Streams and IO Streams are pipe like constructors
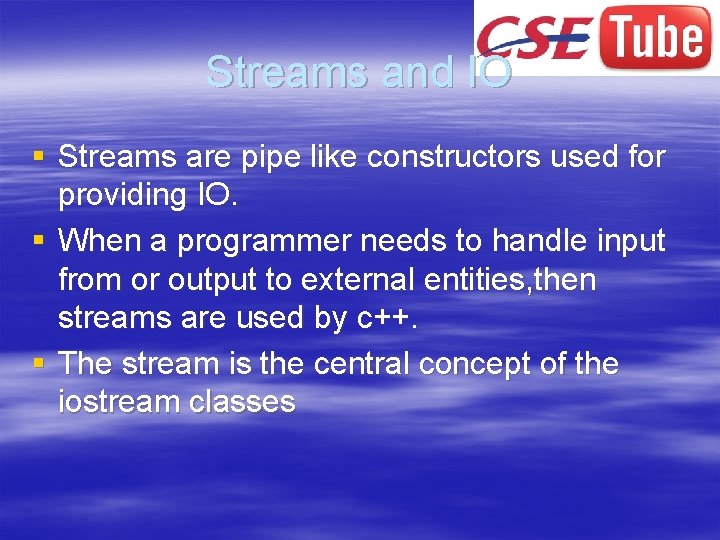
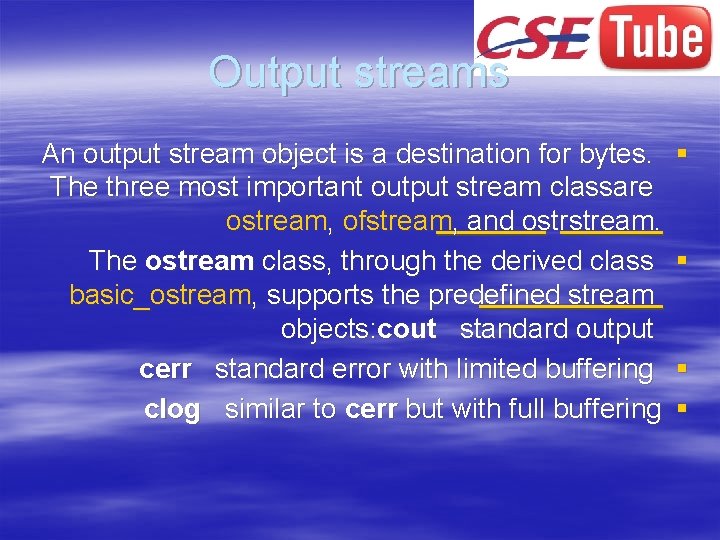
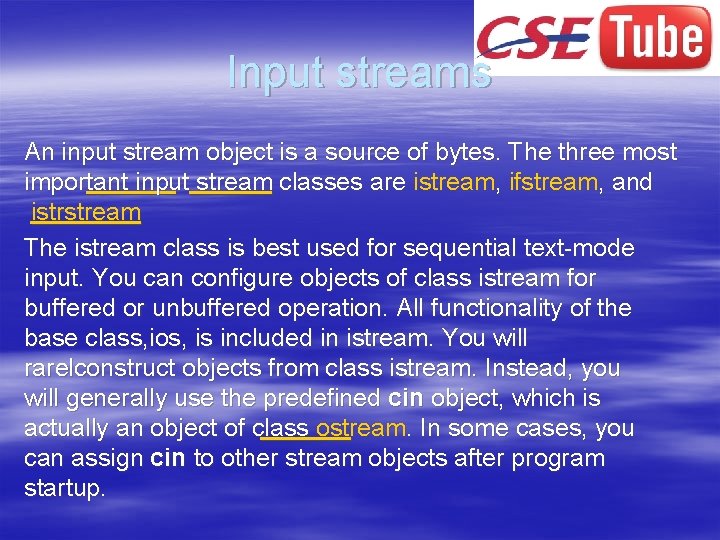
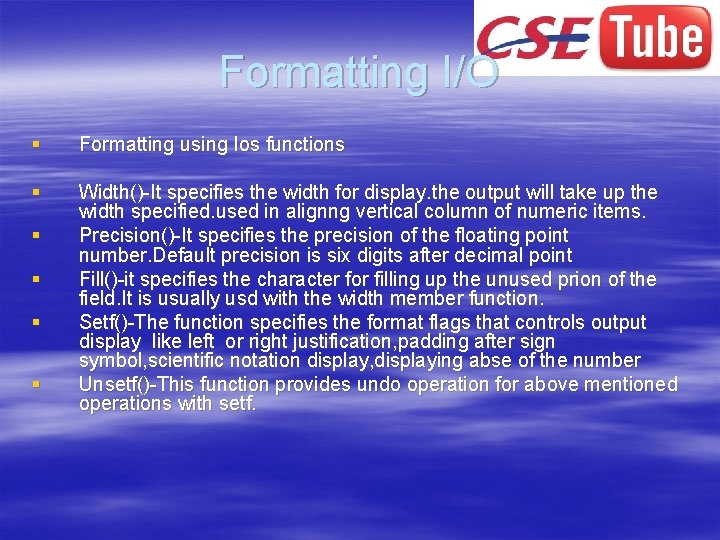
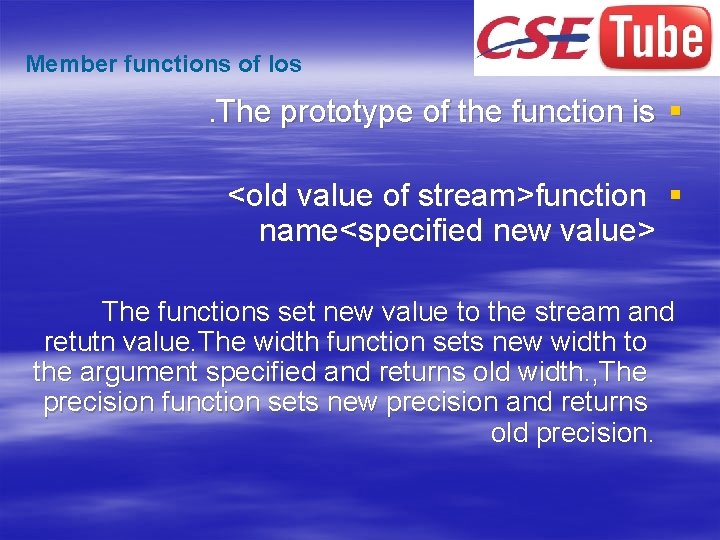
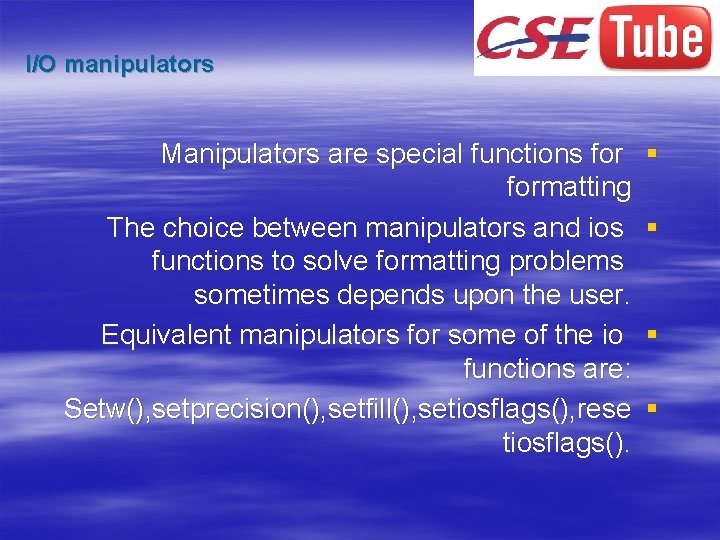
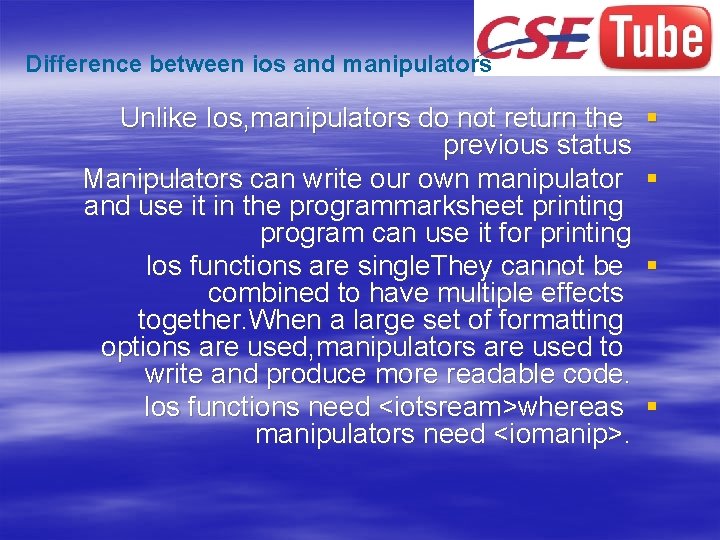
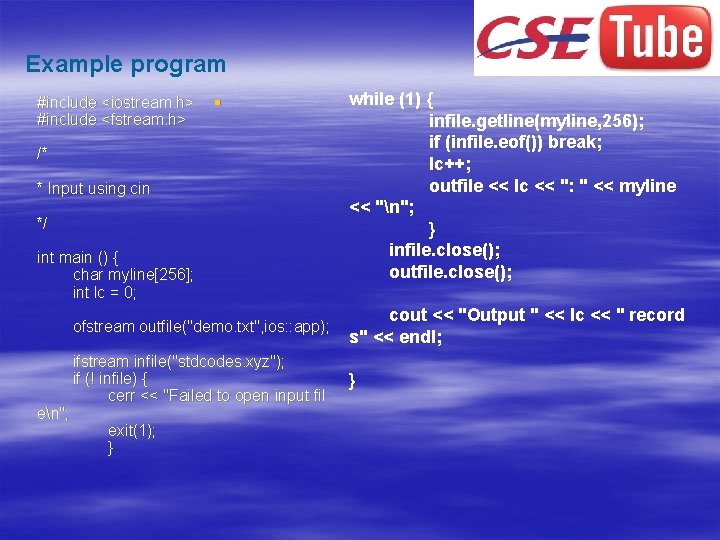
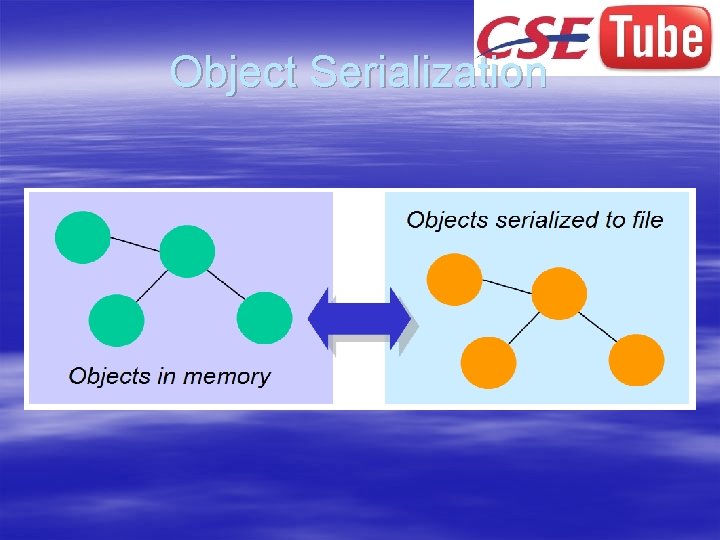
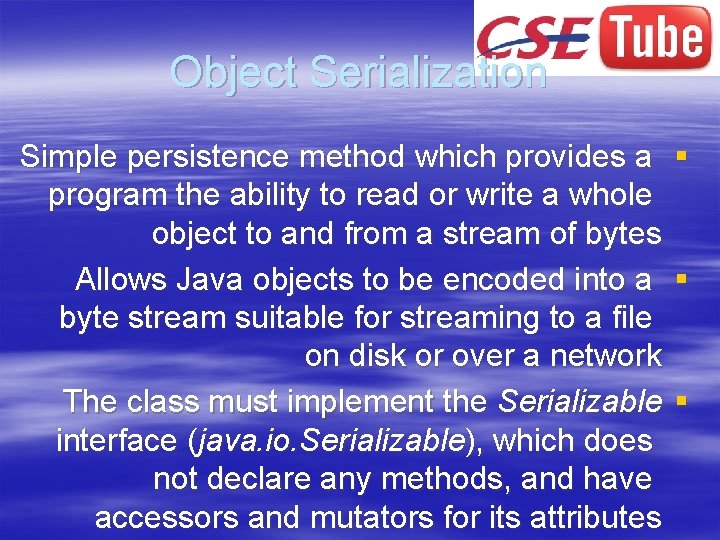
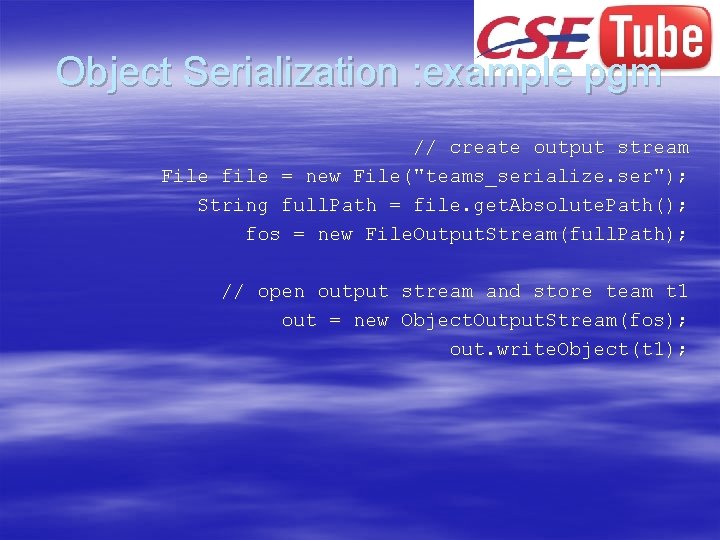
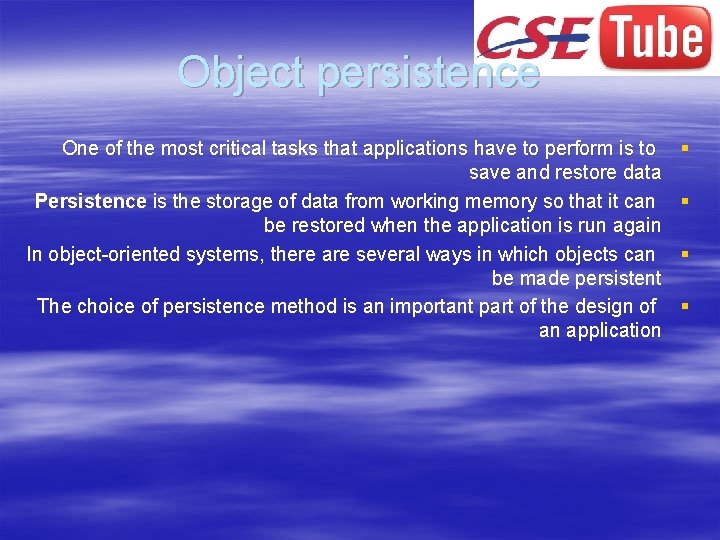
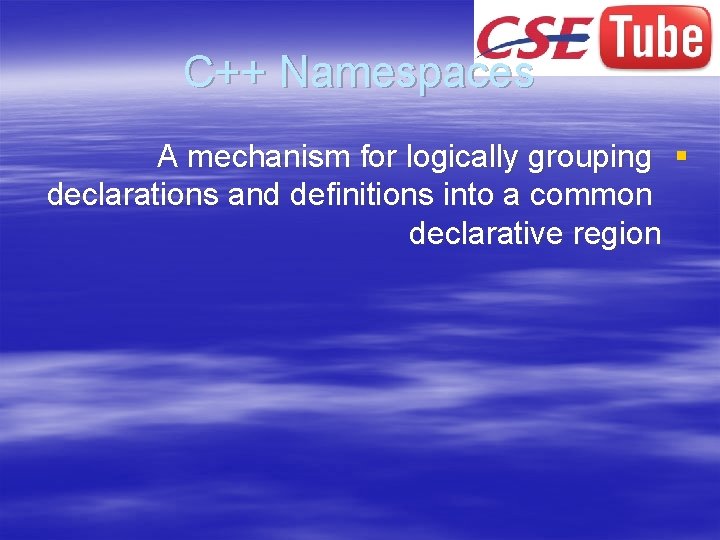
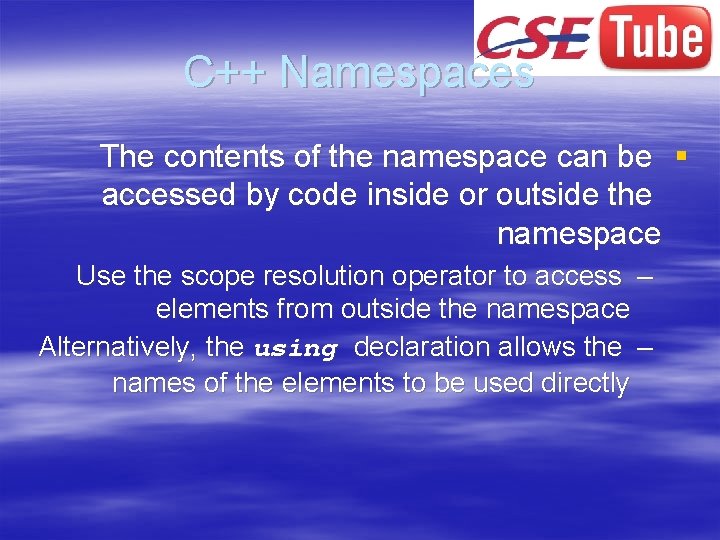
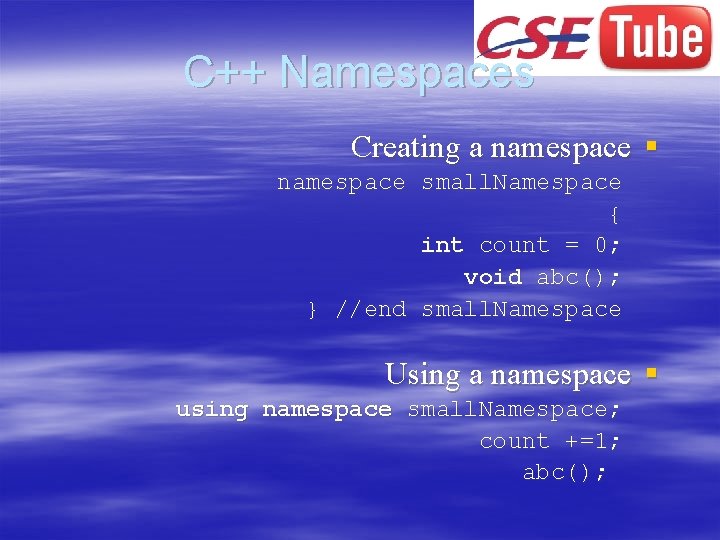
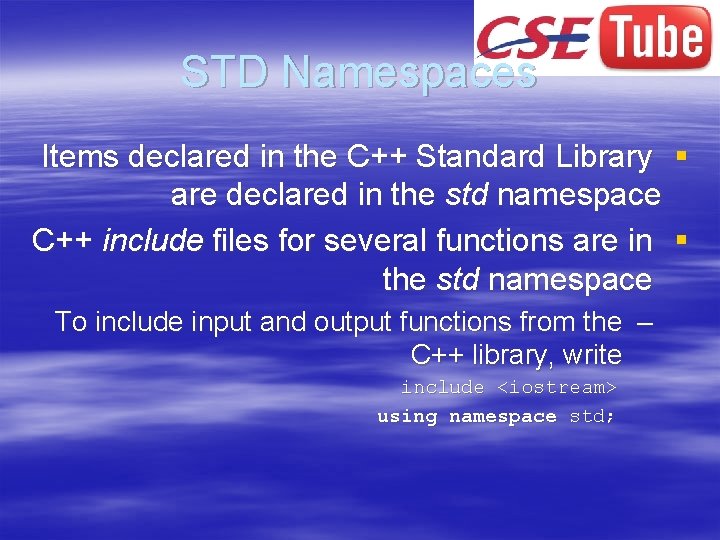
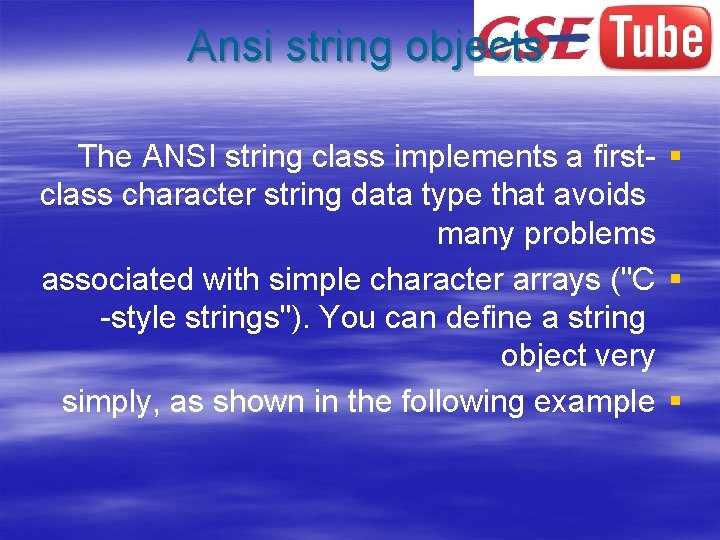
