String Handling in JAVA String Handling provides a
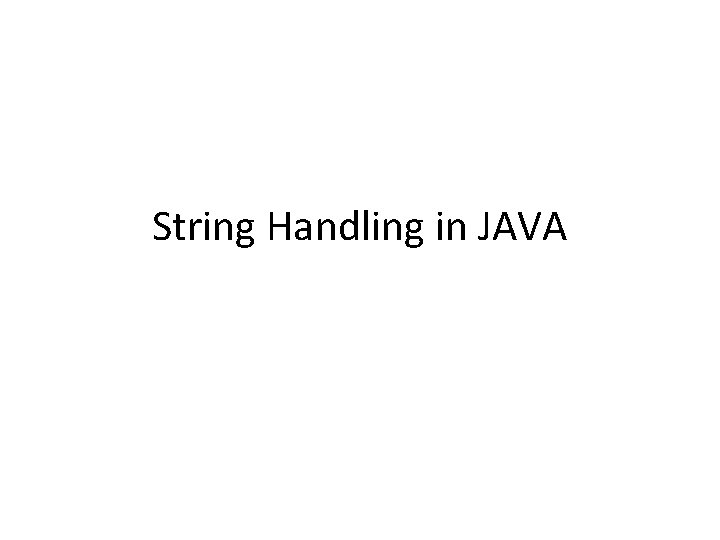
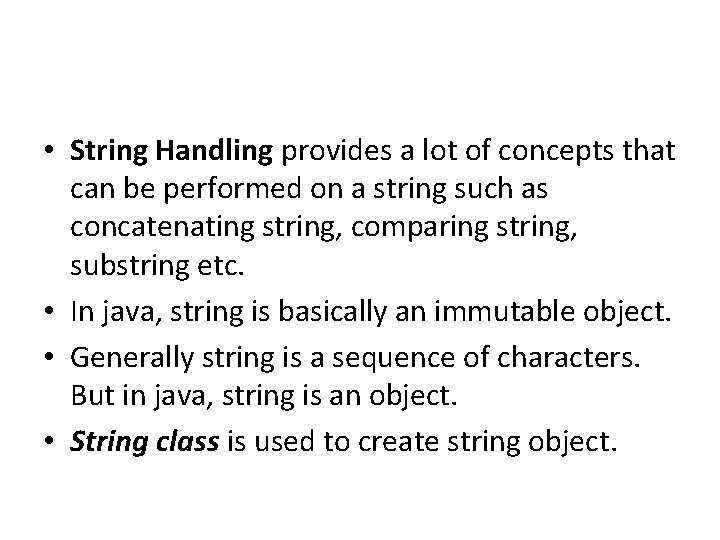
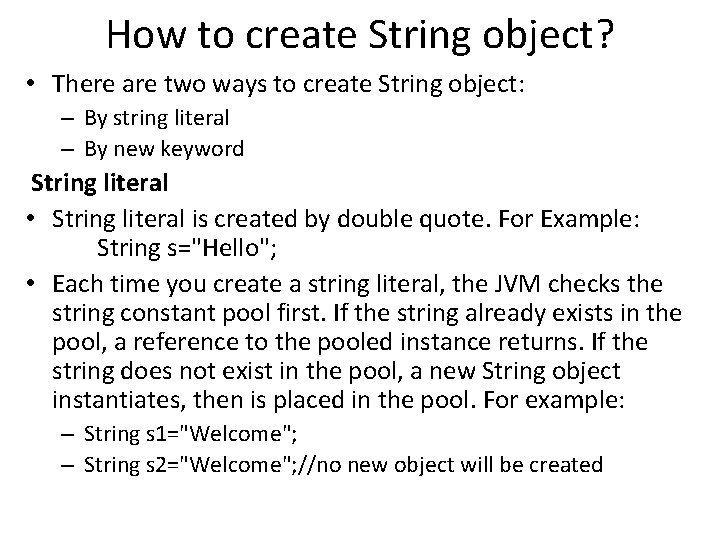
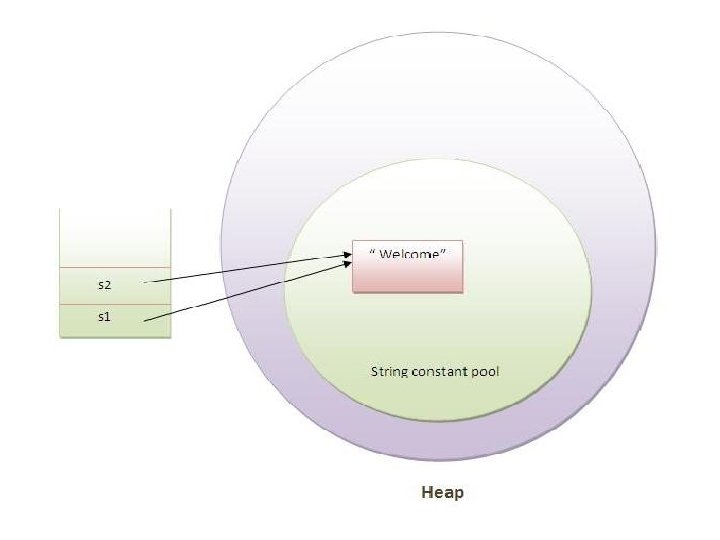
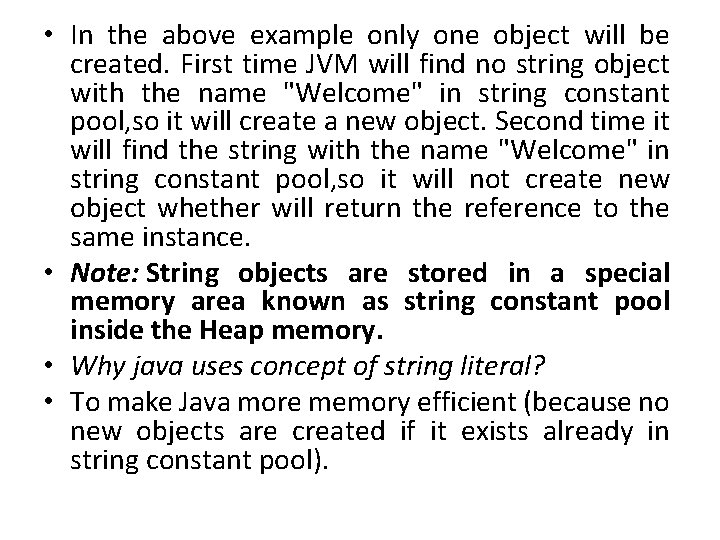
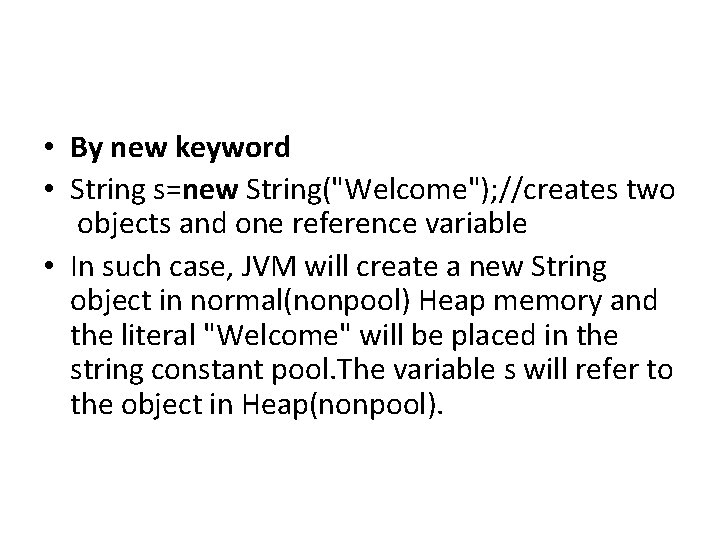
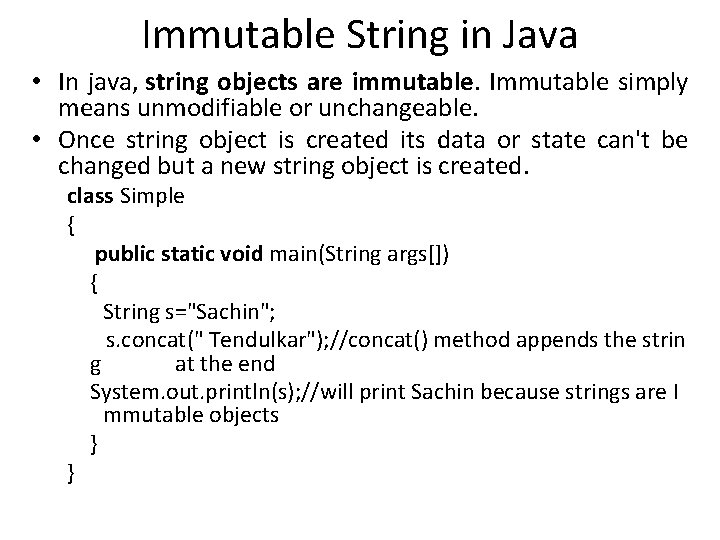
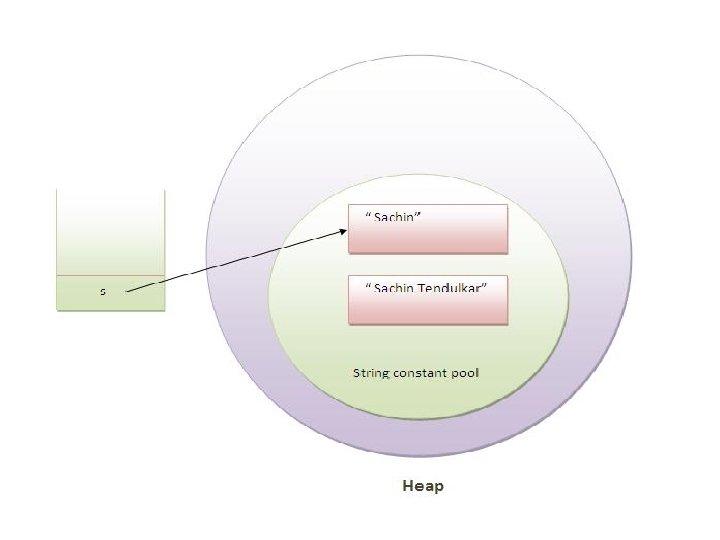
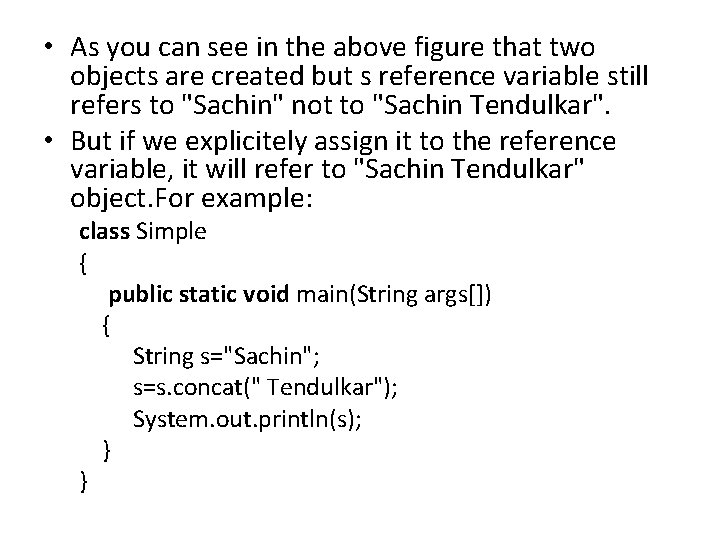
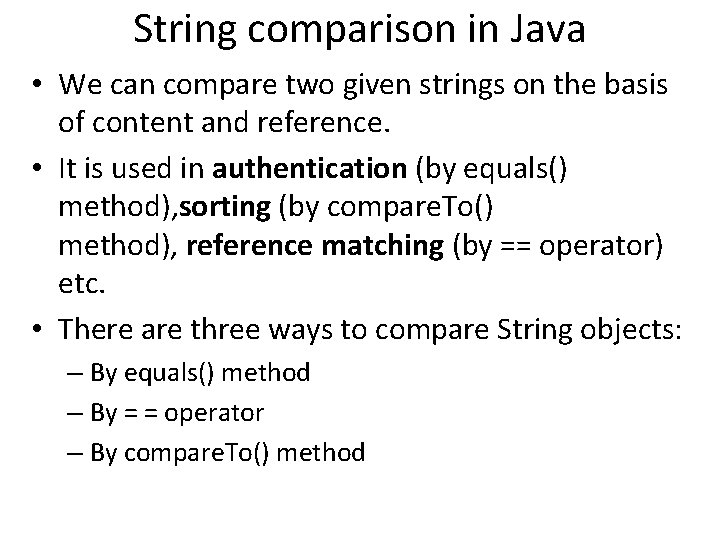
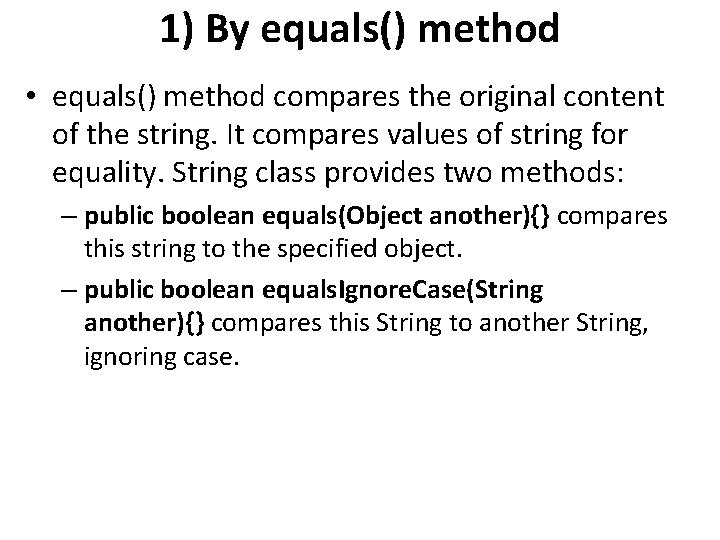
![class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-12.jpg)
