Resource Allocation Centralized Mutex Algorithm l l l
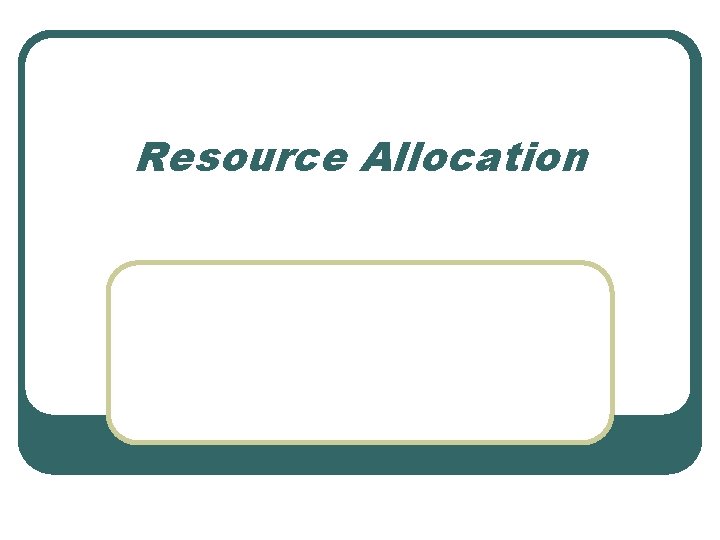
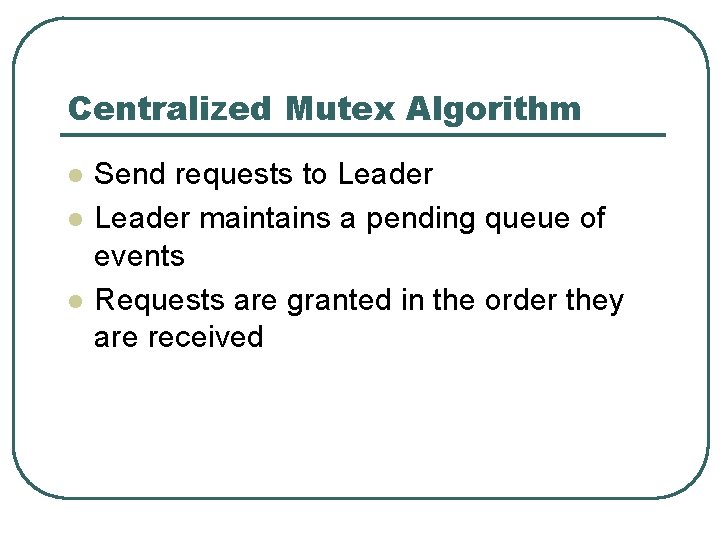
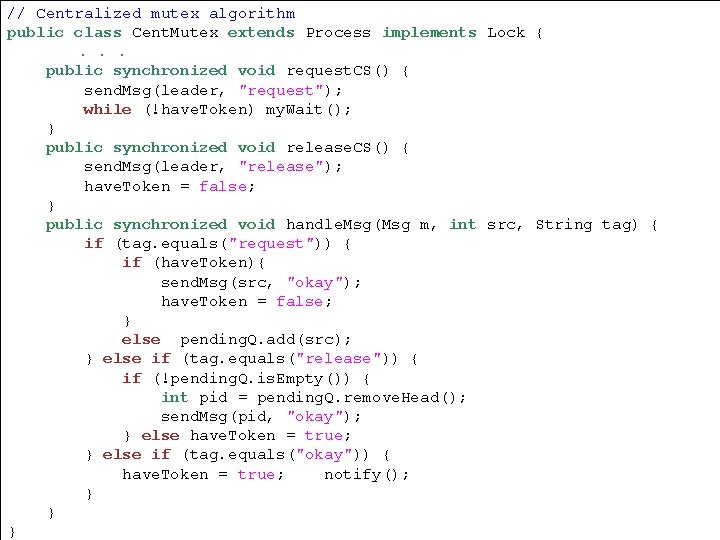
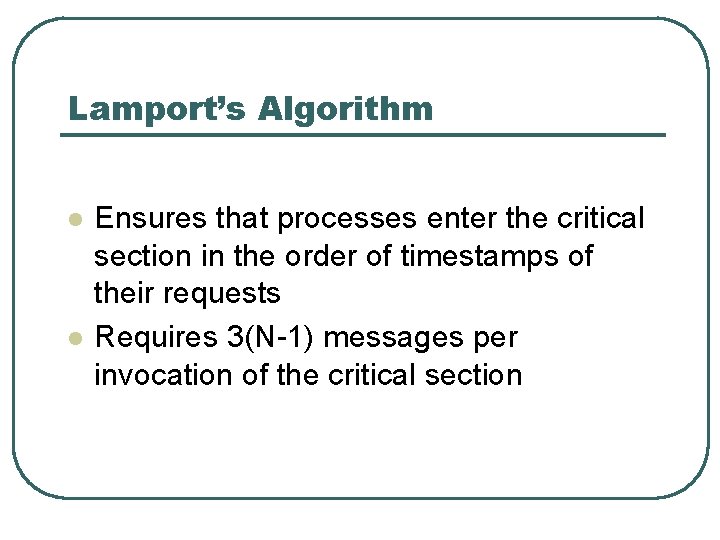
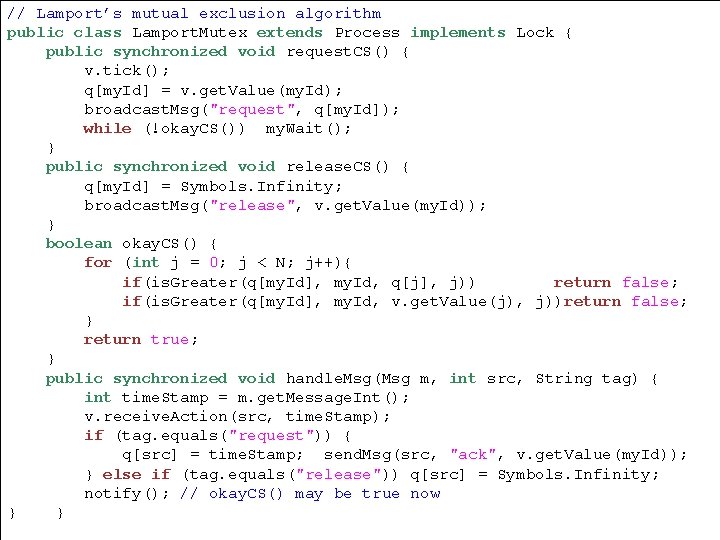
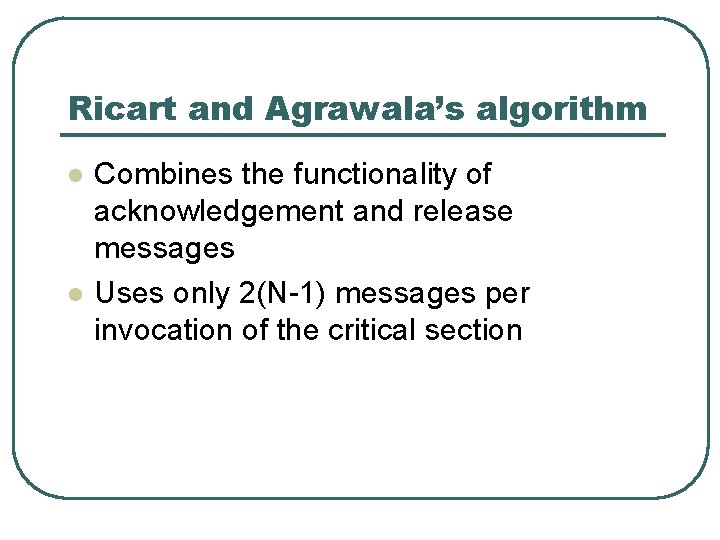
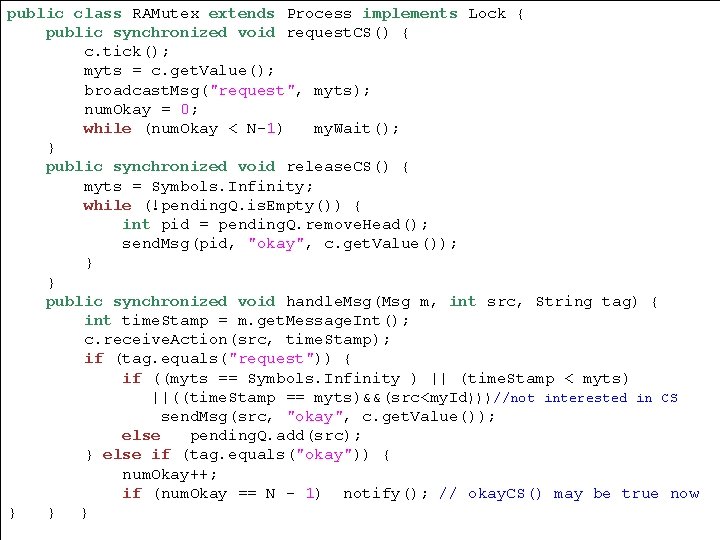
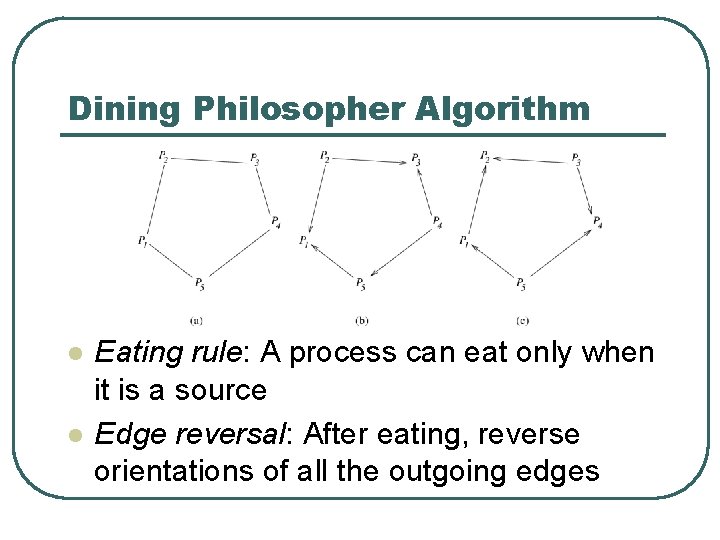
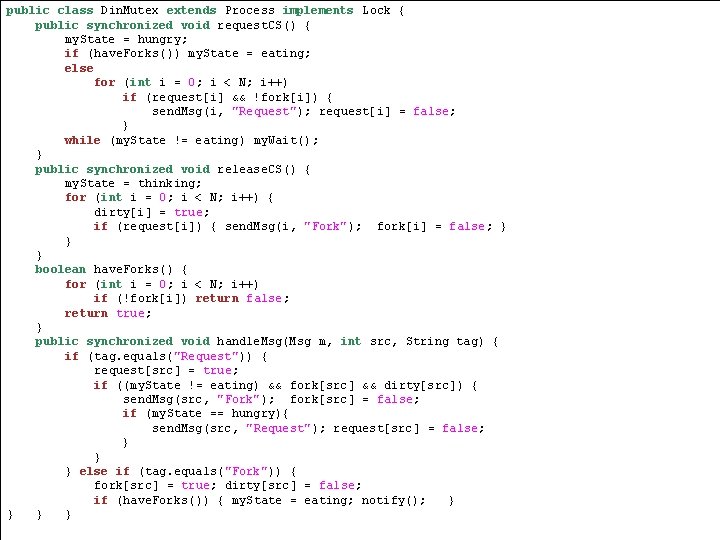
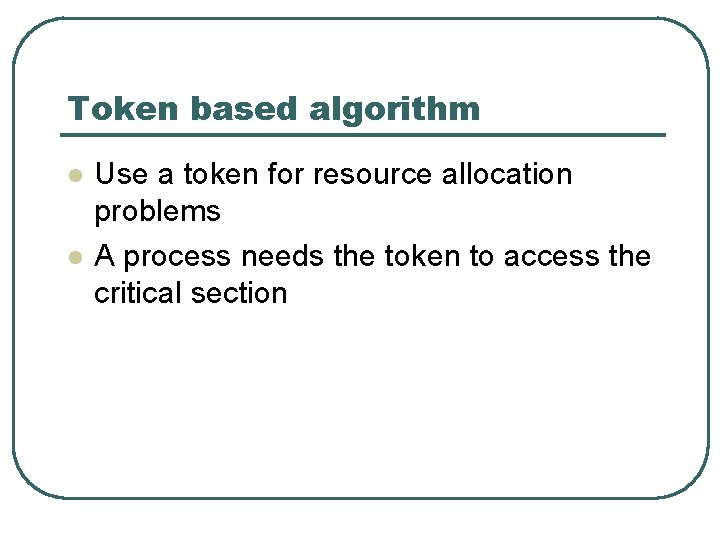
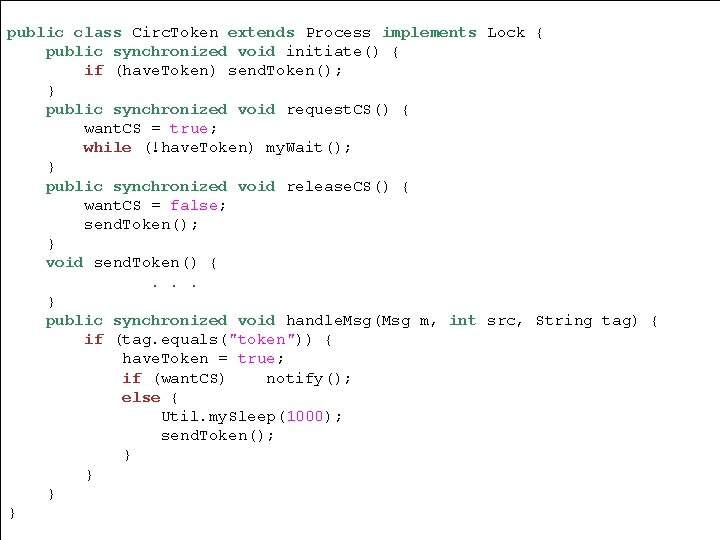
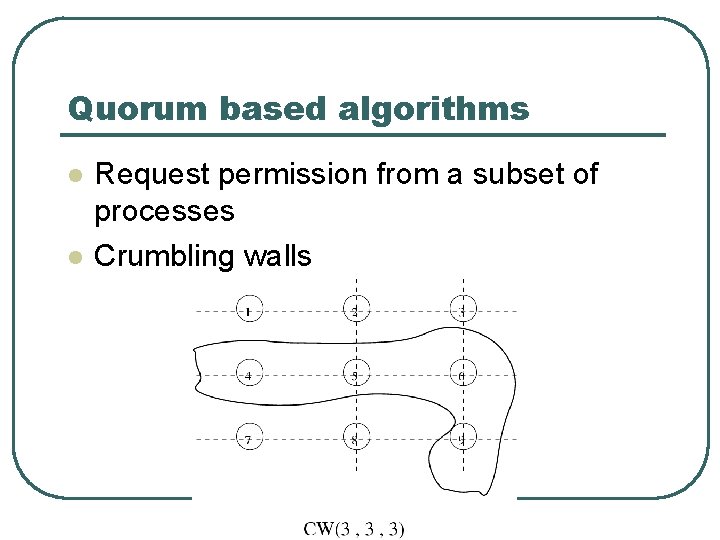
- Slides: 12
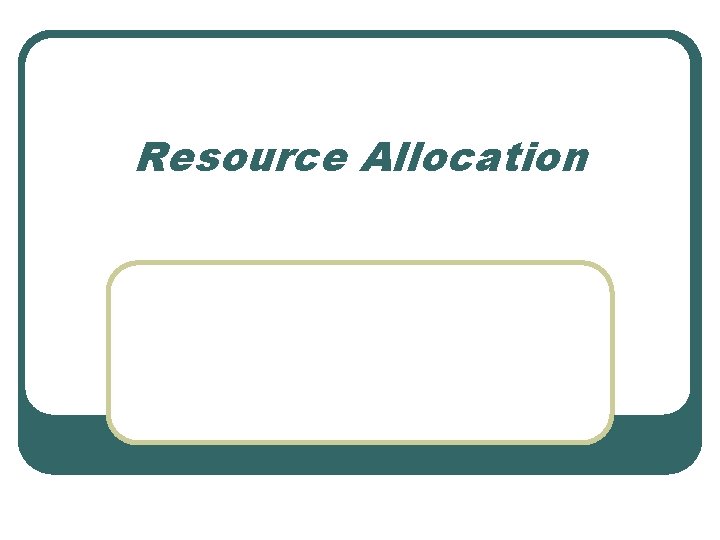
Resource Allocation
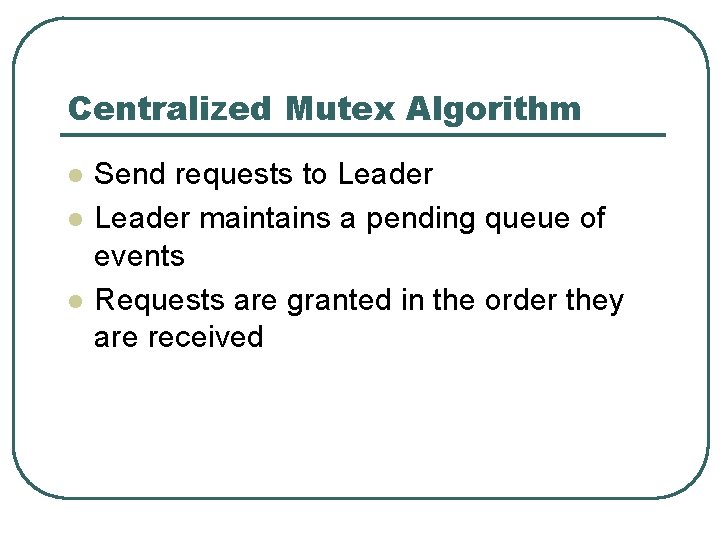
Centralized Mutex Algorithm l l l Send requests to Leader maintains a pending queue of events Requests are granted in the order they are received
