SORTING Michael Tsai 2017328 2 Sorting 3 Applications
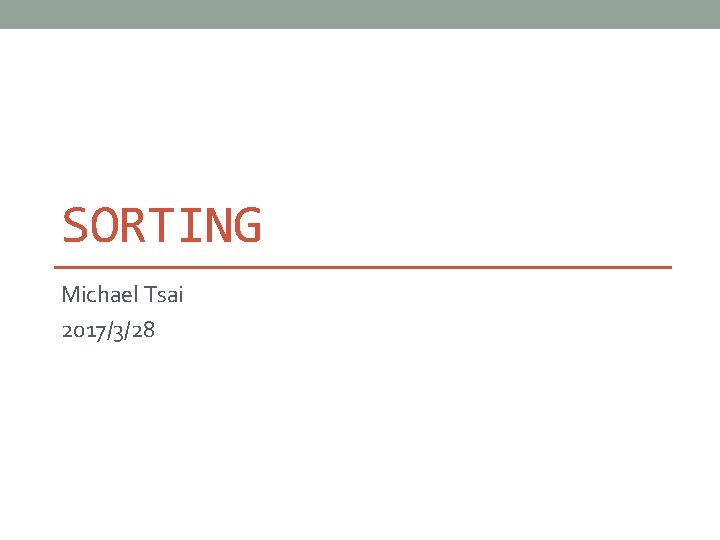
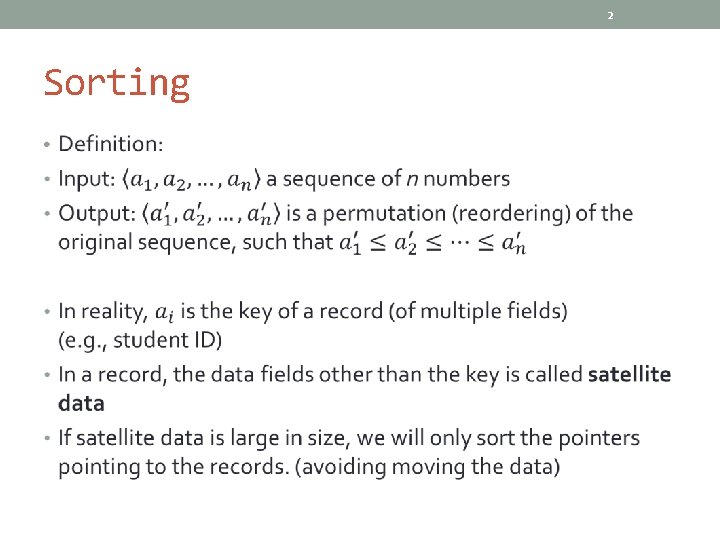
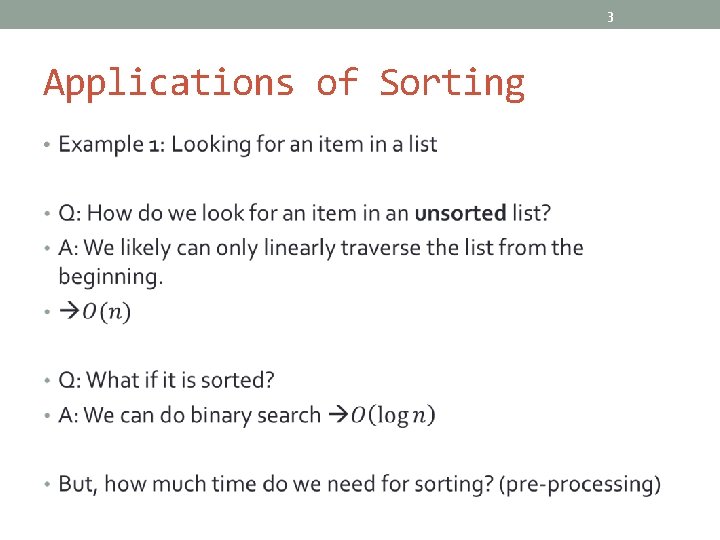
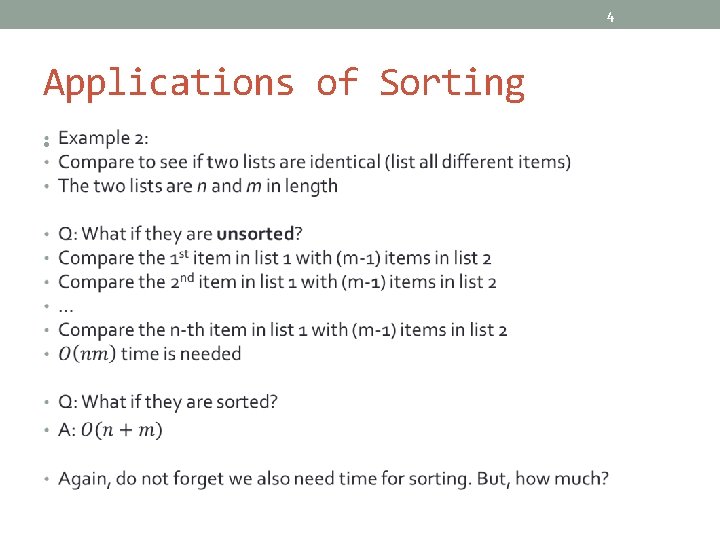
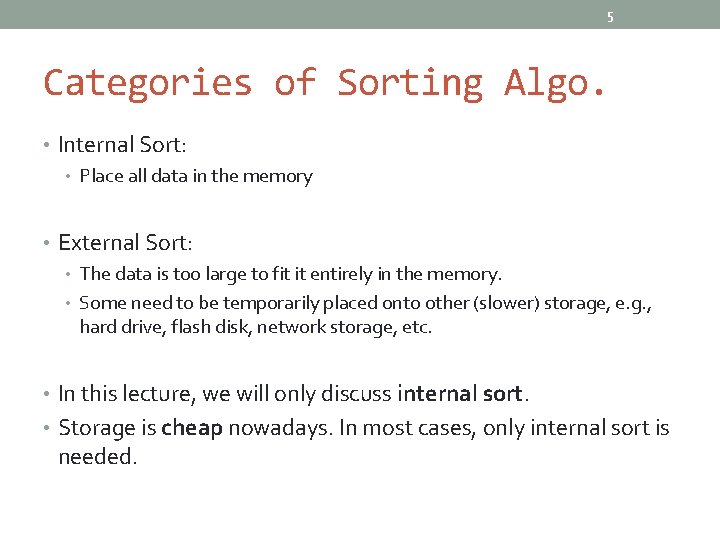
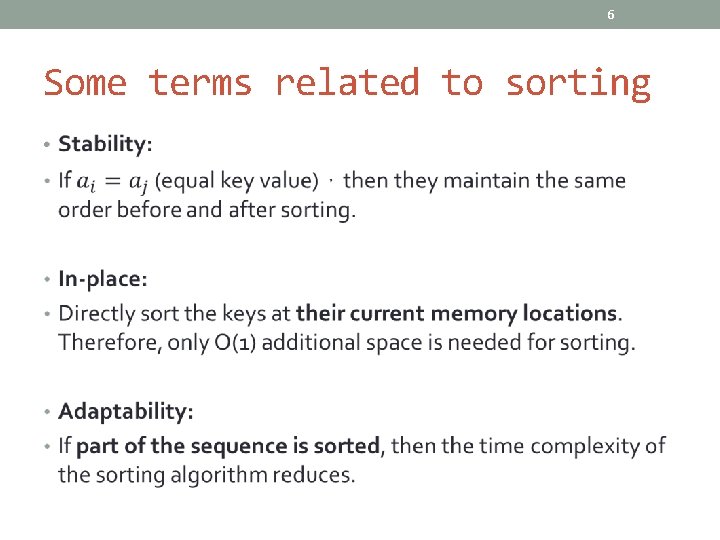
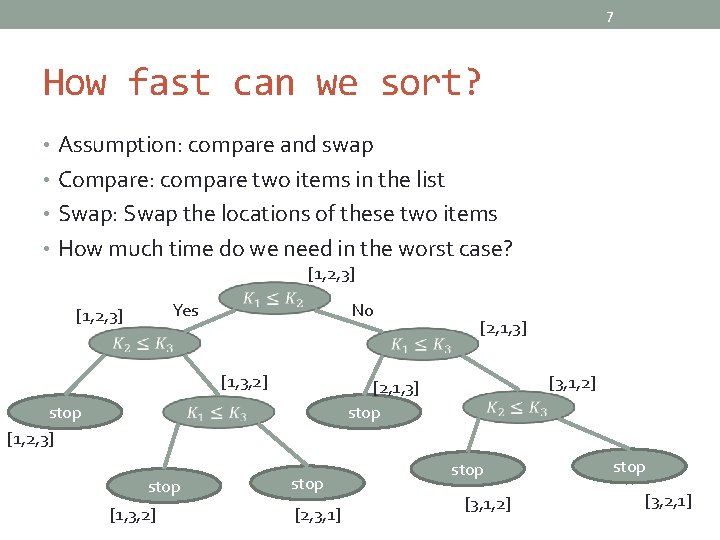
![8 Decision tree for sorting [1, 2, 3] [2, 1, 3] [1, 3, 2] 8 Decision tree for sorting [1, 2, 3] [2, 1, 3] [1, 3, 2]](https://slidetodoc.com/presentation_image_h2/7d775adfc617fdcaa62f4a364da2c0d6/image-8.jpg)
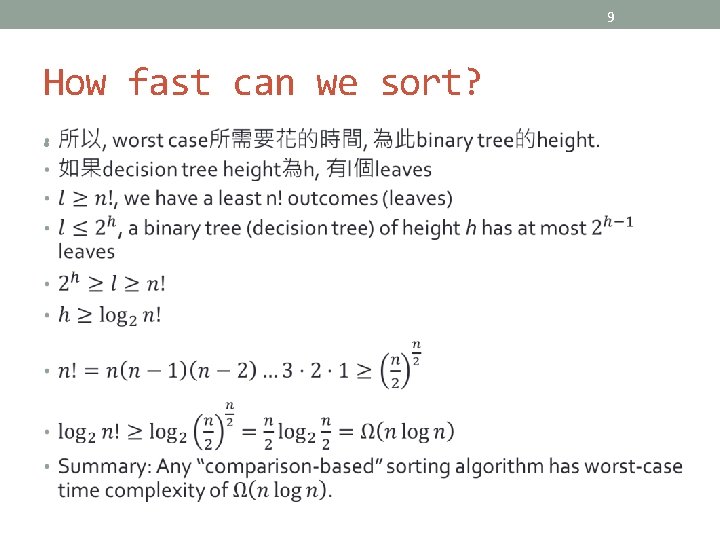
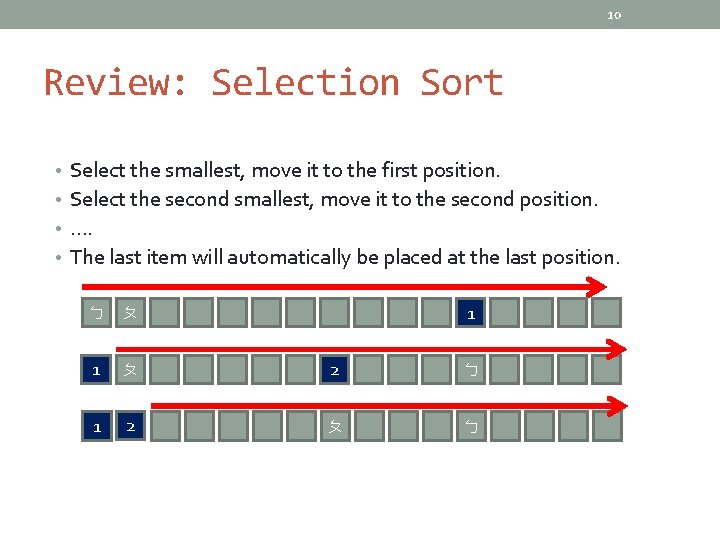
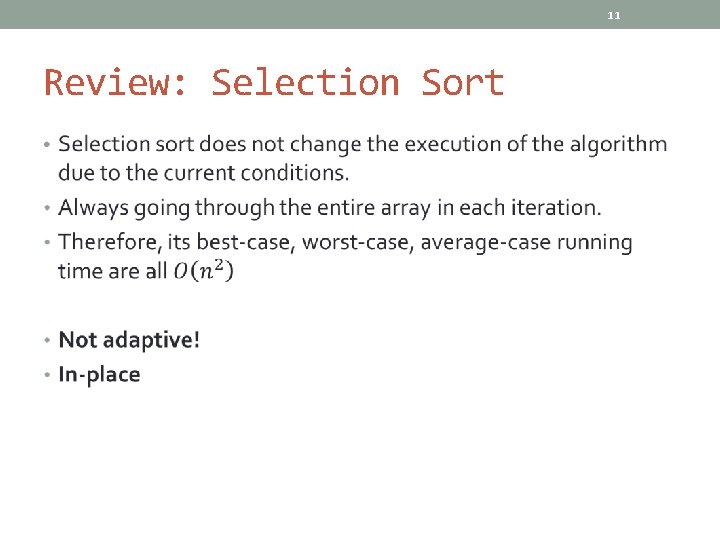
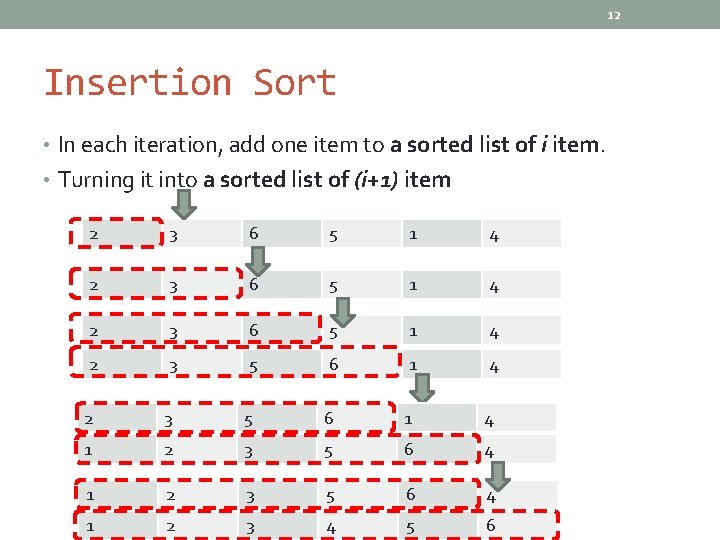
