Recursion Recursion is a math and programming tool
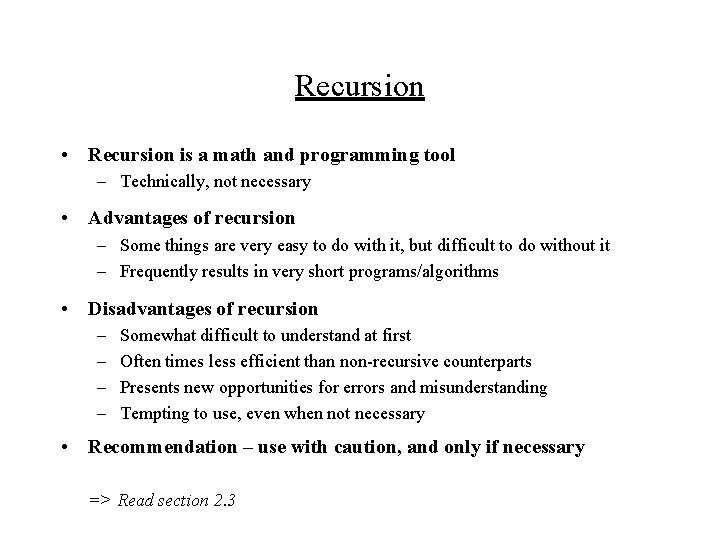
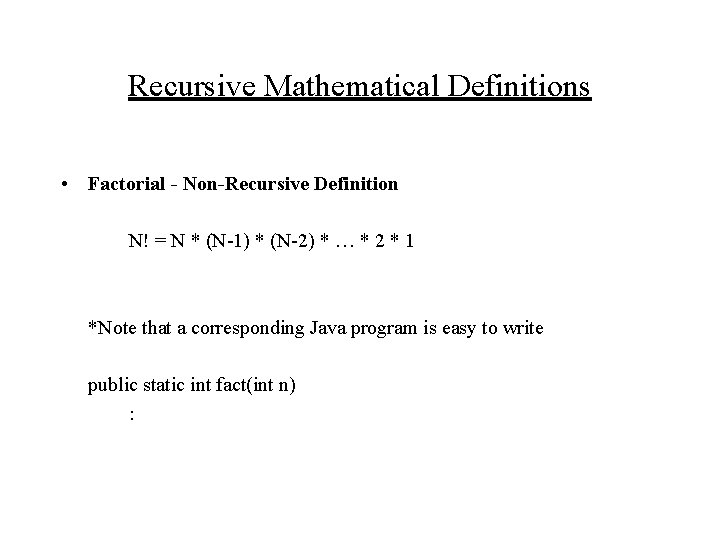
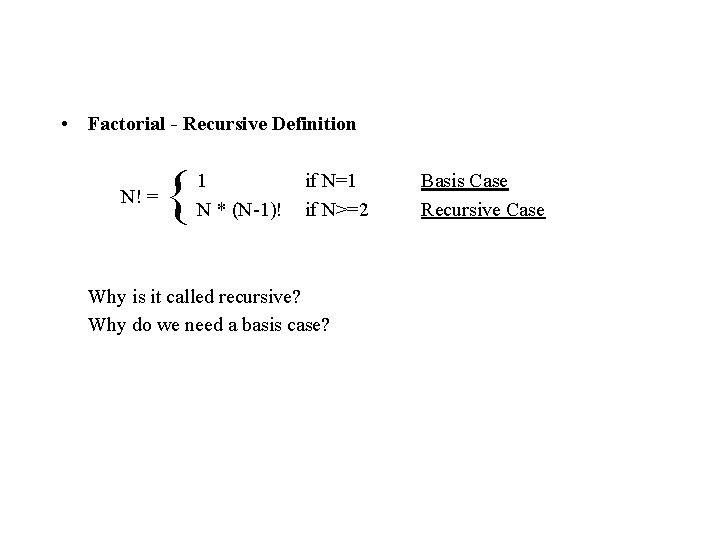
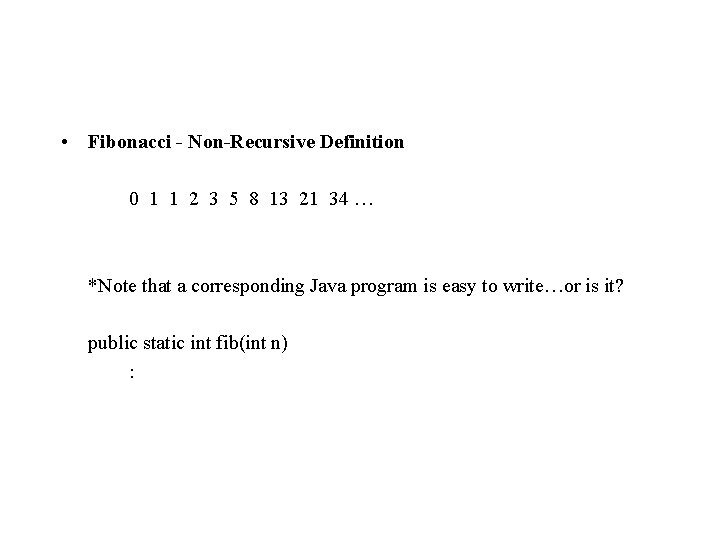
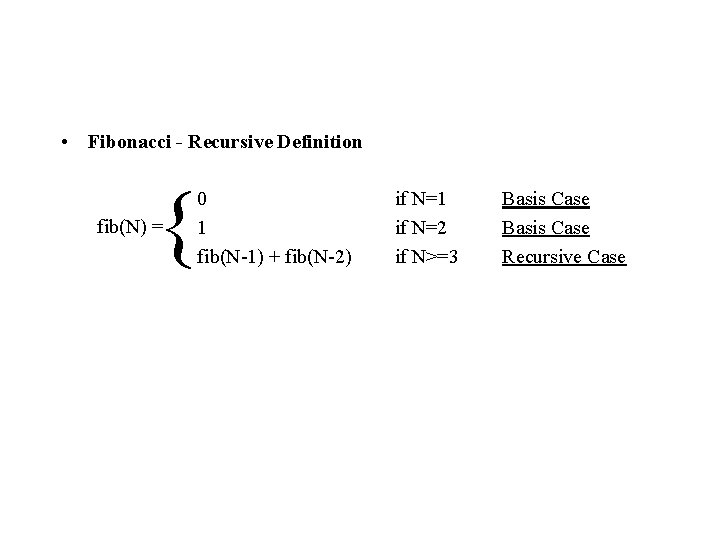
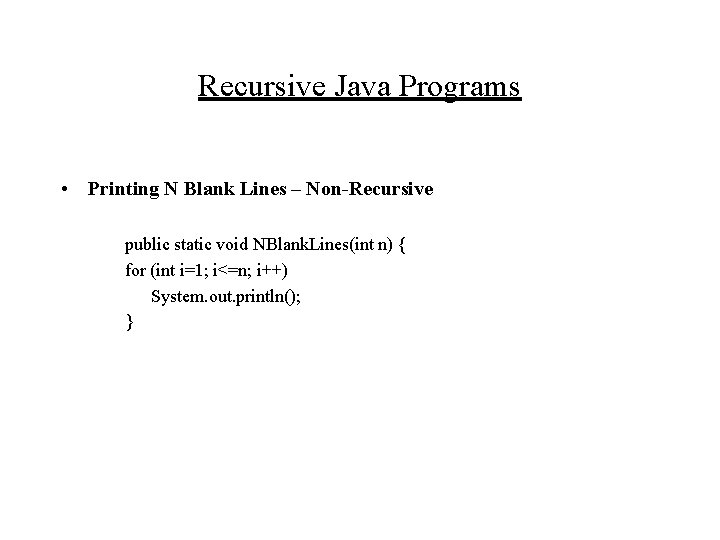
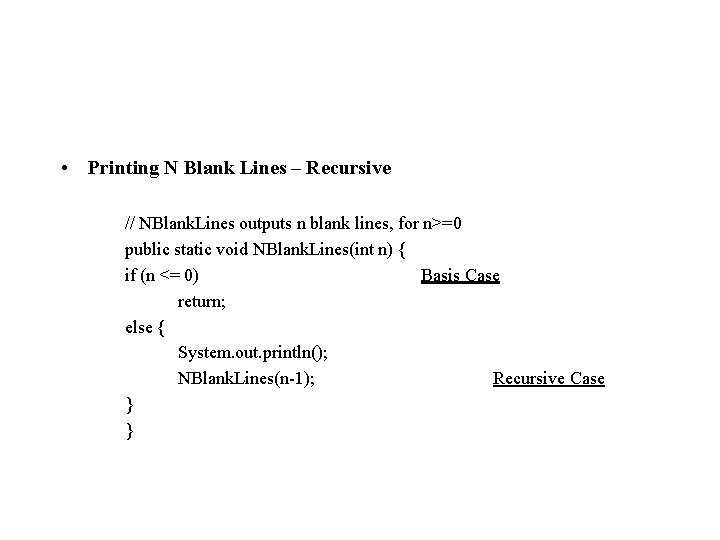
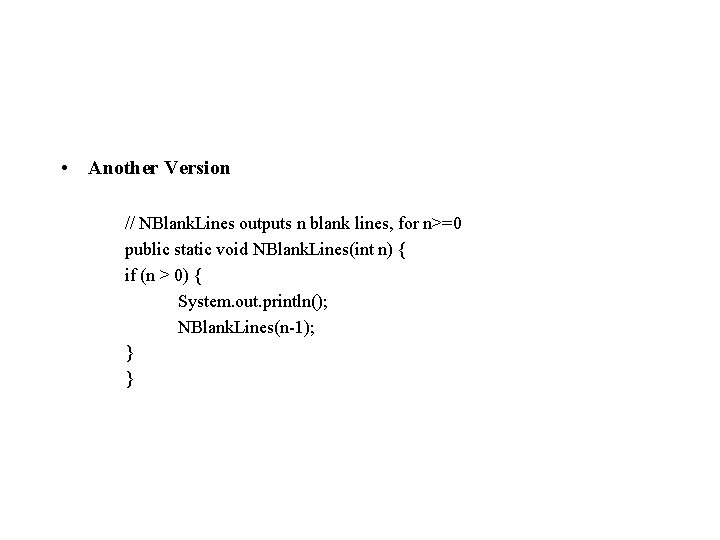
![public static void main(String[] pars) { : NBlank. Lines(3); : } public static void public static void main(String[] pars) { : NBlank. Lines(3); : } public static void](https://slidetodoc.com/presentation_image_h2/0ba0387dea8c5eea91ffedf34fea36ed/image-9.jpg)
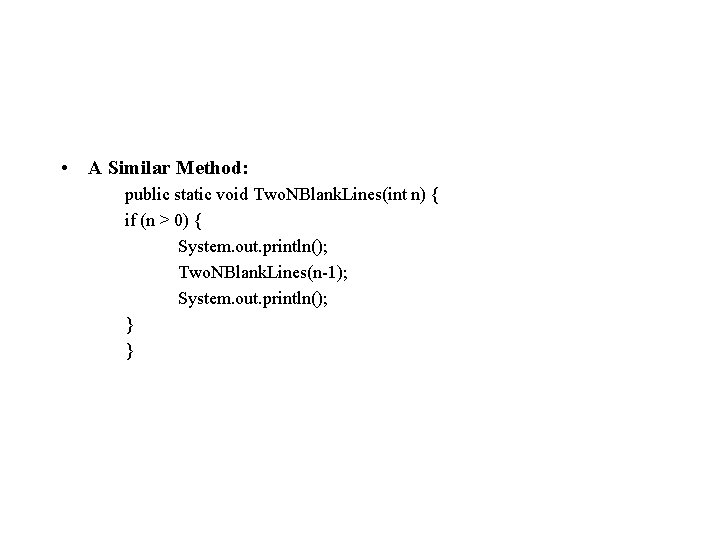
![public static void main(String[] pars) { : Two. NBlank. Lines(2); : } public static public static void main(String[] pars) { : Two. NBlank. Lines(2); : } public static](https://slidetodoc.com/presentation_image_h2/0ba0387dea8c5eea91ffedf34fea36ed/image-11.jpg)