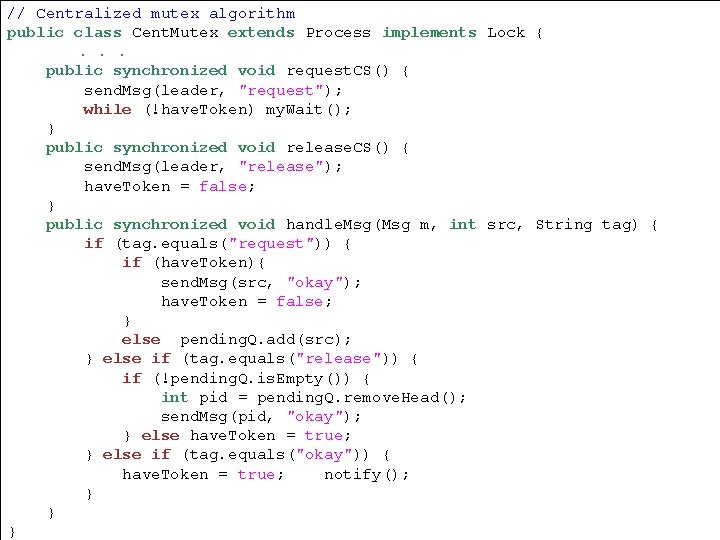
// Centralized mutex algorithm public class Cent. Mutex extends Process implements Lock {. . . public synchronized void request. CS() { send. Msg(leader, "request"); while (!have. Token) my. Wait(); } public synchronized void release. CS() { send. Msg(leader, "release"); have. Token = false; } public synchronized void handle. Msg(Msg m, int src, String tag) { if (tag. equals("request")) { if (have. Token){ send. Msg(src, "okay"); have. Token = false; } else pending. Q. add(src); } else if (tag. equals("release")) { if (!pending. Q. is. Empty()) { int pid = pending. Q. remove. Head(); send. Msg(pid, "okay"); } else have. Token = true; } else if (tag. equals("okay")) { have. Token = true; notify(); } } }
