STRUCT struct product a user defined complex type
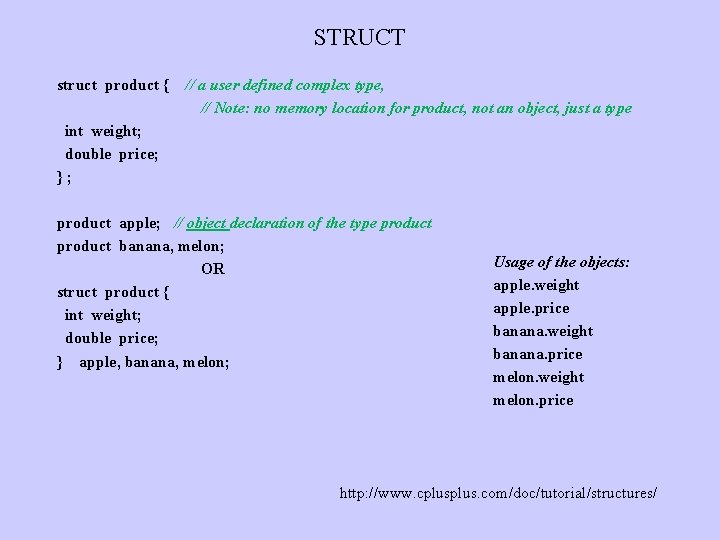
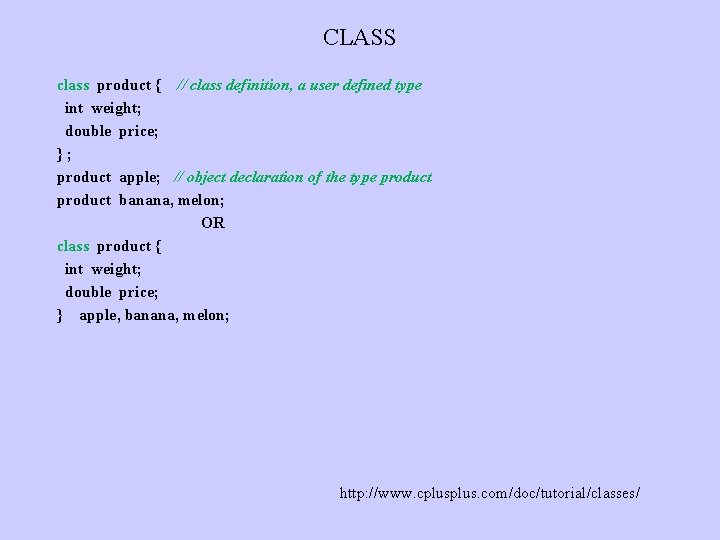
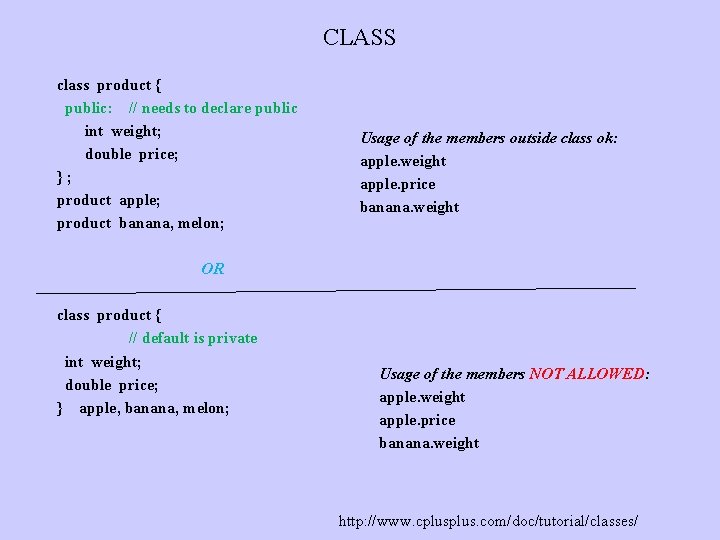
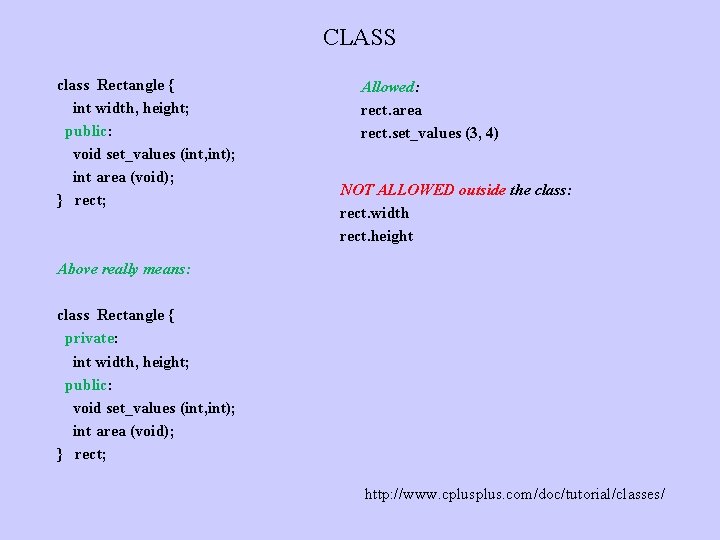
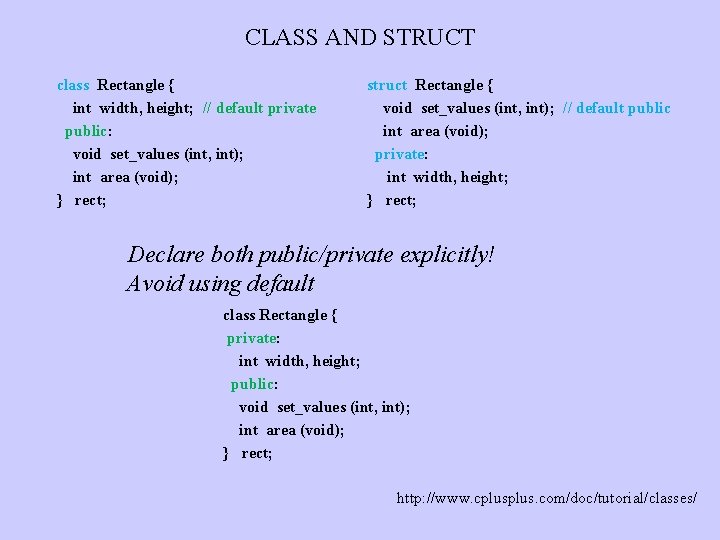
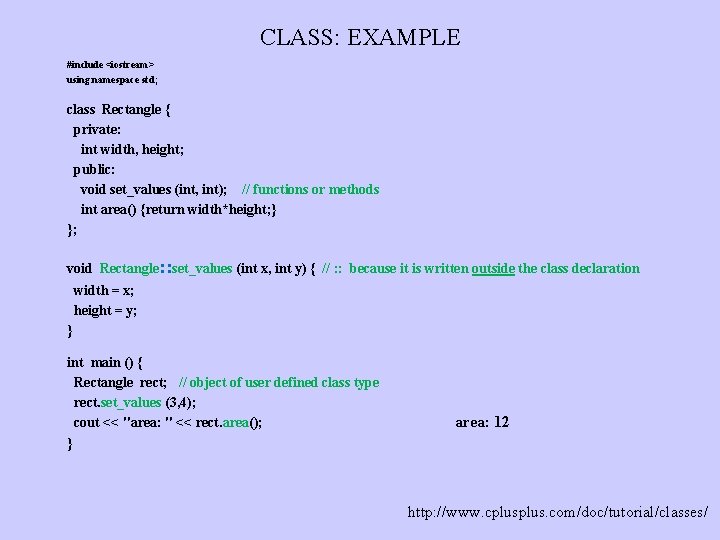
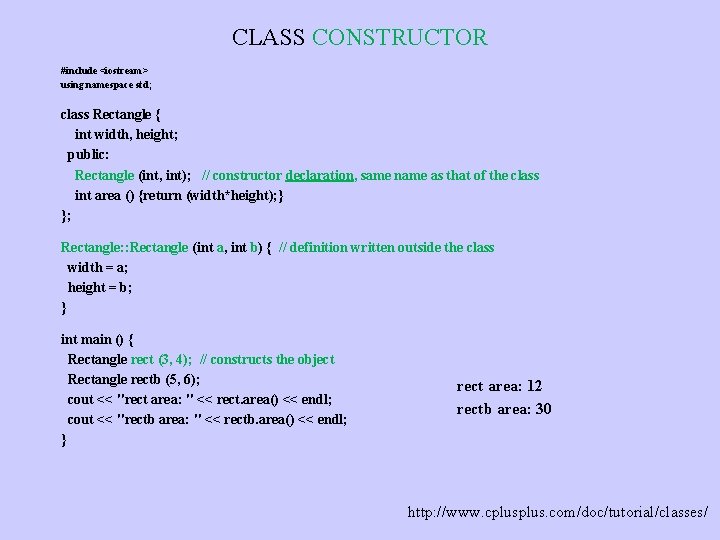
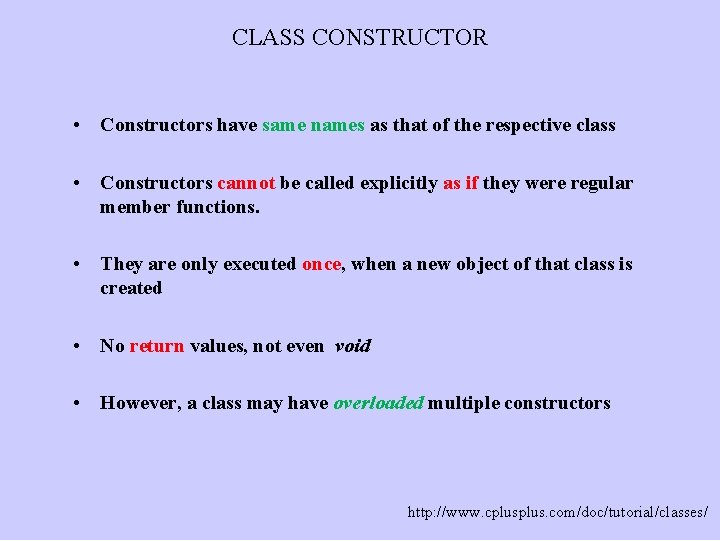
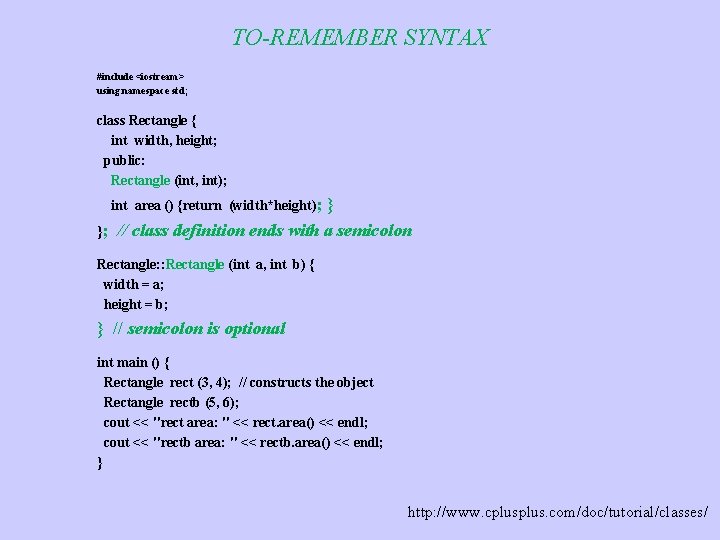
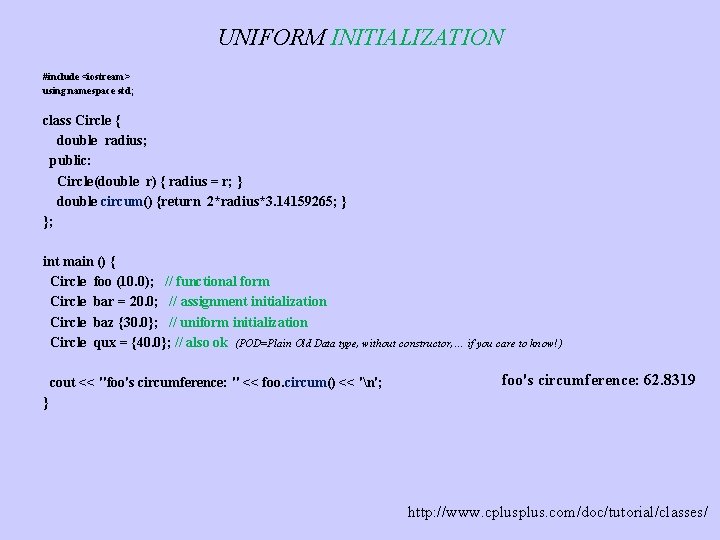
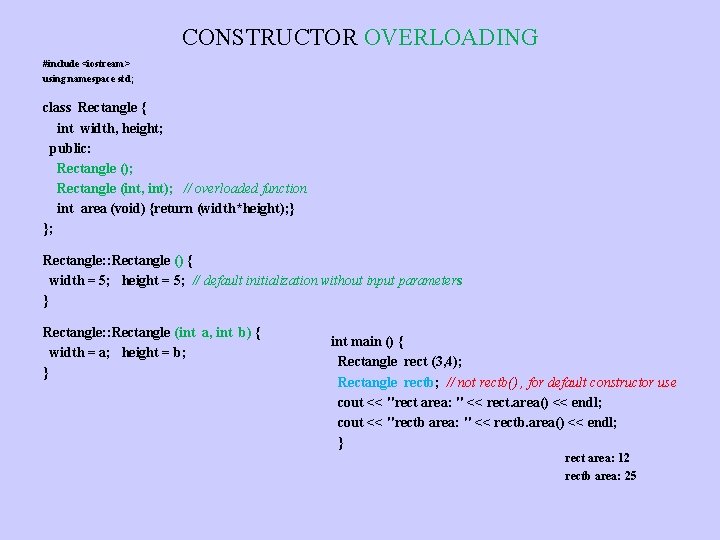
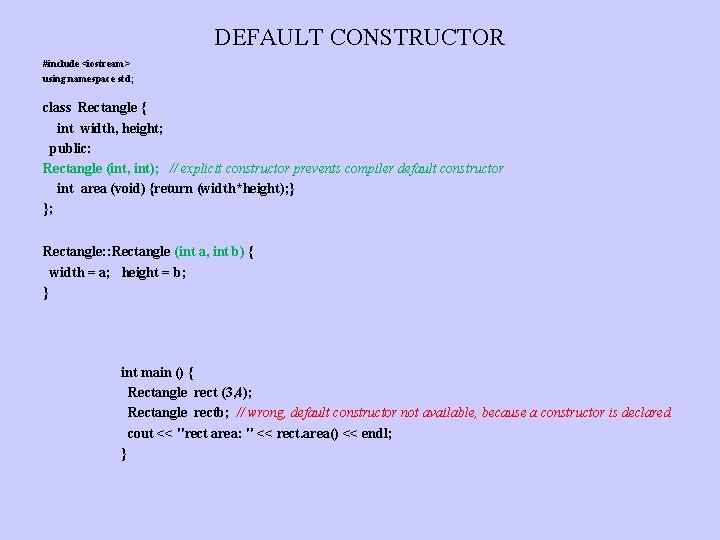
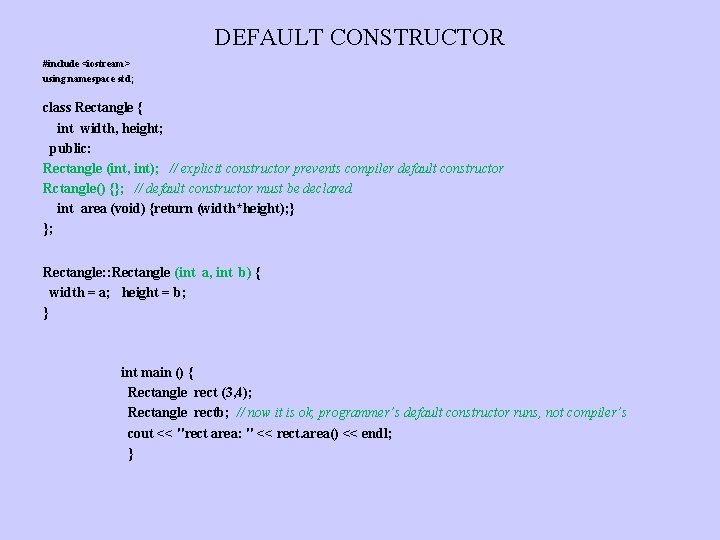
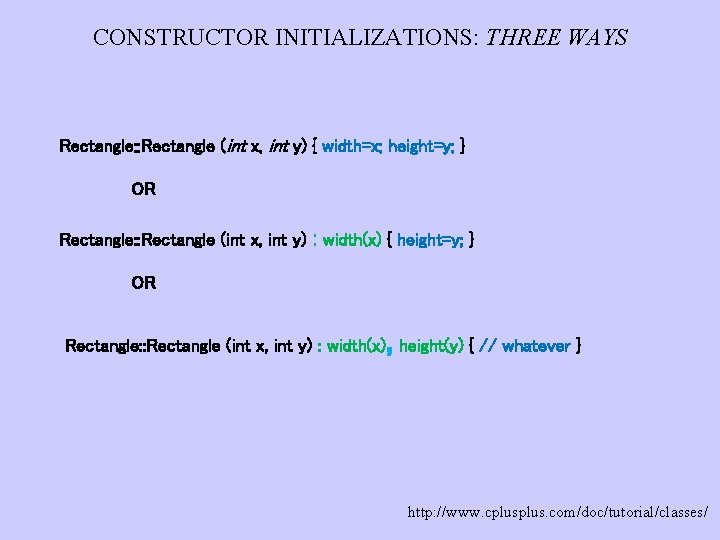
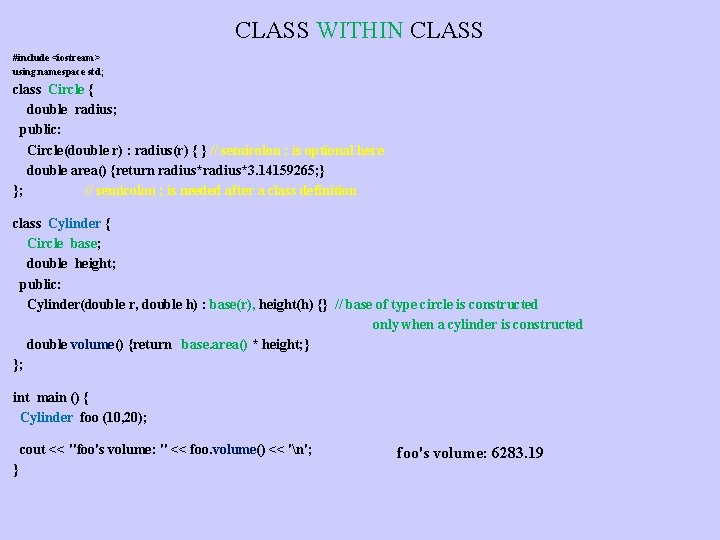
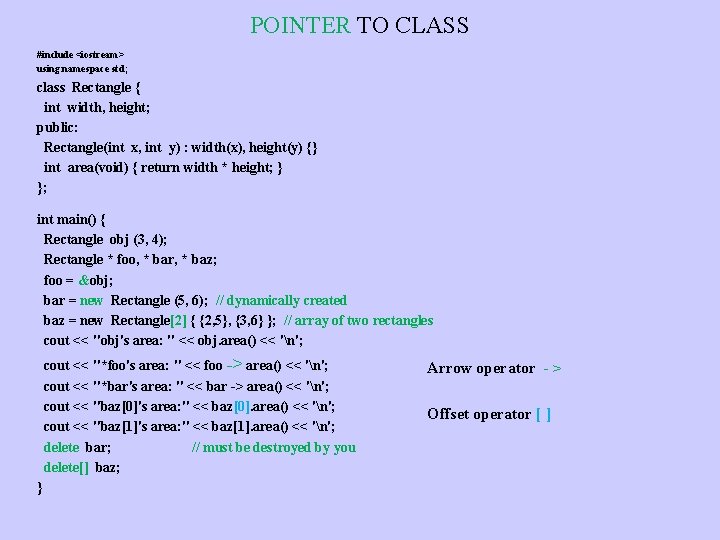
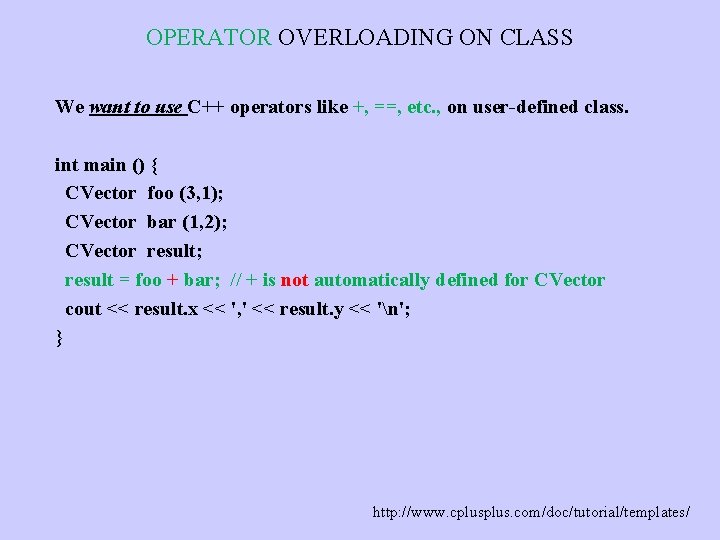
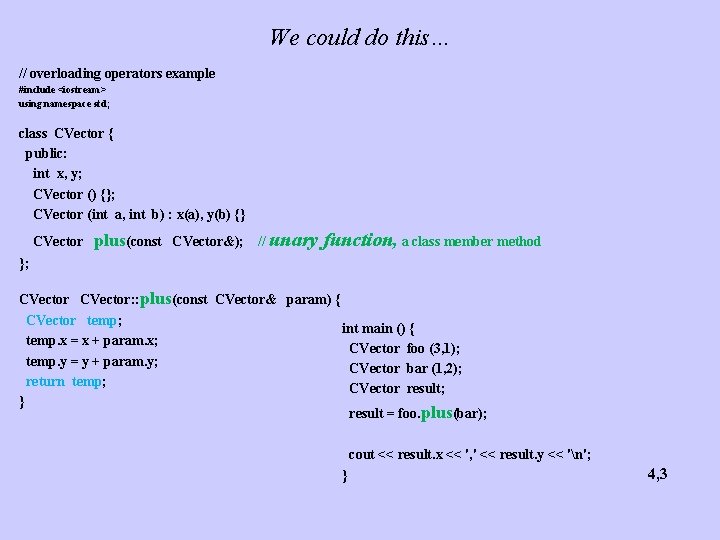
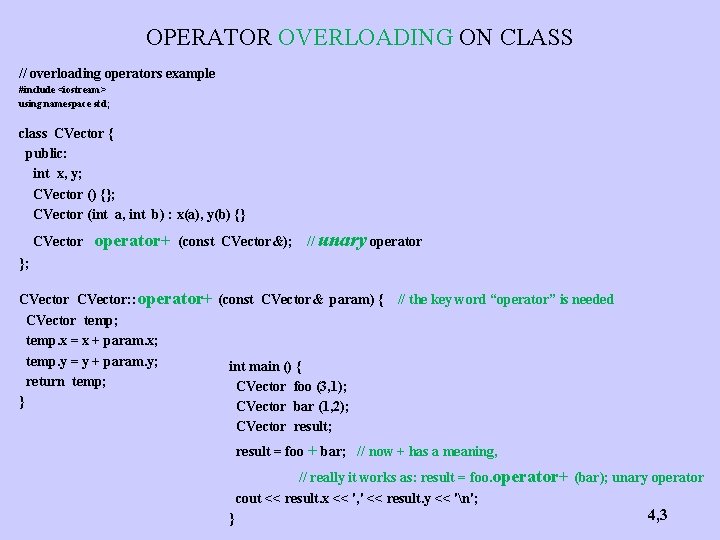
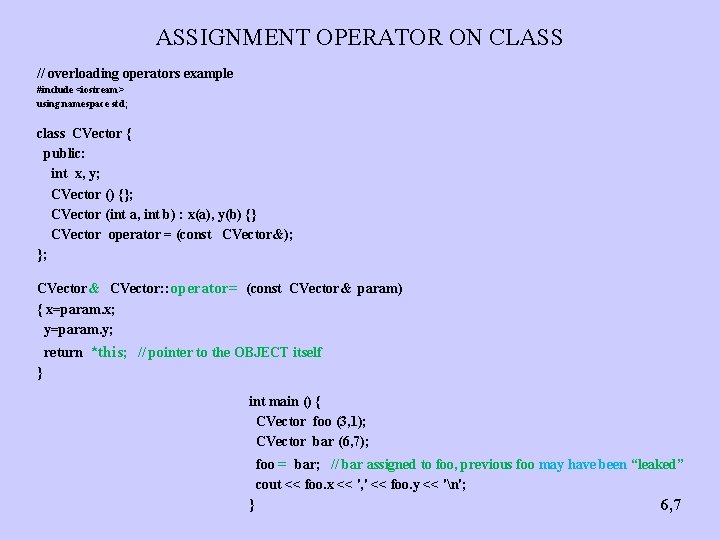
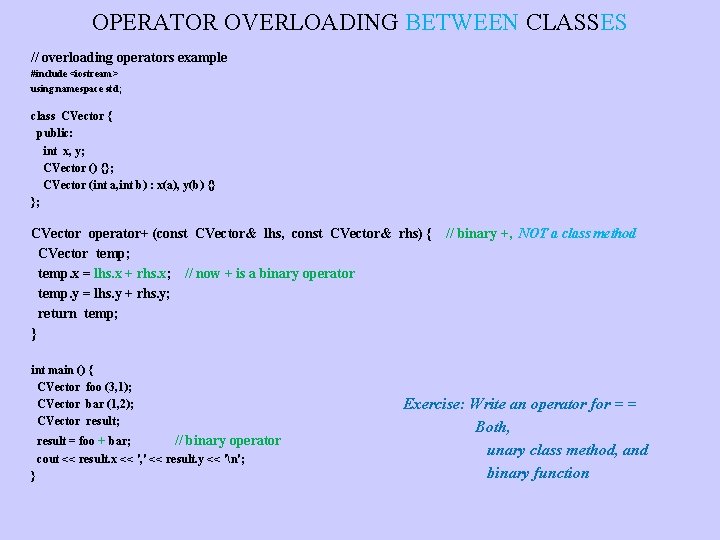
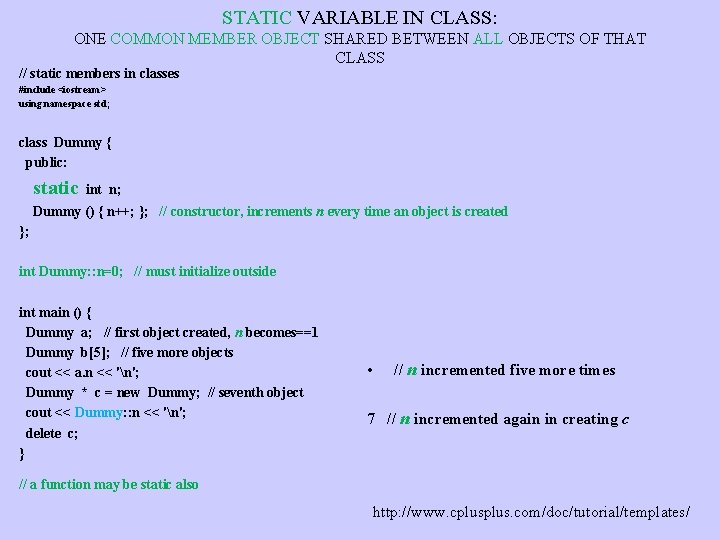
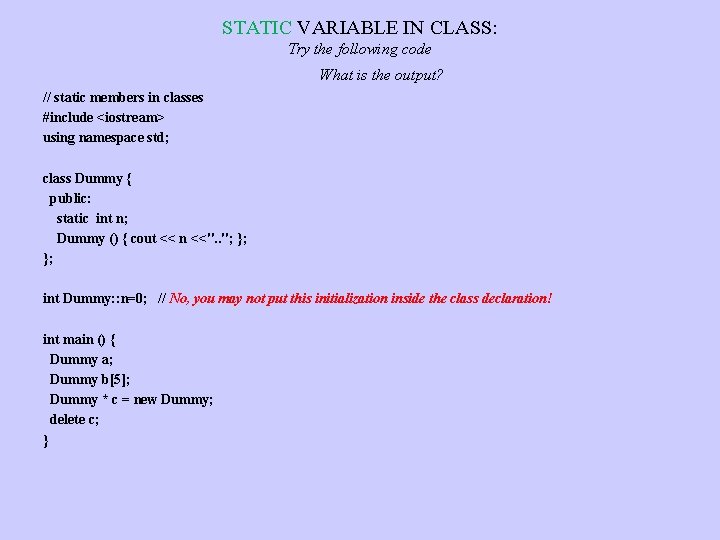
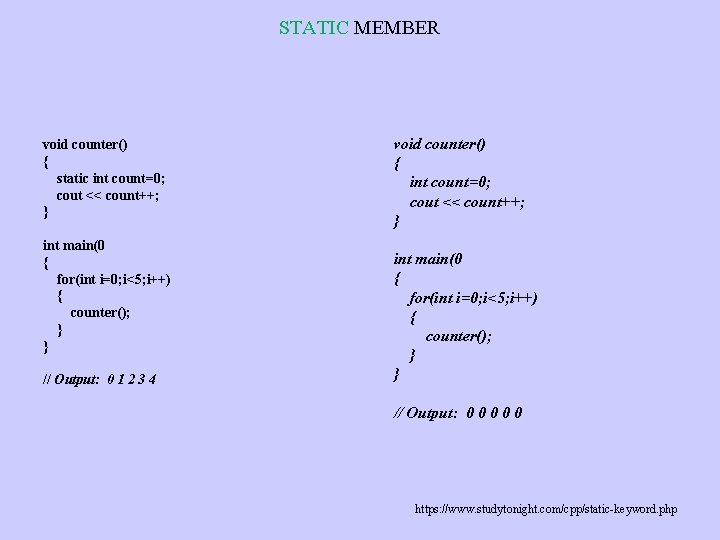
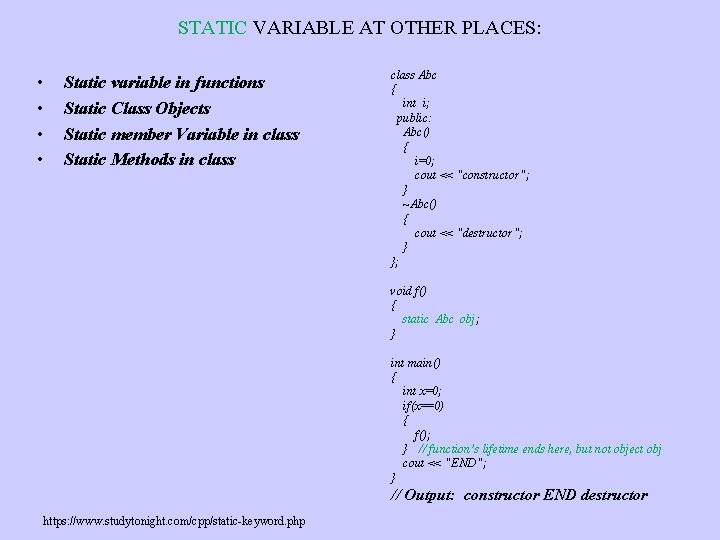
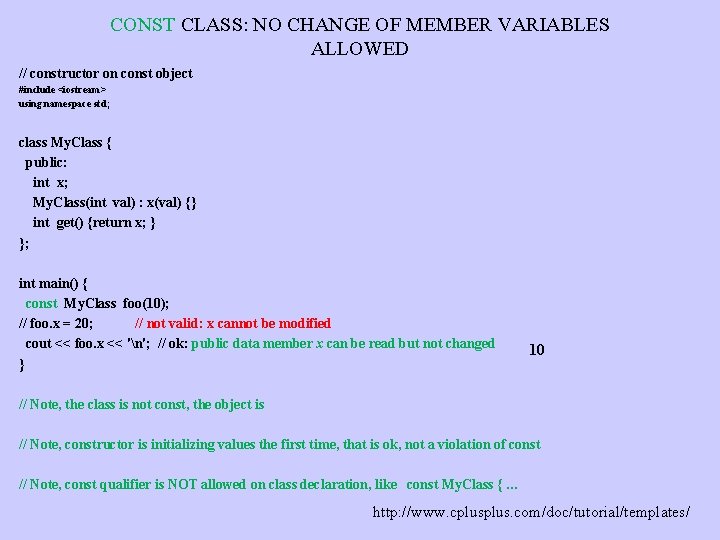
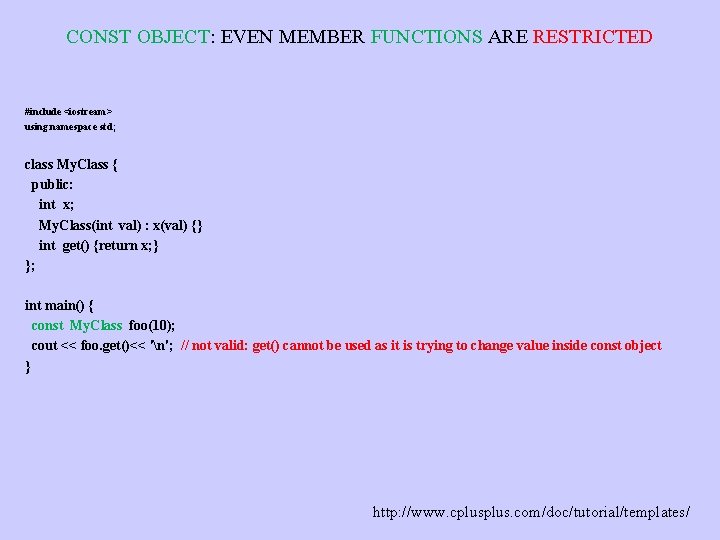
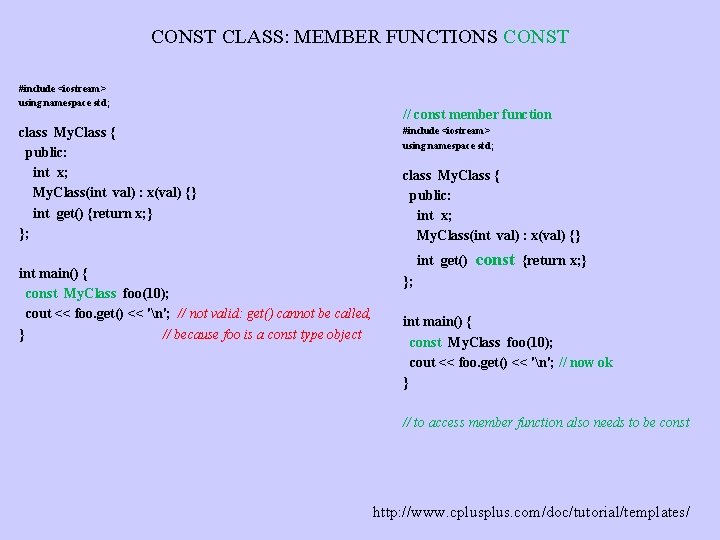
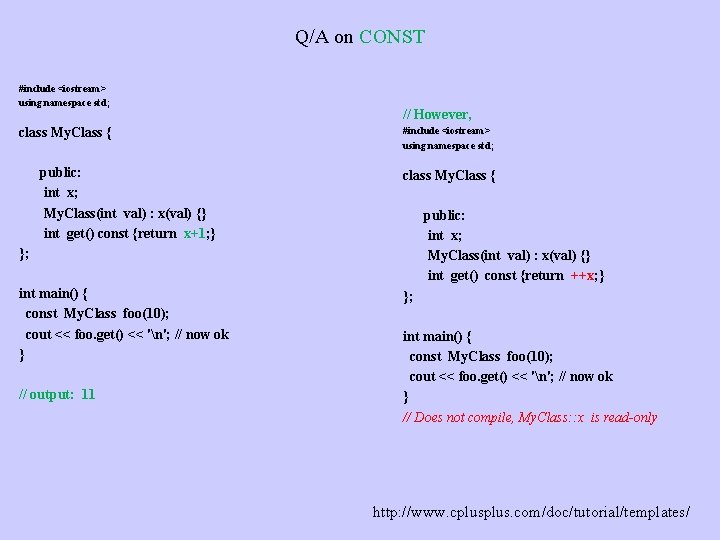
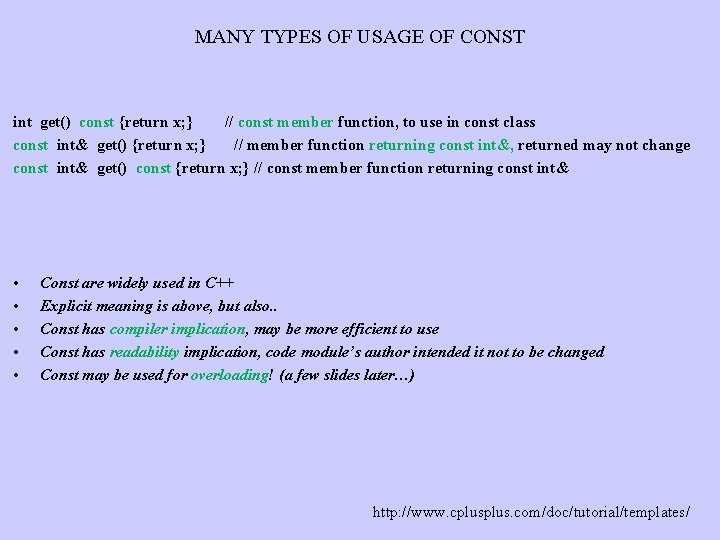
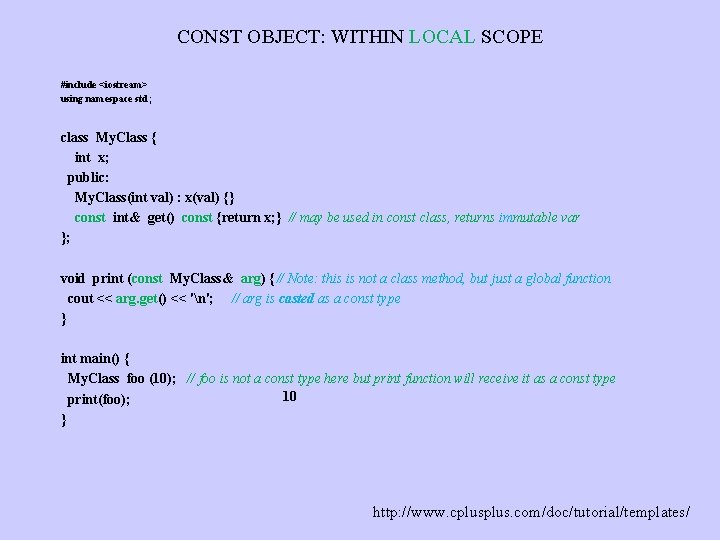
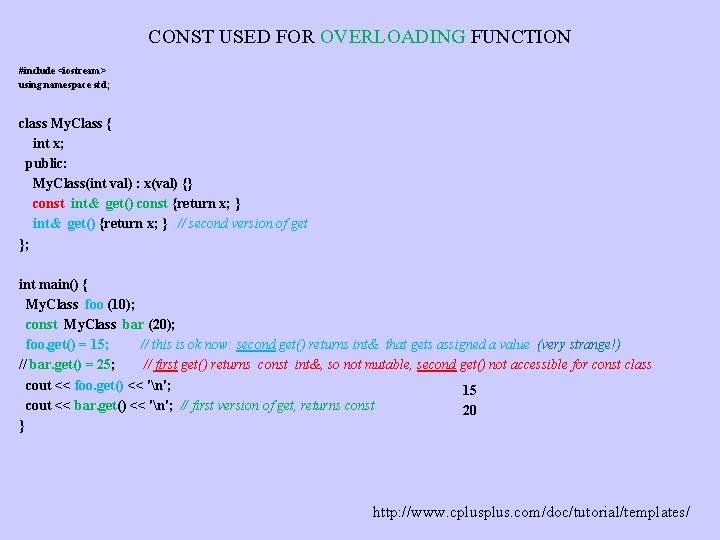
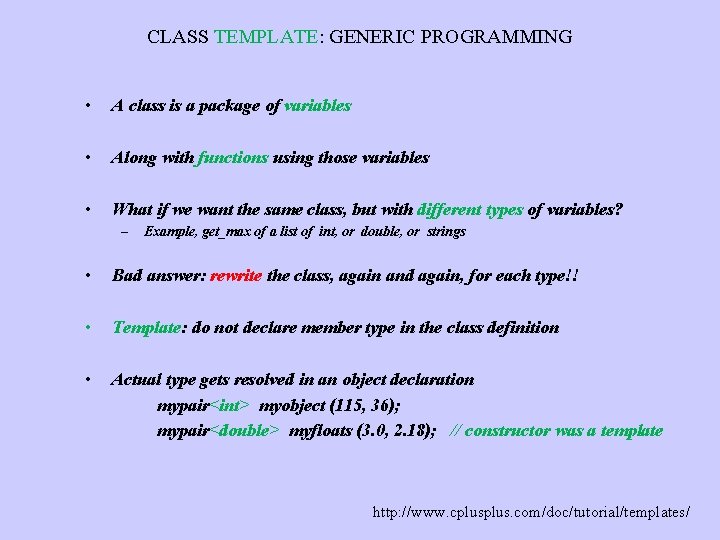
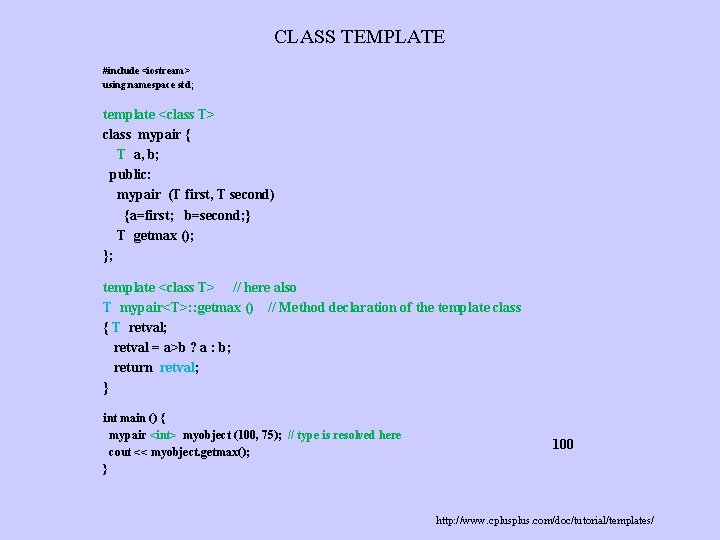
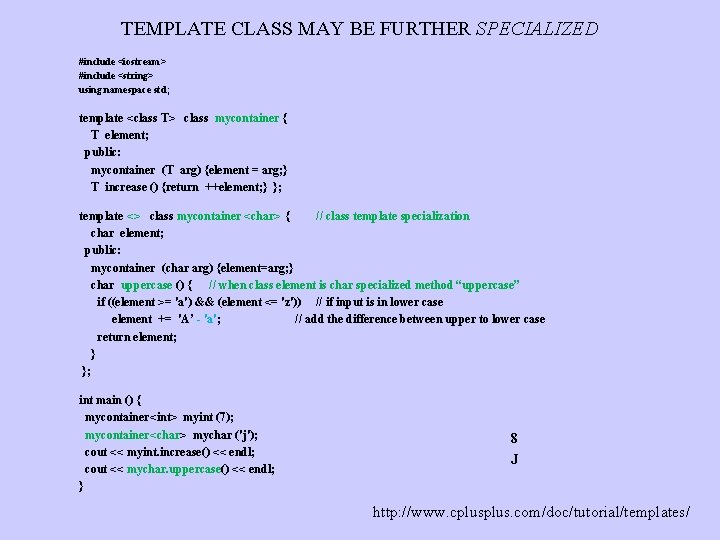
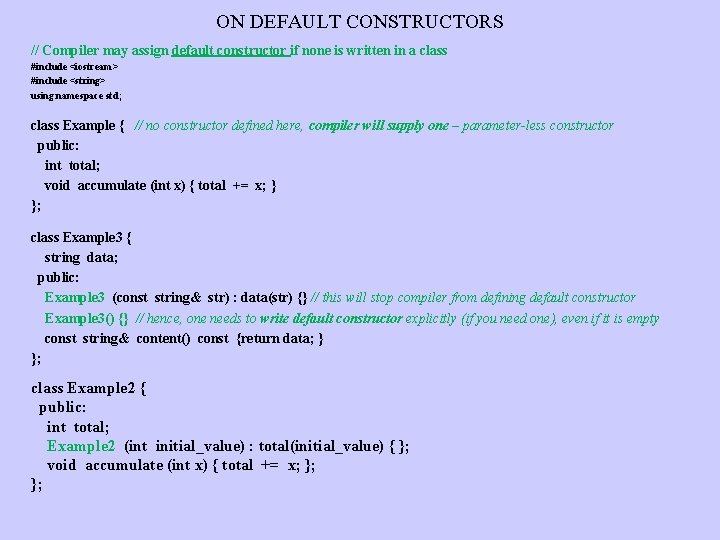
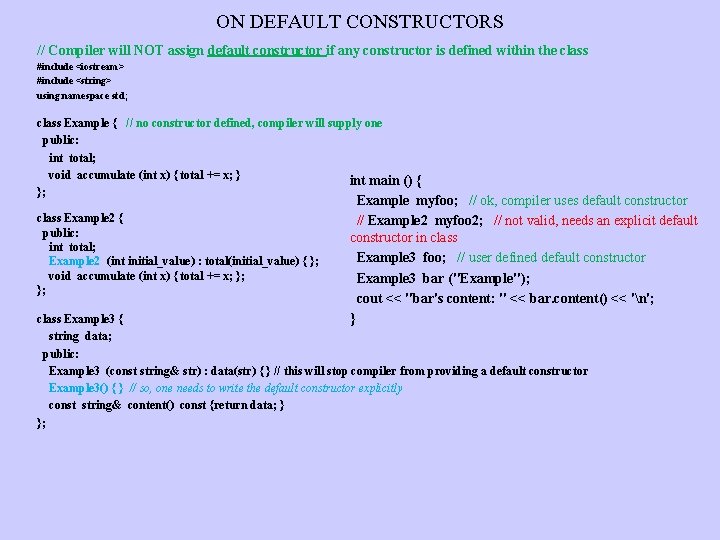
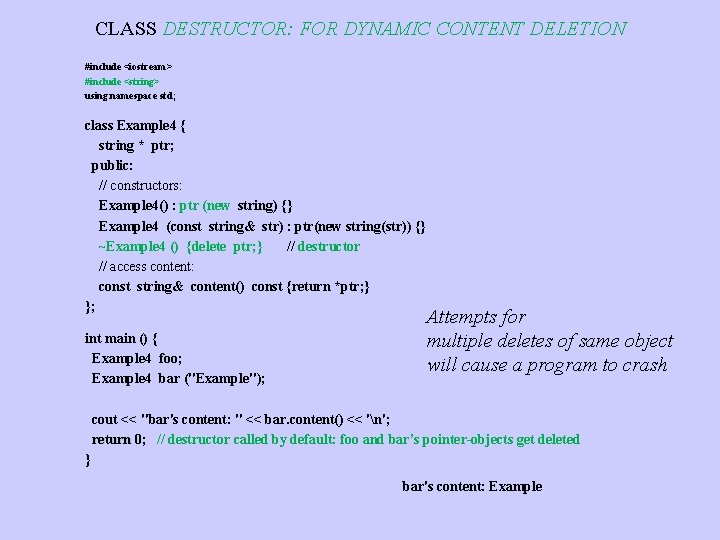
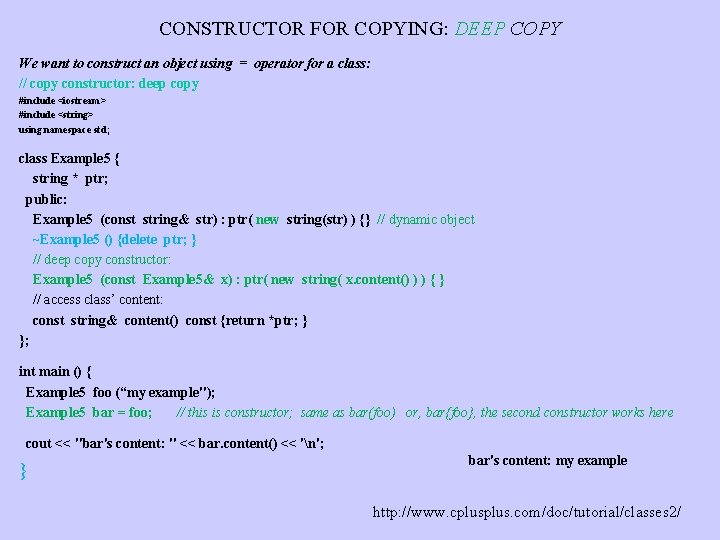
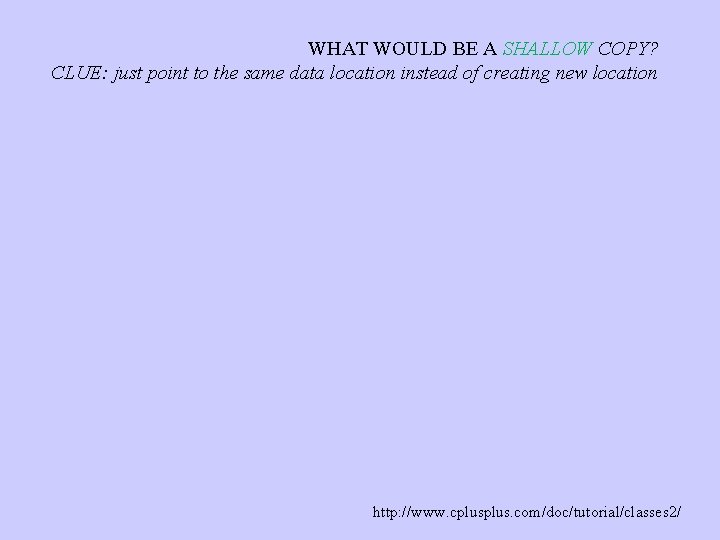
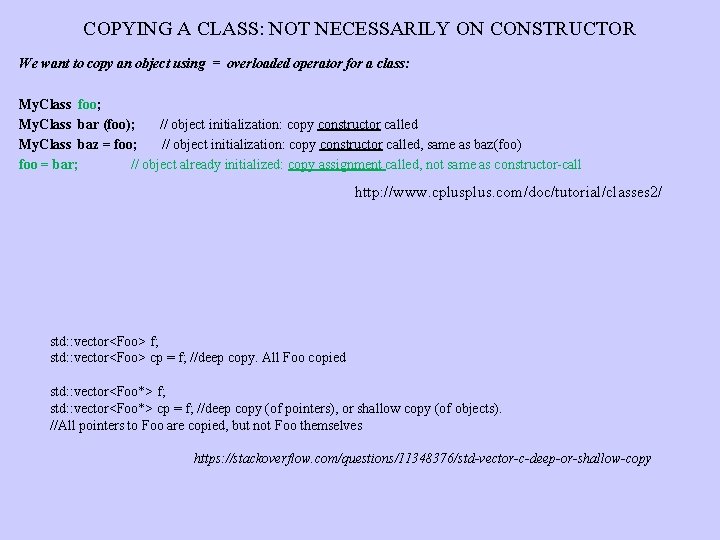
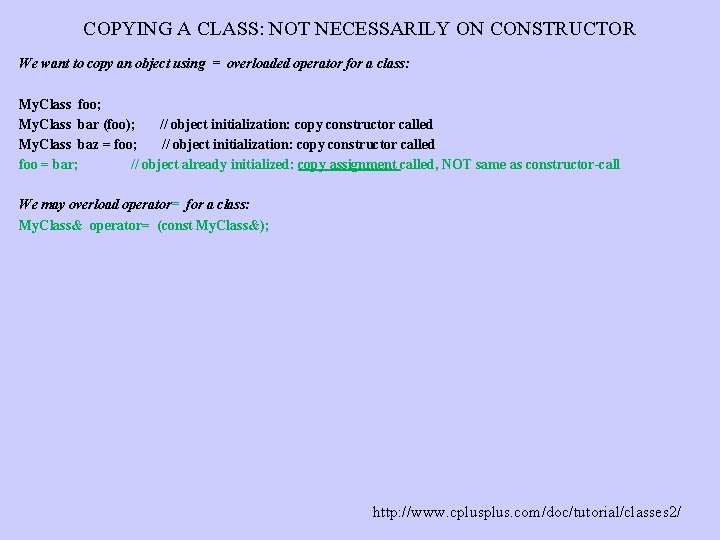
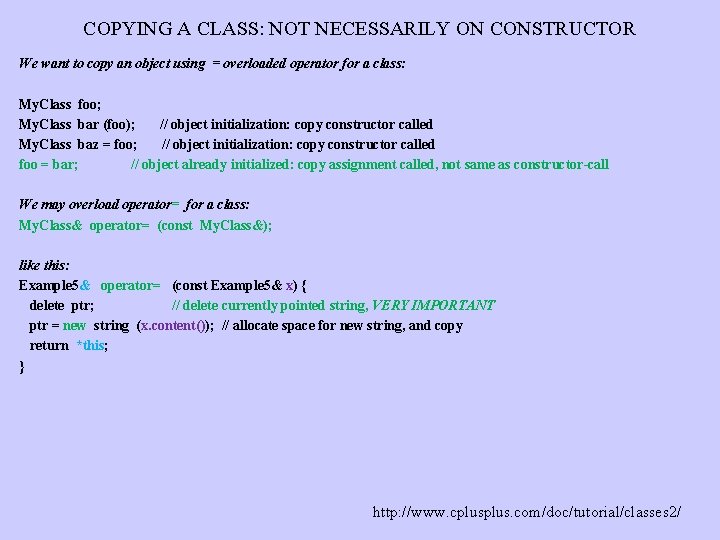
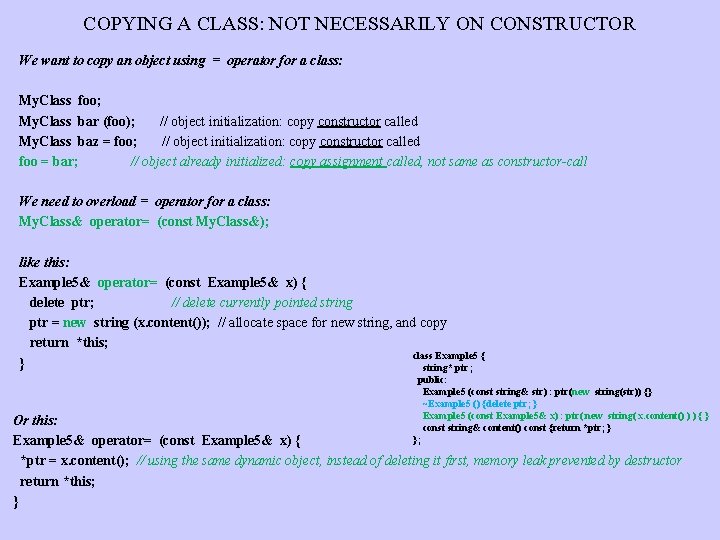
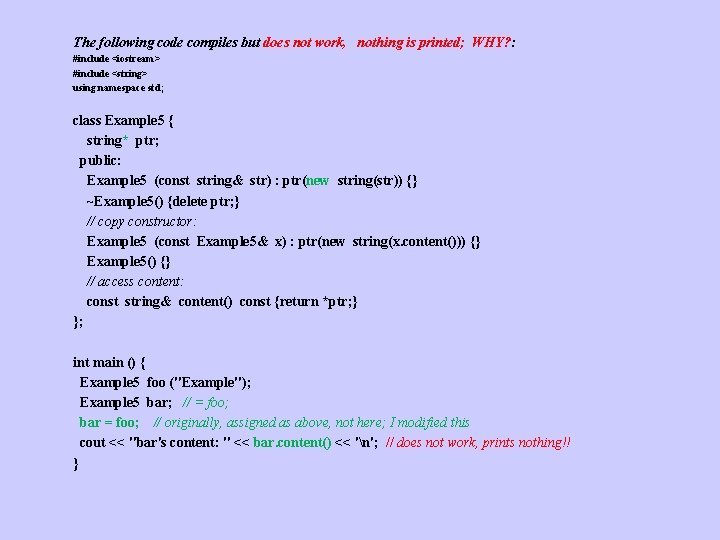
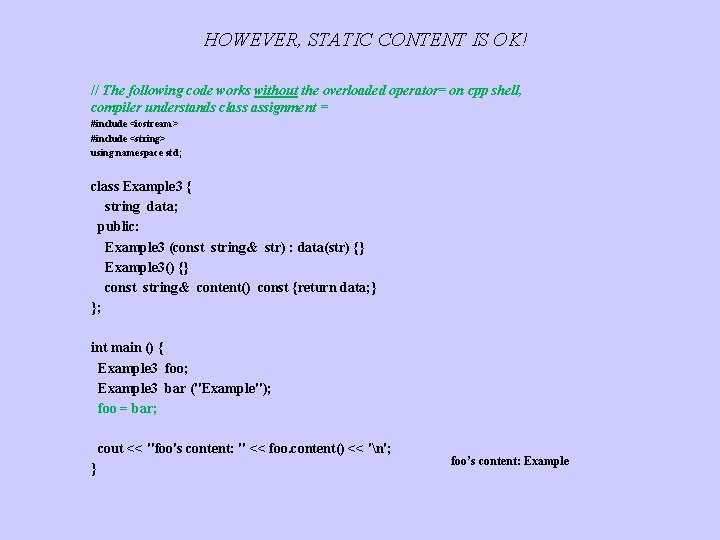
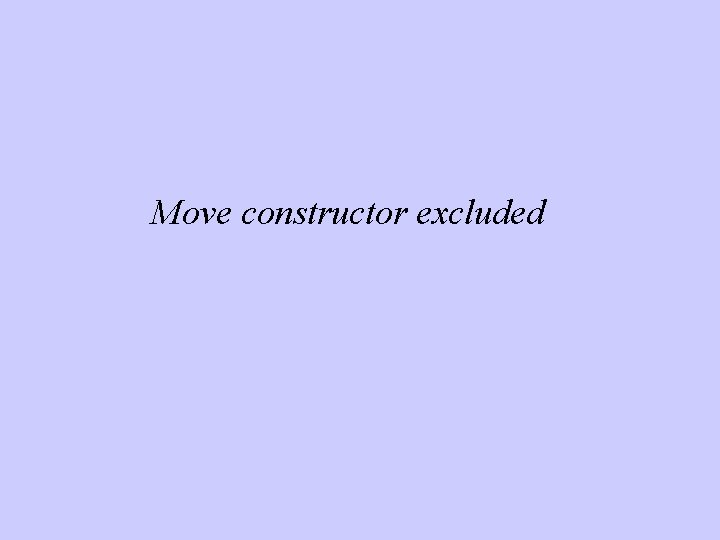
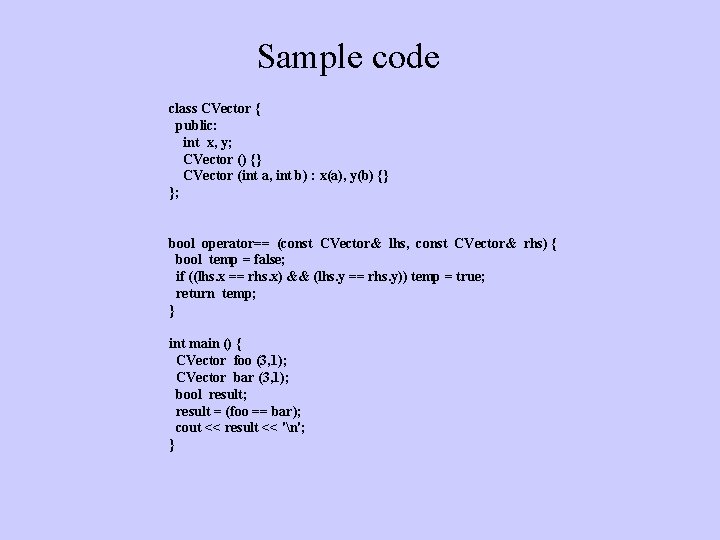
- Slides: 48
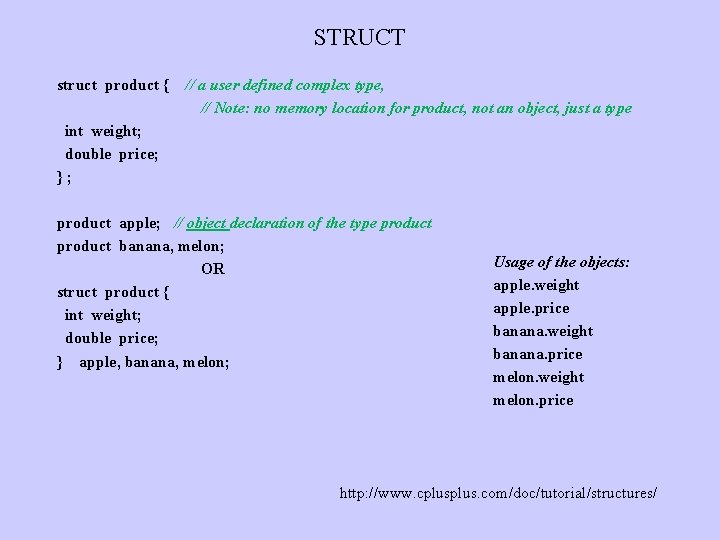
STRUCT struct product { // a user defined complex type, // Note: no memory location for product, not an object, just a type int weight; double price; }; product apple; // object declaration of the type product banana, melon; OR struct product { int weight; double price; } apple, banana, melon; Usage of the objects: apple. weight apple. price banana. weight banana. price melon. weight melon. price http: //www. cplus. com/doc/tutorial/structures/
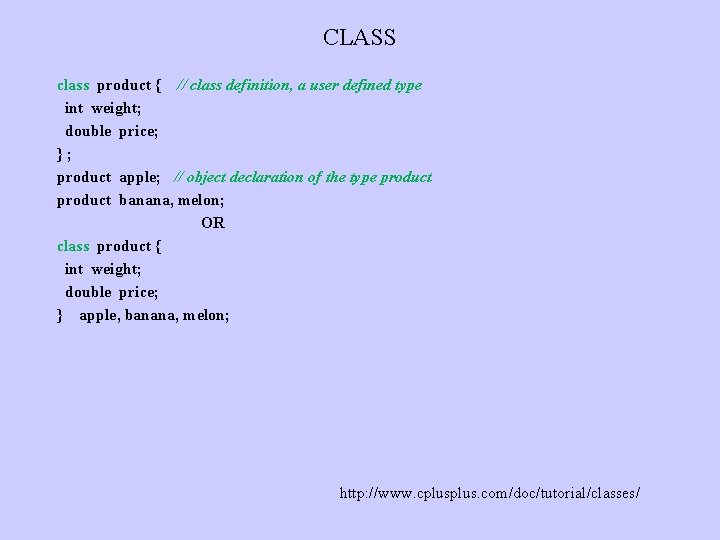
CLASS class product { // class definition, a user defined type int weight; double price; }; product apple; // object declaration of the type product banana, melon; OR class product { int weight; double price; } apple, banana, melon; http: //www. cplus. com/doc/tutorial/classes/
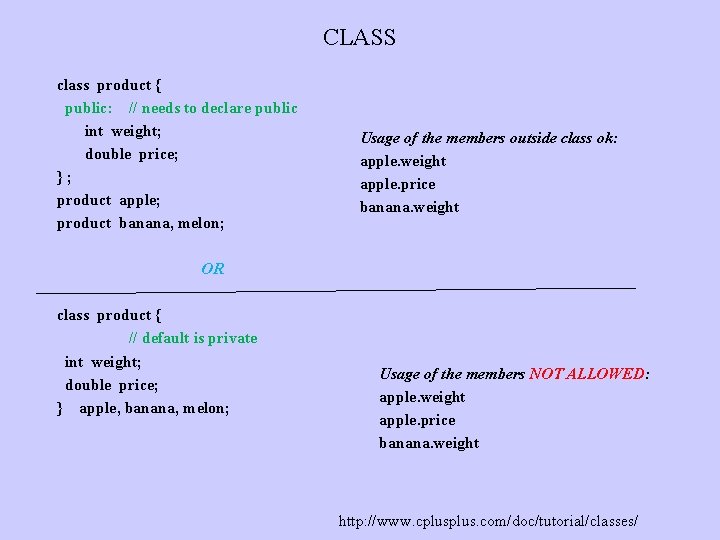
CLASS class product { public: // needs to declare public int weight; double price; }; product apple; product banana, melon; Usage of the members outside class ok: apple. weight apple. price banana. weight OR class product { // default is private int weight; double price; } apple, banana, melon; Usage of the members NOT ALLOWED: apple. weight apple. price banana. weight http: //www. cplus. com/doc/tutorial/classes/
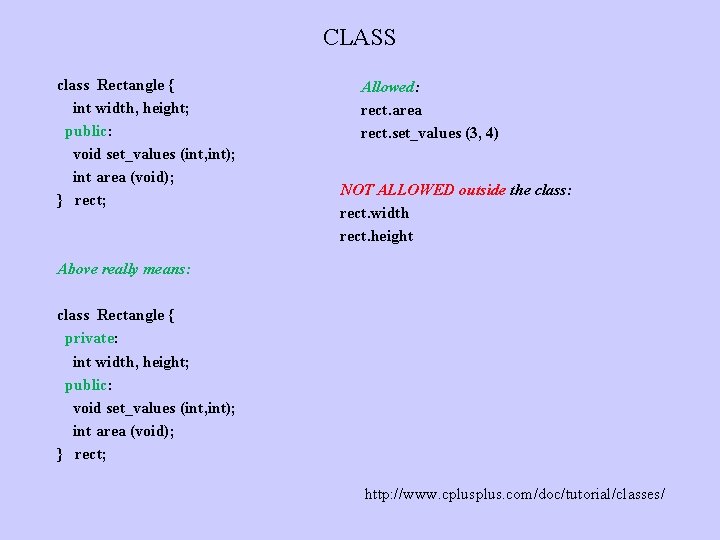
CLASS class Rectangle { int width, height; public: void set_values (int, int); int area (void); } rect; Allowed: rect. area rect. set_values (3, 4) NOT ALLOWED outside the class: rect. width rect. height Above really means: class Rectangle { private: int width, height; public: void set_values (int, int); int area (void); } rect; http: //www. cplus. com/doc/tutorial/classes/
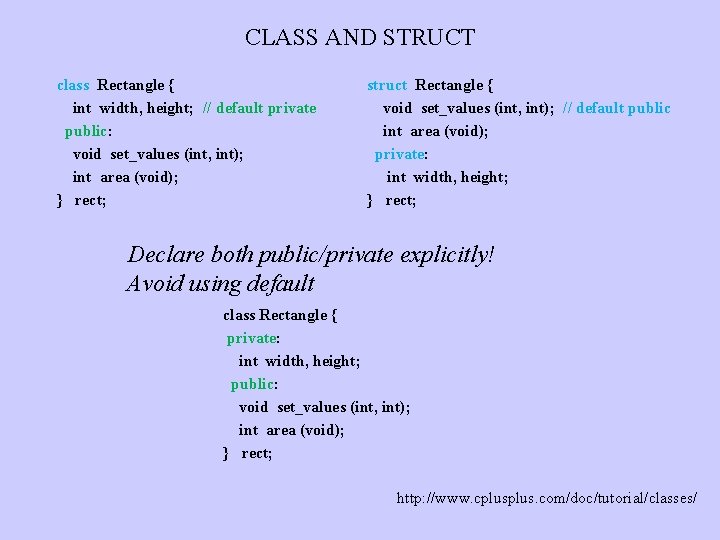