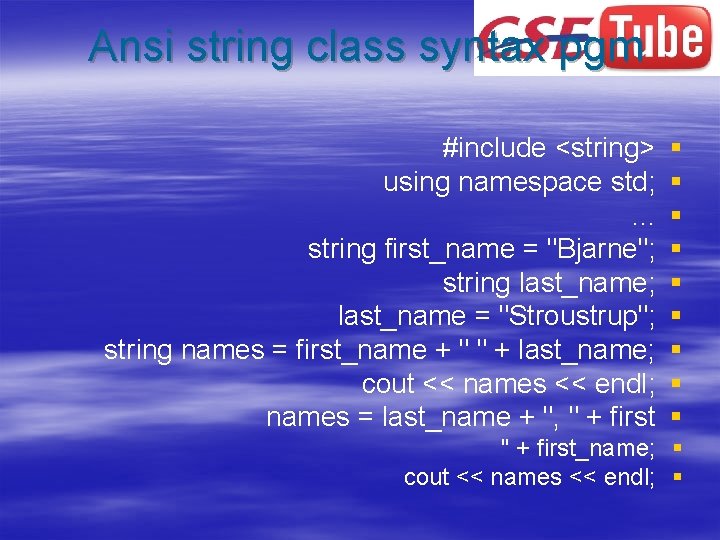
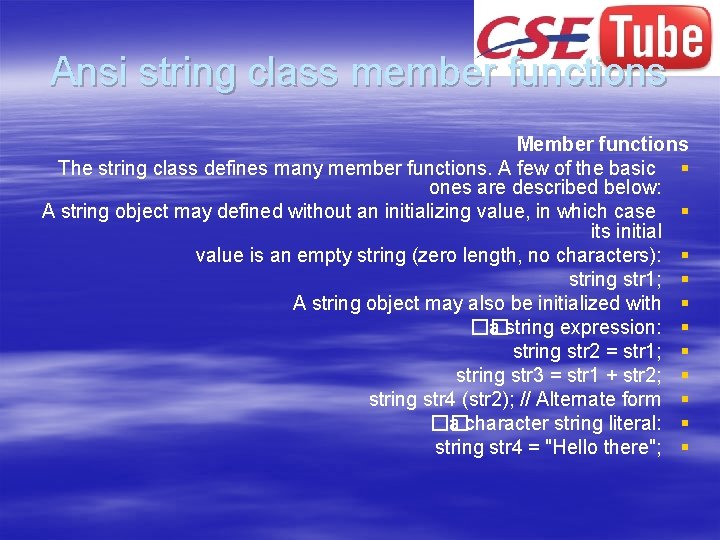
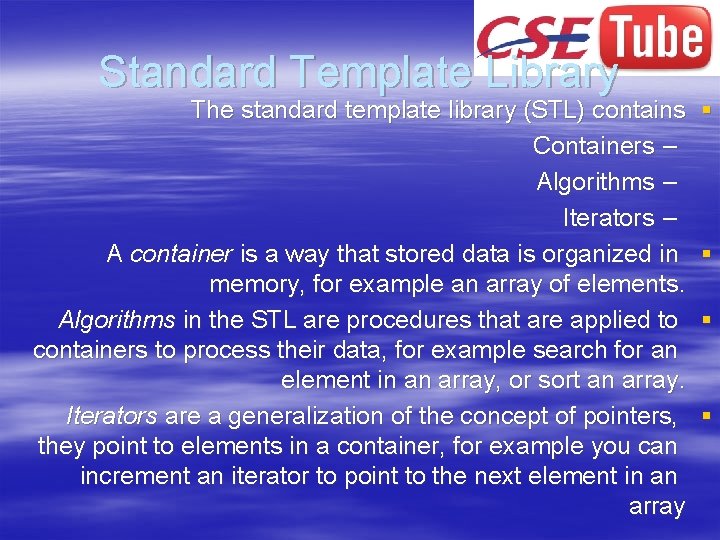
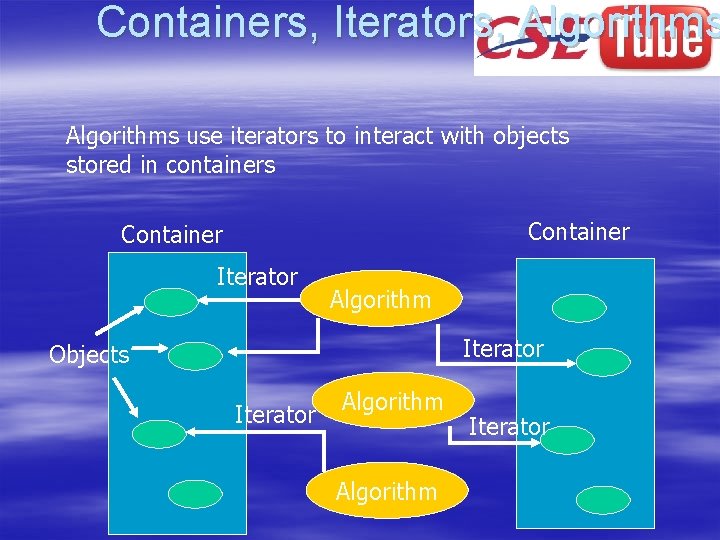
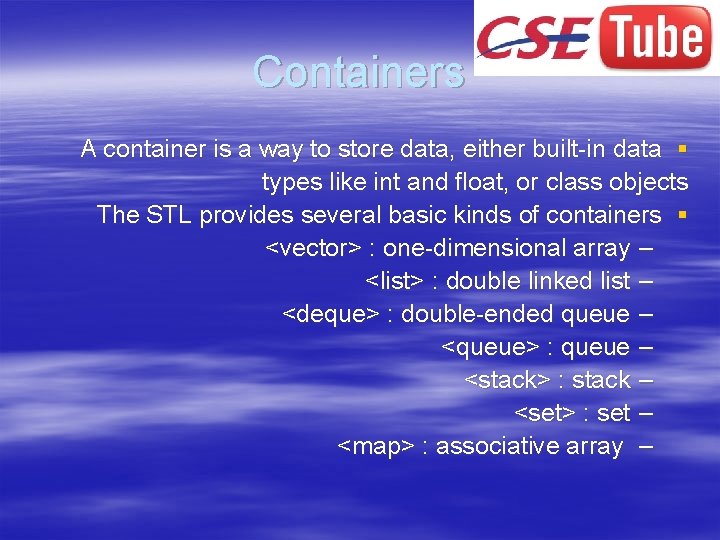
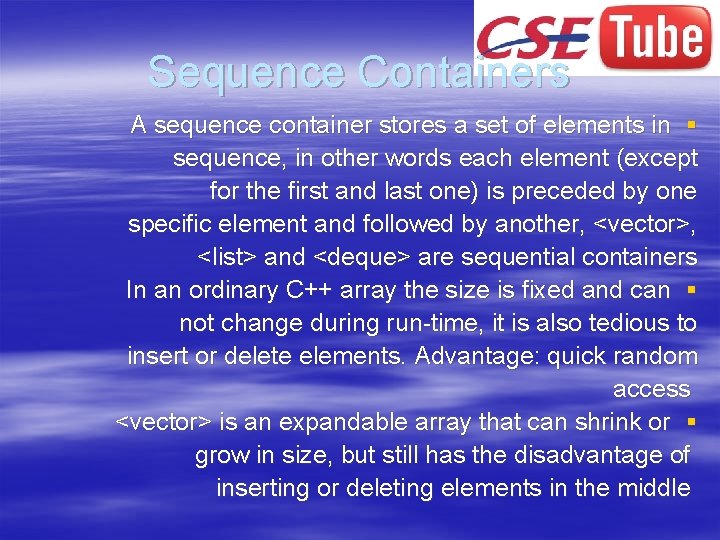
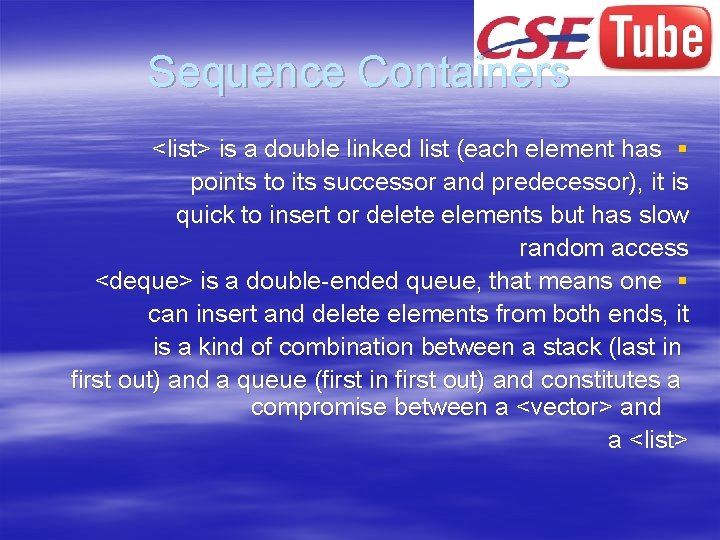
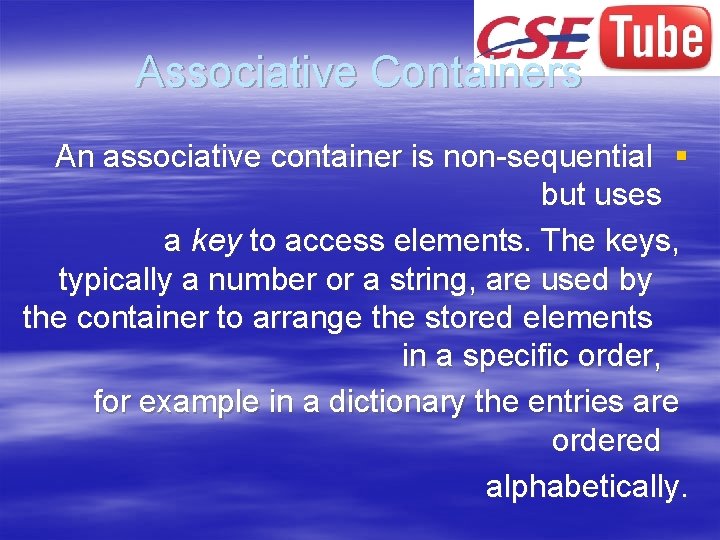
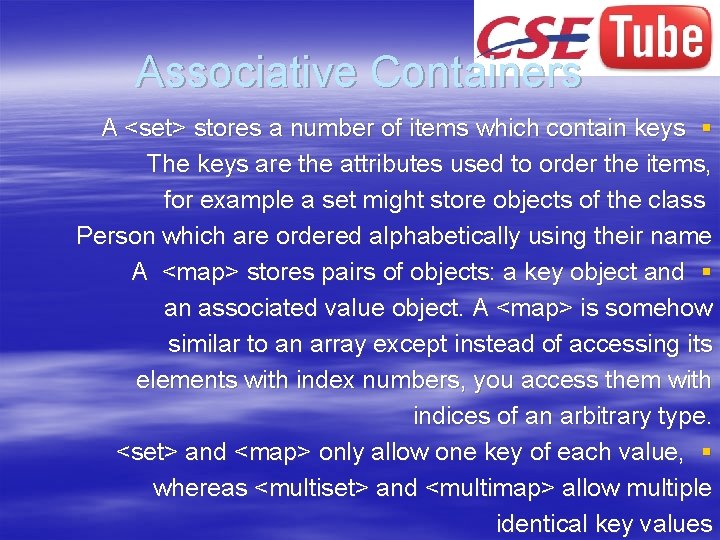
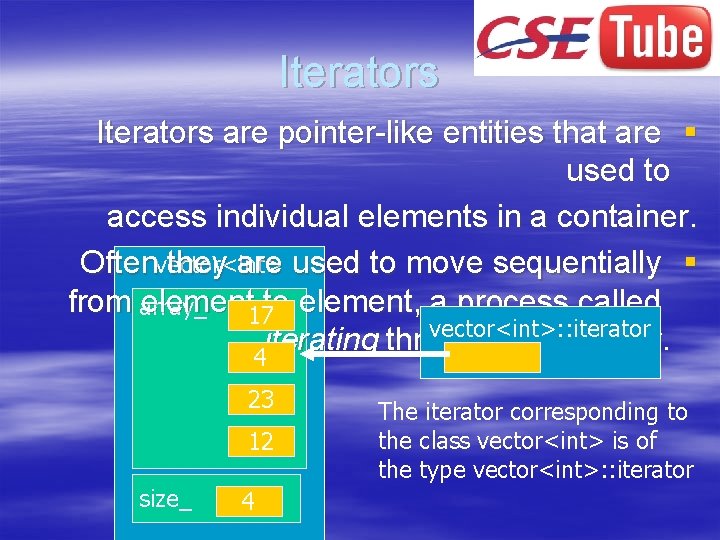
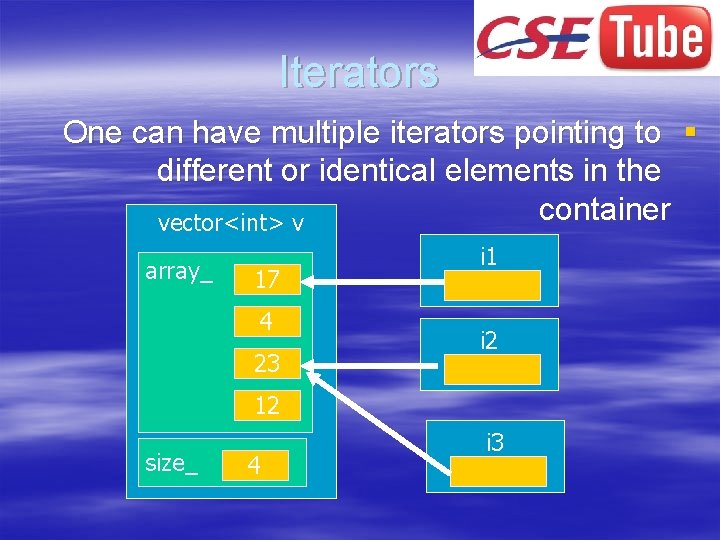
![Iterators #include <vector> #include <iostream> int arr[] = { 12, 3, 17, 8 }; Iterators #include <vector> #include <iostream> int arr[] = { 12, 3, 17, 8 };](https://slidetodoc.com/presentation_image_h/37a4fe6b882dd25e1b4a69c096a99eb4/image-29.jpg)
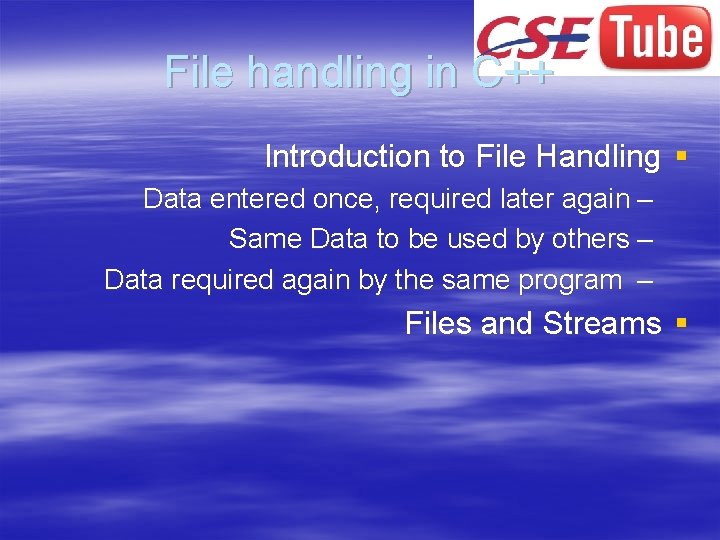
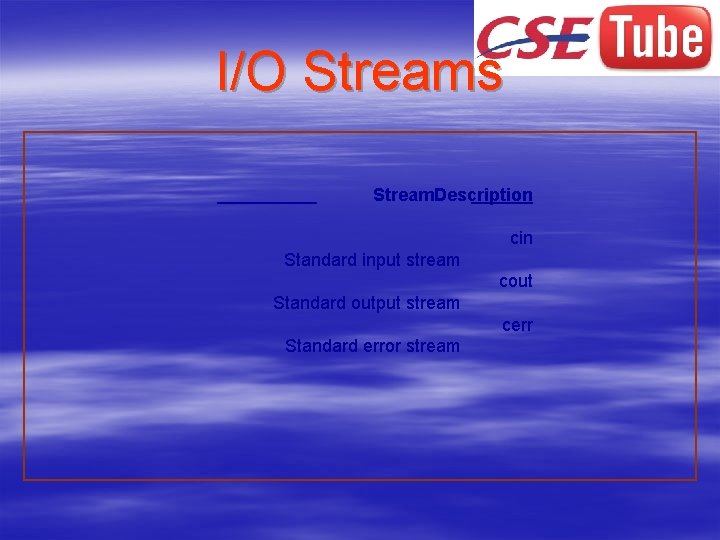
