Recursion Definition n Recursion is a function calling
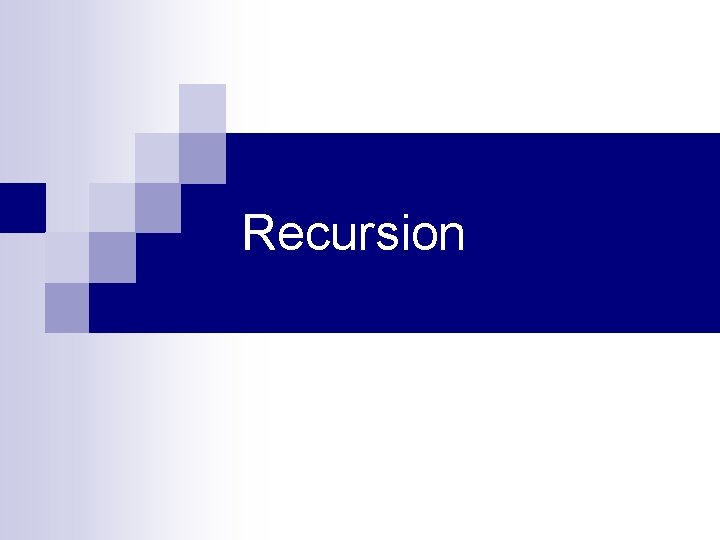
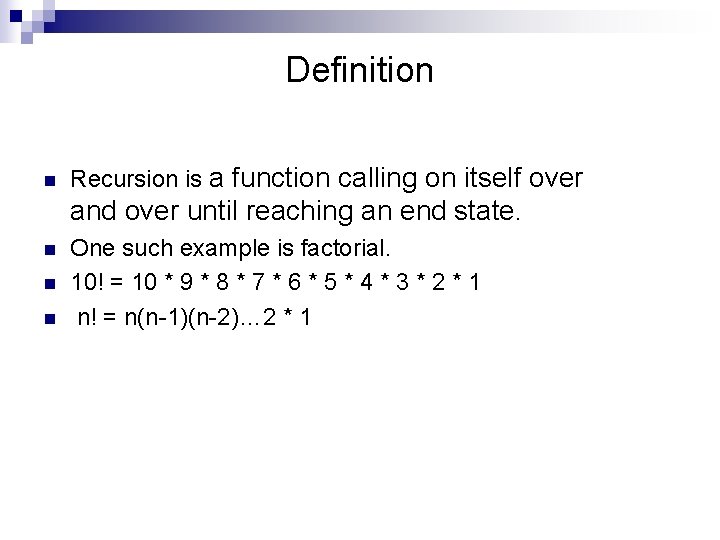
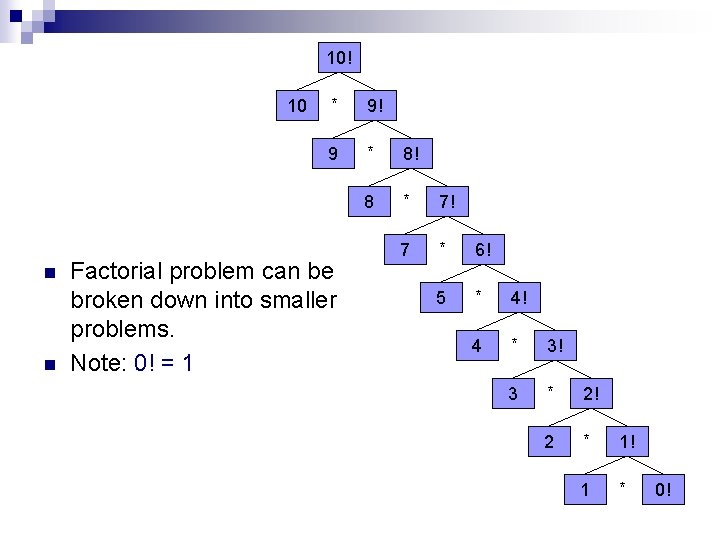
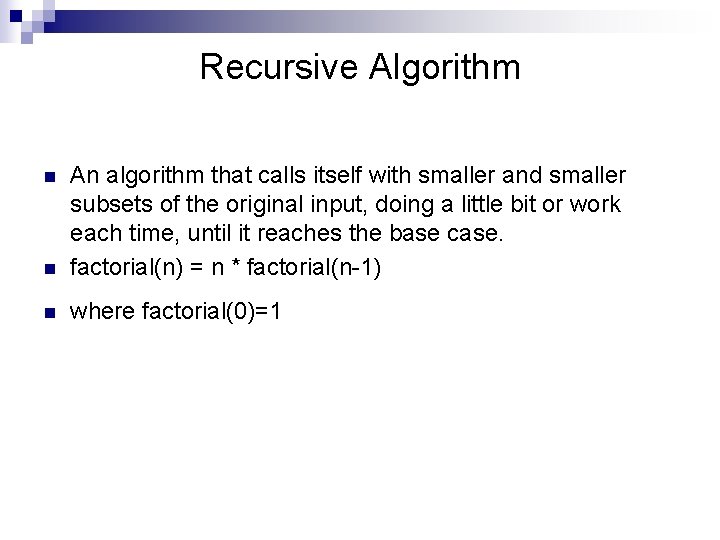
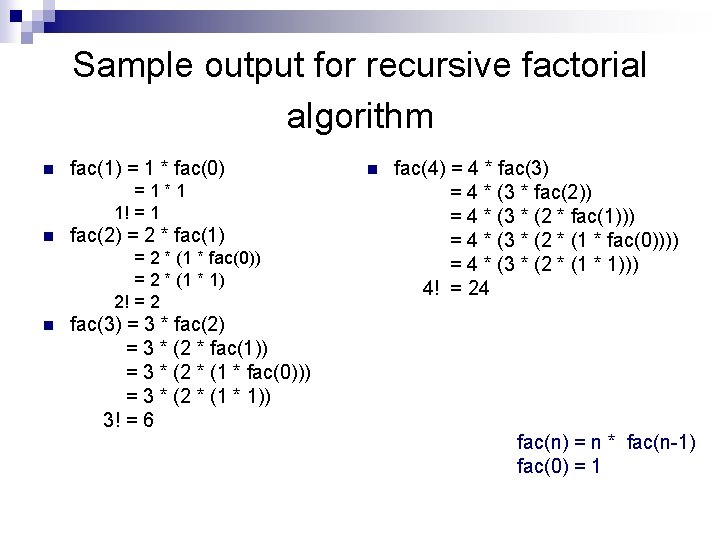
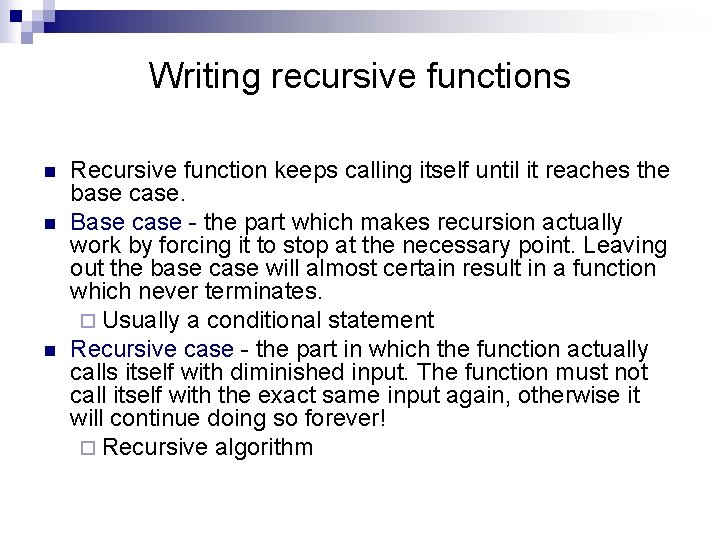
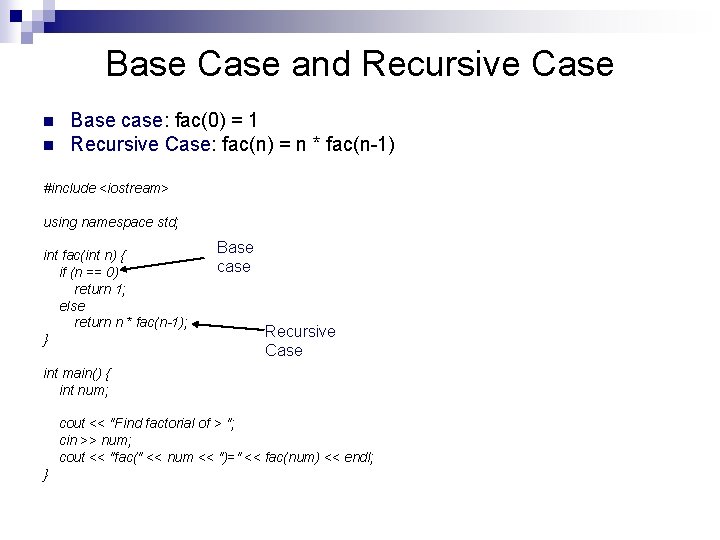
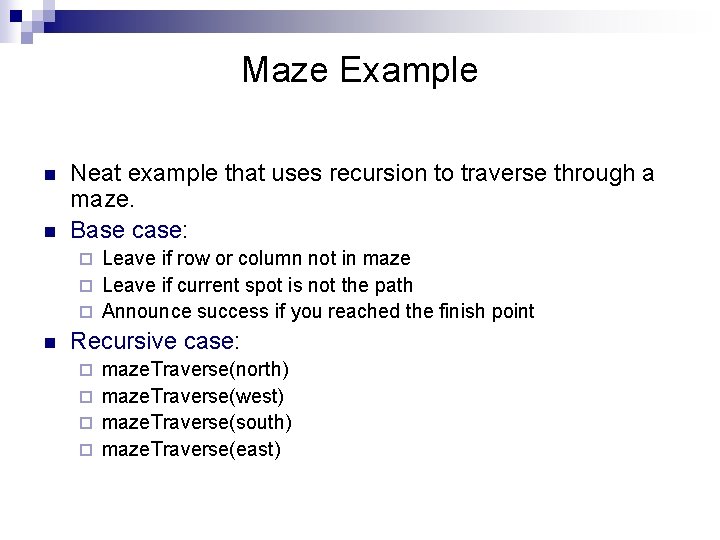
![void maze. Traverse(char maze[12], int start_row, int start_col) { if(start_row>=0 && start_row<12 && start_col>=0 void maze. Traverse(char maze[12], int start_row, int start_col) { if(start_row>=0 && start_row<12 && start_col>=0](https://slidetodoc.com/presentation_image_h/d6e48db286461c75797b8968d2724b6b/image-9.jpg)
