Spring Overview Spring is an open source layered
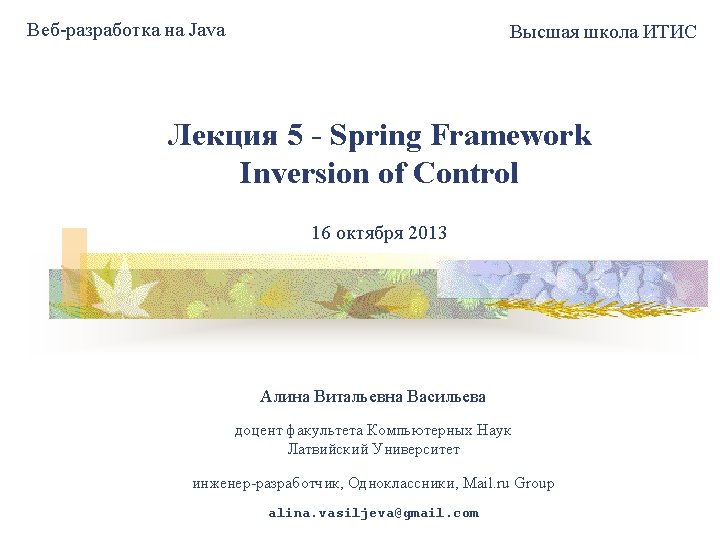
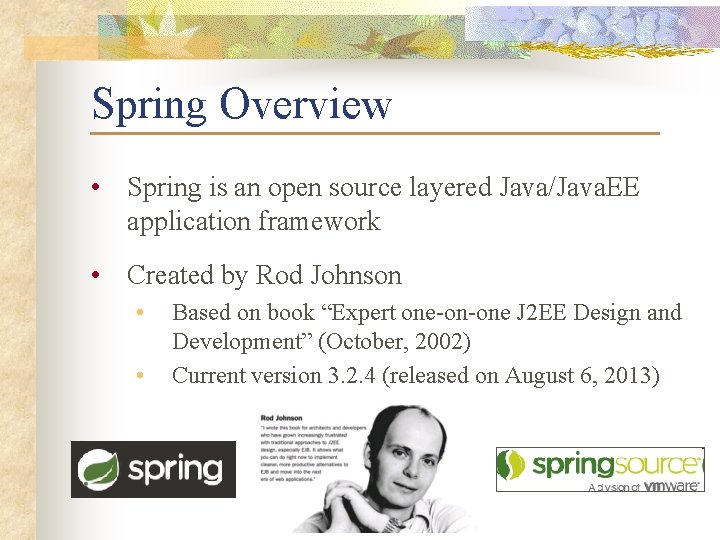
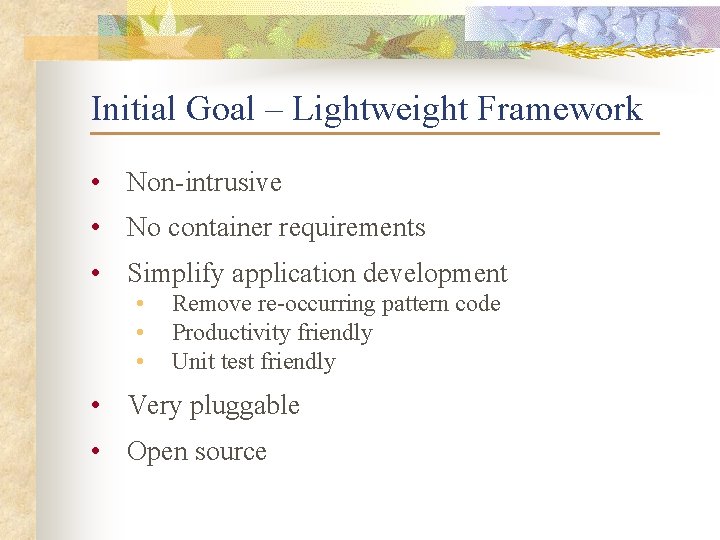
![Spring Mission Statement [1/3] • Java EE should be easier to use • It's Spring Mission Statement [1/3] • Java EE should be easier to use • It's](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-4.jpg)
![Spring Mission Statement [2/3] • Checked exceptions are overused in Java. A framework shouldn't Spring Mission Statement [2/3] • Checked exceptions are overused in Java. A framework shouldn't](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-5.jpg)
![Spring Mission Statement [3/3] • Spring should not compete with good existing solutions, but Spring Mission Statement [3/3] • Spring should not compete with good existing solutions, but](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-6.jpg)
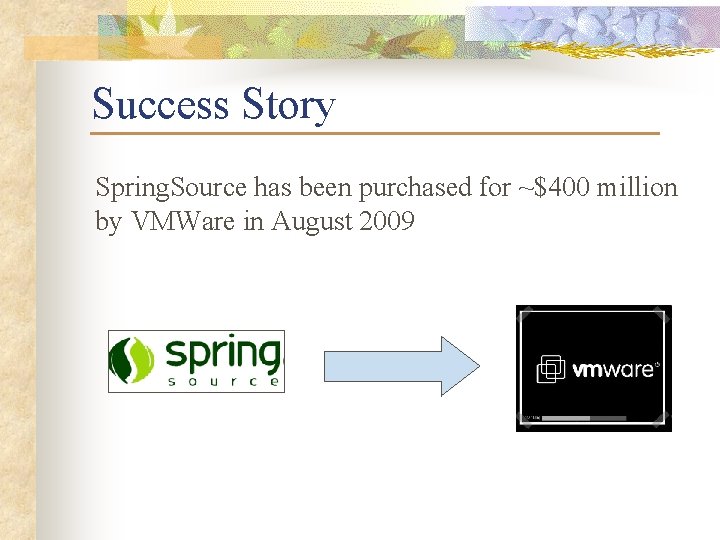
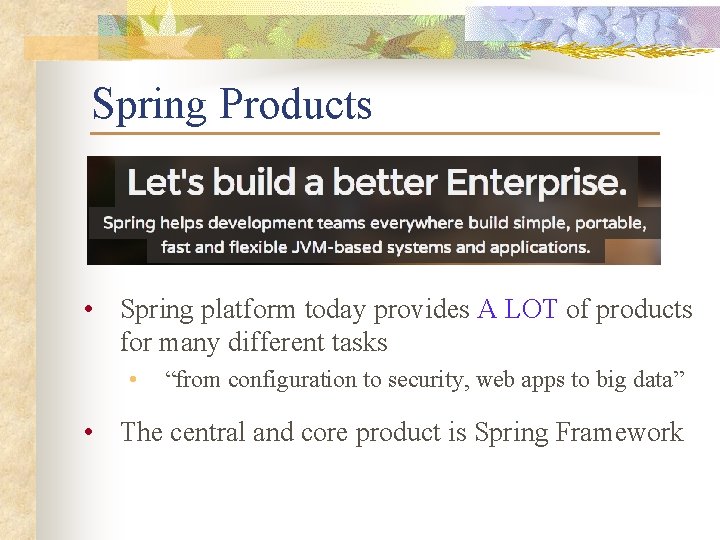
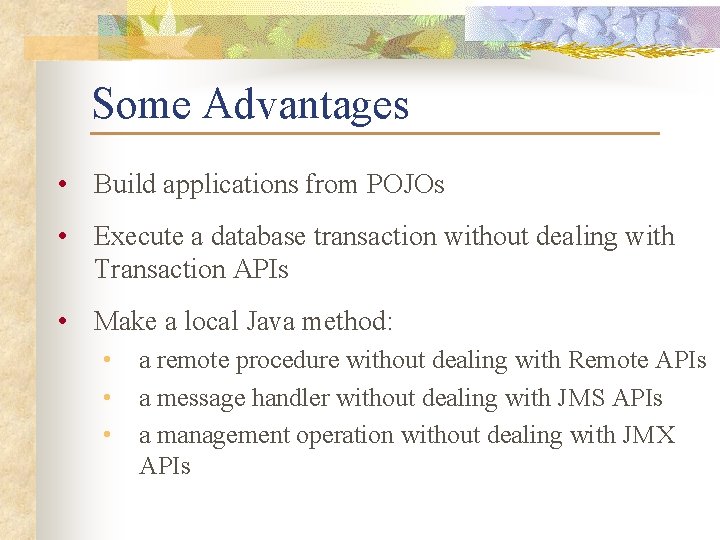
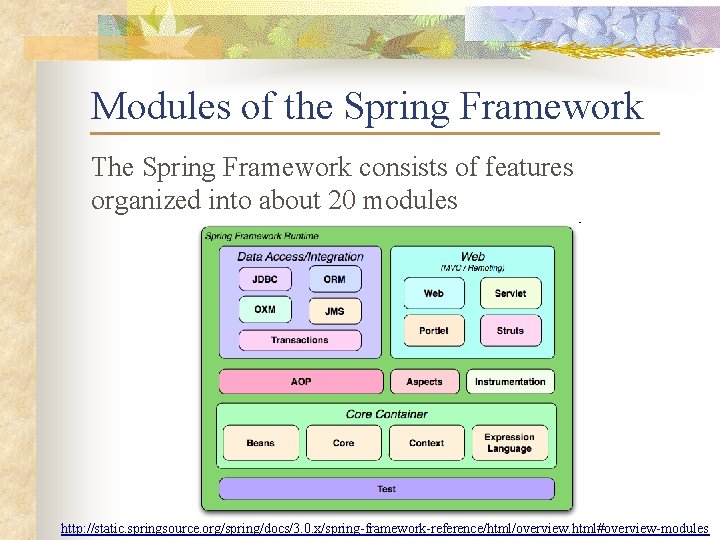
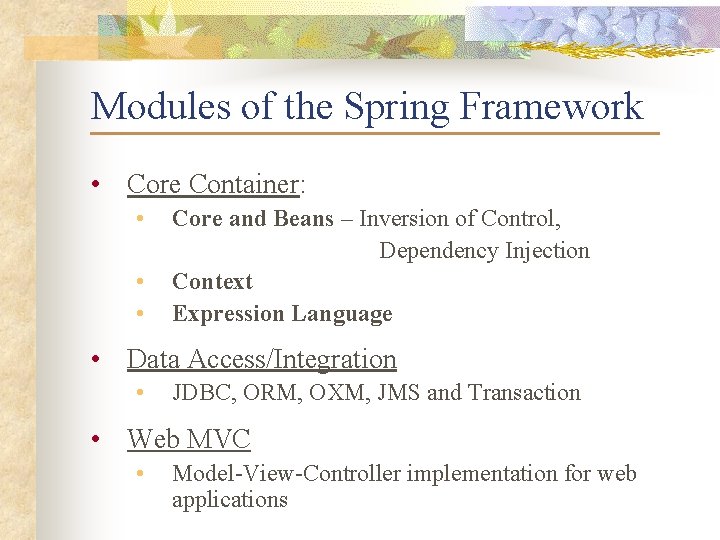
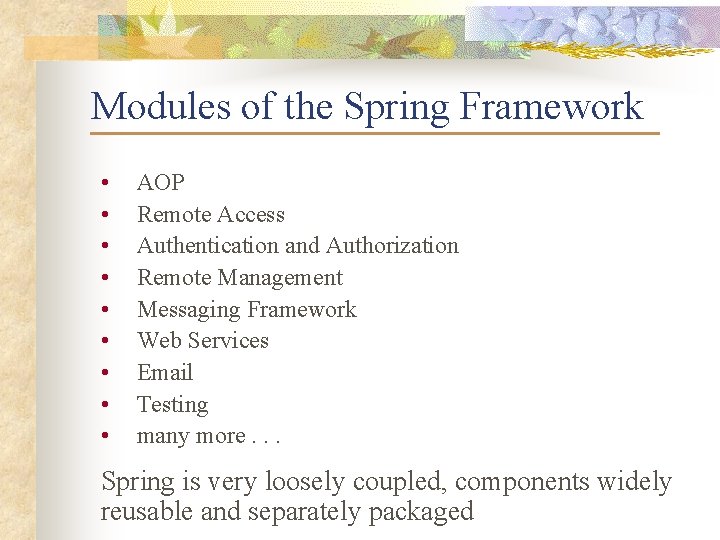
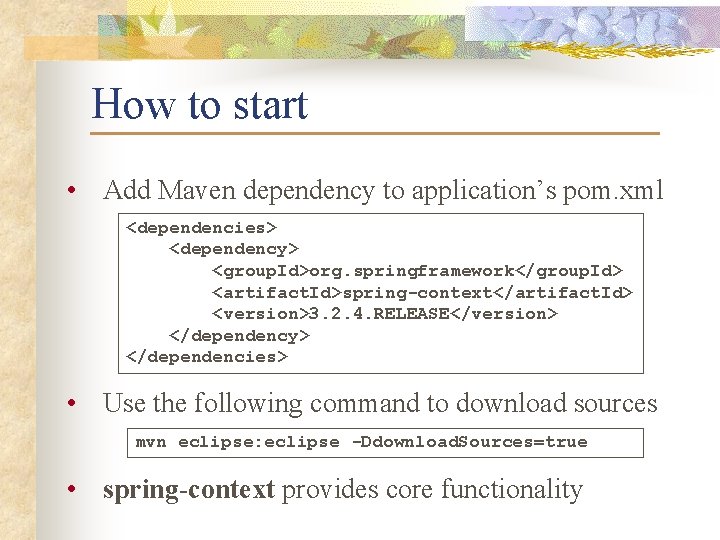
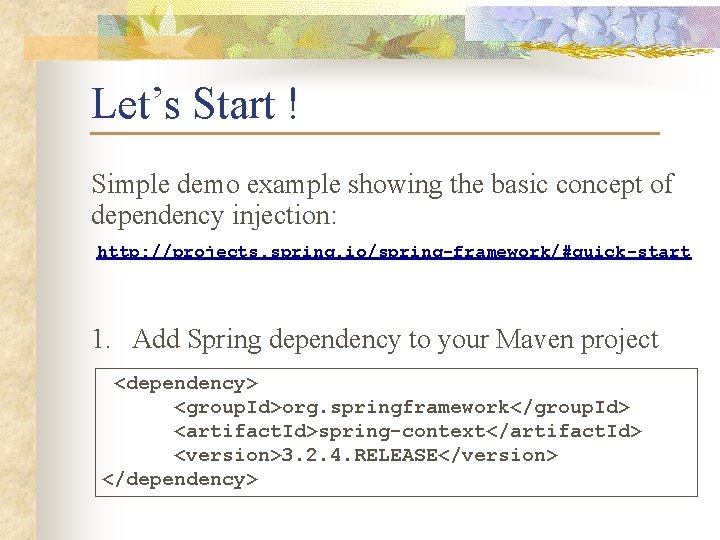
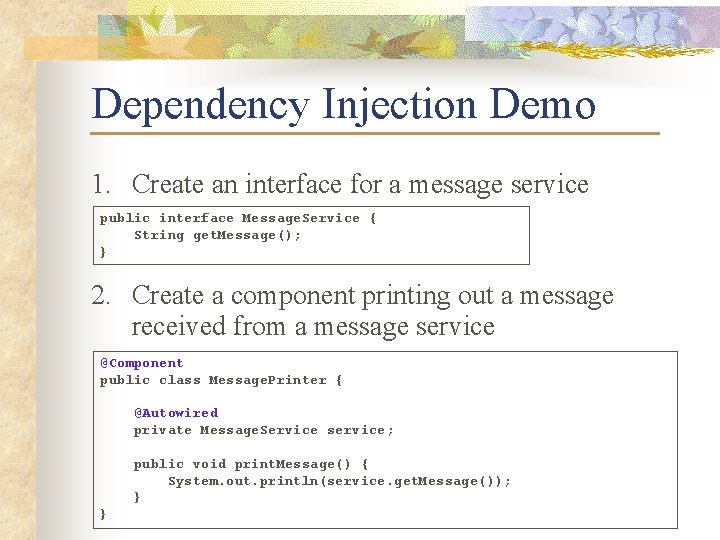
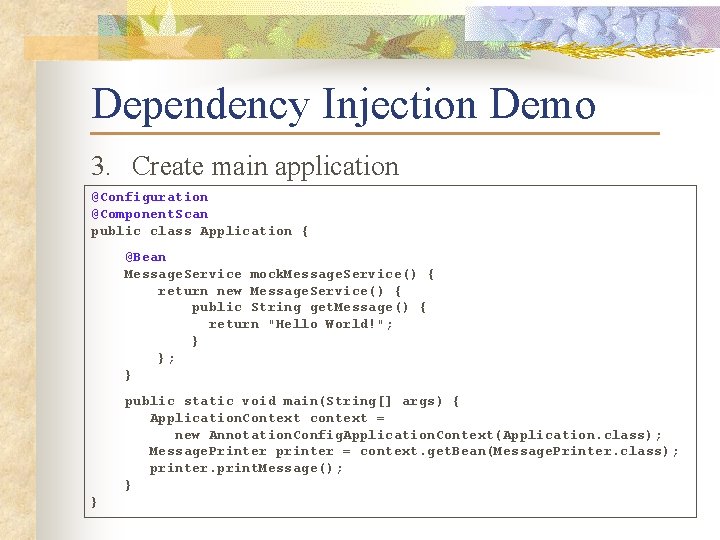
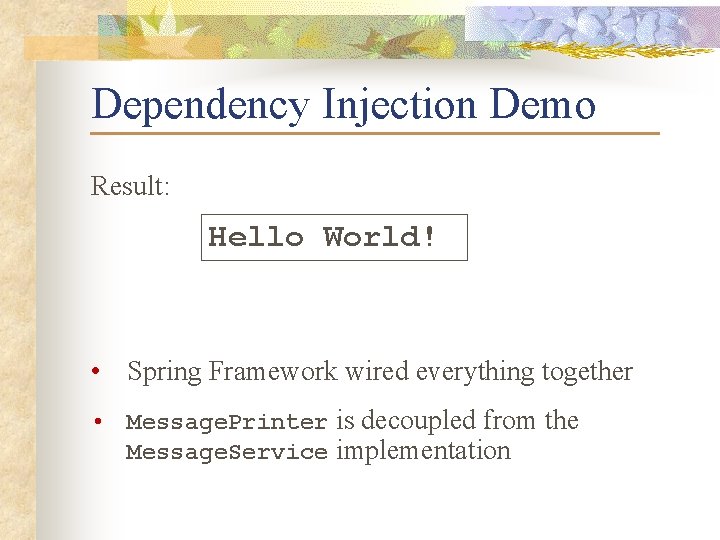
