STRUCTURE OF ANGULAR PROJECTS STRUCTURE OF ANGULAR PROJECTS
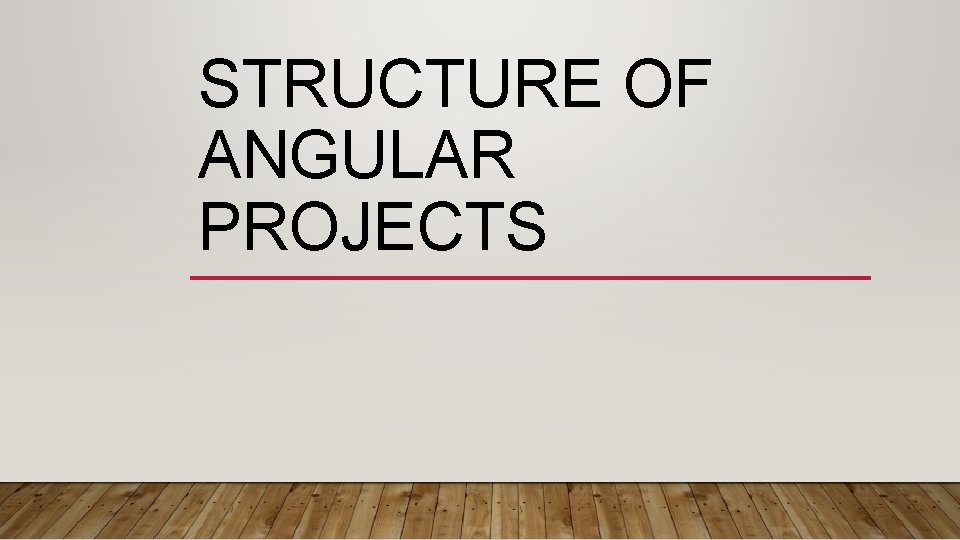
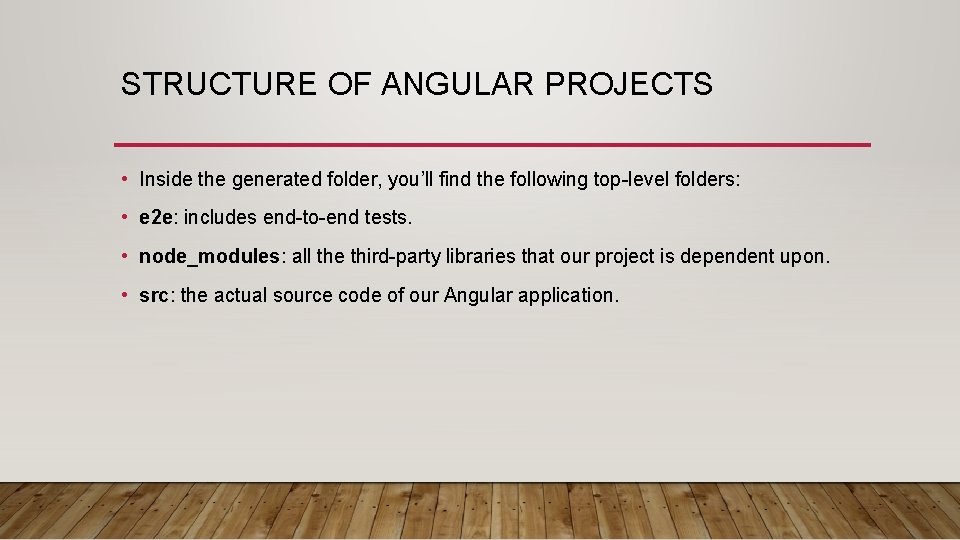
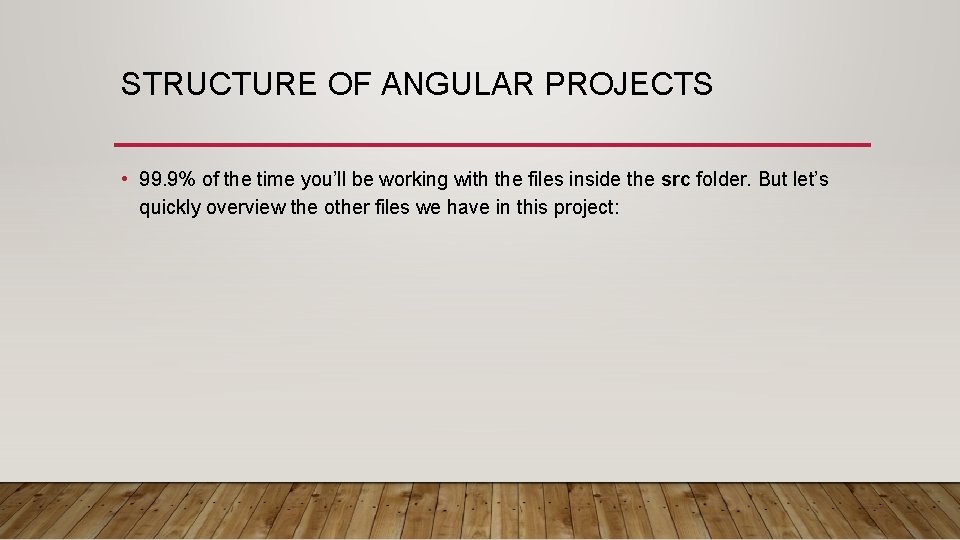
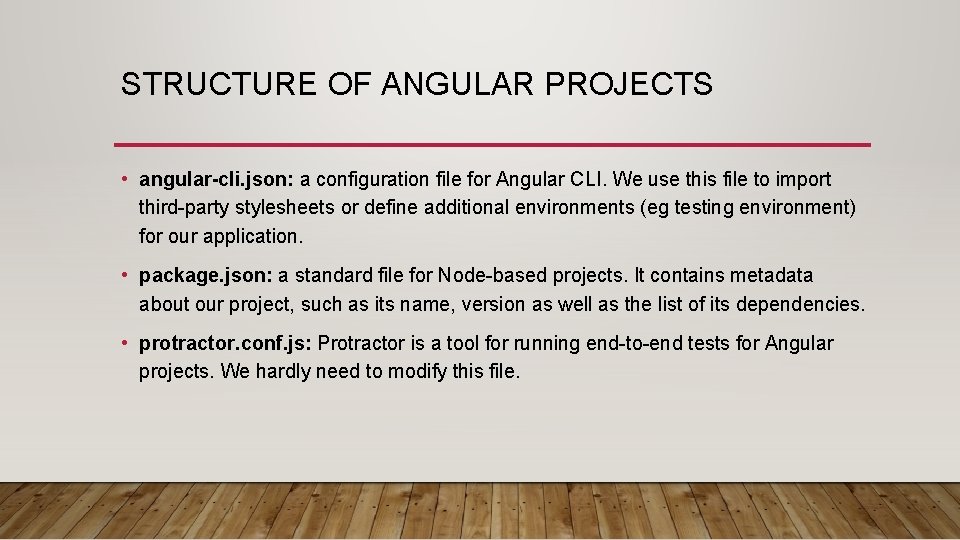
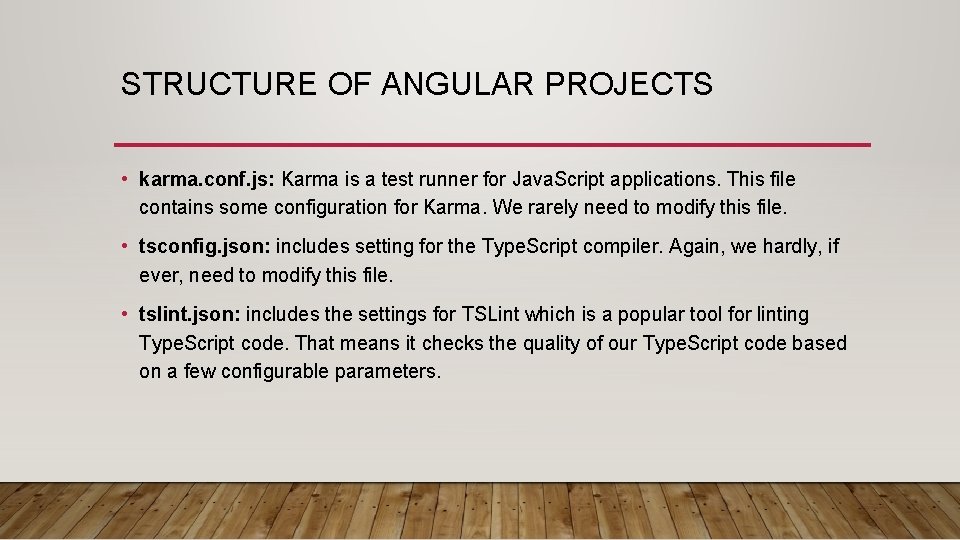
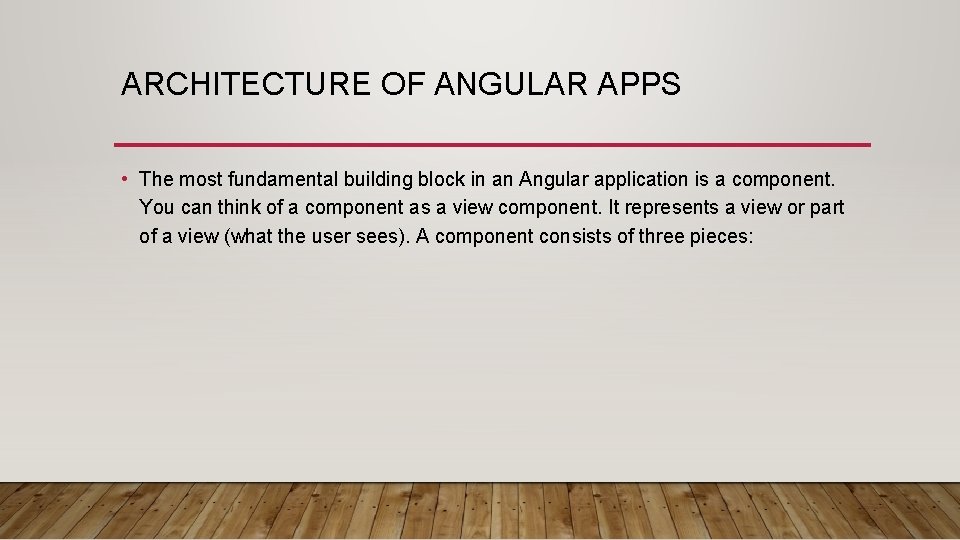
