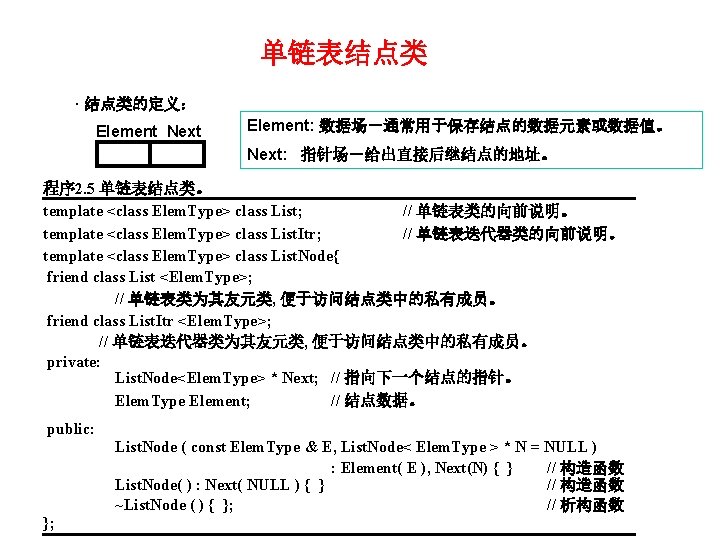
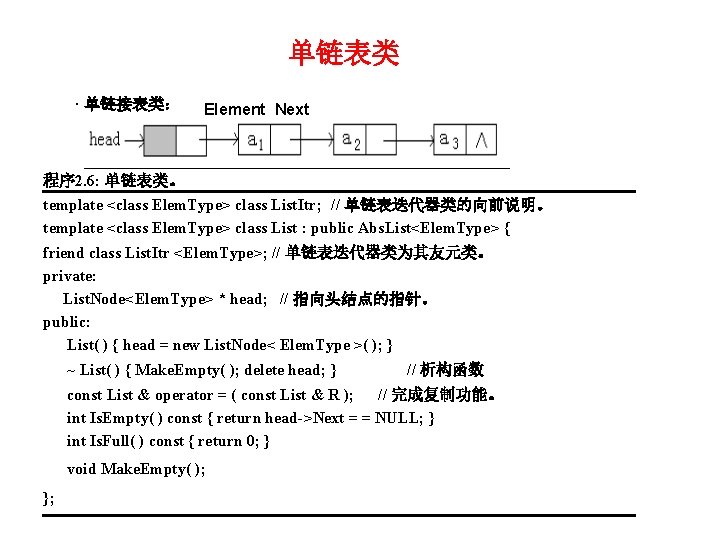
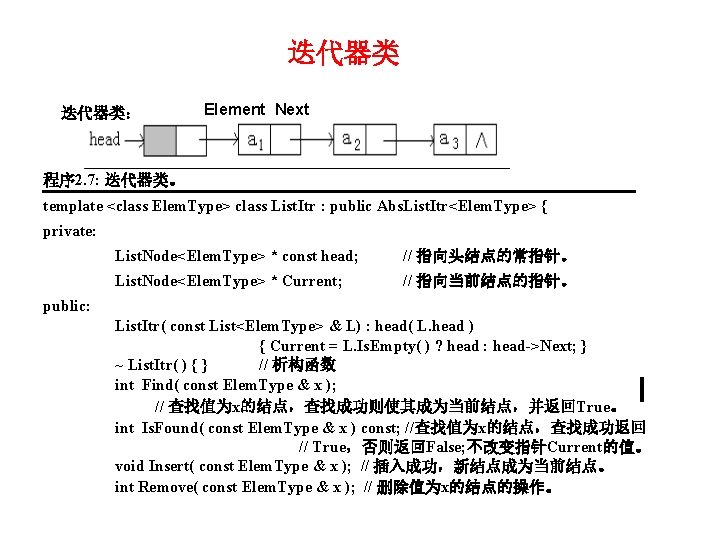
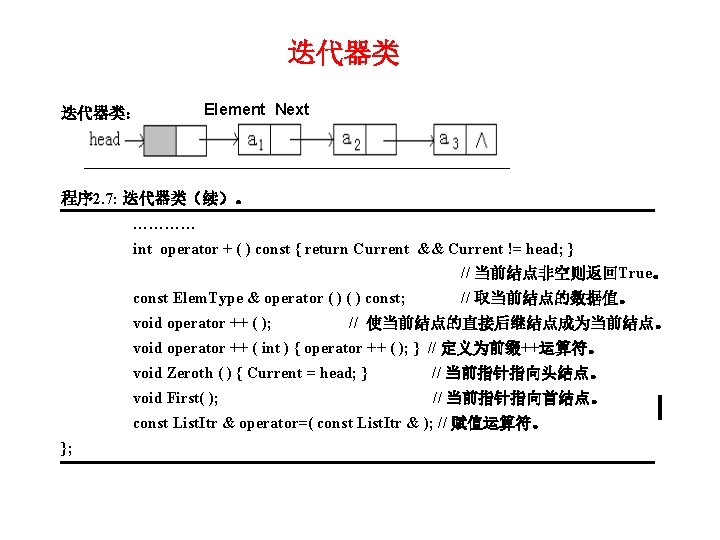
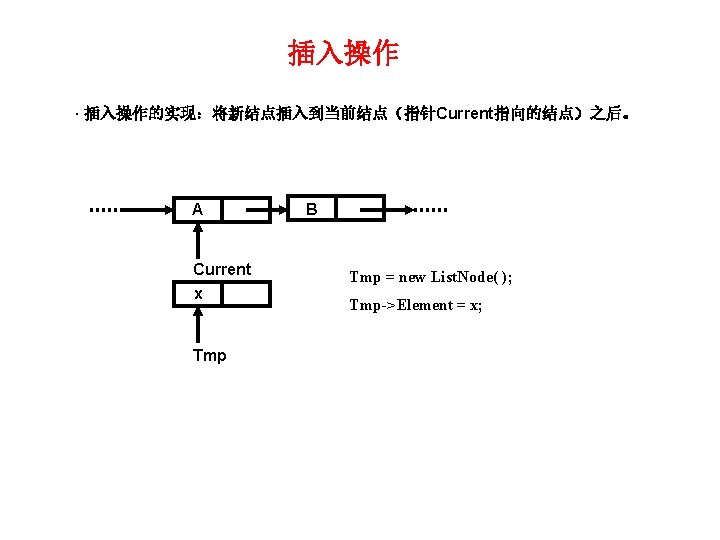
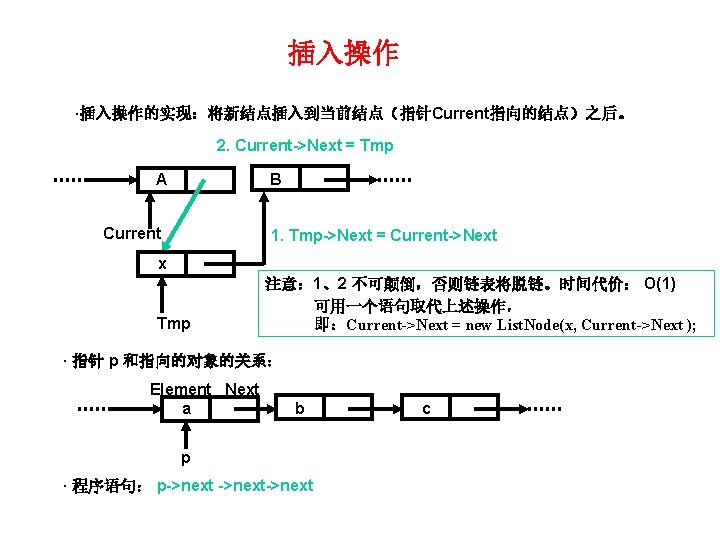
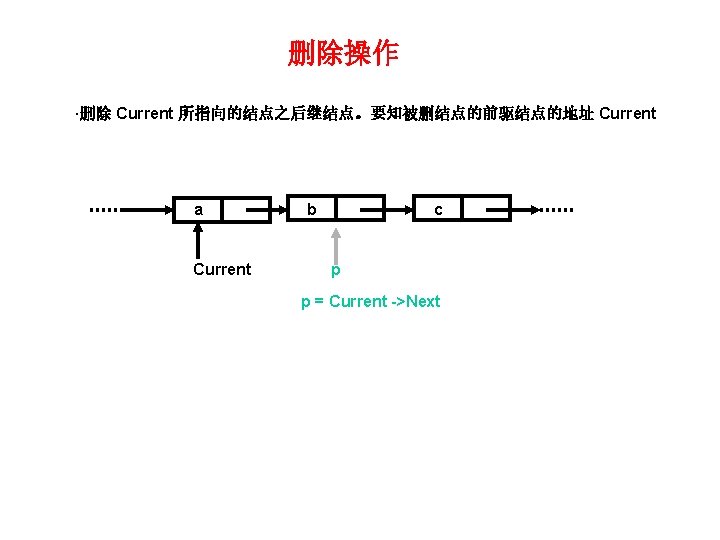
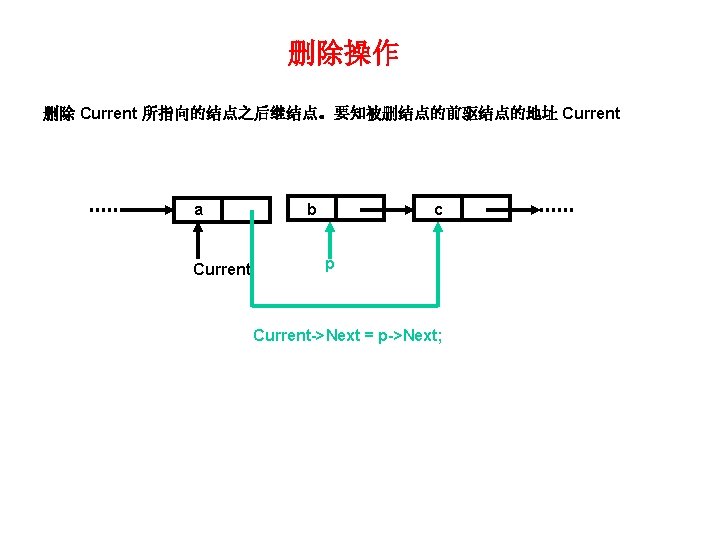
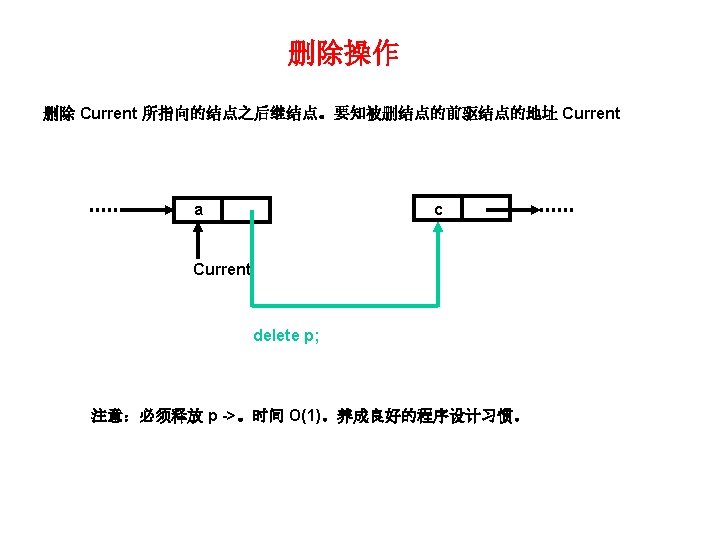
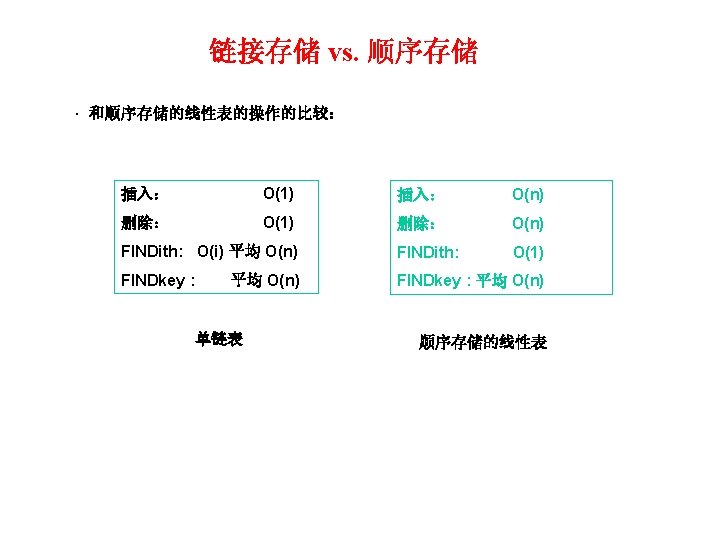
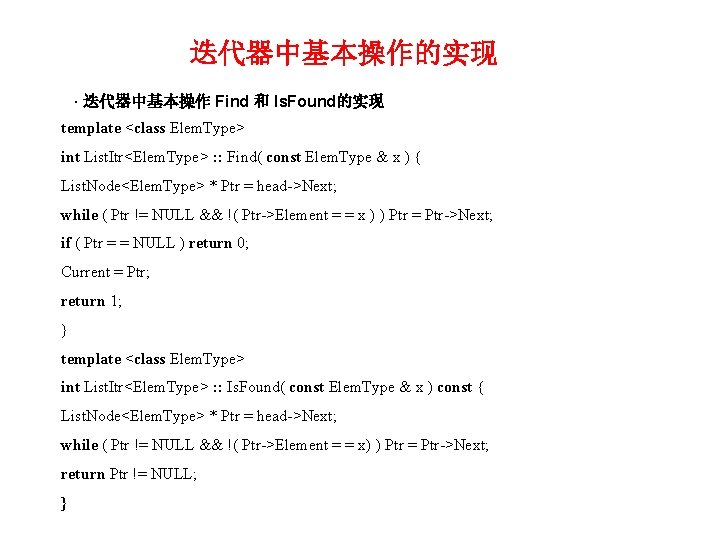
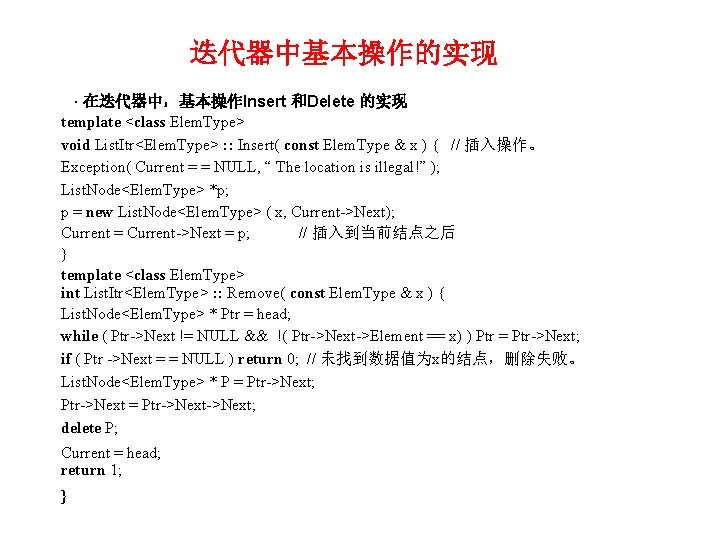
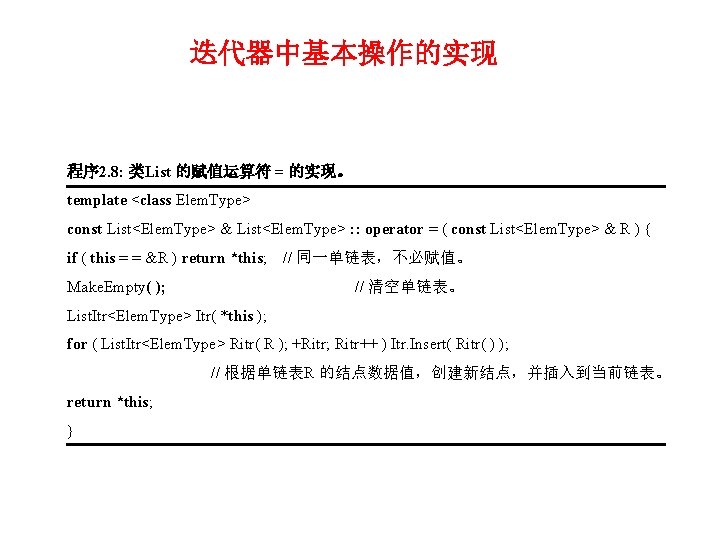
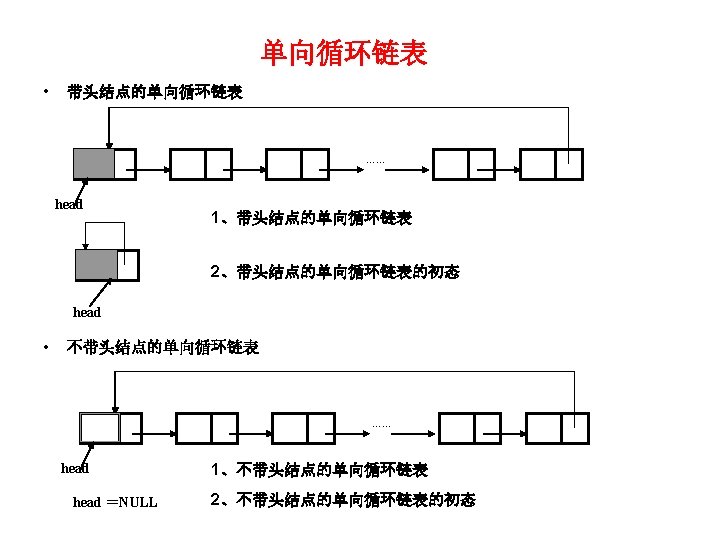
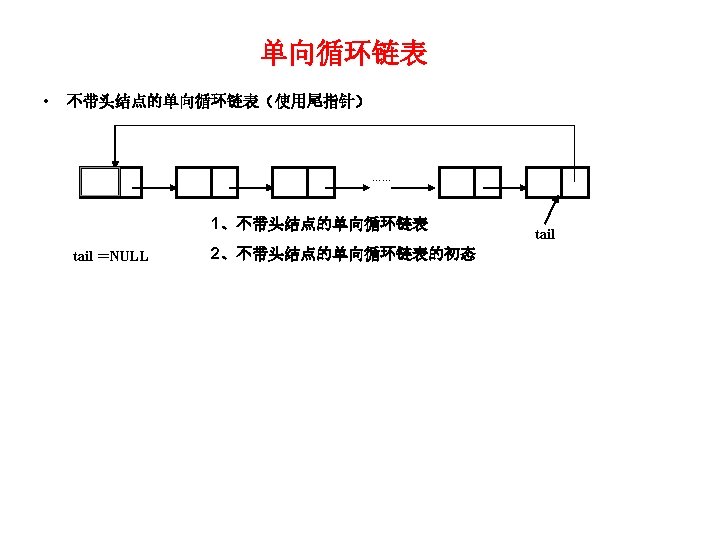
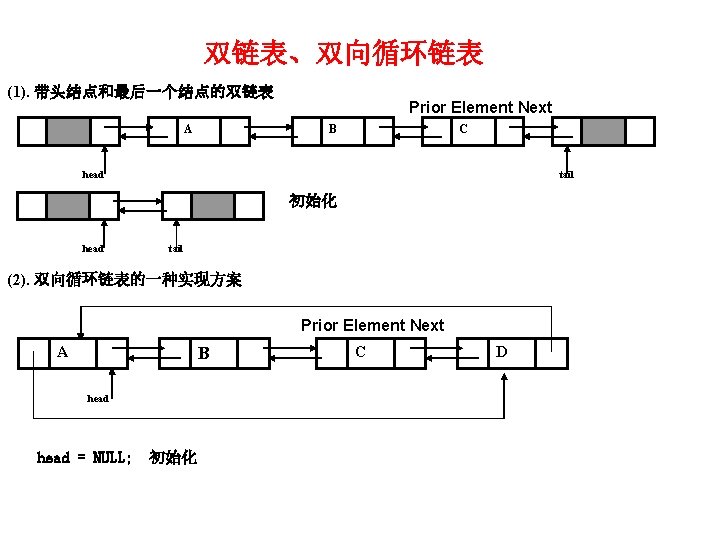
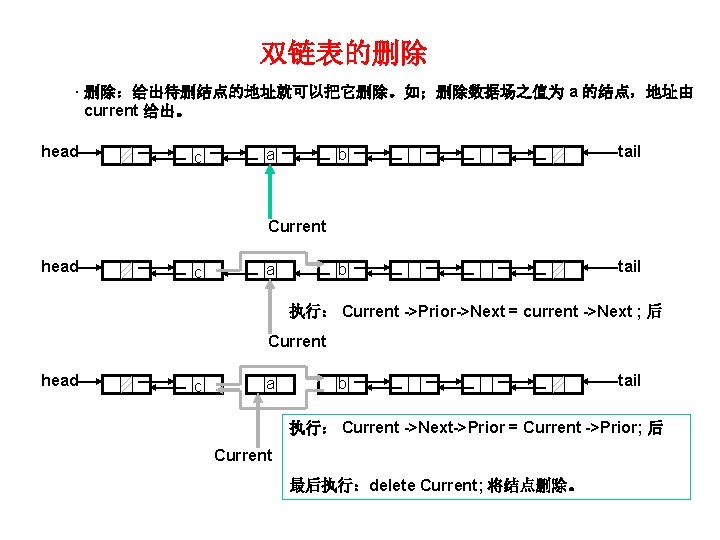
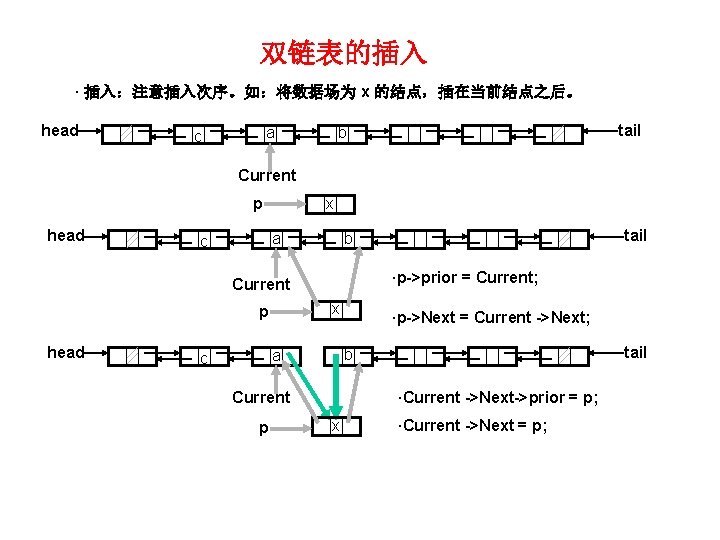
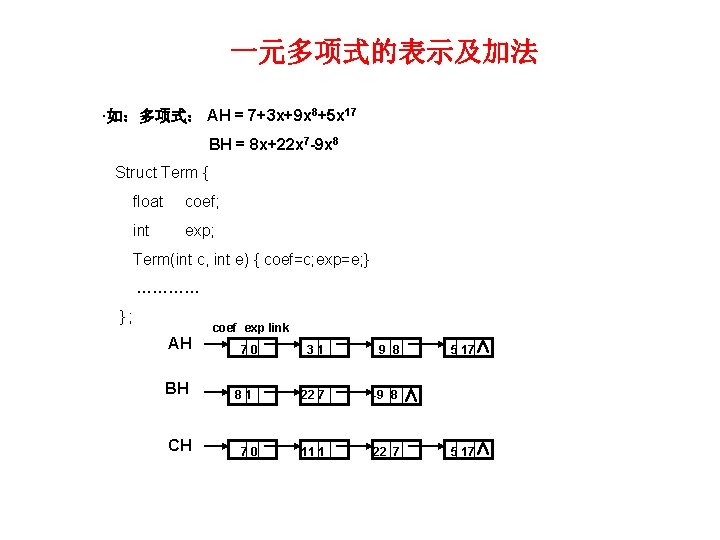
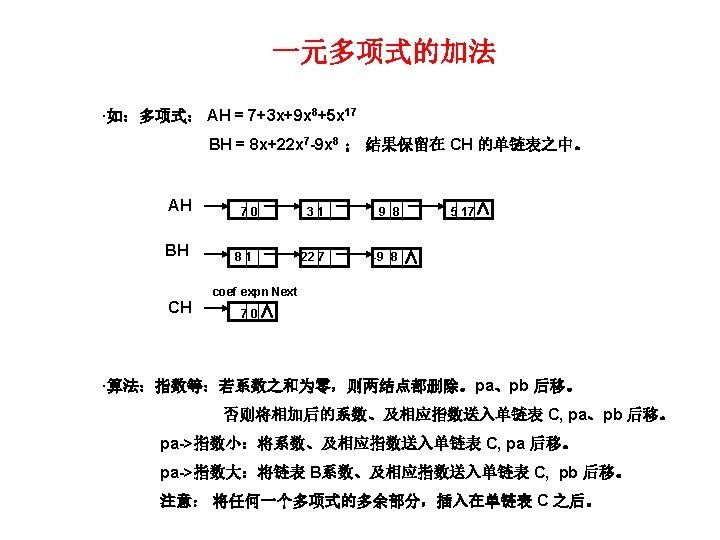
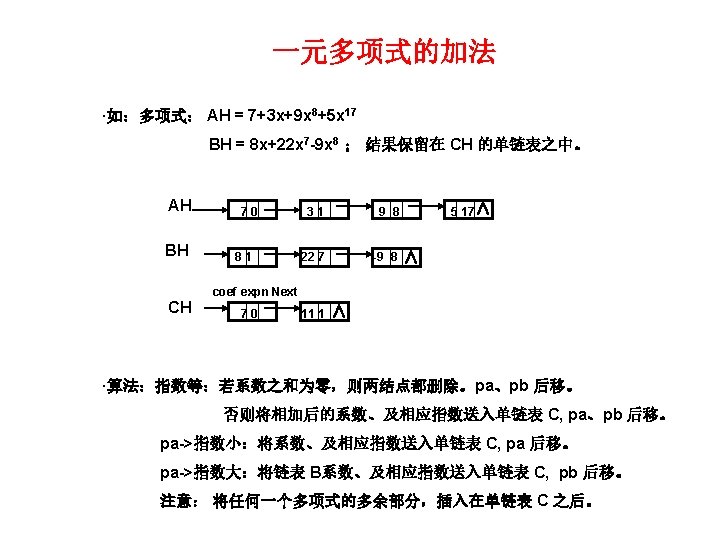
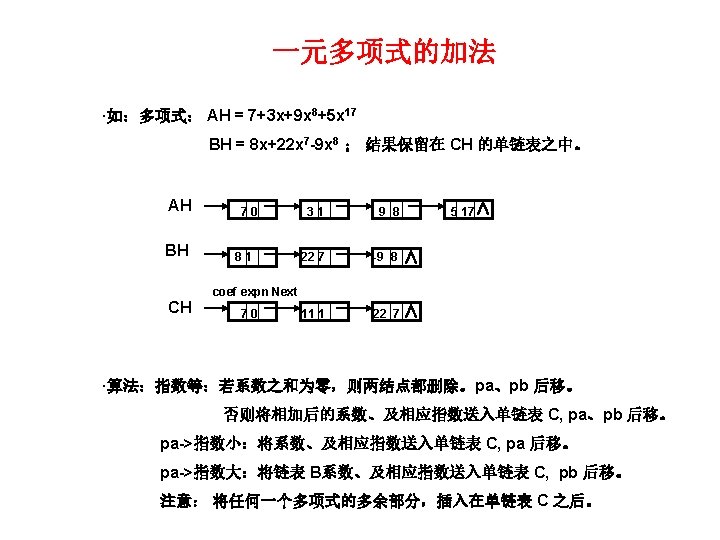
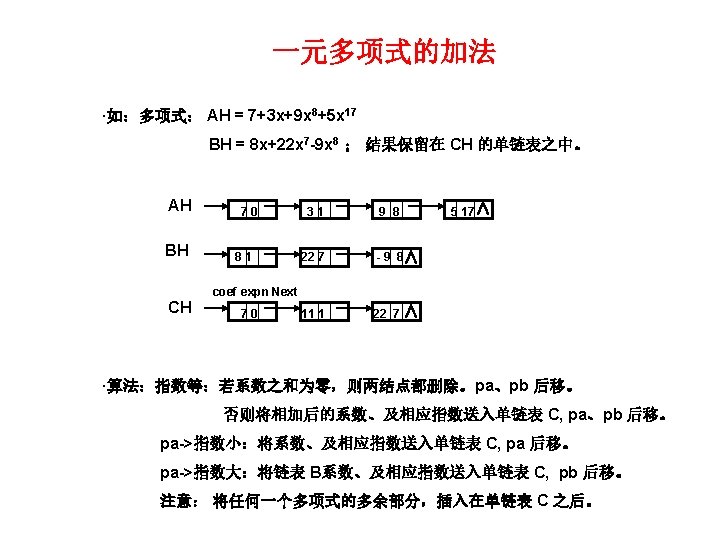
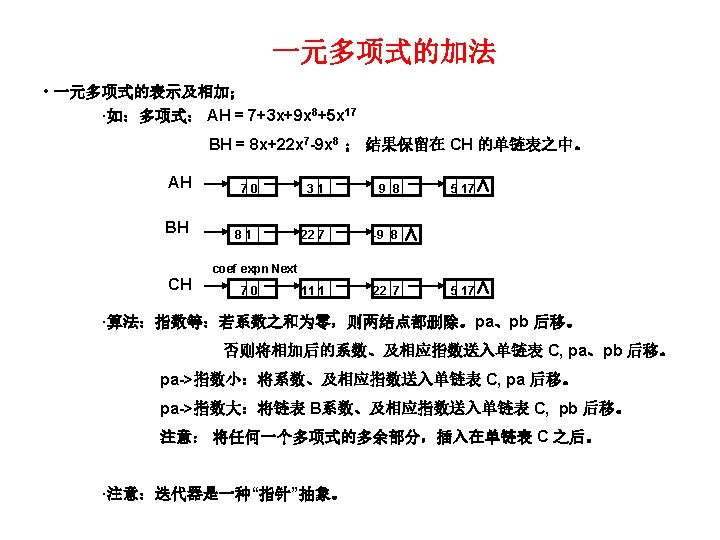
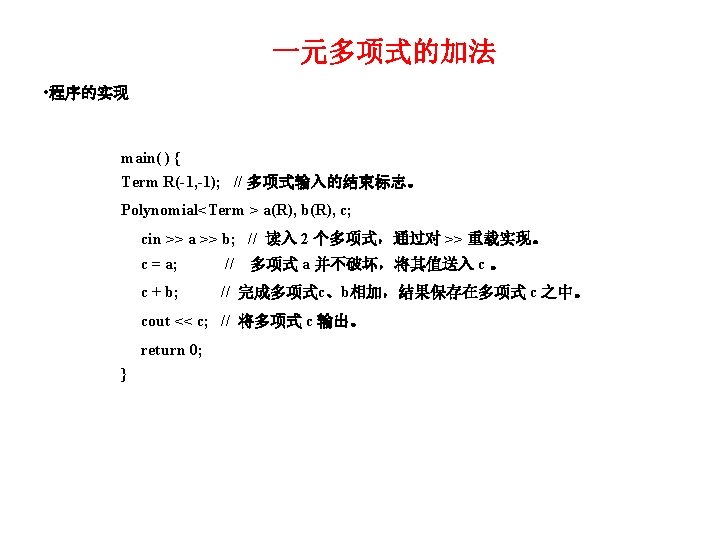
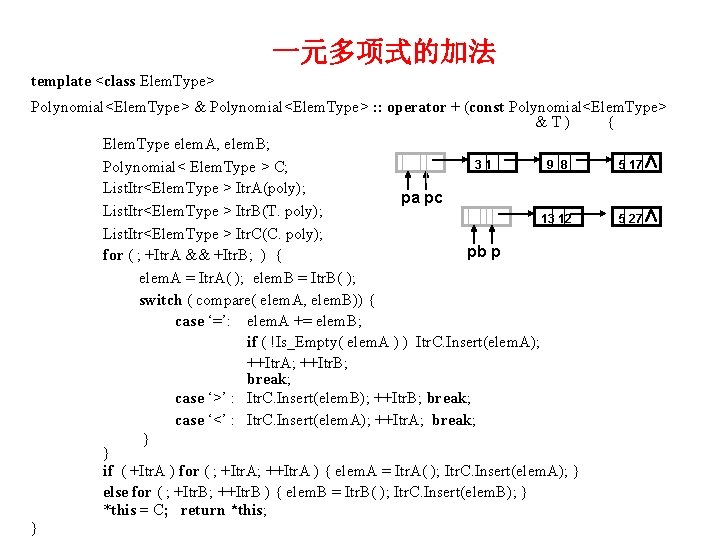
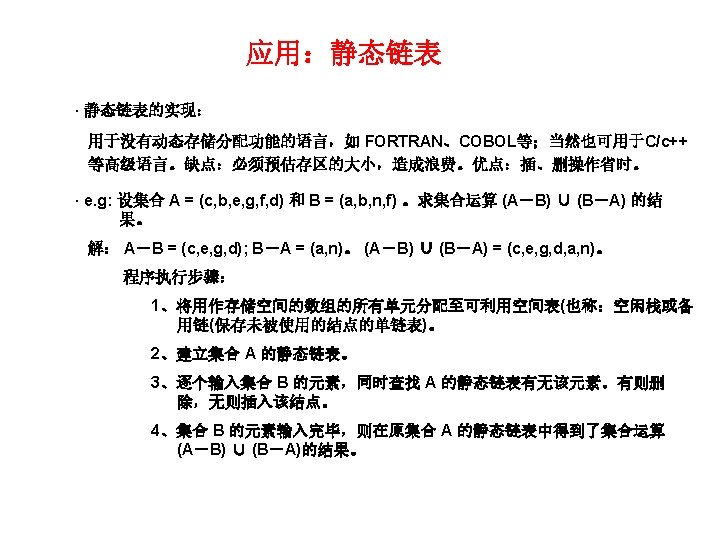
![应用:静态链表 1、将用作存储空间的数组的所有单元分配至空闲栈或备用链。 data cur space[0] 1 space[1] 2 space[2] space[3] 3 space[4] 4 5 应用:静态链表 1、将用作存储空间的数组的所有单元分配至空闲栈或备用链。 data cur space[0] 1 space[1] 2 space[2] space[3] 3 space[4] 4 5](https://slidetodoc.com/presentation_image_h2/d6050df241ccc663b15ba39ffda42a93/image-47.jpg)
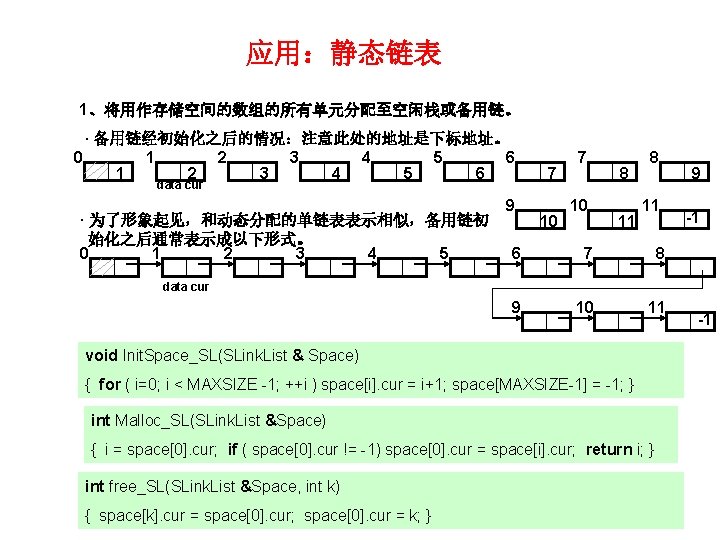
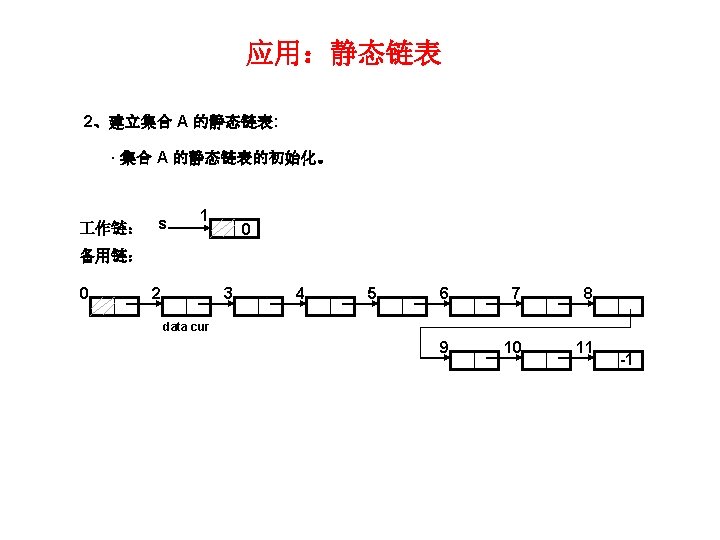
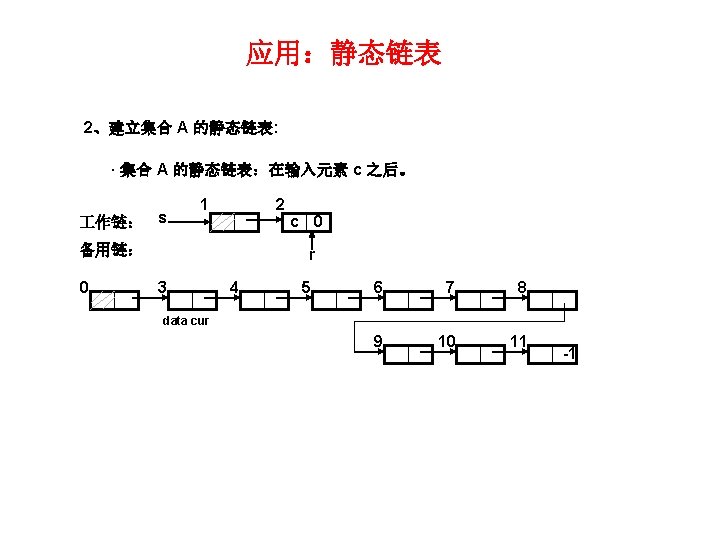
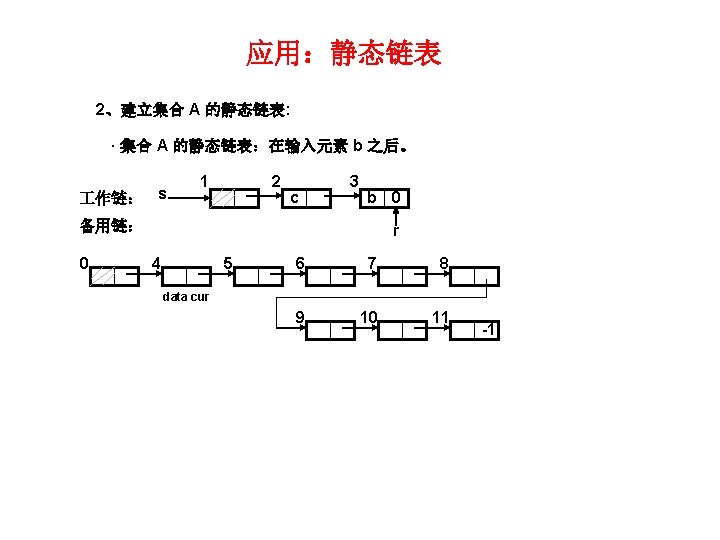
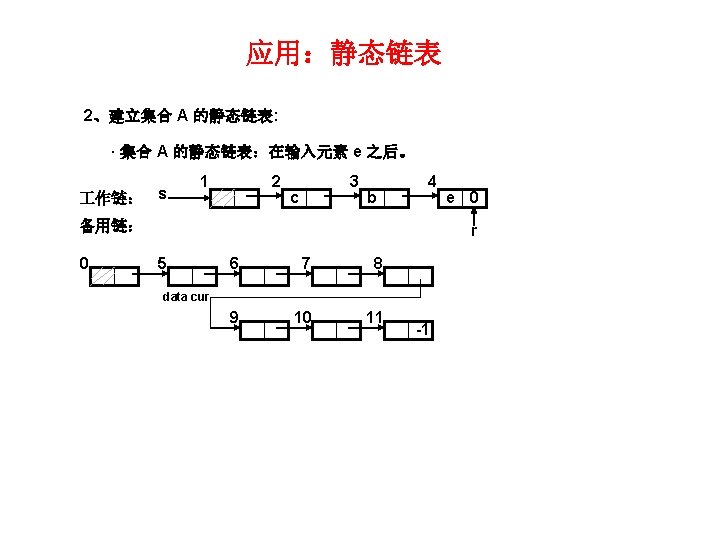
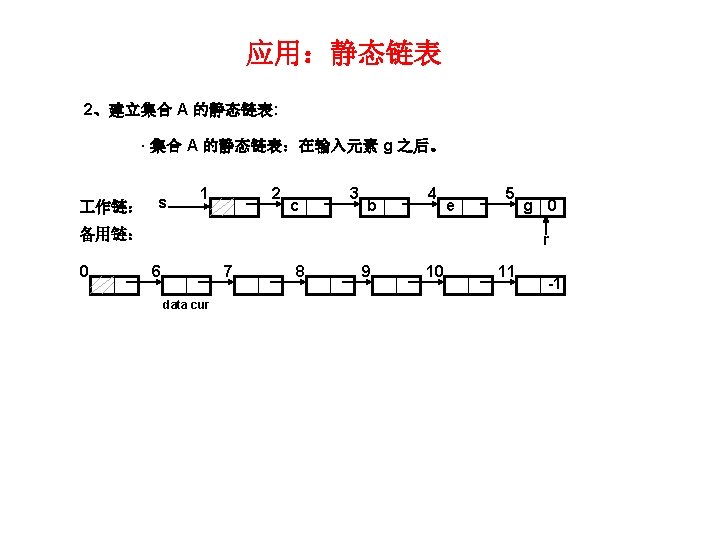
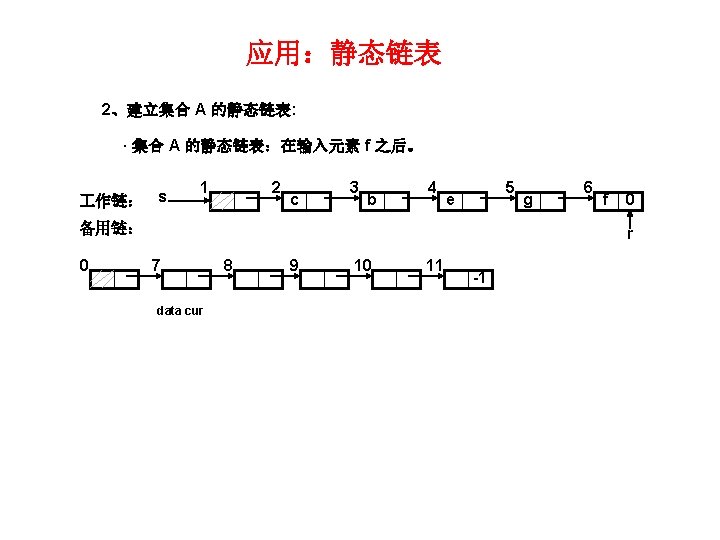
