Recap from last time We saw various different
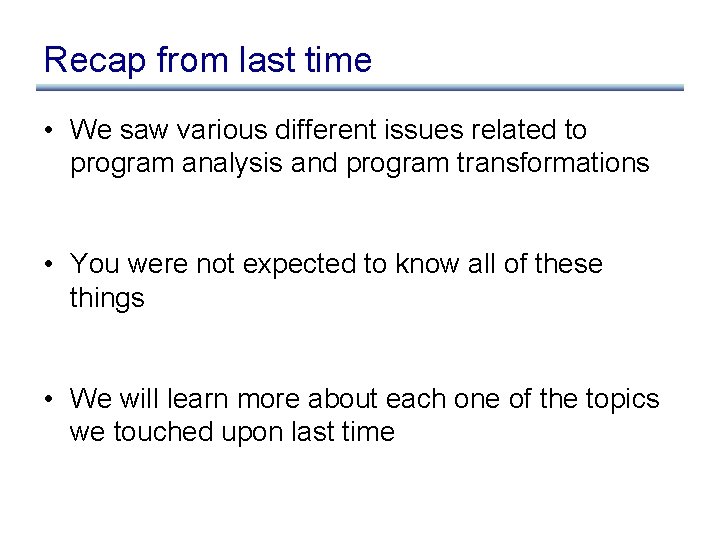
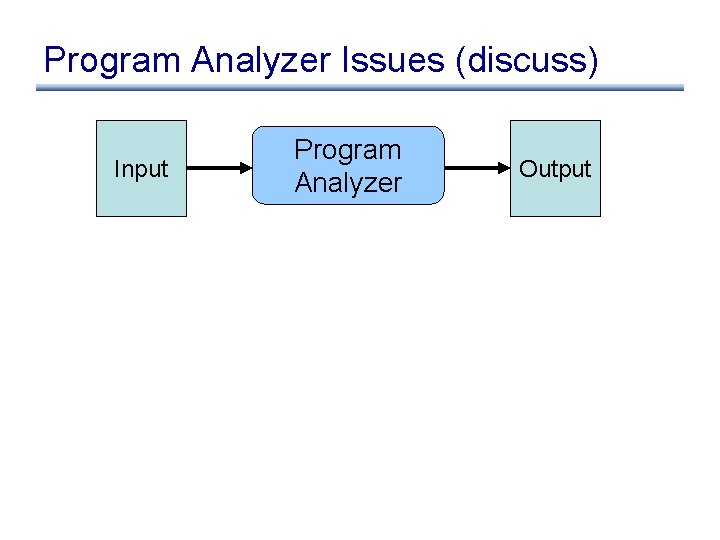
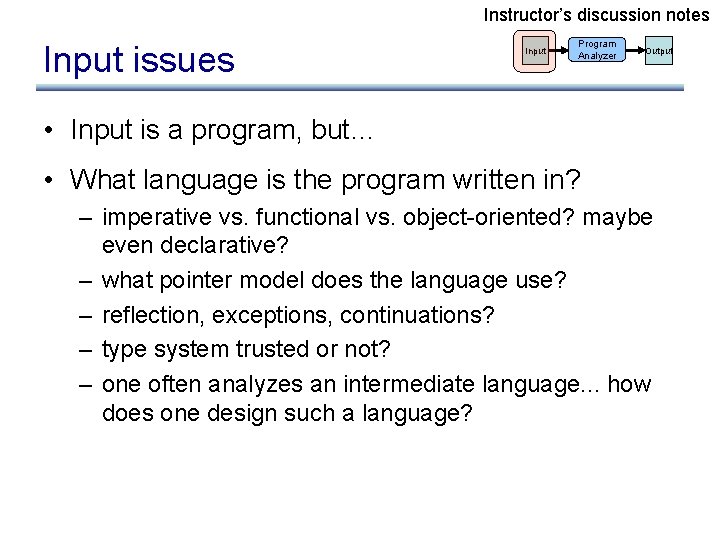
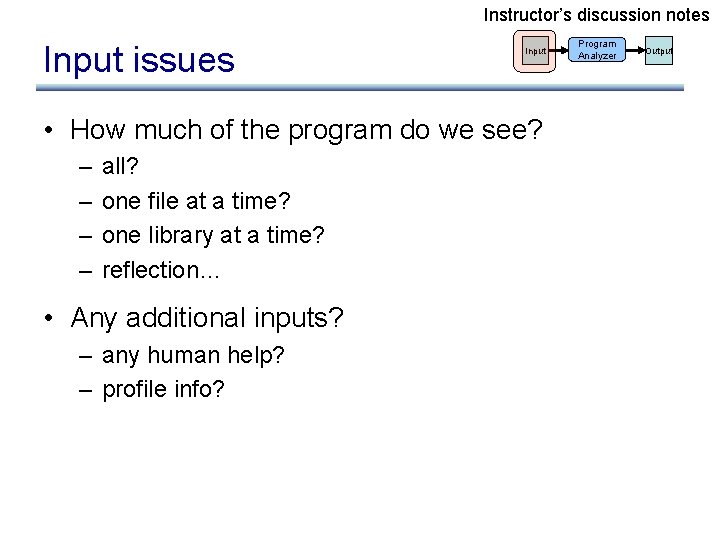
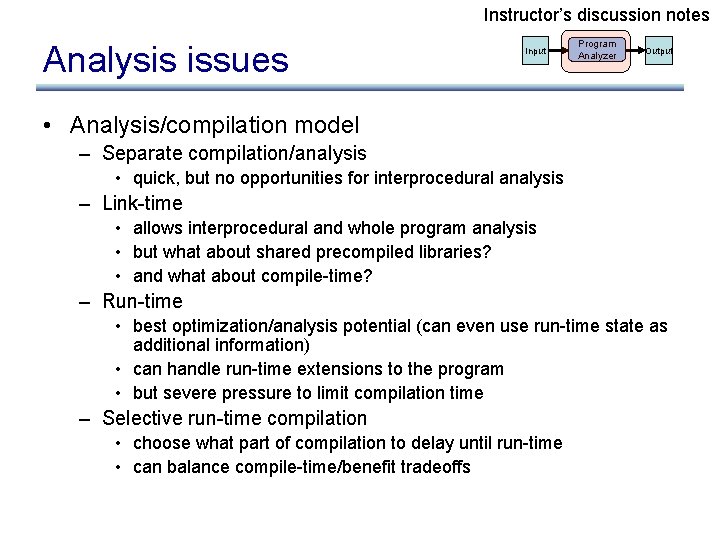
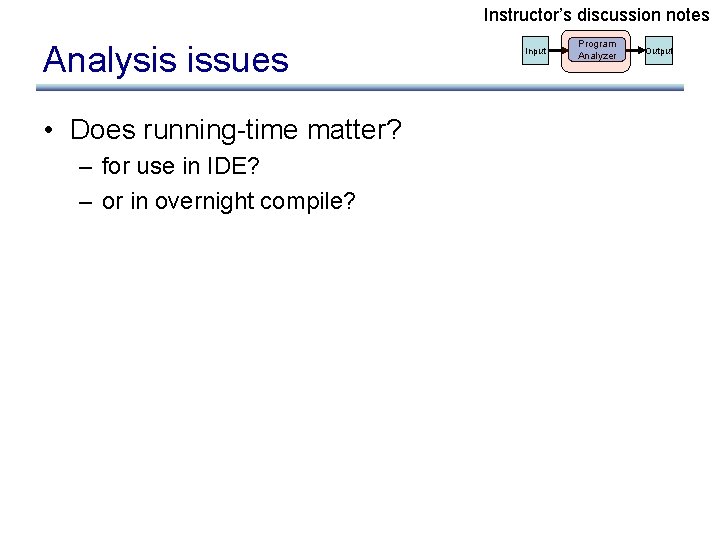
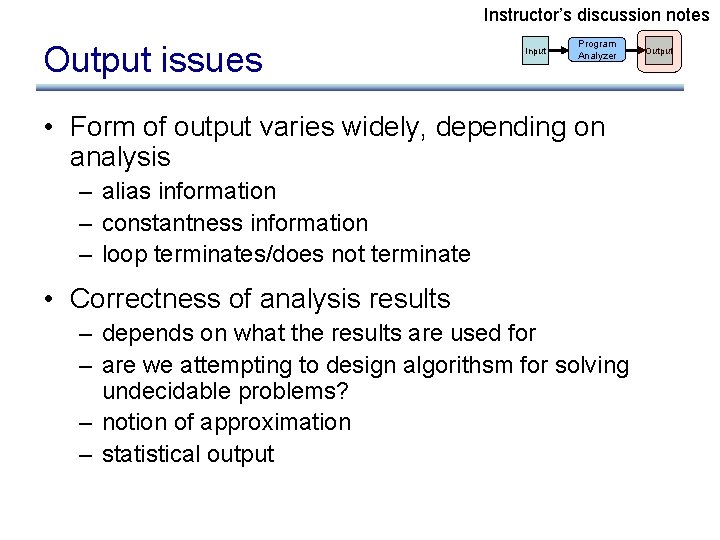
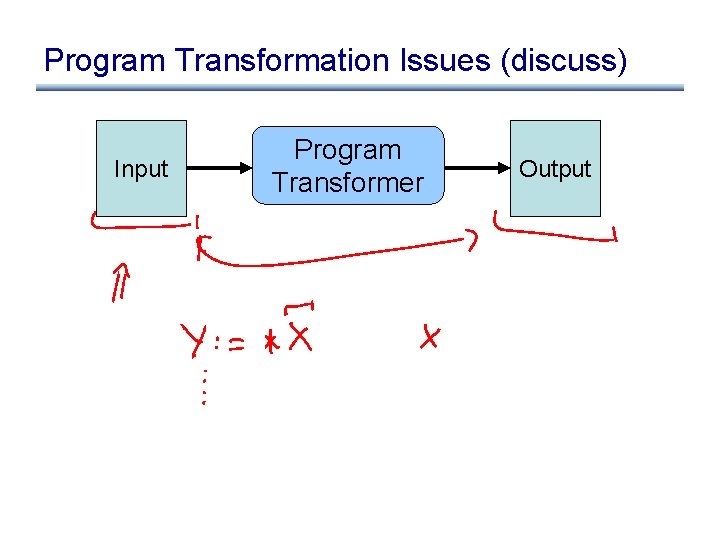
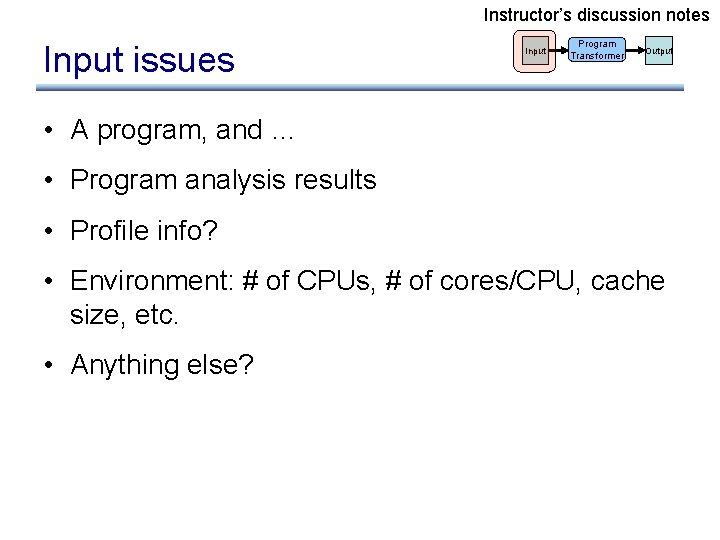
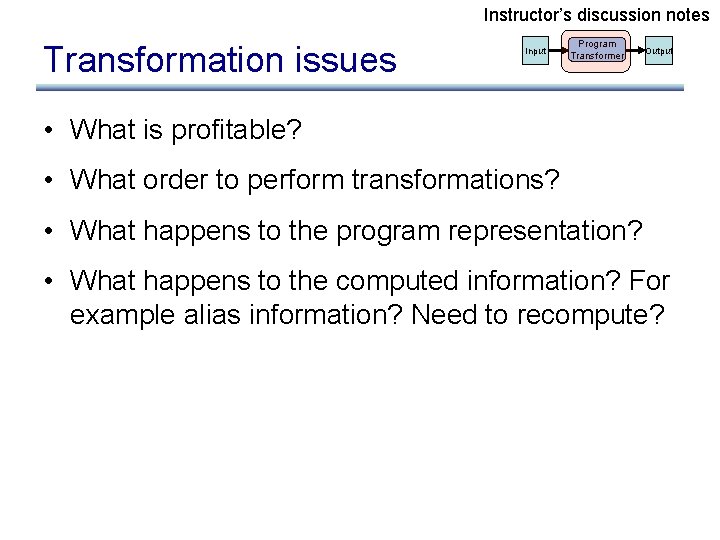
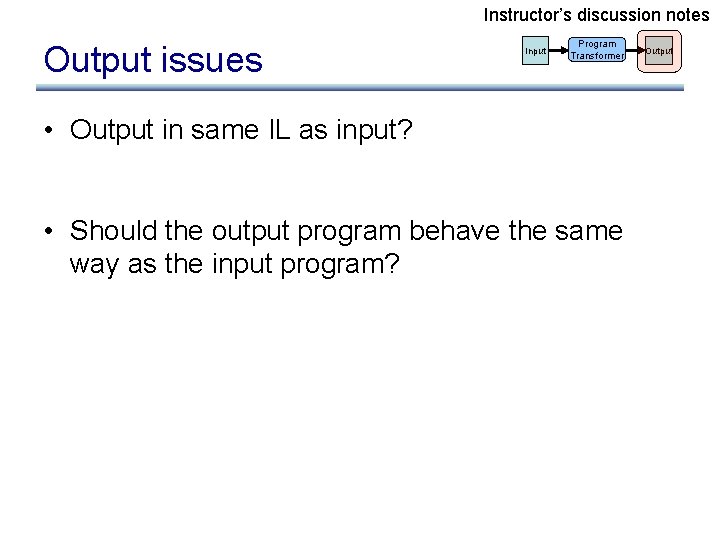
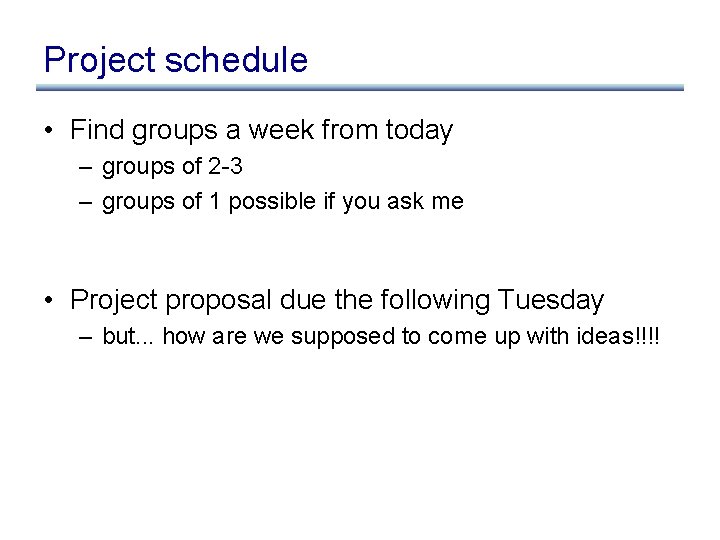
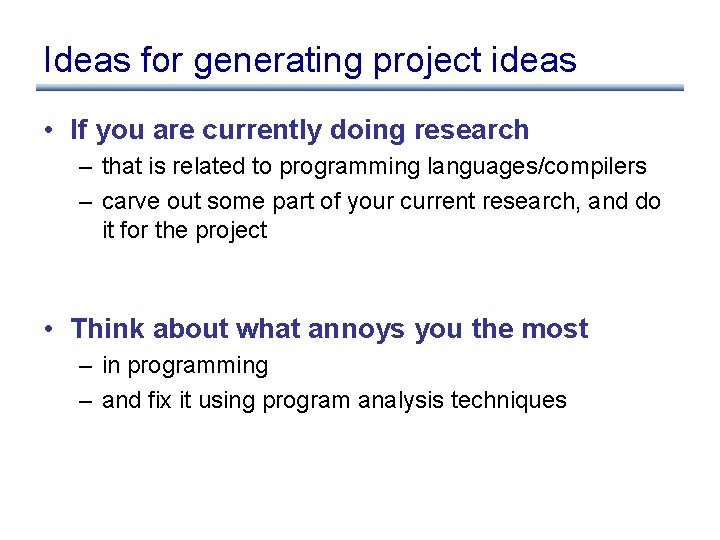
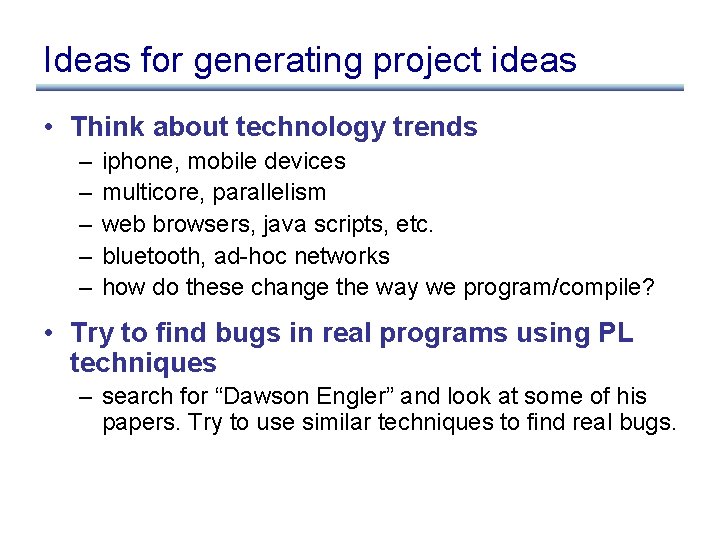
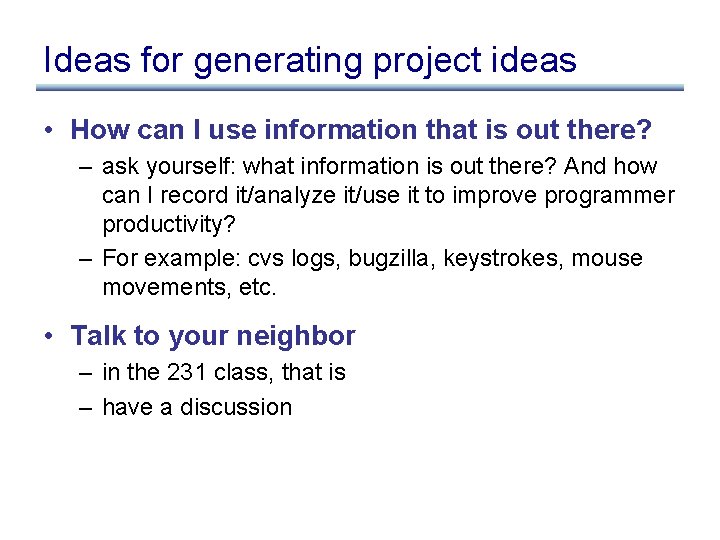
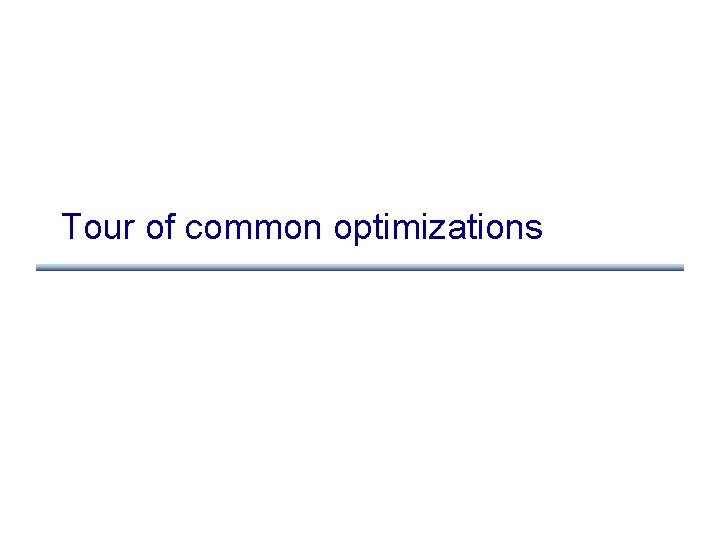
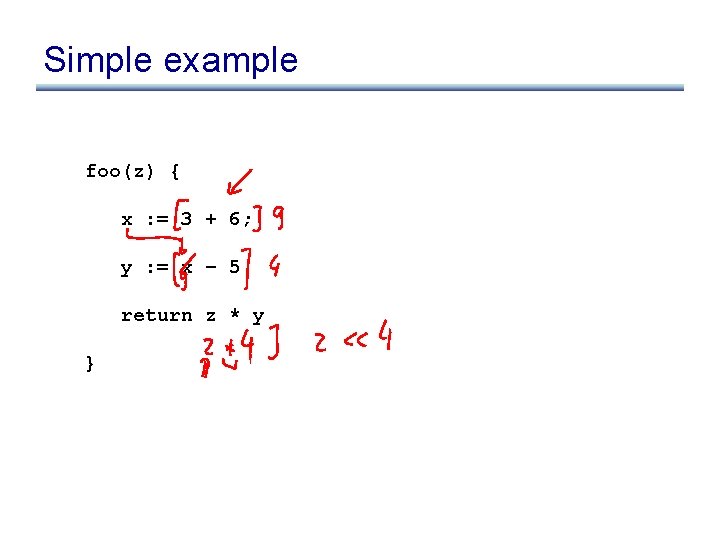
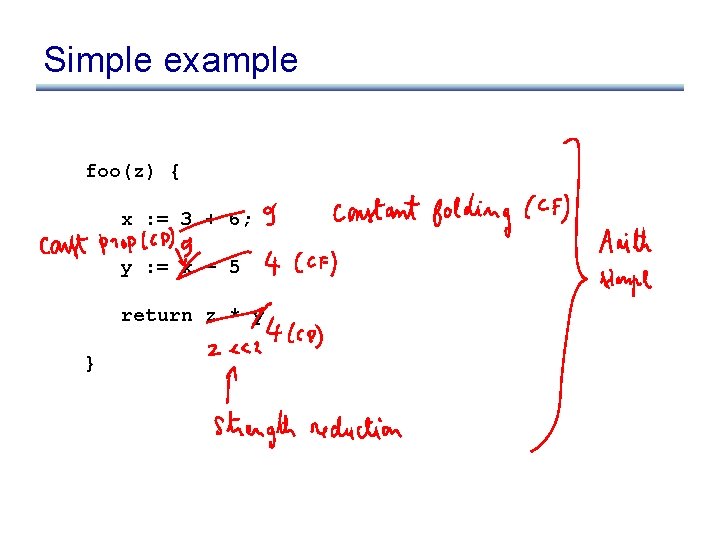
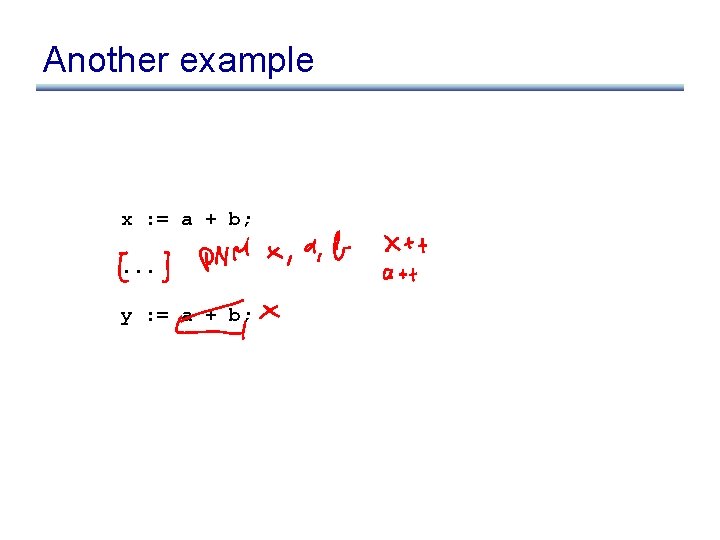
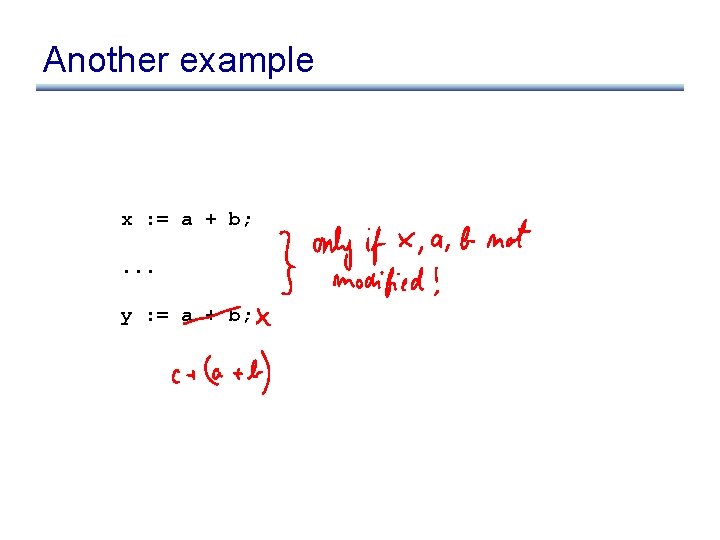
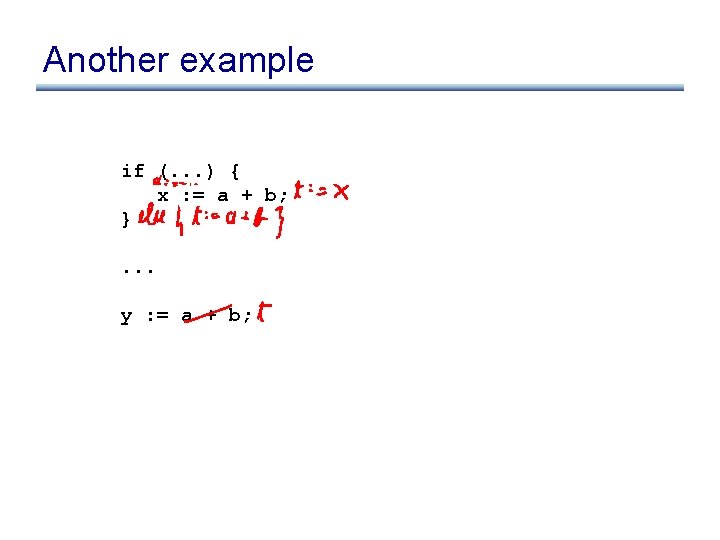
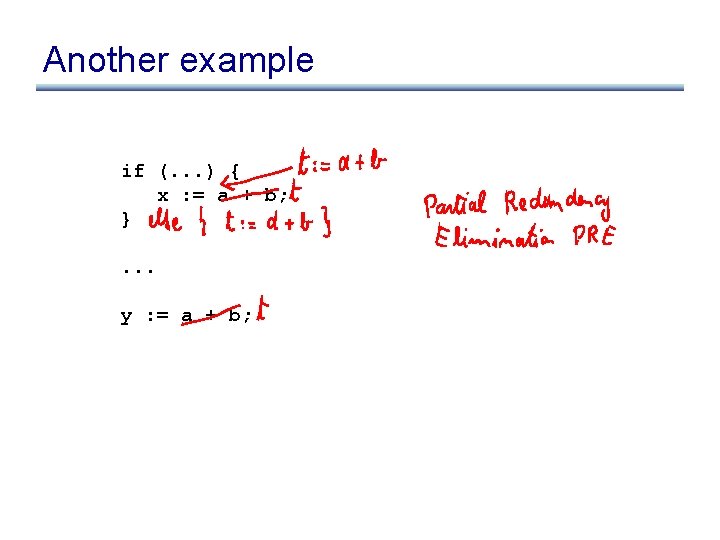
