static void Main using File Stream in File
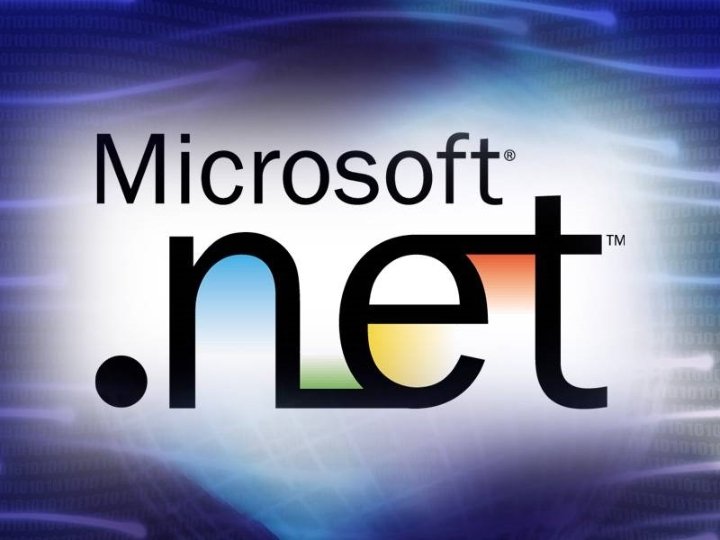
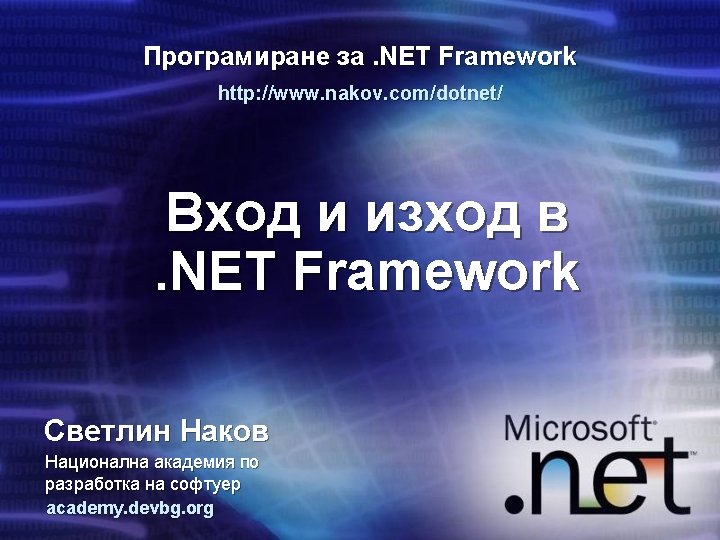
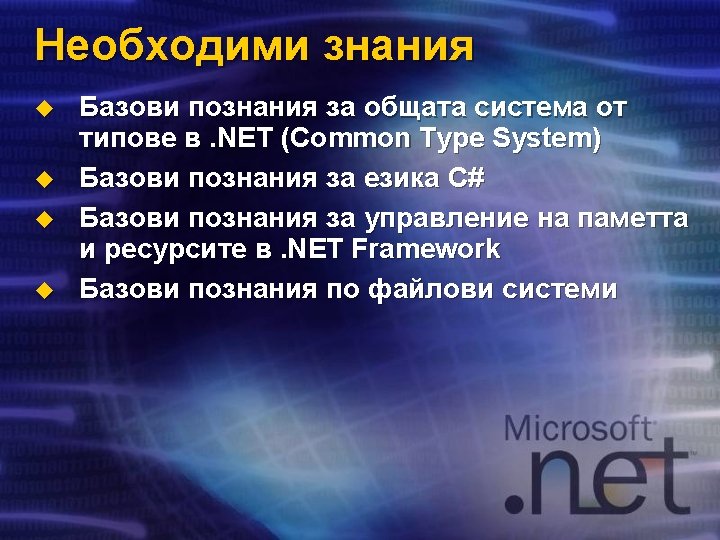
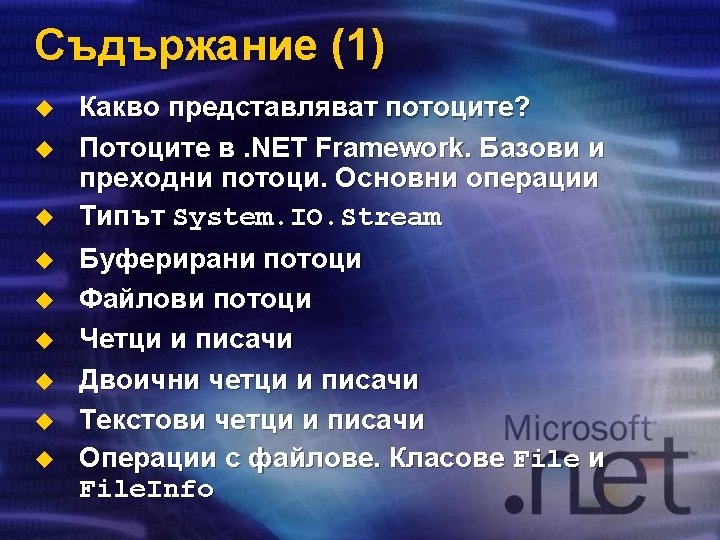
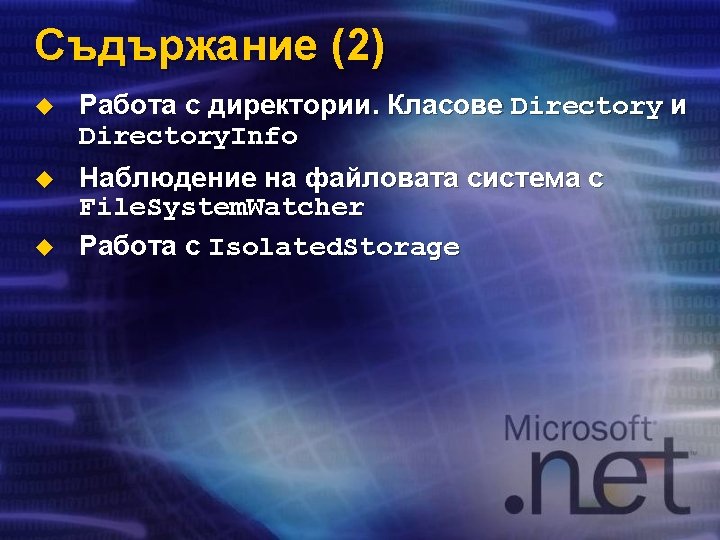
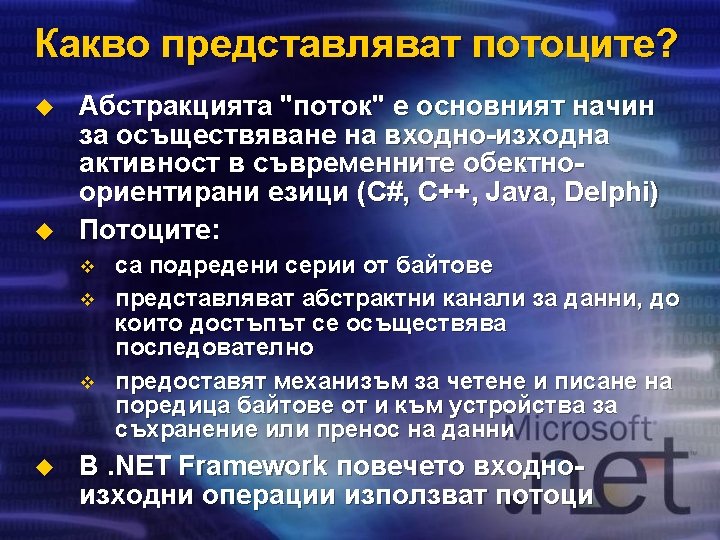
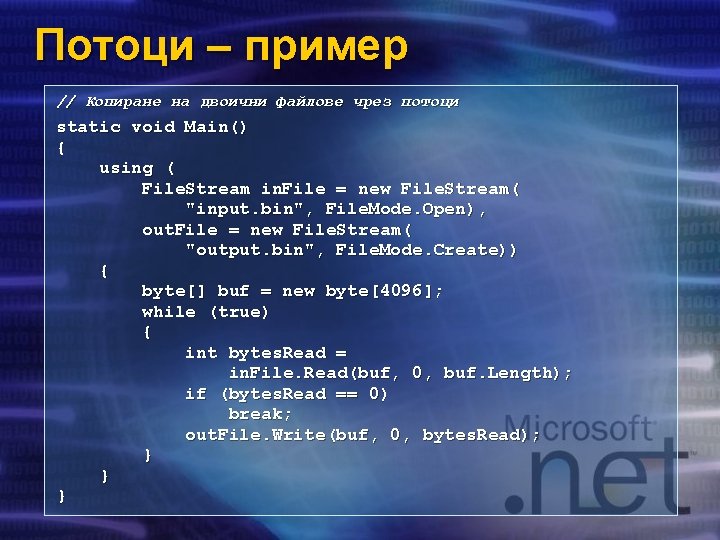
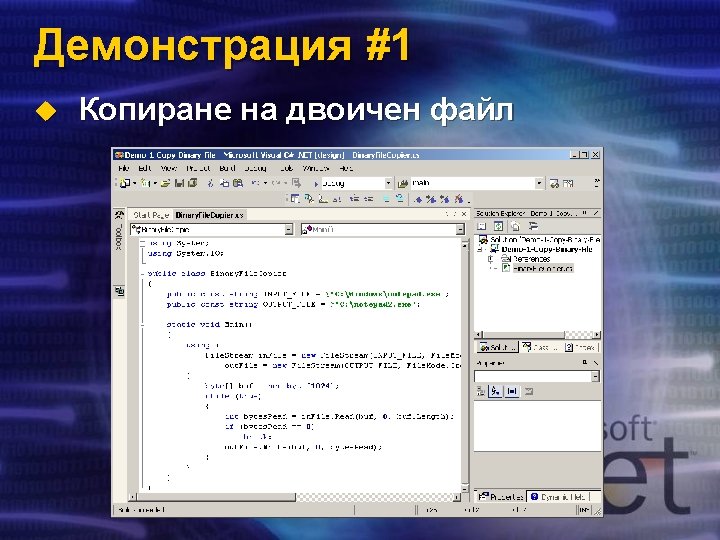
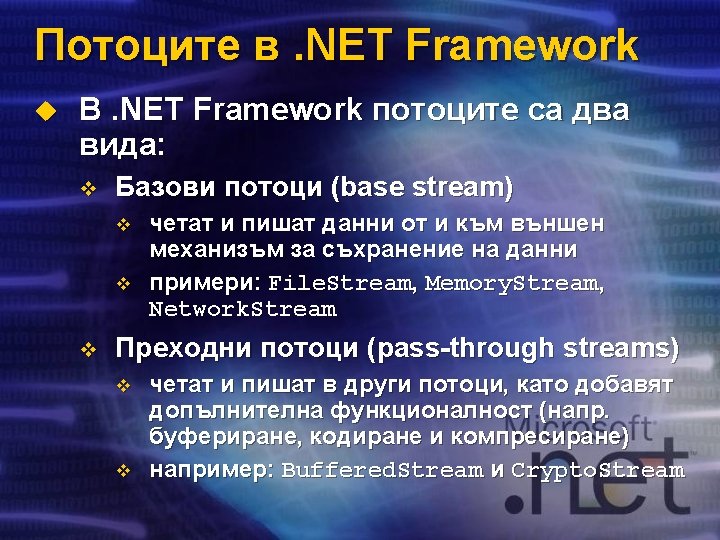
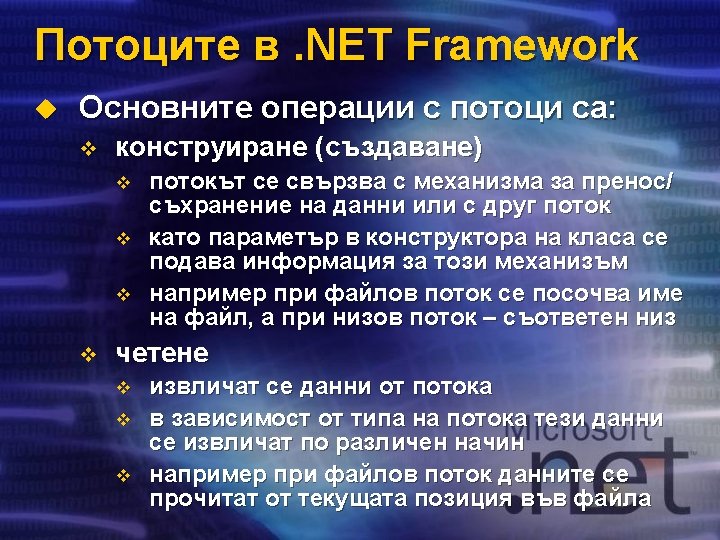
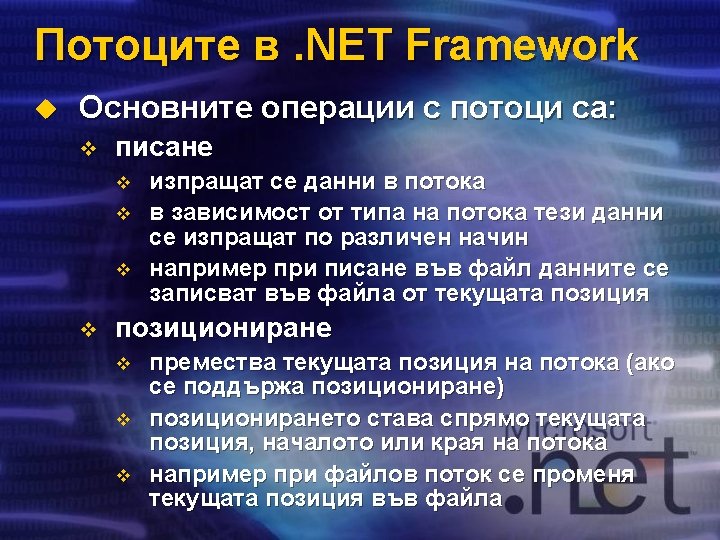
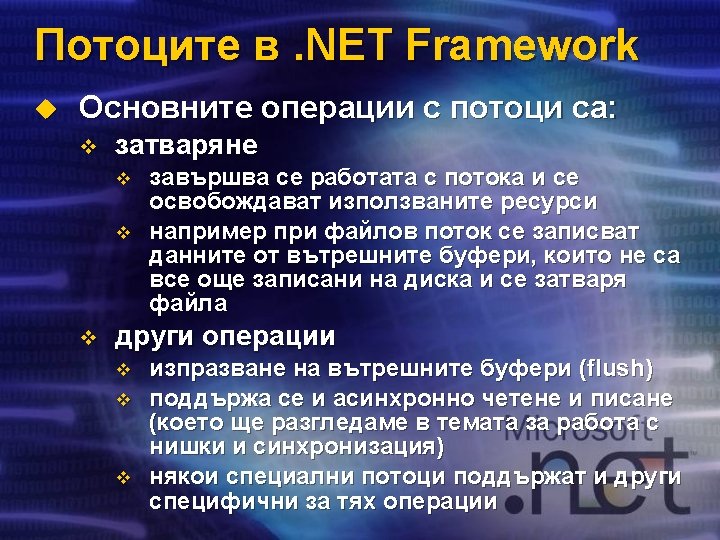
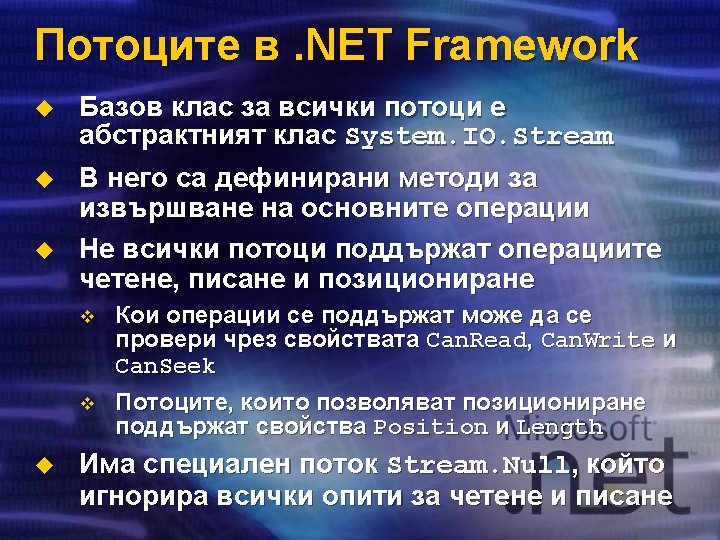
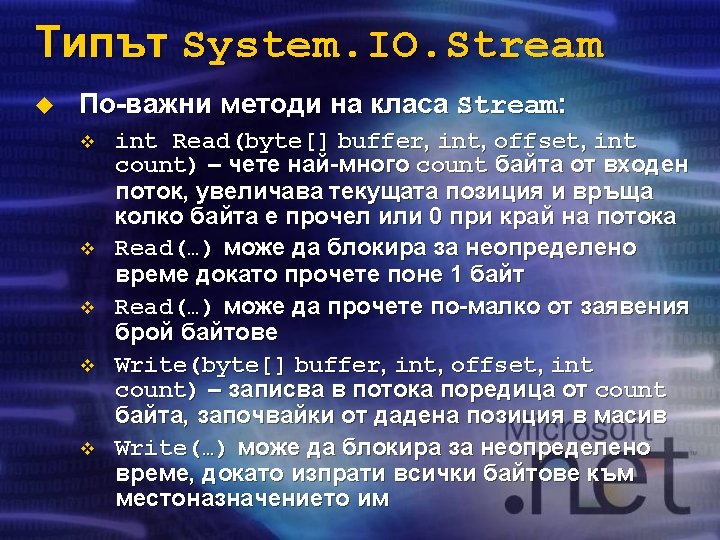
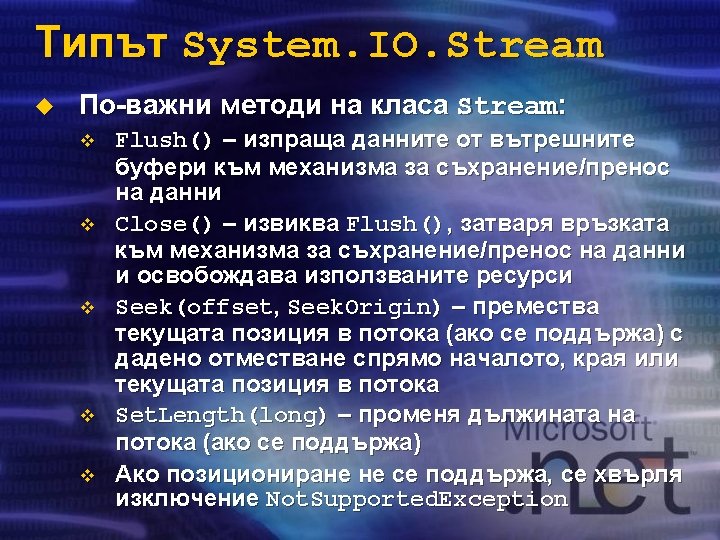
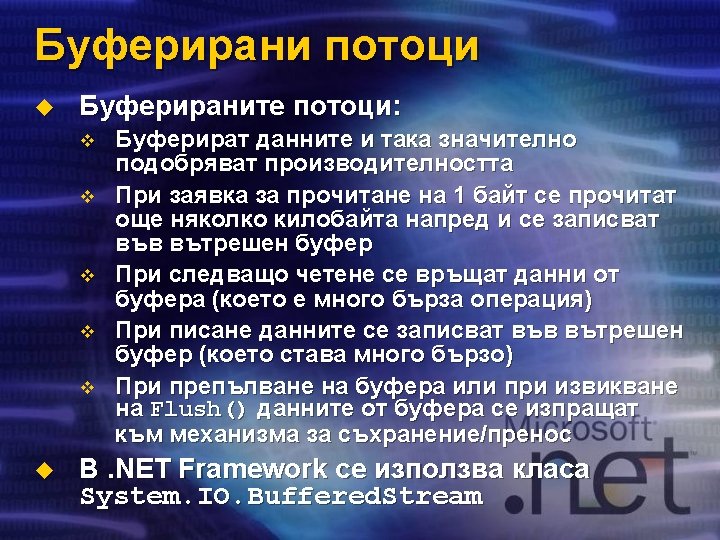
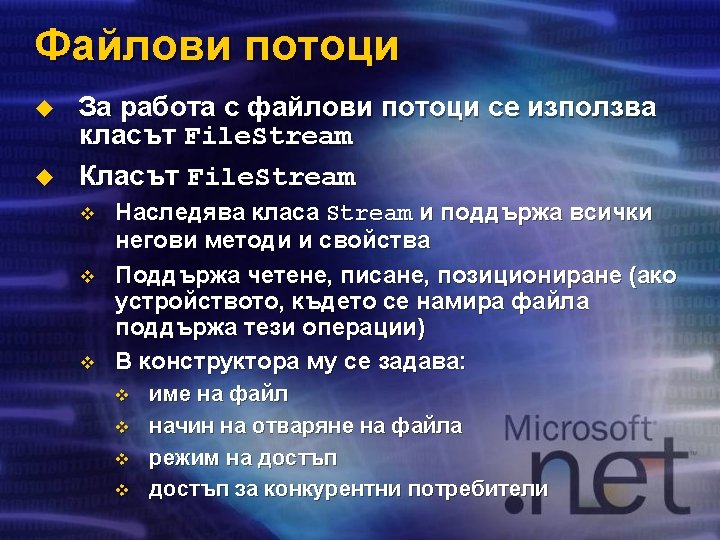
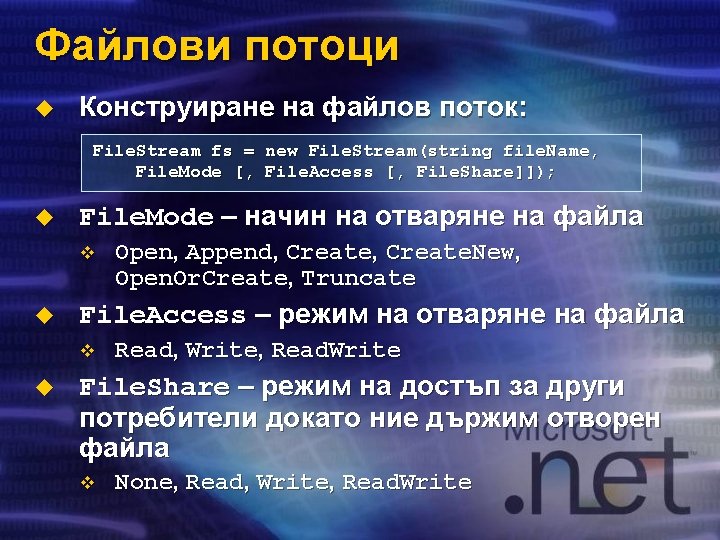
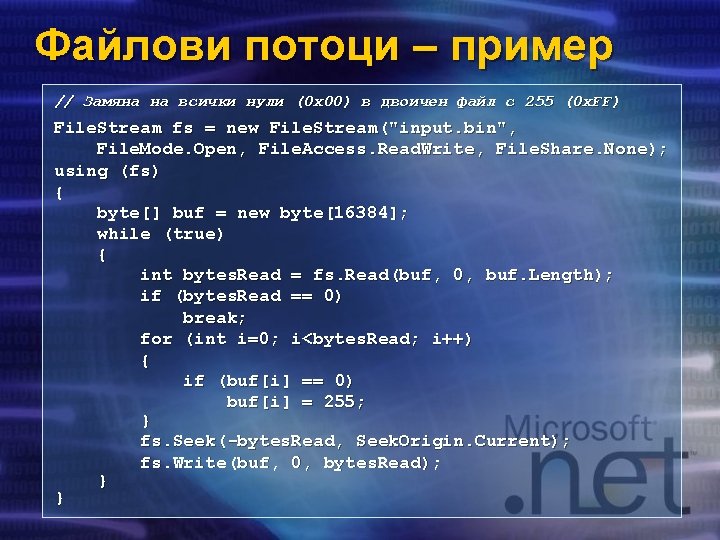
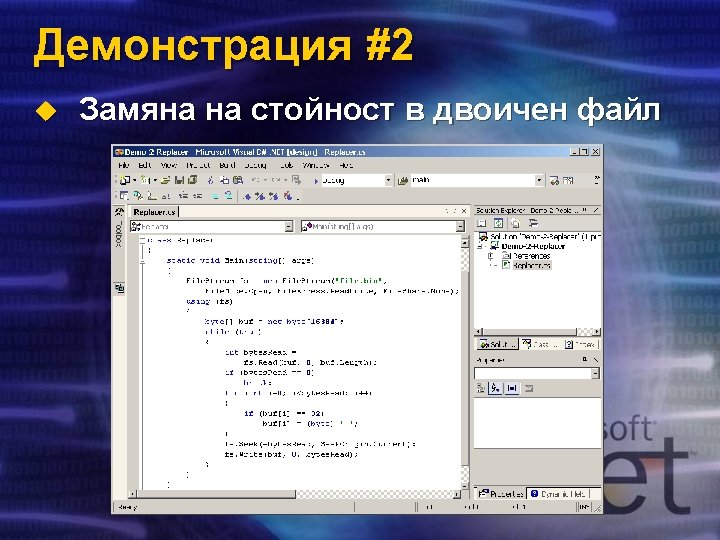
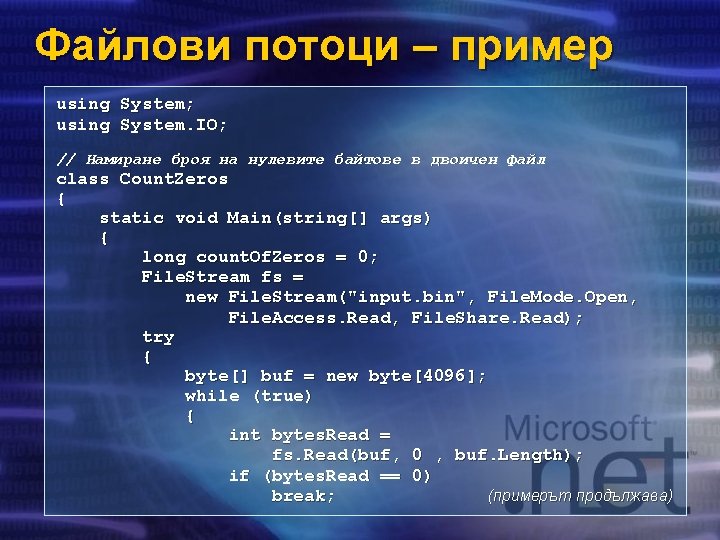
![Файлови потоци – пример for (int i=0; i<bytes. Read; i++) { if (buf[i] == Файлови потоци – пример for (int i=0; i<bytes. Read; i++) { if (buf[i] ==](https://slidetodoc.com/presentation_image_h2/ca5116a0ee53f2b45385e4f2df6fd6b1/image-22.jpg)
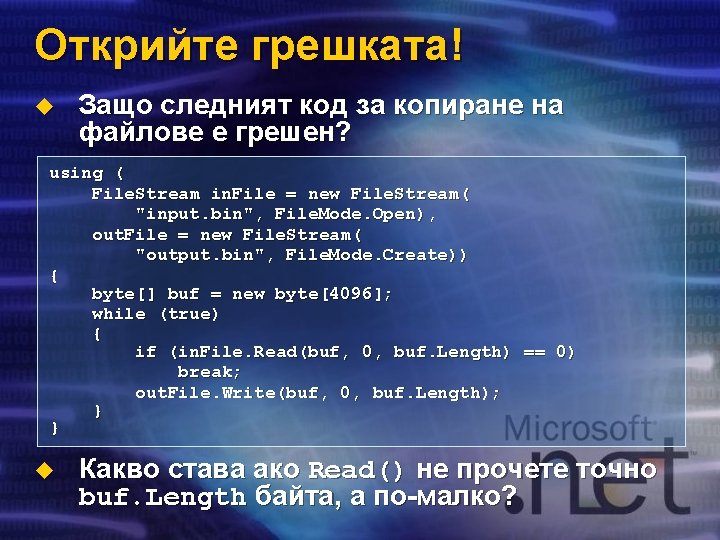
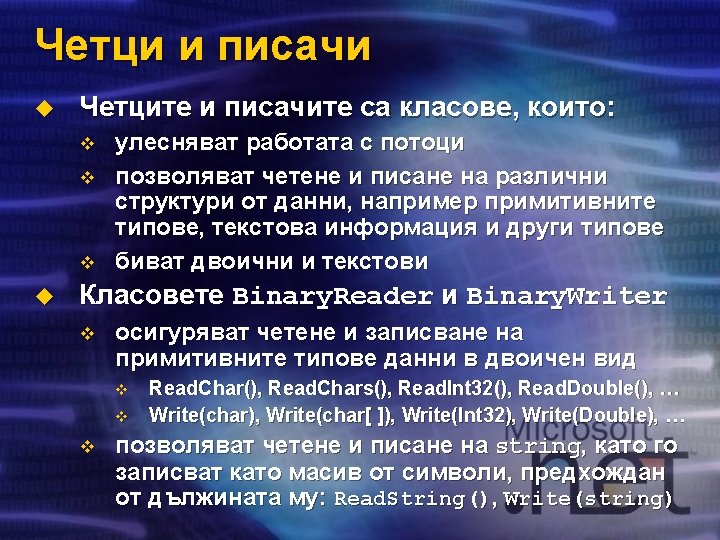
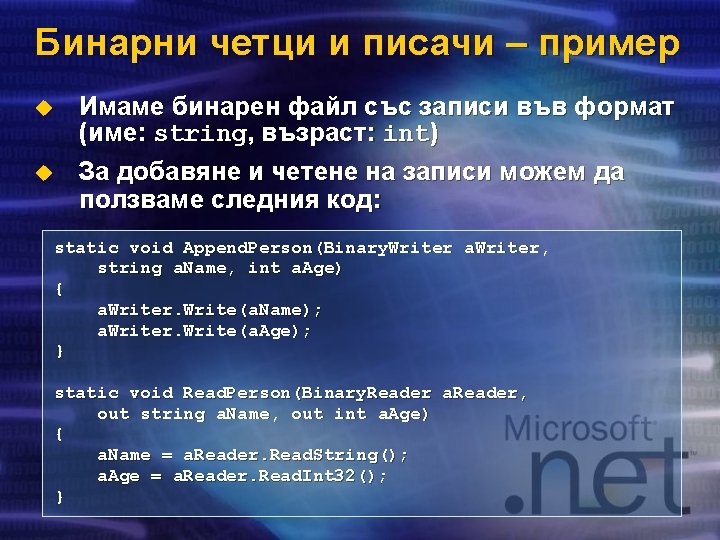
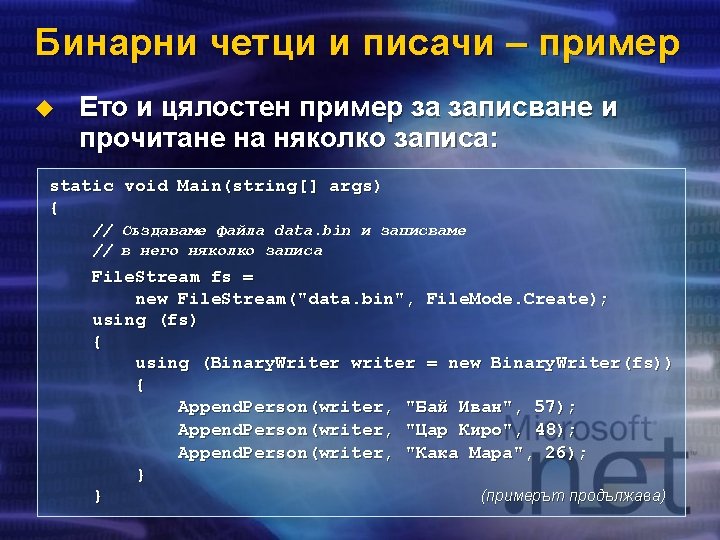
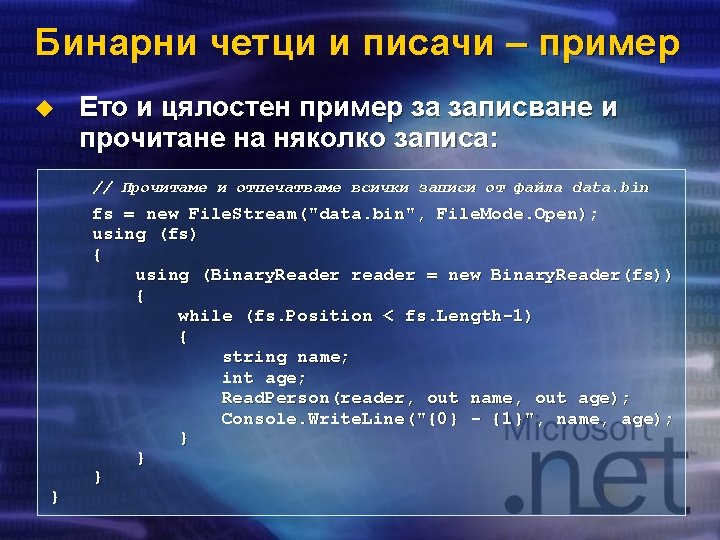
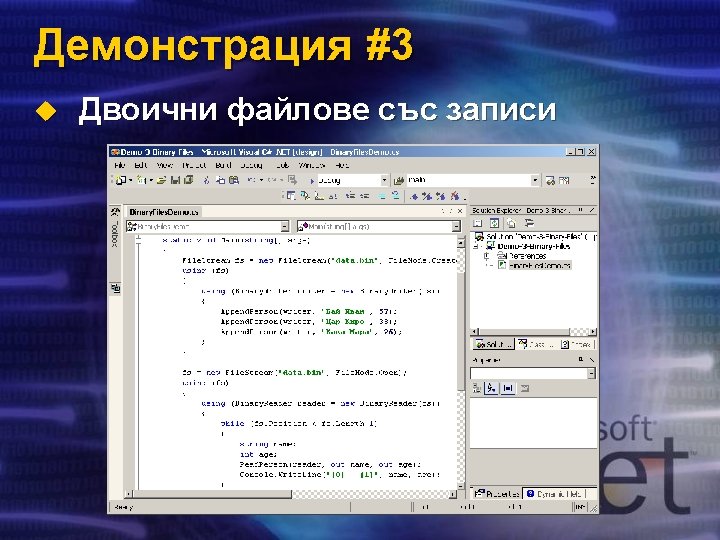
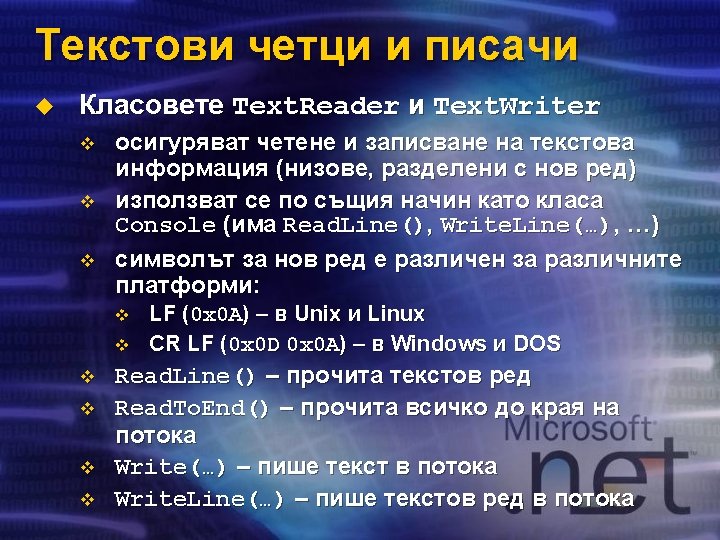
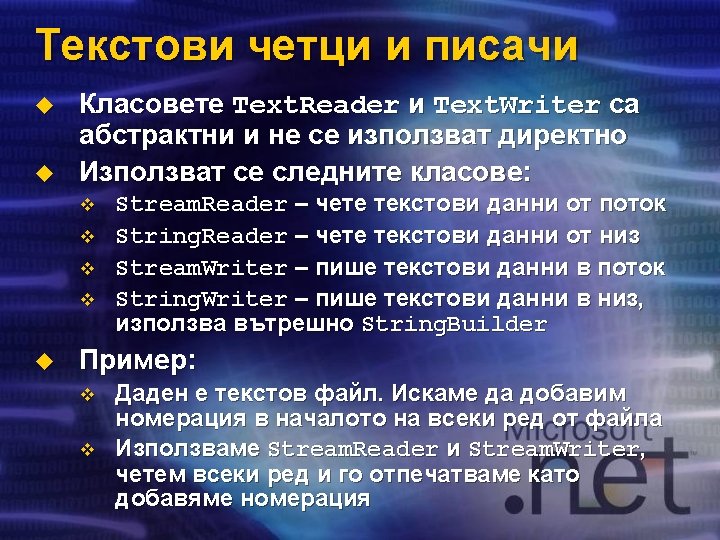
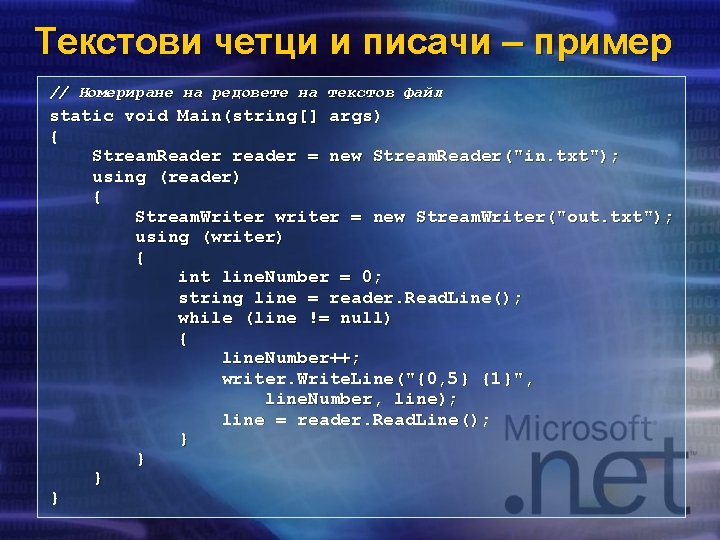
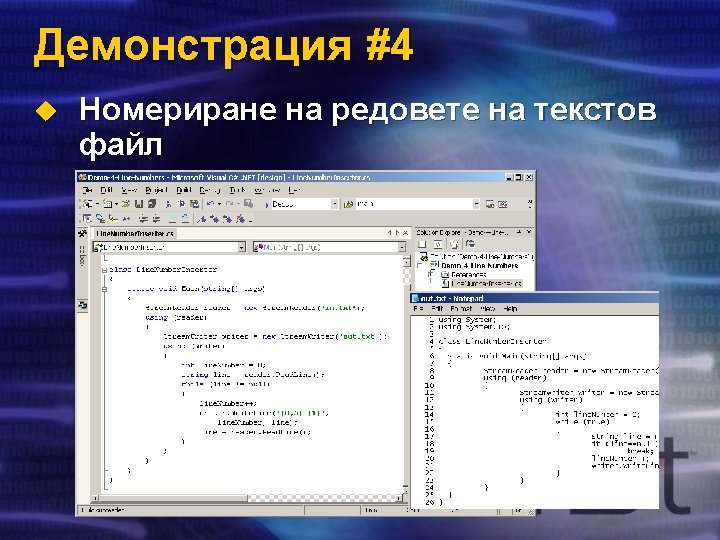
![Текстови четци и писачи – пример // Find-text-in-file Search Utility static void Main(string[] args) Текстови четци и писачи – пример // Find-text-in-file Search Utility static void Main(string[] args)](https://slidetodoc.com/presentation_image_h2/ca5116a0ee53f2b45385e4f2df6fd6b1/image-33.jpg)
