Strings in C Programming CS1001 Structured programming Approach
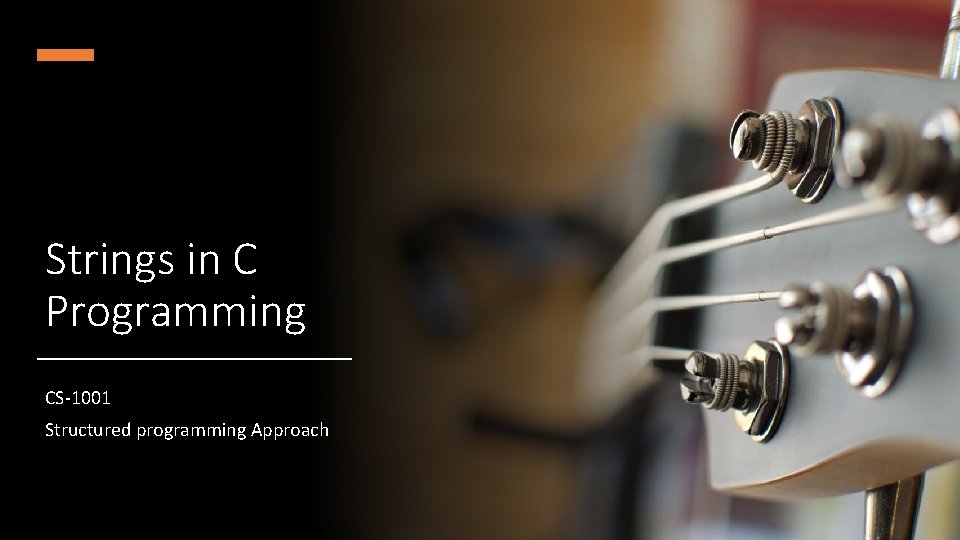
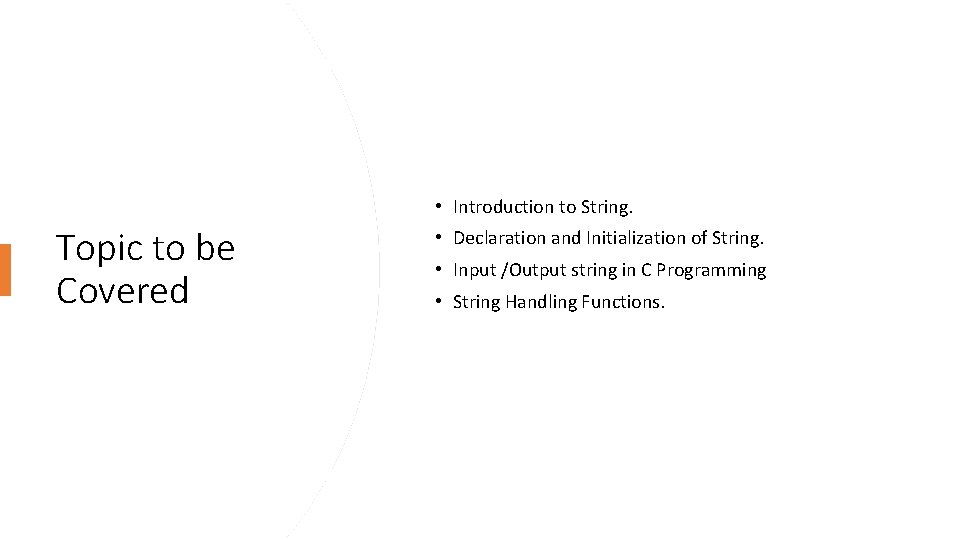
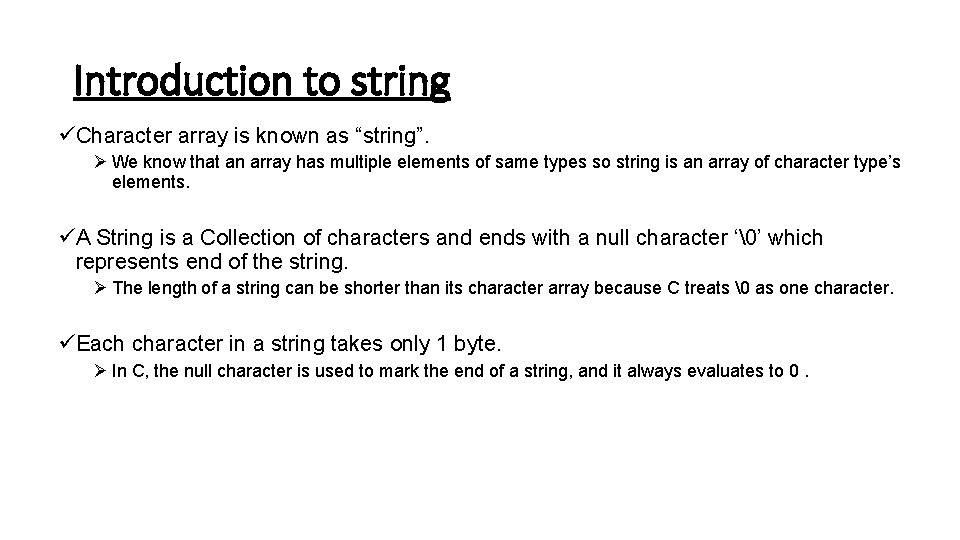
![Example char name[20]; // name is the character array that can contain 20 characters Example char name[20]; // name is the character array that can contain 20 characters](https://slidetodoc.com/presentation_image_h/9a21dbad13b13858c3f792e9c75ae932/image-4.jpg)