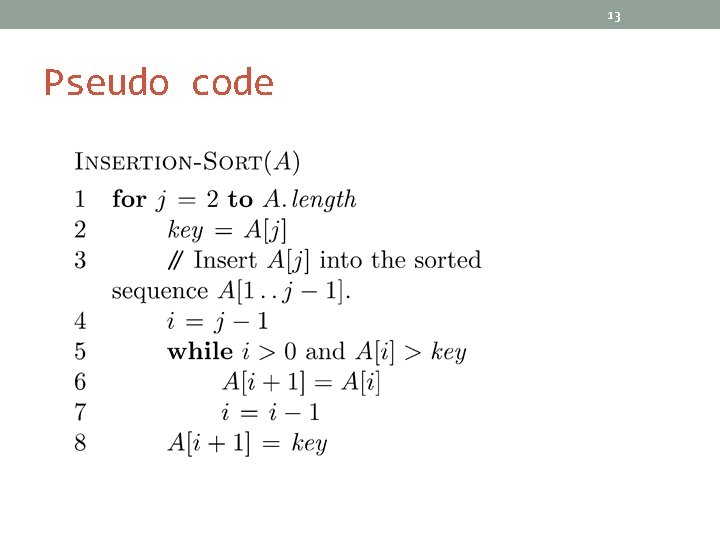
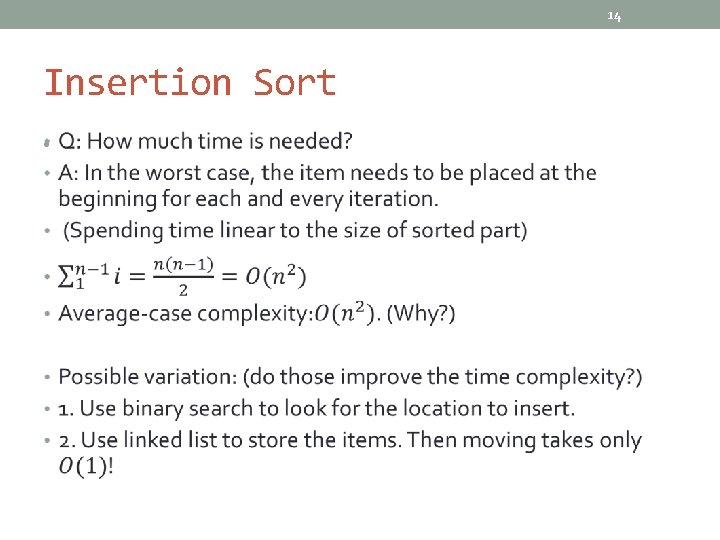
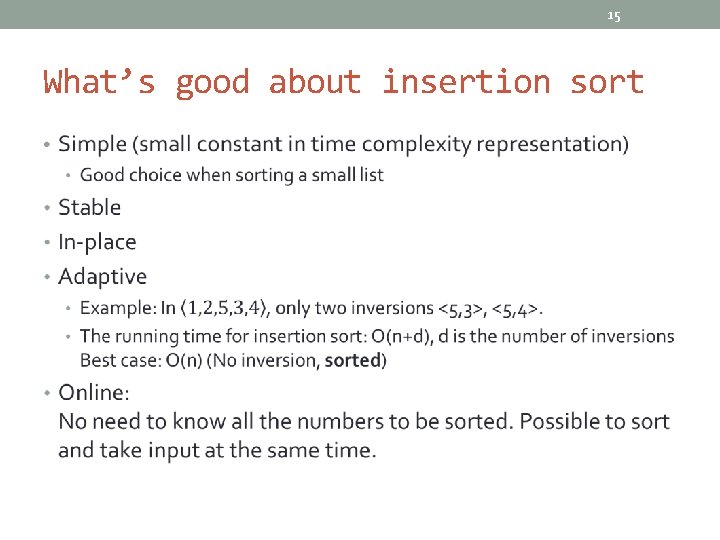
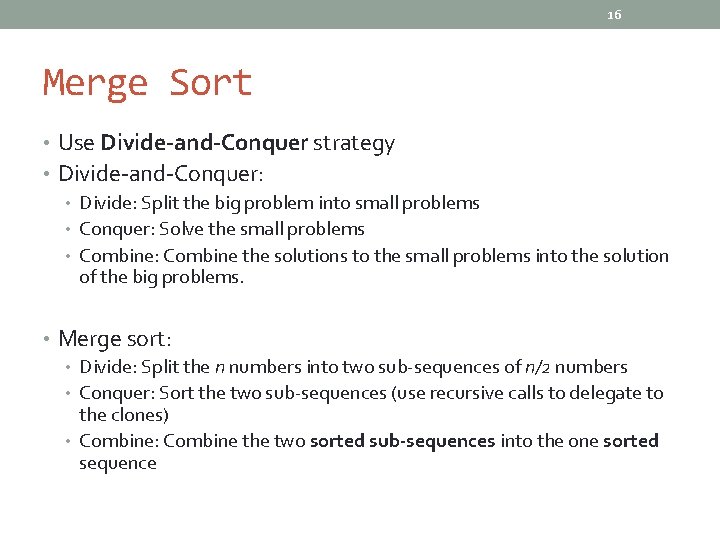
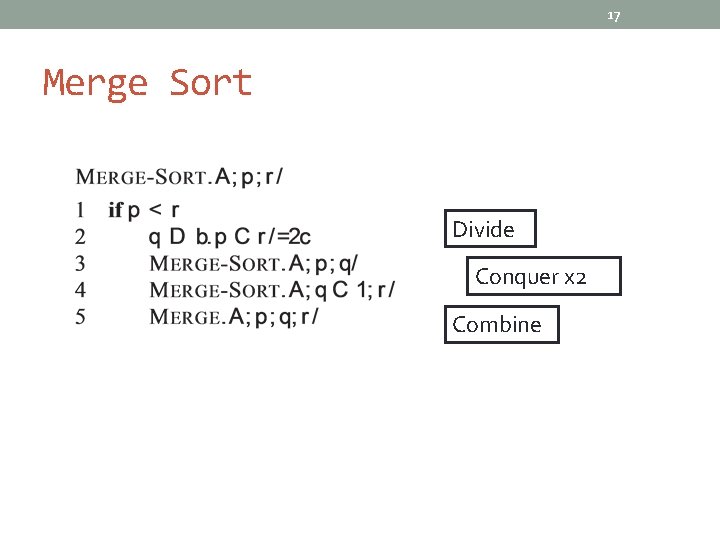
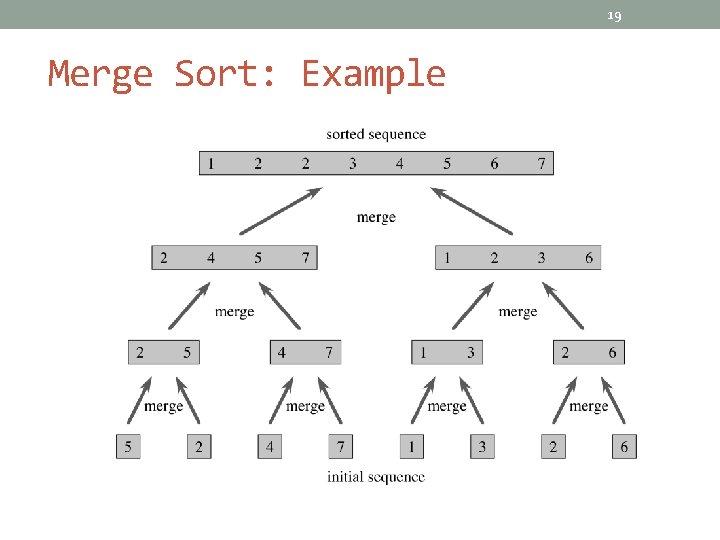
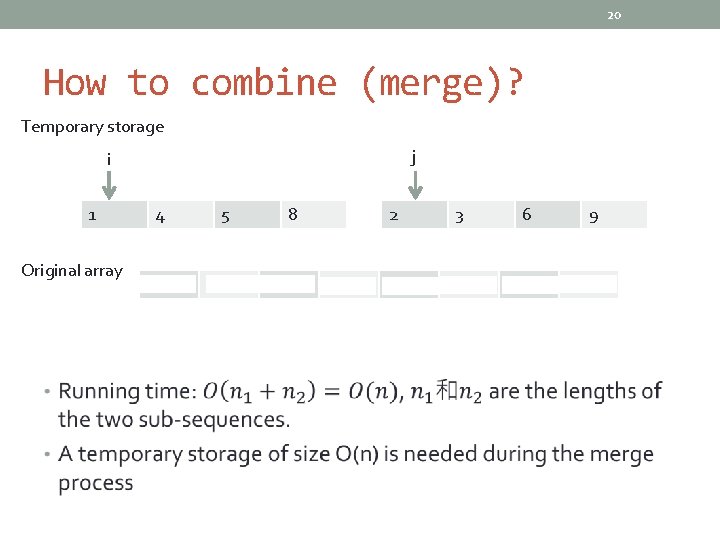
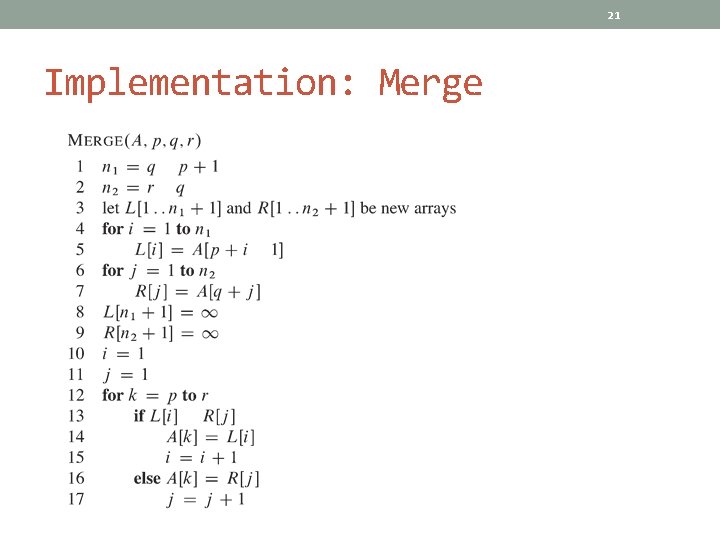
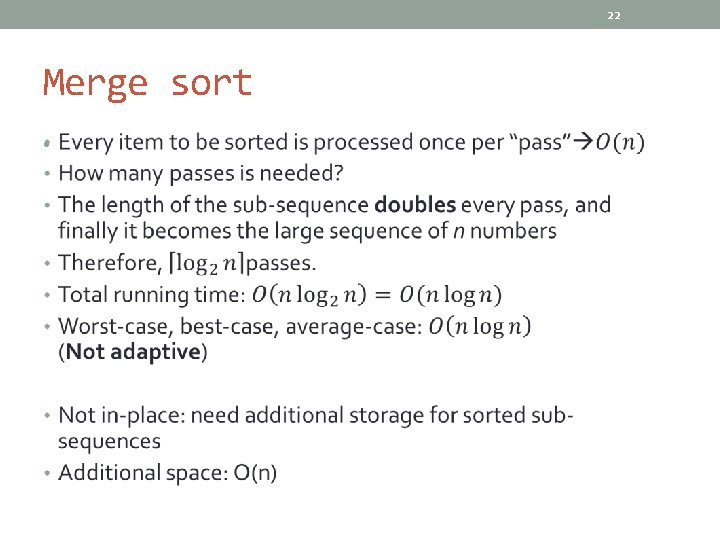
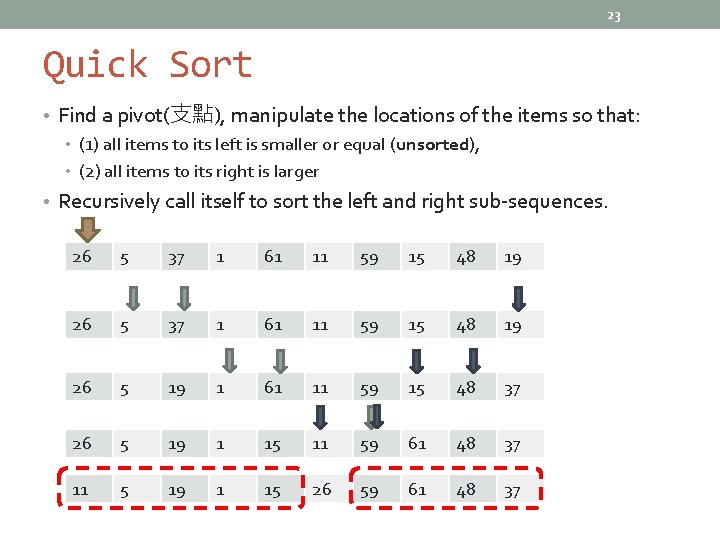
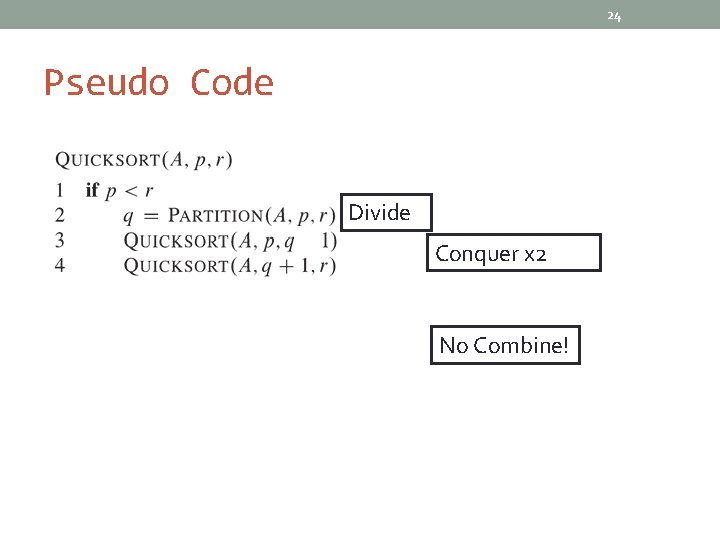
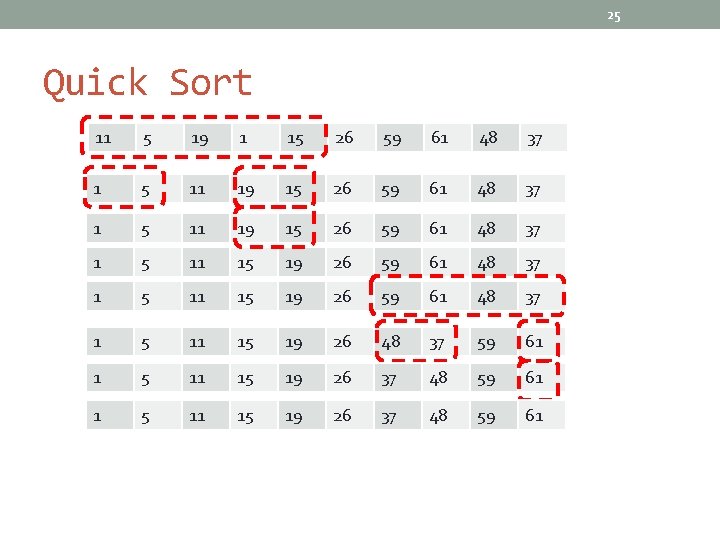
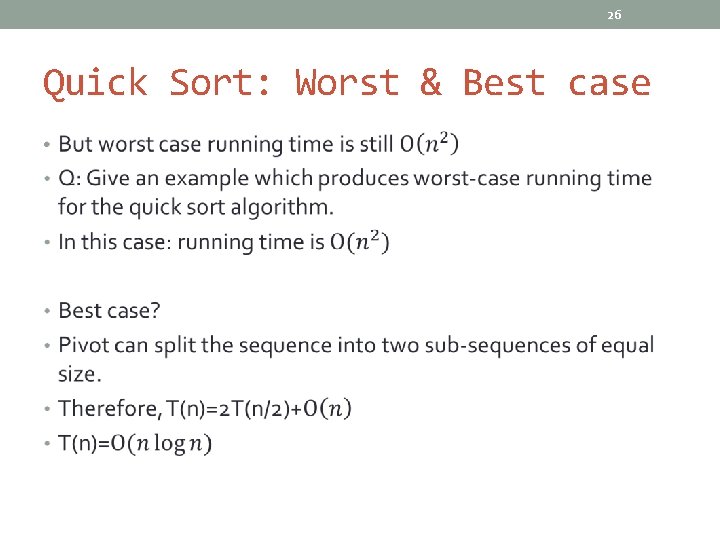
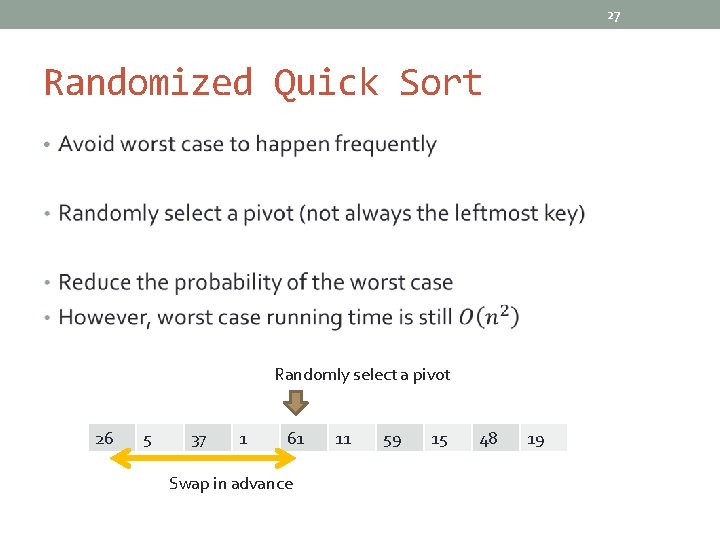
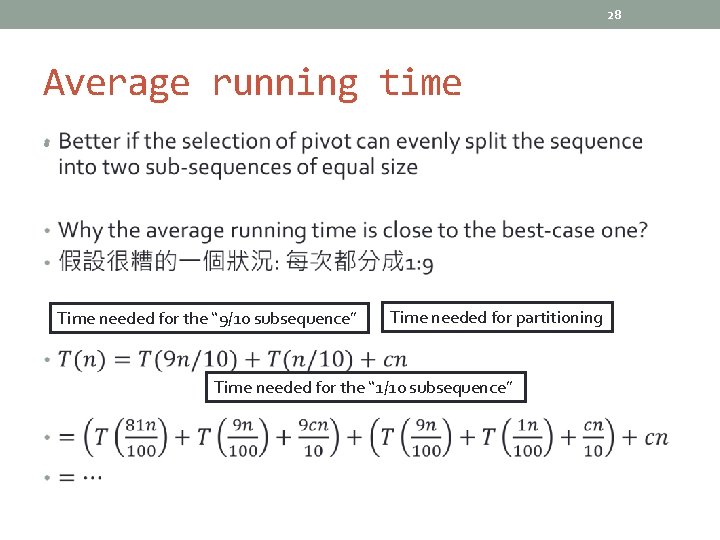
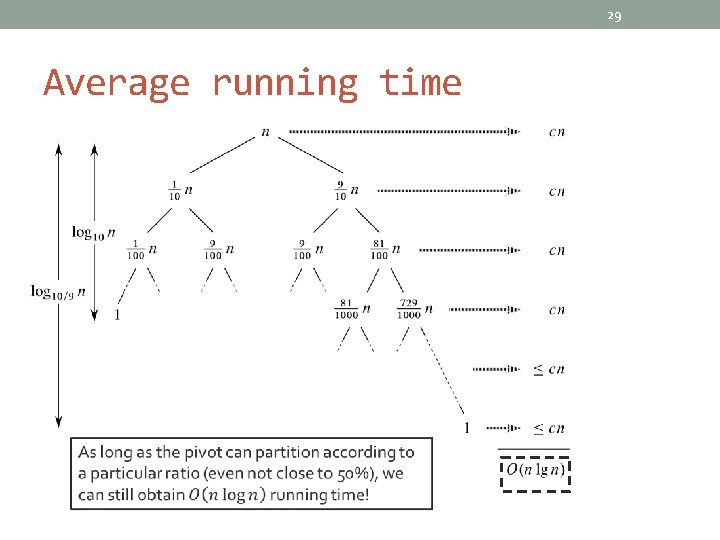
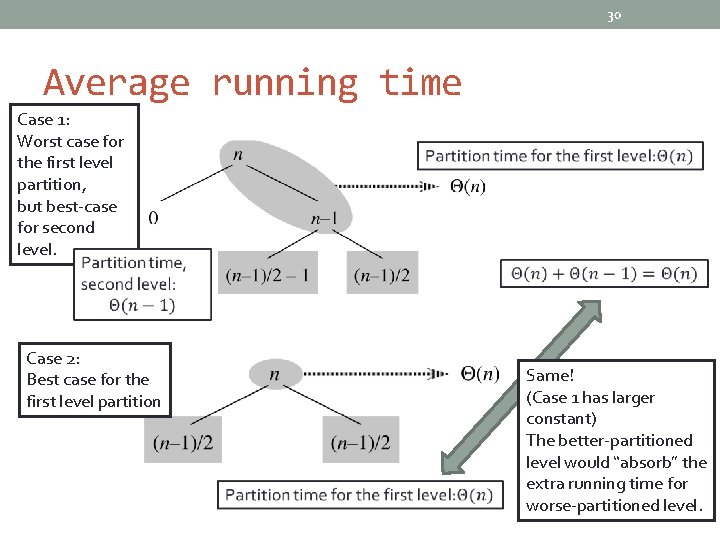
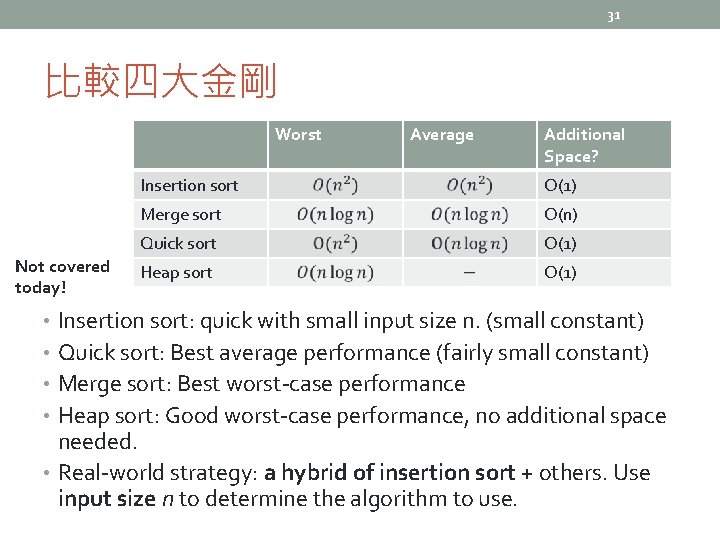
- Slides: 30
