String and character functions include string h include
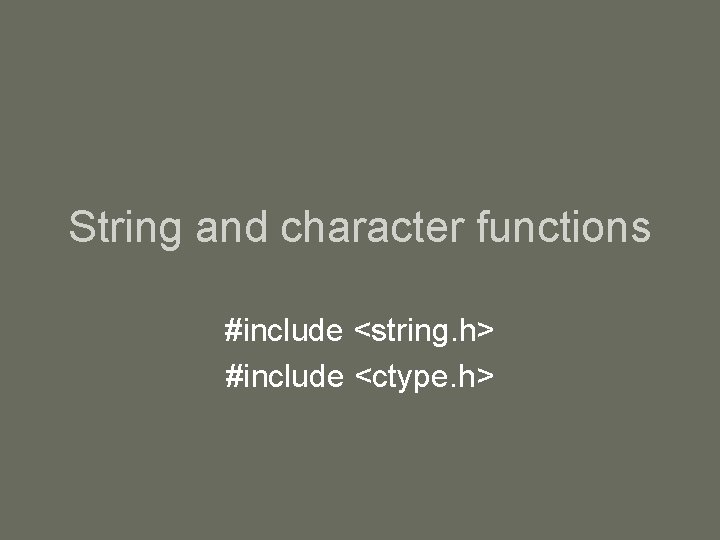
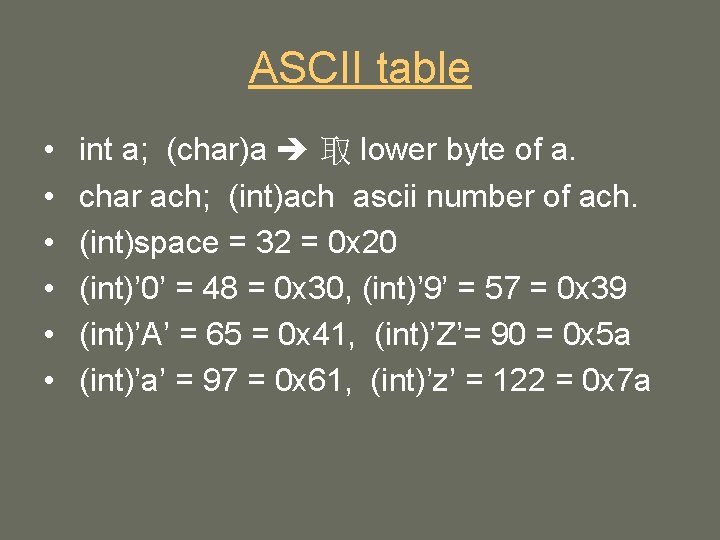
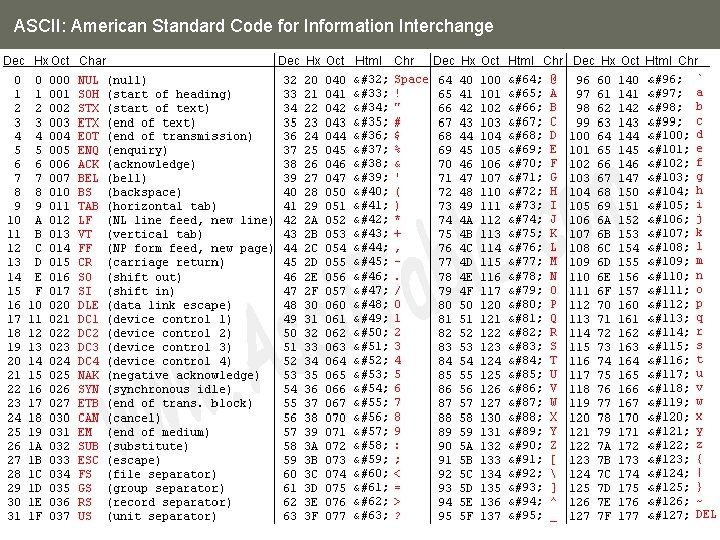
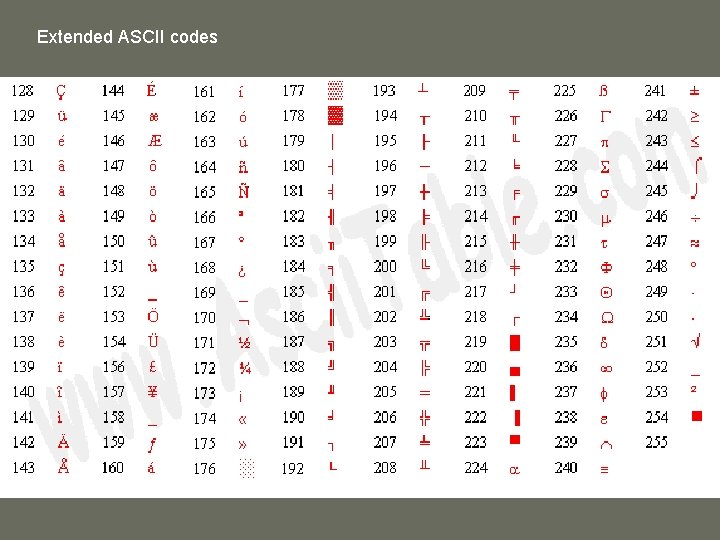
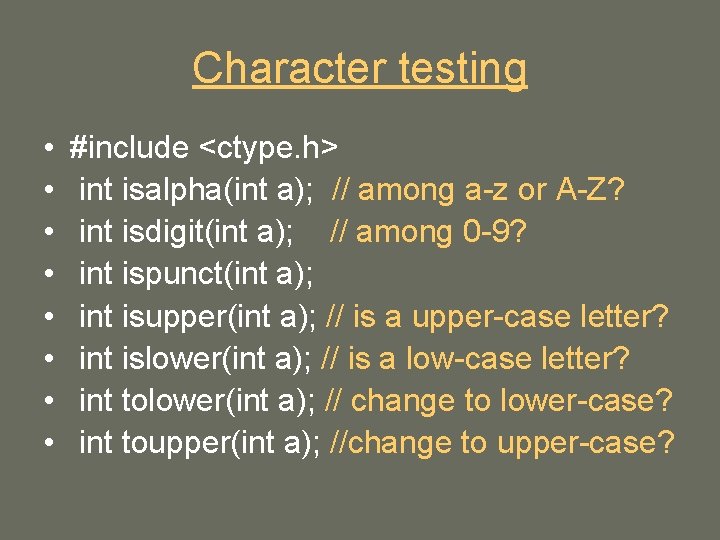
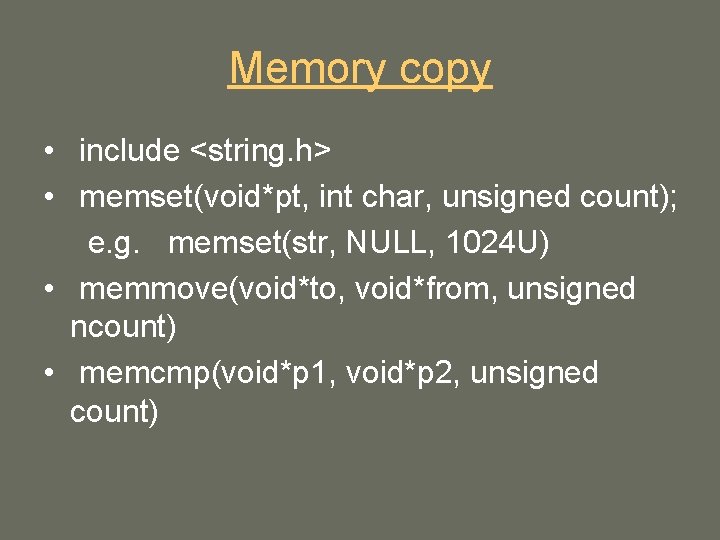
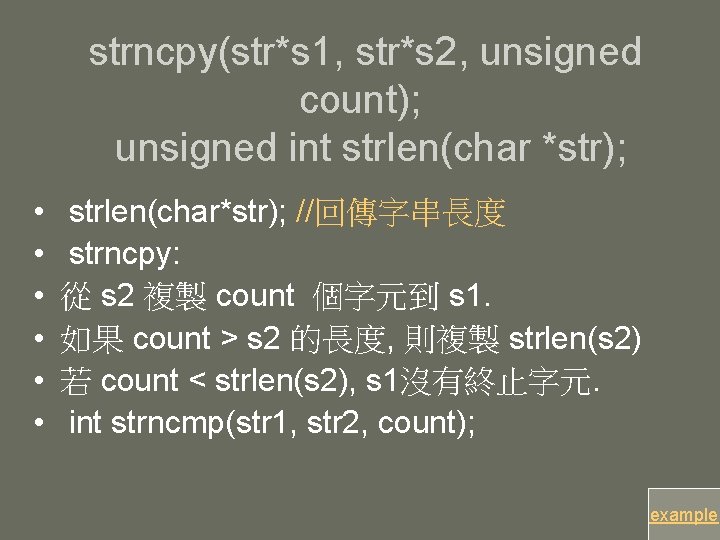