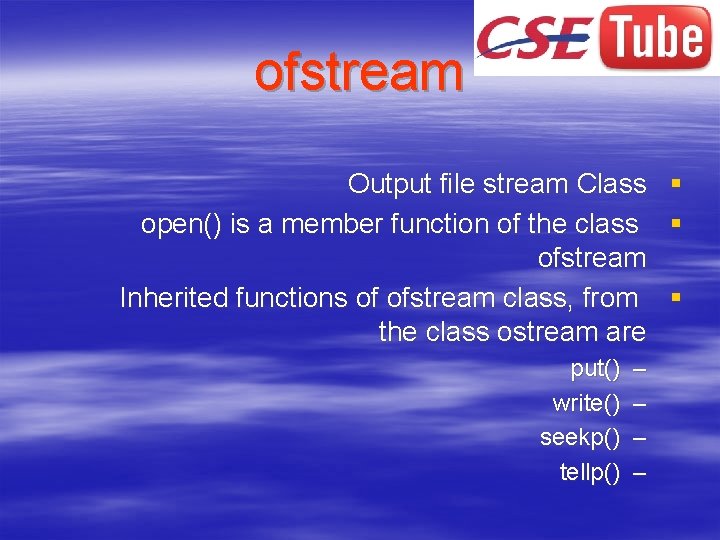
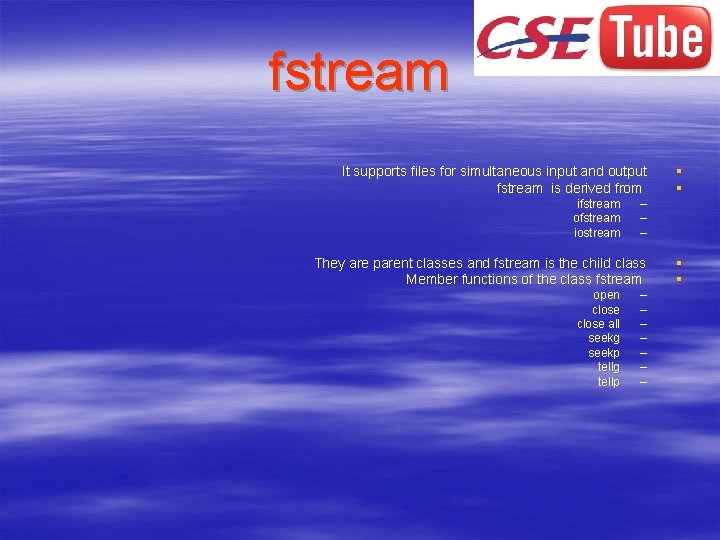
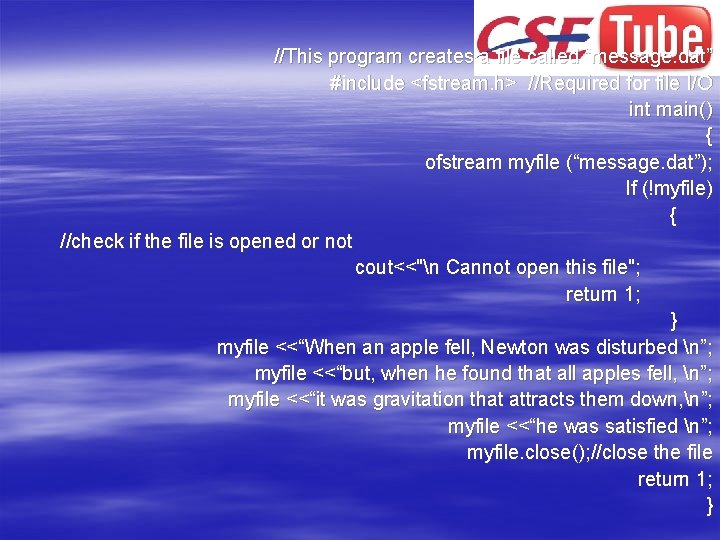
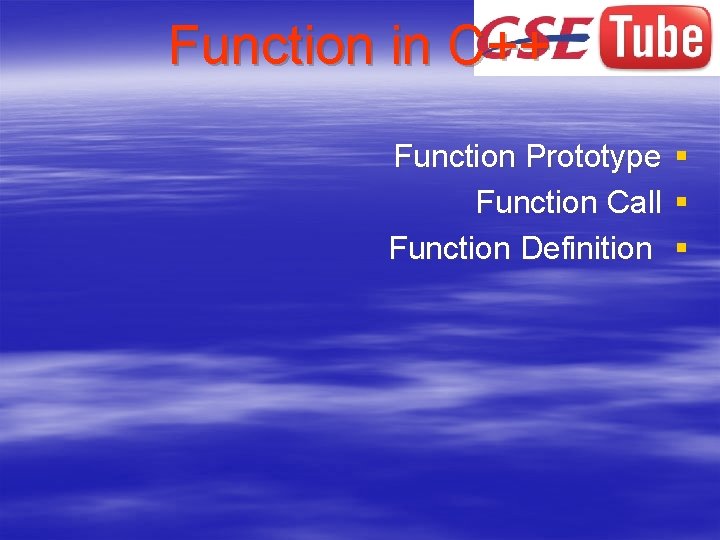
- Slides: 35
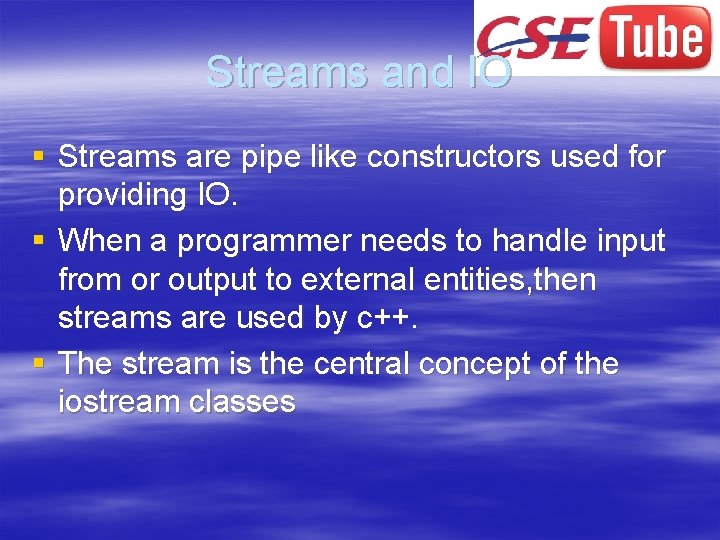
Streams and IO § Streams are pipe like constructors used for providing IO. § When a programmer needs to handle input from or output to external entities, then streams are used by c++. § The stream is the central concept of the iostream classes
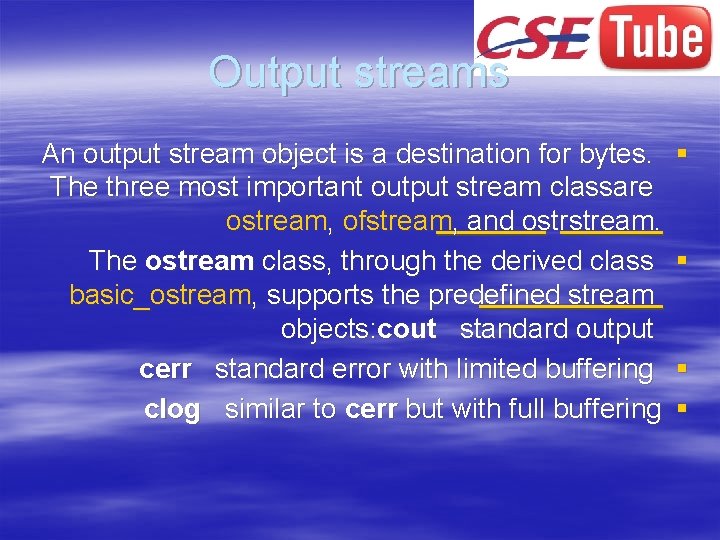
Output streams An output stream object is a destination for bytes. The three most important output stream classare ostream, ofstream, and ostrstream. The ostream class, through the derived class basic_ostream, supports the predefined stream