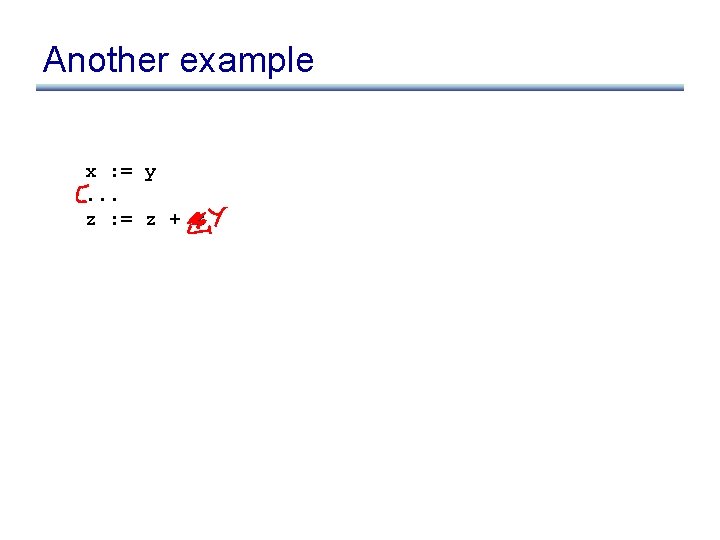
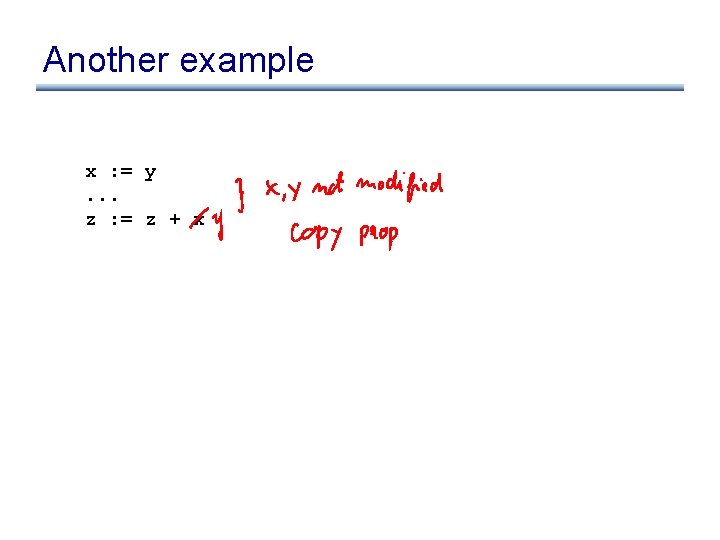
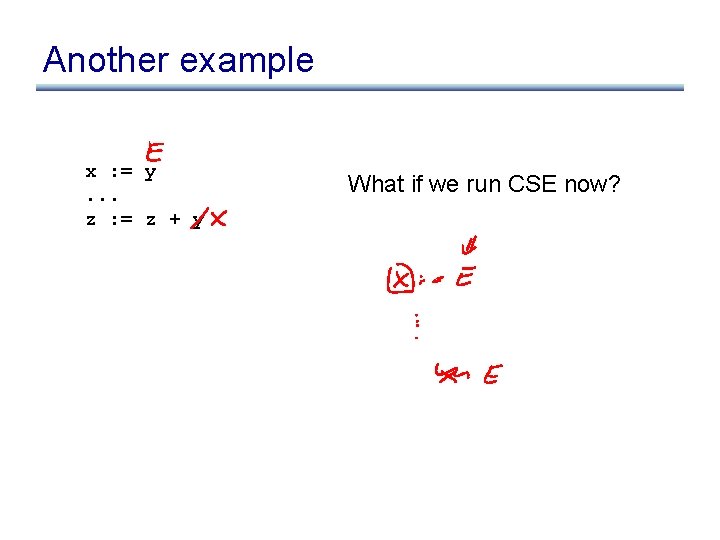
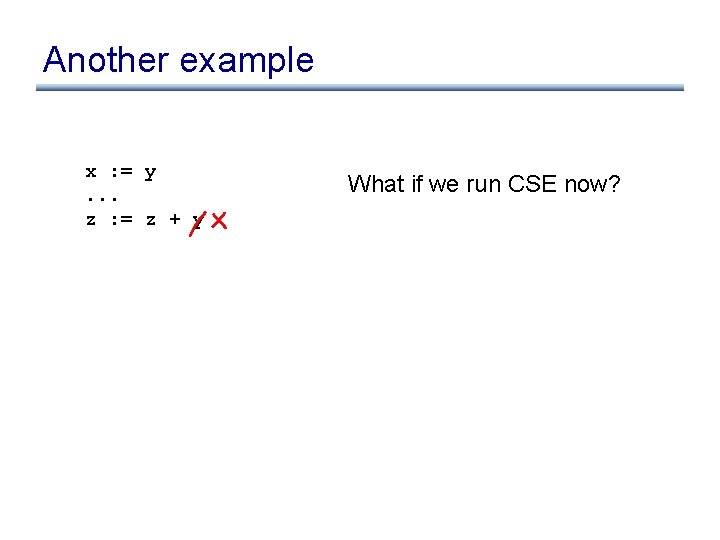
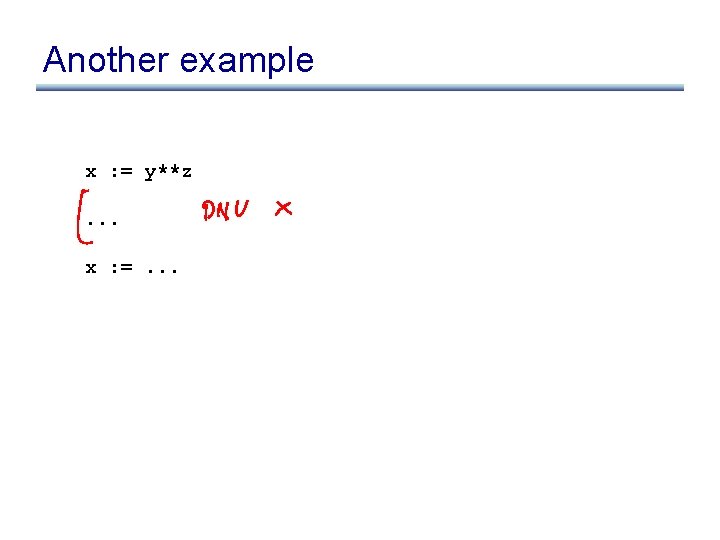
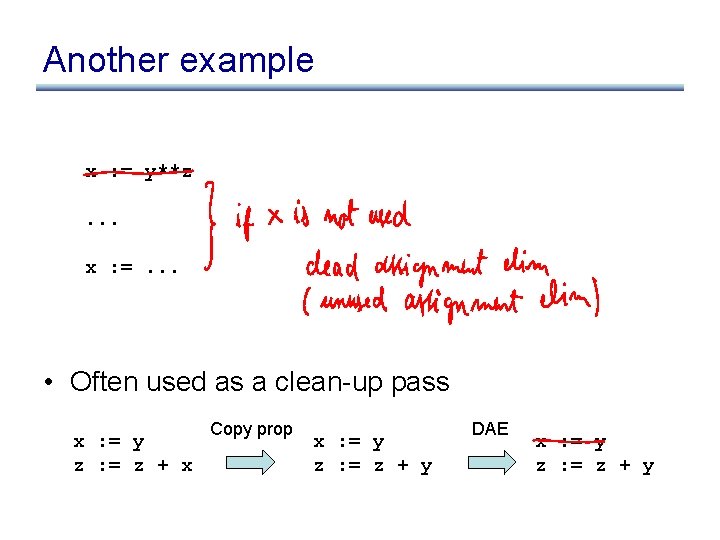
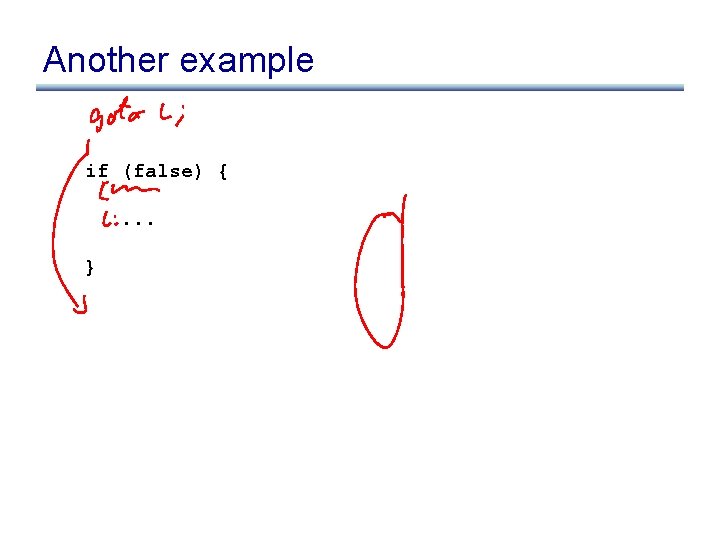
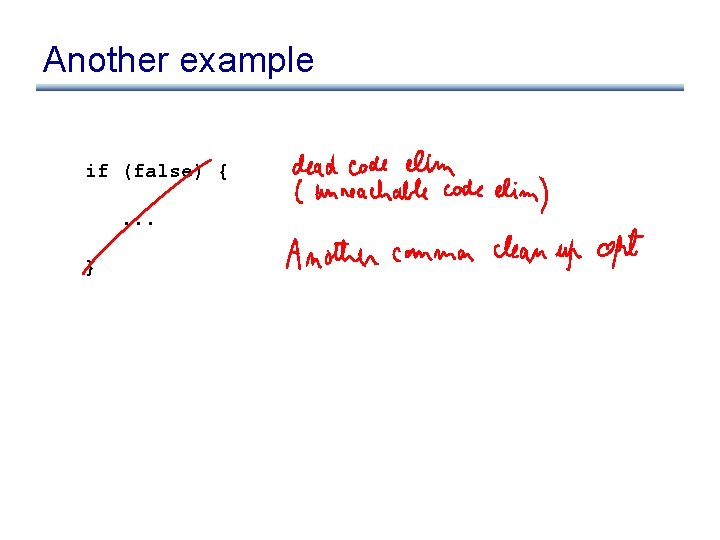
![Another example • In Java: a = new int [10]; for (index = 0; Another example • In Java: a = new int [10]; for (index = 0;](https://slidetodoc.com/presentation_image_h2/8e4865a74e5da4a2f34c9aa2b853d8e2/image-31.jpg)
![Another example • In “lowered” Java: a = new int [10]; for (index = Another example • In “lowered” Java: a = new int [10]; for (index =](https://slidetodoc.com/presentation_image_h2/8e4865a74e5da4a2f34c9aa2b853d8e2/image-32.jpg)
![Another example • In “lowered” Java: a = new int [10]; for (index = Another example • In “lowered” Java: a = new int [10]; for (index =](https://slidetodoc.com/presentation_image_h2/8e4865a74e5da4a2f34c9aa2b853d8e2/image-33.jpg)