![Declaration / Initialization Of String üCharacter arrays can be initialised as char test[ ] Declaration / Initialization Of String üCharacter arrays can be initialised as char test[ ]](https://slidetodoc.com/presentation_image_h/9a21dbad13b13858c3f792e9c75ae932/image-5.jpg)
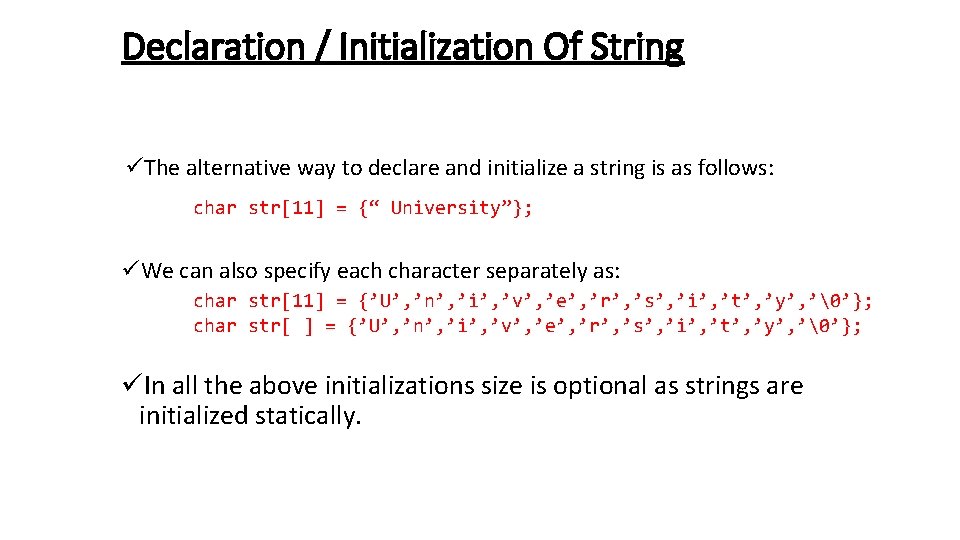
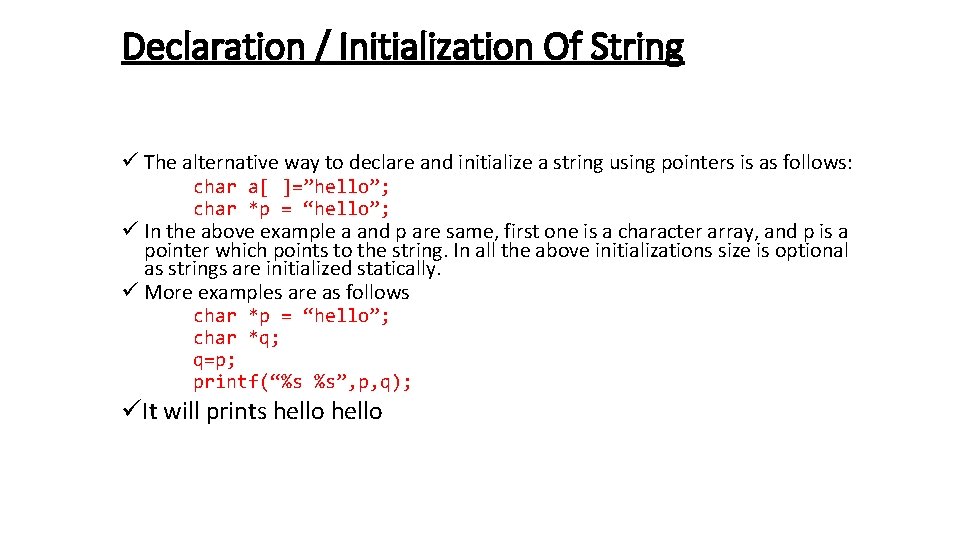
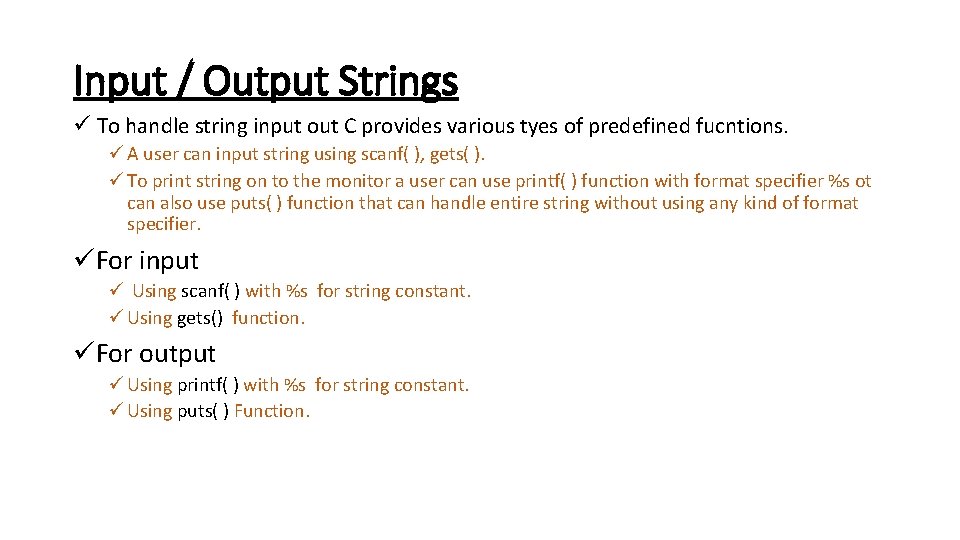
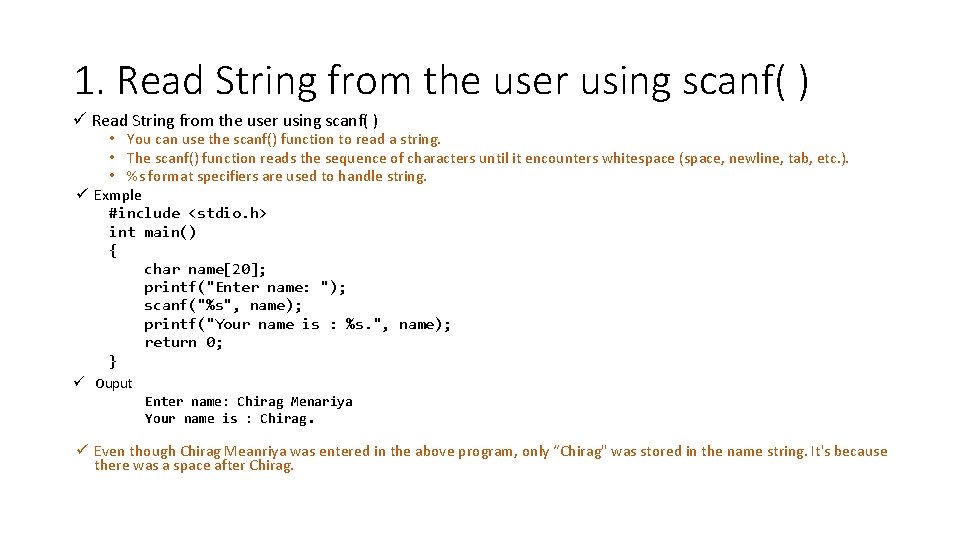
