SE 320 Introduction to Game Development Lecture 5
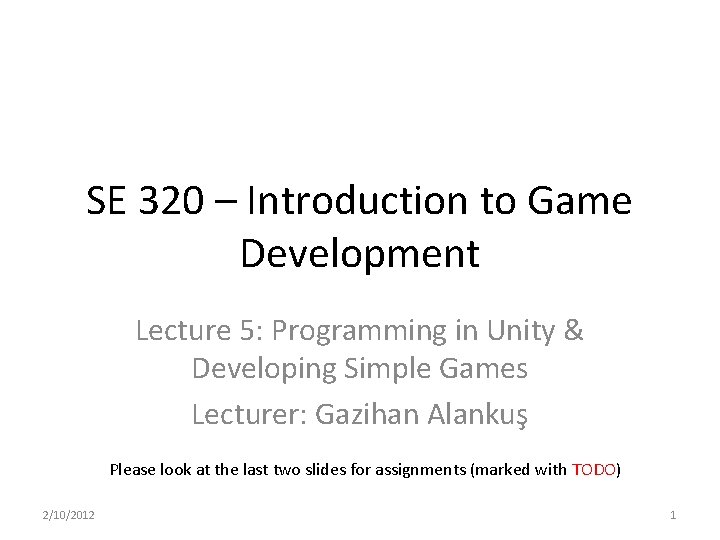
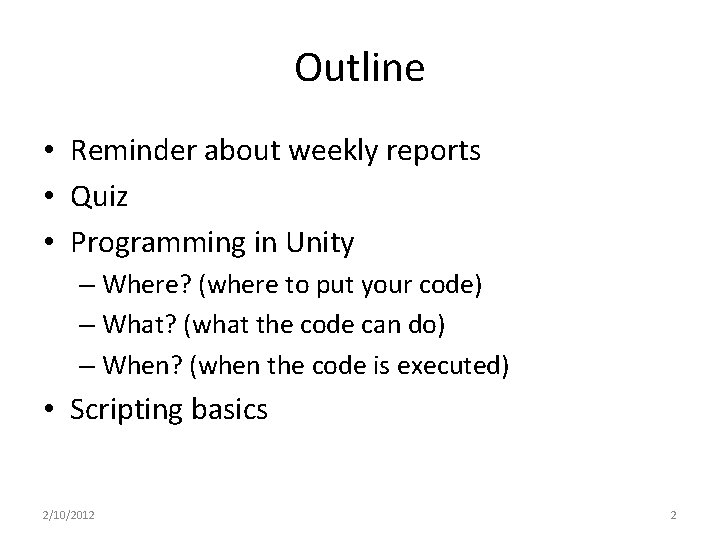
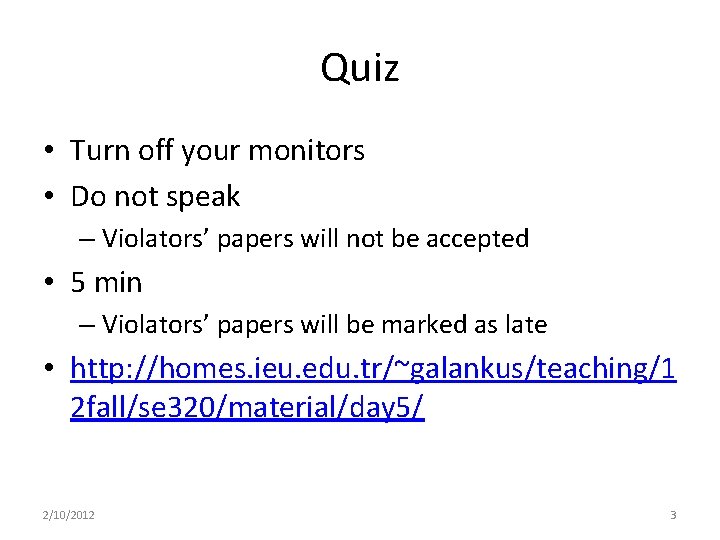
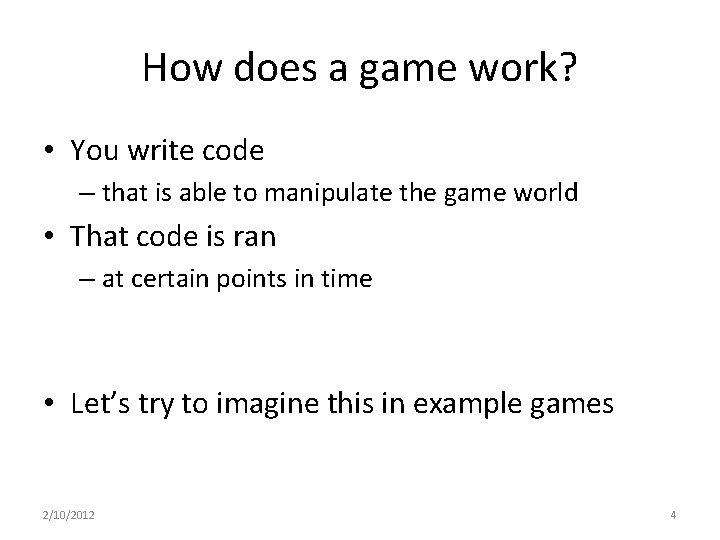
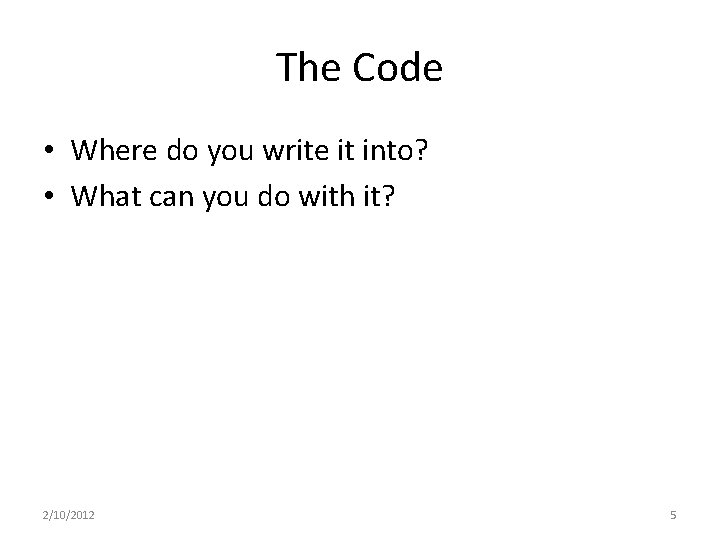
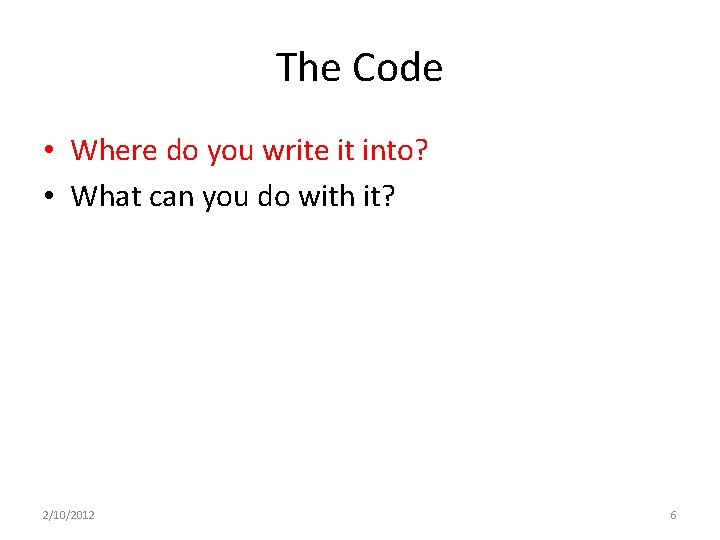
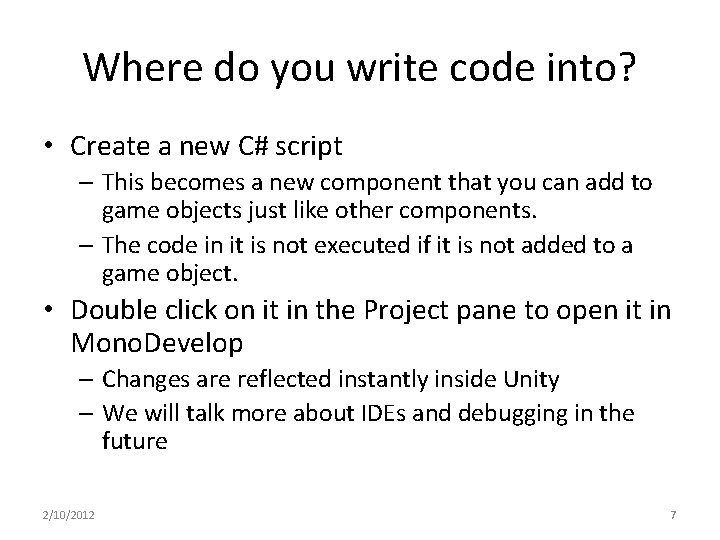
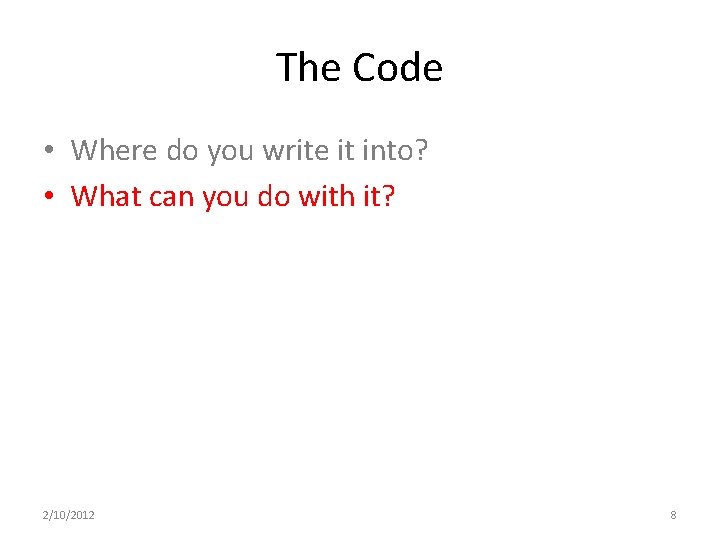
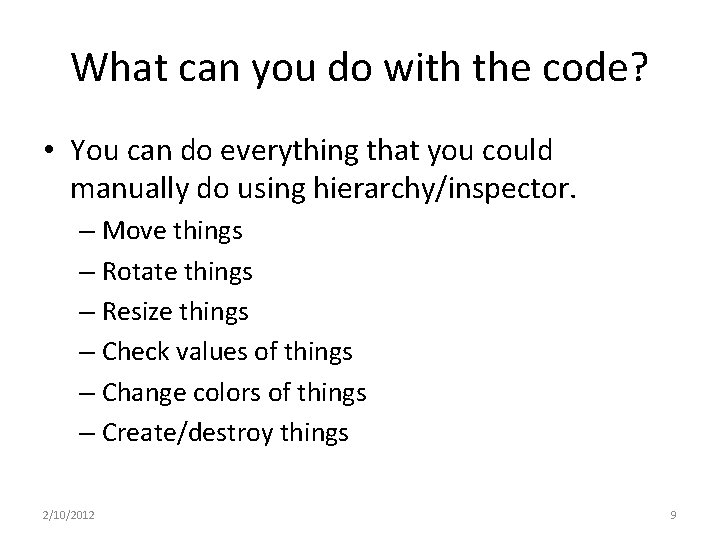
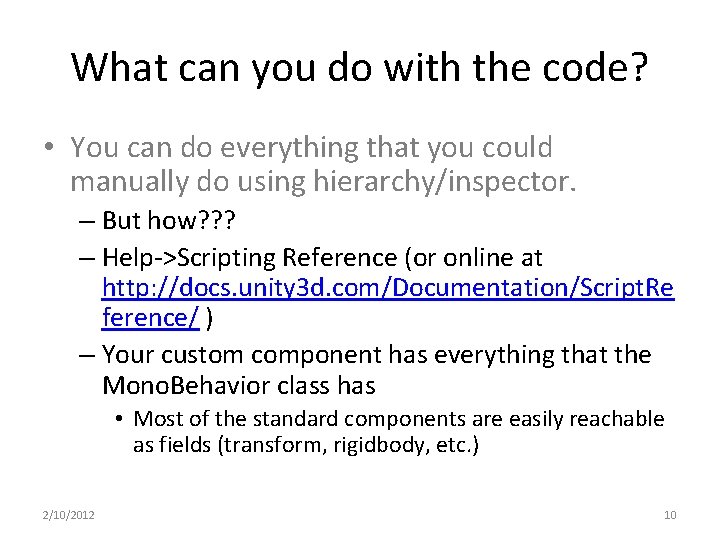
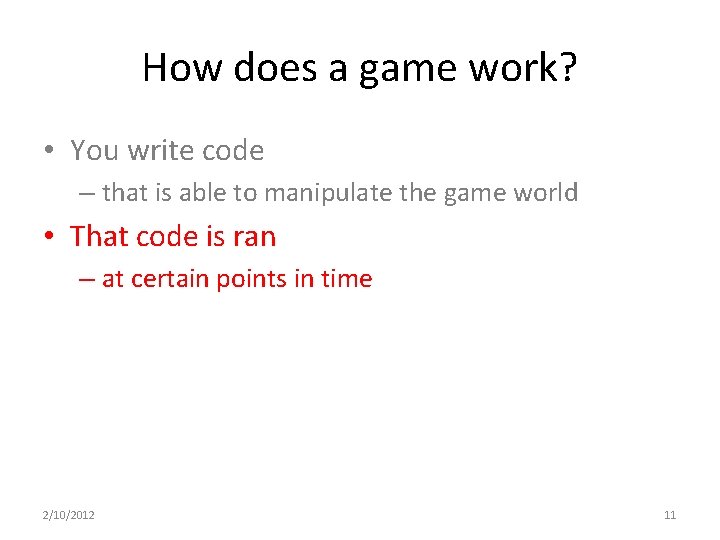
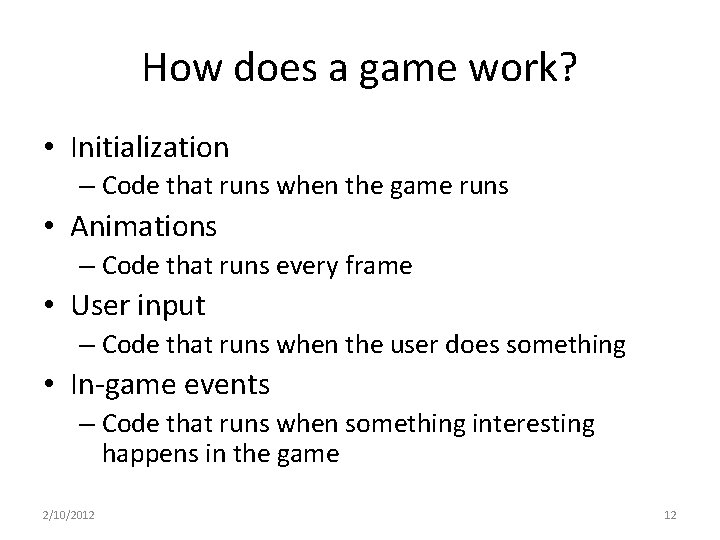
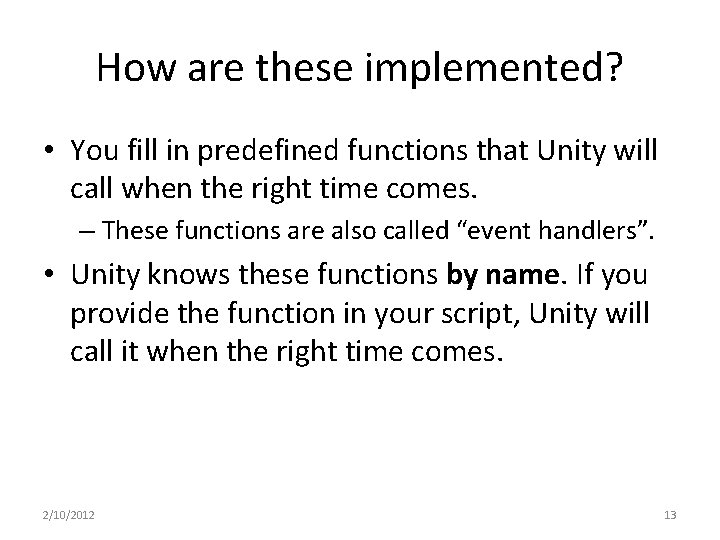
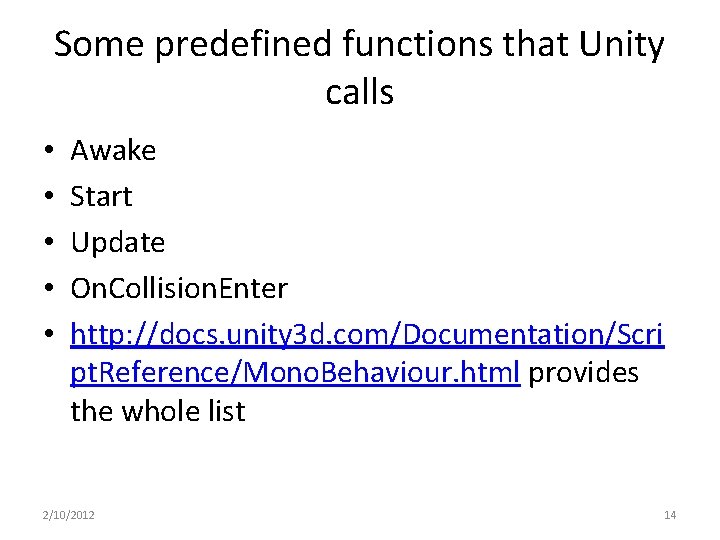
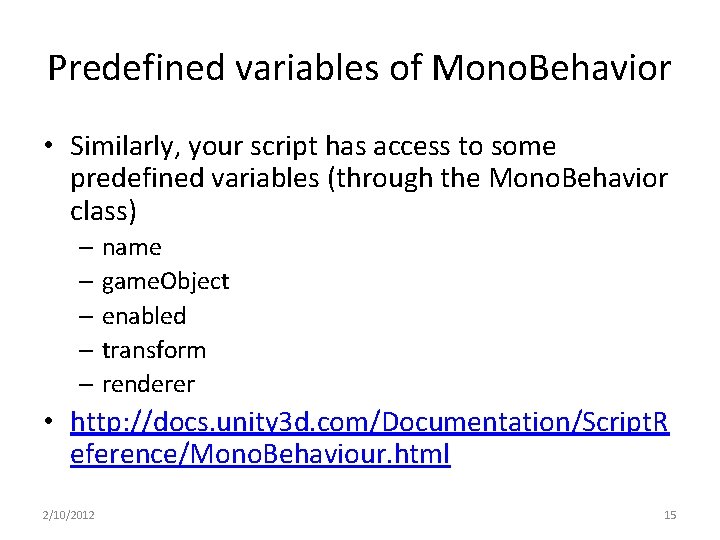
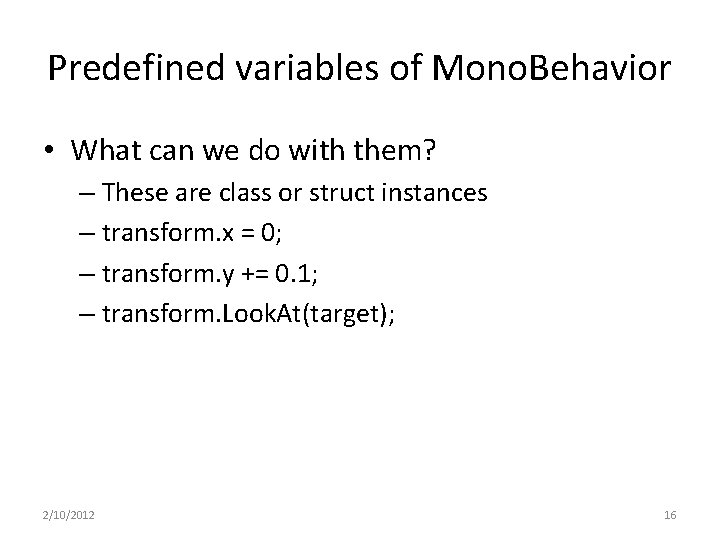
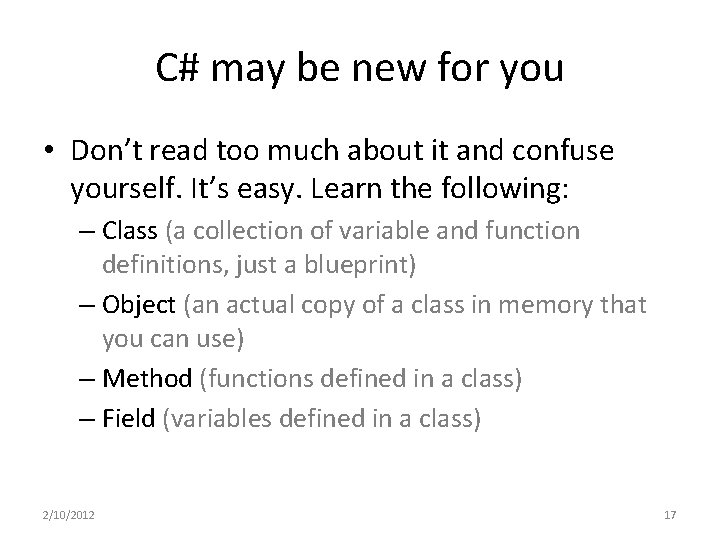
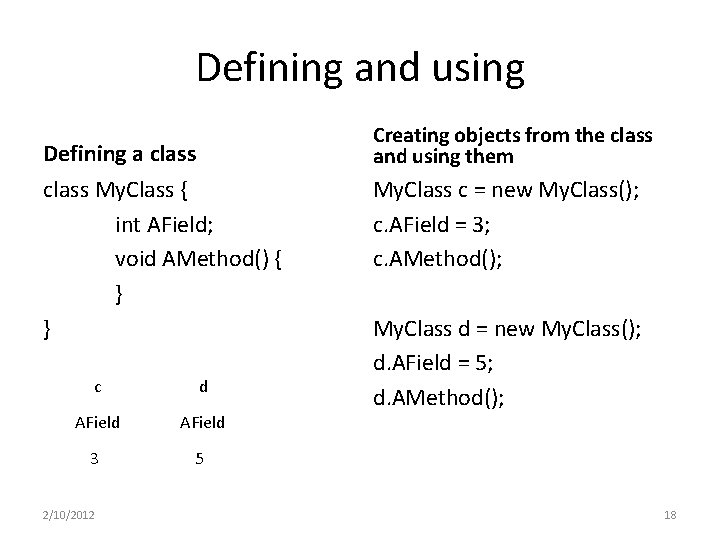
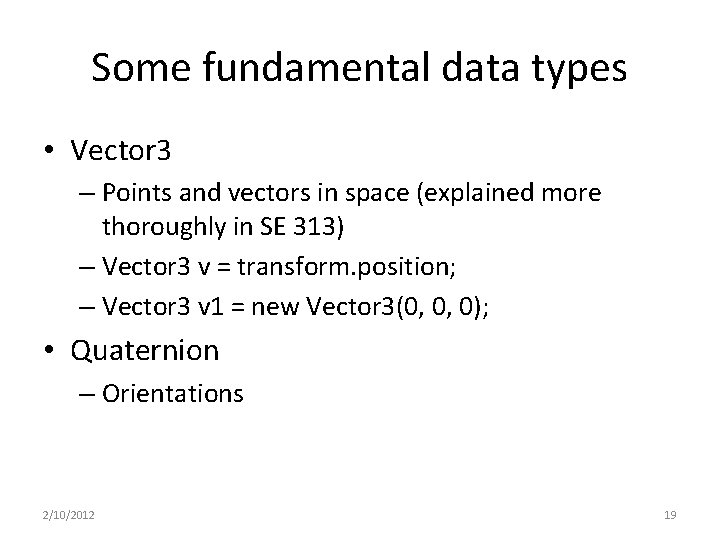
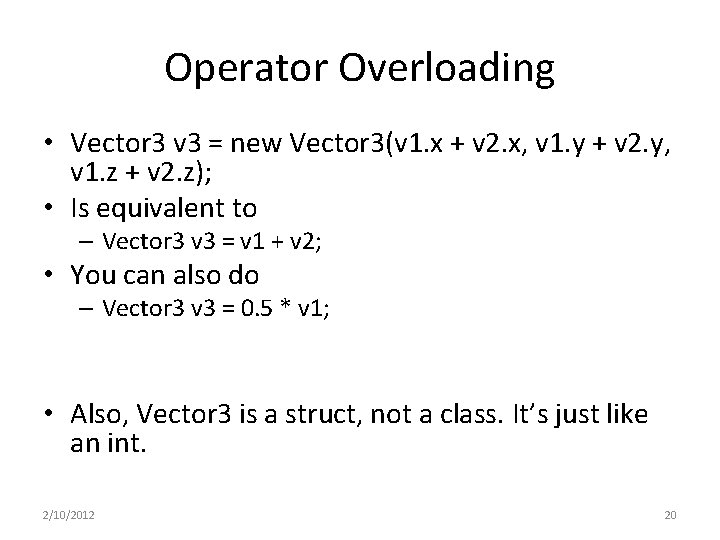
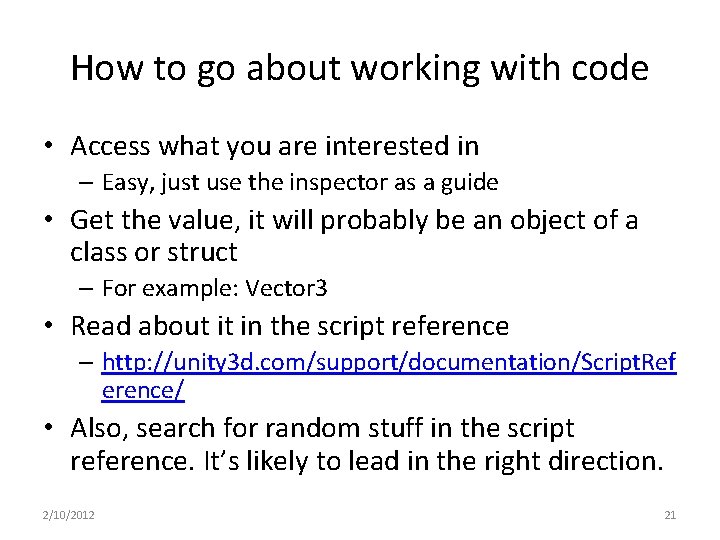
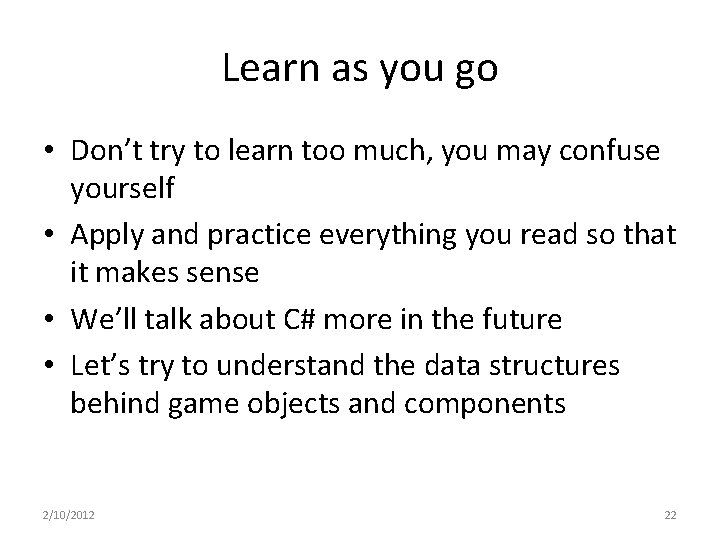
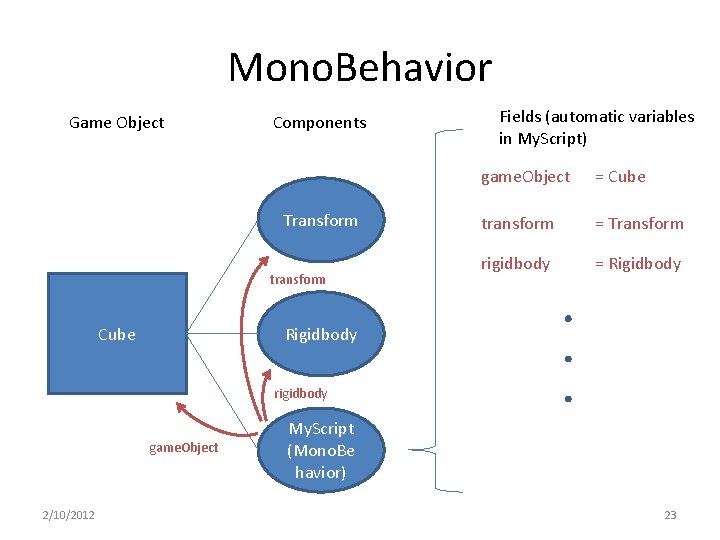
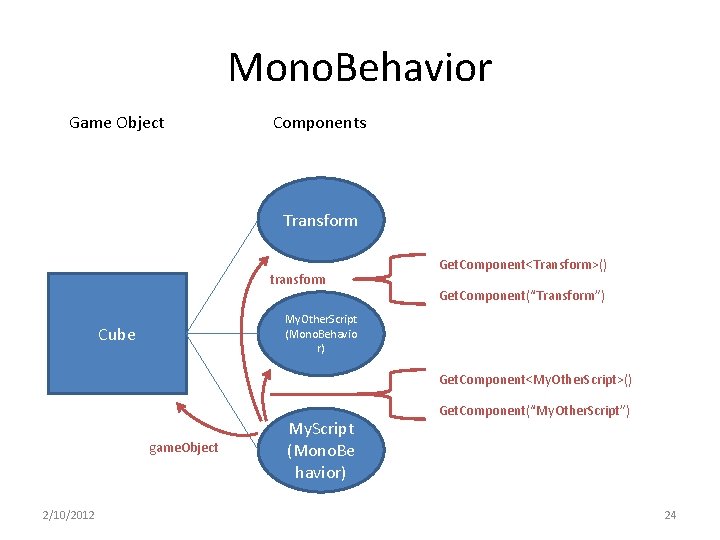
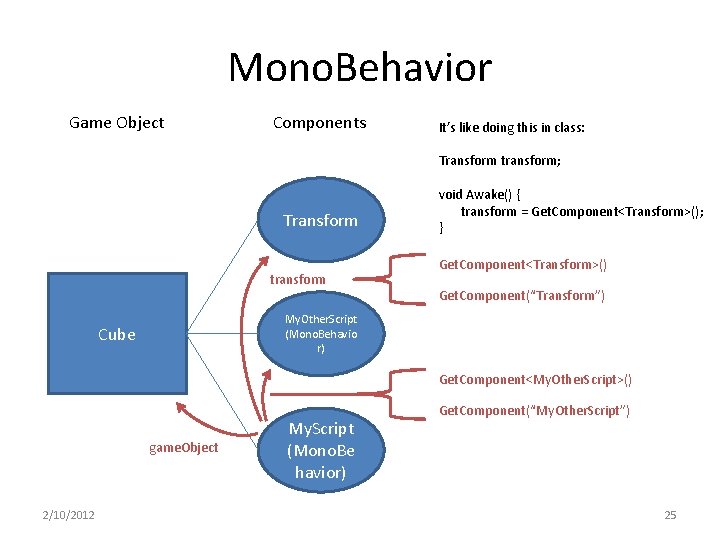
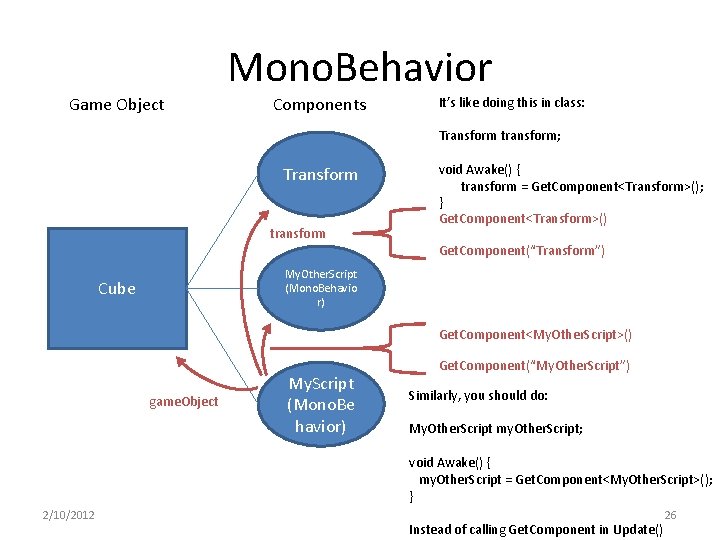
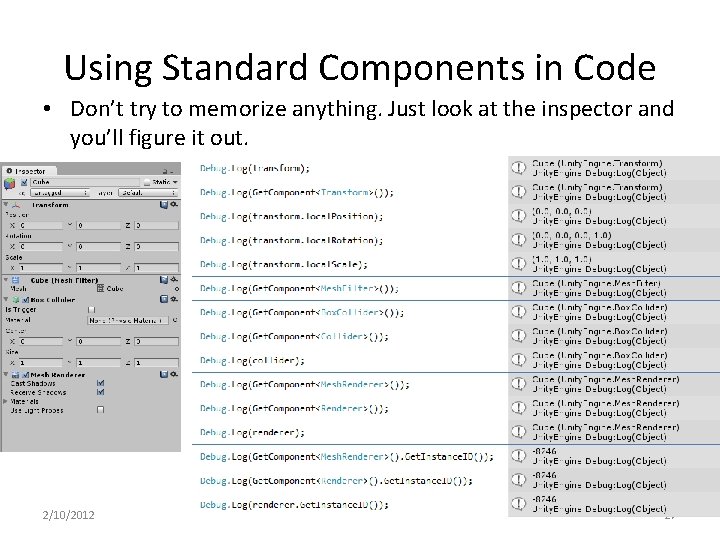
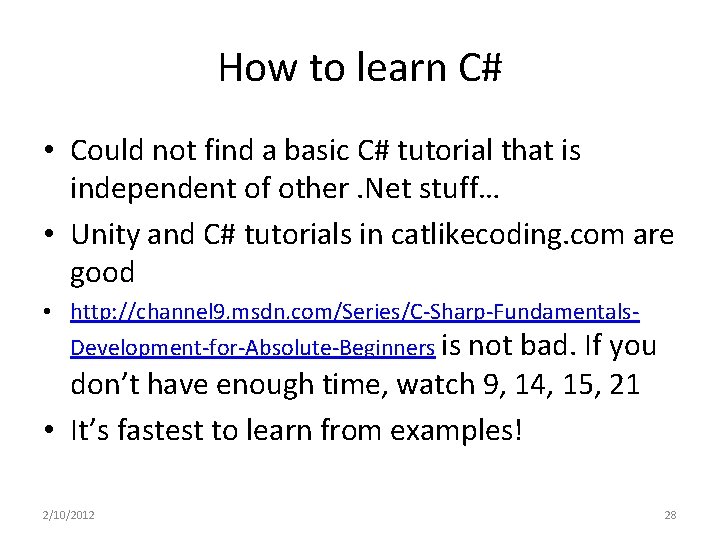
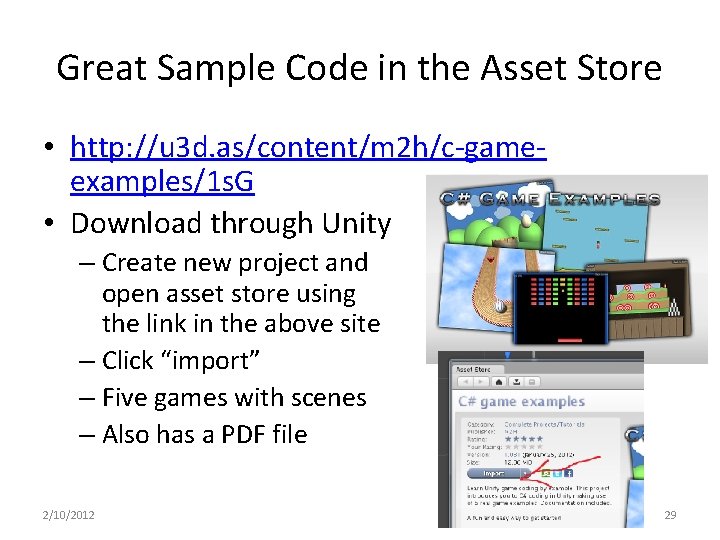
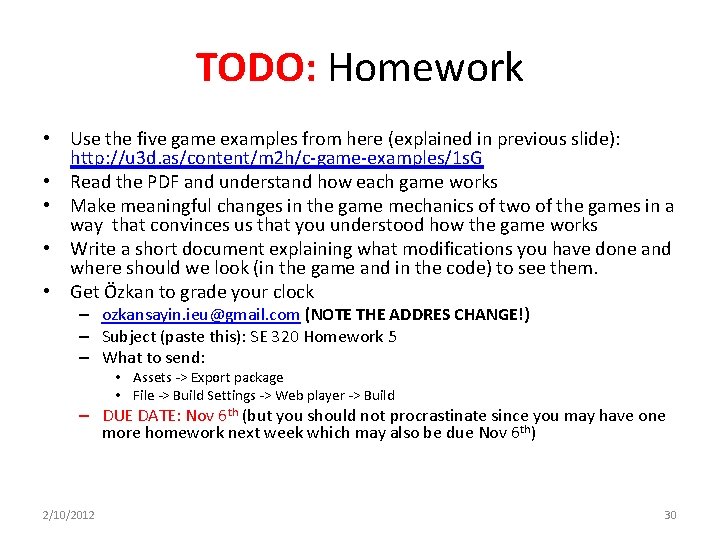
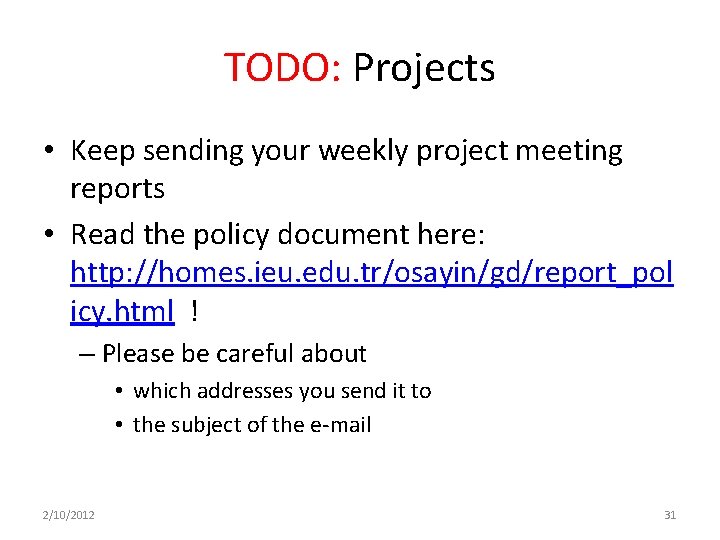
- Slides: 31
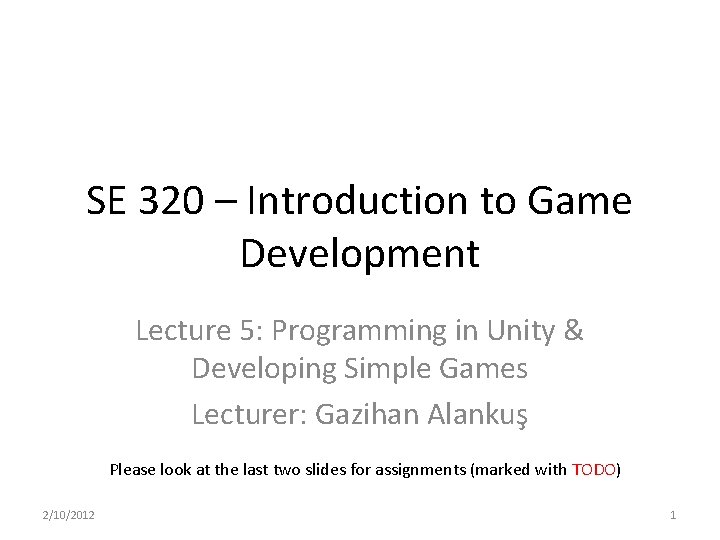
SE 320 – Introduction to Game Development Lecture 5: Programming in Unity & Developing Simple Games Lecturer: Gazihan Alankuş Please look at the last two slides for assignments (marked with TODO) 2/10/2012 1