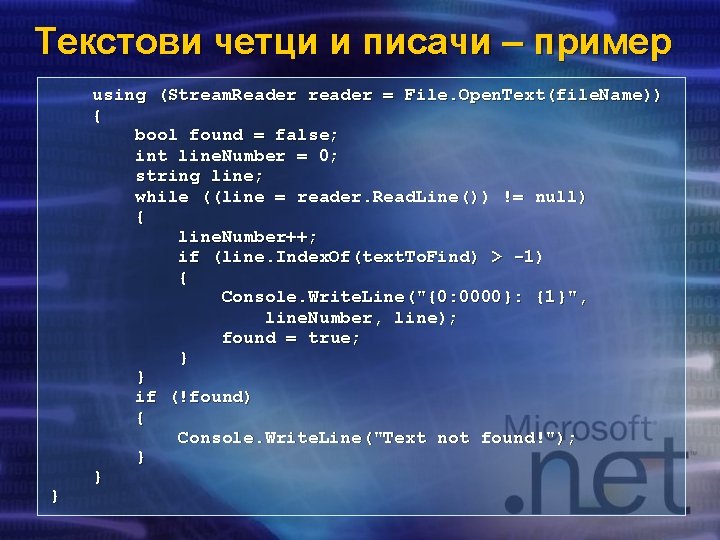
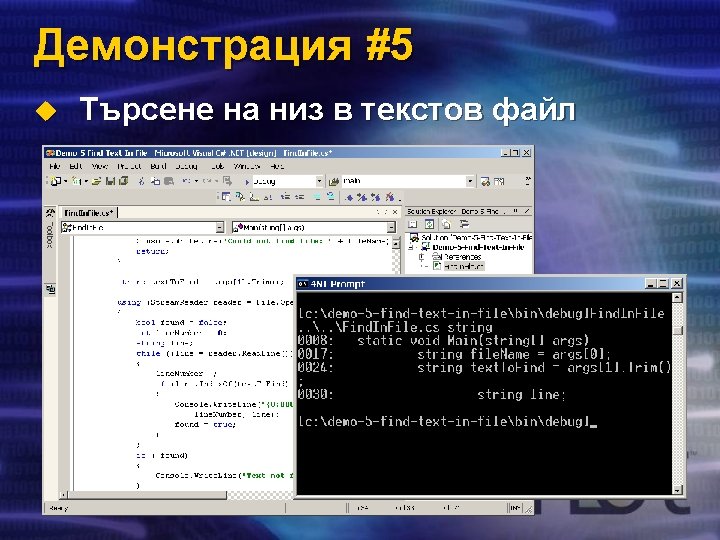
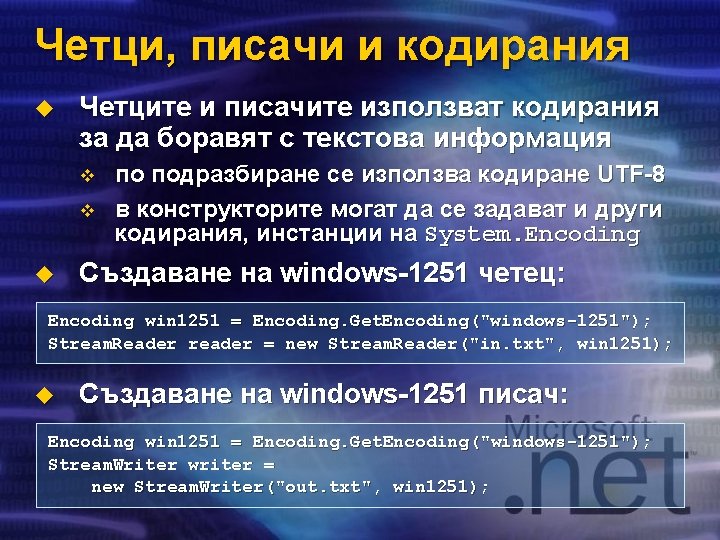
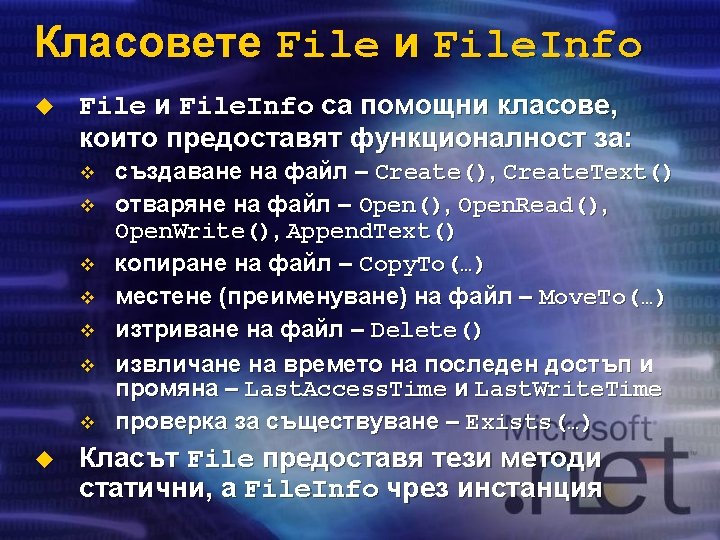
![File и File. Info – пример static void Main(string[] args) { Stream. Writer writer File и File. Info – пример static void Main(string[] args) { Stream. Writer writer](https://slidetodoc.com/presentation_image_h2/ca5116a0ee53f2b45385e4f2df6fd6b1/image-38.jpg)
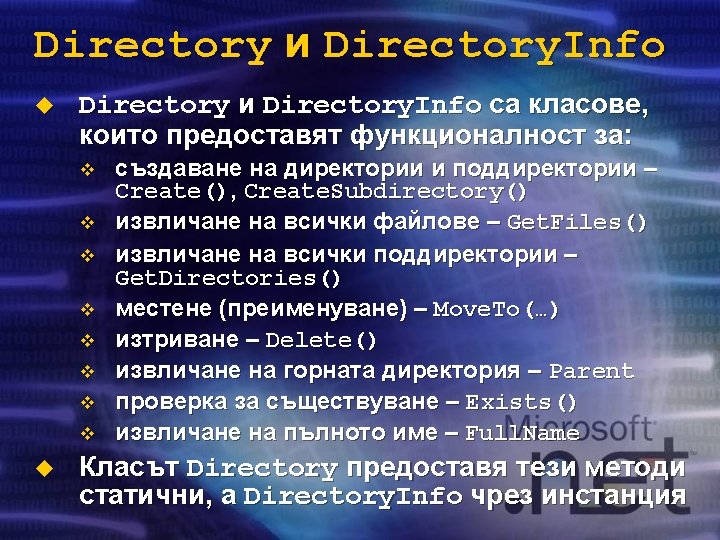
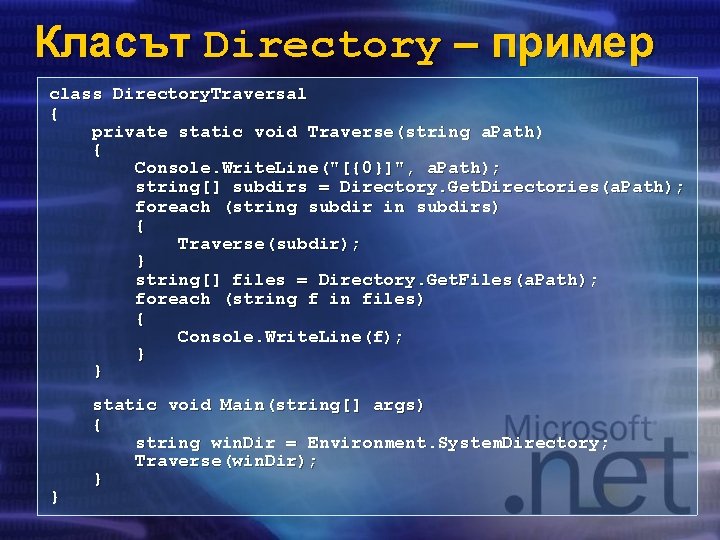
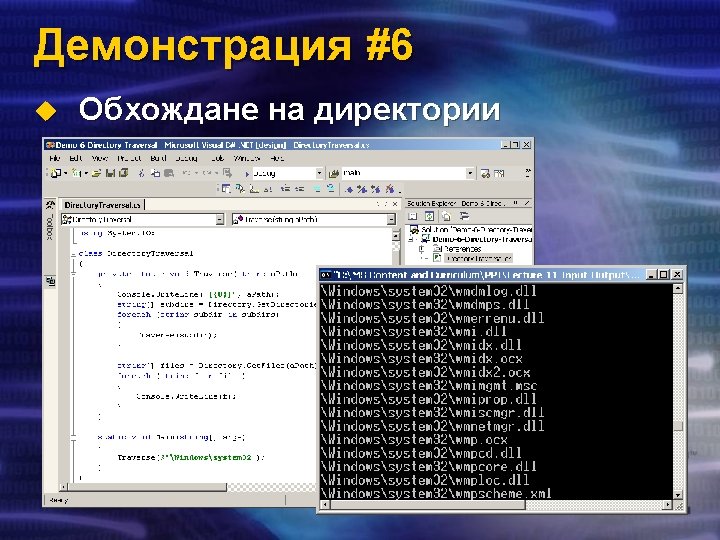
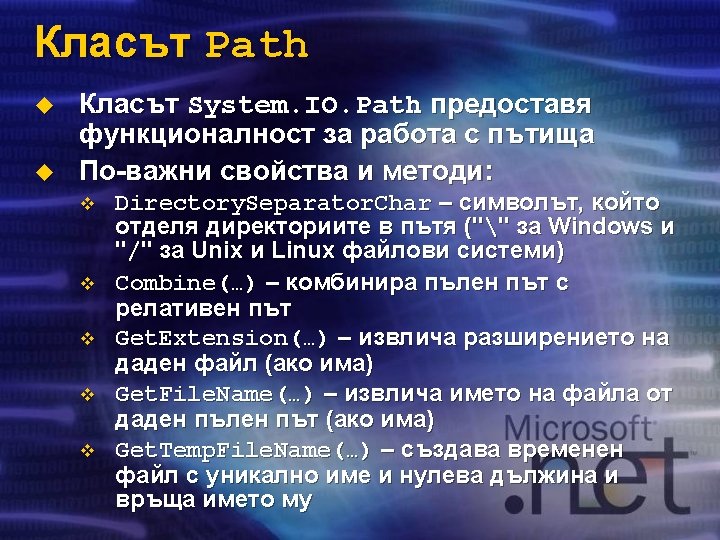
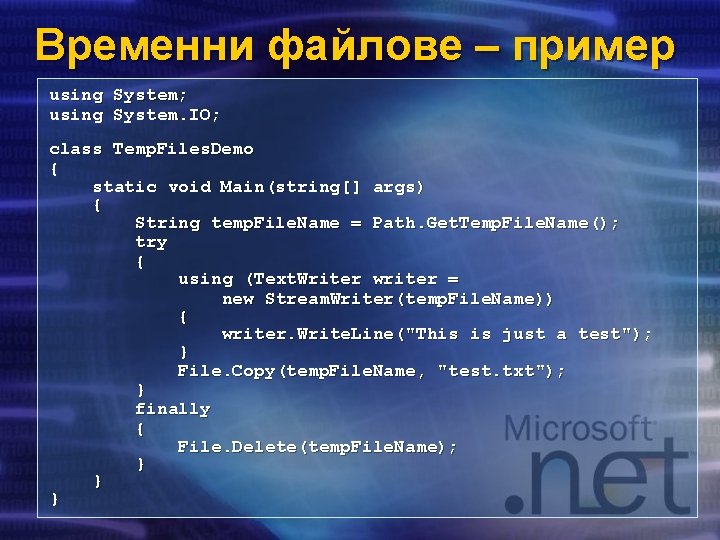
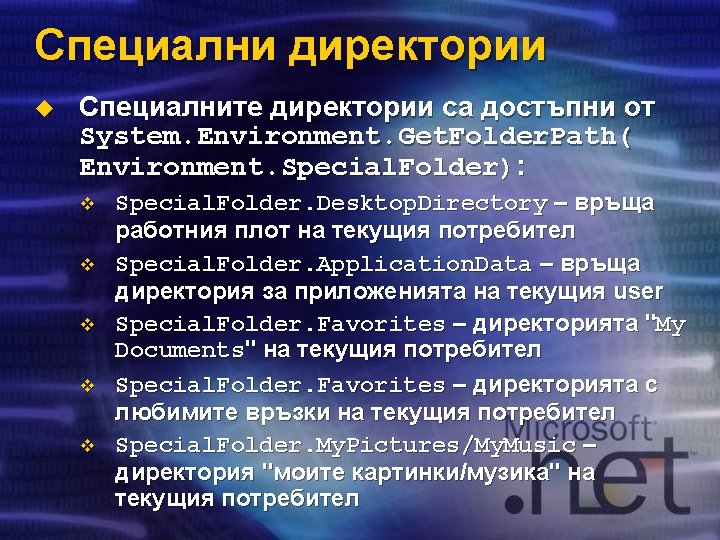
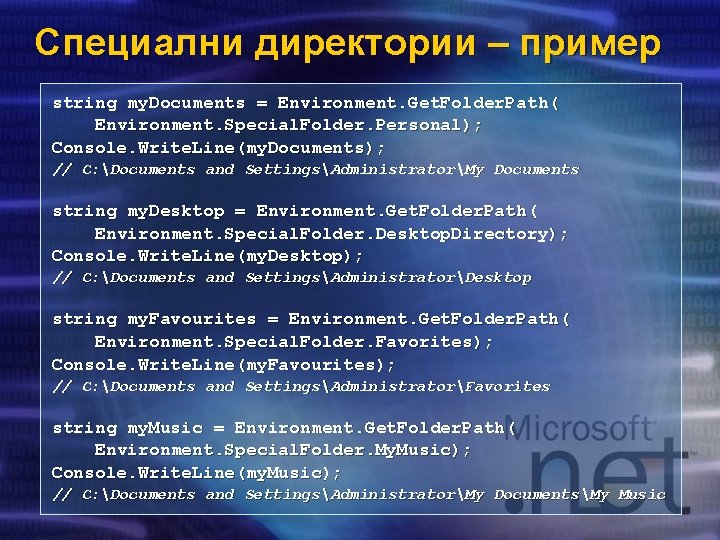
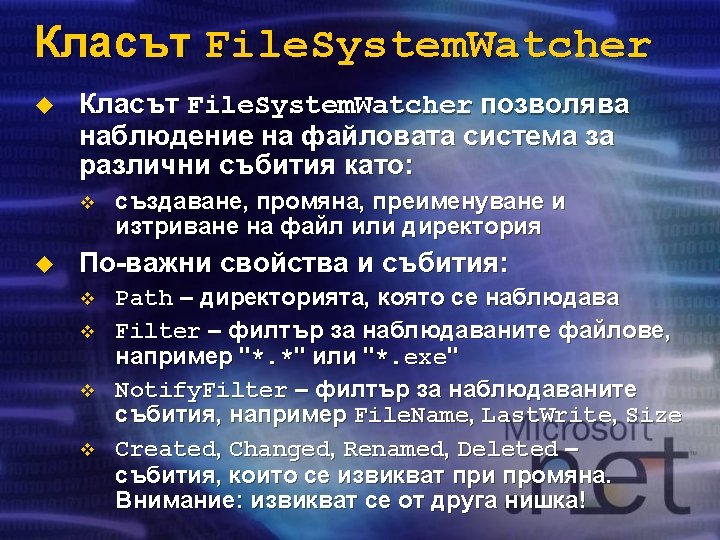
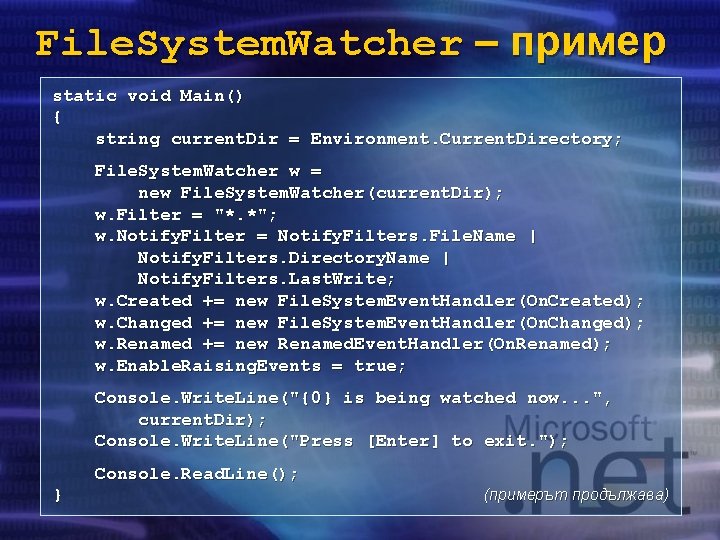
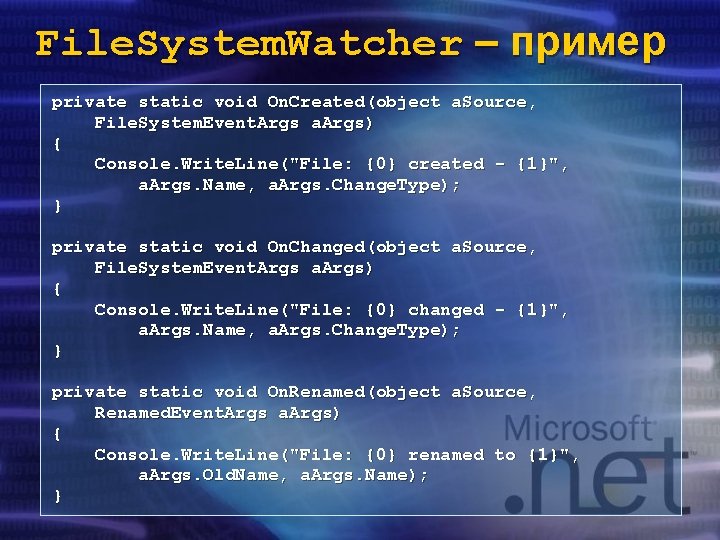
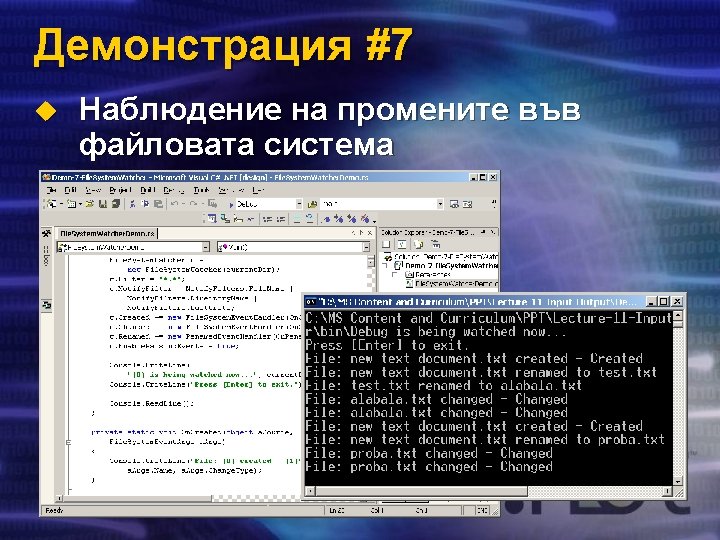
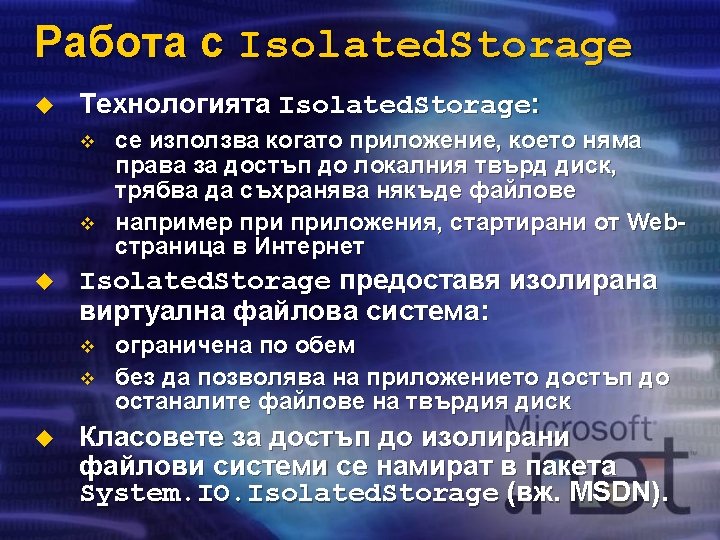
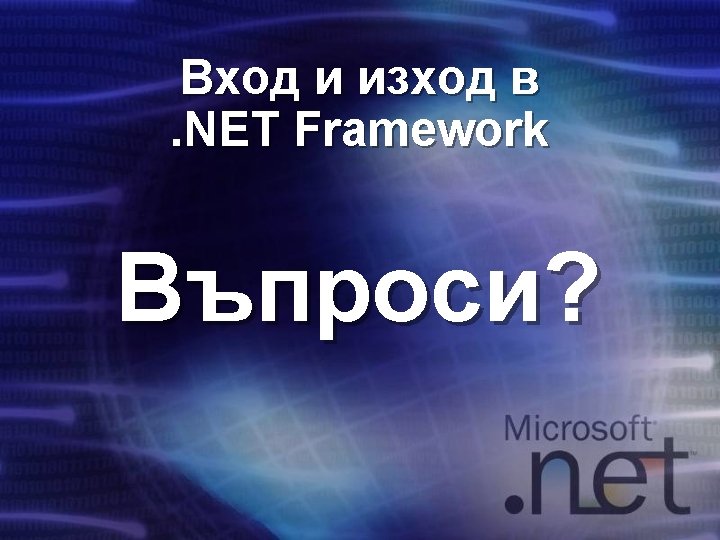
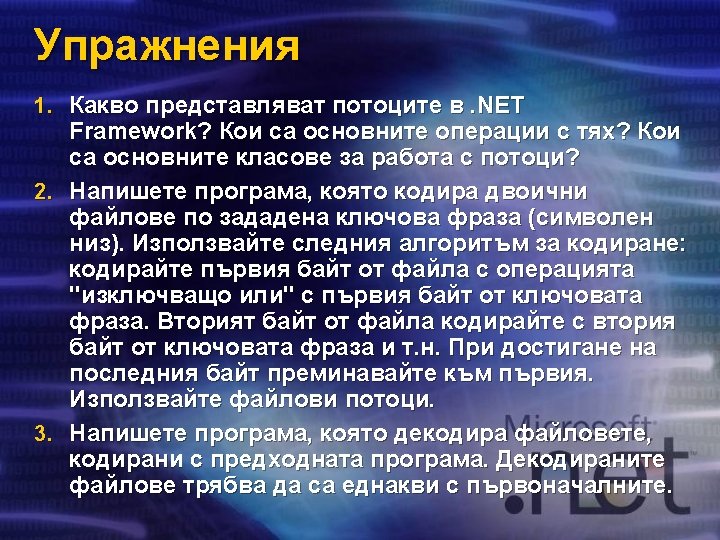
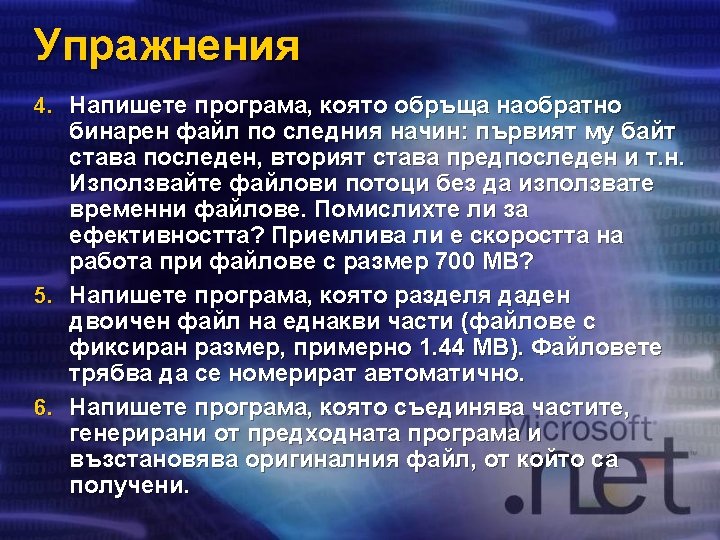
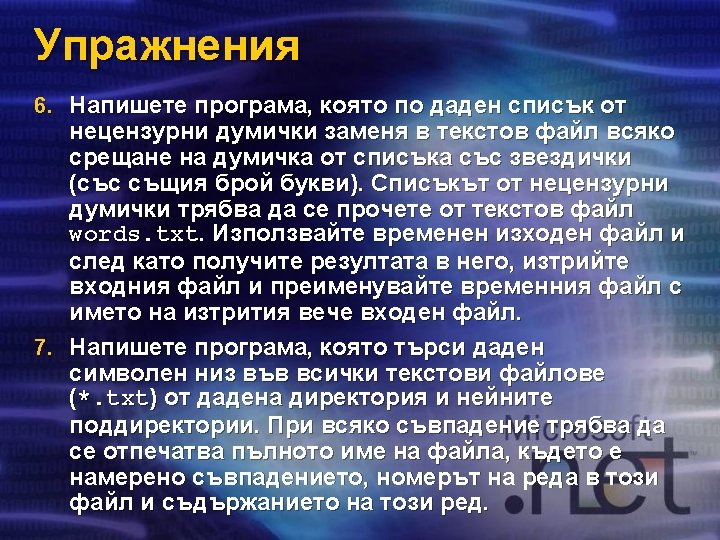
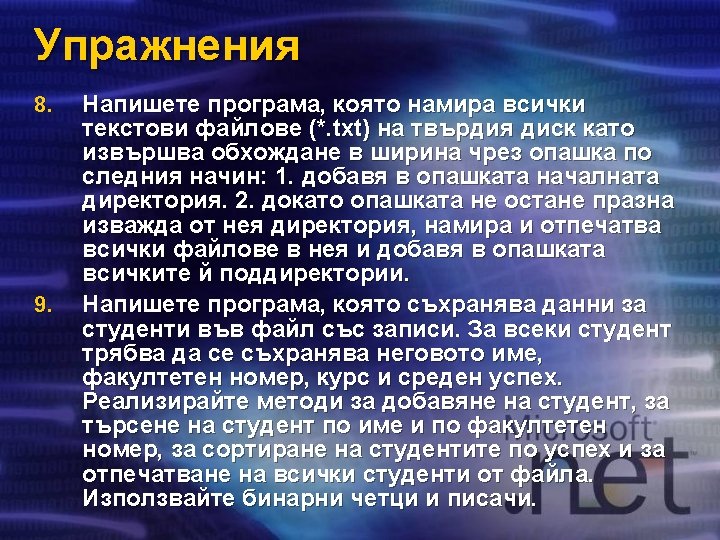
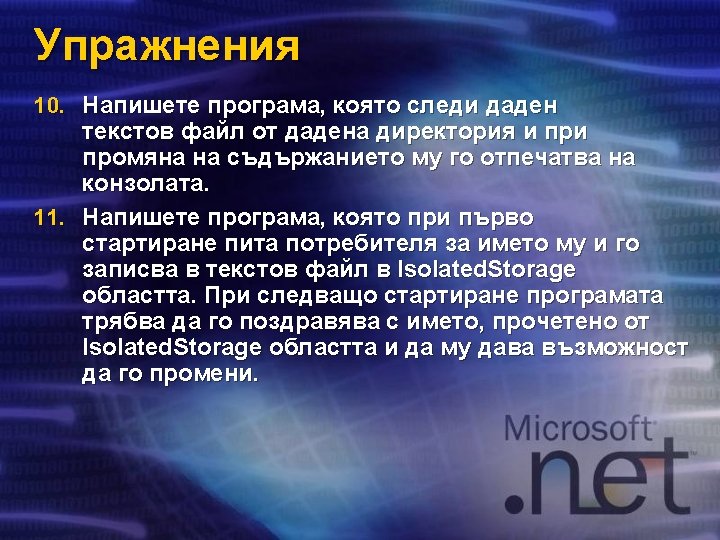
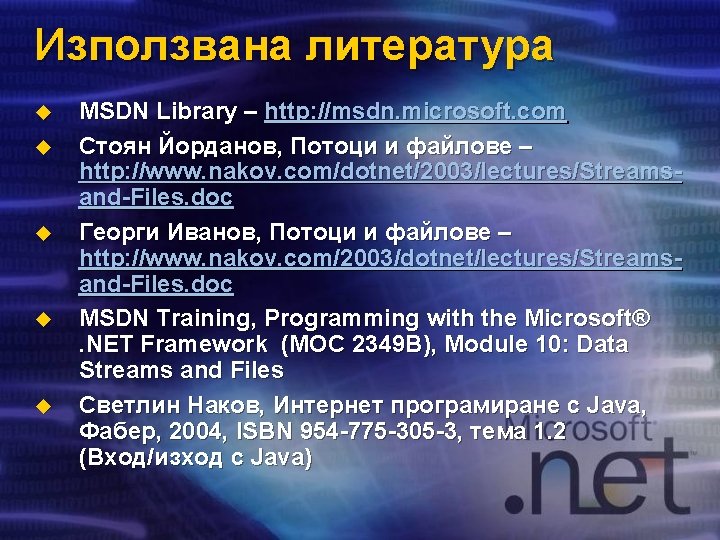
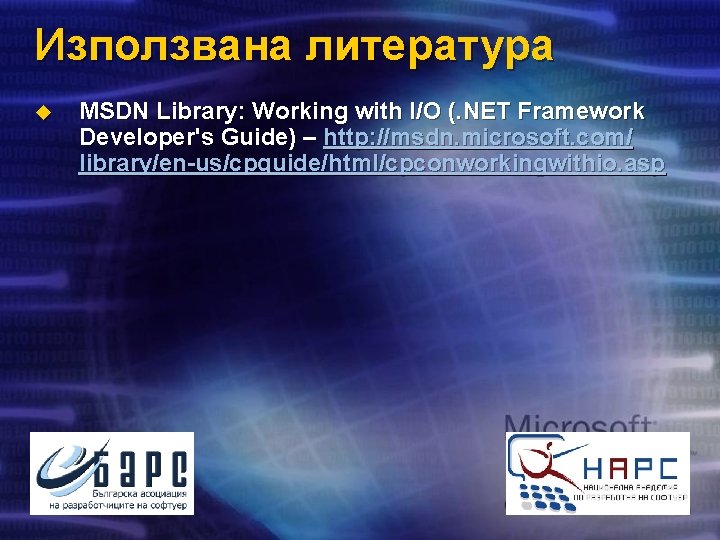
- Slides: 58
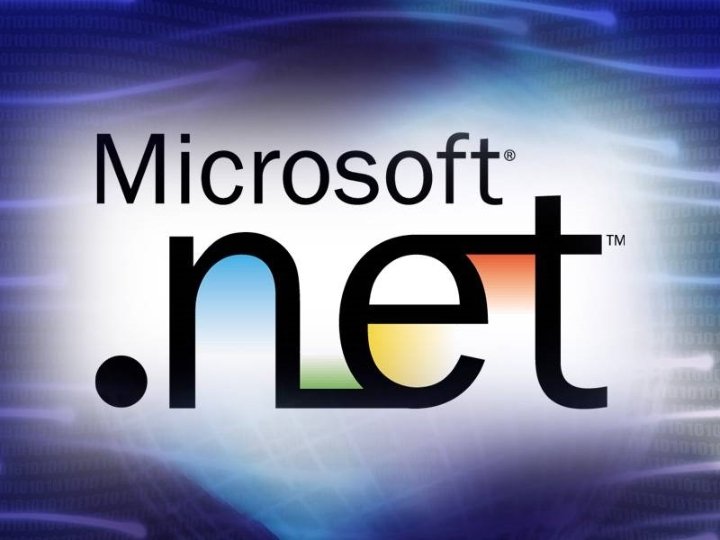
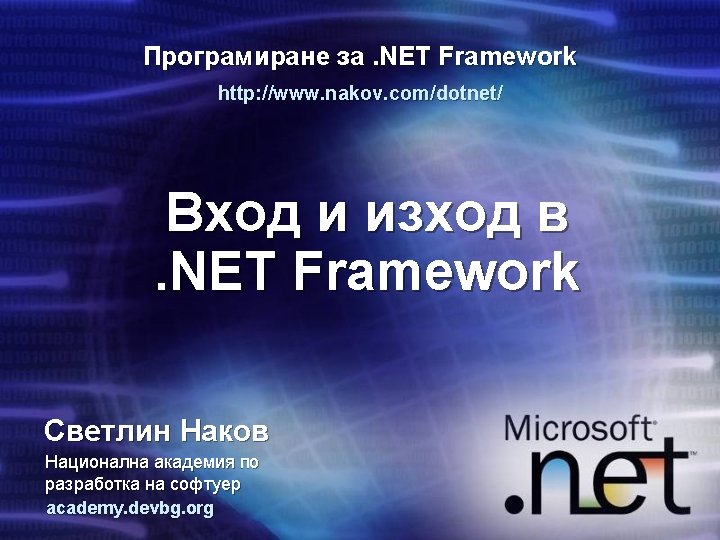
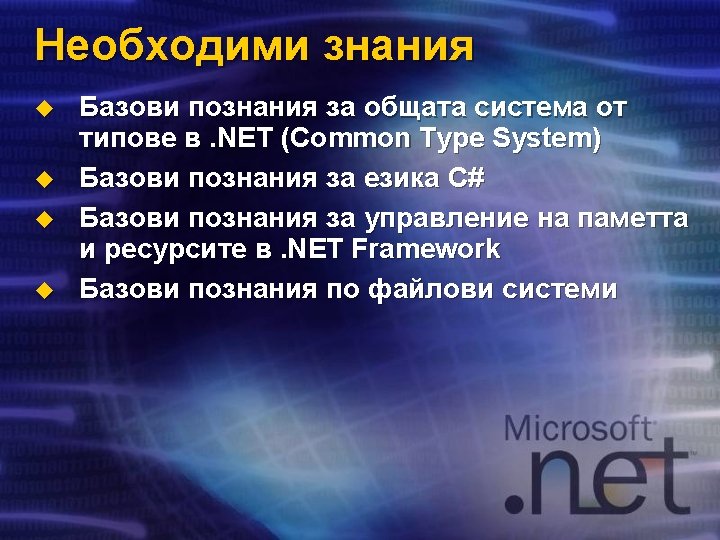
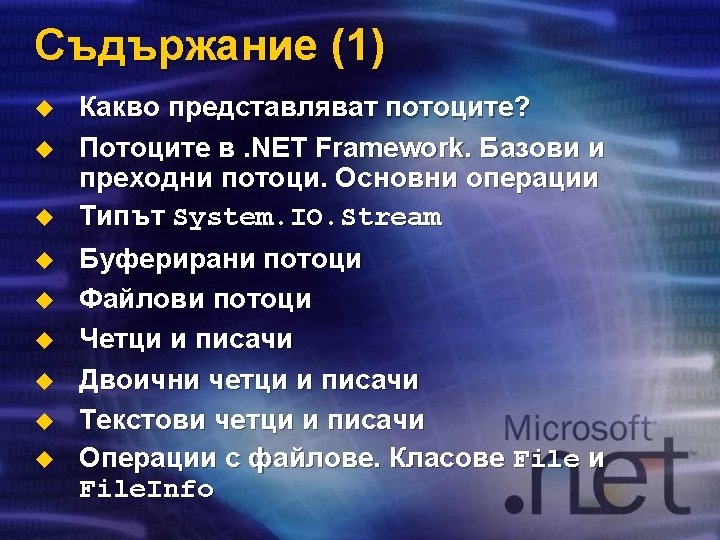
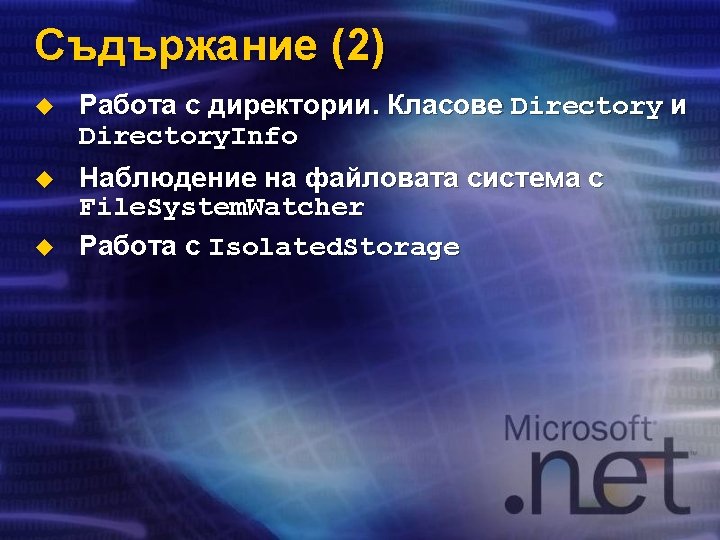
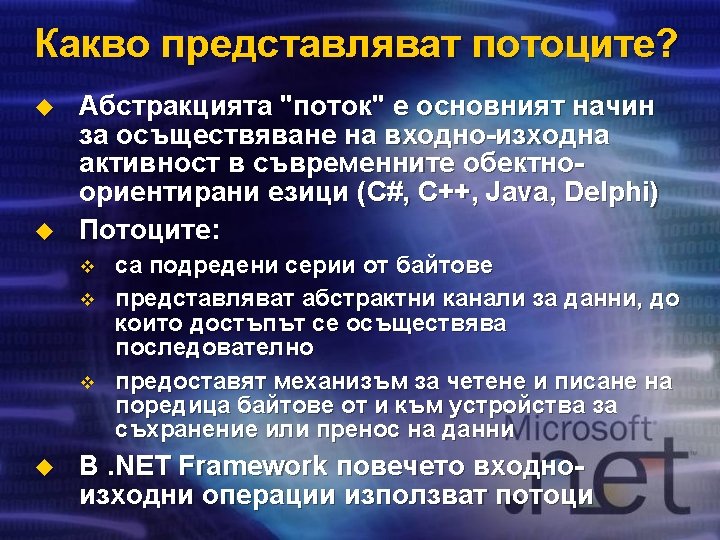
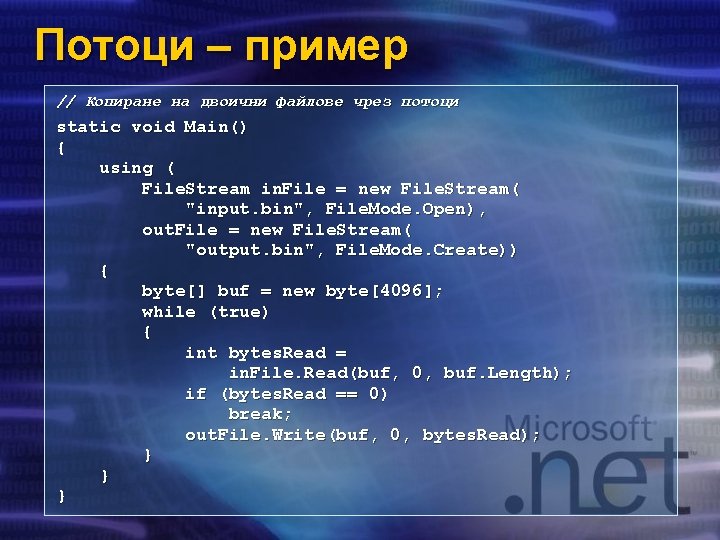
