Structures EKT 120 Computer Programming 1 Outline n
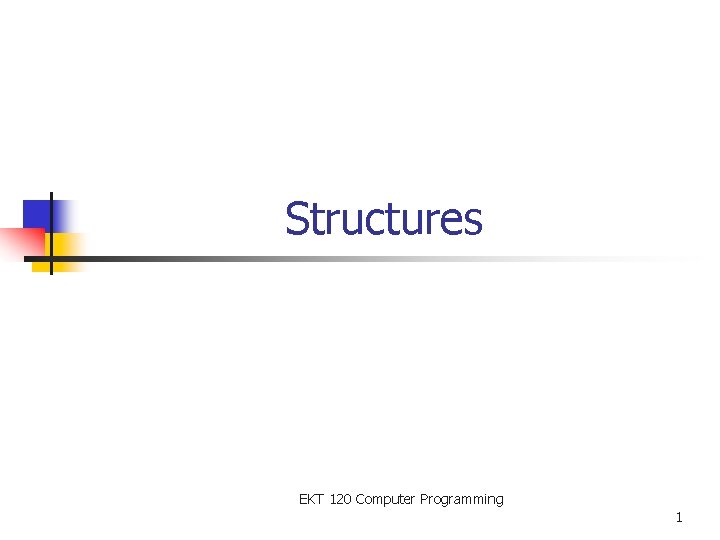
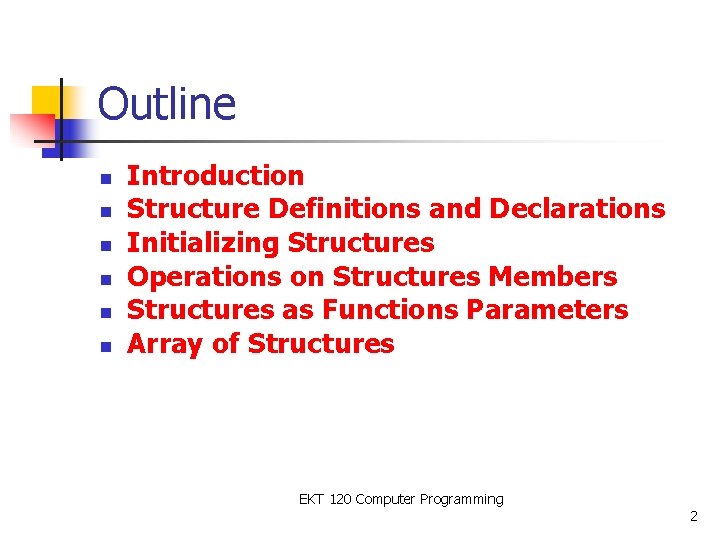
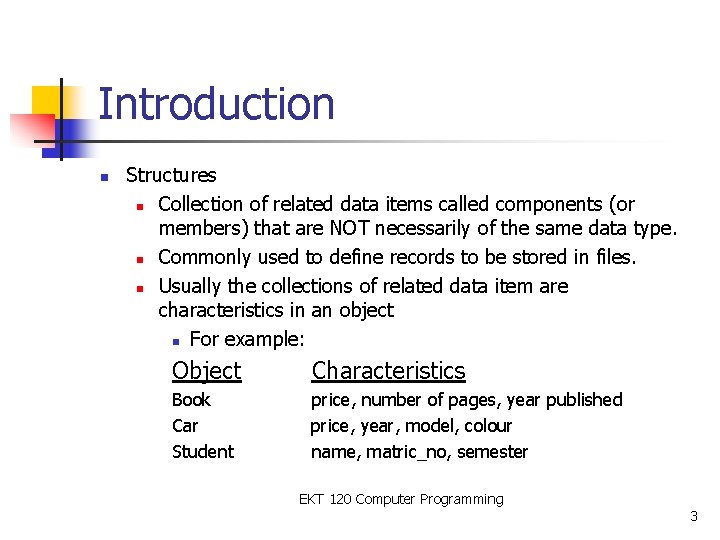
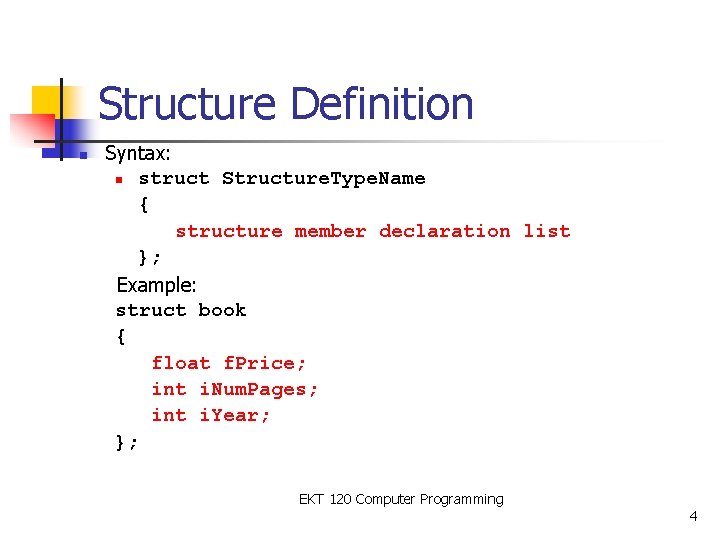
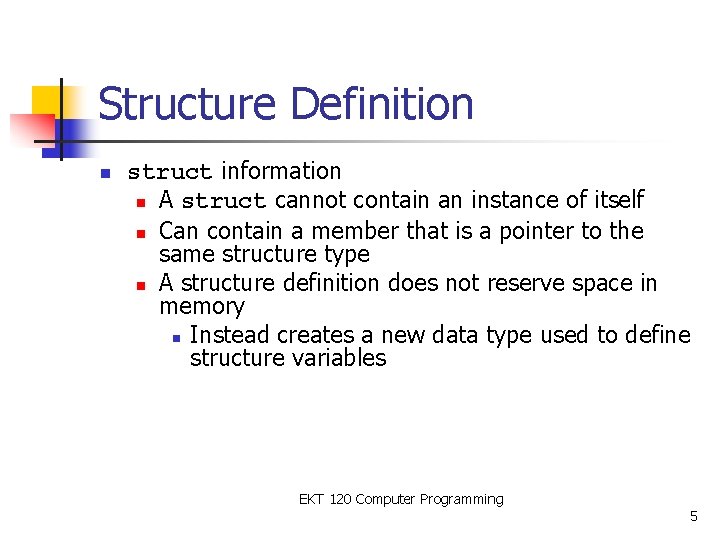
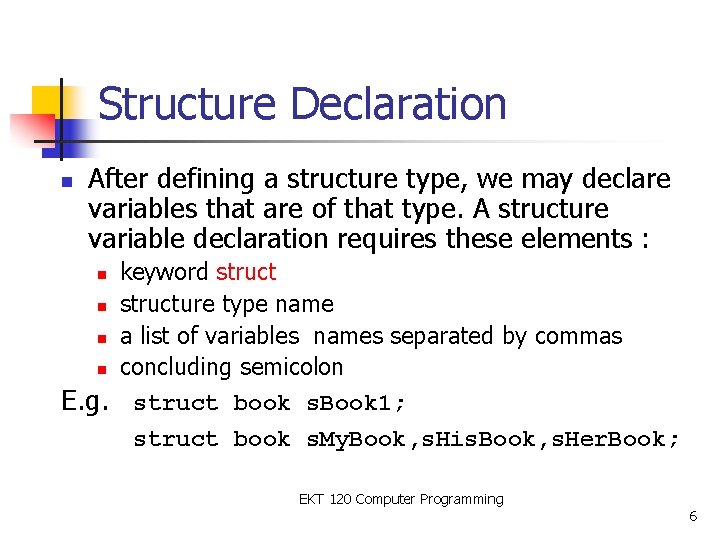
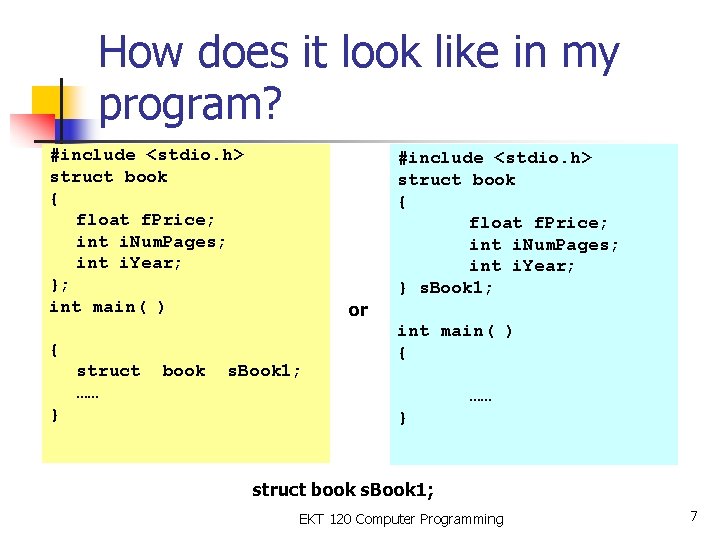
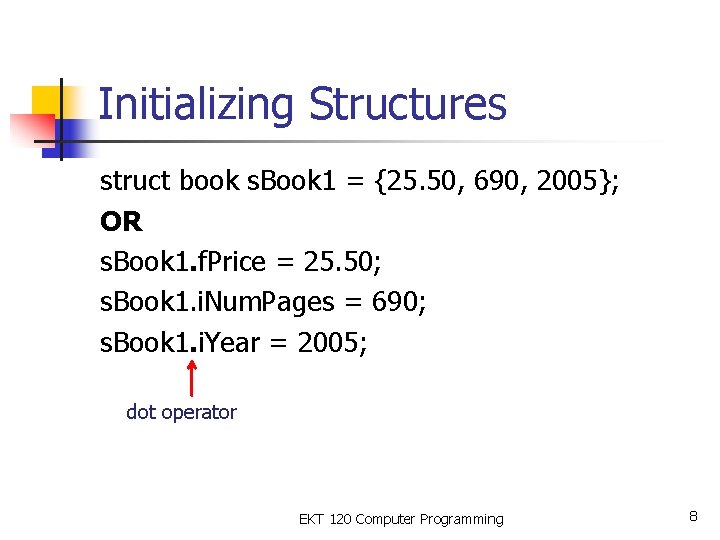
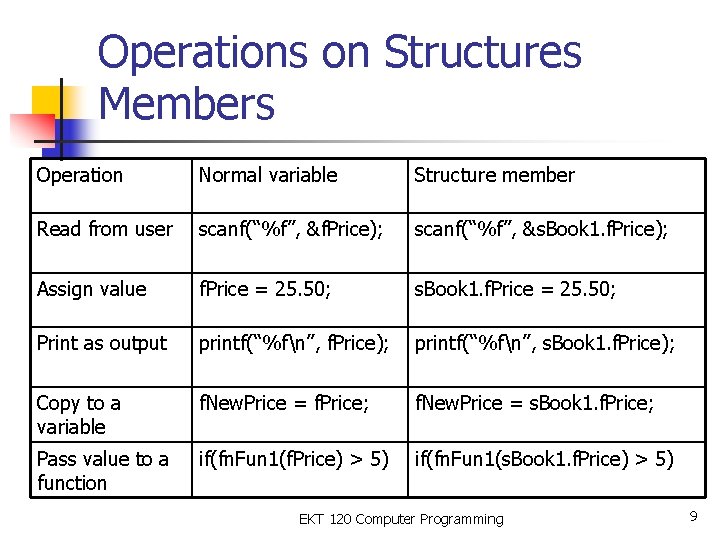
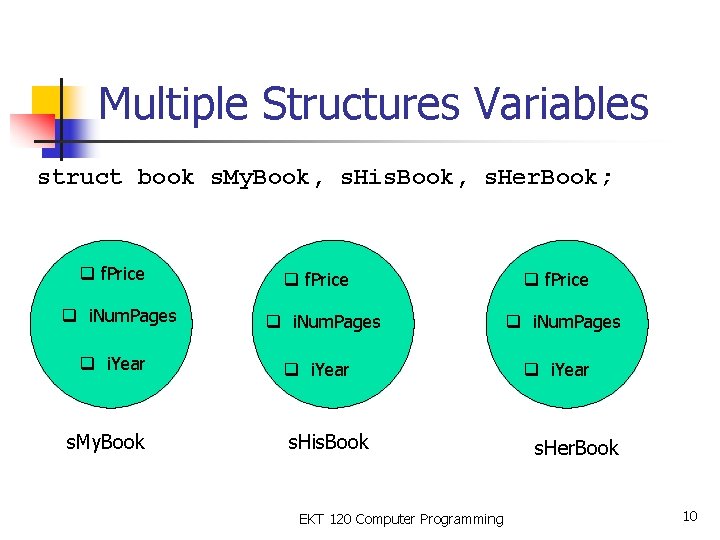
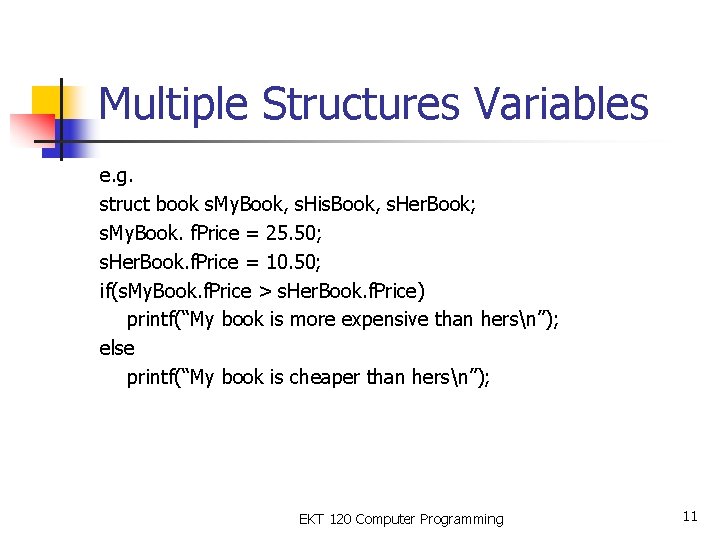
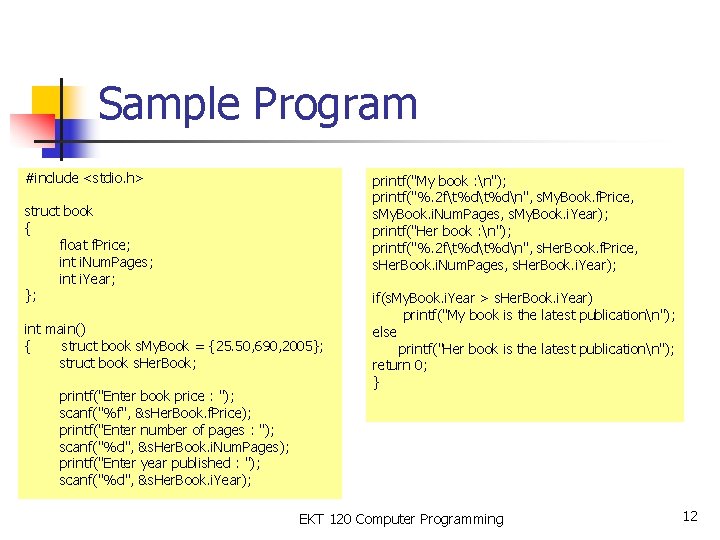
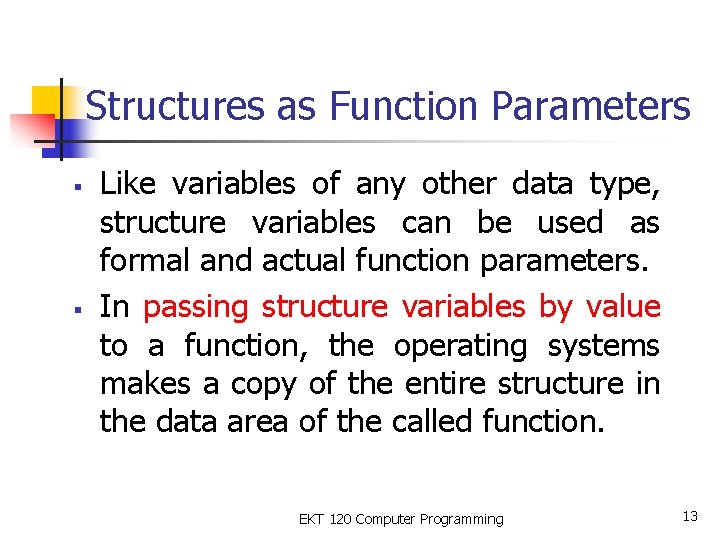
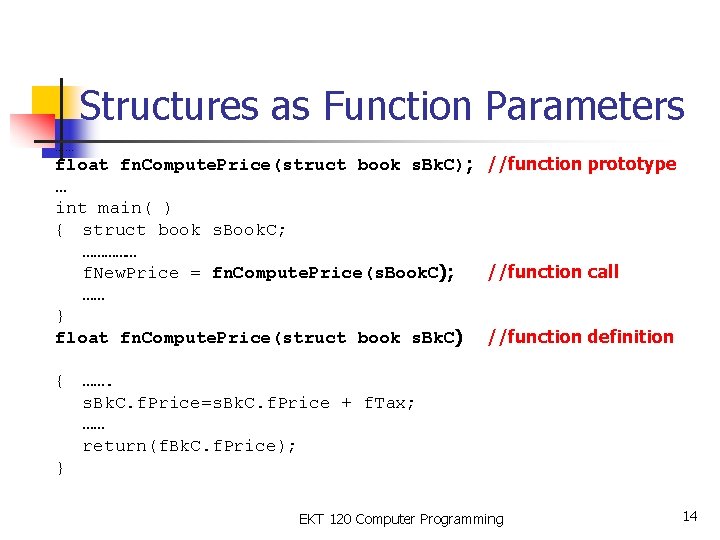
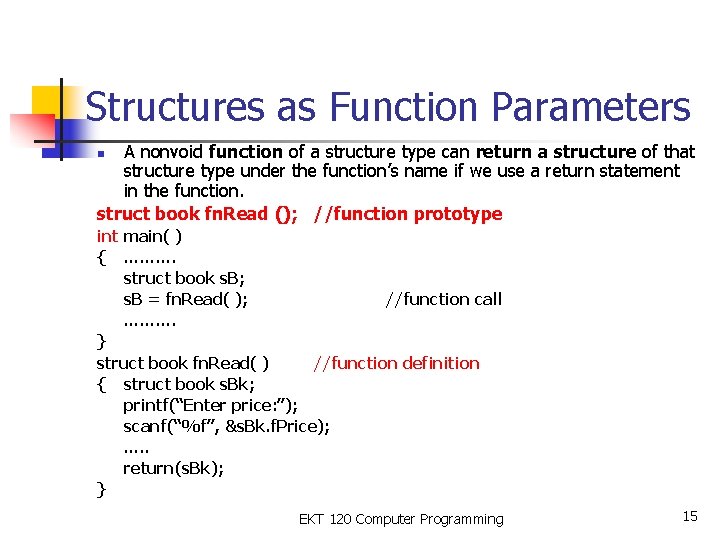
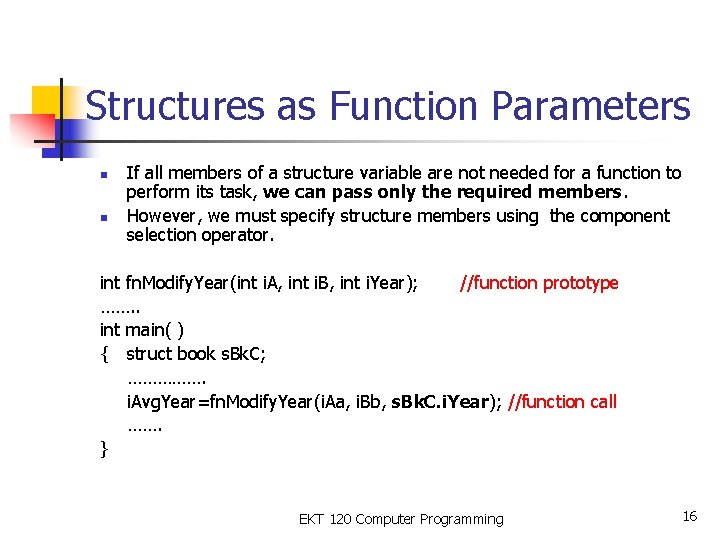
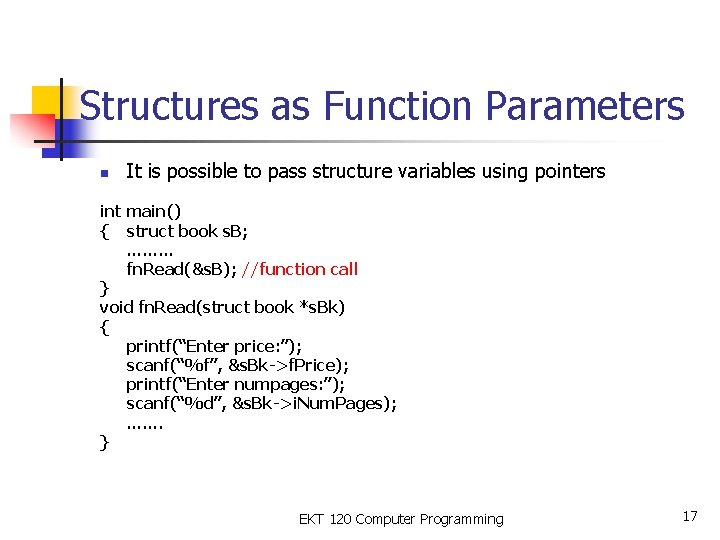
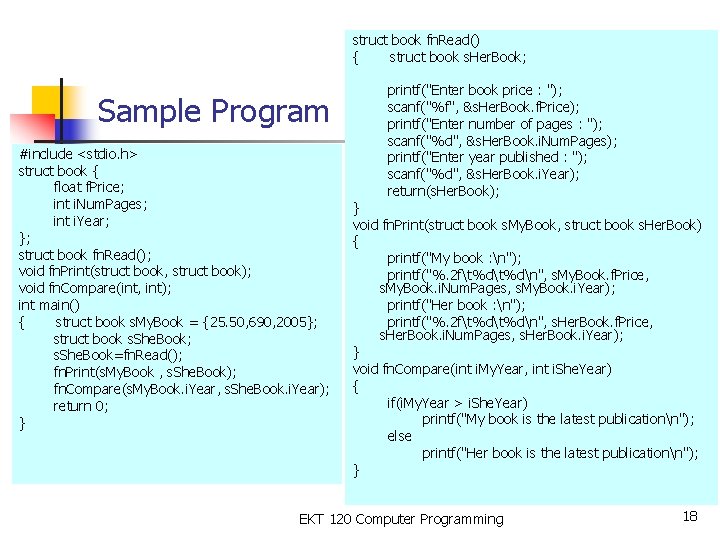
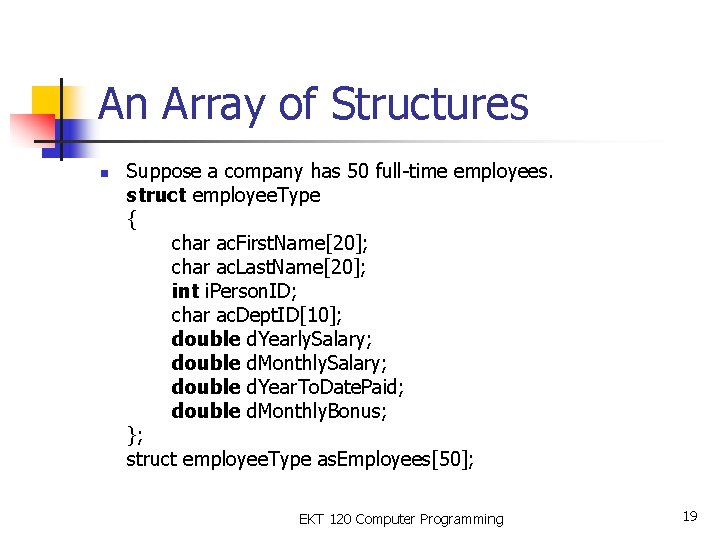
![How it looks like Array of structs as. Employees [0] [1] [2]. . . How it looks like Array of structs as. Employees [0] [1] [2]. . .](https://slidetodoc.com/presentation_image/e7274ca19b6b7994810fdc840719f049/image-20.jpg)
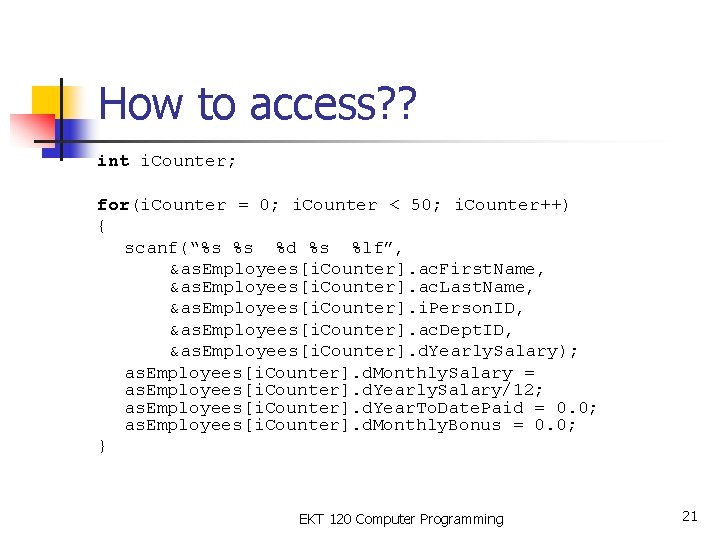
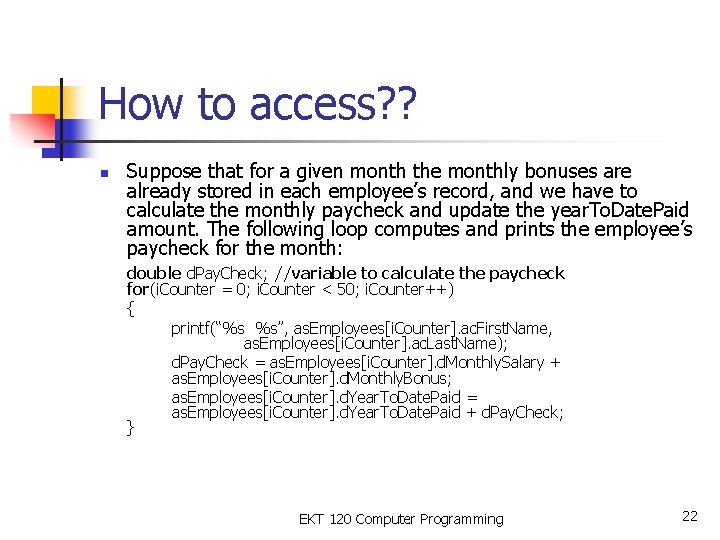
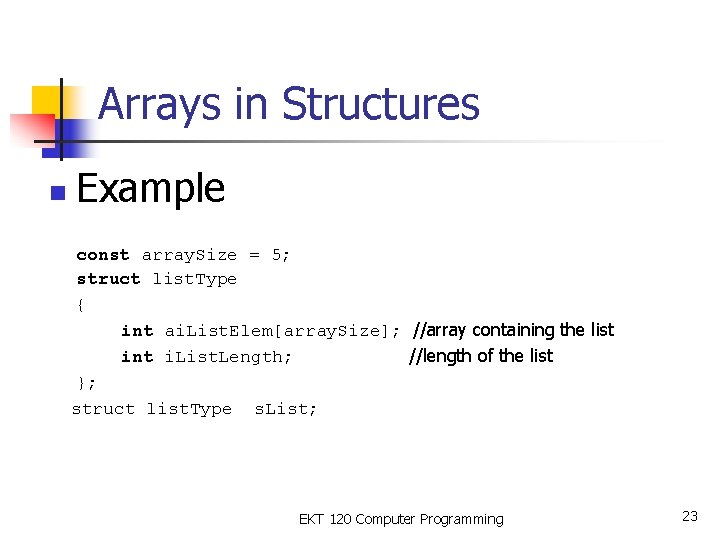
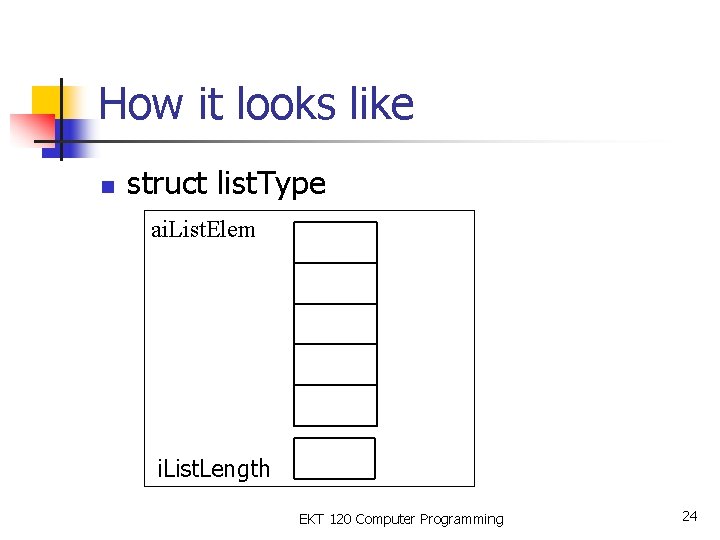
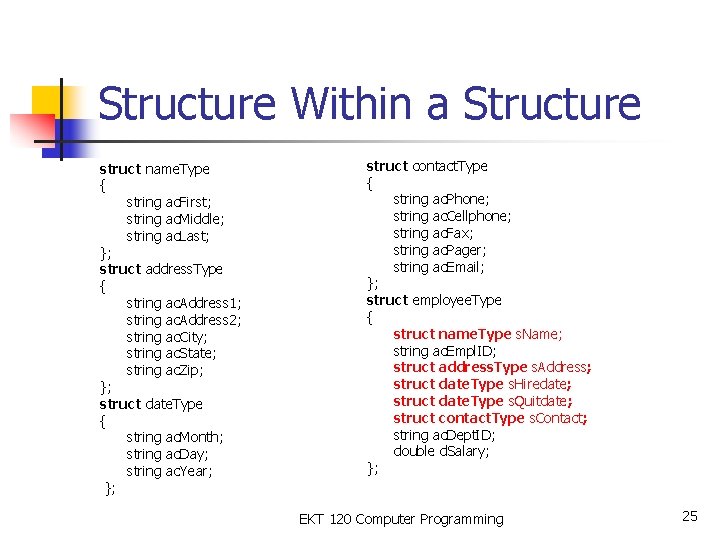
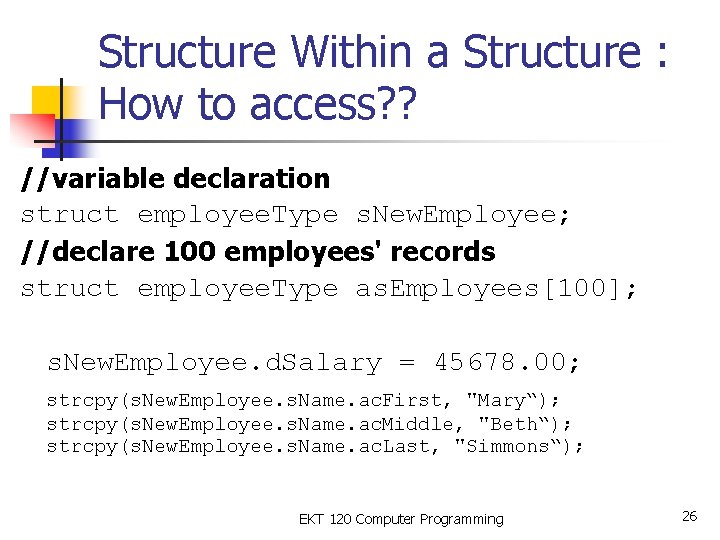
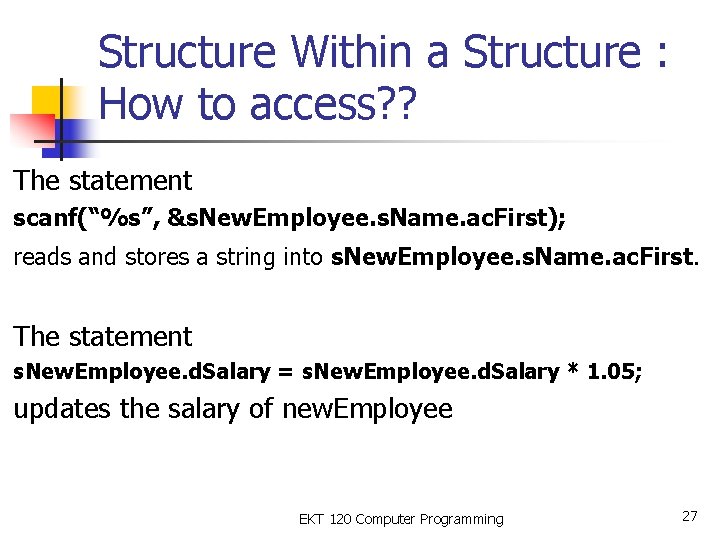
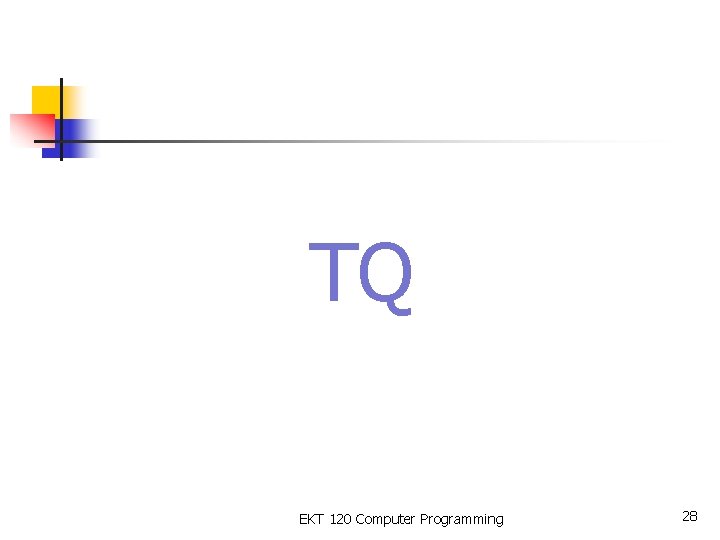
- Slides: 28
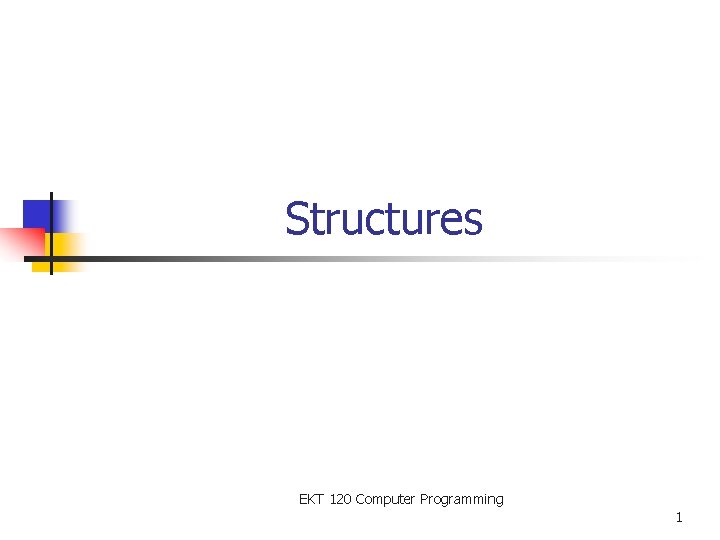
Structures EKT 120 Computer Programming 1
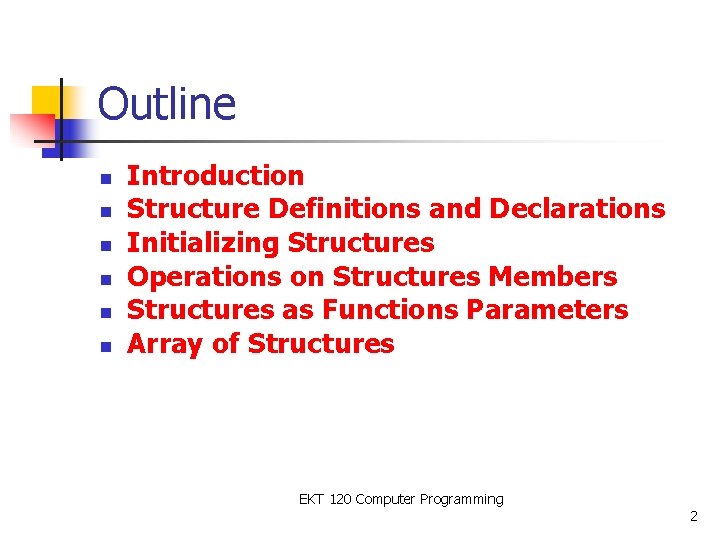
Outline n n n Introduction Structure Definitions and Declarations Initializing Structures Operations on Structures Members Structures as Functions Parameters Array of Structures EKT 120 Computer Programming 2