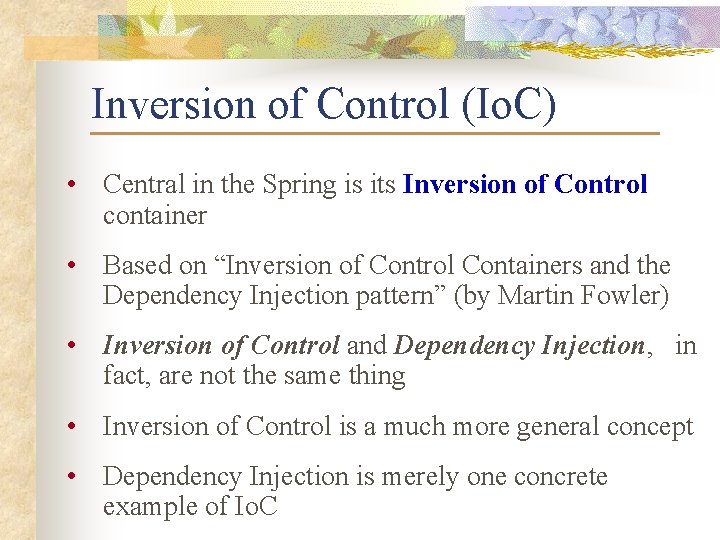
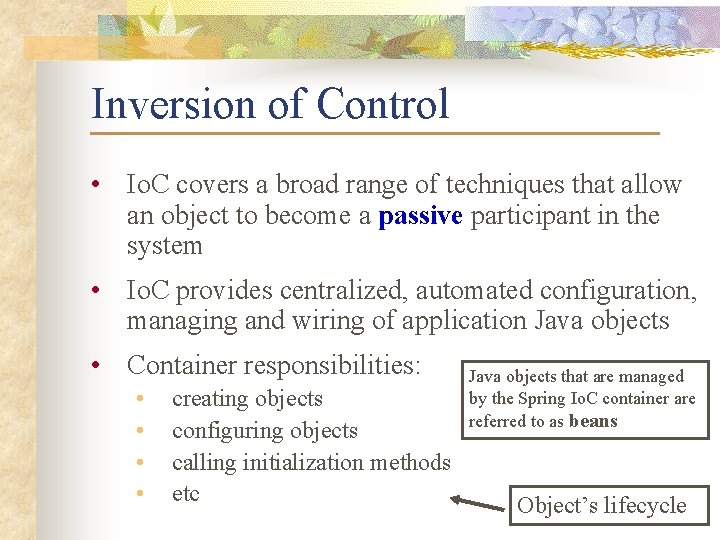
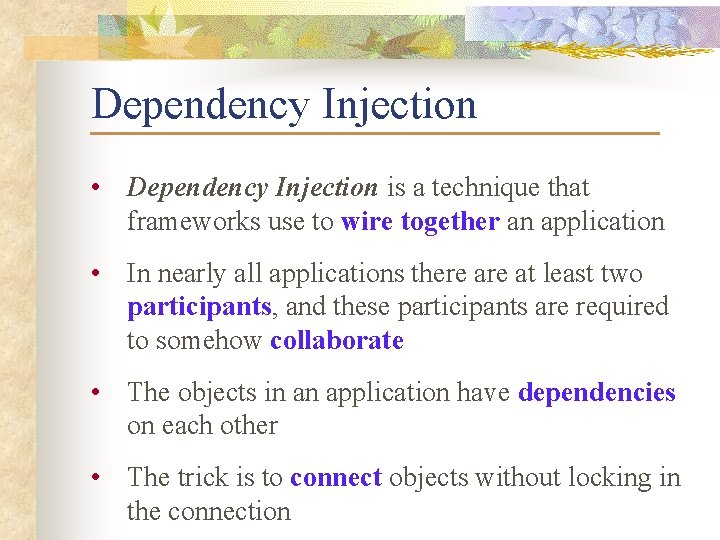
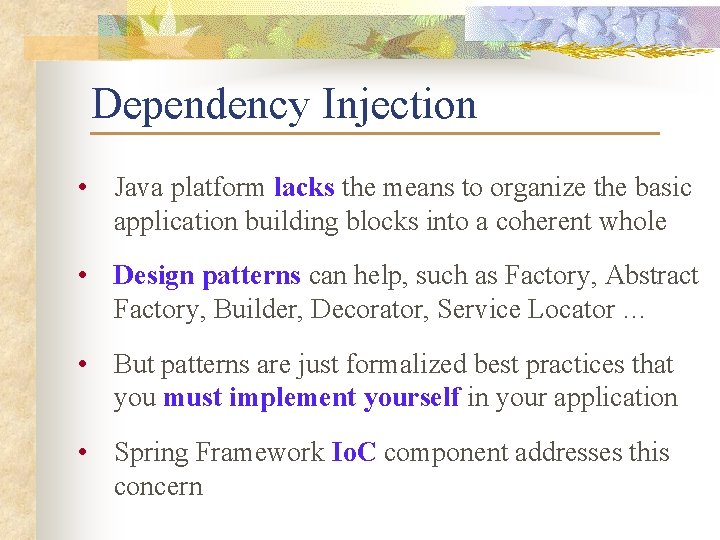
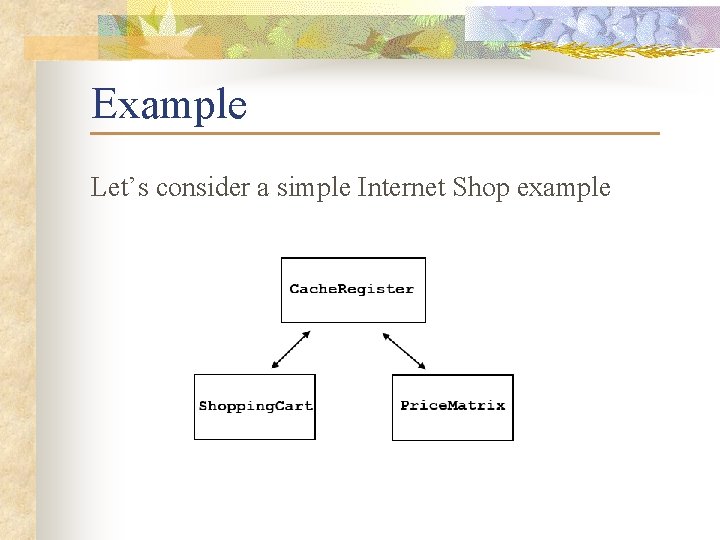
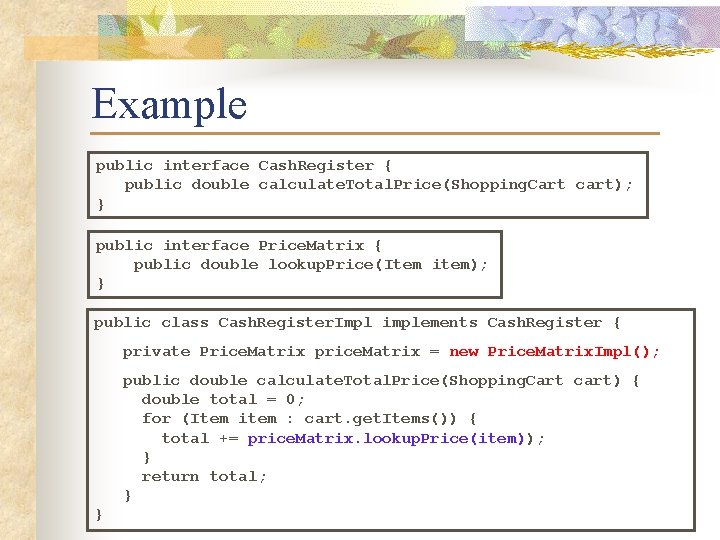
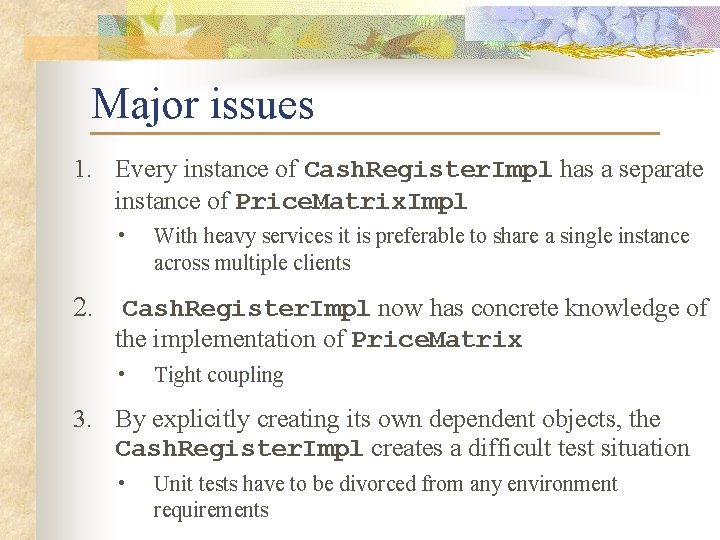
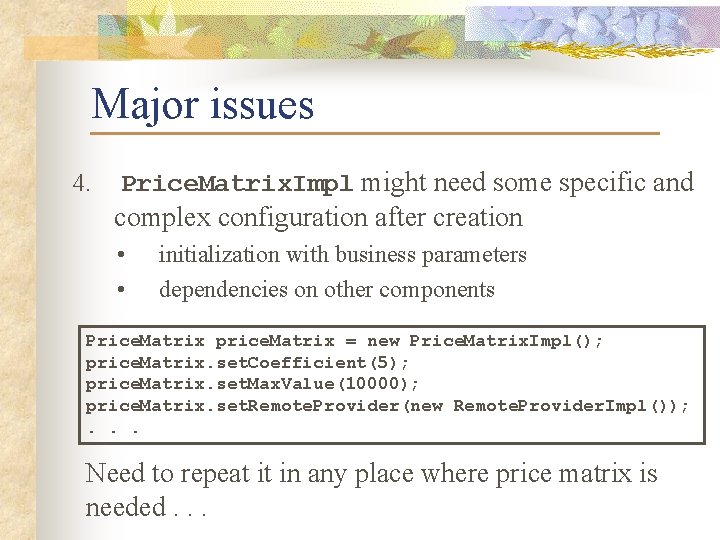
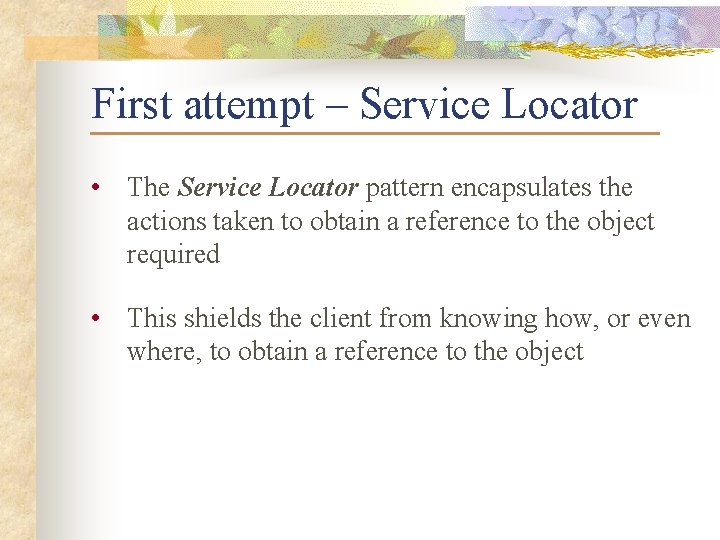
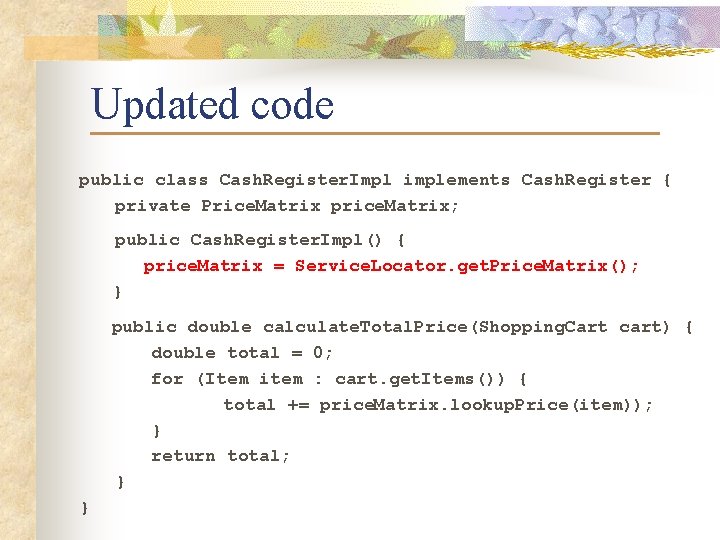
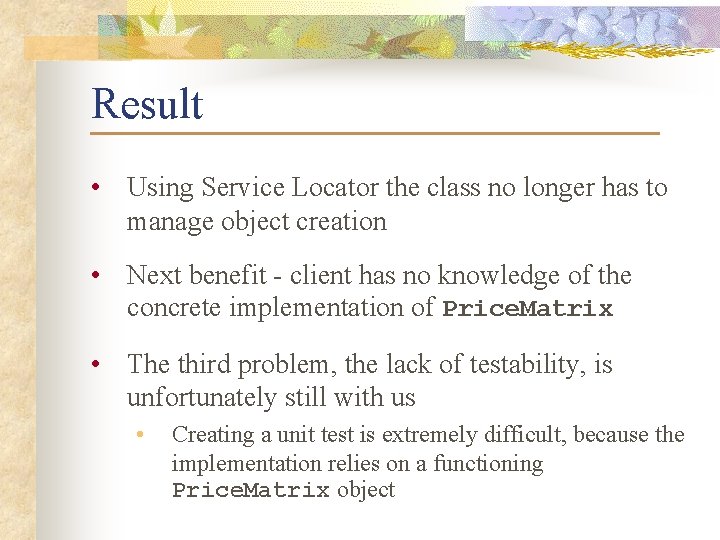
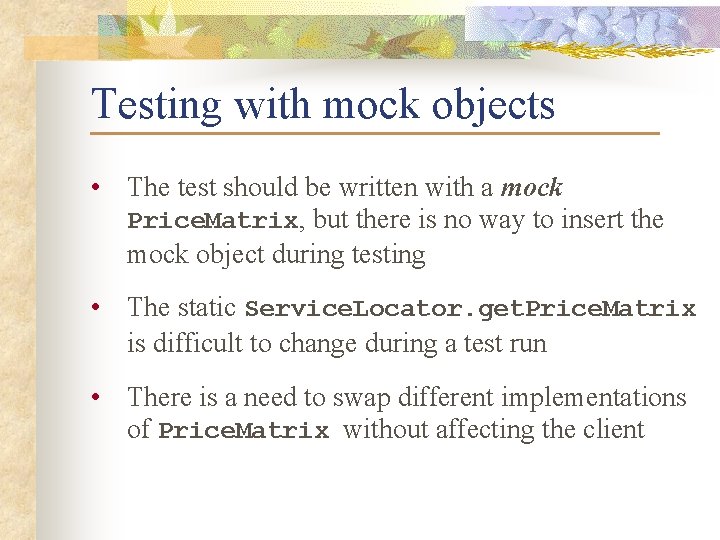
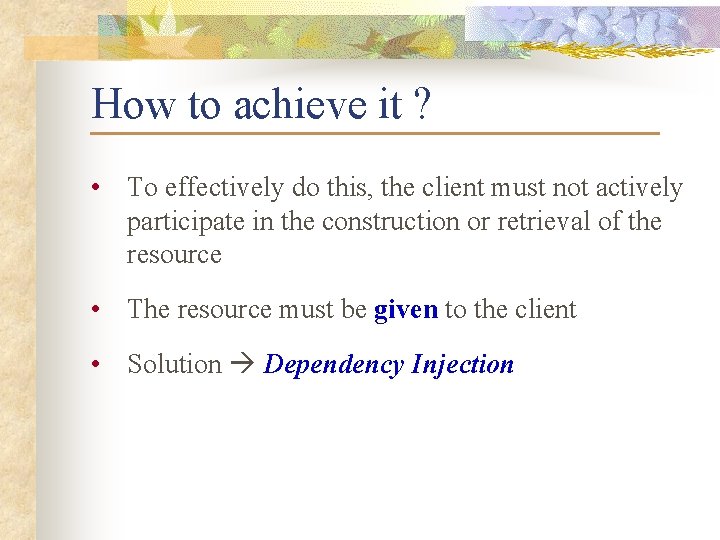
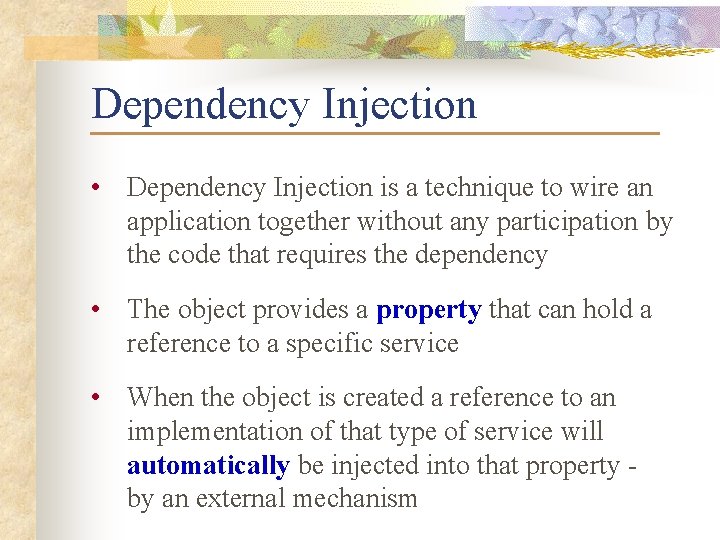
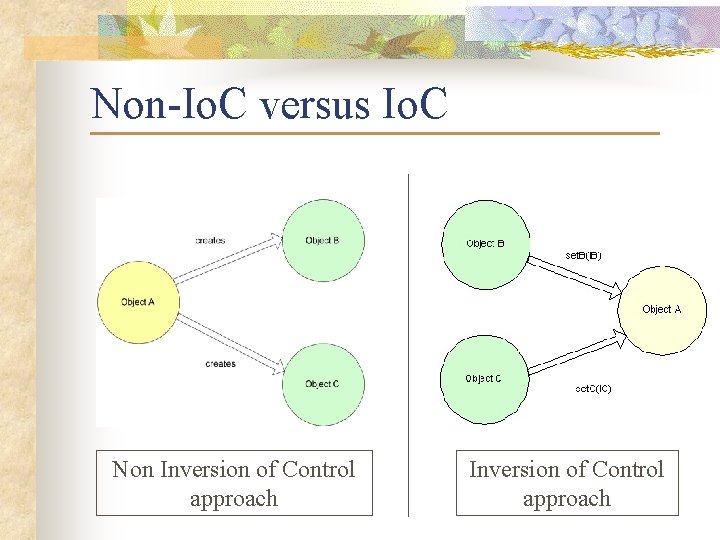
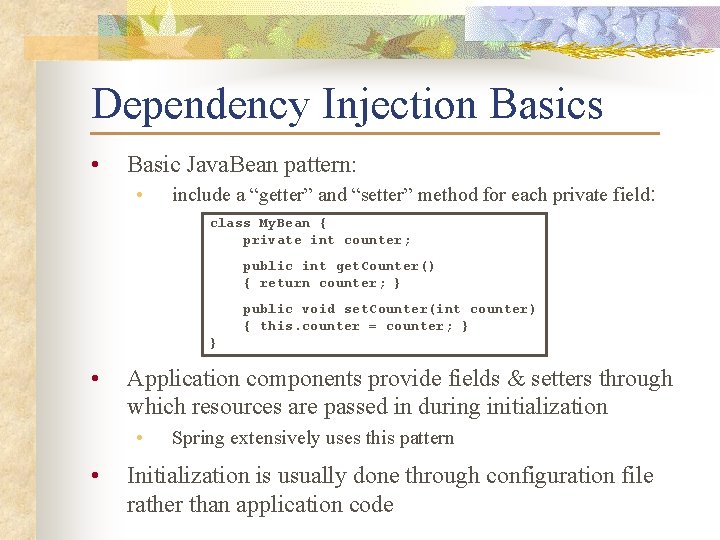
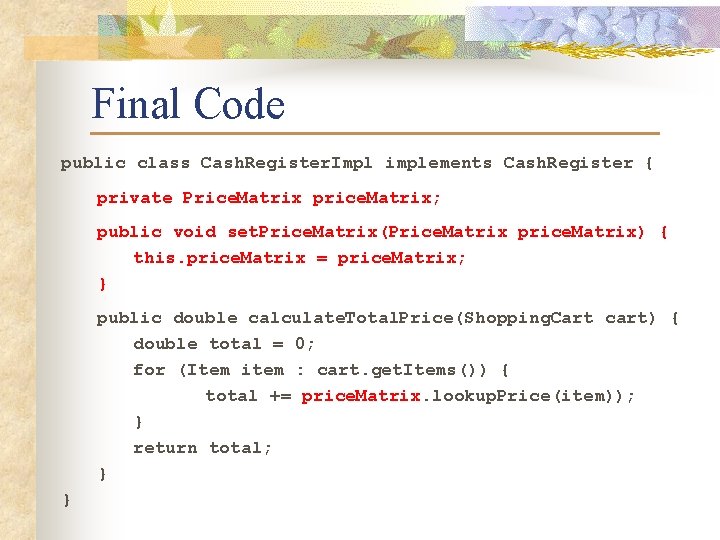
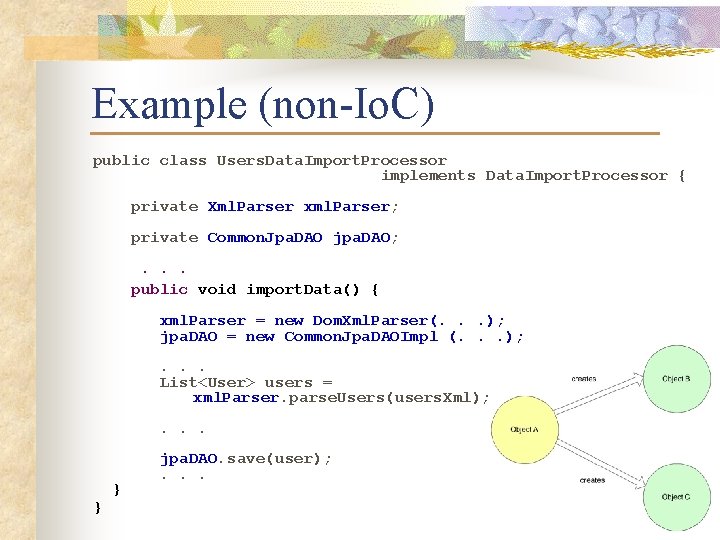
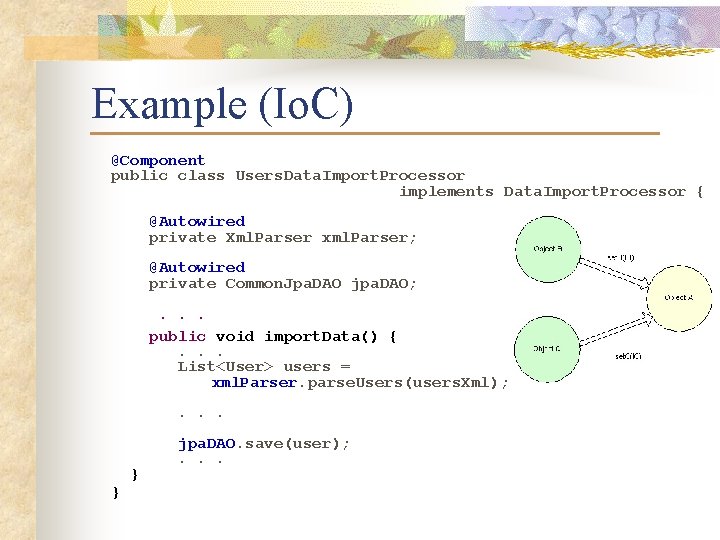
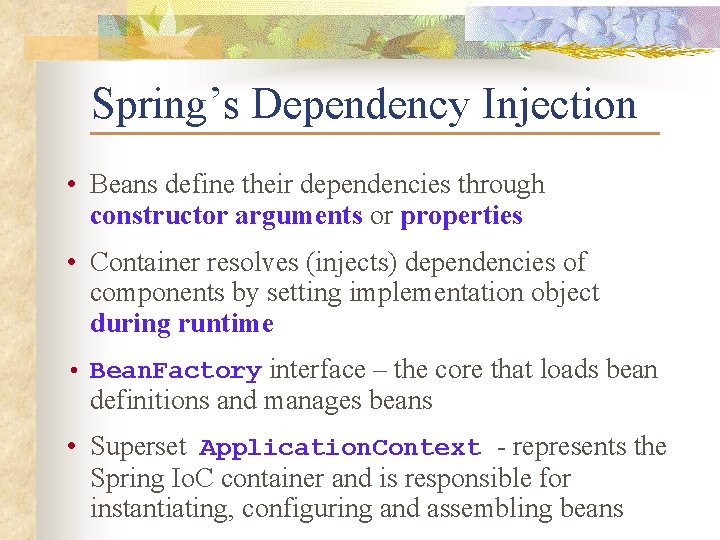
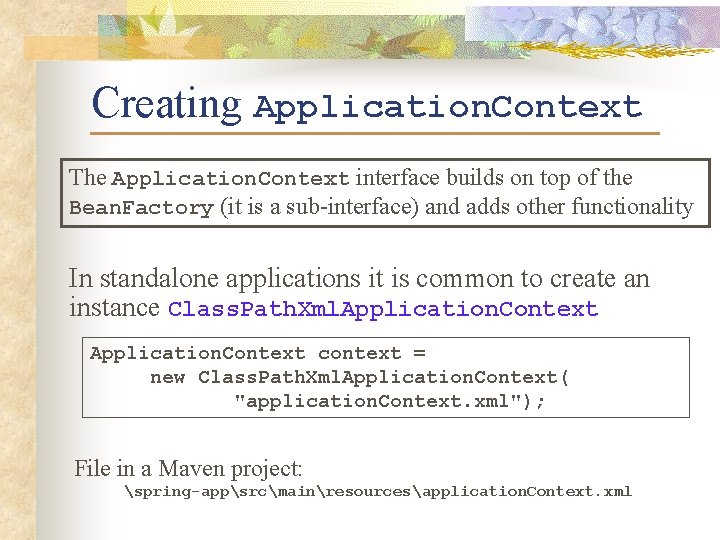
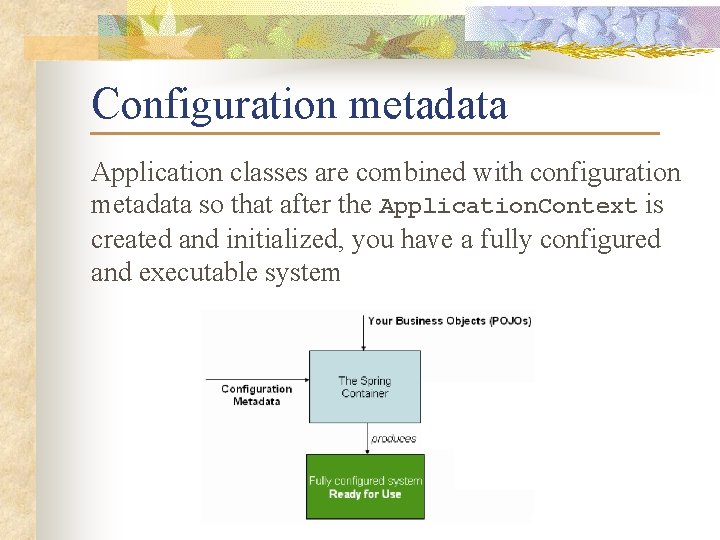
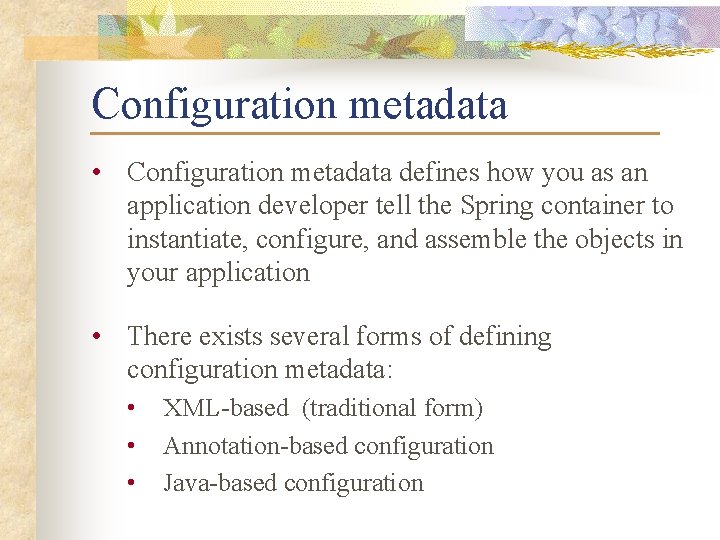
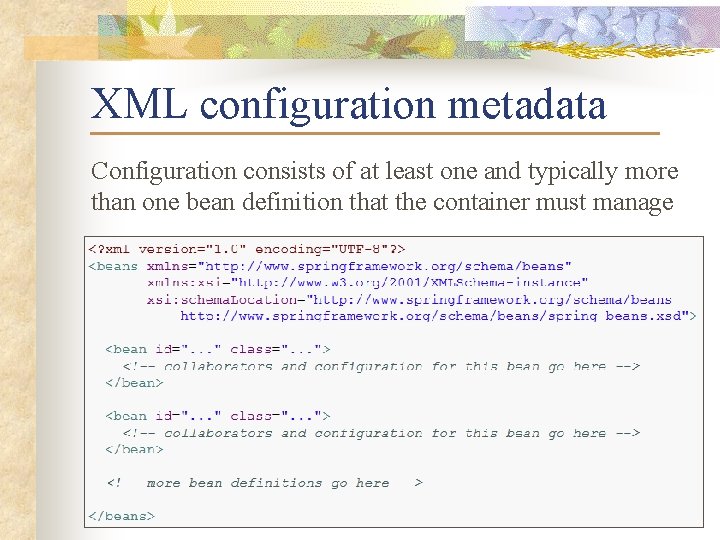
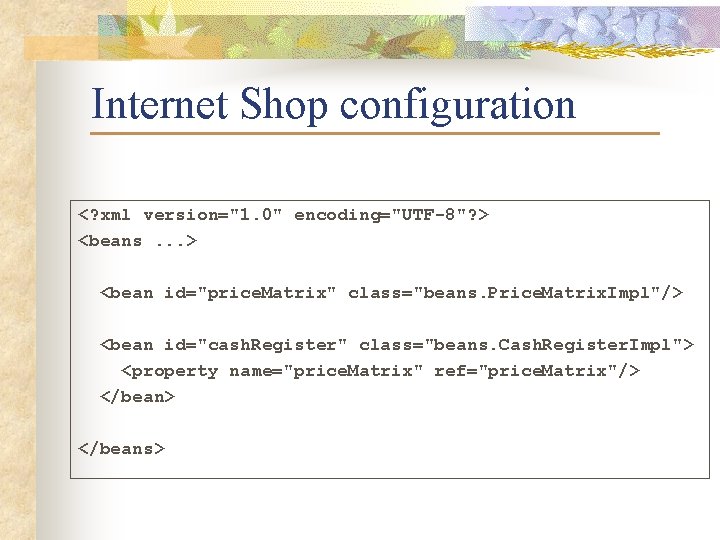
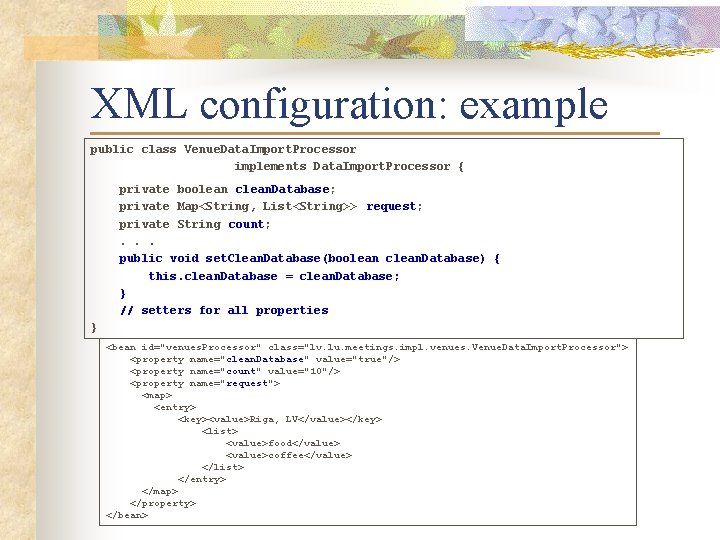
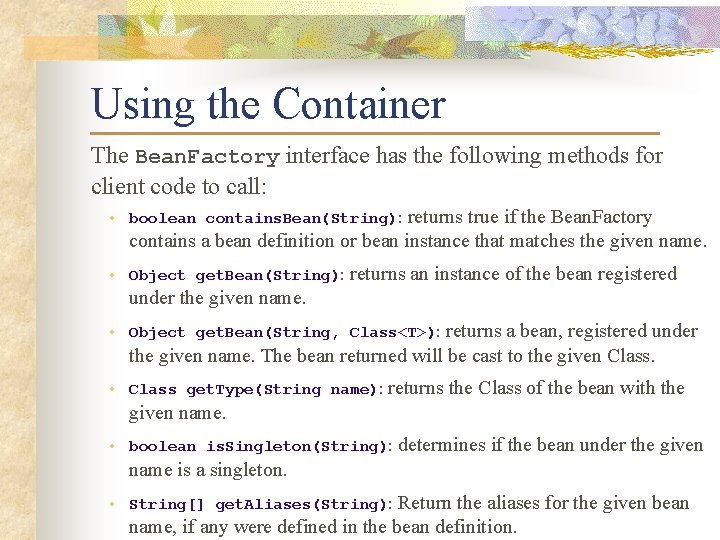
![Main Application public class Internet. Shop. App { public static void main(String[] args){ Application. Main Application public class Internet. Shop. App { public static void main(String[] args){ Application.](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-45.jpg)