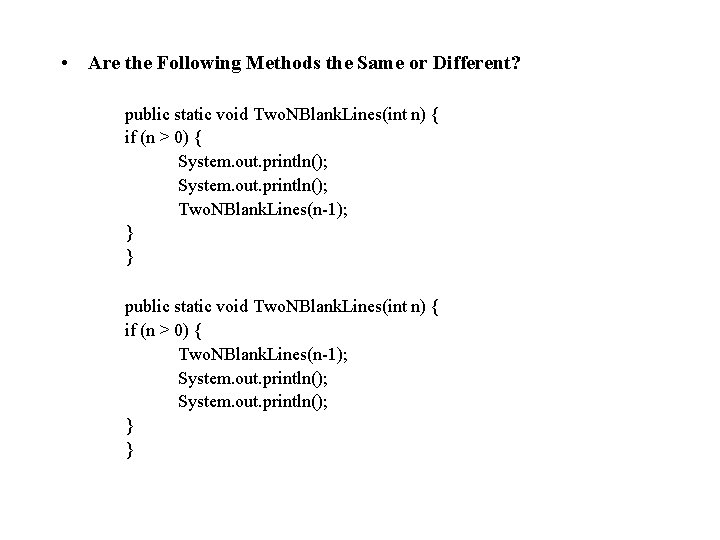
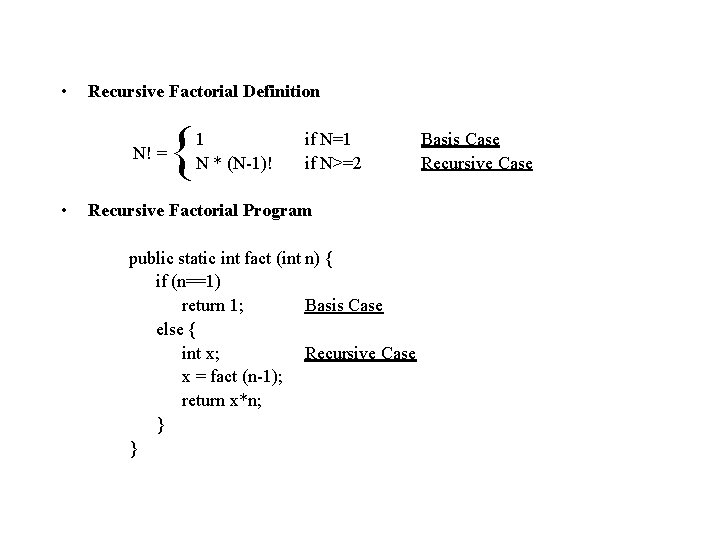
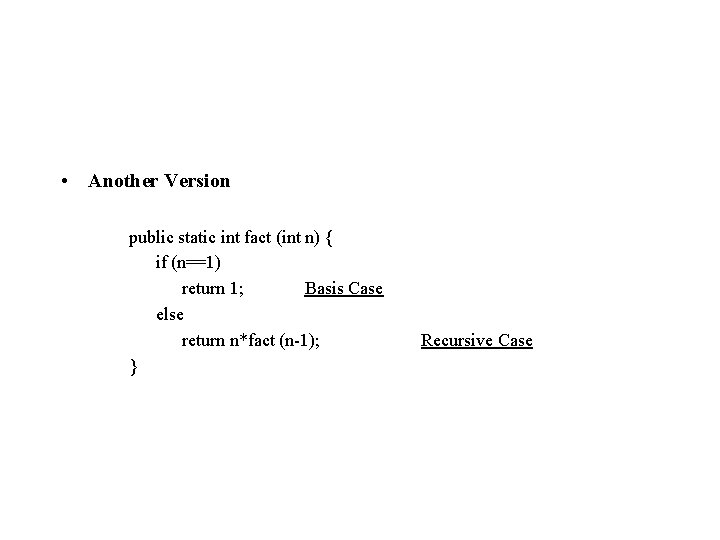
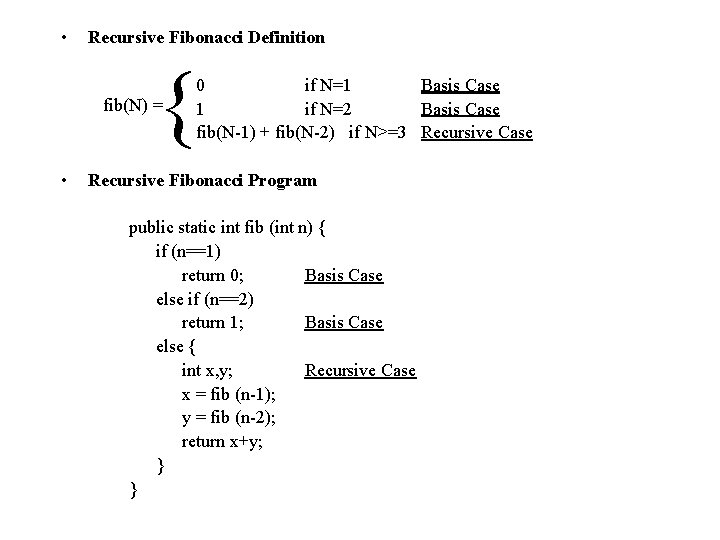
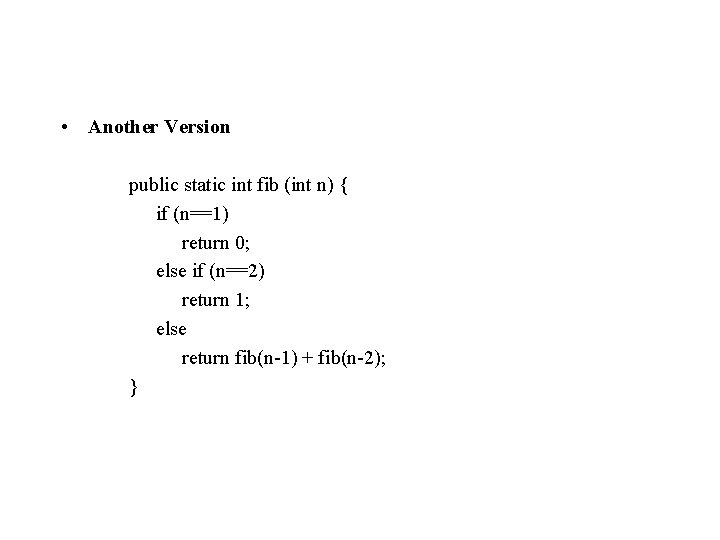
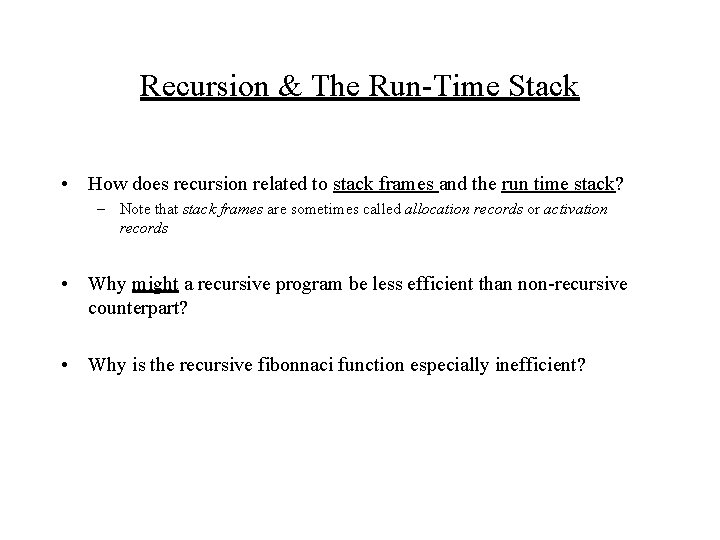
- Slides: 17
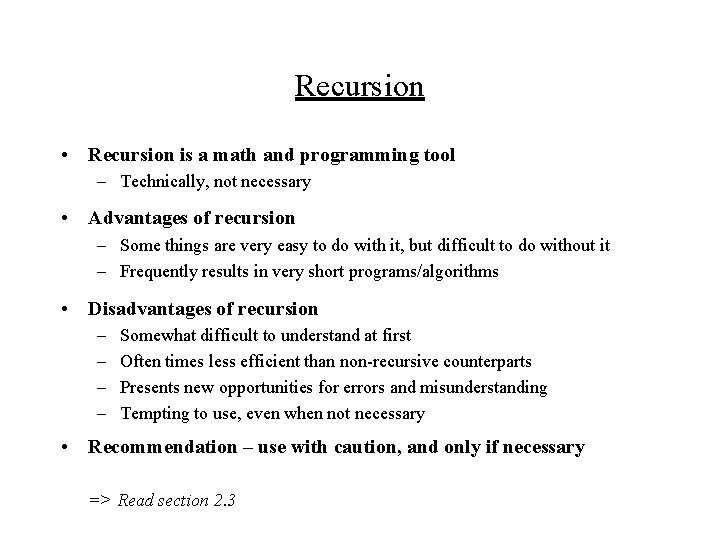
Recursion • Recursion is a math and programming tool – Technically, not necessary • Advantages of recursion – Some things are very easy to do with it, but difficult to do without it – Frequently results in very short programs/algorithms • Disadvantages of recursion – – Somewhat difficult to understand at first Often times less efficient than non-recursive counterparts Presents new opportunities for errors and misunderstanding Tempting to use, even when not necessary • Recommendation – use with caution, and only if necessary => Read section 2. 3