- Slides: 33
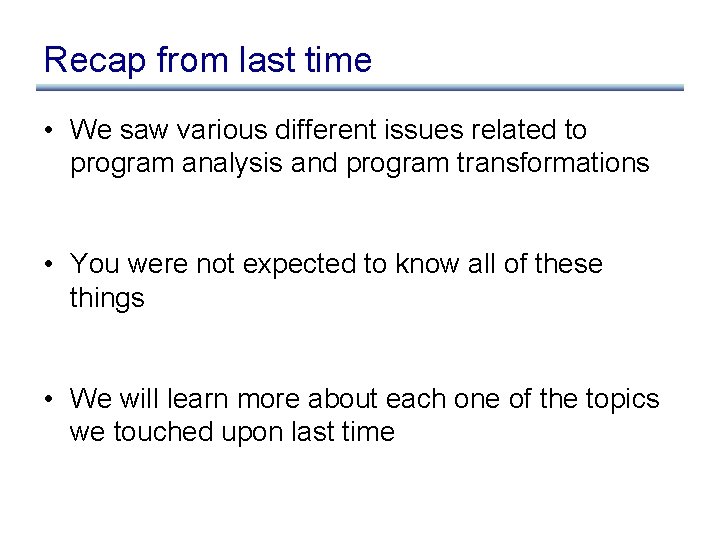
Recap from last time • We saw various different issues related to program analysis and program transformations • You were not expected to know all of these things • We will learn more about each one of the topics we touched upon last time
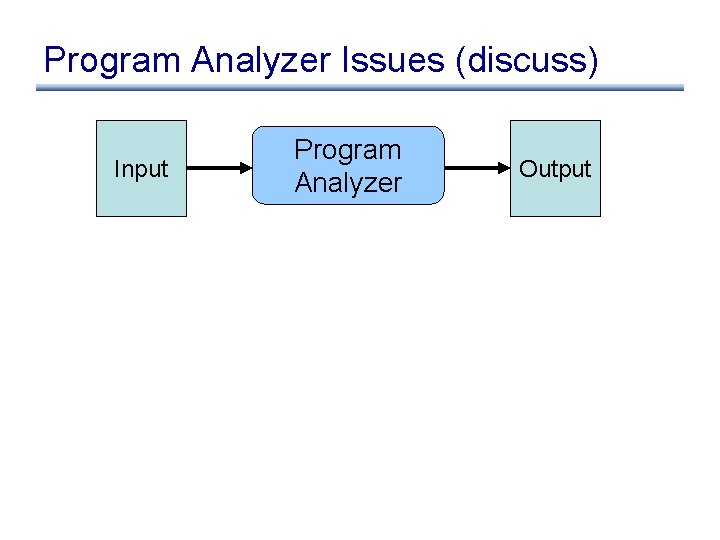
Program Analyzer Issues (discuss) Input Program Analyzer Output
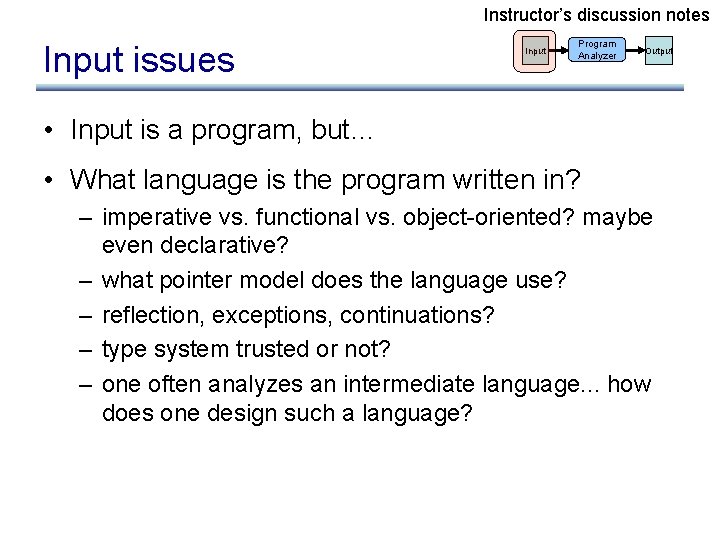
Instructor’s discussion notes Input issues Input Program Analyzer Output • Input is a program, but… • What language is the program written in? – imperative vs. functional vs. object-oriented? maybe even declarative? – what pointer model does the language use? – reflection, exceptions, continuations? – type system trusted or not? – one often analyzes an intermediate language. . . how does one design such a language?