CLASS AND STRUCT class Rectangle { int width, height; // default private public: void set_values (int, int); int area (void); } rect; struct Rectangle { void set_values (int, int); // default public int area (void); private: int width, height; } rect; Declare both public/private explicitly! Avoid using default class Rectangle { private: int width, height; public: void set_values (int, int); int area (void); } rect; http: //www. cplus. com/doc/tutorial/classes/
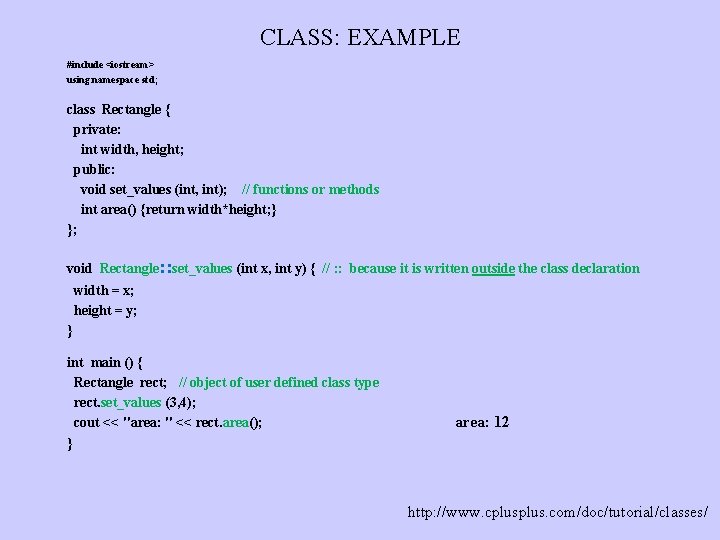
CLASS: EXAMPLE #include <iostream> using namespace std; class Rectangle { private: int width, height; public: void set_values (int, int); // functions or methods int area() {return width*height; } }; void Rectangle: : set_values (int x, int y) { // : : because it is written outside the class declaration width = x; height = y; } int main () { Rectangle rect; // object of user defined class type rect. set_values (3, 4); cout << "area: " << rect. area(); } area: 12 http: //www. cplus. com/doc/tutorial/classes/
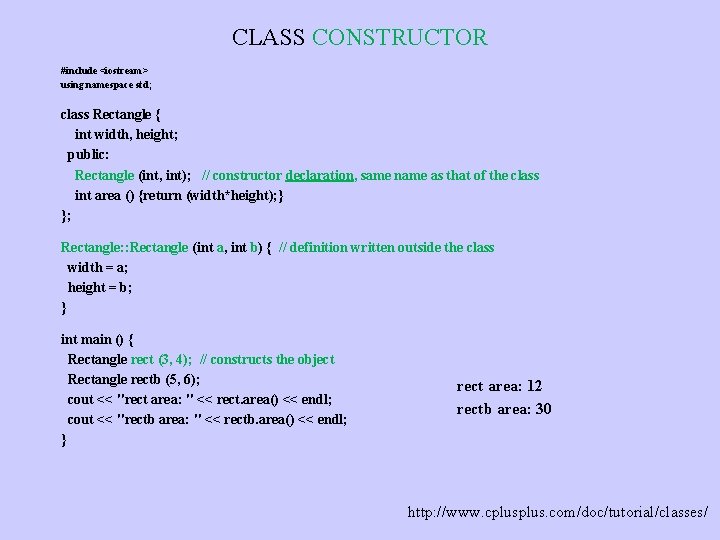
CLASS CONSTRUCTOR #include <iostream> using namespace std; class Rectangle { int width, height; public: Rectangle (int, int); // constructor declaration, same name as that of the class int area () {return (width*height); } }; Rectangle: : Rectangle (int a, int b) { // definition written outside the class width = a; height = b; } int main () { Rectangle rect (3, 4); // constructs the object Rectangle rectb (5, 6); cout << "rect area: " << rect. area() << endl; cout << "rectb area: " << rectb. area() << endl; } rect area: 12 rectb area: 30 http: //www. cplus. com/doc/tutorial/classes/
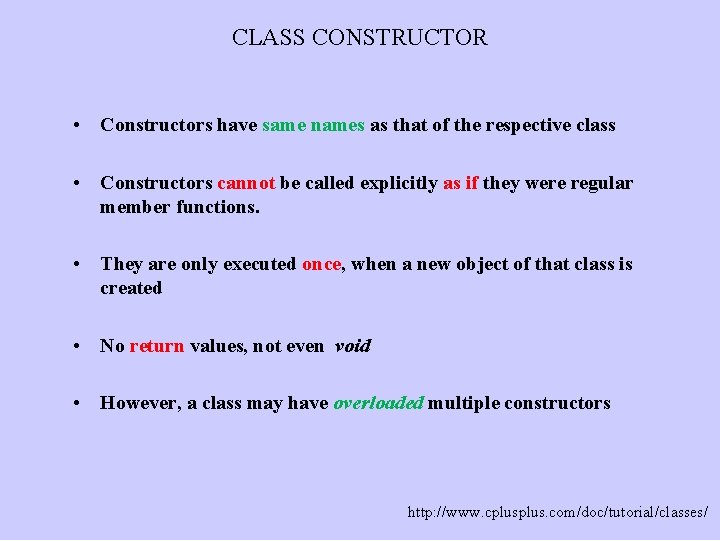
CLASS CONSTRUCTOR • Constructors have same names as that of the respective class • Constructors cannot be called explicitly as if they were regular member functions. • They are only executed once, when a new object of that class is created • No return values, not even void • However, a class may have overloaded multiple constructors http: //www. cplus. com/doc/tutorial/classes/
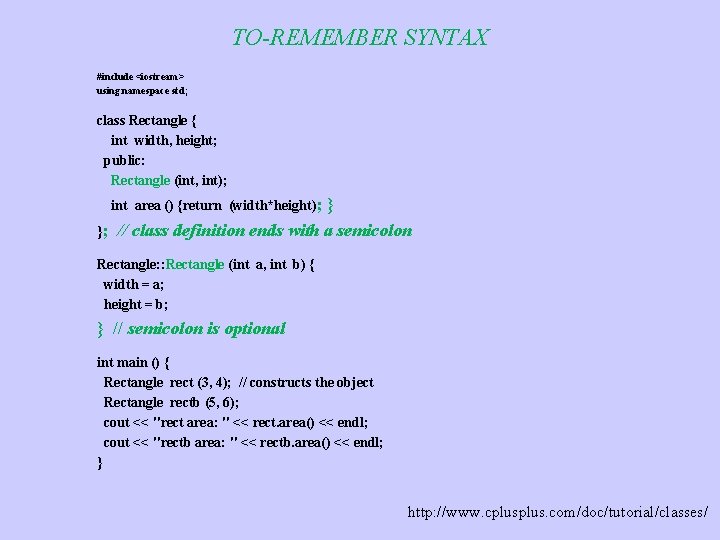
TO-REMEMBER SYNTAX #include <iostream> using namespace std; class Rectangle { int width, height; public: Rectangle (int, int); int area () {return (width*height); } }; // class definition ends with a semicolon Rectangle: : Rectangle (int a, int b) { width = a; height = b; } // semicolon is optional int main () { Rectangle rect (3, 4); // constructs the object Rectangle rectb (5, 6); cout << "rect area: " << rect. area() << endl; cout << "rectb area: " << rectb. area() << endl; } http: //www. cplus. com/doc/tutorial/classes/
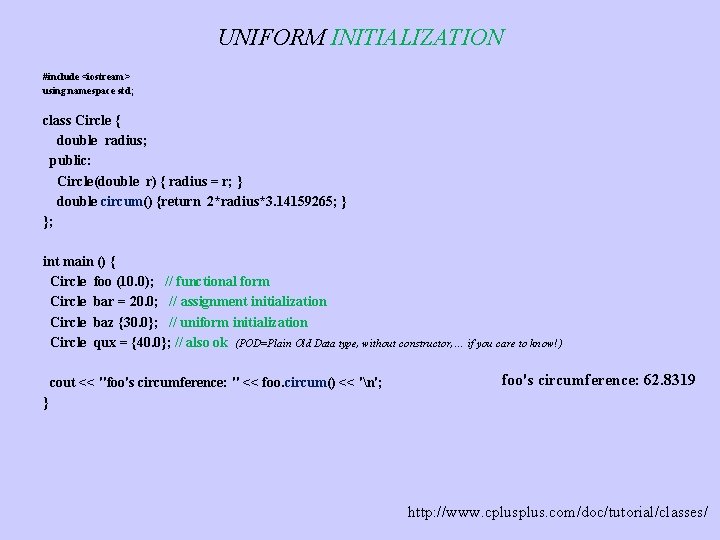
UNIFORM INITIALIZATION #include <iostream> using namespace std; class Circle { double radius; public: Circle(double r) { radius = r; } double circum() {return 2*radius*3. 14159265; } }; int main () { Circle foo (10. 0); // functional form Circle bar = 20. 0; // assignment initialization Circle baz {30. 0}; // uniform initialization Circle qux = {40. 0}; // also ok (POD=Plain Old Data type, without constructor, … if you care to know!) cout << "foo's circumference: " << foo. circum() << 'n'; foo's circumference: 62. 8319 } http: //www. cplus. com/doc/tutorial/classes/
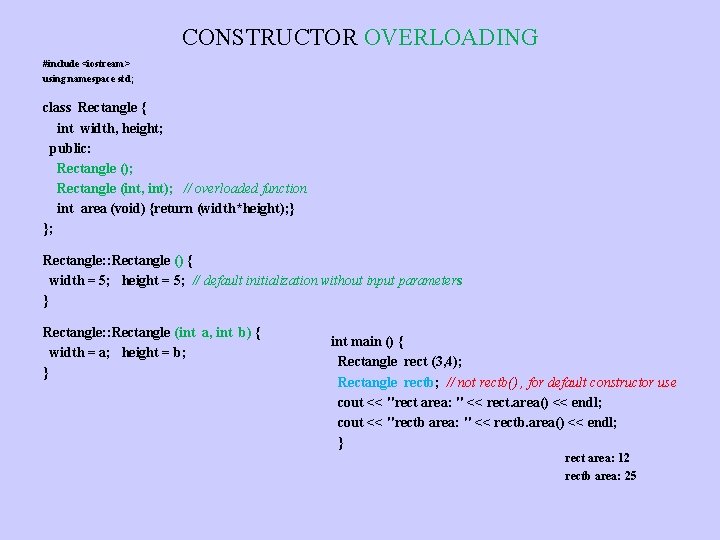
CONSTRUCTOR OVERLOADING #include <iostream> using namespace std; class Rectangle { int width, height; public: Rectangle (); Rectangle (int, int); // overloaded function int area (void) {return (width*height); } }; Rectangle: : Rectangle () { width = 5; height = 5; // default initialization without input parameters } Rectangle: : Rectangle (int a, int b) { width = a; height = b; } int main () { Rectangle rect (3, 4); Rectangle rectb; // not rectb() , for default constructor use cout << "rect area: " << rect. area() << endl; cout << "rectb area: " << rectb. area() << endl; } rect area: 12 rectb area: 25