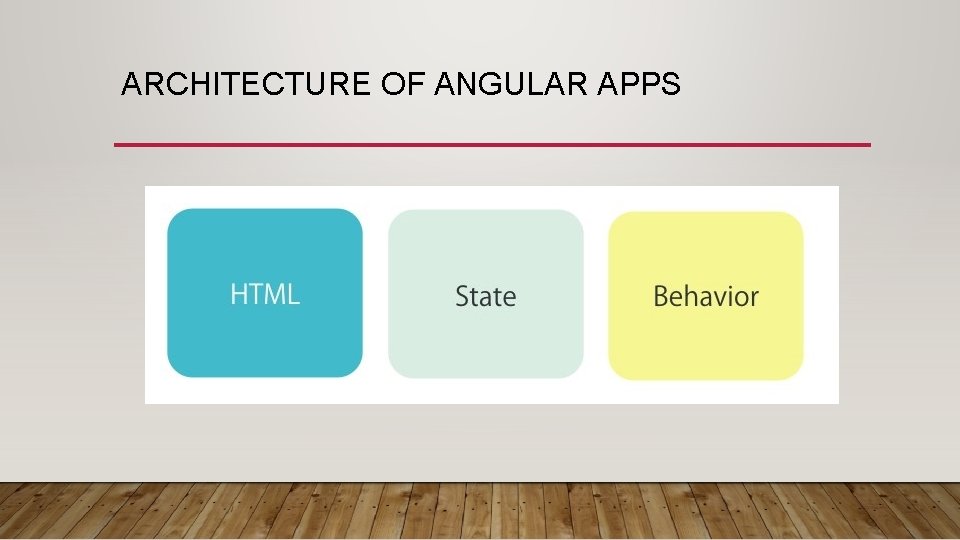
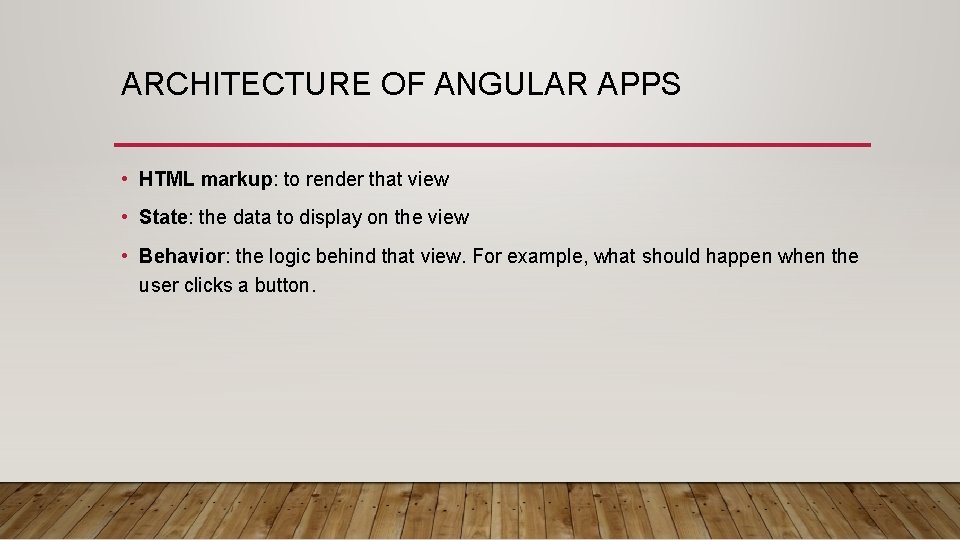
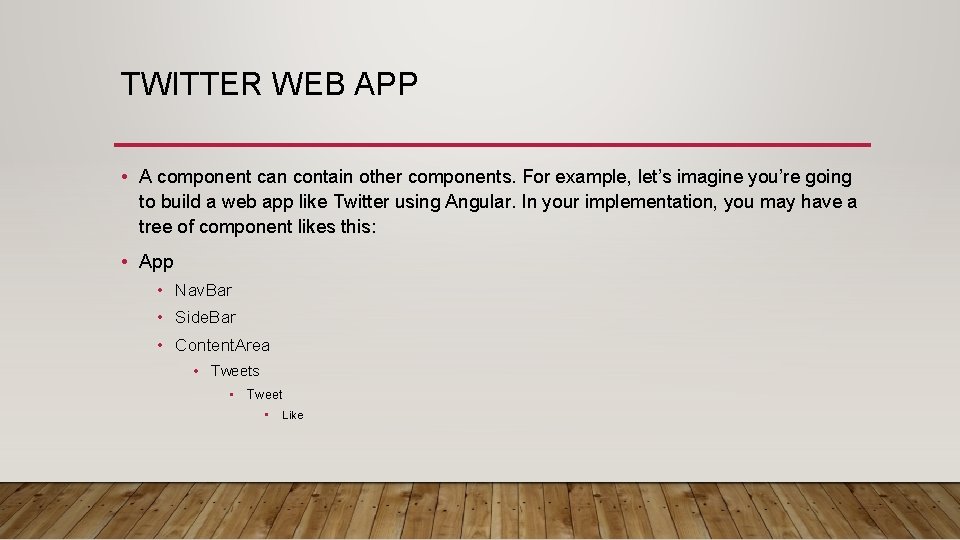
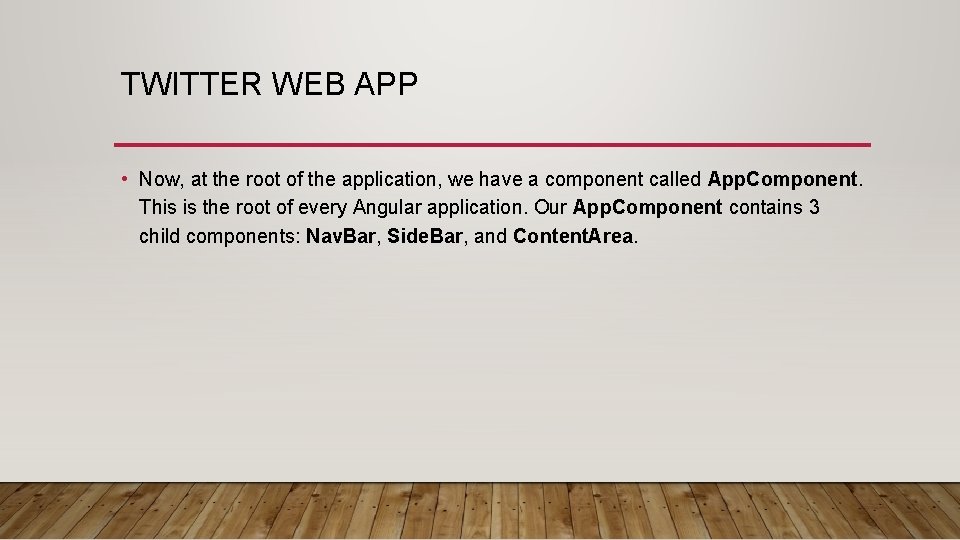
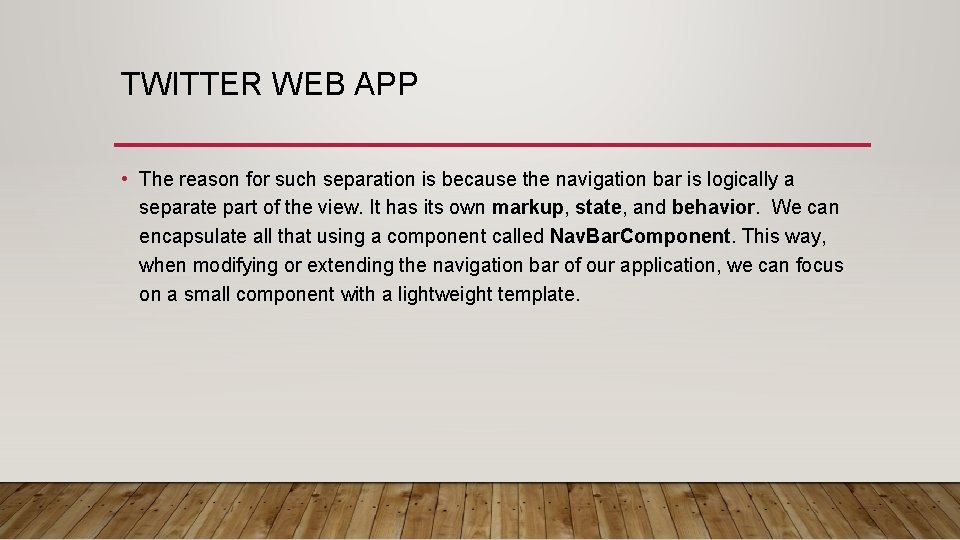
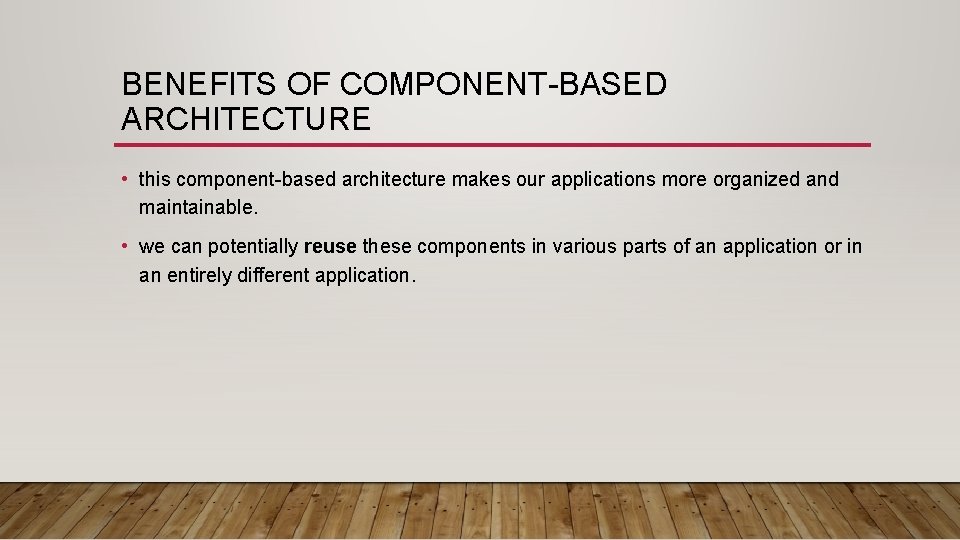
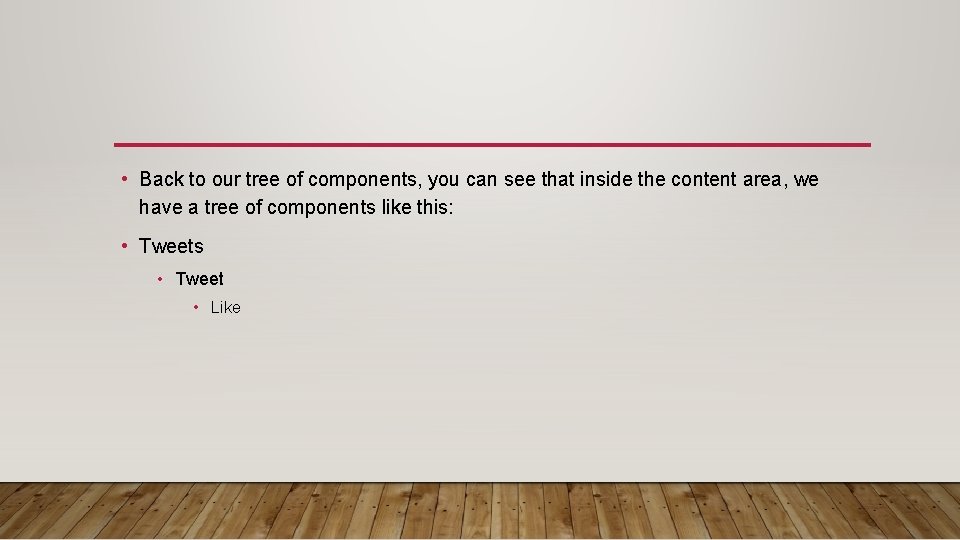
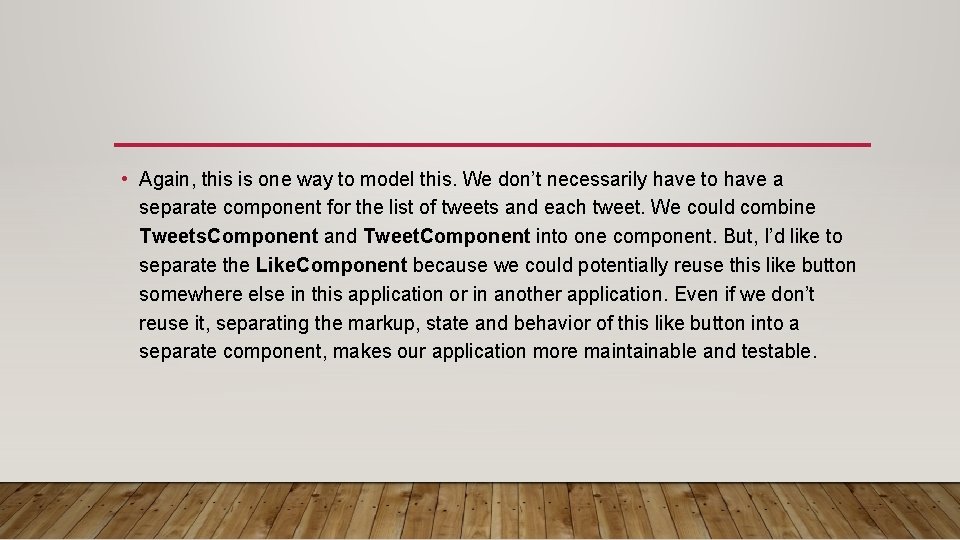