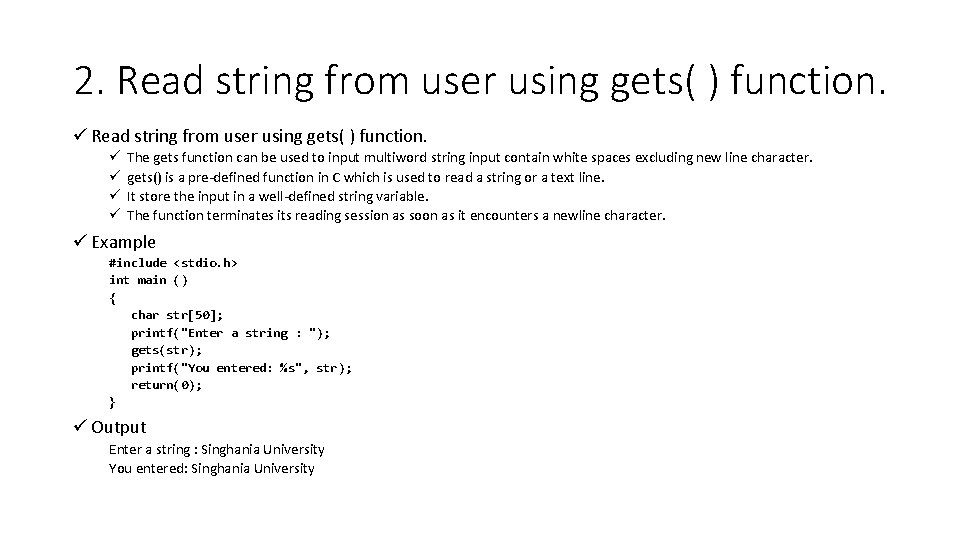
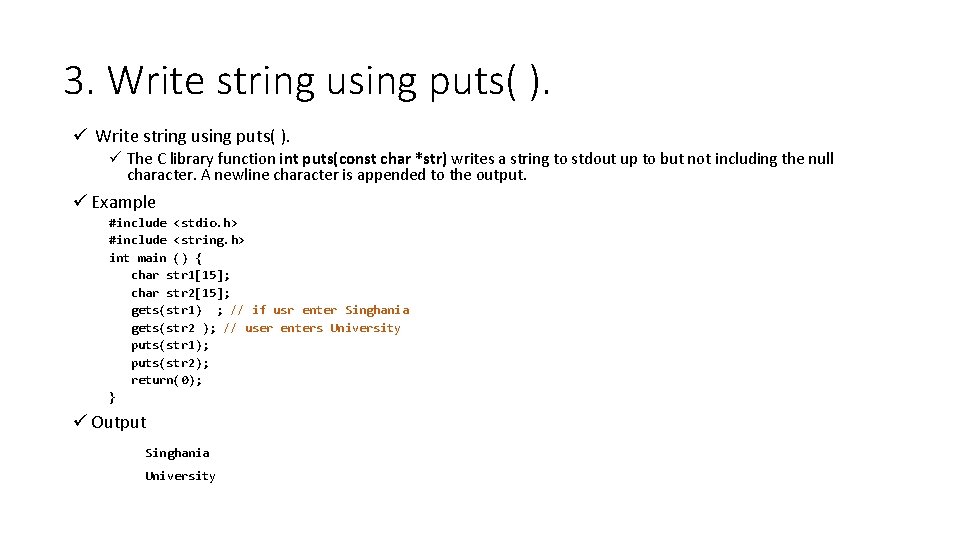
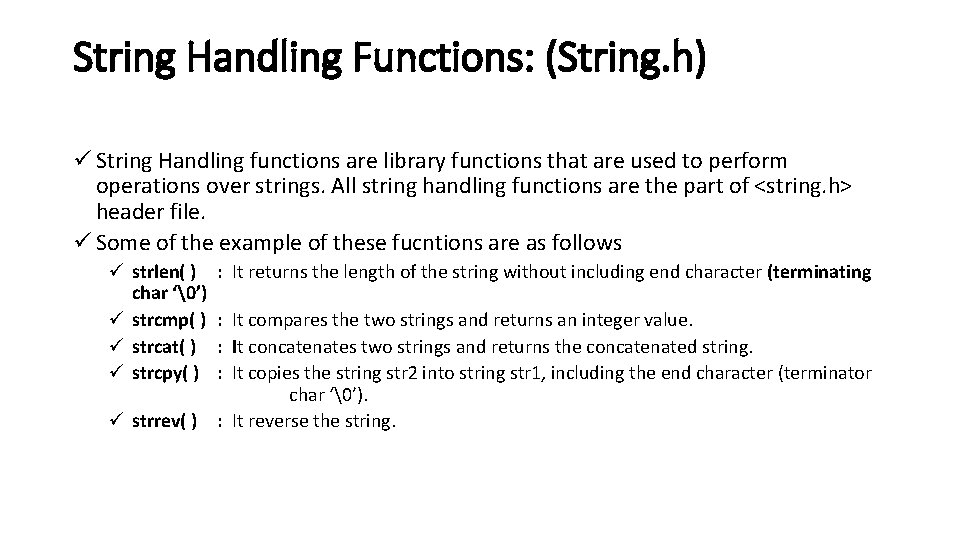
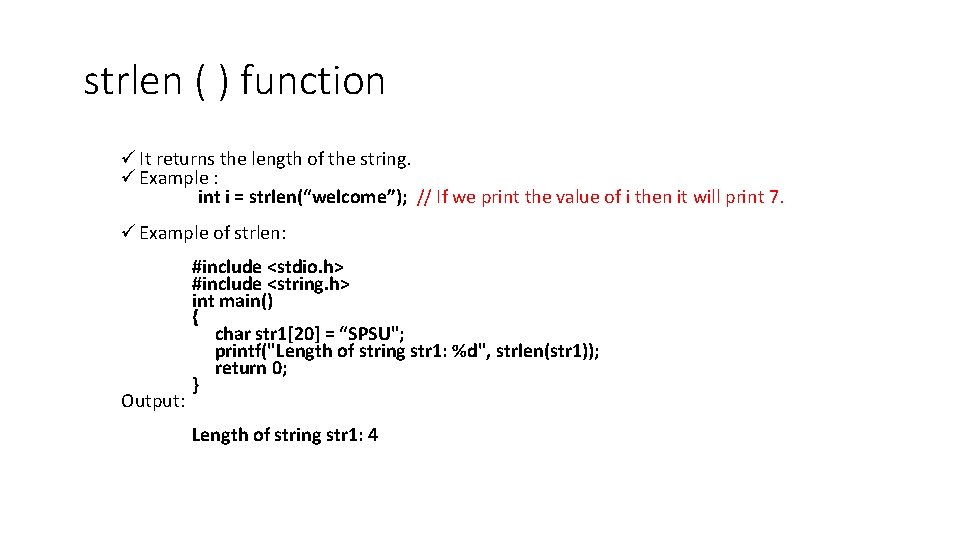
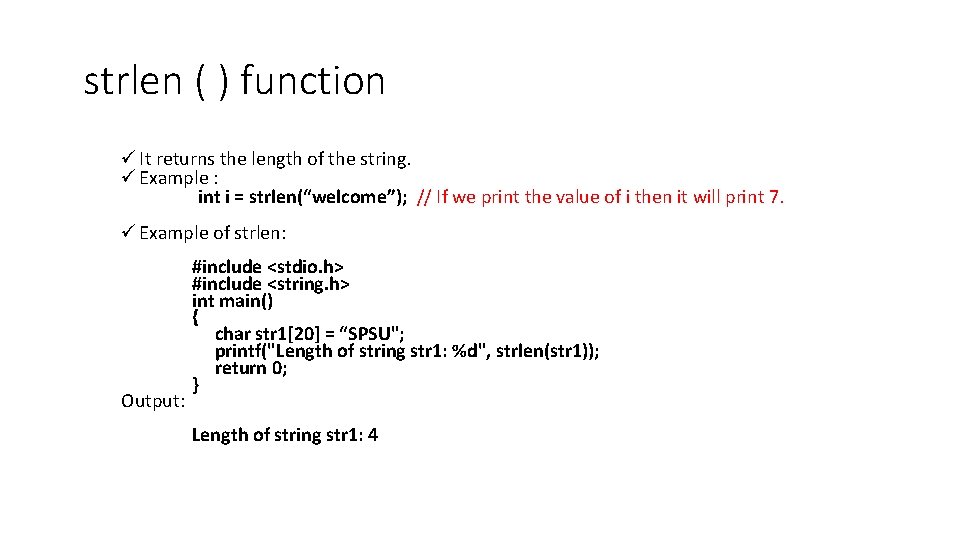
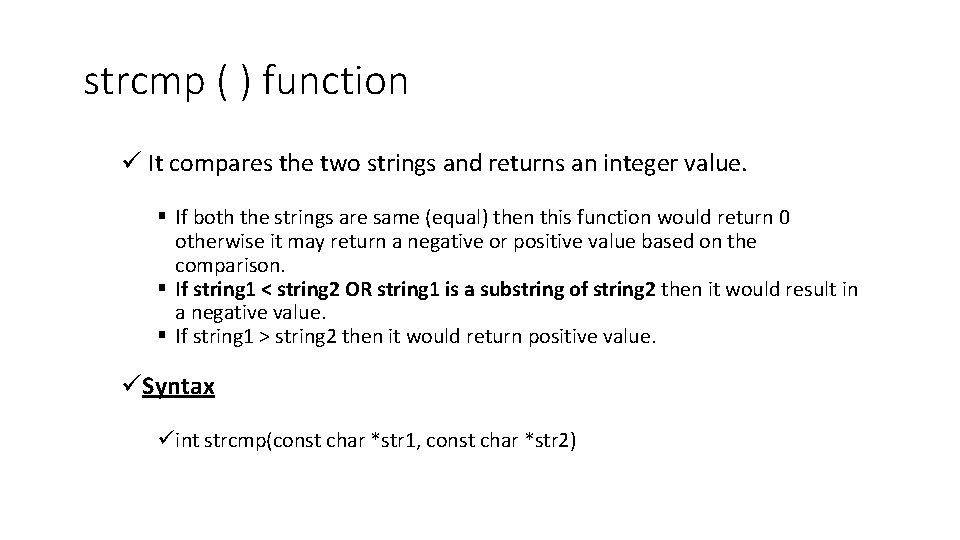
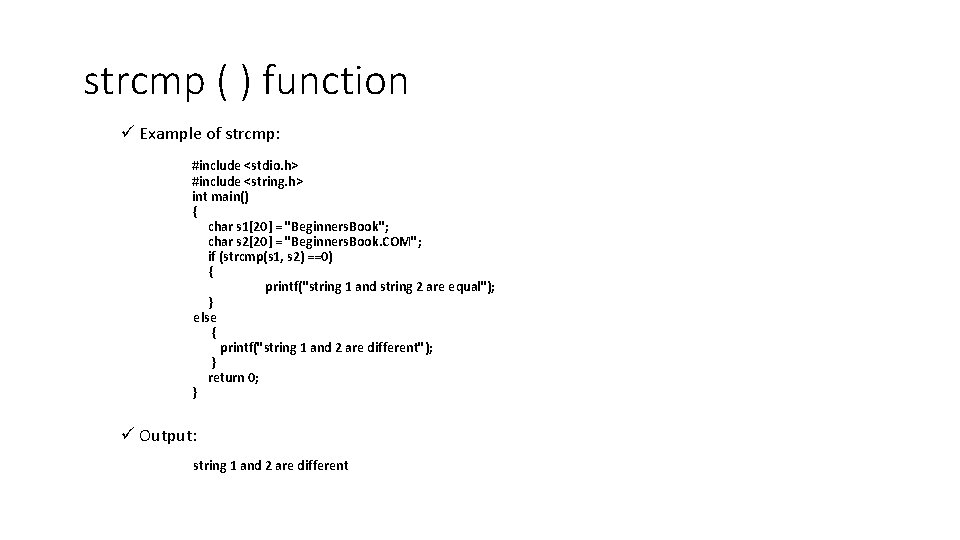
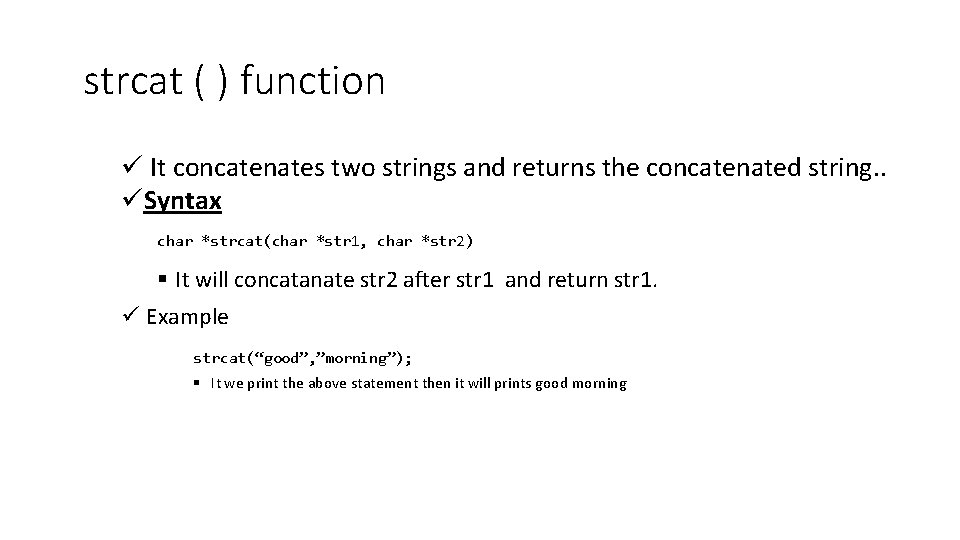
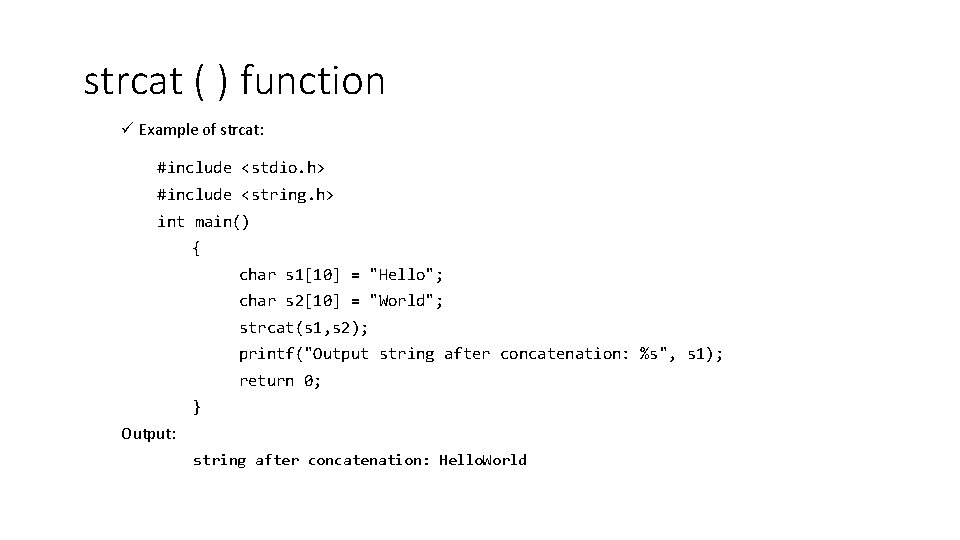
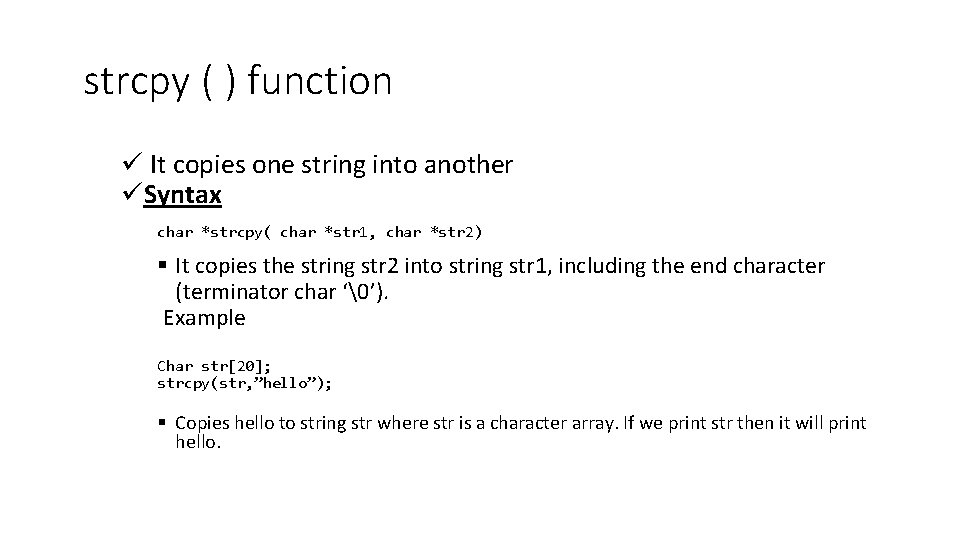
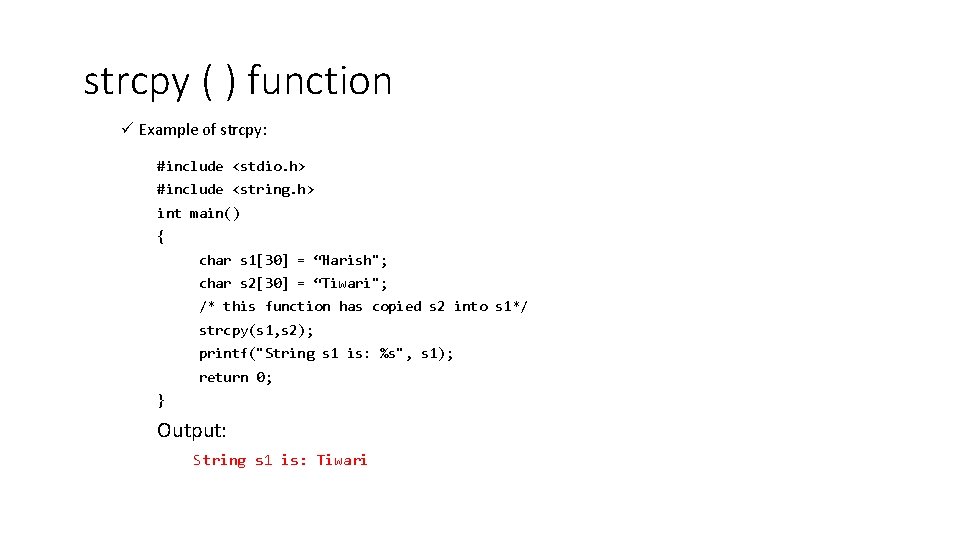