objects: cout standard output cerr standard error with limited buffering clog similar to cerr but with full buffering § §
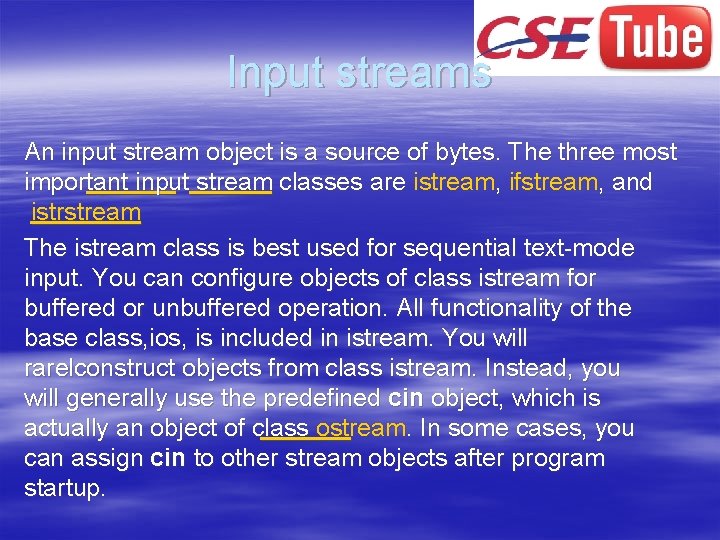
Input streams An input stream object is a source of bytes. The three most important input stream classes are istream, ifstream, and istrstream The istream class is best used for sequential text-mode input. You can configure objects of class istream for buffered or unbuffered operation. All functionality of the base class, ios, is included in istream. You will rarelconstruct objects from class istream. Instead, you will generally use the predefined cin object, which is actually an object of class ostream. In some cases, you can assign cin to other stream objects after program startup.