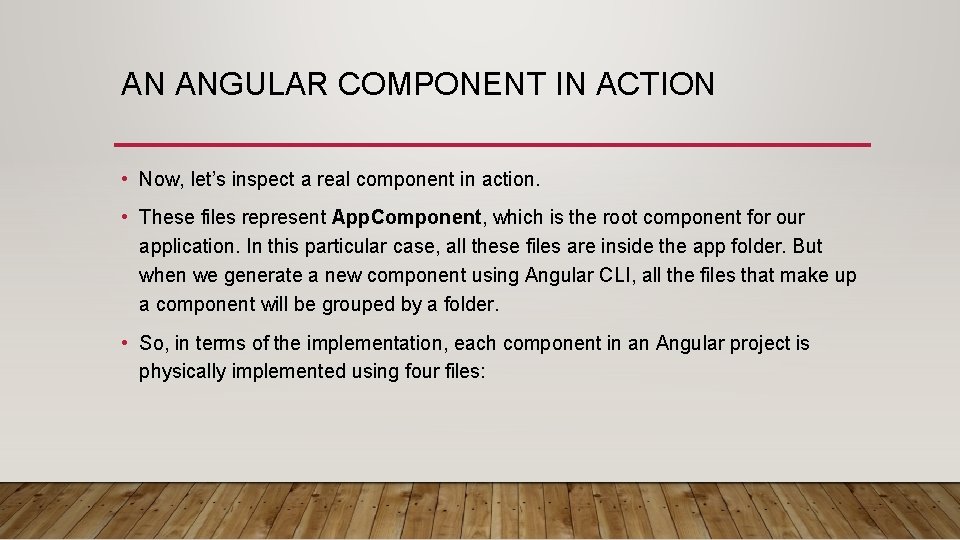
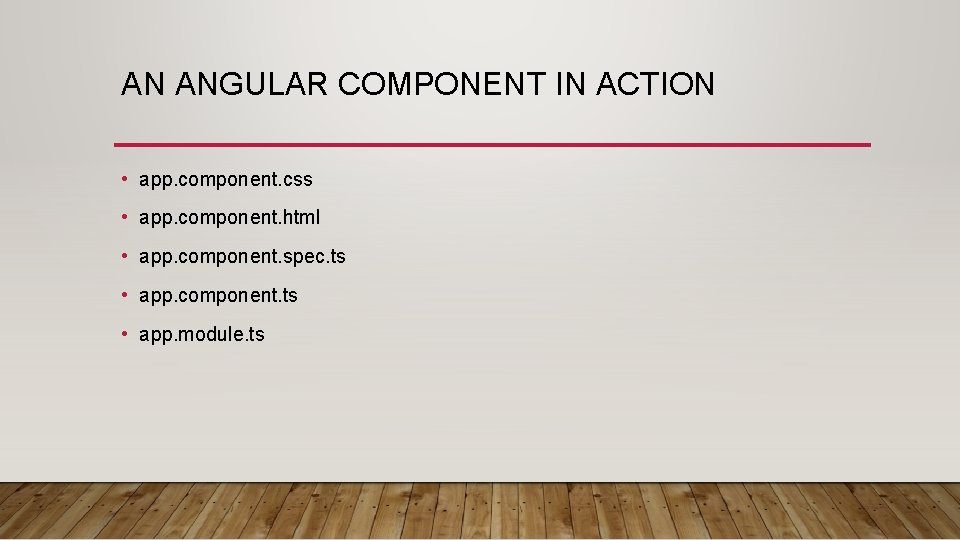
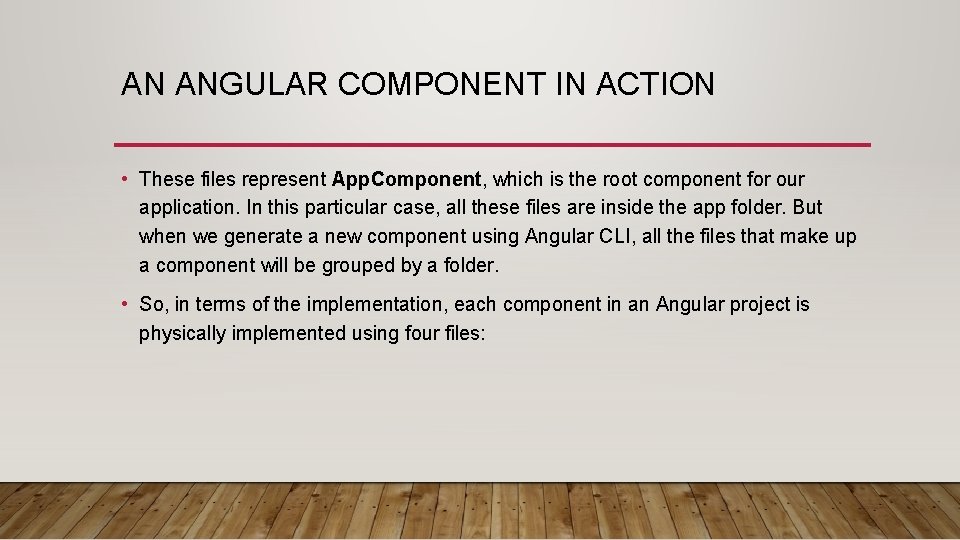
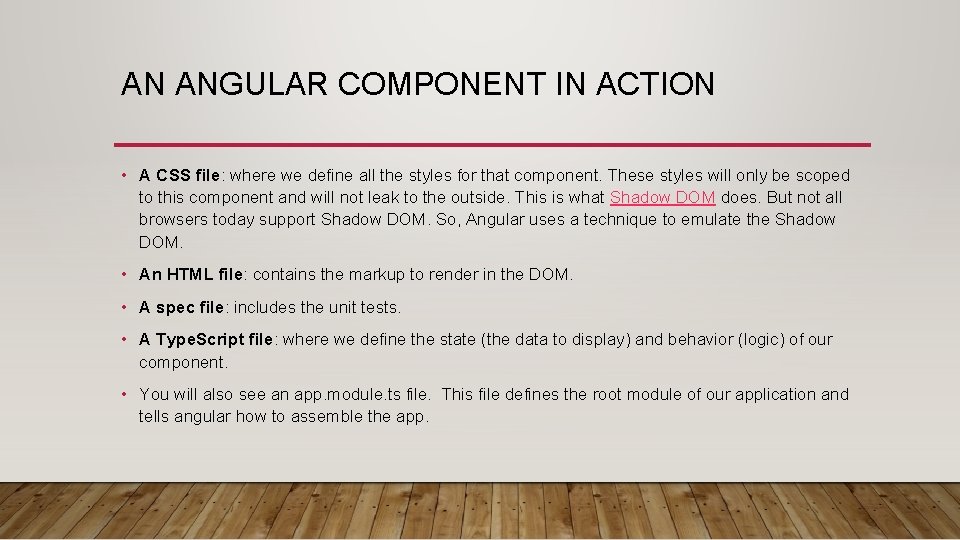
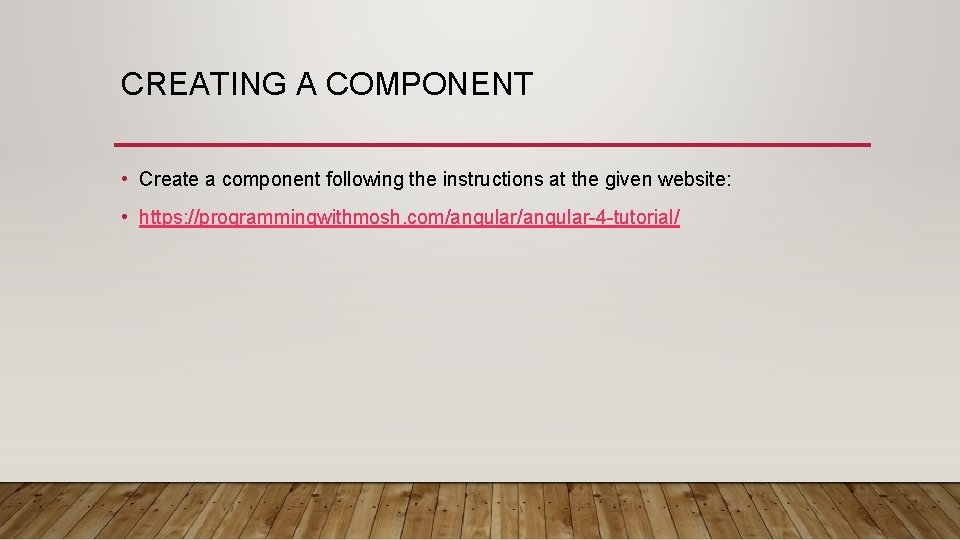
- Slides: 19
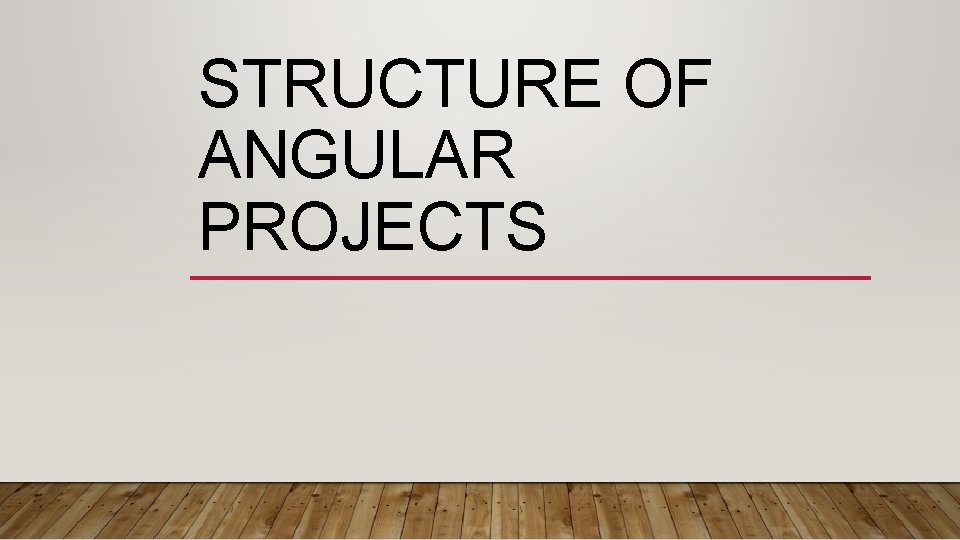
STRUCTURE OF ANGULAR PROJECTS