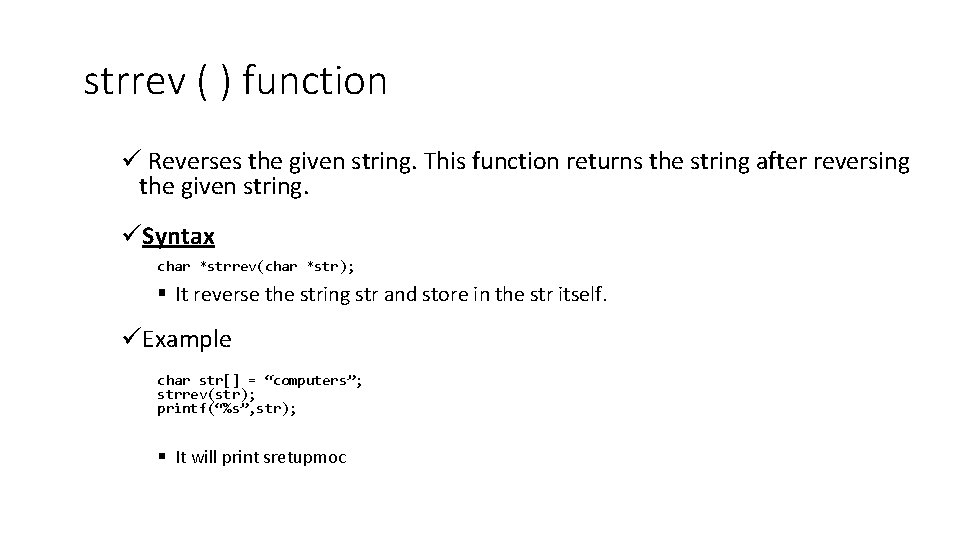
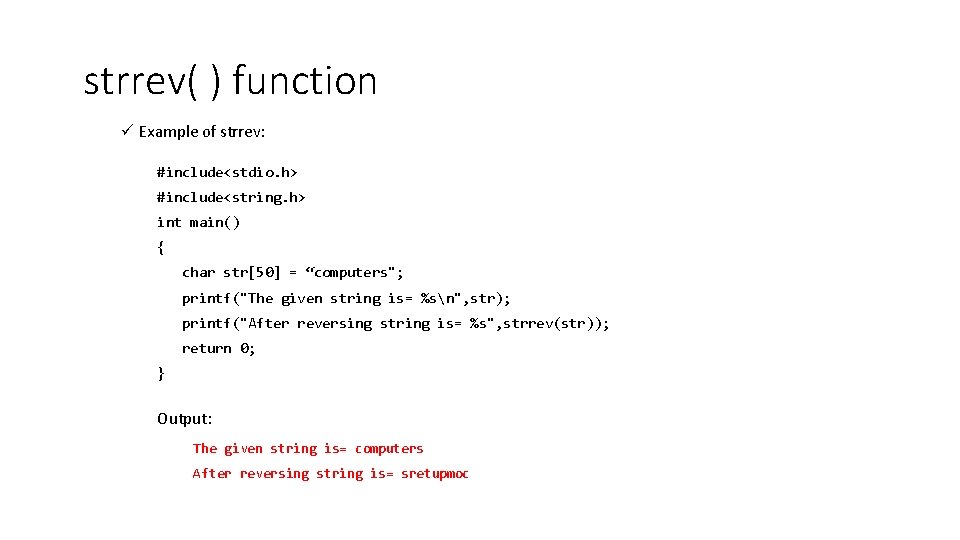
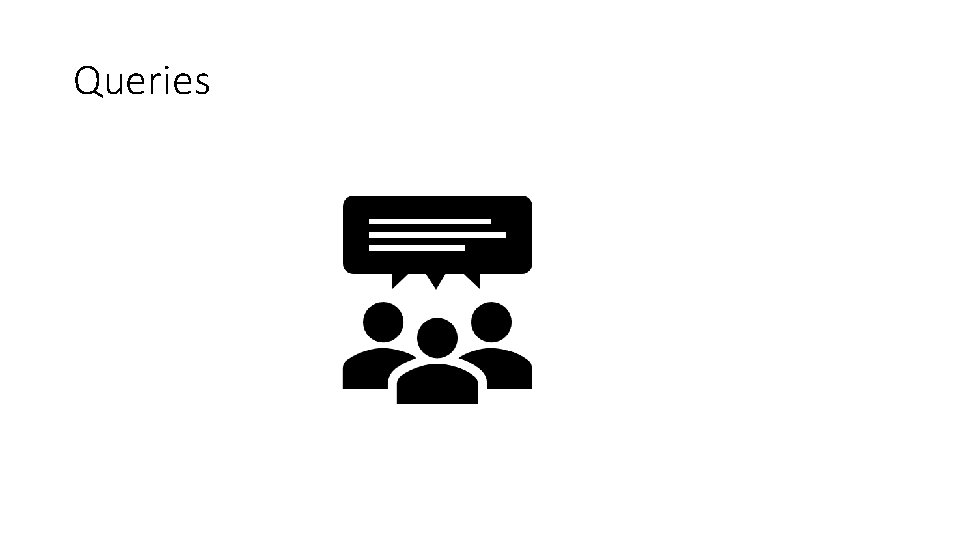
- Slides: 23
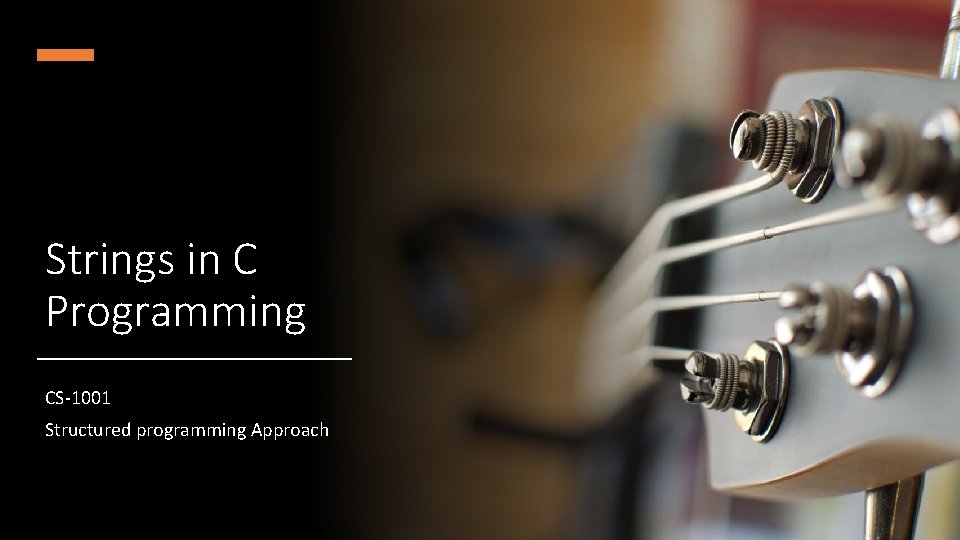
Strings in C Programming CS-1001 Structured programming Approach
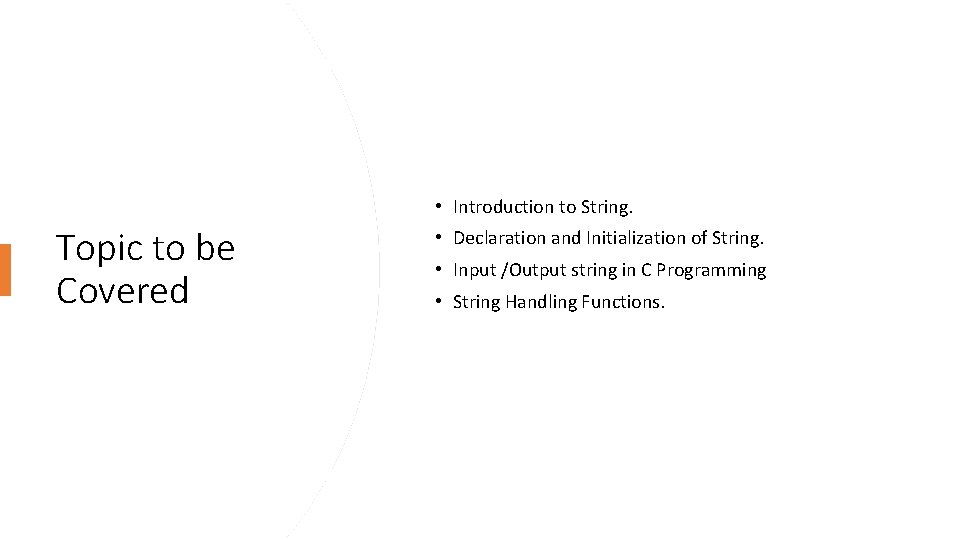
• Introduction to String. Topic to be Covered • Declaration and Initialization of String. • Input /Output string in C Programming • String Handling Functions.