Потоци – пример // Копиране на двоични файлове чрез потоци static void Main() { using ( File. Stream in. File = new File. Stream( "input. bin", File. Mode. Open), out. File = new File. Stream( "output. bin", File. Mode. Create)) { byte[] buf = new byte[4096]; while (true) { int bytes. Read = in. File. Read(buf, 0, buf. Length); if (bytes. Read == 0) break; out. File. Write(buf, 0, bytes. Read); } } }
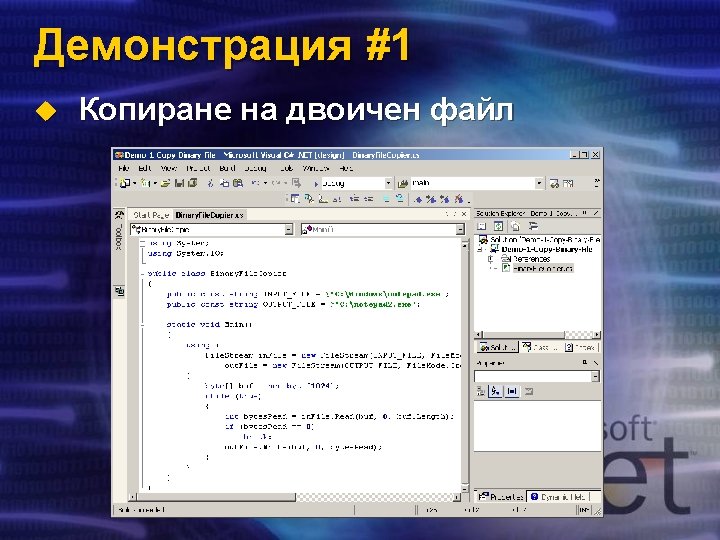
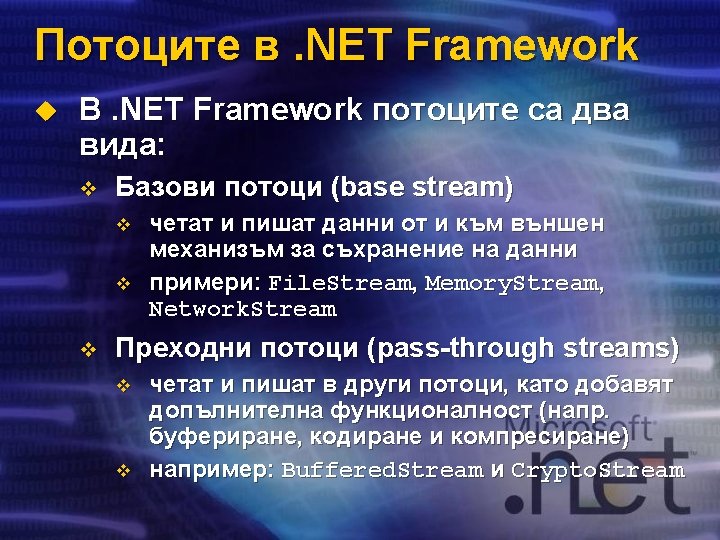
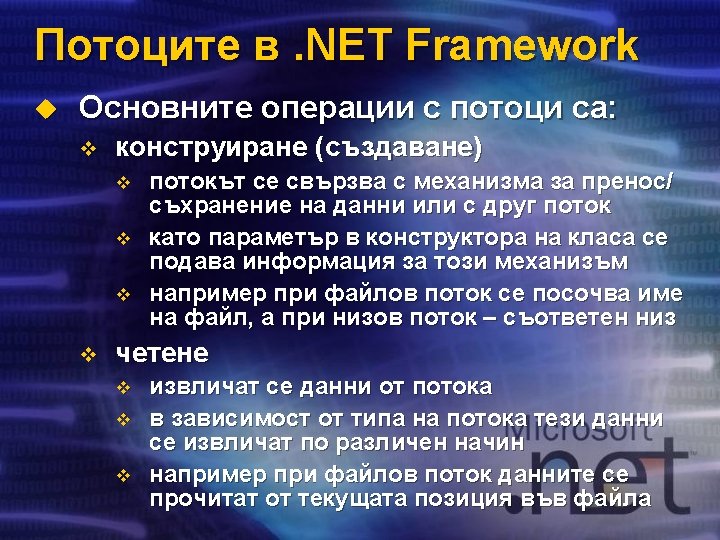
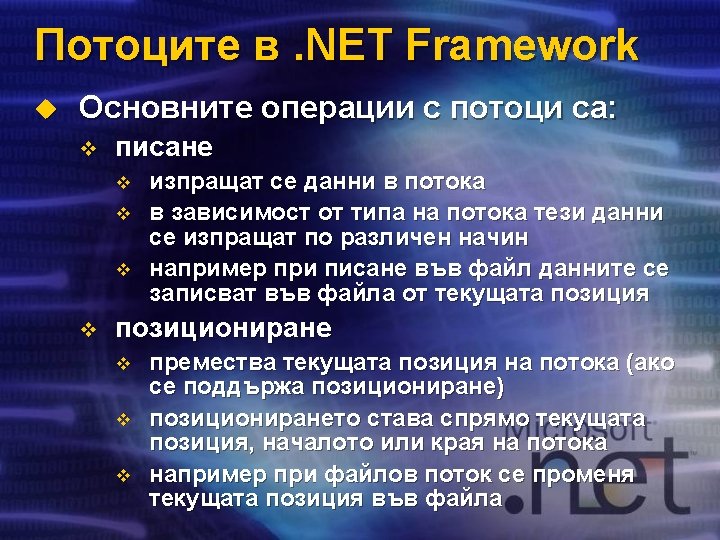
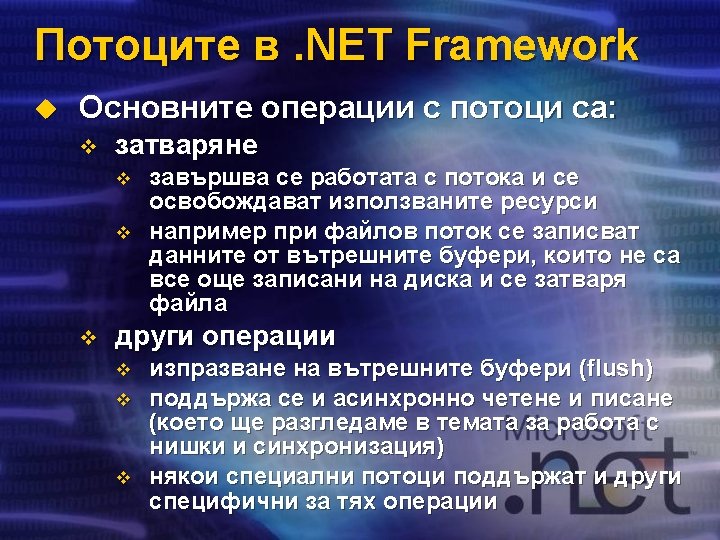
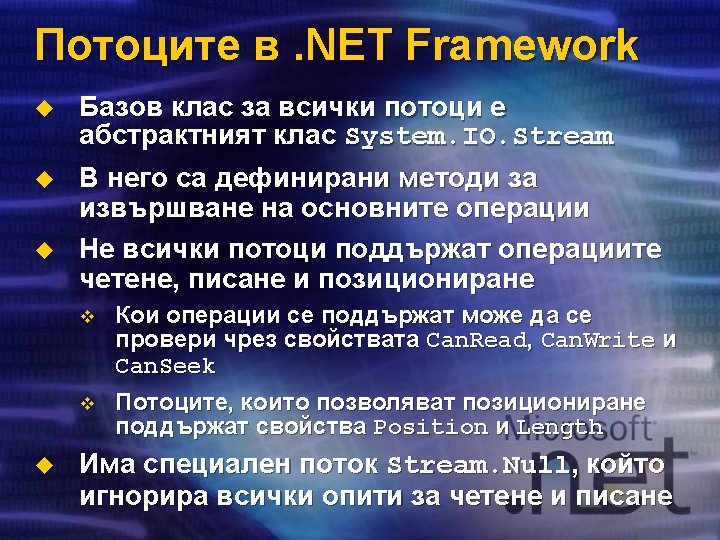
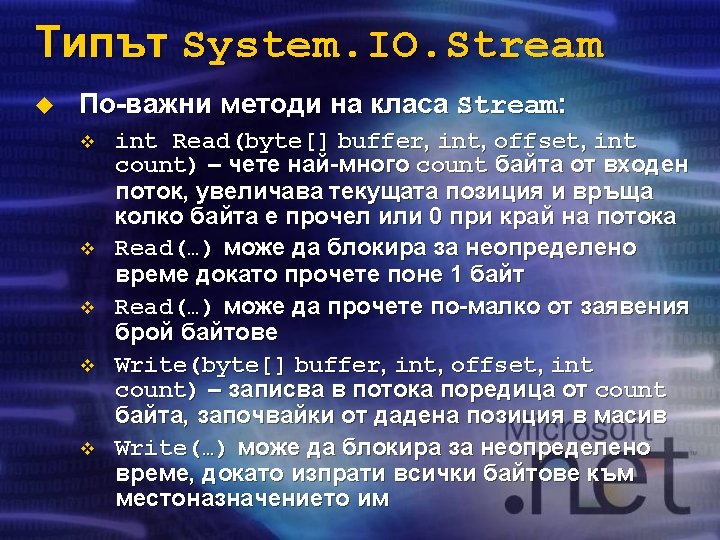
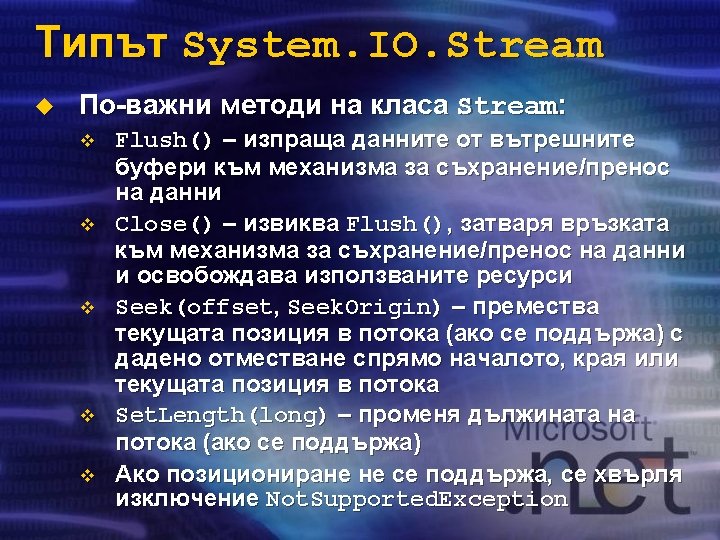
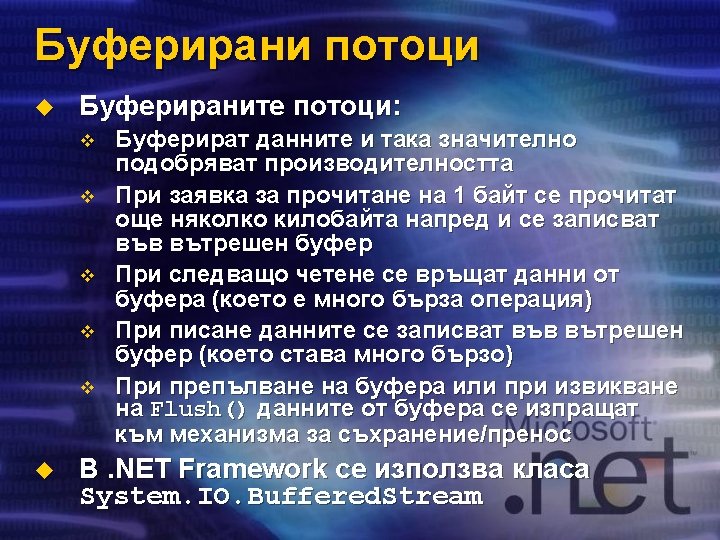
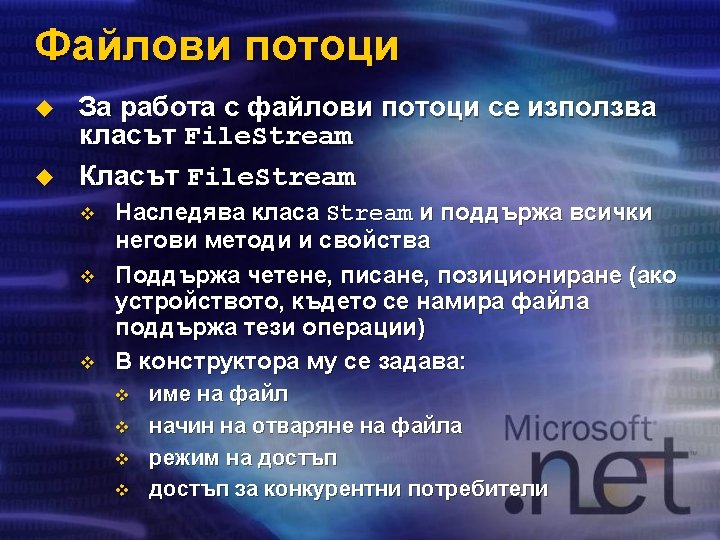
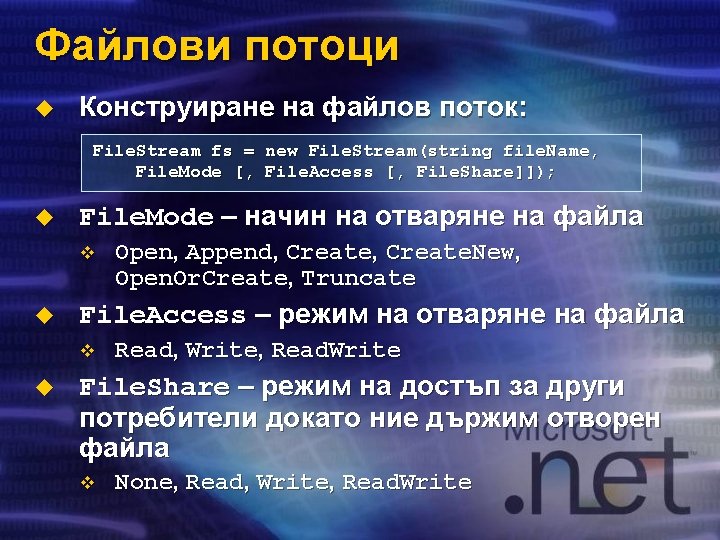
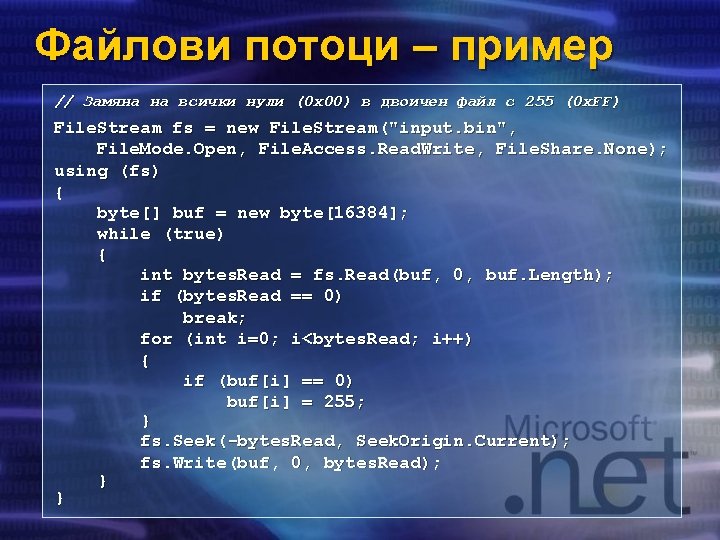
Файлови потоци – пример // Замяна на всички нули (0 x 00) в двоичен файл с 255 (0 x. FF) File. Stream fs = new File. Stream("input. bin", File. Mode. Open, File. Access. Read. Write, File. Share. None); using (fs) { byte[] buf = new byte[16384]; while (true) { int bytes. Read = fs. Read(buf, 0, buf. Length); if (bytes. Read == 0) break; for (int i=0; i<bytes. Read; i++) { if (buf[i] == 0) buf[i] = 255; } fs. Seek(-bytes. Read, Seek. Origin. Current); fs. Write(buf, 0, bytes. Read); } }
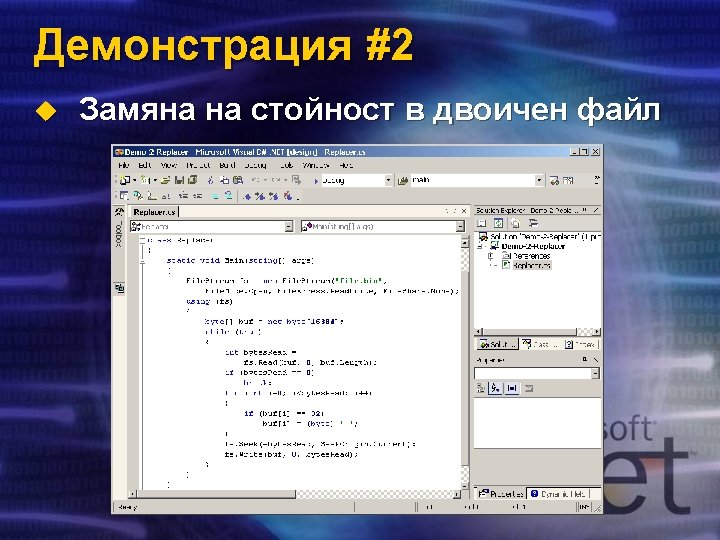
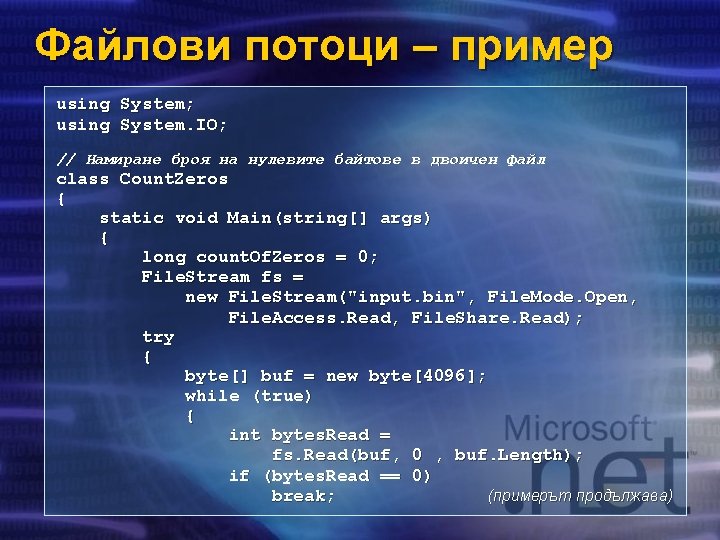
Файлови потоци – пример using System; using System. IO; // Намиране броя на нулевите байтове в двоичен файл class Count. Zeros { static void Main(string[] args) { long count. Of. Zeros = 0; File. Stream fs = new File. Stream("input. bin", File. Mode. Open, File. Access. Read, File. Share. Read); try { byte[] buf = new byte[4096]; while (true) { int bytes. Read = fs. Read(buf, 0 , buf. Length); if (bytes. Read == 0) break; (примерът продължава)