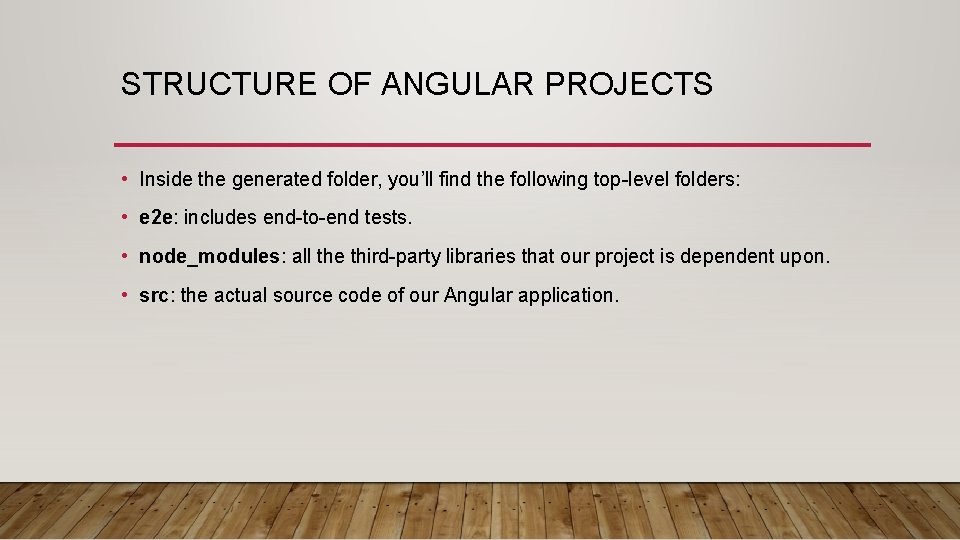
STRUCTURE OF ANGULAR PROJECTS • Inside the generated folder, you’ll find the following top-level folders: • e 2 e: includes end-to-end tests. • node_modules: all the third-party libraries that our project is dependent upon. • src: the actual source code of our Angular application.