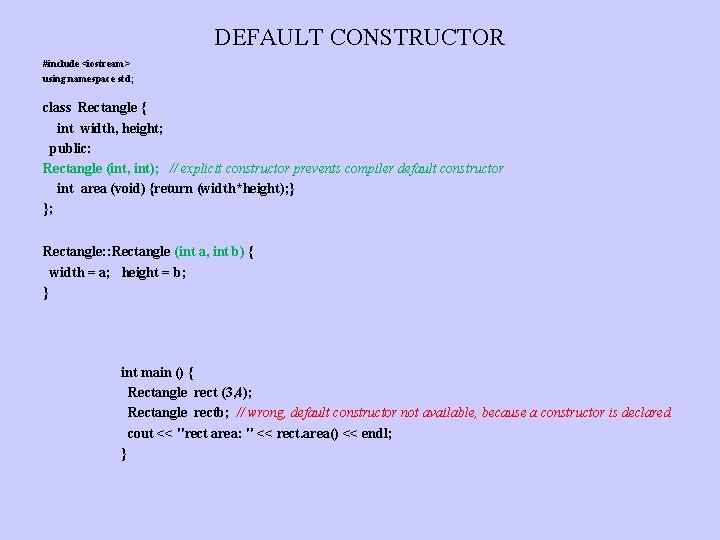
DEFAULT CONSTRUCTOR #include <iostream> using namespace std; class Rectangle { int width, height; public: Rectangle (int, int); // explicit constructor prevents compiler default constructor int area (void) {return (width*height); } }; Rectangle: : Rectangle (int a, int b) { width = a; height = b; } int main () { Rectangle rect (3, 4); Rectangle rectb; // wrong, default constructor not available, because a constructor is declared cout << "rect area: " << rect. area() << endl; }
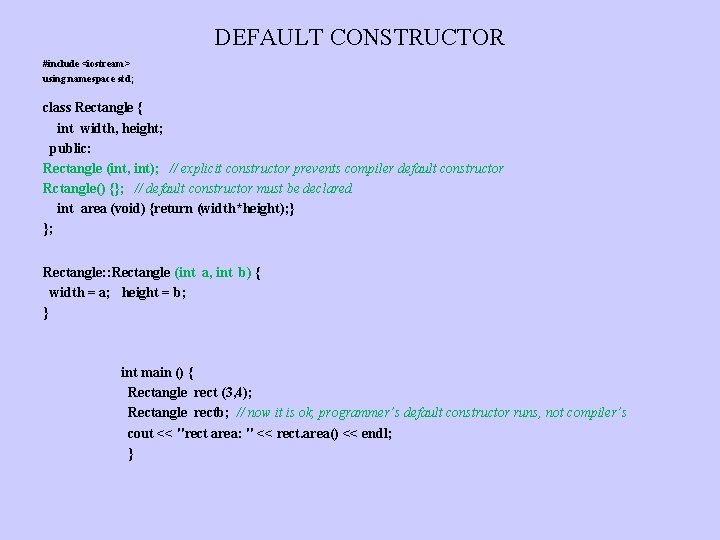
DEFAULT CONSTRUCTOR #include <iostream> using namespace std; class Rectangle { int width, height; public: Rectangle (int, int); // explicit constructor prevents compiler default constructor Rctangle() {}; // default constructor must be declared int area (void) {return (width*height); } }; Rectangle: : Rectangle (int a, int b) { width = a; height = b; } int main () { Rectangle rect (3, 4); Rectangle rectb; // now it is ok, programmer’s default constructor runs, not compiler’s cout << "rect area: " << rect. area() << endl; }
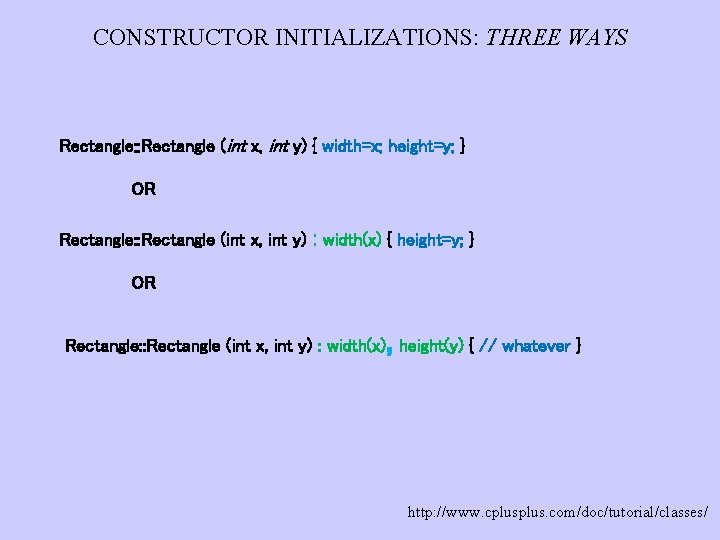
CONSTRUCTOR INITIALIZATIONS: THREE WAYS Rectangle: : Rectangle (int x, int y) { width=x; height=y; } OR Rectangle: : Rectangle (int x, int y) : width(x) { height=y; } OR , Rectangle: : Rectangle (int x, int y) : width(x) height(y) { // whatever } http: //www. cplus. com/doc/tutorial/classes/
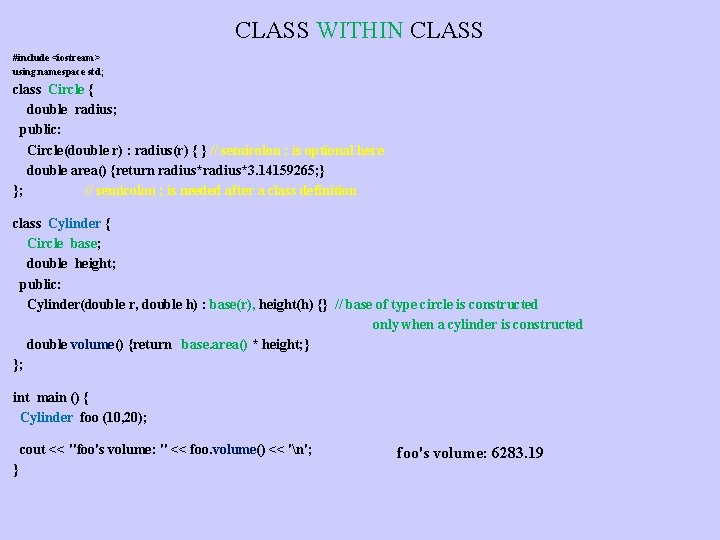
CLASS WITHIN CLASS #include <iostream> using namespace std; class Circle { double radius; public: Circle(double r) : radius(r) { } // semicolon ; is optional here double area() {return radius*3. 14159265; } }; // semicolon ; is needed after a class definition class Cylinder { Circle base; double height; public: Cylinder(double r, double h) : base(r), height(h) {} // base of type circle is constructed only when a cylinder is constructed double volume() {return base. area() * height; } }; int main () { Cylinder foo (10, 20); cout << "foo's volume: " << foo. volume() << 'n'; } foo's volume: 6283. 19
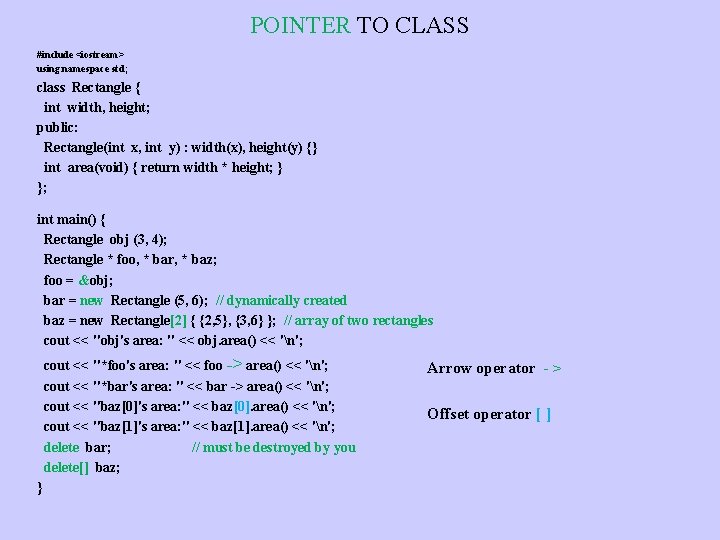
POINTER TO CLASS #include <iostream> using namespace std; class Rectangle { int width, height; public: Rectangle(int x, int y) : width(x), height(y) {} int area(void) { return width * height; } }; int main() { Rectangle obj (3, 4); Rectangle * foo, * bar, * baz; foo = &obj; bar = new Rectangle (5, 6); // dynamically created baz = new Rectangle[2] { {2, 5}, {3, 6} }; // array of two rectangles cout << "obj's area: " << obj. area() << 'n'; cout << "*foo's area: " << foo -> area() << 'n'; cout << "*bar's area: " << bar -> area() << 'n'; cout << "baz[0]'s area: " << baz[0]. area() << 'n'; cout << "baz[1]'s area: " << baz[1]. area() << 'n'; delete bar; // must be destroyed by you delete[] baz; } Arrow operator - > Offset operator [ ]
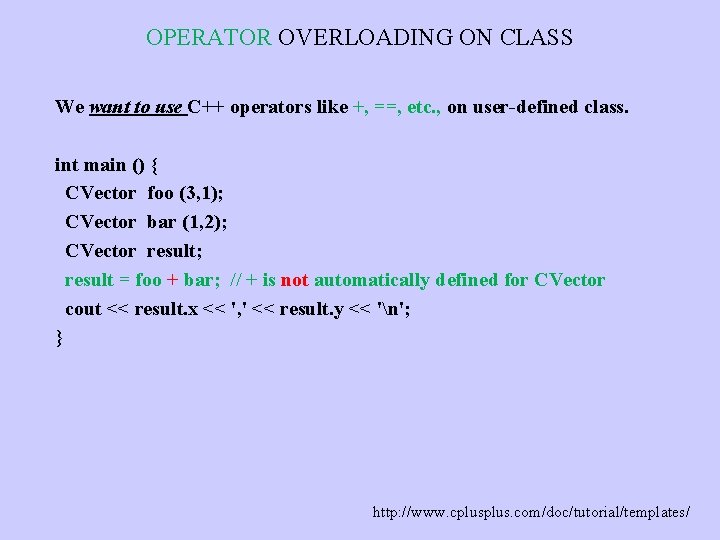
OPERATOR OVERLOADING ON CLASS We want to use C++ operators like +, ==, etc. , on user-defined class. int main () { CVector foo (3, 1); CVector bar (1, 2); CVector result; result = foo + bar; // + is not automatically defined for CVector cout << result. x << ', ' << result. y << 'n'; } http: //www. cplus. com/doc/tutorial/templates/
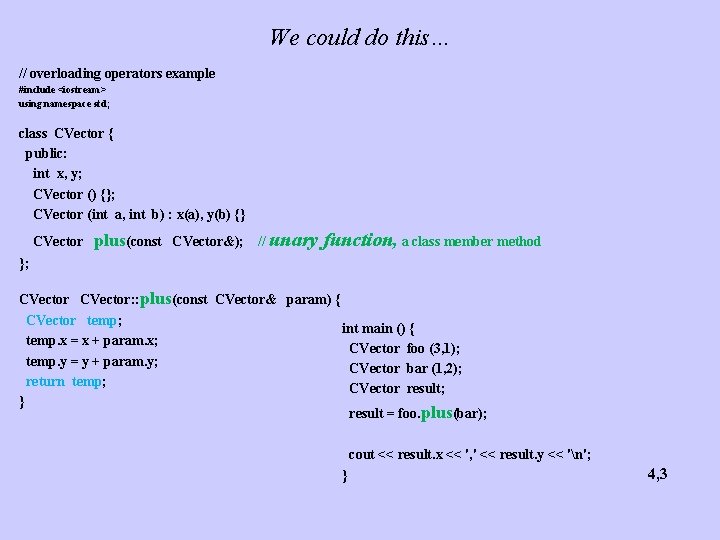
We could do this… // overloading operators example #include <iostream> using namespace std; class CVector { public: int x, y; CVector () {}; CVector (int a, int b) : x(a), y(b) {} CVector plus(const CVector&); // unary function, a class member method }; CVector: : plus(const CVector& param) { CVector temp; temp. x = x + param. x; temp. y = y + param. y; return temp; } int main () { CVector foo (3, 1); CVector bar (1, 2); CVector result; result = foo. plus(bar); cout << result. x << ', ' << result. y << 'n'; } 4, 3