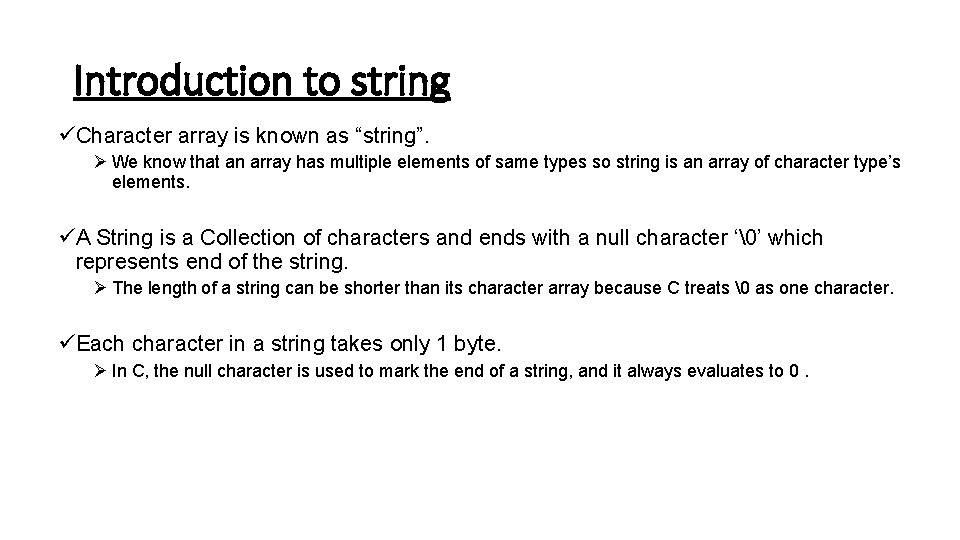
Introduction to string üCharacter array is known as “string”. Ø We know that an array has multiple elements of same types so string is an array of character type’s elements. üA String is a Collection of characters and ends with a null character ‘ ’ which represents end of the string. Ø The length of a string can be shorter than its character array because C treats as one character. üEach character in a string takes only 1 byte. Ø In C, the null character is used to mark the end of a string, and it always evaluates to 0.
![Example char name20 name is the character array that can contain 20 characters Example char name[20]; // name is the character array that can contain 20 characters](https://slidetodoc.com/presentation_image_h/9a21dbad13b13858c3f792e9c75ae932/image-4.jpg)
Example char name[20]; // name is the character array that can contain 20 characters including ‘ ’ character. char name[5] = {‘S’, ‘P’, ‘S’, ‘U’, ‘ ’}; char name[ ] = {‘S’, ‘P’, ‘S’, ‘U’, ‘ ’}; ü String Constant • A series of characters enclosed in double quotes (“”) is called a string constant. • The C compiler will automatically add a null character ( ) at the end of a string constant to indicate the end of the string. • Example : char name[ ]= “SPSU”;
![Declaration Initialization Of String üCharacter arrays can be initialised as char test Declaration / Initialization Of String üCharacter arrays can be initialised as char test[ ]](https://slidetodoc.com/presentation_image_h/9a21dbad13b13858c3f792e9c75ae932/image-5.jpg)
Declaration / Initialization Of String üCharacter arrays can be initialised as char test[ ] = {‘a’, ’e’, ’i’, ’o’, ’u’, ’ ’}; or üA string can be initialized by writing the string in double quotation marks as follows. In this case it is not necessary to specify null character explicitly. char test[ ] = “aeiou”; // NULL character is automatically upended in this case.
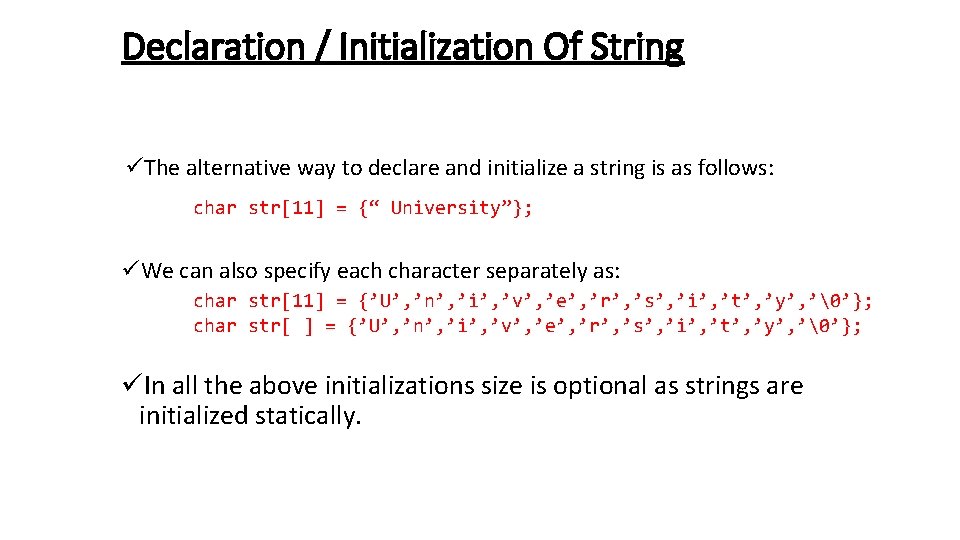
Declaration / Initialization Of String üThe alternative way to declare and initialize a string is as follows: char str[11] = {“ University”}; üWe can also specify each character separately as: char str[11] = {’U’, ’n’, ’i’, ’v’, ’e’, ’r’, ’s’, ’i’, ’t’, ’y’, ’ ’}; char str[ ] = {’U’, ’n’, ’i’, ’v’, ’e’, ’r’, ’s’, ’i’, ’t’, ’y’, ’ ’}; üIn all the above initializations size is optional as strings are initialized statically.
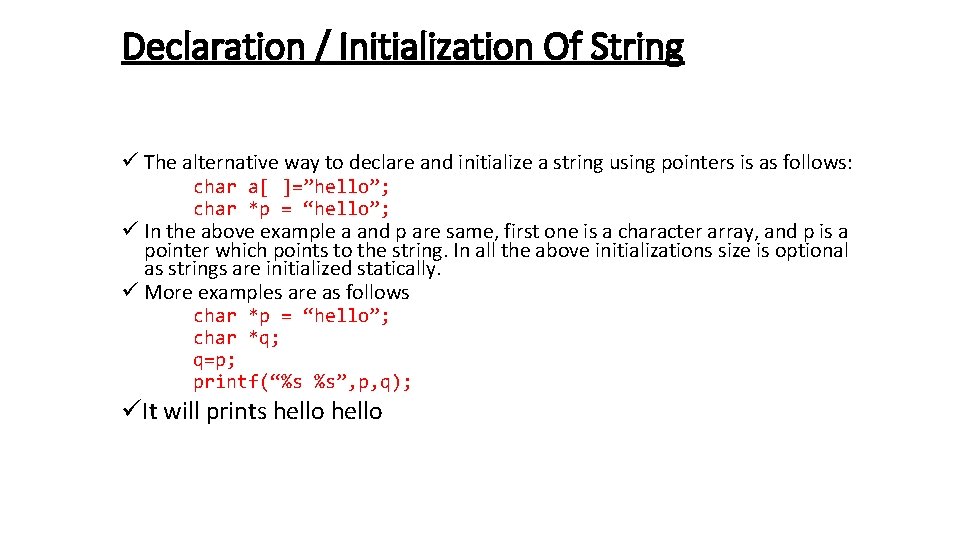
Declaration / Initialization Of String ü The alternative way to declare and initialize a string using pointers is as follows: char a[ ]=”hello”; char *p = “hello”; ü In the above example a and p are same, first one is a character array, and p is a pointer which points to the string. In all the above initializations size is optional as strings are initialized statically. ü More examples are as follows char *p = “hello”; char *q; q=p; printf(“%s %s”, p, q); üIt will prints hello
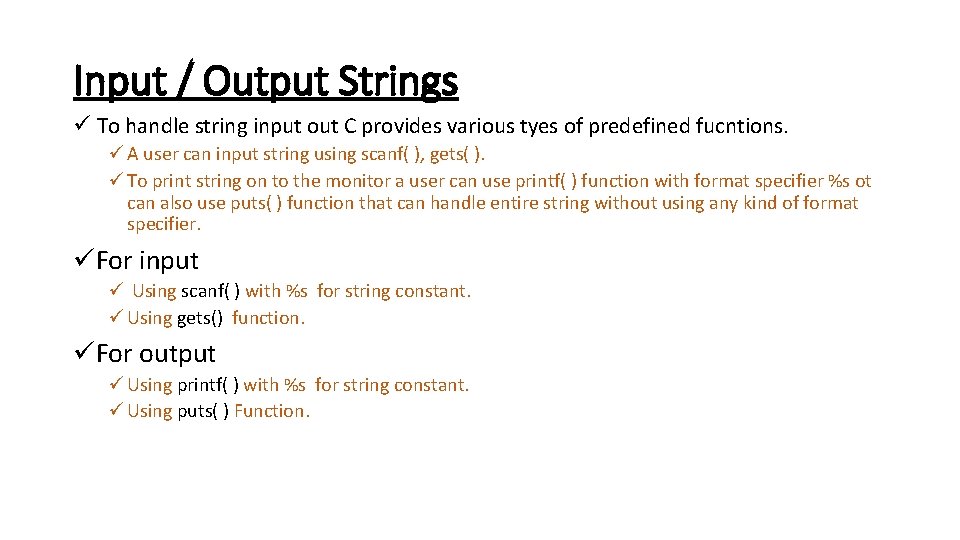
Input / Output Strings ü To handle string input out C provides various tyes of predefined fucntions. ü A user can input string using scanf( ), gets( ). ü To print string on to the monitor a user can use printf( ) function with format specifier %s ot can also use puts( ) function that can handle entire string without using any kind of format specifier. üFor input ü Using scanf( ) with %s for string constant. ü Using gets() function. üFor output ü Using printf( ) with %s for string constant. ü Using puts( ) Function.