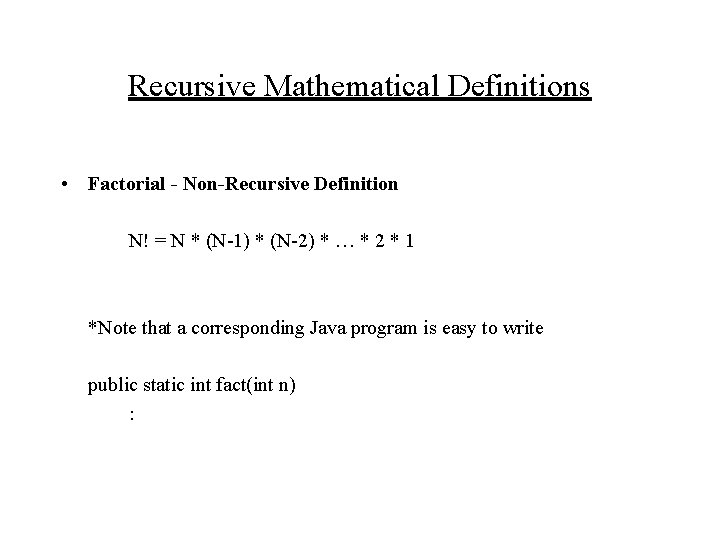
Recursive Mathematical Definitions • Factorial - Non-Recursive Definition N! = N * (N-1) * (N-2) * … * 2 * 1 *Note that a corresponding Java program is easy to write public static int fact(int n) :
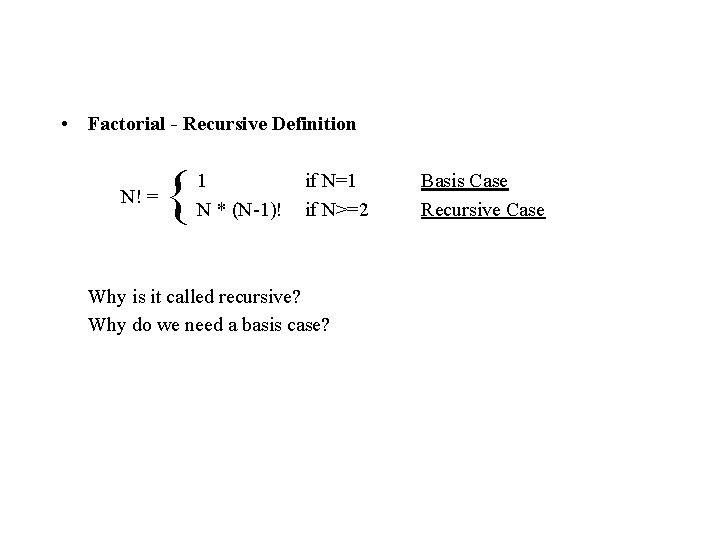
• Factorial - Recursive Definition N! = { 1 N * (N-1)! if N=1 if N>=2 Why is it called recursive? Why do we need a basis case? Basis Case Recursive Case
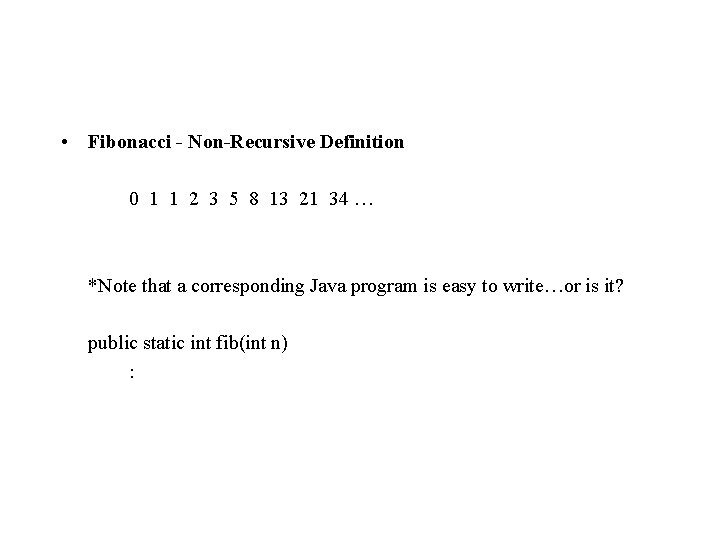
• Fibonacci - Non-Recursive Definition 0 1 1 2 3 5 8 13 21 34 … *Note that a corresponding Java program is easy to write…or is it? public static int fib(int n) :
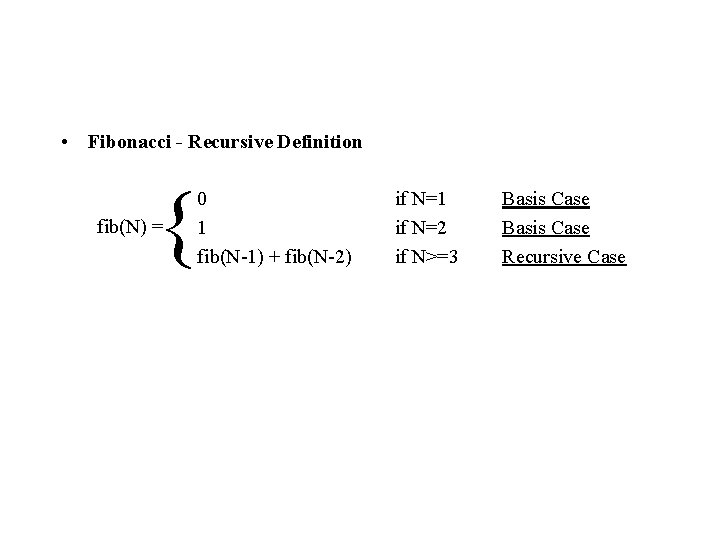
• Fibonacci - Recursive Definition { fib(N) = 0 1 fib(N-1) + fib(N-2) if N=1 if N=2 if N>=3 Basis Case Recursive Case
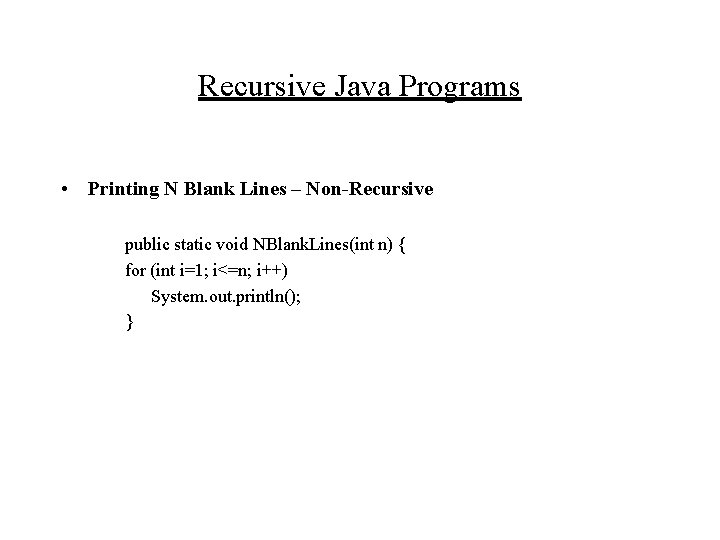
