Simple Geometrical Transformations Simple geometrical transformations are those
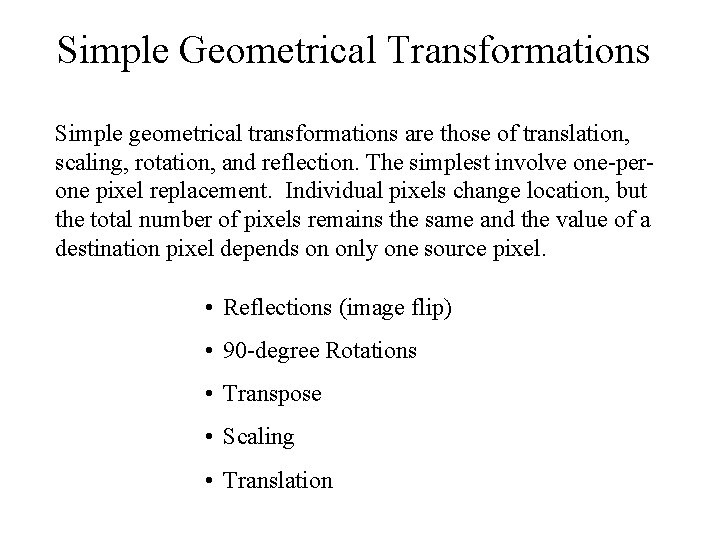
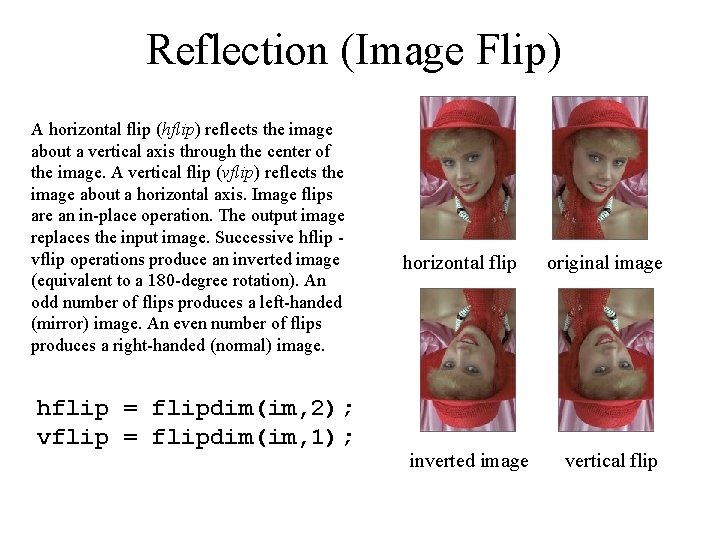
![Transpose out = permute(im, [2 1 3]); For a simple grayscale image we can Transpose out = permute(im, [2 1 3]); For a simple grayscale image we can](https://slidetodoc.com/presentation_image_h2/4976a5ac3e903d6b27a83e926942df1c/image-3.jpg)
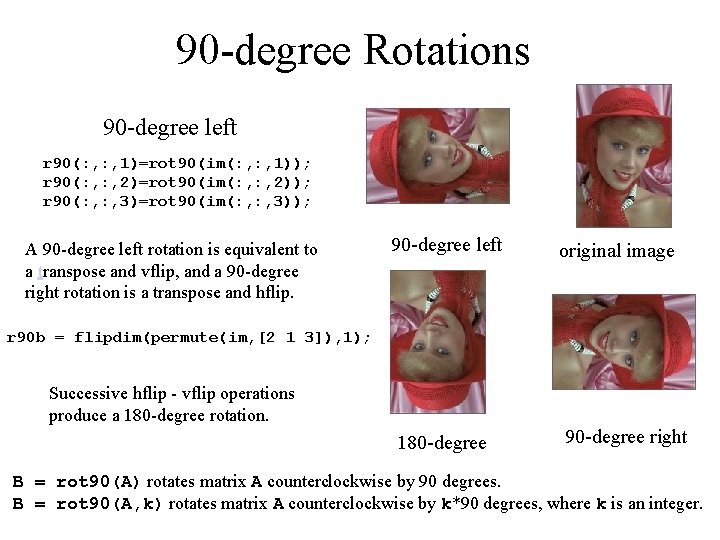
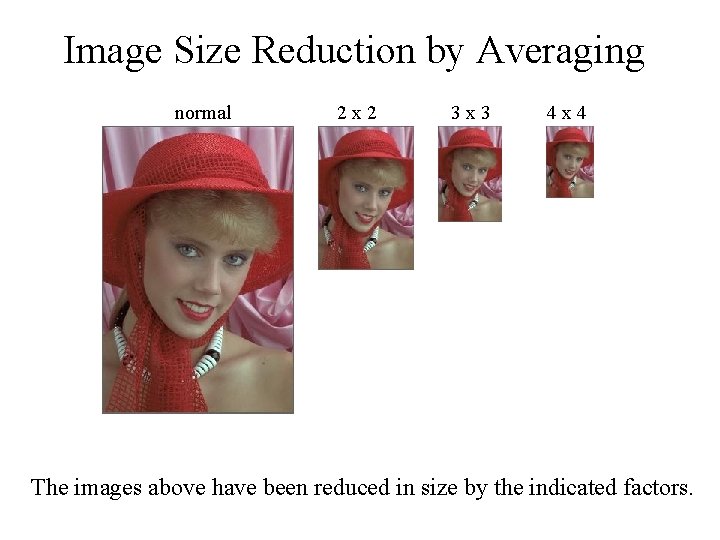
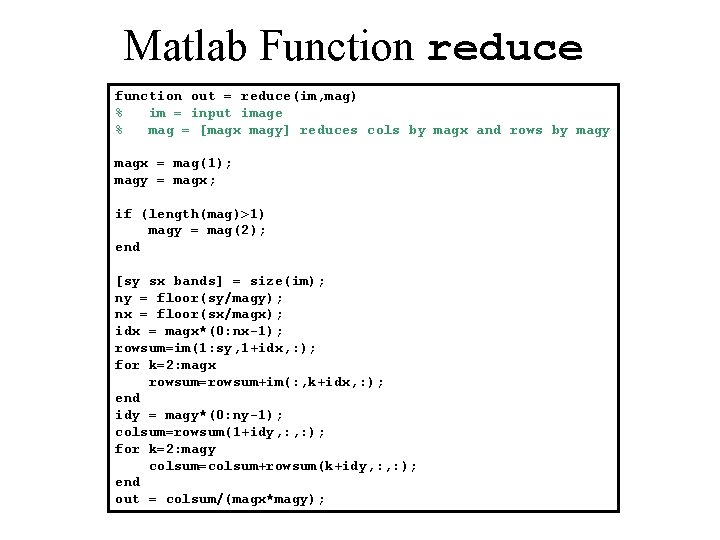
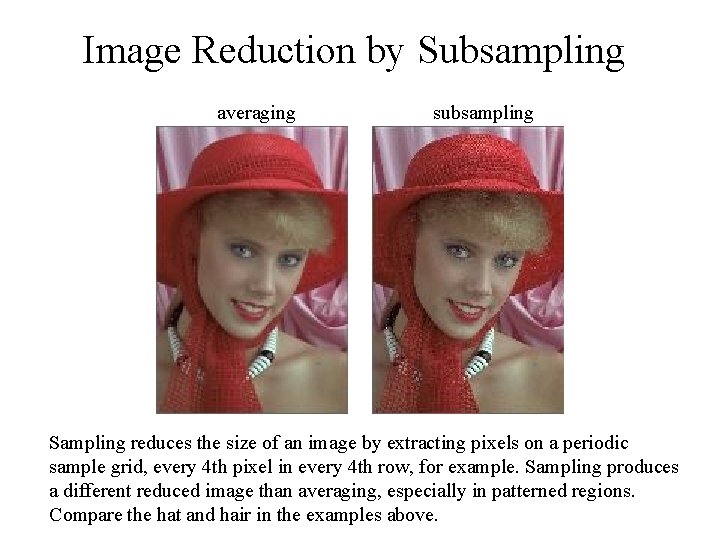
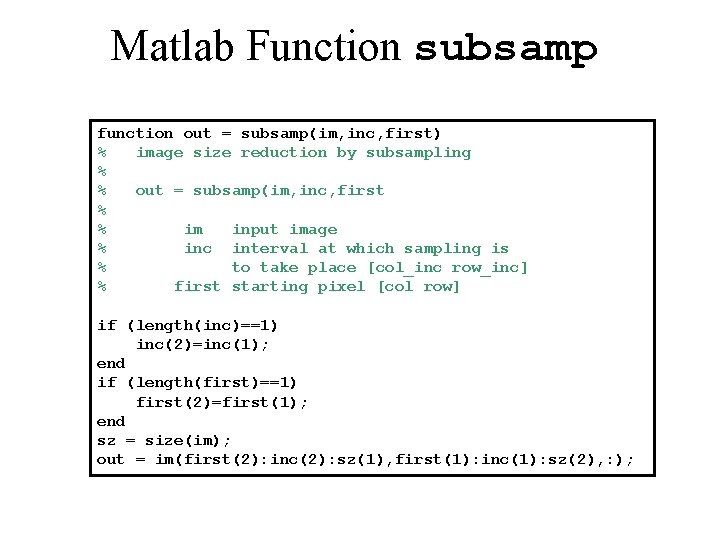
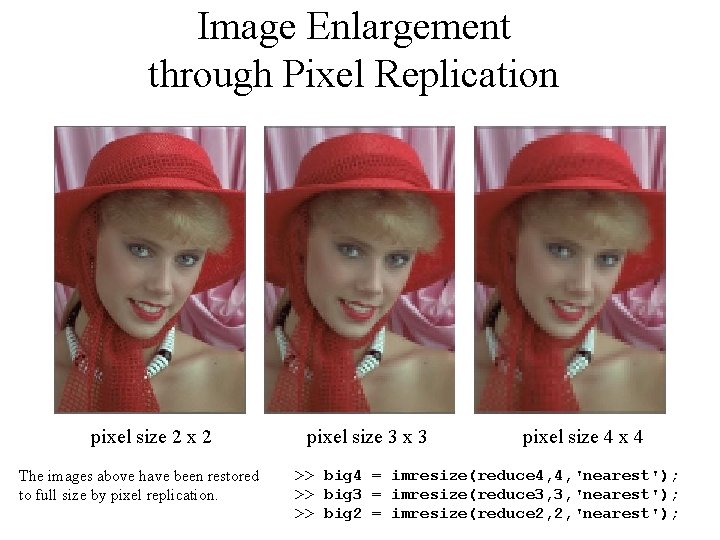