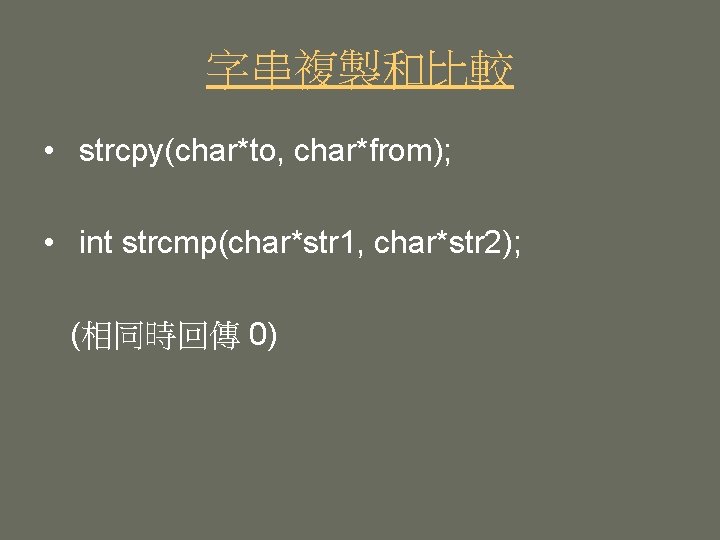
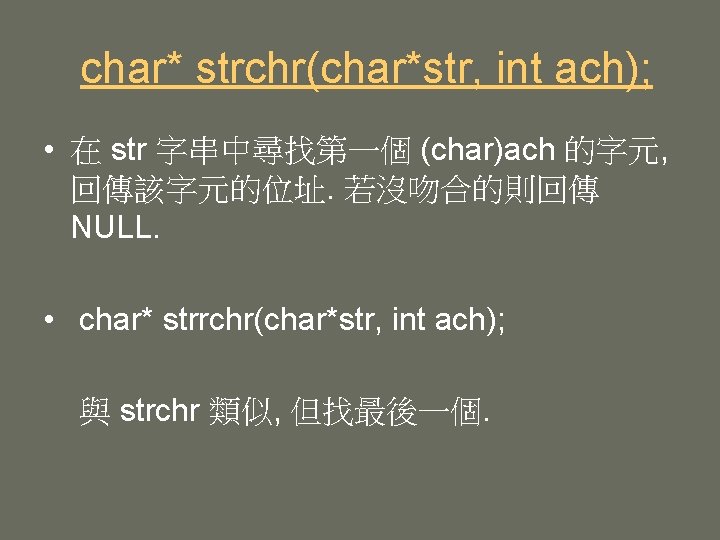
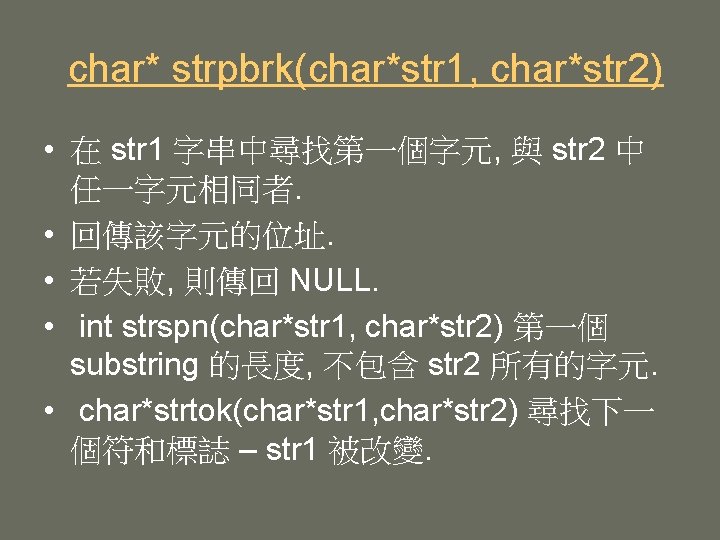
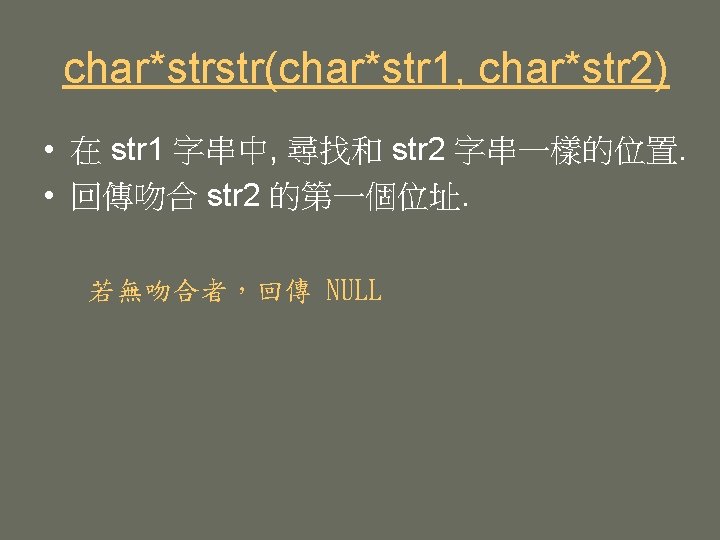
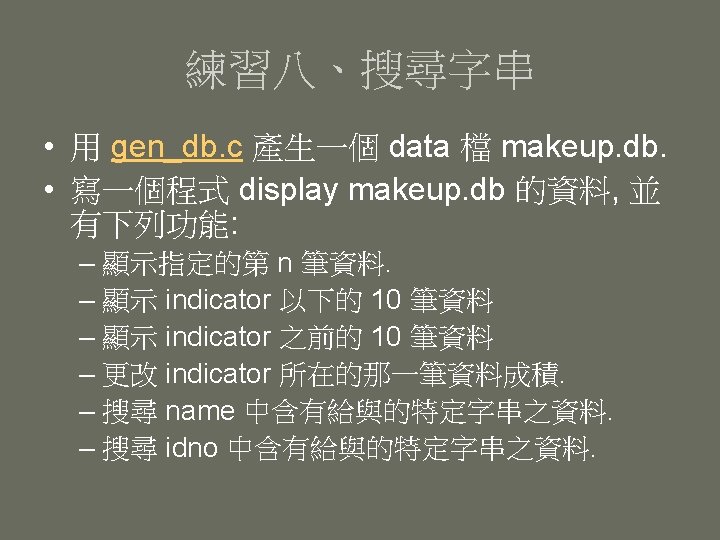
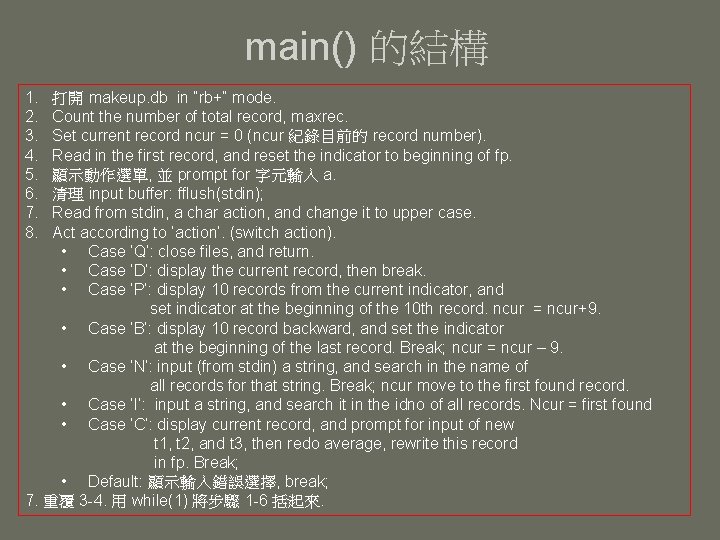
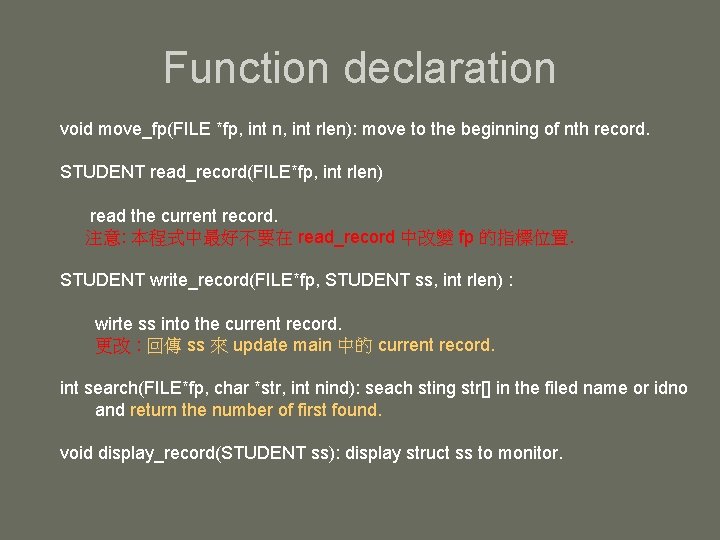
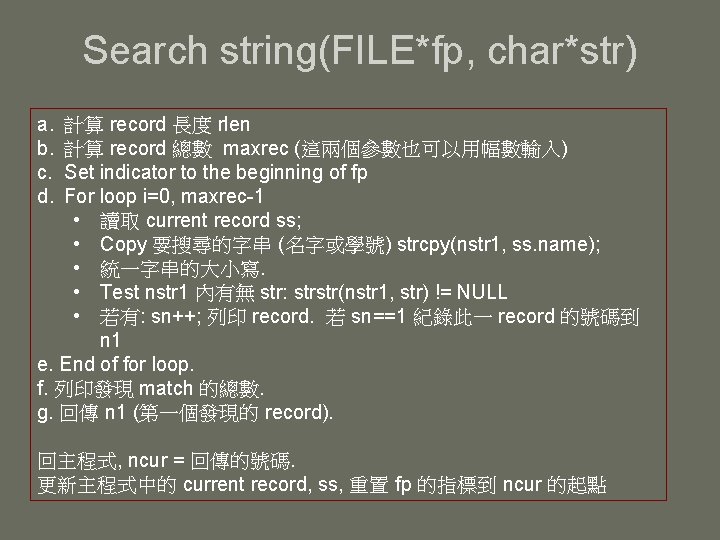