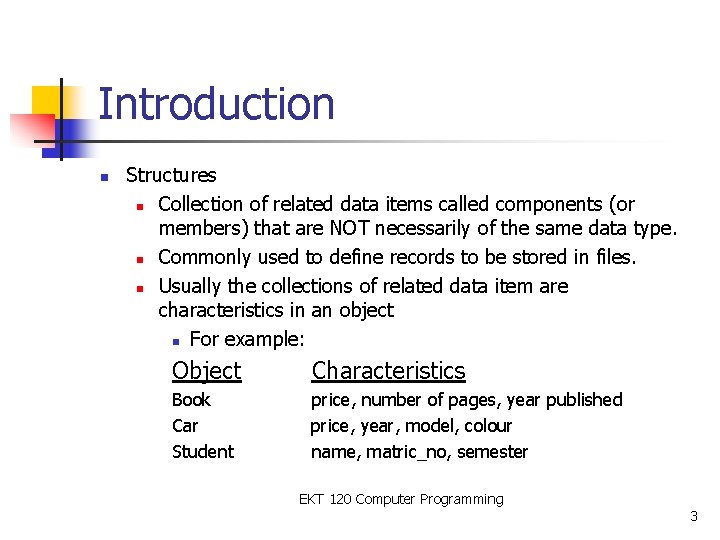
Introduction n Structures n Collection of related data items called components (or members) that are NOT necessarily of the same data type. n Commonly used to define records to be stored in files. n Usually the collections of related data item are characteristics in an object n For example: Object Characteristics Book Car Student price, number of pages, year published price, year, model, colour name, matric_no, semester EKT 120 Computer Programming 3
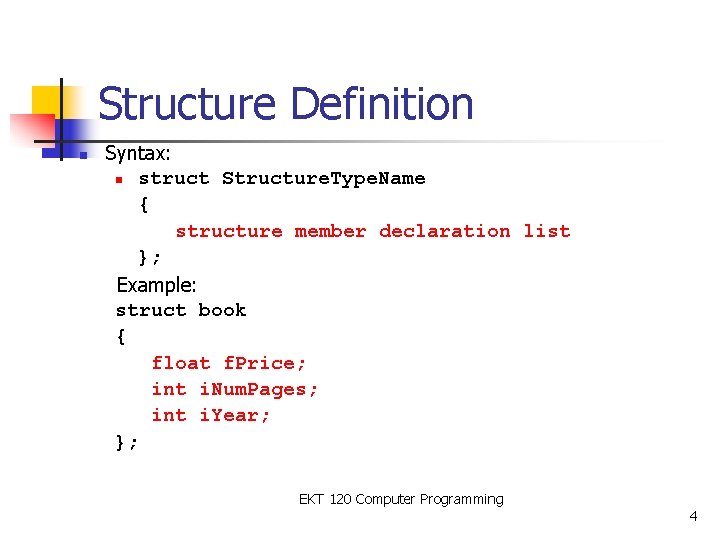
Structure Definition n Syntax: n struct Structure. Type. Name { structure member declaration list }; Example: struct book { float f. Price; int i. Num. Pages; int i. Year; }; EKT 120 Computer Programming 4
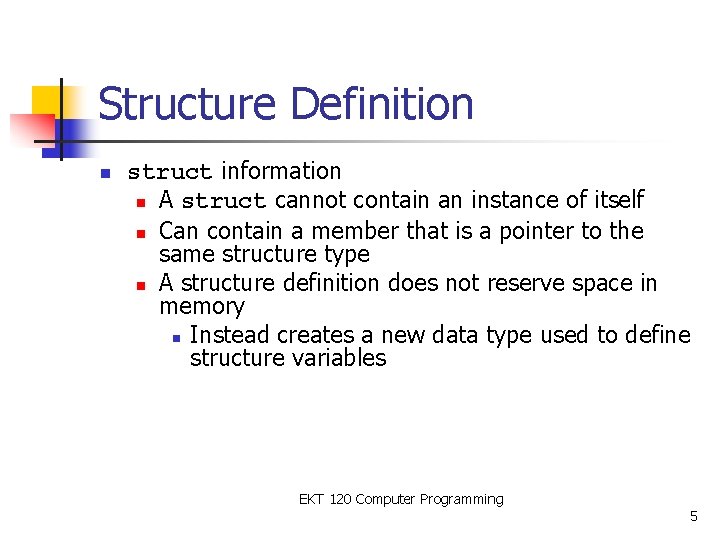
Structure Definition n struct information n A struct cannot contain an instance of itself n Can contain a member that is a pointer to the same structure type n A structure definition does not reserve space in memory n Instead creates a new data type used to define structure variables EKT 120 Computer Programming 5
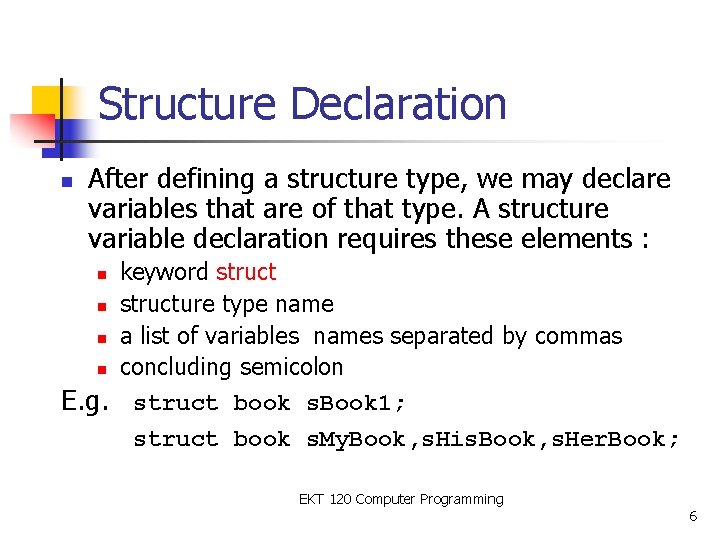
Structure Declaration n After defining a structure type, we may declare variables that are of that type. A structure variable declaration requires these elements : keyword struct n structure type name n a list of variables names separated by commas n concluding semicolon E. g. struct book s. Book 1; struct book s. My. Book, s. His. Book, s. Her. Book; n EKT 120 Computer Programming 6
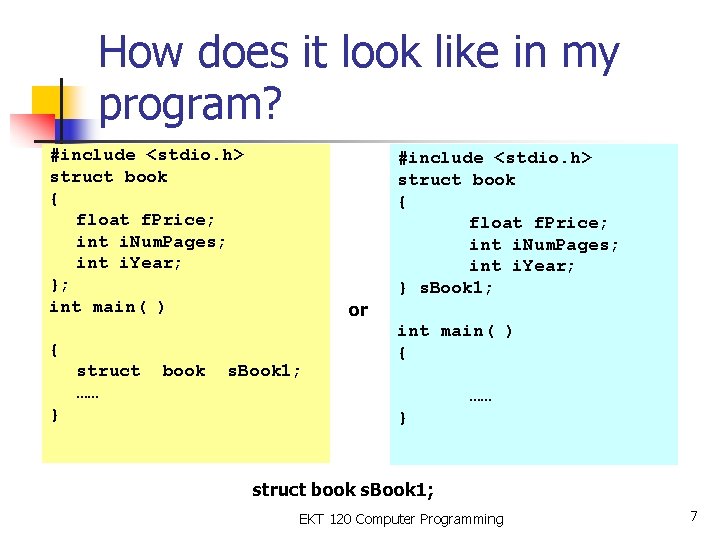
How does it look like in my program? #include <stdio. h> struct book { float f. Price; int i. Num. Pages; int i. Year; }; int main( ) or { struct …… } book s. Book 1; #include <stdio. h> struct book { float f. Price; int i. Num. Pages; int i. Year; } s. Book 1; int main( ) { …… } struct book s. Book 1; EKT 120 Computer Programming 7
