Sorting Dr Yingwu Zhu Sorting n n Consider
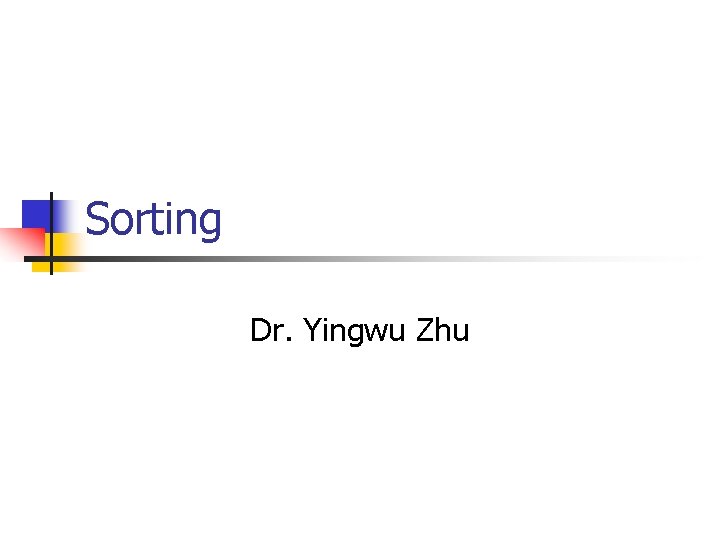
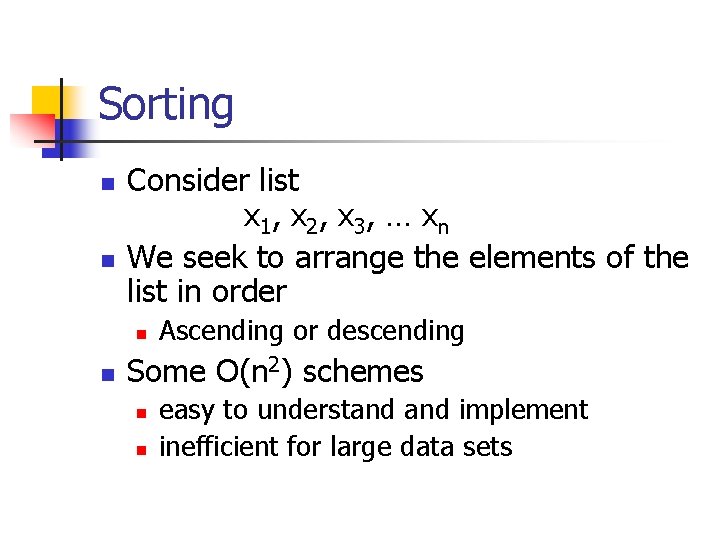
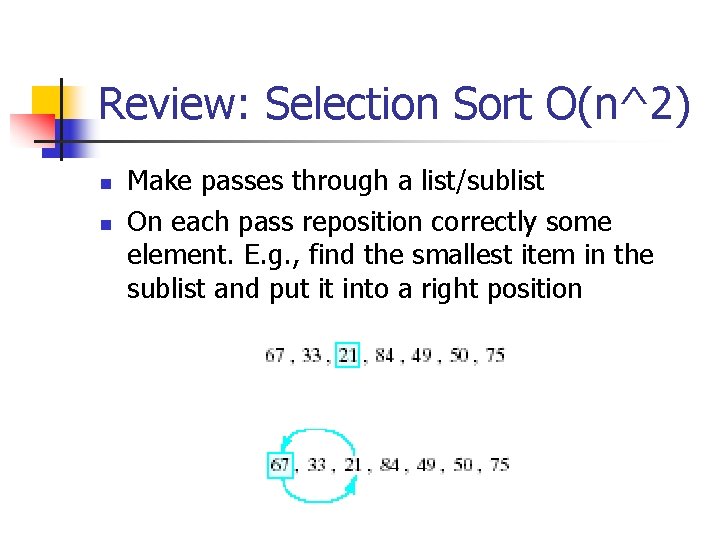
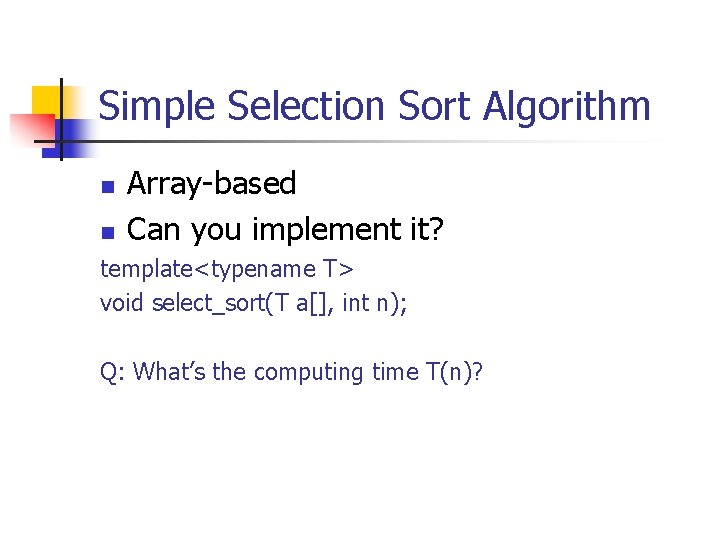
![Simple Selection Sort Algorithm template <typename T> void select_sort(int a[], int n) { for Simple Selection Sort Algorithm template <typename T> void select_sort(int a[], int n) { for](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-5.jpg)
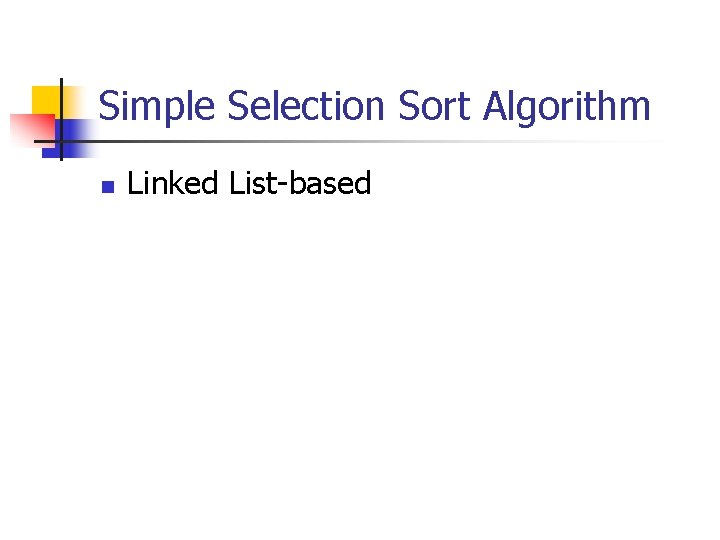
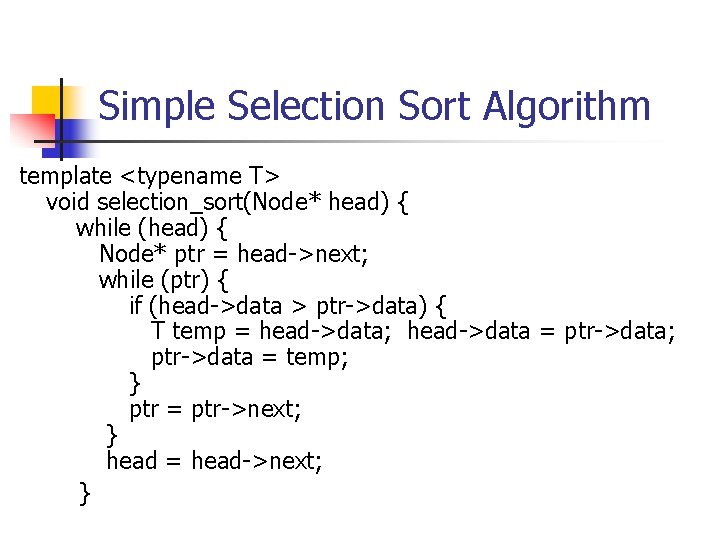
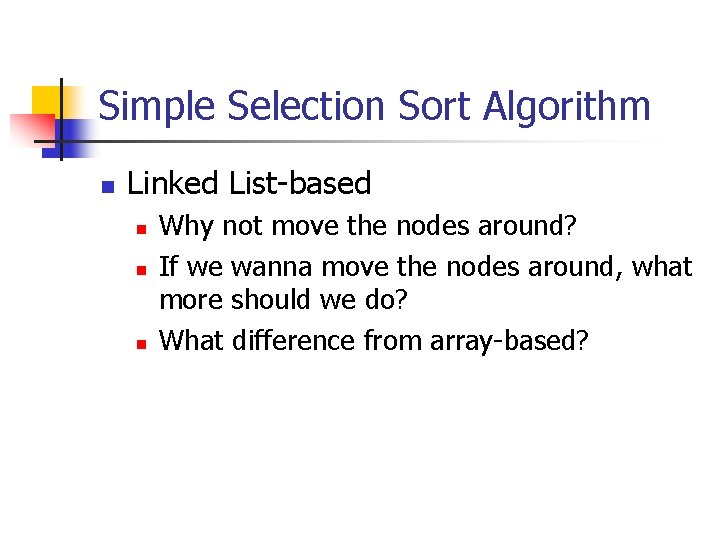
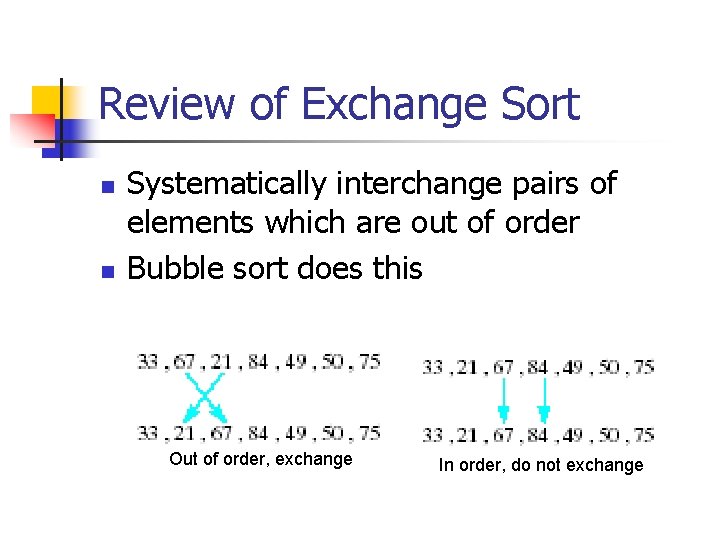
![Bubble Sort n n void bubble_sort(int a[], int n) Can you write one in Bubble Sort n n void bubble_sort(int a[], int n) Can you write one in](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-10.jpg)