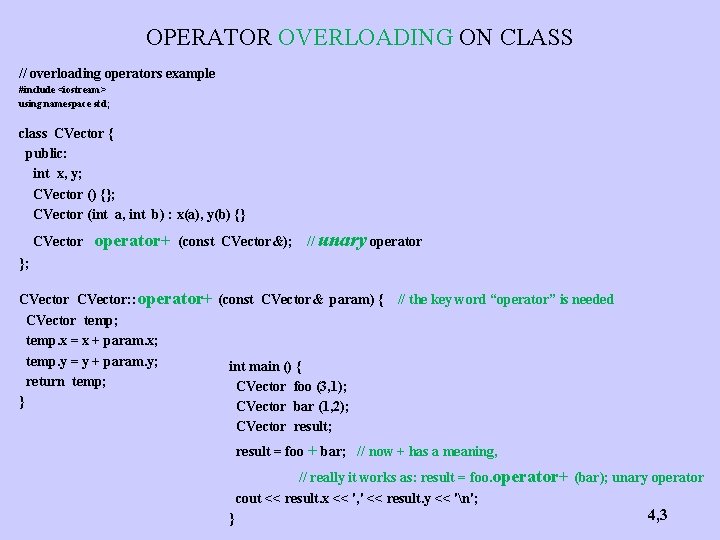
OPERATOR OVERLOADING ON CLASS // overloading operators example #include <iostream> using namespace std; class CVector { public: int x, y; CVector () {}; CVector (int a, int b) : x(a), y(b) {} CVector operator+ (const CVector&); // unary operator }; CVector: : operator+ (const CVector& param) { CVector temp; temp. x = x + param. x; temp. y = y + param. y; return temp; } // the key word “operator” is needed int main () { CVector foo (3, 1); CVector bar (1, 2); CVector result; result = foo + bar; // now + has a meaning, // really it works as: result = foo. operator+ (bar); unary operator cout << result. x << ', ' << result. y << 'n'; } 4, 3
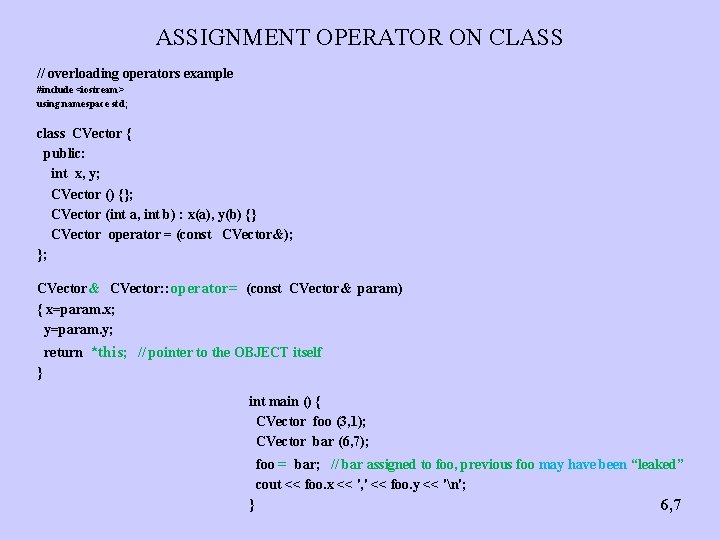
ASSIGNMENT OPERATOR ON CLASS // overloading operators example #include <iostream> using namespace std; class CVector { public: int x, y; CVector () {}; CVector (int a, int b) : x(a), y(b) {} CVector operator = (const CVector&); }; CVector& CVector: : operator= (const CVector& param) { x=param. x; y=param. y; return *this; // pointer to the OBJECT itself } int main () { CVector foo (3, 1); CVector bar (6, 7); foo = bar; // bar assigned to foo, previous foo may have been “leaked” cout << foo. x << ', ' << foo. y << 'n'; } 6, 7
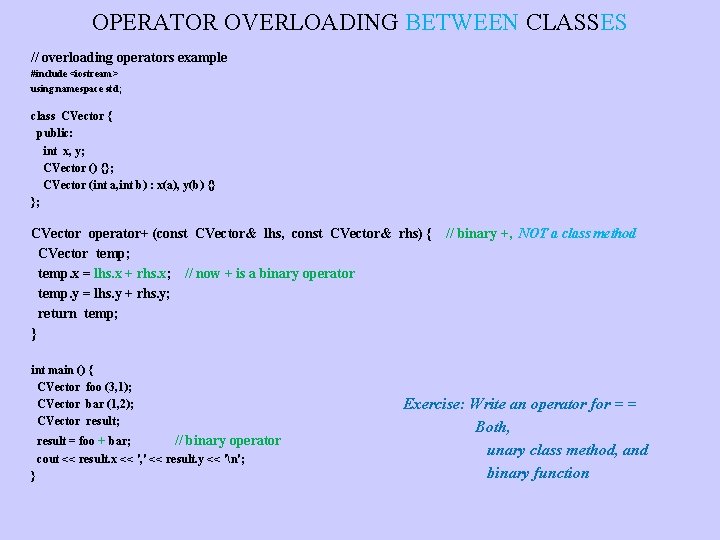
OPERATOR OVERLOADING BETWEEN CLASSES // overloading operators example #include <iostream> using namespace std; class CVector { public: int x, y; CVector () {}; CVector (int a, int b) : x(a), y(b) {} }; CVector operator+ (const CVector& lhs, const CVector& rhs) { CVector temp; temp. x = lhs. x + rhs. x; // now + is a binary operator temp. y = lhs. y + rhs. y; return temp; } int main () { CVector foo (3, 1); CVector bar (1, 2); CVector result; result = foo + bar; // binary operator cout << result. x << ', ' << result. y << 'n'; } // binary +, NOT a class method Exercise: Write an operator for = = Both, unary class method, and binary function
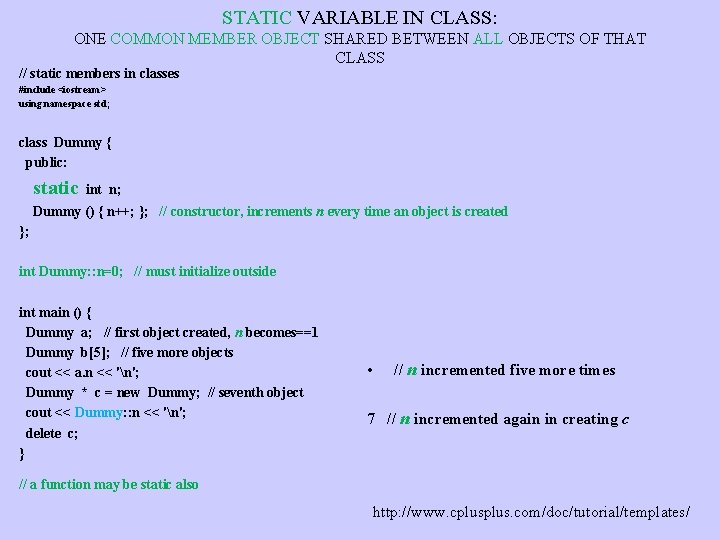
STATIC VARIABLE IN CLASS: ONE COMMON MEMBER OBJECT SHARED BETWEEN ALL OBJECTS OF THAT CLASS // static members in classes #include <iostream> using namespace std; class Dummy { public: static int n; Dummy () { n++; }; // constructor, increments n every time an object is created }; int Dummy: : n=0; // must initialize outside int main () { Dummy a; // first object created, n becomes==1 Dummy b[5]; // five more objects cout << a. n << 'n'; Dummy * c = new Dummy; // seventh object cout << Dummy: : n << 'n'; delete c; } • // n incremented five more times 7 // n incremented again in creating c // a function may be static also http: //www. cplus. com/doc/tutorial/templates/
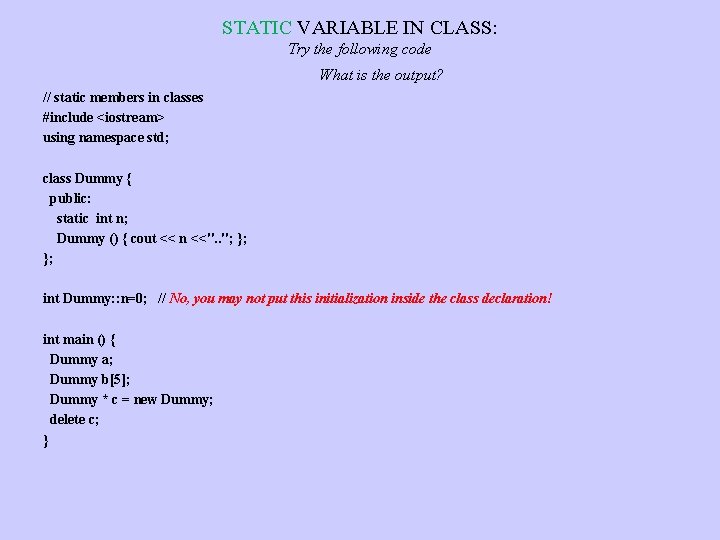
STATIC VARIABLE IN CLASS: Try the following code What is the output? // static members in classes #include <iostream> using namespace std; class Dummy { public: static int n; Dummy () { cout << n <<". . "; }; }; int Dummy: : n=0; // No, you may not put this initialization inside the class declaration! int main () { Dummy a; Dummy b[5]; Dummy * c = new Dummy; delete c; }
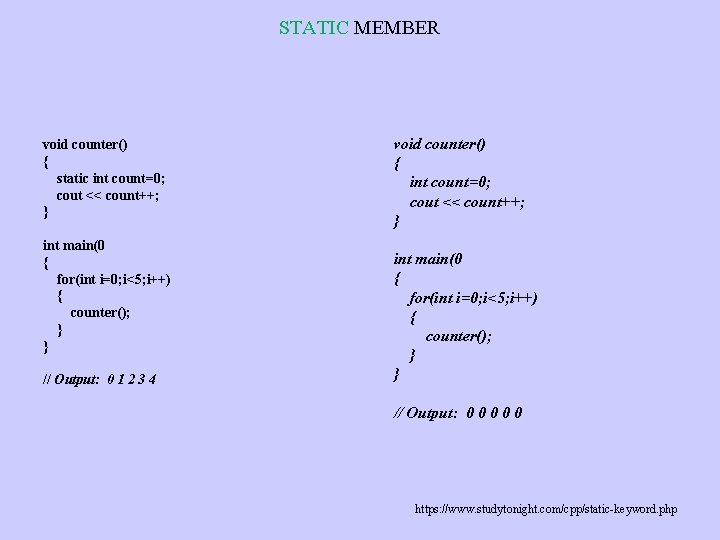
STATIC MEMBER void counter() { static int count=0; cout << count++; } int main(0 { for(int i=0; i<5; i++) { counter(); } } // Output: 0 1 2 3 4 void counter() { int count=0; cout << count++; } int main(0 { for(int i=0; i<5; i++) { counter(); } } // Output: 0 0 0 https: //www. studytonight. com/cpp/static-keyword. php
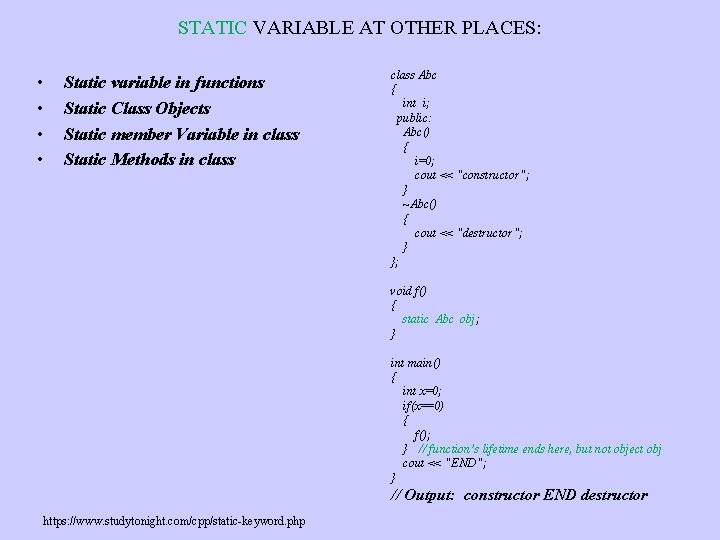