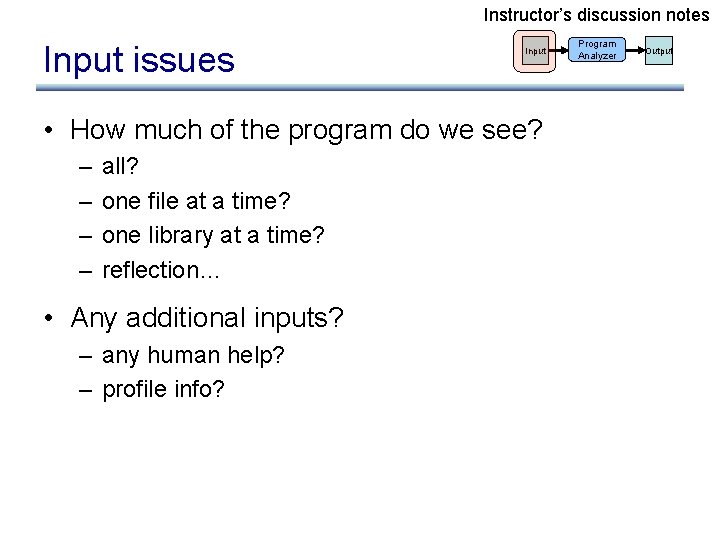
Instructor’s discussion notes Input issues Input • How much of the program do we see? – – all? one file at a time? one library at a time? reflection… • Any additional inputs? – any human help? – profile info? Program Analyzer Output
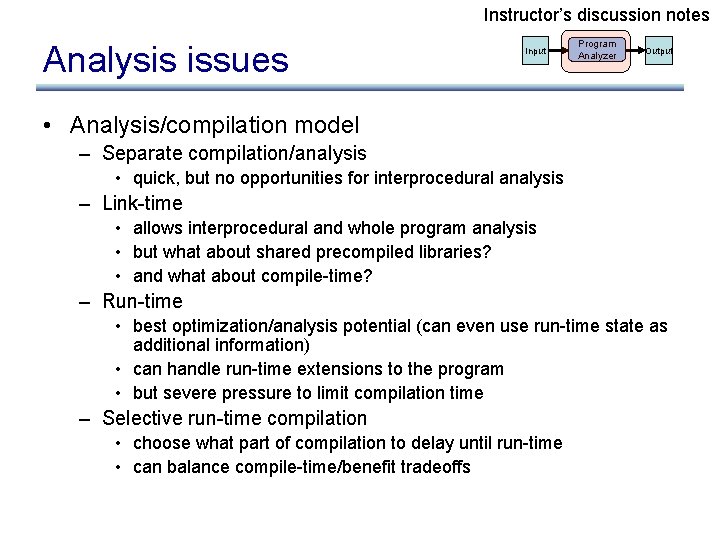
Instructor’s discussion notes Analysis issues Input Program Analyzer Output • Analysis/compilation model – Separate compilation/analysis • quick, but no opportunities for interprocedural analysis – Link-time • allows interprocedural and whole program analysis • but what about shared precompiled libraries? • and what about compile-time? – Run-time • best optimization/analysis potential (can even use run-time state as additional information) • can handle run-time extensions to the program • but severe pressure to limit compilation time – Selective run-time compilation • choose what part of compilation to delay until run-time • can balance compile-time/benefit tradeoffs