![//Example of equals. Ignore. Case(String) method class Simple{ public static void main(String args[]){ String //Example of equals. Ignore. Case(String) method class Simple{ public static void main(String args[]){ String](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-13.jpg)
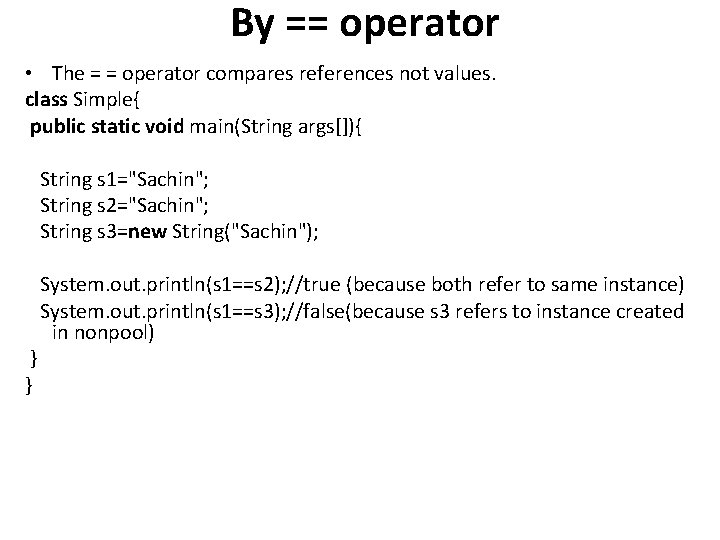
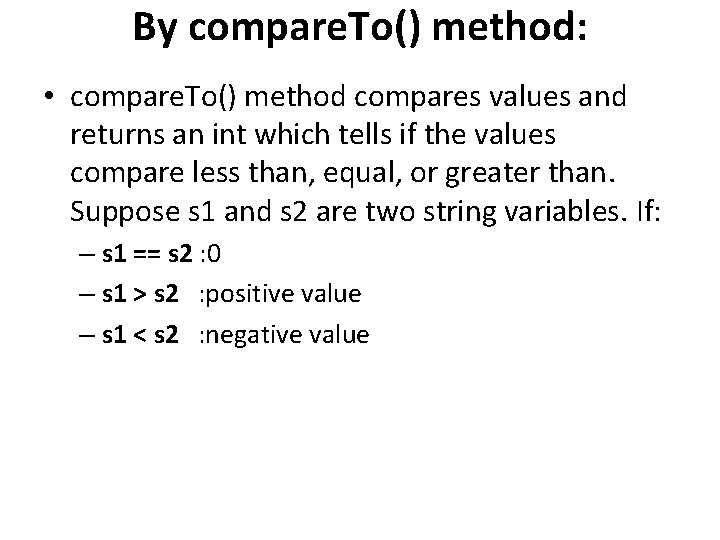
![class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-16.jpg)
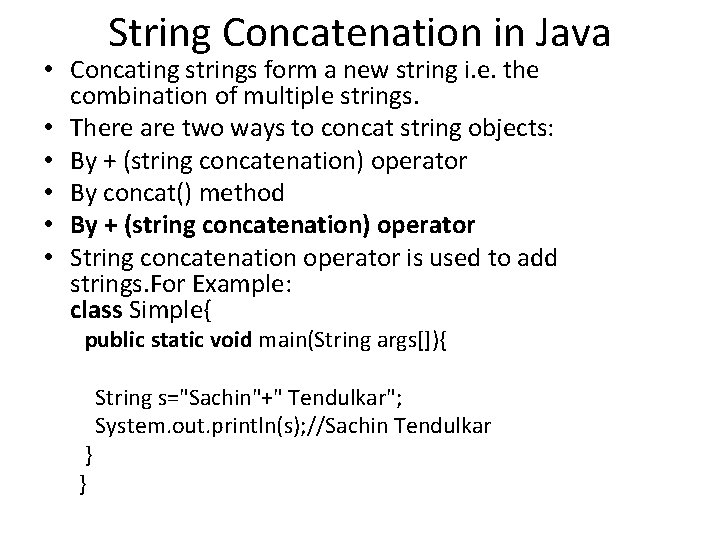