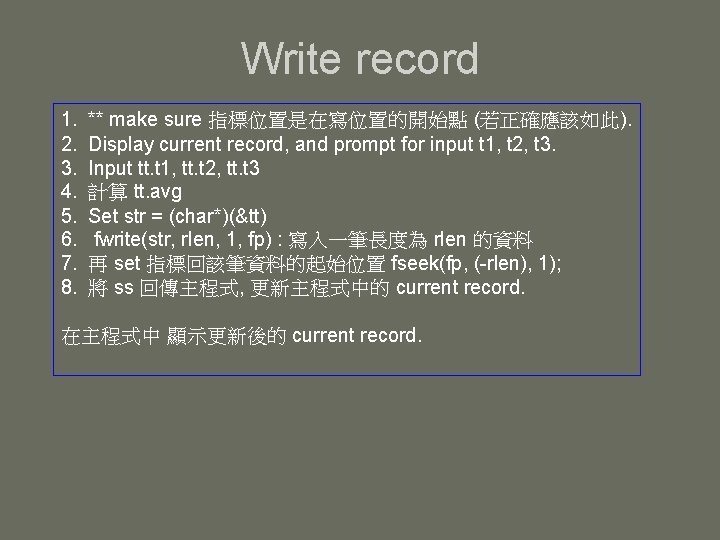
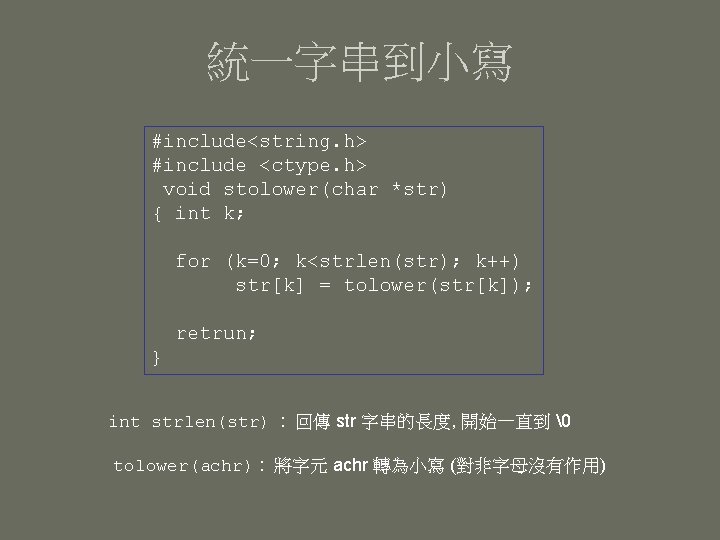
- Slides: 17
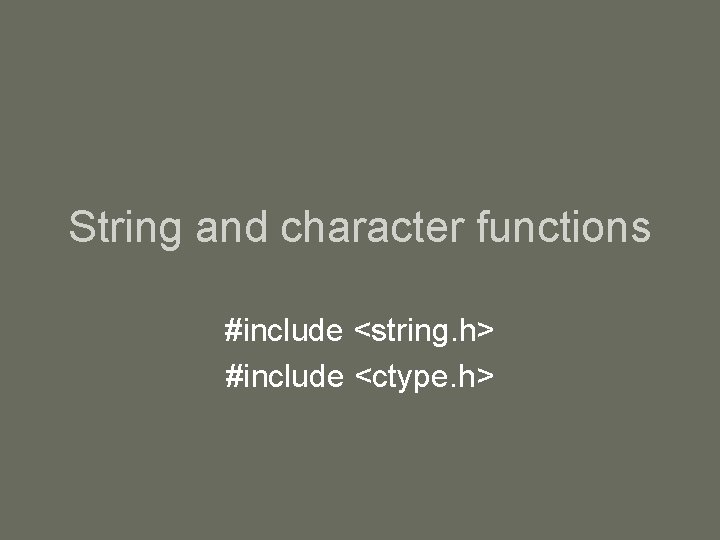
String and character functions #include <string. h> #include <ctype. h>
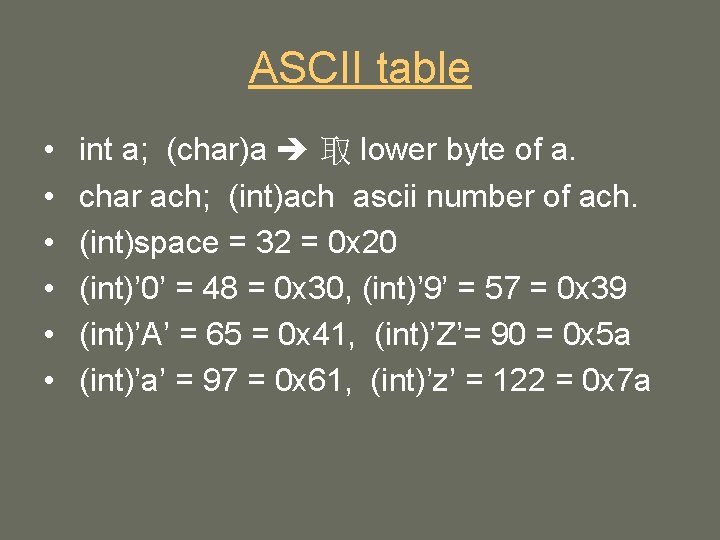
ASCII table • • • int a; (char)a 取 lower byte of a. char ach; (int)ach ascii number of ach. (int)space = 32 = 0 x 20 (int)’ 0’ = 48 = 0 x 30, (int)’ 9’ = 57 = 0 x 39 (int)’A’ = 65 = 0 x 41, (int)’Z’= 90 = 0 x 5 a (int)’a’ = 97 = 0 x 61, (int)’z’ = 122 = 0 x 7 a
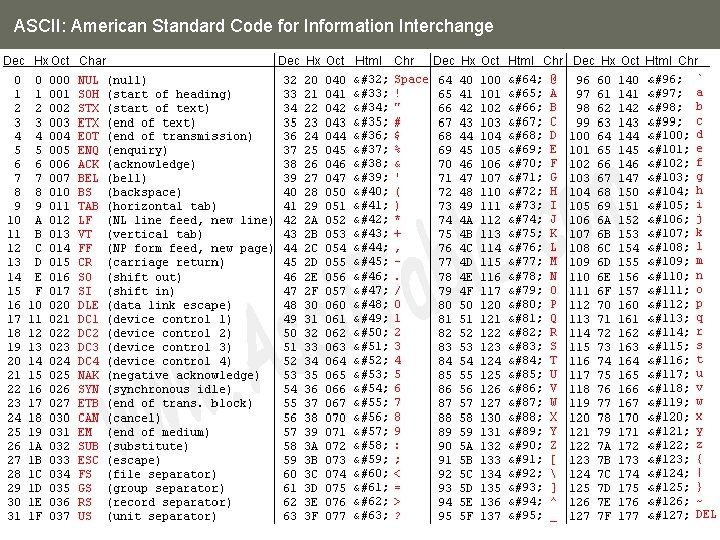
ASCII: American Standard Code for Information Interchange
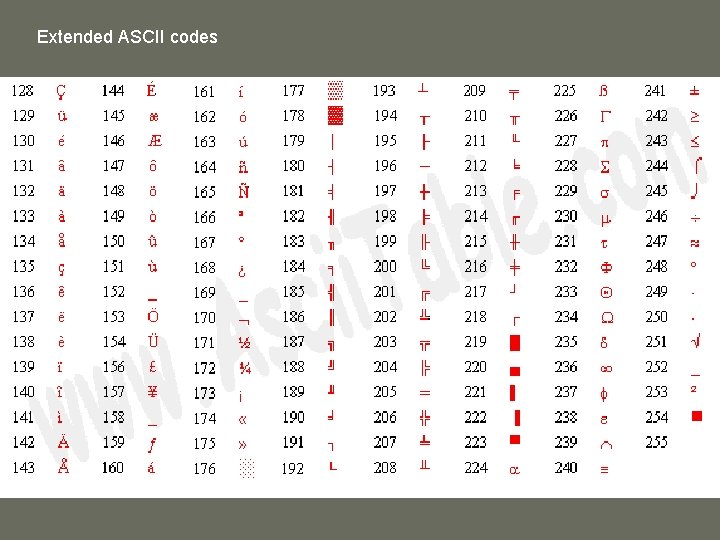
Extended ASCII codes