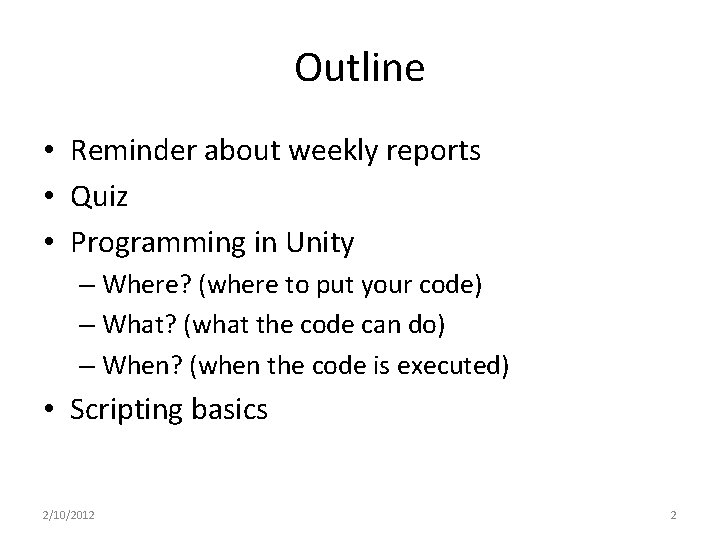
Outline • Reminder about weekly reports • Quiz • Programming in Unity – Where? (where to put your code) – What? (what the code can do) – When? (when the code is executed) • Scripting basics 2/10/2012 2
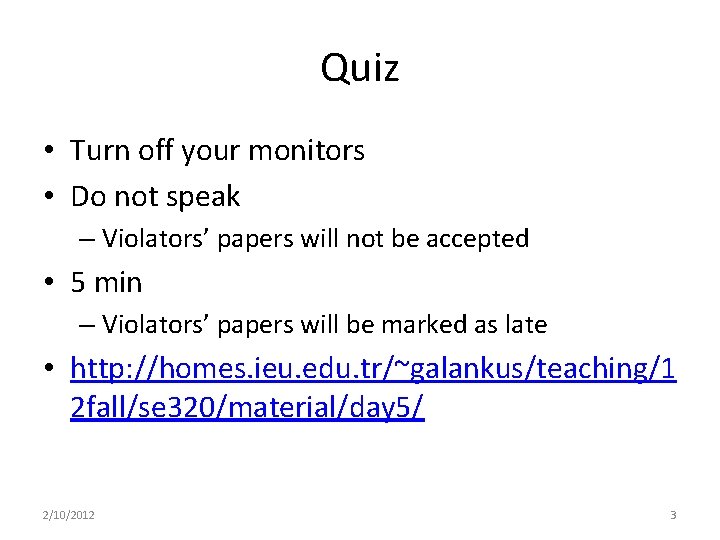
Quiz • Turn off your monitors • Do not speak – Violators’ papers will not be accepted • 5 min – Violators’ papers will be marked as late • http: //homes. ieu. edu. tr/~galankus/teaching/1 2 fall/se 320/material/day 5/ 2/10/2012 3
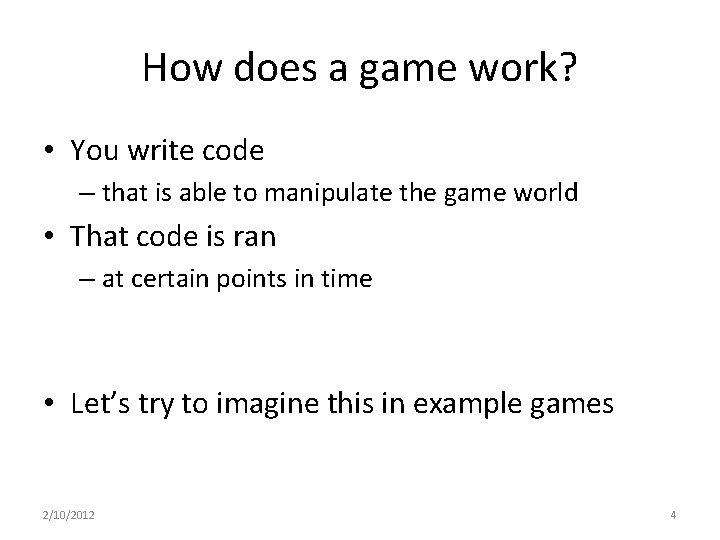
How does a game work? • You write code – that is able to manipulate the game world • That code is ran – at certain points in time • Let’s try to imagine this in example games 2/10/2012 4
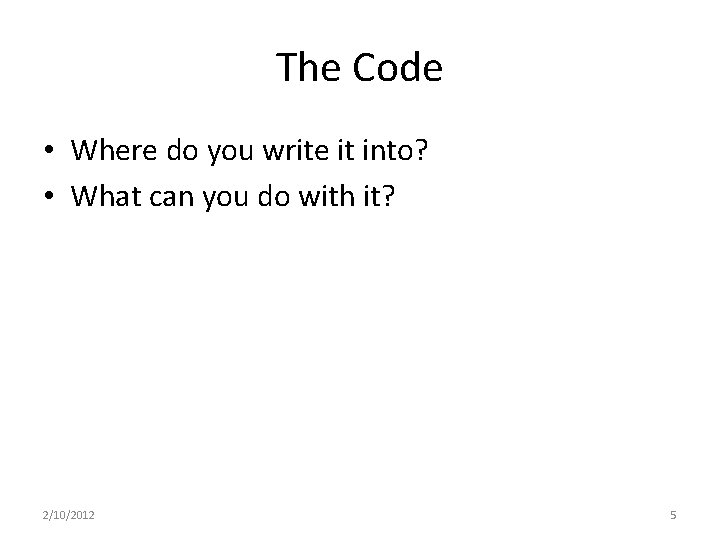
The Code • Where do you write it into? • What can you do with it? 2/10/2012 5
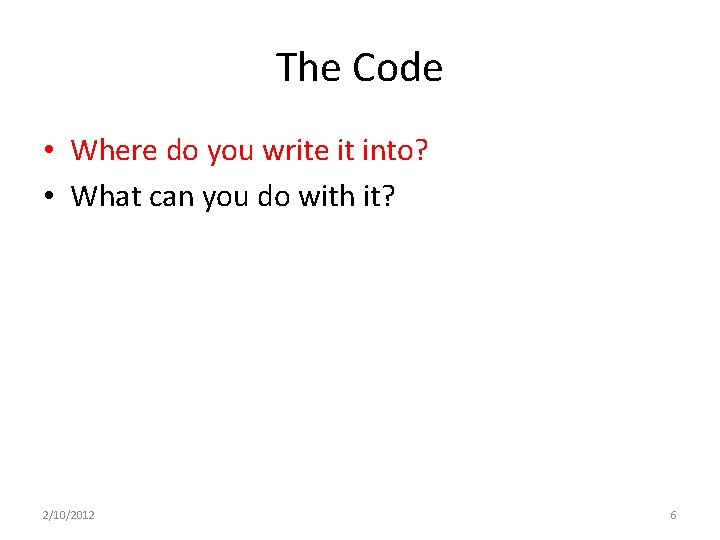
The Code • Where do you write it into? • What can you do with it? 2/10/2012 6
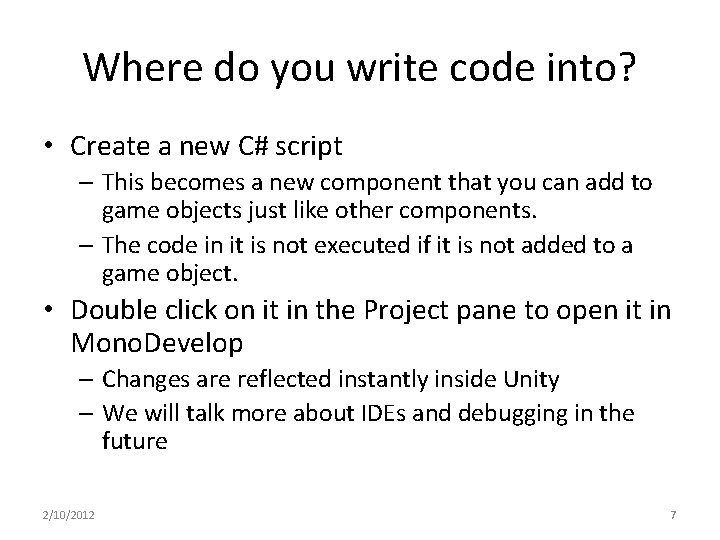
Where do you write code into? • Create a new C# script – This becomes a new component that you can add to game objects just like other components. – The code in it is not executed if it is not added to a game object. • Double click on it in the Project pane to open it in Mono. Develop – Changes are reflected instantly inside Unity – We will talk more about IDEs and debugging in the future 2/10/2012 7
