selfreference 2 struct node int data 1 char
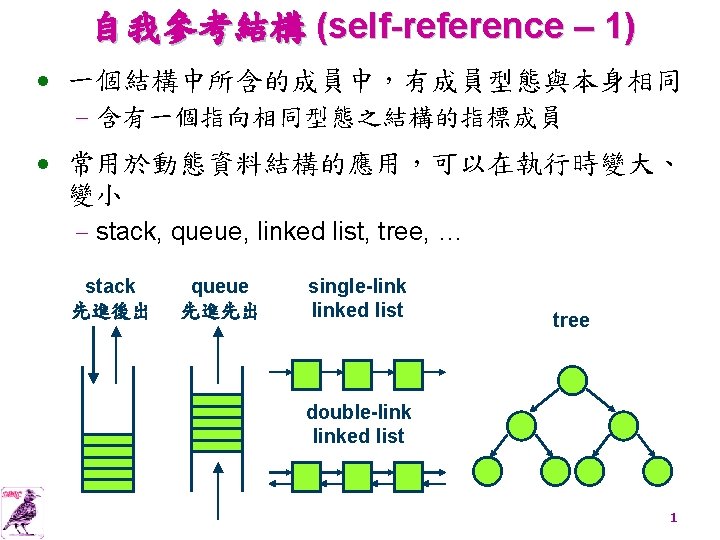
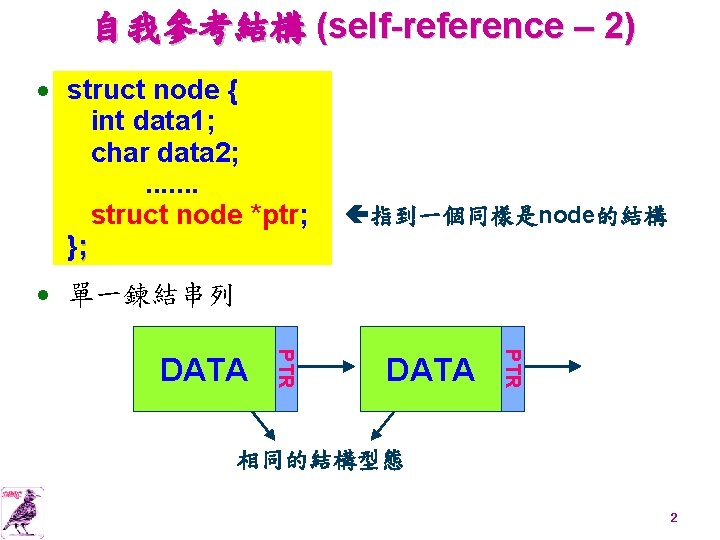
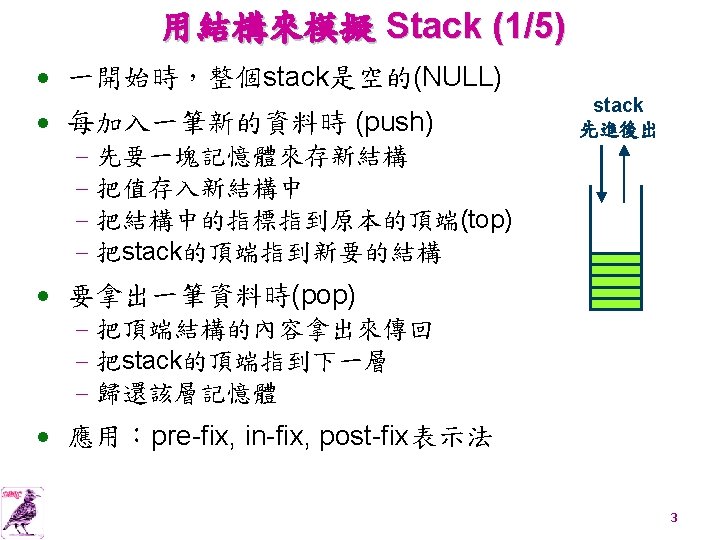
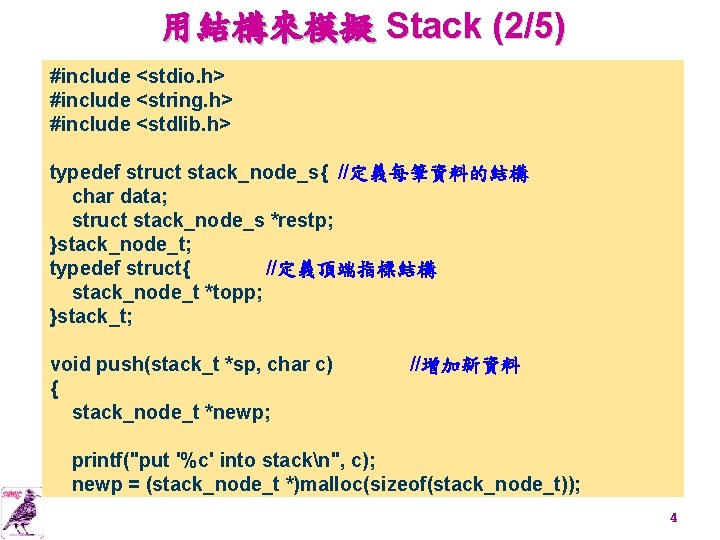
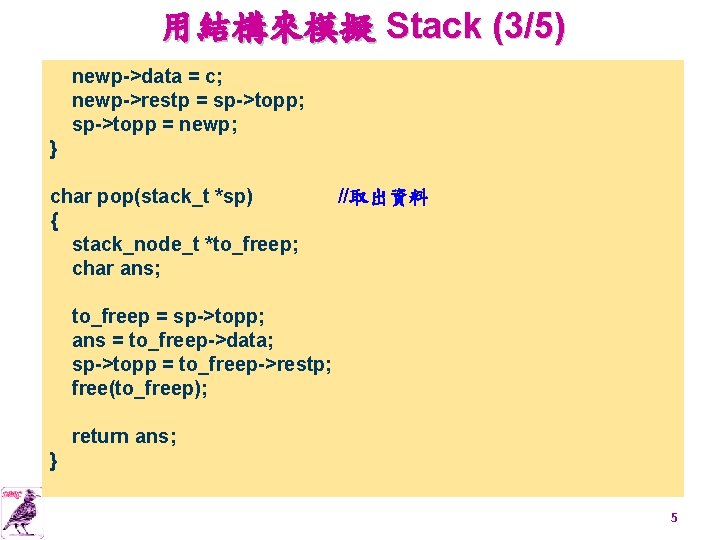
![用結構來模擬 Stack (4/5) int main(void) { stack_t s = {NULL}; //程式一開始stack是空的 char str[80]; int 用結構來模擬 Stack (4/5) int main(void) { stack_t s = {NULL}; //程式一開始stack是空的 char str[80]; int](https://slidetodoc.com/presentation_image/4f0c38a0253619c288a131c0a8c77f43/image-6.jpg)
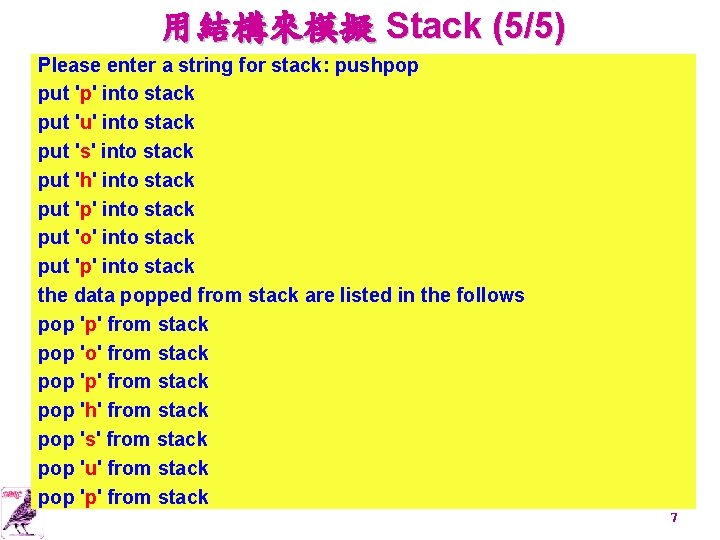
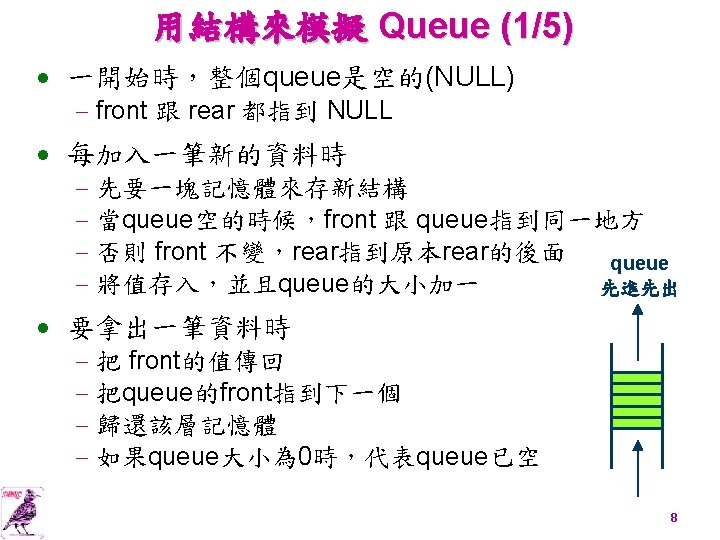
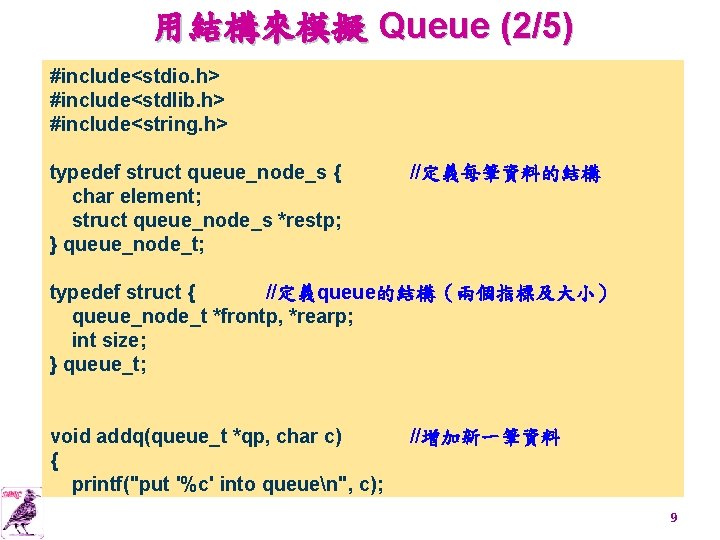
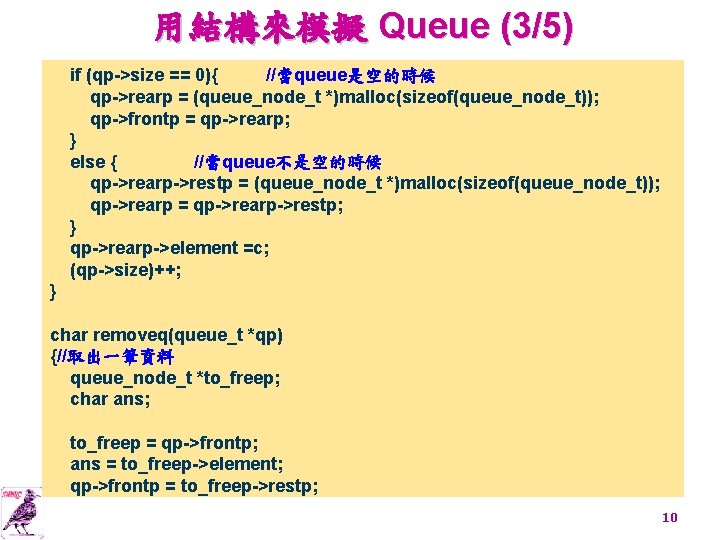
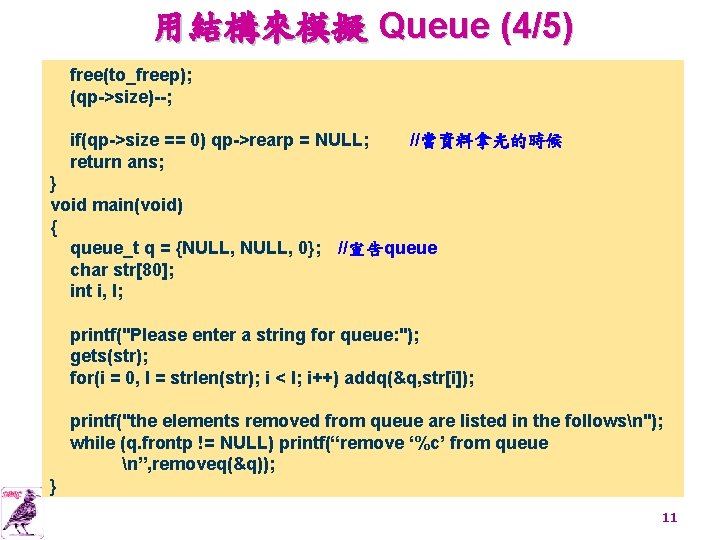
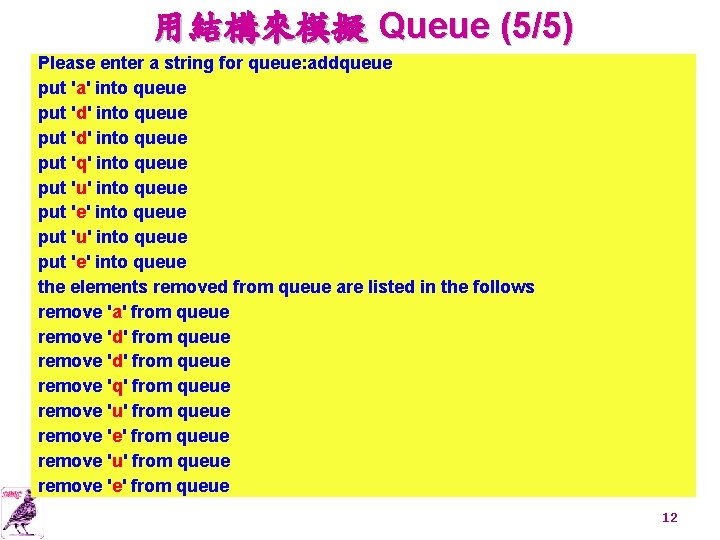
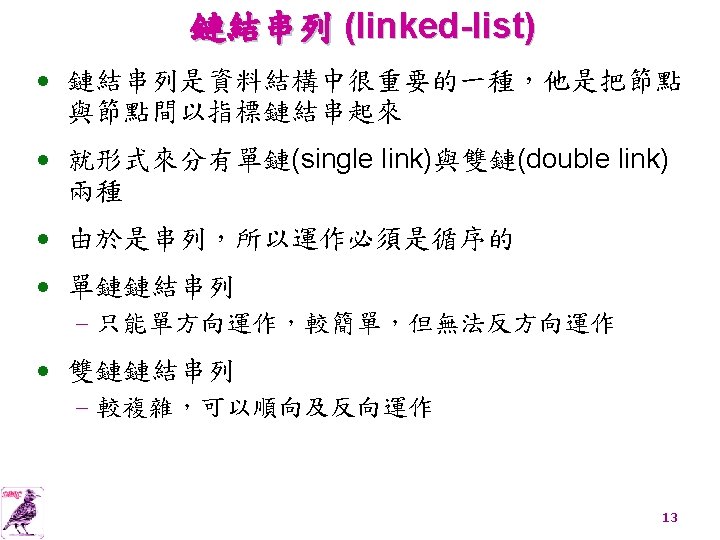
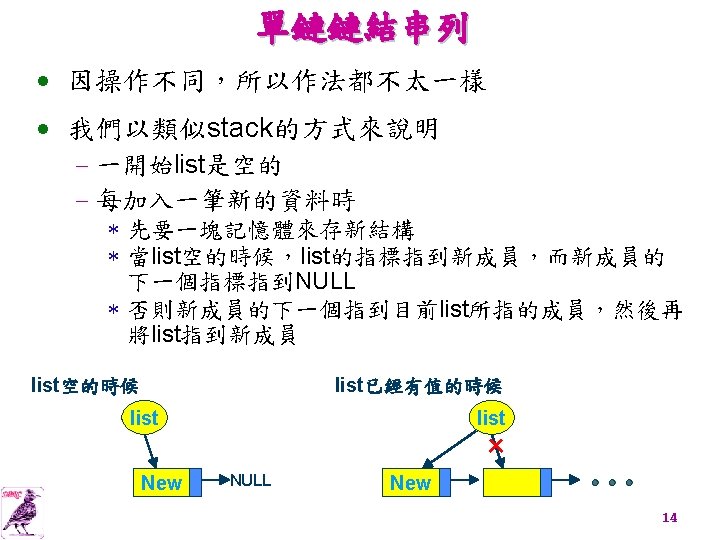
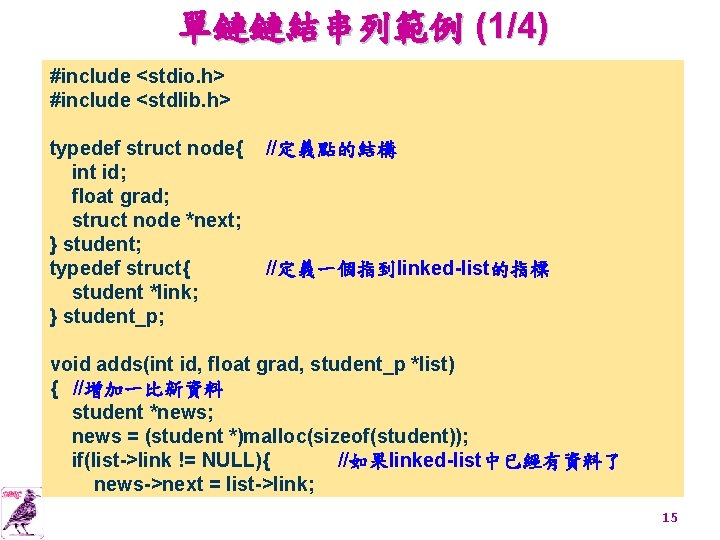
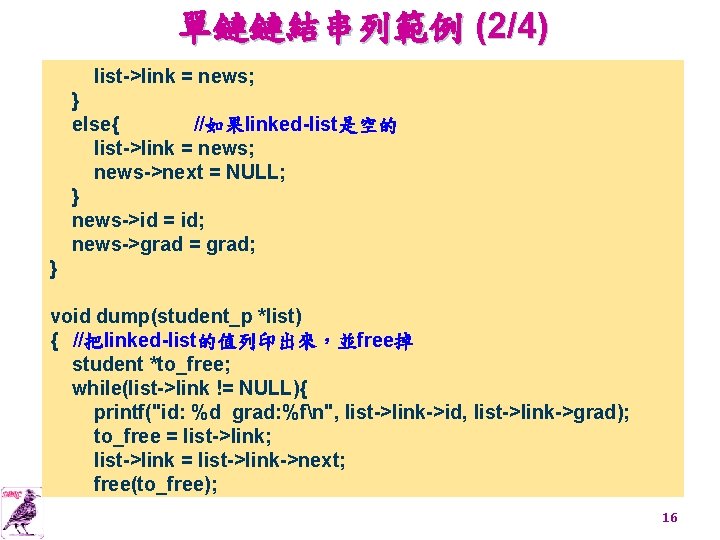
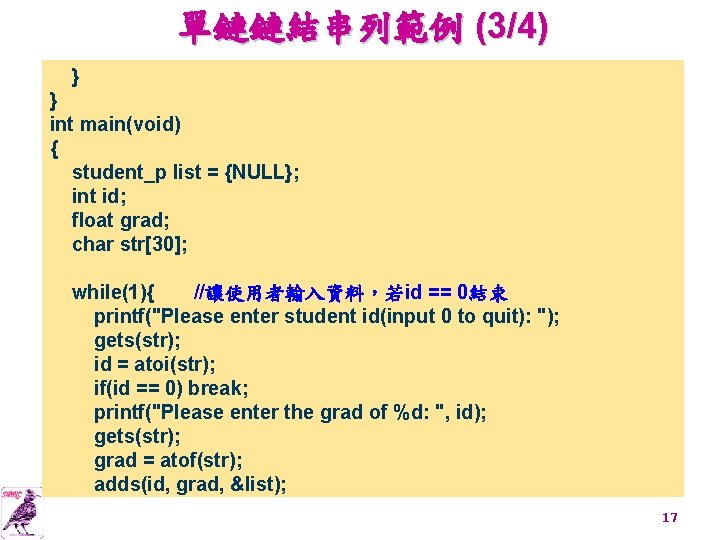
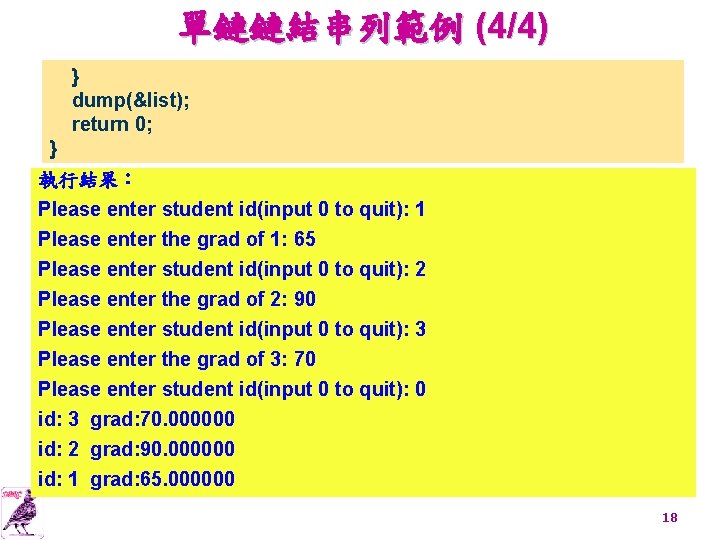
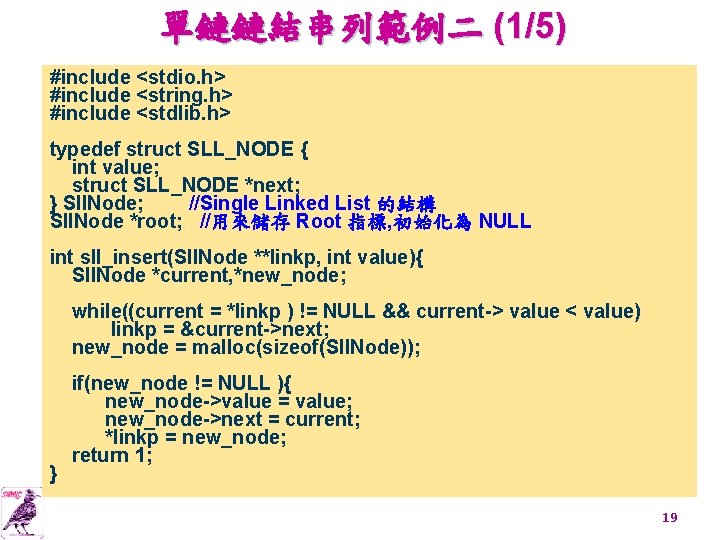
