Teach Scheme Reach Java Adelphi University Friday morning
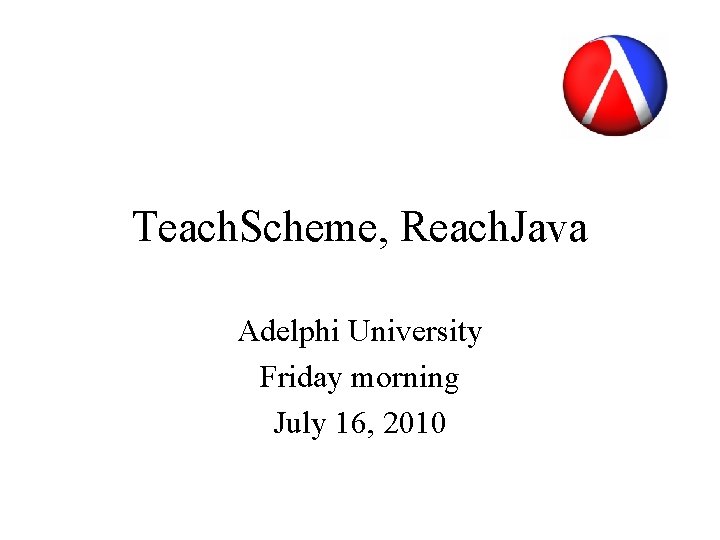
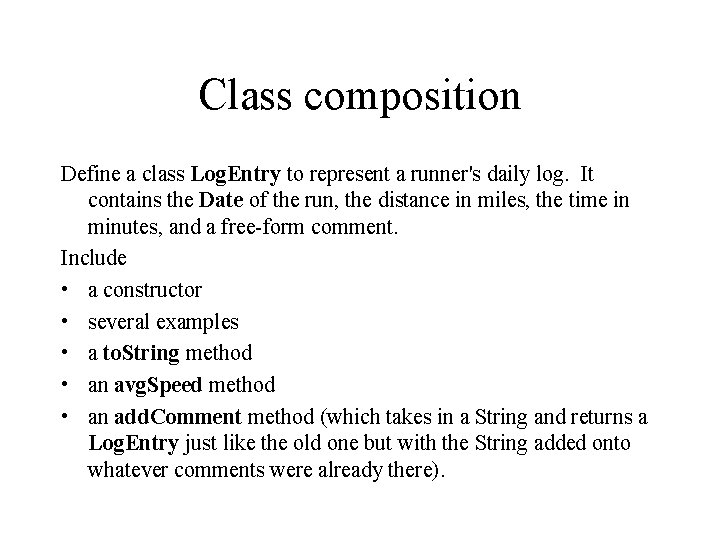
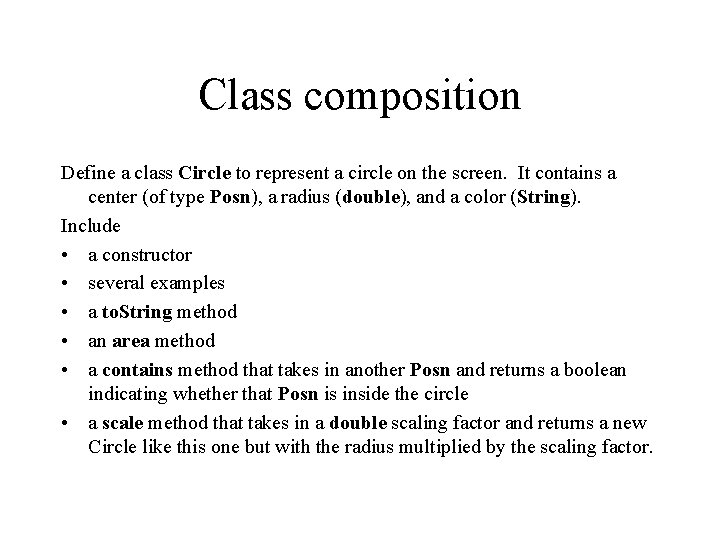
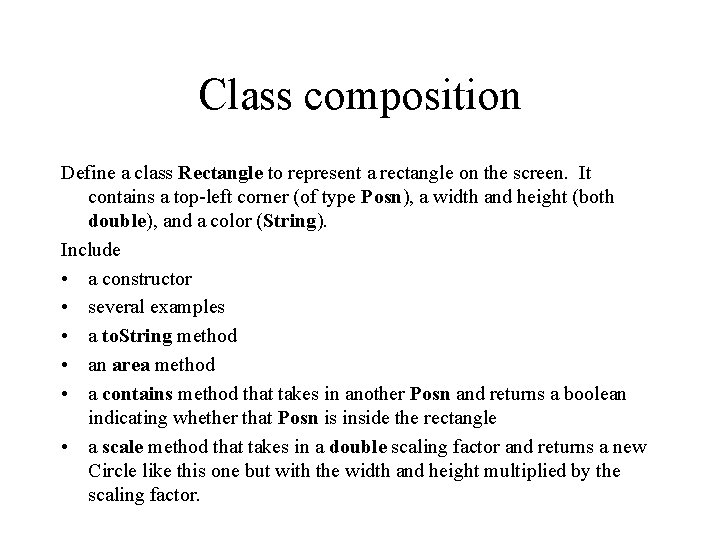
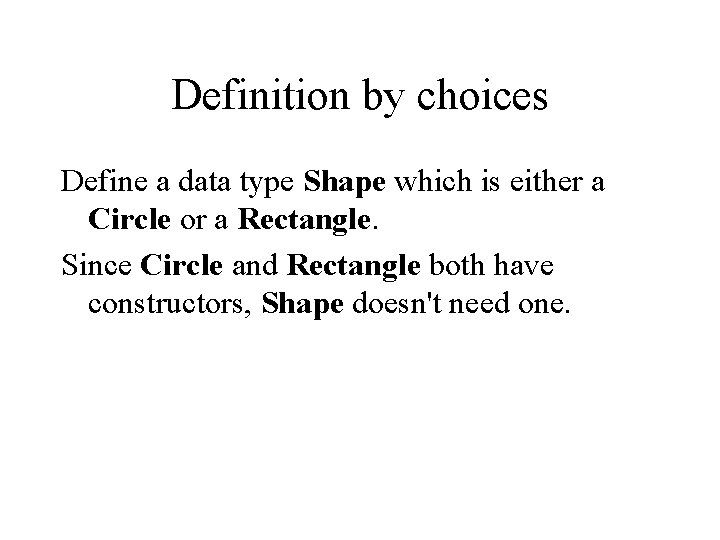
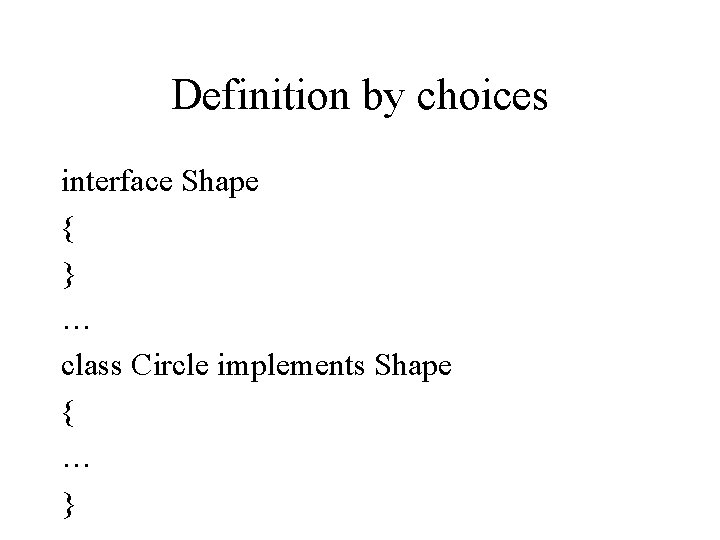
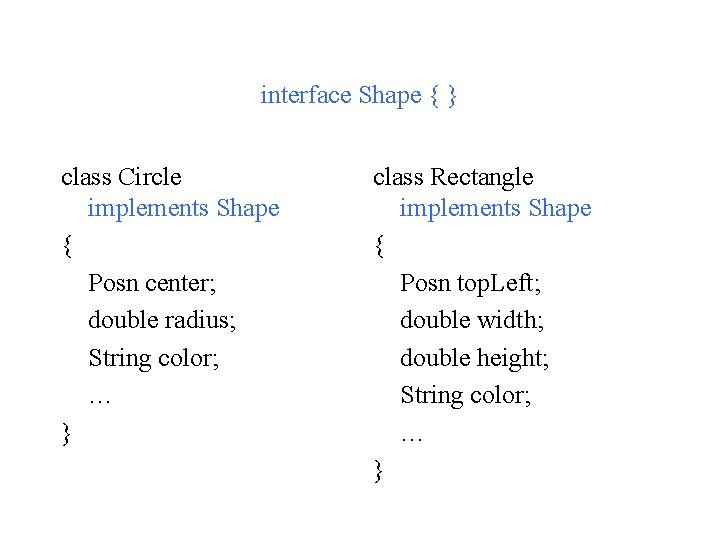
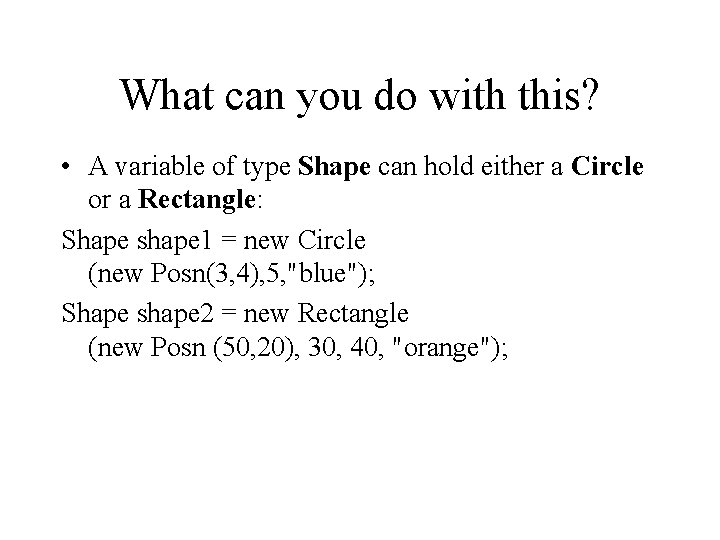
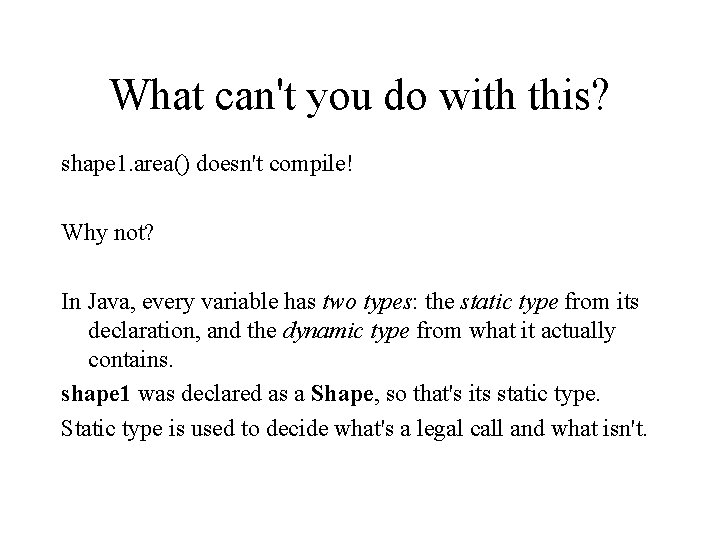
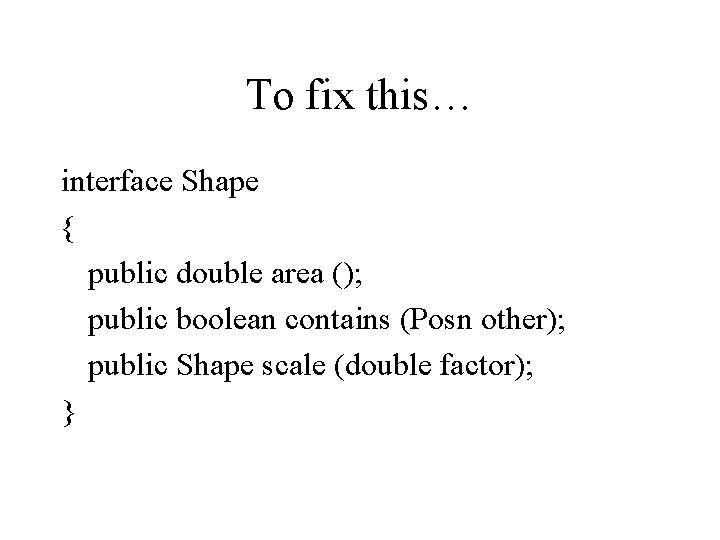
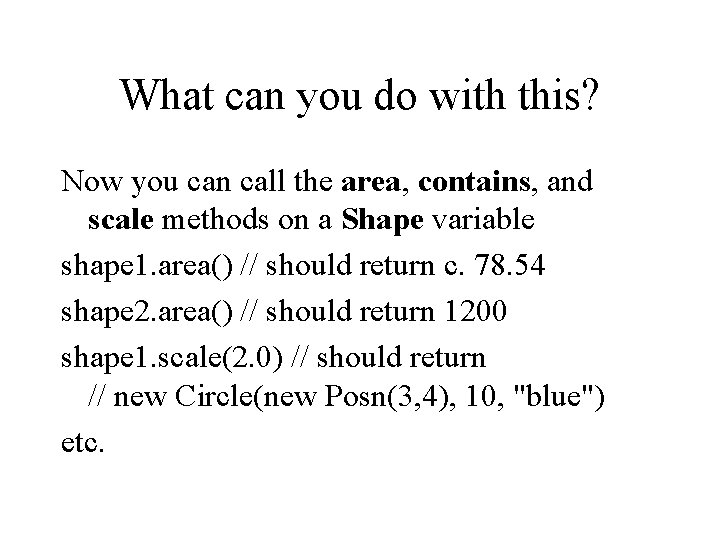
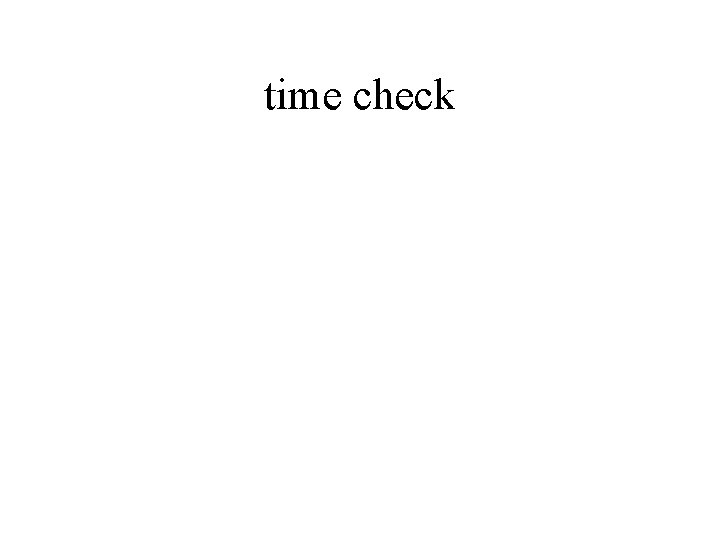
