Strings What can we do with Strings concatenate
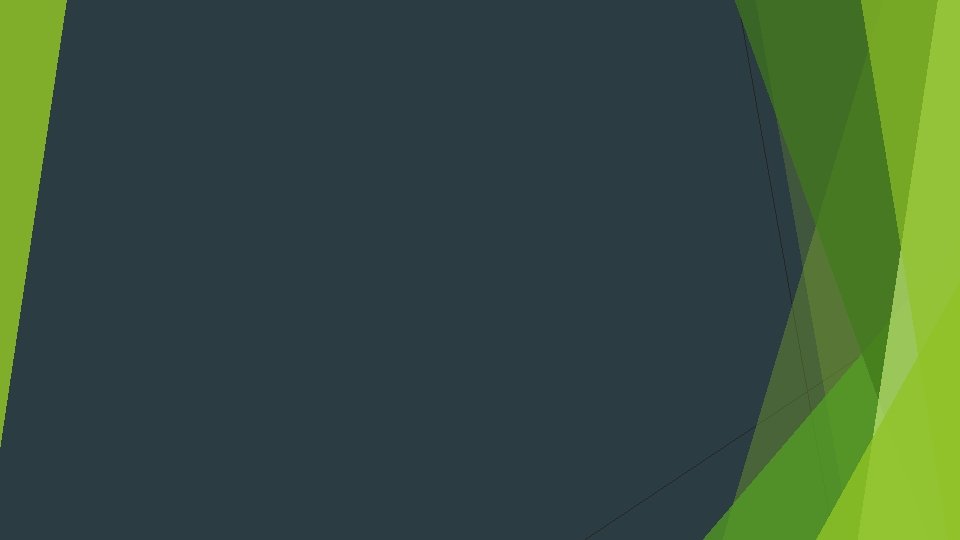
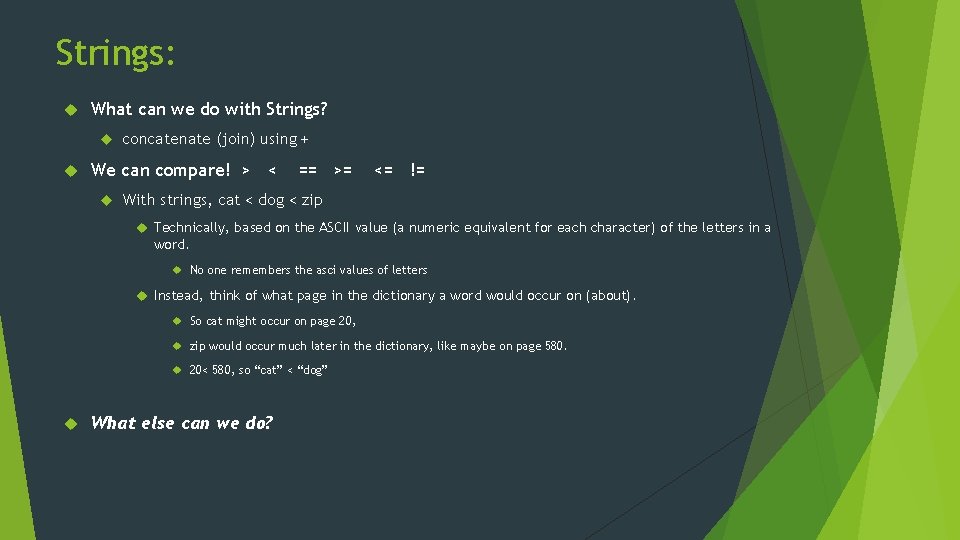
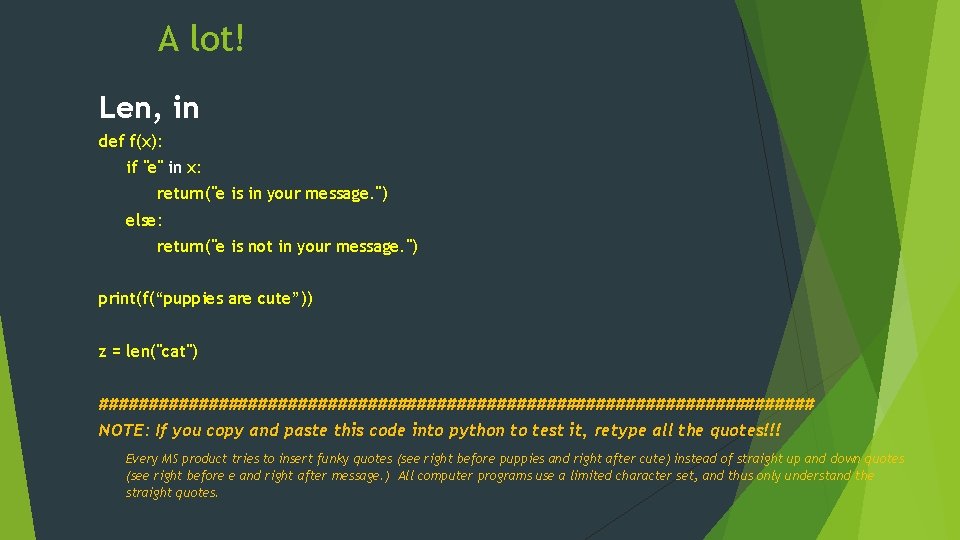
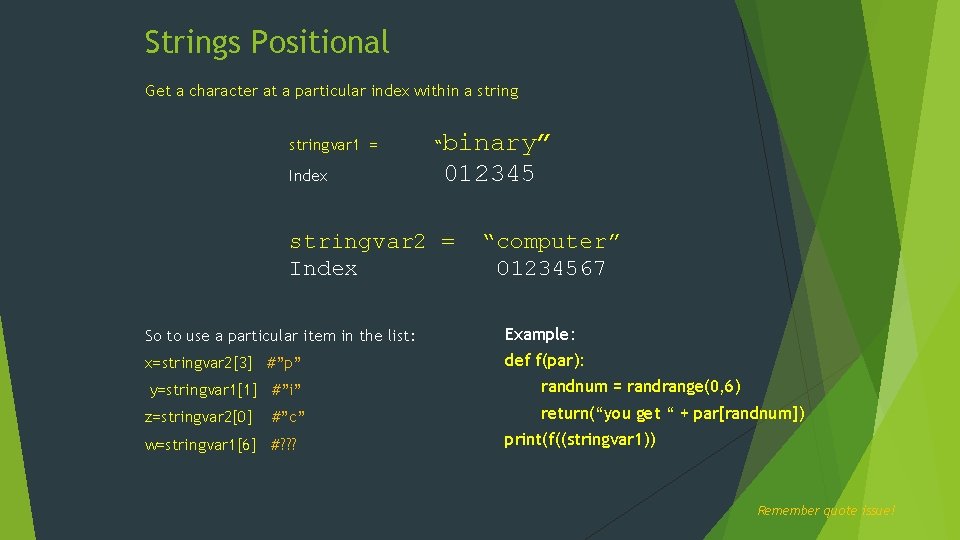
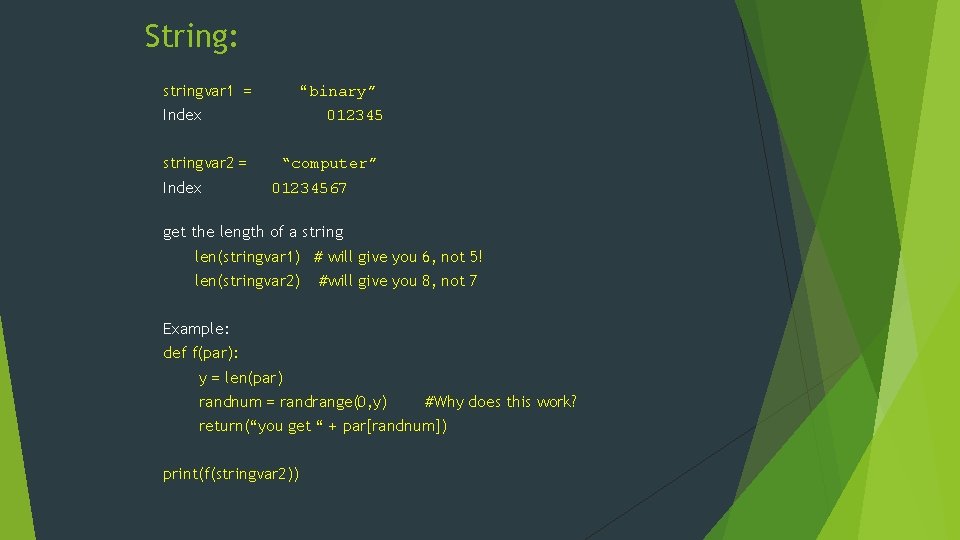
![We can now do: def f(y): x=0 while (x < len(y)): print(y[x]) x+=1 return We can now do: def f(y): x=0 while (x < len(y)): print(y[x]) x+=1 return](https://slidetodoc.com/presentation_image_h2/ba42252a495265b5a88330af7ed41532/image-6.jpg)
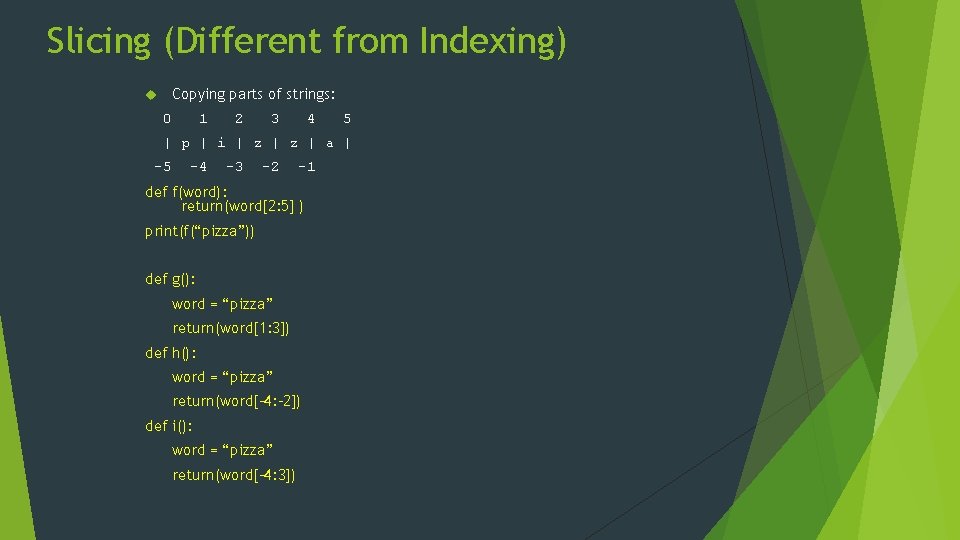
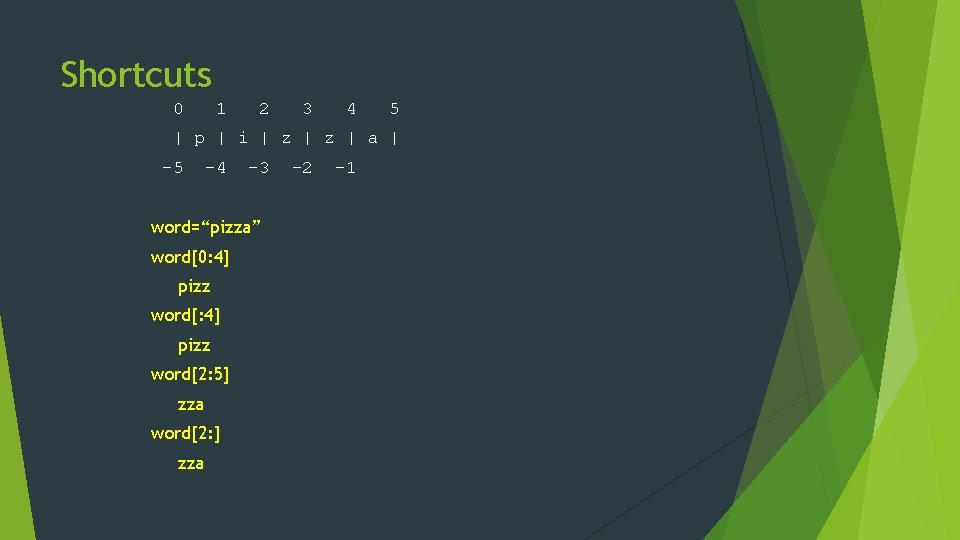
![# display a slice def g(s, f, wd): return("wd["+str(s)+": "+str(f)+"] is "+wd[s: f]) print(g(3, # display a slice def g(s, f, wd): return("wd["+str(s)+": "+str(f)+"] is "+wd[s: f]) print(g(3,](https://slidetodoc.com/presentation_image_h2/ba42252a495265b5a88330af7ed41532/image-9.jpg)
- Slides: 9
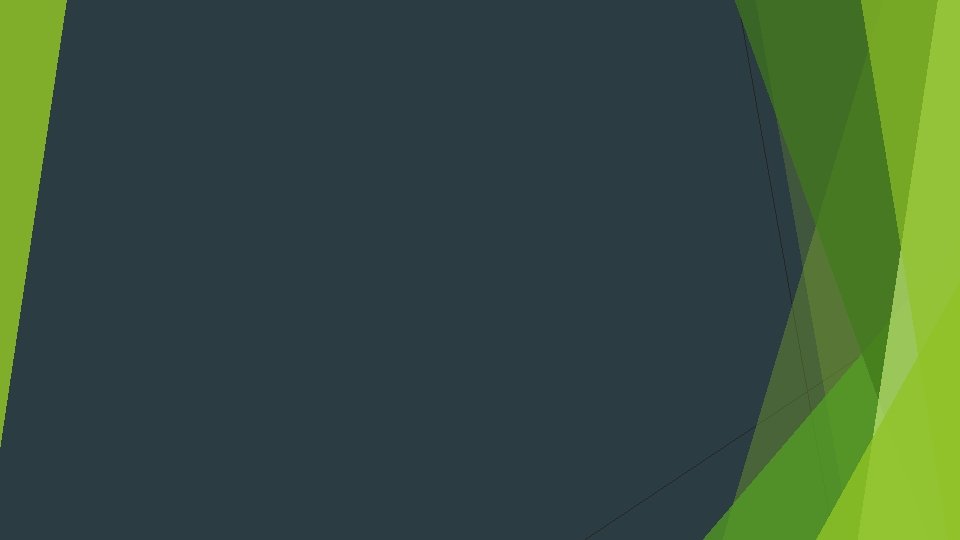
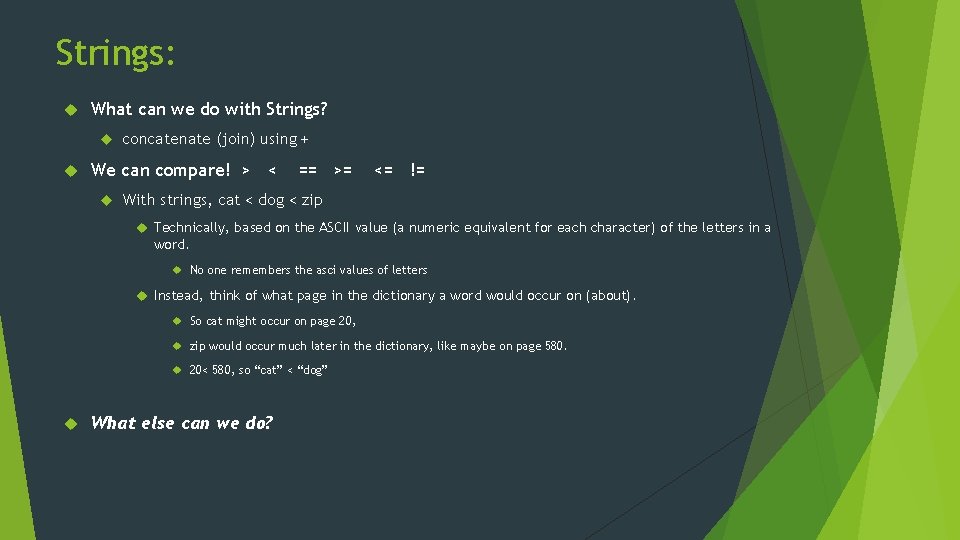
Strings: What can we do with Strings? concatenate (join) using + We can compare! > < == >= <= != With strings, cat < dog < zip Technically, based on the ASCII value (a numeric equivalent for each character) of the letters in a word. No one remembers the asci values of letters Instead, think of what page in the dictionary a word would occur on (about). So cat might occur on page 20, zip would occur much later in the dictionary, like maybe on page 580. 20< 580, so “cat” < “dog” What else can we do?