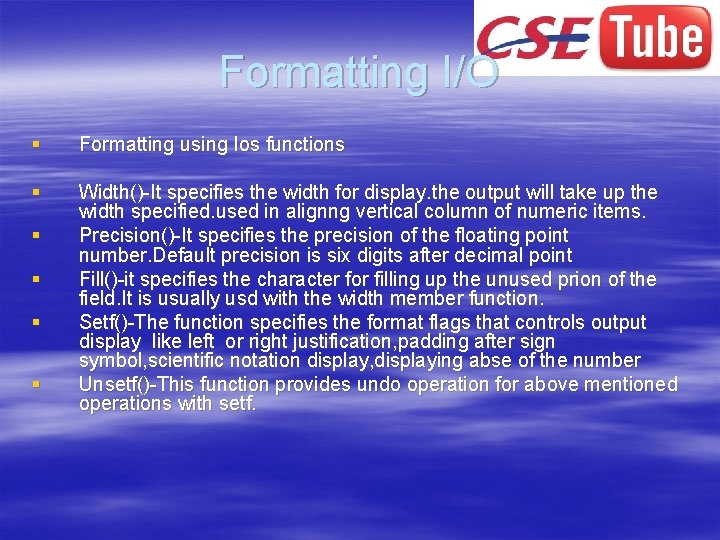
Formatting I/O § Formatting using Ios functions § Width()-It specifies the width for display. the output will take up the width specified. used in alignng vertical column of numeric items. Precision()-It specifies the precision of the floating point number. Default precision is six digits after decimal point Fill()-it specifies the character for filling up the unused prion of the field. It is usually usd with the width member function. Setf()-The function specifies the format flags that controls output display like left or right justification, padding after sign symbol, scientific notation display, displaying abse of the number Unsetf()-This function provides undo operation for above mentioned operations with setf. § §
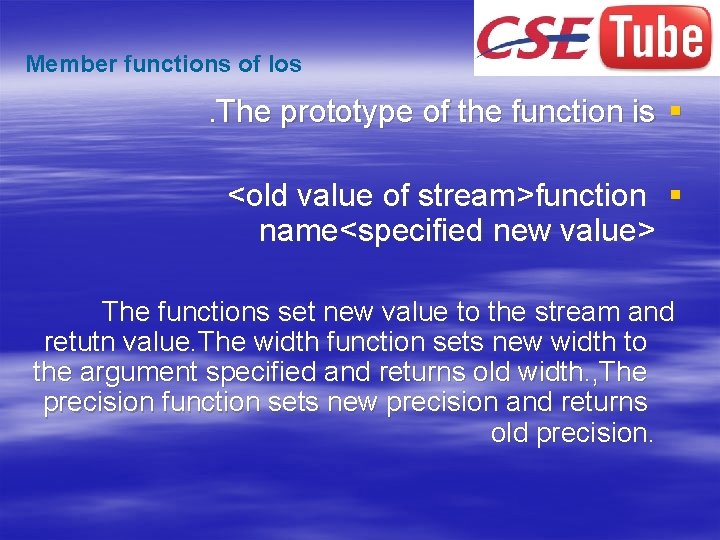
Member functions of Ios . The prototype of the function is § <old value of stream>function § name<specified new value> The functions set new value to the stream and retutn value. The width function sets new width to the argument specified and returns old width. , The precision function sets new precision and returns old precision.
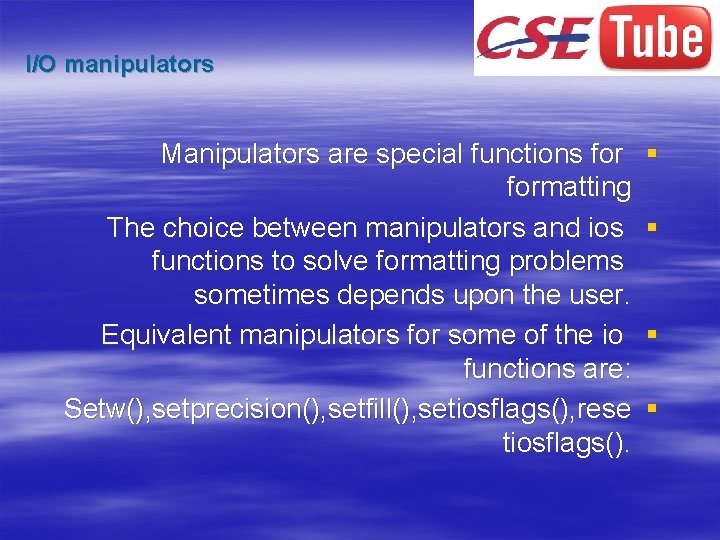
I/O manipulators Manipulators are special functions formatting The choice between manipulators and ios functions to solve formatting problems sometimes depends upon the user. Equivalent manipulators for some of the io functions are: Setw(), setprecision(), setfill(), setiosflags(), rese tiosflags(). § §
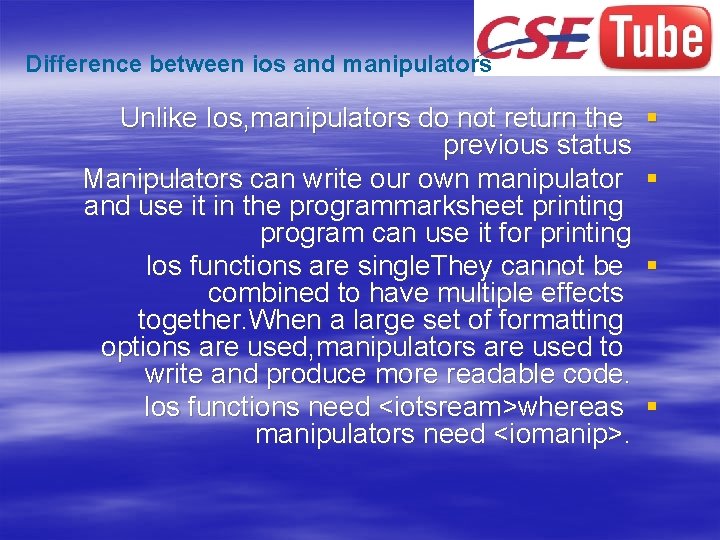
Difference between ios and manipulators Unlike Ios, manipulators do not return the previous status Manipulators can write our own manipulator and use it in the programmarksheet printing program can use it for printing Ios functions are single. They cannot be combined to have multiple effects together. When a large set of formatting options are used, manipulators are used to write and produce more readable code. Ios functions need <iotsream>whereas manipulators need <iomanip>. § §
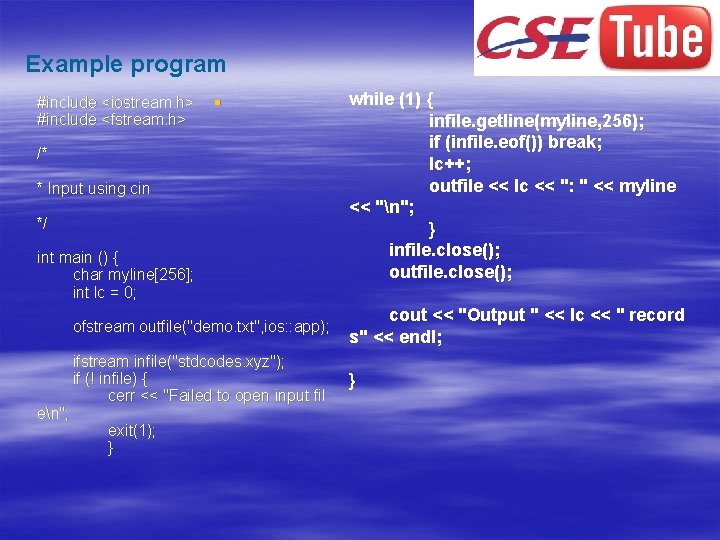
Example program #include <iostream. h> #include <fstream. h> § /* * Input using cin */ int main () { char myline[256]; int lc = 0; ofstream outfile("demo. txt", ios: : app); ifstream infile("stdcodes. xyz"); if (! infile) { cerr << "Failed to open input fil en"; exit(1); } while (1) { infile. getline(myline, 256); if (infile. eof()) break; lc++; outfile << lc << ": " << myline << "n"; } infile. close(); outfile. close(); cout << "Output " << lc << " record s" << endl; }
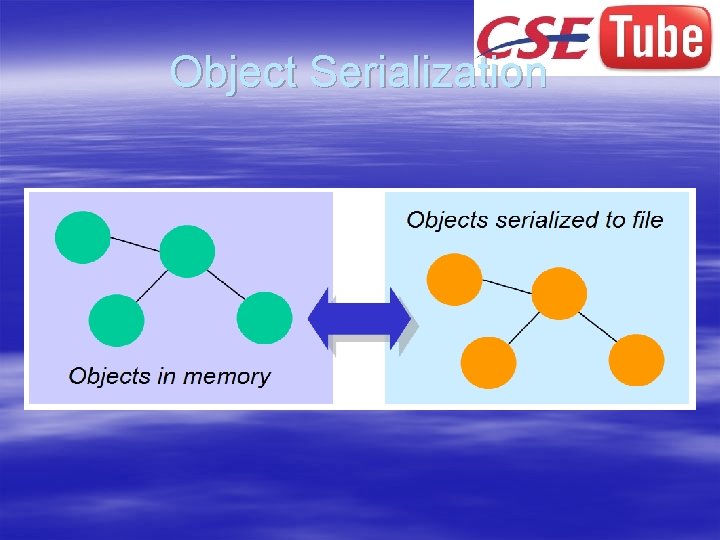
Object Serialization
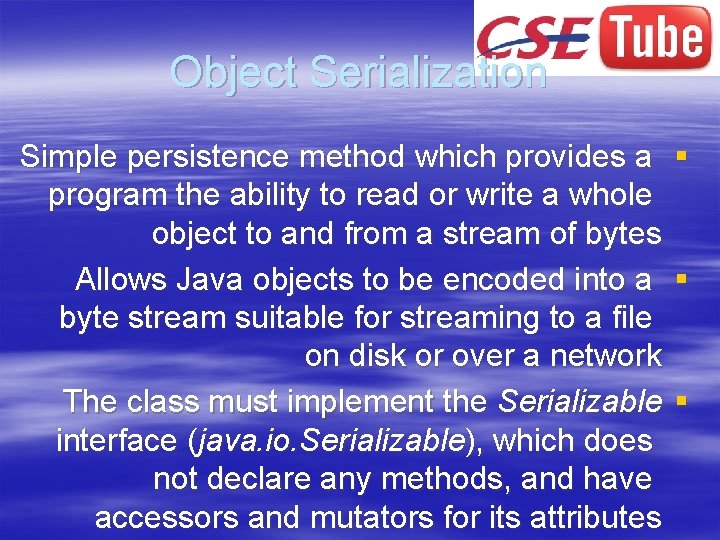
Object Serialization Simple persistence method which provides a § program the ability to read or write a whole object to and from a stream of bytes Allows Java objects to be encoded into a § byte stream suitable for streaming to a file on disk or over a network The class must implement the Serializable § interface (java. io. Serializable), which does not declare any methods, and have accessors and mutators for its attributes