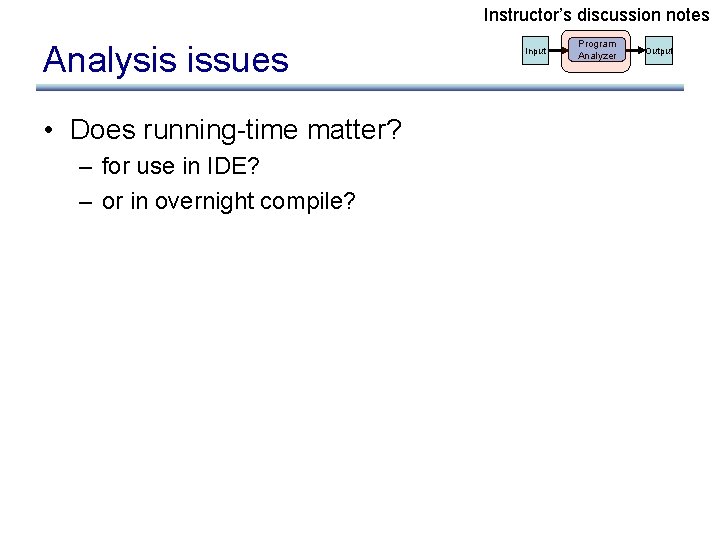
Instructor’s discussion notes Analysis issues • Does running-time matter? – for use in IDE? – or in overnight compile? Input Program Analyzer Output
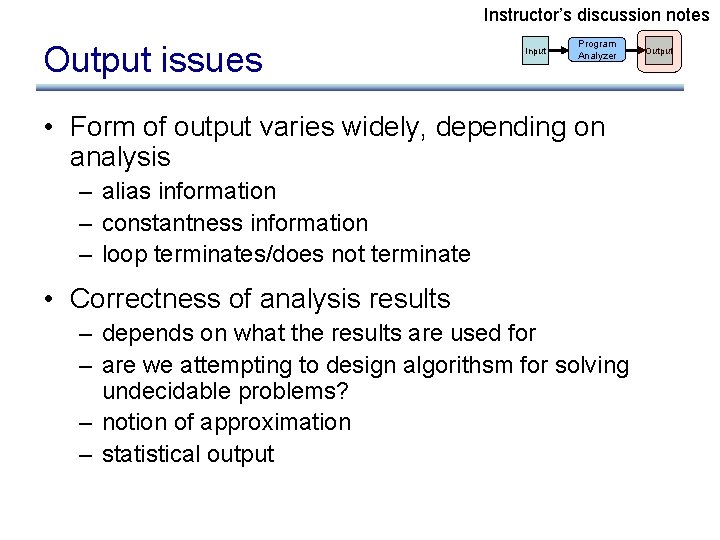
Instructor’s discussion notes Output issues Input Program Analyzer • Form of output varies widely, depending on analysis – alias information – constantness information – loop terminates/does not terminate • Correctness of analysis results – depends on what the results are used for – are we attempting to design algorithsm for solving undecidable problems? – notion of approximation – statistical output Output
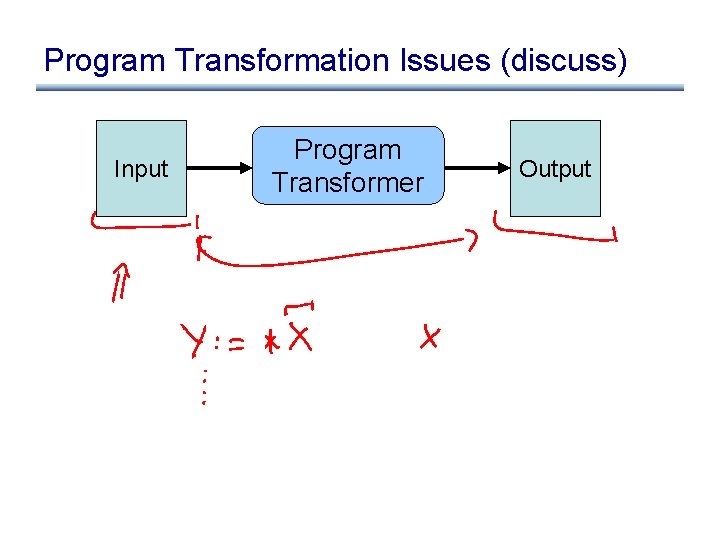
Program Transformation Issues (discuss) Input Program Transformer Output
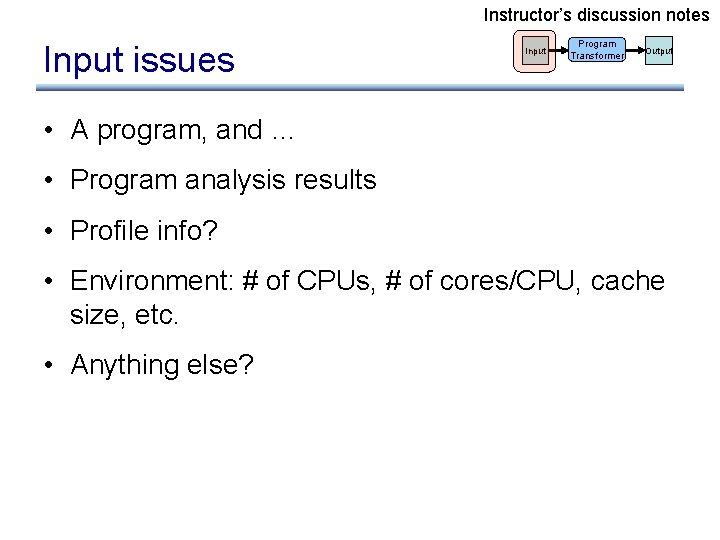