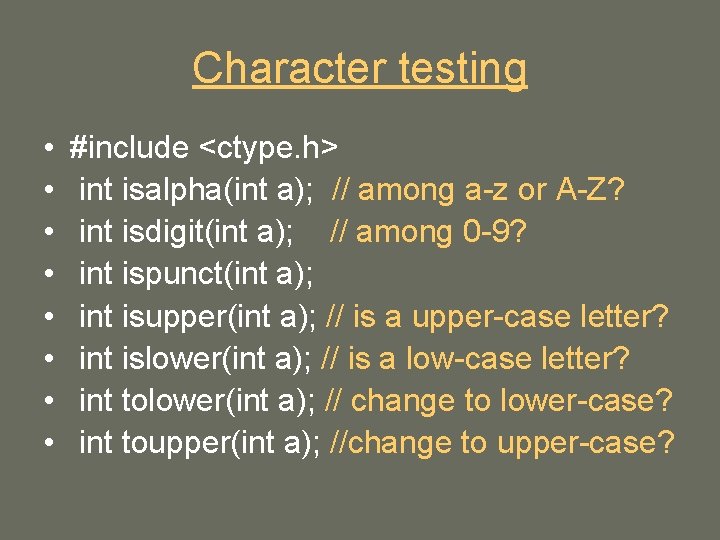
Character testing • • #include <ctype. h> int isalpha(int a); // among a-z or A-Z? int isdigit(int a); // among 0 -9? int ispunct(int a); int isupper(int a); // is a upper-case letter? int islower(int a); // is a low-case letter? int tolower(int a); // change to lower-case? int toupper(int a); //change to upper-case?
