Searching and Sorting Chapter 14 What is searching
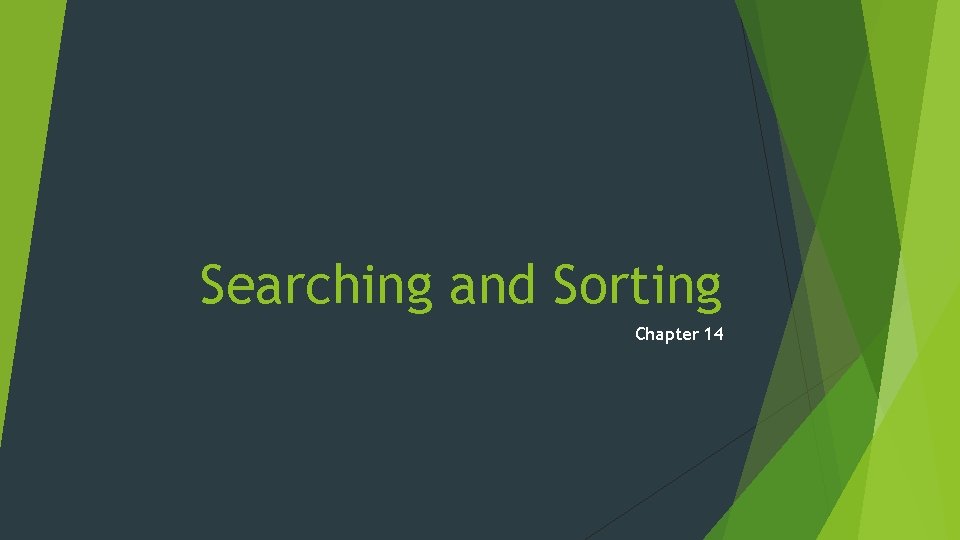
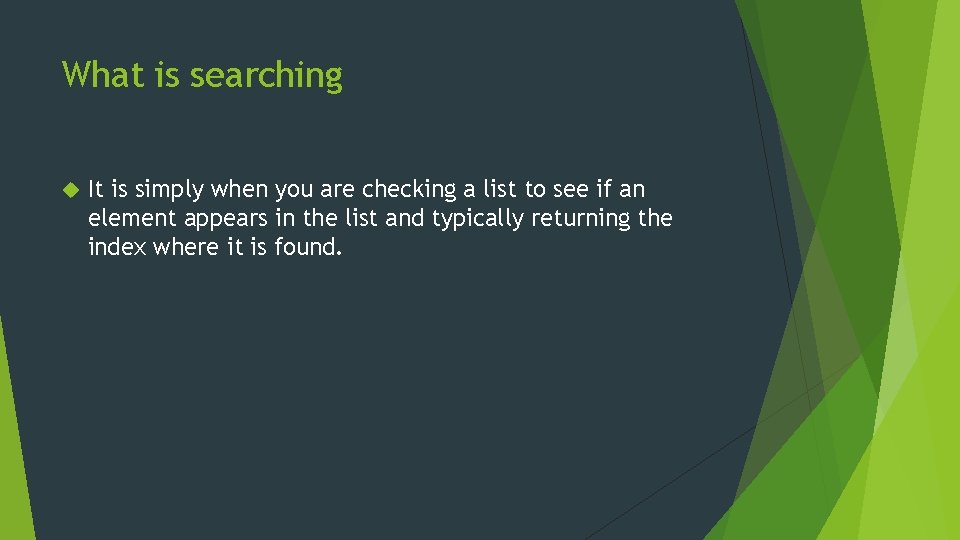
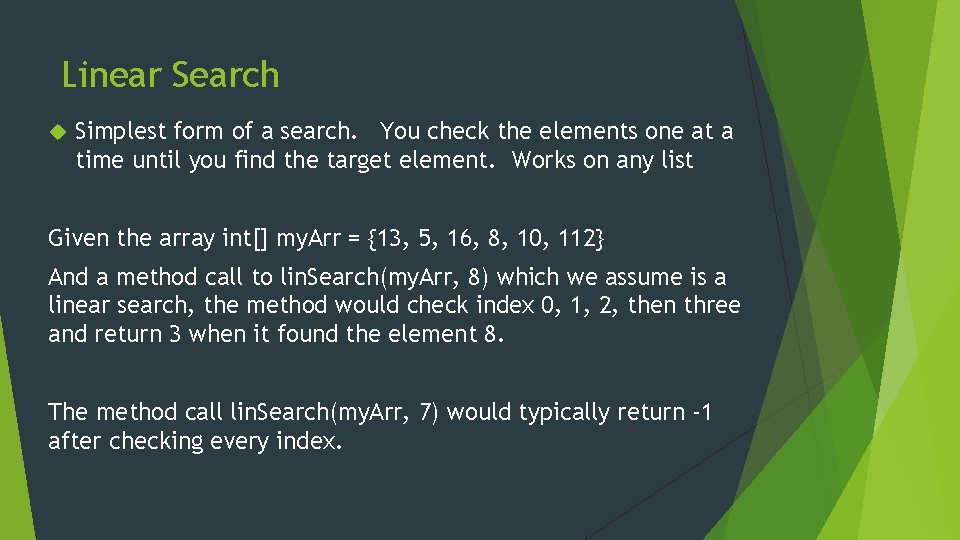
![Sample implementation public int(int[] arr, int target) { for ( int i = 0; Sample implementation public int(int[] arr, int target) { for ( int i = 0;](https://slidetodoc.com/presentation_image_h2/4b2d9057f6bda7a49c6b44ac211b1af4/image-4.jpg)