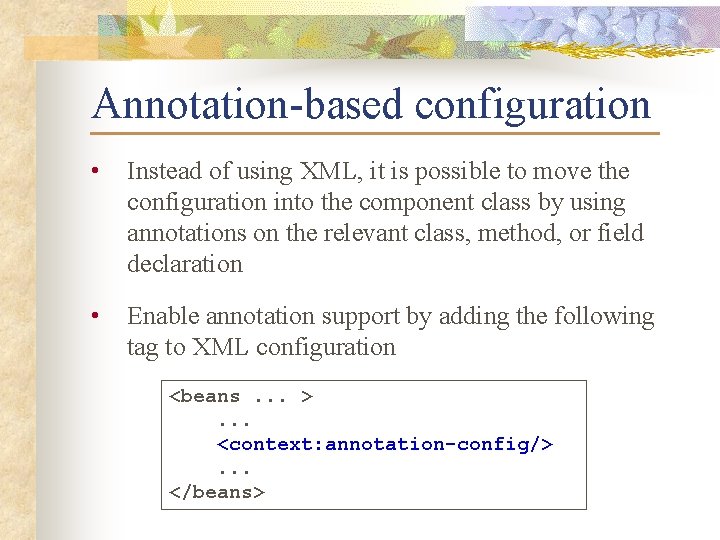
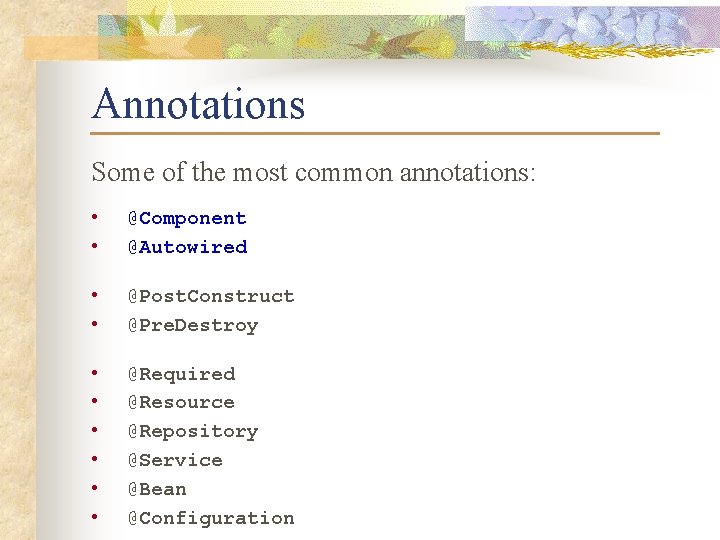
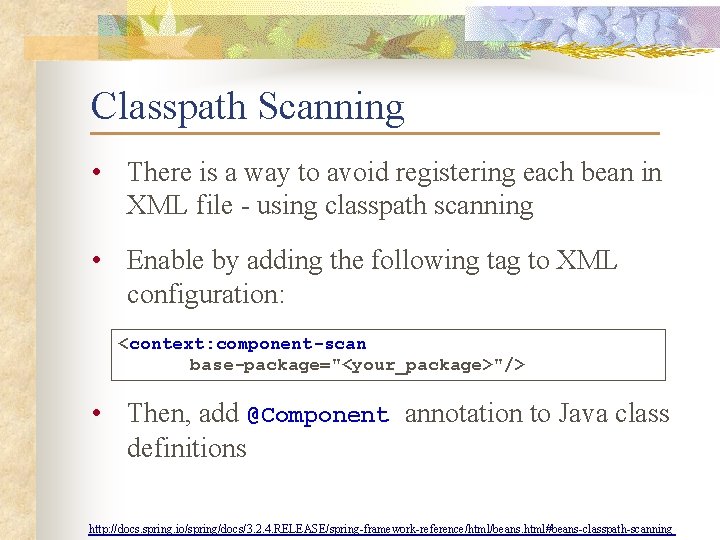
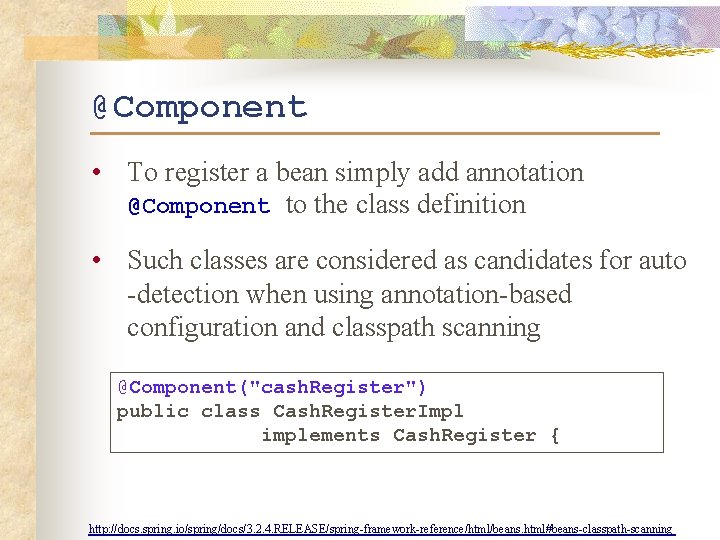
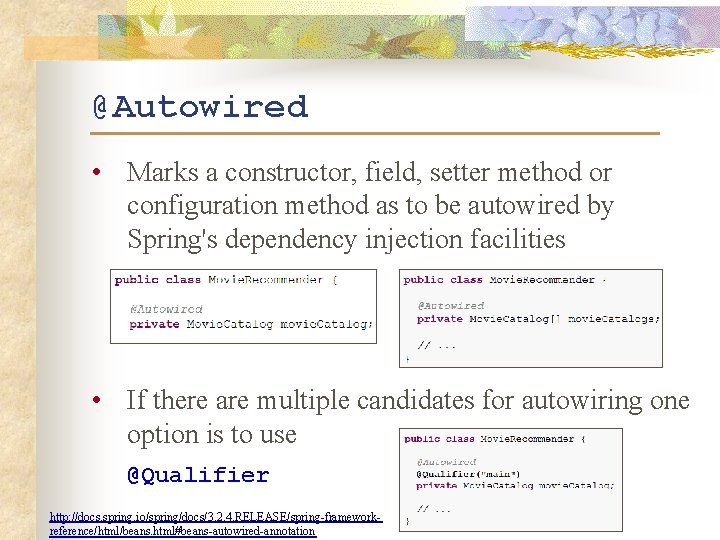
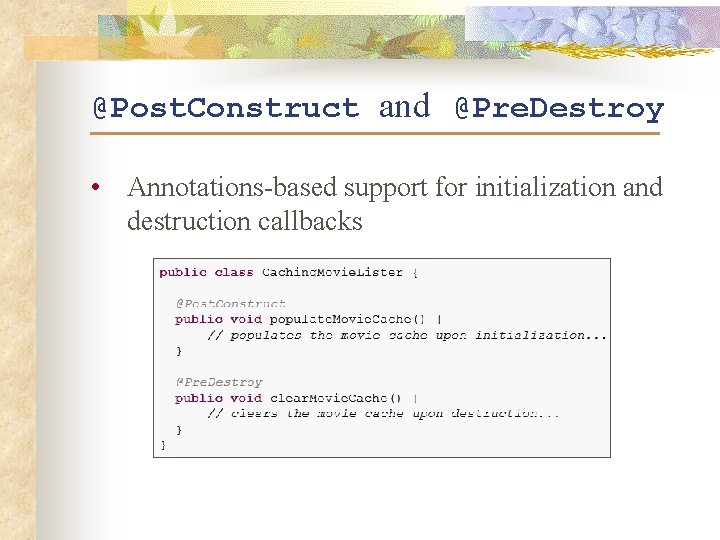
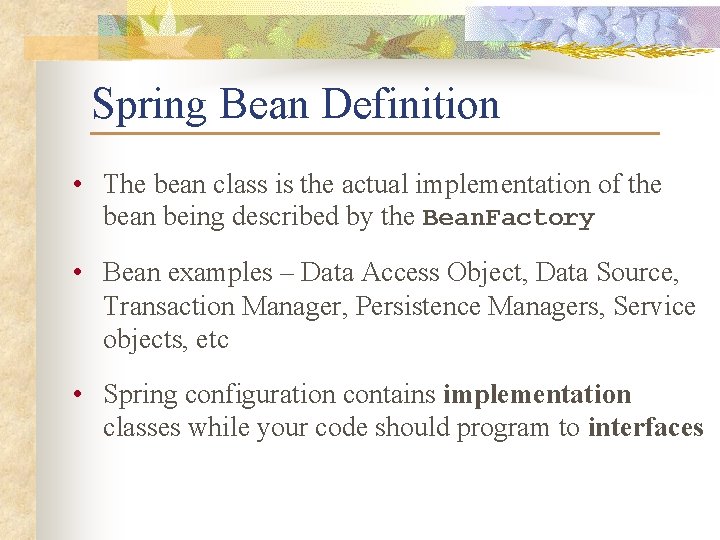
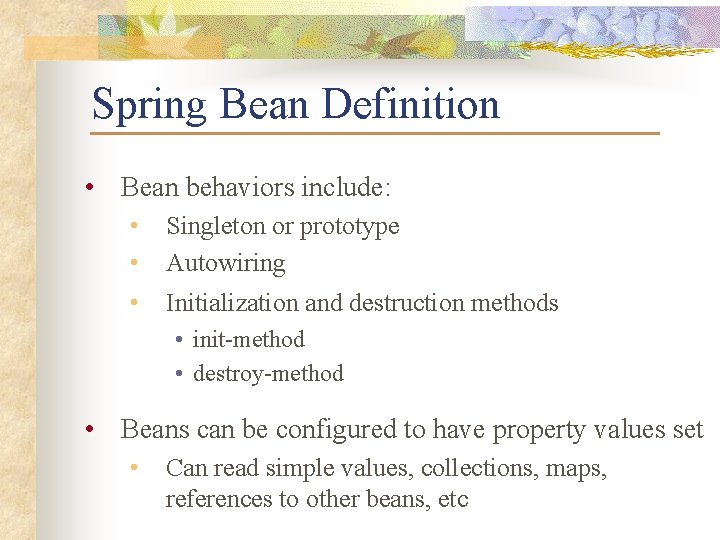
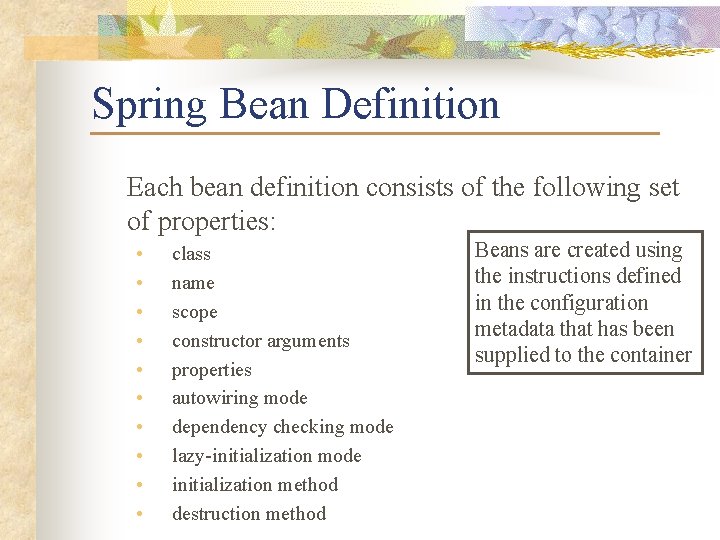
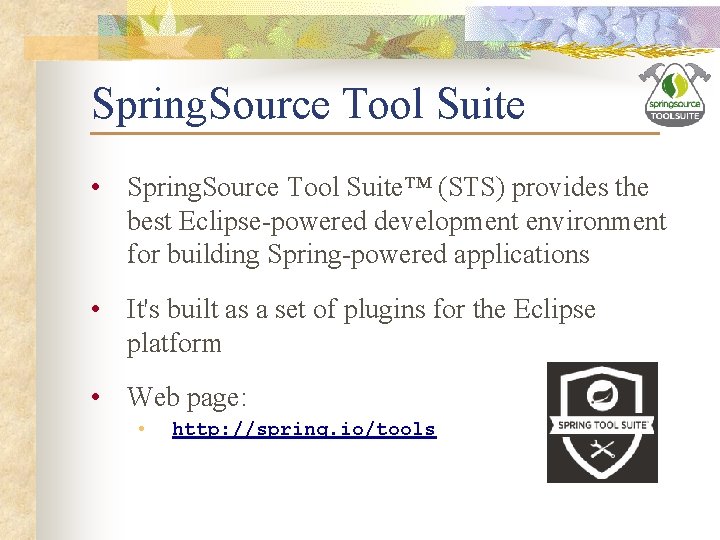
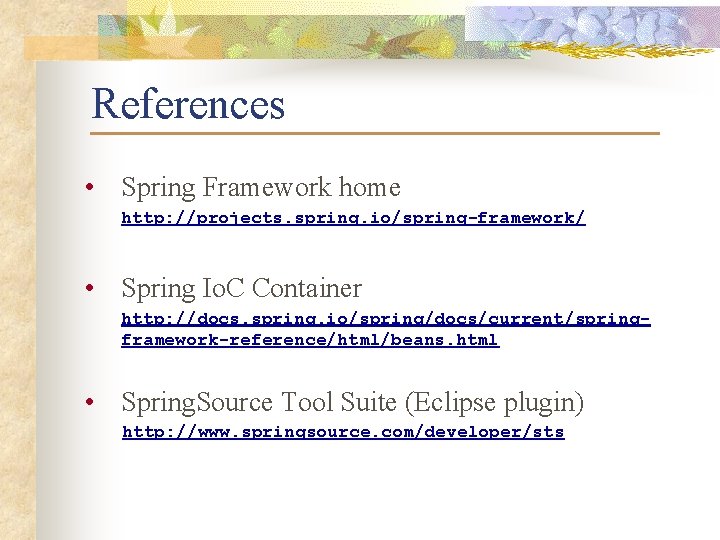
- Slides: 56
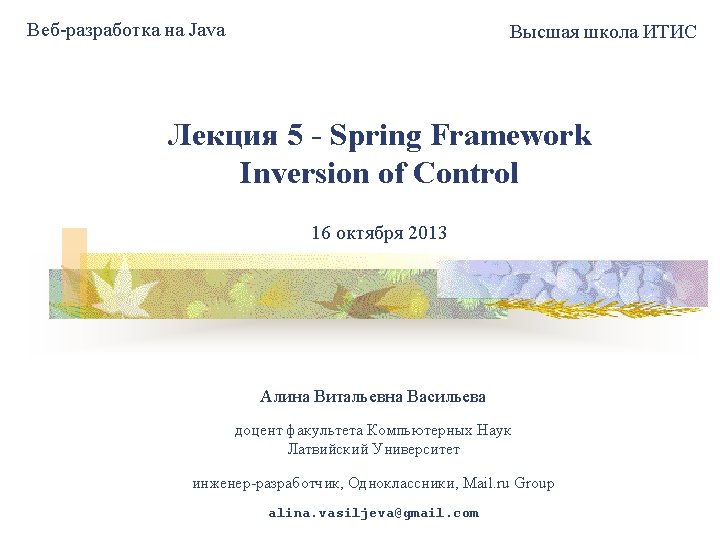
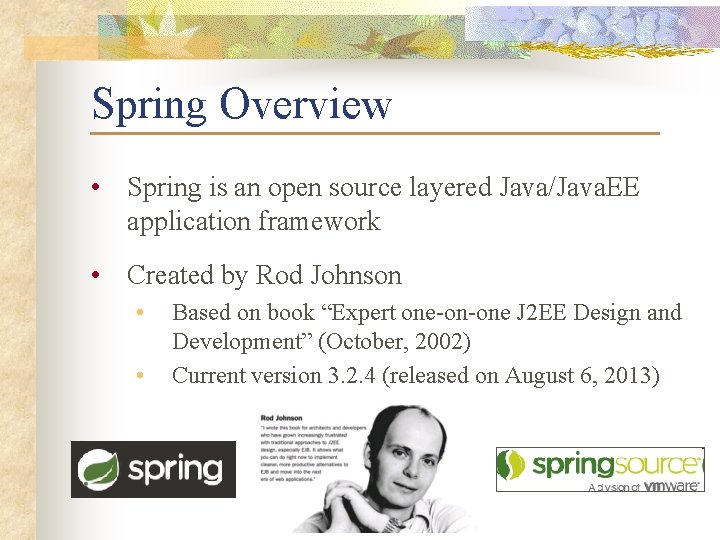
Spring Overview • Spring is an open source layered Java/Java. EE application framework • Created by Rod Johnson • • Based on book “Expert one-on-one J 2 EE Design and Development” (October, 2002) Current version 3. 2. 4 (released on August 6, 2013)
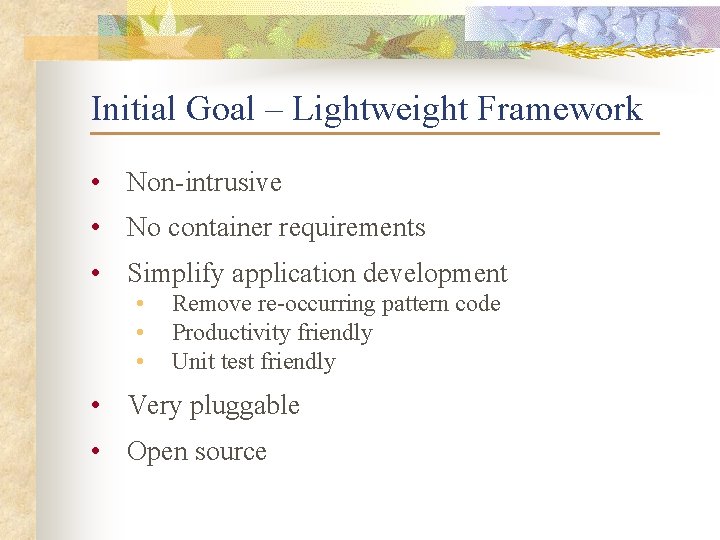
Initial Goal – Lightweight Framework • Non-intrusive • No container requirements • Simplify application development • • • Remove re-occurring pattern code Productivity friendly Unit test friendly • Very pluggable • Open source
![Spring Mission Statement 13 Java EE should be easier to use Its Spring Mission Statement [1/3] • Java EE should be easier to use • It's](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-4.jpg)
Spring Mission Statement [1/3] • Java EE should be easier to use • It's best to program to interfaces, rather than classes. Spring reduces the complexity cost of using interfaces to zero. • Java. Beans (POJO) offer a great way of configuring applications • OO design is more important than any implementation technology, such as Java EE
![Spring Mission Statement 23 Checked exceptions are overused in Java A framework shouldnt Spring Mission Statement [2/3] • Checked exceptions are overused in Java. A framework shouldn't](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-5.jpg)
Spring Mission Statement [2/3] • Checked exceptions are overused in Java. A framework shouldn't force you to catch exceptions you're unlikely to be able to recover from. • Testability is essential, and a framework such as Spring should help make your code easier to test • Spring should be a pleasure to use • Your application code should not depend on Spring APIs
![Spring Mission Statement 33 Spring should not compete with good existing solutions but Spring Mission Statement [3/3] • Spring should not compete with good existing solutions, but](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-6.jpg)
Spring Mission Statement [3/3] • Spring should not compete with good existing solutions, but should foster integration. For example, Hibernate is a great objectrelational mapping solution. Don't need to develop another one.