Instructor’s discussion notes Input issues Input Program Transformer Output • A program, and … • Program analysis results • Profile info? • Environment: # of CPUs, # of cores/CPU, cache size, etc. • Anything else?
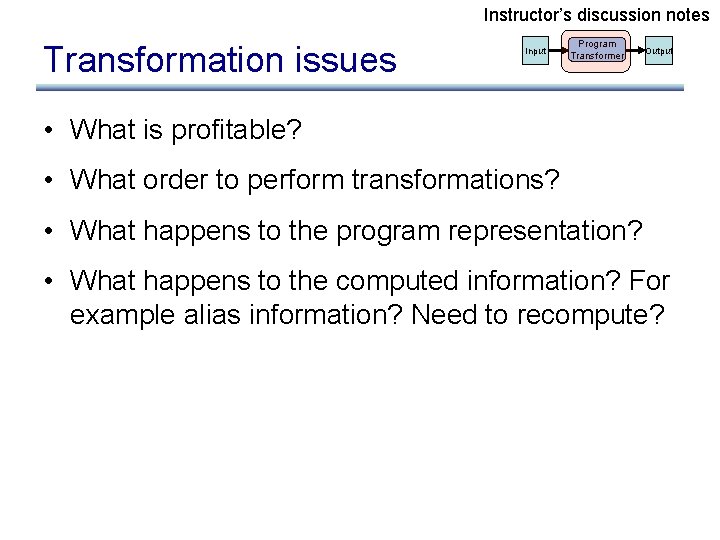
Instructor’s discussion notes Transformation issues Input Program Transformer Output • What is profitable? • What order to perform transformations? • What happens to the program representation? • What happens to the computed information? For example alias information? Need to recompute?
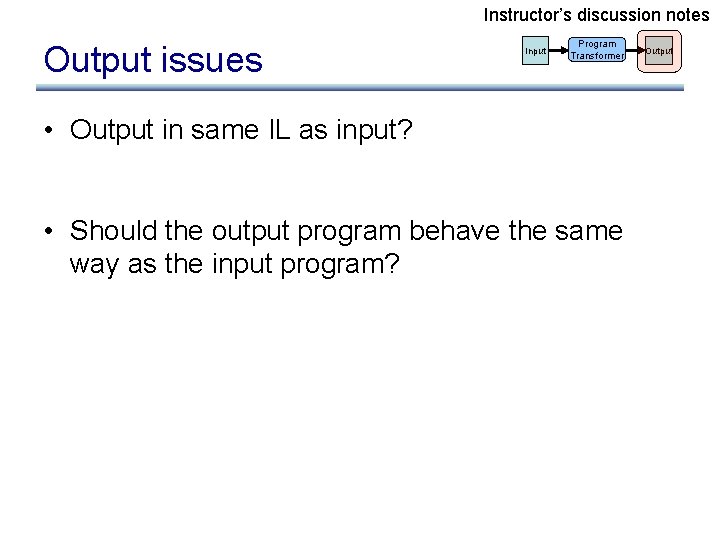
Instructor’s discussion notes Output issues Input Program Transformer • Output in same IL as input? • Should the output program behave the same way as the input program? Output
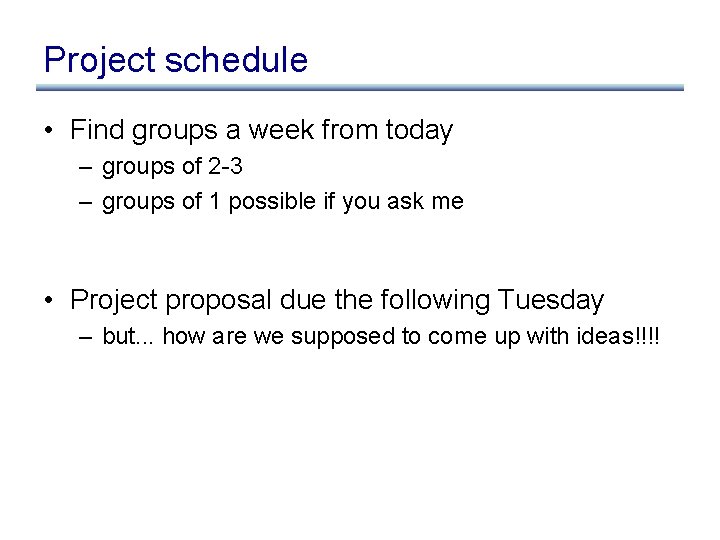
Project schedule • Find groups a week from today – groups of 2 -3 – groups of 1 possible if you ask me • Project proposal due the following Tuesday – but. . . how are we supposed to come up with ideas!!!!