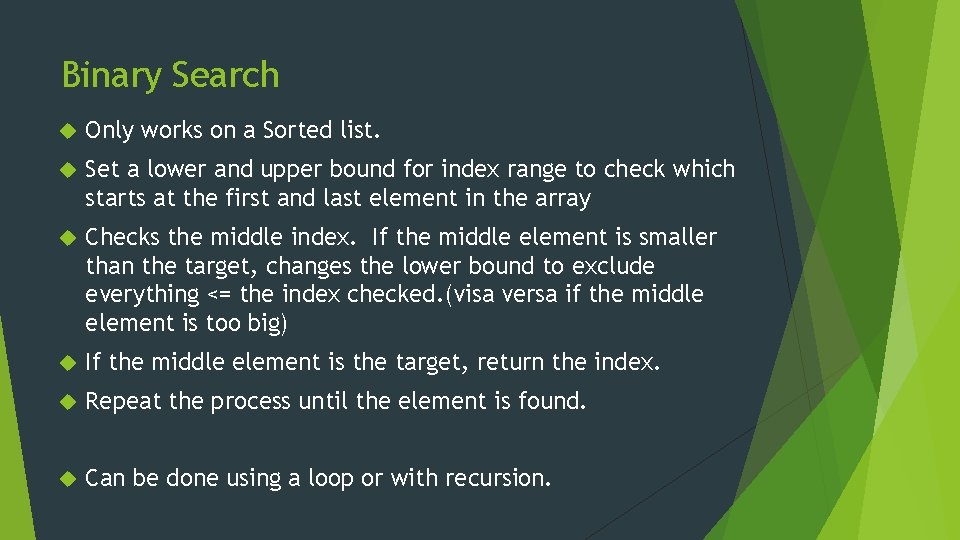
![Binary Search Given int[] my. Arr = {2, 4, 6, 8, 10, 12, 14, Binary Search Given int[] my. Arr = {2, 4, 6, 8, 10, 12, 14,](https://slidetodoc.com/presentation_image_h2/4b2d9057f6bda7a49c6b44ac211b1af4/image-6.jpg)
![Sample implementation public int bin. Search(int[] sorted. Array, int target) { int