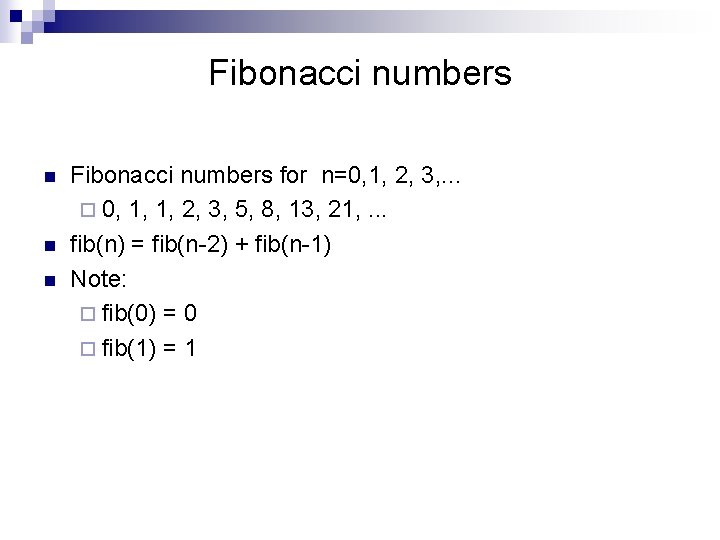
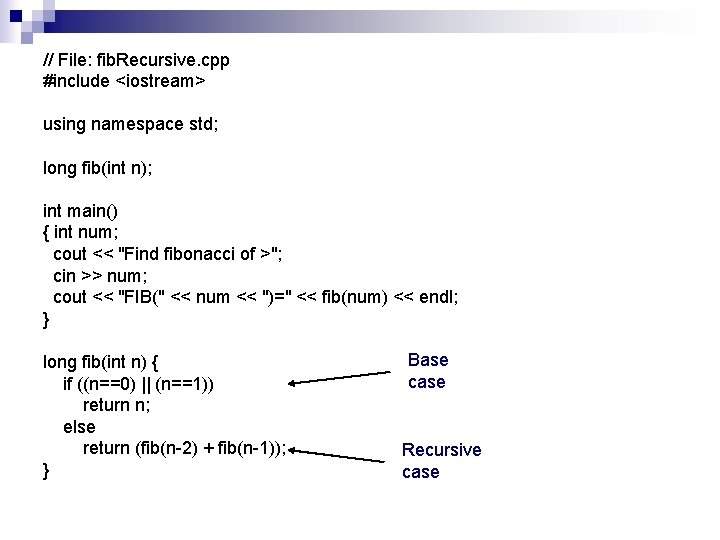
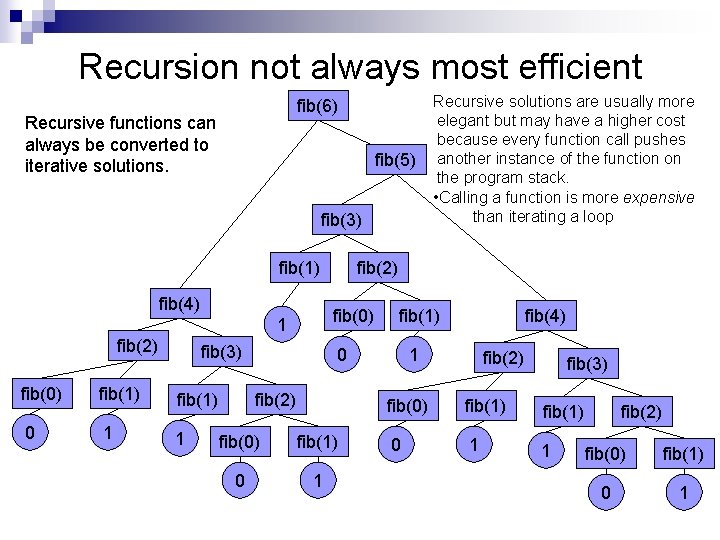
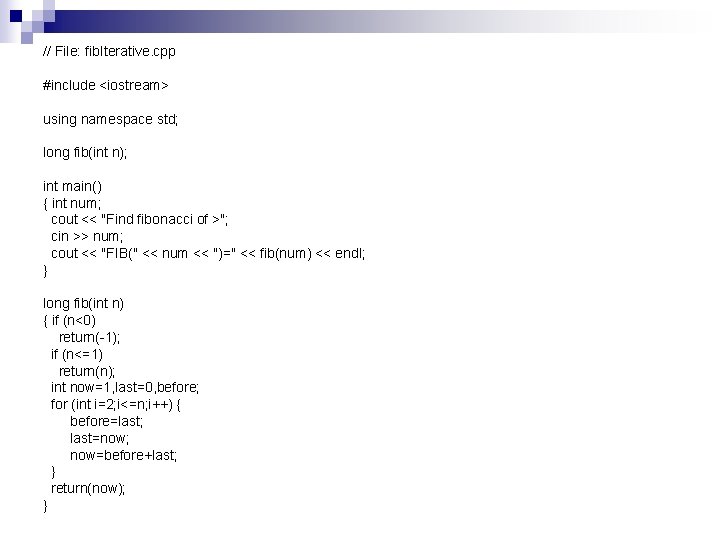
- Slides: 13
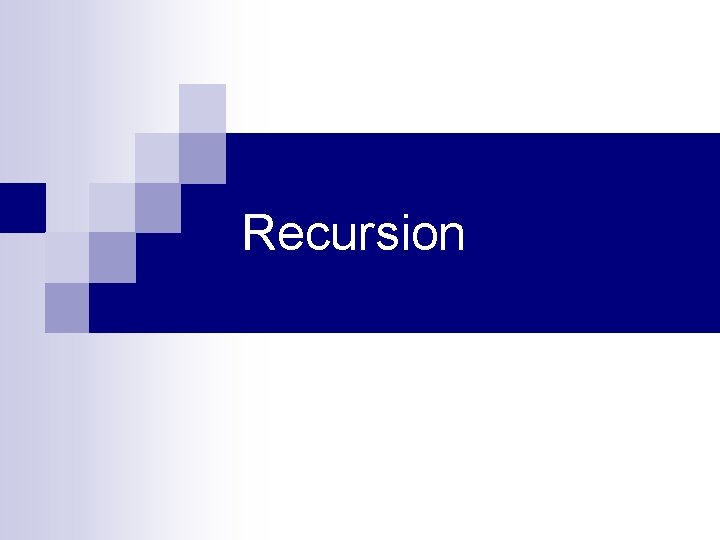
Recursion
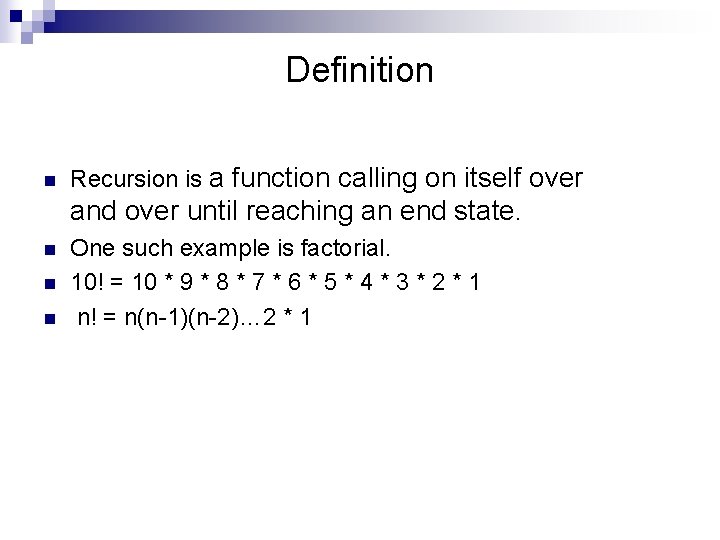
Definition n Recursion is a function calling on itself over and over until reaching an end state. n n n One such example is factorial. 10! = 10 * 9 * 8 * 7 * 6 * 5 * 4 * 3 * 2 * 1 n! = n(n-1)(n-2)… 2 * 1
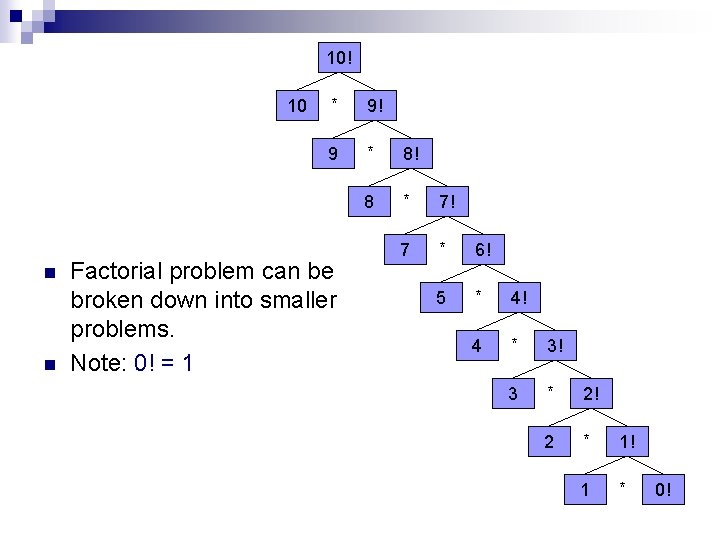
10! 10 n n * 9! 9 * 8! 8 * 7! 7 * 6! 5 * 4! 4 * 3! 3 * 2! 2 * 1! 1 * Factorial problem can be broken down into smaller problems. Note: 0! = 1 0!
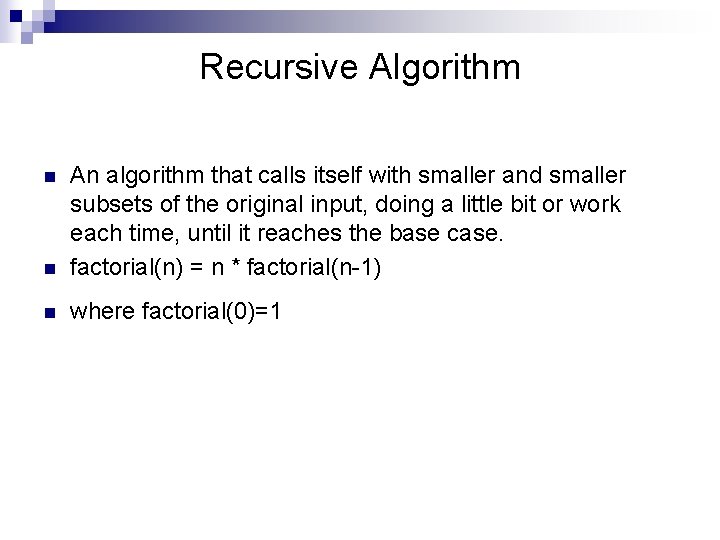