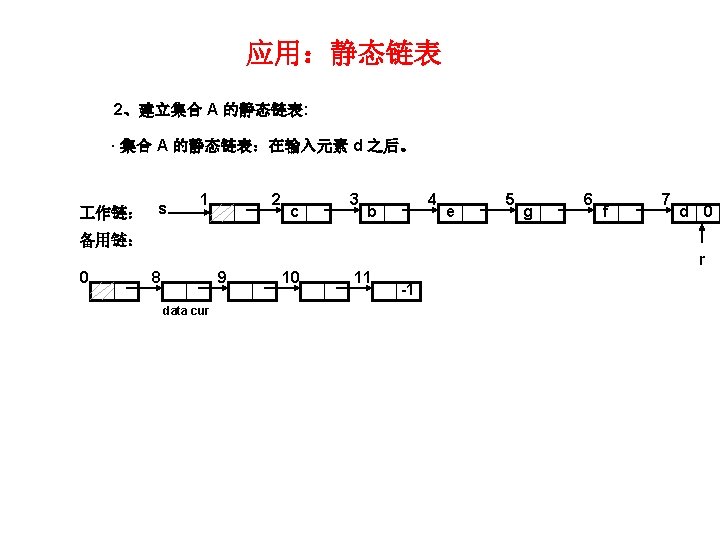
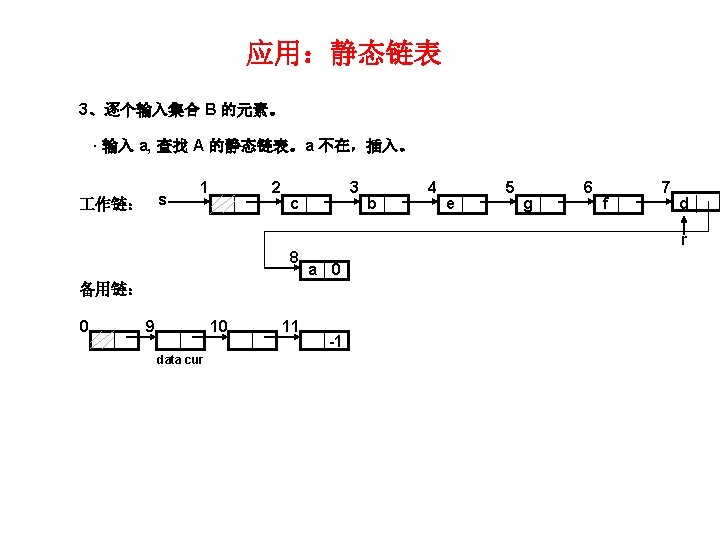
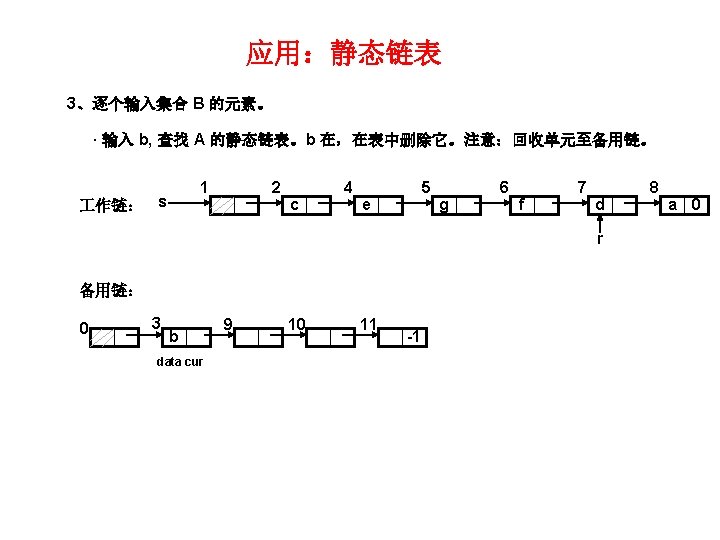
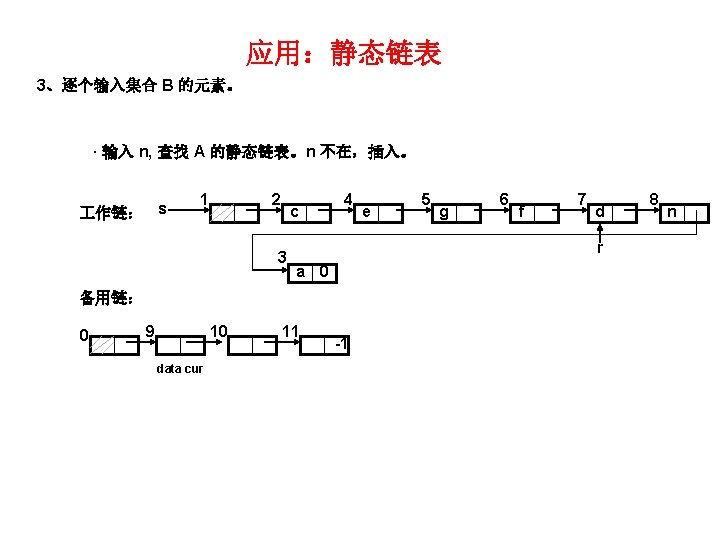
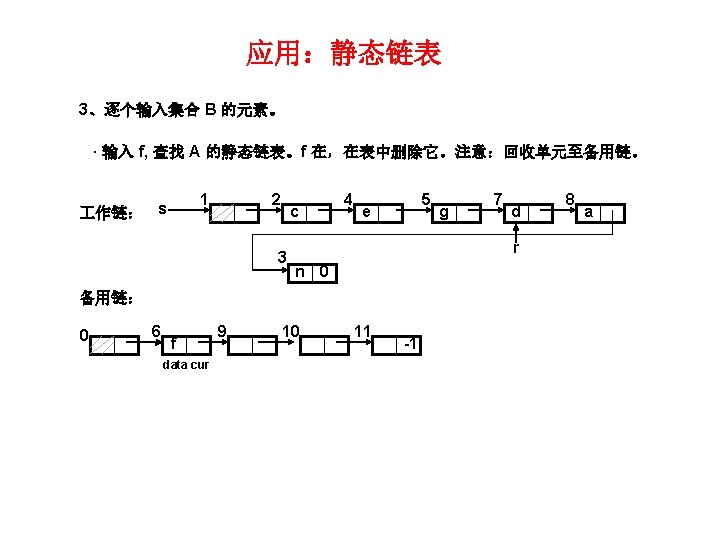
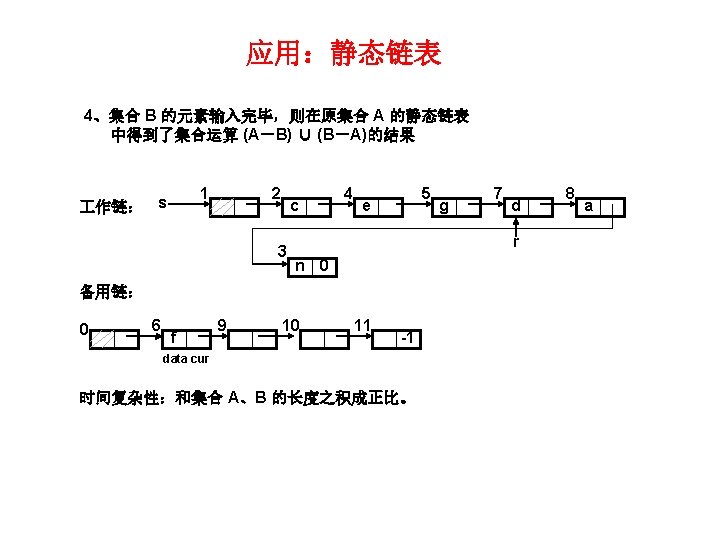
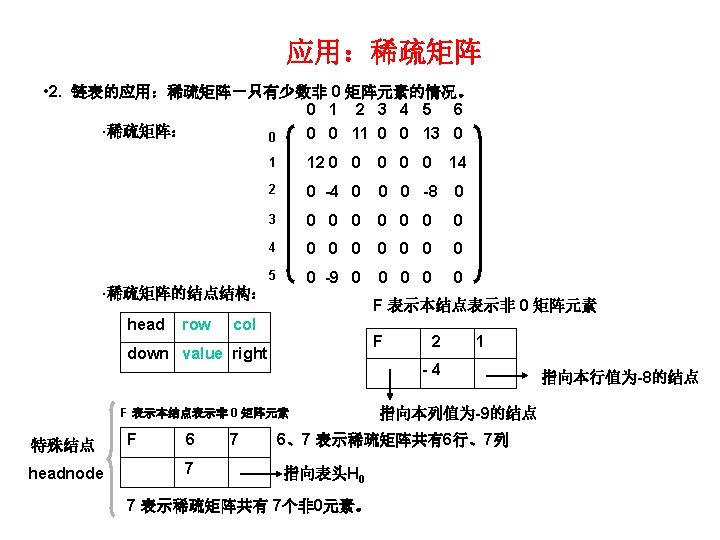
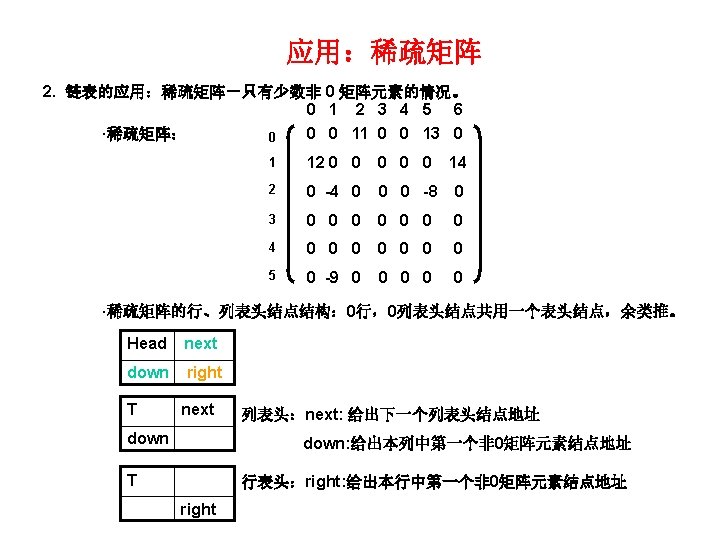
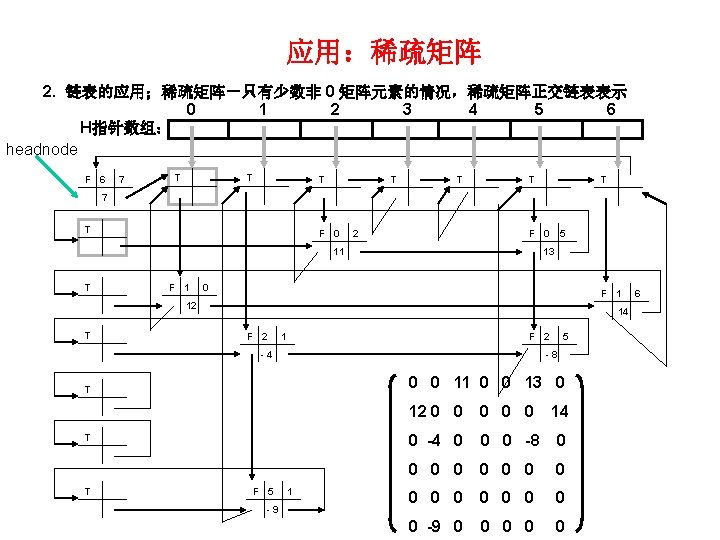
- Slides: 63
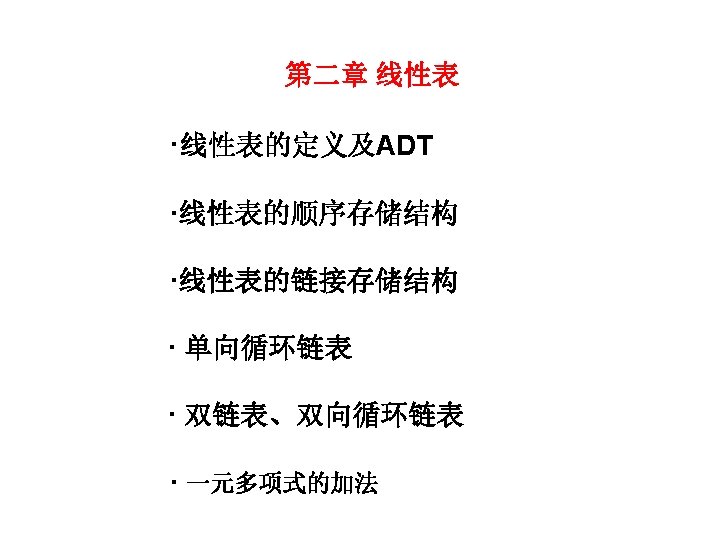
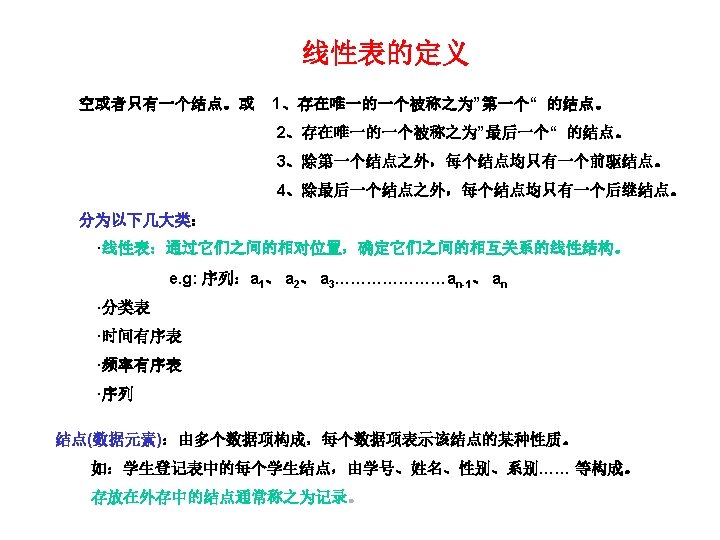
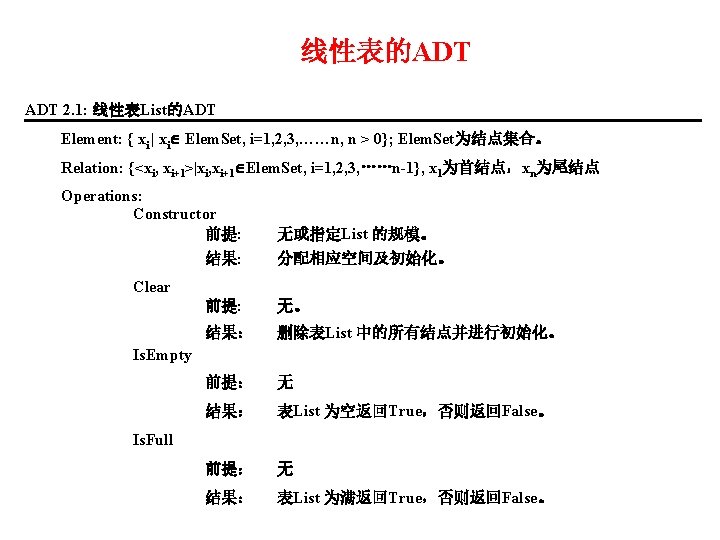
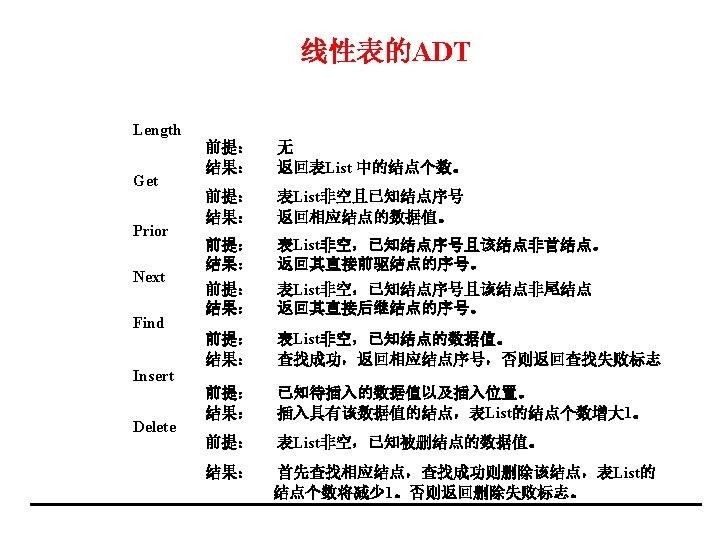
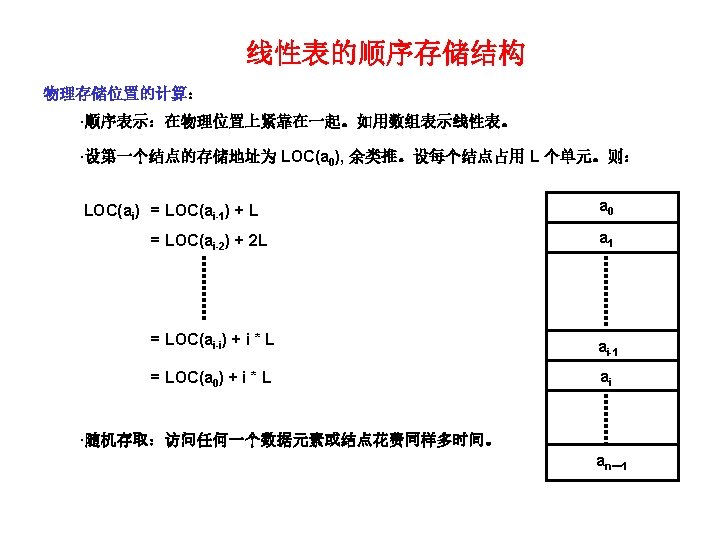
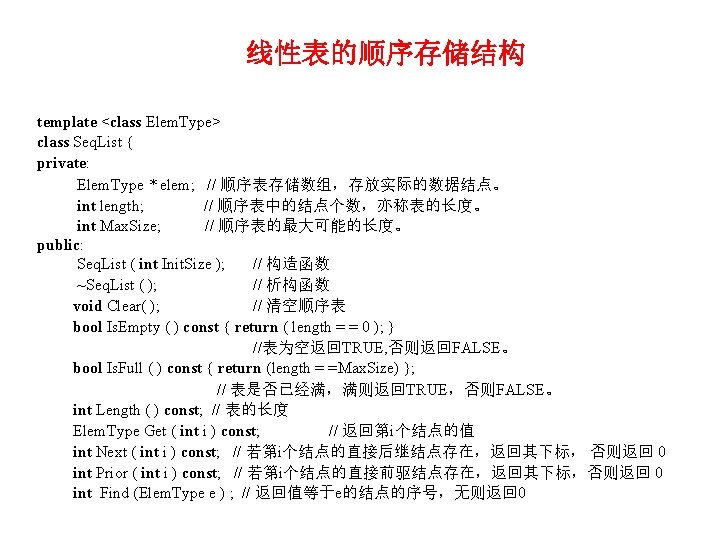
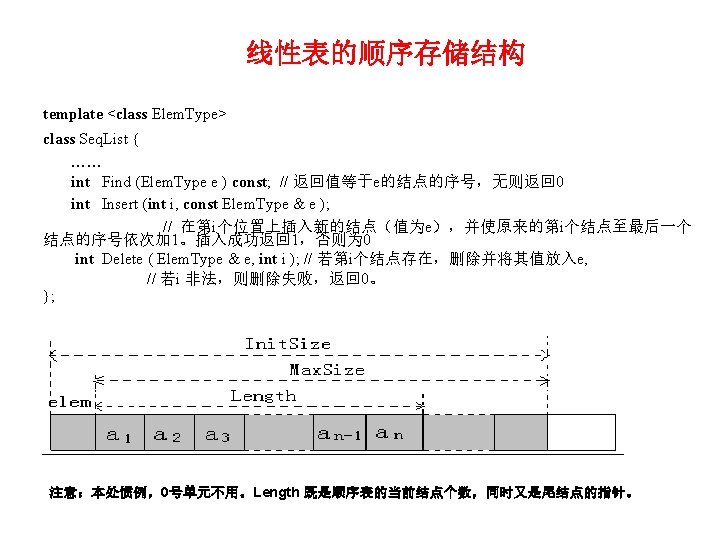
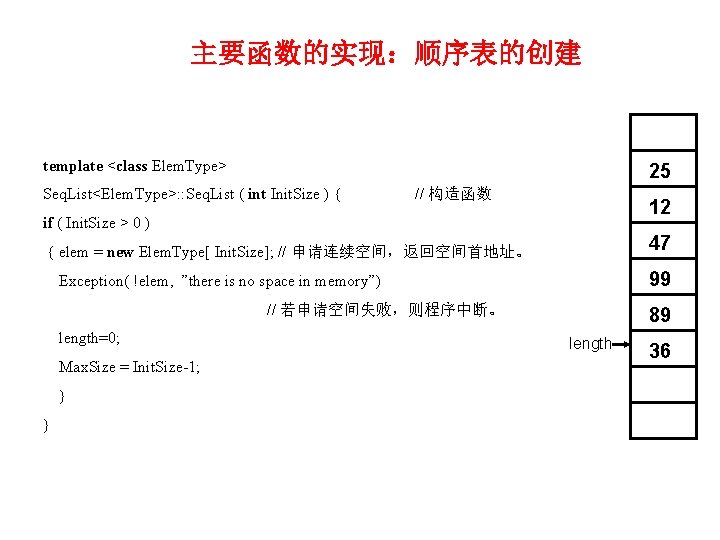
主要函数的实现:顺序表的创建 template <class Elem. Type> 25 Seq. List<Elem. Type>: : Seq. List ( int Init. Size ) { // 构造函数 12 if ( Init. Size > 0 ) 47 { elem = new Elem. Type[ Init. Size]; // 申请连续空间,返回空间首地址。 99 Exception( !elem, ”there is no space in memory”) // 若申请空间失败,则程序中断。 length=0; Max. Size = Init. Size-1; } } 89 length 36
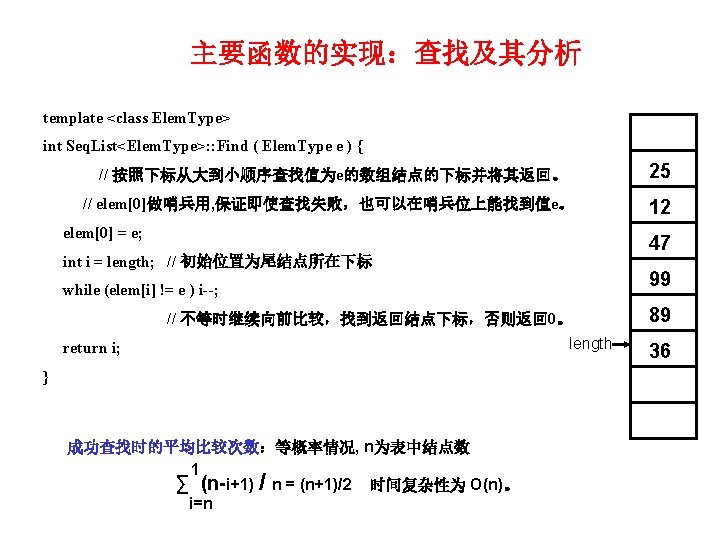
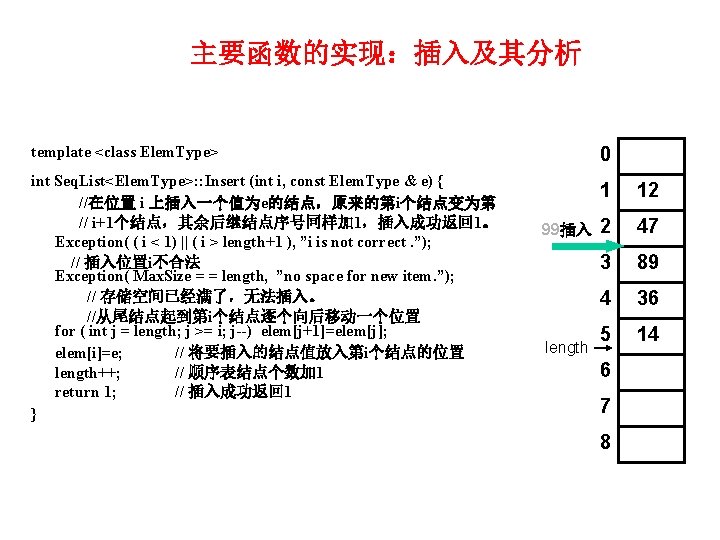
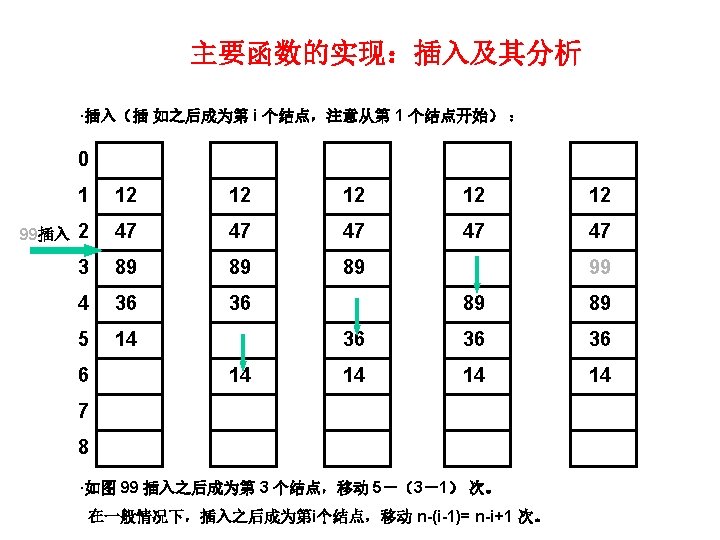
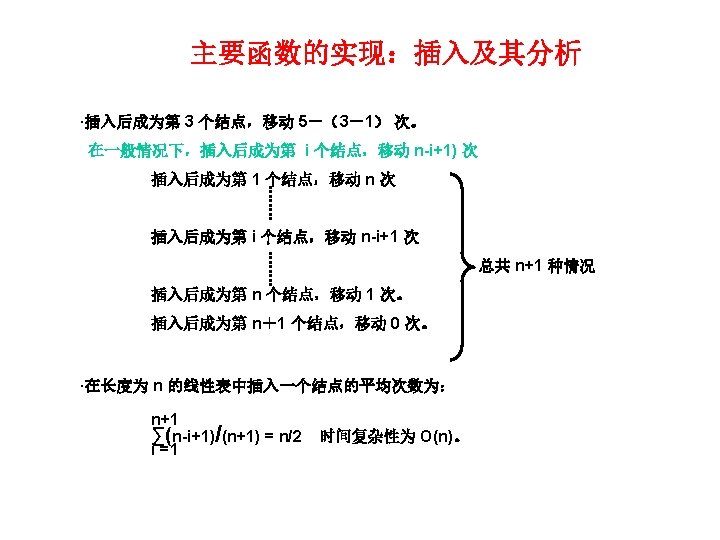
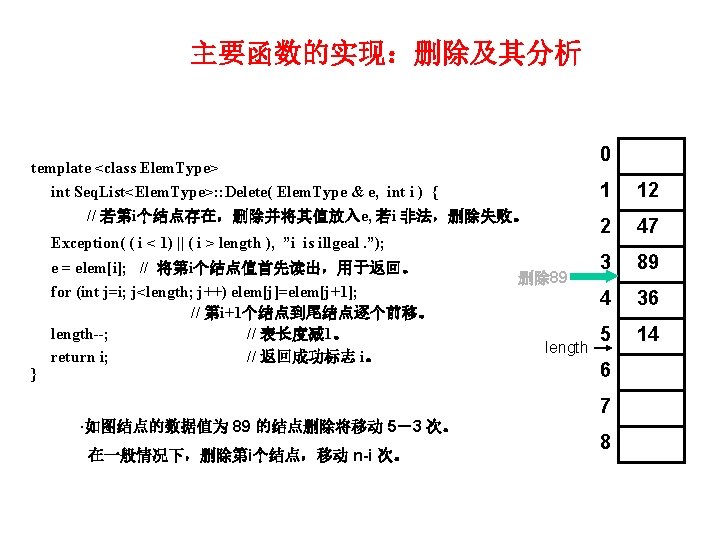
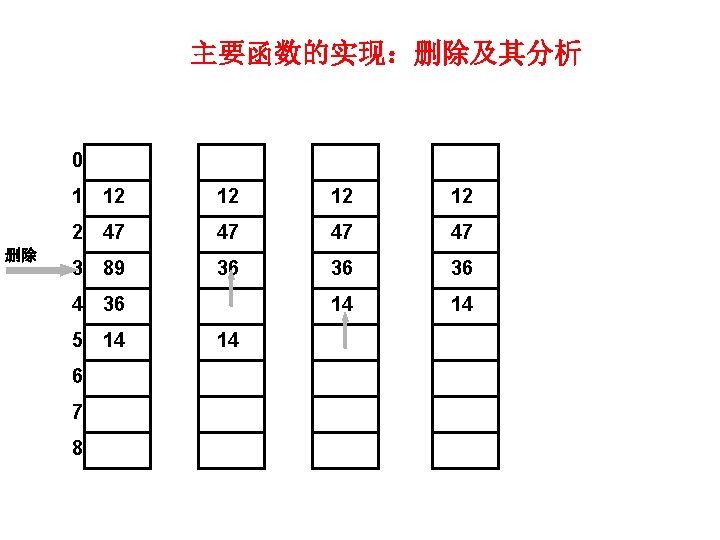
主要函数的实现:删除及其分析 0 删除 1 12 12 2 47 47 3 89 36 36 36 14 14 4 36 5 14 6 7 8 14
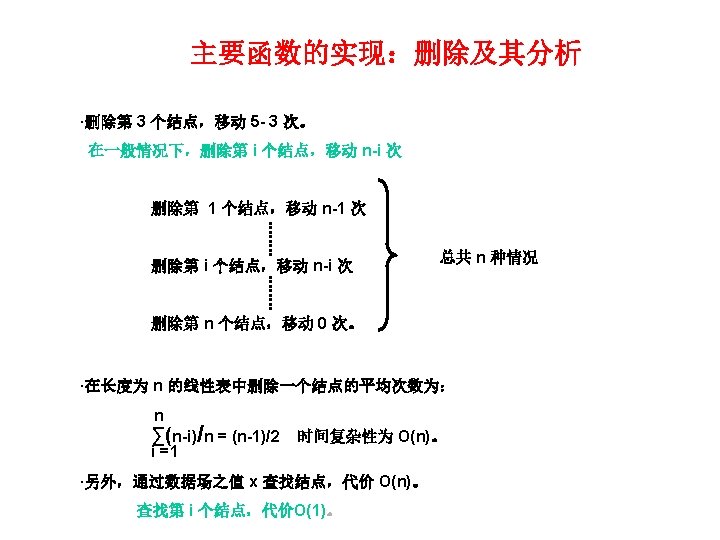
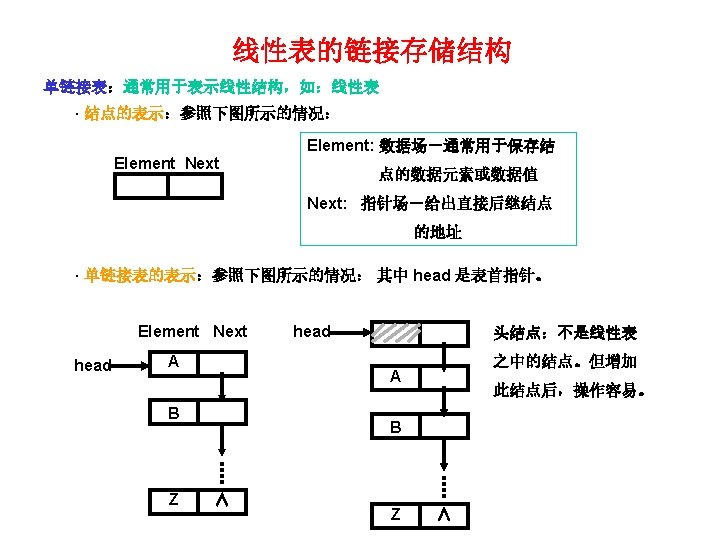
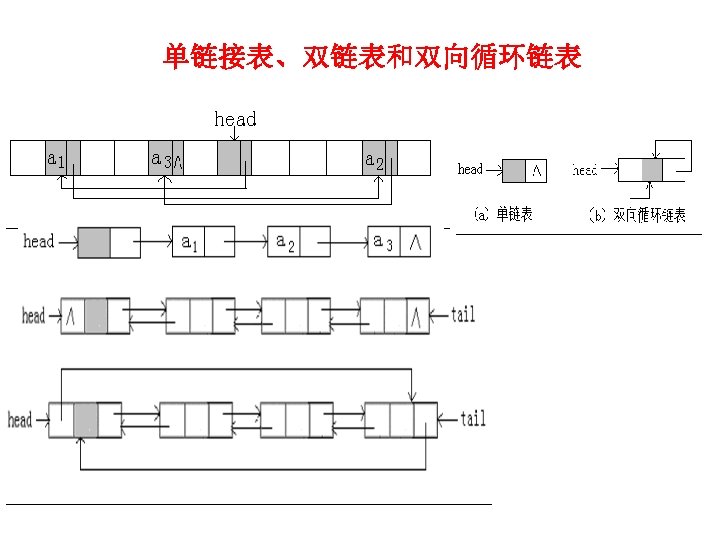
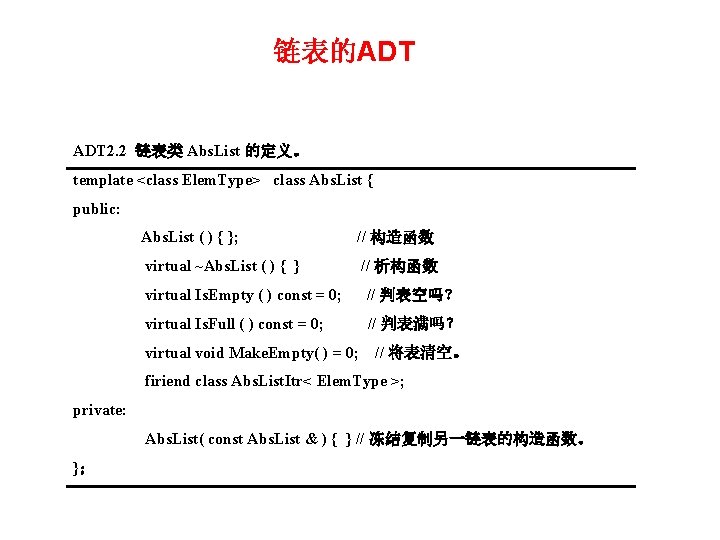