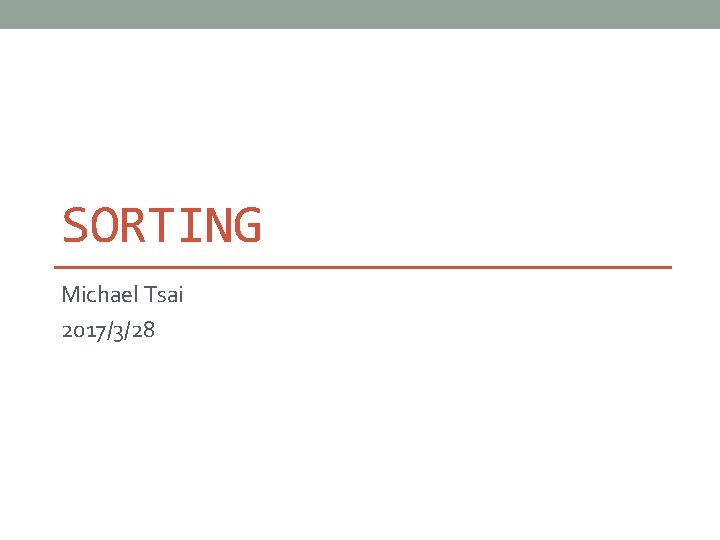
SORTING Michael Tsai 2017/3/28
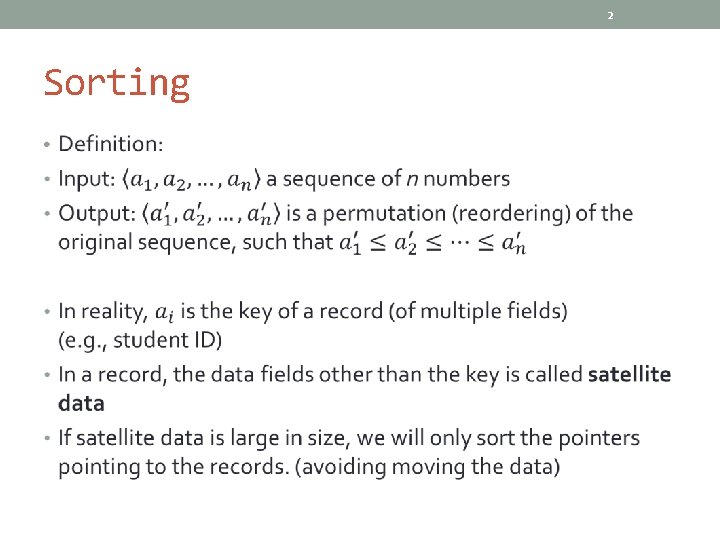
2 Sorting •
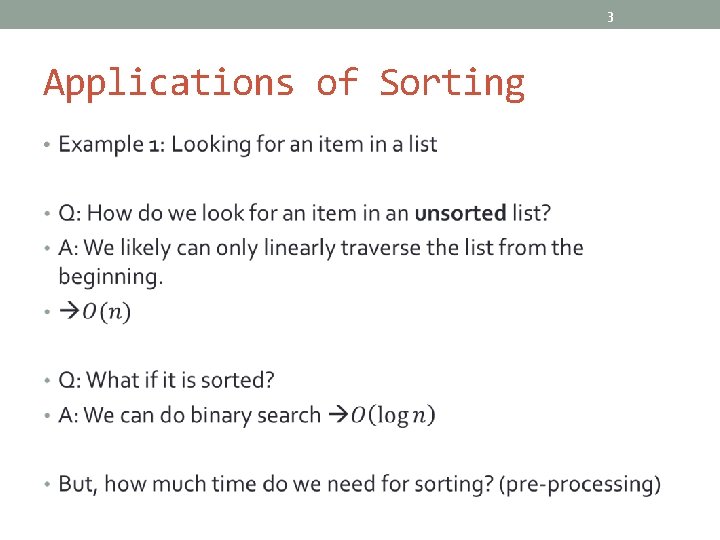
3 Applications of Sorting •
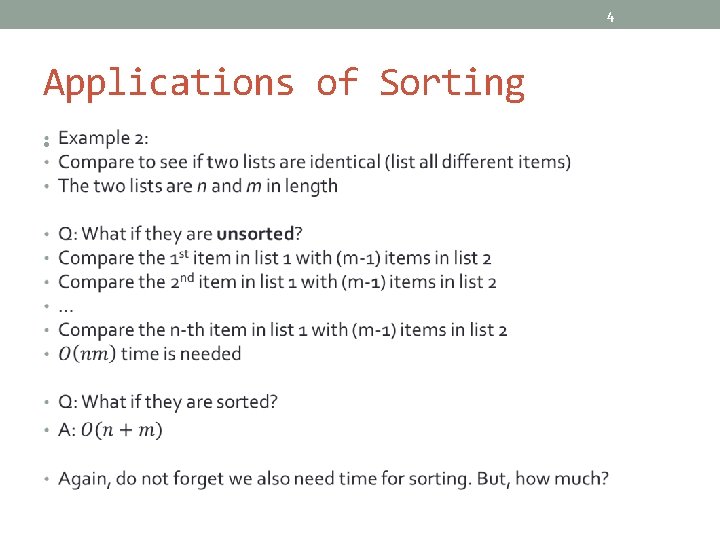
4 Applications of Sorting •
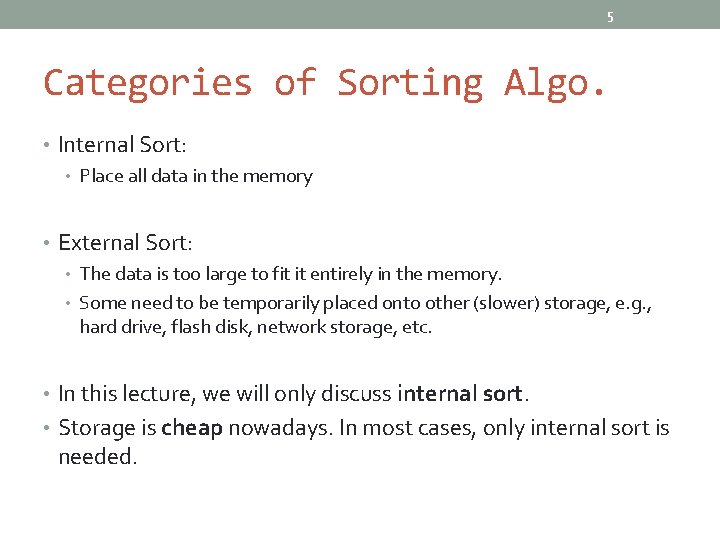
5 Categories of Sorting Algo. • Internal Sort: • Place all data in the memory • External Sort: • The data is too large to fit it entirely in the memory. • Some need to be temporarily placed onto other (slower) storage, e. g. , hard drive, flash disk, network storage, etc. • In this lecture, we will only discuss internal sort. • Storage is cheap nowadays. In most cases, only internal sort is needed.
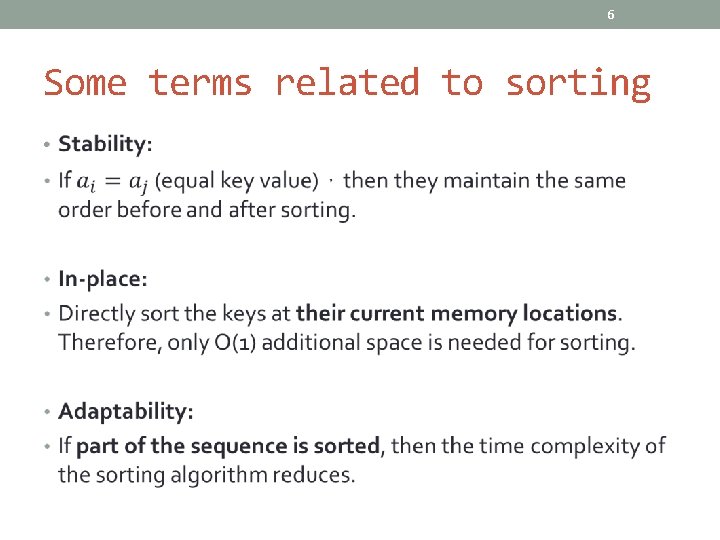