![Bubble Sort void bubble_sort(int a[], int n) { int num_compares = n-1; //first should Bubble Sort void bubble_sort(int a[], int n) { int num_compares = n-1; //first should](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-11.jpg)
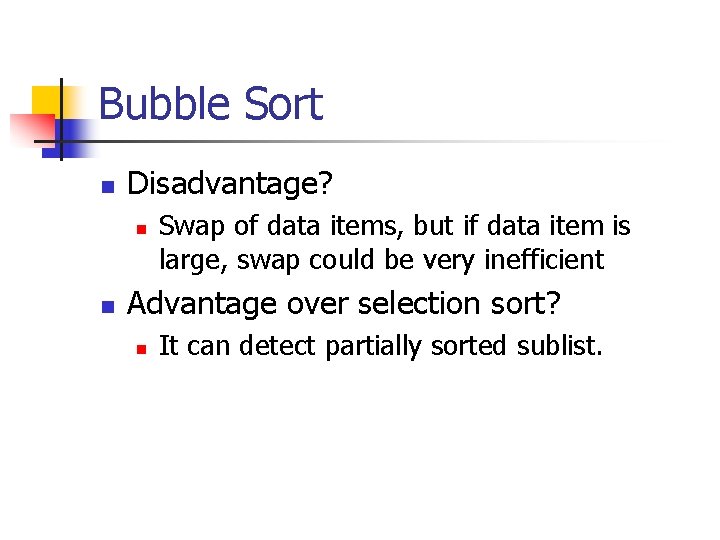
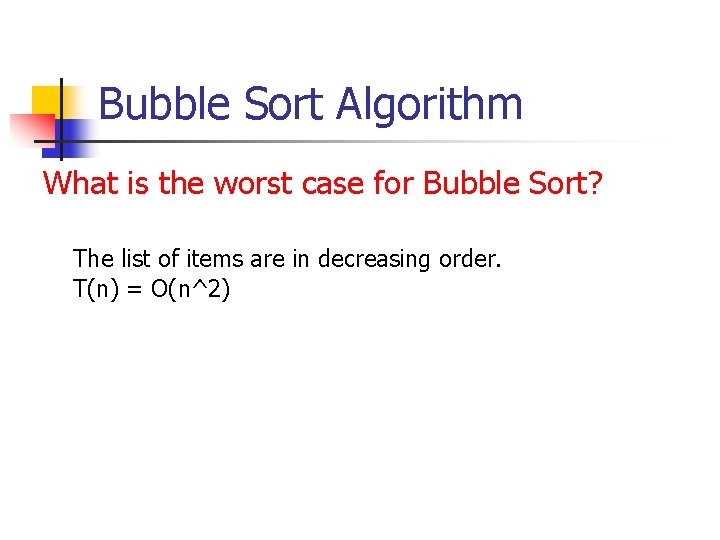
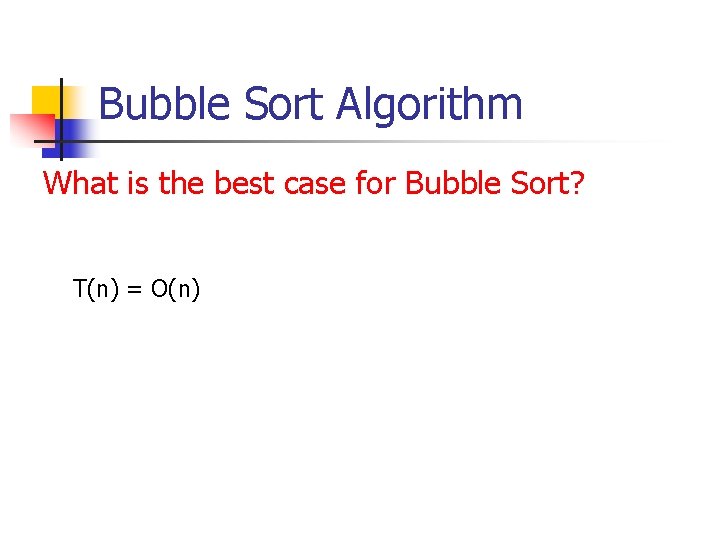
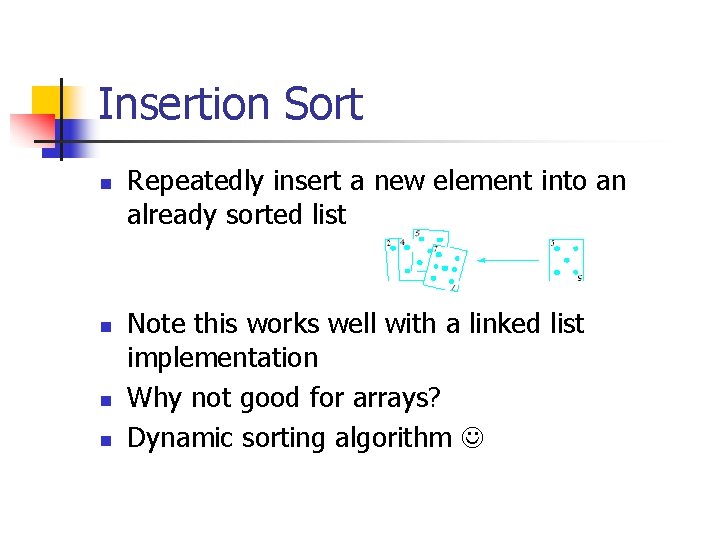
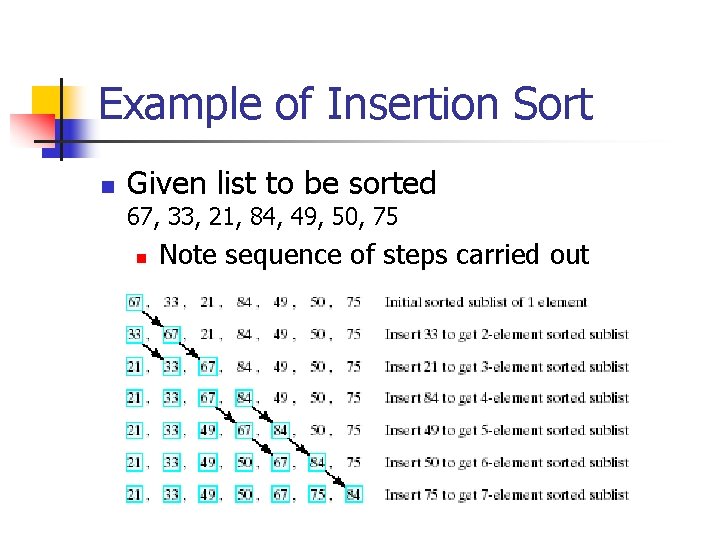
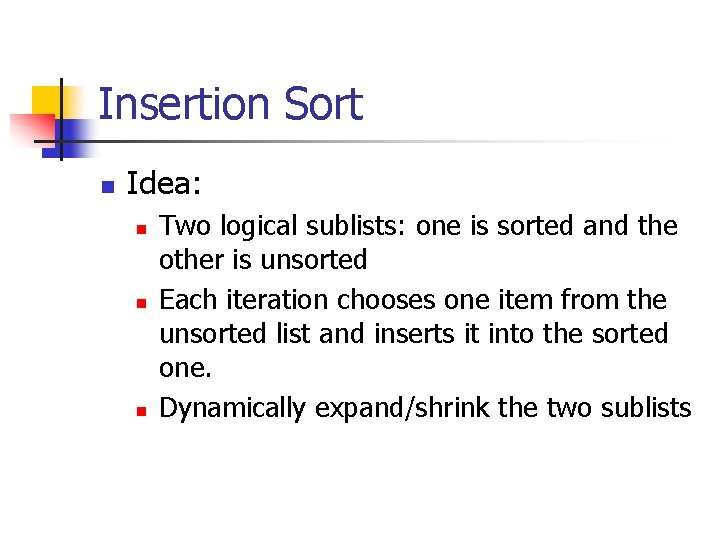
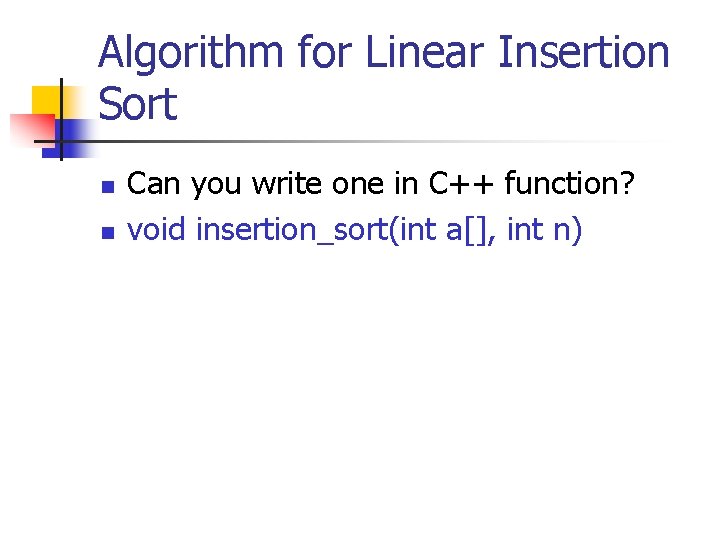
![Algorithm for Linear Insertion Sort void insertion_sort(int a[], int n) { int temp; for Algorithm for Linear Insertion Sort void insertion_sort(int a[], int n) { int temp; for](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-19.jpg)