链表的ADT 2. 2 链表类 Abs. List 的定义。 template <class Elem. Type> class Abs. List { public: Abs. List ( ) { }; // 构造函数 virtual ~Abs. List ( ) { } // 析构函数 virtual Is. Empty ( ) const = 0; // 判表空吗? virtual Is. Full ( ) const = 0; // 判表满吗? virtual void Make. Empty( ) = 0; // 将表清空。 firiend class Abs. List. Itr< Elem. Type >; private: Abs. List( const Abs. List & ) { } // 冻结复制另一链表的构造函数。 };
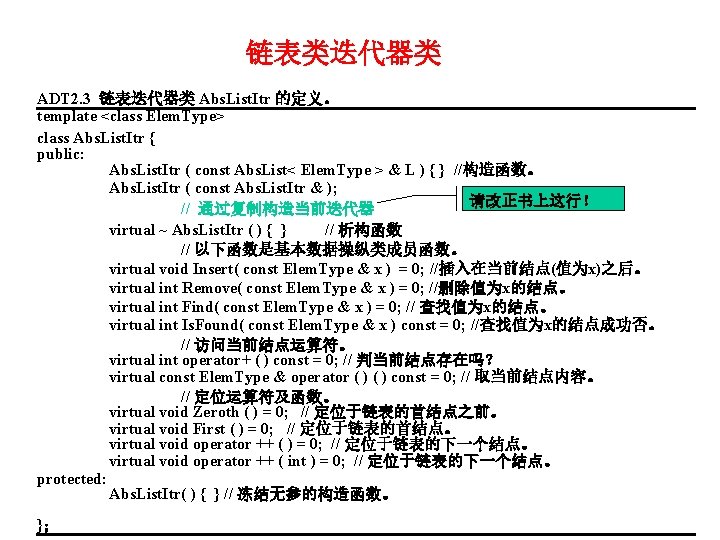
链表类迭代器类 ADT 2. 3 链表迭代器类 Abs. List. Itr 的定义。 template <class Elem. Type> class Abs. List. Itr { public: Abs. List. Itr ( const Abs. List< Elem. Type > & L ) { } //构造函数。 Abs. List. Itr ( const Abs. List. Itr & ); 请改正书上这行! // 通过复制构造当前迭代器 virtual ~ Abs. List. Itr ( ) { } // 析构函数 // 以下函数是基本数据操纵类成员函数。 virtual void Insert( const Elem. Type & x ) = 0; //插入在当前结点(值为x)之后。 virtual int Remove( const Elem. Type & x ) = 0; //删除值为x的结点。 virtual int Find( const Elem. Type & x ) = 0; // 查找值为x的结点。 virtual int Is. Found( const Elem. Type & x ) const = 0; //查找值为x的结点成功否。 // 访问当前结点运算符。 virtual int operator+ ( ) const = 0; // 判当前结点存在吗? virtual const Elem. Type & operator ( ) const = 0; // 取当前结点内容。 // 定位运算符及函数。 virtual void Zeroth ( ) = 0; // 定位于链表的首结点之前。 virtual void First ( ) = 0; // 定位于链表的首结点。 virtual void operator ++ ( ) = 0; // 定位于链表的下一个结点。 virtual void operator ++ ( int ) = 0; // 定位于链表的下一个结点。 protected: Abs. List. Itr( ) { } // 冻结无参的构造函数。 };
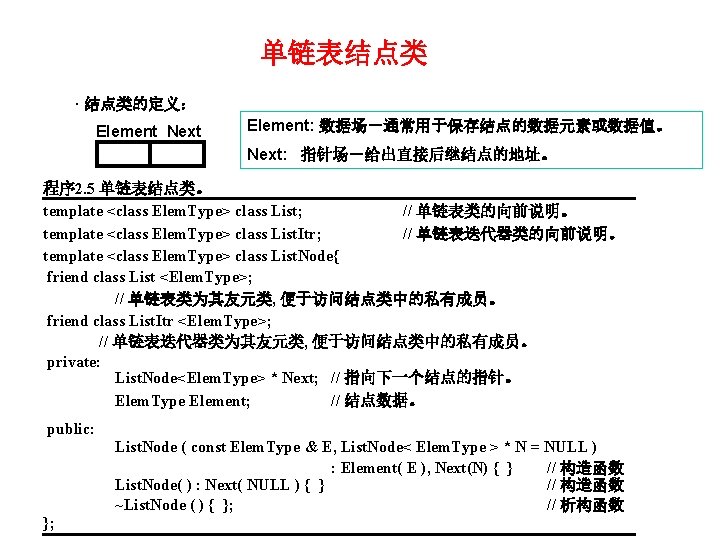
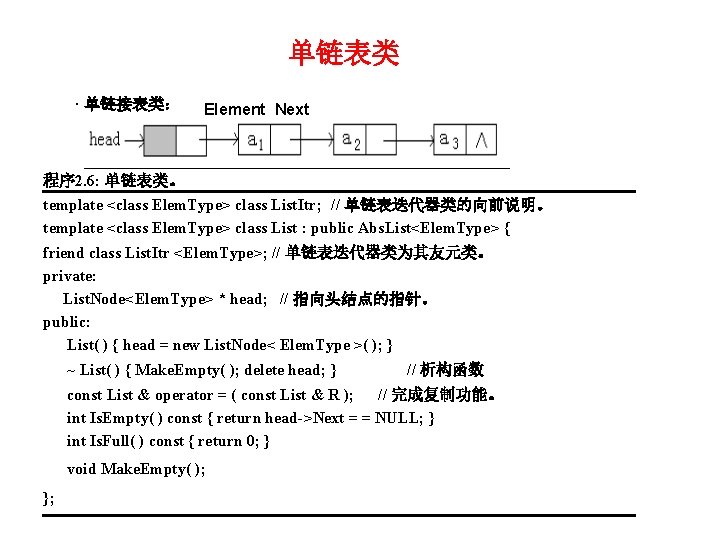
单链表类 · 单链接表类: Element Next 程序 2. 6: 单链表类。 template <class Elem. Type> class List. Itr; // 单链表迭代器类的向前说明。 template <class Elem. Type> class List : public Abs. List<Elem. Type> { friend class List. Itr <Elem. Type>; // 单链表迭代器类为其友元类。 private: List. Node<Elem. Type> * head; // 指向头结点的指针。 public: List( ) { head = new List. Node< Elem. Type >( ); } ~ List( ) { Make. Empty( ); delete head; } // 析构函数 const List & operator = ( const List & R ); // 完成复制功能。 int Is. Empty( ) const { return head->Next = = NULL; } int Is. Full( ) const { return 0; } void Make. Empty( ); };