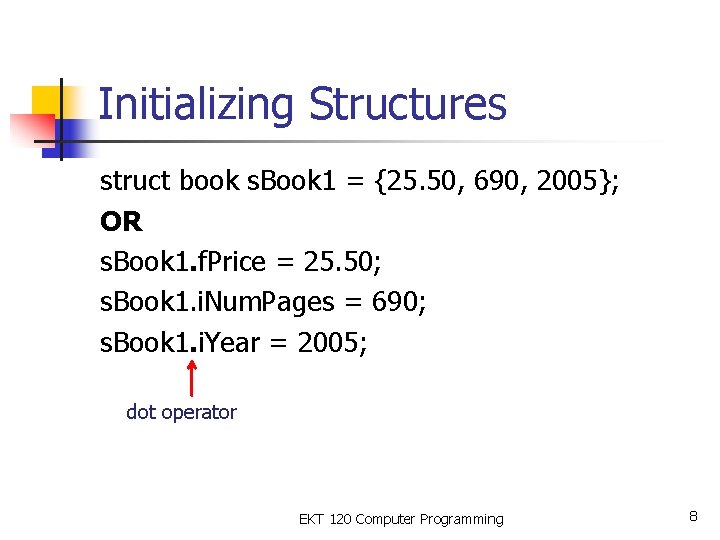
Initializing Structures struct book s. Book 1 = {25. 50, 690, 2005}; OR s. Book 1. f. Price = 25. 50; s. Book 1. i. Num. Pages = 690; s. Book 1. i. Year = 2005; dot operator EKT 120 Computer Programming 8
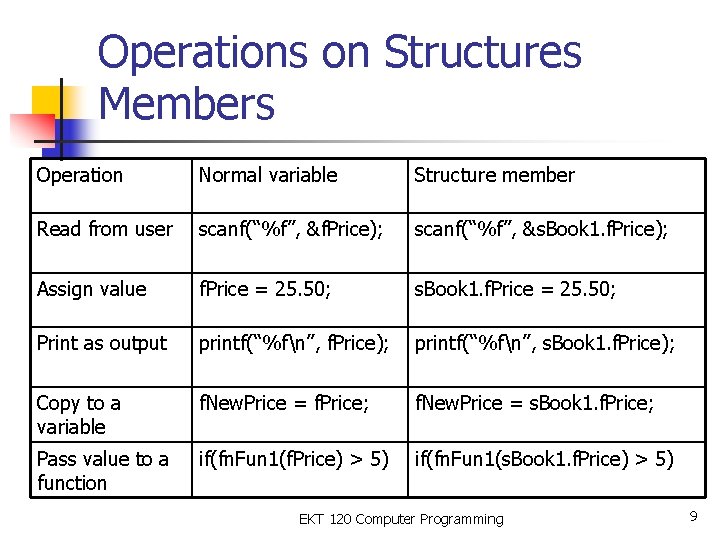
Operations on Structures Members Operation Normal variable Structure member Read from user scanf(“%f”, &f. Price); scanf(“%f”, &s. Book 1. f. Price); Assign value f. Price = 25. 50; s. Book 1. f. Price = 25. 50; Print as output printf(“%fn”, f. Price); printf(“%fn”, s. Book 1. f. Price); Copy to a variable f. New. Price = f. Price; f. New. Price = s. Book 1. f. Price; Pass value to a function if(fn. Fun 1(f. Price) > 5) if(fn. Fun 1(s. Book 1. f. Price) > 5) EKT 120 Computer Programming 9
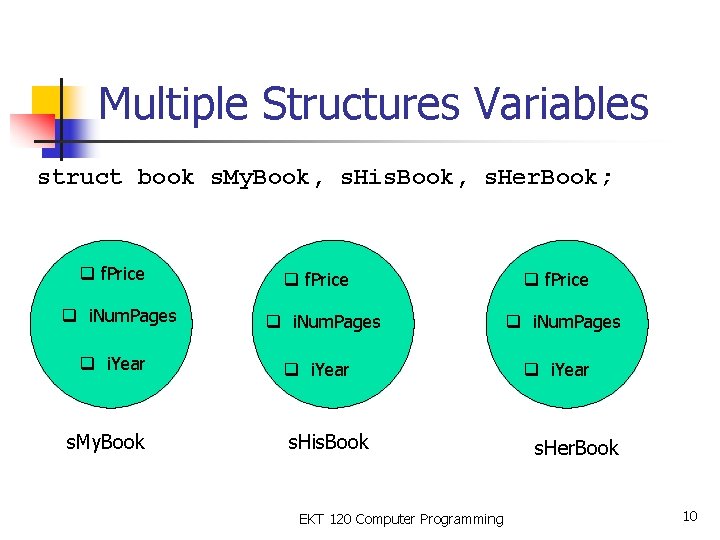
Multiple Structures Variables struct book s. My. Book, s. His. Book, s. Her. Book; q f. Price q i. Num. Pages q i. Year s. My. Book q f. Price q i. Num. Pages q i. Year s. His. Book EKT 120 Computer Programming q f. Price q i. Num. Pages q i. Year s. Her. Book 10
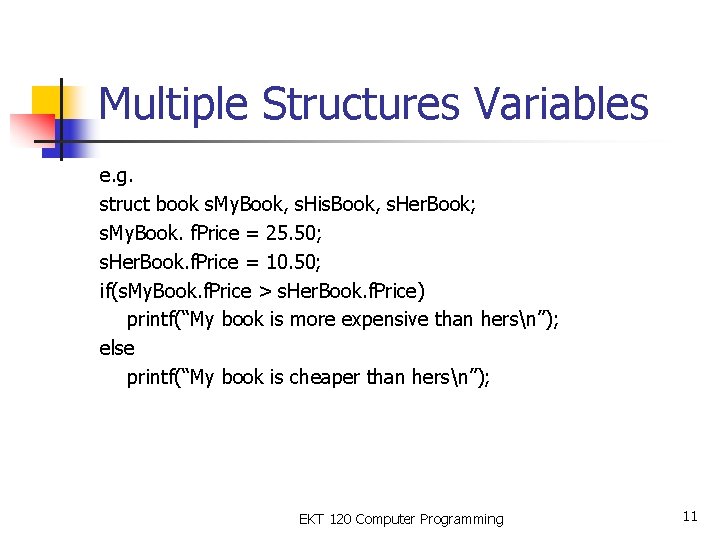
Multiple Structures Variables e. g. struct book s. My. Book, s. His. Book, s. Her. Book; s. My. Book. f. Price = 25. 50; s. Her. Book. f. Price = 10. 50; if(s. My. Book. f. Price > s. Her. Book. f. Price) printf(“My book is more expensive than hersn”); else printf(“My book is cheaper than hersn”); EKT 120 Computer Programming 11
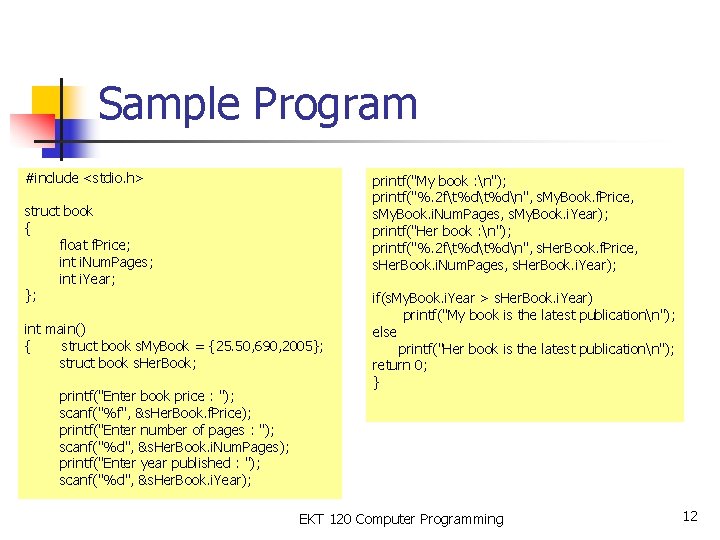