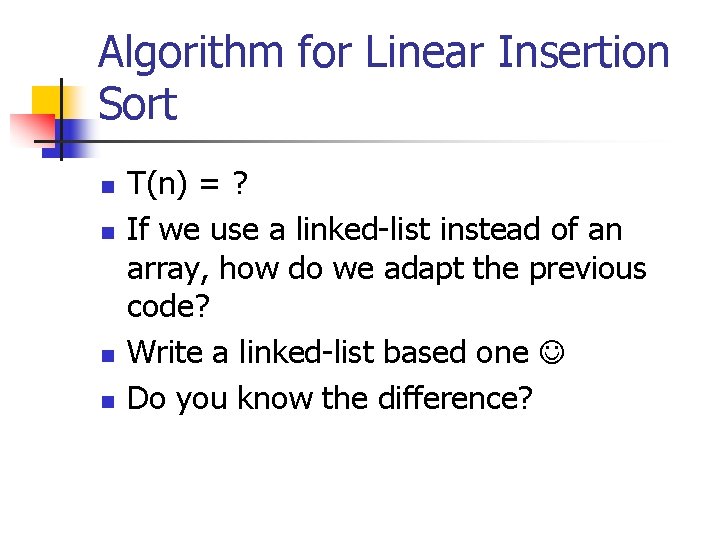
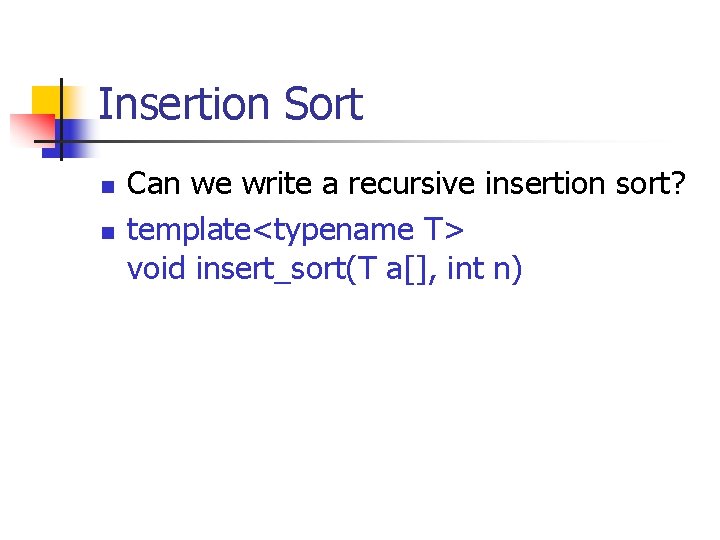
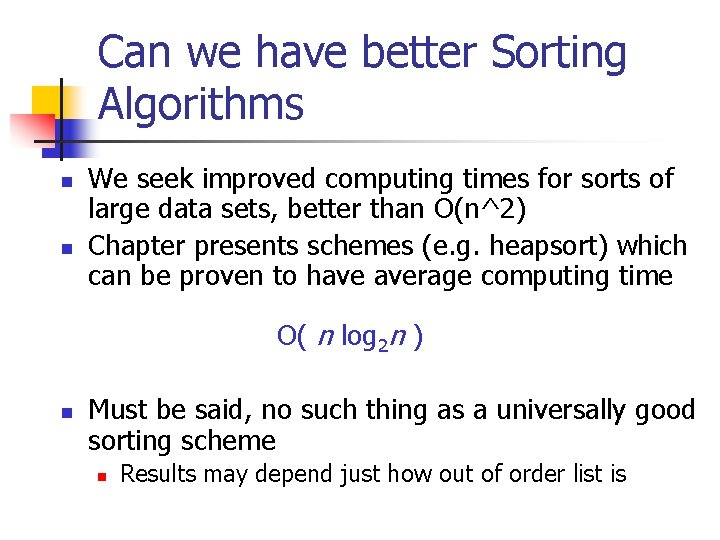
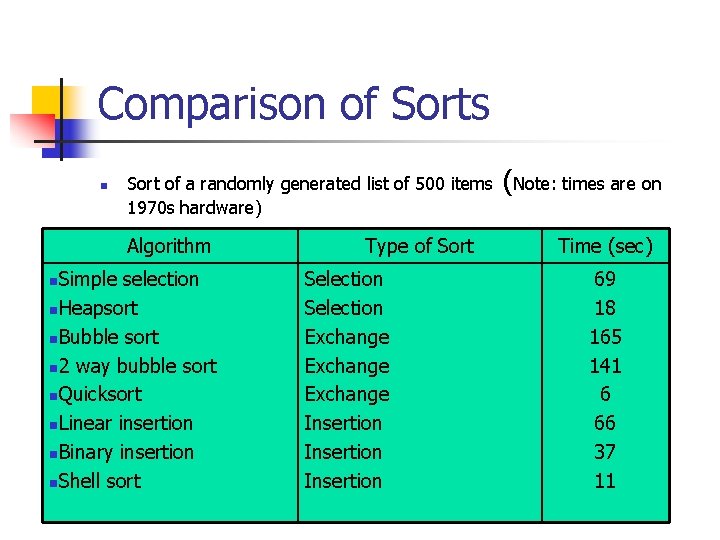
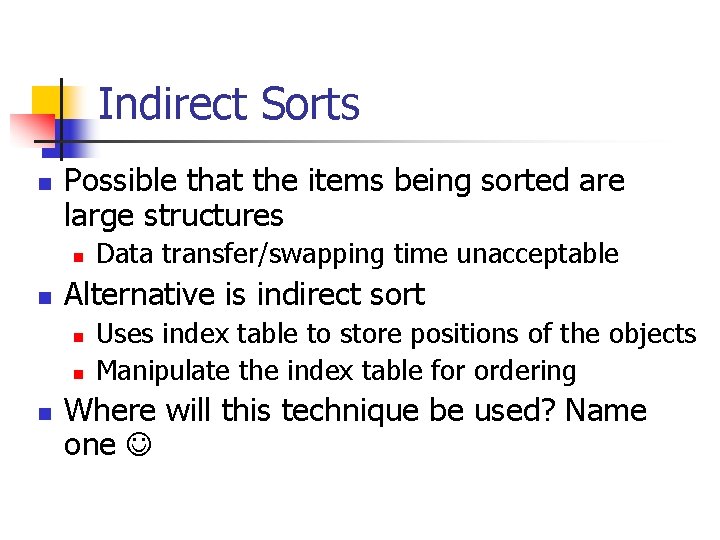
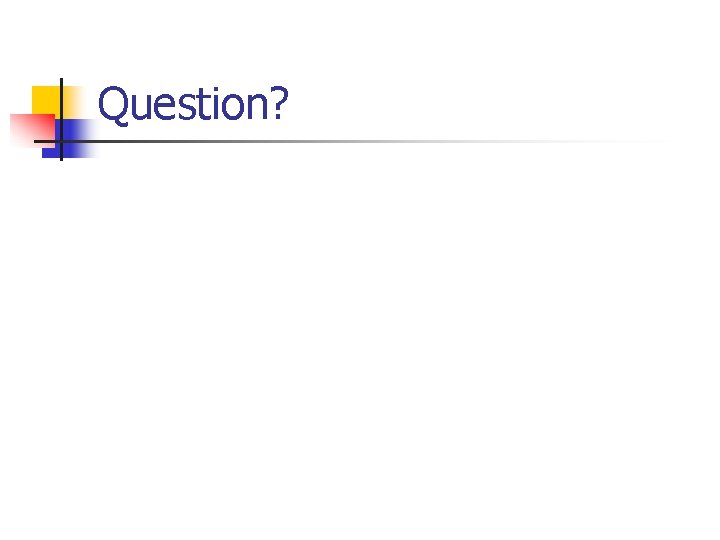
- Slides: 25
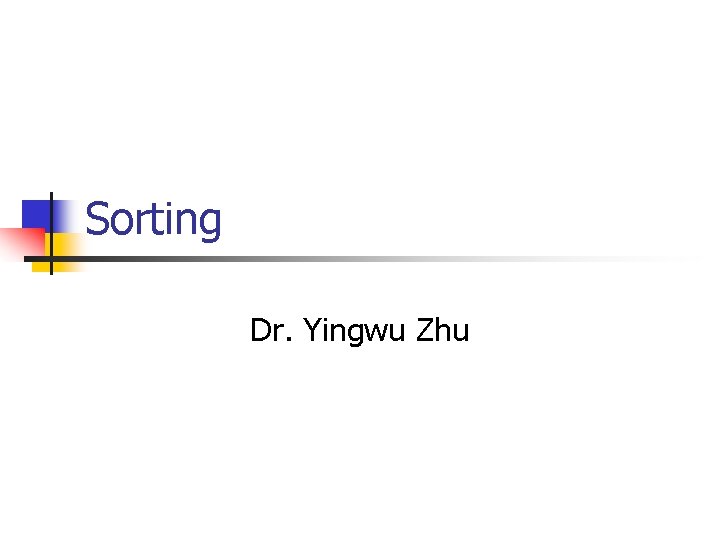
Sorting Dr. Yingwu Zhu
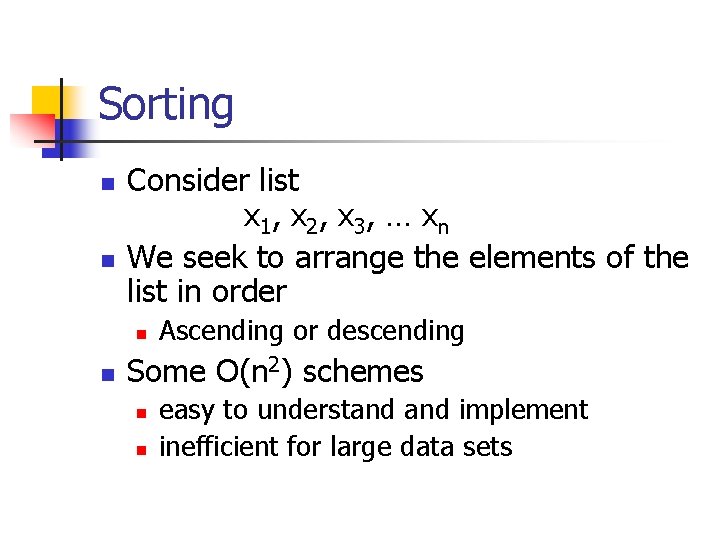
Sorting n n Consider list x 1 , x 2 , x 3 , … xn We seek to arrange the elements of the list in order n n Ascending or descending Some O(n 2) schemes n n easy to understand implement inefficient for large data sets
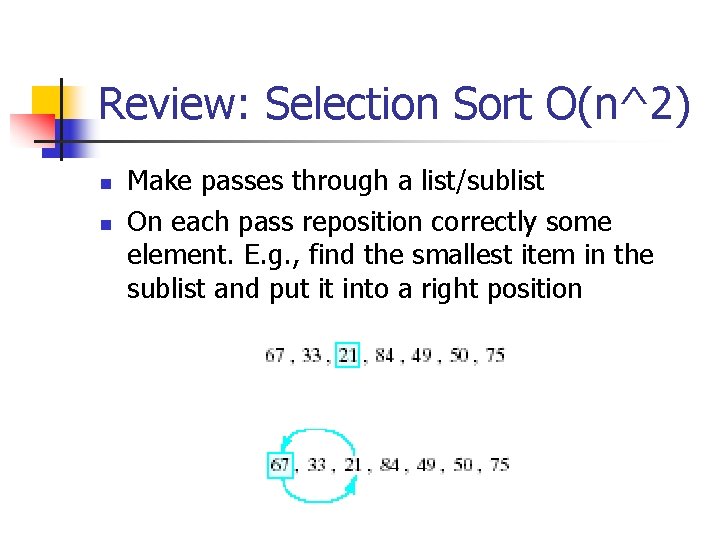