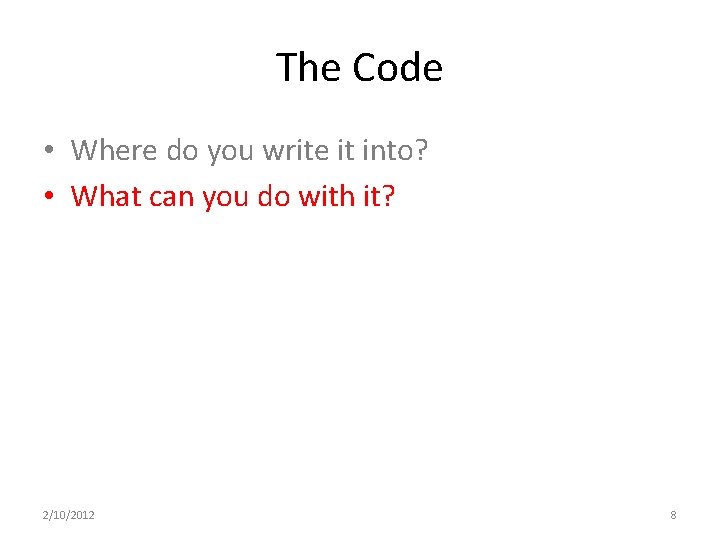
The Code • Where do you write it into? • What can you do with it? 2/10/2012 8
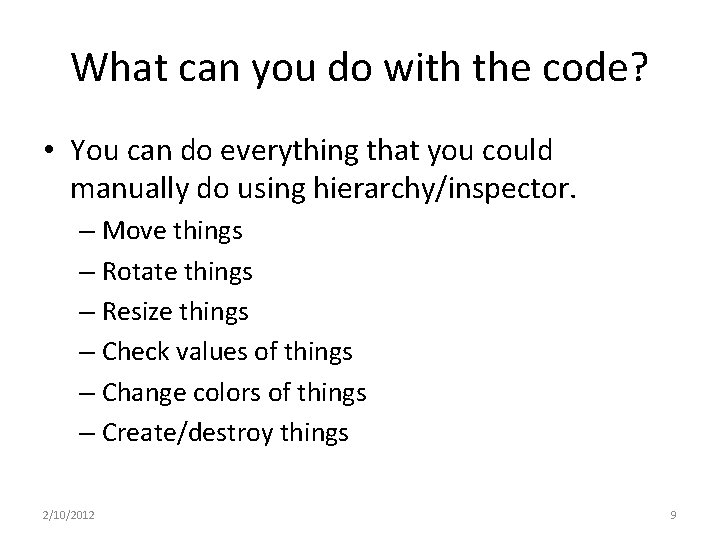
What can you do with the code? • You can do everything that you could manually do using hierarchy/inspector. – Move things – Rotate things – Resize things – Check values of things – Change colors of things – Create/destroy things 2/10/2012 9
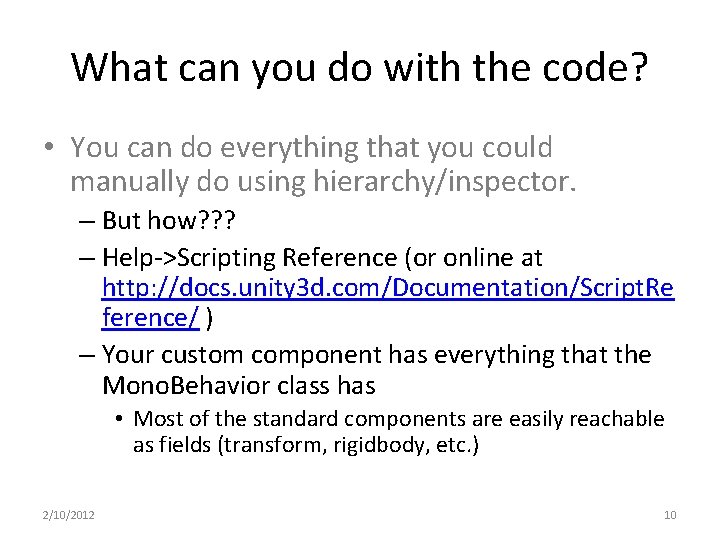
What can you do with the code? • You can do everything that you could manually do using hierarchy/inspector. – But how? ? ? – Help->Scripting Reference (or online at http: //docs. unity 3 d. com/Documentation/Script. Re ference/ ) – Your custom component has everything that the Mono. Behavior class has • Most of the standard components are easily reachable as fields (transform, rigidbody, etc. ) 2/10/2012 10
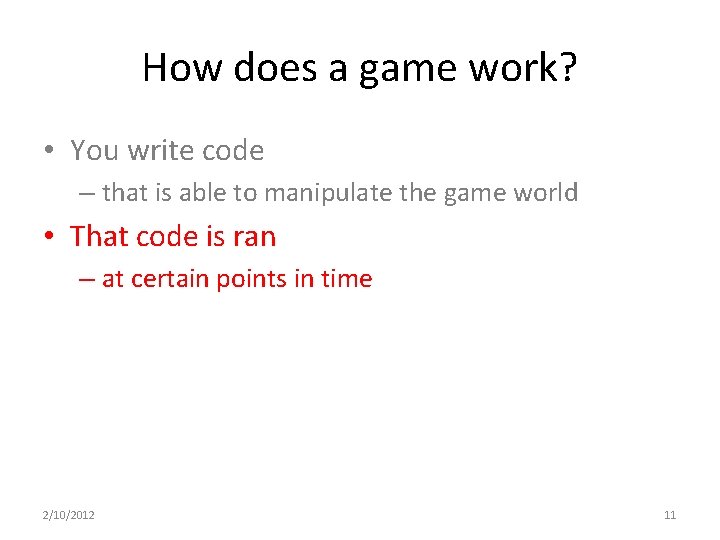
How does a game work? • You write code – that is able to manipulate the game world • That code is ran – at certain points in time 2/10/2012 11
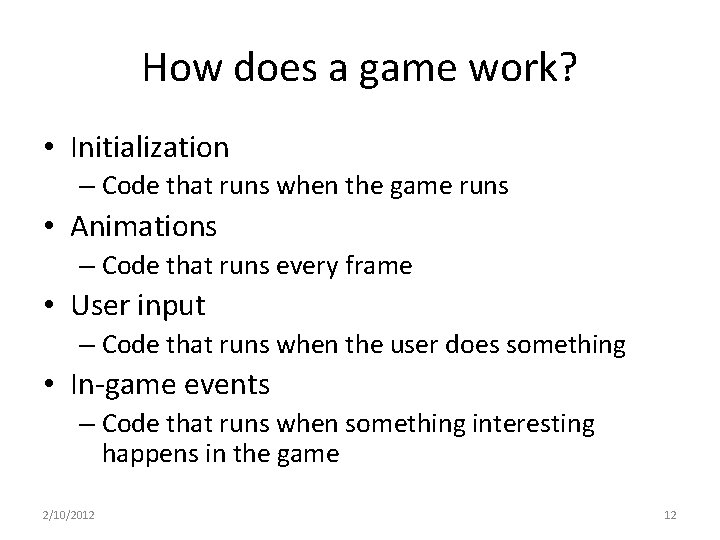
How does a game work? • Initialization – Code that runs when the game runs • Animations – Code that runs every frame • User input – Code that runs when the user does something • In-game events – Code that runs when something interesting happens in the game 2/10/2012 12
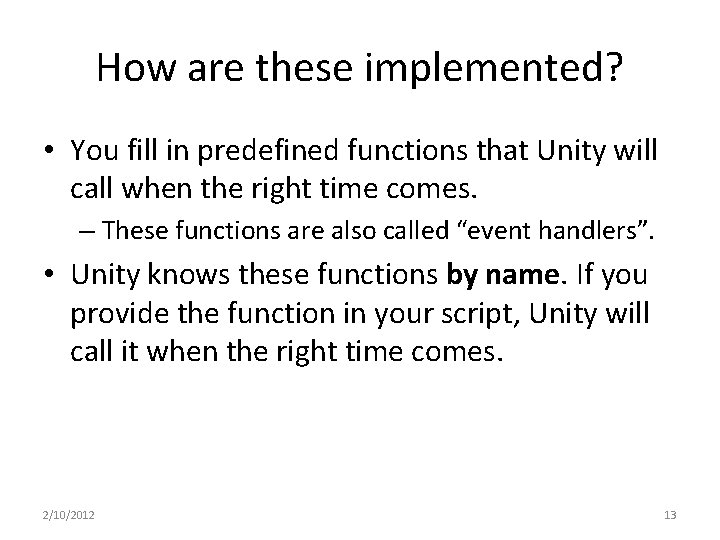