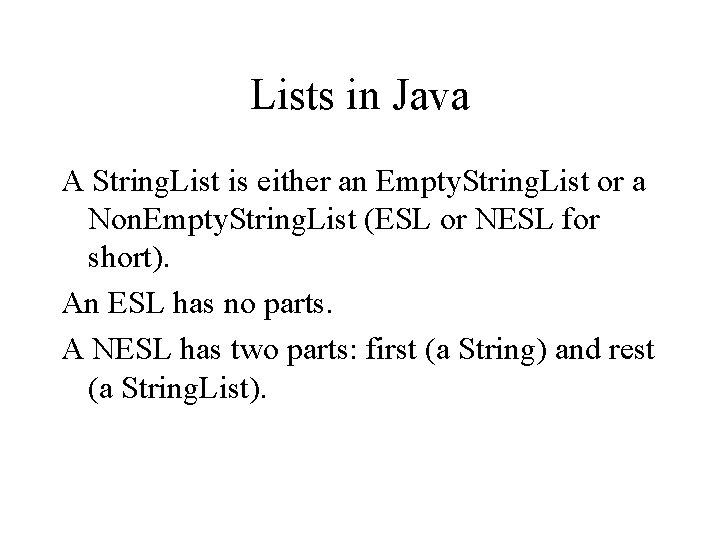
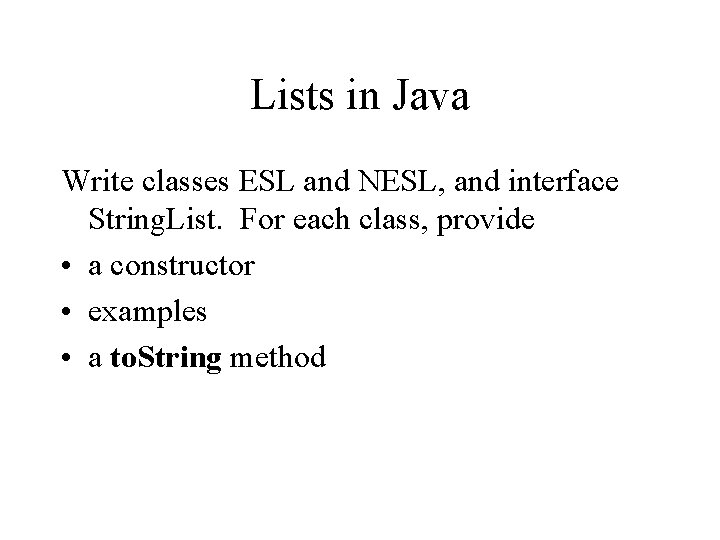
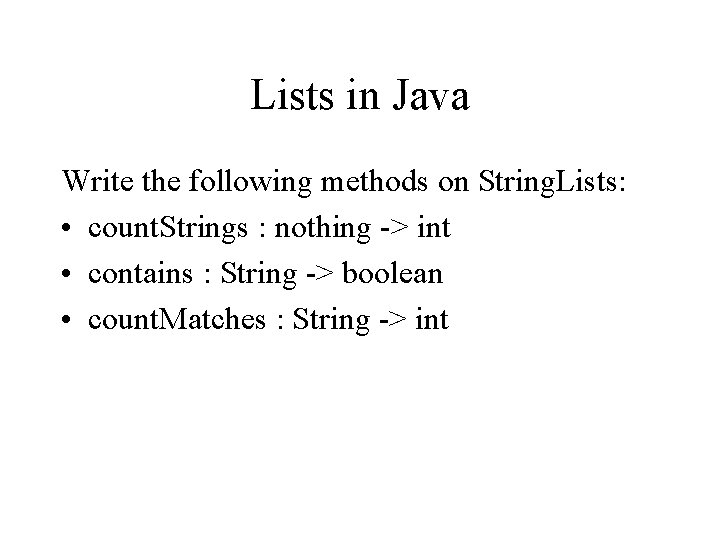
![Recall add-up function (define (add-up nums) (cond [(empty? nums) 0] [(cons? nums) (+ (first Recall add-up function (define (add-up nums) (cond [(empty? nums) 0] [(cons? nums) (+ (first](https://slidetodoc.com/presentation_image/1ac9f442f43961e8cc4c89e300ba22f2/image-16.jpg)
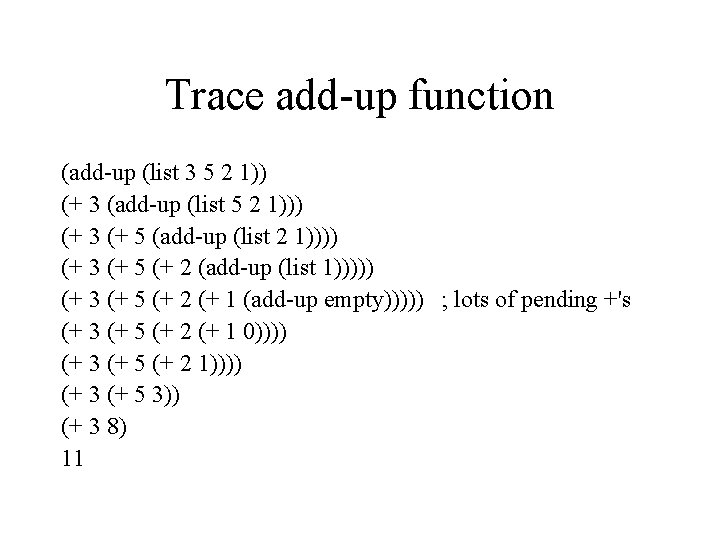