Review: Selection Sort O(n^2) n n Make passes through a list/sublist On each pass reposition correctly some element. E. g. , find the smallest item in the sublist and put it into a right position
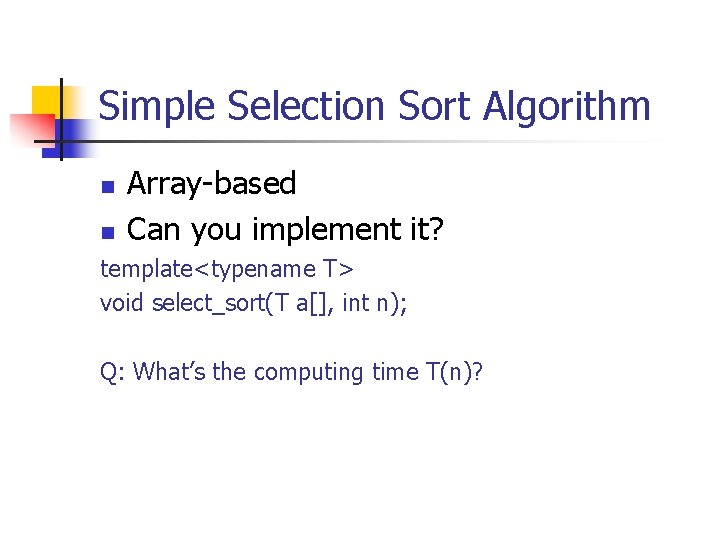
Simple Selection Sort Algorithm n n Array-based Can you implement it? template<typename T> void select_sort(T a[], int n); Q: What’s the computing time T(n)?
![Simple Selection Sort Algorithm template typename T void selectsortint a int n for Simple Selection Sort Algorithm template <typename T> void select_sort(int a[], int n) { for](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-5.jpg)
Simple Selection Sort Algorithm template <typename T> void select_sort(int a[], int n) { for (int i=0; i < n-1; i++) { int min_pos = i; for (int j = i+1; j < n; j++) { if (a[min_pos] > a[j]) min_pos = j; } if (min_pos != i) { T temp = a[i]; a[i] = a[min_pos]; a[min_pos] = temp; } } } T(n) = ?
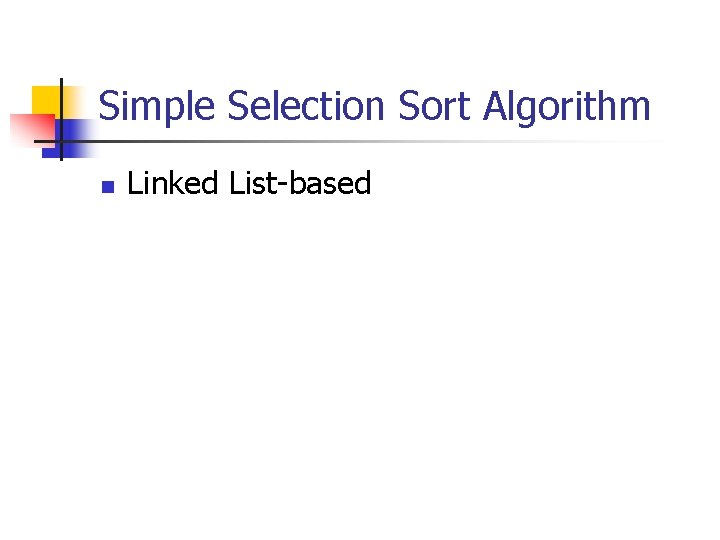
Simple Selection Sort Algorithm n Linked List-based
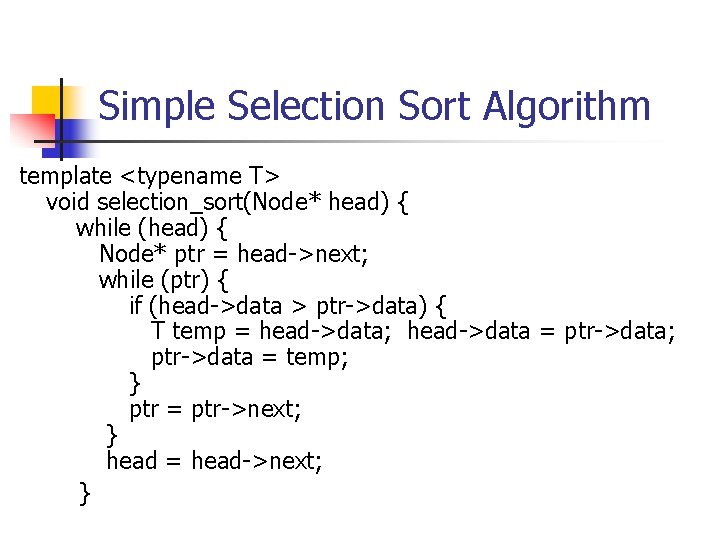
Simple Selection Sort Algorithm template <typename T> void selection_sort(Node* head) { while (head) { Node* ptr = head->next; while (ptr) { if (head->data > ptr->data) { T temp = head->data; head->data = ptr->data; ptr->data = temp; } ptr = ptr->next; } head = head->next; }
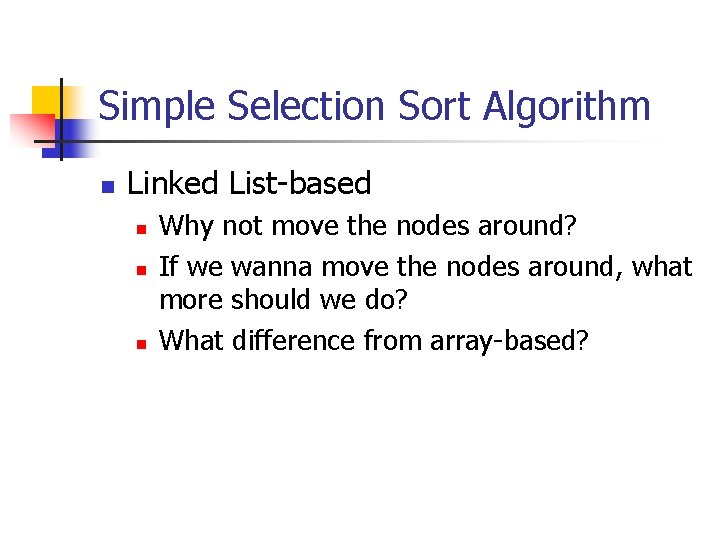
Simple Selection Sort Algorithm n Linked List-based n n n Why not move the nodes around? If we wanna move the nodes around, what more should we do? What difference from array-based?