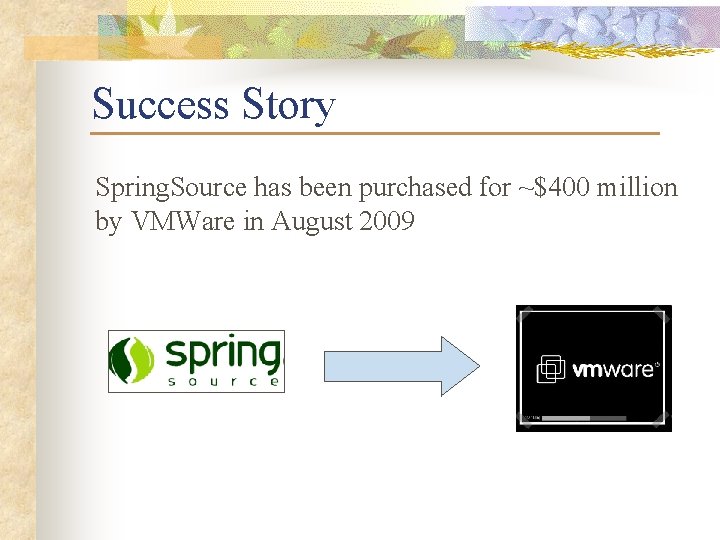
Success Story Spring. Source has been purchased for ~$400 million by VMWare in August 2009
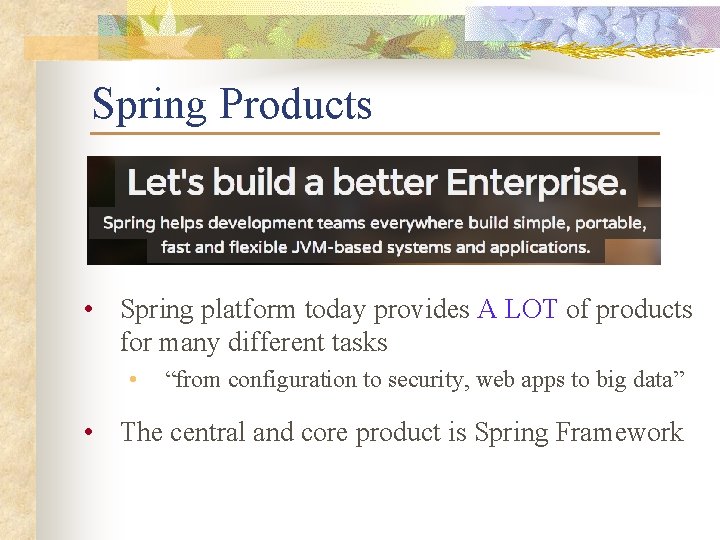
Spring Products • Spring platform today provides A LOT of products for many different tasks • “from configuration to security, web apps to big data” • The central and core product is Spring Framework
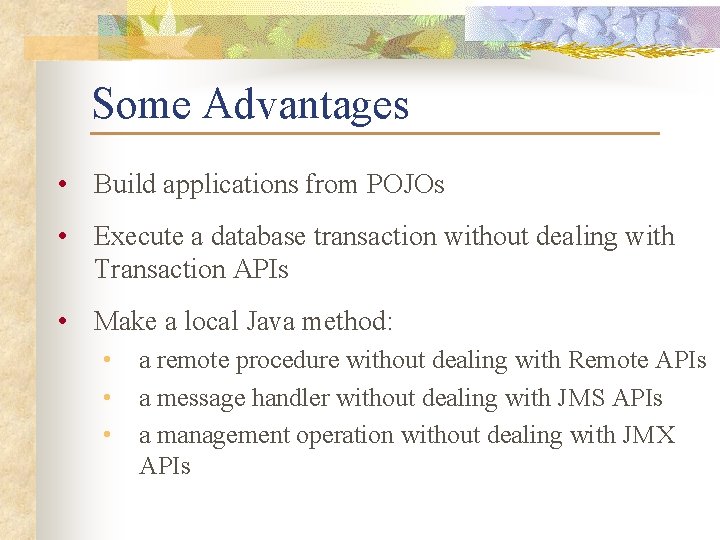
Some Advantages • Build applications from POJOs • Execute a database transaction without dealing with Transaction APIs • Make a local Java method: • • • a remote procedure without dealing with Remote APIs a message handler without dealing with JMS APIs a management operation without dealing with JMX APIs
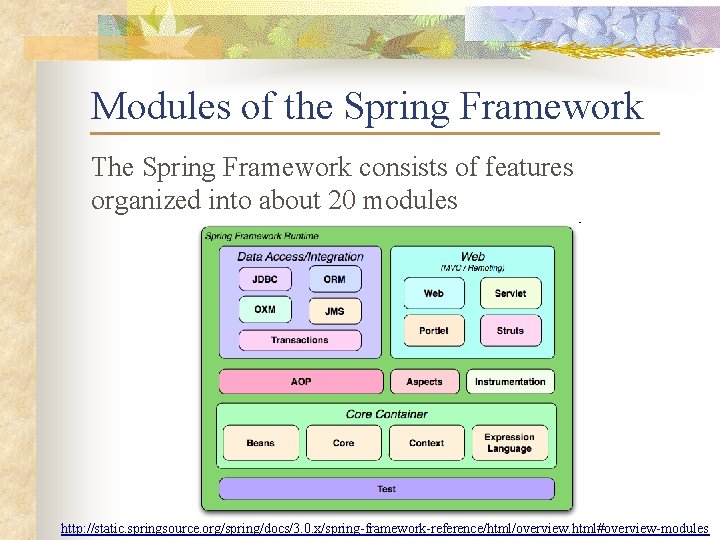
Modules of the Spring Framework The Spring Framework consists of features organized into about 20 modules http: //static. springsource. org/spring/docs/3. 0. x/spring-framework-reference/html/overview. html#overview-modules
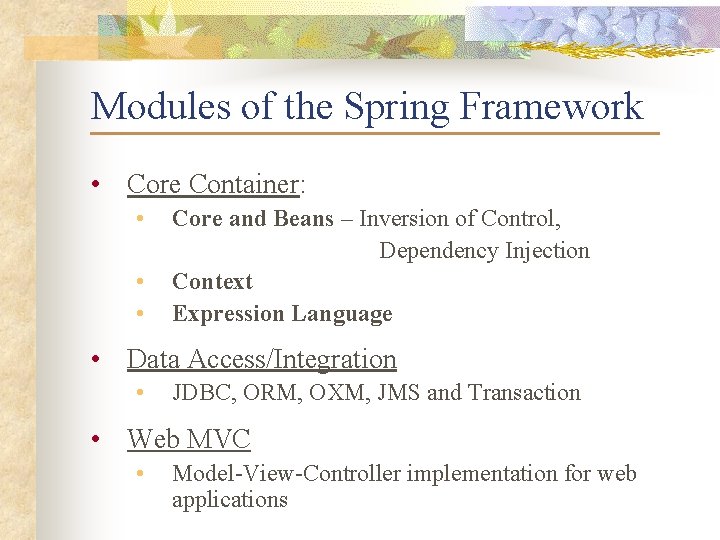
Modules of the Spring Framework • Core Container: • • • Core and Beans – Inversion of Control, Dependency Injection Context Expression Language • Data Access/Integration • JDBC, ORM, OXM, JMS and Transaction • Web MVC • Model-View-Controller implementation for web applications
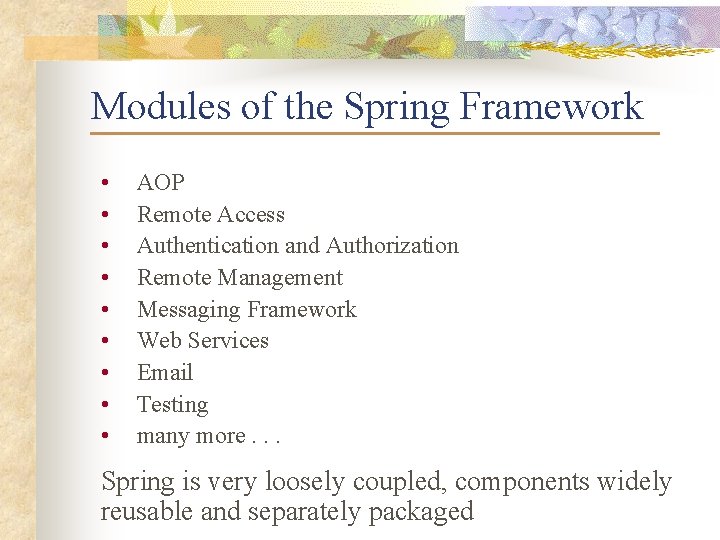
Modules of the Spring Framework • • • AOP Remote Access Authentication and Authorization Remote Management Messaging Framework Web Services Email Testing many more. . . Spring is very loosely coupled, components widely reusable and separately packaged
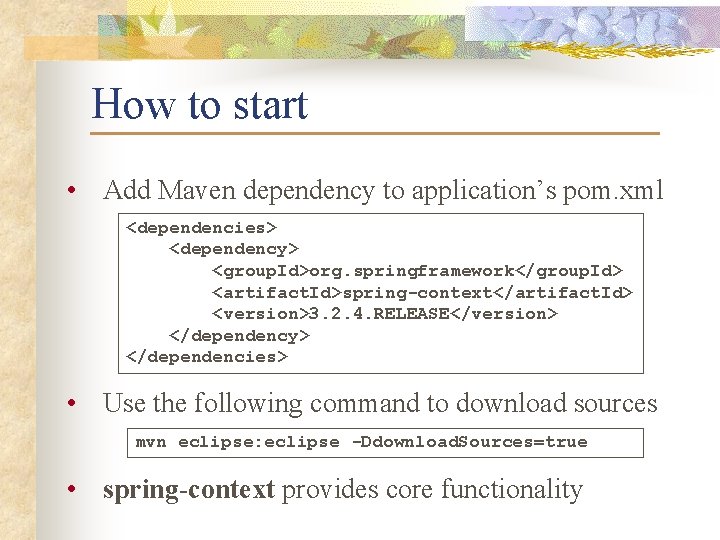
How to start • Add Maven dependency to application’s pom. xml <dependencies> <dependency> <group. Id>org. springframework</group. Id> <artifact. Id>spring-context</artifact. Id> <version>3. 2. 4. RELEASE</version> </dependency> </dependencies> • Use the following command to download sources mvn eclipse: eclipse -Ddownload. Sources=true • spring-context provides core functionality
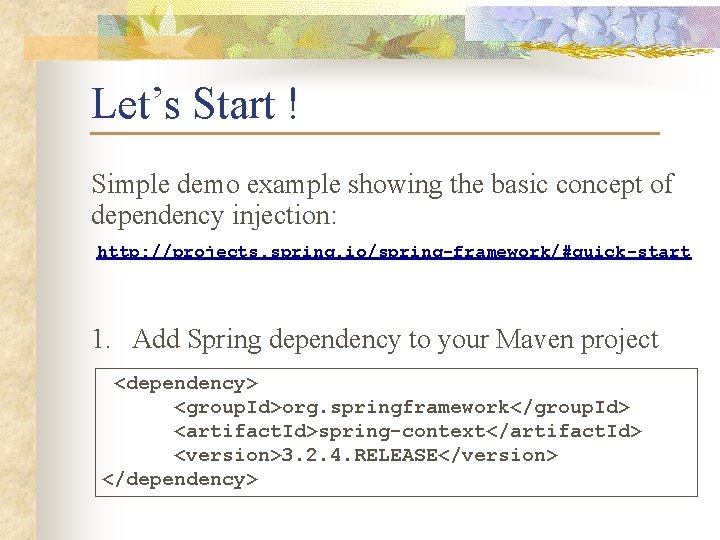
Let’s Start ! Simple demo example showing the basic concept of dependency injection: http: //projects. spring. io/spring-framework/#quick-start 1. Add Spring dependency to your Maven project <dependency> <group. Id>org. springframework</group. Id> <artifact. Id>spring-context</artifact. Id> <version>3. 2. 4. RELEASE</version> </dependency>
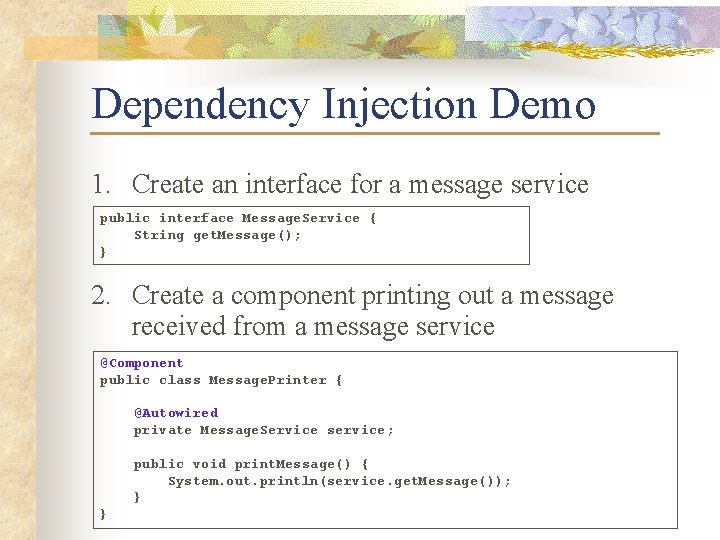
Dependency Injection Demo 1. Create an interface for a message service public interface Message. Service { String get. Message(); } 2. Create a component printing out a message received from a message service @Component public class Message. Printer { @Autowired private Message. Service service; public void print. Message() { System. out. println(service. get. Message()); } }
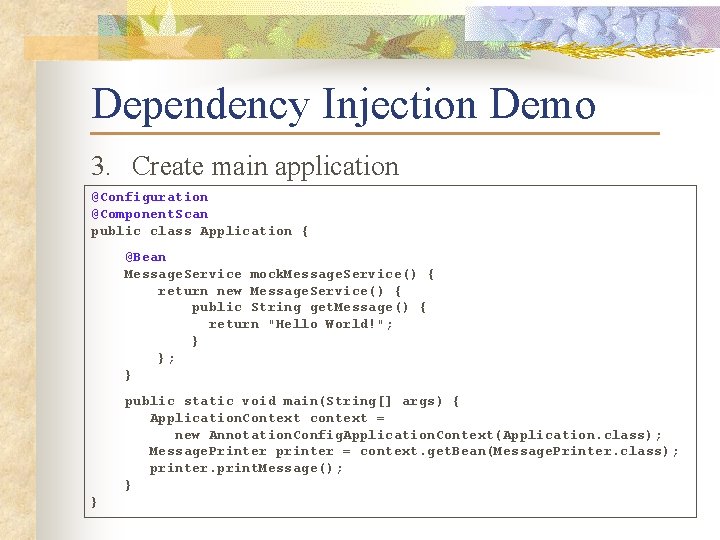
Dependency Injection Demo 3. Create main application @Configuration @Component. Scan public class Application { @Bean Message. Service mock. Message. Service() { return new Message. Service() { public String get. Message() { return "Hello World!"; } }; } public static void main(String[] args) { Application. Context context = new Annotation. Config. Application. Context(Application. class); Message. Printer printer = context. get. Bean(Message. Printer. class); printer. print. Message(); } }
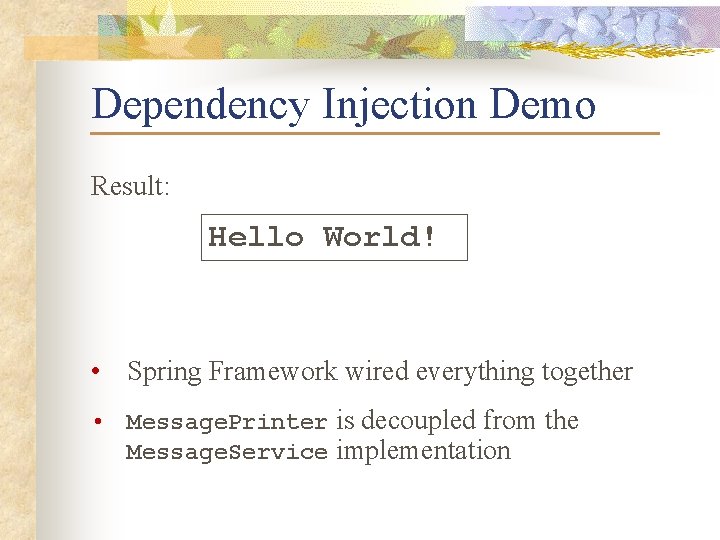
Dependency Injection Demo Result: Hello World! • Spring Framework wired everything together • Message. Printer is decoupled from the Message. Service implementation
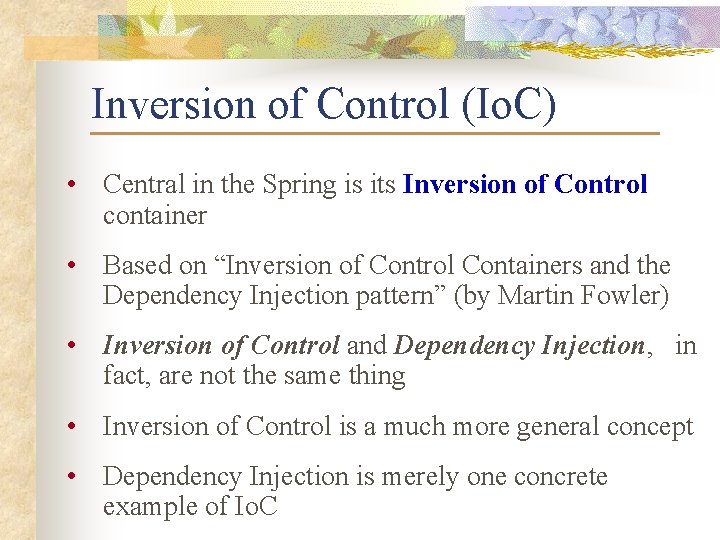
Inversion of Control (Io. C) • Central in the Spring is its Inversion of Control container • Based on “Inversion of Control Containers and the Dependency Injection pattern” (by Martin Fowler) • Inversion of Control and Dependency Injection, in fact, are not the same thing • Inversion of Control is a much more general concept • Dependency Injection is merely one concrete example of Io. C
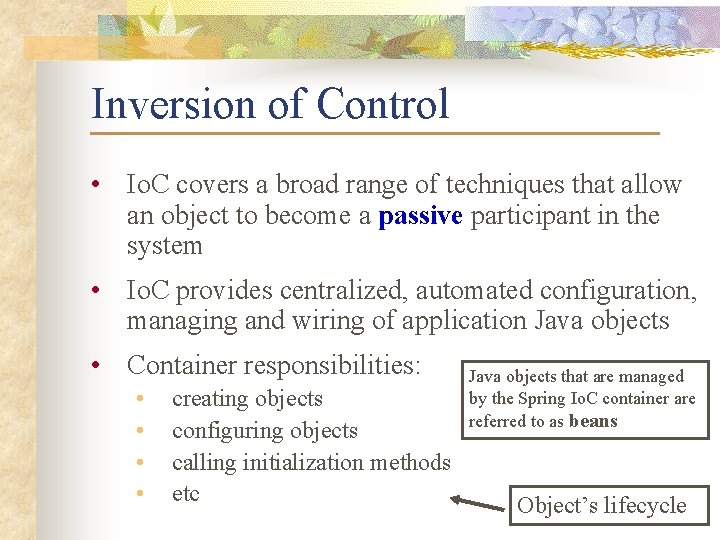
Inversion of Control • Io. C covers a broad range of techniques that allow an object to become a passive participant in the system • Io. C provides centralized, automated configuration, managing and wiring of application Java objects • Container responsibilities: • • creating objects configuring objects calling initialization methods etc Java objects that are managed by the Spring Io. C container are referred to as beans Object’s lifecycle
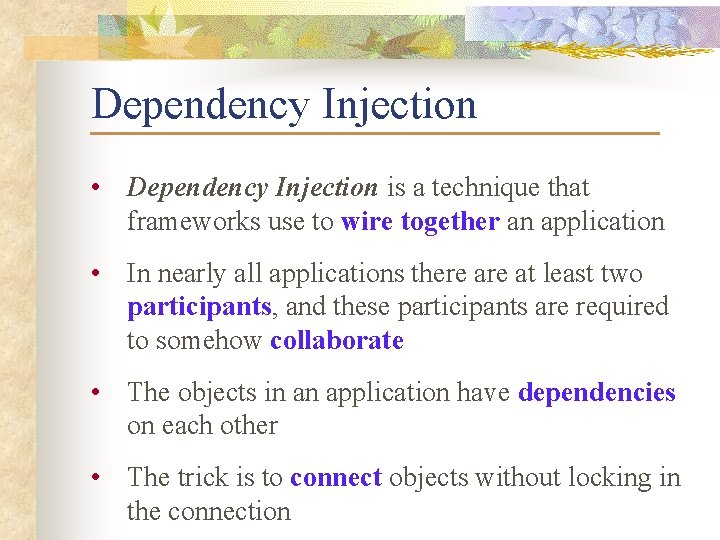
Dependency Injection • Dependency Injection is a technique that frameworks use to wire together an application • In nearly all applications there at least two participants, and these participants are required to somehow collaborate • The objects in an application have dependencies on each other • The trick is to connect objects without locking in the connection
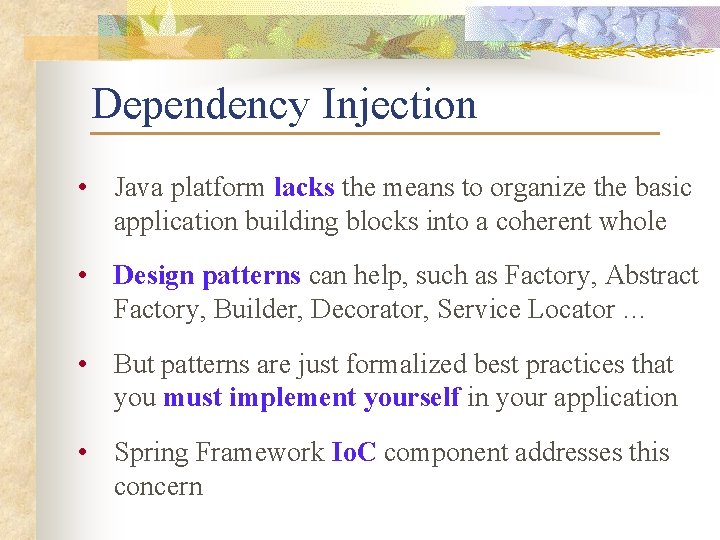
Dependency Injection • Java platform lacks the means to organize the basic application building blocks into a coherent whole • Design patterns can help, such as Factory, Abstract Factory, Builder, Decorator, Service Locator … • But patterns are just formalized best practices that you must implement yourself in your application • Spring Framework Io. C component addresses this concern