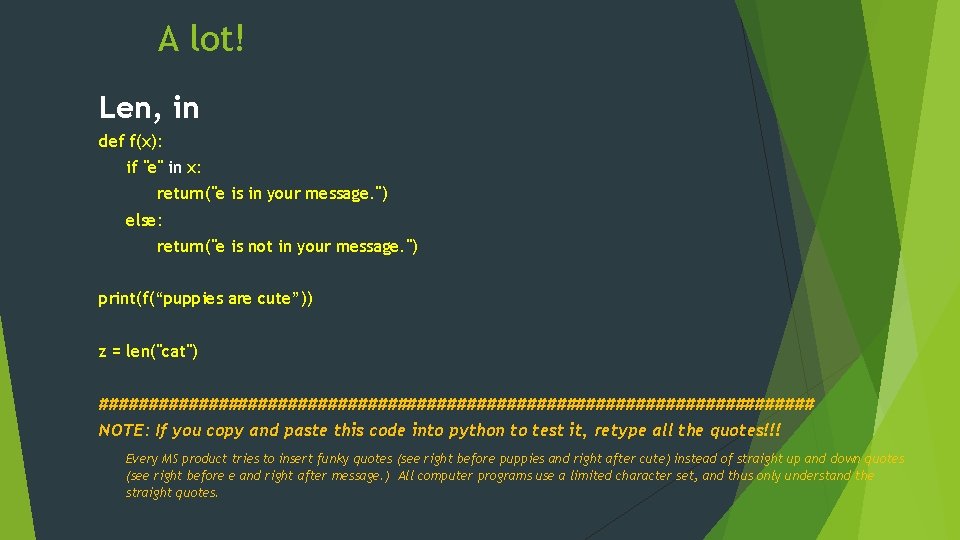
A lot! Len, in def f(x): if "e" in x: return("e is in your message. ") else: return("e is not in your message. ") print(f(“puppies are cute”)) z = len("cat") #################################### NOTE: If you copy and paste this code into python to test it, retype all the quotes!!! Every MS product tries to insert funky quotes (see right before puppies and right after cute) instead of straight up and down quotes (see right before e and right after message. ) All computer programs use a limited character set, and thus only understand the straight quotes.
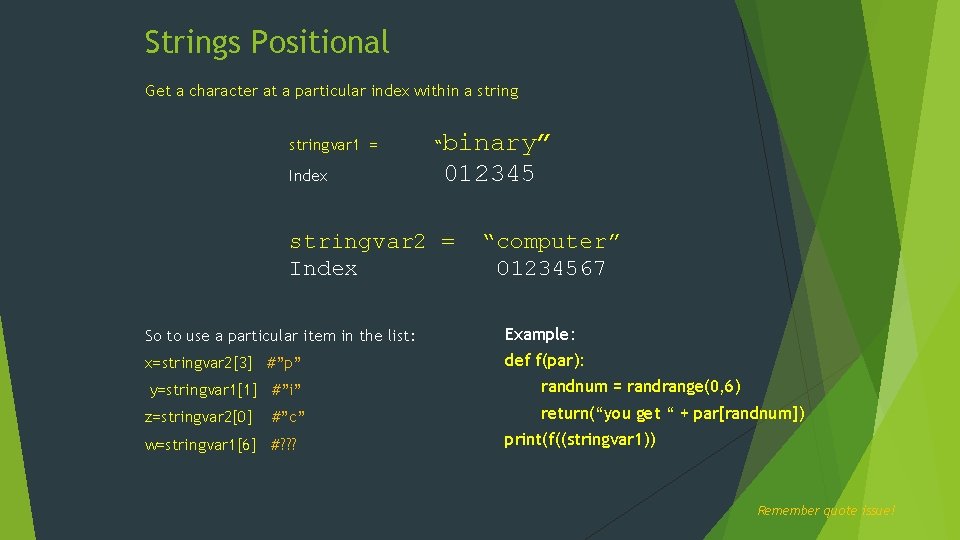
Strings Positional Get a character at a particular index within a stringvar 1 = Index “ binary” 012345 stringvar 2 = Index “computer” 01234567 So to use a particular item in the list: Example: x=stringvar 2[3] #”p” def f(par): y=stringvar 1[1] #”i” z=stringvar 2[0] #”c” w=stringvar 1[6] #? ? ? randnum = randrange(0, 6) return(“you get “ + par[randnum]) print(f((stringvar 1)) Remember quote issue!