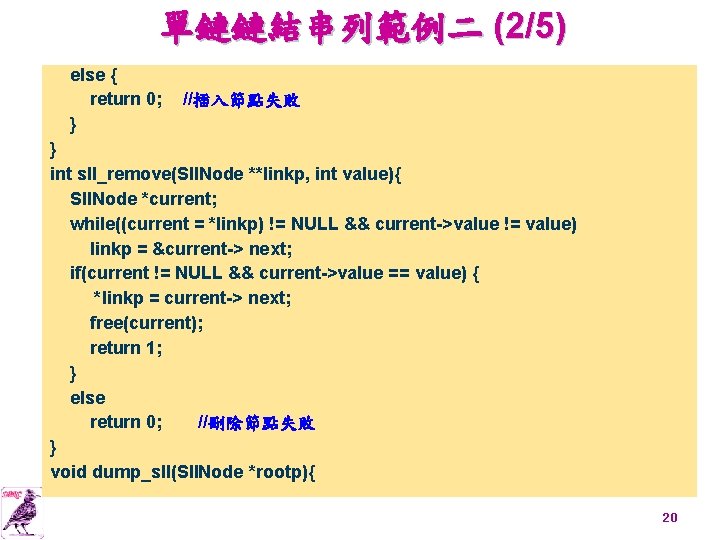
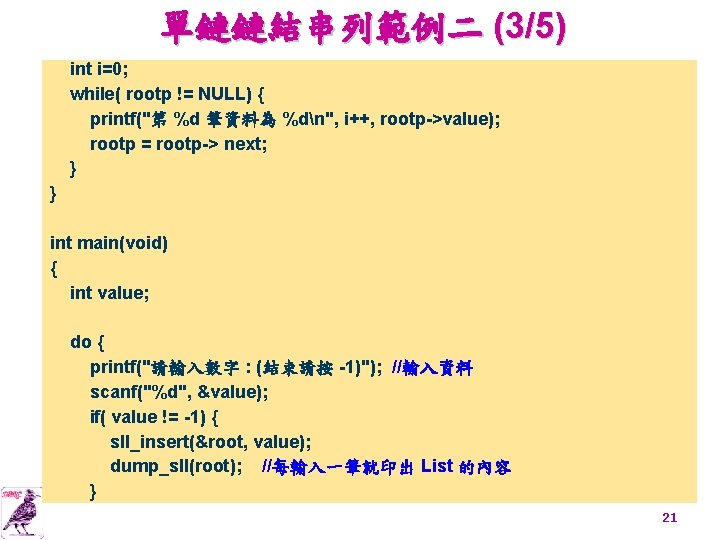
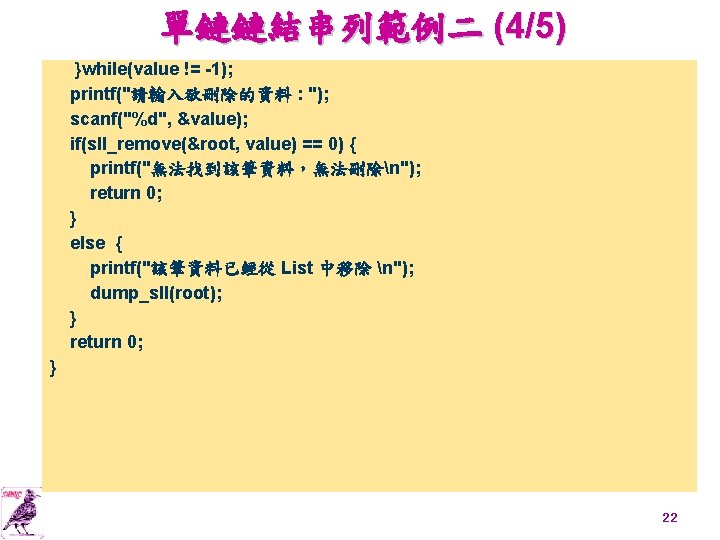
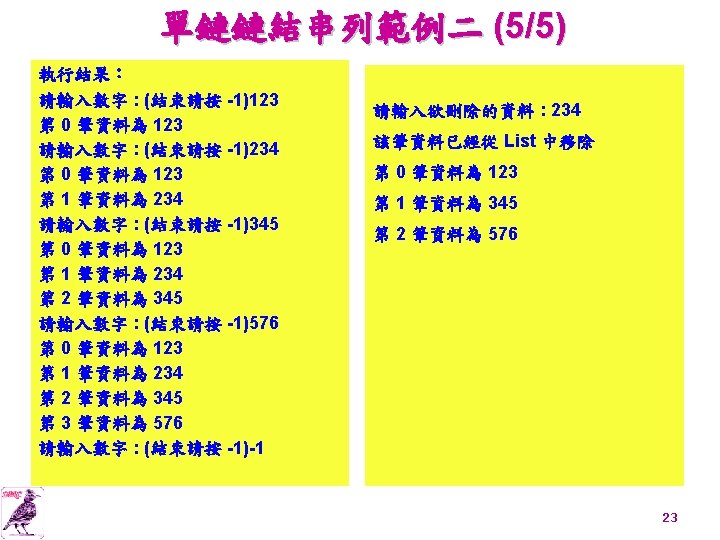
- Slides: 23
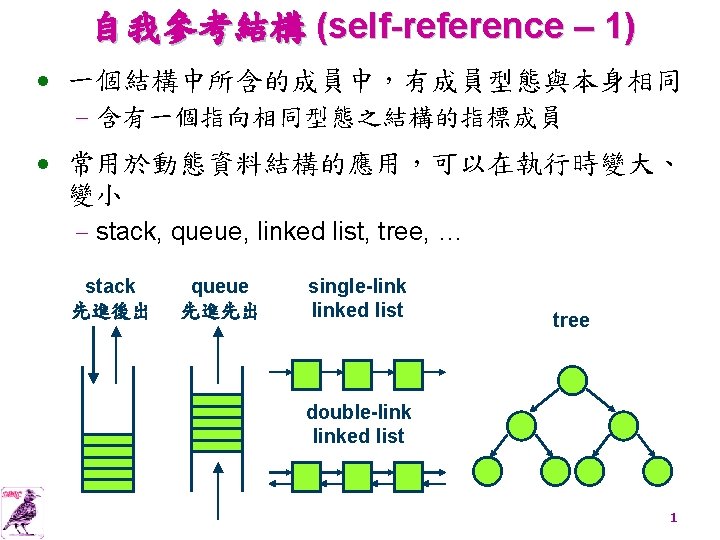
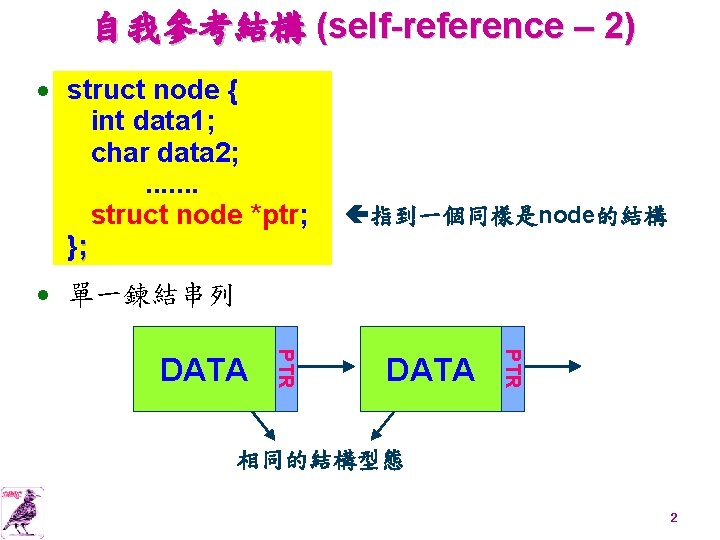
自我參考結構 (self-reference – 2) · struct node { int data 1; char data 2; . . . . struct node *ptr; 指到一個同樣是node的結構 }; · 單一鍊結串列 DATA PTR DATA 相同的結構型態 2
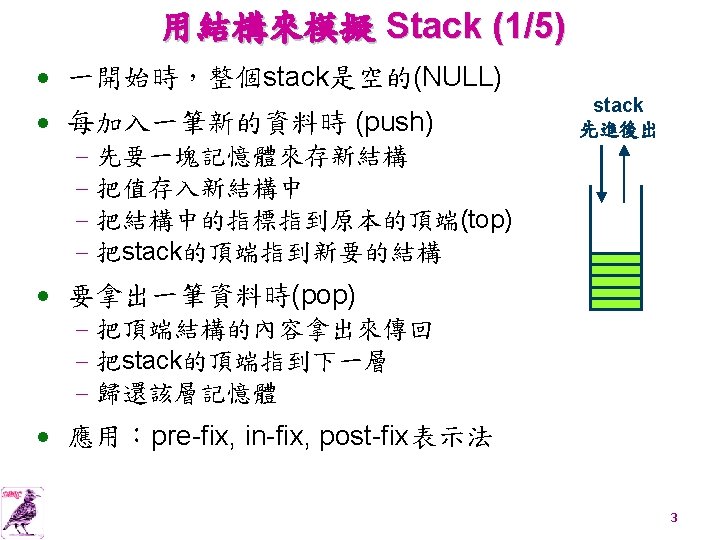
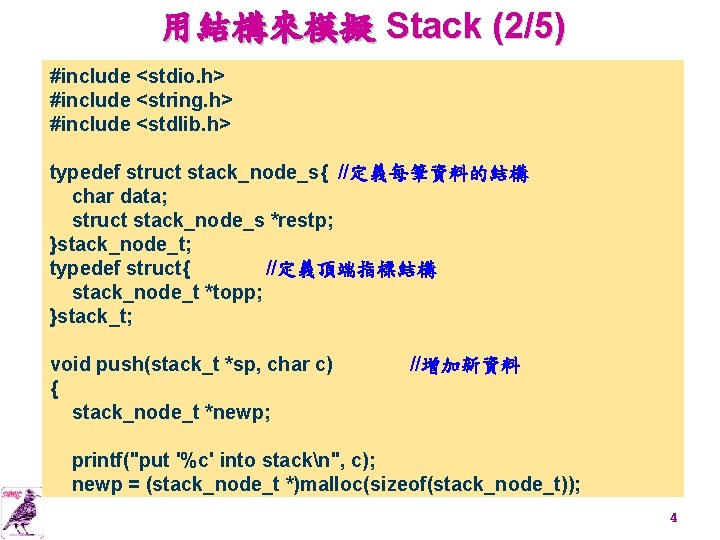
用結構來模擬 Stack (2/5) #include <stdio. h> #include <string. h> #include <stdlib. h> typedef struct stack_node_s{ //定義每筆資料的結構 char data; struct stack_node_s *restp; }stack_node_t; typedef struct{ //定義頂端指標結構 stack_node_t *topp; }stack_t; void push(stack_t *sp, char c) { stack_node_t *newp; //增加新資料 printf("put '%c' into stackn", c); newp = (stack_node_t *)malloc(sizeof(stack_node_t)); 4
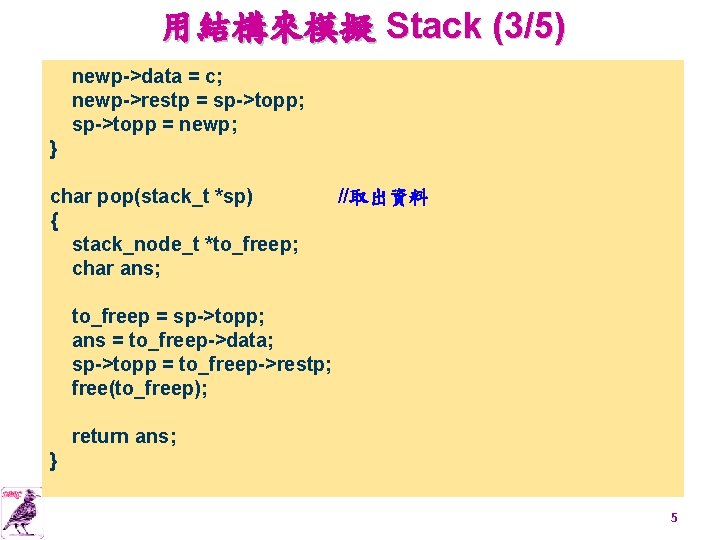
