System programming file management CS 2204 Class meeting
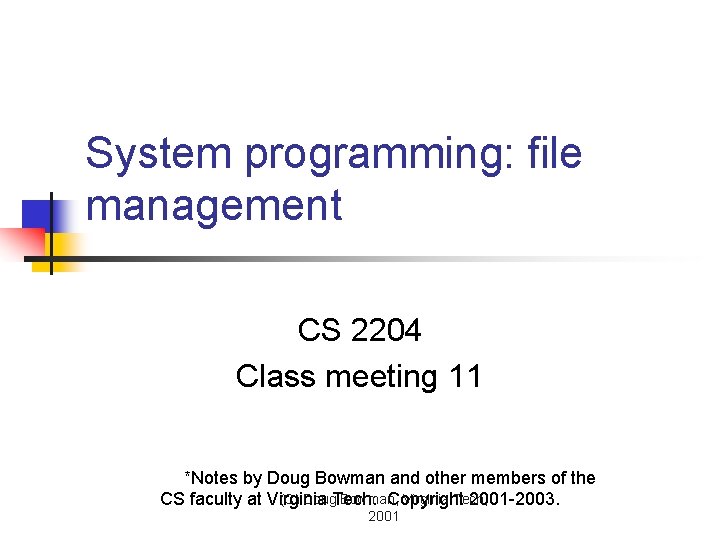
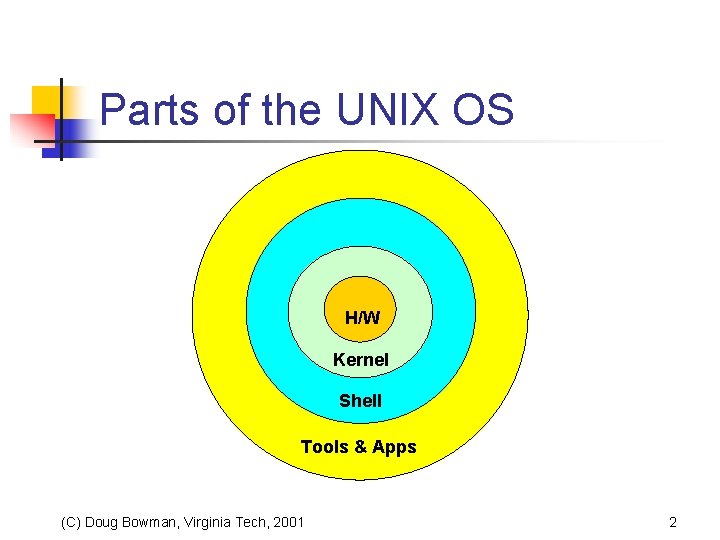
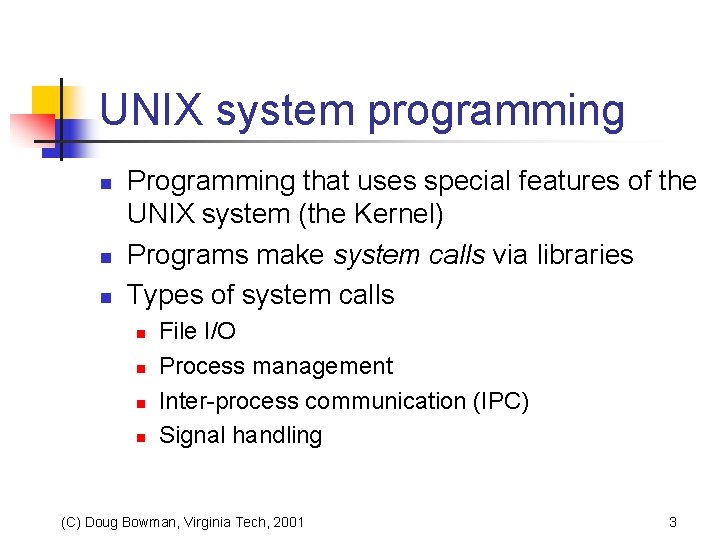
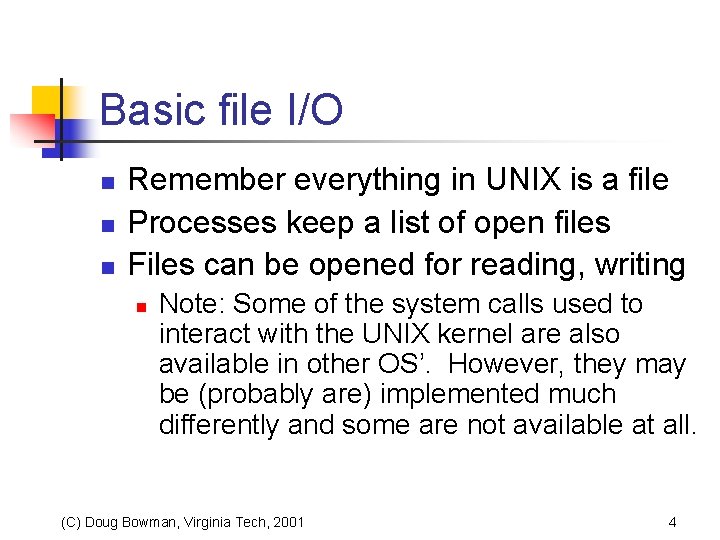
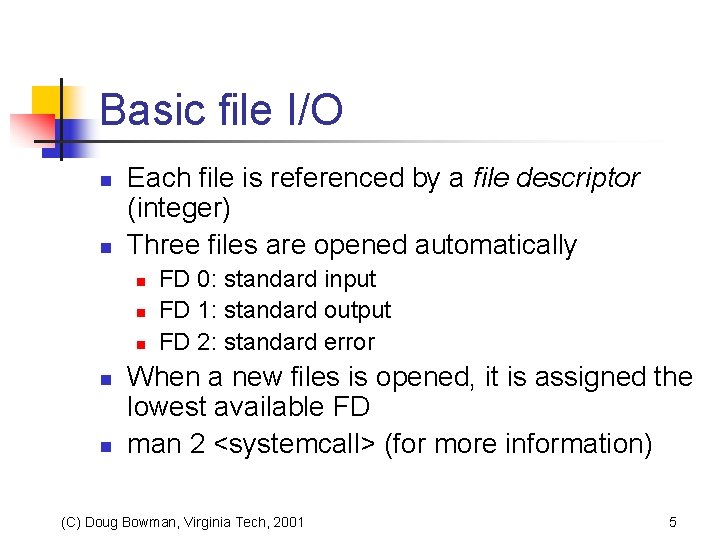
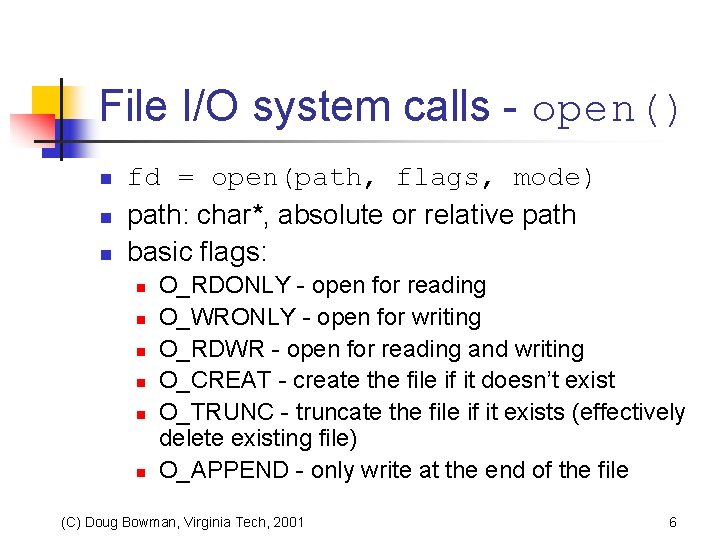
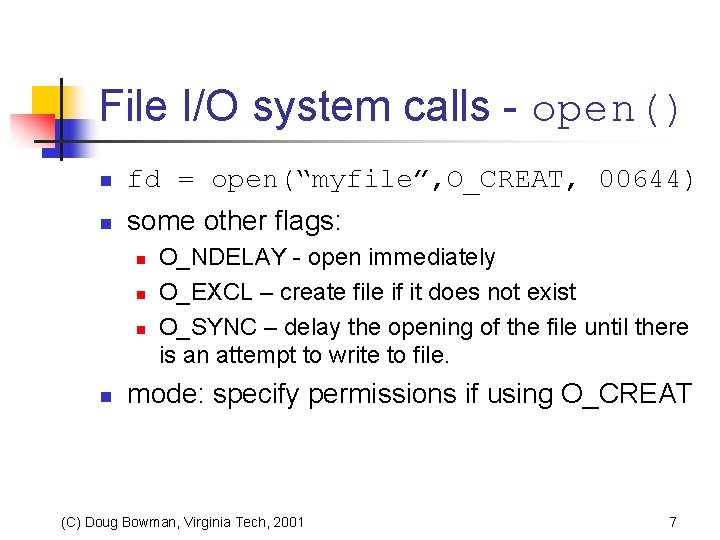
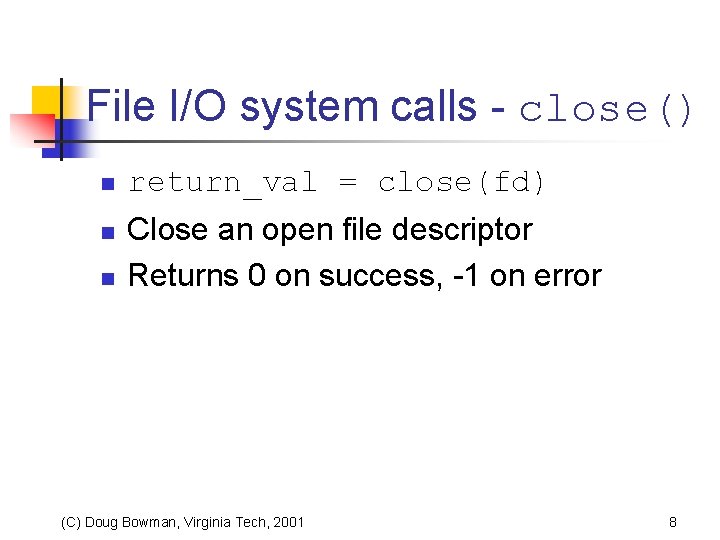
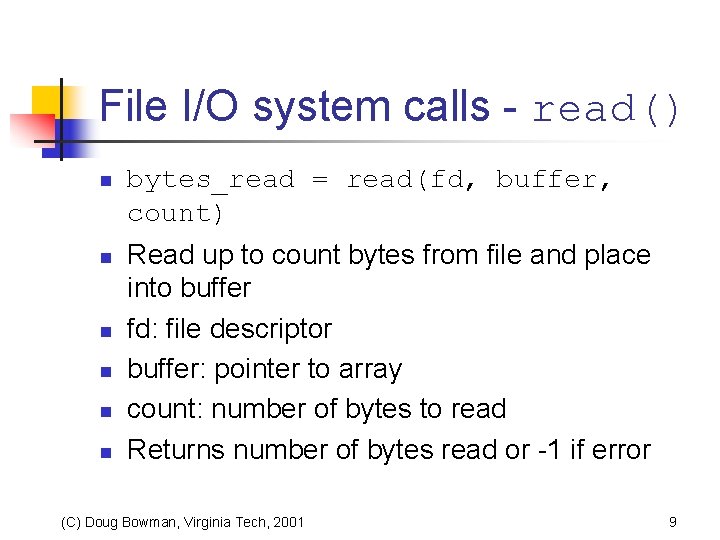
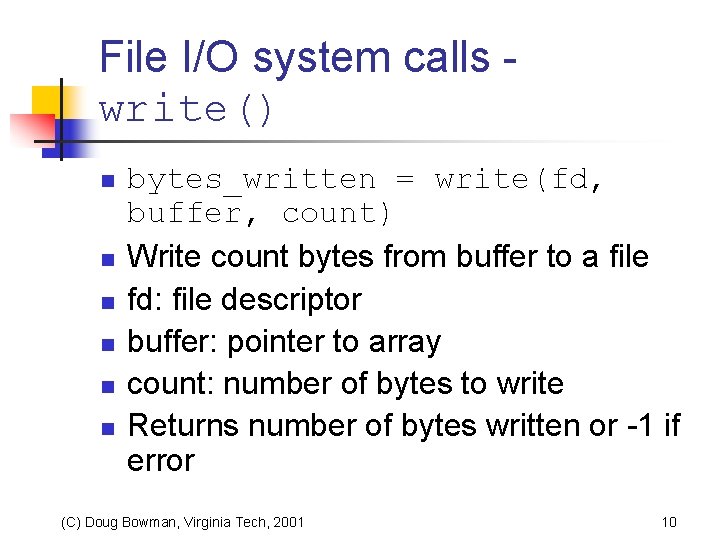
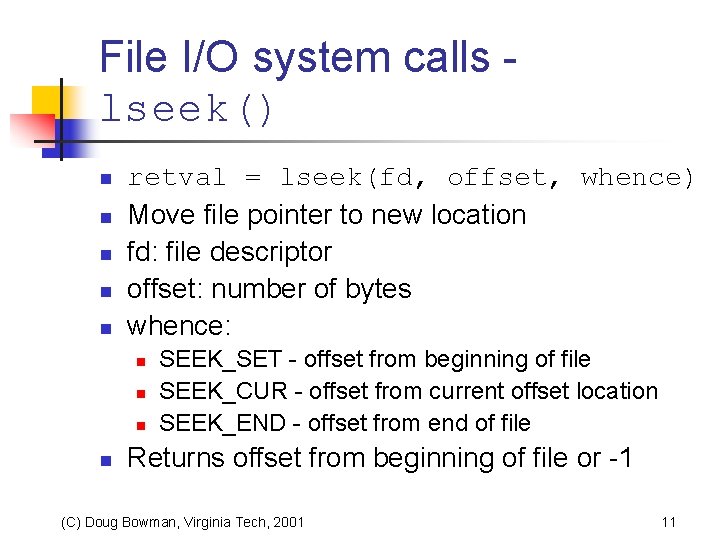
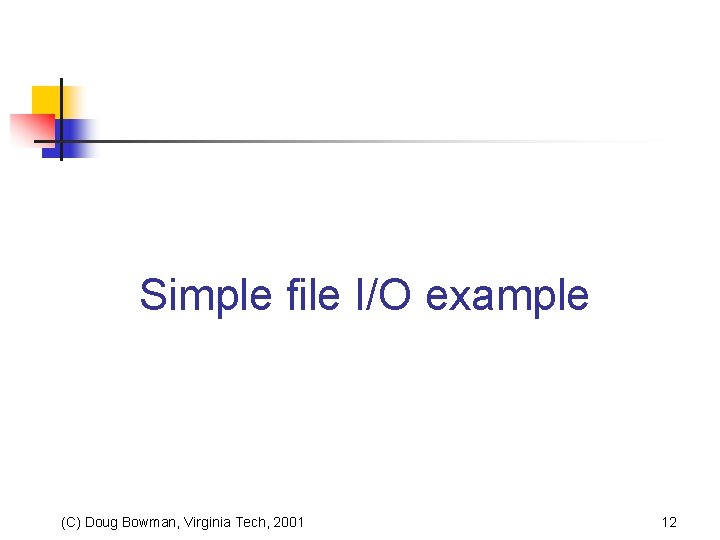
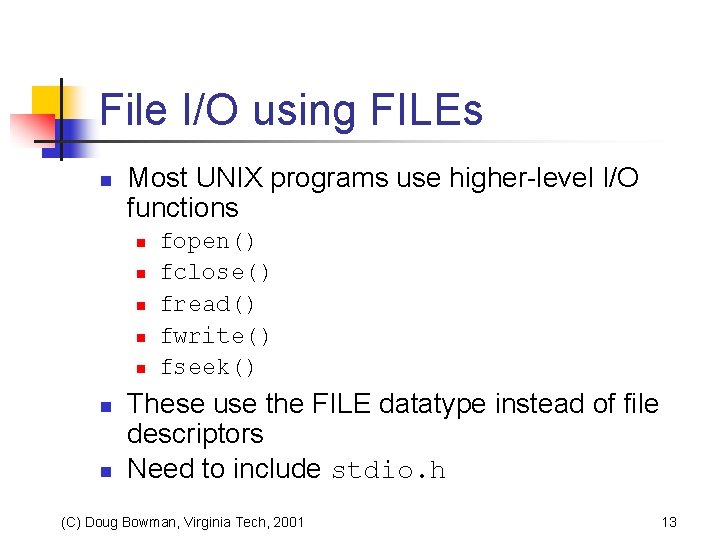
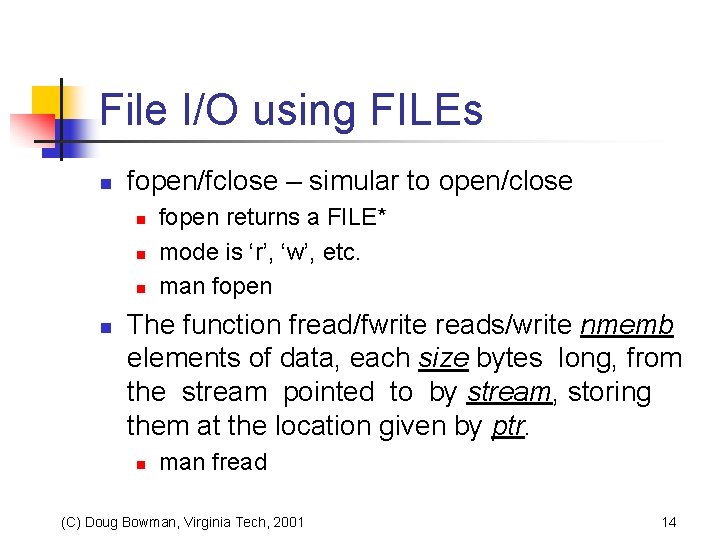
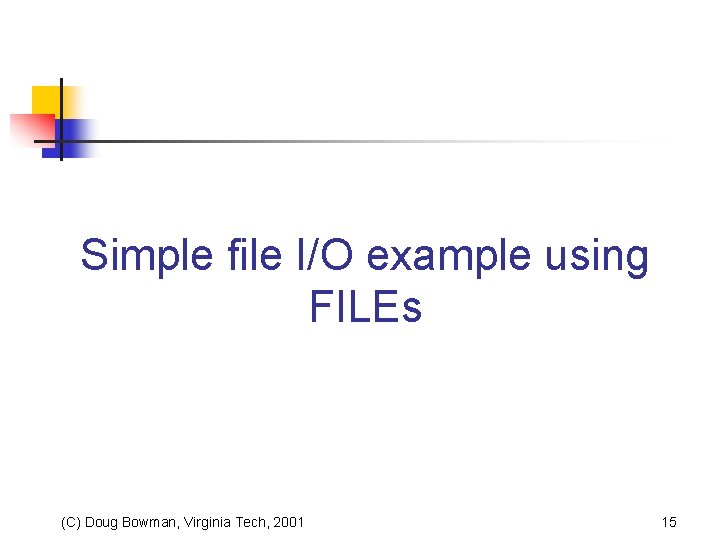
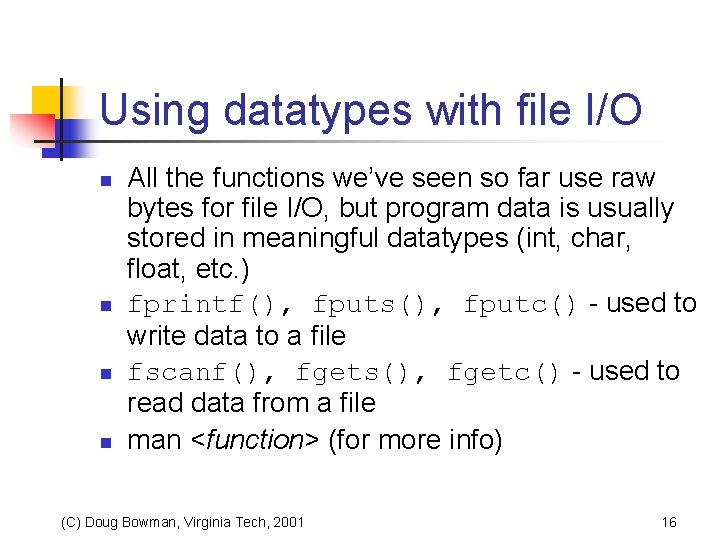
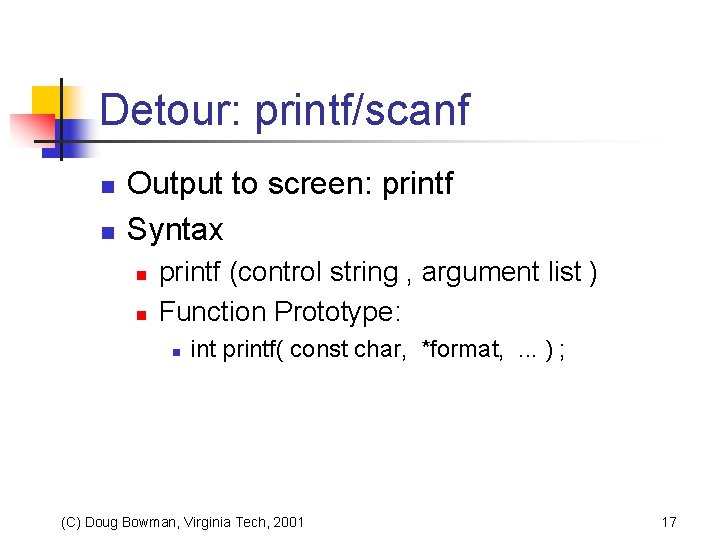
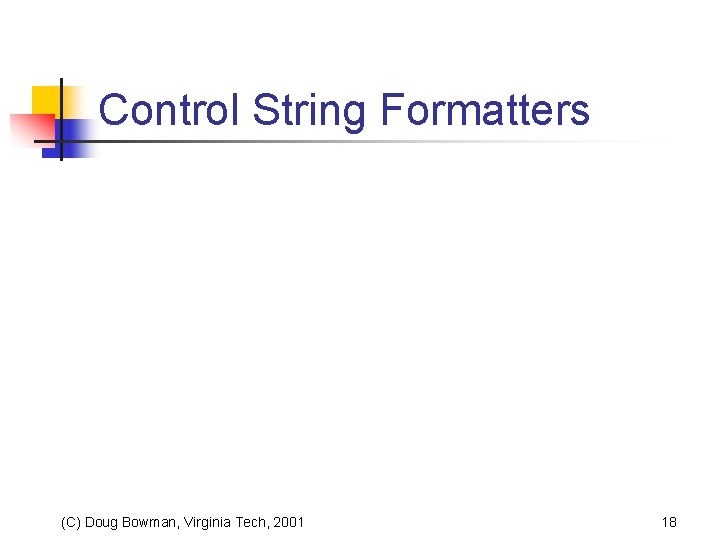
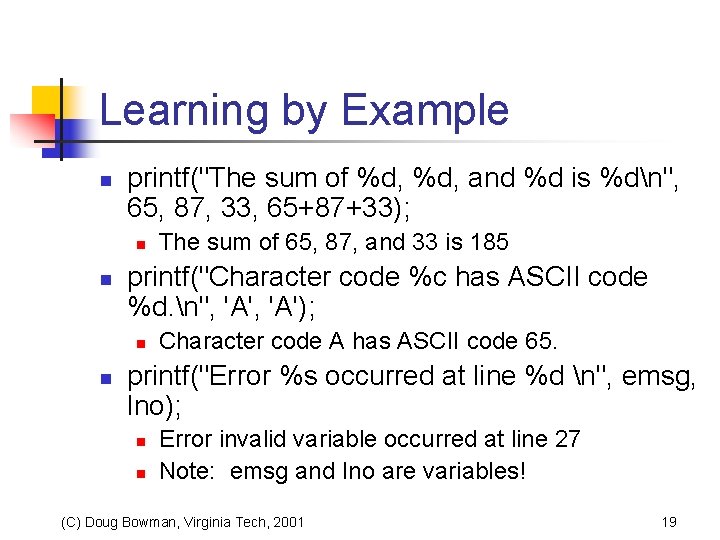
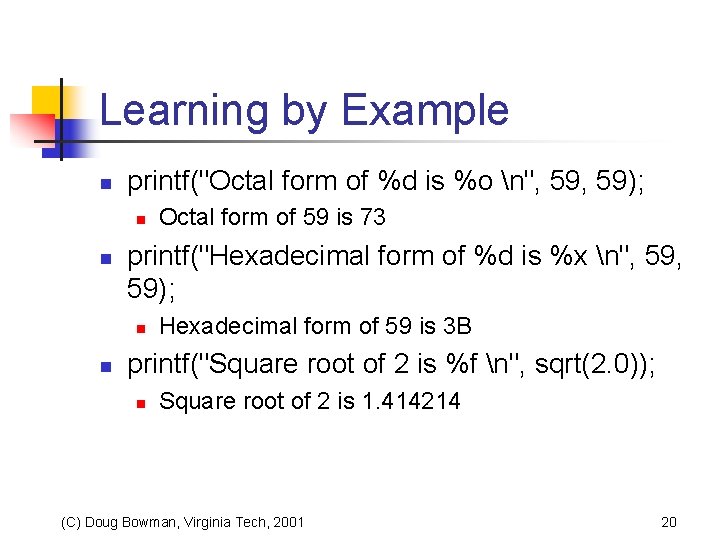
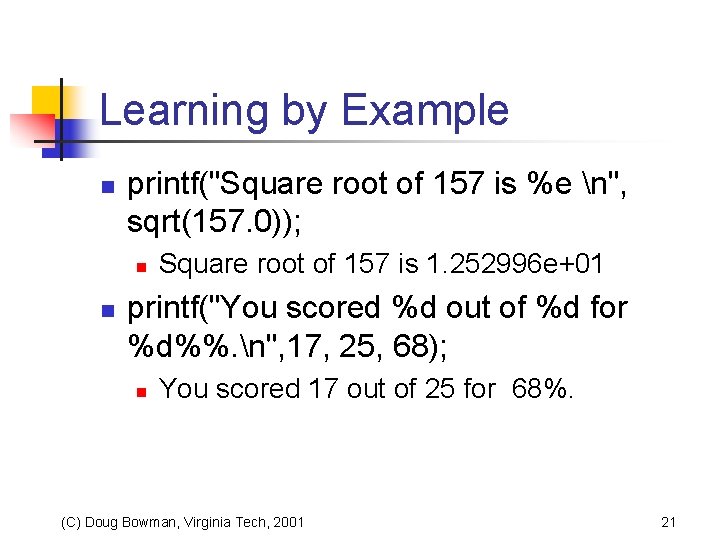
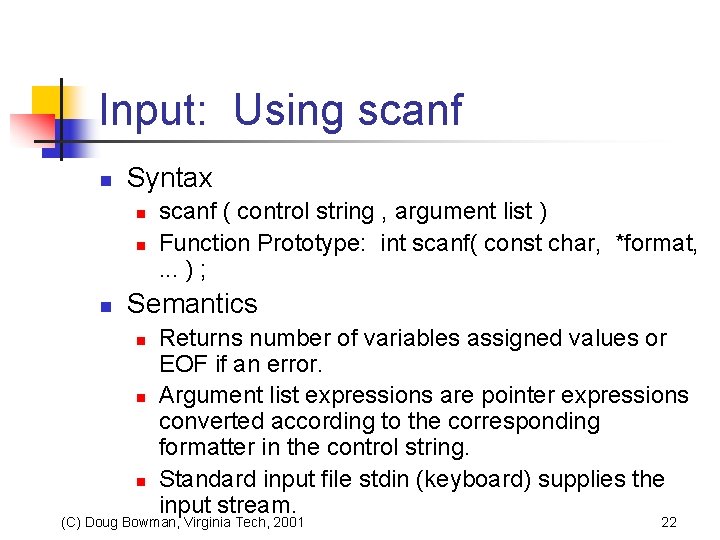
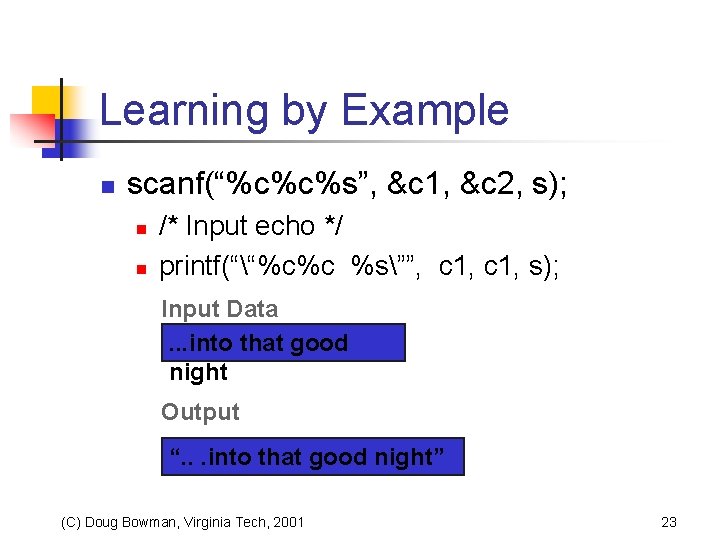
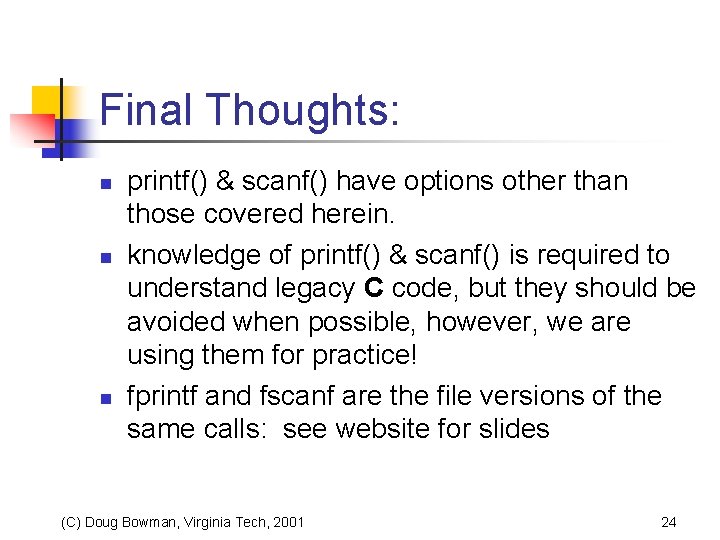
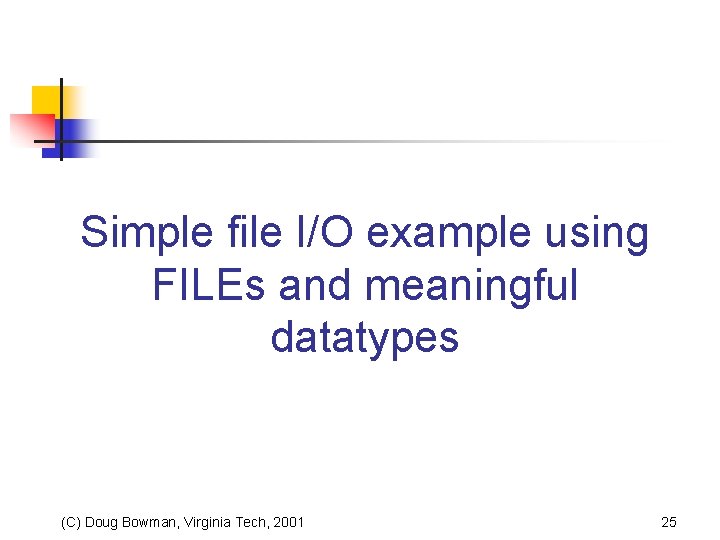