STATIC VARIABLE AT OTHER PLACES: • • Static variable in functions Static Class Objects Static member Variable in class Static Methods in class Abc { int i; public: Abc() { i=0; cout << "constructor"; } ~Abc() { cout << "destructor"; } }; void f() { static Abc obj; } int main() { int x=0; if(x==0) { f(); } // function’s lifetime ends here, but not object obj cout << "END"; } // Output: constructor END destructor https: //www. studytonight. com/cpp/static-keyword. php
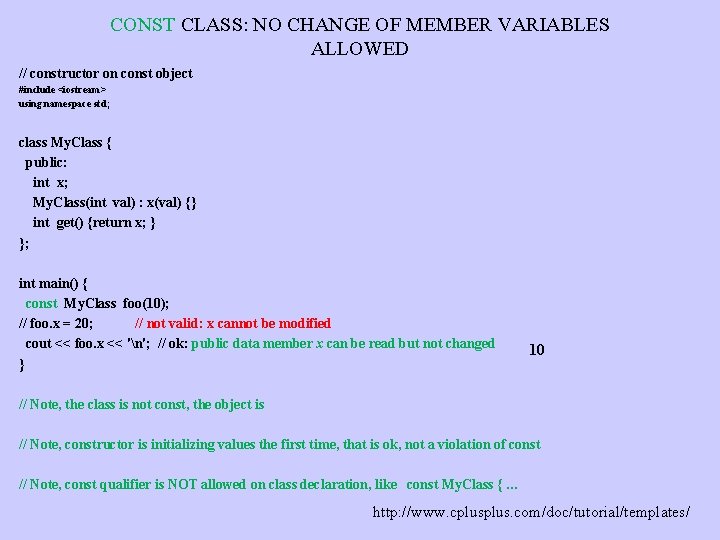
CONST CLASS: NO CHANGE OF MEMBER VARIABLES ALLOWED // constructor on const object #include <iostream> using namespace std; class My. Class { public: int x; My. Class(int val) : x(val) {} int get() {return x; } }; int main() { const My. Class foo(10); // foo. x = 20; // not valid: x cannot be modified cout << foo. x << 'n'; // ok: public data member x can be read but not changed } 10 // Note, the class is not const, the object is // Note, constructor is initializing values the first time, that is ok, not a violation of const // Note, const qualifier is NOT allowed on class declaration, like const My. Class { … http: //www. cplus. com/doc/tutorial/templates/
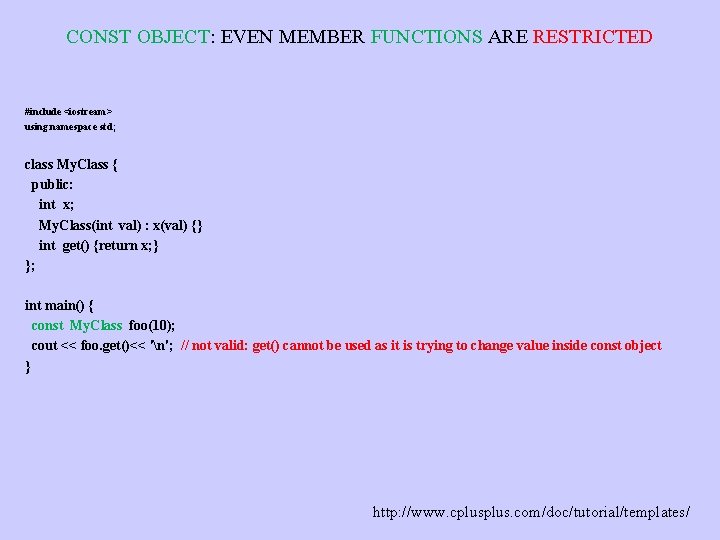
CONST OBJECT: EVEN MEMBER FUNCTIONS ARE RESTRICTED #include <iostream> using namespace std; class My. Class { public: int x; My. Class(int val) : x(val) {} int get() {return x; } }; int main() { const My. Class foo(10); cout << foo. get()<< 'n'; // not valid: get() cannot be used as it is trying to change value inside const object } http: //www. cplus. com/doc/tutorial/templates/
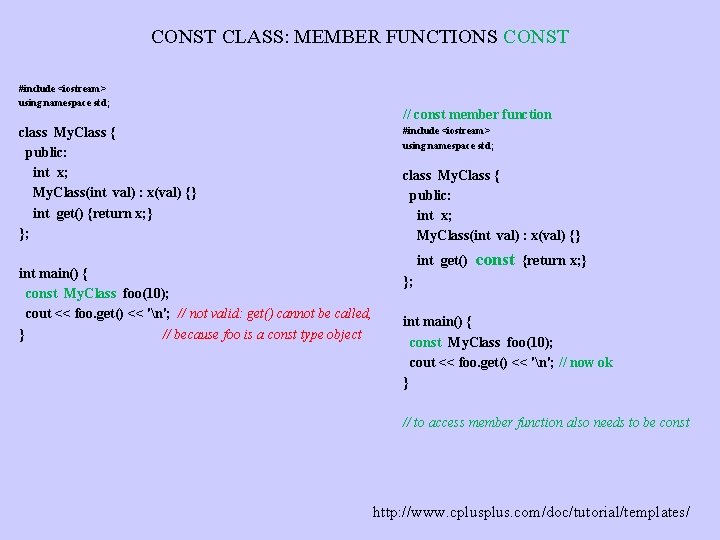
CONST CLASS: MEMBER FUNCTIONS CONST #include <iostream> using namespace std; class My. Class { public: int x; My. Class(int val) : x(val) {} int get() {return x; } }; int main() { const My. Class foo(10); cout << foo. get() << 'n'; // not valid: get() cannot be called, } // because foo is a const type object // const member function #include <iostream> using namespace std; class My. Class { public: int x; My. Class(int val) : x(val) {} int get() const {return x; } }; int main() { const My. Class foo(10); cout << foo. get() << 'n'; // now ok } // to access member function also needs to be const http: //www. cplus. com/doc/tutorial/templates/
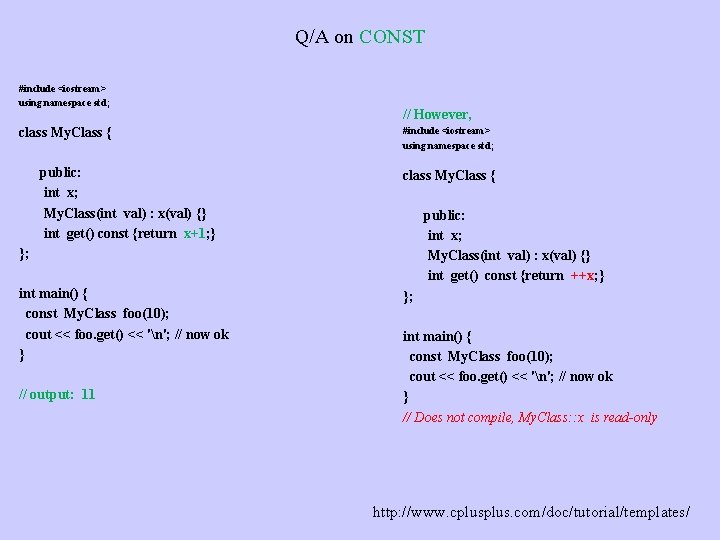
Q/A on CONST #include <iostream> using namespace std; class My. Class { public: int x; My. Class(int val) : x(val) {} int get() const {return x+1; } // However, #include <iostream> using namespace std; class My. Class { public: int x; My. Class(int val) : x(val) {} int get() const {return ++x; } }; int main() { const My. Class foo(10); cout << foo. get() << 'n'; // now ok } // output: 11 }; int main() { const My. Class foo(10); cout << foo. get() << 'n'; // now ok } // Does not compile, My. Class: : x is read-only http: //www. cplus. com/doc/tutorial/templates/
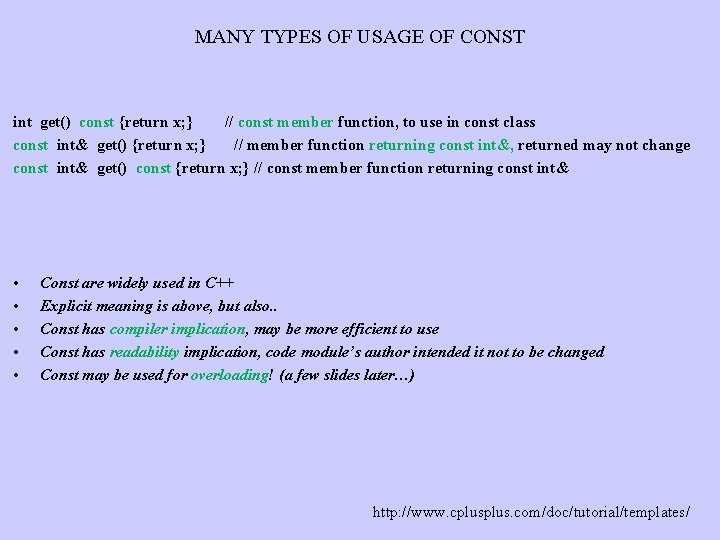
MANY TYPES OF USAGE OF CONST int get() const {return x; } // const member function, to use in const class const int& get() {return x; } // member function returning const int&, returned may not change const int& get() const {return x; } // const member function returning const int& • • • Const are widely used in C++ Explicit meaning is above, but also. . Const has compiler implication, may be more efficient to use Const has readability implication, code module’s author intended it not to be changed Const may be used for overloading! (a few slides later…) http: //www. cplus. com/doc/tutorial/templates/
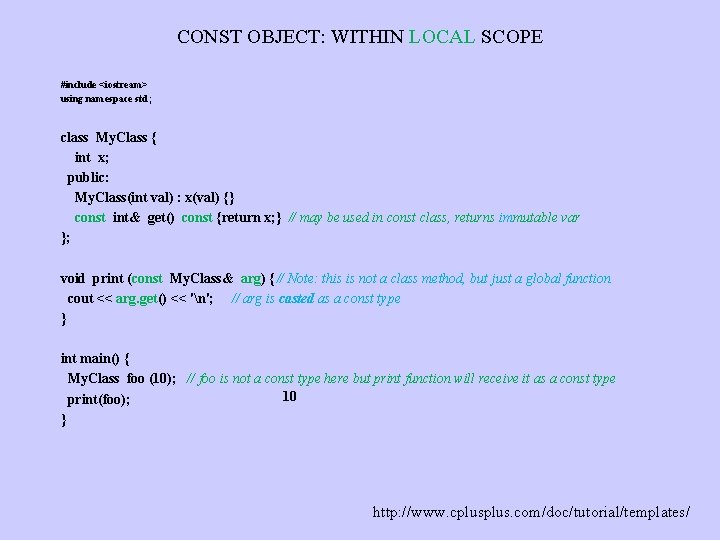
CONST OBJECT: WITHIN LOCAL SCOPE #include <iostream> using namespace std; class My. Class { int x; public: My. Class(int val) : x(val) {} const int& get() const {return x; } // may be used in const class, returns immutable var }; void print (const My. Class& arg) { // Note: this is not a class method, but just a global function cout << arg. get() << 'n'; // arg is casted as a const type } int main() { My. Class foo (10); // foo is not a const type here but print function will receive it as a const type 10 print(foo); } http: //www. cplus. com/doc/tutorial/templates/