low = Sample implementation public int bin. Search(int[] sorted. Array, int target) { int low =](https://slidetodoc.com/presentation_image_h2/4b2d9057f6bda7a49c6b44ac211b1af4/image-7.jpg)
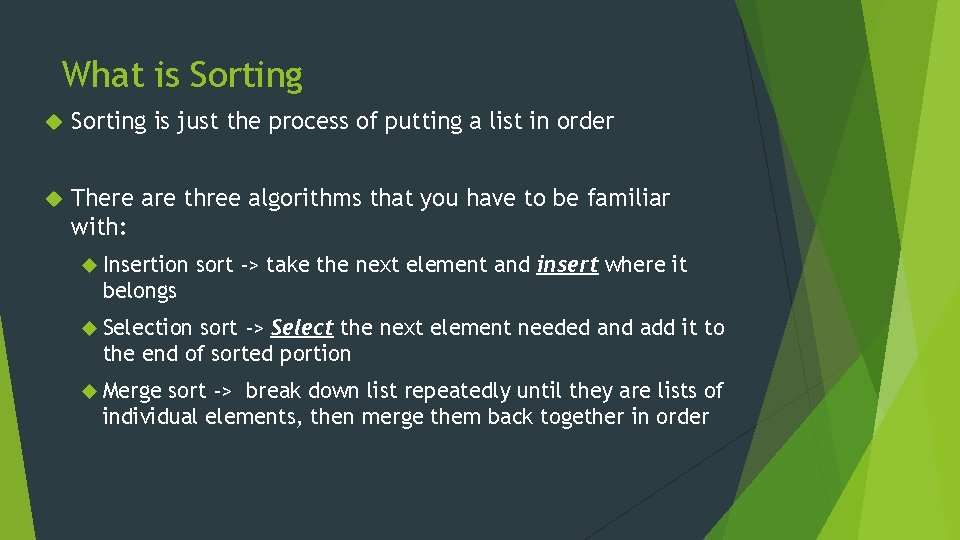
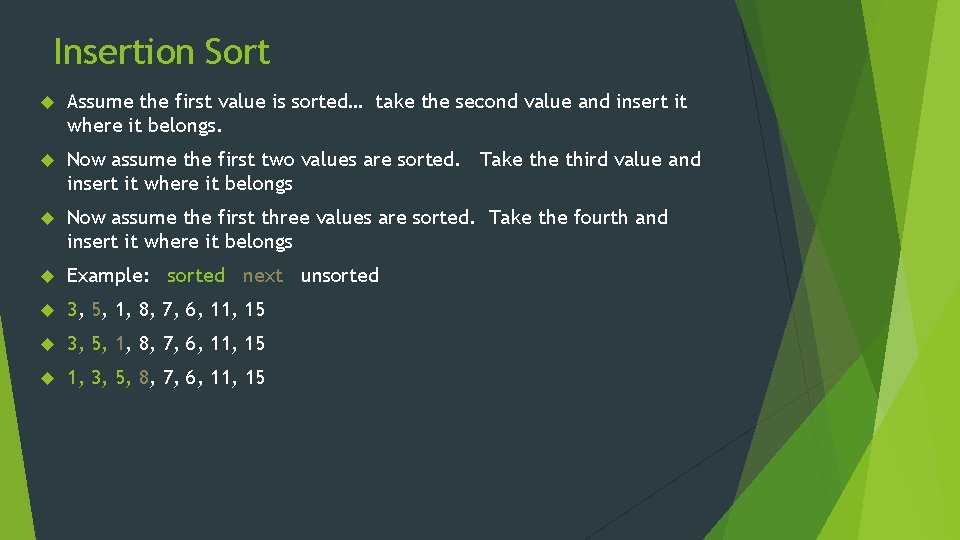
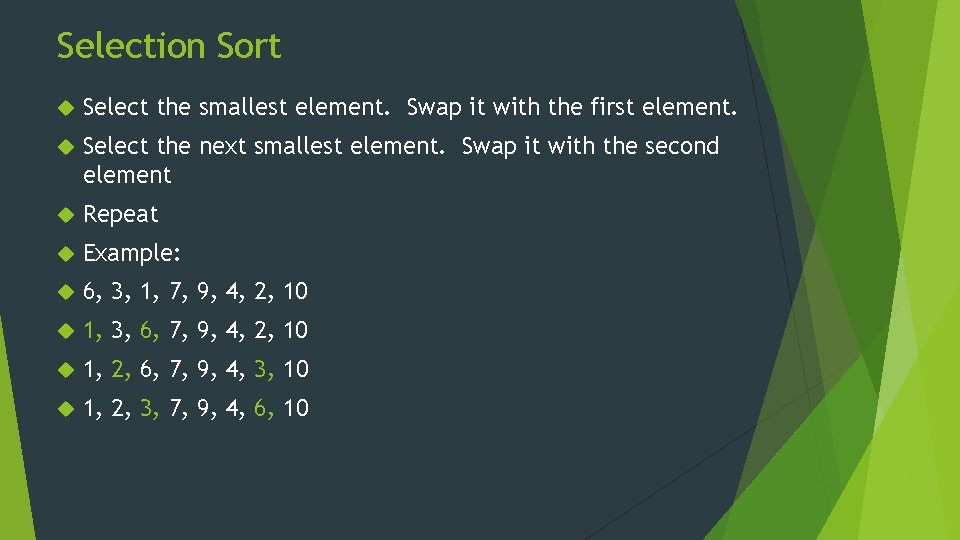
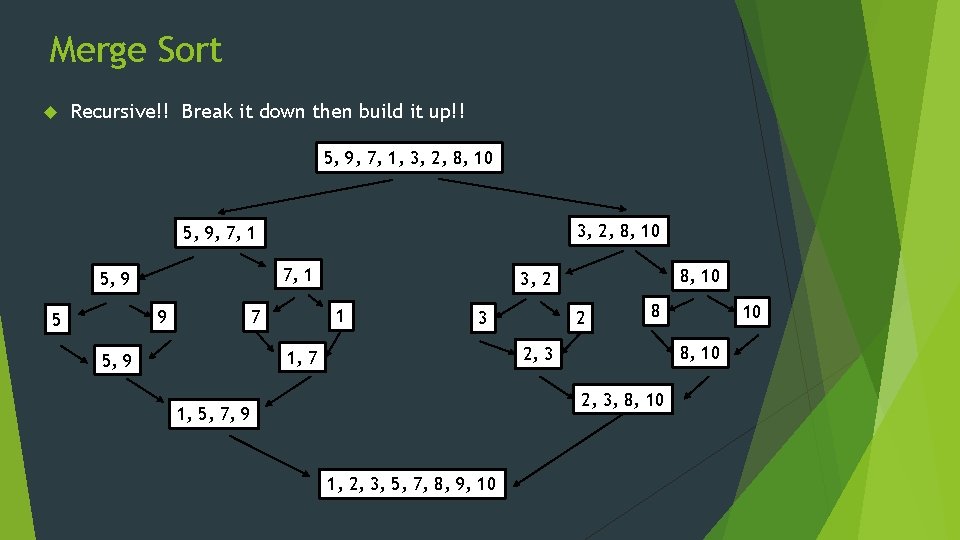
- Slides: 11