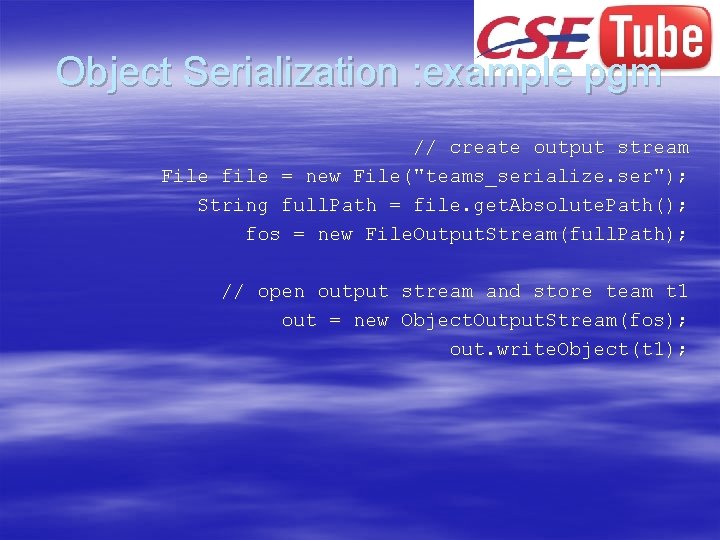
Object Serialization : example pgm // create output stream File file = new File("teams_serialize. ser"); String full. Path = file. get. Absolute. Path(); fos = new File. Output. Stream(full. Path); // open output stream and store team t 1 out = new Object. Output. Stream(fos); out. write. Object(t 1);
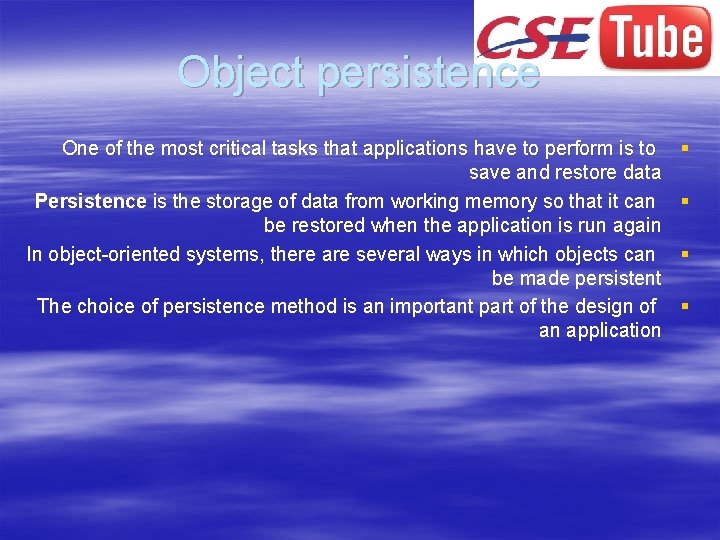
Object persistence One of the most critical tasks that applications have to perform is to save and restore data Persistence is the storage of data from working memory so that it can be restored when the application is run again In object-oriented systems, there are several ways in which objects can be made persistent The choice of persistence method is an important part of the design of an application § §
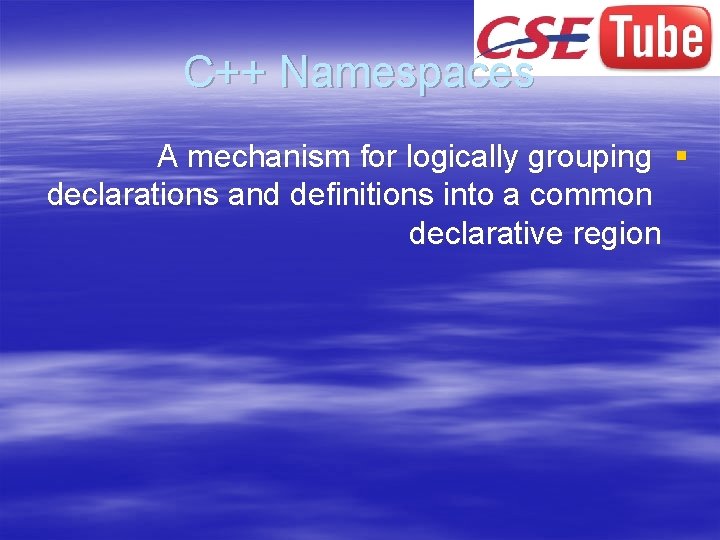
C++ Namespaces A mechanism for logically grouping § declarations and definitions into a common declarative region
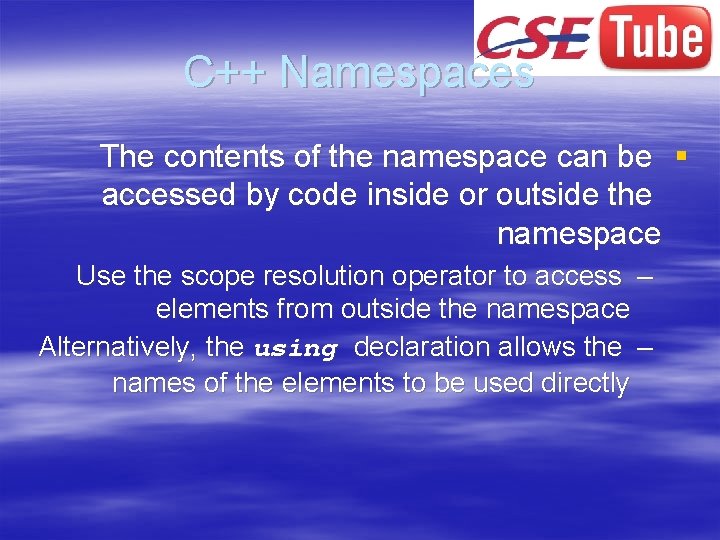
C++ Namespaces The contents of the namespace can be § accessed by code inside or outside the namespace Use the scope resolution operator to access – elements from outside the namespace Alternatively, the using declaration allows the – names of the elements to be used directly
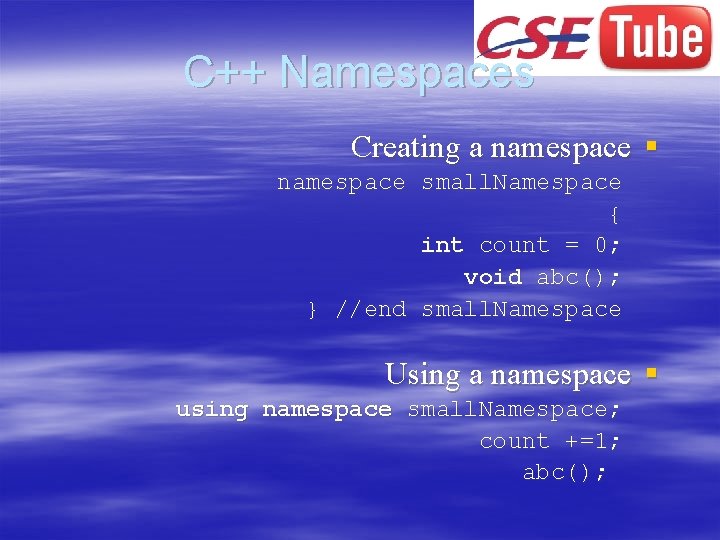
C++ Namespaces Creating a namespace § namespace small. Namespace { int count = 0; void abc(); } //end small. Namespace Using a namespace § using namespace small. Namespace; count +=1; abc();
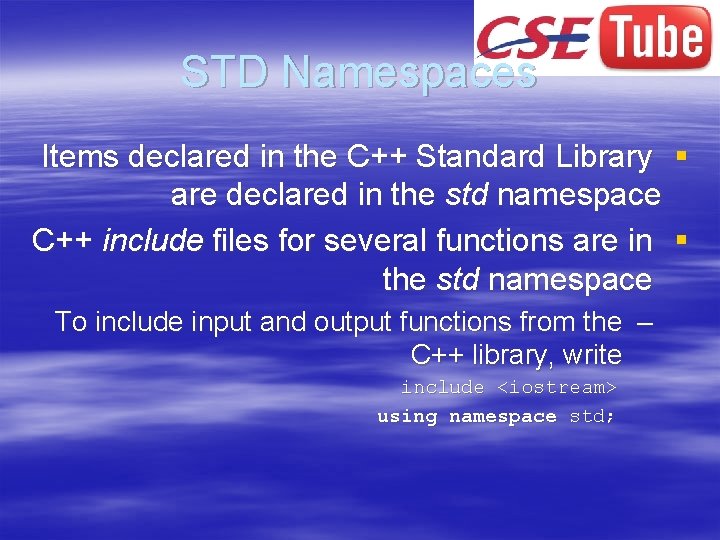
STD Namespaces Items declared in the C++ Standard Library § are declared in the std namespace C++ include files for several functions are in § the std namespace To include input and output functions from the – C++ library, write include <iostream> using namespace std;
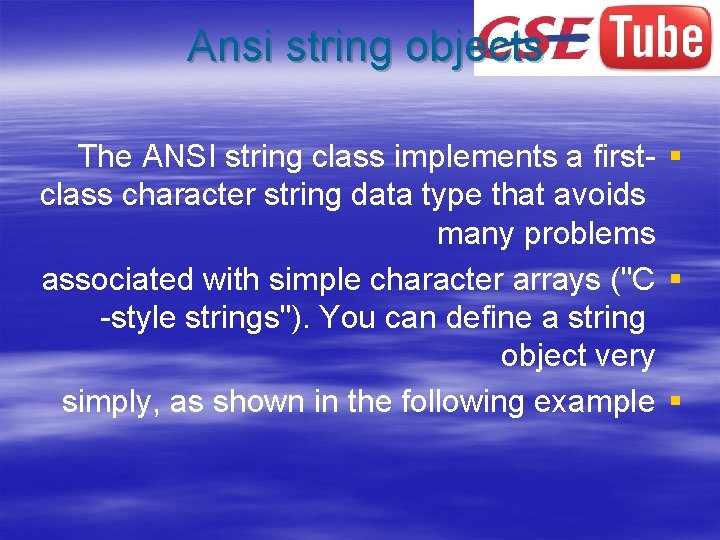
Ansi string objects The ANSI string class implements a first- § class character string data type that avoids many problems associated with simple character arrays ("C § -style strings"). You can define a string object very simply, as shown in the following example §
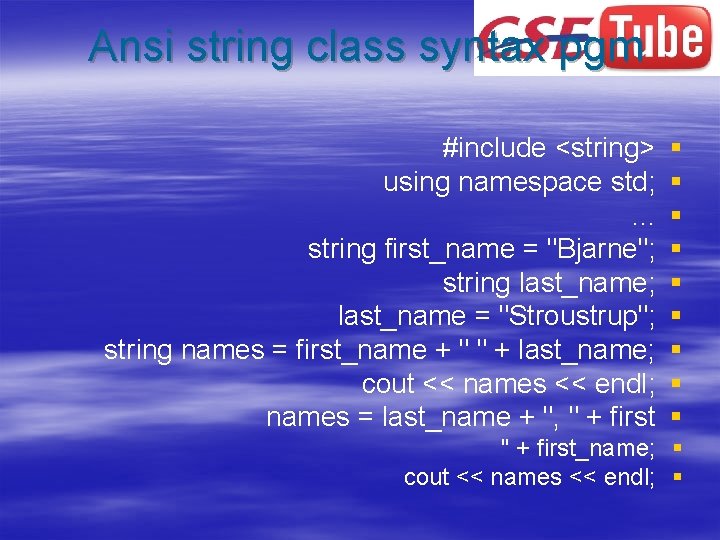
Ansi string class syntax pgm #include <string> using namespace std; . . . string first_name = "Bjarne"; string last_name; last_name = "Stroustrup"; string names = first_name + " " + last_name; cout << names << endl; names = last_name + ", " + first § § § § § " + first_name; § cout << names << endl; §
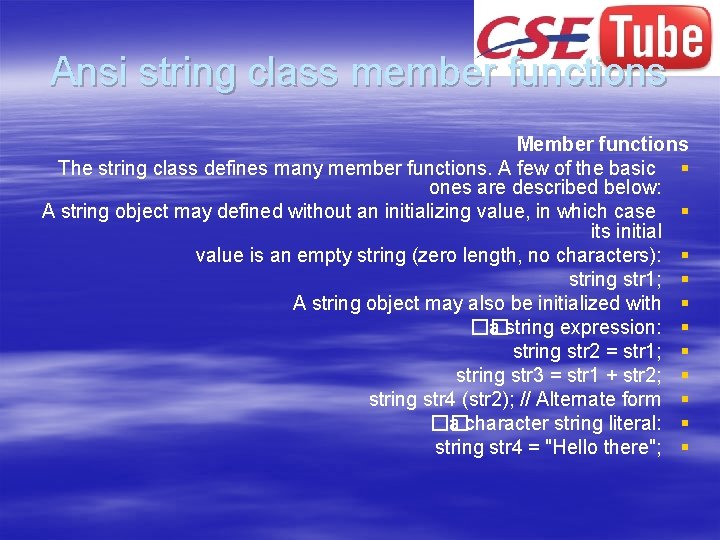