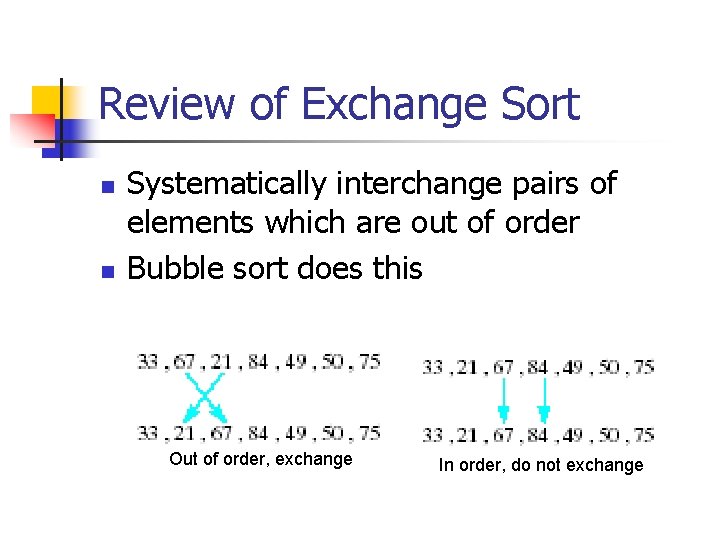
Review of Exchange Sort n n Systematically interchange pairs of elements which are out of order Bubble sort does this Out of order, exchange In order, do not exchange
![Bubble Sort n n void bubblesortint a int n Can you write one in Bubble Sort n n void bubble_sort(int a[], int n) Can you write one in](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-10.jpg)
Bubble Sort n n void bubble_sort(int a[], int n) Can you write one in C++ function?
![Bubble Sort void bubblesortint a int n int numcompares n1 first should Bubble Sort void bubble_sort(int a[], int n) { int num_compares = n-1; //first should](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-11.jpg)
Bubble Sort void bubble_sort(int a[], int n) { int num_compares = n-1; //first should do n-1 comparisons while (num_compares > 0) { int last = 0; //why need this? for (int i=0; i<num_compares; i++) { if (a[i] > a[i+1]) { swap(a[i], a[i+1]); last = i; } //end if num_compares = last; } //end while } // thinking: why need last = i? ? ? The purpose of last?
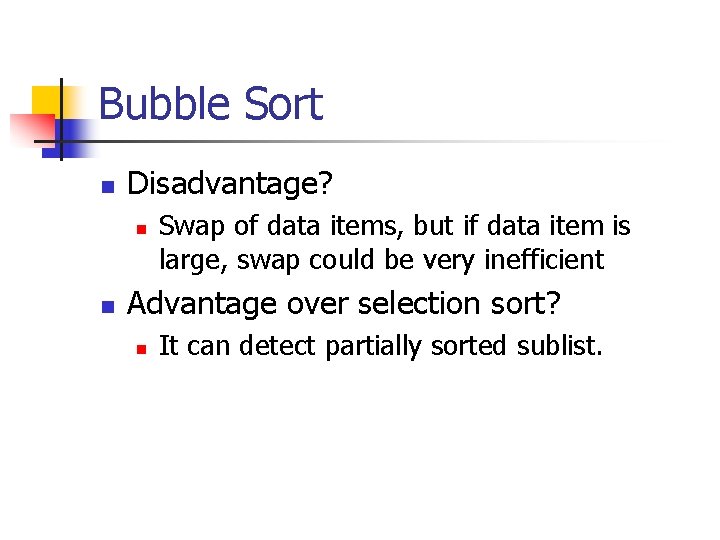
Bubble Sort n Disadvantage? n n Swap of data items, but if data item is large, swap could be very inefficient Advantage over selection sort? n It can detect partially sorted sublist.
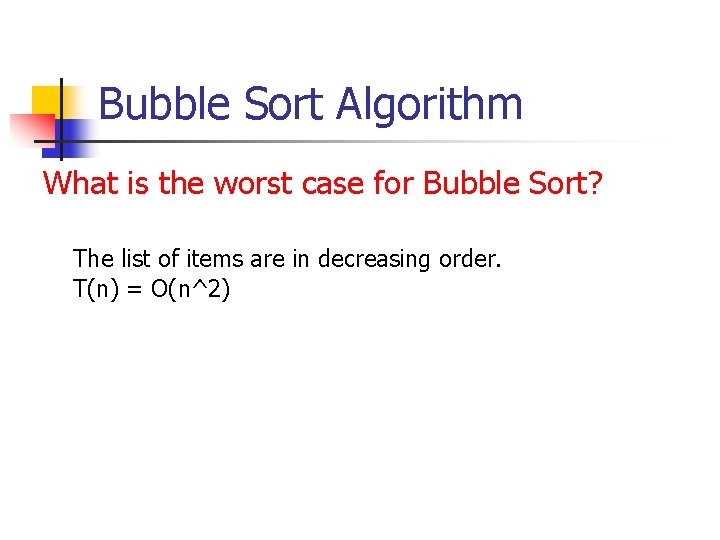
Bubble Sort Algorithm What is the worst case for Bubble Sort? The list of items are in decreasing order. T(n) = O(n^2)
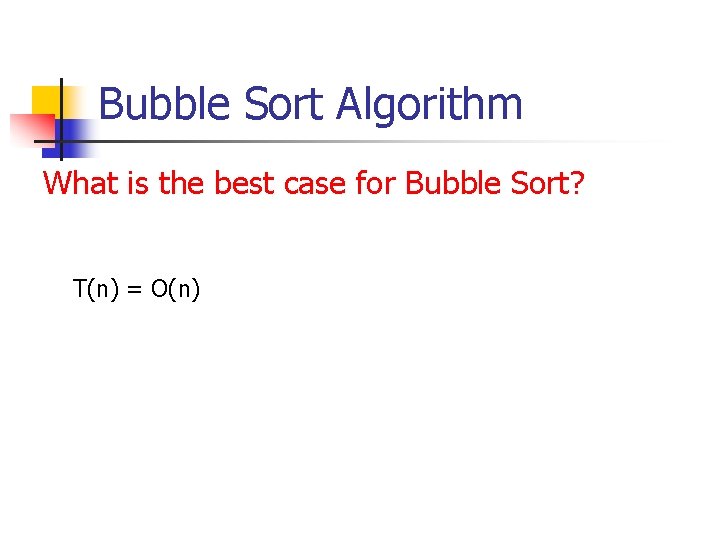
Bubble Sort Algorithm What is the best case for Bubble Sort? T(n) = O(n)