![Another approach (define (add-up-accum nums so-far) (cond [(empty? nums) so-far] [(cons? nums) (add-up-accum (rest Another approach (define (add-up-accum nums so-far) (cond [(empty? nums) so-far] [(cons? nums) (add-up-accum (rest](https://slidetodoc.com/presentation_image/1ac9f442f43961e8cc4c89e300ba22f2/image-18.jpg)
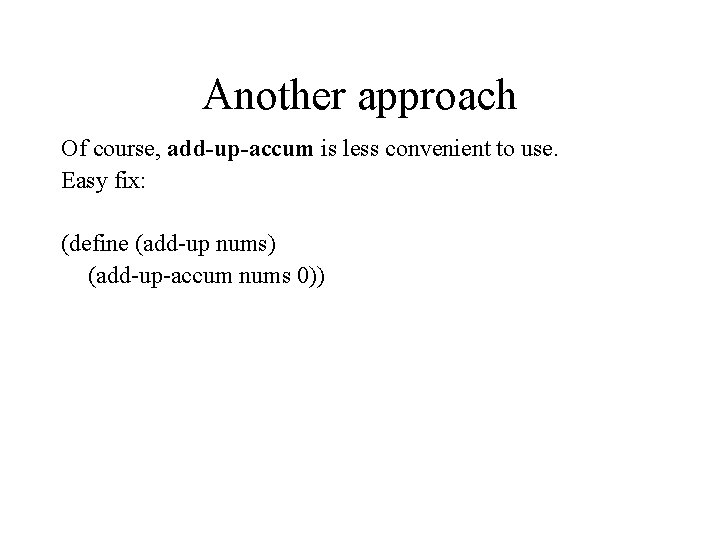
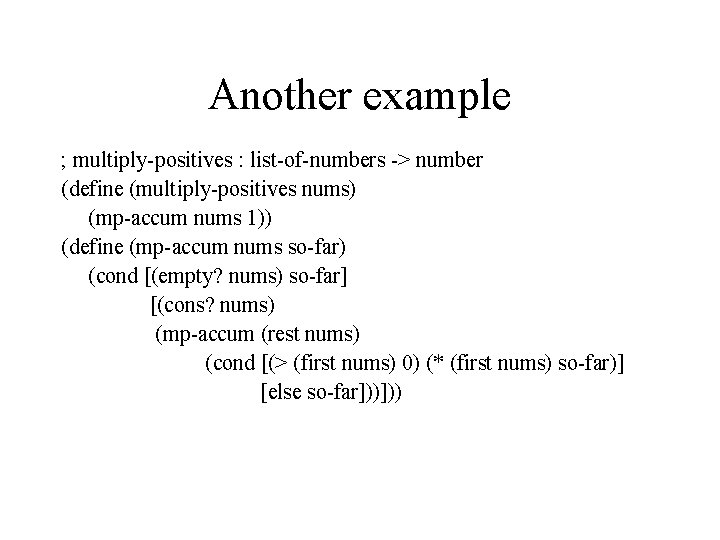
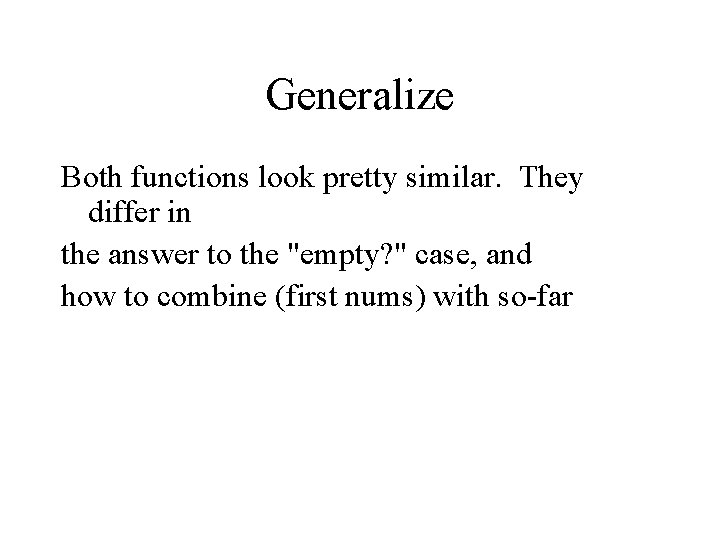
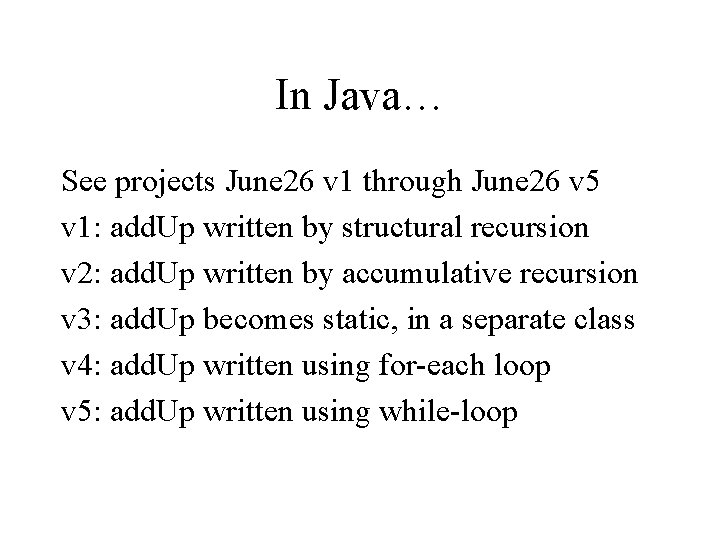
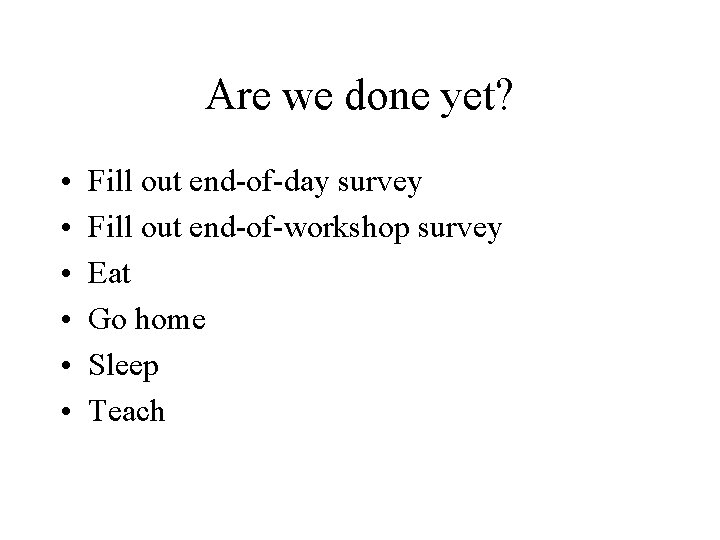
- Slides: 23
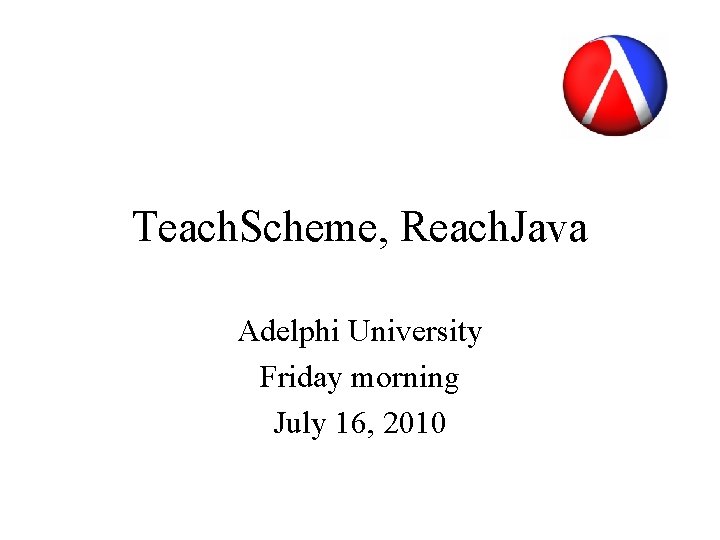