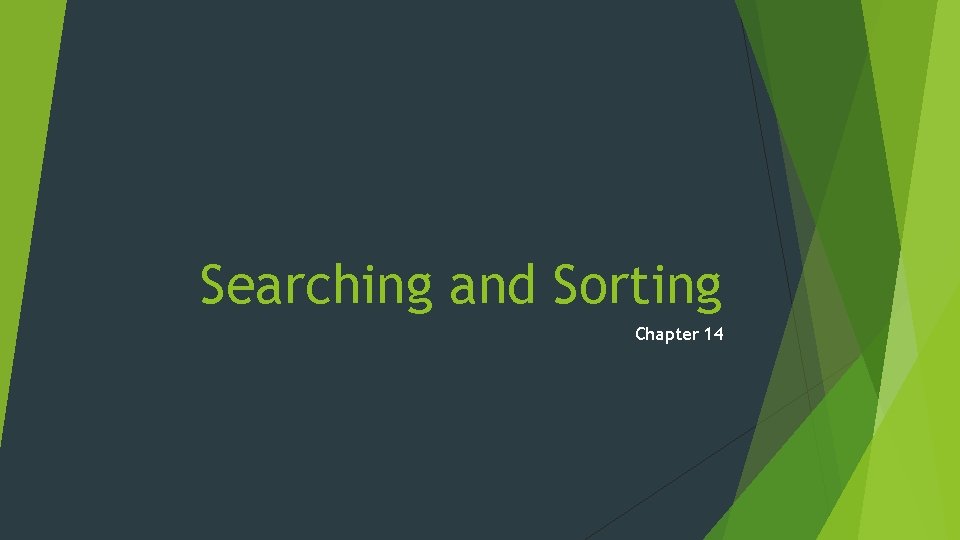
Searching and Sorting Chapter 14
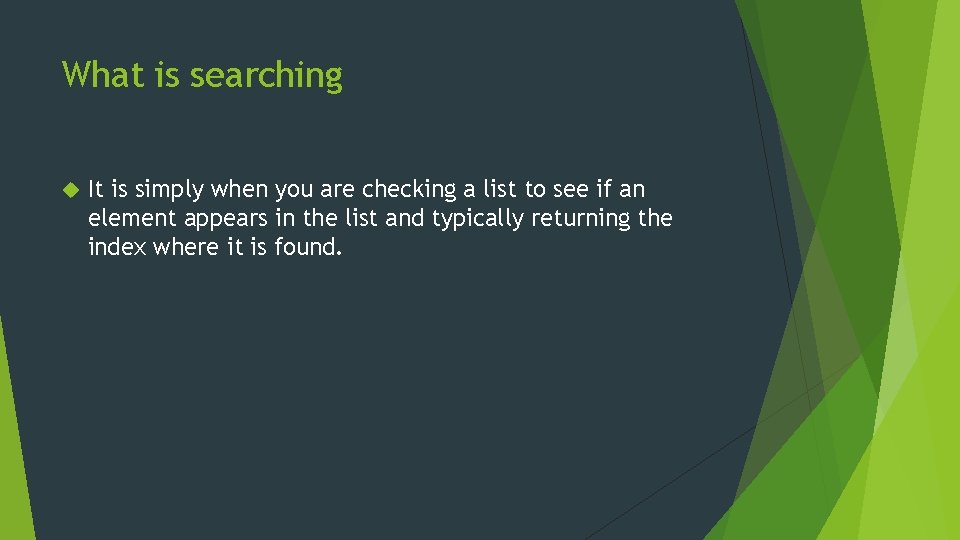
What is searching It is simply when you are checking a list to see if an element appears in the list and typically returning the index where it is found.
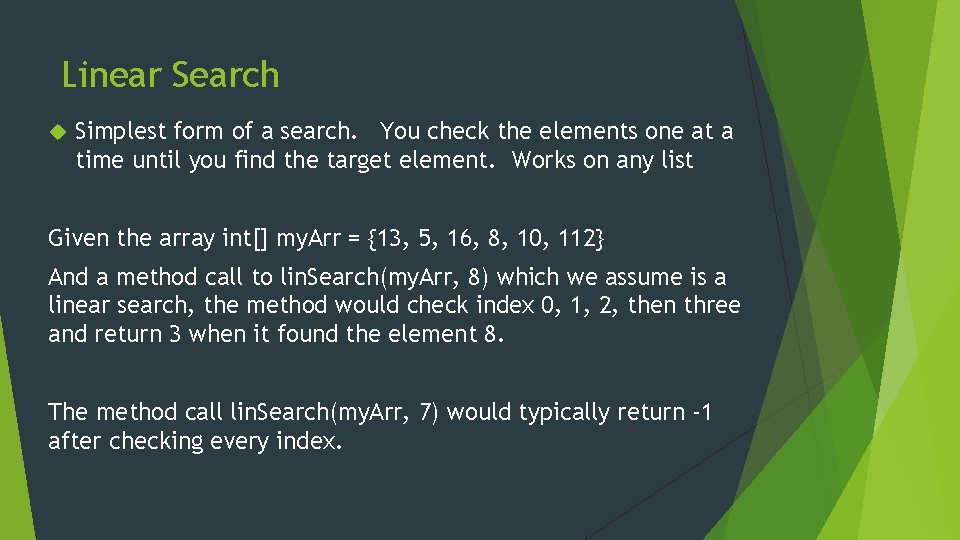
Linear Search Simplest form of a search. You check the elements one at a time until you find the target element. Works on any list Given the array int[] my. Arr = {13, 5, 16, 8, 10, 112} And a method call to lin. Search(my. Arr, 8) which we assume is a linear search, the method would check index 0, 1, 2, then three and return 3 when it found the element 8. The method call lin. Search(my. Arr, 7) would typically return -1 after checking every index.