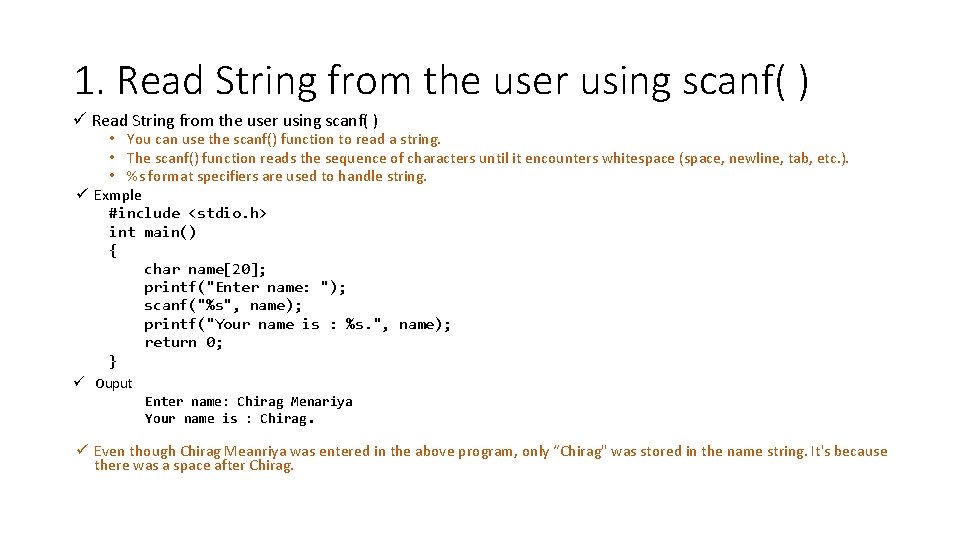
1. Read String from the user using scanf( ) ü Read String from the user using scanf( ) • You can use the scanf() function to read a string. • The scanf() function reads the sequence of characters until it encounters whitespace (space, newline, tab, etc. ). • %s format specifiers are used to handle string. ü Exmple #include <stdio. h> int main() { char name[20]; printf("Enter name: "); scanf("%s", name); printf("Your name is : %s. ", name); return 0; } ü Ouput Enter name: Chirag Menariya Your name is : Chirag. ü Even though Chirag Meanriya was entered in the above program, only “Chirag" was stored in the name string. It's because there was a space after Chirag.
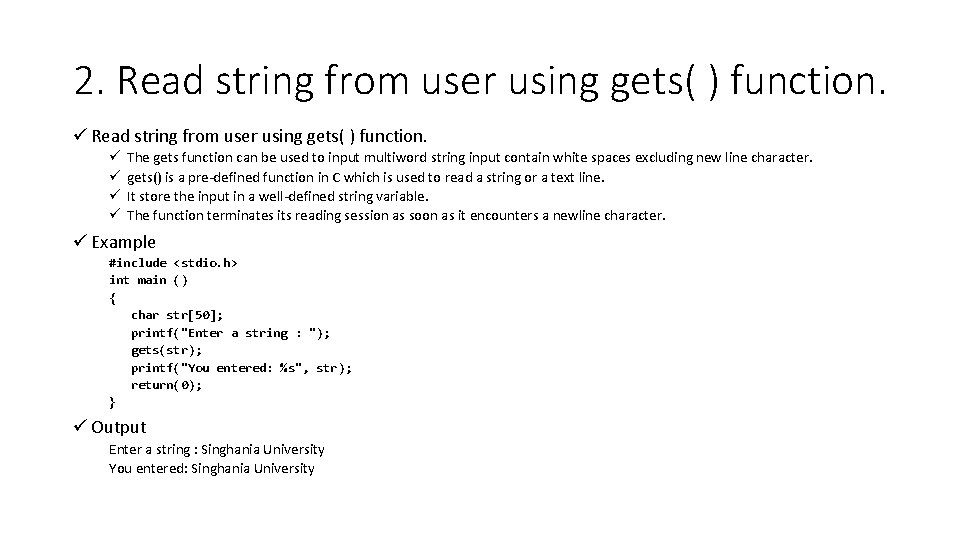
2. Read string from user using gets( ) function. ü ü ü ü The gets function can be used to input multiword string input contain white spaces excluding new line character. gets() is a pre-defined function in C which is used to read a string or a text line. It store the input in a well-defined string variable. The function terminates its reading session as soon as it encounters a newline character. ü Example #include <stdio. h> int main () { char str[50]; printf("Enter a string : "); gets(str); printf("You entered: %s", str); return(0); } ü Output Enter a string : Singhania University You entered: Singhania University
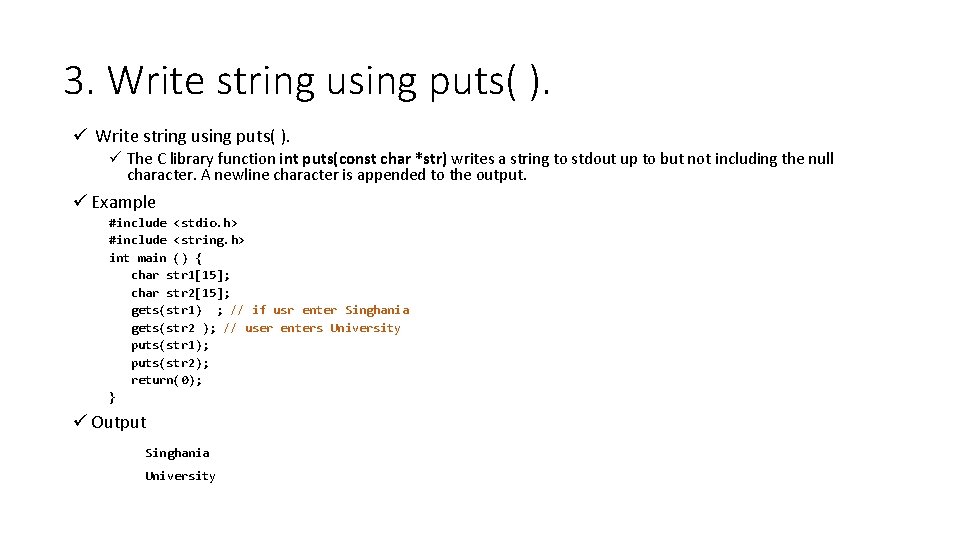
3. Write string using puts( ). ü The C library function int puts(const char *str) writes a string to stdout up to but not including the null character. A newline character is appended to the output. ü Example #include <stdio. h> #include <string. h> int main () { char str 1[15]; char str 2[15]; gets(str 1) ; // if usr enter Singhania gets(str 2 ); // user enters University puts(str 1); puts(str 2); return(0); } ü Output Singhania University
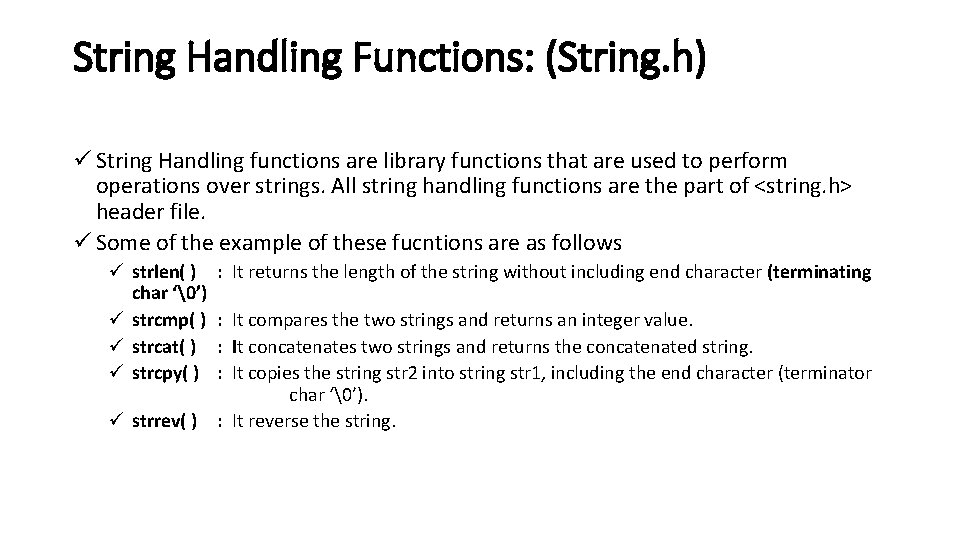
String Handling Functions: (String. h) ü String Handling functions are library functions that are used to perform operations over strings. All string handling functions are the part of <string. h> header file. ü Some of the example of these fucntions are as follows ü strlen( ) char ‘ ’) ü strcmp( ) ü strcat( ) ü strcpy( ) : It returns the length of the string without including end character (terminating : It compares the two strings and returns an integer value. : It concatenates two strings and returns the concatenated string. : It copies the string str 2 into string str 1, including the end character (terminator char ‘ ’). ü strrev( ) : It reverse the string.