How are these implemented? • You fill in predefined functions that Unity will call when the right time comes. – These functions are also called “event handlers”. • Unity knows these functions by name. If you provide the function in your script, Unity will call it when the right time comes. 2/10/2012 13
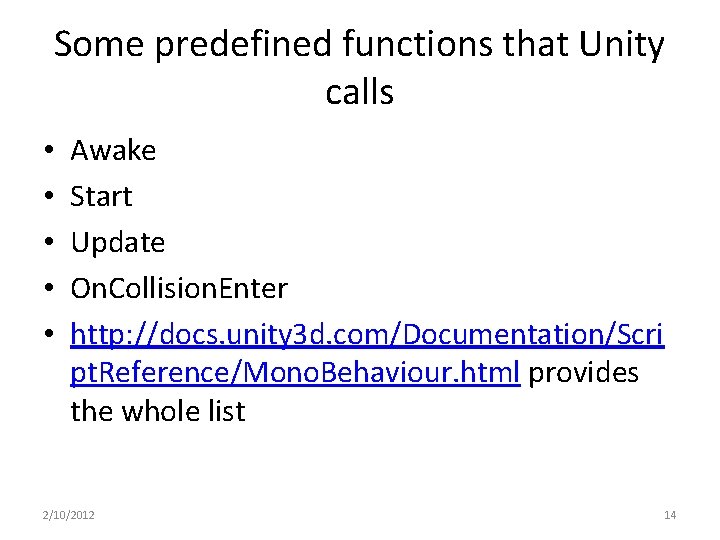
Some predefined functions that Unity calls • • • Awake Start Update On. Collision. Enter http: //docs. unity 3 d. com/Documentation/Scri pt. Reference/Mono. Behaviour. html provides the whole list 2/10/2012 14
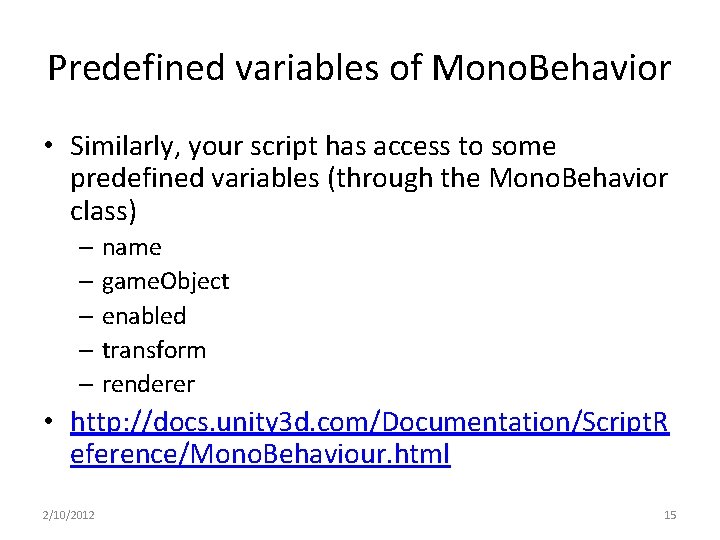
Predefined variables of Mono. Behavior • Similarly, your script has access to some predefined variables (through the Mono. Behavior class) – name – game. Object – enabled – transform – renderer • http: //docs. unity 3 d. com/Documentation/Script. R eference/Mono. Behaviour. html 2/10/2012 15
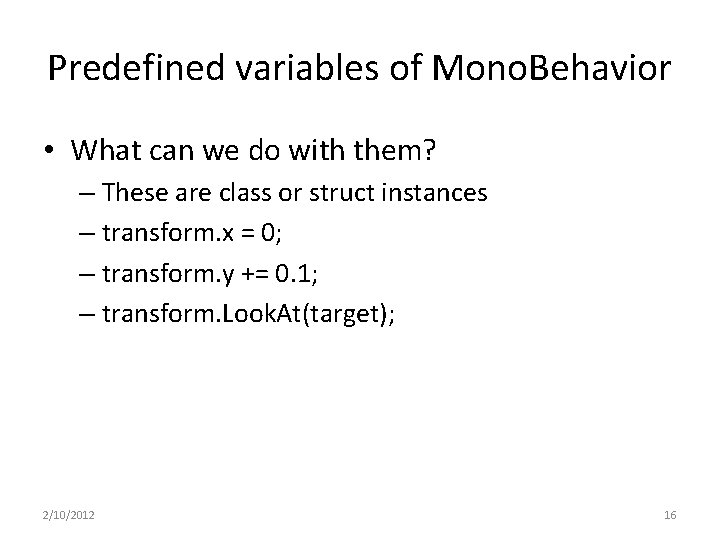
Predefined variables of Mono. Behavior • What can we do with them? – These are class or struct instances – transform. x = 0; – transform. y += 0. 1; – transform. Look. At(target); 2/10/2012 16
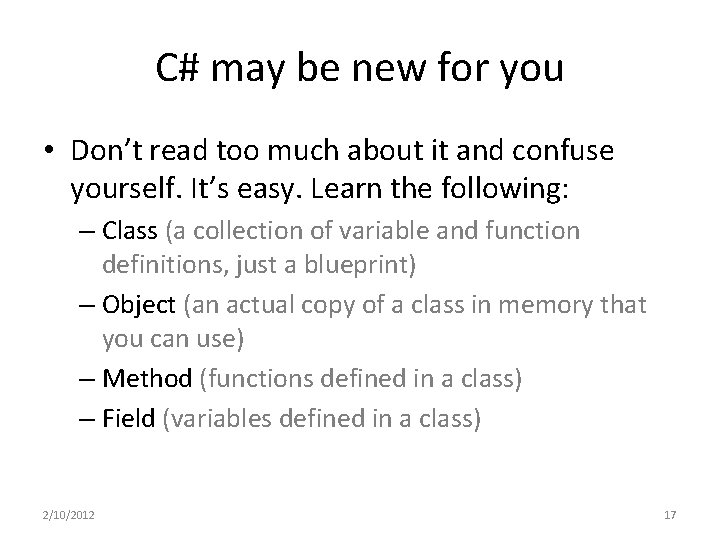
C# may be new for you • Don’t read too much about it and confuse yourself. It’s easy. Learn the following: – Class (a collection of variable and function definitions, just a blueprint) – Object (an actual copy of a class in memory that you can use) – Method (functions defined in a class) – Field (variables defined in a class) 2/10/2012 17
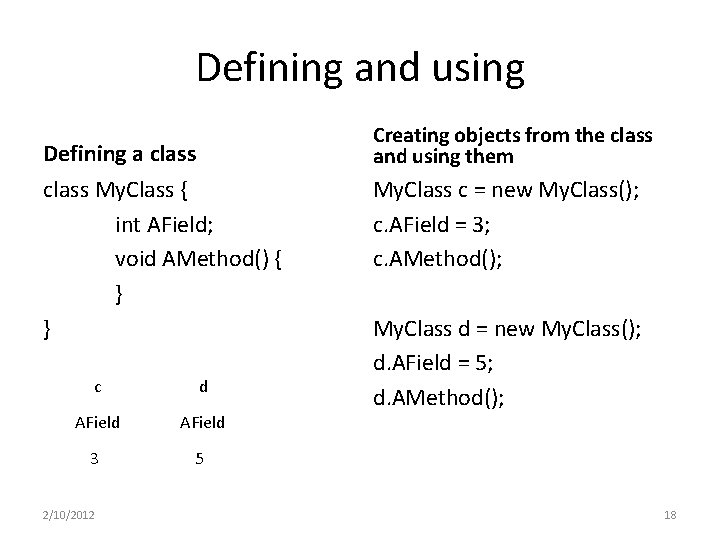