- Slides: 25
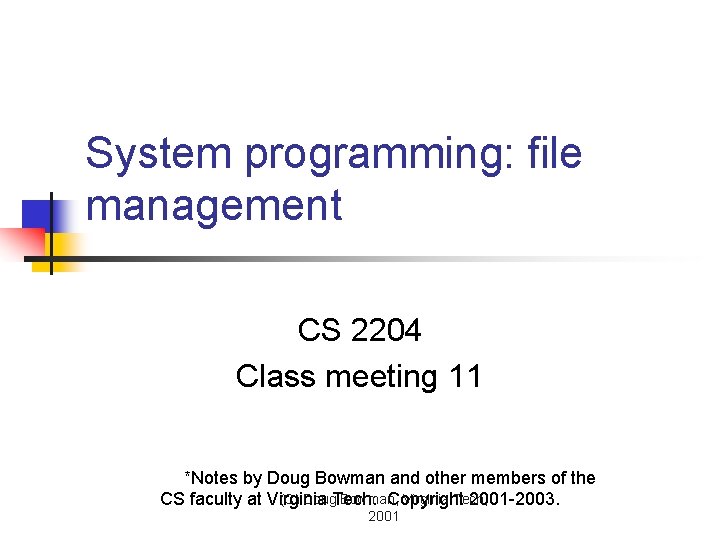
System programming: file management CS 2204 Class meeting 11 *Notes by Doug Bowman and other members of the (C) Doug. Tech. Bowman, Virginia Tech, CS faculty at Virginia Copyright 2001 -2003. 2001
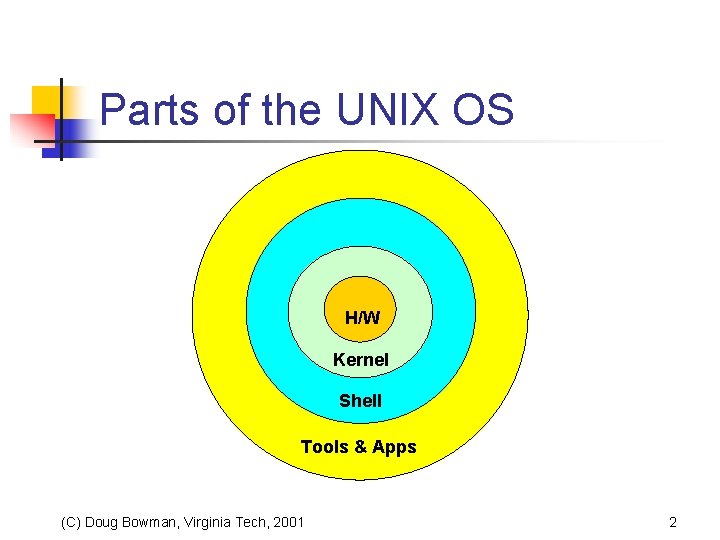
Parts of the UNIX OS H/W Kernel Shell Tools & Apps (C) Doug Bowman, Virginia Tech, 2001 2
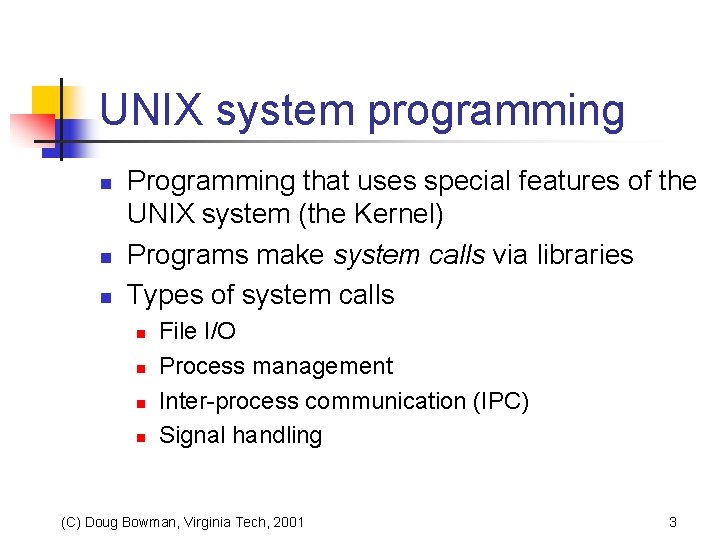
UNIX system programming n n n Programming that uses special features of the UNIX system (the Kernel) Programs make system calls via libraries Types of system calls n n File I/O Process management Inter-process communication (IPC) Signal handling (C) Doug Bowman, Virginia Tech, 2001 3
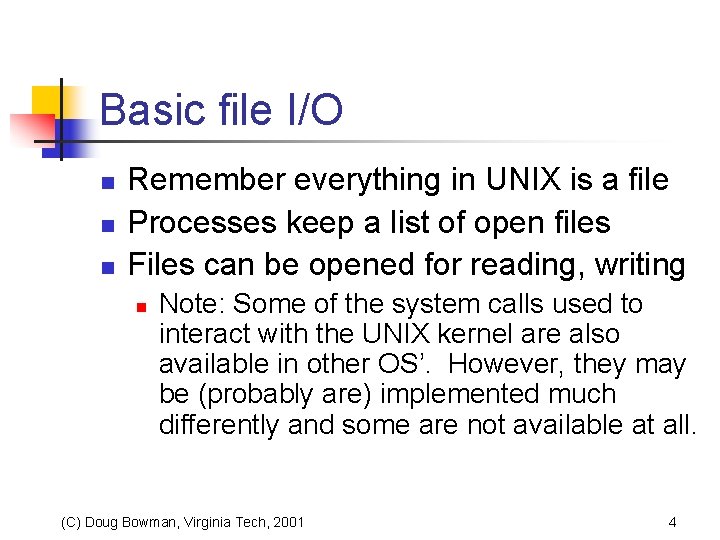
Basic file I/O n n n Remember everything in UNIX is a file Processes keep a list of open files Files can be opened for reading, writing n Note: Some of the system calls used to interact with the UNIX kernel are also available in other OS’. However, they may be (probably are) implemented much differently and some are not available at all. (C) Doug Bowman, Virginia Tech, 2001 4