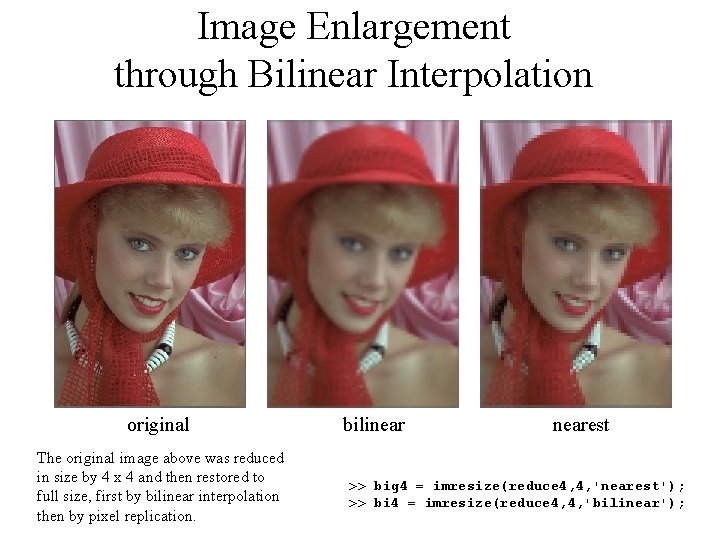
![Cyclic Translation ishift(normal, [0 80]); ishift(normal, [80 80]); The pixels and rows of the Cyclic Translation ishift(normal, [0 80]); ishift(normal, [80 80]); The pixels and rows of the](https://slidetodoc.com/presentation_image_h2/4976a5ac3e903d6b27a83e926942df1c/image-11.jpg)
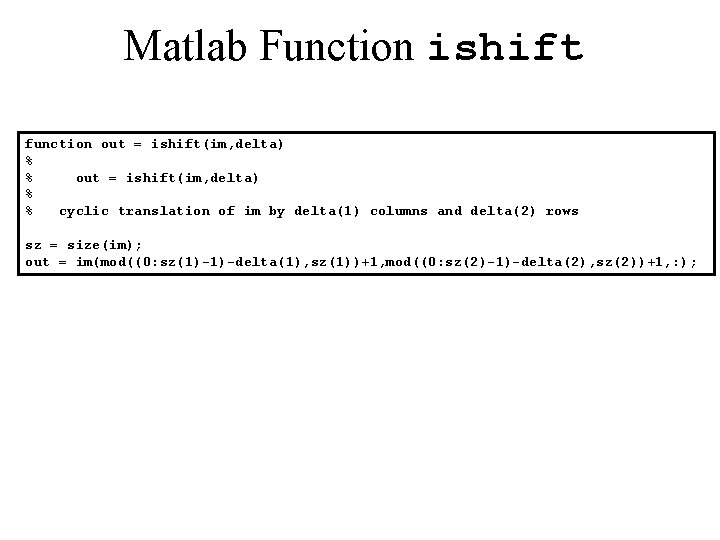
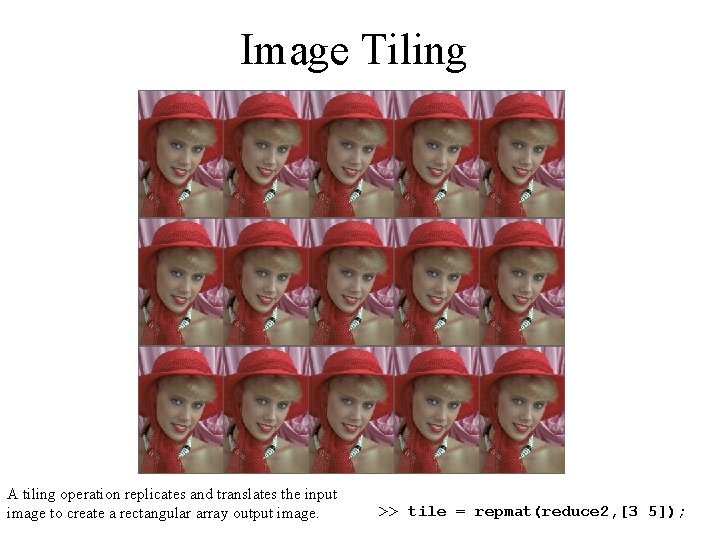
- Slides: 13
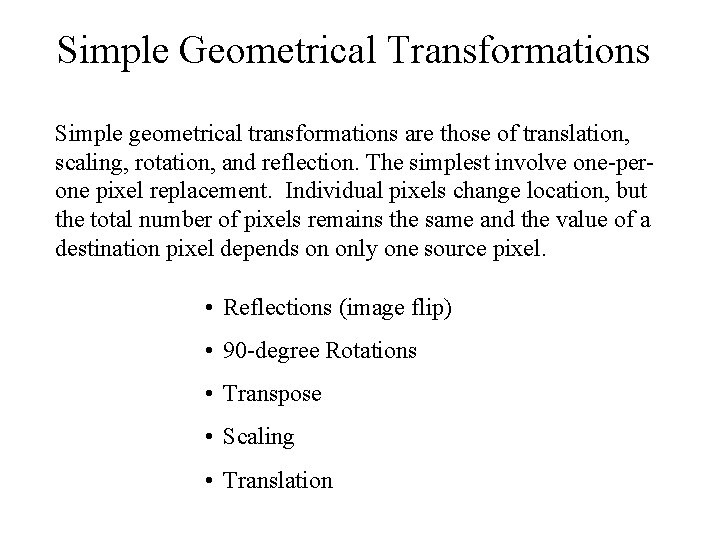
Simple Geometrical Transformations Simple geometrical transformations are those of translation, scaling, rotation, and reflection. The simplest involve one-perone pixel replacement. Individual pixels change location, but