用結構來模擬 Stack (3/5) newp->data = c; newp->restp = sp->topp; sp->topp = newp; } char pop(stack_t *sp) { stack_node_t *to_freep; char ans; //取出資料 to_freep = sp->topp; ans = to_freep->data; sp->topp = to_freep->restp; free(to_freep); return ans; } 5
![用結構來模擬 Stack 45 int mainvoid stackt s NULL 程式一開始stack是空的 char str80 int 用結構來模擬 Stack (4/5) int main(void) { stack_t s = {NULL}; //程式一開始stack是空的 char str[80]; int](https://slidetodoc.com/presentation_image/4f0c38a0253619c288a131c0a8c77f43/image-6.jpg)
用結構來模擬 Stack (4/5) int main(void) { stack_t s = {NULL}; //程式一開始stack是空的 char str[80]; int i, l; printf(“Please enter a string for stack: "); gets(str); for(i = 0, l = strlen(str); i < l; i++) push(&s, str[i]); printf("the data popped from stack are listed in the followsn"); while (s. topp != NULL) printf("pop '%c' from stackn", pop(&s)); return 0; } 6
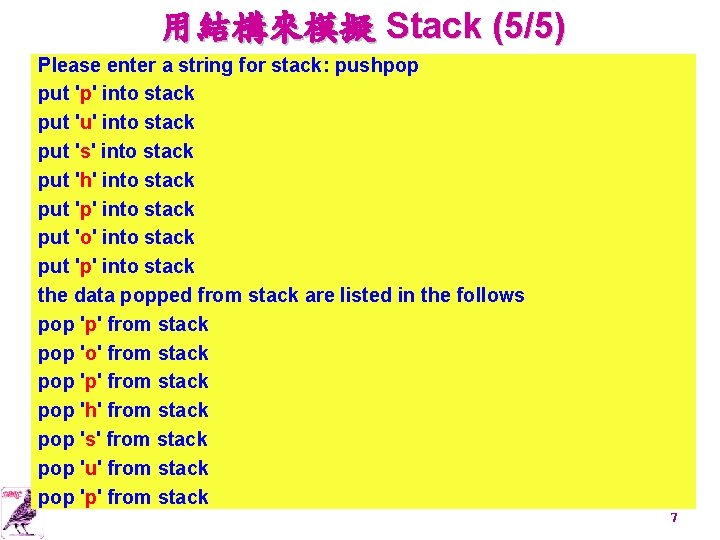
用結構來模擬 Stack (5/5) Please enter a string for stack: pushpop put 'p' into stack put 'u' into stack put 's' into stack put 'h' into stack put 'p' into stack put 'o' into stack put 'p' into stack the data popped from stack are listed in the follows pop 'p' from stack pop 'o' from stack pop 'p' from stack pop 'h' from stack pop 's' from stack pop 'u' from stack pop 'p' from stack 7