Recursive Java Programs • Printing N Blank Lines – Non-Recursive public static void NBlank. Lines(int n) { for (int i=1; i<=n; i++) System. out. println(); }
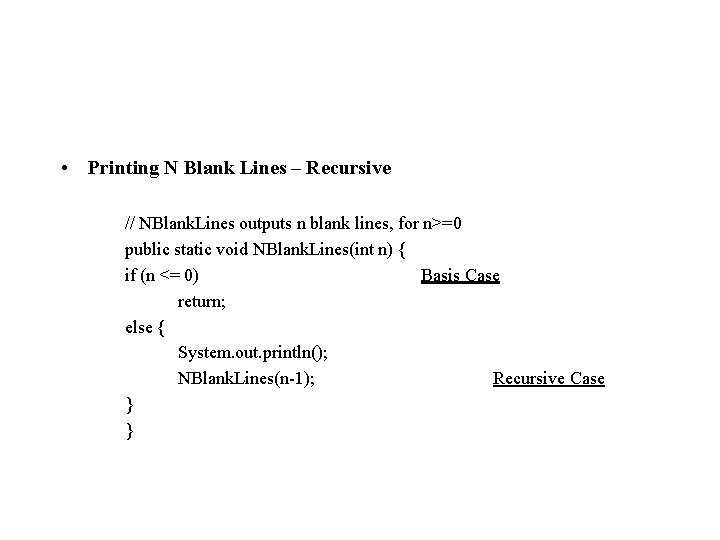
• Printing N Blank Lines – Recursive // NBlank. Lines outputs n blank lines, for n>=0 public static void NBlank. Lines(int n) { if (n <= 0) Basis Case return; else { System. out. println(); NBlank. Lines(n-1); Recursive Case } }
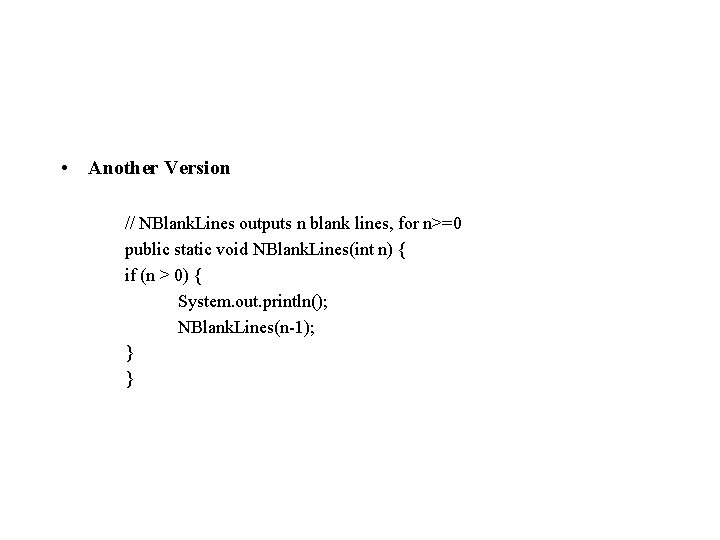
• Another Version // NBlank. Lines outputs n blank lines, for n>=0 public static void NBlank. Lines(int n) { if (n > 0) { System. out. println(); NBlank. Lines(n-1); } }
![public static void mainString pars NBlank Lines3 public static void public static void main(String[] pars) { : NBlank. Lines(3); : } public static void](https://slidetodoc.com/presentation_image_h2/0ba0387dea8c5eea91ffedf34fea36ed/image-9.jpg)