the total number of pixels remains the same and the value of a destination pixel depends on only one source pixel. • Reflections (image flip) • 90 -degree Rotations • Transpose • Scaling • Translation
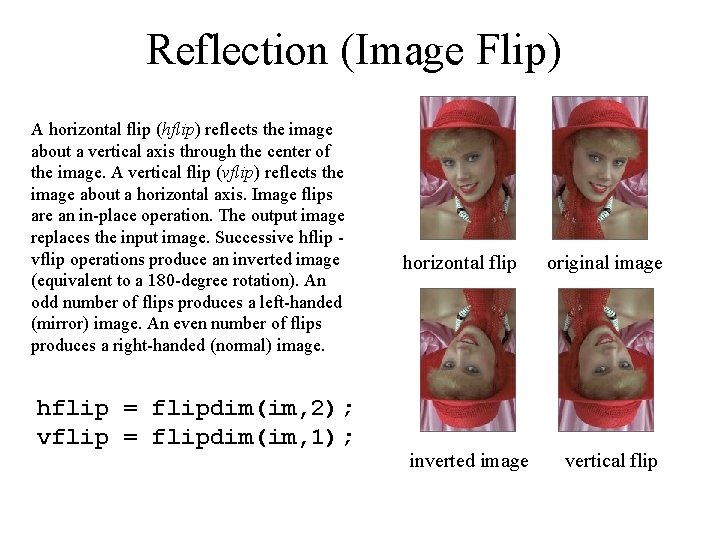
Reflection (Image Flip) A horizontal flip (hflip) reflects the image about a vertical axis through the center of the image. A vertical flip (vflip) reflects the image about a horizontal axis. Image flips are an in-place operation. The output image replaces the input image. Successive hflip vflip operations produce an inverted image (equivalent to a 180 -degree rotation). An odd number of flips produces a left-handed (mirror) image. An even number of flips produces a right-handed (normal) image. hflip = flipdim(im, 2); vflip = flipdim(im, 1); horizontal flip inverted image original image vertical flip
![Transpose out permuteim 2 1 3 For a simple grayscale image we can Transpose out = permute(im, [2 1 3]); For a simple grayscale image we can](https://slidetodoc.com/presentation_image_h2/4976a5ac3e903d6b27a83e926942df1c/image-3.jpg)
Transpose out = permute(im, [2 1 3]); For a simple grayscale image we can do >> gray = rgb 2 gray(im); >> tpose = gray';
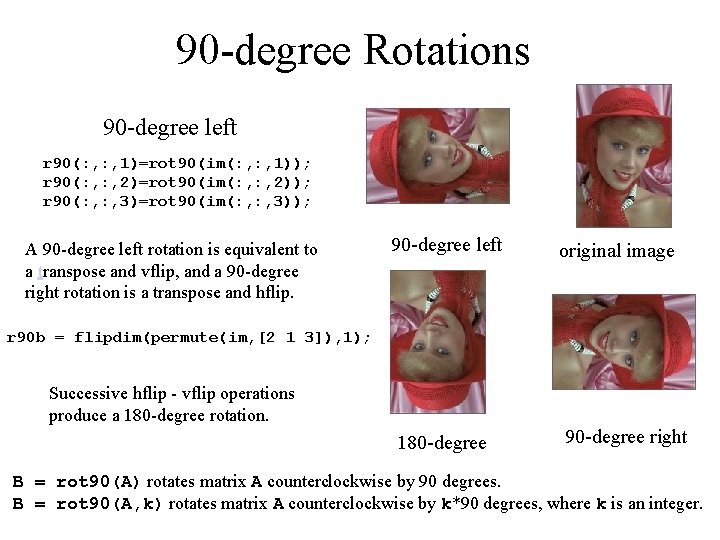