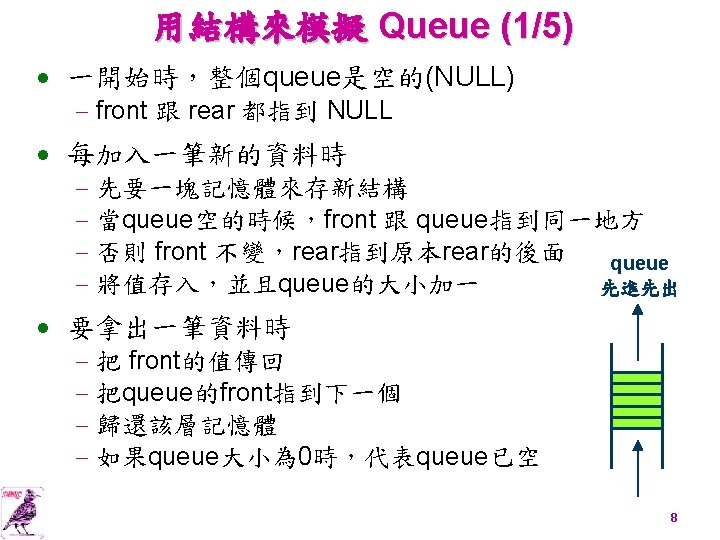
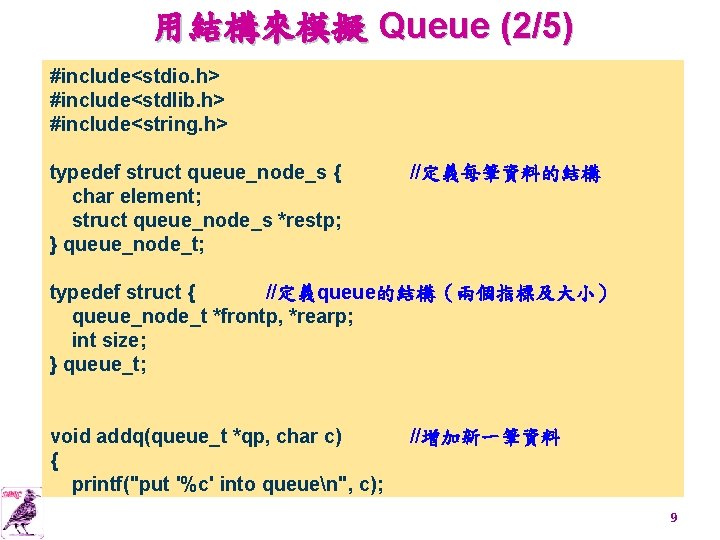
用結構來模擬 Queue (2/5) #include<stdio. h> #include<stdlib. h> #include<string. h> typedef struct queue_node_s { char element; struct queue_node_s *restp; } queue_node_t; //定義每筆資料的結構 typedef struct { //定義queue的結構(兩個指標及大小) queue_node_t *frontp, *rearp; int size; } queue_t; void addq(queue_t *qp, char c) { printf("put '%c' into queuen", c); //增加新一筆資料 9
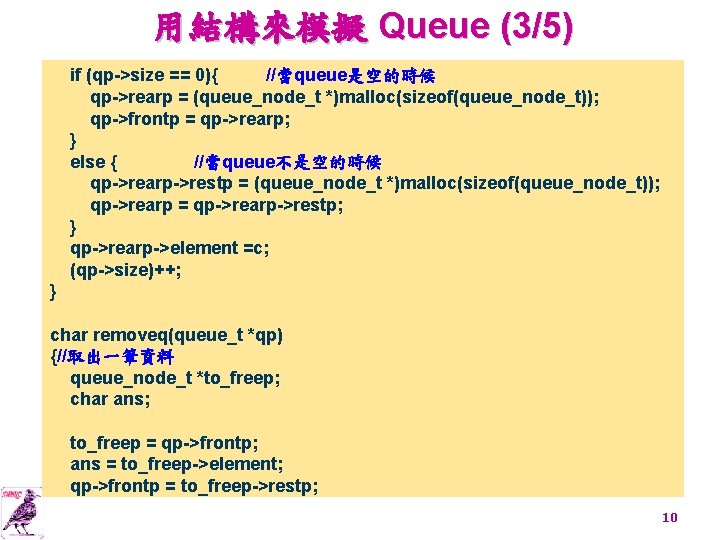
用結構來模擬 Queue (3/5) if (qp->size == 0){ //當queue是空的時候 qp->rearp = (queue_node_t *)malloc(sizeof(queue_node_t)); qp->frontp = qp->rearp; } else { //當queue不是空的時候 qp->rearp->restp = (queue_node_t *)malloc(sizeof(queue_node_t)); qp->rearp = qp->rearp->restp; } qp->rearp->element =c; (qp->size)++; } char removeq(queue_t *qp) {//取出一筆資料 queue_node_t *to_freep; char ans; to_freep = qp->frontp; ans = to_freep->element; qp->frontp = to_freep->restp; 10