![class Simple{ public static void main(String args[]){ String s=50+30+"Sachin"+40+40; System. out. println(s); } } class Simple{ public static void main(String args[]){ String s=50+30+"Sachin"+40+40; System. out. println(s); } }](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-18.jpg)
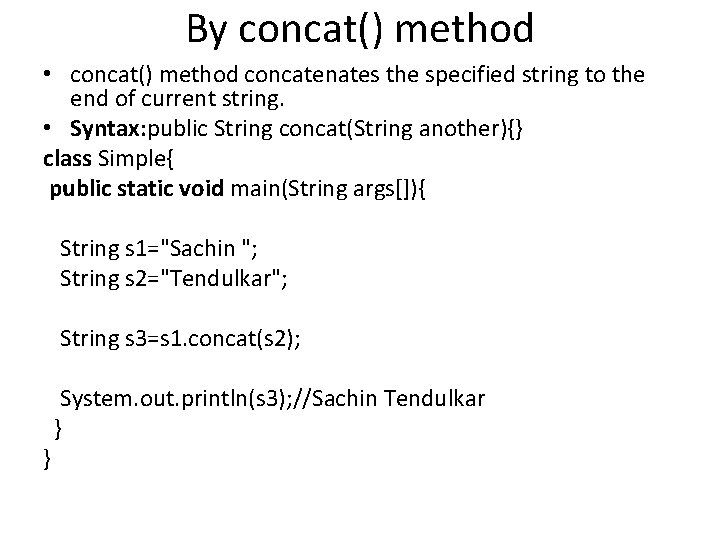
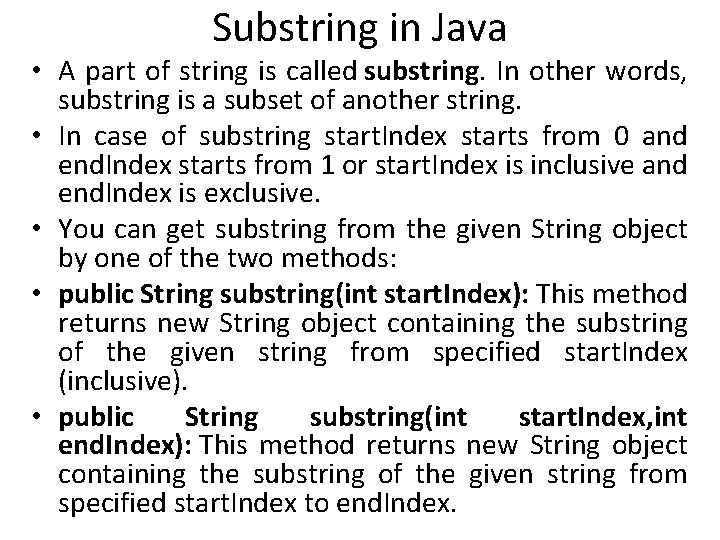
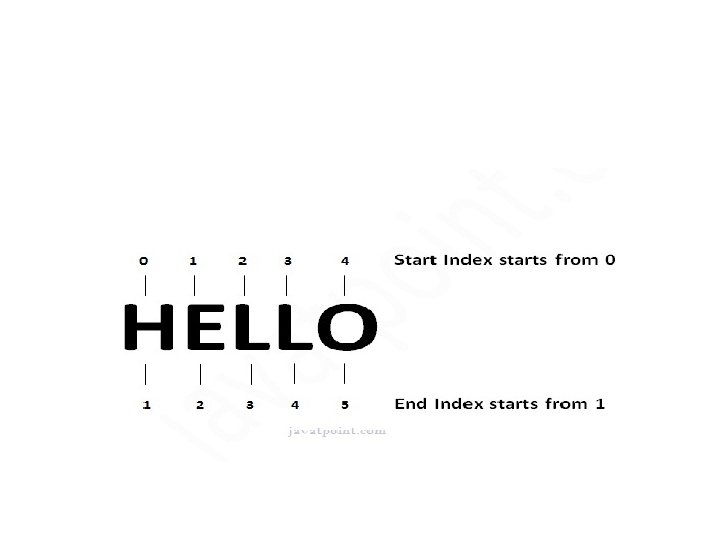
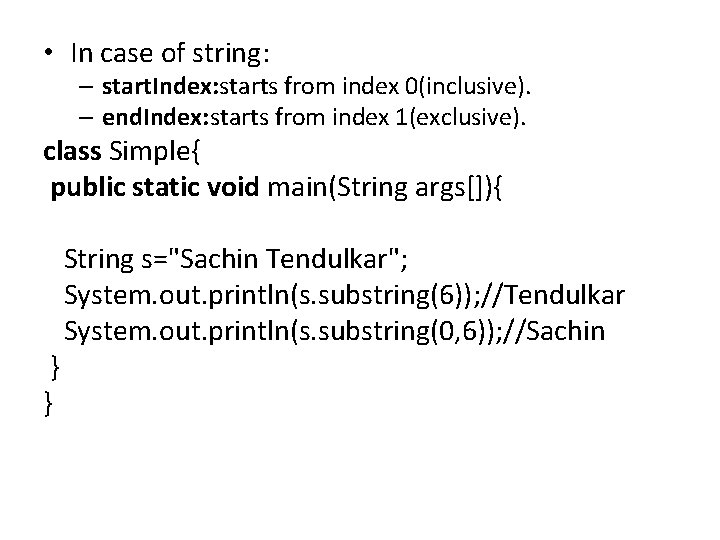
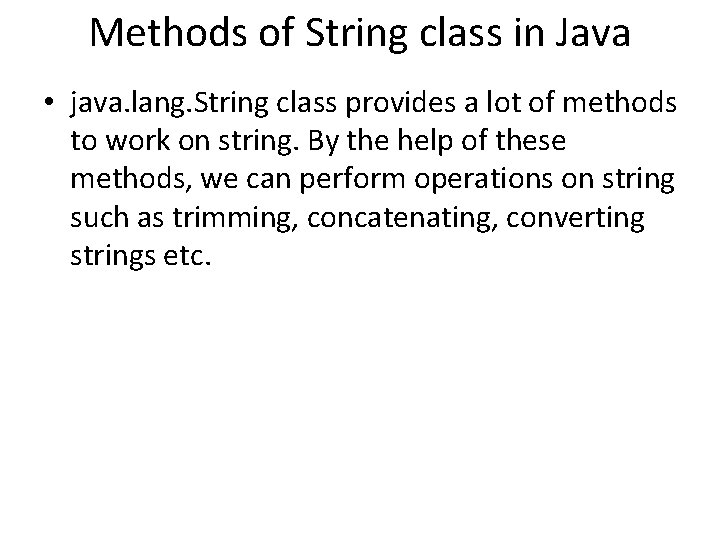
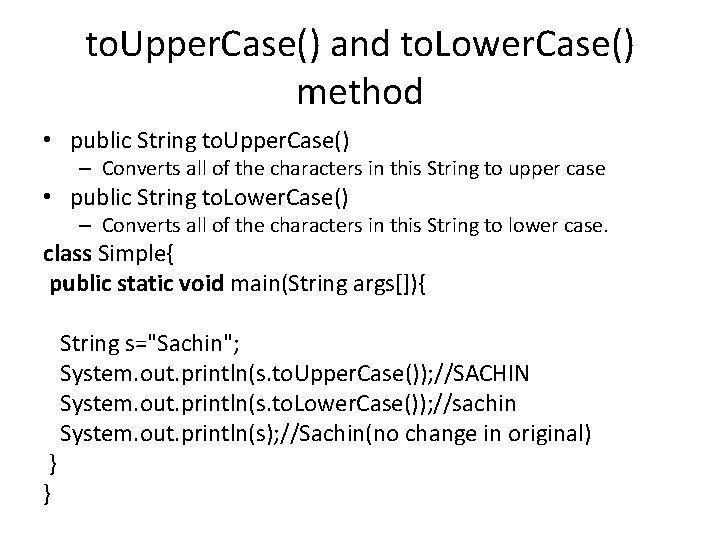
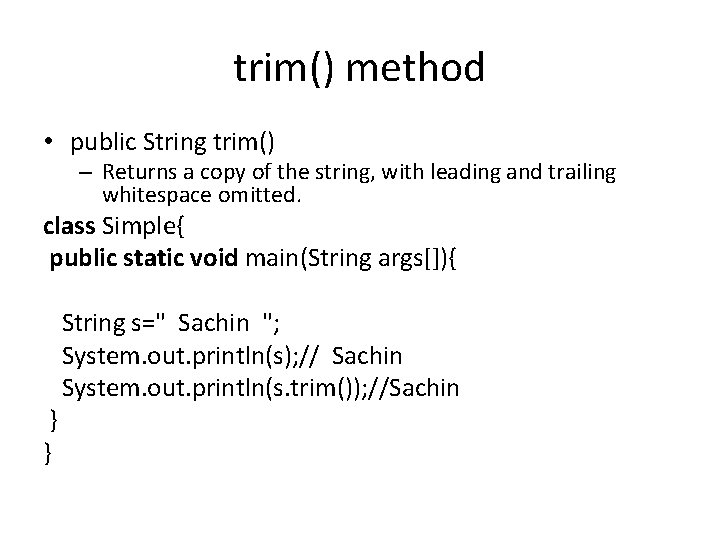