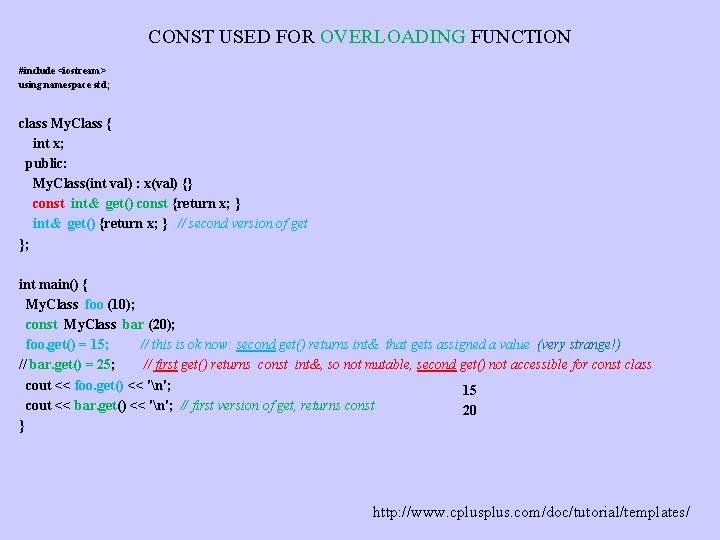
CONST USED FOR OVERLOADING FUNCTION #include <iostream> using namespace std; class My. Class { int x; public: My. Class(int val) : x(val) {} const int& get() const {return x; } int& get() {return x; } // second version of get }; int main() { My. Class foo (10); const My. Class bar (20); foo. get() = 15; // this is ok now: second get() returns int& that gets assigned a value (very strange!) // bar. get() = 25; // first get() returns const int&, so not mutable, second get() not accessible for const class cout << foo. get() << 'n'; 15 cout << bar. get() << 'n'; // first version of get, returns const 20 } http: //www. cplus. com/doc/tutorial/templates/
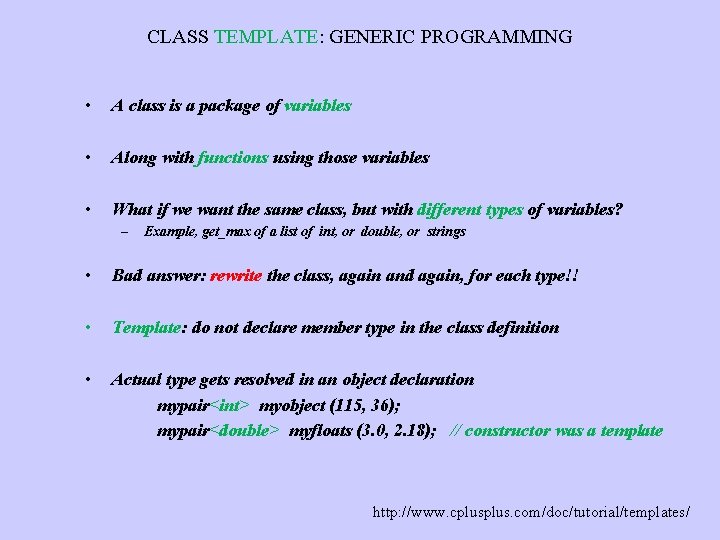
CLASS TEMPLATE: GENERIC PROGRAMMING • A class is a package of variables • Along with functions using those variables • What if we want the same class, but with different types of variables? – Example, get_max of a list of int, or double, or strings • Bad answer: rewrite the class, again and again, for each type!! • Template: do not declare member type in the class definition • Actual type gets resolved in an object declaration mypair<int> myobject (115, 36); mypair<double> myfloats (3. 0, 2. 18); // constructor was a template http: //www. cplus. com/doc/tutorial/templates/
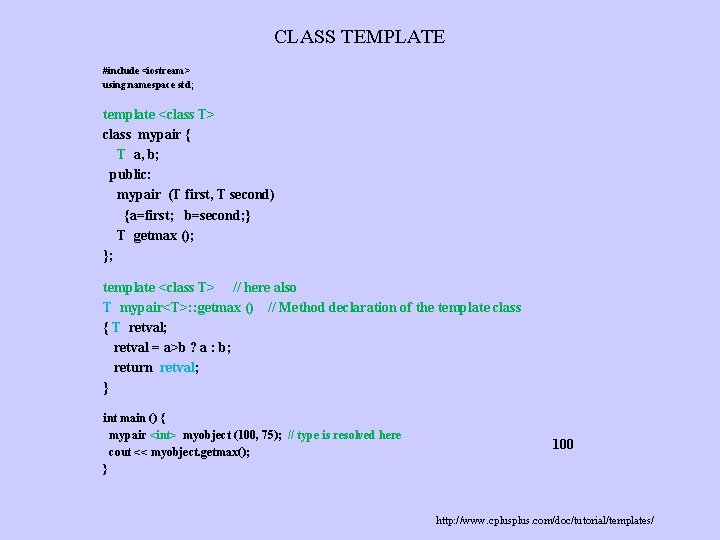
CLASS TEMPLATE #include <iostream> using namespace std; template <class T> class mypair { T a, b; public: mypair (T first, T second) {a=first; b=second; } T getmax (); }; template <class T> // here also T mypair<T>: : getmax () // Method declaration of the template class { T retval; retval = a>b ? a : b; return retval; } int main () { mypair <int> myobject (100, 75); // type is resolved here cout << myobject. getmax(); } 100 http: //www. cplus. com/doc/tutorial/templates/
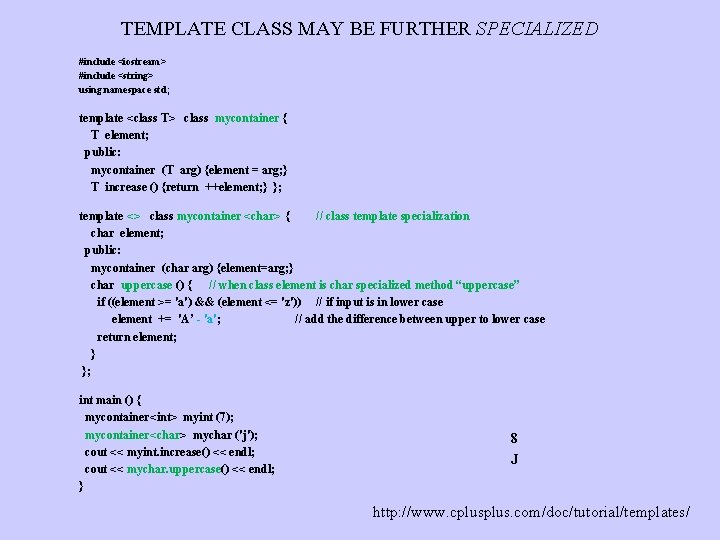
TEMPLATE CLASS MAY BE FURTHER SPECIALIZED #include <iostream> #include <string> using namespace std; template <class T> class mycontainer { T element; public: mycontainer (T arg) {element = arg; } T increase () {return ++element; } }; template <> class mycontainer <char> { // class template specialization char element; public: mycontainer (char arg) {element=arg; } char uppercase () { // when class element is char specialized method “uppercase” if ((element >= 'a') && (element <= 'z')) // if input is in lower case element += 'A’ - 'a'; // add the difference between upper to lower case return element; } }; int main () { mycontainer<int> myint (7); mycontainer<char> mychar ('j'); cout << myint. increase() << endl; cout << mychar. uppercase() << endl; } 8 J http: //www. cplus. com/doc/tutorial/templates/
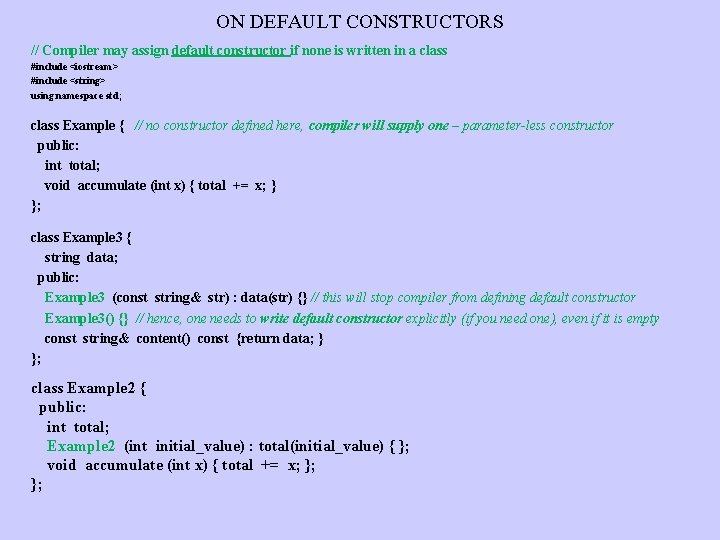
ON DEFAULT CONSTRUCTORS // Compiler may assign default constructor if none is written in a class #include <iostream> #include <string> using namespace std; class Example { // no constructor defined here, compiler will supply one – parameter-less constructor public: int total; void accumulate (int x) { total += x; } }; class Example 3 { string data; public: Example 3 (const string& str) : data(str) {} // this will stop compiler from defining default constructor Example 3() {} // hence, one needs to write default constructor explicitly (if you need one), even if it is empty const string& content() const {return data; } }; class Example 2 { public: int total; Example 2 (int initial_value) : total(initial_value) { }; void accumulate (int x) { total += x; }; };
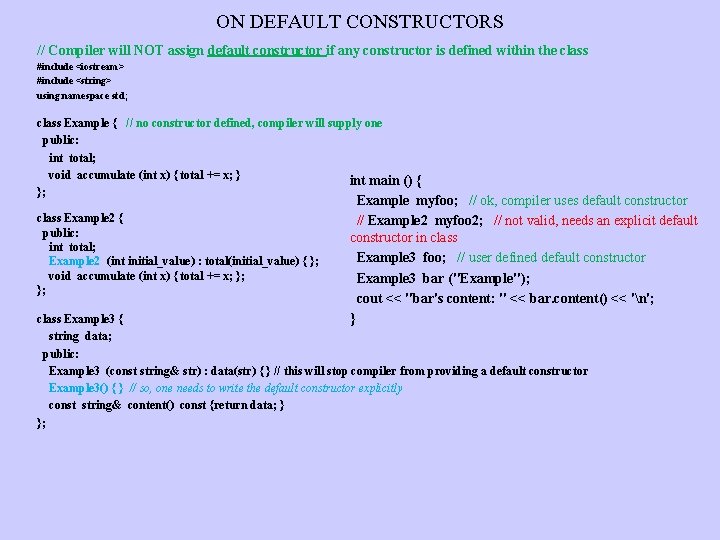