public static void main(String[] pars) { : NBlank. Lines(3); : } public static void NBlank. Lines(int n) { if (n > 0) { System. out. println(); NBlank. Lines(n-1); } } n=3 public static void NBlank. Lines(int n) { if (n > 0) { System. out. println(); NBlank. Lines(n-1); } } n=2 public static void NBlank. Lines(int n) { if (n > 0) { System. out. println(); NBlank. Lines(n-1); } } n=1 public static void NBlank. Lines(int n) { if (n > 0) { System. out. println(); NBlank. Lines(n-1); } } n=0
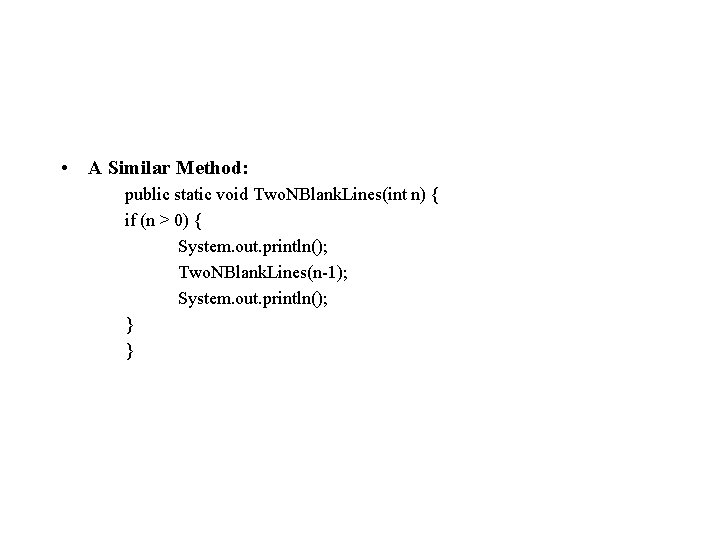
• A Similar Method: public static void Two. NBlank. Lines(int n) { if (n > 0) { System. out. println(); Two. NBlank. Lines(n-1); System. out. println(); } }
![public static void mainString pars Two NBlank Lines2 public static public static void main(String[] pars) { : Two. NBlank. Lines(2); : } public static](https://slidetodoc.com/presentation_image_h2/0ba0387dea8c5eea91ffedf34fea36ed/image-11.jpg)