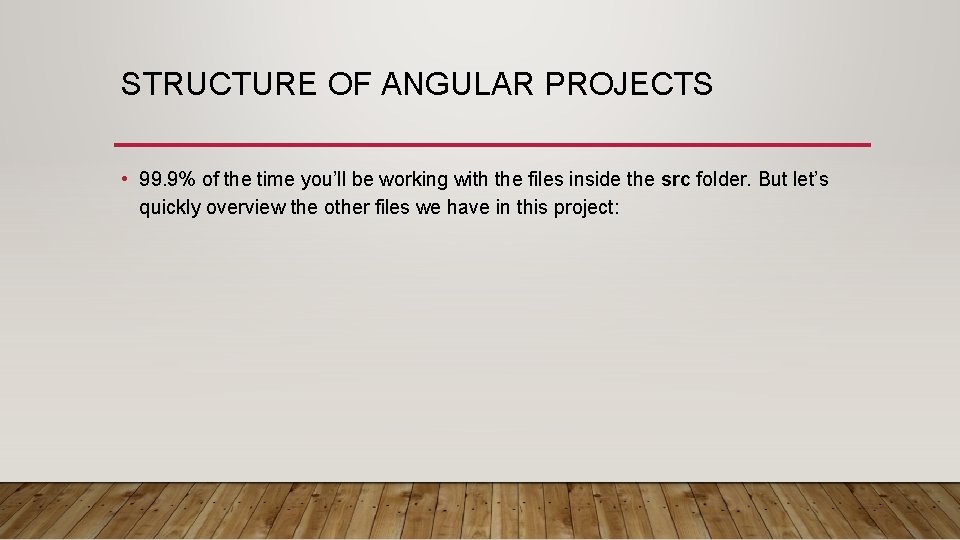
STRUCTURE OF ANGULAR PROJECTS • 99. 9% of the time you’ll be working with the files inside the src folder. But let’s quickly overview the other files we have in this project:
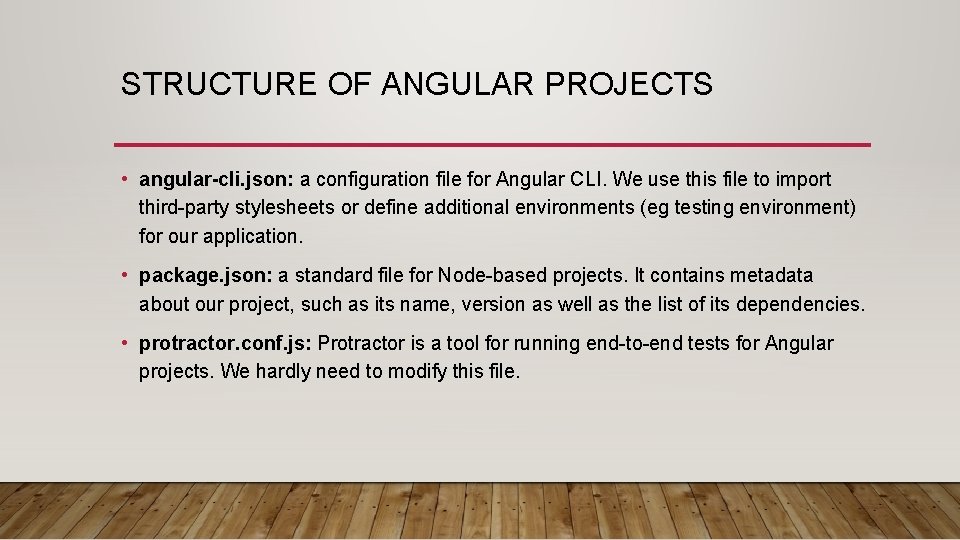
STRUCTURE OF ANGULAR PROJECTS • angular-cli. json: a configuration file for Angular CLI. We use this file to import third-party stylesheets or define additional environments (eg testing environment) for our application. • package. json: a standard file for Node-based projects. It contains metadata about our project, such as its name, version as well as the list of its dependencies. • protractor. conf. js: Protractor is a tool for running end-to-end tests for Angular projects. We hardly need to modify this file.
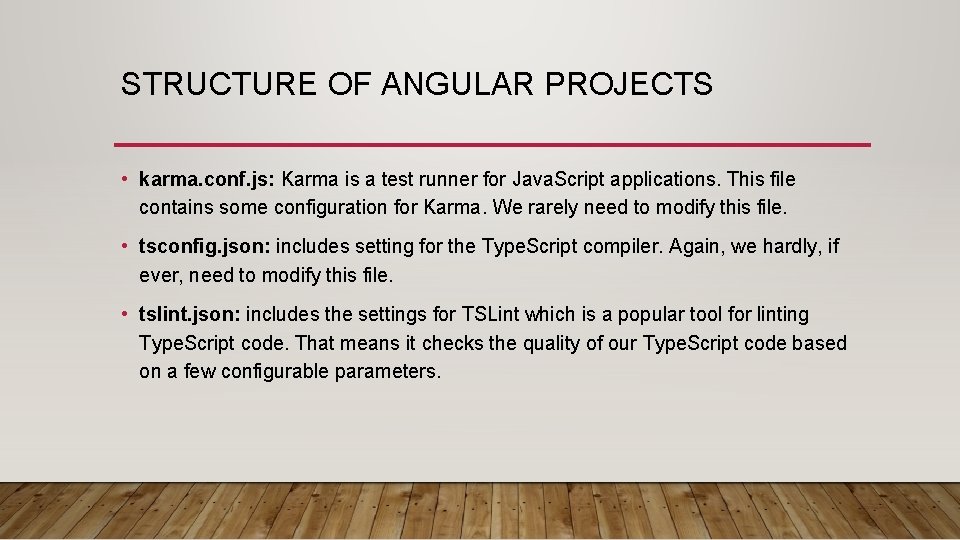
STRUCTURE OF ANGULAR PROJECTS • karma. conf. js: Karma is a test runner for Java. Script applications. This file contains some configuration for Karma. We rarely need to modify this file. • tsconfig. json: includes setting for the Type. Script compiler. Again, we hardly, if ever, need to modify this file. • tslint. json: includes the settings for TSLint which is a popular tool for linting Type. Script code. That means it checks the quality of our Type. Script code based on a few configurable parameters.