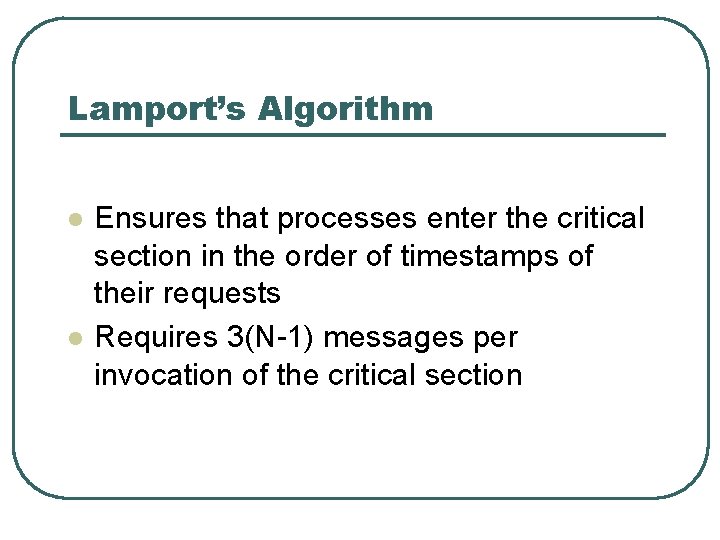
Lamport’s Algorithm l l Ensures that processes enter the critical section in the order of timestamps of their requests Requires 3(N-1) messages per invocation of the critical section
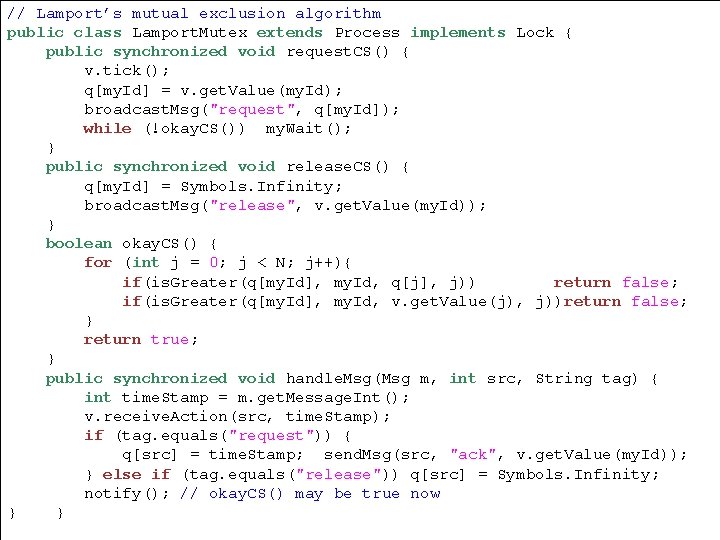
// Lamport’s mutual exclusion algorithm public class Lamport. Mutex extends Process implements Lock { public synchronized void request. CS() { v. tick(); q[my. Id] = v. get. Value(my. Id); broadcast. Msg("request", q[my. Id]); while (!okay. CS()) my. Wait(); } public synchronized void release. CS() { q[my. Id] = Symbols. Infinity; broadcast. Msg("release", v. get. Value(my. Id)); } boolean okay. CS() { for (int j = 0; j < N; j++){ if(is. Greater(q[my. Id], my. Id, q[j], j)) return false; if(is. Greater(q[my. Id], my. Id, v. get. Value(j), j))return false; } return true; } public synchronized void handle. Msg(Msg m, int src, String tag) { int time. Stamp = m. get. Message. Int(); v. receive. Action(src, time. Stamp); if (tag. equals("request")) { q[src] = time. Stamp; send. Msg(src, "ack", v. get. Value(my. Id)); } else if (tag. equals("release")) q[src] = Symbols. Infinity; notify(); // okay. CS() may be true now } }
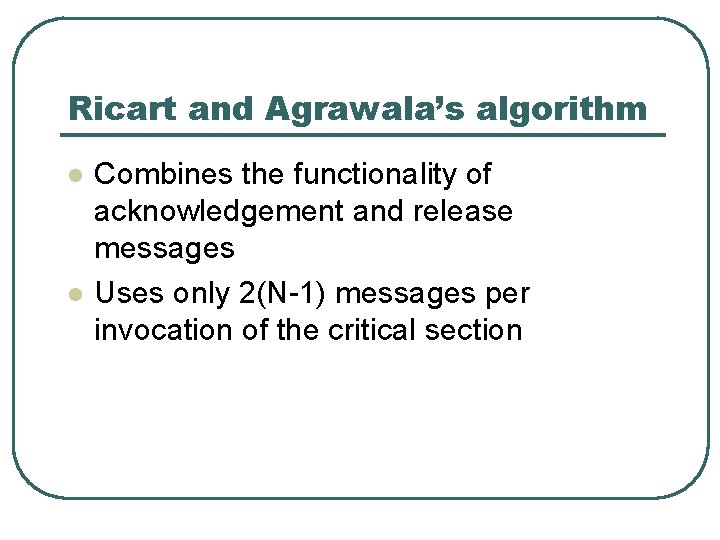
Ricart and Agrawala’s algorithm l l Combines the functionality of acknowledgement and release messages Uses only 2(N-1) messages per invocation of the critical section
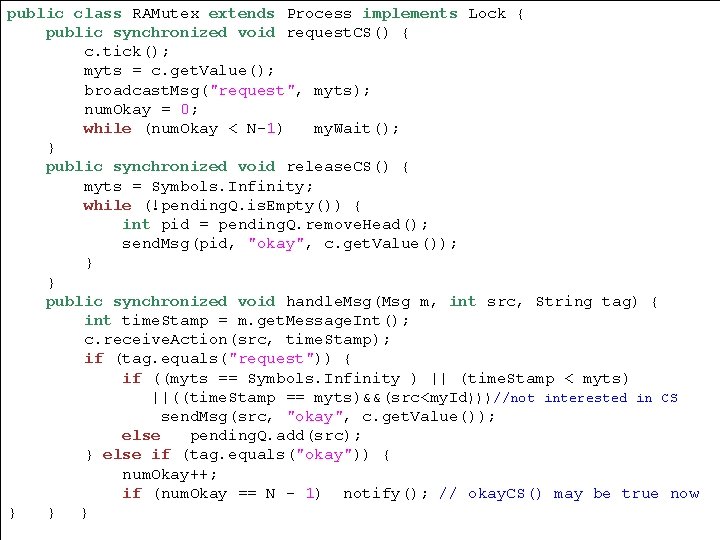