Defining and using Creating objects from the class and using them Defining a class My. Class { int AField; void AMethod() { } } c d AField 3 5 2/10/2012 My. Class c = new My. Class(); c. AField = 3; c. AMethod(); My. Class d = new My. Class(); d. AField = 5; d. AMethod(); 18
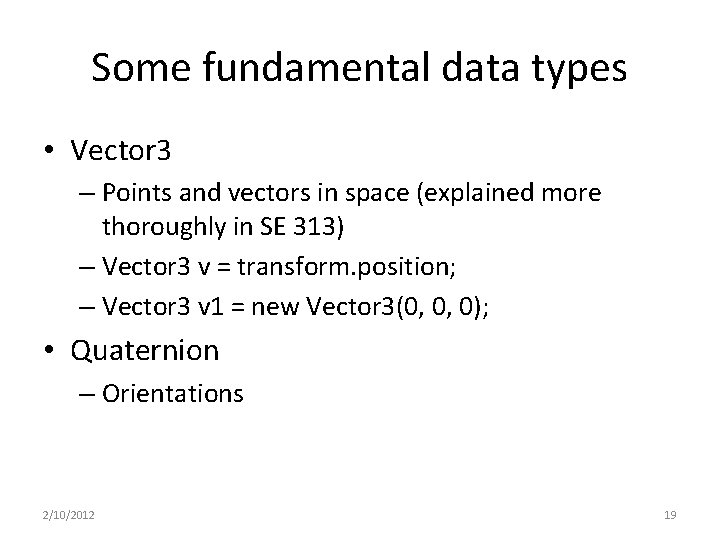
Some fundamental data types • Vector 3 – Points and vectors in space (explained more thoroughly in SE 313) – Vector 3 v = transform. position; – Vector 3 v 1 = new Vector 3(0, 0, 0); • Quaternion – Orientations 2/10/2012 19
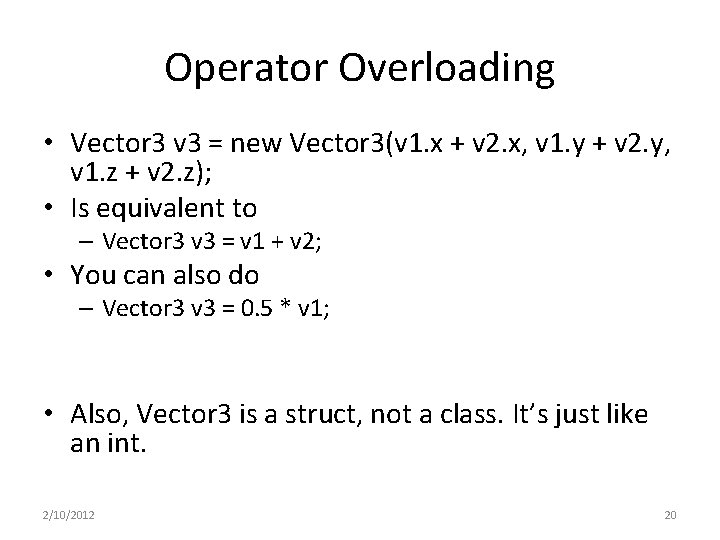
Operator Overloading • Vector 3 v 3 = new Vector 3(v 1. x + v 2. x, v 1. y + v 2. y, v 1. z + v 2. z); • Is equivalent to – Vector 3 v 3 = v 1 + v 2; • You can also do – Vector 3 v 3 = 0. 5 * v 1; • Also, Vector 3 is a struct, not a class. It’s just like an int. 2/10/2012 20
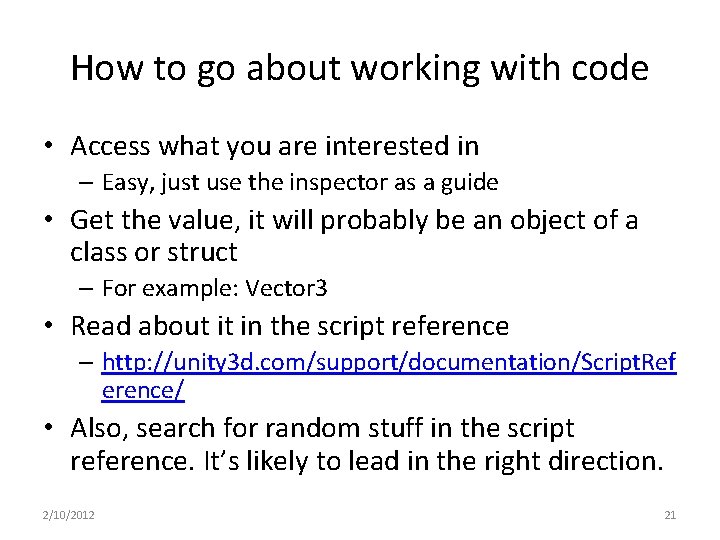
How to go about working with code • Access what you are interested in – Easy, just use the inspector as a guide • Get the value, it will probably be an object of a class or struct – For example: Vector 3 • Read about it in the script reference – http: //unity 3 d. com/support/documentation/Script. Ref erence/ • Also, search for random stuff in the script reference. It’s likely to lead in the right direction. 2/10/2012 21
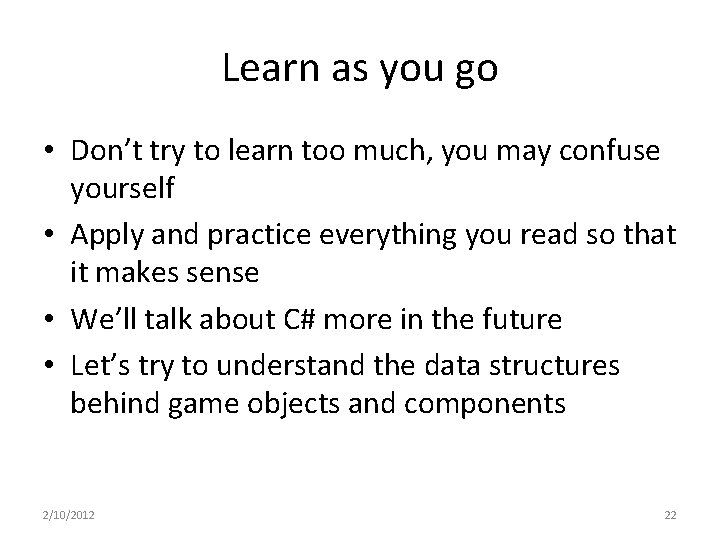
Learn as you go • Don’t try to learn too much, you may confuse yourself • Apply and practice everything you read so that it makes sense • We’ll talk about C# more in the future • Let’s try to understand the data structures behind game objects and components 2/10/2012 22