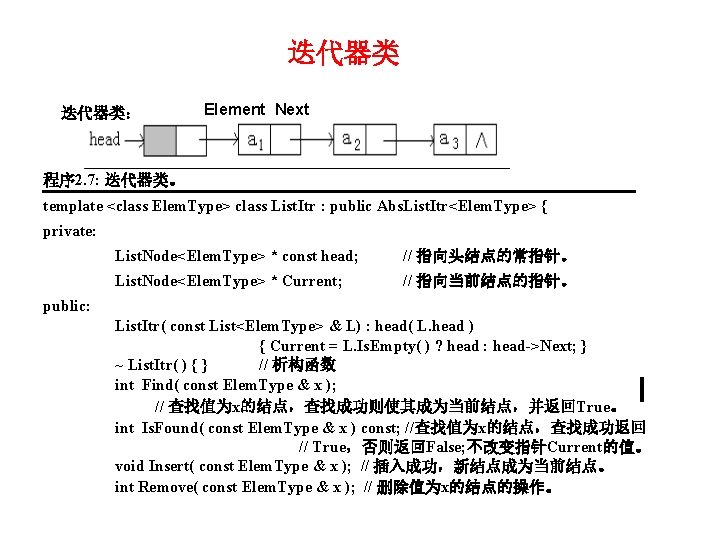
迭代器类: Element Next 程序 2. 7: 迭代器类。 template <class Elem. Type> class List. Itr : public Abs. List. Itr<Elem. Type> { private: List. Node<Elem. Type> * const head; // 指向头结点的常指针。 List. Node<Elem. Type> * Current; // 指向当前结点的指针。 public: List. Itr( const List<Elem. Type> & L) : head( L. head ) { Current = L. Is. Empty( ) ? head : head->Next; } ~ List. Itr( ) { } // 析构函数 int Find( const Elem. Type & x ); // 查找值为x的结点,查找成功则使其成为当前结点,并返回True。 int Is. Found( const Elem. Type & x ) const; //查找值为x的结点,查找成功返回 // True,否则返回False; 不改变指针Current的值。 void Insert( const Elem. Type & x ); // 插入成功,新结点成为当前结点。 int Remove( const Elem. Type & x ); // 删除值为x的结点的操作。
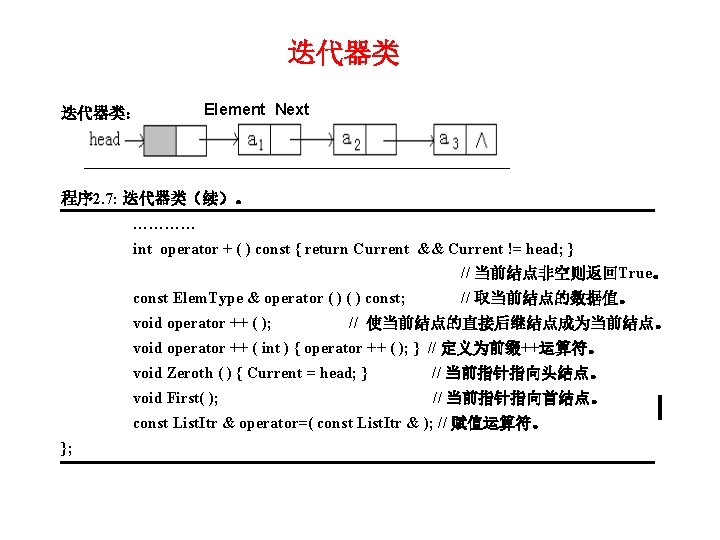
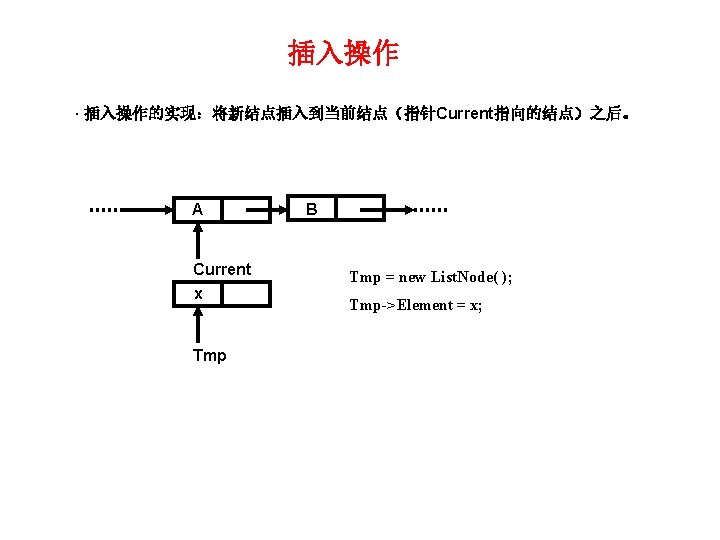
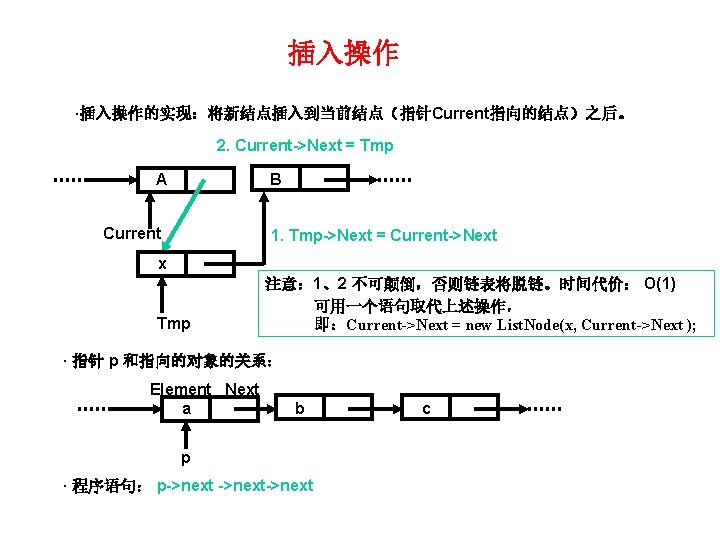
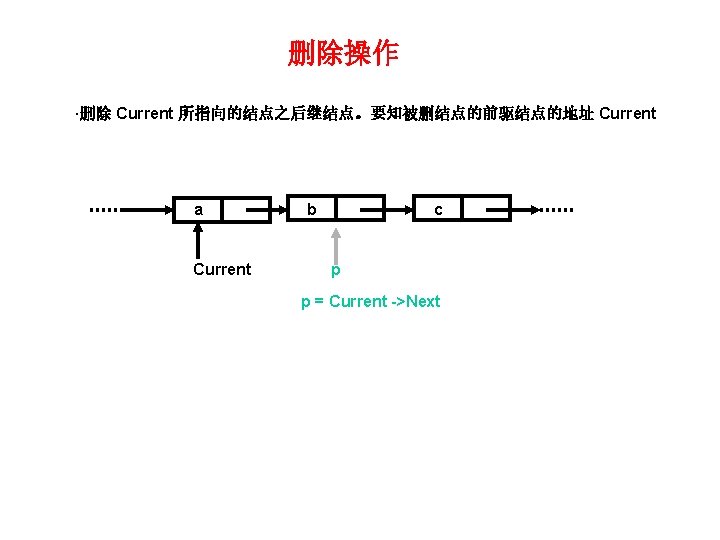
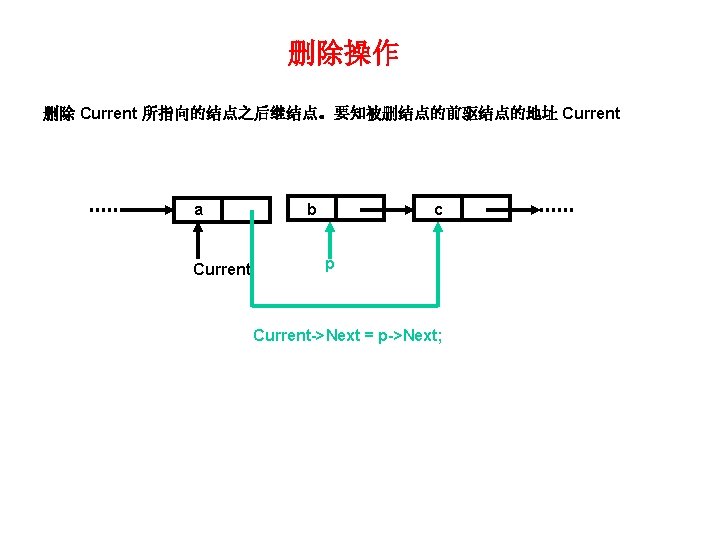
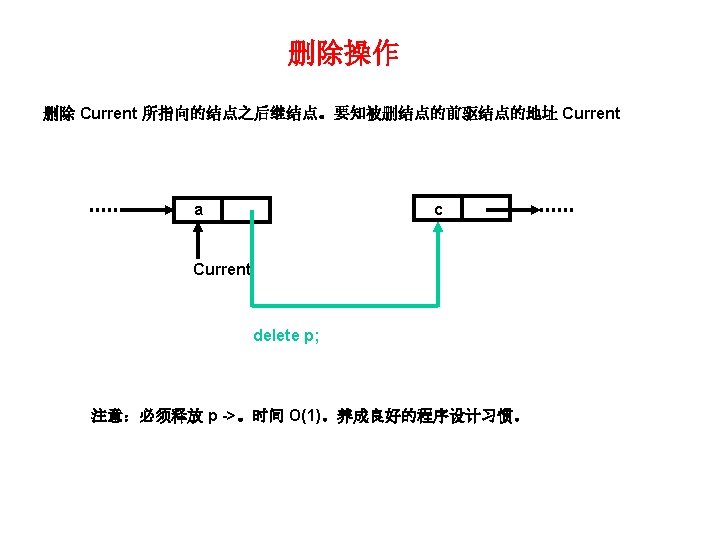
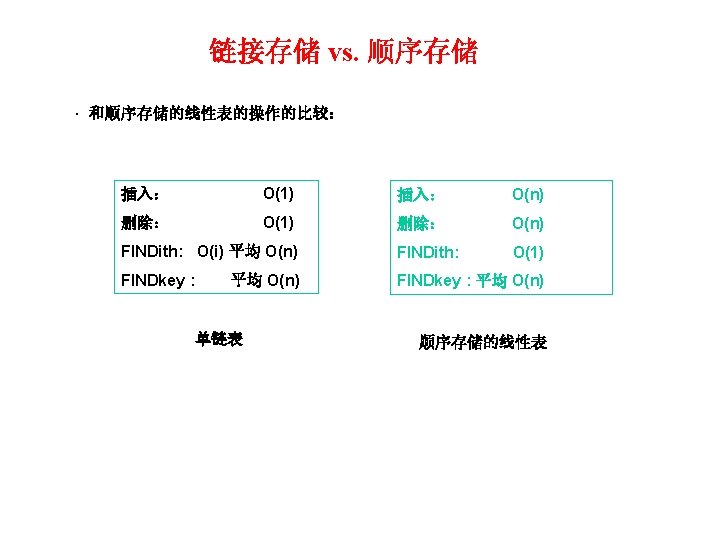
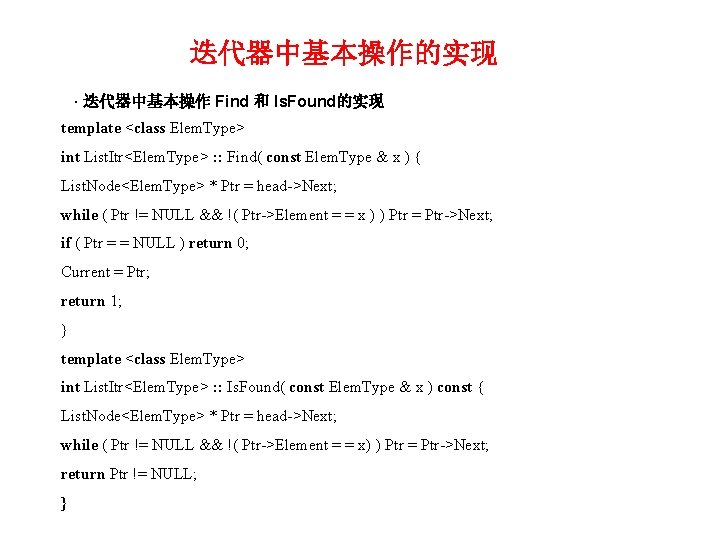
迭代器中基本操作的实现 · 迭代器中基本操作 Find 和 Is. Found的实现 template <class Elem. Type> int List. Itr<Elem. Type> : : Find( const Elem. Type & x ) { List. Node<Elem. Type> * Ptr = head->Next; while ( Ptr != NULL && !( Ptr->Element = = x ) ) Ptr = Ptr->Next; if ( Ptr = = NULL ) return 0; Current = Ptr; return 1; } template <class Elem. Type> int List. Itr<Elem. Type> : : Is. Found( const Elem. Type & x ) const { List. Node<Elem. Type> * Ptr = head->Next; while ( Ptr != NULL && !( Ptr->Element = = x) ) Ptr = Ptr->Next; return Ptr != NULL; }