Teach. Scheme, Reach. Java Adelphi University Friday morning July 16, 2010
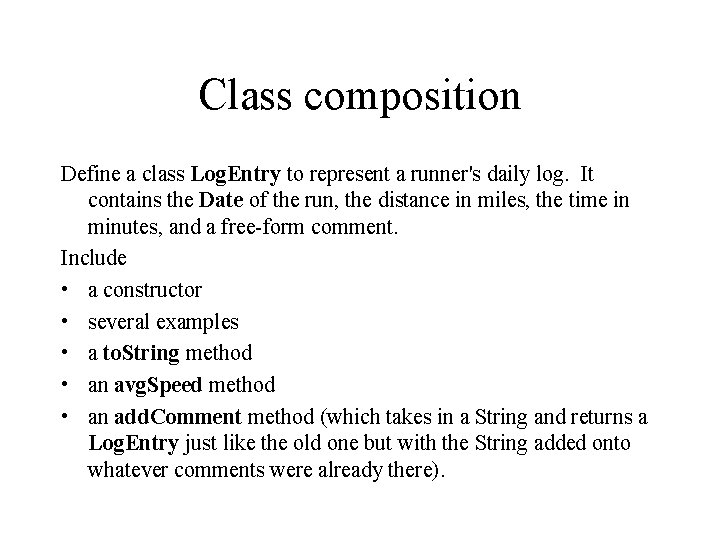
Class composition Define a class Log. Entry to represent a runner's daily log. It contains the Date of the run, the distance in miles, the time in minutes, and a free-form comment. Include • a constructor • several examples • a to. String method • an avg. Speed method • an add. Comment method (which takes in a String and returns a Log. Entry just like the old one but with the String added onto whatever comments were already there).