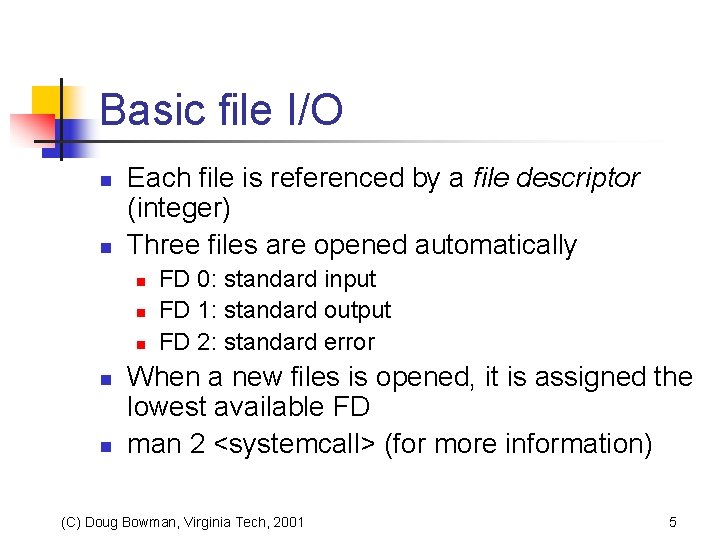
Basic file I/O n n Each file is referenced by a file descriptor (integer) Three files are opened automatically n n n FD 0: standard input FD 1: standard output FD 2: standard error When a new files is opened, it is assigned the lowest available FD man 2 <systemcall> (for more information) (C) Doug Bowman, Virginia Tech, 2001 5
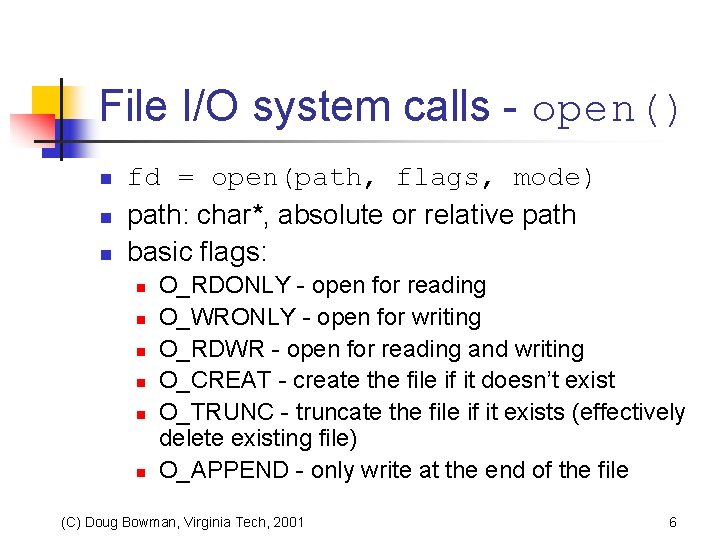
File I/O system calls - open() n n n fd = open(path, flags, mode) path: char*, absolute or relative path basic flags: n n n O_RDONLY - open for reading O_WRONLY - open for writing O_RDWR - open for reading and writing O_CREAT - create the file if it doesn’t exist O_TRUNC - truncate the file if it exists (effectively delete existing file) O_APPEND - only write at the end of the file (C) Doug Bowman, Virginia Tech, 2001 6
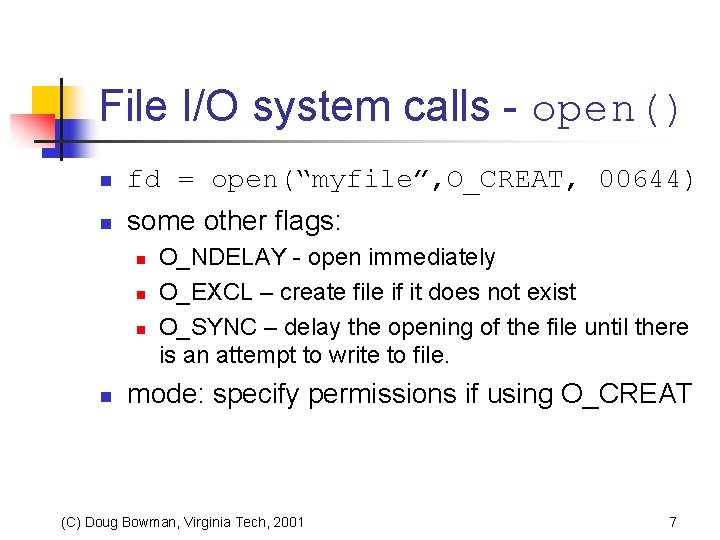
File I/O system calls - open() n fd = open(“myfile”, O_CREAT, 00644) n some other flags: n n O_NDELAY - open immediately O_EXCL – create file if it does not exist O_SYNC – delay the opening of the file until there is an attempt to write to file. mode: specify permissions if using O_CREAT (C) Doug Bowman, Virginia Tech, 2001 7