Recursive Algorithm n An algorithm that calls itself with smaller and smaller subsets of the original input, doing a little bit or work each time, until it reaches the base case. factorial(n) = n * factorial(n-1) n where factorial(0)=1 n
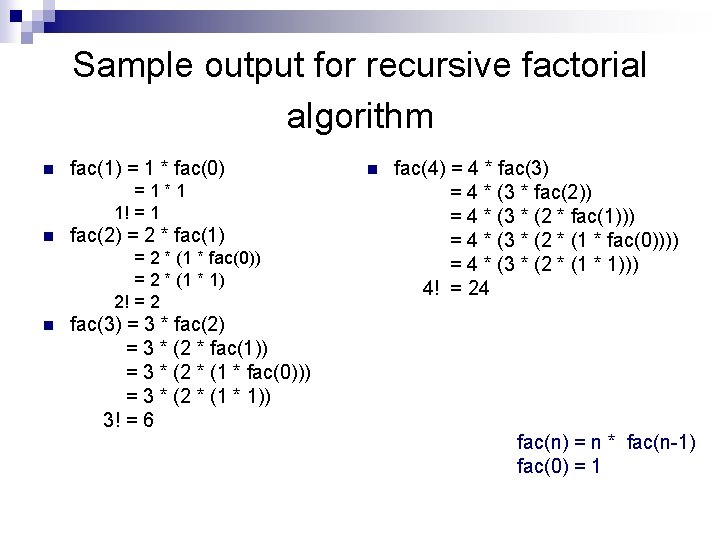
Sample output for recursive factorial algorithm n fac(1) = 1 * fac(0) =1*1 1! = 1 n fac(2) = 2 * fac(1) = 2 * (1 * fac(0)) = 2 * (1 * 1) 2! = 2 n fac(3) = 3 * fac(2) = 3 * (2 * fac(1)) = 3 * (2 * (1 * fac(0))) = 3 * (2 * (1 * 1)) 3! = 6 n fac(4) = 4 * fac(3) = 4 * (3 * fac(2)) = 4 * (3 * (2 * fac(1))) = 4 * (3 * (2 * (1 * fac(0)))) = 4 * (3 * (2 * (1 * 1))) 4! = 24 fac(n) = n * fac(n-1) fac(0) = 1
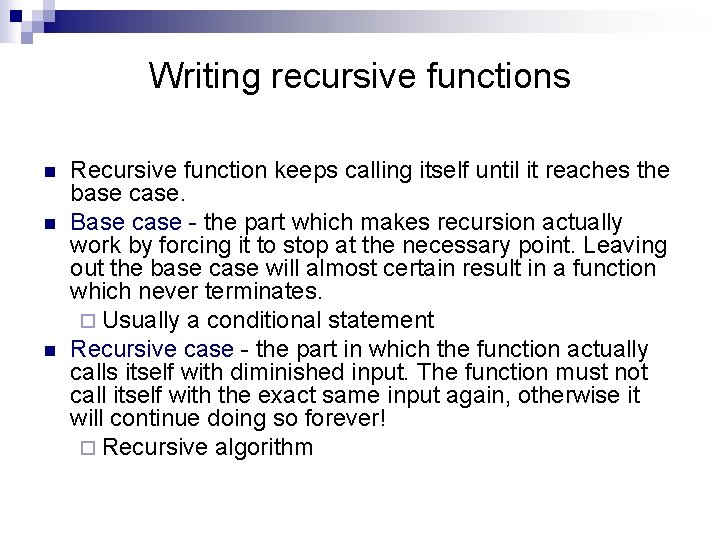