Sample Program #include <stdio. h> printf("My book : n"); printf("%. 2 ft%dn", s. My. Book. f. Price, s. My. Book. i. Num. Pages, s. My. Book. i. Year); printf("Her book : n"); printf("%. 2 ft%dn", s. Her. Book. f. Price, s. Her. Book. i. Num. Pages, s. Her. Book. i. Year); struct book { float f. Price; int i. Num. Pages; int i. Year; }; int main() { struct book s. My. Book = {25. 50, 690, 2005}; struct book s. Her. Book; printf("Enter book price : "); scanf("%f", &s. Her. Book. f. Price); printf("Enter number of pages : "); scanf("%d", &s. Her. Book. i. Num. Pages); printf("Enter year published : "); scanf("%d", &s. Her. Book. i. Year); if(s. My. Book. i. Year > s. Her. Book. i. Year) printf("My book is the latest publicationn"); else printf("Her book is the latest publicationn"); return 0; } EKT 120 Computer Programming 12
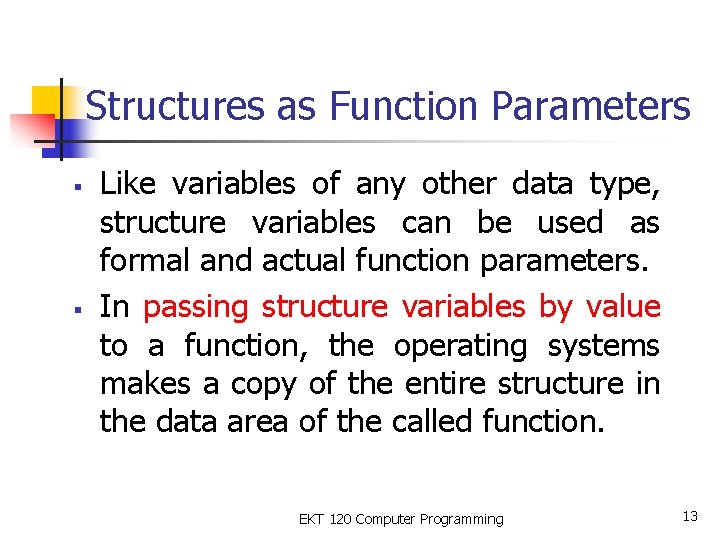
Structures as Function Parameters § § Like variables of any other data type, structure variables can be used as formal and actual function parameters. In passing structure variables by value to a function, the operating systems makes a copy of the entire structure in the data area of the called function. EKT 120 Computer Programming 13
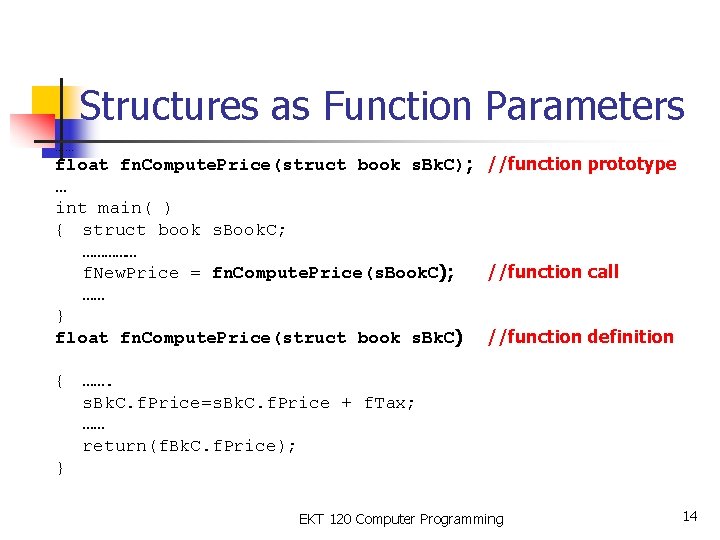
Structures as Function Parameters …… float fn. Compute. Price(struct book s. Bk. C); //function prototype … int main( ) { struct book s. Book. C; …………… f. New. Price = fn. Compute. Price(s. Book. C); //function call …… } float fn. Compute. Price(struct book s. Bk. C) //function definition { ……. s. Bk. C. f. Price=s. Bk. C. f. Price + f. Tax; …… return(f. Bk. C. f. Price); } EKT 120 Computer Programming 14
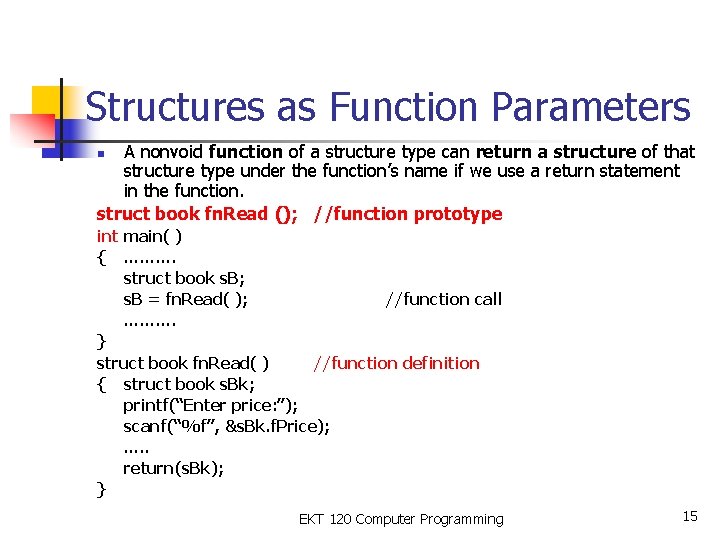