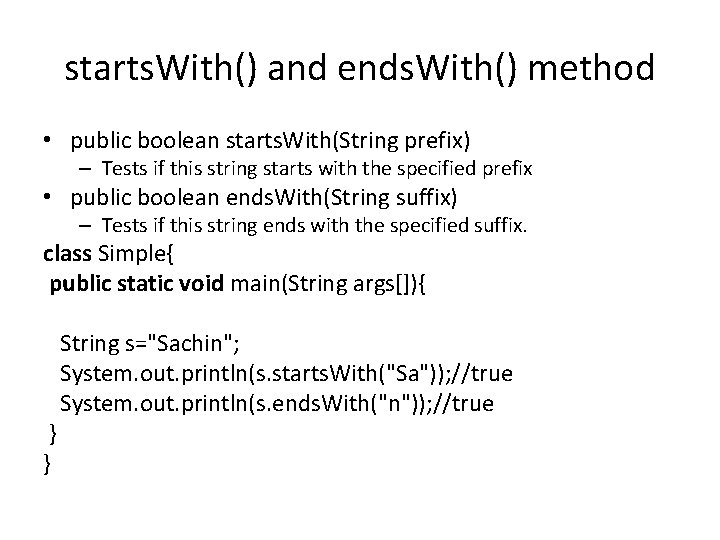
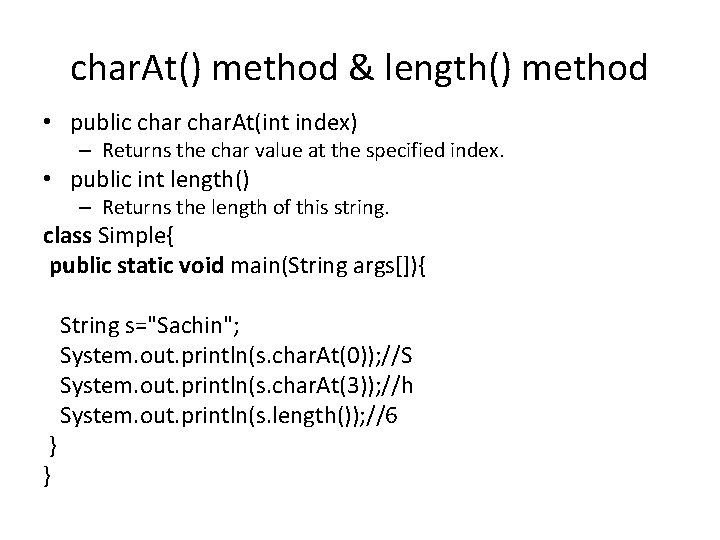
- Slides: 27
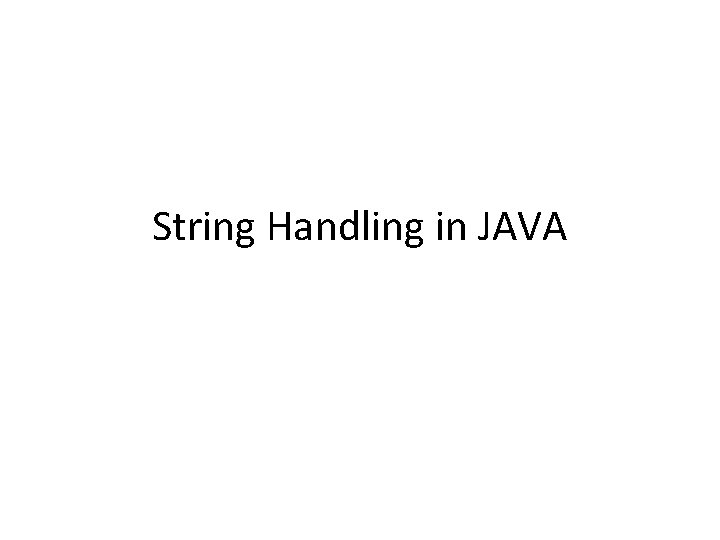
String Handling in JAVA
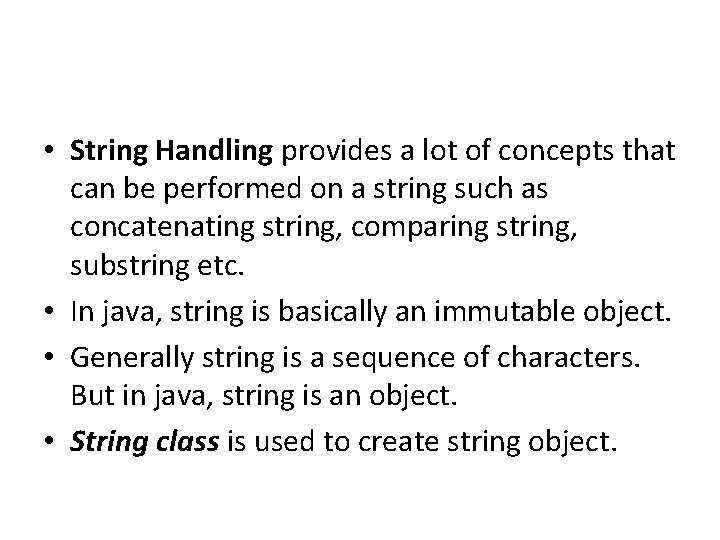
• String Handling provides a lot of concepts that can be performed on a string such as concatenating string, comparing string, substring etc. • In java, string is basically an immutable object. • Generally string is a sequence of characters. But in java, string is an object. • String class is used to create string object.