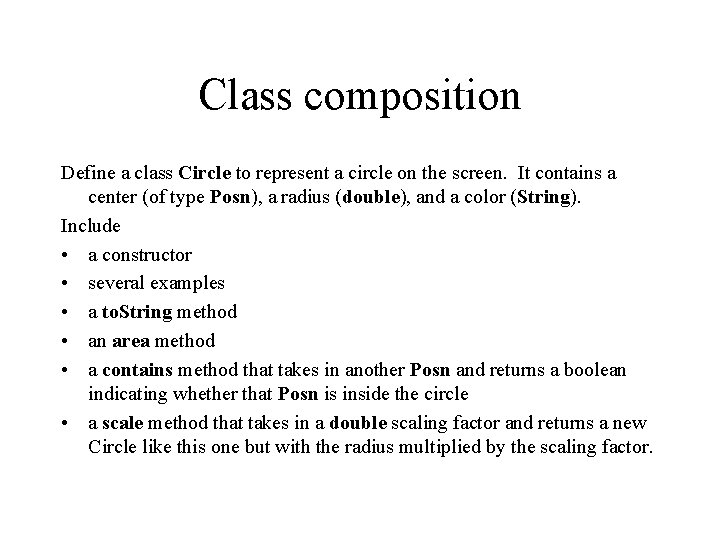
Class composition Define a class Circle to represent a circle on the screen. It contains a center (of type Posn), a radius (double), and a color (String). Include • a constructor • several examples • a to. String method • an area method • a contains method that takes in another Posn and returns a boolean indicating whether that Posn is inside the circle • a scale method that takes in a double scaling factor and returns a new Circle like this one but with the radius multiplied by the scaling factor.
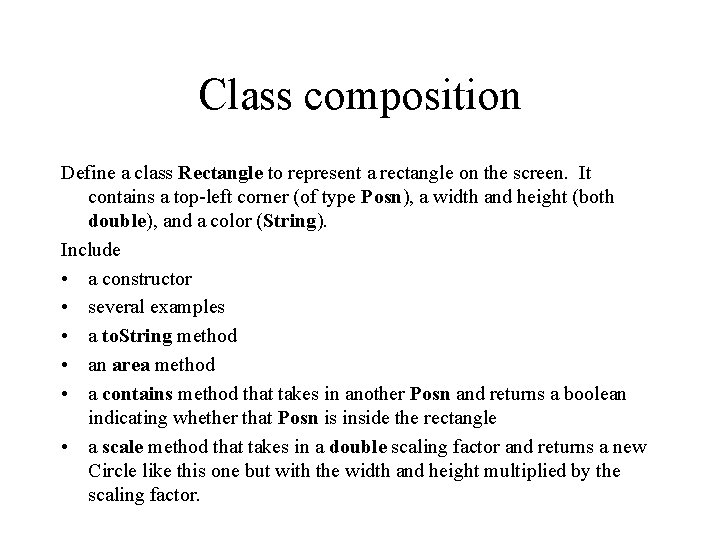
Class composition Define a class Rectangle to represent a rectangle on the screen. It contains a top-left corner (of type Posn), a width and height (both double), and a color (String). Include • a constructor • several examples • a to. String method • an area method • a contains method that takes in another Posn and returns a boolean indicating whether that Posn is inside the rectangle • a scale method that takes in a double scaling factor and returns a new Circle like this one but with the width and height multiplied by the scaling factor.
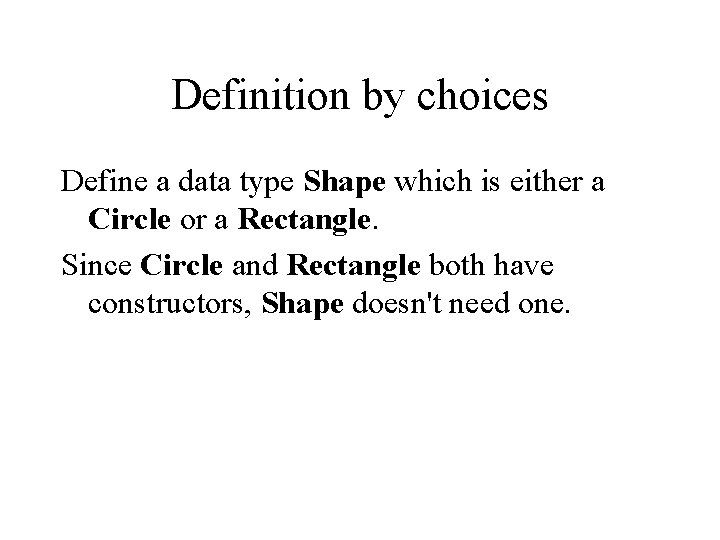
Definition by choices Define a data type Shape which is either a Circle or a Rectangle. Since Circle and Rectangle both have constructors, Shape doesn't need one.
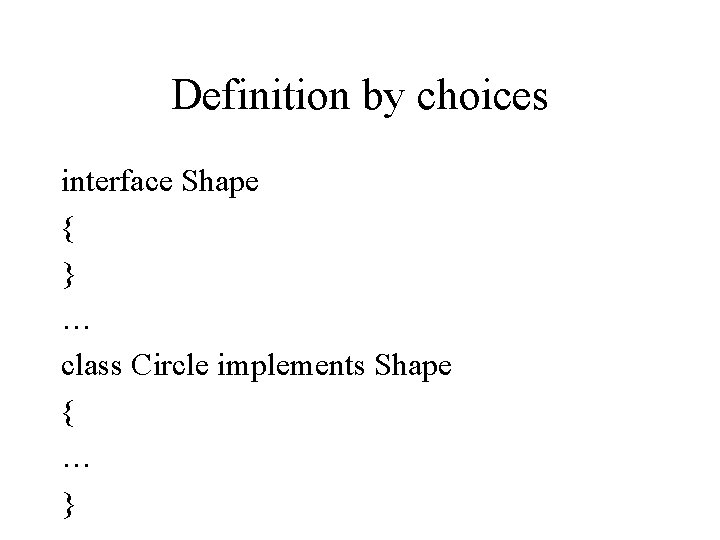
Definition by choices interface Shape { } … class Circle implements Shape { … }
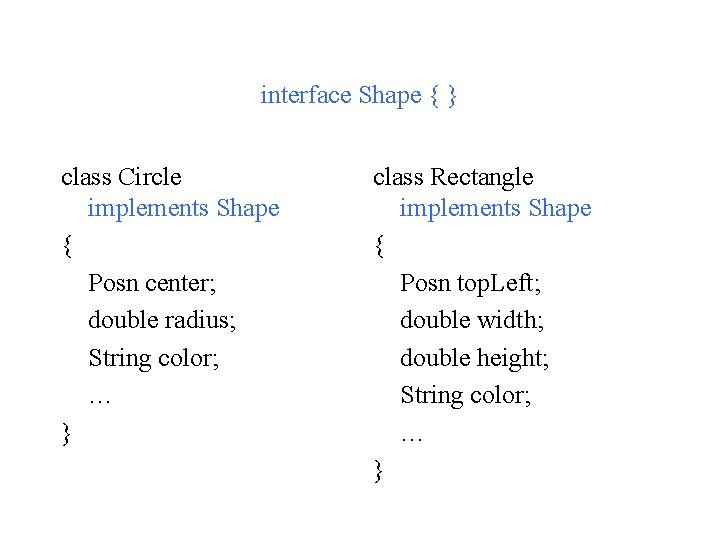