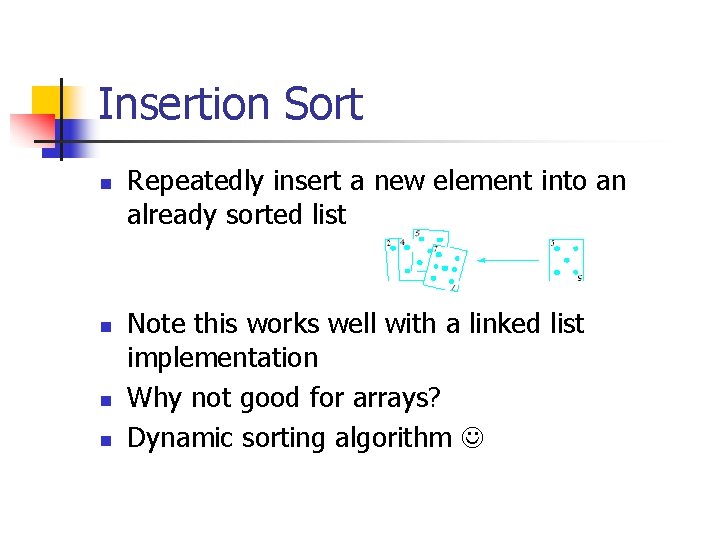
Insertion Sort n n Repeatedly insert a new element into an already sorted list Note this works well with a linked list implementation Why not good for arrays? Dynamic sorting algorithm
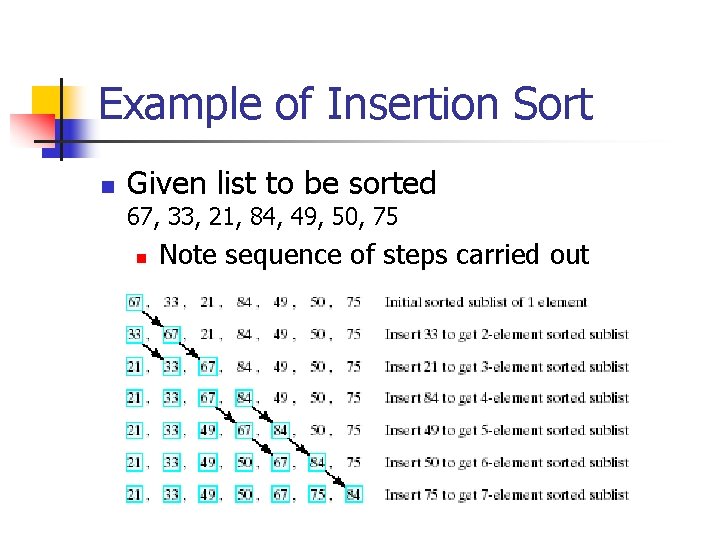
Example of Insertion Sort n Given list to be sorted 67, 33, 21, 84, 49, 50, 75 n Note sequence of steps carried out
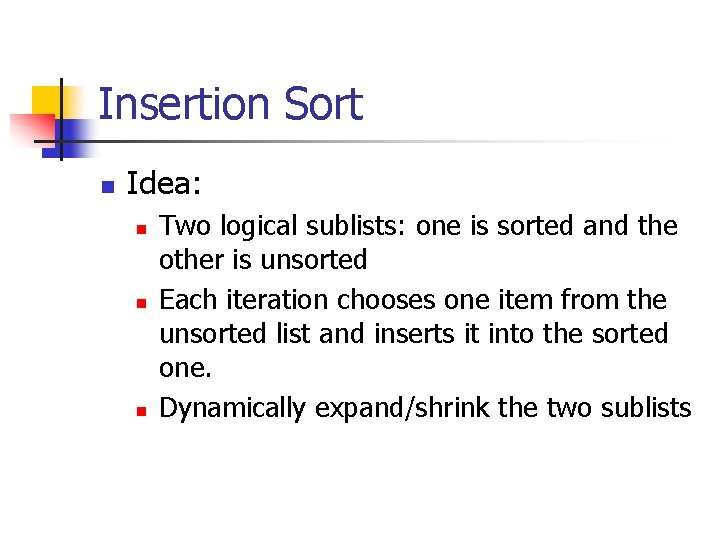
Insertion Sort n Idea: n n n Two logical sublists: one is sorted and the other is unsorted Each iteration chooses one item from the unsorted list and inserts it into the sorted one. Dynamically expand/shrink the two sublists
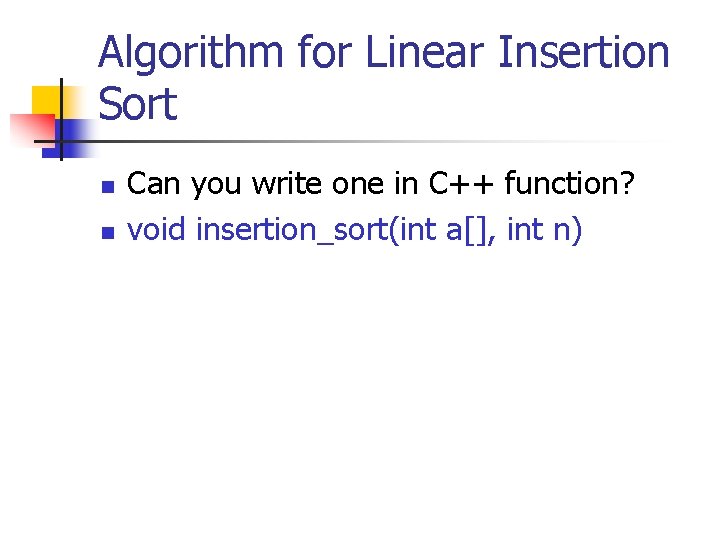
Algorithm for Linear Insertion Sort n n Can you write one in C++ function? void insertion_sort(int a[], int n)
![Algorithm for Linear Insertion Sort void insertionsortint a int n int temp for Algorithm for Linear Insertion Sort void insertion_sort(int a[], int n) { int temp; for](https://slidetodoc.com/presentation_image_h2/45f7c9434ccea0e736b917f39313b494/image-19.jpg)
Algorithm for Linear Insertion Sort void insertion_sort(int a[], int n) { int temp; for (int i = 1; i<n; i++) { temp = a[i]; // keep the item to be inserted int j; for (j = i-1; j >= 0; j--) //go through the sorted sublist if (temp < a[j]) a[j+1] = a[j]; //shift the items else break; a[j+1] = temp; } //end for }
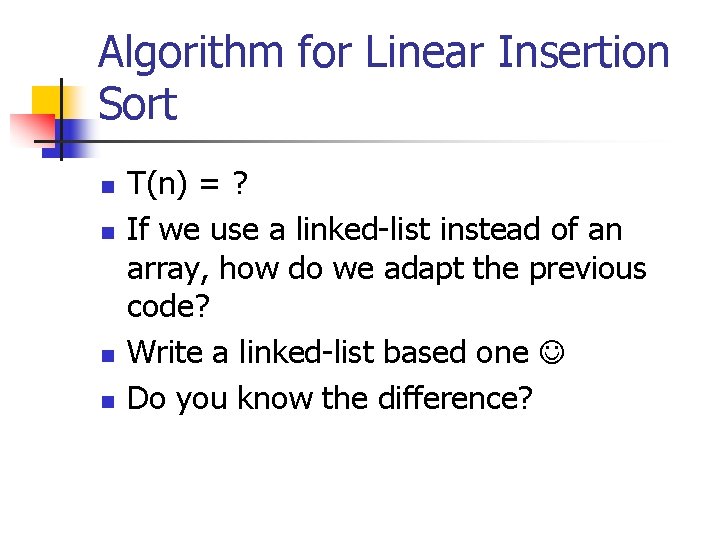
Algorithm for Linear Insertion Sort n n T(n) = ? If we use a linked-list instead of an array, how do we adapt the previous code? Write a linked-list based one Do you know the difference?