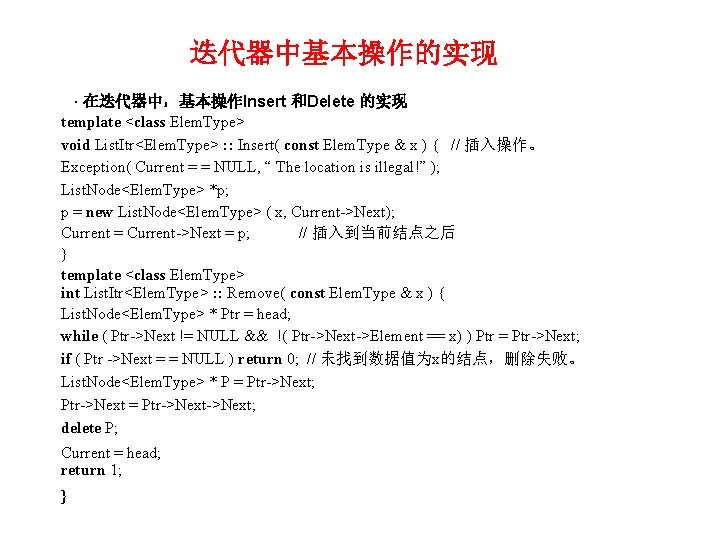
迭代器中基本操作的实现 · 在迭代器中,基本操作Insert 和Delete 的实现 template <class Elem. Type> void List. Itr<Elem. Type> : : Insert( const Elem. Type & x ) { // 插入操作。 Exception( Current = = NULL, “ The location is illegal!” ); List. Node<Elem. Type> *p; p = new List. Node<Elem. Type> ( x, Current->Next); Current = Current->Next = p; // 插入到当前结点之后 } template <class Elem. Type> int List. Itr<Elem. Type> : : Remove( const Elem. Type & x ) { List. Node<Elem. Type> * Ptr = head; while ( Ptr->Next != NULL && !( Ptr->Next->Element == x) ) Ptr = Ptr->Next; if ( Ptr ->Next = = NULL ) return 0; // 未找到数据值为x的结点,删除失败。 List. Node<Elem. Type> * P = Ptr->Next; Ptr->Next = Ptr->Next; delete P; Current = head; return 1; }
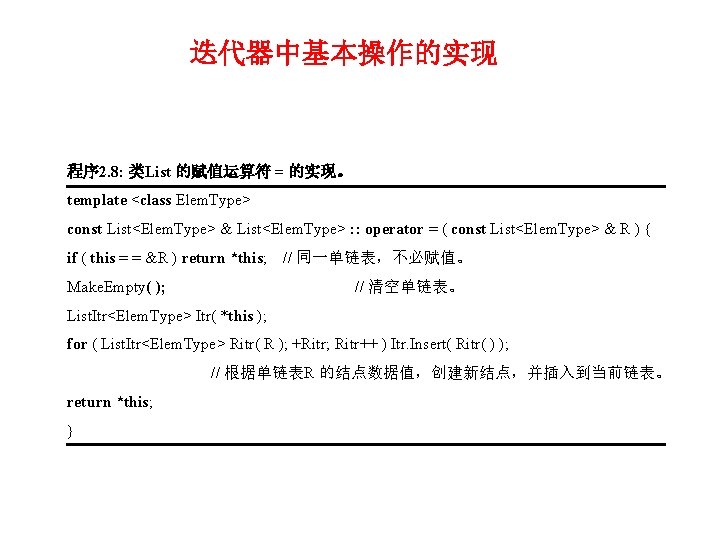
迭代器中基本操作的实现 程序 2. 8: 类List 的赋值运算符 = 的实现。 template <class Elem. Type> const List<Elem. Type> & List<Elem. Type> : : operator = ( const List<Elem. Type> & R ) { if ( this = = &R ) return *this; // 同一单链表,不必赋值。 Make. Empty( ); // 清空单链表。 List. Itr<Elem. Type> Itr( *this ); for ( List. Itr<Elem. Type> Ritr( R ); +Ritr; Ritr++ ) Itr. Insert( Ritr( ) ); // 根据单链表R 的结点数据值,创建新结点,并插入到当前链表。 return *this; }
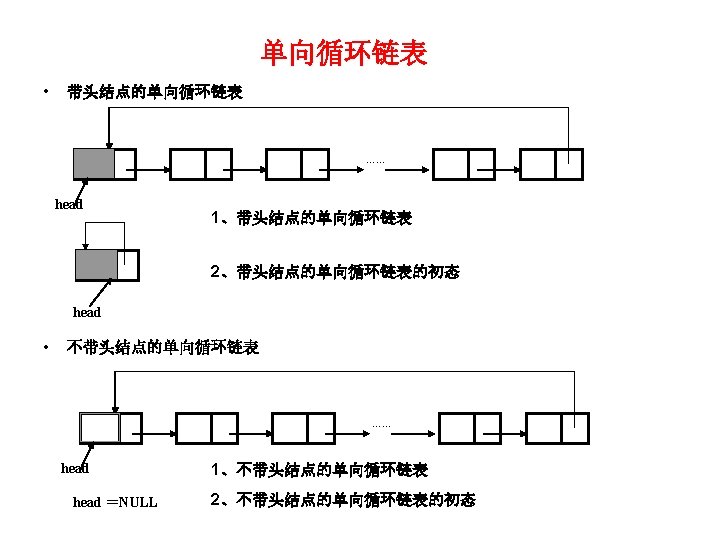
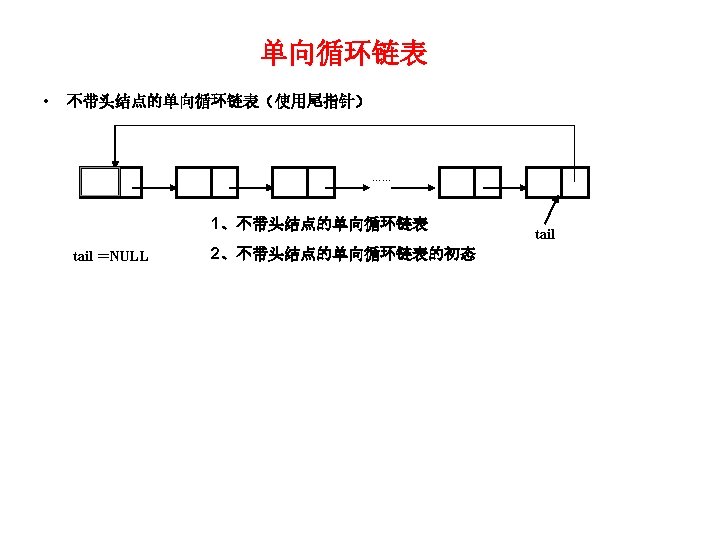
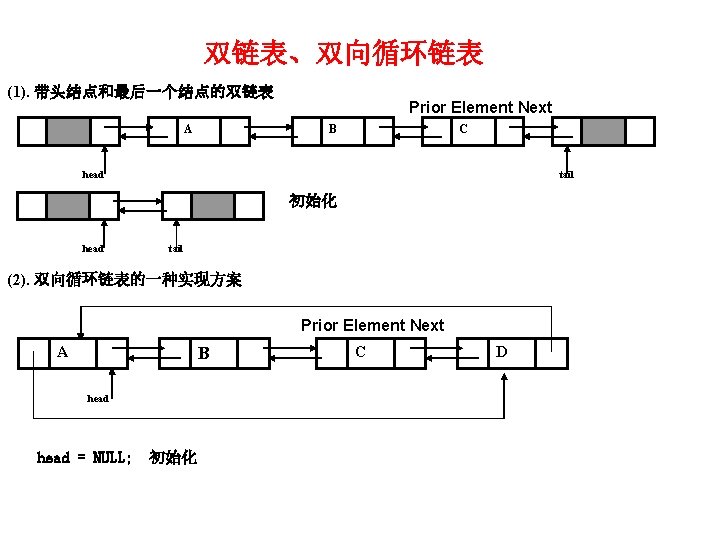
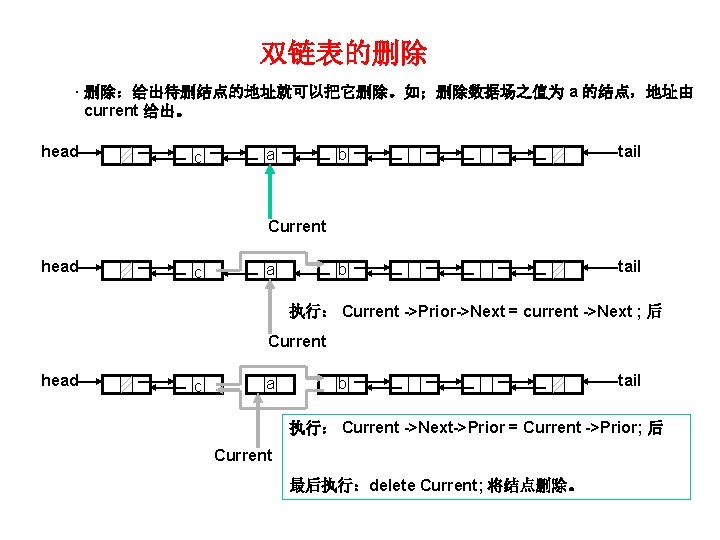
双链表的删除 · 删除:给出待删结点的地址就可以把它删除。如;删除数据场之值为 a 的结点,地址由 current 给出。 head c a b tail Current head c a 执行: Current ->Prior->Next = current ->Next ; 后 Current head c a b tail 执行: Current ->Next->Prior = Current ->Prior; 后 Current 最后执行:delete Current; 将结点删除。
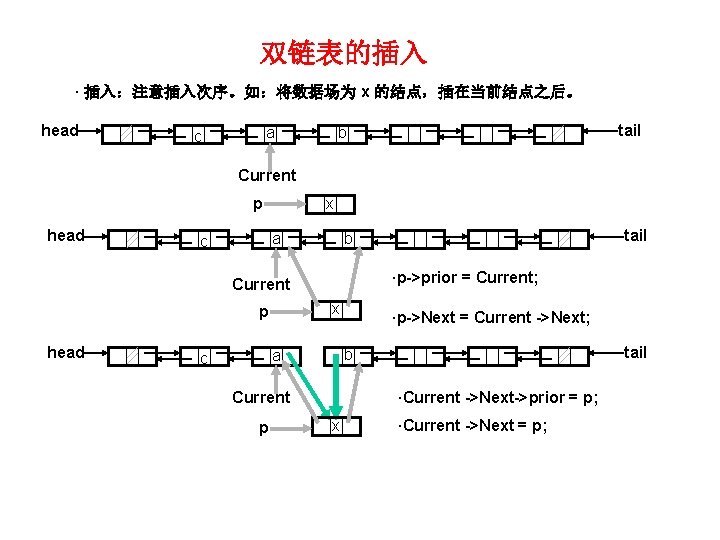