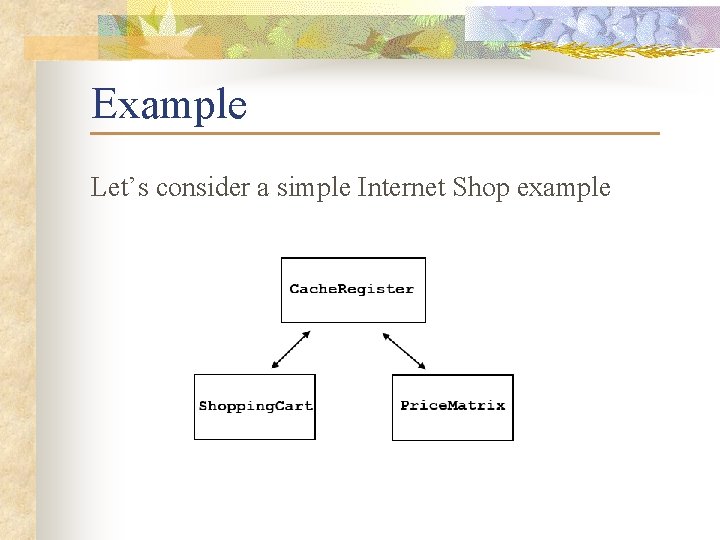
Example Let’s consider a simple Internet Shop example
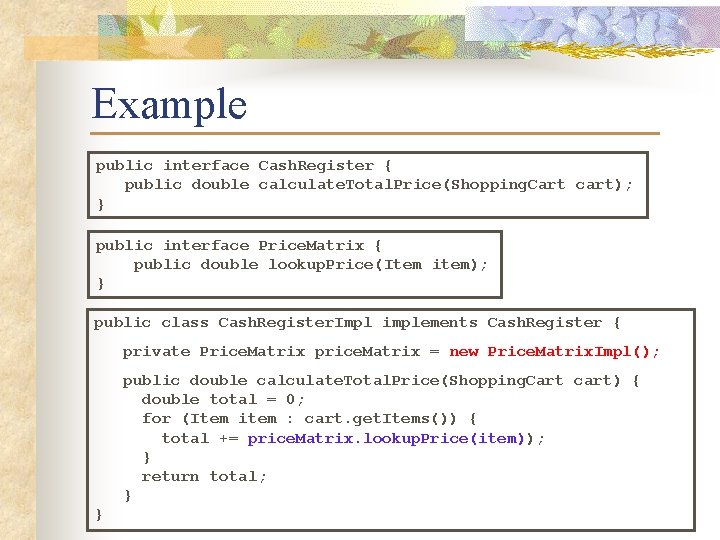
Example public interface Cash. Register { public double calculate. Total. Price(Shopping. Cart cart); } public interface Price. Matrix { public double lookup. Price(Item item); } public class Cash. Register. Impl implements Cash. Register { private Price. Matrix price. Matrix = new Price. Matrix. Impl(); public double calculate. Total. Price(Shopping. Cart cart) { double total = 0; for (Item item : cart. get. Items()) { total += price. Matrix. lookup. Price(item)); } return total; } }
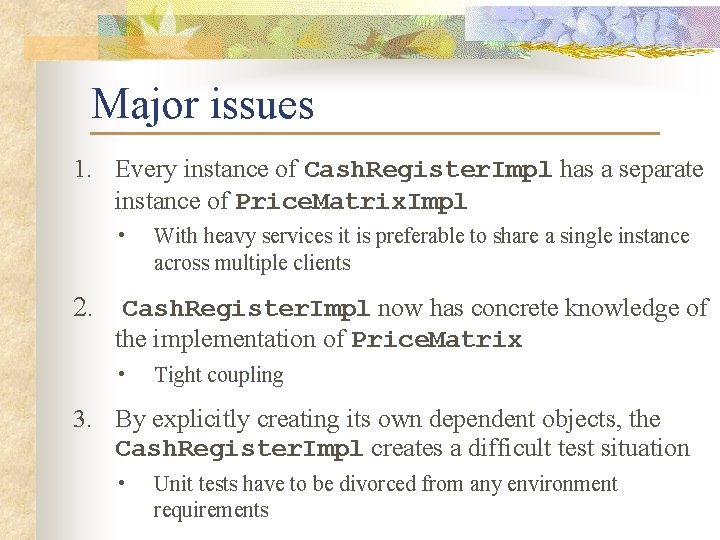
Major issues 1. Every instance of Cash. Register. Impl has a separate instance of Price. Matrix. Impl • With heavy services it is preferable to share a single instance across multiple clients 2. Cash. Register. Impl now has concrete knowledge of the implementation of Price. Matrix • Tight coupling 3. By explicitly creating its own dependent objects, the Cash. Register. Impl creates a difficult test situation • Unit tests have to be divorced from any environment requirements
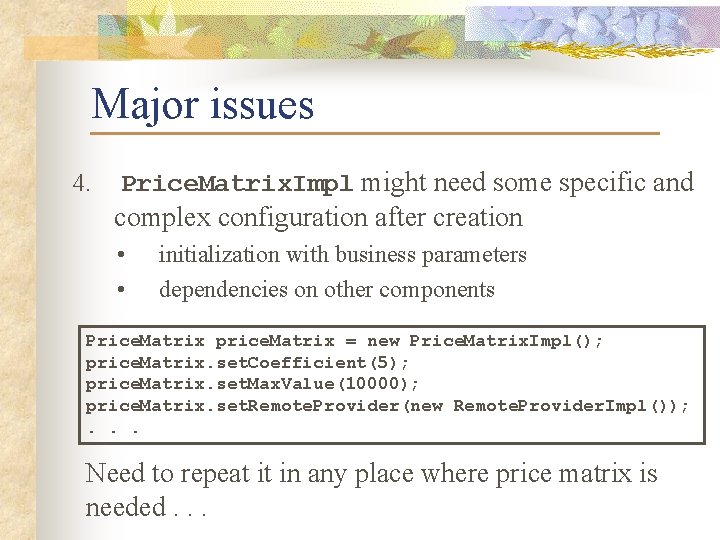
Major issues 4. Price. Matrix. Impl might need some specific and complex configuration after creation • • initialization with business parameters dependencies on other components Price. Matrix price. Matrix = new Price. Matrix. Impl(); price. Matrix. set. Coefficient(5); price. Matrix. set. Max. Value(10000); price. Matrix. set. Remote. Provider(new Remote. Provider. Impl()); . . . Need to repeat it in any place where price matrix is needed. . .
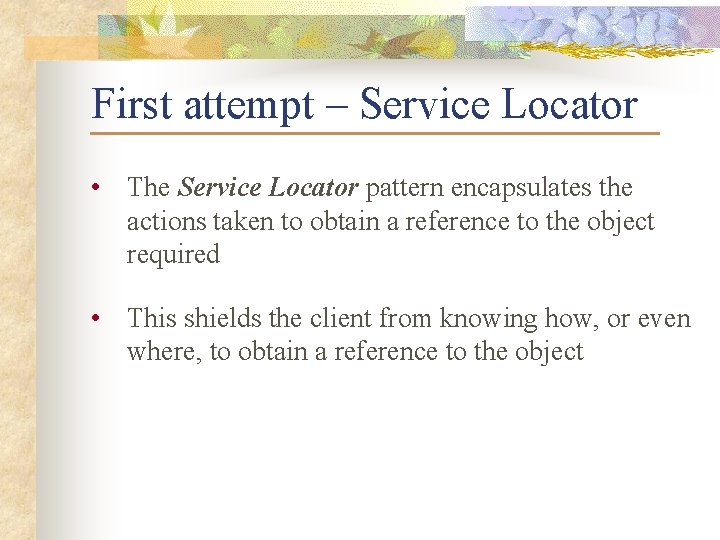
First attempt – Service Locator • The Service Locator pattern encapsulates the actions taken to obtain a reference to the object required • This shields the client from knowing how, or even where, to obtain a reference to the object
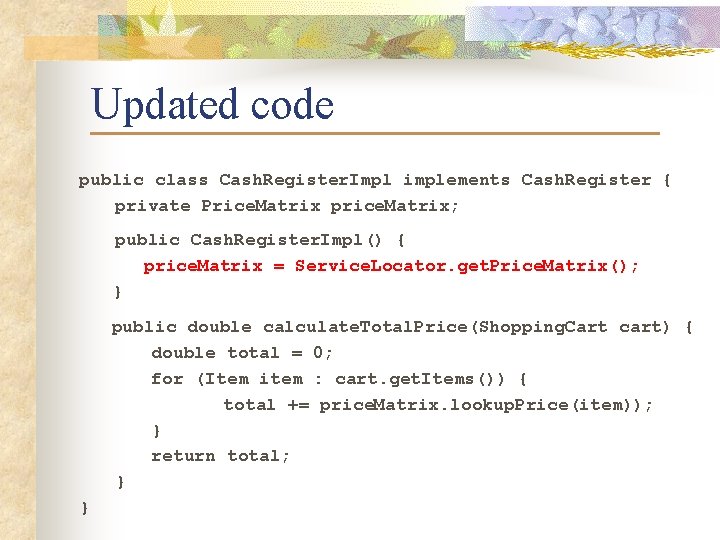
Updated code public class Cash. Register. Impl implements Cash. Register { private Price. Matrix price. Matrix; public Cash. Register. Impl() { price. Matrix = Service. Locator. get. Price. Matrix(); } public double calculate. Total. Price(Shopping. Cart cart) { double total = 0; for (Item item : cart. get. Items()) { total += price. Matrix. lookup. Price(item)); } return total; } }
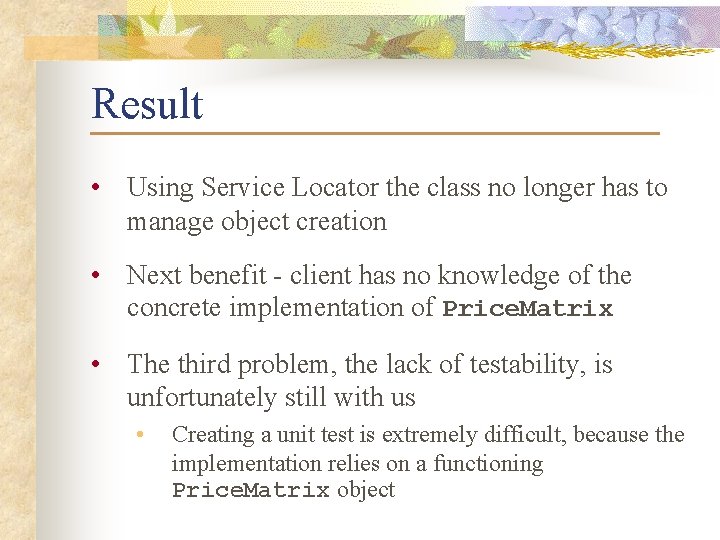
Result • Using Service Locator the class no longer has to manage object creation • Next benefit - client has no knowledge of the concrete implementation of Price. Matrix • The third problem, the lack of testability, is unfortunately still with us • Creating a unit test is extremely difficult, because the implementation relies on a functioning Price. Matrix object
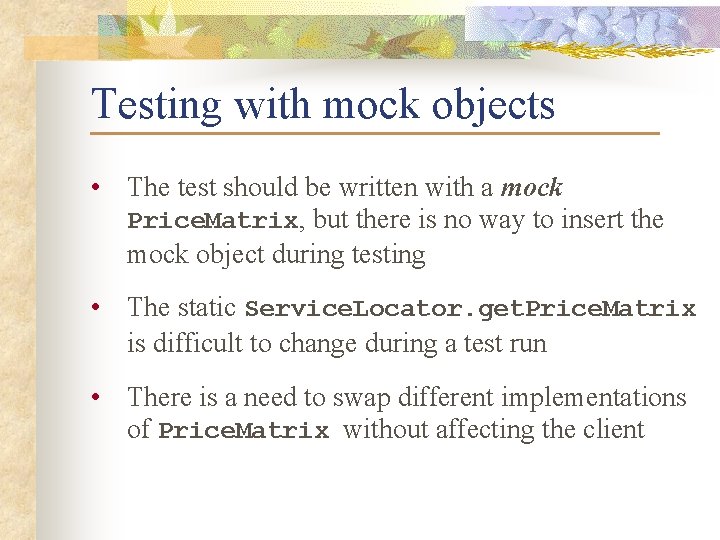
Testing with mock objects • The test should be written with a mock Price. Matrix, but there is no way to insert the mock object during testing • The static Service. Locator. get. Price. Matrix is difficult to change during a test run • There is a need to swap different implementations of Price. Matrix without affecting the client
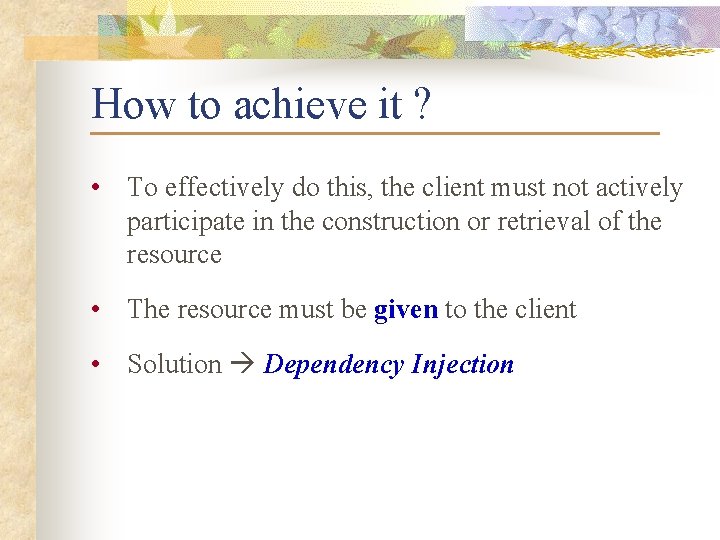
How to achieve it ? • To effectively do this, the client must not actively participate in the construction or retrieval of the resource • The resource must be given to the client • Solution Dependency Injection
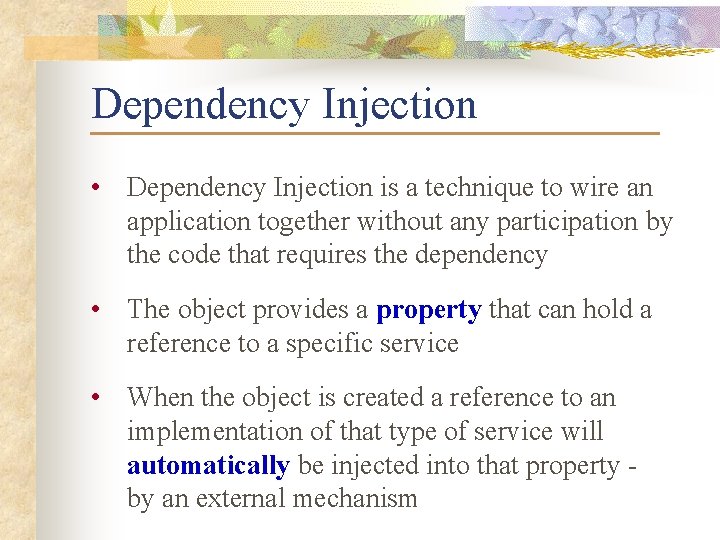
Dependency Injection • Dependency Injection is a technique to wire an application together without any participation by the code that requires the dependency • The object provides a property that can hold a reference to a specific service • When the object is created a reference to an implementation of that type of service will automatically be injected into that property by an external mechanism
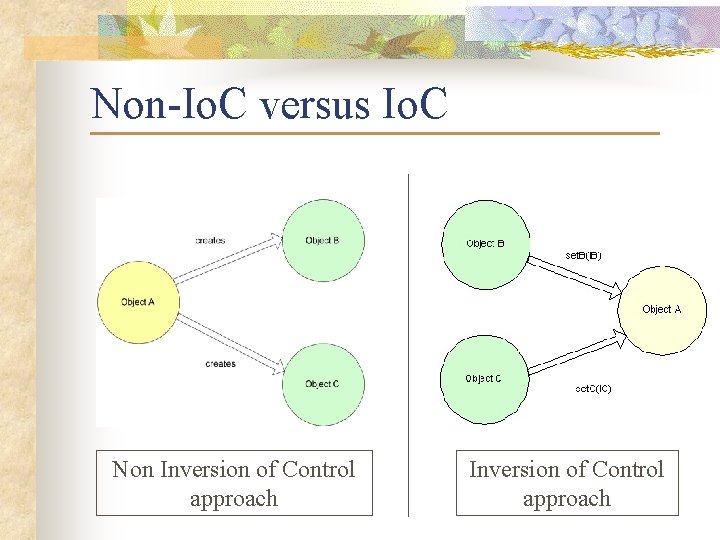
Non-Io. C versus Io. C Non Inversion of Control approach
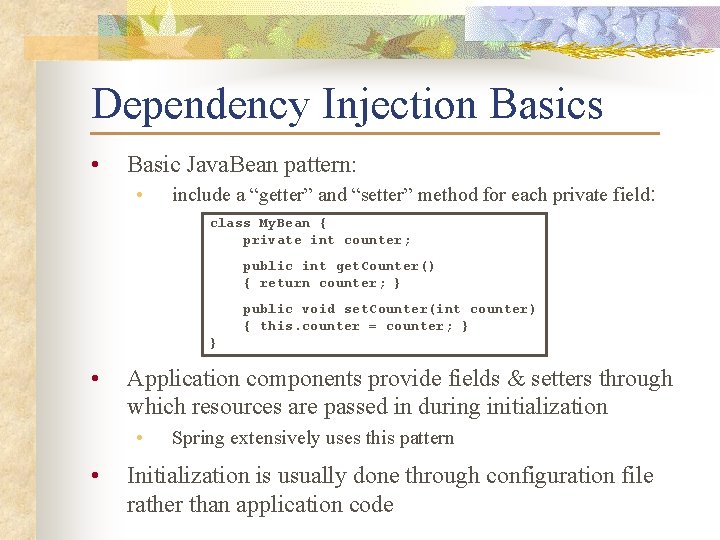
Dependency Injection Basics • Basic Java. Bean pattern: • include a “getter” and “setter” method for each private field: class My. Bean { private int counter; public int get. Counter() { return counter; } public void set. Counter(int counter) { this. counter = counter; } } • Application components provide fields & setters through which resources are passed in during initialization • • Spring extensively uses this pattern Initialization is usually done through configuration file rather than application code
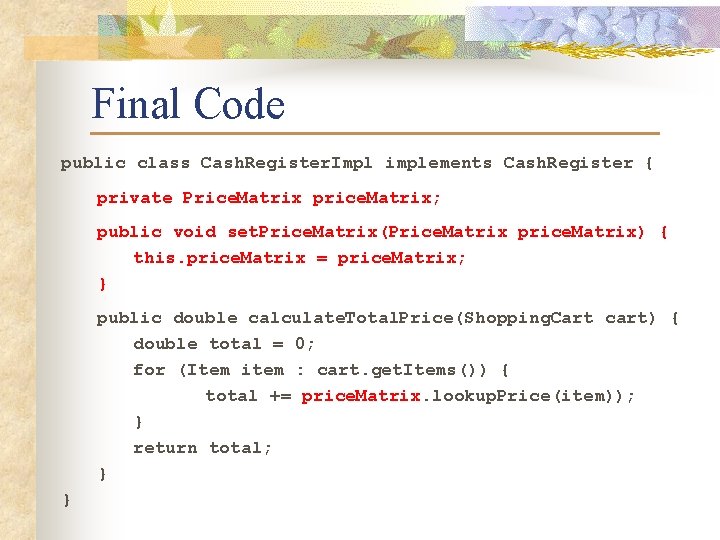
Final Code public class Cash. Register. Impl implements Cash. Register { private Price. Matrix price. Matrix; public void set. Price. Matrix(Price. Matrix price. Matrix) { this. price. Matrix = price. Matrix; } public double calculate. Total. Price(Shopping. Cart cart) { double total = 0; for (Item item : cart. get. Items()) { total += price. Matrix. lookup. Price(item)); } return total; } }
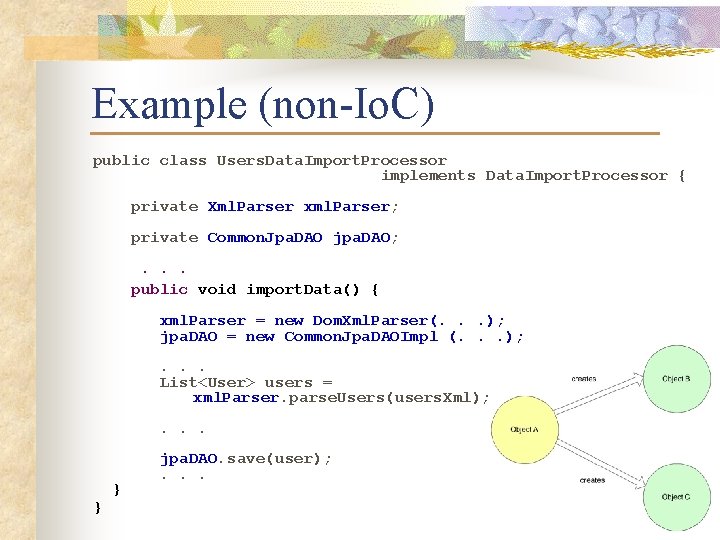
Example (non-Io. C) public class Users. Data. Import. Processor implements Data. Import. Processor { private Xml. Parser xml. Parser; private Common. Jpa. DAO jpa. DAO; . . . public void import. Data() { xml. Parser = new Dom. Xml. Parser(. . . ); jpa. DAO = new Common. Jpa. DAOImpl (. . . ); . . . List<User> users = xml. Parser. parse. Users(users. Xml); . . . } } jpa. DAO. save(user); . . .