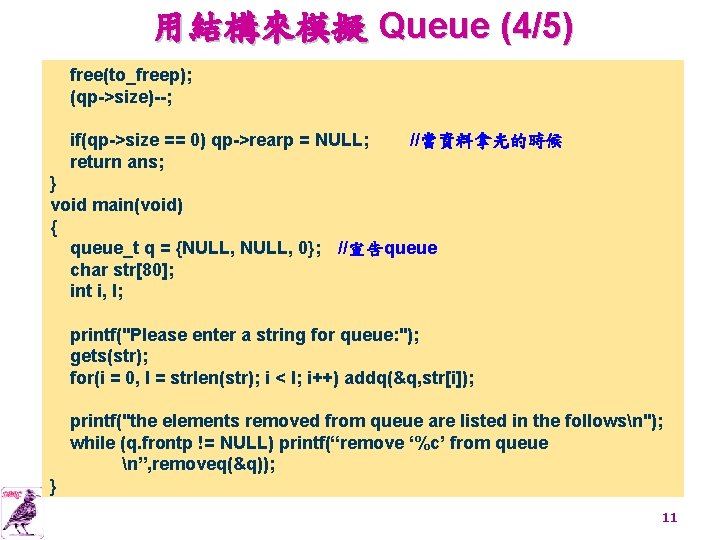
用結構來模擬 Queue (4/5) free(to_freep); (qp->size)--; if(qp->size == 0) qp->rearp = NULL; //當資料拿光的時候 return ans; } void main(void) { queue_t q = {NULL, 0}; //宣告queue char str[80]; int i, l; printf("Please enter a string for queue: "); gets(str); for(i = 0, l = strlen(str); i < l; i++) addq(&q, str[i]); printf("the elements removed from queue are listed in the followsn"); while (q. frontp != NULL) printf(“remove ‘%c’ from queue n”, removeq(&q)); } 11
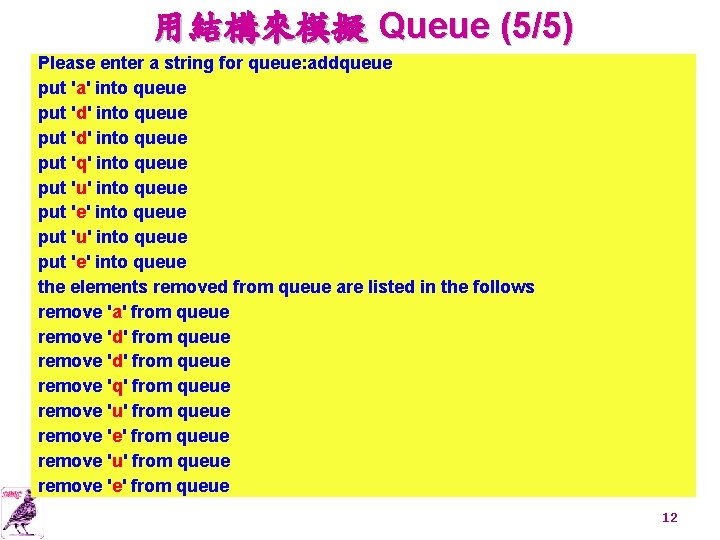
用結構來模擬 Queue (5/5) Please enter a string for queue: addqueue put 'a' into queue put 'd' into queue put 'q' into queue put 'u' into queue put 'e' into queue the elements removed from queue are listed in the follows remove 'a' from queue remove 'd' from queue remove 'q' from queue remove 'u' from queue remove 'e' from queue 12
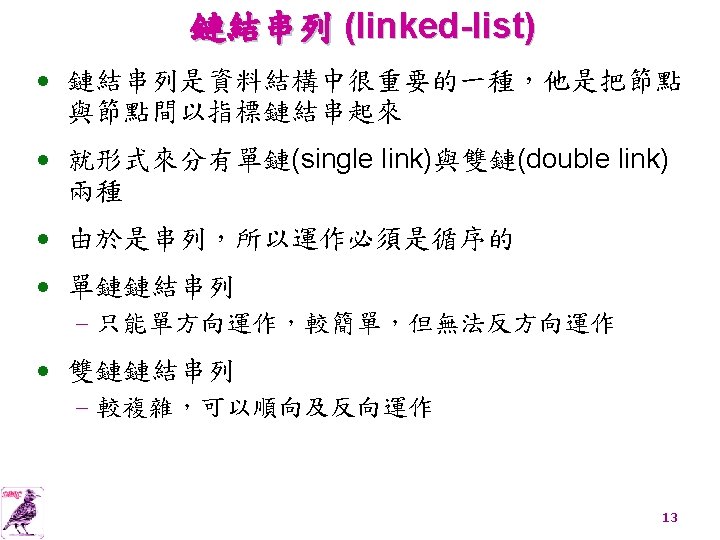
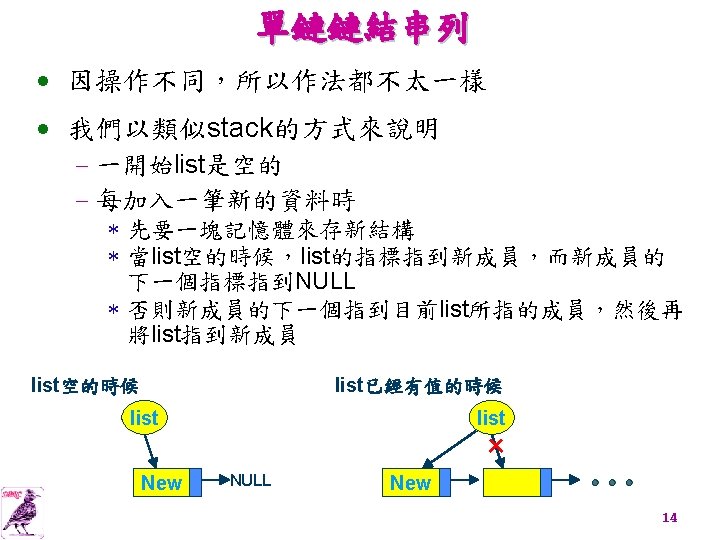
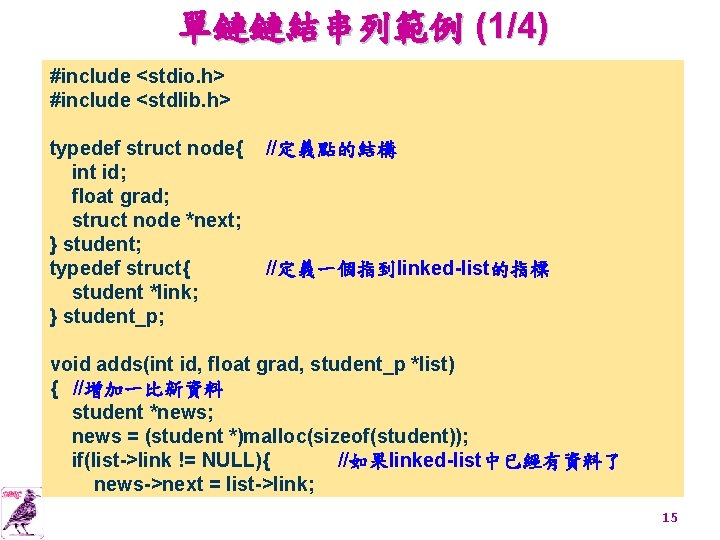