![Файлови потоци пример for int i0 ibytes Read i if bufi Файлови потоци – пример for (int i=0; i<bytes. Read; i++) { if (buf[i] ==](https://slidetodoc.com/presentation_image_h2/ca5116a0ee53f2b45385e4f2df6fd6b1/image-22.jpg)
Файлови потоци – пример for (int i=0; i<bytes. Read; i++) { if (buf[i] == 0) count. Of. Zeros++; } } } finally { fs. Close(); } Console. Write. Line( "The count of zeros in the file is {0}. ", count. Of. Zeros); } }
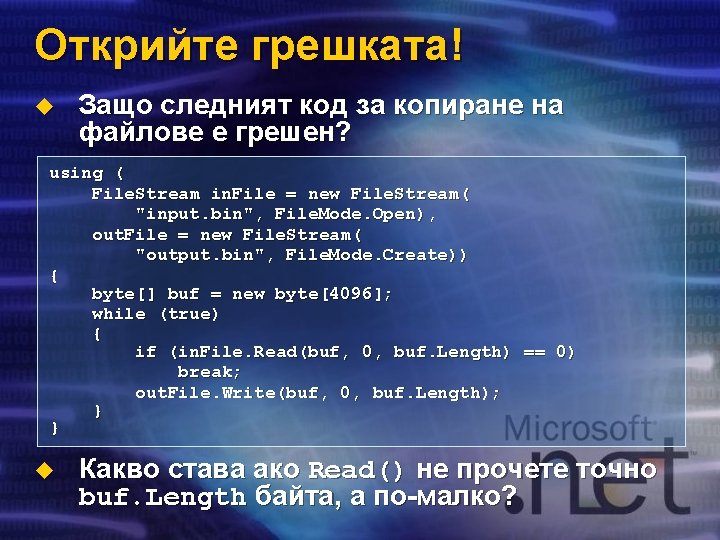
Открийте грешката! u Защо следният код за копиране на файлове е грешен? using ( File. Stream in. File = new File. Stream( "input. bin", File. Mode. Open), out. File = new File. Stream( "output. bin", File. Mode. Create)) { byte[] buf = new byte[4096]; while (true) { if (in. File. Read(buf, 0, buf. Length) == 0) break; out. File. Write(buf, 0, buf. Length); } } u Какво става ако Read() не прочете точно buf. Length байта, а по-малко?
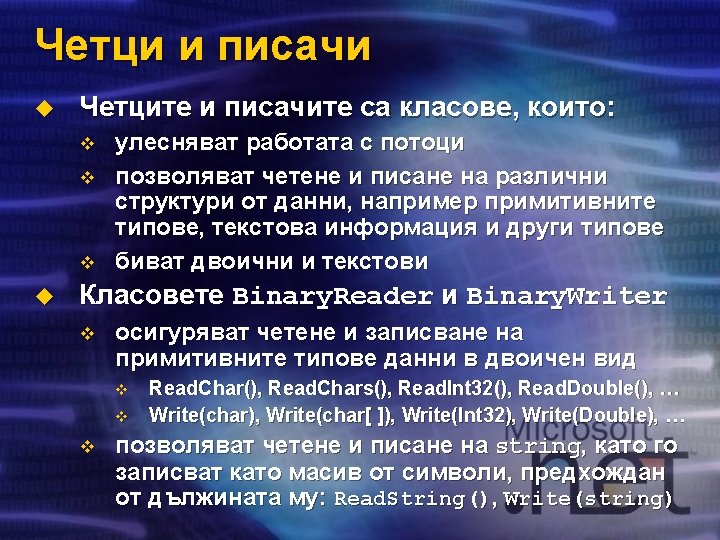
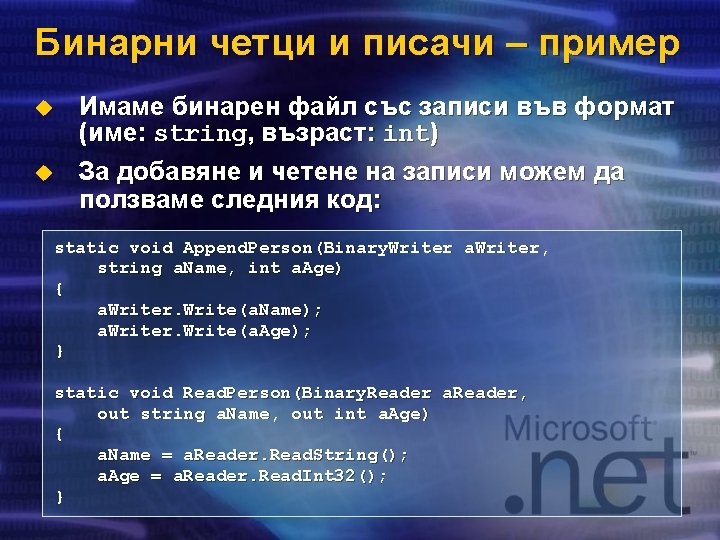
Бинарни четци и писачи – пример u u Имаме бинарен файл със записи във формат (име: string, възраст: int) За добавяне и четене на записи можем да ползваме следния код: static void Append. Person(Binary. Writer a. Writer, string a. Name, int a. Age) { a. Writer. Write(a. Name); a. Writer. Write(a. Age); } static void Read. Person(Binary. Reader a. Reader, out string a. Name, out int a. Age) { a. Name = a. Reader. Read. String(); a. Age = a. Reader. Read. Int 32(); }
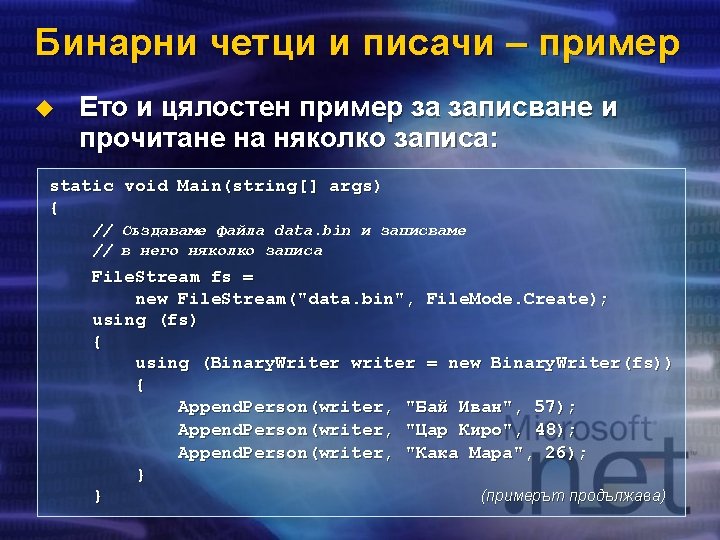
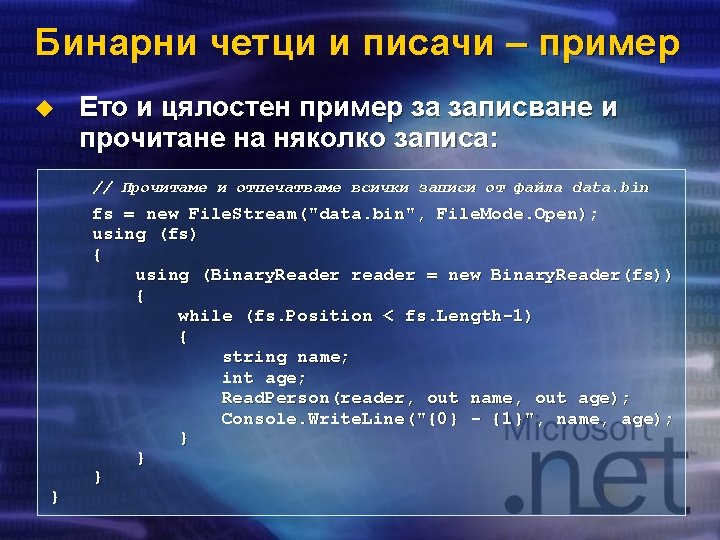
Бинарни четци и писачи – пример u Ето и цялостен пример за записване и прочитане на няколко записа: // Прочитаме и отпечатваме всички записи от файла data. bin } fs = new File. Stream("data. bin", File. Mode. Open); using (fs) { using (Binary. Reader reader = new Binary. Reader(fs)) { while (fs. Position < fs. Length-1) { string name; int age; Read. Person(reader, out name, out age); Console. Write. Line("{0} - {1}", name, age); } } }