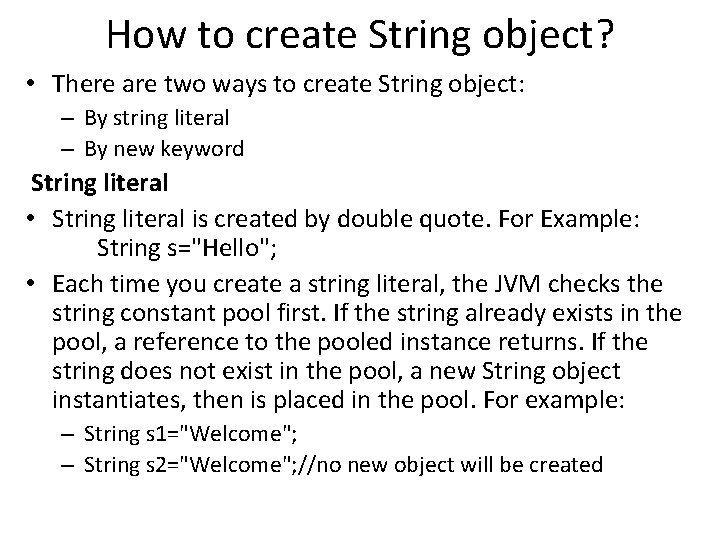
How to create String object? • There are two ways to create String object: – By string literal – By new keyword String literal • String literal is created by double quote. For Example: String s="Hello"; • Each time you create a string literal, the JVM checks the string constant pool first. If the string already exists in the pool, a reference to the pooled instance returns. If the string does not exist in the pool, a new String object instantiates, then is placed in the pool. For example: – String s 1="Welcome"; – String s 2="Welcome"; //no new object will be created
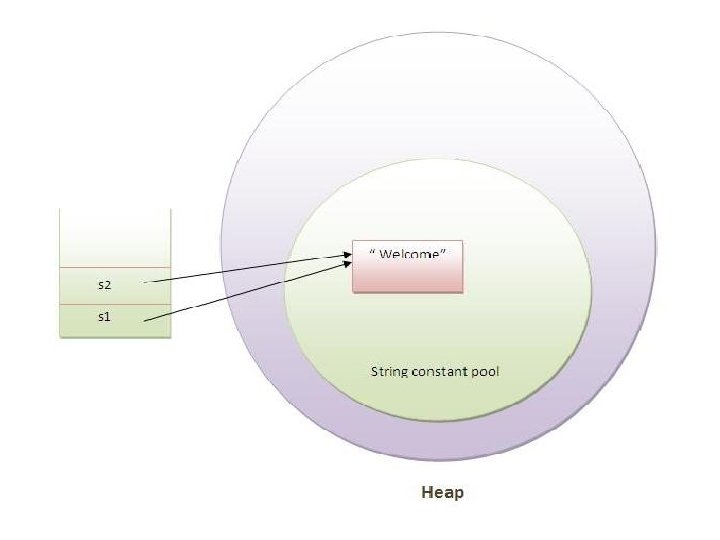
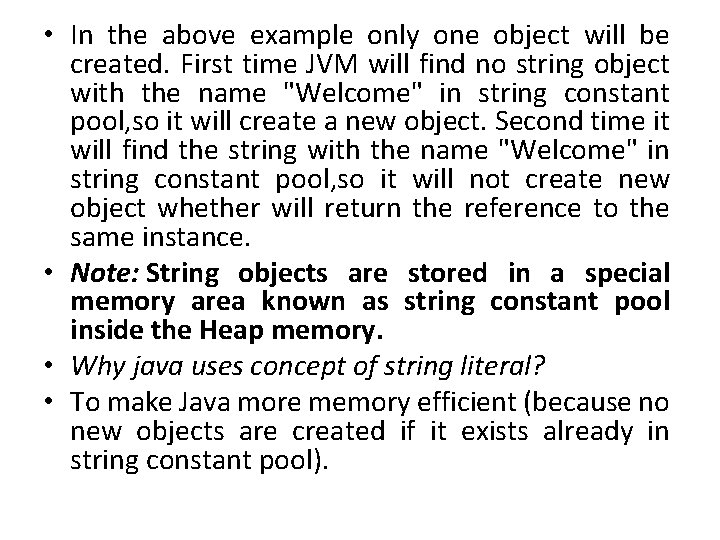
• In the above example only one object will be created. First time JVM will find no string object with the name "Welcome" in string constant pool, so it will create a new object. Second time it will find the string with the name "Welcome" in string constant pool, so it will not create new object whether will return the reference to the same instance. • Note: String objects are stored in a special memory area known as string constant pool inside the Heap memory. • Why java uses concept of string literal? • To make Java more memory efficient (because no new objects are created if it exists already in string constant pool).
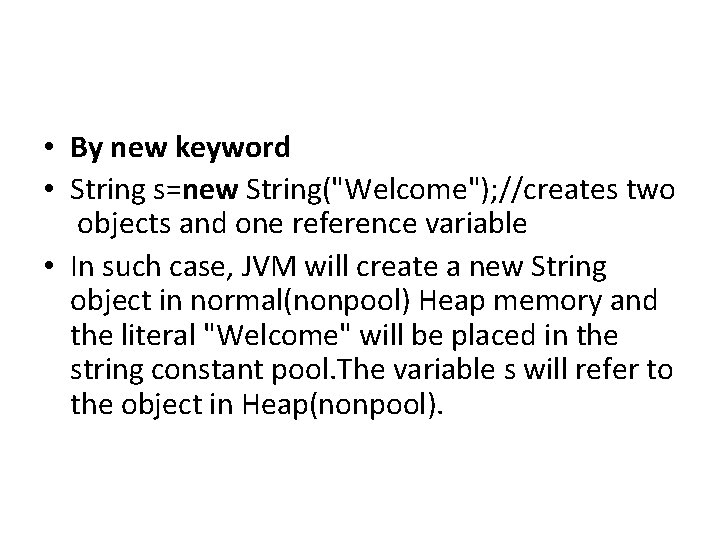
• By new keyword • String s=new String("Welcome"); //creates two objects and one reference variable • In such case, JVM will create a new String object in normal(nonpool) Heap memory and the literal "Welcome" will be placed in the string constant pool. The variable s will refer to the object in Heap(nonpool).
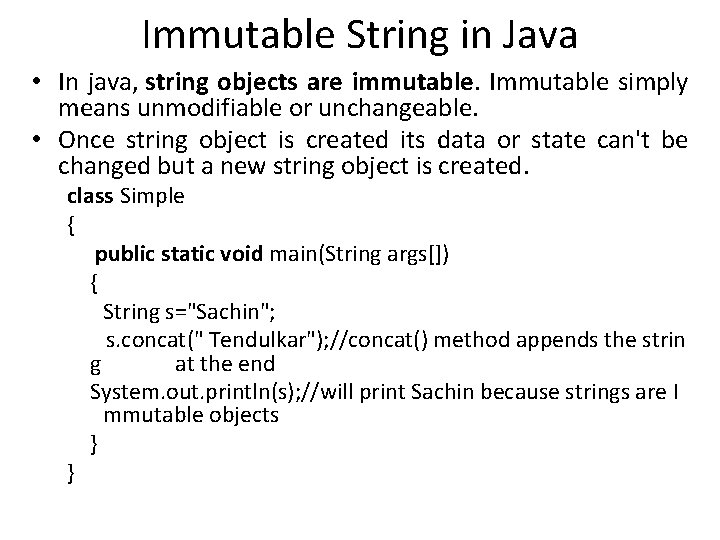
Immutable String in Java • In java, string objects are immutable. Immutable simply means unmodifiable or unchangeable. • Once string object is created its data or state can't be changed but a new string object is created. class Simple { public static void main(String args[]) { String s="Sachin"; s. concat(" Tendulkar"); //concat() method appends the strin g at the end System. out. println(s); //will print Sachin because strings are I mmutable objects } }
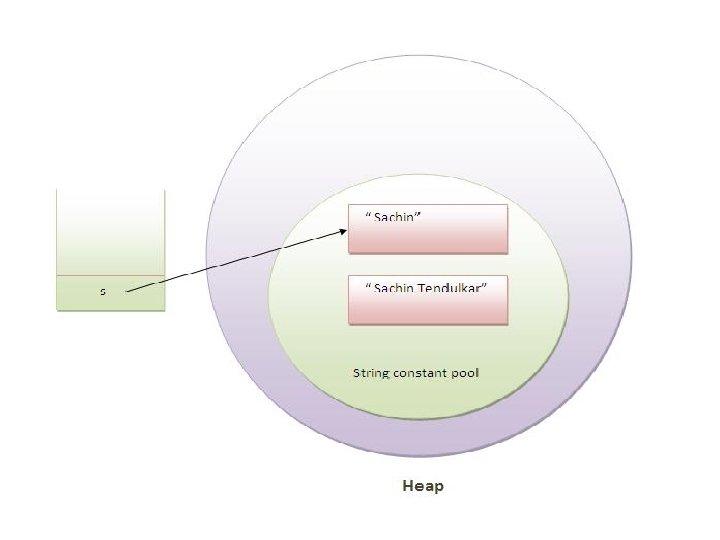
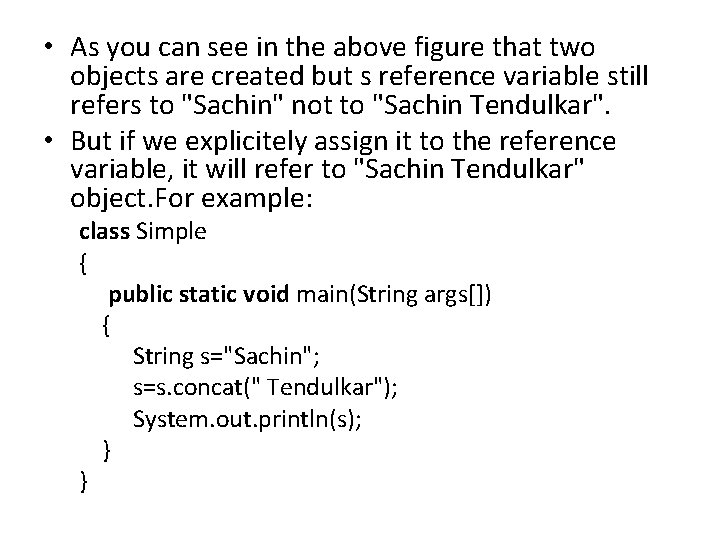
• As you can see in the above figure that two objects are created but s reference variable still refers to "Sachin" not to "Sachin Tendulkar". • But if we explicitely assign it to the reference variable, it will refer to "Sachin Tendulkar" object. For example: class Simple { public static void main(String args[]) { String s="Sachin"; s=s. concat(" Tendulkar"); System. out. println(s); } }
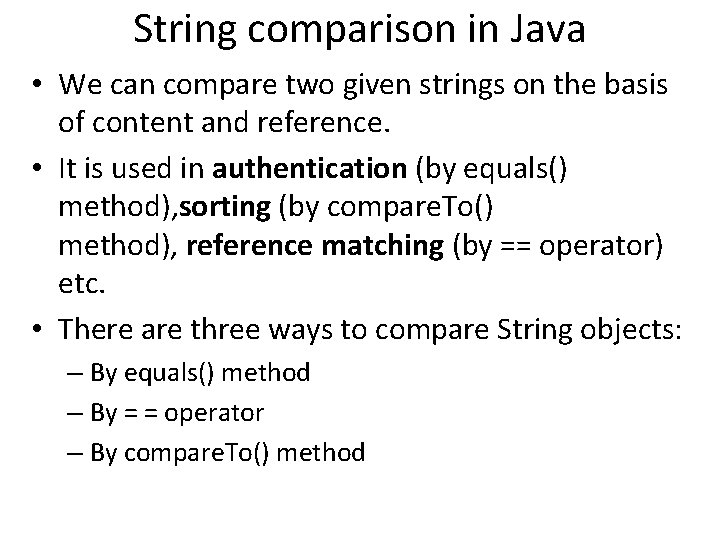