單鏈鏈結串列範例 (1/4) #include <stdio. h> #include <stdlib. h> typedef struct node{ int id; float grad; struct node *next; } student; typedef struct{ student *link; } student_p; //定義點的結構 //定義一個指到linked-list的指標 void adds(int id, float grad, student_p *list) { //增加一比新資料 student *news; news = (student *)malloc(sizeof(student)); if(list->link != NULL){ //如果linked-list中已經有資料了 news->next = list->link; 15
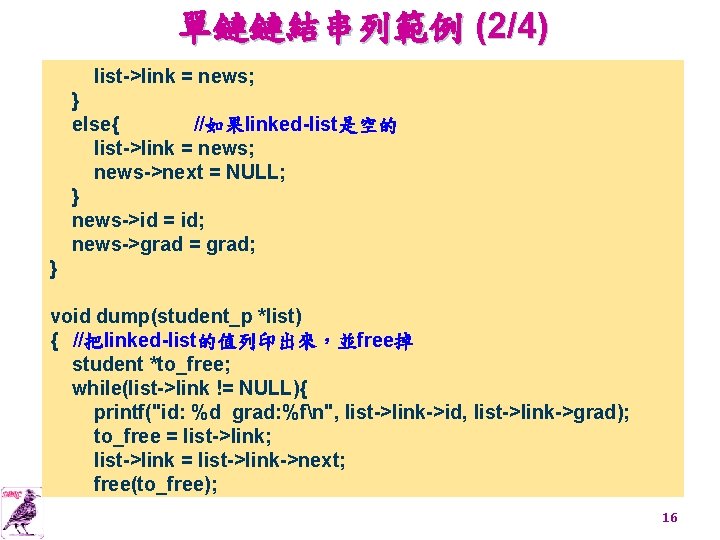
單鏈鏈結串列範例 (2/4) list->link = news; } else{ //如果linked-list是空的 list->link = news; news->next = NULL; } news->id = id; news->grad = grad; } void dump(student_p *list) { //把linked-list的值列印出來,並free掉 student *to_free; while(list->link != NULL){ printf("id: %d grad: %fn", list->link->id, list->link->grad); to_free = list->link; list->link = list->link->next; free(to_free); 16
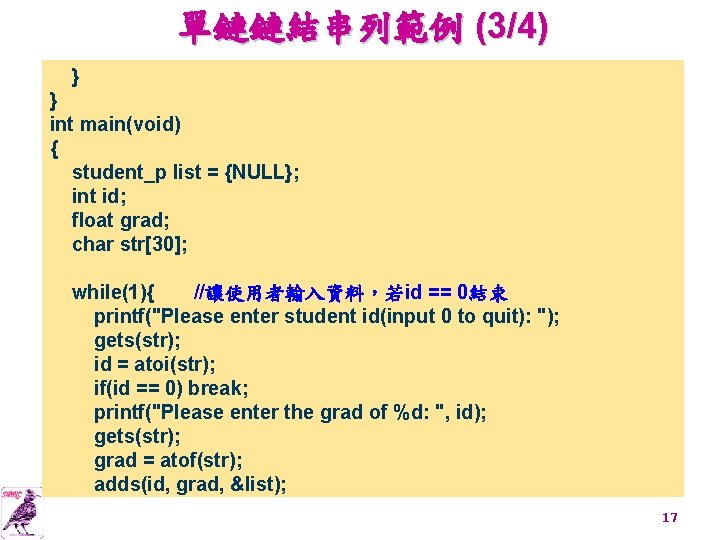
單鏈鏈結串列範例 (3/4) } } int main(void) { student_p list = {NULL}; int id; float grad; char str[30]; while(1){ //讓使用者輸入資料,若id == 0結束 printf("Please enter student id(input 0 to quit): "); gets(str); id = atoi(str); if(id == 0) break; printf("Please enter the grad of %d: ", id); gets(str); grad = atof(str); adds(id, grad, &list); 17