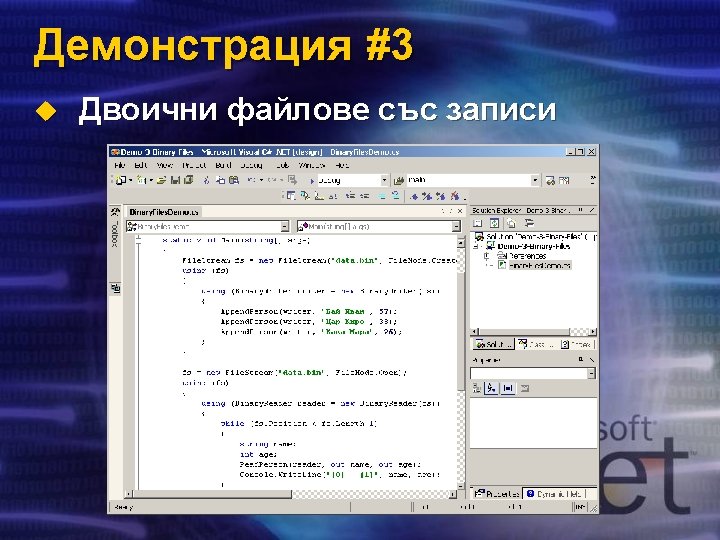
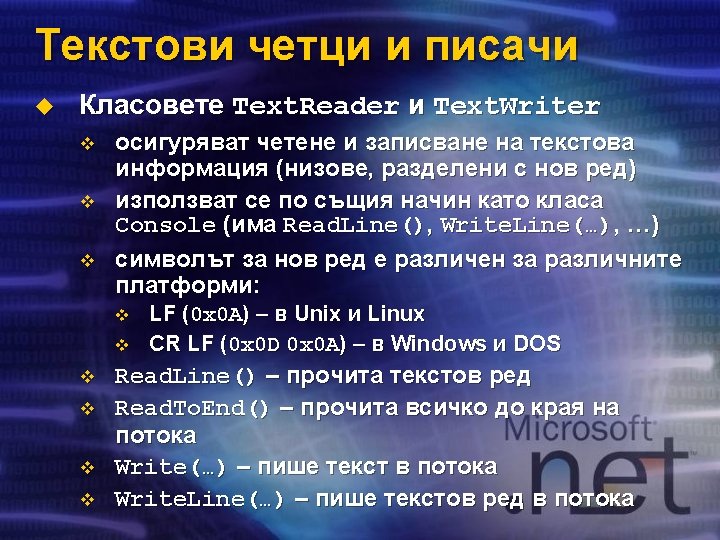
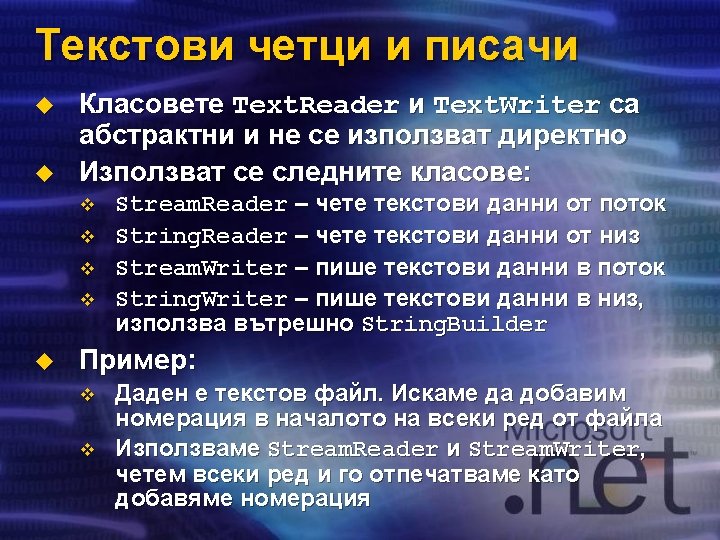
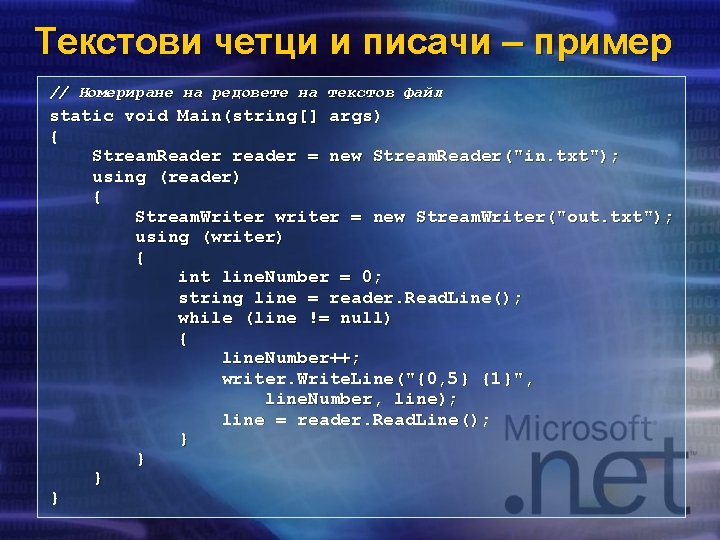
Текстови четци и писачи – пример // Номериране на редовете на текстов файл static void Main(string[] args) { Stream. Reader reader = new Stream. Reader("in. txt"); using (reader) { Stream. Writer writer = new Stream. Writer("out. txt"); using (writer) { int line. Number = 0; string line = reader. Read. Line(); while (line != null) { line. Number++; writer. Write. Line("{0, 5} {1}", line. Number, line); line = reader. Read. Line(); } }
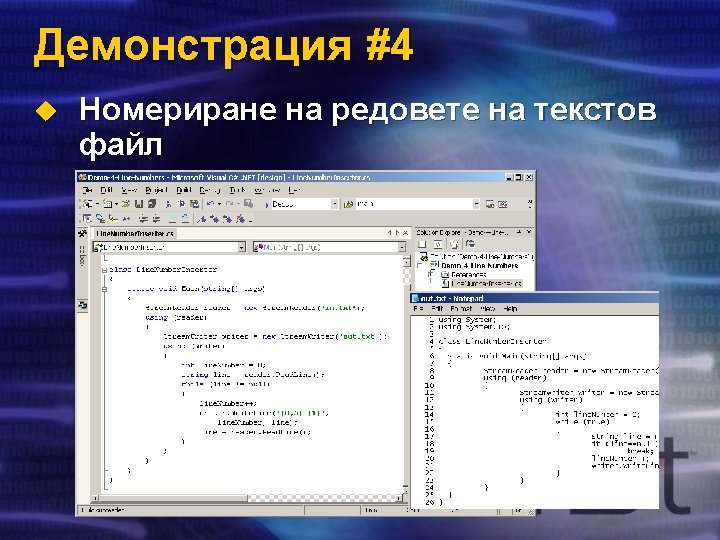
![Текстови четци и писачи пример Findtextinfile Search Utility static void Mainstring args Текстови четци и писачи – пример // Find-text-in-file Search Utility static void Main(string[] args)](https://slidetodoc.com/presentation_image_h2/ca5116a0ee53f2b45385e4f2df6fd6b1/image-33.jpg)
Текстови четци и писачи – пример // Find-text-in-file Search Utility static void Main(string[] args) { if (args. Length < 2) { Console. Write. Line("Use: Find. In. File file text"); return; } string file. Name = args[0]; if (!File. Exists(file. Name)) { Console. Write. Line( "Could not find file: " + file. Name); return; } string text. To. Find = args[1]. Trim(); (примерът продължава)
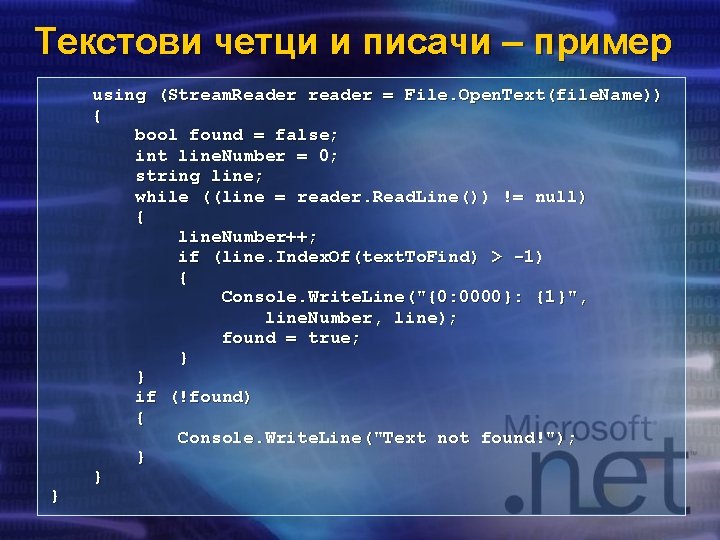
Текстови четци и писачи – пример } using (Stream. Reader reader = File. Open. Text(file. Name)) { bool found = false; int line. Number = 0; string line; while ((line = reader. Read. Line()) != null) { line. Number++; if (line. Index. Of(text. To. Find) > -1) { Console. Write. Line("{0: 0000}: {1}", line. Number, line); found = true; } } if (!found) { Console. Write. Line("Text not found!"); } }