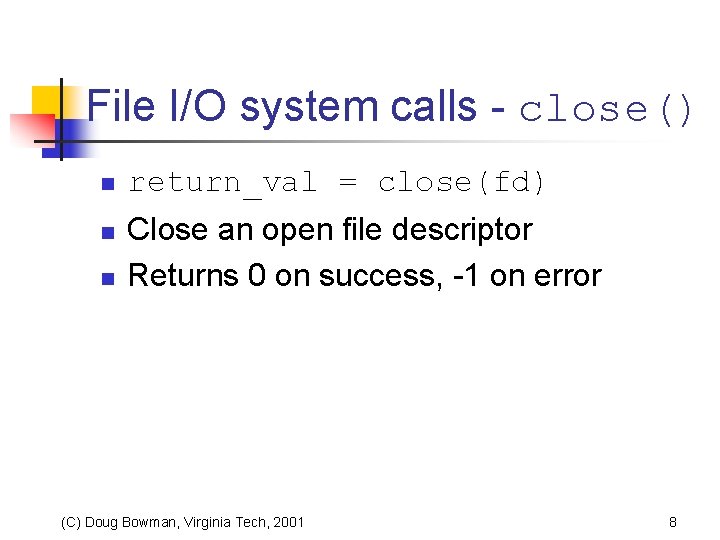
File I/O system calls - close() n n n return_val = close(fd) Close an open file descriptor Returns 0 on success, -1 on error (C) Doug Bowman, Virginia Tech, 2001 8
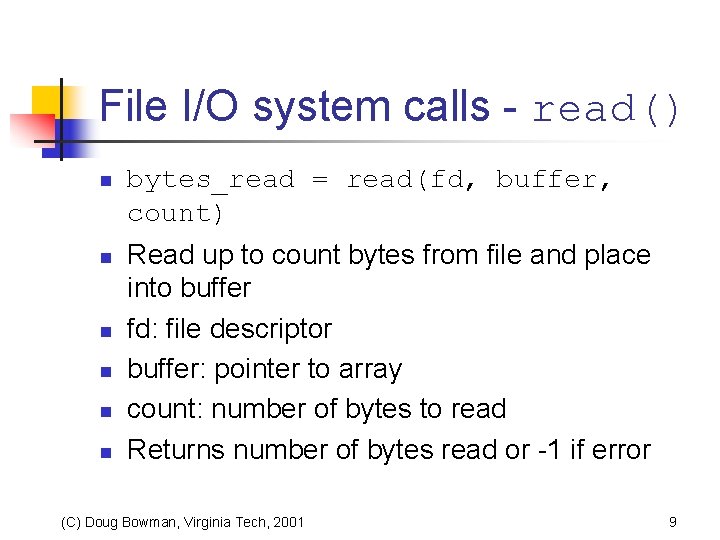
File I/O system calls - read() n n n bytes_read = read(fd, buffer, count) Read up to count bytes from file and place into buffer fd: file descriptor buffer: pointer to array count: number of bytes to read Returns number of bytes read or -1 if error (C) Doug Bowman, Virginia Tech, 2001 9
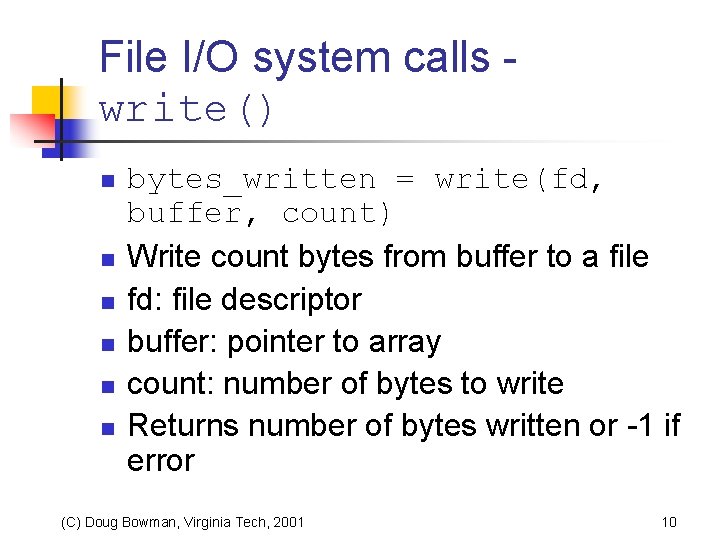
File I/O system calls write() n n n bytes_written = write(fd, buffer, count) Write count bytes from buffer to a file fd: file descriptor buffer: pointer to array count: number of bytes to write Returns number of bytes written or -1 if error (C) Doug Bowman, Virginia Tech, 2001 10
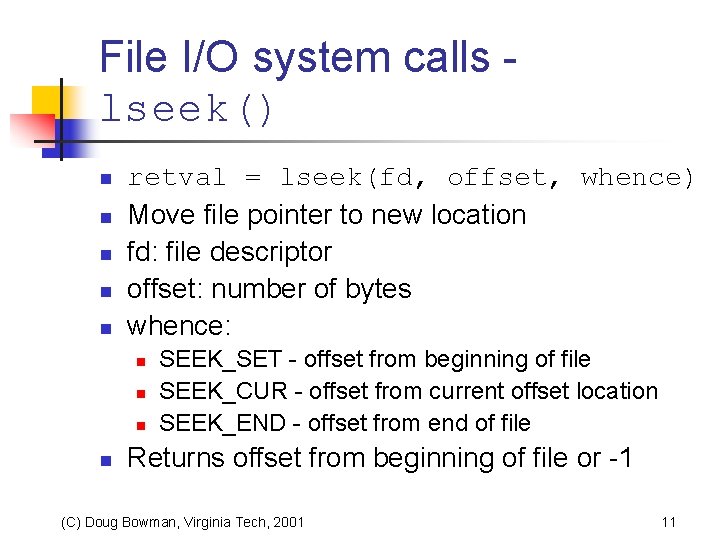