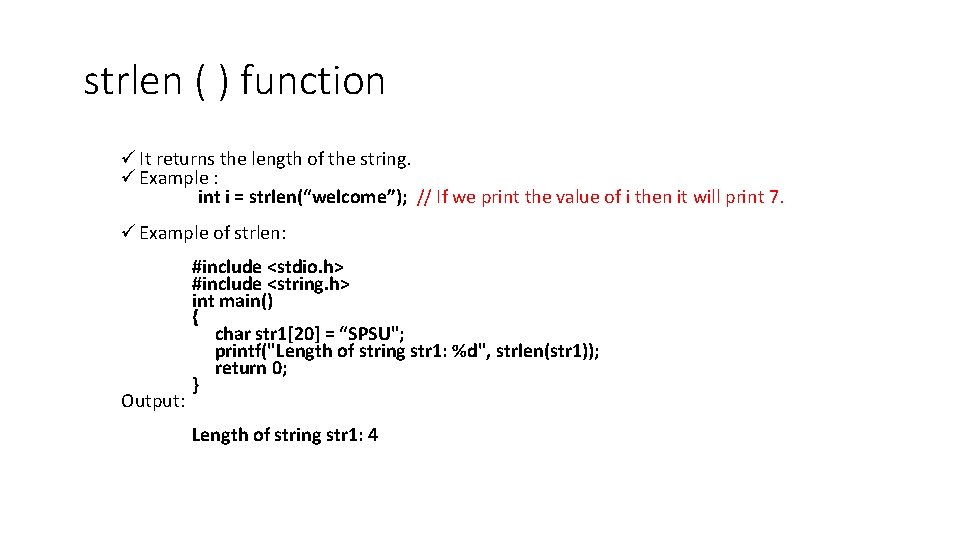
strlen ( ) function ü It returns the length of the string. ü Example : int i = strlen(“welcome”); // If we print the value of i then it will print 7. ü Example of strlen: Output: #include <stdio. h> #include <string. h> int main() { char str 1[20] = “SPSU"; printf("Length of string str 1: %d", strlen(str 1)); return 0; } Length of string str 1: 4
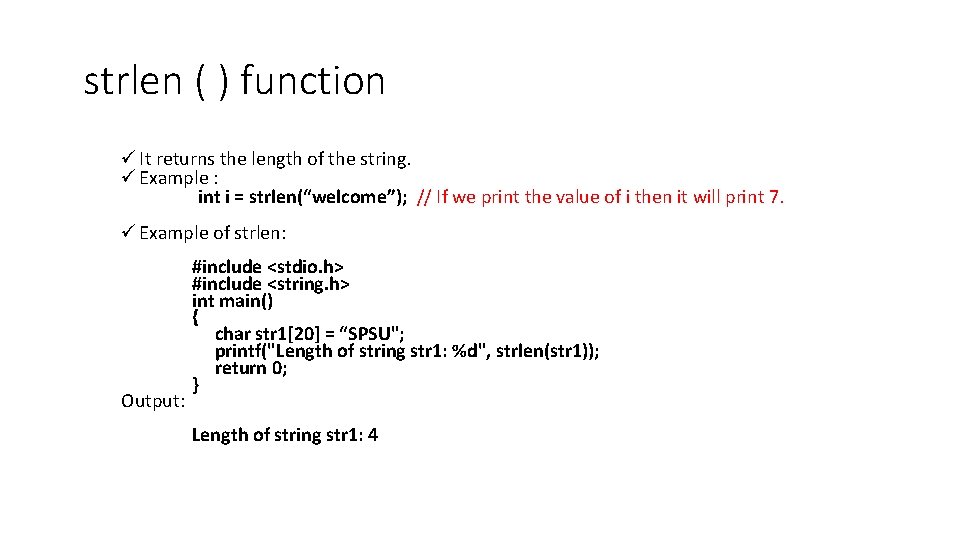
strlen ( ) function ü It returns the length of the string. ü Example : int i = strlen(“welcome”); // If we print the value of i then it will print 7. ü Example of strlen: Output: #include <stdio. h> #include <string. h> int main() { char str 1[20] = “SPSU"; printf("Length of string str 1: %d", strlen(str 1)); return 0; } Length of string str 1: 4
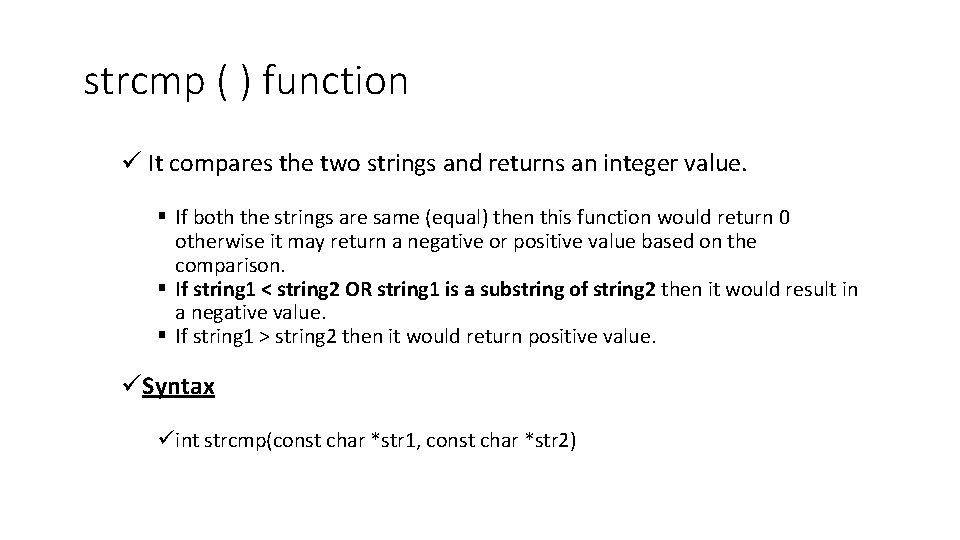
strcmp ( ) function ü It compares the two strings and returns an integer value. § If both the strings are same (equal) then this function would return 0 otherwise it may return a negative or positive value based on the comparison. § If string 1 < string 2 OR string 1 is a substring of string 2 then it would result in a negative value. § If string 1 > string 2 then it would return positive value. üSyntax üint strcmp(const char *str 1, const char *str 2)
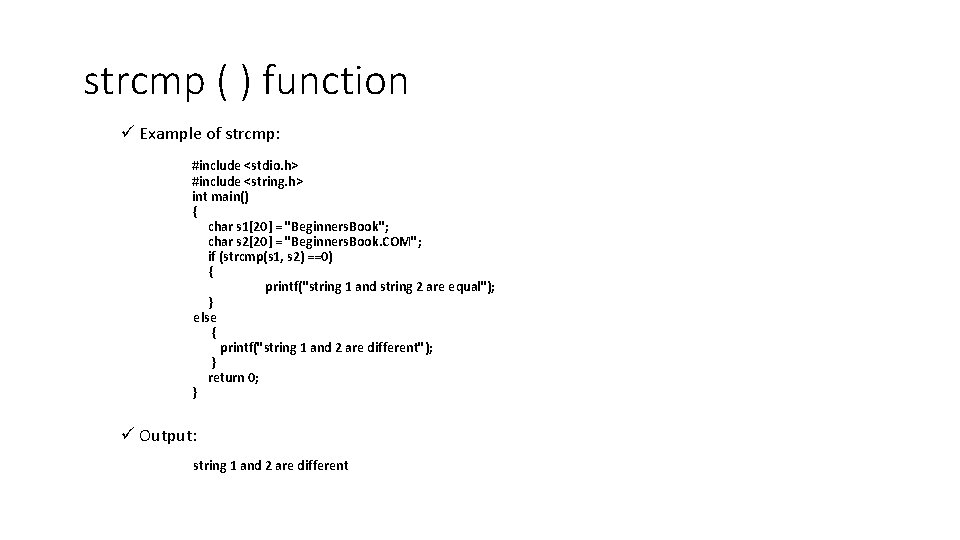
strcmp ( ) function ü Example of strcmp: #include <stdio. h> #include <string. h> int main() { char s 1[20] = "Beginners. Book"; char s 2[20] = "Beginners. Book. COM"; if (strcmp(s 1, s 2) ==0) { printf("string 1 and string 2 are equal"); } else { printf("string 1 and 2 are different"); } return 0; } ü Output: string 1 and 2 are different
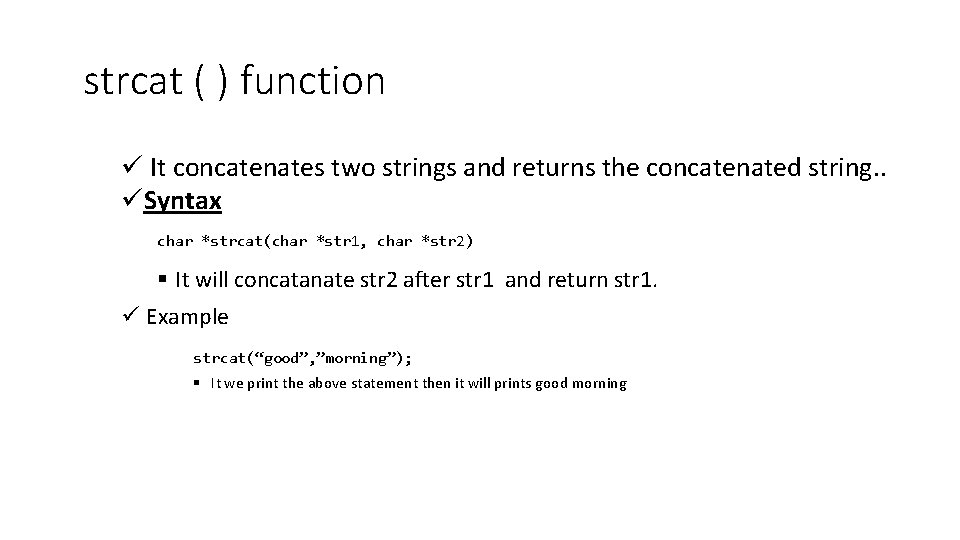
strcat ( ) function ü It concatenates two strings and returns the concatenated string. . üSyntax char *strcat(char *str 1, char *str 2) § It will concatanate str 2 after str 1 and return str 1. ü Example strcat(“good”, ”morning”); § It we print the above statement then it will prints good morning
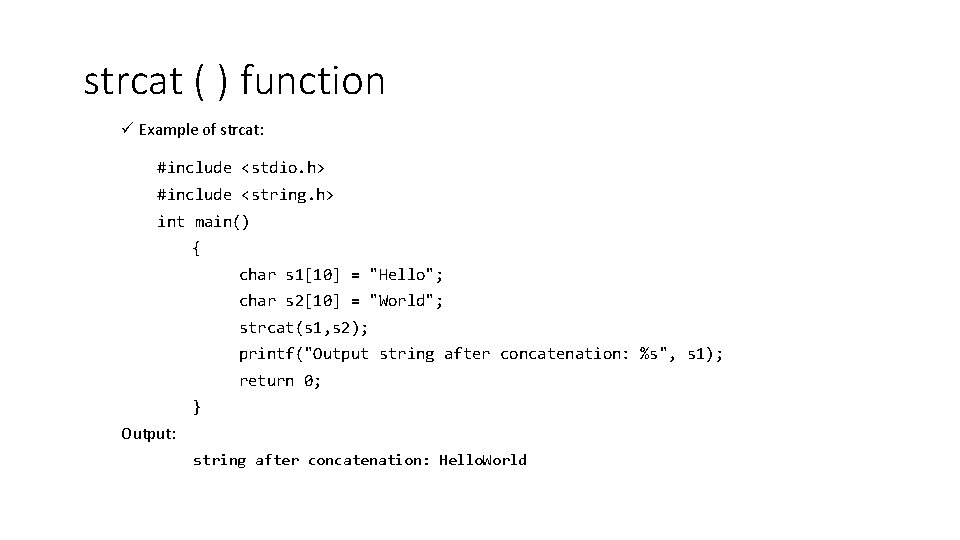
strcat ( ) function ü Example of strcat: #include <stdio. h> #include <string. h> int main() { char s 1[10] = "Hello"; char s 2[10] = "World"; strcat(s 1, s 2); printf("Output string after concatenation: %s", s 1); return 0; } Output: string after concatenation: Hello. World
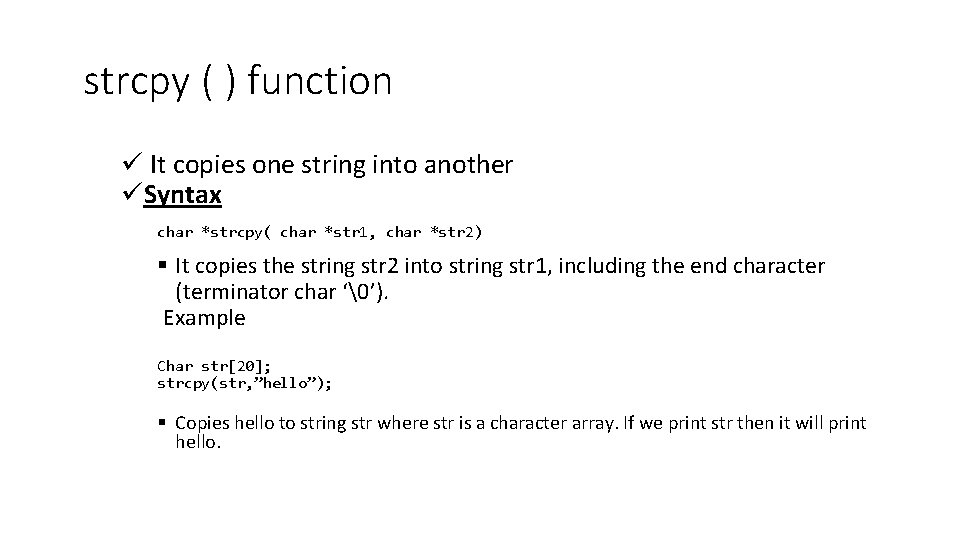
strcpy ( ) function ü It copies one string into another üSyntax char *strcpy( char *str 1, char *str 2) § It copies the string str 2 into string str 1, including the end character (terminator char ‘ ’). Example Char str[20]; strcpy(str, ”hello”); § Copies hello to string str where str is a character array. If we print str then it will print hello.