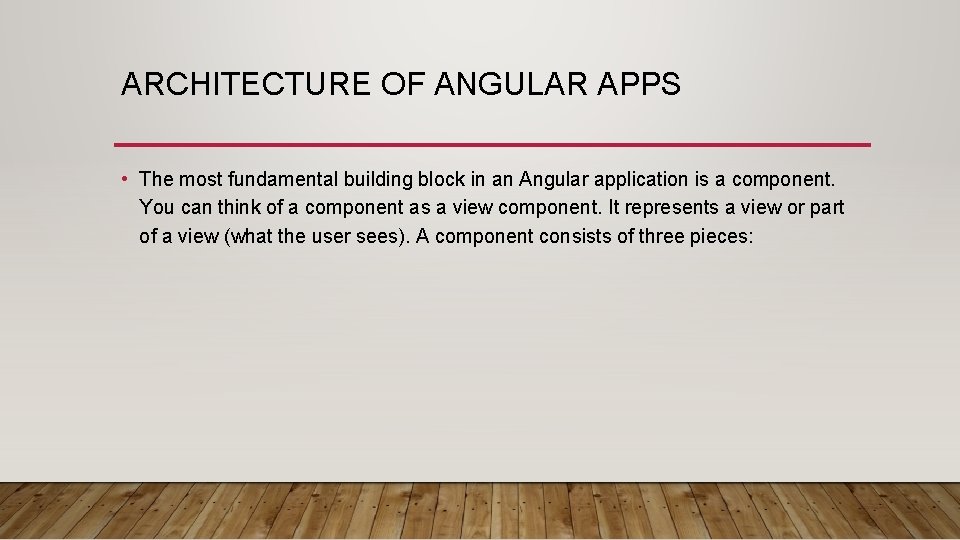
ARCHITECTURE OF ANGULAR APPS • The most fundamental building block in an Angular application is a component. You can think of a component as a view component. It represents a view or part of a view (what the user sees). A component consists of three pieces:
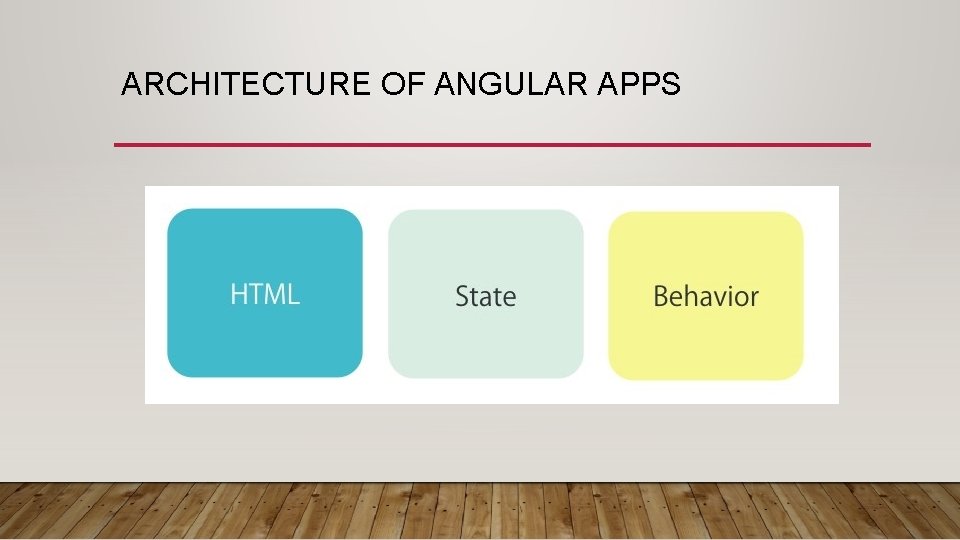
ARCHITECTURE OF ANGULAR APPS
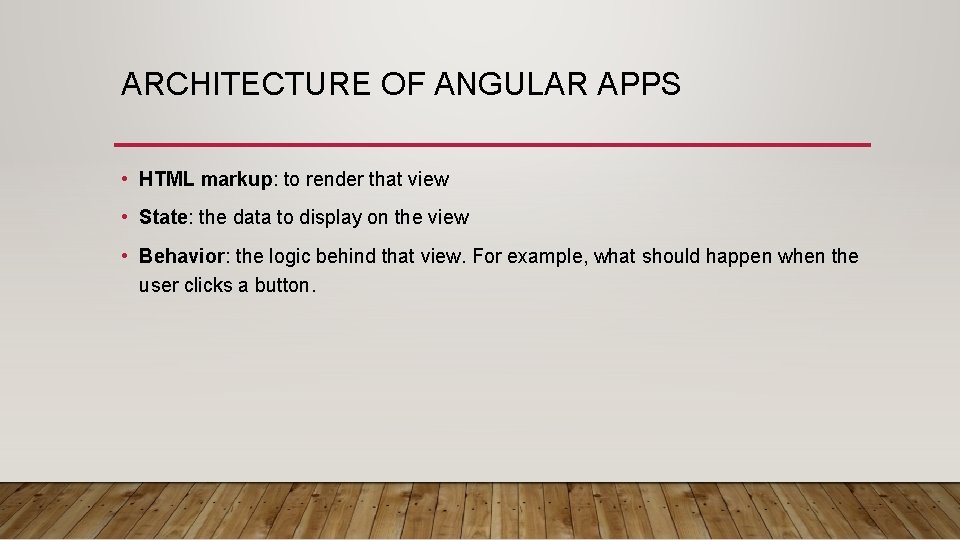
ARCHITECTURE OF ANGULAR APPS • HTML markup: to render that view • State: the data to display on the view • Behavior: the logic behind that view. For example, what should happen when the user clicks a button.
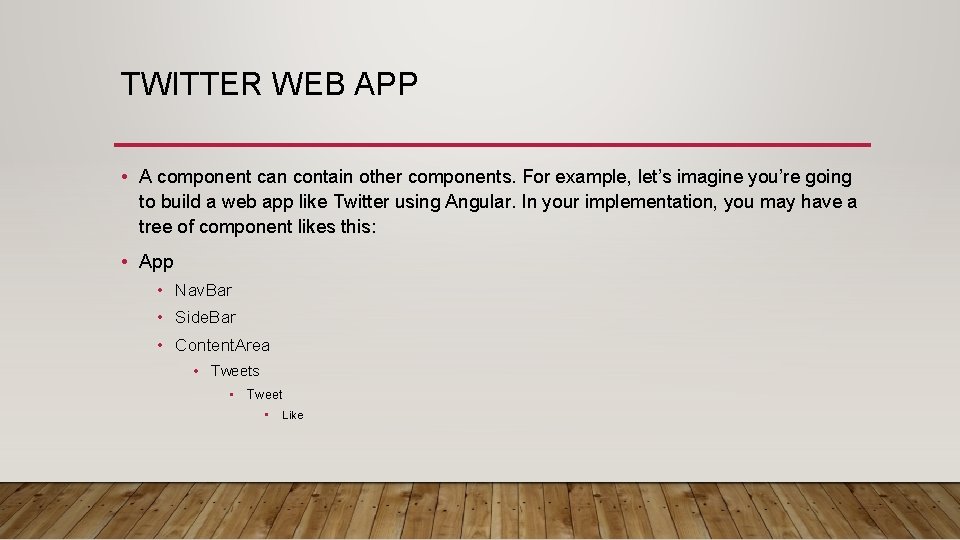
TWITTER WEB APP • A component can contain other components. For example, let’s imagine you’re going to build a web app like Twitter using Angular. In your implementation, you may have a tree of component likes this: • App • Nav. Bar • Side. Bar • Content. Area • Tweets • Tweet • Like
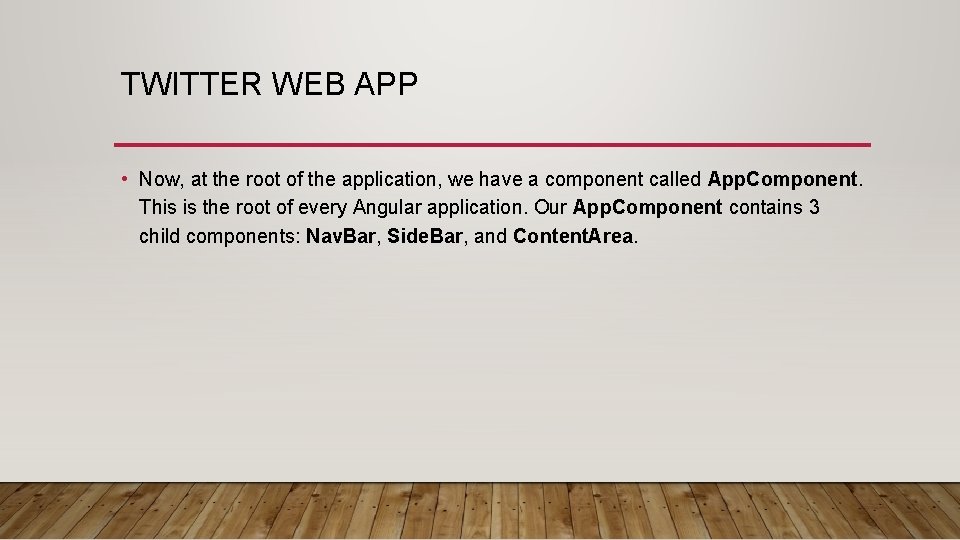
TWITTER WEB APP • Now, at the root of the application, we have a component called App. Component. This is the root of every Angular application. Our App. Component contains 3 child components: Nav. Bar, Side. Bar, and Content. Area.