ON DEFAULT CONSTRUCTORS // Compiler will NOT assign default constructor if any constructor is defined within the class #include <iostream> #include <string> using namespace std; class Example { // no constructor defined, compiler will supply one public: int total; void accumulate (int x) { total += x; } int main () { }; class Example 2 { public: int total; Example 2 (int initial_value) : total(initial_value) { }; void accumulate (int x) { total += x; }; }; Example myfoo; // ok, compiler uses default constructor // Example 2 myfoo 2; // not valid, needs an explicit default constructor in class Example 3 foo; // user defined default constructor Example 3 bar ("Example"); cout << "bar's content: " << bar. content() << 'n'; } class Example 3 { string data; public: Example 3 (const string& str) : data(str) { } // this will stop compiler from providing a default constructor Example 3() { } // so, one needs to write the default constructor explicitly const string& content() const {return data; } };
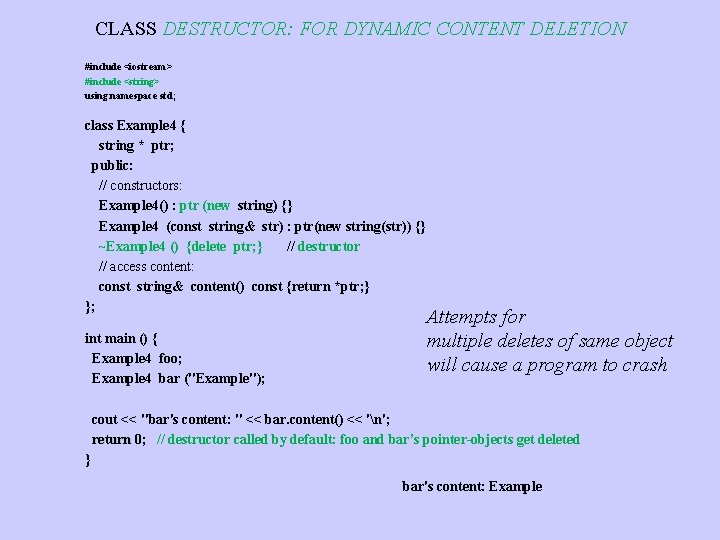
CLASS DESTRUCTOR: FOR DYNAMIC CONTENT DELETION #include <iostream> #include <string> using namespace std; class Example 4 { string * ptr; public: // constructors: Example 4() : ptr (new string) {} Example 4 (const string& str) : ptr(new string(str)) {} ~Example 4 () {delete ptr; } // destructor // access content: const string& content() const {return *ptr; } }; int main () { Example 4 foo; Example 4 bar ("Example"); Attempts for multiple deletes of same object will cause a program to crash cout << "bar's content: " << bar. content() << 'n'; return 0; // destructor called by default: foo and bar’s pointer-objects get deleted } bar's content: Example
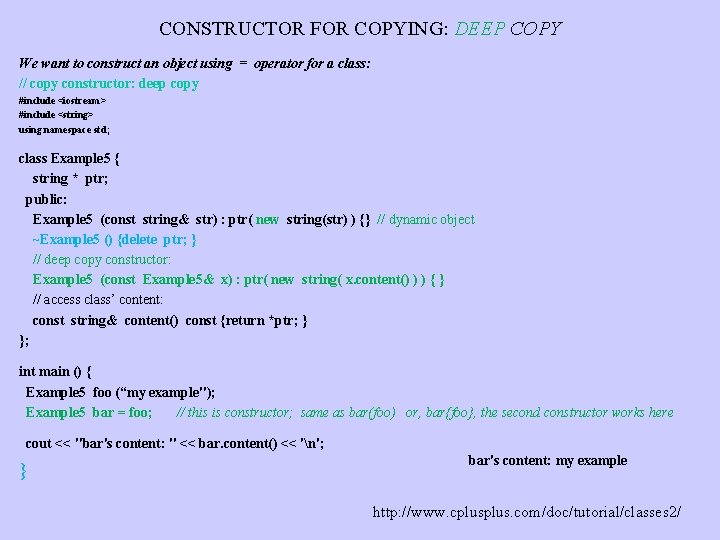
CONSTRUCTOR FOR COPYING: DEEP COPY We want to construct an object using = operator for a class: // copy constructor: deep copy #include <iostream> #include <string> using namespace std; class Example 5 { string * ptr; public: Example 5 (const string& str) : ptr( new string(str) ) {} // dynamic object ~Example 5 () {delete ptr; } // deep copy constructor: Example 5 (const Example 5& x) : ptr( new string( x. content() ) ) { } // access class’ content: const string& content() const {return *ptr; } }; int main () { Example 5 foo (“my example"); Example 5 bar = foo; // this is constructor; same as bar(foo) or, bar{foo}, the second constructor works here cout << "bar's content: " << bar. content() << 'n'; } bar's content: my example http: //www. cplus. com/doc/tutorial/classes 2/
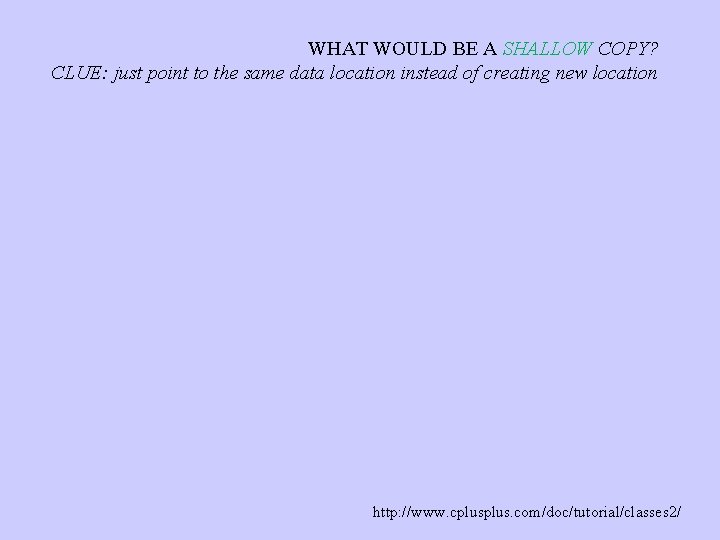
WHAT WOULD BE A SHALLOW COPY? CLUE: just point to the same data location instead of creating new location http: //www. cplus. com/doc/tutorial/classes 2/
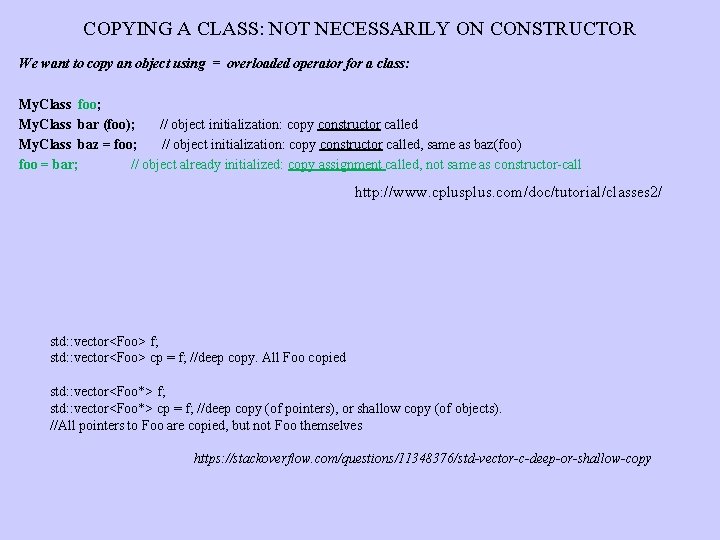
COPYING A CLASS: NOT NECESSARILY ON CONSTRUCTOR We want to copy an object using = overloaded operator for a class: My. Class foo; My. Class bar (foo); // object initialization: copy constructor called My. Class baz = foo; // object initialization: copy constructor called, same as baz(foo) foo = bar; // object already initialized: copy assignment called, not same as constructor-call http: //www. cplus. com/doc/tutorial/classes 2/ std: : vector<Foo> f; std: : vector<Foo> cp = f; //deep copy. All Foo copied std: : vector<Foo*> f; std: : vector<Foo*> cp = f; //deep copy (of pointers), or shallow copy (of objects). //All pointers to Foo are copied, but not Foo themselves https: //stackoverflow. com/questions/11348376/std-vector-c-deep-or-shallow-copy
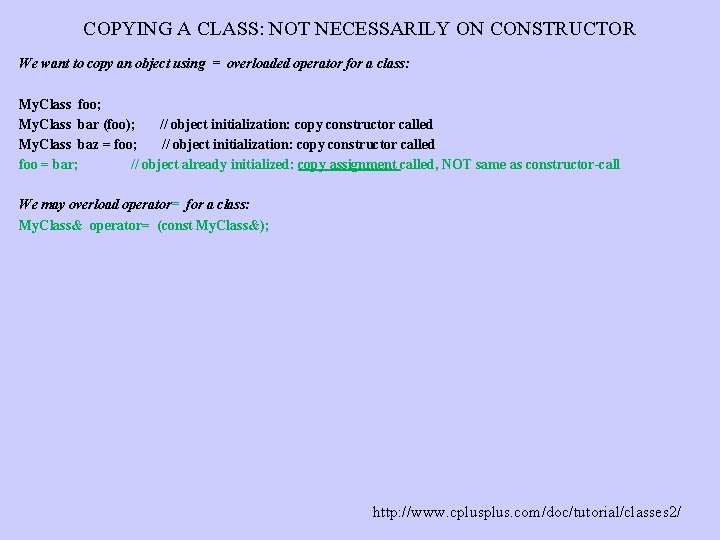
COPYING A CLASS: NOT NECESSARILY ON CONSTRUCTOR We want to copy an object using = overloaded operator for a class: My. Class foo; My. Class bar (foo); // object initialization: copy constructor called My. Class baz = foo; // object initialization: copy constructor called foo = bar; // object already initialized: copy assignment called, NOT same as constructor-call We may overload operator= for a class: My. Class& operator= (const My. Class&); http: //www. cplus. com/doc/tutorial/classes 2/