90 -degree Rotations 90 -degree left r 90(: , 1)=rot 90(im(: , 1)); r 90(: , 2)=rot 90(im(: , 2)); r 90(: , 3)=rot 90(im(: , 3)); A 90 -degree left rotation is equivalent to a transpose and vflip, and a 90 -degree right rotation is a transpose and hflip. 90 -degree left original image r 90 b = flipdim(permute(im, [2 1 3]), 1); Successive hflip - vflip operations produce a 180 -degree rotation. 180 -degree 90 -degree right B = rot 90(A) rotates matrix A counterclockwise by 90 degrees. B = rot 90(A, k) rotates matrix A counterclockwise by k*90 degrees, where k is an integer.
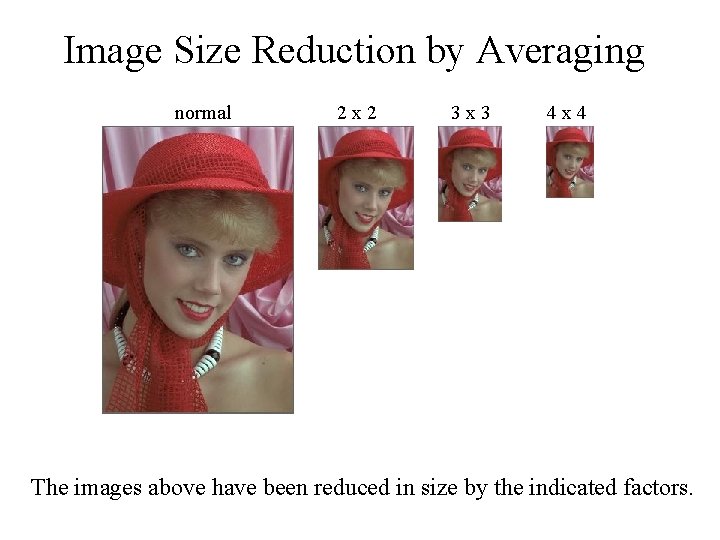
Image Size Reduction by Averaging normal 2 x 2 3 x 3 4 x 4 The images above have been reduced in size by the indicated factors.