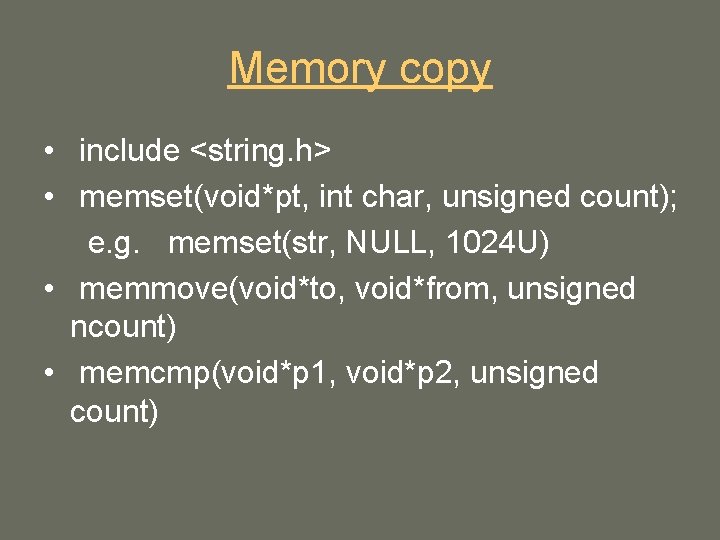
Memory copy • include <string. h> • memset(void*pt, int char, unsigned count); e. g. memset(str, NULL, 1024 U) • memmove(void*to, void*from, unsigned ncount) • memcmp(void*p 1, void*p 2, unsigned count)
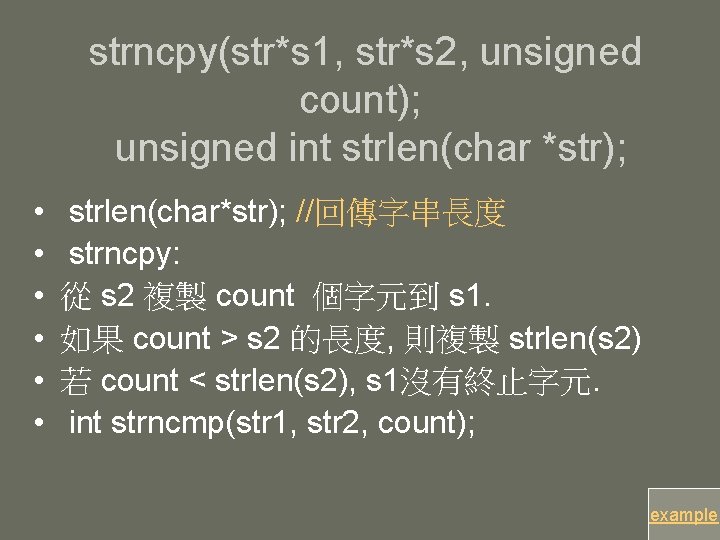
strncpy(str*s 1, str*s 2, unsigned count); unsigned int strlen(char *str); • • • strlen(char*str); //回傳字串長度 strncpy: 從 s 2 複製 count 個字元到 s 1. 如果 count > s 2 的長度, 則複製 strlen(s 2) 若 count < strlen(s 2), s 1沒有終止字元. int strncmp(str 1, str 2, count); example
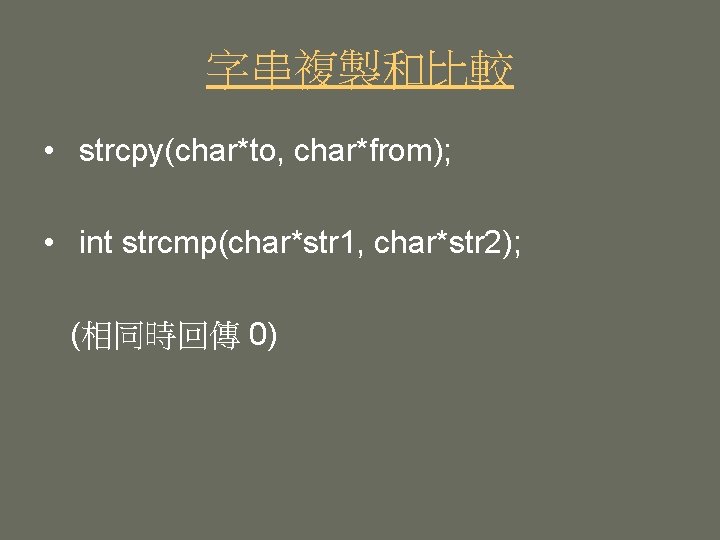
字串複製和比較 • strcpy(char*to, char*from); • int strcmp(char*str 1, char*str 2); (相同時回傳 0)
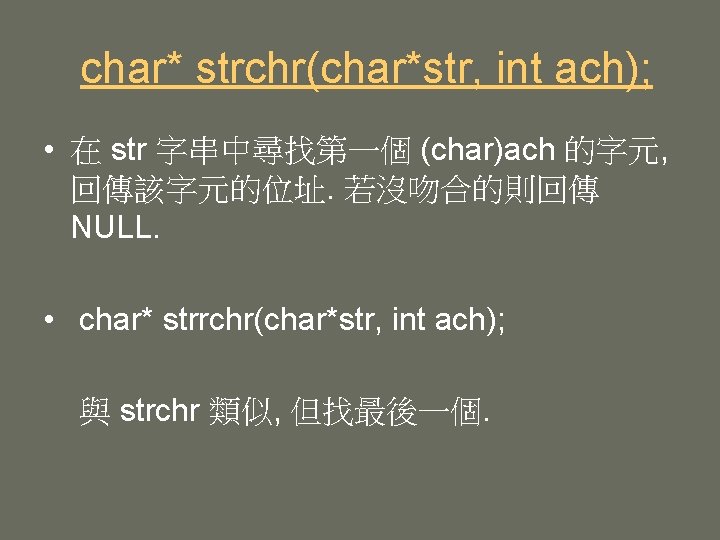
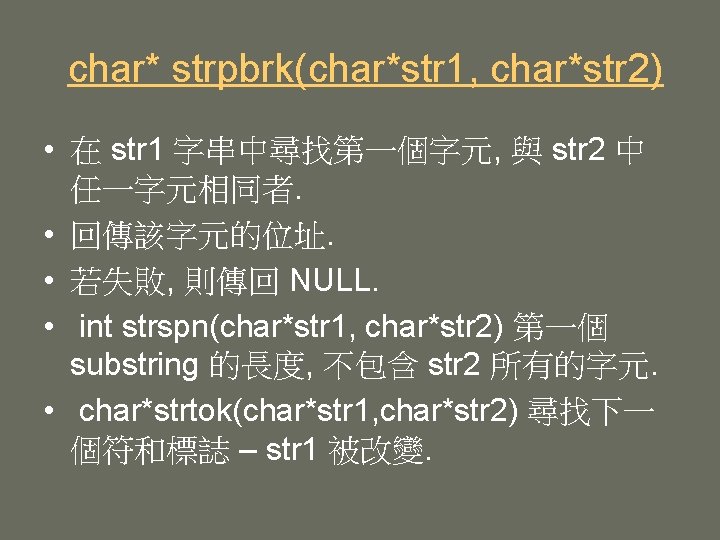
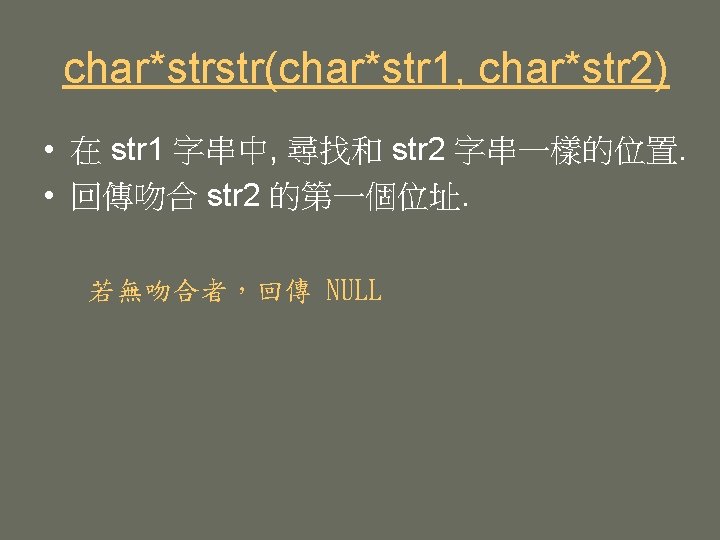
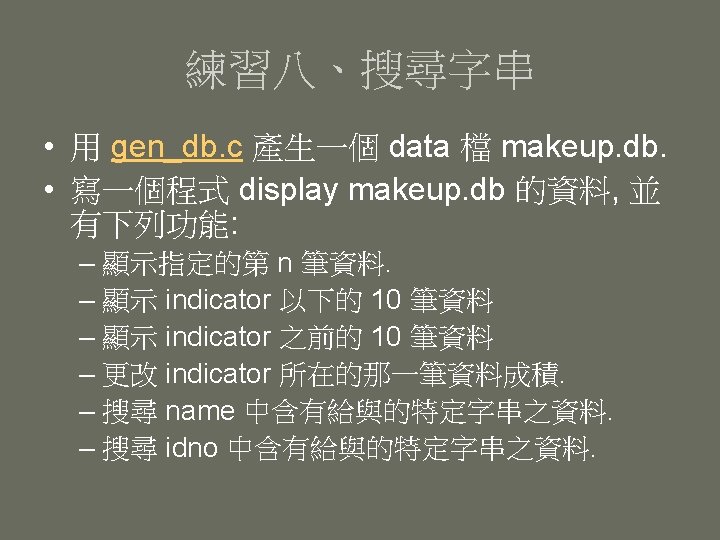
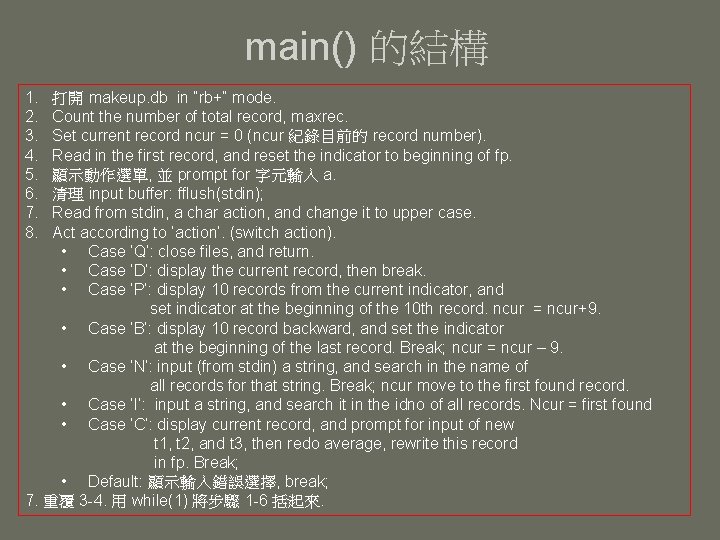
main() 的結構 1. 2. 3. 4. 5. 6. 7. 8. 打開 makeup. db in “rb+” mode. Count the number of total record, maxrec. Set current record ncur = 0 (ncur 紀錄目前的 record number). Read in the first record, and reset the indicator to beginning of fp. 顯示動作選單, 並 prompt for 字元輸入 a. 