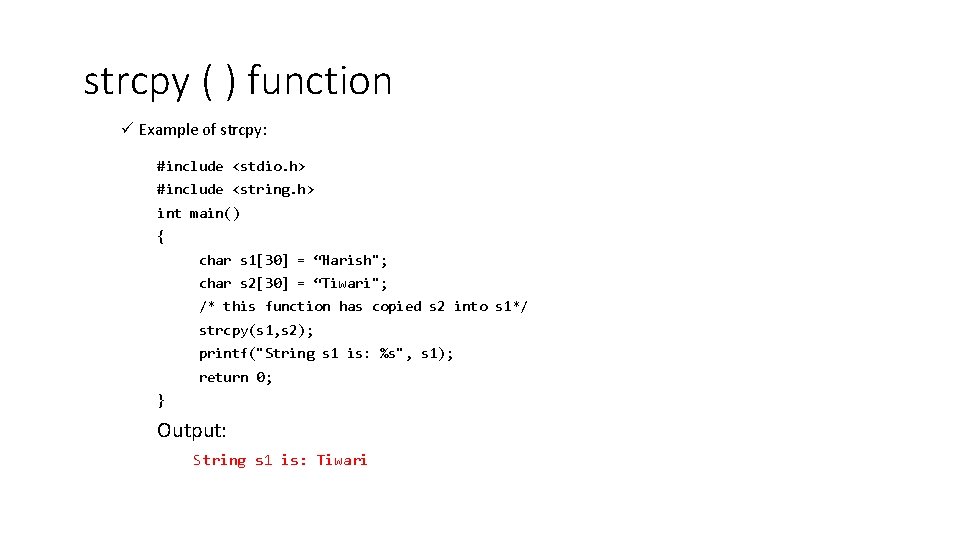
strcpy ( ) function ü Example of strcpy: #include <stdio. h> #include <string. h> int main() { char s 1[30] = “Harish"; char s 2[30] = “Tiwari"; /* this function has copied s 2 into s 1*/ strcpy(s 1, s 2); printf("String s 1 is: %s", s 1); return 0; } Output: String s 1 is: Tiwari
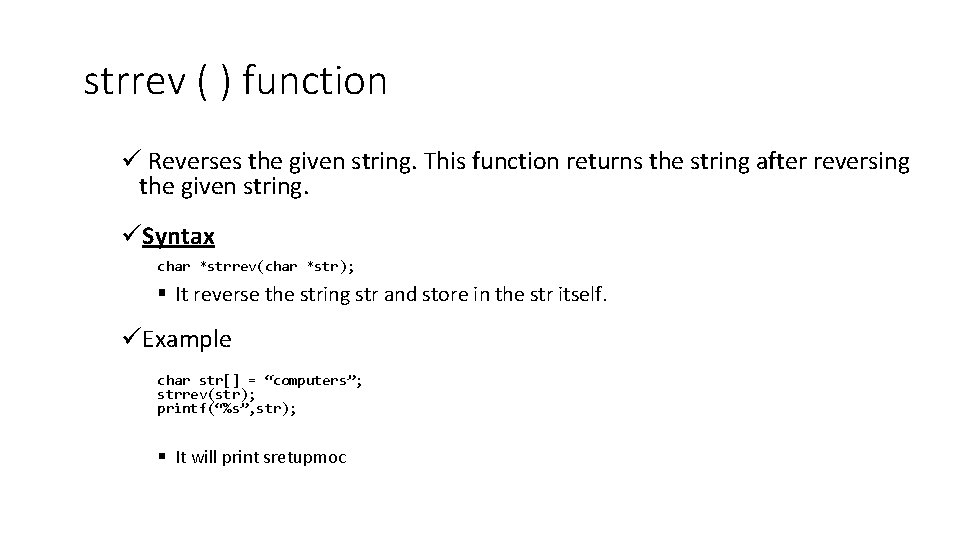
strrev ( ) function ü Reverses the given string. This function returns the string after reversing the given string. üSyntax char *strrev(char *str); § It reverse the string str and store in the str itself. üExample char str[] = “computers”; strrev(str); printf(“%s”, str); § It will print sretupmoc
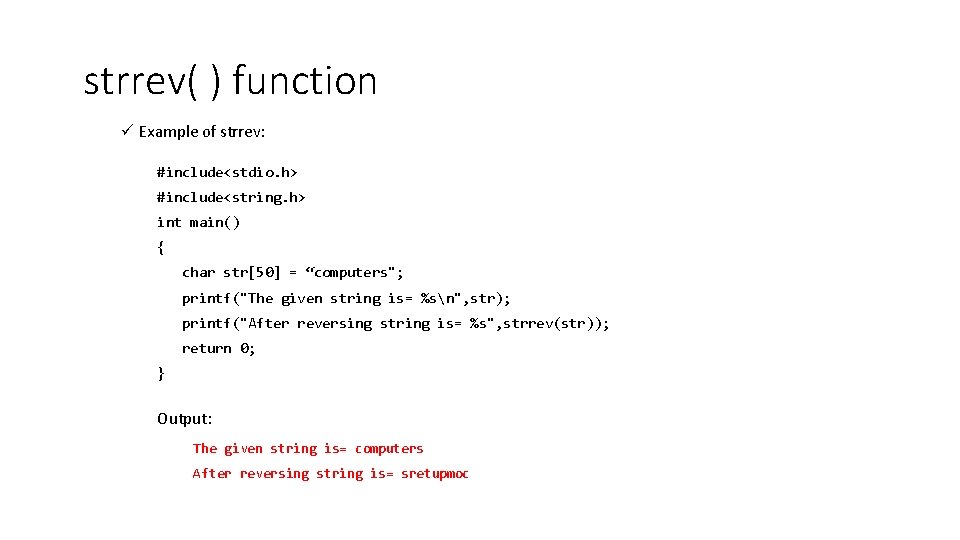
strrev( ) function ü Example of strrev: #include<stdio. h> #include<string. h> int main() { char str[50] = “computers"; printf("The given string is= %sn", str); printf("After reversing string is= %s", strrev(str)); return 0; } Output: The given string is= computers After reversing string is= sretupmoc
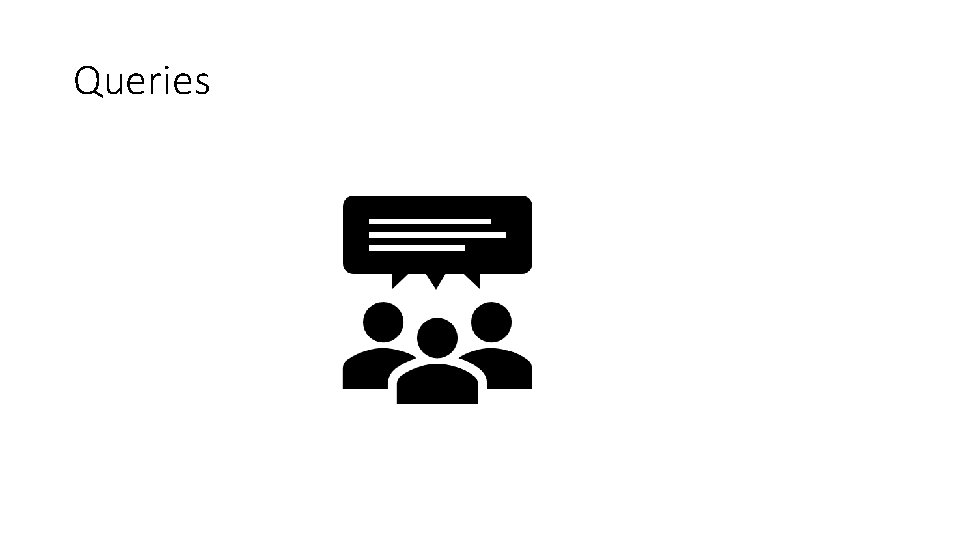
Queries