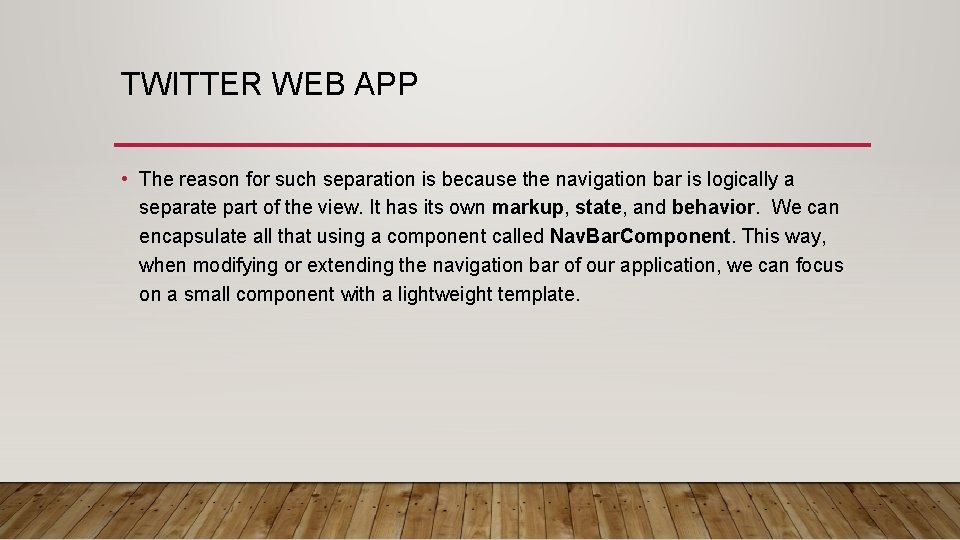
TWITTER WEB APP • The reason for such separation is because the navigation bar is logically a separate part of the view. It has its own markup, state, and behavior. We can encapsulate all that using a component called Nav. Bar. Component. This way, when modifying or extending the navigation bar of our application, we can focus on a small component with a lightweight template.
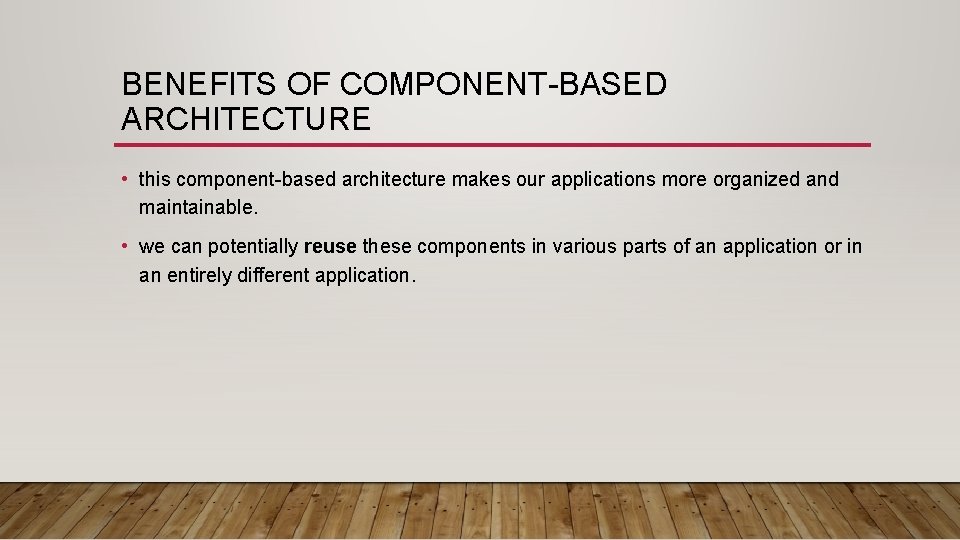
BENEFITS OF COMPONENT-BASED ARCHITECTURE • this component-based architecture makes our applications more organized and maintainable. • we can potentially reuse these components in various parts of an application or in an entirely different application.
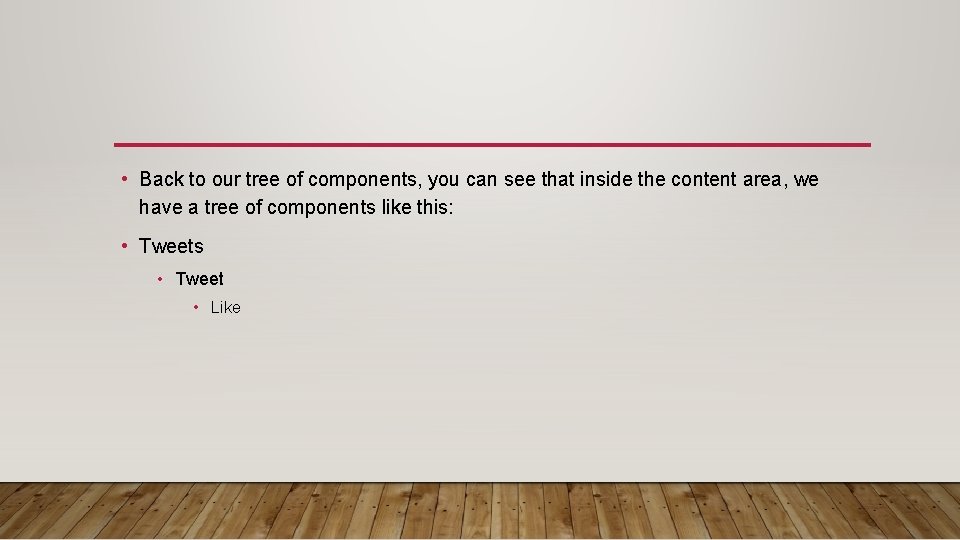
• Back to our tree of components, you can see that inside the content area, we have a tree of components like this: • Tweets • Tweet • Like
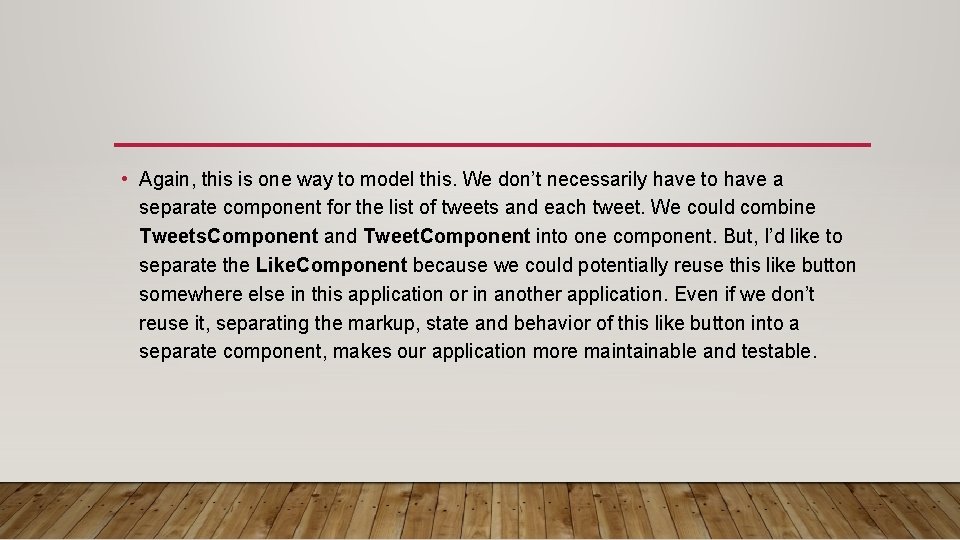
• Again, this is one way to model this. We don’t necessarily have to have a separate component for the list of tweets and each tweet. We could combine Tweets. Component and Tweet. Component into one component. But, I’d like to separate the Like. Component because we could potentially reuse this like button somewhere else in this application or in another application. Even if we don’t reuse it, separating the markup, state and behavior of this like button into a separate component, makes our application more maintainable and testable.