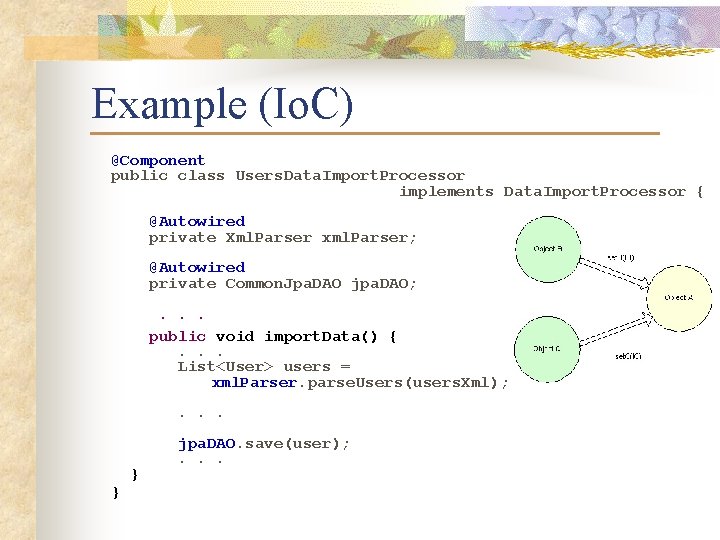
Example (Io. C) @Component public class Users. Data. Import. Processor implements Data. Import. Processor { @Autowired private Xml. Parser xml. Parser; @Autowired private Common. Jpa. DAO jpa. DAO; . . . public void import. Data() {. . . List<User> users = xml. Parser. parse. Users(users. Xml); . . . } } jpa. DAO. save(user); . . .
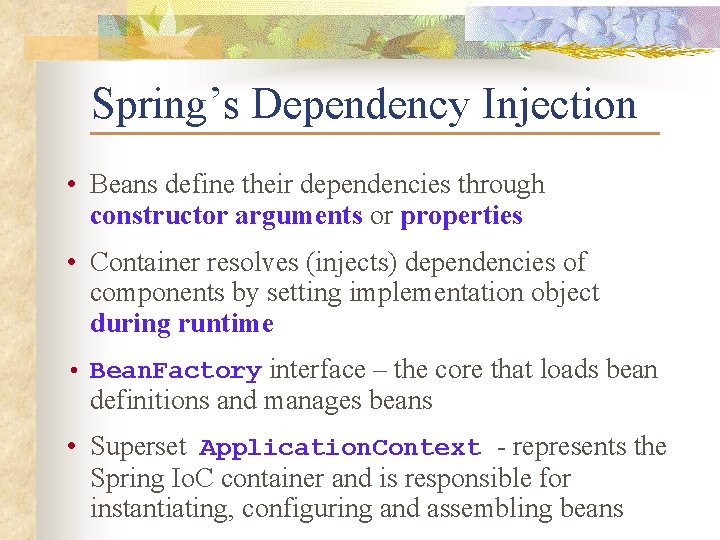
Spring’s Dependency Injection • Beans define their dependencies through constructor arguments or properties • Container resolves (injects) dependencies of components by setting implementation object during runtime • Bean. Factory interface – the core that loads bean definitions and manages beans • Superset Application. Context - represents the Spring Io. C container and is responsible for instantiating, configuring and assembling beans
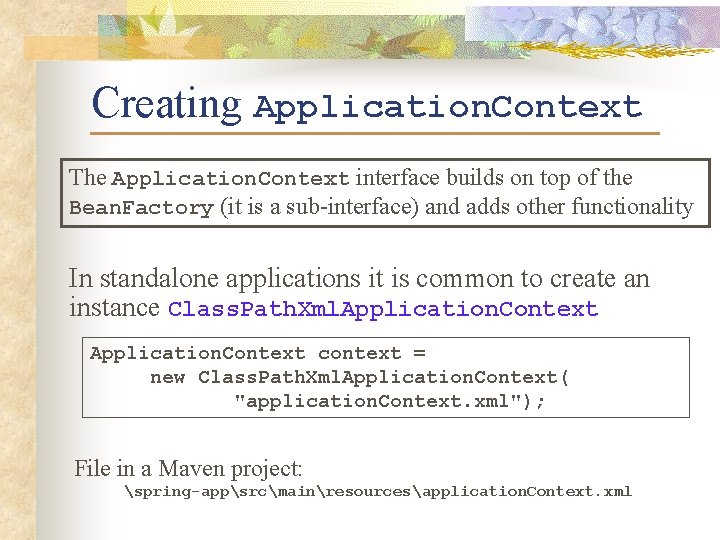
Creating Application. Context The Application. Context interface builds on top of the Bean. Factory (it is a sub-interface) and adds other functionality In standalone applications it is common to create an instance Class. Path. Xml. Application. Context context = new Class. Path. Xml. Application. Context( "application. Context. xml"); File in a Maven project: spring-appsrcmainresourcesapplication. Context. xml
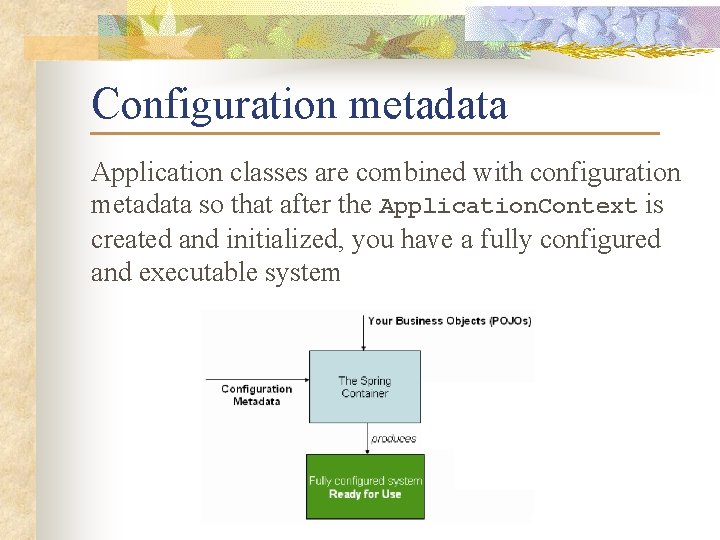
Configuration metadata Application classes are combined with configuration metadata so that after the Application. Context is created and initialized, you have a fully configured and executable system
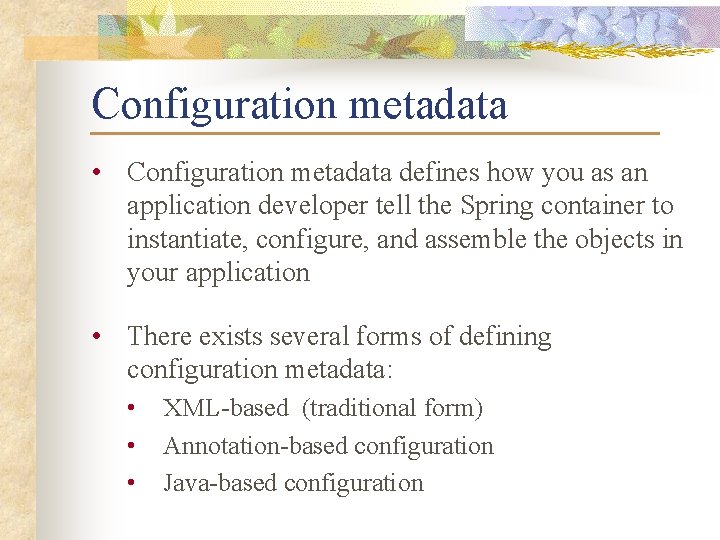
Configuration metadata • Configuration metadata defines how you as an application developer tell the Spring container to instantiate, configure, and assemble the objects in your application • There exists several forms of defining configuration metadata: • • • XML-based (traditional form) Annotation-based configuration Java-based configuration
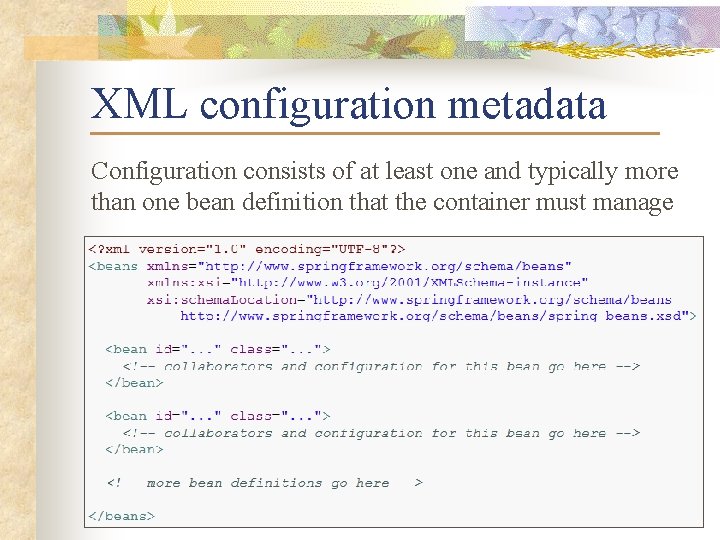
XML configuration metadata Configuration consists of at least one and typically more than one bean definition that the container must manage
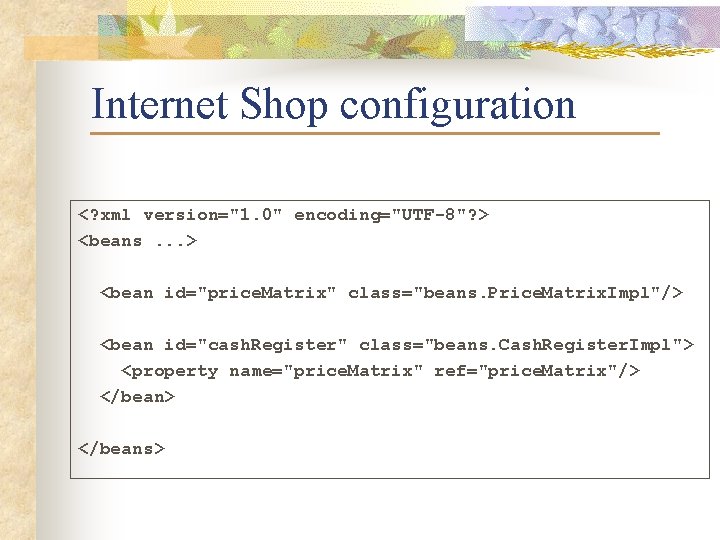
Internet Shop configuration <? xml version="1. 0" encoding="UTF-8"? > <beans. . . > <bean id="price. Matrix" class="beans. Price. Matrix. Impl"/> <bean id="cash. Register" class="beans. Cash. Register. Impl"> <property name="price. Matrix" ref="price. Matrix"/> </beans>
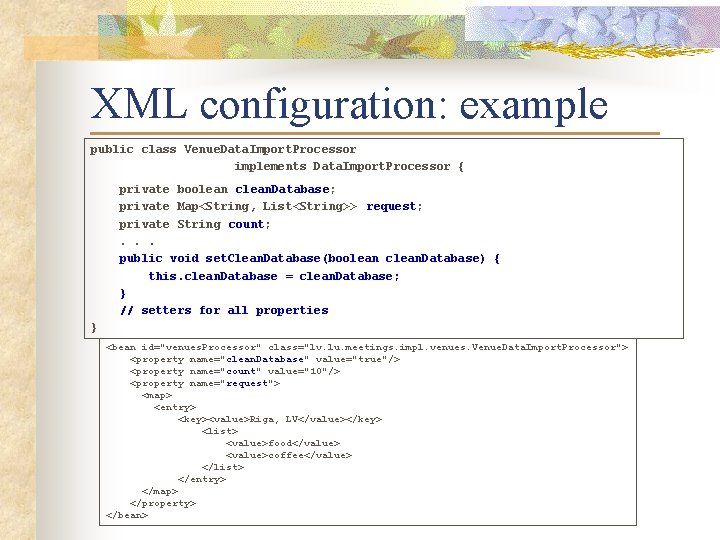
XML configuration: example public class Venue. Data. Import. Processor implements Data. Import. Processor { private boolean clean. Database; private Map<String, List<String>> request; private String count; . . . public void set. Clean. Database(boolean clean. Database) { this. clean. Database = clean. Database; } // setters for all properties } <bean id="venues. Processor" class="lv. lu. meetings. impl. venues. Venue. Data. Import. Processor"> <property name="clean. Database" value="true"/> <property name="count" value="10"/> <property name="request"> <map> <entry> <key><value>Riga, LV</value></key> <list> <value>food</value> <value>coffee</value> </list> </entry> </map> </property> </bean>
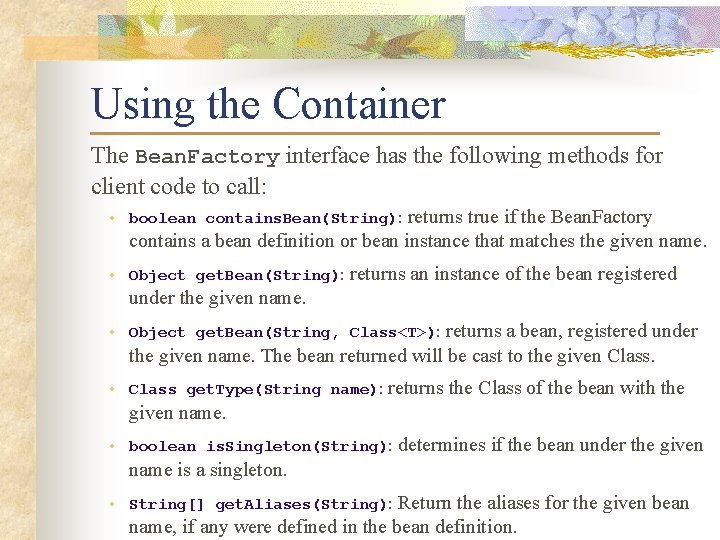
Using the Container The Bean. Factory interface has the following methods for client code to call: • boolean contains. Bean(String): returns true if the Bean. Factory contains a bean definition or bean instance that matches the given name. • Object get. Bean(String): returns an instance of the bean registered under the given name. • Object get. Bean(String, Class<T>): returns a bean, registered under the given name. The bean returned will be cast to the given Class. • Class get. Type(String name): returns the Class of the bean with the given name. • boolean is. Singleton(String): determines if the bean under the given name is a singleton. • String[] get. Aliases(String): Return the aliases for the given bean name, if any were defined in the bean definition.