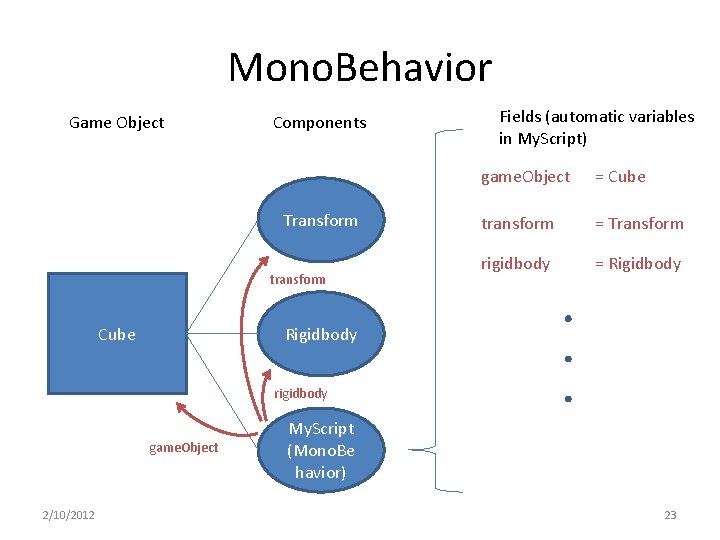
Mono. Behavior Game Object Components Transform transform Fields (automatic variables in My. Script) game. Object = Cube transform = Transform rigidbody = Rigidbody Cube rigidbody game. Object 2/10/2012 My. Script (Mono. Be havior) 23
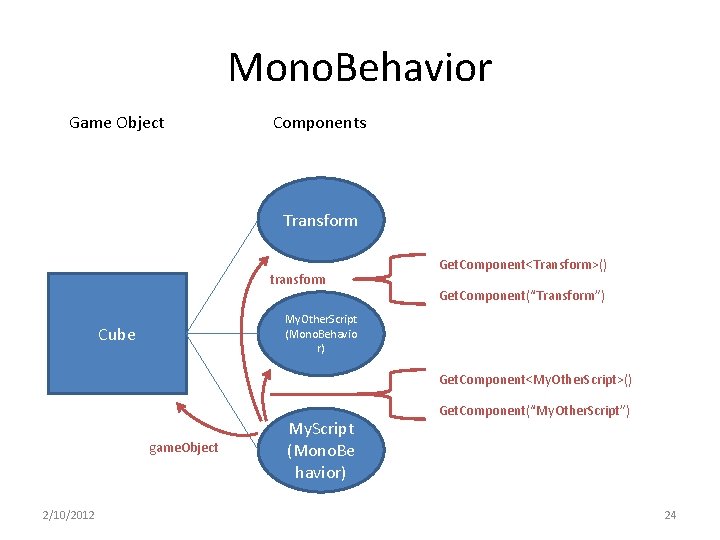
Mono. Behavior Game Object Components Transform transform Get. Component<Transform>() Get. Component(“Transform”) My. Other. Script (Mono. Behavio r) Cube Get. Component<My. Other. Script>() game. Object 2/10/2012 My. Script (Mono. Be havior) Get. Component(“My. Other. Script”) 24
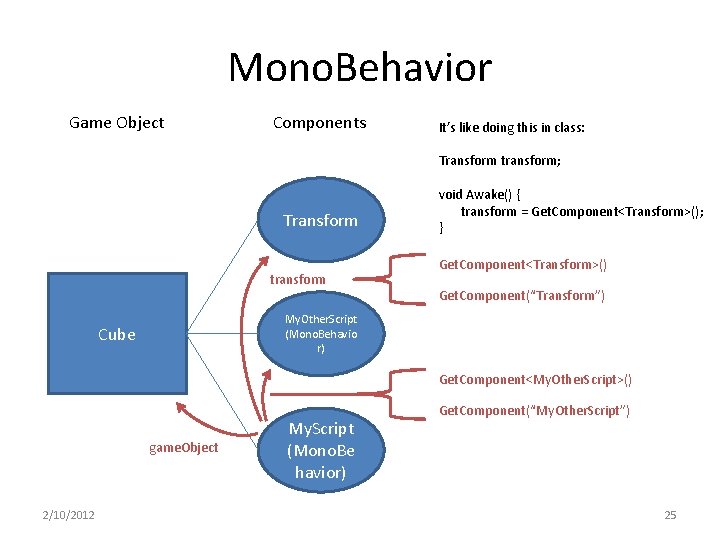
Mono. Behavior Game Object Components It’s like doing this in class: Transform transform; Transform transform void Awake() { transform = Get. Component<Transform>(); } Get. Component<Transform>() Get. Component(“Transform”) My. Other. Script (Mono. Behavio r) Cube Get. Component<My. Other. Script>() game. Object 2/10/2012 My. Script (Mono. Be havior) Get. Component(“My. Other. Script”) 25
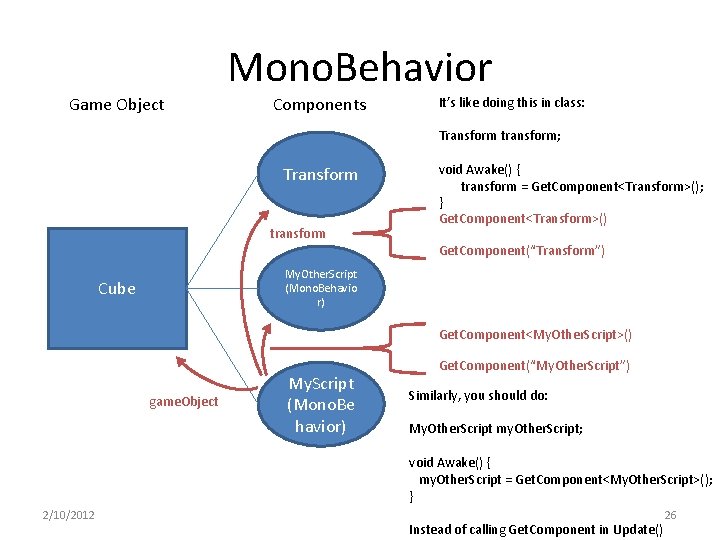
Mono. Behavior Game Object Components It’s like doing this in class: Transform transform; Transform transform void Awake() { transform = Get. Component<Transform>(); } Get. Component<Transform>() Get. Component(“Transform”) My. Other. Script (Mono. Behavio r) Cube Get. Component<My. Other. Script>() game. Object My. Script (Mono. Be havior) Get. Component(“My. Other. Script”) Similarly, you should do: My. Other. Script my. Other. Script; void Awake() { my. Other. Script = Get. Component<My. Other. Script>(); } 2/10/2012 Instead of calling Get. Component in Update() 26