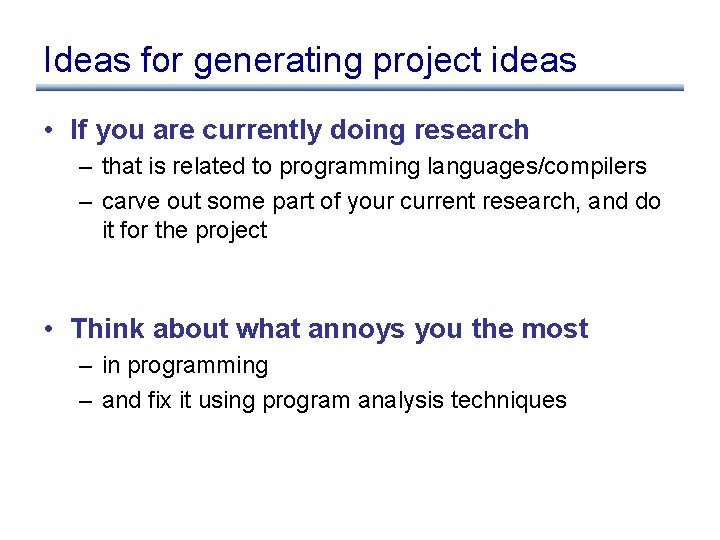
Ideas for generating project ideas • If you are currently doing research – that is related to programming languages/compilers – carve out some part of your current research, and do it for the project • Think about what annoys you the most – in programming – and fix it using program analysis techniques
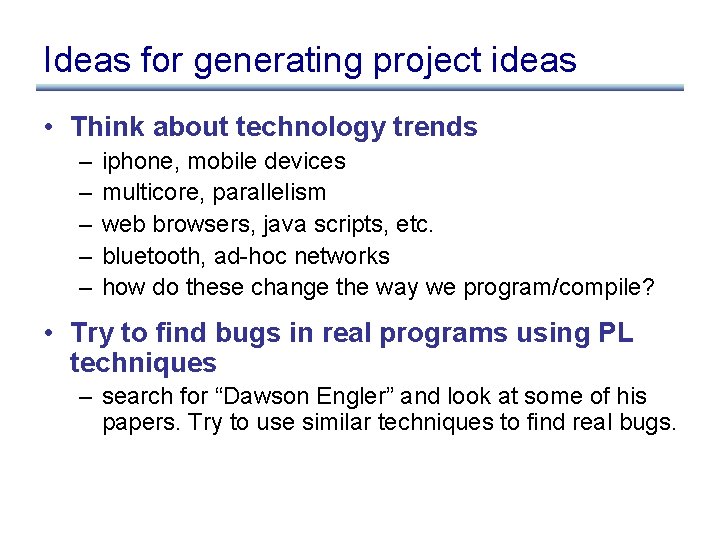
Ideas for generating project ideas • Think about technology trends – – – iphone, mobile devices multicore, parallelism web browsers, java scripts, etc. bluetooth, ad-hoc networks how do these change the way we program/compile? • Try to find bugs in real programs using PL techniques – search for “Dawson Engler” and look at some of his papers. Try to use similar techniques to find real bugs.
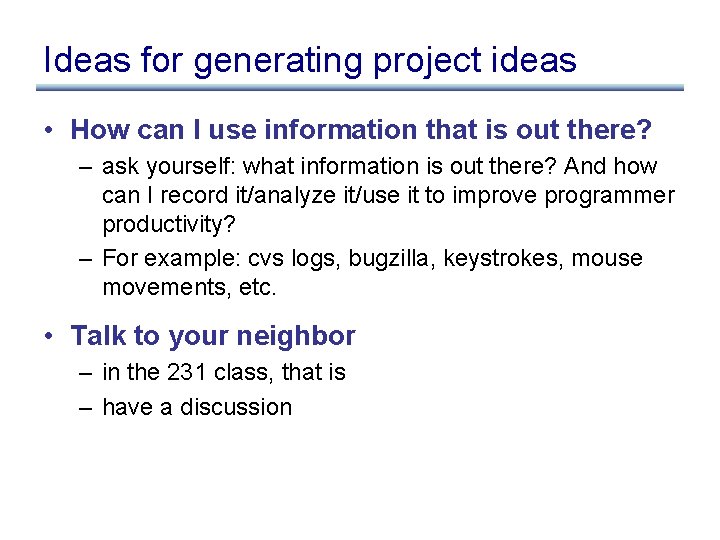
Ideas for generating project ideas • How can I use information that is out there? – ask yourself: what information is out there? And how can I record it/analyze it/use it to improve programmer productivity? – For example: cvs logs, bugzilla, keystrokes, mouse movements, etc. • Talk to your neighbor – in the 231 class, that is – have a discussion