6 Some terms related to sorting •
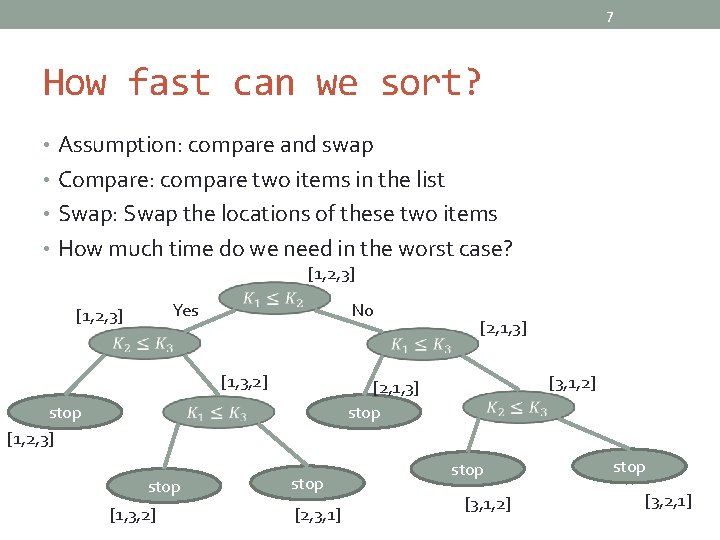
7 How fast can we sort? • Assumption: compare and swap • Compare: compare two items in the list • Swap: Swap the locations of these two items • How much time do we need in the worst case? [1, 2, 3] Yes [1, 2, 3] No [1, 3, 2] [3, 1, 2] [2, 1, 3] stop [1, 2, 3] stop [2, 1, 3] stop [2, 3, 1] stop [3, 1, 2] stop [3, 2, 1]
![8 Decision tree for sorting 1 2 3 2 1 3 1 3 2 8 Decision tree for sorting [1, 2, 3] [2, 1, 3] [1, 3, 2]](https://slidetodoc.com/presentation_image_h2/7d775adfc617fdcaa62f4a364da2c0d6/image-8.jpg)
8 Decision tree for sorting [1, 2, 3] [2, 1, 3] [1, 3, 2] [2, 1, 3] stop [1, 2, 3] stop [1, 3, 2] • stop [2, 3, 1] stop [3, 1, 2] stop [3, 2, 1]