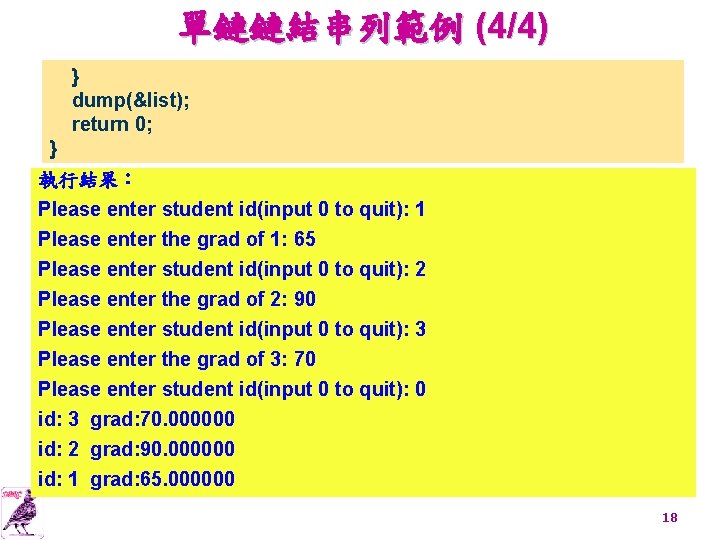
單鏈鏈結串列範例 (4/4) } dump(&list); return 0; } 執行結果: Please enter student id(input 0 to quit): 1 Please enter the grad of 1: 65 Please enter student id(input 0 to quit): 2 Please enter the grad of 2: 90 Please enter student id(input 0 to quit): 3 Please enter the grad of 3: 70 Please enter student id(input 0 to quit): 0 id: 3 grad: 70. 000000 id: 2 grad: 90. 000000 id: 1 grad: 65. 000000 18
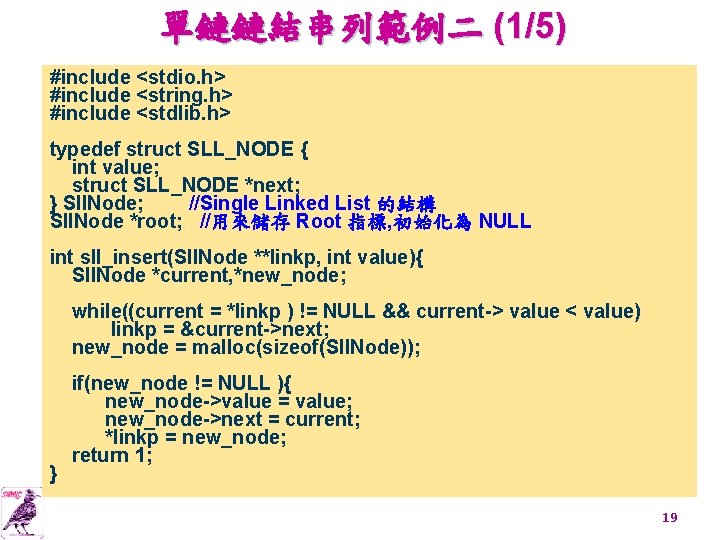
單鏈鏈結串列範例二 (1/5) #include <stdio. h> #include <string. h> #include <stdlib. h> typedef struct SLL_NODE { int value; struct SLL_NODE *next; } Sll. Node; //Single Linked List 的結構 Sll. Node *root; //用來儲存 Root 指標, 初始化為 NULL int sll_insert(Sll. Node **linkp, int value){ Sll. Node *current, *new_node; while((current = *linkp ) != NULL && current-> value < value) linkp = ¤t->next; new_node = malloc(sizeof(Sll. Node)); if(new_node != NULL ){ new_node->value = value; new_node->next = current; *linkp = new_node; return 1; } 19