Writing recursive functions n n n Recursive function keeps calling itself until it reaches the base case. Base case - the part which makes recursion actually work by forcing it to stop at the necessary point. Leaving out the base case will almost certain result in a function which never terminates. ¨ Usually a conditional statement Recursive case - the part in which the function actually calls itself with diminished input. The function must not call itself with the exact same input again, otherwise it will continue doing so forever! ¨ Recursive algorithm
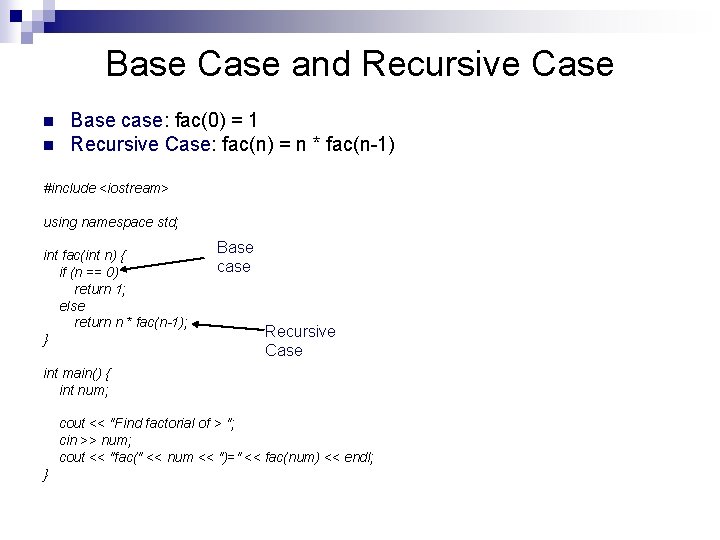
Base Case and Recursive Case n n Base case: fac(0) = 1 Recursive Case: fac(n) = n * fac(n-1) #include <iostream> using namespace std; int fac(int n) { if (n == 0) return 1; else