双链表的插入 · 插入:注意插入次序。如:将数据场为 x 的结点,插在当前结点之后。 head a c tail b Current p head x a c ·p->prior = Current; Current x p head Current p ·p->Next = Current ->Next; tail b a c tail b ·Current ->Next->prior = p; x ·Current ->Next = p;
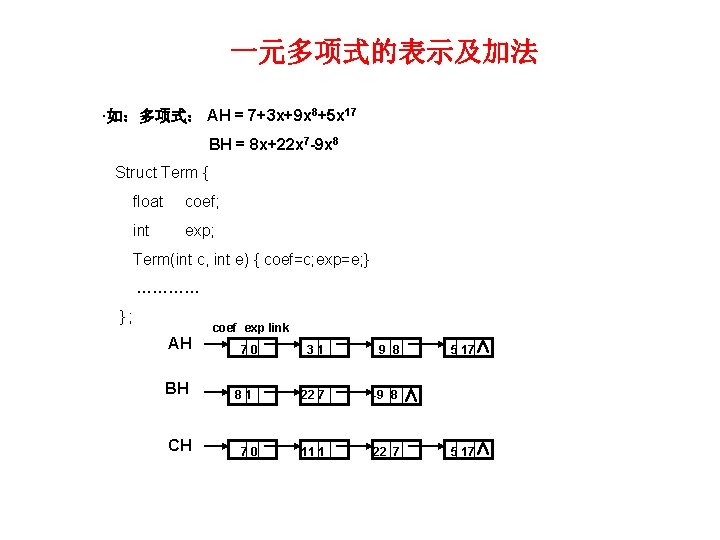
一元多项式的表示及加法 ·如:多项式: AH = 7+3 x+9 x 8+5 x 17 BH = 8 x+22 x 7 -9 x 8 Struct Term { float coef; int exp; Term(int c, int e) { coef=c; exp=e; } ………… }; coef exp link AH BH CH 70 81 70 31 9 8 22 7 -9 8 11 1 22 7 5 17 ∧ ∧ 5 17 ∧
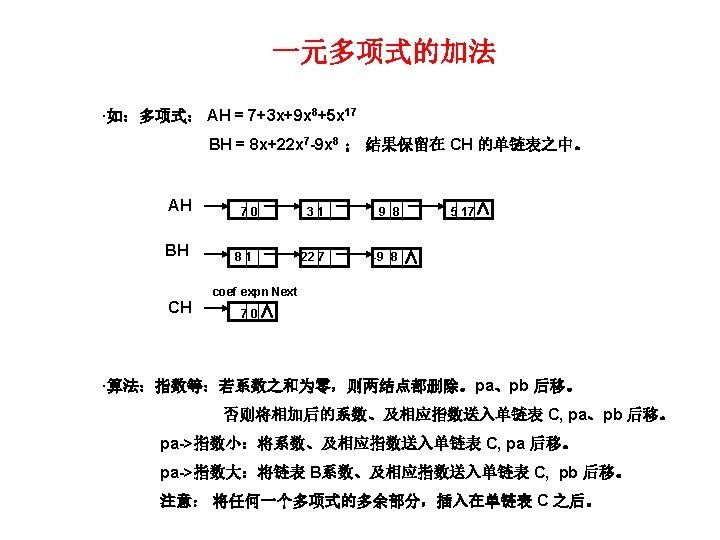
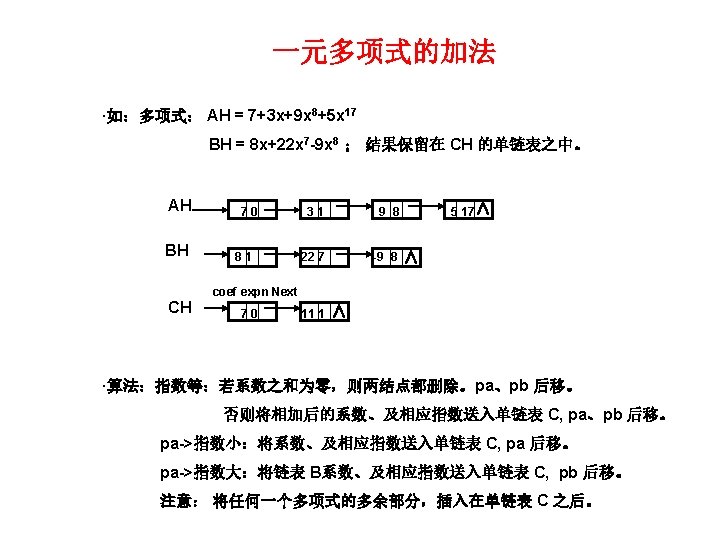
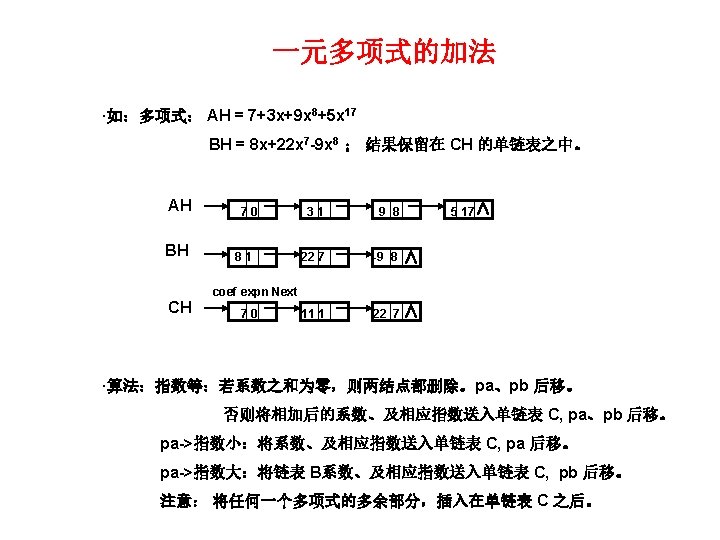
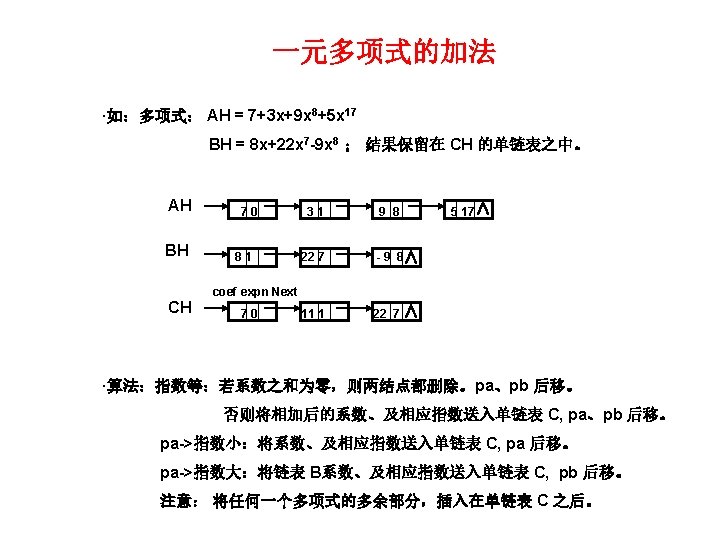
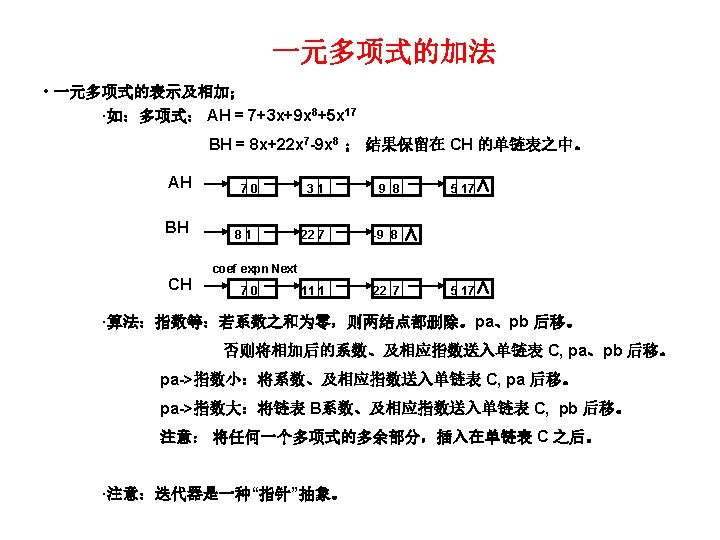
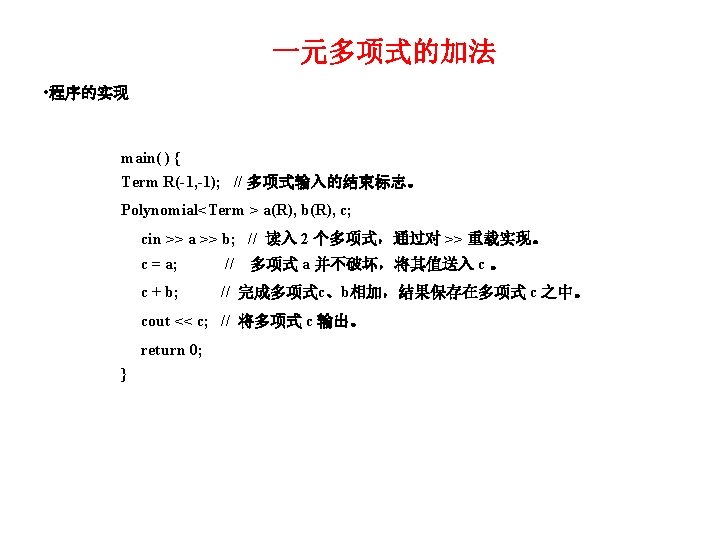
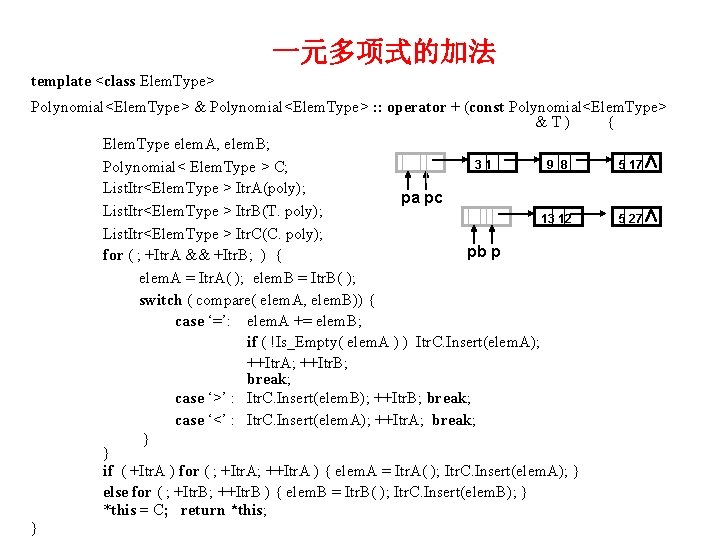
一元多项式的加法 template <class Elem. Type> Polynomial<Elem. Type> & Polynomial<Elem. Type> : : operator + (const Polynomial<Elem. Type> &T) { Elem. Type elem. A, elem. B; 31 9 8 5 17 ∧ Polynomial< Elem. Type > C; List. Itr<Elem. Type > Itr. A(poly); pa pc List. Itr<Elem. Type > Itr. B(T. poly); 13 12 5 27 ∧ List. Itr<Elem. Type > Itr. C(C. poly); pb p for ( ; +Itr. A && +Itr. B; ) { elem. A = Itr. A( ); elem. B = Itr. B( ); switch ( compare( elem. A, elem. B)) { case ‘=’: elem. A += elem. B; if ( !Is_Empty( elem. A ) ) Itr. C. Insert(elem. A); ++Itr. A; ++Itr. B; break; case ‘>’ : Itr. C. Insert(elem. B); ++Itr. B; break; case ‘<’ : Itr. C. Insert(elem. A); ++Itr. A; break; } } if ( +Itr. A ) for ( ; +Itr. A; ++Itr. A ) { elem. A = Itr. A( ); Itr. C. Insert(elem. A); } else for ( ; +Itr. B; ++Itr. B ) { elem. B = Itr. B( ); Itr. C. Insert(elem. B); } *this = C; return *this; }
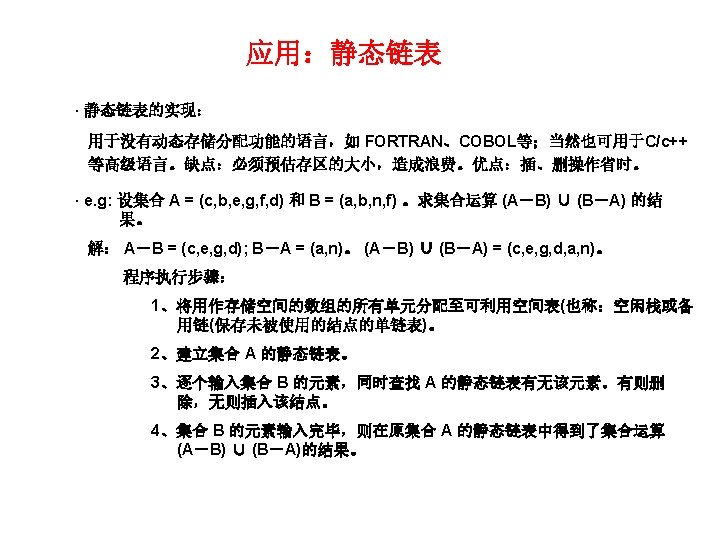