Structures as Function Parameters A nonvoid function of a structure type can return a structure of that structure type under the function’s name if we use a return statement in the function. struct book fn. Read (); //function prototype n int main( ) { ………. struct book s. B; s. B = fn. Read( ); //function call ………. } struct book fn. Read( ) //function definition { struct book s. Bk; printf(“Enter price: ”); scanf(“%f”, &s. Bk. f. Price); …. . return(s. Bk); } EKT 120 Computer Programming 15
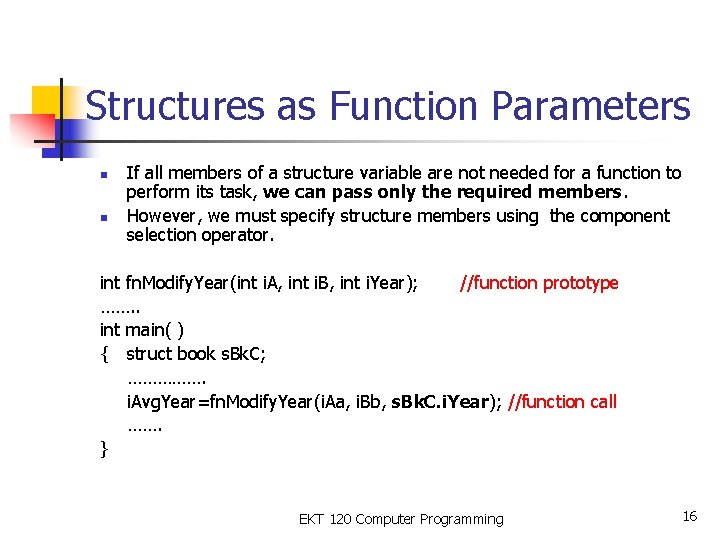
Structures as Function Parameters n n If all members of a structure variable are not needed for a function to perform its task, we can pass only the required members. However, we must specify structure members using the component selection operator. int fn. Modify. Year(int i. A, int i. B, int i. Year); //function prototype ……. . int main( ) { struct book s. Bk. C; ……………. i. Avg. Year=fn. Modify. Year(i. Aa, i. Bb, s. Bk. C. i. Year); //function call ……. } EKT 120 Computer Programming 16
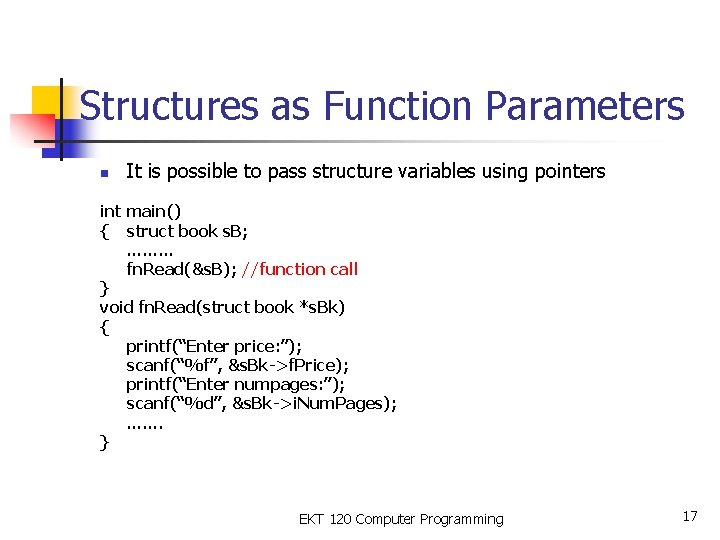
Structures as Function Parameters n It is possible to pass structure variables using pointers int main() { struct book s. B; ……… fn. Read(&s. B); //function call } void fn. Read(struct book *s. Bk) { printf(“Enter price: ”); scanf(“%f”, &s. Bk->f. Price); printf(“Enter numpages: ”); scanf(“%d”, &s. Bk->i. Num. Pages); ……. } EKT 120 Computer Programming 17
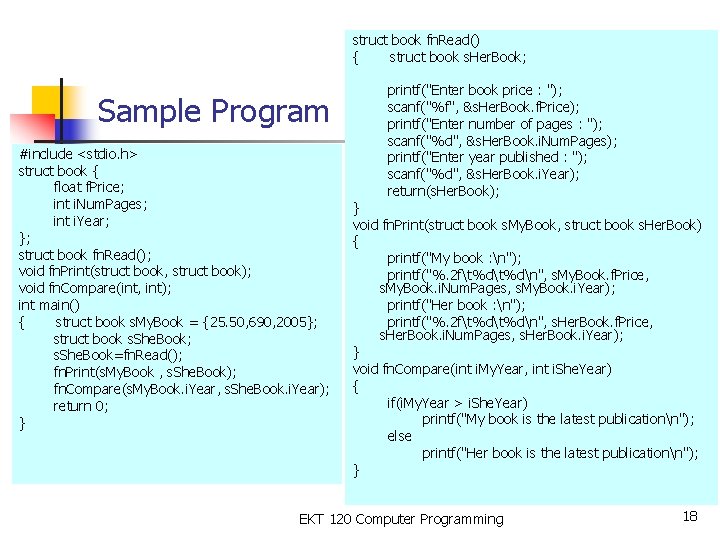
struct book fn. Read() { struct book s. Her. Book; Sample Program #include <stdio. h> struct book { float f. Price; int i. Num. Pages; int i. Year; }; struct book fn. Read(); void fn. Print(struct book, struct book); void fn. Compare(int, int); int main() { struct book s. My. Book = {25. 