interface Shape { } class Circle implements Shape { Posn center; double radius; String color; … } class Rectangle implements Shape { Posn top. Left; double width; double height; String color; … }
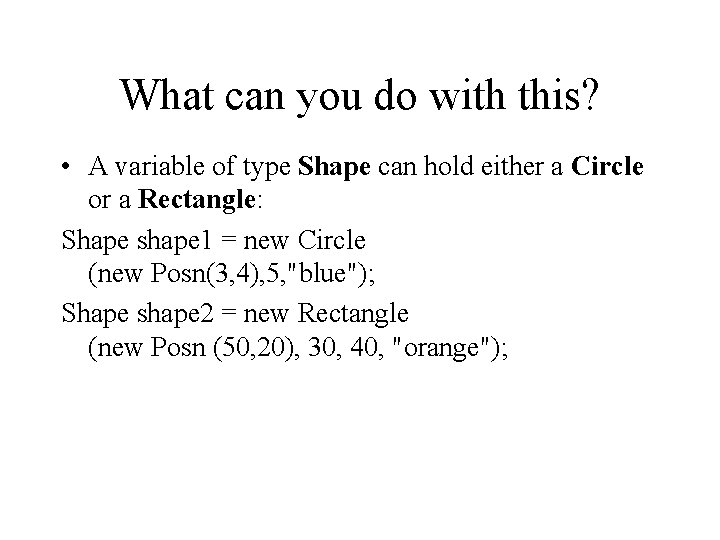
What can you do with this? • A variable of type Shape can hold either a Circle or a Rectangle: Shape shape 1 = new Circle (new Posn(3, 4), 5, "blue"); Shape shape 2 = new Rectangle (new Posn (50, 20), 30, 40, "orange");
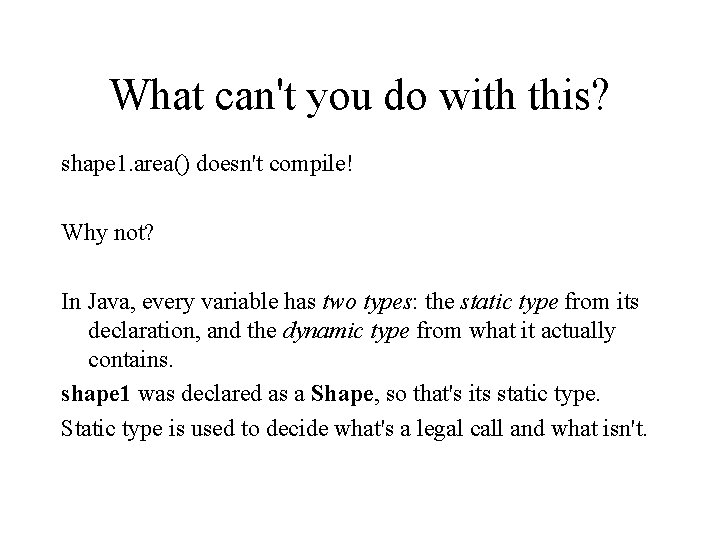
What can't you do with this? shape 1. area() doesn't compile! Why not? In Java, every variable has two types: the static type from its declaration, and the dynamic type from what it actually contains. shape 1 was declared as a Shape, so that's its static type. Static type is used to decide what's a legal call and what isn't.
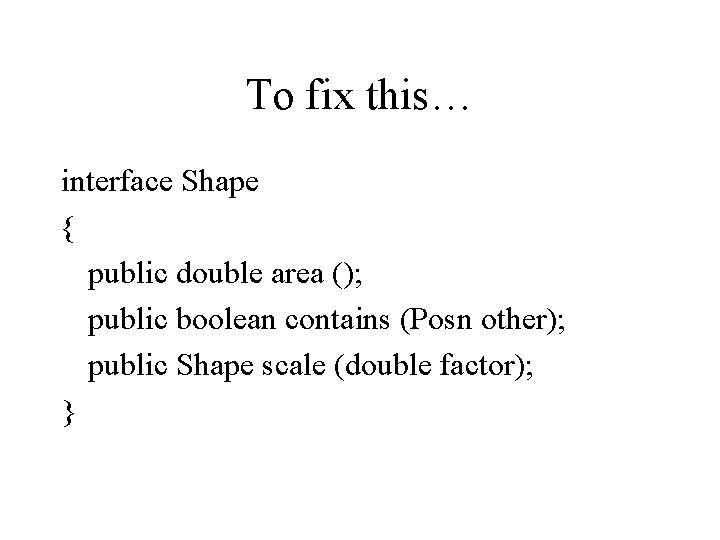
To fix this… interface Shape { public double area (); public boolean contains (Posn other); public Shape scale (double factor); }
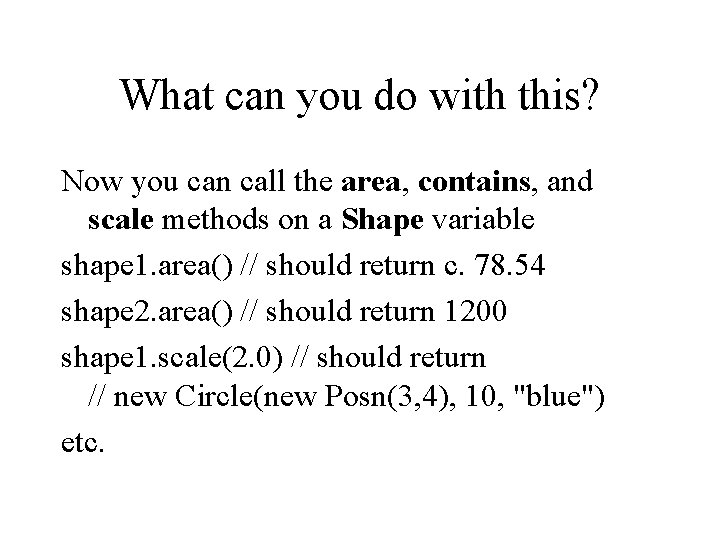
What can you do with this? Now you can call the area, contains, and scale methods on a Shape variable shape 1. area() // should return c. 78. 54 shape 2. area() // should return 1200 shape 1. scale(2. 0) // should return // new Circle(new Posn(3, 4), 10, "blue") etc.
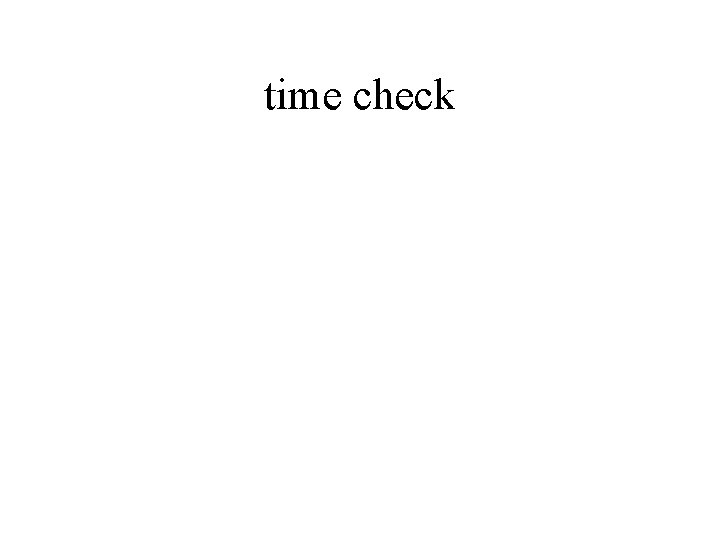
time check
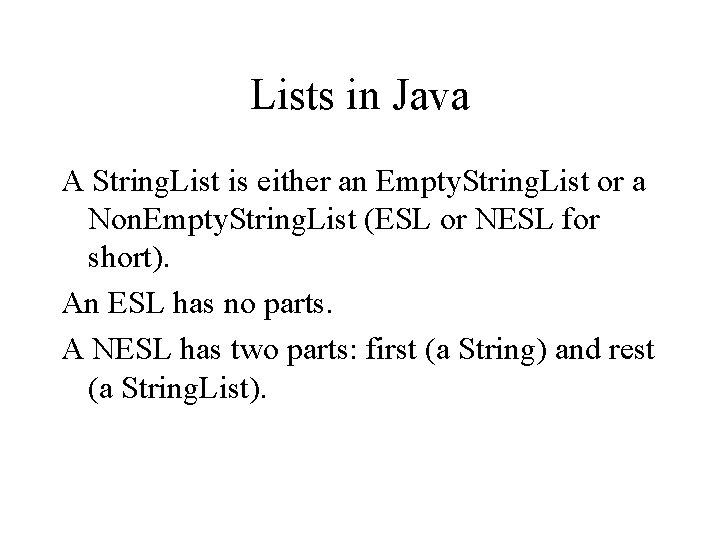
Lists in Java A String. List is either an Empty. String. List or a Non. Empty. String. List (ESL or NESL for short). An ESL has no parts. A NESL has two parts: first (a String) and rest (a String. List).