return n * fac(n-1); } Base case Recursive Case int main() { int num; cout << "Find factorial of > "; cin >> num; cout << "fac(" << num << ")=" << fac(num) << endl; }
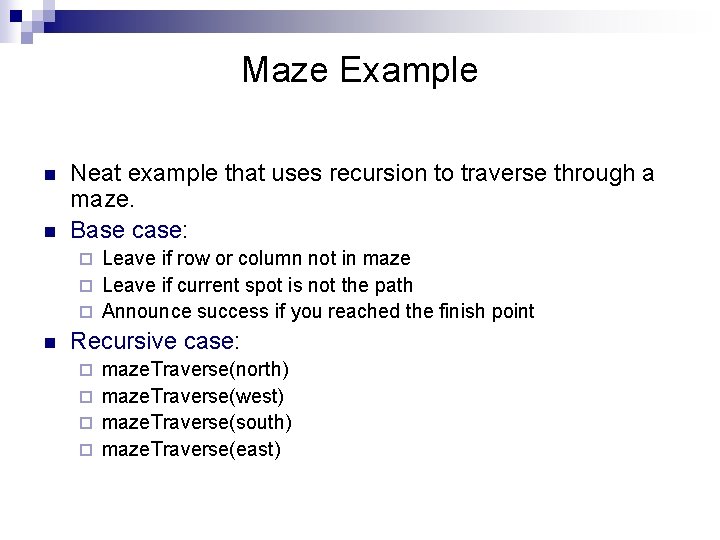
Maze Example n n Neat example that uses recursion to traverse through a maze. Base case: Leave if row or column not in maze ¨ Leave if current spot is not the path ¨ Announce success if you reached the finish point ¨ n Recursive case: maze. Traverse(north) ¨ maze. Traverse(west) ¨ maze. Traverse(south) ¨ maze. Traverse(east) ¨
![void maze Traversechar maze12 int startrow int startcol ifstartrow0 startrow12 startcol0 void maze. Traverse(char maze[12], int start_row, int start_col) { if(start_row>=0 && start_row<12 && start_col>=0](https://slidetodoc.com/presentation_image_h/d6e48db286461c75797b8968d2724b6b/image-9.jpg)
void maze. Traverse(char maze[12], int start_row, int start_col) { if(start_row>=0 && start_row<12 && start_col>=0 && start_col<12) { if(start_row==4 && start_col==11) cout<<"success"<<endl; if(maze[start_row][start_col]=='. ') { maze[start_row][start_col]='x'; print_array(maze, 12); maze. Traverse(maze, start_row-1, start_col); maze. Traverse(maze, start_row, start_col-1); maze. Traverse(maze, start_row+1, start_col); maze. Traverse(maze, start_row, start_col+1); maze[start_row][start_col]='*'; } } } Base case Recursive case
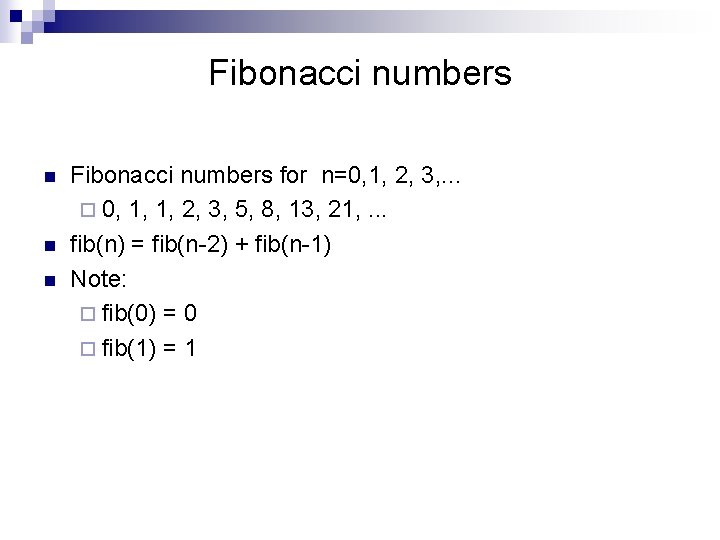