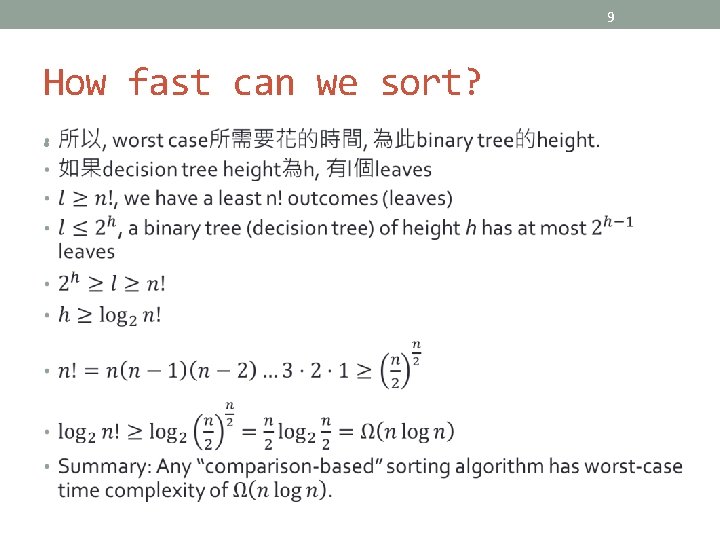
9 How fast can we sort? •
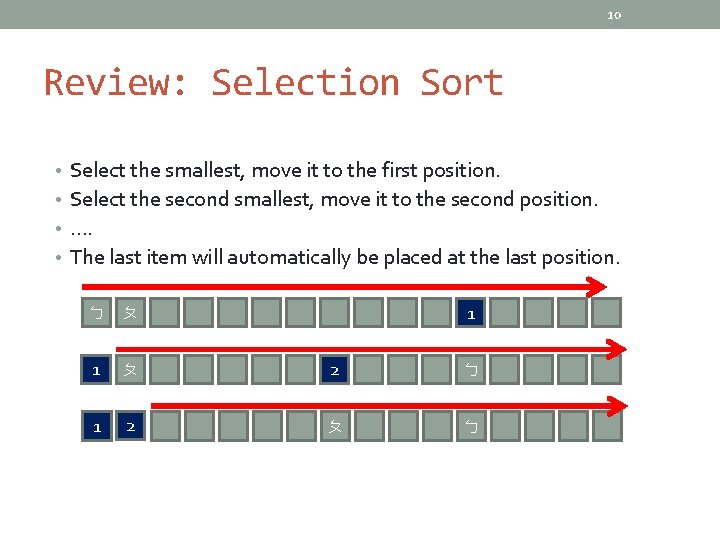
10 Review: Selection Sort • Select the smallest, move it to the first position. • Select the second smallest, move it to the second position. • …. • The last item will automatically be placed at the last position. ㄅ ㄆ 1 1 ㄆ 2 ㄅ 1 2 ㄆ ㄅ
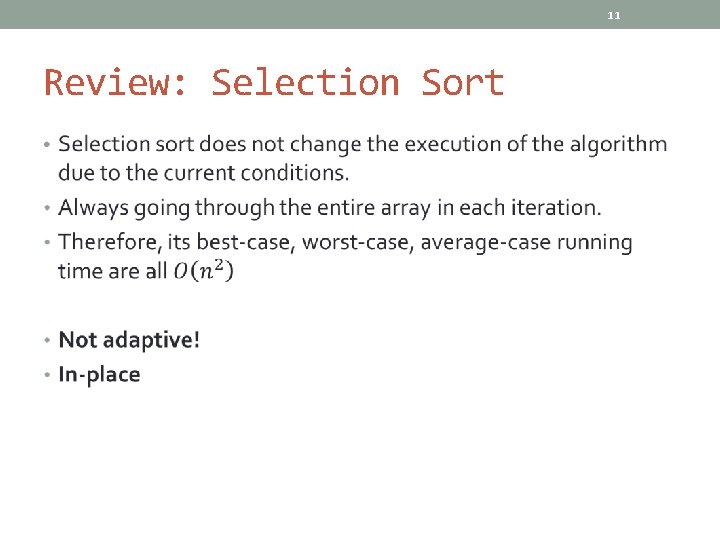
11 Review: Selection Sort •
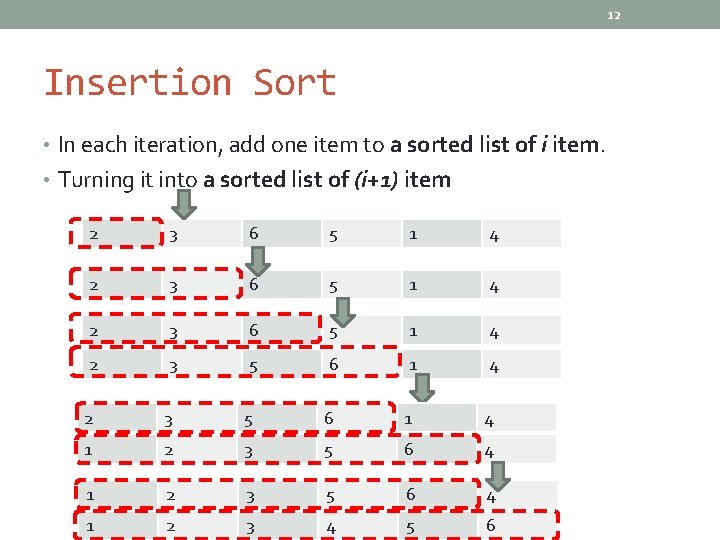
12 Insertion Sort • In each iteration, add one item to a sorted list of i item. • Turning it into a sorted list of (i+1) item 2 3 6 5 1 4 2 3 5 6 1 4 1 2 3 5 6 4 1 2 3 4 5 6
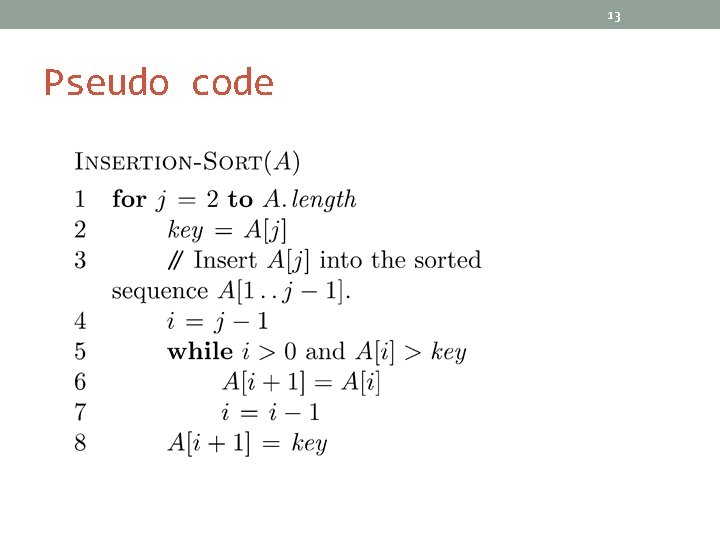
13 Pseudo code
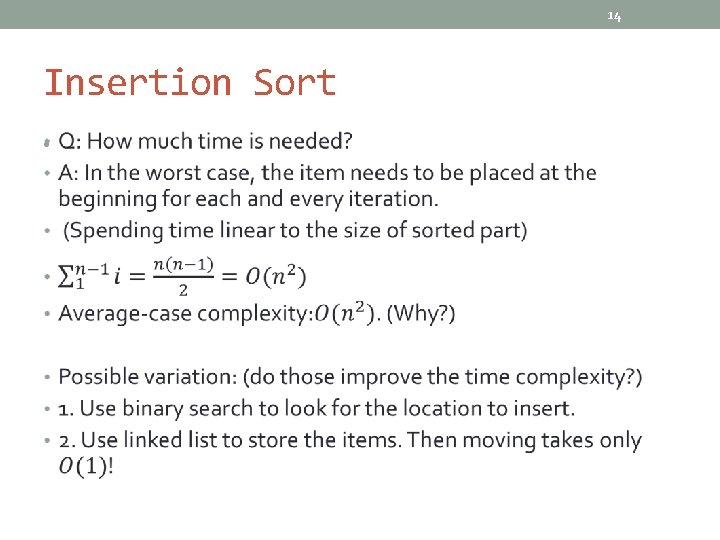