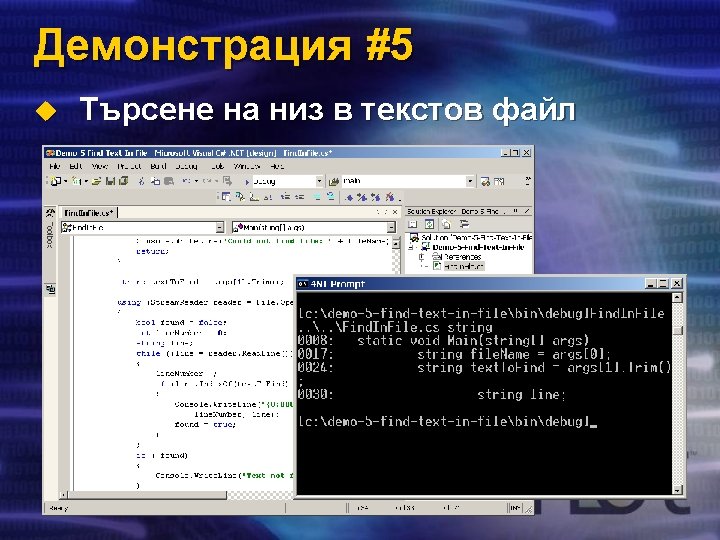
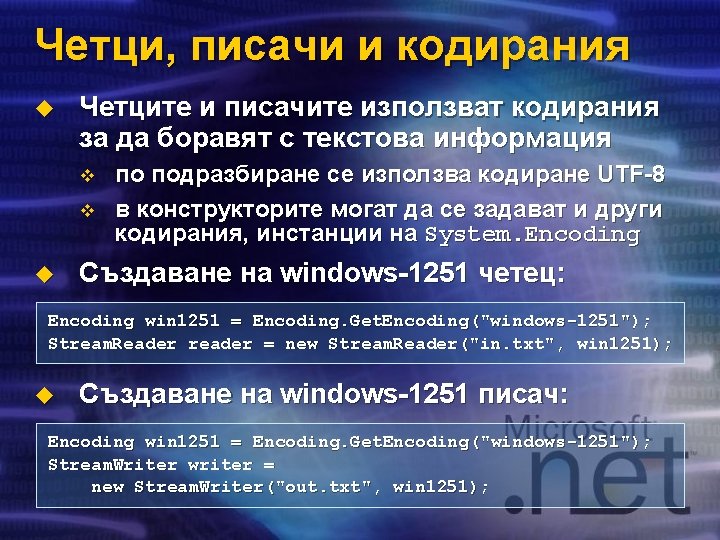
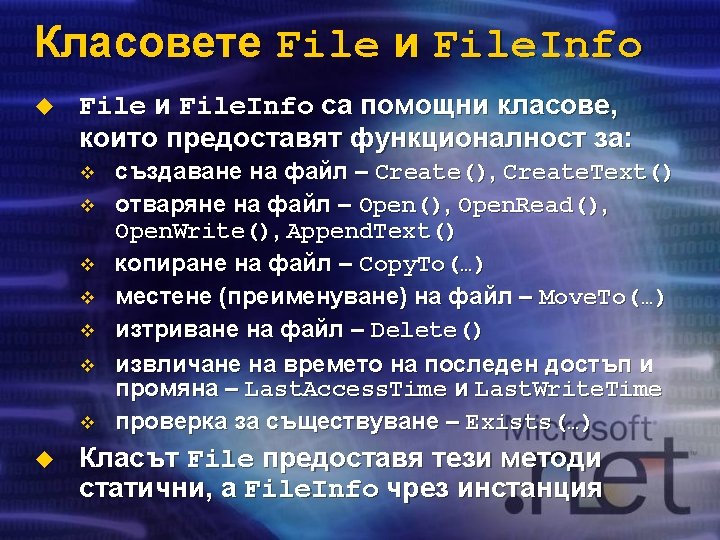
![File и File Info пример static void Mainstring args Stream Writer writer File и File. Info – пример static void Main(string[] args) { Stream. Writer writer](https://slidetodoc.com/presentation_image_h2/ca5116a0ee53f2b45385e4f2df6fd6b1/image-38.jpg)
File и File. Info – пример static void Main(string[] args) { Stream. Writer writer = File. Create. Text("test 1. txt"); using (writer) { writer. Write. Line("Налей ми бира!"); } File. Info file. Info = new File. Info("test 1. txt"); file. Info. Copy. To("test 2. txt", true); file. Info. Copy. To("test 3. txt", true); if (File. Exists("test 4. txt")) { File. Delete("test 4. txt"); } File. Move("test 3. txt", "test 4. txt"); }
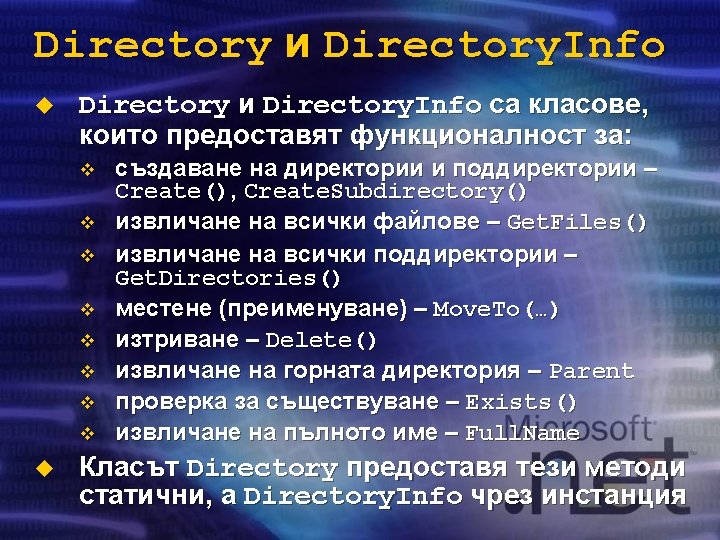
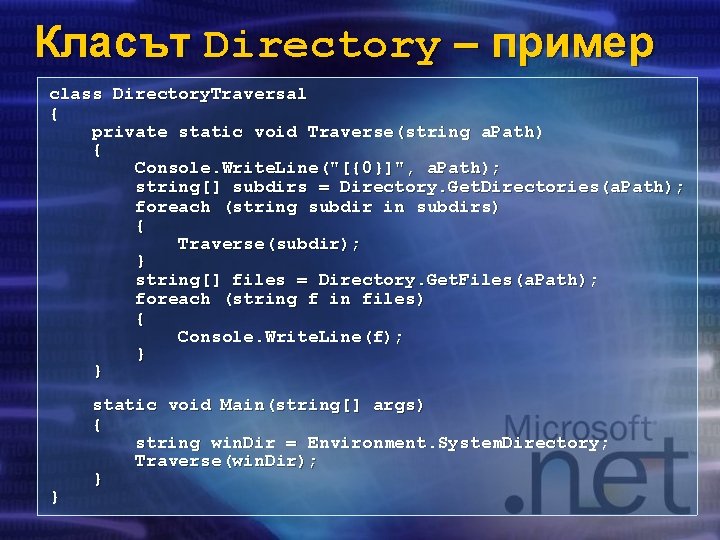
Класът Directory – пример class Directory. Traversal { private static void Traverse(string a. Path) { Console. Write. Line("[{0}]", a. Path); string[] subdirs = Directory. Get. Directories(a. Path); foreach (string subdir in subdirs) { Traverse(subdir); } string[] files = Directory. Get. Files(a. Path); foreach (string f in files) { Console. Write. Line(f); } } } static void Main(string[] args) { string win. Dir = Environment. System. Directory; Traverse(win. Dir); }
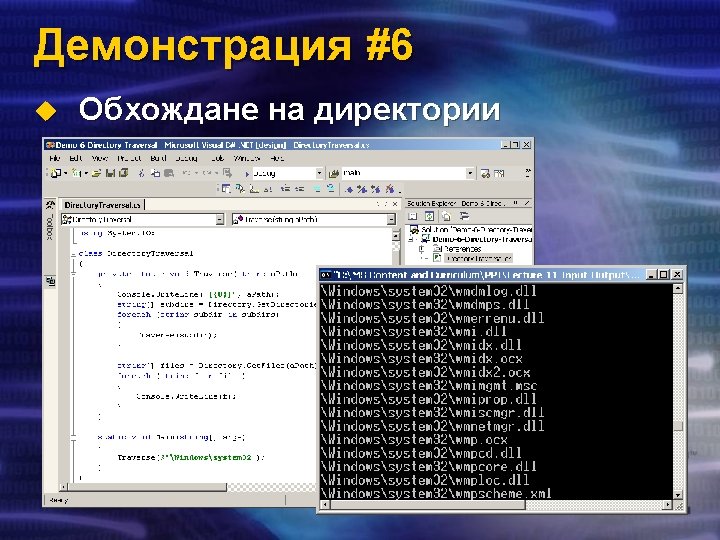
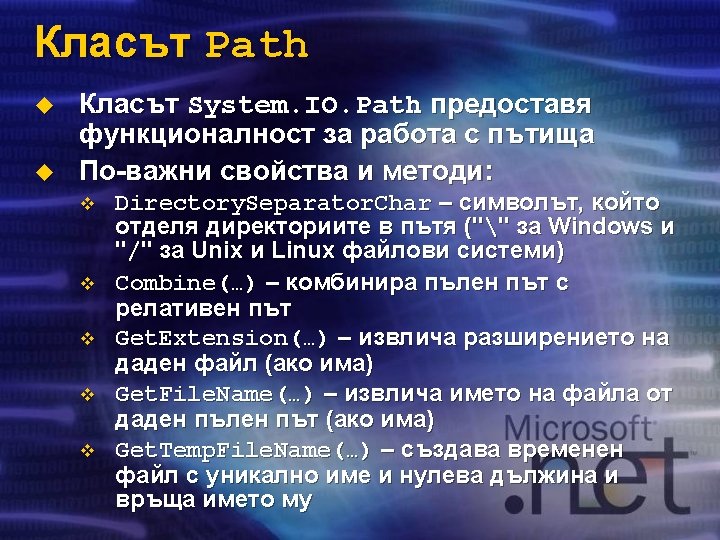
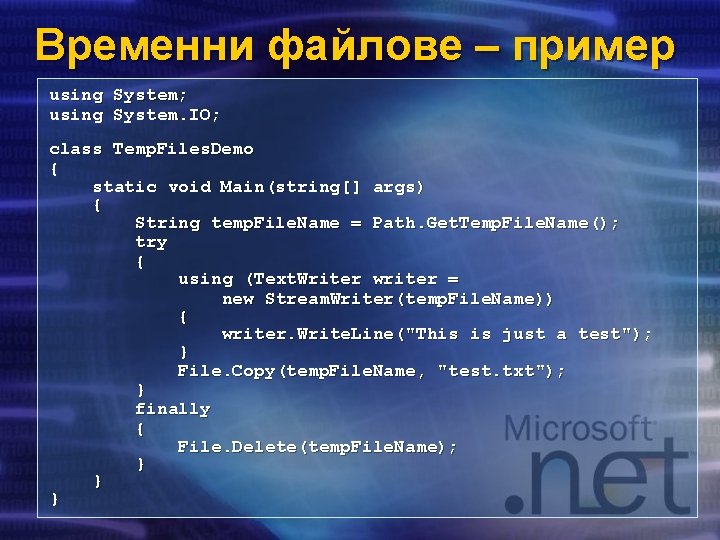