Fibonacci numbers n n n Fibonacci numbers for n=0, 1, 2, 3, . . . ¨ 0, 1, 1, 2, 3, 5, 8, 13, 21, . . . fib(n) = fib(n-2) + fib(n-1) Note: ¨ fib(0) = 0 ¨ fib(1) = 1
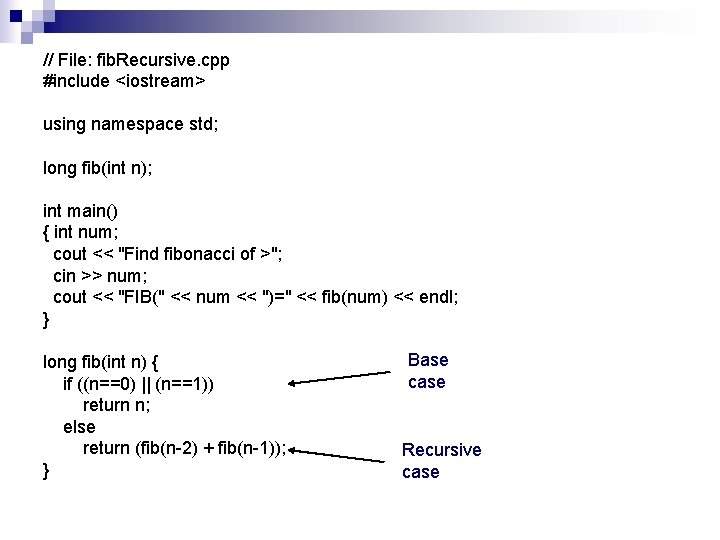
// File: fib. Recursive. cpp #include <iostream> using namespace std; long fib(int n); int main() { int num; cout << "Find fibonacci of >"; cin >> num; cout << "FIB(" << num << ")=" << fib(num) << endl; } long fib(int n) { if ((n==0) || (n==1)) return n; else return (fib(n-2) + fib(n-1)); } Base case Recursive case
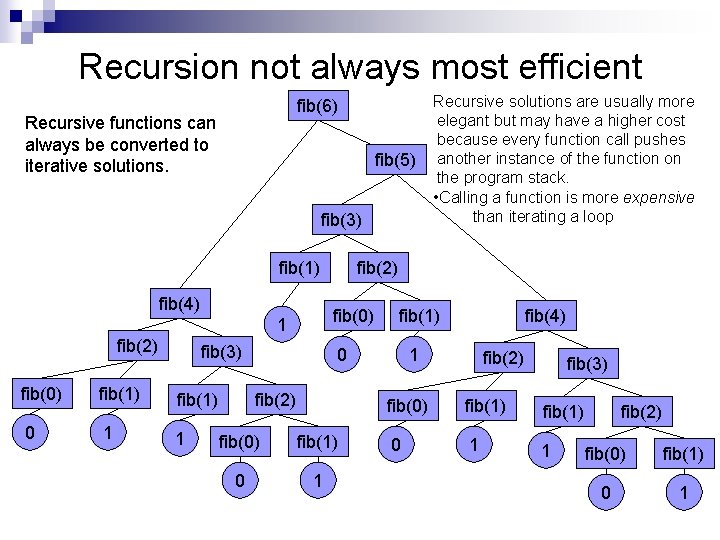
Recursion not always most efficient fib(6) Recursive functions can always be converted to iterative solutions. fib(5) fib(3) fib(1) fib(4) fib(2) fib(3) fib(0) fib(1) 0 1 1 fib(2) fib(0) 1 Recursive solutions