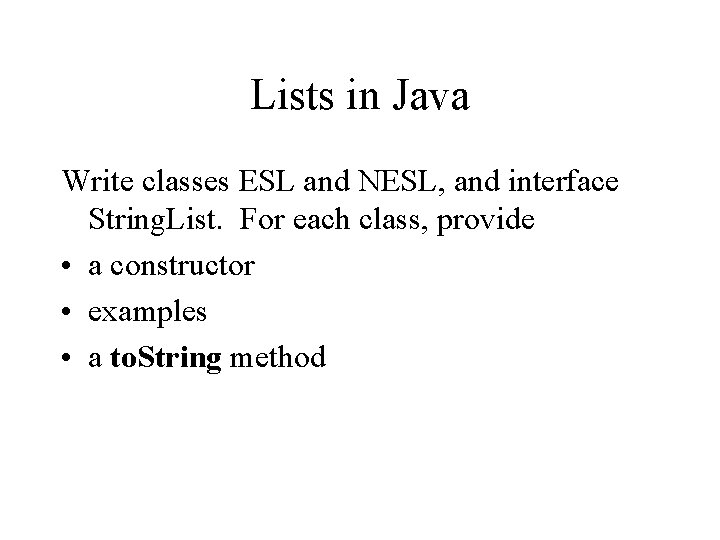
Lists in Java Write classes ESL and NESL, and interface String. List. For each class, provide • a constructor • examples • a to. String method
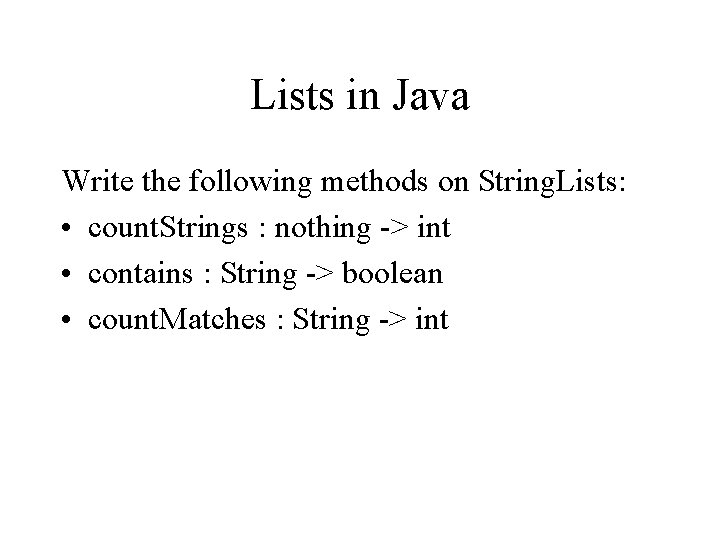
Lists in Java Write the following methods on String. Lists: • count. Strings : nothing -> int • contains : String -> boolean • count. Matches : String -> int
![Recall addup function define addup nums cond empty nums 0 cons nums first Recall add-up function (define (add-up nums) (cond [(empty? nums) 0] [(cons? nums) (+ (first](https://slidetodoc.com/presentation_image/1ac9f442f43961e8cc4c89e300ba22f2/image-16.jpg)
Recall add-up function (define (add-up nums) (cond [(empty? nums) 0] [(cons? nums) (+ (first nums) (add-up (rest nums)))]))
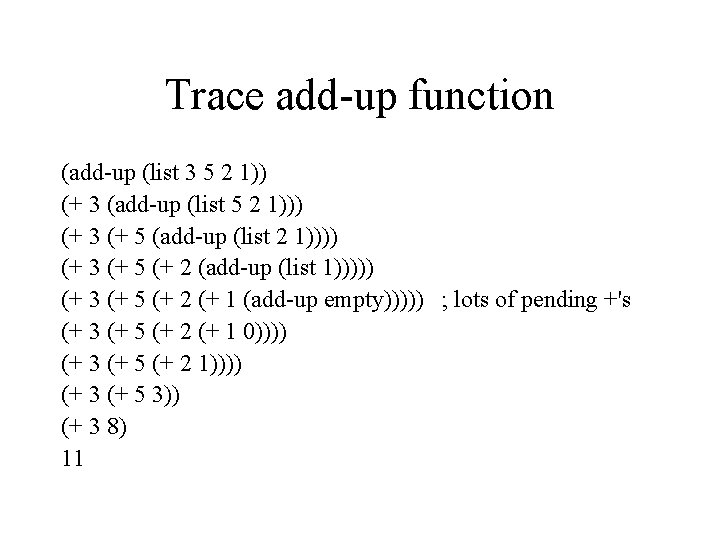
Trace add-up function (add-up (list 3 5 2 1)) (+ 3 (add-up (list 5 2 1))) (+ 3 (+ 5 (add-up (list 2 1)))) (+ 3 (+ 5 (+ 2 (add-up (list 1))))) (+ 3 (+ 5 (+ 2 (+ 1 (add-up empty))))) ; lots of pending +'s (+ 3 (+ 5 (+ 2 (+ 1 0)))) (+ 3 (+ 5 (+ 2 1)))) (+ 3 (+ 5 3)) (+ 3 8) 11
![Another approach define addupaccum nums sofar cond empty nums sofar cons nums addupaccum rest Another approach (define (add-up-accum nums so-far) (cond [(empty? nums) so-far] [(cons? nums) (add-up-accum (rest](https://slidetodoc.com/presentation_image/1ac9f442f43961e8cc4c89e300ba22f2/image-18.jpg)
Another approach (define (add-up-accum nums so-far) (cond [(empty? nums) so-far] [(cons? nums) (add-up-accum (rest nums) (+ (first nums) so-far))])) (add-up-accum (list 3 5 2 1) 0) (add-up-accum (list 5 2 1) (+ 3 0)) (add-up-accum (list 5 2 1) 3) (add-up-accum (list 2 1) (+ 5 3)) (add-up-accum (list 2 1) 8) (add-up-accum (list 1) (+ 2 8)) (add-up-accum (list 1) 10) (add-up-accum empty (+ 1 10)) (add-up-accum empty 11) 11 ; never more than 1 pending +