Временни файлове – пример using System; using System. IO; class Temp. Files. Demo { static void Main(string[] args) { String temp. File. Name = Path. Get. Temp. File. Name(); try { using (Text. Writer writer = new Stream. Writer(temp. File. Name)) { writer. Write. Line("This is just a test"); } File. Copy(temp. File. Name, "test. txt"); } finally { File. Delete(temp. File. Name); } } }
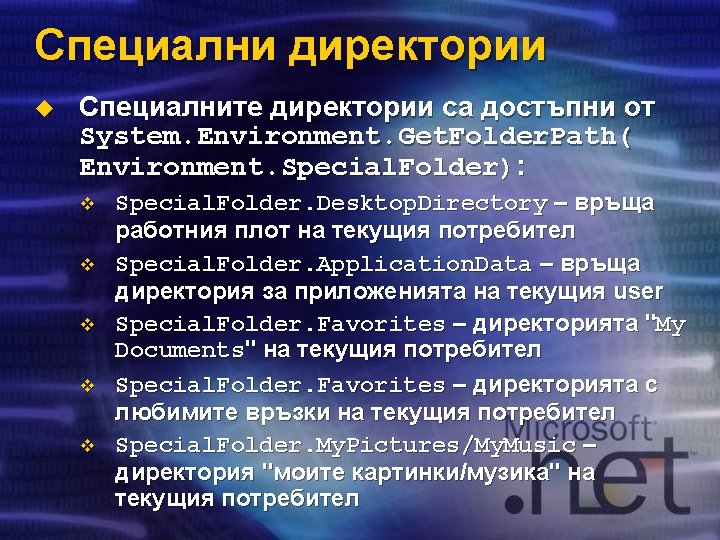
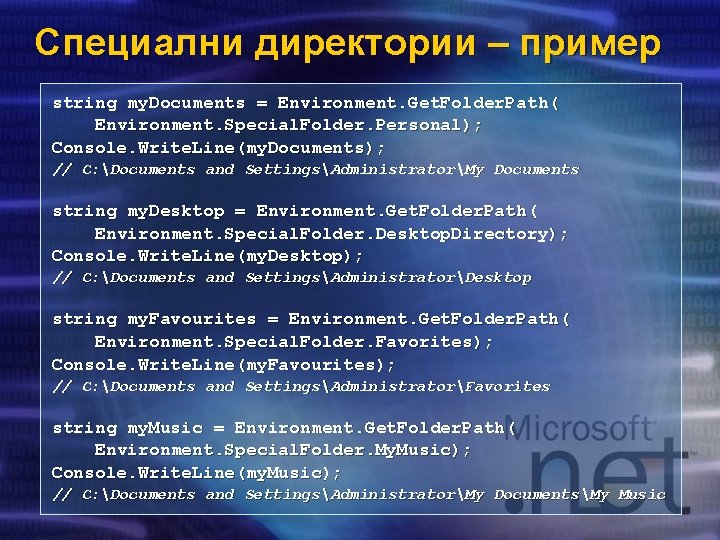
Специални директории – пример string my. Documents = Environment. Get. Folder. Path( Environment. Special. Folder. Personal); Console. Write. Line(my. Documents); // C: Documents and SettingsAdministratorMy Documents string my. Desktop = Environment. Get. Folder. Path( Environment. Special. Folder. Desktop. Directory); Console. Write. Line(my. Desktop); // C: Documents and SettingsAdministratorDesktop string my. Favourites = Environment. Get. Folder. Path( Environment. Special. Folder. Favorites); Console. Write. Line(my. Favourites); // C: Documents and SettingsAdministratorFavorites string my. Music = Environment. Get. Folder. Path( Environment. Special. Folder. My. Music); Console. Write. Line(my. Music); // C: Documents and SettingsAdministratorMy DocumentsMy Music
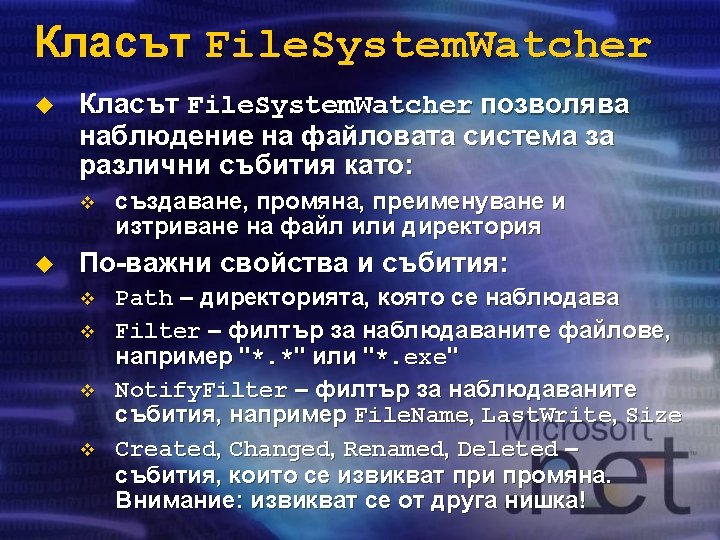
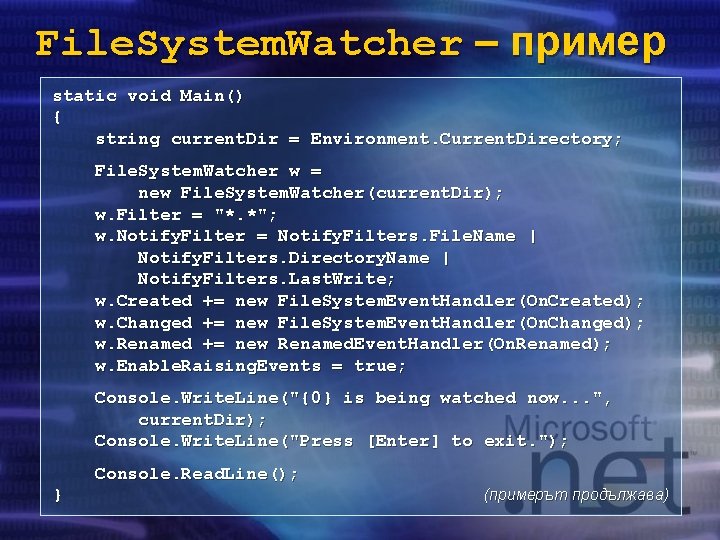
File. System. Watcher – пример static void Main() { string current. Dir = Environment. Current. Directory; File. System. Watcher w = new File. System. Watcher(current. Dir); w. Filter = "*. *"; w. Notify. Filter = Notify. Filters. File. Name | Notify. Filters. Directory. Name | Notify. Filters. Last. Write; w. Created += new File. System. Event. Handler(On. Created); w. Changed += new File. System. Event. Handler(On. Changed); w. Renamed += new Renamed. Event. Handler(On. Renamed); w. Enable. Raising. Events = true; Console. Write. Line("{0} is being watched now. . . ", current. Dir); Console. Write. Line("Press [Enter] to exit. "); Console. Read. Line(); } (примерът продължава)