File I/O system calls lseek() n n n retval = lseek(fd, offset, whence) Move file pointer to new location fd: file descriptor offset: number of bytes whence: n n SEEK_SET - offset from beginning of file SEEK_CUR - offset from current offset location SEEK_END - offset from end of file Returns offset from beginning of file or -1 (C) Doug Bowman, Virginia Tech, 2001 11
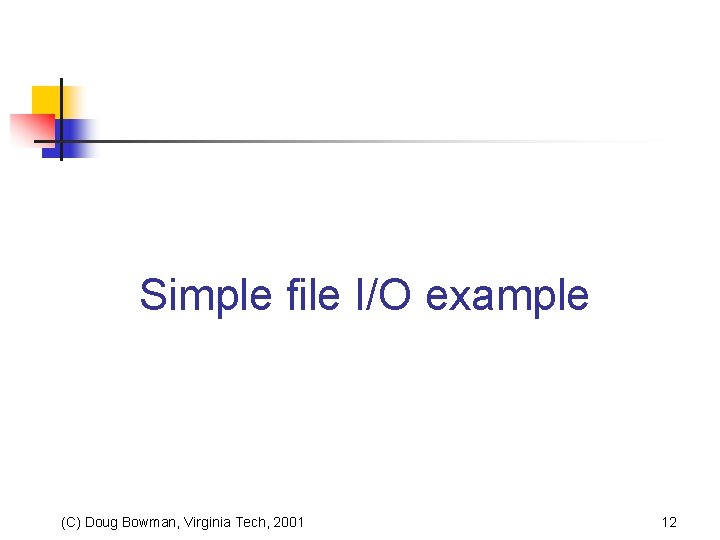
Simple file I/O example (C) Doug Bowman, Virginia Tech, 2001 12
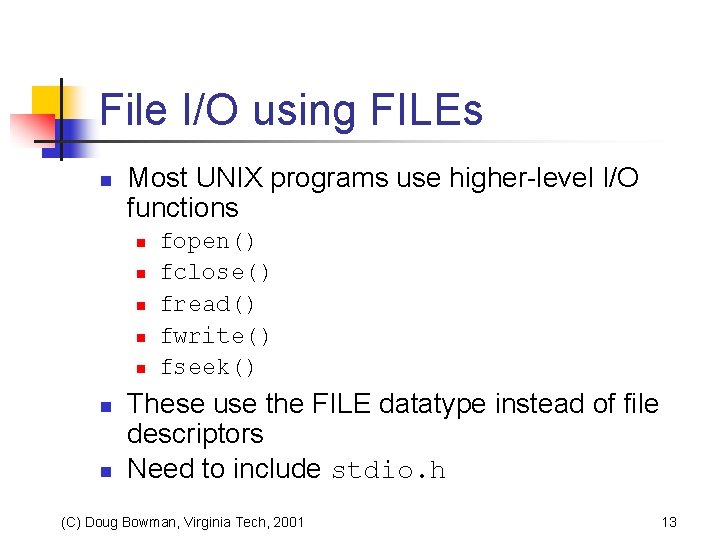
File I/O using FILEs n Most UNIX programs use higher-level I/O functions n n n n fopen() fclose() fread() fwrite() fseek() These use the FILE datatype instead of file descriptors Need to include stdio. h (C) Doug Bowman, Virginia Tech, 2001 13
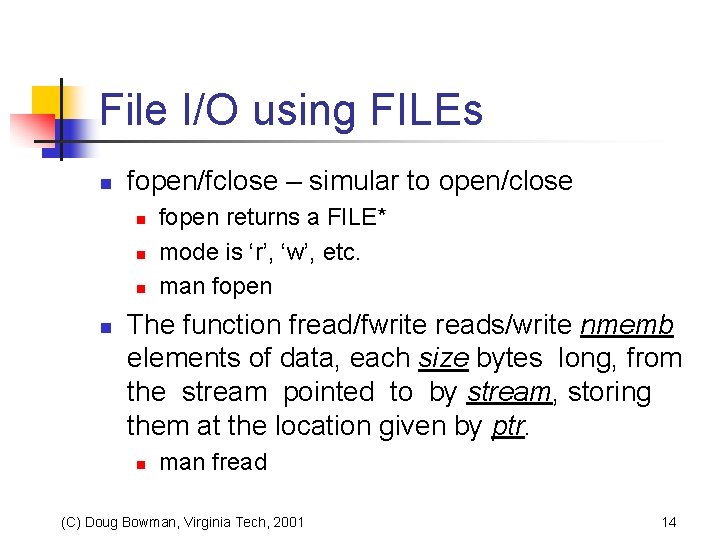
File I/O using FILEs n fopen/fclose – simular to open/close n n fopen returns a FILE* mode is ‘r’, ‘w’, etc. man fopen The function fread/fwrite reads/write nmemb elements of data, each size bytes long, from the stream pointed to by stream, storing them at the location given by ptr. n man fread (C) Doug Bowman, Virginia Tech, 2001 14