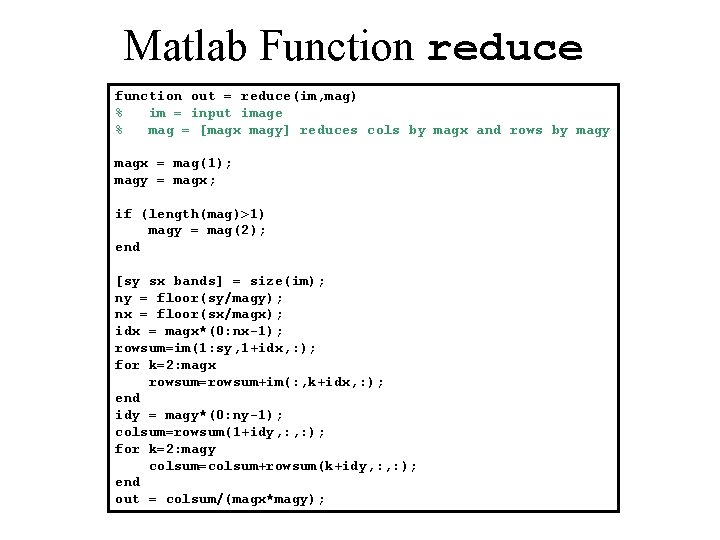
Matlab Function reduce function out = reduce(im, mag) % im = input image % mag = [magx magy] reduces cols by magx and rows by magx = mag(1); magy = magx; if (length(mag)>1) magy = mag(2); end [sy sx bands] = size(im); ny = floor(sy/magy); nx = floor(sx/magx); idx = magx*(0: nx-1); rowsum=im(1: sy, 1+idx, : ); for k=2: magx rowsum=rowsum+im(: , k+idx, : ); end idy = magy*(0: ny-1); colsum=rowsum(1+idy, : ); for k=2: magy colsum=colsum+rowsum(k+idy, : ); end out = colsum/(magx*magy);
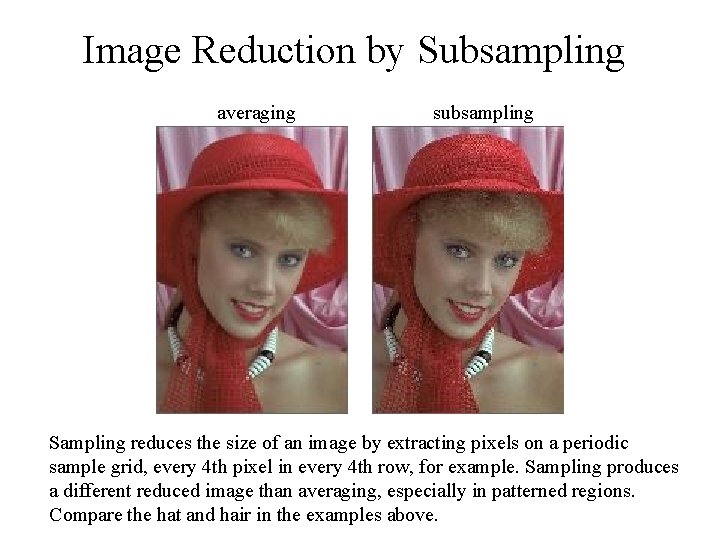
Image Reduction by Subsampling averaging subsampling Sampling reduces the size of an image by extracting pixels on a periodic sample grid, every 4 th pixel in every 4 th row, for example. Sampling produces a different reduced image than averaging, especially in patterned regions. Compare the hat and hair in the examples above.