![Sample implementation public intint arr int target for int i 0 Sample implementation public int(int[] arr, int target) { for ( int i = 0;](https://slidetodoc.com/presentation_image_h2/4b2d9057f6bda7a49c6b44ac211b1af4/image-4.jpg)
Sample implementation public int(int[] arr, int target) { for ( int i = 0; i < arr. length; i++) { if (arr[i] == target) return I; } return -1; }
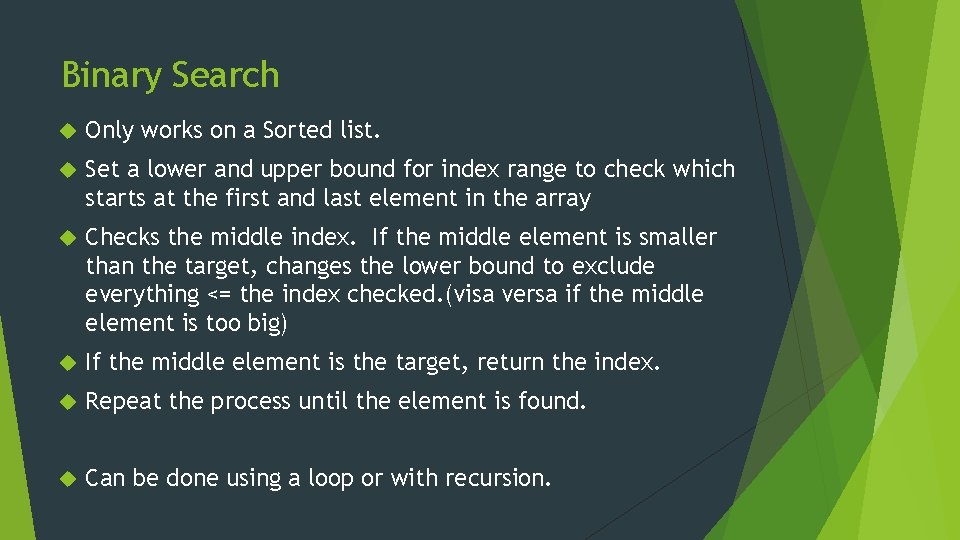
Binary Search Only works on a Sorted list. Set a lower and upper bound for index range to check which starts at the first and last element in the array Checks the middle index. If the middle element is smaller than the target, changes the lower bound to exclude everything <= the index checked. (visa versa if the middle element is too big) If the middle element is the target, return the index. Repeat the process until the element is found. Can be done using a loop or with recursion.
![Binary Search Given int my Arr 2 4 6 8 10 12 14 Binary Search Given int[] my. Arr = {2, 4, 6, 8, 10, 12, 14,](https://slidetodoc.com/presentation_image_h2/4b2d9057f6bda7a49c6b44ac211b1af4/image-6.jpg)
Binary Search Given int[] my. Arr = {2, 4, 6, 8, 10, 12, 14, 16}; Assuming bin. Search is an implemented binary search, the method call bin. Search(my. Arr, 12) would do the following: Set lower. Bound = 0, and upper. Bound = 7 Find middle index by averaging: (0+7)/2 = 3 (integer div) Check index 3 which is 8… 8 <12 so all data from index 0 -3 can be excluded. Set lower. Bound = 4 Repeat: find middle index: (4 + 7) / 2 = 5 Index 5 contains element 12, our target. Return 5.