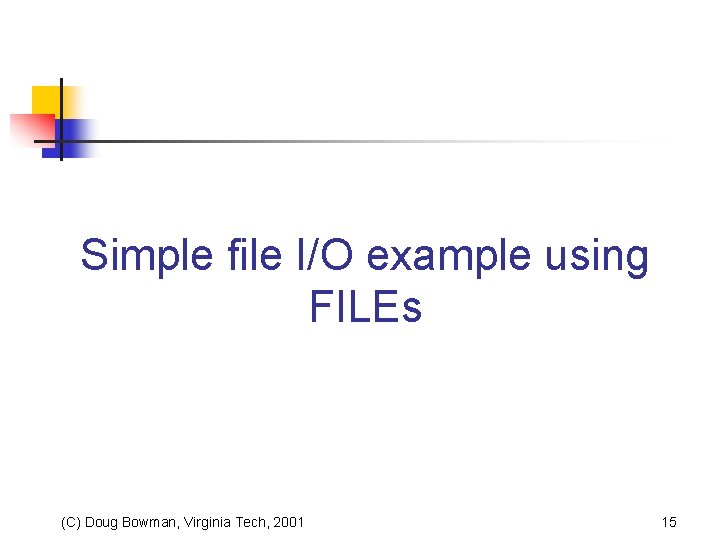
Simple file I/O example using FILEs (C) Doug Bowman, Virginia Tech, 2001 15
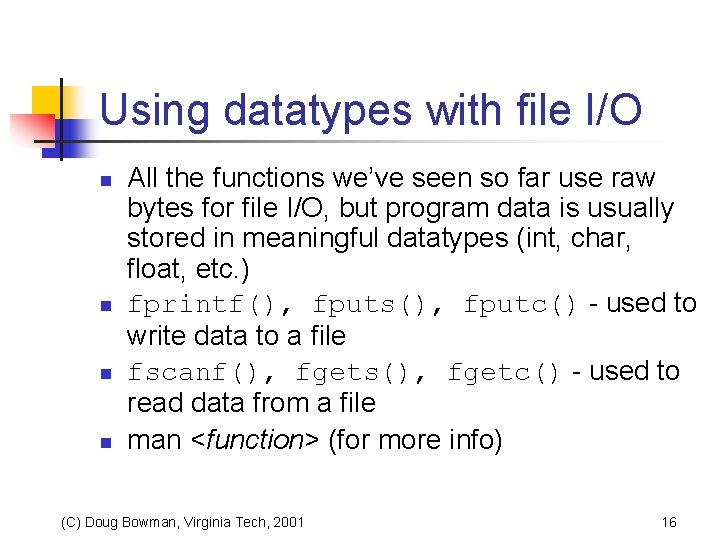
Using datatypes with file I/O n n All the functions we’ve seen so far use raw bytes for file I/O, but program data is usually stored in meaningful datatypes (int, char, float, etc. ) fprintf(), fputs(), fputc() - used to write data to a file fscanf(), fgets(), fgetc() - used to read data from a file man <function> (for more info) (C) Doug Bowman, Virginia Tech, 2001 16
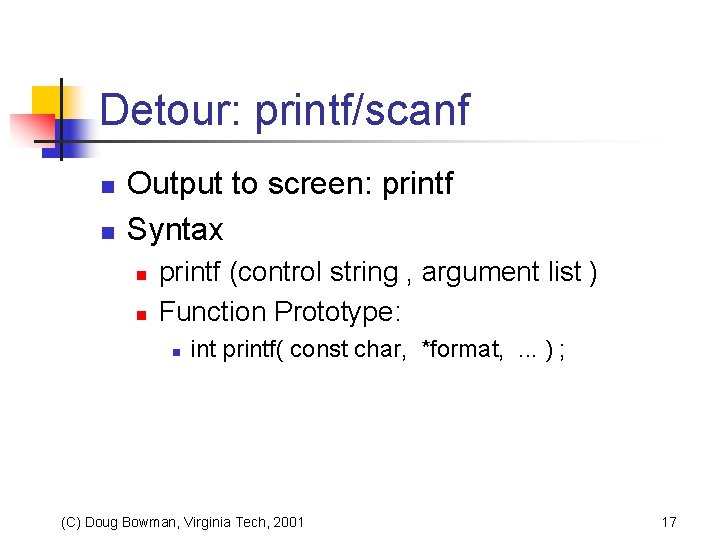
Detour: printf/scanf n n Output to screen: printf Syntax n n printf (control string , argument list ) Function Prototype: n int printf( const char, *format, . . . ) ; (C) Doug Bowman, Virginia Tech, 2001 17
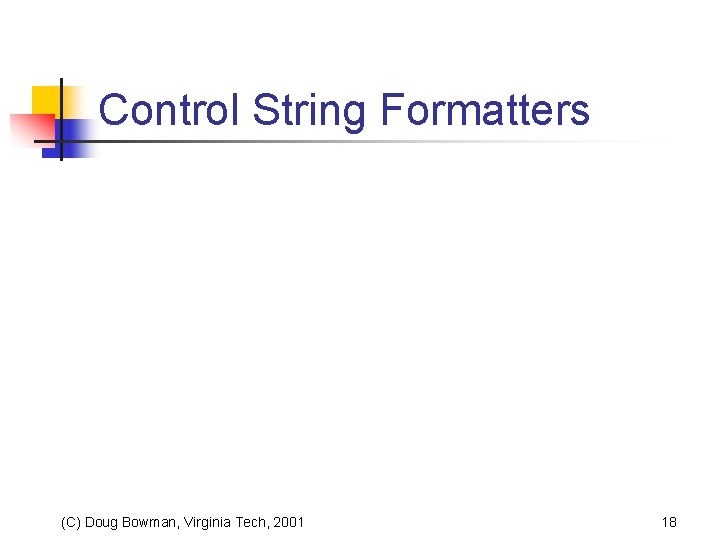
Control String Formatters (C) Doug Bowman, Virginia Tech, 2001 18
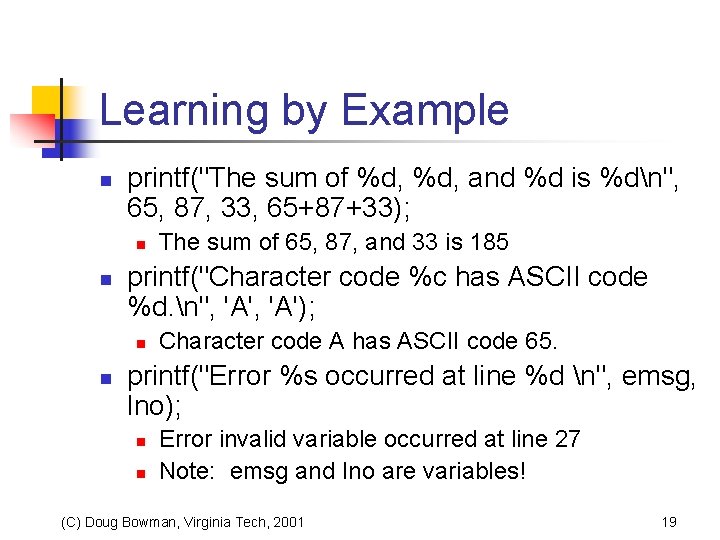