Ansi string class member functions Member functions The string class defines many member functions. A few of the basic § ones are described below: A string object may defined without an initializing value, in which case § its initial value is an empty string (zero length, no characters): § string str 1; § A string object may also be initialized with § �� a string expression: § string str 2 = str 1; § string str 3 = str 1 + str 2; § string str 4 (str 2); // Alternate form § �� a character string literal: § string str 4 = "Hello there"; §
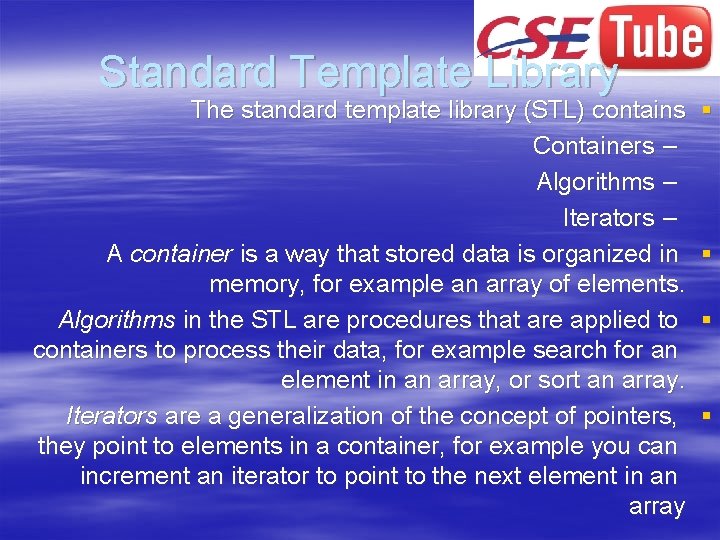
Standard Template Library The standard template library (STL) contains Containers – Algorithms – Iterators – A container is a way that stored data is organized in memory, for example an array of elements. Algorithms in the STL are procedures that are applied to containers to process their data, for example search for an element in an array, or sort an array. Iterators are a generalization of the concept of pointers, they point to elements in a container, for example you can increment an iterator to point to the next element in an array § §
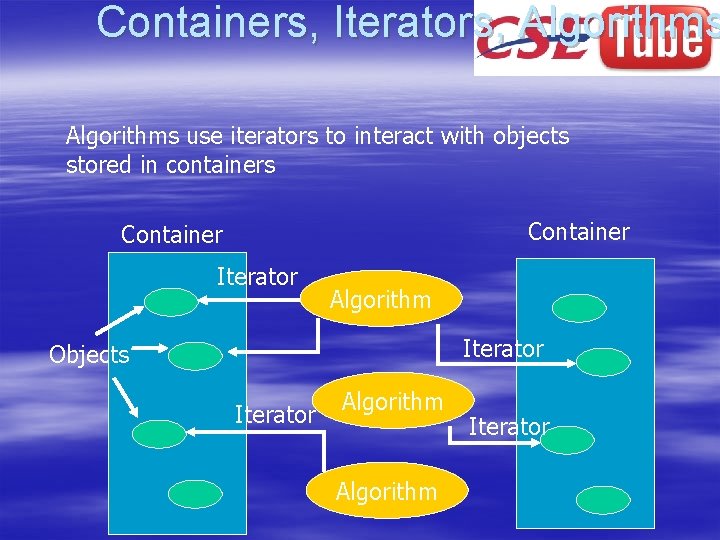
Containers, Iterators, Algorithms use iterators to interact with objects stored in containers Container Iterator Algorithm Iterator Objects Iterator Algorithm Iterator
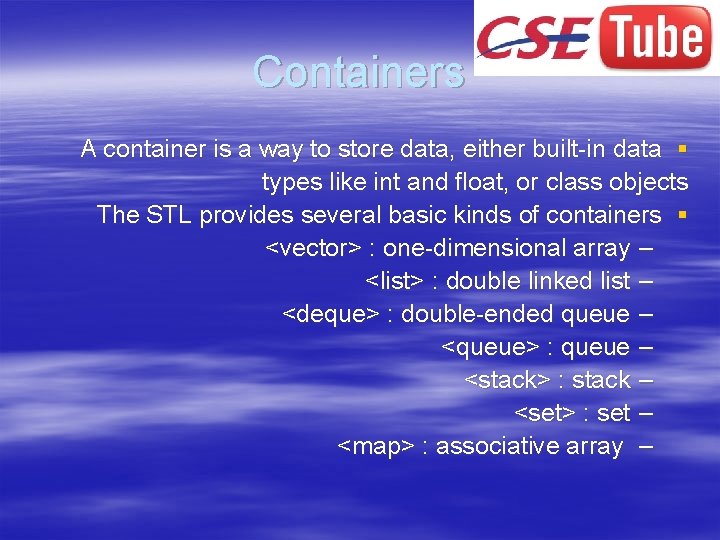
Containers A container is a way to store data, either built-in data § types like int and float, or class objects The STL provides several basic kinds of containers § <vector> : one-dimensional array – <list> : double linked list – <deque> : double-ended queue – <queue> : queue – <stack> : stack – <set> : set – <map> : associative array –
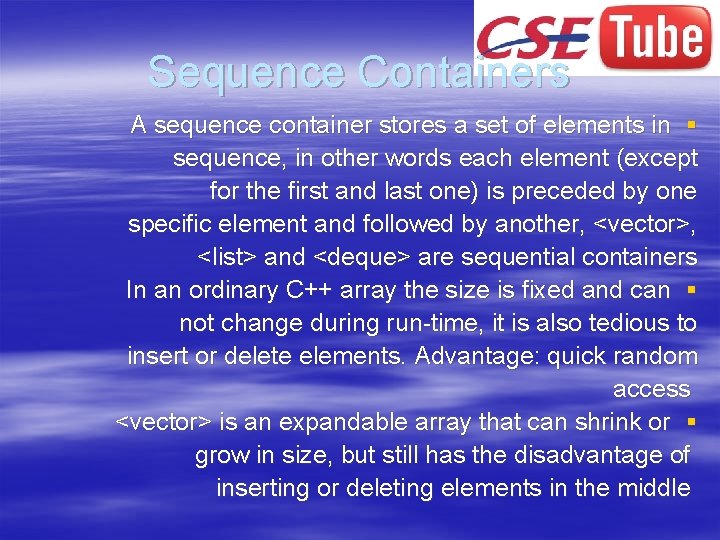
Sequence Containers A sequence container stores a set of elements in § sequence, in other words each element (except for the first and last one) is preceded by one specific element and followed by another, <vector>, <list> and <deque> are sequential containers In an ordinary C++ array the size is fixed and can § not change during run-time, it is also tedious to insert or delete elements. Advantage: quick random access <vector> is an expandable array that can shrink or § grow in size, but still has the disadvantage of inserting or deleting elements in the middle
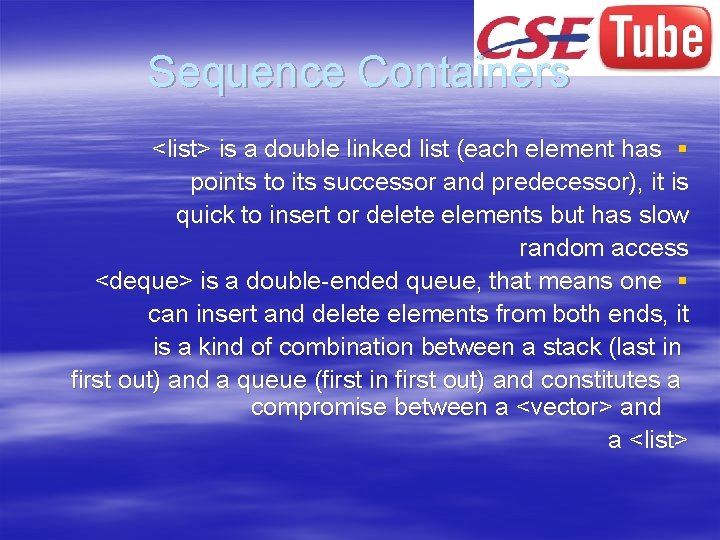
Sequence Containers <list> is a double linked list (each element has § points to its successor and predecessor), it is quick to insert or delete elements but has slow random access <deque> is a double-ended queue, that means one § can insert and delete elements from both ends, it is a kind of combination between a stack (last in first out) and a queue (first in first out) and constitutes a compromise between a <vector> and a <list>
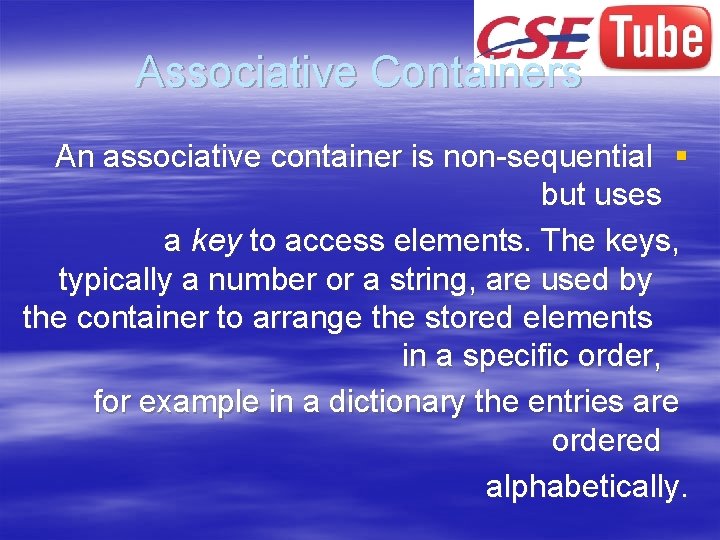
Associative Containers An associative container is non-sequential § but uses a key to access elements. The keys, typically a number or a string, are used by the container to arrange the stored elements in a specific order, for example in a dictionary the entries are ordered alphabetically.