![Sample implementation public int bin Searchint sorted Array int target int low Sample implementation public int bin. Search(int[] sorted. Array, int target) { int low =](https://slidetodoc.com/presentation_image_h2/4b2d9057f6bda7a49c6b44ac211b1af4/image-7.jpg)
Sample implementation public int bin. Search(int[] sorted. Array, int target) { int low = 0, high = sorted. Array. length-1; while (low <= high) { int mid = (low + high) / 2; if (sorted. Array[mid] < target) low = mid + 1; else if (sorted. Array[mid] > target) high = mid - 1; else if (sorted. Array[mid] == key) { return mid; } } return -1; }
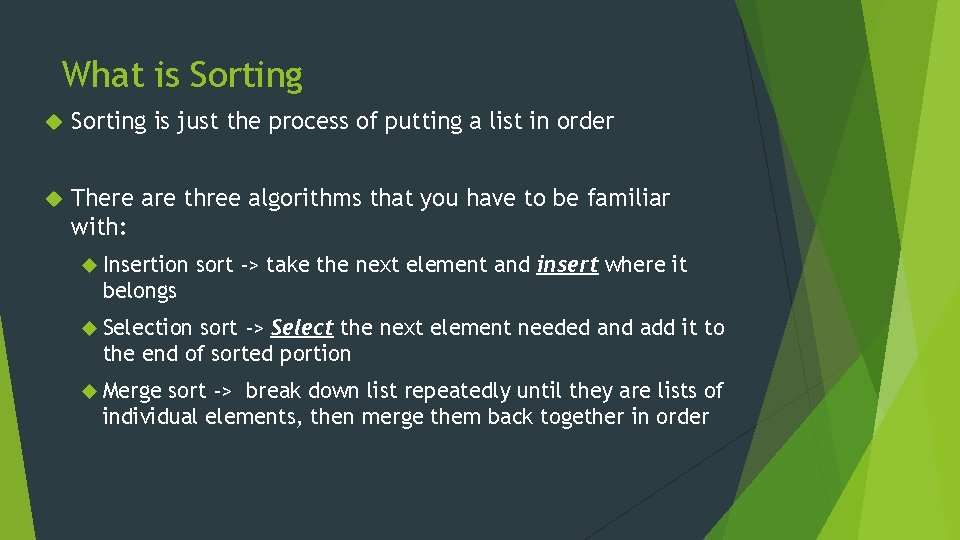
What is Sorting is just the process of putting a list in order There are three algorithms that you have to be familiar with: Insertion sort -> take the next element and insert where it belongs Selection sort -> Select the next element needed and add it to the end of sorted portion Merge sort -> break down list repeatedly until they are lists of individual elements, then merge them back together in order