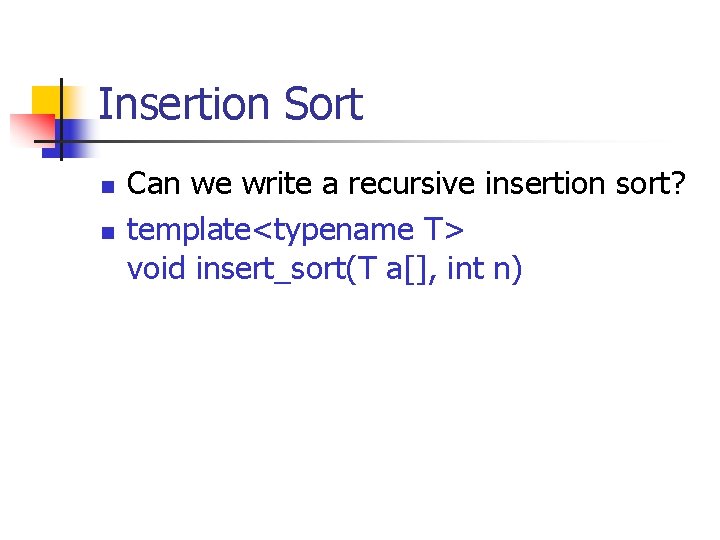
Insertion Sort n n Can we write a recursive insertion sort? template<typename T> void insert_sort(T a[], int n)
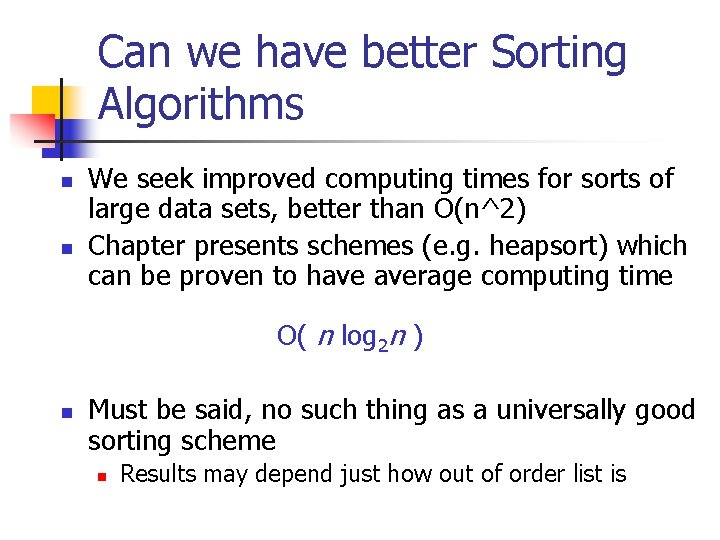
Can we have better Sorting Algorithms n n We seek improved computing times for sorts of large data sets, better than O(n^2) Chapter presents schemes (e. g. heapsort) which can be proven to have average computing time O( n log 2 n ) n Must be said, no such thing as a universally good sorting scheme n Results may depend just how out of order list is
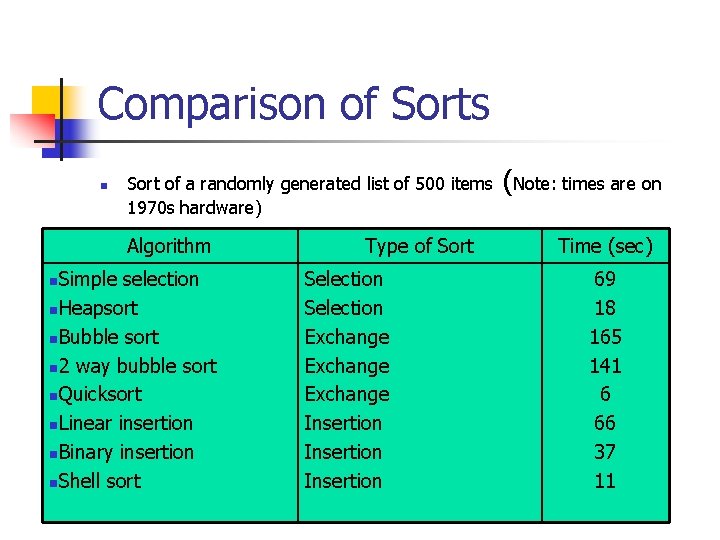
Comparison of Sorts n Sort of a randomly generated list of 500 items 1970 s hardware) Algorithm Simple selection n. Heapsort n. Bubble sort n 2 way bubble sort n. Quicksort n. Linear insertion n. Binary insertion n. Shell sort n Type of Sort Selection Exchange Insertion (Note: times are on Time (sec) 69 18 165 141 6 66 37 11
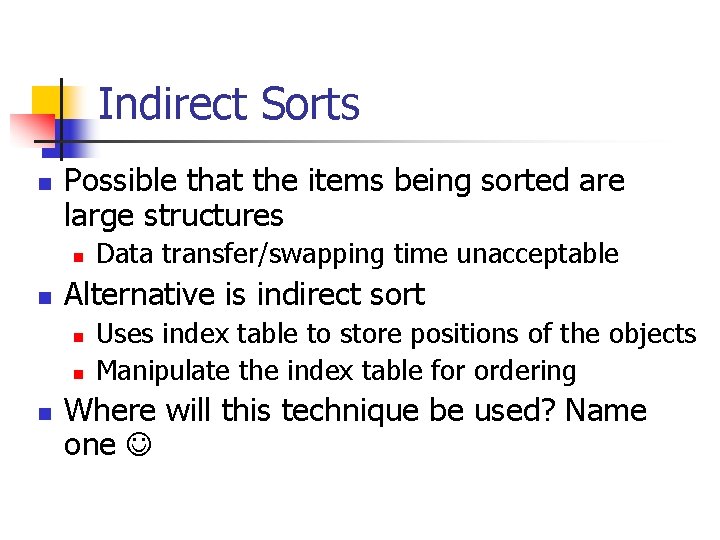
Indirect Sorts n Possible that the items being sorted are large structures n n Alternative is indirect sort n n n Data transfer/swapping time unacceptable Uses index table to store positions of the objects Manipulate the index table for ordering Where will this technique be used? Name one
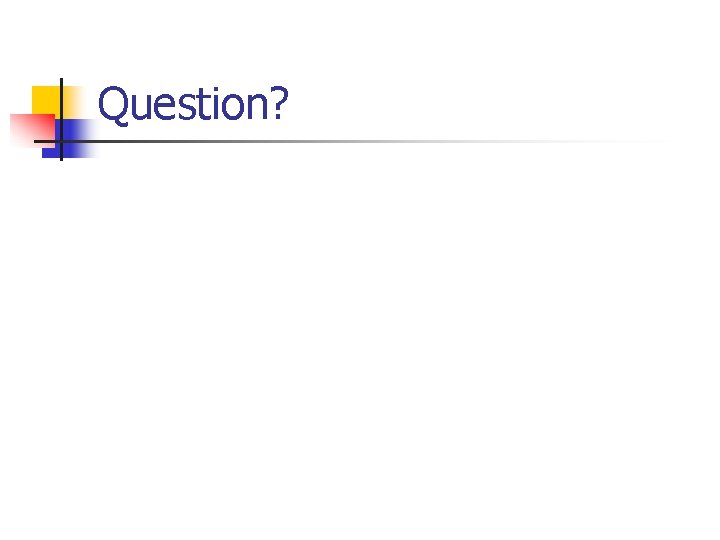
Question?