Learning by Example n printf("The sum of %d, and %d is %dn", 65, 87, 33, 65+87+33); n n printf("Character code %c has ASCII code %d. n", 'A'); n n The sum of 65, 87, and 33 is 185 Character code A has ASCII code 65. printf("Error %s occurred at line %d n", emsg, lno); n n Error invalid variable occurred at line 27 Note: emsg and Ino are variables! (C) Doug Bowman, Virginia Tech, 2001 19
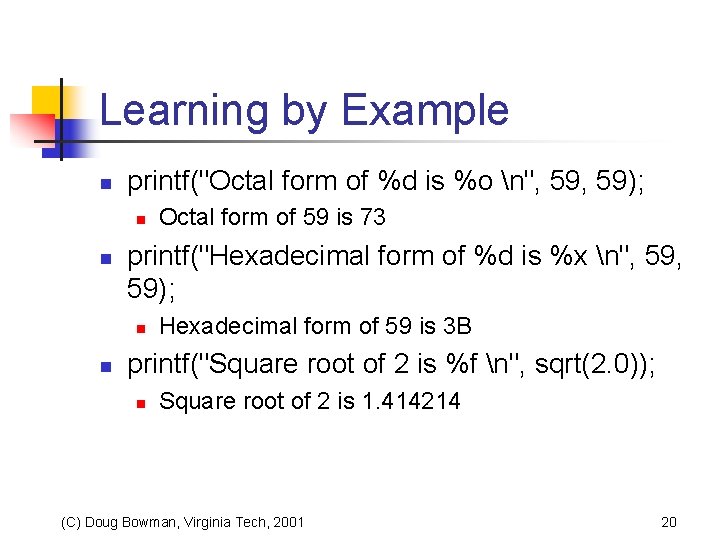
Learning by Example n printf("Octal form of %d is %o n", 59); n n printf("Hexadecimal form of %d is %x n", 59); n n Octal form of 59 is 73 Hexadecimal form of 59 is 3 B printf("Square root of 2 is %f n", sqrt(2. 0)); n Square root of 2 is 1. 414214 (C) Doug Bowman, Virginia Tech, 2001 20
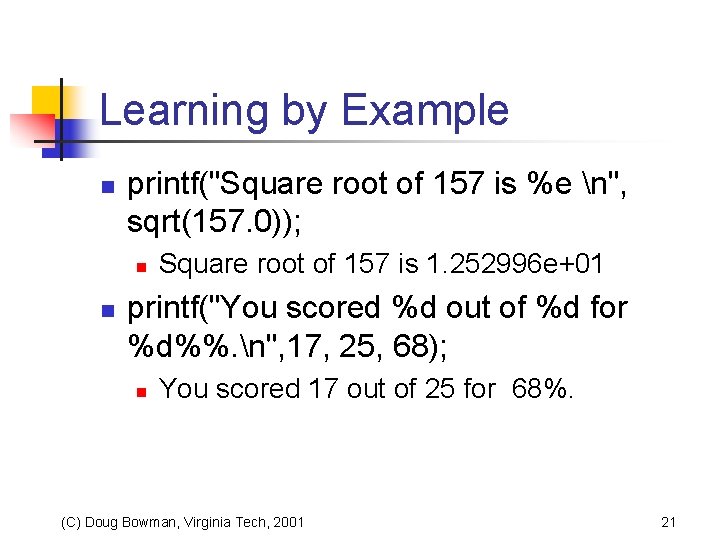
Learning by Example n printf("Square root of 157 is %e n", sqrt(157. 0)); n n Square root of 157 is 1. 252996 e+01 printf("You scored %d out of %d for %d%%. n", 17, 25, 68); n You scored 17 out of 25 for 68%. (C) Doug Bowman, Virginia Tech, 2001 21
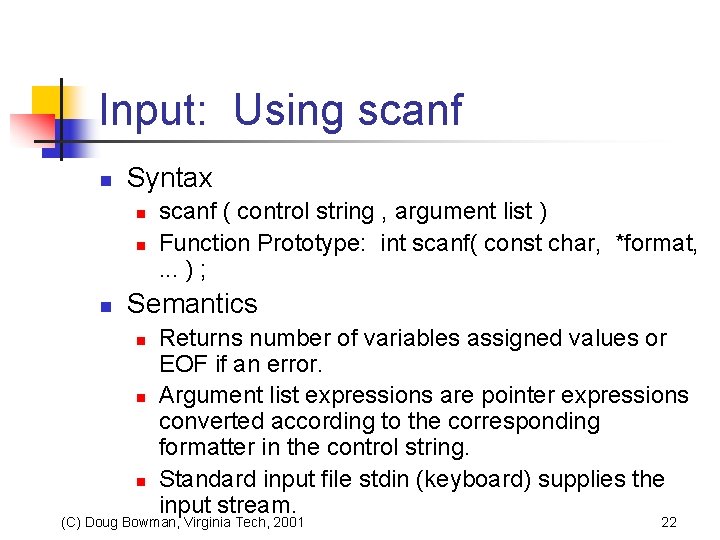
Input: Using scanf n Syntax n n n scanf ( control string , argument list ) Function Prototype: int scanf( const char, *format, . . . ) ; Semantics n n n Returns number