![应用静态链表 1将用作存储空间的数组的所有单元分配至空闲栈或备用链 data cur space0 1 space1 2 space2 space3 3 space4 4 5 应用:静态链表 1、将用作存储空间的数组的所有单元分配至空闲栈或备用链。 data cur space[0] 1 space[1] 2 space[2] space[3] 3 space[4] 4 5](https://slidetodoc.com/presentation_image_h2/d6050df241ccc663b15ba39ffda42a93/image-47.jpg)
应用:静态链表 1、将用作存储空间的数组的所有单元分配至空闲栈或备用链。 data cur space[0] 1 space[1] 2 space[2] space[3] 3 space[4] 4 5 space[5] 6 space[6] space[7] 7 space[8] 8 9 space[9] 10 space[10] space[11] 11 -1
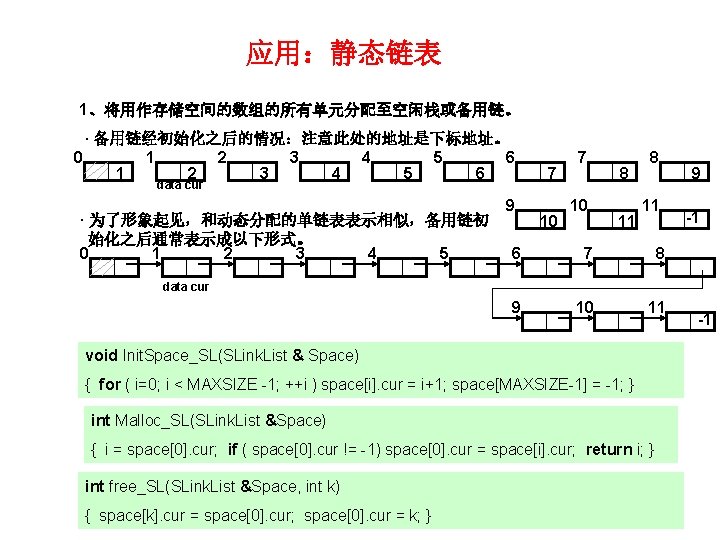
应用:静态链表 1、将用作存储空间的数组的所有单元分配至空闲栈或备用链。 · 备用链经初始化之后的情况:注意此处的地址是下标地址。 0 1 2 3 4 5 6 data cur · 为了形象起见,和动态分配的单链表表示相似,备用链初 始化之后通常表示成以下形式。 0 1 2 3 4 5 9 7 10 8 11 6 7 8 9 10 11 9 -1 data cur void Init. Space_SL(SLink. List & Space) { for ( i=0; i < MAXSIZE -1; ++i ) space[i]. cur = i+1; space[MAXSIZE-1] = -1; } int Malloc_SL(SLink. List &Space) { i = space[0]. cur; if ( space[0]. cur != -1) space[0]. cur = space[i]. cur; return i; } int free_SL(SLink. List &Space, int k) { space[k]. cur = space[0]. cur; space[0]. cur = k; } -1
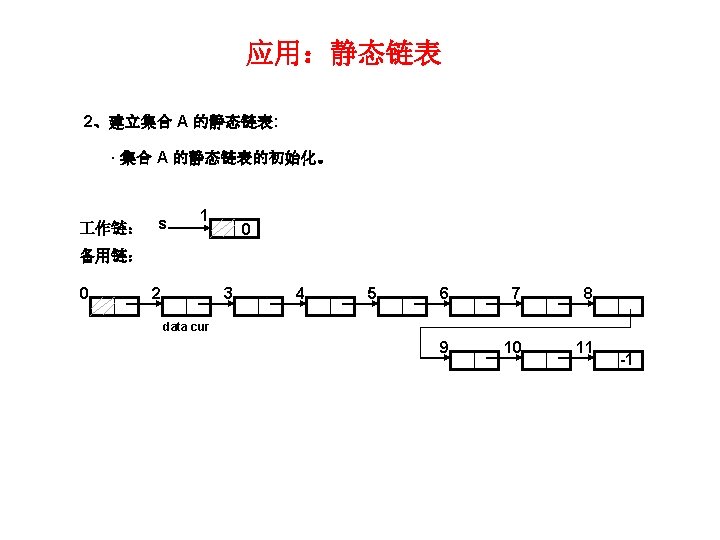
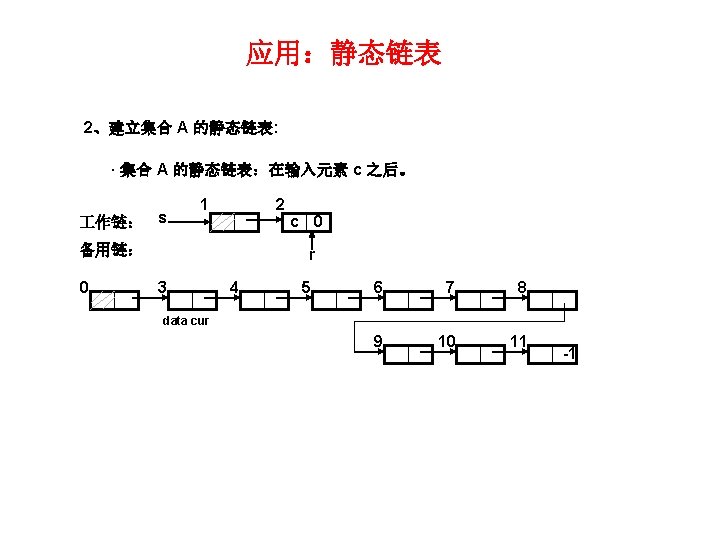
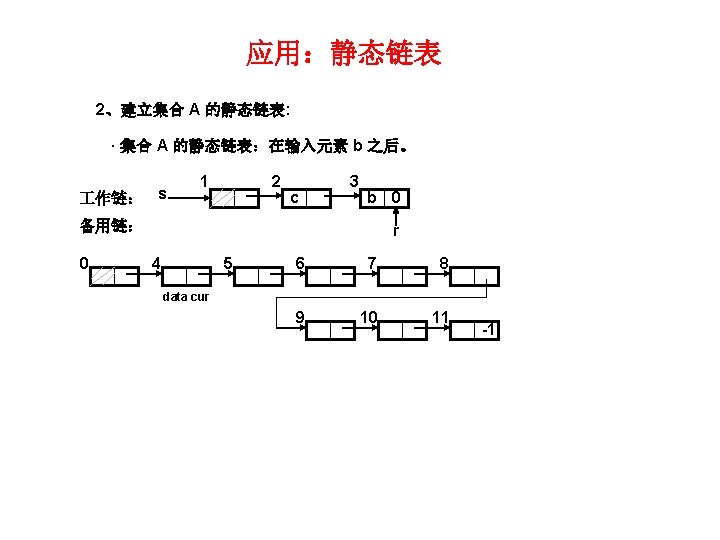
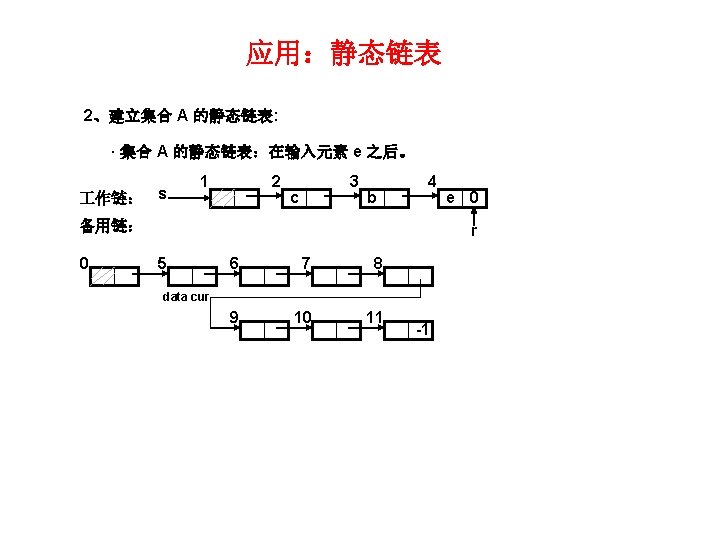
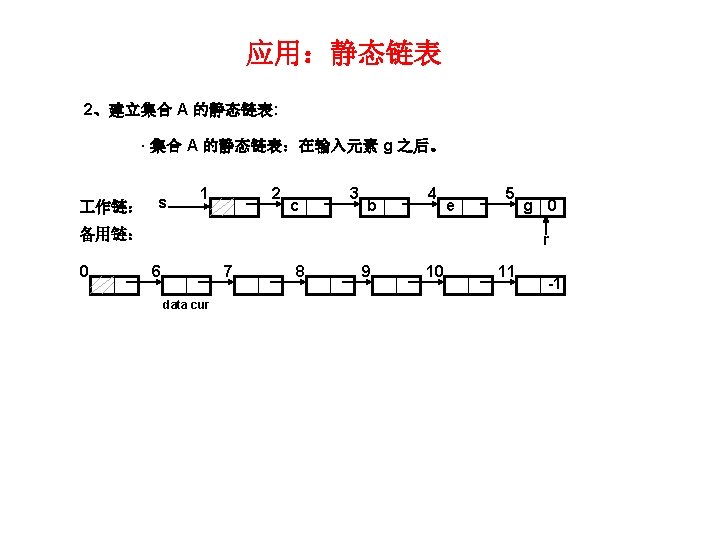
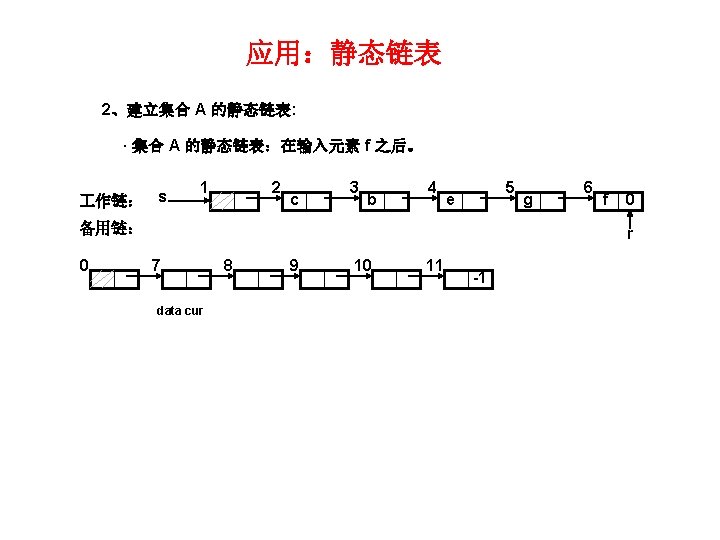
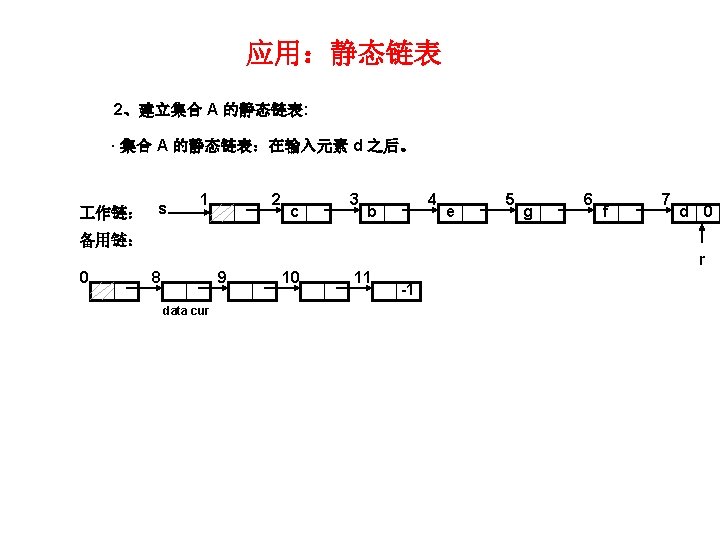
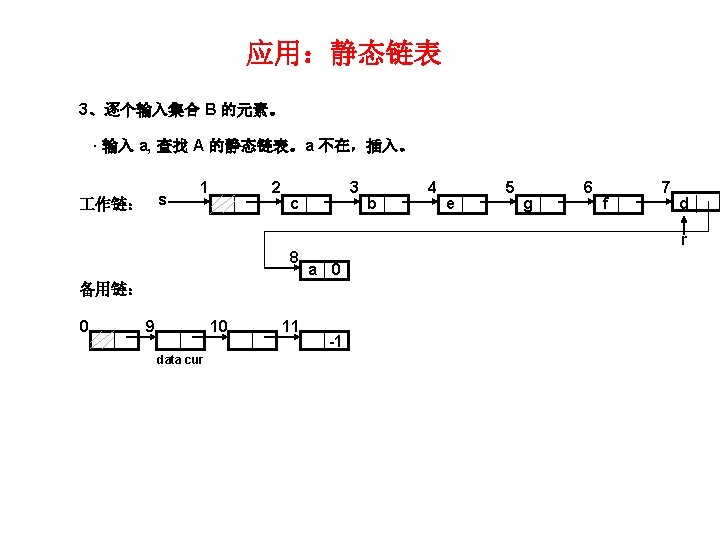
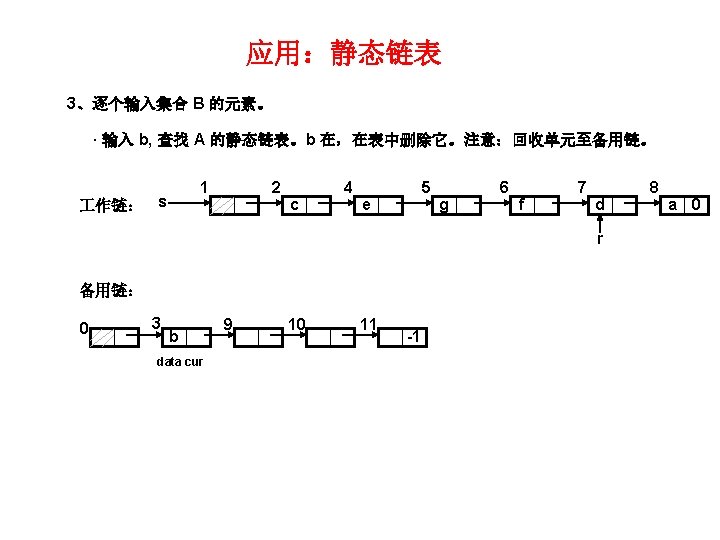
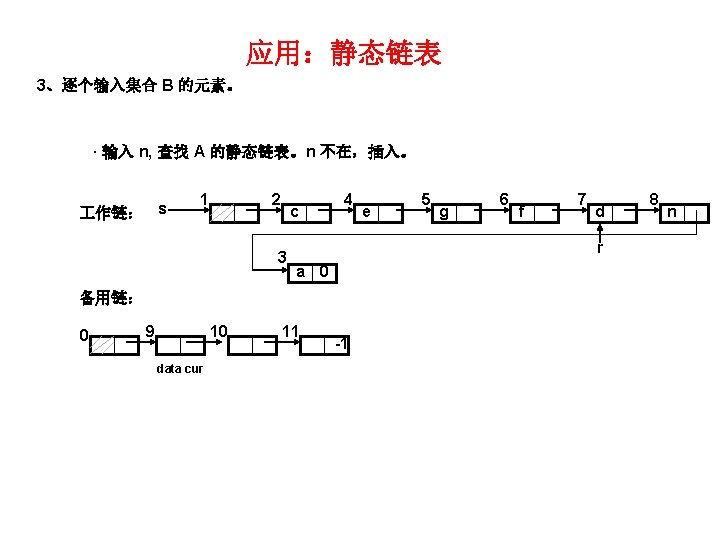
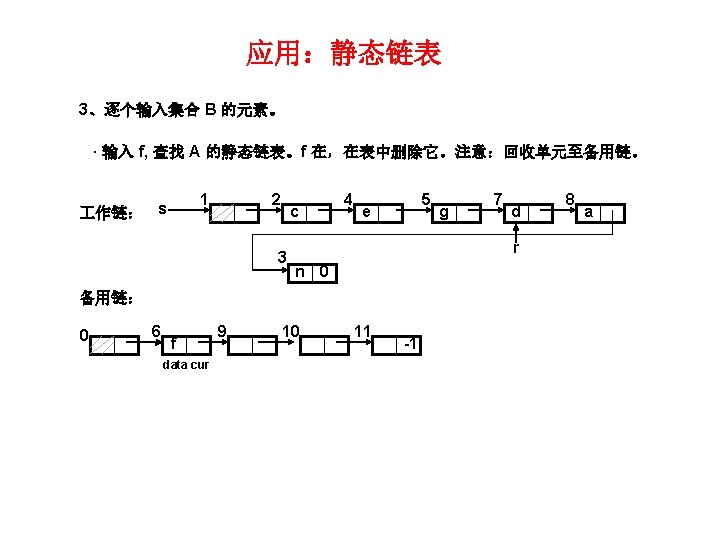
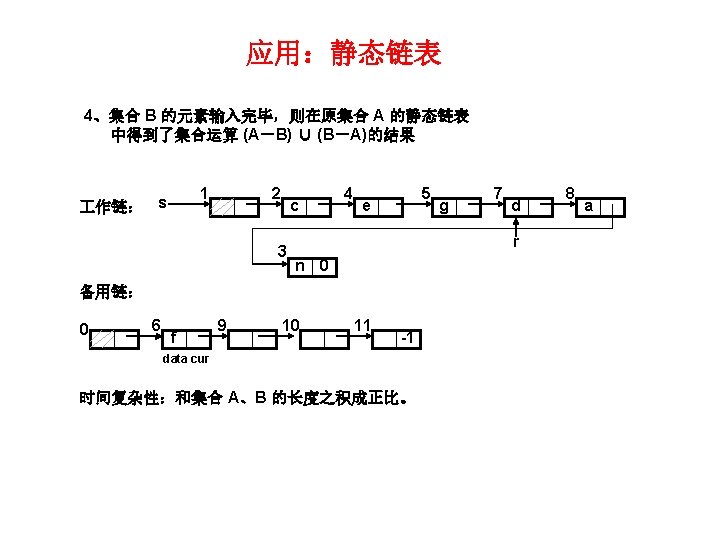
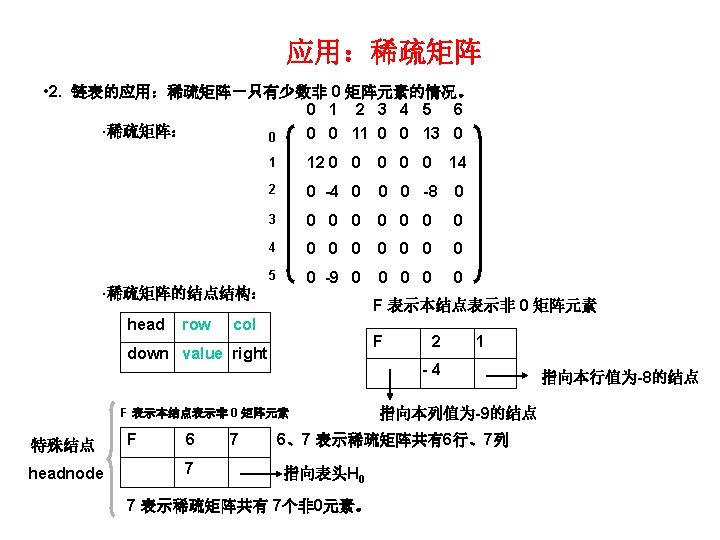
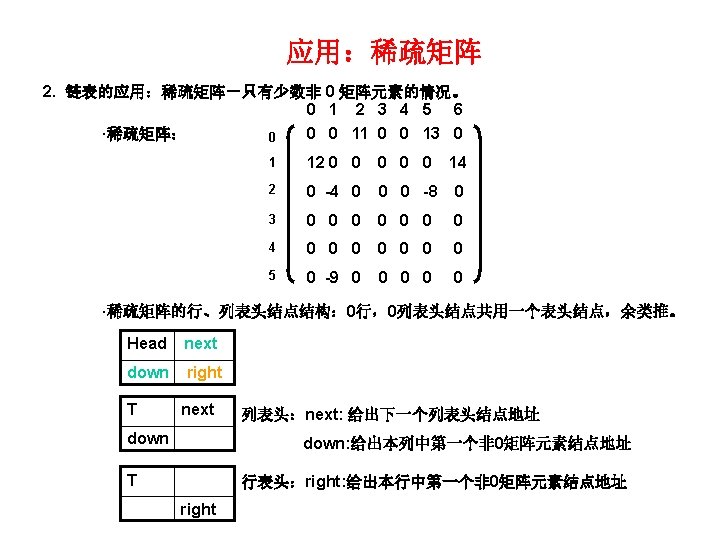
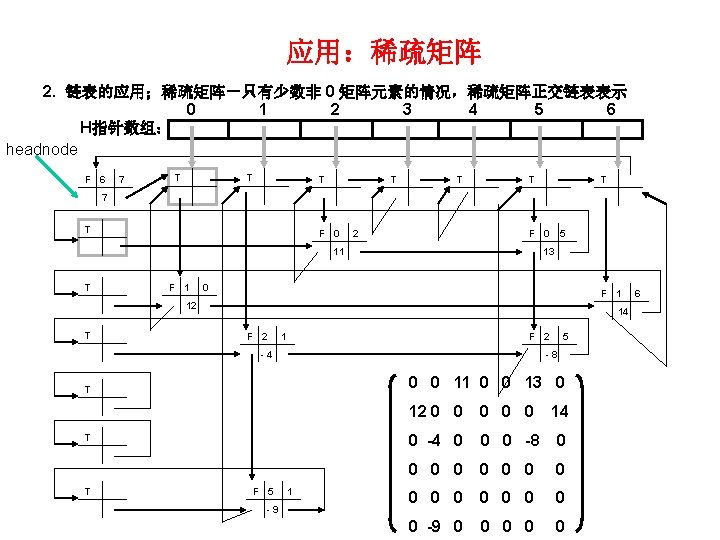
Art critique sentence starters
R90d elem
Elem
Daniell elem
Karn partridge algorithm
Ocaml seq
Seq gan