50, 690, 2005}; struct book s. She. Book; s. She. Book=fn. Read(); fn. Print(s. My. Book , s. She. Book); fn. Compare(s. My. Book. i. Year, s. She. Book. i. Year); return 0; } printf("Enter book price : "); scanf("%f", &s. Her. Book. f. Price); printf("Enter number of pages : "); scanf("%d", &s. Her. Book. i. Num. Pages); printf("Enter year published : "); scanf("%d", &s. Her. Book. i. Year); return(s. Her. Book); } void fn. Print(struct book s. My. Book, struct book s. Her. Book) { printf("My book : n"); printf("%. 2 ft%dn", s. My. Book. f. Price, s. My. Book. i. Num. Pages, s. My. Book. i. Year); printf("Her book : n"); printf("%. 2 ft%dn", s. Her. Book. f. Price, s. Her. Book. i. Num. Pages, s. Her. Book. i. Year); } void fn. Compare(int i. My. Year, int i. She. Year) { if(i. My. Year > i. She. Year) printf("My book is the latest publicationn"); else printf("Her book is the latest publicationn"); } EKT 120 Computer Programming 18
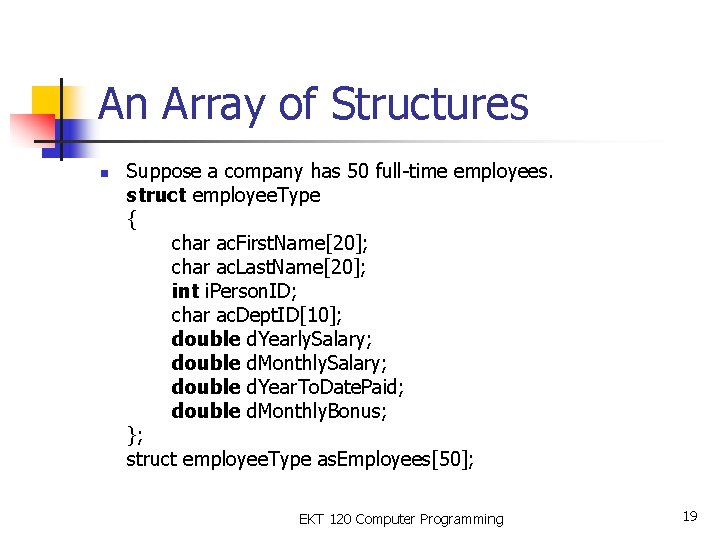
An Array of Structures n Suppose a company has 50 full-time employees. struct employee. Type { char ac. First. Name[20]; char ac. Last. Name[20]; int i. Person. ID; char ac. Dept. ID[10]; double d. Yearly. Salary; double d. Monthly. Salary; double d. Year. To. Date. Paid; double d. Monthly. Bonus; }; struct employee. Type as. Employees[50]; EKT 120 Computer Programming 19
![How it looks like Array of structs as Employees 0 1 2 How it looks like Array of structs as. Employees [0] [1] [2]. . .](https://slidetodoc.com/presentation_image/e7274ca19b6b7994810fdc840719f049/image-20.jpg)
How it looks like Array of structs as. Employees [0] [1] [2]. . . [25] [26]. . . [48] [49] as. Employees[2] ac. First. Name ac. Last. Name i. Person. ID ac. Dept. ID d. Yearly. Salary d. Monthly. Salary d. Year. To. Date. Paid d. Monthly. Bonus EKT 120 Computer Programming 20