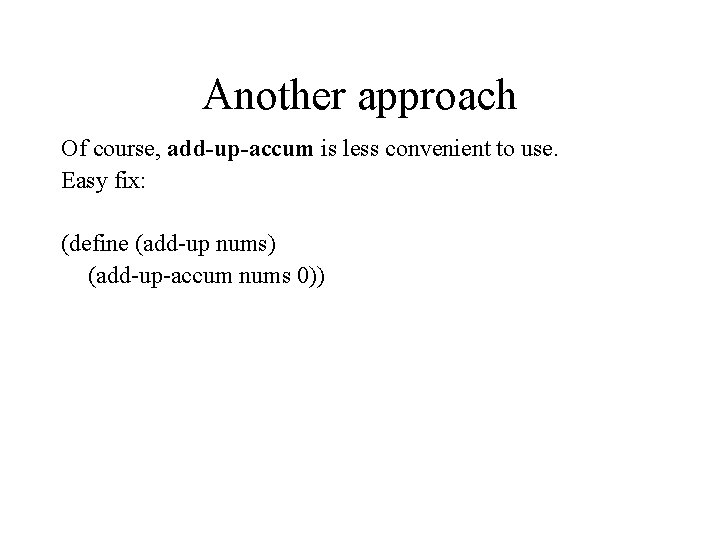
Another approach Of course, add-up-accum is less convenient to use. Easy fix: (define (add-up nums) (add-up-accum nums 0))
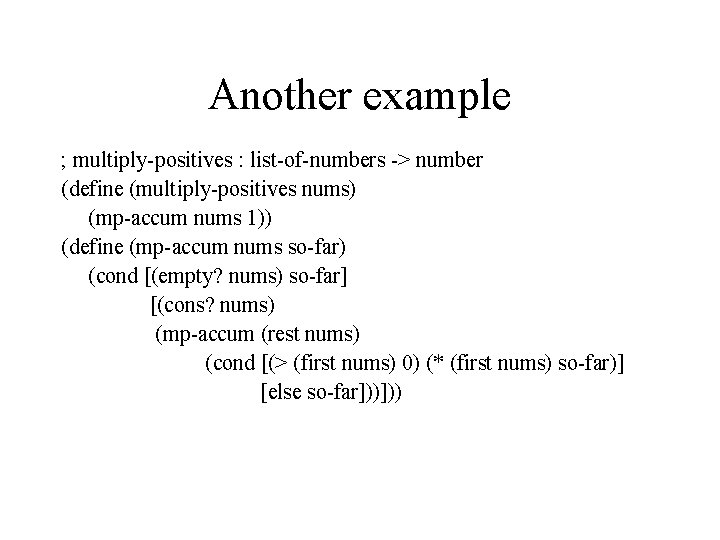
Another example ; multiply-positives : list-of-numbers -> number (define (multiply-positives nums) (mp-accum nums 1)) (define (mp-accum nums so-far) (cond [(empty? nums) so-far] [(cons? nums) (mp-accum (rest nums) (cond [(> (first nums) 0) (* (first nums) so-far)] [else so-far]))]))
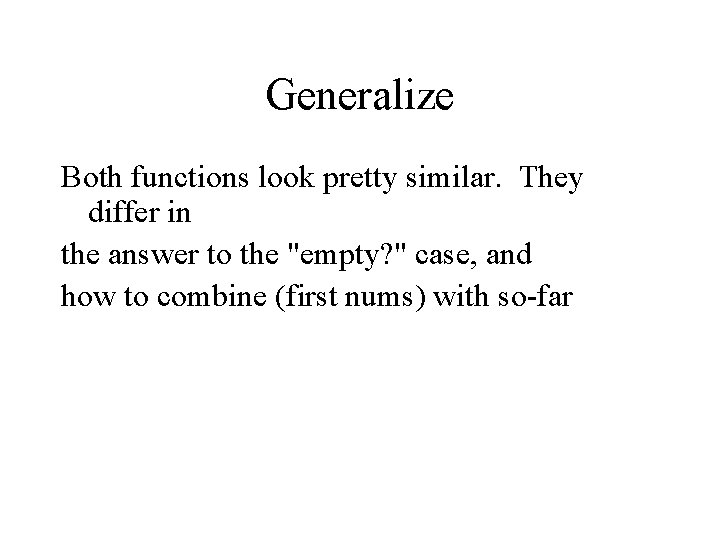
Generalize Both functions look pretty similar. They differ in the answer to the "empty? " case, and how to combine (first nums) with so-far
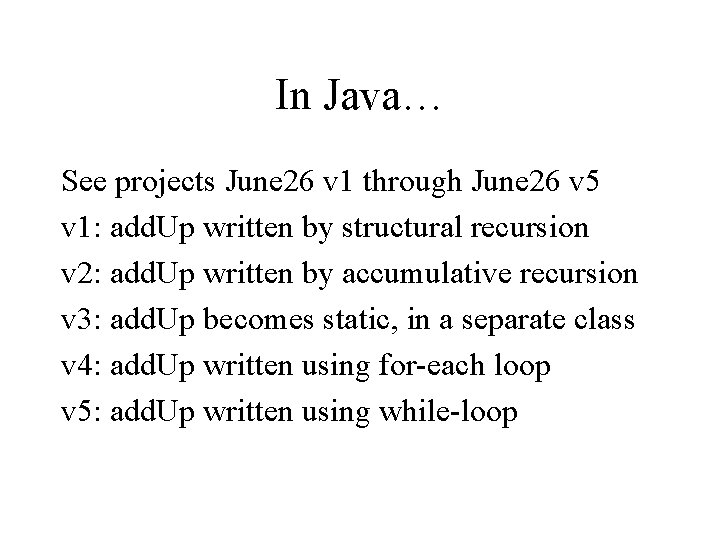
In Java… See projects June 26 v 1 through June 26 v 5 v 1: add. Up written by structural recursion v 2: add. Up written by accumulative recursion v 3: add. Up becomes static, in a separate class v 4: add. Up written using for-each loop v 5: add. Up written using while-loop
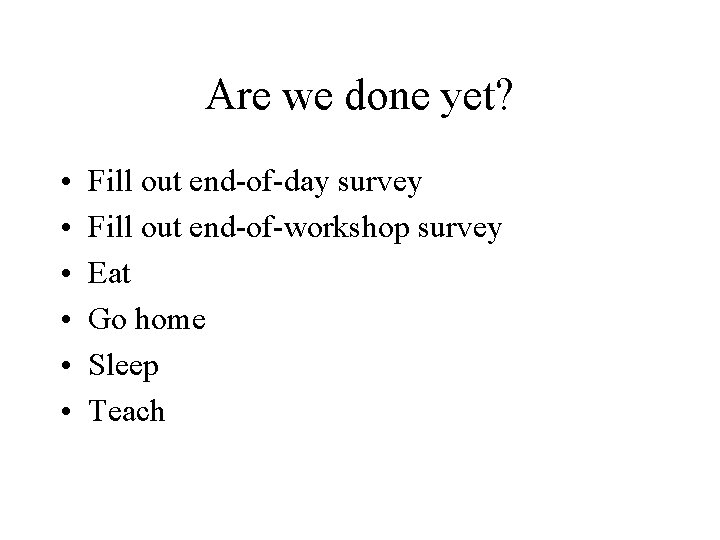
Are we done yet? • • • Fill out end-of-day survey Fill out end-of-workshop survey Eat Go home Sleep Teach
Adelphi moodle
Friday morning prayer
Snowman mad libs
Happy friday
Good morning friends friday
Adelphi early learning center
Adelphi research steroids
Emerson degreeworks
Adelphi definition
Good compounding practices
3 domain scheme and 5 kingdom scheme
Stata schemes
Consumer financial services pensacola fl
No one loves me
Good morning class!
Ding ding ding good morning
Good afternoon, teacher
Good morning everybody or everyone
Hello friend! i am glad to see you!
Hello formal
Buenas tardes
Monash unistart support scholarship
Import java.util.*
Import java.util.*