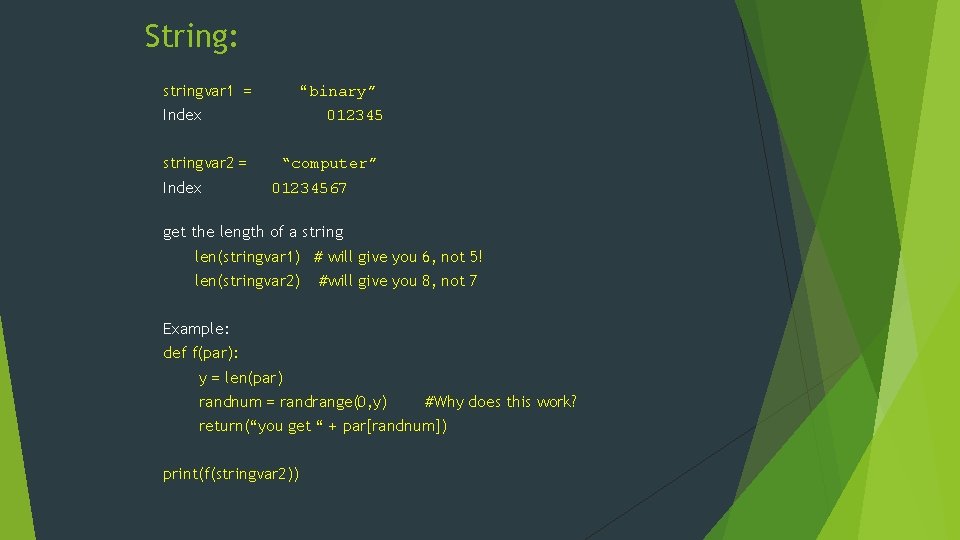
String: stringvar 1 = “binary” Index stringvar 2 = Index 012345 “computer” 01234567 get the length of a string len(stringvar 1) # will give you 6, not 5! len(stringvar 2) #will give you 8, not 7 Example: def f(par): y = len(par) randnum = randrange(0, y) #Why does this work? return(“you get “ + par[randnum]) print(f(stringvar 2))
![We can now do def fy x0 while x leny printyx x1 return We can now do: def f(y): x=0 while (x < len(y)): print(y[x]) x+=1 return](https://slidetodoc.com/presentation_image_h2/ba42252a495265b5a88330af7ed41532/image-6.jpg)
We can now do: def f(y): x=0 while (x < len(y)): print(y[x]) x+=1 return f(“kluge”)
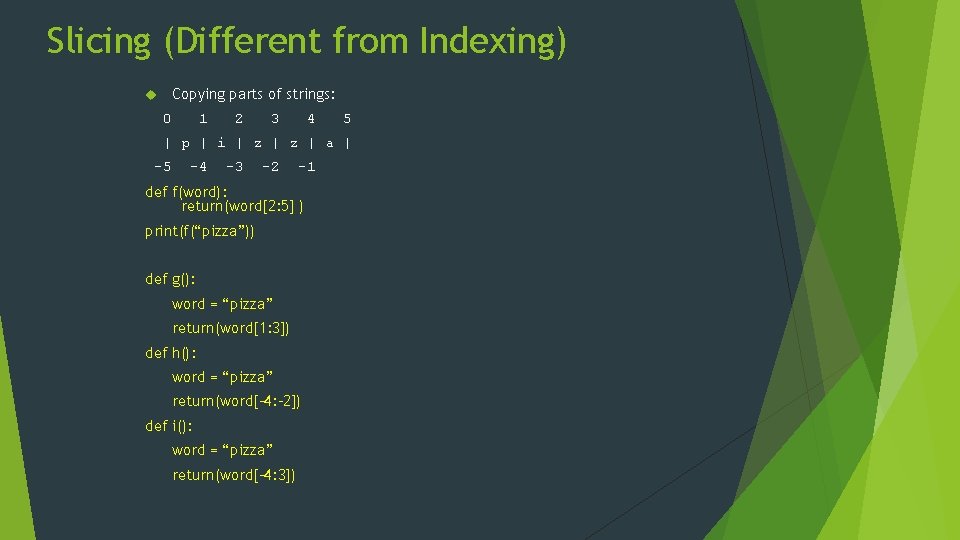