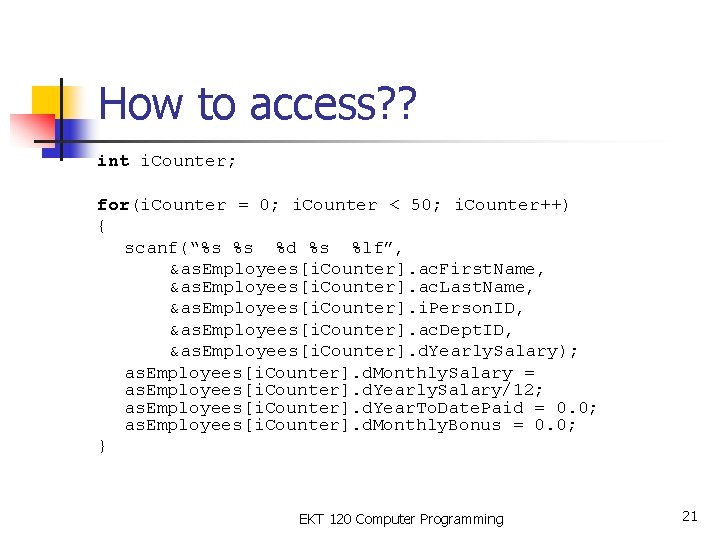
How to access? ? int i. Counter; for(i. Counter = 0; i. Counter < 50; i. Counter++) { scanf(“%s %s %d %s %lf”, &as. Employees[i. Counter]. ac. First. Name, &as. Employees[i. Counter]. ac. Last. Name, &as. Employees[i. Counter]. i. Person. ID, &as. Employees[i. Counter]. ac. Dept. ID, &as. Employees[i. Counter]. d. Yearly. Salary); as. Employees[i. Counter]. d. Monthly. Salary = as. Employees[i. Counter]. d. Yearly. Salary/12; as. Employees[i. Counter]. d. Year. To. Date. Paid = 0. 0; as. Employees[i. Counter]. d. Monthly. Bonus = 0. 0; } EKT 120 Computer Programming 21
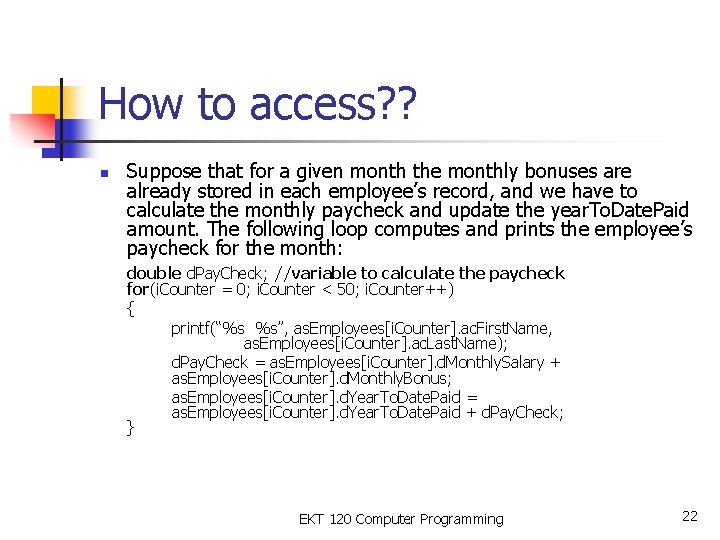
How to access? ? n Suppose that for a given month the monthly bonuses are already stored in each employee’s record, and we have to calculate the monthly paycheck and update the year. To. Date. Paid amount. The following loop computes and prints the employee’s paycheck for the month: double d. Pay. Check; //variable to calculate the paycheck for(i. Counter = 0; i. Counter < 50; i. Counter++) { printf(“%s %s”, as. Employees[i. Counter]. ac. First. Name, as. Employees[i. Counter]. ac. Last. Name); d. Pay. Check = as. Employees[i. Counter]. d. Monthly. Salary + as. Employees[i. Counter]. d. Monthly. Bonus; as. Employees[i. Counter]. d. Year. To. Date. Paid = as. Employees[i. Counter]. d. Year. To. Date. Paid + d. Pay. Check; } EKT 120 Computer Programming 22
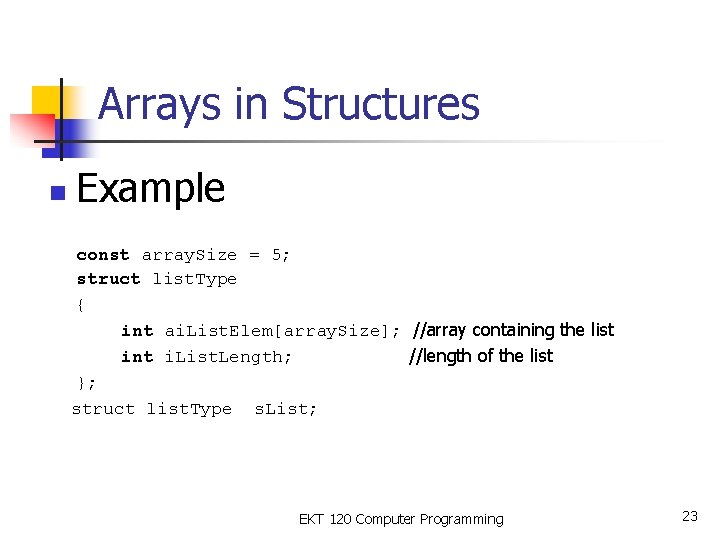
Arrays in Structures n Example const array. Size = 5; struct list. Type { int ai. List. Elem[array. Size]; //array containing the list int i. List. Length; //length of the list }; struct list. Type s. List; EKT 120 Computer Programming 23
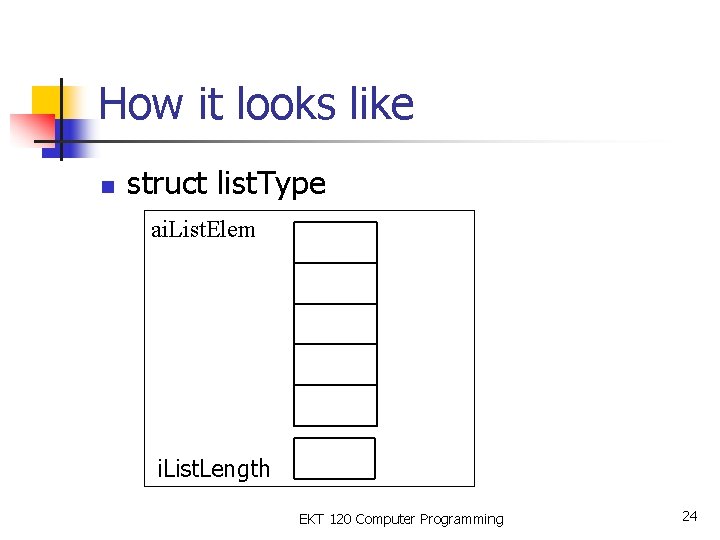