String comparison in Java • We can compare two given strings on the basis of content and reference. • It is used in authentication (by equals() method), sorting (by compare. To() method), reference matching (by == operator) etc. • There are three ways to compare String objects: – By equals() method – By = = operator – By compare. To() method
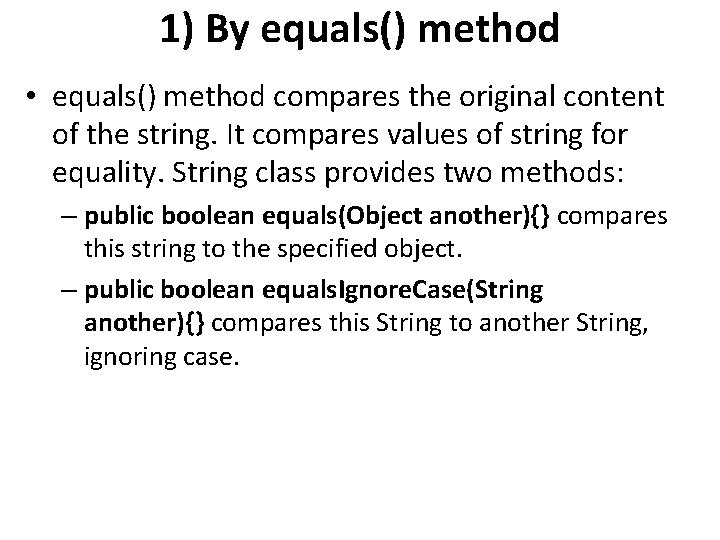
1) By equals() method • equals() method compares the original content of the string. It compares values of string for equality. String class provides two methods: – public boolean equals(Object another){} compares this string to the specified object. – public boolean equals. Ignore. Case(String another){} compares this String to another String, ignoring case.
![class Simple public static void mainString args String s 1Sachin String s 2Sachin String class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-12.jpg)
class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String s 3=new String("Sachin"); String s 4="Saurav"; System. out. println(s 1. equals(s 2)); //true System. out. println(s 1. equals(s 3)); //true System. out. println(s 1. equals(s 4)); //false } }
![Example of equals Ignore CaseString method class Simple public static void mainString args String //Example of equals. Ignore. Case(String) method class Simple{ public static void main(String args[]){ String](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-13.jpg)
//Example of equals. Ignore. Case(String) method class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="SACHIN"; System. out. println(s 1. equals(s 2)); //false System. out. println(s 1. equals. Ignore. Case(s 3)); //tru e } }
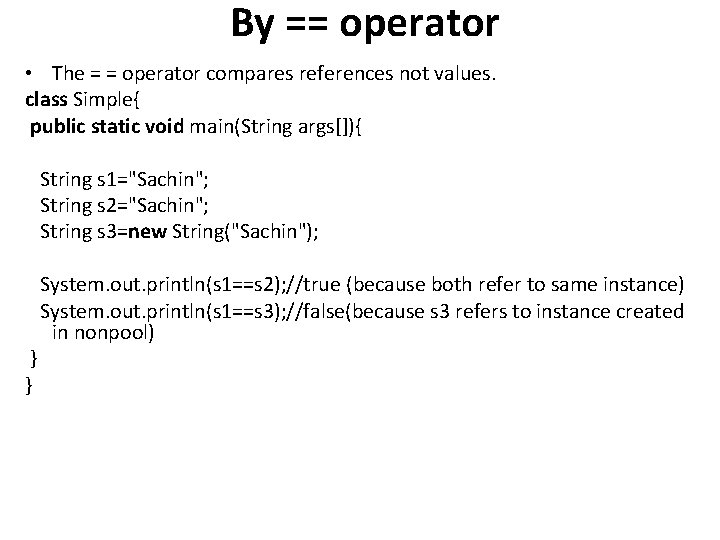
By == operator • The = = operator compares references not values. class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String s 3=new String("Sachin"); System. out. println(s 1==s 2); //true (because both refer to same instance) System. out. println(s 1==s 3); //false(because s 3 refers to instance created in nonpool) } }
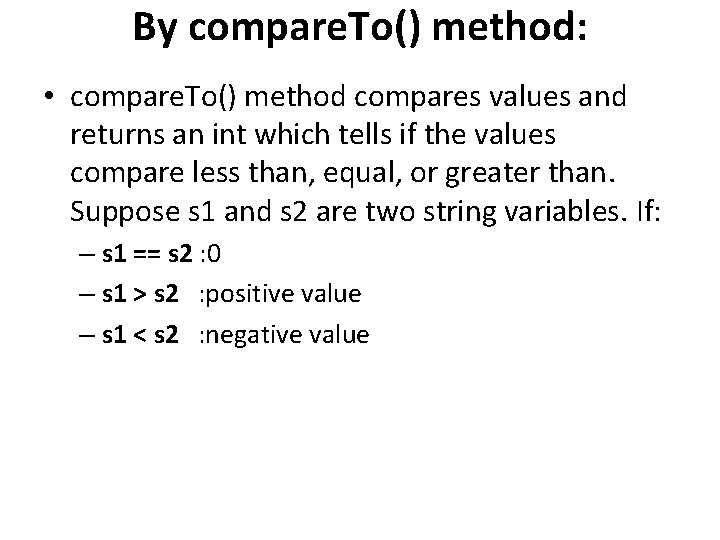
By compare. To() method: • compare. To() method compares values and returns an int which tells if the values compare less than, equal, or greater than. Suppose s 1 and s 2 are two string variables. If: – s 1 == s 2 : 0 – s 1 > s 2 : positive value – s 1 < s 2 : negative value
![class Simple public static void mainString args String s 1Sachin String s 2Sachin String class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-16.jpg)
class Simple{ public static void main(String args[]){ String s 1="Sachin"; String s 2="Sachin"; String s 3="Ratan"; System. out. println(s 1. compare. To(s 2)); //0 System. out. println(s 1. compare. To(s 3)); //1(because s 1>s 3) System. out. println(s 3. compare. To(s 1)); //1(because s 3 < s 1 ) } }