![Main Application public class Internet Shop App public static void mainString args Application Main Application public class Internet. Shop. App { public static void main(String[] args){ Application.](https://slidetodoc.com/presentation_image_h/214fcbf23145492ba5432140448dad2c/image-45.jpg)
Main Application public class Internet. Shop. App { public static void main(String[] args){ Application. Context app. Ctxt = new Class. Path. Xml. Application. Context( "/application. Context. xml"); Cash. Register cash. Register = (Cash. Register)app. Ctxt. get. Bean("cash. Register"); System. out. println("Total price: " + cash. Register. calculate. Total. Price( get. Shorring. Cart())); System. exit(0); } }
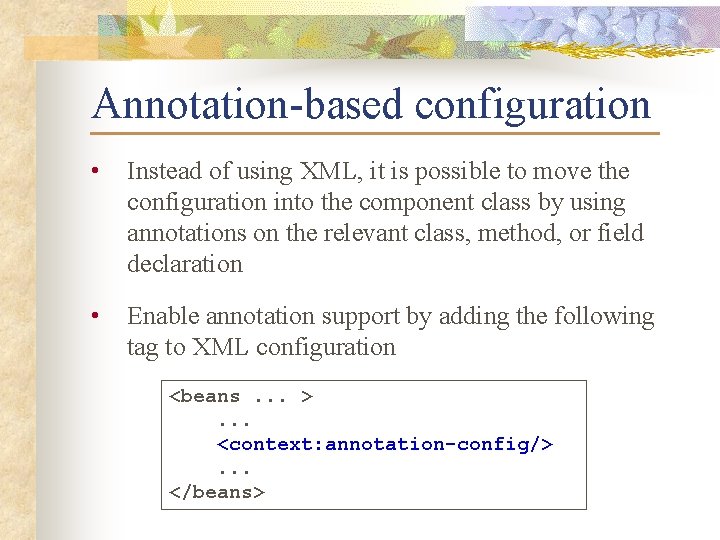
Annotation-based configuration • Instead of using XML, it is possible to move the configuration into the component class by using annotations on the relevant class, method, or field declaration • Enable annotation support by adding the following tag to XML configuration <beans. . . >. . . <context: annotation-config/>. . . </beans>
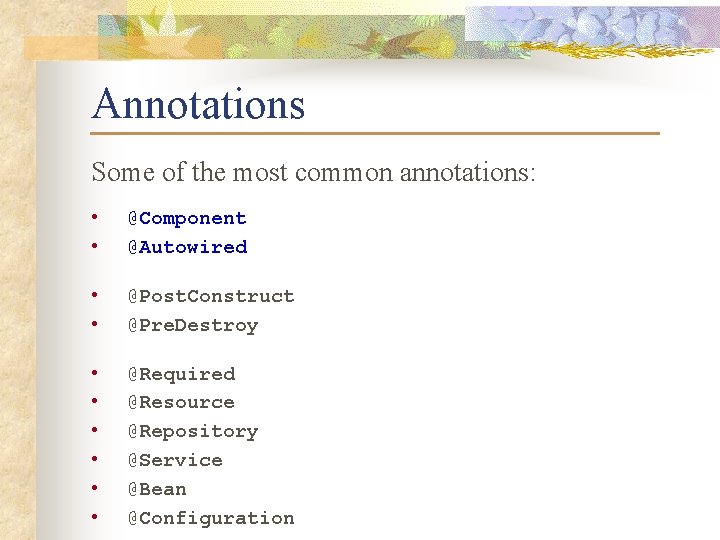
Annotations Some of the most common annotations: • • @Component @Autowired • • @Post. Construct @Pre. Destroy • • • @Required @Resource @Repository @Service @Bean @Configuration
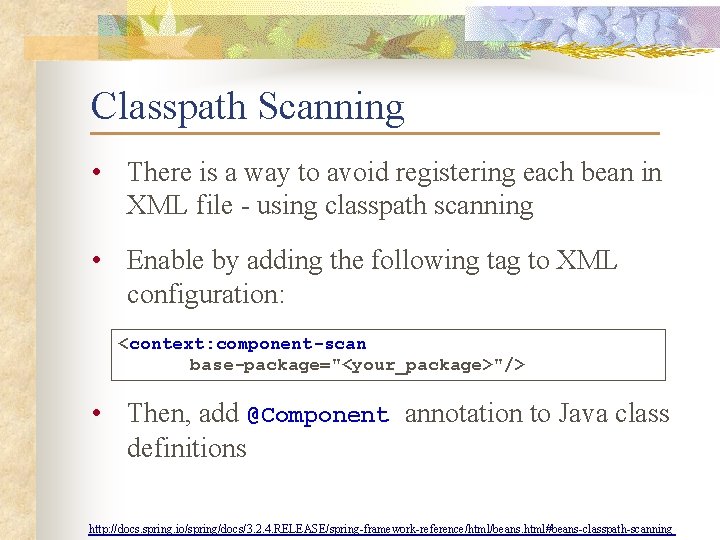
Classpath Scanning • There is a way to avoid registering each bean in XML file - using classpath scanning • Enable by adding the following tag to XML configuration: <context: component-scan base-package="<your_package>"/> • Then, add @Component annotation to Java class definitions http: //docs. spring. io/spring/docs/3. 2. 4. RELEASE/spring-framework-reference/html/beans. html#beans-classpath-scanning
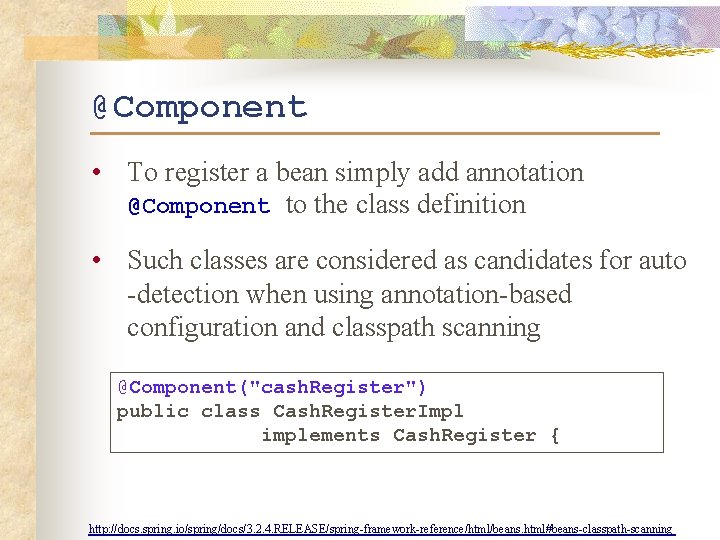
@Component • To register a bean simply add annotation @Component to the class definition • Such classes are considered as candidates for auto -detection when using annotation-based configuration and classpath scanning @Component("cash. Register") public class Cash. Register. Impl implements Cash. Register { http: //docs. spring. io/spring/docs/3. 2. 4. RELEASE/spring-framework-reference/html/beans. html#beans-classpath-scanning
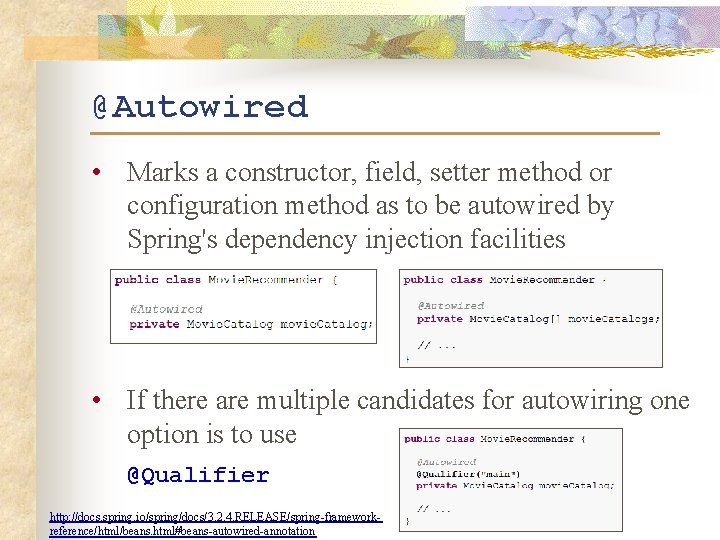
@Autowired • Marks a constructor, field, setter method or configuration method as to be autowired by Spring's dependency injection facilities • If there are multiple candidates for autowiring one option is to use @Qualifier http: //docs. spring. io/spring/docs/3. 2. 4. RELEASE/spring-frameworkreference/html/beans. html#beans-autowired-annotation
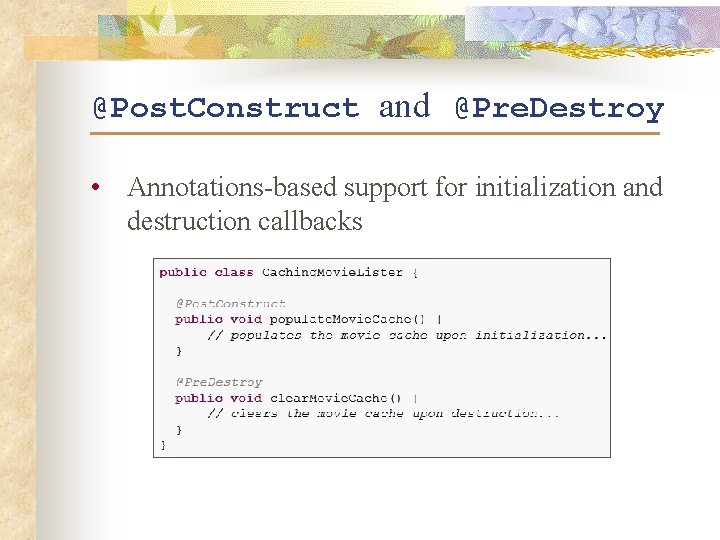
@Post. Construct and @Pre. Destroy • Annotations-based support for initialization and destruction callbacks
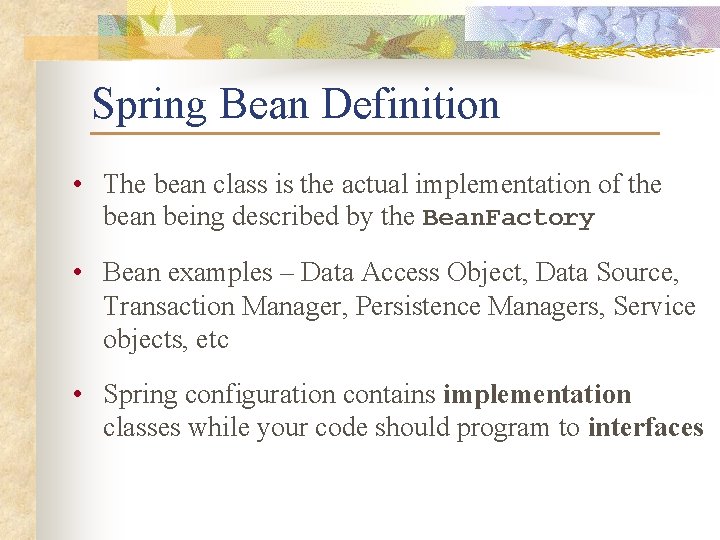
Spring Bean Definition • The bean class is the actual implementation of the bean being described by the Bean. Factory • Bean examples – Data Access Object, Data Source, Transaction Manager, Persistence Managers, Service objects, etc • Spring configuration contains implementation classes while your code should program to interfaces
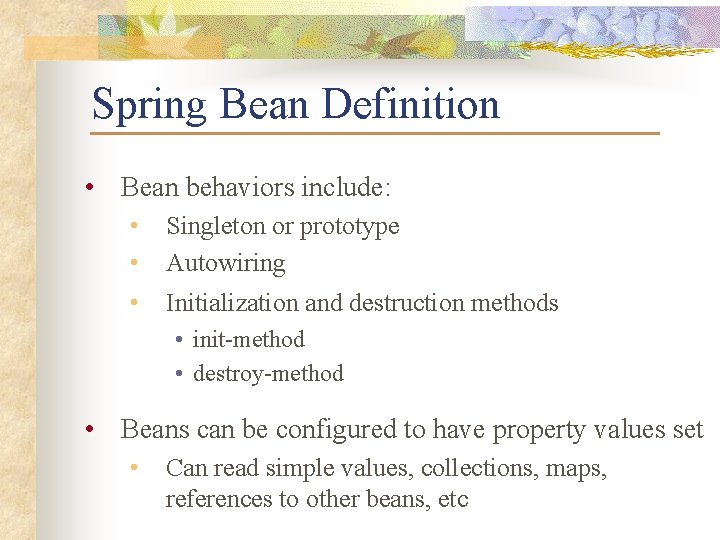
Spring Bean Definition • Bean behaviors include: • • Singleton or prototype Autowiring • Initialization and destruction methods • init-method • destroy-method • Beans can be configured to have property values set • Can read simple values, collections, maps, references to other beans, etc
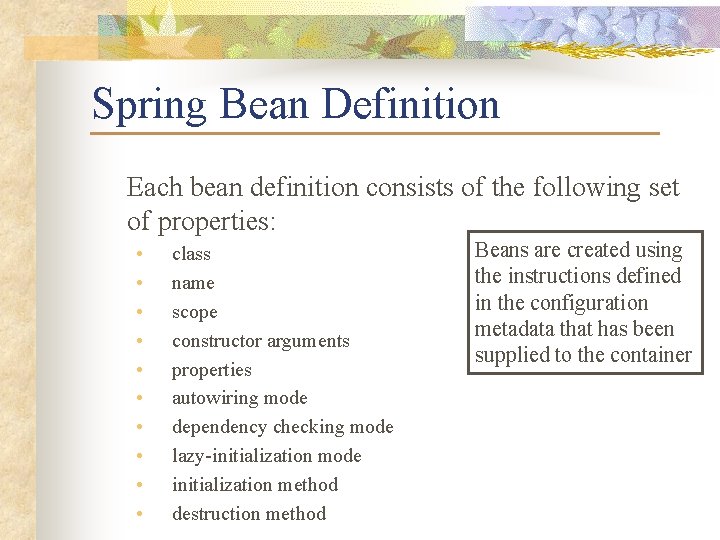
Spring Bean Definition Each bean definition consists of the following set of properties: • • • class name scope constructor arguments properties autowiring mode dependency checking mode lazy-initialization mode initialization method destruction method Beans are created using the instructions defined in the configuration metadata that has been supplied to the container
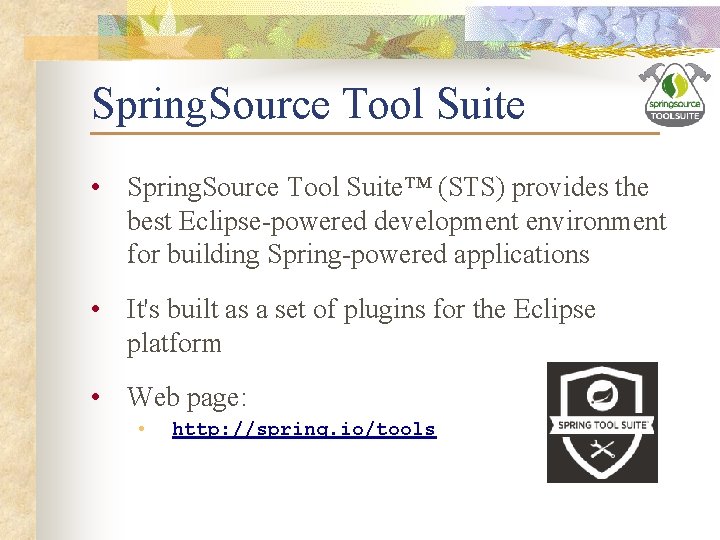
Spring. Source Tool Suite • Spring. Source Tool Suite™ (STS) provides the best Eclipse-powered development environment for building Spring-powered applications • It's built as a set of plugins for the Eclipse platform • Web page: • http: //spring. io/tools
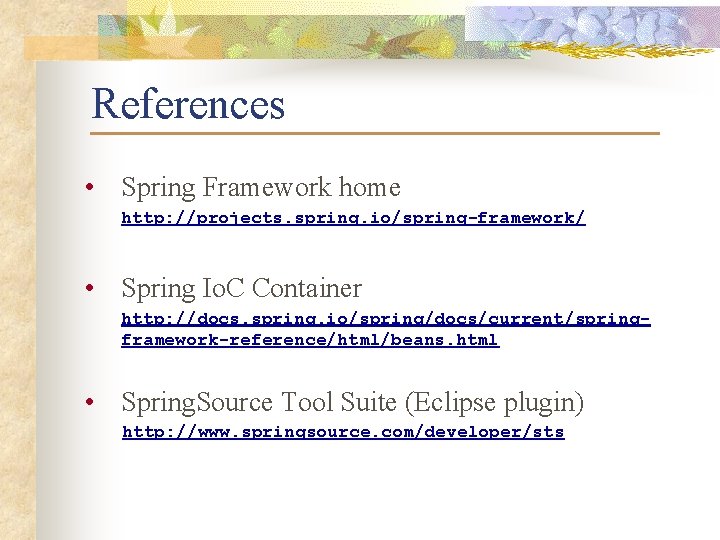
References • Spring Framework home http: //projects. spring. io/spring-framework/ • Spring Io. C Container http: //docs. spring. io/spring/docs/current/springframework-reference/html/beans. html • Spring. Source Tool Suite (Eclipse plugin) http: //www. springsource. com/developer/sts