Illumina
Index.php?seq=
Tuxedo suite rna seq
Slide-seq
Seq monk
Seq artinya
Slide-seq
Per base sequence quality
Bagaimana cara membuka menu format pada microsoft publisher
How is function overloading different from template class
Class list log observation
Bin tree
Template class t t func(t a)
Todaysclass
Package mypackage; class first { /* class body */ }
Abstract class vs concrete class
Lower boundary of the modal class
Class i vs class ii mhc
Abstract concrete class relationship
Fdt
Sd and sdelta
Discriminative stimulus psychology definition
Therapeutic class and pharmacologic class
Class maths student student1 class student string name
Unordered stem and leaf plot
In greenfoot, you can cast an actor class to a world class?
Static class loading and dynamic class loading
Esd class 0
Static uml diagrams
Class 2 class 3
Public class test subject extends test class
Package mypackage class first class body
Class third class
C++ class implementation
Component class has composite class as collaborator
Field type is an abstract class
Match the correct type of fire to the appropriate class
Is it possible to create an object of an empty class type
Hunt's algorithm
A class is an example of a structured data type.
Is hyper v type 1 or type 2
Type 1 and type 2 muscle fibres
Type 1 error vs type 2 error example
Type 2 vs type 1 error
Rock cycle
Type a and type b personality theory
Sublimation defence mechanism
Myotonic dystrophy.
Null type and deflection type instruments
Clause type 0
Difference between type 1 and type 2 error
Hypothesis testing examples
Type checking in compiler design
A and b blood type offspring
Type 1 vs type 2 fibers
Hit type 1 vs type 2
Types of moulds