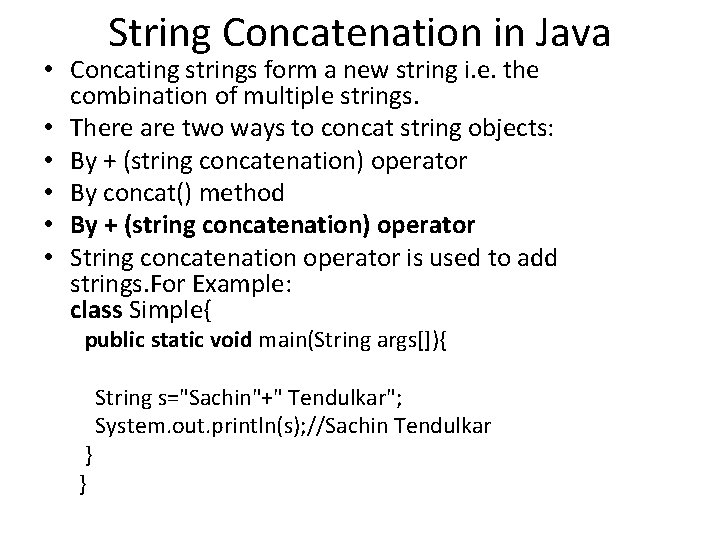
String Concatenation in Java • Concating strings form a new string i. e. the combination of multiple strings. • There are two ways to concat string objects: • By + (string concatenation) operator • By concat() method • By + (string concatenation) operator • String concatenation operator is used to add strings. For Example: class Simple{ public static void main(String args[]){ String s="Sachin"+" Tendulkar"; System. out. println(s); //Sachin Tendulkar } }
![class Simple public static void mainString args String s5030Sachin4040 System out printlns class Simple{ public static void main(String args[]){ String s=50+30+"Sachin"+40+40; System. out. println(s); } }](https://slidetodoc.com/presentation_image_h/0dc4974f54bcd8fa17d4f3ed9541ef60/image-18.jpg)
class Simple{ public static void main(String args[]){ String s=50+30+"Sachin"+40+40; System. out. println(s); } }
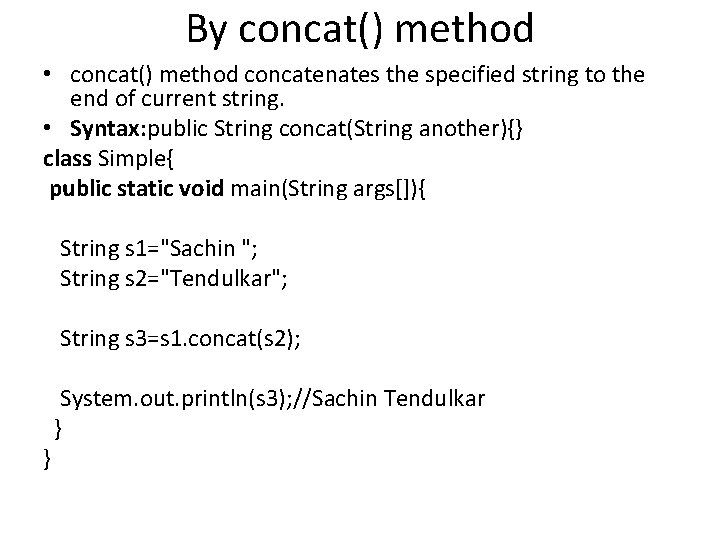
By concat() method • concat() method concatenates the specified string to the end of current string. • Syntax: public String concat(String another){} class Simple{ public static void main(String args[]){ String s 1="Sachin "; String s 2="Tendulkar"; String s 3=s 1. concat(s 2); System. out. println(s 3); //Sachin Tendulkar } }
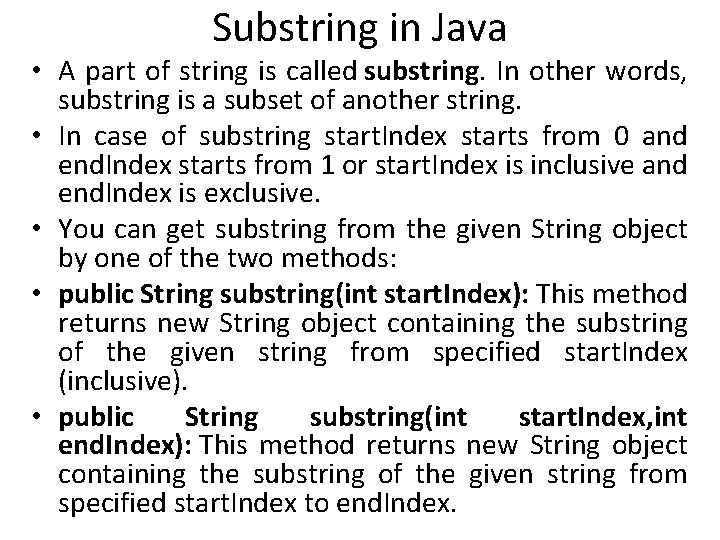
Substring in Java • A part of string is called substring. In other words, substring is a subset of another string. • In case of substring start. Index starts from 0 and end. Index starts from 1 or start. Index is inclusive and end. Index is exclusive. • You can get substring from the given String object by one of the two methods: • public String substring(int start. Index): This method returns new String object containing the substring of the given string from specified start. Index (inclusive). • public String substring(int start. Index, int end. Index): This method returns new String object containing the substring of the given string from specified start. Index to end. Index.
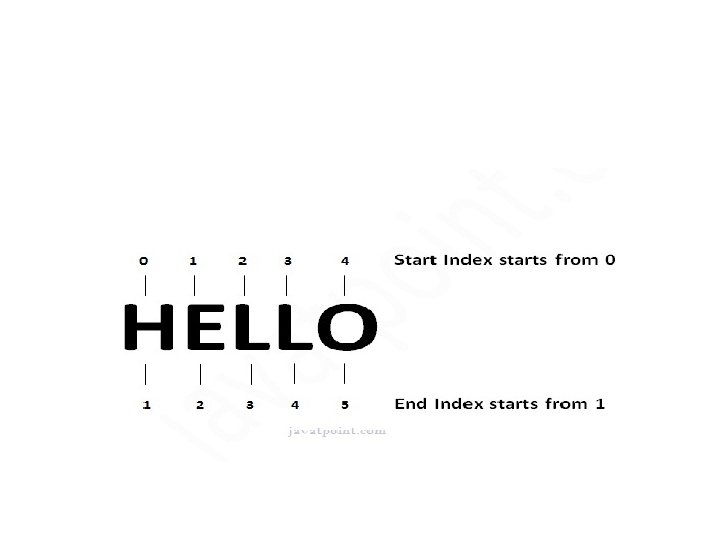
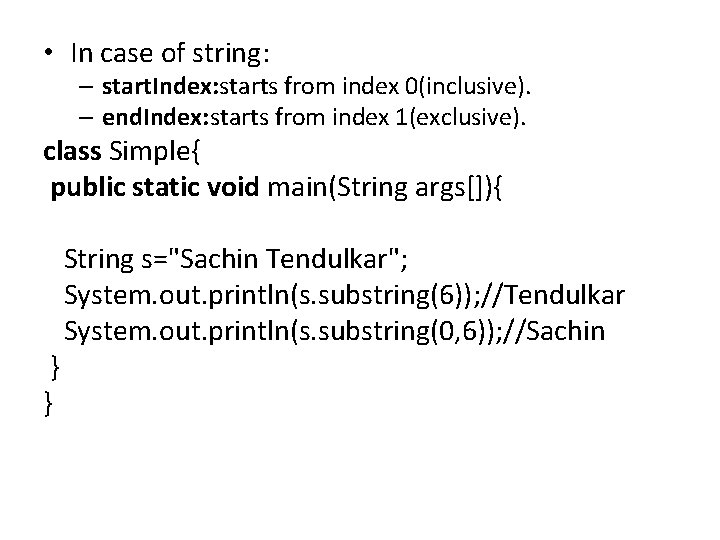
• In case of string: – start. Index: starts from index 0(inclusive). – end. Index: starts from index 1(exclusive). class Simple{ public static void main(String args[]){ String s="Sachin Tendulkar"; System. out. println(s. substring(6)); //Tendulkar System. out. println(s. substring(0, 6)); //Sachin } }
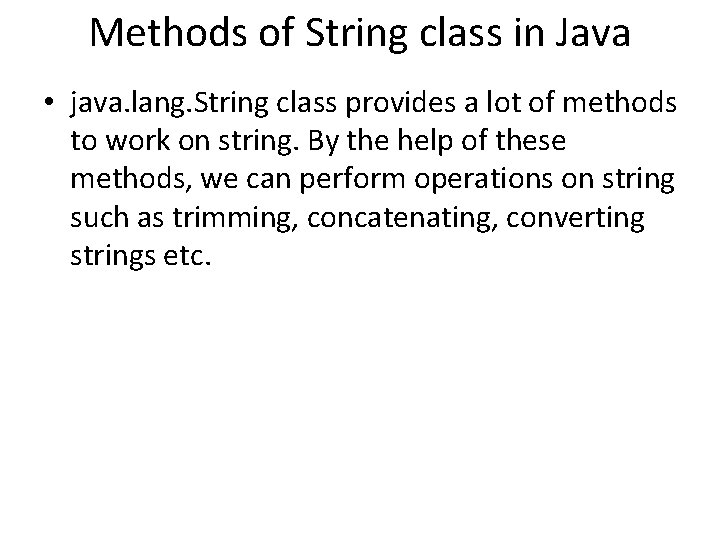
Methods of String class in Java • java. lang. String class provides a lot of methods to work on string. By the help of these methods, we can perform operations on string such as trimming, concatenating, converting strings etc.