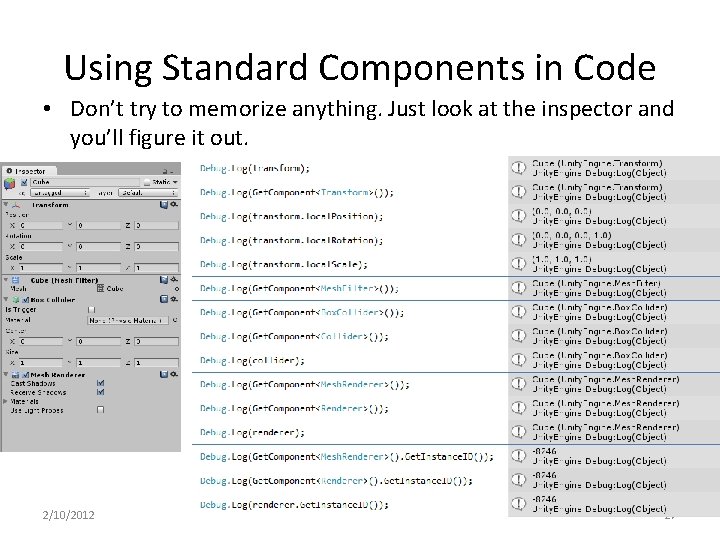
Using Standard Components in Code • Don’t try to memorize anything. Just look at the inspector and you’ll figure it out. 2/10/2012 27
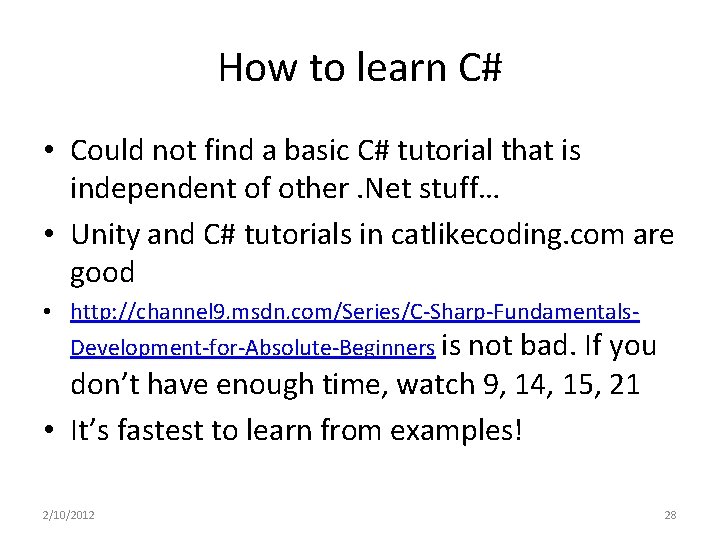
How to learn C# • Could not find a basic C# tutorial that is independent of other. Net stuff… • Unity and C# tutorials in catlikecoding. com are good • http: //channel 9. msdn. com/Series/C-Sharp-Fundamentals. Development-for-Absolute-Beginners is not bad. If you don’t have enough time, watch 9, 14, 15, 21 • It’s fastest to learn from examples! 2/10/2012 28
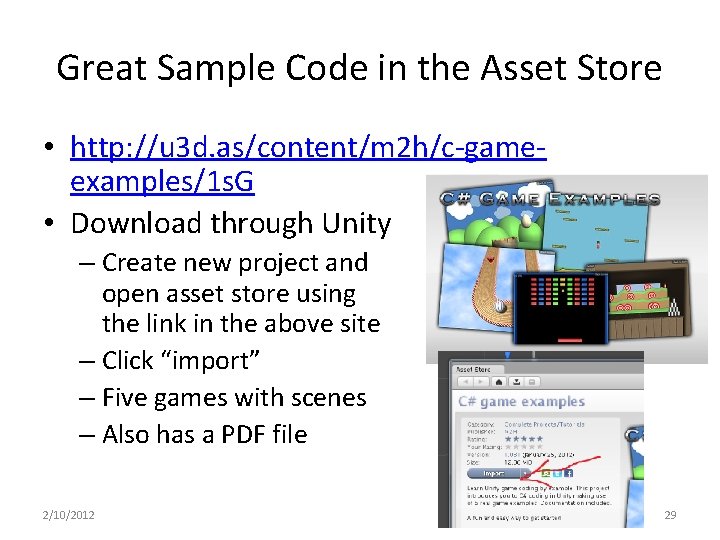
Great Sample Code in the Asset Store • http: //u 3 d. as/content/m 2 h/c-gameexamples/1 s. G • Download through Unity – Create new project and open asset store using the link in the above site – Click “import” – Five games with scenes – Also has a PDF file 2/10/2012 29
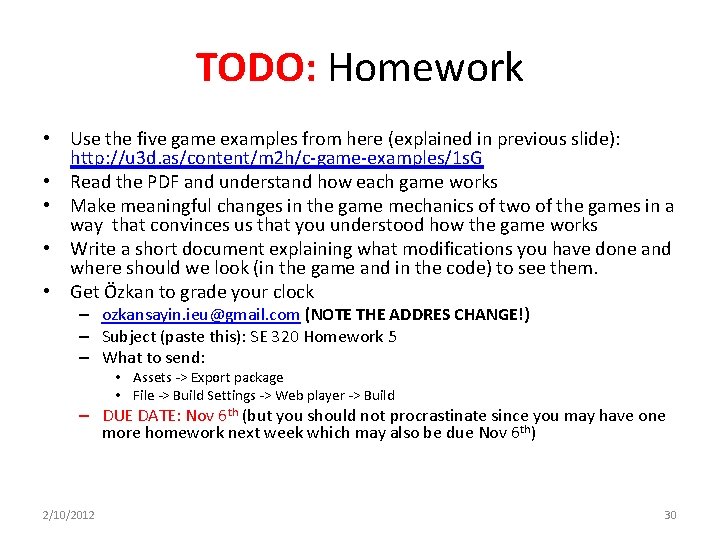
TODO: Homework • Use the five game examples from here (explained in previous slide): http: //u 3 d. as/content/m 2 h/c-game-examples/1 s. G • Read the PDF and understand how each game works • Make meaningful changes in the game mechanics of two of the games in a way that convinces us that you understood how the game works • Write a short document