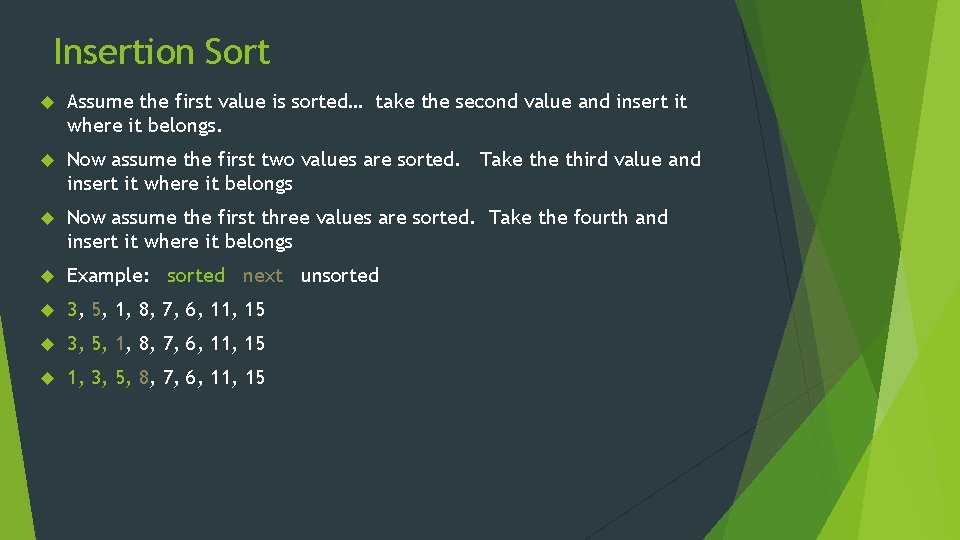
Insertion Sort Assume the first value is sorted… take the second value and insert it where it belongs. Now assume the first two values are sorted. Take third value and insert it where it belongs Now assume the first three values are sorted. Take the fourth and insert it where it belongs Example: sorted next unsorted 3, 5, 1, 8, 7, 6, 11, 15 1, 3, 5, 8, 7, 6, 11, 15
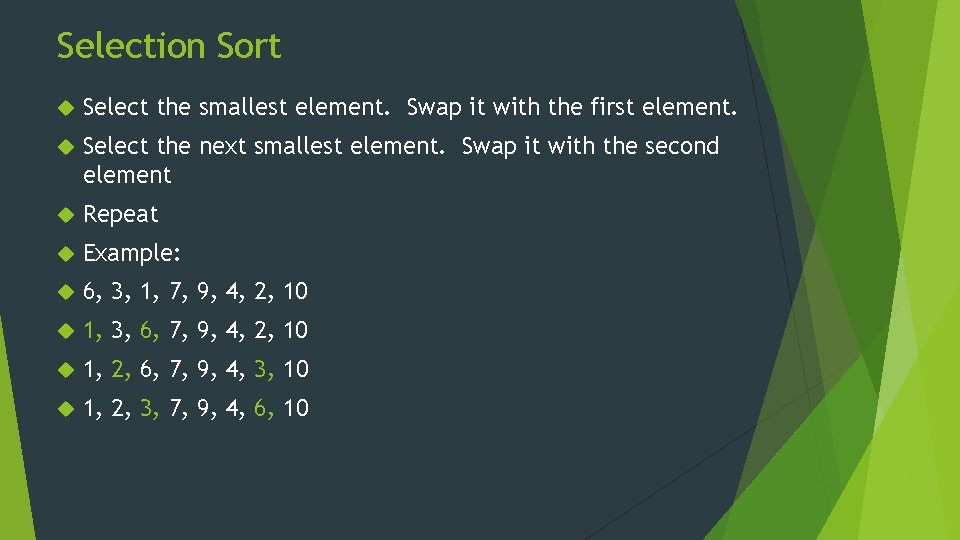
Selection Sort Select the smallest element. Swap it with the first element. Select the next smallest element. Swap it with the second element Repeat Example: 6, 3, 1, 7, 9, 4, 2, 10 1, 3, 6, 7, 9, 4, 2, 10 1, 2, 6, 7, 9, 4, 3, 10 1, 2, 3, 7, 9, 4, 6, 10
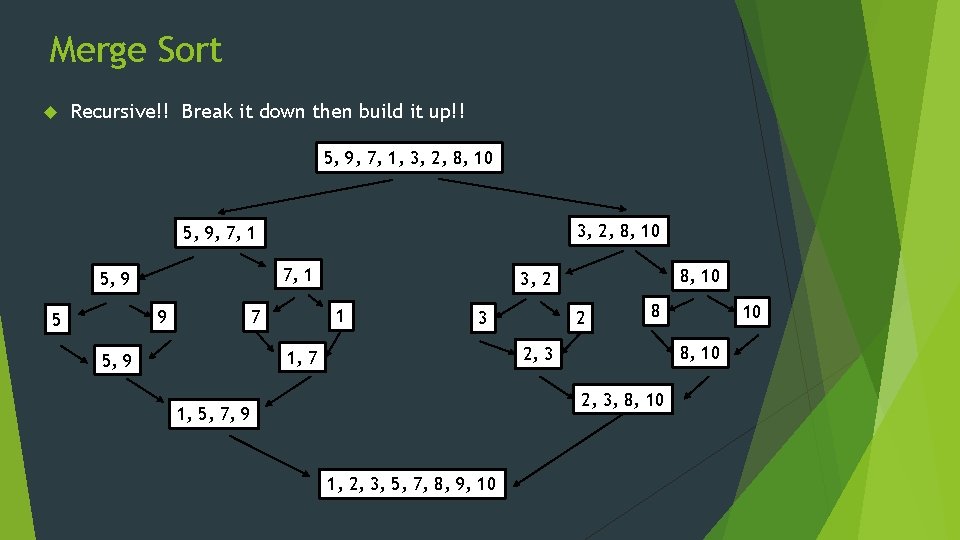
Merge Sort Recursive!! Break it down then build it up!! 5, 9, 7, 1, 3, 2, 8, 10 5, 9, 7, 1 5, 9 1 7 9 5 3 2 8 2, 3, 8, 10 1, 5, 7, 9 1, 2, 3, 5, 7, 8, 9, 10 10 8, 10 2, 3 1, 7 5, 9 8, 10 3, 2