Slicing (Different from Indexing) Copying parts of strings: 0 1 2 3 4 5 | p | i | z | a | -5 -4 -3 -2 -1 def f(word): return(word[2: 5] ) print(f(“pizza”)) def g(): word = “pizza” return(word[1: 3]) def h(): word = “pizza” return(word[-4: -2]) def i(): word = “pizza” return(word[-4: 3])
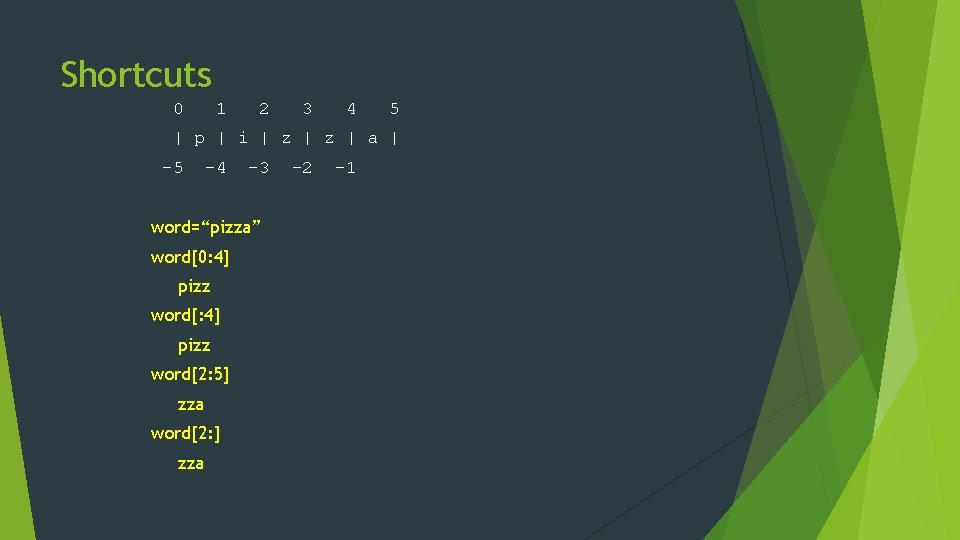
Shortcuts 0 1 2 3 4 5 | p | i | z | a | -5 -4 -3 word=“pizza” word[0: 4] pizz word[2: 5] zza word[2: ] zza -2 -1
![display a slice def gs f wd returnwdstrs strf is wds f printg3 # display a slice def g(s, f, wd): return("wd["+str(s)+": "+str(f)+"] is "+wd[s: f]) print(g(3,](https://slidetodoc.com/presentation_image_h2/ba42252a495265b5a88330af7ed41532/image-9.jpg)
# display a slice def g(s, f, wd): return("wd["+str(s)+": "+str(f)+"] is "+wd[s: f]) print(g(3, 7, "sesquipedalian"))