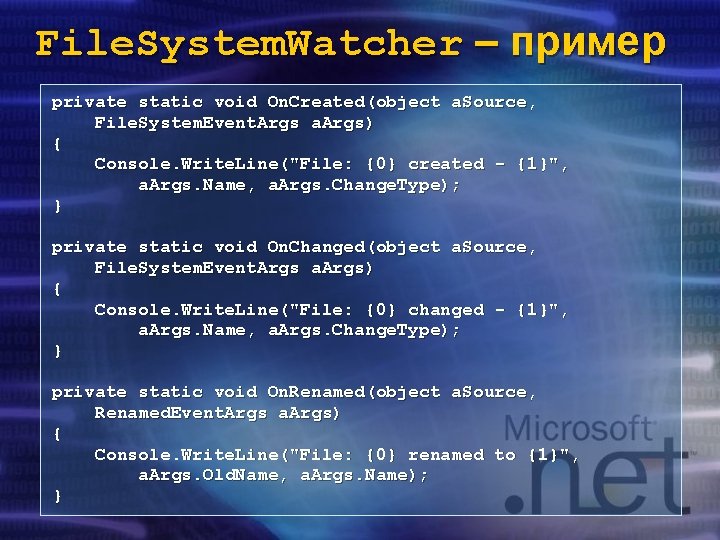
File. System. Watcher – пример private static void On. Created(object a. Source, File. System. Event. Args a. Args) { Console. Write. Line("File: {0} created - {1}", a. Args. Name, a. Args. Change. Type); } private static void On. Changed(object a. Source, File. System. Event. Args a. Args) { Console. Write. Line("File: {0} changed - {1}", a. Args. Name, a. Args. Change. Type); } private static void On. Renamed(object a. Source, Renamed. Event. Args a. Args) { Console. Write. Line("File: {0} renamed to {1}", a. Args. Old. Name, a. Args. Name); }
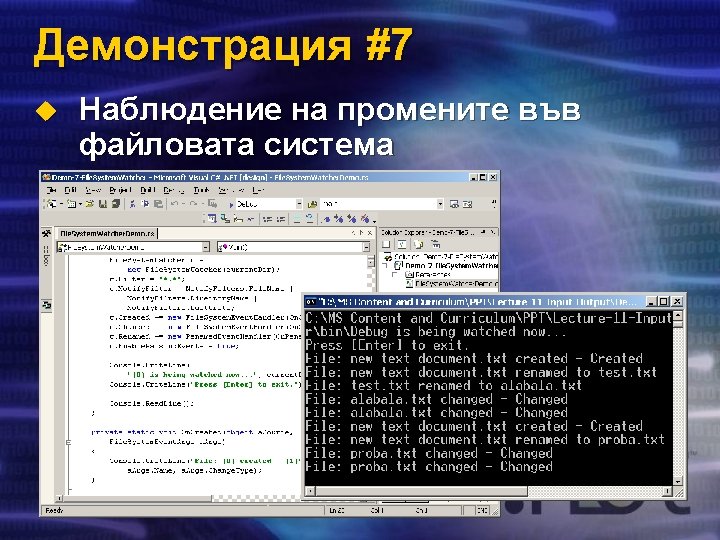
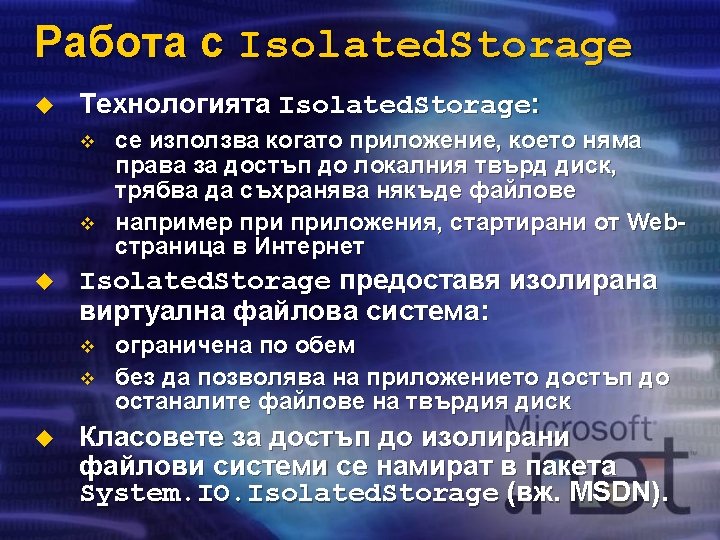
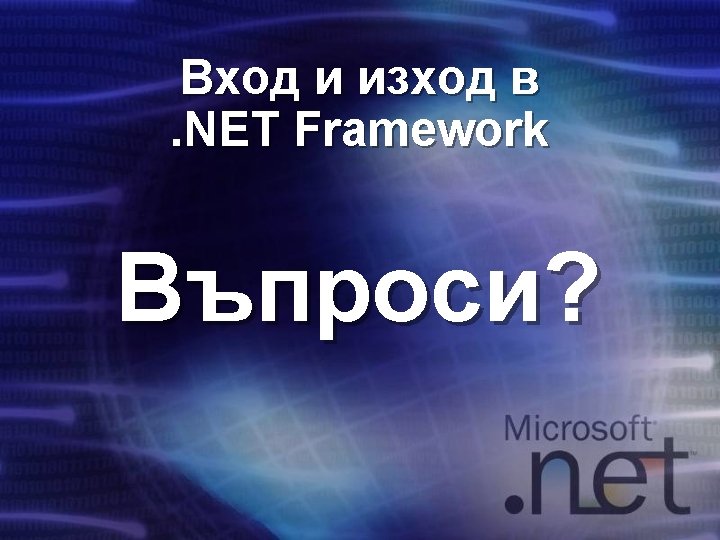
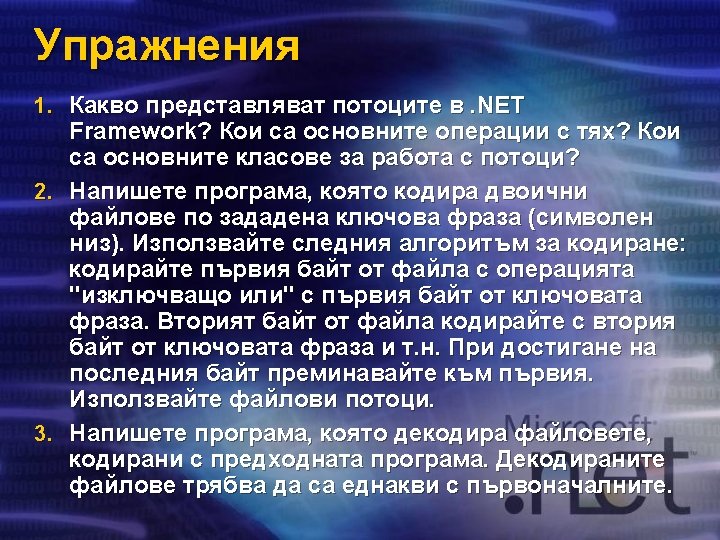
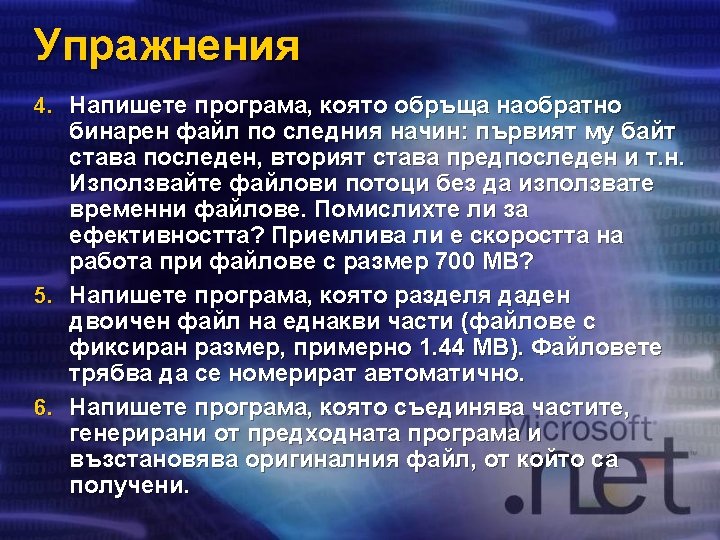
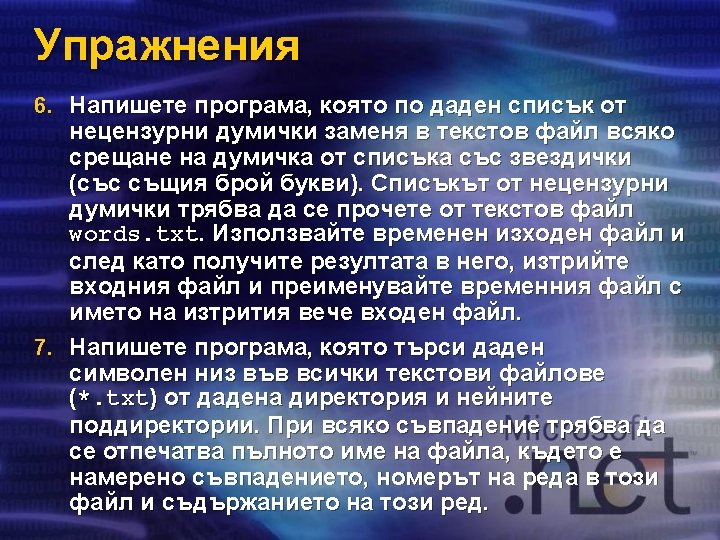
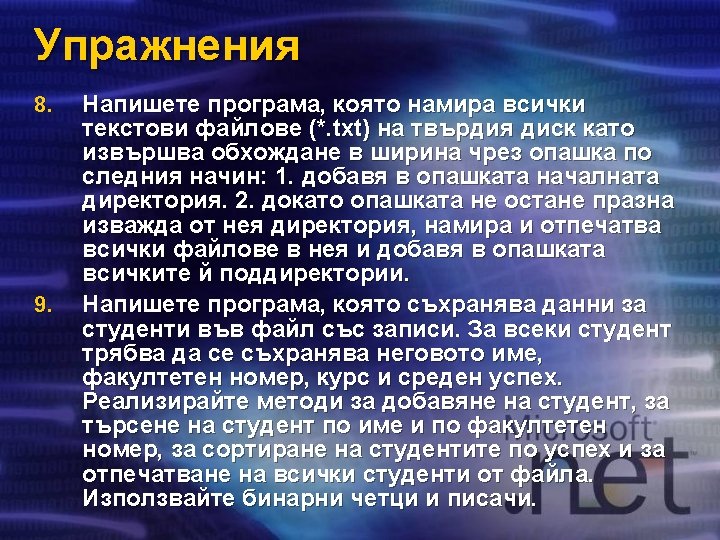
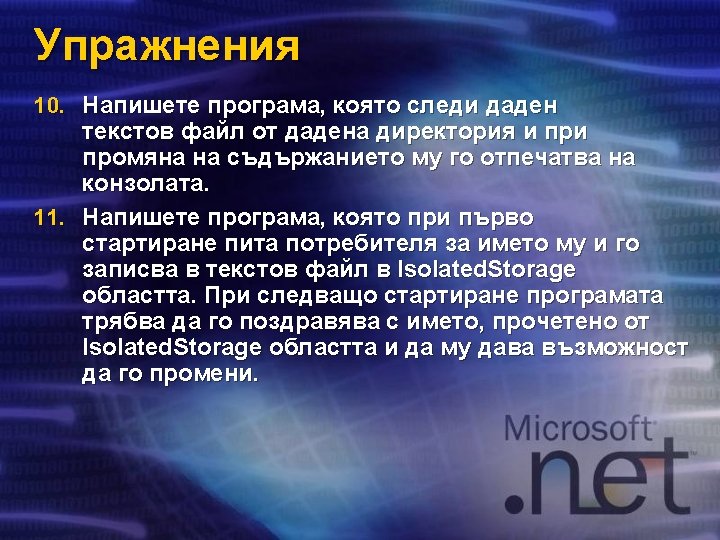
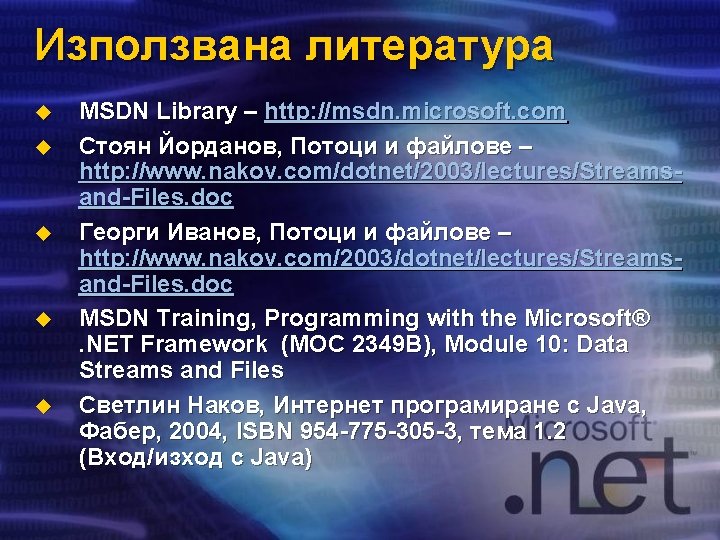
Използвана литература u u u MSDN Library – http: //msdn. microsoft. com Стоян Йорданов, Потоци и файлове – http: //www. nakov. com/dotnet/2003/lectures/Streamsand-Files. doc Георги Иванов, Потоци и файлове – http: //www. nakov. com/2003/dotnet/lectures/Streamsand-Files. doc MSDN Training, Programming with the Microsoft®. NET Framework (MOC 2349 B), Module 10: Data Streams and Files Светлин Наков, Интернет програмиране с Java, Фабер, 2004, ISBN 954 -775 -305 -3, тема 1. 2 (Вход/изход с Java)
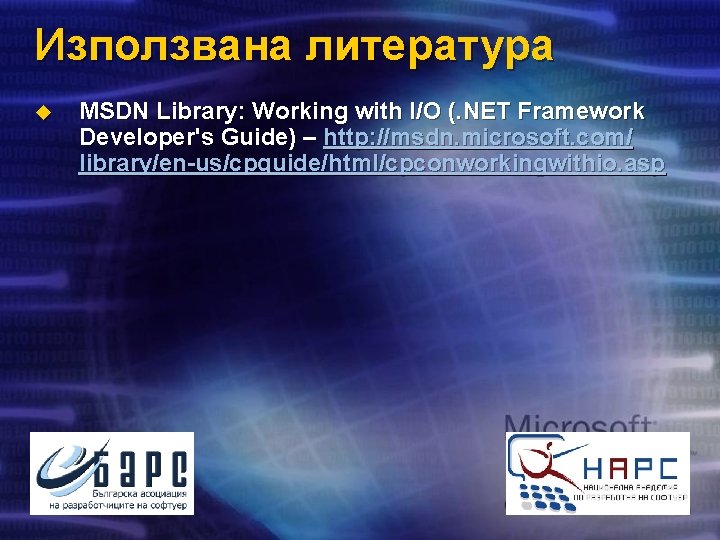
Използвана литература u MSDN Library: Working with I/O (. NET Framework Developer's Guide) – http: //msdn. microsoft. com/ library/en-us/cpguide/html/cpconworkingwithio. asp