public class RAMutex extends Process implements Lock { public synchronized void request. CS() { c. tick(); myts = c. get. Value(); broadcast. Msg("request", myts); num. Okay = 0; while (num. Okay < N-1) my. Wait(); } public synchronized void release. CS() { myts = Symbols. Infinity; while (!pending. Q. is. Empty()) { int pid = pending. Q. remove. Head(); send. Msg(pid, "okay", c. get. Value()); } } public synchronized void handle. Msg(Msg m, int src, String tag) { int time. Stamp = m. get. Message. Int(); c. receive. Action(src, time. Stamp); if (tag. equals("request")) { if ((myts == Symbols. Infinity ) || (time. Stamp < myts) ||((time. Stamp == myts)&&(src<my. Id)))//not interested in CS send. Msg(src, "okay", c. get. Value()); else pending. Q. add(src); } else if (tag. equals("okay")) { num. Okay++; if (num. Okay == N - 1) notify(); // okay. CS() may be true now } } }
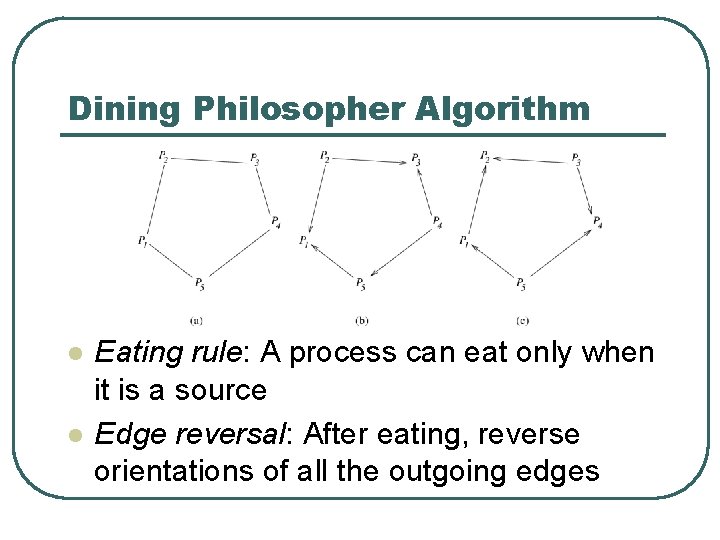
Dining Philosopher Algorithm l l Eating rule: A process can eat only when it is a source Edge reversal: After eating, reverse orientations of all the outgoing edges
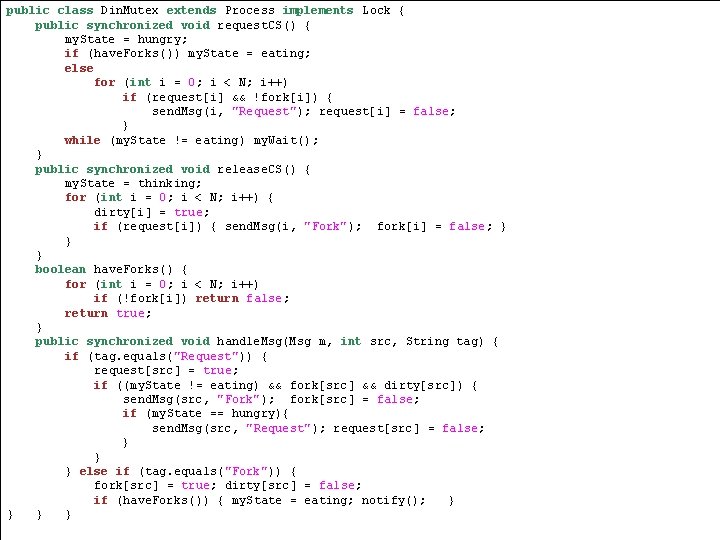
public class Din. Mutex extends Process implements Lock { public synchronized void request. CS() { my. State = hungry; if (have. Forks()) my. State = eating; else for (int i = 0; i < N; i++) if (request[i] && !fork[i]) { send. Msg(i, "Request"); request[i] = false; } while (my. State != eating) my. Wait(); } public synchronized void release. CS() { my. State = thinking; for (int i = 0; i < N; i++) { dirty[i] = true; if (request[i]) { send. Msg(i, "Fork"); fork[i] = false; } } } boolean have. Forks() { for (int i = 0; i < N; i++) if (!fork[i]) return false; return true; } public synchronized void handle. Msg(Msg m, int src, String tag) { if (tag. equals("Request")) { request[src] = true; if ((my. State != eating) && fork[src] && dirty[src]) { send. Msg(src, "Fork"); fork[src] = false; if (my. State == hungry){ send. Msg(src, "Request"); request[src] = false; } } } else if (tag. equals("Fork")) { fork[src] = true; dirty[src] = false; if (have. Forks()) { my. State = eating; notify(); } }
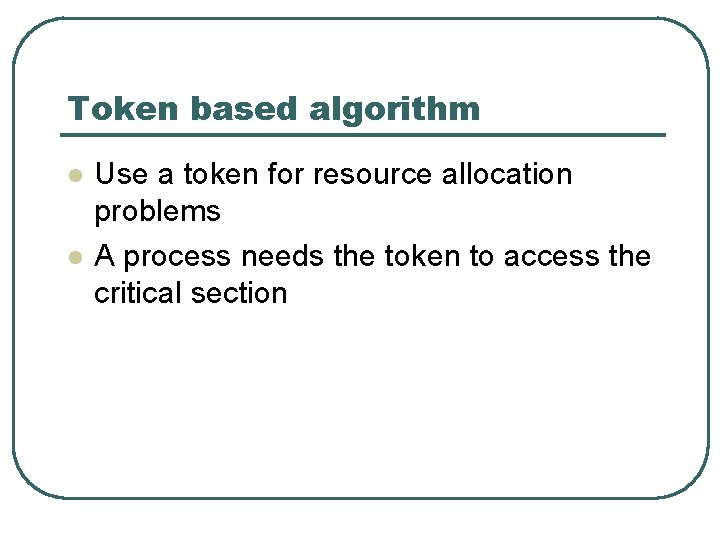
Token based algorithm l l Use a token for resource allocation problems A process needs the token to access the critical section