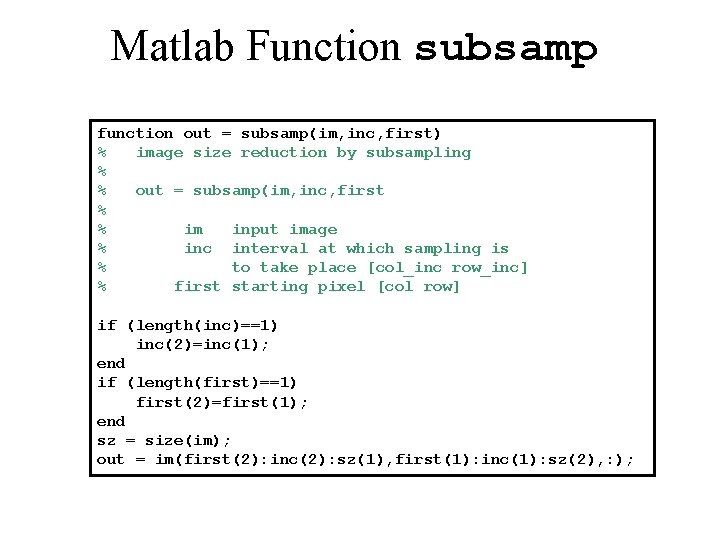
Matlab Function subsamp function out = subsamp(im, inc, first) % image size reduction by subsampling % % out = subsamp(im, inc, first % % im input image % inc interval at which sampling is % to take place [col_inc row_inc] % first starting pixel [col row] if (length(inc)==1) inc(2)=inc(1); end if (length(first)==1) first(2)=first(1); end sz = size(im); out = im(first(2): inc(2): sz(1), first(1): inc(1): sz(2), : );
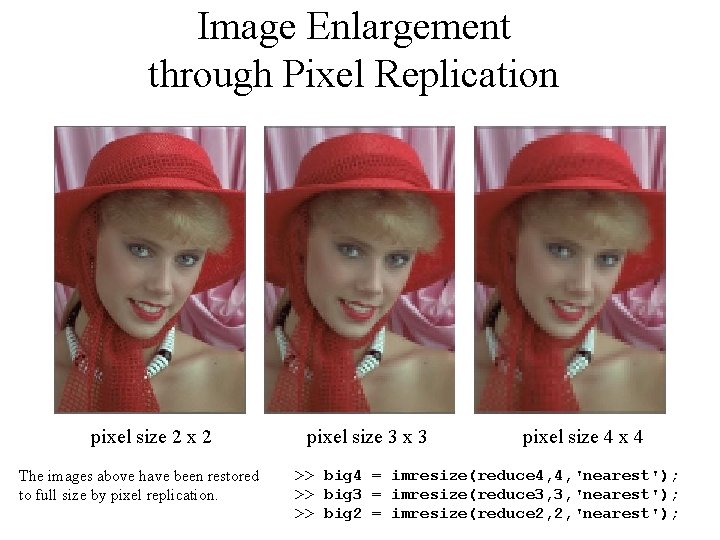
Image Enlargement through Pixel Replication pixel size 2 x 2 The images above have been restored to full size by pixel replication. pixel size 3 x 3 pixel size 4 x 4 >> big 4 = imresize(reduce 4, 4, 'nearest'); >> big 3 = imresize(reduce 3, 3, 'nearest'); >> big 2 = imresize(reduce 2, 2, 'nearest');
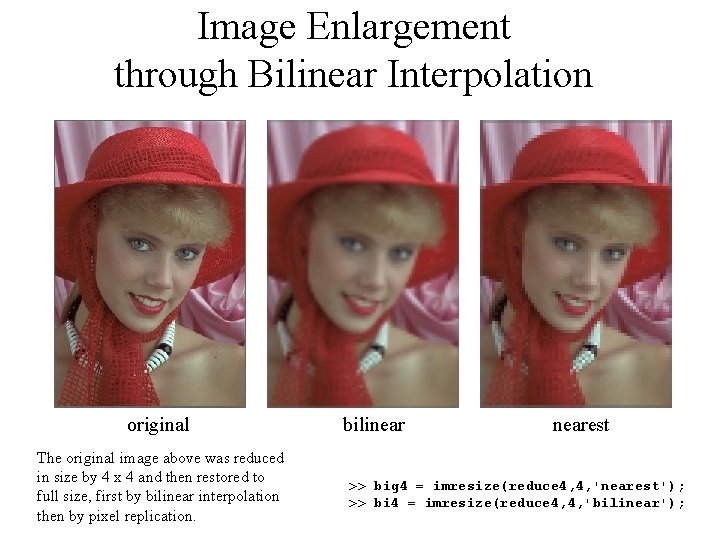
Image Enlargement through Bilinear Interpolation original The original image above was reduced in size by 4 x 4 and then restored to full size, first by bilinear interpolation then by pixel replication. bilinearest >> big 4 = imresize(reduce 4, 4, 'nearest'); >> bi 4 = imresize(reduce 4, 4, 'bilinear');
![Cyclic Translation ishiftnormal 0 80 ishiftnormal 80 80 The pixels and rows of the Cyclic Translation ishift(normal, [0 80]); ishift(normal, [80 80]); The pixels and rows of the](https://slidetodoc.com/presentation_image_h2/4976a5ac3e903d6b27a83e926942df1c/image-11.jpg)
Cyclic Translation ishift(normal, [0 80]); ishift(normal, [80 80]); The pixels and rows of the images above have been shifted with wraparound. Pixels shifted off the image to the right are inserted on the left. Rows moved down off the image are inserted at the top.