of variables assigned values or EOF if an error. Argument list expressions are pointer expressions converted according to the corresponding formatter in the control string. Standard input file stdin (keyboard) supplies the input stream. (C) Doug Bowman, Virginia Tech, 2001 22
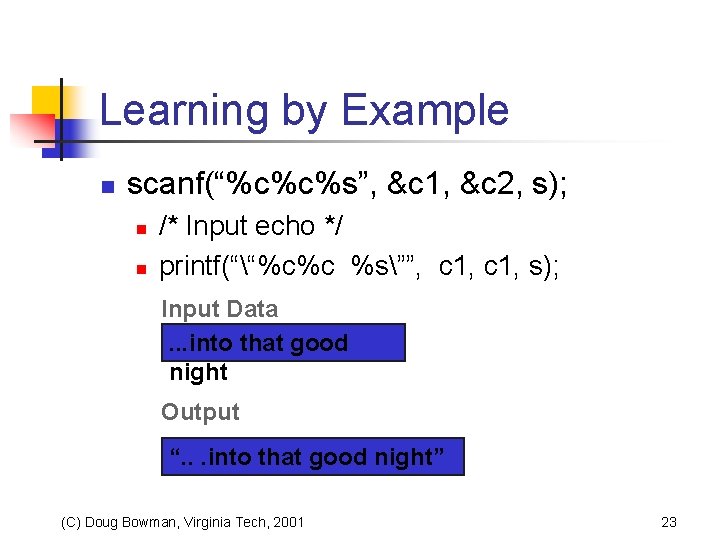
Learning by Example n scanf(“%c%c%s”, &c 1, &c 2, s); n n /* Input echo */ printf(““%c%c %s””, c 1, s); Input Data. . . into that good night Output “. . . into that good night” (C) Doug Bowman, Virginia Tech, 2001 23
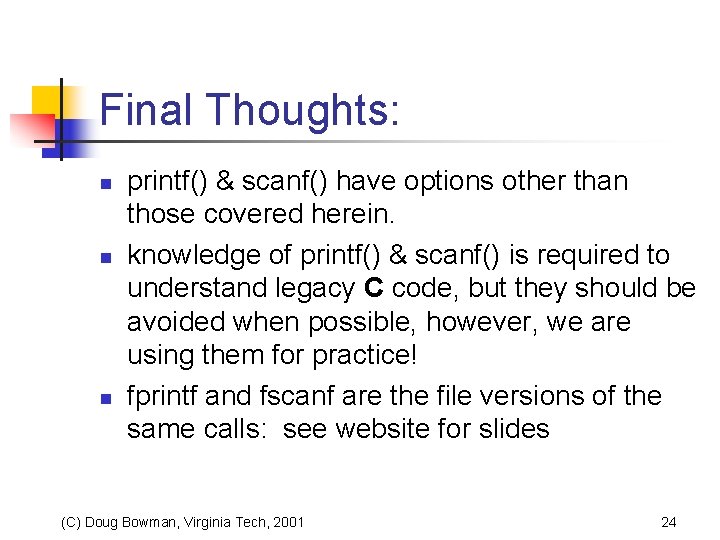
Final Thoughts: n n n printf() & scanf() have options other than those covered herein. knowledge of printf() & scanf() is required to understand legacy C code, but they should be avoided when possible, however, we are using them for practice! fprintf and fscanf are the file versions of the same calls: see website for slides (C) Doug Bowman, Virginia Tech, 2001 24
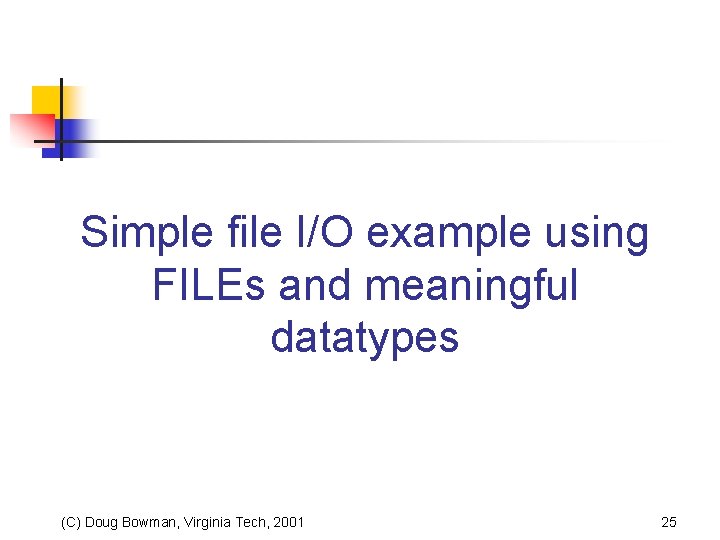
Simple file I/O example using FILEs and meaningful datatypes (C) Doug Bowman, Virginia Tech, 2001 25