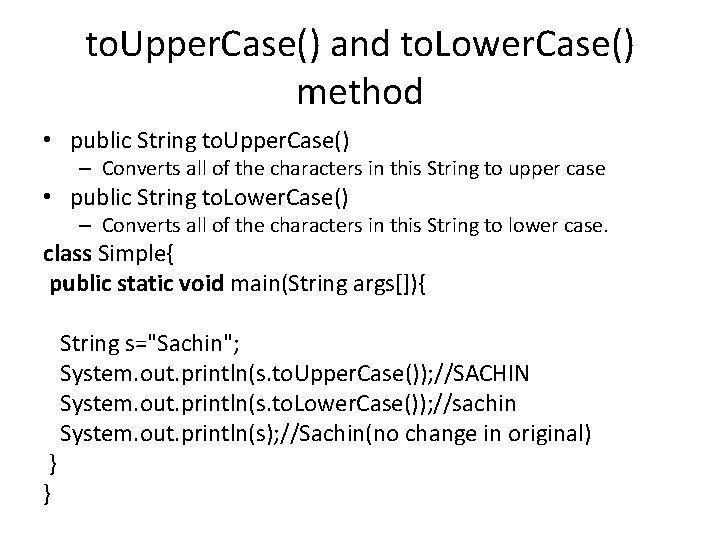
to. Upper. Case() and to. Lower. Case() method • public String to. Upper. Case() – Converts all of the characters in this String to upper case • public String to. Lower. Case() – Converts all of the characters in this String to lower case. class Simple{ public static void main(String args[]){ String s="Sachin"; System. out. println(s. to. Upper. Case()); //SACHIN System. out. println(s. to. Lower. Case()); //sachin System. out. println(s); //Sachin(no change in original) } }
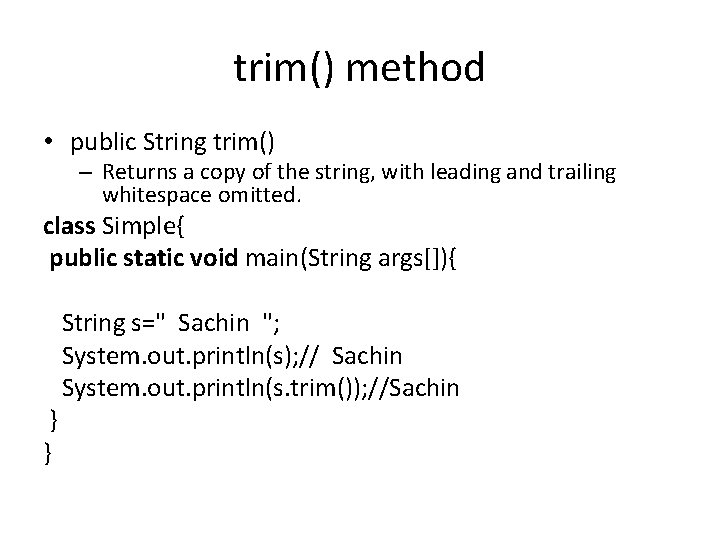
trim() method • public String trim() – Returns a copy of the string, with leading and trailing whitespace omitted. class Simple{ public static void main(String args[]){ String s=" Sachin "; System. out. println(s); // Sachin System. out. println(s. trim()); //Sachin } }
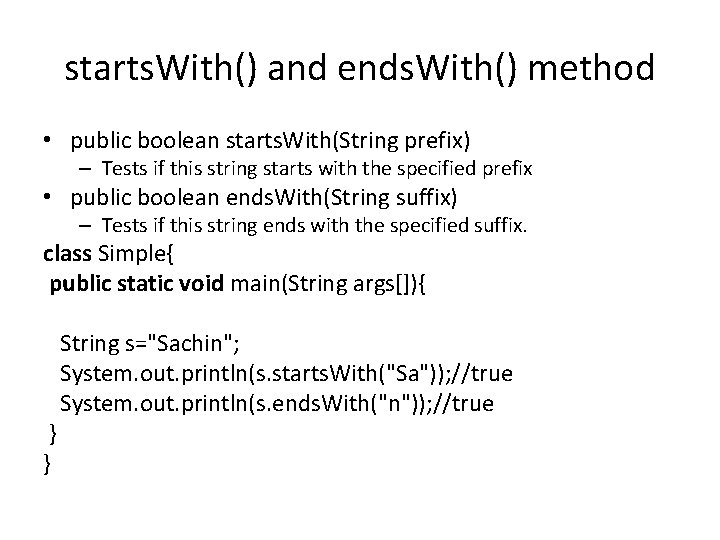
starts. With() and ends. With() method • public boolean starts. With(String prefix) – Tests if this string starts with the specified prefix • public boolean ends. With(String suffix) – Tests if this string ends with the specified suffix. class Simple{ public static void main(String args[]){ String s="Sachin"; System. out. println(s. starts. With("Sa")); //true System. out. println(s. ends. With("n")); //true } }
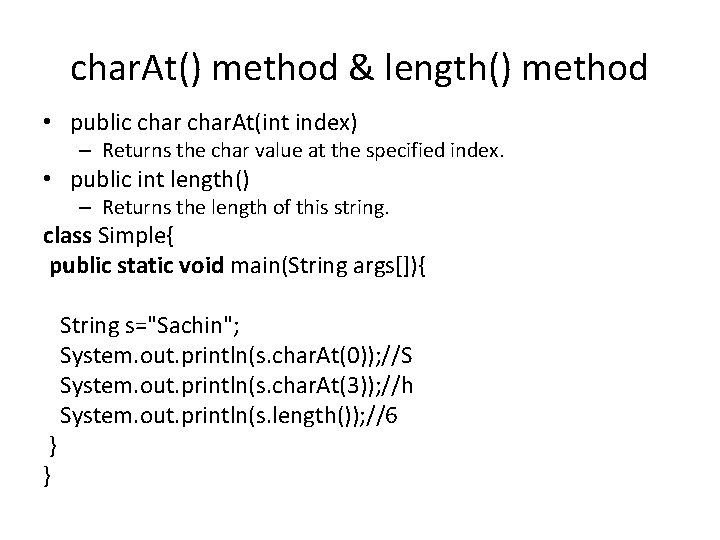
char. At() method & length() method • public char. At(int index) – Returns the char value at the specified index. • public int length() – Returns the length of this string. class Simple{ public static void main(String args[]){ String s="Sachin"; System. out. println(s. char. At(0)); //S System. out. println(s. char. At(3)); //h System. out. println(s. length()); //6 } }