public static void main(String[] pars) { : Two. NBlank. Lines(2); : } public static void Two. NBlank. Lines(int n) { if (n > 0) { System. out. println(); Two. NBlank. Lines(n-1); System. out. println(); } } n=2 public static void Two. NBlank. Lines(int n) { if (n > 0) { System. out. println(); Two. NBlank. Lines(n-1); System. out. println(); } } n=1 public static void Two. NBlank. Lines(int n) { if (n > 0) { System. out. println(); Two. NBlank. Lines(n-1); System. out. println(); } } n=0
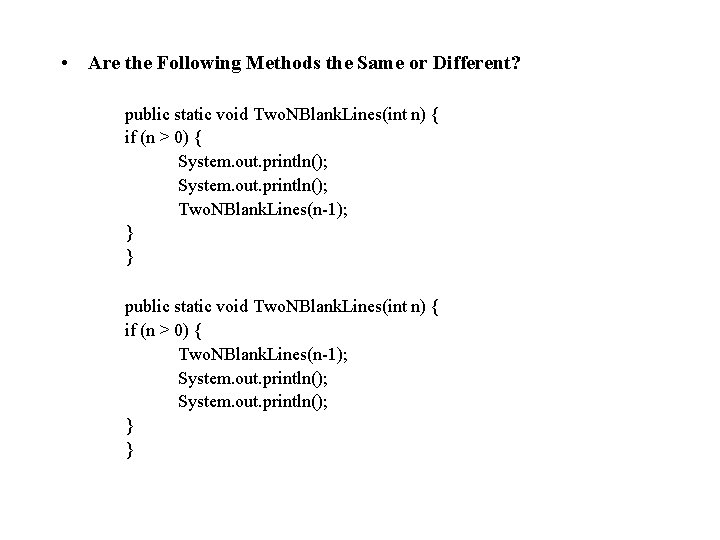
• Are the Following Methods the Same or Different? public static void Two. NBlank. Lines(int n) { if (n > 0) { System. out. println(); Two. NBlank. Lines(n-1); } } public static void Two. NBlank. Lines(int n) { if (n > 0) { Two. NBlank. Lines(n-1); System. out. println(); } }
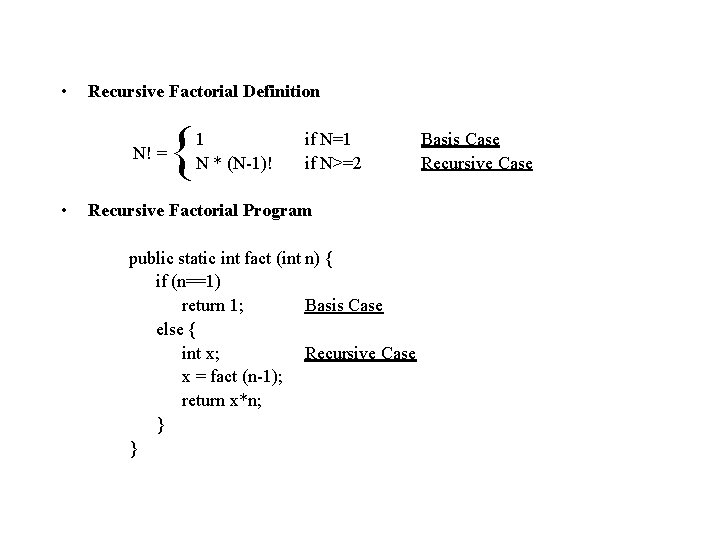