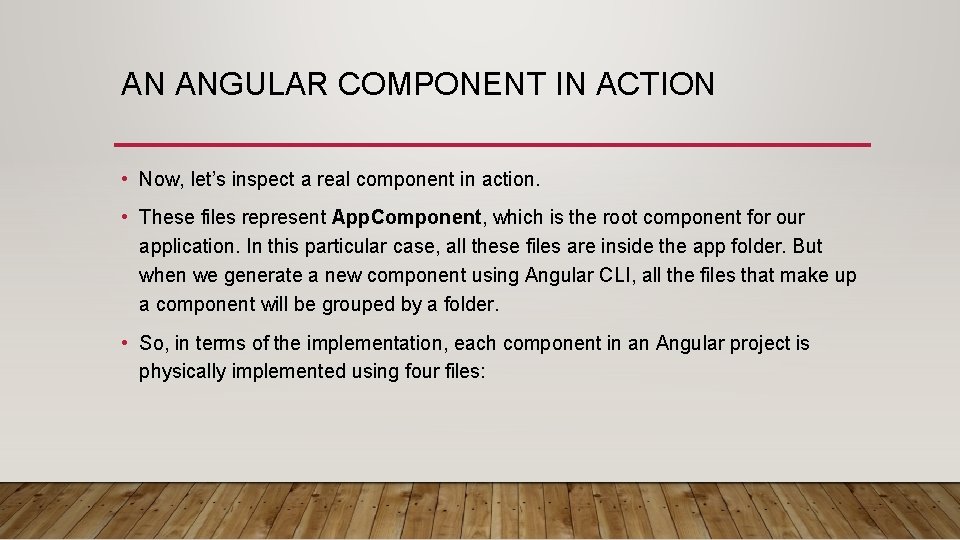
AN ANGULAR COMPONENT IN ACTION • Now, let’s inspect a real component in action. • These files represent App. Component, which is the root component for our application. In this particular case, all these files are inside the app folder. But when we generate a new component using Angular CLI, all the files that make up a component will be grouped by a folder. • So, in terms of the implementation, each component in an Angular project is physically implemented using four files:
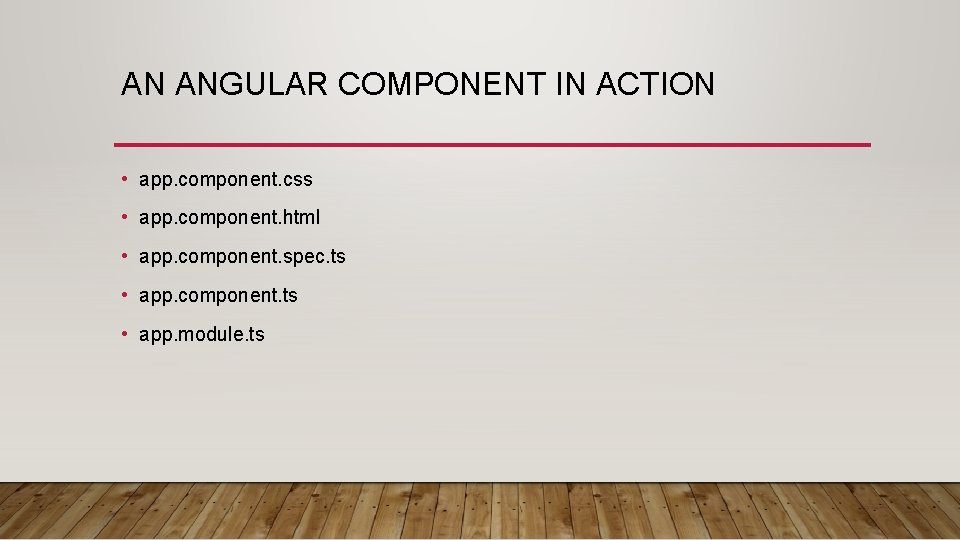
AN ANGULAR COMPONENT IN ACTION • app. component. css • app. component. html • app. component. spec. ts • app. component. ts • app. module. ts
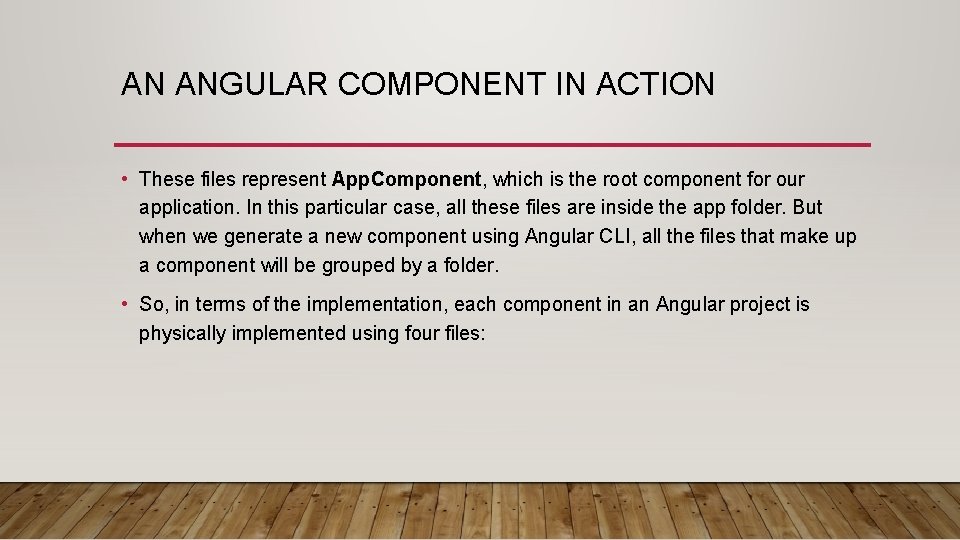
AN ANGULAR COMPONENT IN ACTION • These files represent App. Component, which is the root component for our application. In this particular case, all these files are inside the app folder. But when we generate a new component using Angular CLI, all the files that make up a component will be grouped by a folder. • So, in terms of the implementation, each component in an Angular project is physically implemented using four files:
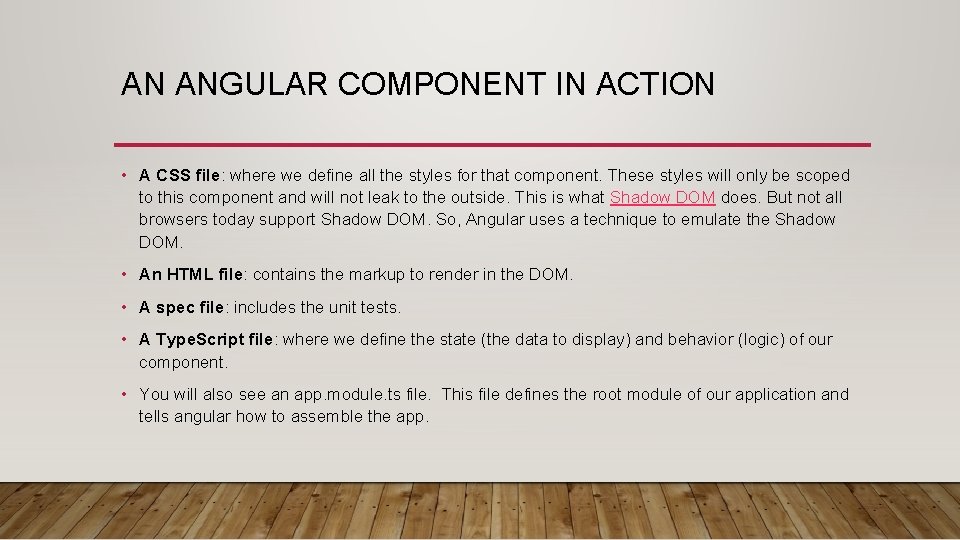
AN ANGULAR COMPONENT IN ACTION • A CSS file: where we define all the styles for that component. These styles will only be scoped to this component and will not leak to the outside. This is what Shadow DOM does. But not all browsers today support Shadow DOM. So, Angular uses a technique to emulate the Shadow DOM. • An HTML file: contains the markup to render in the DOM. • A spec file: includes the unit tests. • A Type. Script file: where we define the state (the data to display) and behavior (logic) of our component. • You will also see an app. module. ts file. This file defines the root module of our application and tells angular how to assemble the app.
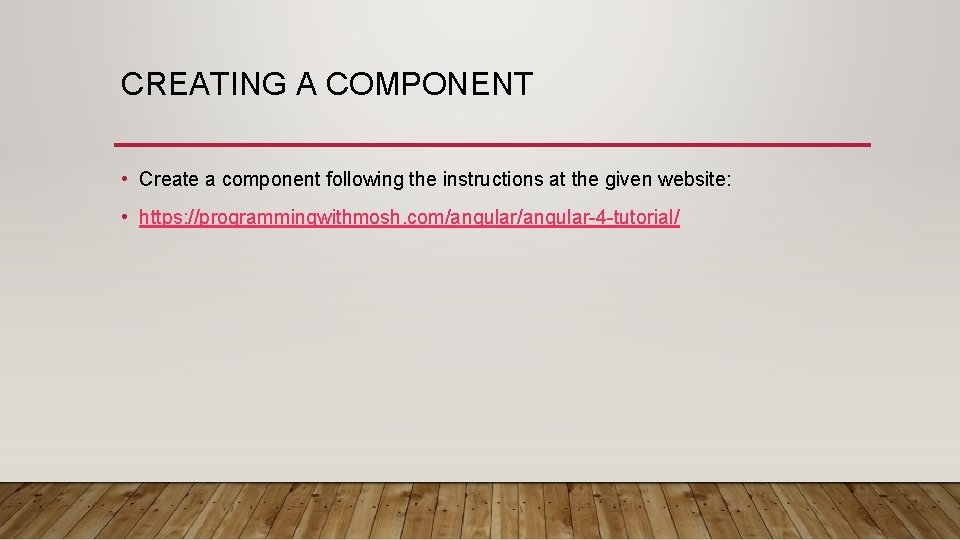
CREATING A COMPONENT • Create a component following the instructions at the given website: • https: //programmingwithmosh. com/angular-4 -tutorial/