14 Insertion Sort •
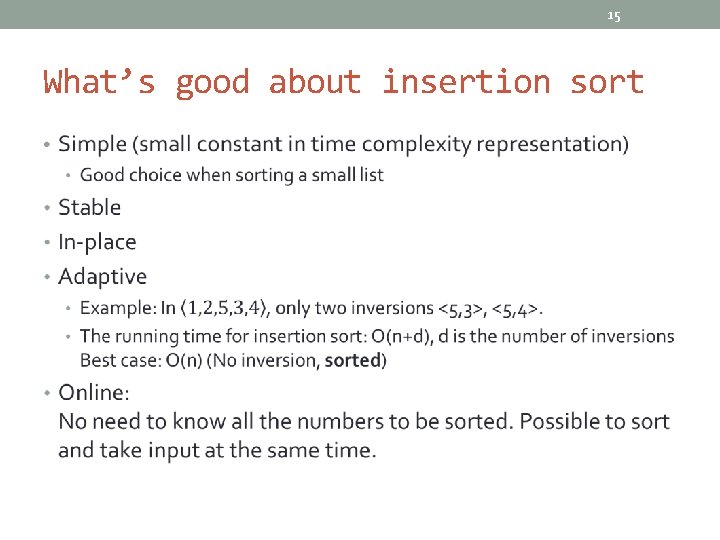
15 What’s good about insertion sort •
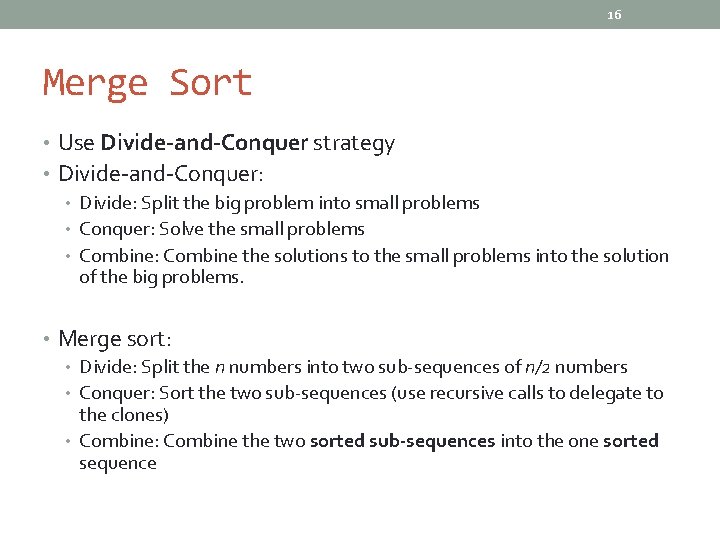
16 Merge Sort • Use Divide-and-Conquer strategy • Divide-and-Conquer: • Divide: Split the big problem into small problems • Conquer: Solve the small problems • Combine: Combine the solutions to the small problems into the solution of the big problems. • Merge sort: • Divide: Split the n numbers into two sub-sequences of n/2 numbers • Conquer: Sort the two sub-sequences (use recursive calls to delegate to the clones) • Combine: Combine the two sorted sub-sequences into the one sorted sequence
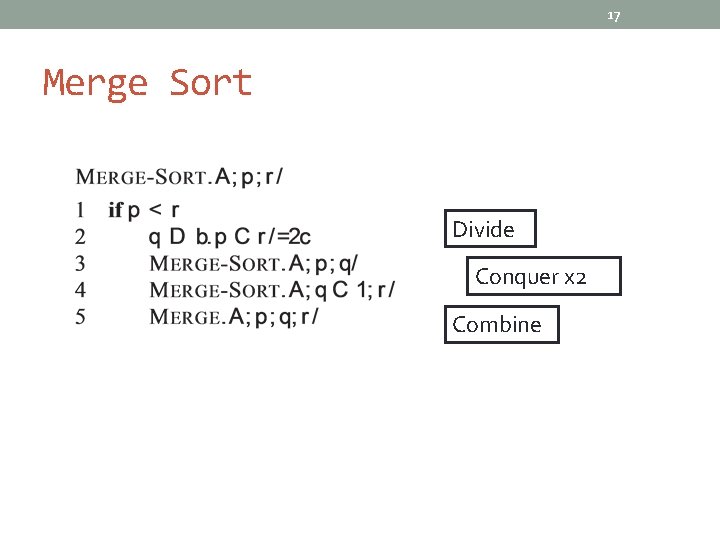
17 Merge Sort Divide Conquer x 2 Combine