• Recursive Factorial Definition N! = • { 1 N * (N-1)! if N=1 if N>=2 Recursive Factorial Program public static int fact (int n) { if (n==1) return 1; Basis Case else { int x; Recursive Case x = fact (n-1); return x*n; } } Basis Case Recursive Case
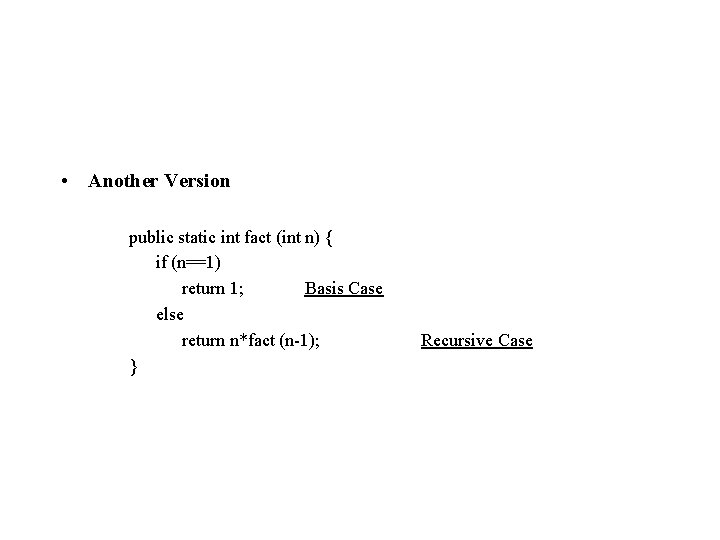
• Another Version public static int fact (int n) { if (n==1) return 1; Basis Case else return n*fact (n-1); } Recursive Case
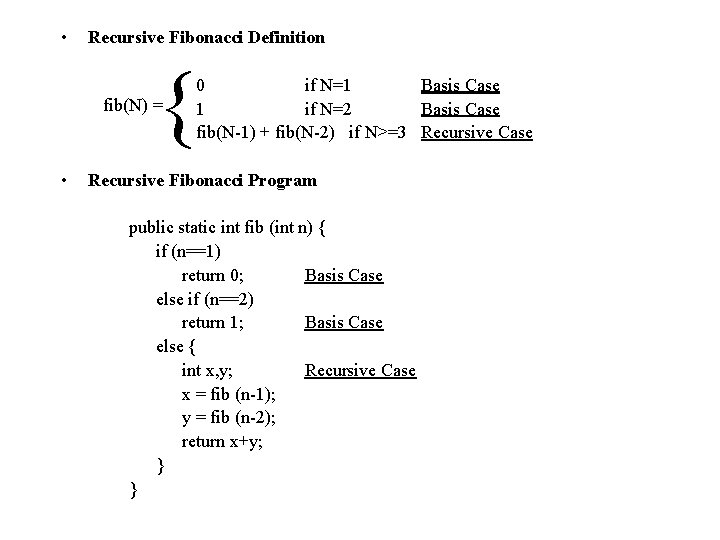
• Recursive Fibonacci Definition { fib(N) = • 0 if N=1 Basis Case 1 if N=2 Basis Case fib(N-1) + fib(N-2) if N>=3 Recursive Case Recursive Fibonacci Program public static int fib (int n) { if (n==1) return 0; Basis Case else if (n==2) return 1; Basis Case else { int x, y; Recursive Case x = fib (n-1); y = fib (n-2); return x+y; } }
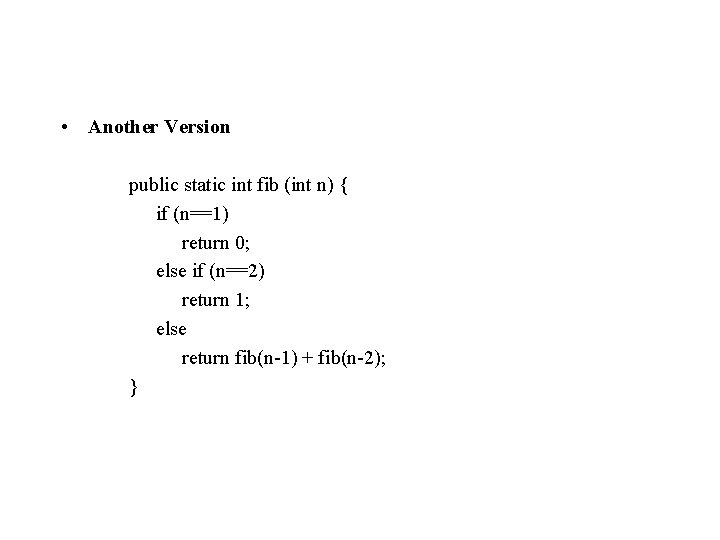
• Another Version public static int fib (int n) { if (n==1) return 0; else if (n==2) return 1; else return fib(n-1) + fib(n-2); }