清理 input buffer: fflush(stdin); Read from stdin, a char action, and change it to upper case. Act according to ‘action’. (switch action). • Case ‘Q’: close files, and return. • Case ‘D’: display the current record, then break. • Case ‘P’: display 10 records from the current indicator, and set indicator at the beginning of the 10 th record. ncur = ncur+9. • Case ‘B’: display 10 record backward, and set the indicator at the beginning of the last record. Break; ncur = ncur – 9. • Case ‘N’: input (from stdin) a string, and search in the name of all records for that string. Break; ncur move to the first found record. • Case ‘I’: input a string, and search it in the idno of all records. Ncur = first found • Case ‘C’: display current record, and prompt for input of new t 1, t 2, and t 3, then redo average, rewrite this record in fp. Break; • Default: 顯示輸入錯誤選擇, break; 7. 重覆 3 -4. 用 while(1) 將步驟 1 -6 括起來.
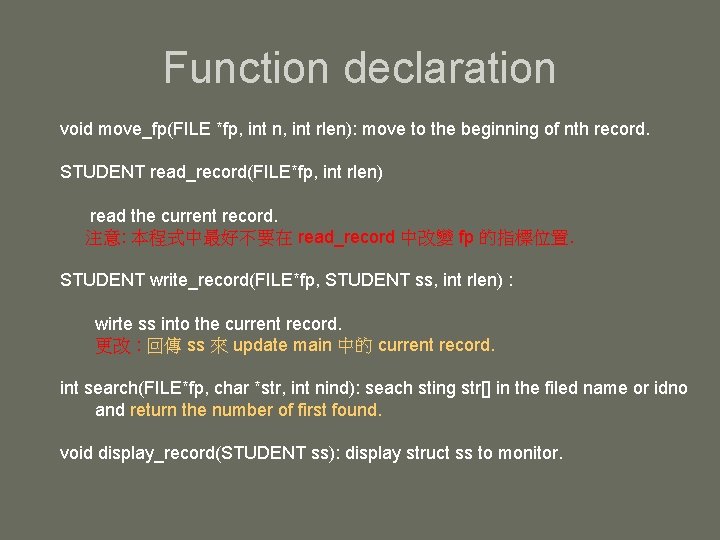
Function declaration void move_fp(FILE *fp, int n, int rlen): move to the beginning of nth record. STUDENT read_record(FILE*fp, int rlen) read the current record. 注意: 本程式中最好不要在 read_record 中改變 fp 的指標位置. STUDENT write_record(FILE*fp, STUDENT ss, int rlen) : wirte ss into the current record. 更改 : 回傳 ss 來 update main 中的 current record. int search(FILE*fp, char *str, int nind): seach sting str[] in the filed name or idno and return the number of first found. void display_record(STUDENT ss): display struct ss to monitor.