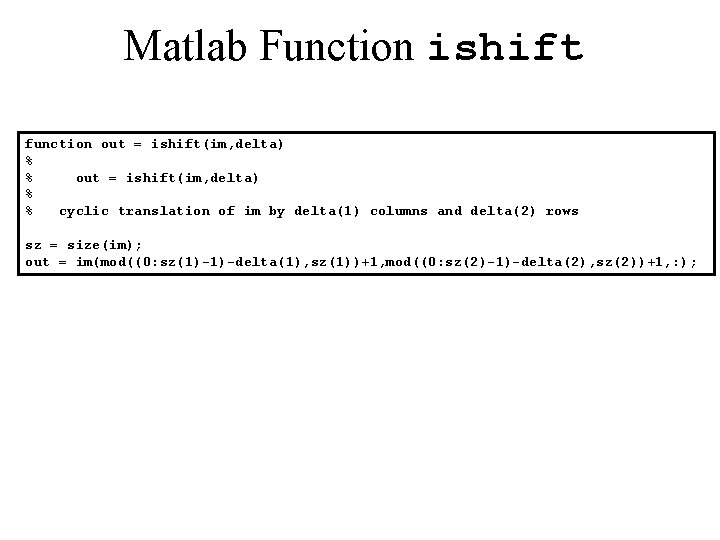
Matlab Function ishift function out = ishift(im, delta) % % cyclic translation of im by delta(1) columns and delta(2) rows sz = size(im); out = im(mod((0: sz(1)-1)-delta(1), sz(1))+1, mod((0: sz(2)-1)-delta(2), sz(2))+1, : );
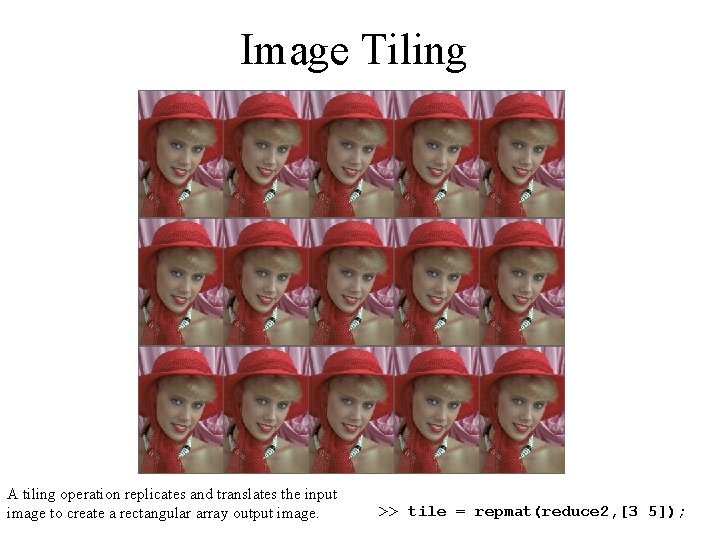
Image Tiling A tiling operation replicates and translates the input image to create a rectangular array output image. >> tile = repmat(reduce 2, [3 5]);
Mikael ferm
Geometric vs structural isomers
Gdt symbol
Geometric tolerance
Permissible straightness tolerance
Geometric optics ppt
Geometrical
Geometrical optics
Geometrical data
Geometric representation of complex numbers
Surface area of cylinder
Geometrical spreading
Geometrical shadow
Geometrical isomerism in coordination compounds