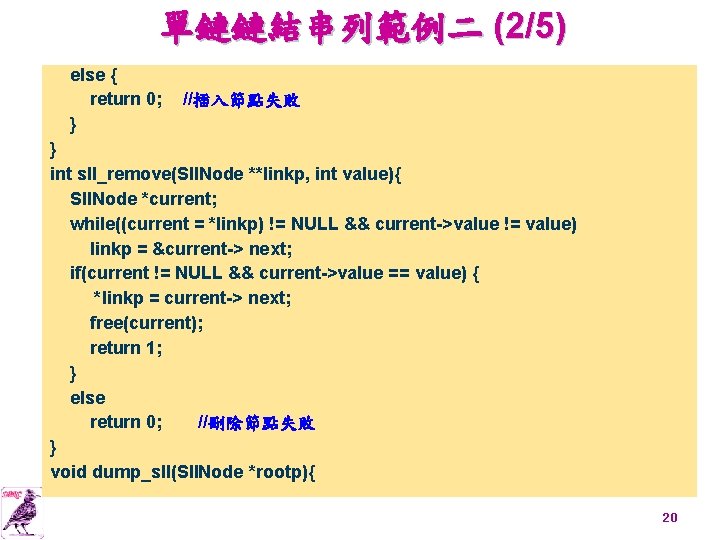
單鏈鏈結串列範例二 (2/5) else { return 0; //插入節點失敗 } } int sll_remove(Sll. Node **linkp, int value){ Sll. Node *current; while((current = *linkp) != NULL && current->value != value) linkp = ¤t-> next; if(current != NULL && current->value == value) { *linkp = current-> next; free(current); return 1; } else return 0; //刪除節點失敗 } void dump_sll(Sll. Node *rootp){ 20
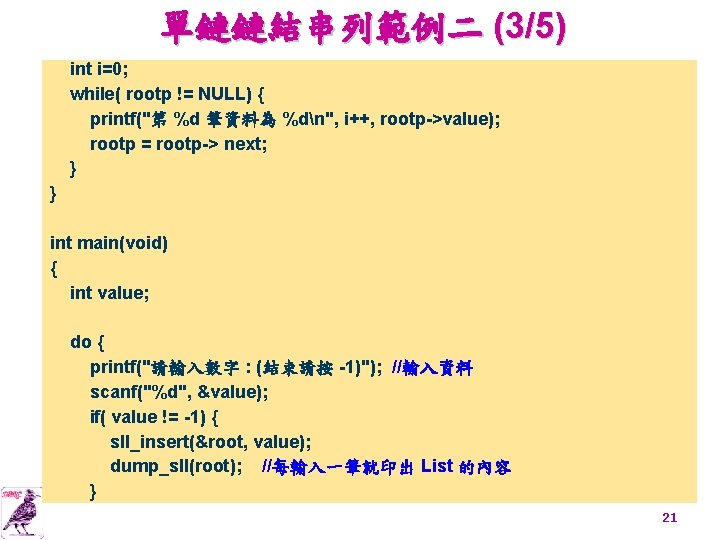
單鏈鏈結串列範例二 (3/5) int i=0; while( rootp != NULL) { printf("第 %d 筆資料為 %dn", i++, rootp->value); rootp = rootp-> next; } } int main(void) { int value; do { printf("請輸入數字 : (結束請按 -1)"); //輸入資料 scanf("%d", &value); if( value != -1) { sll_insert(&root, value); dump_sll(root); //每輸入一筆就印出 List 的內容 } 21
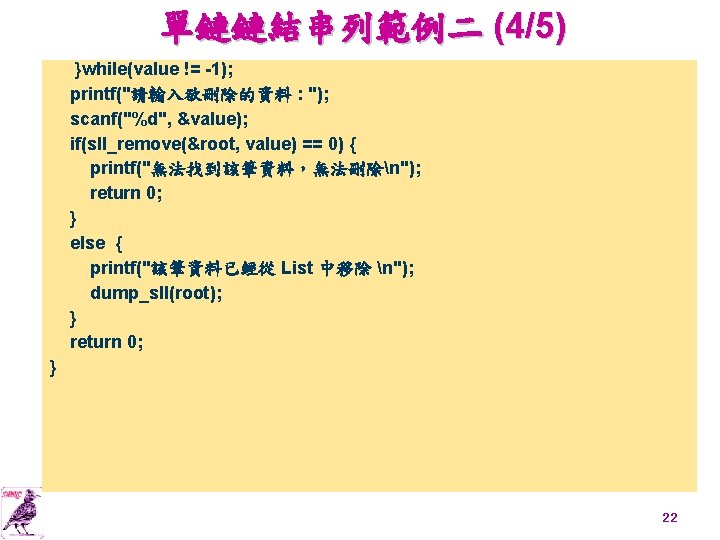
單鏈鏈結串列範例二 (4/5) }while(value != -1); printf("請輸入欲刪除的資料 : "); scanf("%d", &value); if(sll_remove(&root, value) == 0) { printf("無法找到該筆資料,無法刪除n"); return 0; } else { printf("該筆資料已經從 List 中移除 n"); dump_sll(root); } return 0; } 22
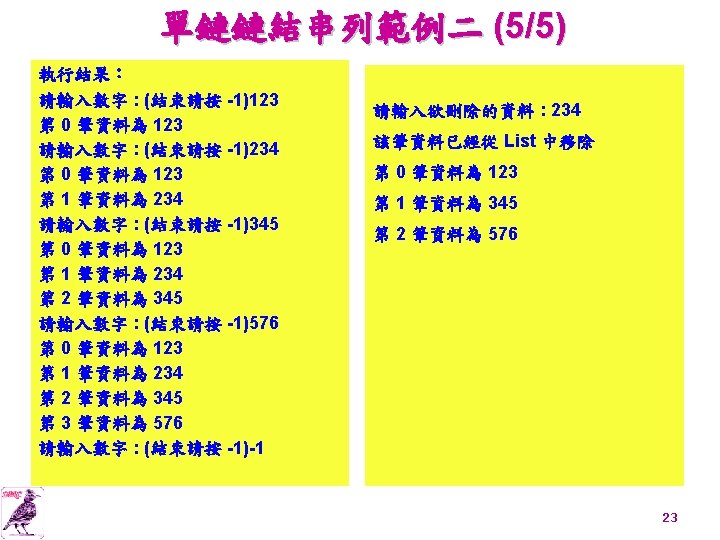