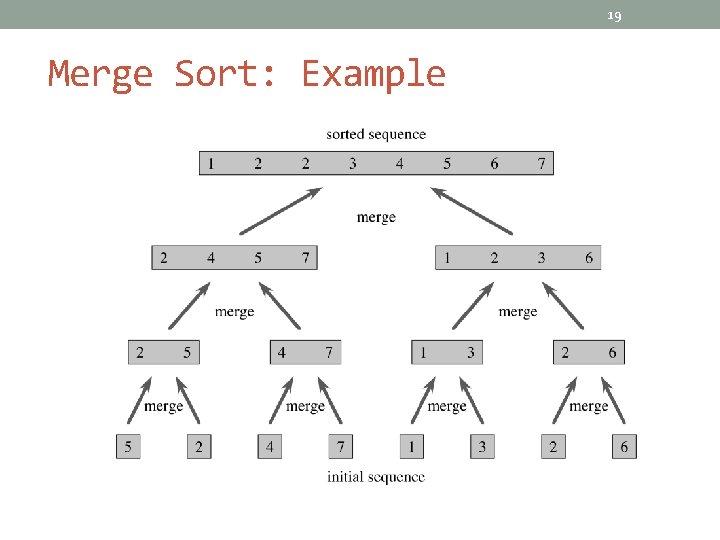
19 Merge Sort: Example
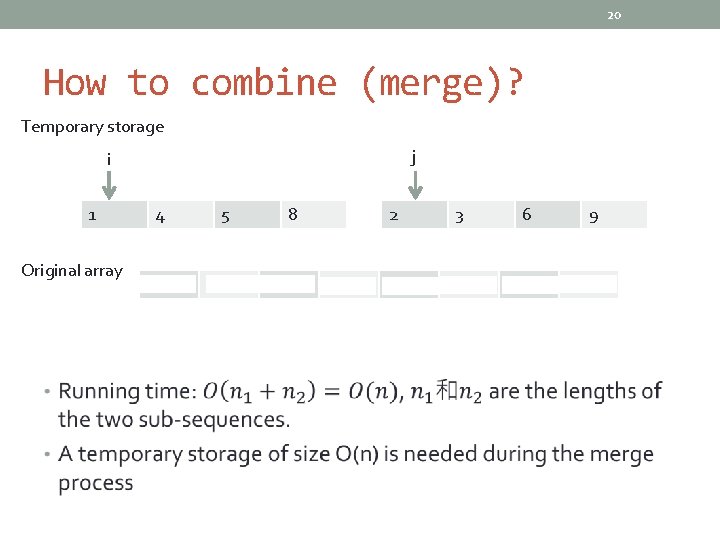
20 How to combine (merge)? Temporary storage j i 1 Original array 4 1 5 2 8 3 2 4 5 3 6 6 8 9 9
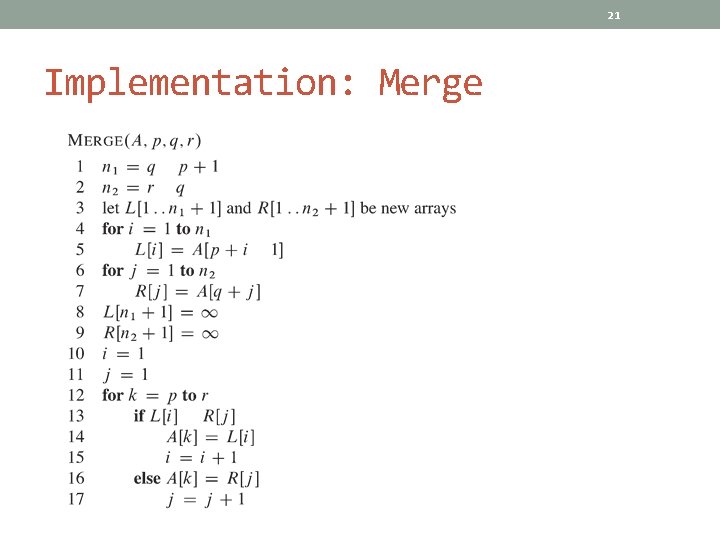
21 Implementation: Merge
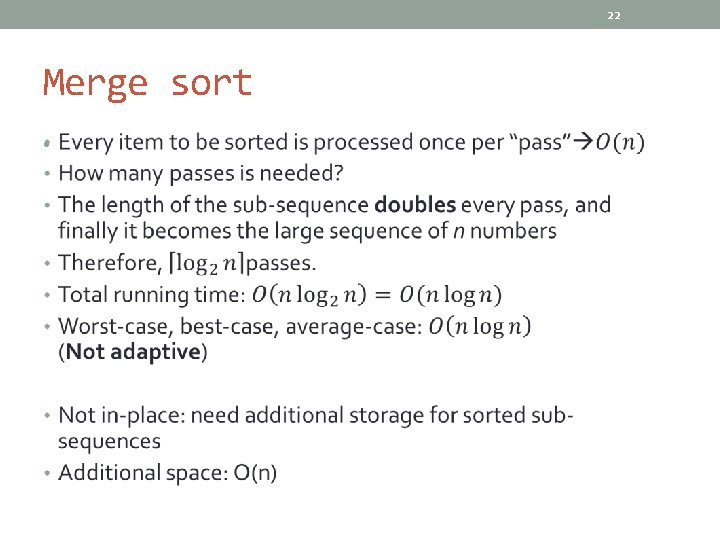
22 Merge sort •
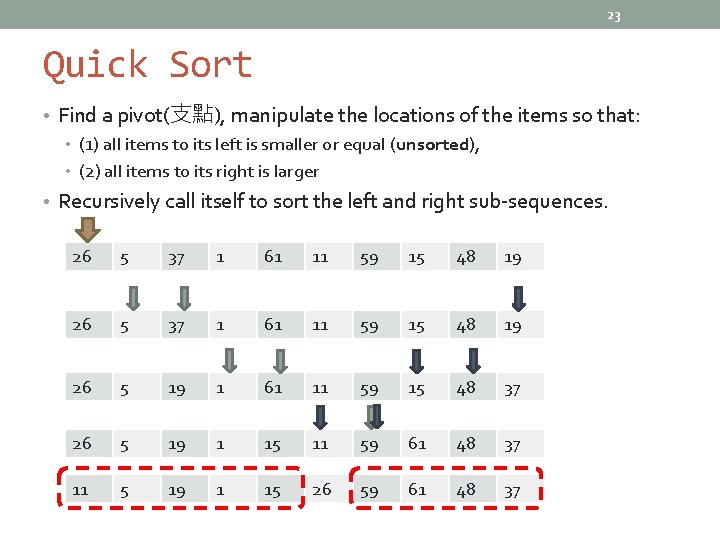
23 Quick Sort • Find a pivot(支點), manipulate the locations of the items so that: • (1) all items to its left is smaller or equal (unsorted), • (2) all items to its right is larger • Recursively call itself to sort the left and right sub-sequences. 26 5 37 1 61 11 59 15 48 19 26 5 19 1 61 11 59 15 48 37 26 5 19 1 15 11 59 61 48 37 11 5 19 1 15 26 59 61 48 37