explaining what modifications you have done and where should we look (in the game and in the code) to see them. • Get Özkan to grade your clock – ozkansayin. ieu@gmail. com (NOTE THE ADDRES CHANGE!) – Subject (paste this): SE 320 Homework 5 – What to send: • Assets -> Export package • File -> Build Settings -> Web player -> Build – DUE DATE: Nov 6 th (but you should not procrastinate since you may have one more homework next week which may also be due Nov 6 th) 2/10/2012 30
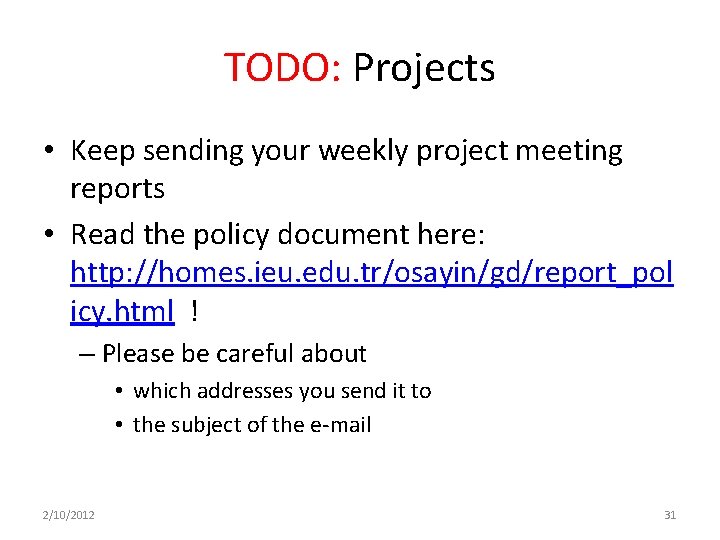
TODO: Projects • Keep sending your weekly project meeting reports • Read the policy document here: http: //homes. ieu. edu. tr/osayin/gd/report_pol icy. html ! – Please be careful about • which addresses you send it to • the subject of the e-mail 2/10/2012 31
01:640:244 lecture notes - lecture 15: plat, idah, farad
Game development essentials: an introduction
Game design lecture
Introduction to biochemistry lecture notes
Introduction to psychology lecture
Introduction to algorithms lecture notes
Pirate game theory
The farm game board game
A formal approach to game design and game research
Game lab game theory
Liar game game theory
Liar game game theory
Pms 320 navy
Notifier 320 manual
Introduccion de la nia 320
Isa 320 materiality
Miaa-320
Dynamixel pinout
Cos 320
The new futura 320 laser printer
The new futura 320 laser printer
Cyranose 320 ebay
Simplifying radicals
How many sig figs in 320
Tms 320
Orea form 320
Cmpt 320
Aae 320
Ubc cpsc 320
Cosc 320
Cos 320°
320 strategy