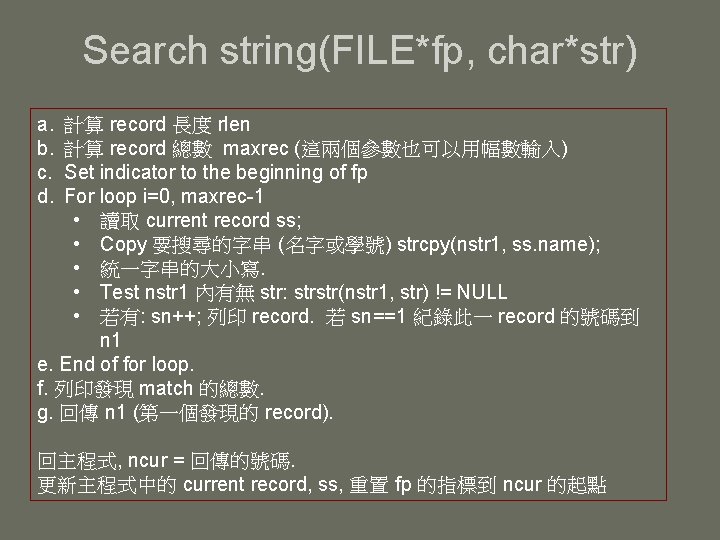
Search string(FILE*fp, char*str) a. b. c. d. 計算 record 長度 rlen 計算 record 總數 maxrec (這兩個參數也可以用幅數輸入) Set indicator to the beginning of fp For loop i=0, maxrec-1 • 讀取 current record ss; • Copy 要搜尋的字串 (名字或學號) strcpy(nstr 1, ss. name); • 統一字串的大小寫. • Test nstr 1 內有無 str: strstr(nstr 1, str) != NULL • 若有: sn++; 列印 record. 若 sn==1 紀錄此一 record 的號碼到 n 1 e. End of for loop. f. 列印發現 match 的總數. g. 回傳 n 1 (第一個發現的 record). 回主程式, ncur = 回傳的號碼. 更新主程式中的 current record, ss, 重置 fp 的指標到 ncur 的起點
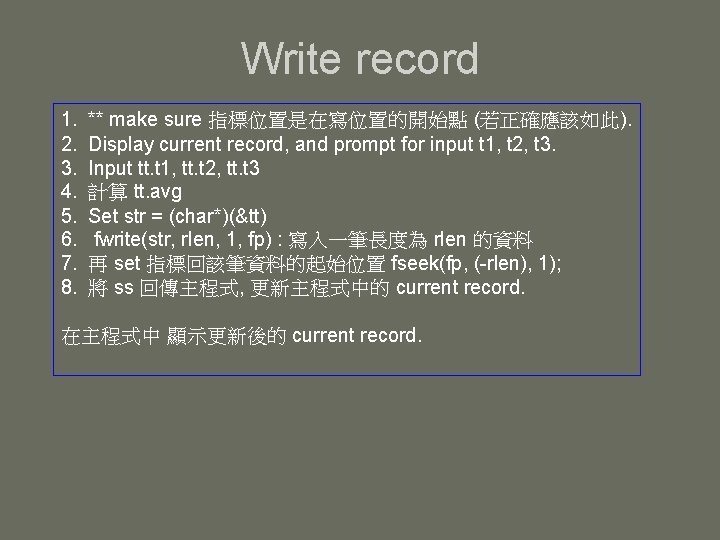
Write record 1. 2. 3. 4. 5. 6. 7. 8. ** make sure 指標位置是在寫位置的開始點 (若正確應該如此). Display current record, and prompt for input t 1, t 2, t 3. Input tt. t 1, tt. t 2, tt. t 3 計算 tt. avg Set str = (char*)(&tt) fwrite(str, rlen, 1, fp) : 寫入一筆長度為 rlen 的資料 再 set 指標回該筆資料的起始位置 fseek(fp, (-rlen), 1); 將 ss 回傳主程式, 更新主程式中的 current record. 在主程式中 顯示更新後的 current record.
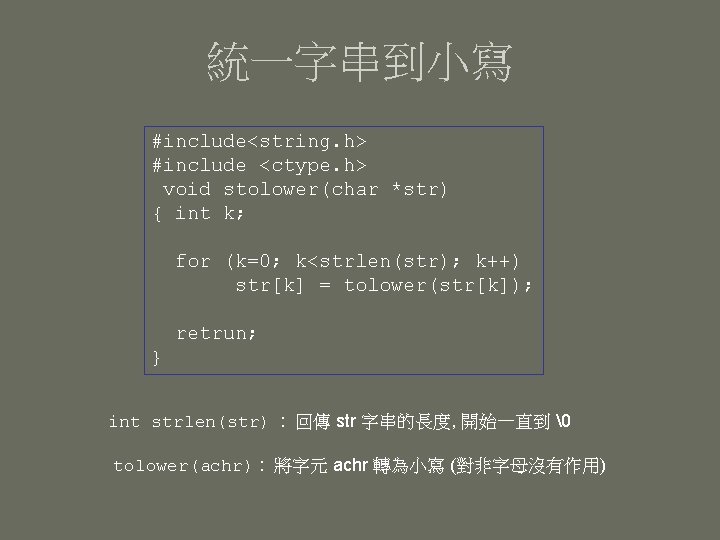
統一字串到小寫 #include<string. h> #include <ctype. h> void stolower(char *str) { int k; for (k=0; k<strlen(str); k++) str[k] = tolower(str[k]); retrun; } int strlen(str) : 回傳 str 字串的長度, 開始一直到 tolower(achr) : 將字元 achr 轉為小寫 (對非字母沒有作用)
#include stdio.h #include stdlib.h #include string.h
#include stdio.h #include stdlib.h int main()
Licenseid=string&content=string&/paramsxml=string
C++ include iostream
#include iostream using namespace std
Template
#include string
#include stdio.h #include conio.h #include stdlib.h
Const int size =18 string *tbl2
Public class person string name
String[::-1]
Difference between string and character
#include "stdafx.h"