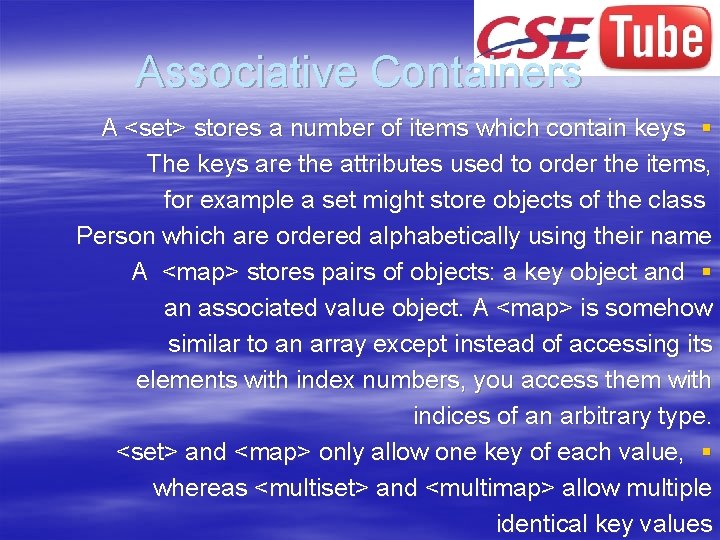
Associative Containers A <set> stores a number of items which contain keys § The keys are the attributes used to order the items, for example a set might store objects of the class Person which are ordered alphabetically using their name A <map> stores pairs of objects: a key object and § an associated value object. A <map> is somehow similar to an array except instead of accessing its elements with index numbers, you access them with indices of an arbitrary type. <set> and <map> only allow one key of each value, § whereas <multiset> and <multimap> allow multiple identical key values
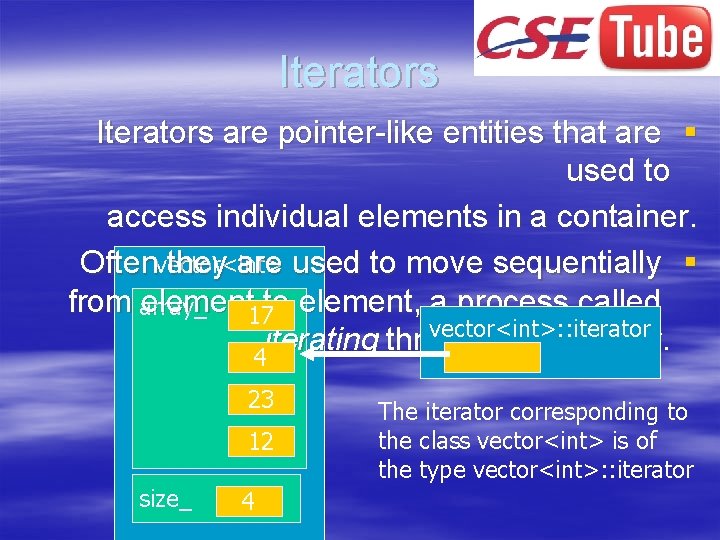
Iterators are pointer-like entities that are § used to access individual elements in a container. vector<int> Often they are used to move sequentially § from element to element, a process called array_ 17 vector<int>: : iterator iterating through a container. 4 23 12 size_ 4 The iterator corresponding to the class vector<int> is of the type vector<int>: : iterator
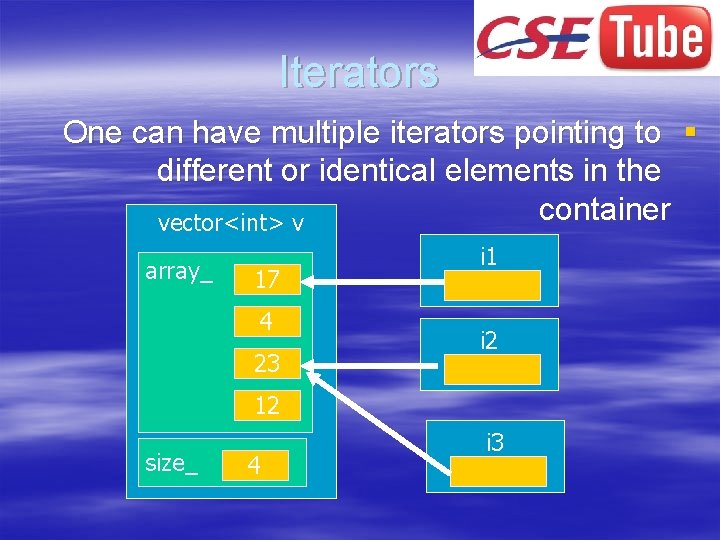
Iterators One can have multiple iterators pointing to § different or identical elements in the container vector<int> v array_ 17 4 23 i 1 i 2 12 size_ 4 i 3
![Iterators include vector include iostream int arr 12 3 17 8 Iterators #include <vector> #include <iostream> int arr[] = { 12, 3, 17, 8 };](https://slidetodoc.com/presentation_image_h/37a4fe6b882dd25e1b4a69c096a99eb4/image-29.jpg)
Iterators #include <vector> #include <iostream> int arr[] = { 12, 3, 17, 8 }; // standard C array vector<int> v(arr, arr+4); // initialize vector with C array for (vector<int>: : iterator i=v. begin(); i!=v. end(); i++) // initialize i with pointer to first element of v // i++ increment iterator, move iterator to next element { cout << *i << ” ”; // de-referencing iterator returns the // value of the element the iterator points at } cout << endl;
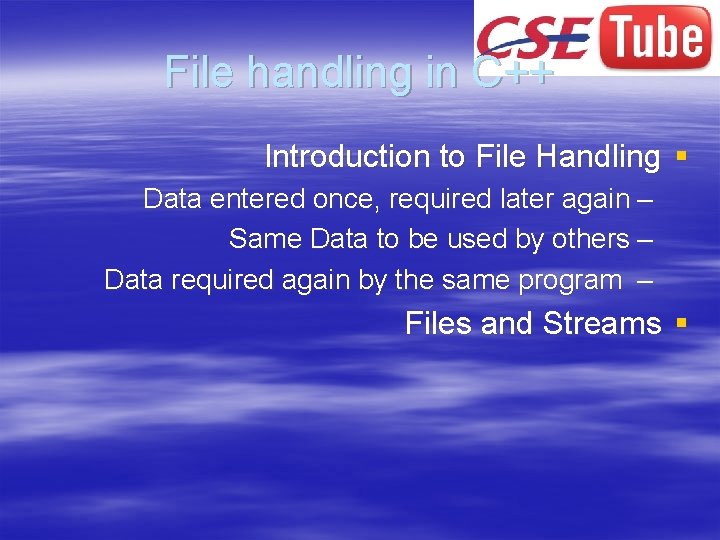
File handling in C++ Introduction to File Handling § Data entered once, required later again – Same Data to be used by others – Data required again by the same program – Files and Streams §
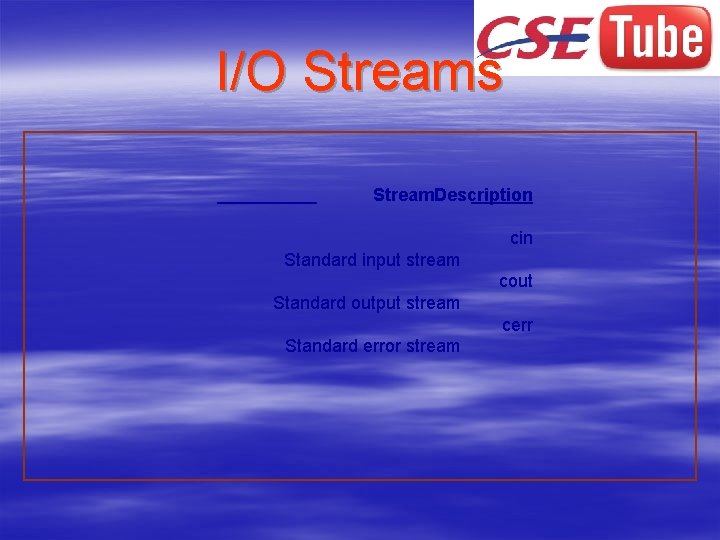
I/O Streams Stream. Description cin Standard input stream cout Standard output stream cerr Standard error stream
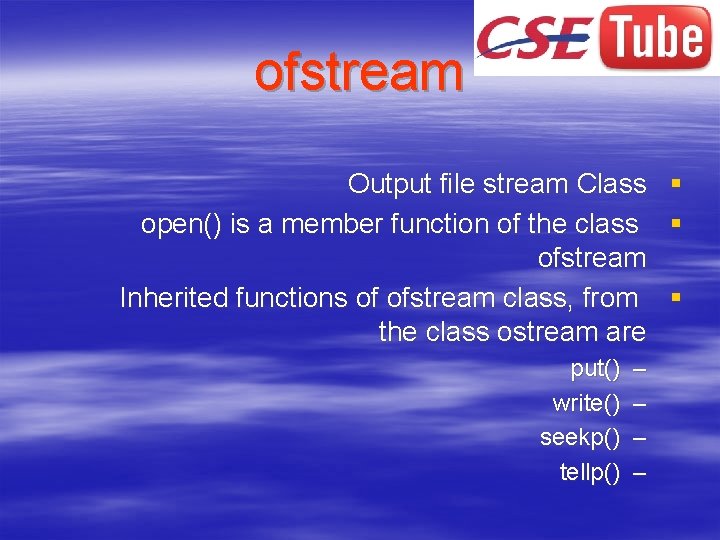
ofstream Output file stream Class open() is a member function of the class ofstream Inherited functions of ofstream class, from the class ostream are put() write() seekp() tellp() – – § § §
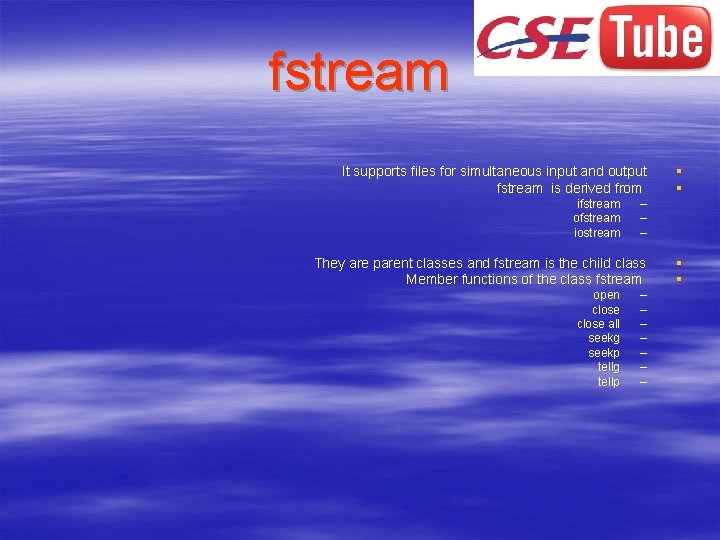
fstream It supports files for simultaneous input and output fstream is derived from ifstream ofstream iostream – – – They are parent classes and fstream is the child class Member functions of the class fstream open close all seekg seekp tellg tellp – – – – § §
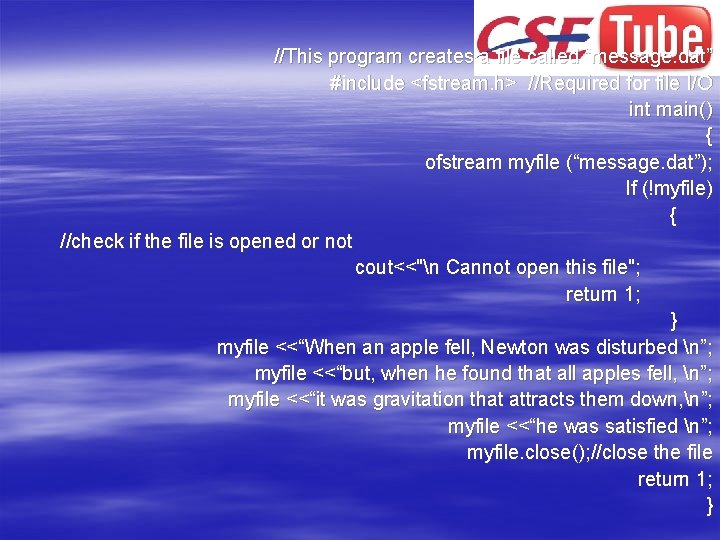
//This program creates a file called “message. dat” #include <fstream. h> //Required for file I/O int main() { ofstream myfile (“message. dat”); If (!myfile) { //check if the file is opened or not cout<<"n Cannot open this file"; return 1; } myfile <<“When an apple fell, Newton was disturbed n”; myfile <<“but, when he found that all apples fell, n”; myfile <<“it was gravitation that attracts them down, n”; myfile <<“he was satisfied n”; myfile. close(); //close the file return 1; }
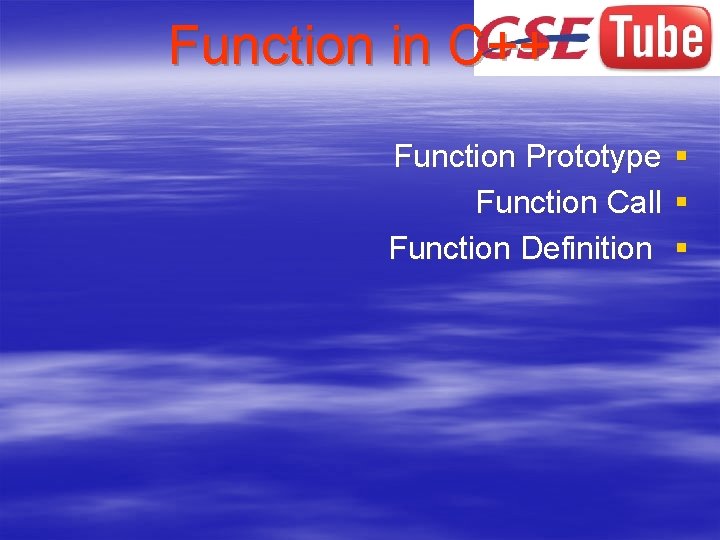
Function in C++ Function Prototype Function Call Function Definition § § §