are usually more elegant but may have a higher cost because every function call pushes another instance of the function on the program stack. • Calling a function is more expensive than iterating a loop fib(1) 0 fib(2) fib(0) fib(1) 0 1 fib(4) 1 fib(2) fib(3) fib(0) fib(1) 0 1 1 fib(2) fib(0) fib(1) 0 1
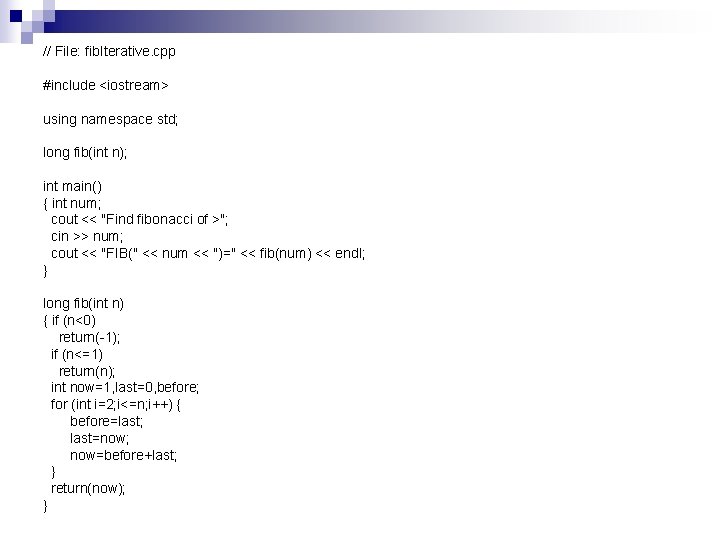
// File: fib. Iterative. cpp #include <iostream> using namespace std; long fib(int n); int main() { int num; cout << "Find fibonacci of >"; cin >> num; cout << "FIB(" << num << ")=" << fib(num) << endl; } long fib(int n) { if (n<0) return(-1); if (n<=1) return(n); int now=1, last=0, before; for (int i=2; i<=n; i++) { before=last; last=now; now=before+last; } return(now); }