How it looks like n struct list. Type ai. List. Elem i. List. Length EKT 120 Computer Programming 24
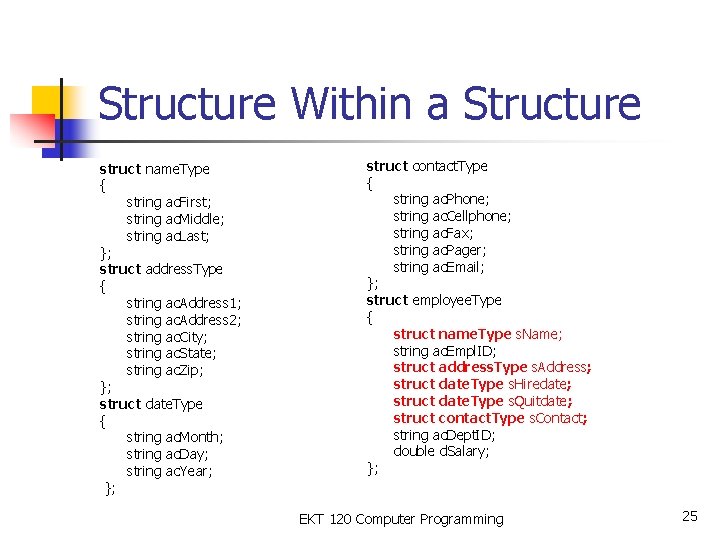
Structure Within a Structure struct name. Type { string ac. First; string ac. Middle; string ac. Last; }; struct address. Type { string ac. Address 1; string ac. Address 2; string ac. City; string ac. State; string ac. Zip; }; struct date. Type { string ac. Month; string ac. Day; string ac. Year; }; struct contact. Type { string ac. Phone; string ac. Cellphone; string ac. Fax; string ac. Pager; string ac. Email; }; struct employee. Type { struct name. Type s. Name; string ac. Empl. ID; struct address. Type s. Address; struct date. Type s. Hiredate; struct date. Type s. Quitdate; struct contact. Type s. Contact; string ac. Dept. ID; double d. Salary; }; EKT 120 Computer Programming 25
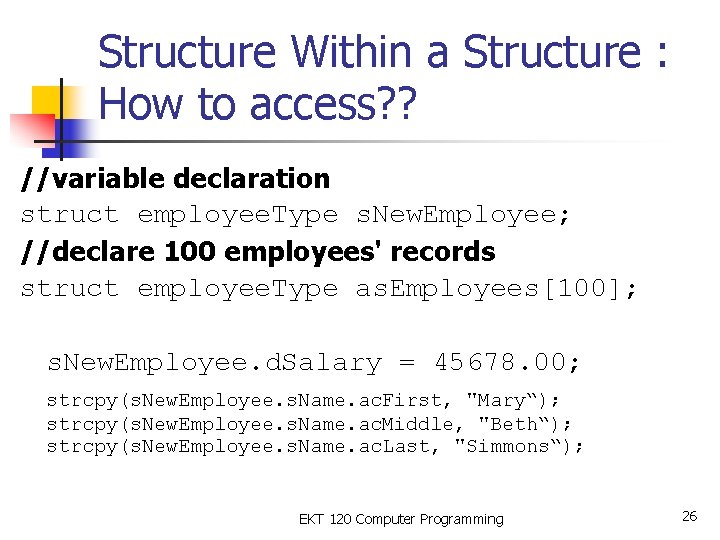
Structure Within a Structure : How to access? ? //variable declaration struct employee. Type s. New. Employee; //declare 100 employees' records struct employee. Type as. Employees[100]; s. New. Employee. d. Salary = 45678. 00; strcpy(s. New. Employee. s. Name. ac. First, "Mary“); strcpy(s. New. Employee. s. Name. ac. Middle, "Beth“); strcpy(s. New. Employee. s. Name. ac. Last, "Simmons“); EKT 120 Computer Programming 26
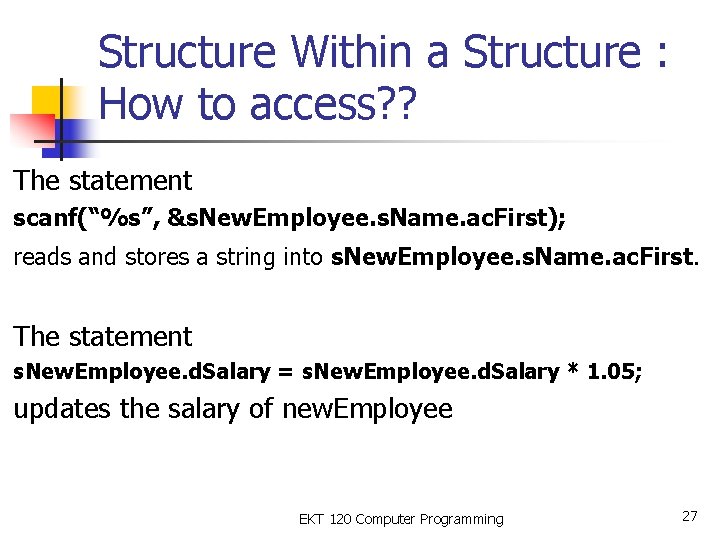
Structure Within a Structure : How to access? ? The statement scanf(“%s”, &s. New. Employee. s. Name. ac. First); reads and stores a string into s. New. Employee. s. Name. ac. First. The statement s. New. Employee. d. Salary = s. New. Employee. d. Salary * 1. 05; updates the salary of new. Employee EKT 120 Computer Programming 27
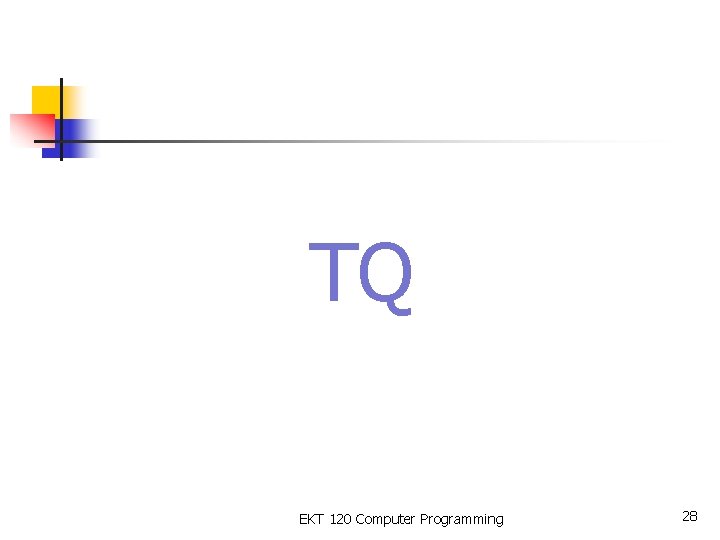
TQ EKT 120 Computer Programming 28