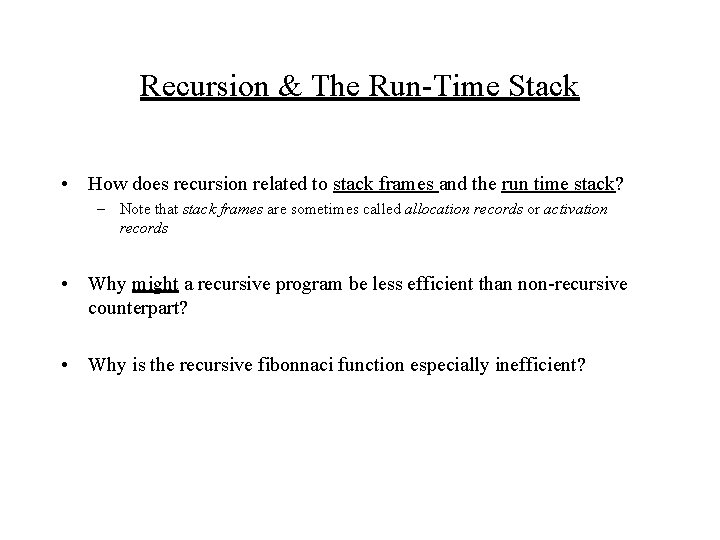
Recursion & The Run-Time Stack • How does recursion related to stack frames and the run time stack? – Note that stack frames are sometimes called allocation records or activation records • Why might a recursive program be less efficient than non-recursive counterpart? • Why is the recursive fibonnaci function especially inefficient?
To understand recursion you must understand recursion
Recursive thought
Dynamic programming recursion example
Bh&m
What is recursion can be a powerful tool for solving?
Recursive form
Recursion in discrete mathematics
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is system programing
Linear vs integer programming
Definisi integer
Metode grafis
Potter's tool is data cleaning tool
Hit the button
Using recursion in models and decision making
Iterative vs recursive
Using recursion in models and decision making sheet 3