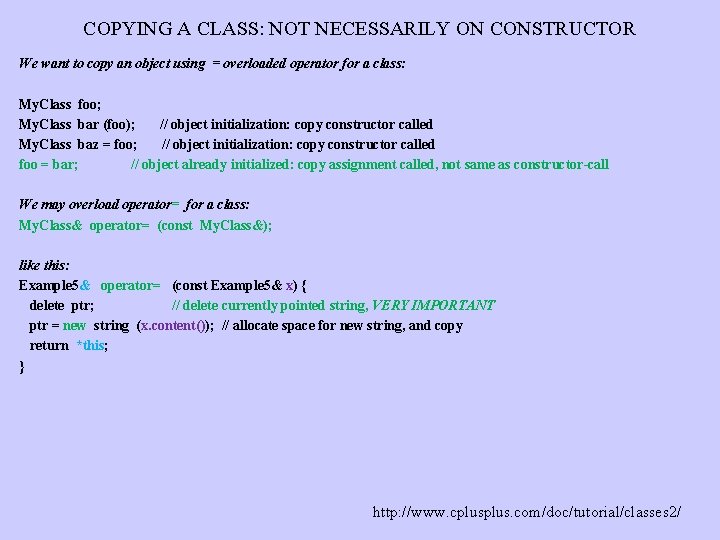
COPYING A CLASS: NOT NECESSARILY ON CONSTRUCTOR We want to copy an object using = overloaded operator for a class: My. Class foo; My. Class bar (foo); // object initialization: copy constructor called My. Class baz = foo; // object initialization: copy constructor called foo = bar; // object already initialized: copy assignment called, not same as constructor-call We may overload operator= for a class: My. Class& operator= (const My. Class&); like this: Example 5& operator= (const Example 5& x) { delete ptr; // delete currently pointed string, VERY IMPORTANT ptr = new string (x. content()); // allocate space for new string, and copy return *this; } http: //www. cplus. com/doc/tutorial/classes 2/
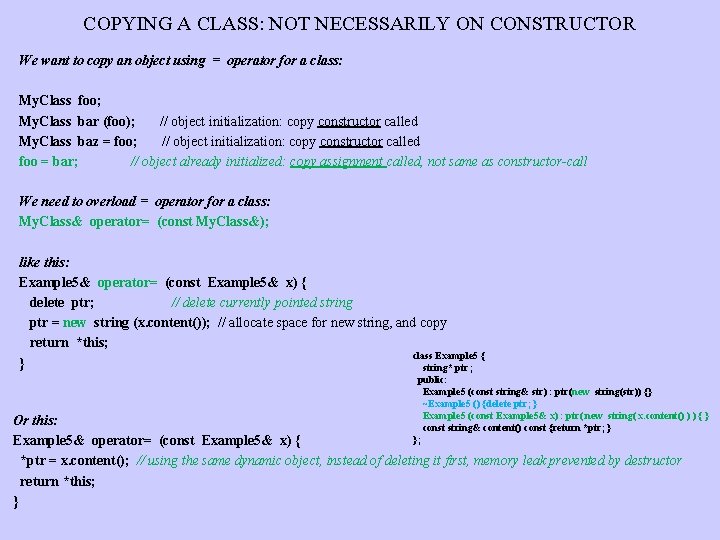
COPYING A CLASS: NOT NECESSARILY ON CONSTRUCTOR We want to copy an object using = operator for a class: My. Class foo; My. Class bar (foo); // object initialization: copy constructor called My. Class baz = foo; // object initialization: copy constructor called foo = bar; // object already initialized: copy assignment called, not same as constructor-call We need to overload = operator for a class: My. Class& operator= (const My. Class&); like this: Example 5& operator= (const Example 5& x) { delete ptr; // delete currently pointed string ptr = new string (x. content()); // allocate space for new string, and copy return *this; class Example 5 { } string* ptr; public: Example 5 (const string& str) : ptr(new string(str)) {} ~Example 5 () {delete ptr; } Example 5 (const Example 5& x) : ptr( new string( x. content() ) ) { } const string& content() const {return *ptr; } }; Or this: Example 5& operator= (const Example 5& x) { *ptr = x. content(); // using the same dynamic object, instead of deleting it first, memory leak prevented by destructor return *this; }
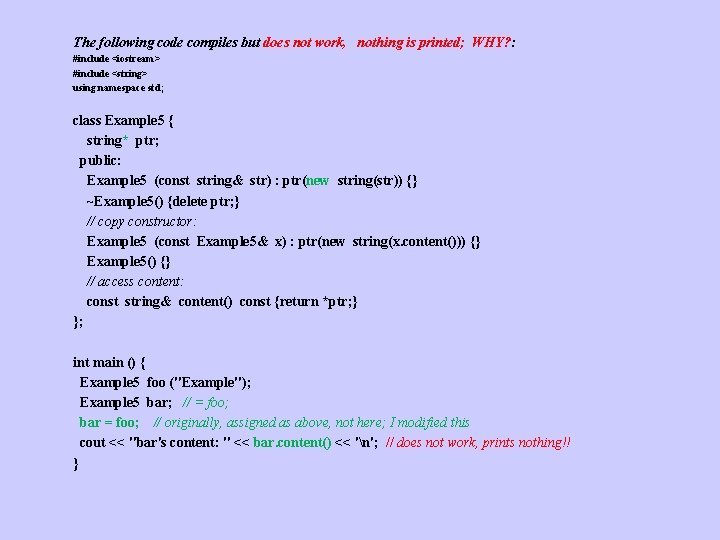
The following code compiles but does not work, nothing is printed; WHY? : #include <iostream> #include <string> using namespace std; class Example 5 { string* ptr; public: Example 5 (const string& str) : ptr(new string(str)) {} ~Example 5() {delete ptr; } // copy constructor: Example 5 (const Example 5& x) : ptr(new string(x. content())) {} Example 5() {} // access content: const string& content() const {return *ptr; } }; int main () { Example 5 foo ("Example"); Example 5 bar; // = foo; bar = foo; // originally, assigned as above, not here; I modified this cout << "bar's content: " << bar. content() << 'n'; // does not work, prints nothing!! }
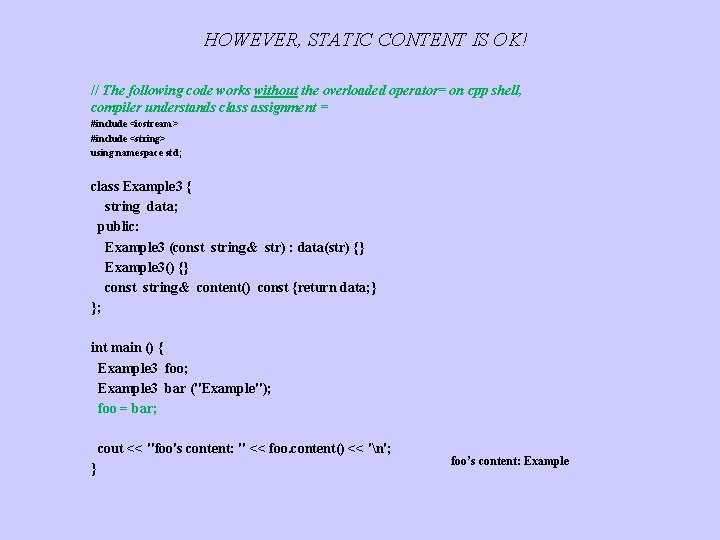
HOWEVER, STATIC CONTENT IS OK! // The following code works without the overloaded operator= on cpp shell, compiler understands class assignment = #include <iostream> #include <string> using namespace std; class Example 3 { string data; public: Example 3 (const string& str) : data(str) {} Example 3() {} const string& content() const {return data; } }; int main () { Example 3 foo; Example 3 bar ("Example"); foo = bar; cout << "foo's content: " << foo. content() << 'n'; } foo’s content: Example
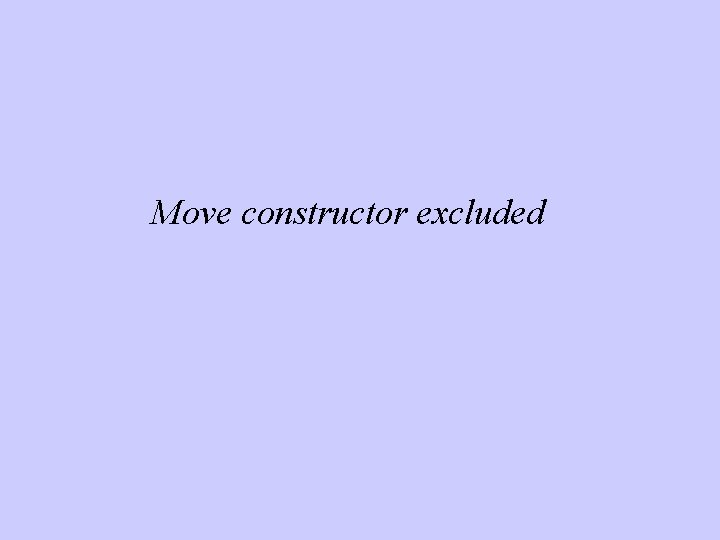
Move constructor excluded
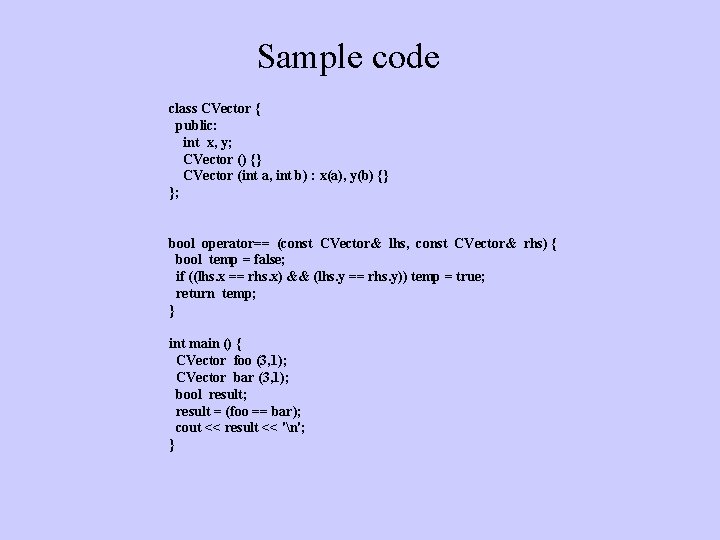
Sample code class CVector { public: int x, y; CVector () {} CVector (int a, int b) : x(a), y(b) {} }; bool operator== (const CVector& lhs, const CVector& rhs) { bool temp = false; if ((lhs. x == rhs. x) && (lhs. y == rhs. y)) temp = true; return temp; } int main () { CVector foo (3, 1); CVector bar (3, 1); bool result; result = (foo == bar); cout << result << 'n'; }