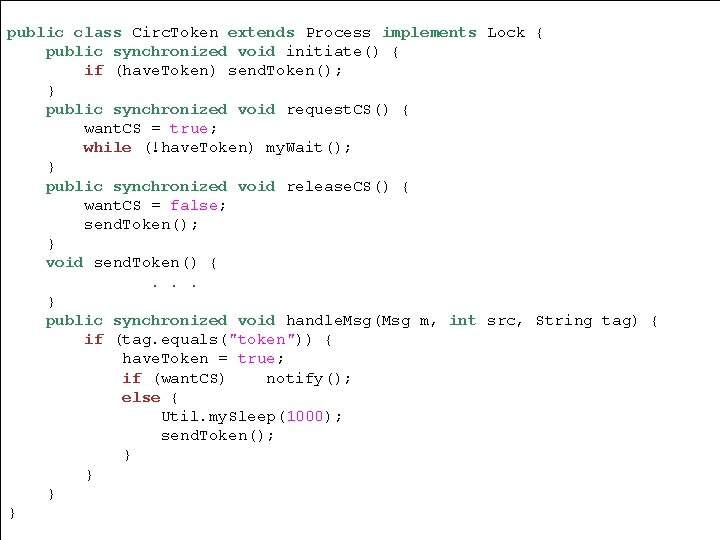
public class Circ. Token extends Process implements Lock { public synchronized void initiate() { if (have. Token) send. Token(); } public synchronized void request. CS() { want. CS = true; while (!have. Token) my. Wait(); } public synchronized void release. CS() { want. CS = false; send. Token(); } void send. Token() {. . . } public synchronized void handle. Msg(Msg m, int src, String tag) { if (tag. equals("token")) { have. Token = true; if (want. CS) notify(); else { Util. my. Sleep(1000); send. Token(); } }
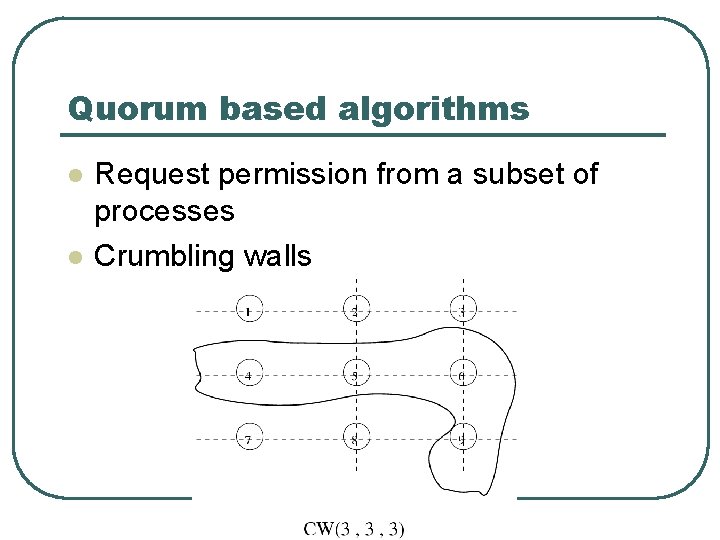
Quorum based algorithms l l Request permission from a subset of processes Crumbling walls