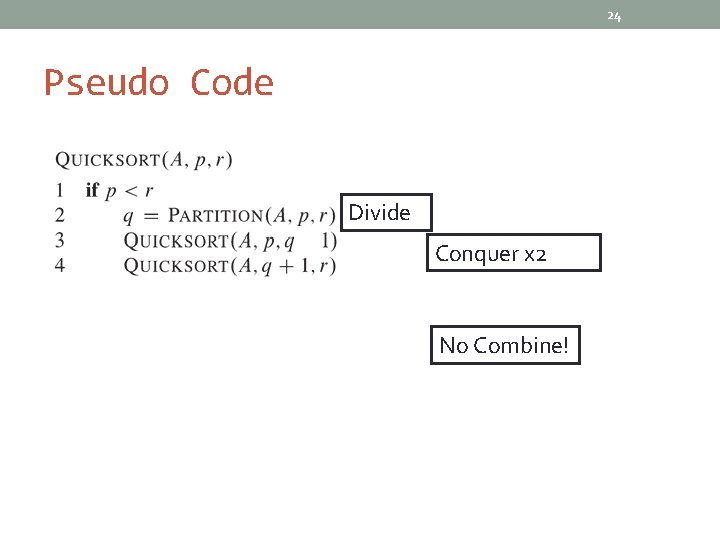
24 Pseudo Code Divide Conquer x 2 No Combine!
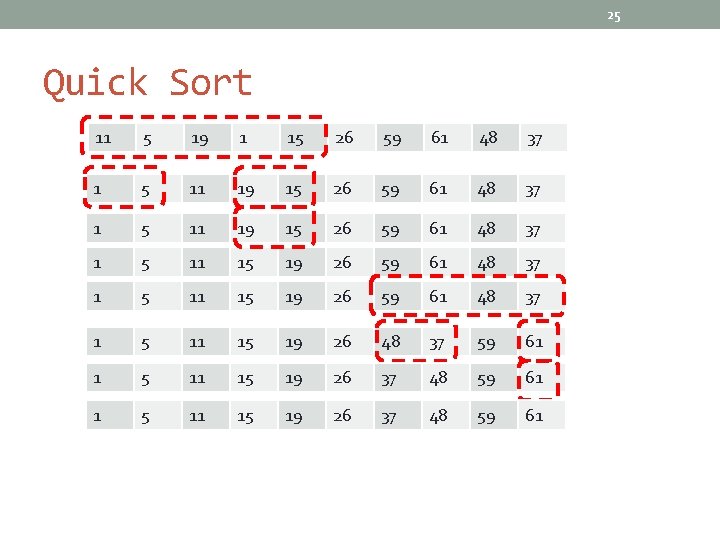
25 Quick Sort 11 5 19 1 15 26 59 61 48 37 1 5 11 19 15 26 59 61 48 37 1 5 11 15 19 26 48 37 59 61 1 5 11 15 19 26 37 48 59 61
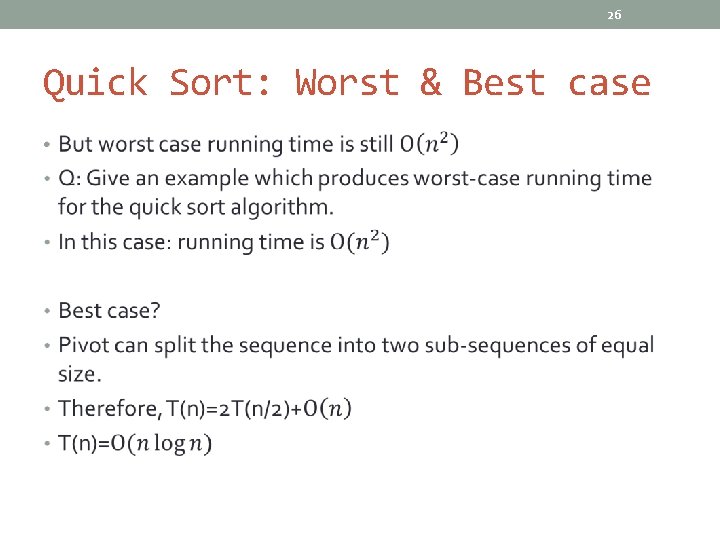
26 Quick Sort: Worst & Best case •
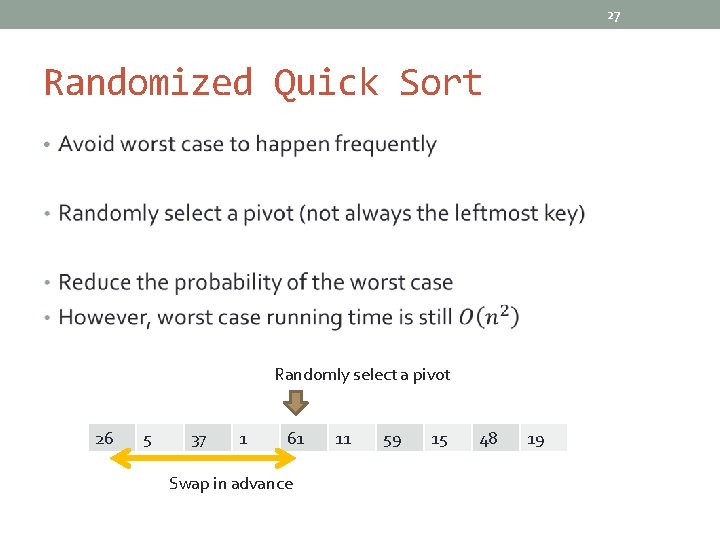
27 Randomized Quick Sort • Randomly select a pivot 26 5 37 1 61 Swap in advance 11 59 15 48 19
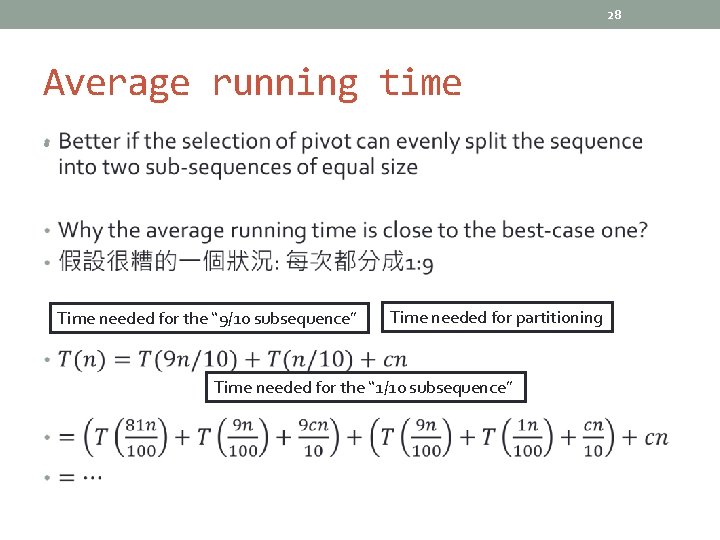
28 Average running time • Time needed for the “ 9/10 subsequence” Time needed for partitioning Time needed for the “ 1/10 subsequence”
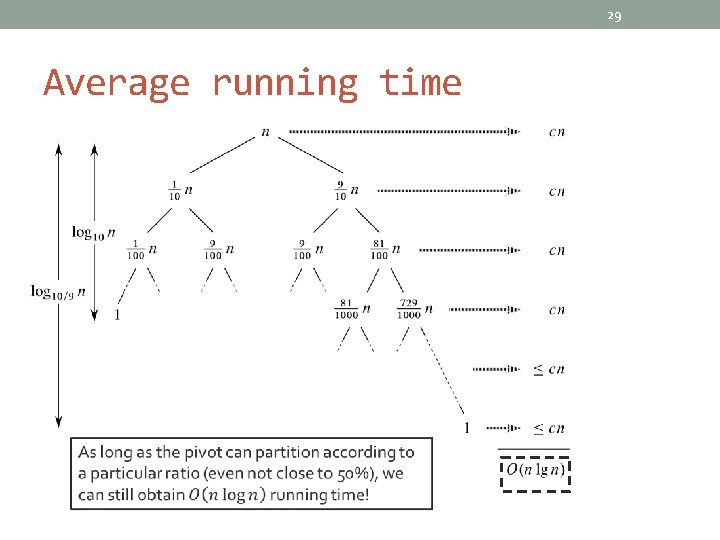
29 Average running time
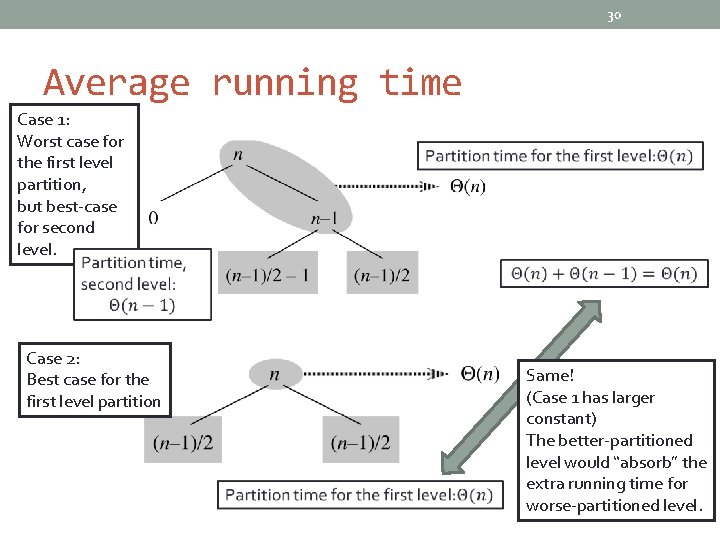
30 Average running time Case 1: Worst case for the first level partition, but best-case for second level. Case 2: Best case for the first level partition Same! (Case 1 has larger constant) The better-partitioned level would “absorb” the extra running time for worse-partitioned level.
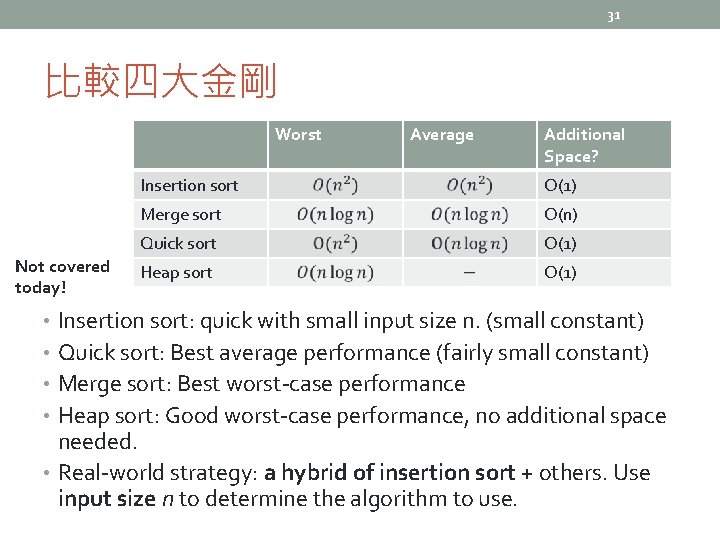
31 比較四大金剛 Worst Not covered today! Average Additional Space? Insertion sort O(1) Merge sort O(n) Quick sort O(1) Heap sort O(1) • Insertion sort: quick with small input size n. (small constant) • Quick sort: Best average performance (fairly small constant) • Merge sort: Best worst-case performance • Heap sort: Good worst-case performance, no additional space needed. • Real-world strategy: a hybrid of insertion sort + others. Use input size n to determine the algorithm to use.
Internal sorting and external sorting
Yu dai tsai
Eddie tsai
Splunk ftr
Chin-chung tsai
Wen-hsuan tsai
Vukovar rat
Introduction to snmp
Shannon tsai
Robin tsai
Effectively communicate meaning
Beth tsai
Charlene tsai
Physical and chemical properties sorting activity
External sorting techniques
Nyc recycling sorting
Card sorting machine
Evaporation mixtures
Efficiency of sorting algorithms
Internal sorting
Internal sorting takes place in
External sorting algorithms
Quadratic sorting algorithms
Tools and equipment in swine production
Sorting dan searching
Lesson 2 assignment a sort of sorts answers
Sorting quadrilaterals
10 methods of separating mixtures
Grading in food processing
Lower bounds for sorting
Sediment sorting