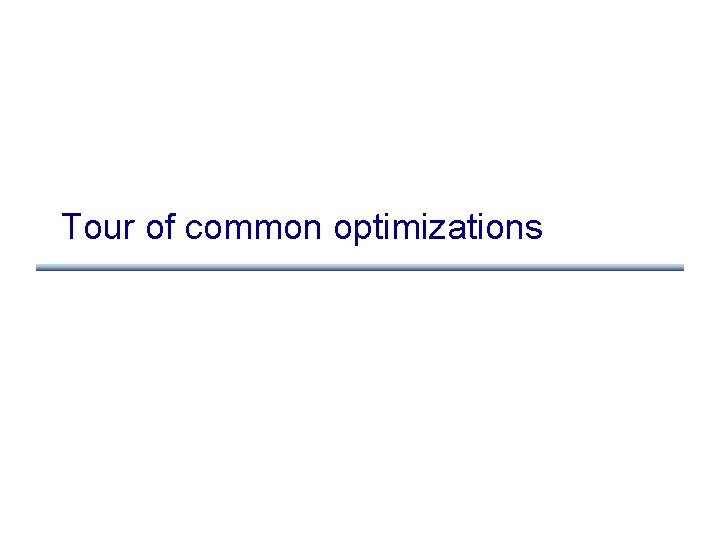
Tour of common optimizations
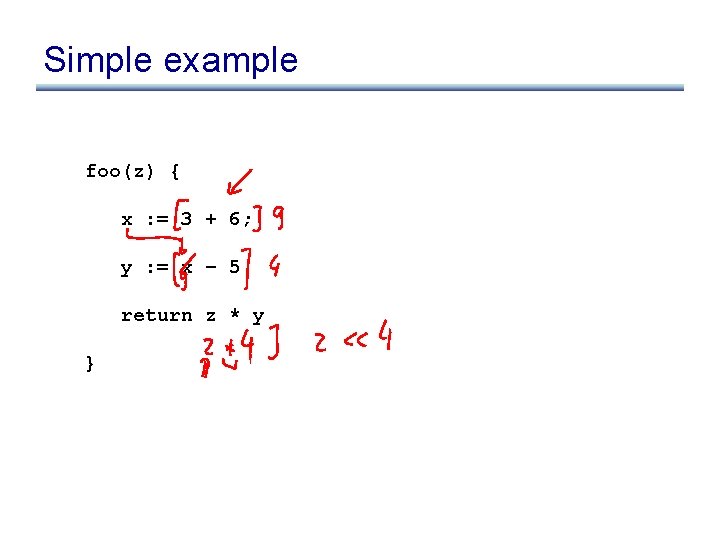
Simple example foo(z) { x : = 3 + 6; y : = x – 5 return z * y }
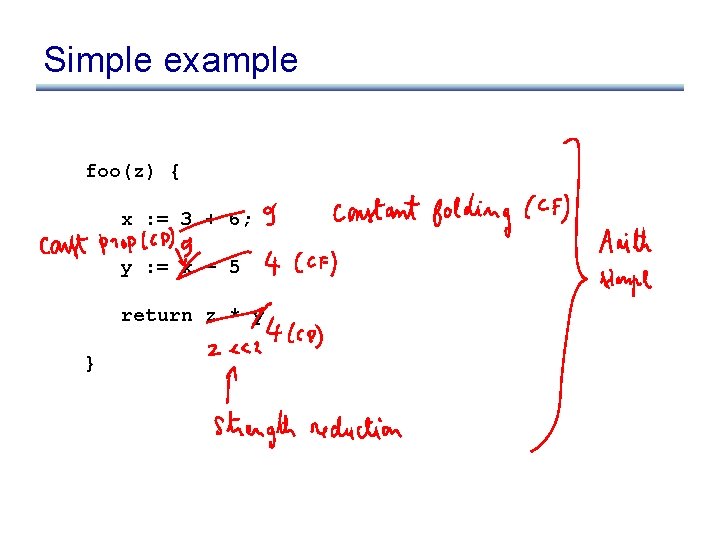
Simple example foo(z) { x : = 3 + 6; y : = x – 5 return z * y }
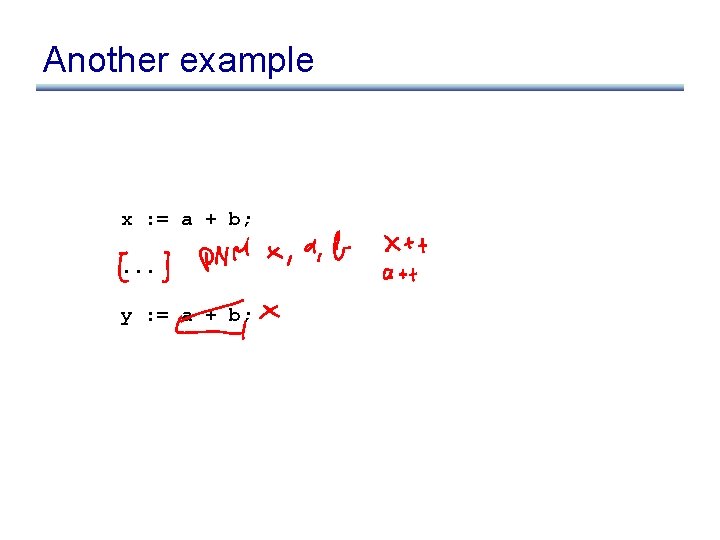
Another example x : = a + b; . . . y : = a + b;
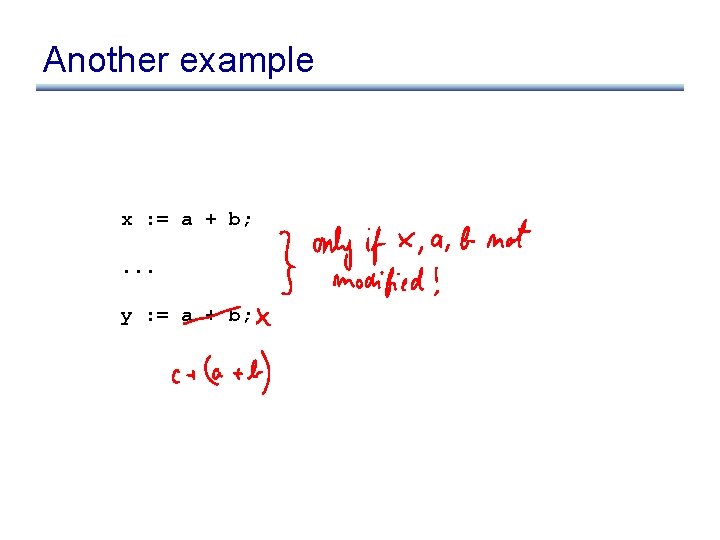
Another example x : = a + b; . . . y : = a + b;
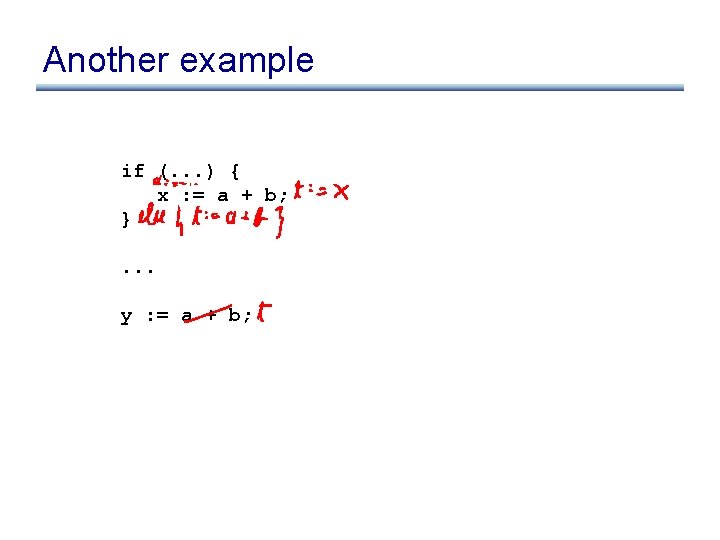
Another example if (. . . ) { x : = a + b; }. . . y : = a + b;
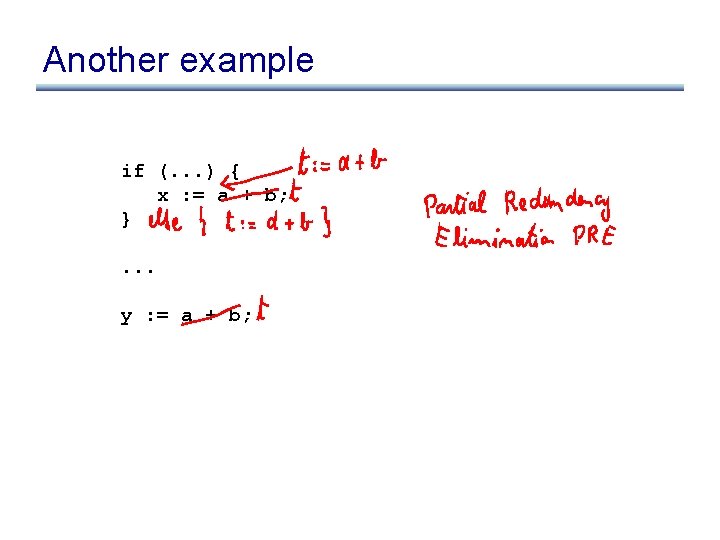
Another example if (. . . ) { x : = a + b; }. . . y : = a + b;
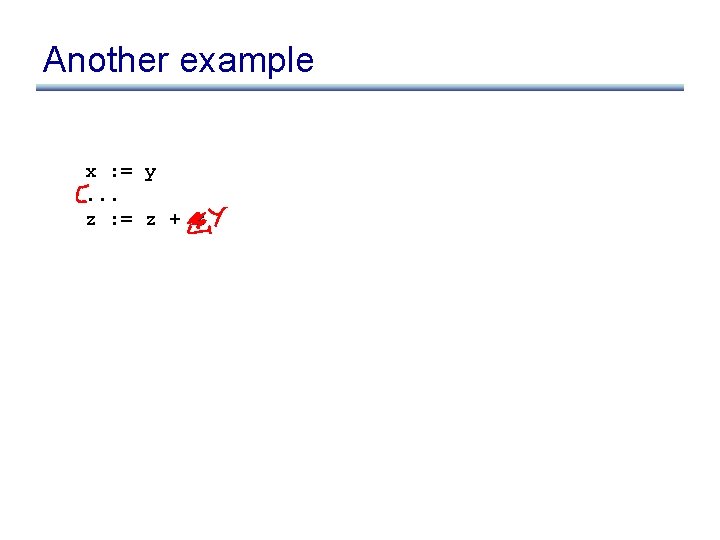
Another example x : = y. . . z : = z + x
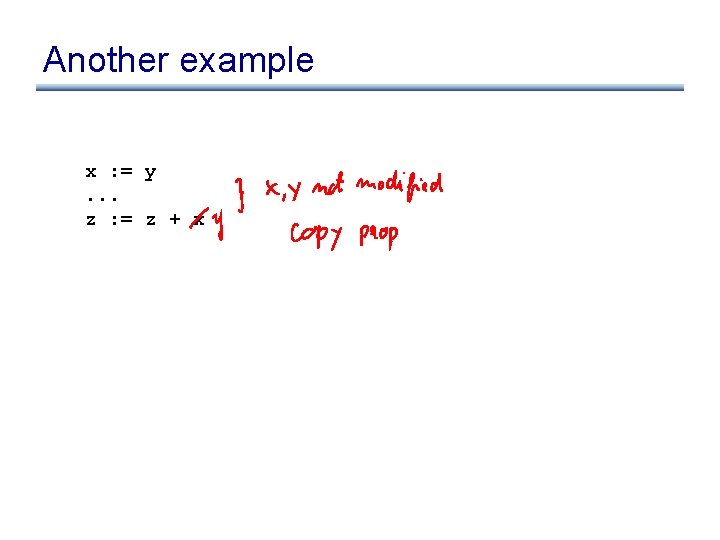
Another example x : = y. . . z : = z + x
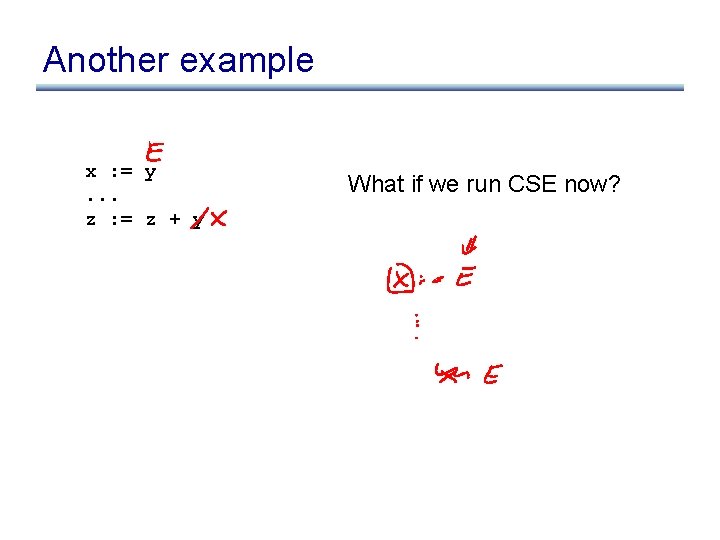
Another example x : = y. . . z : = z + y What if we run CSE now?
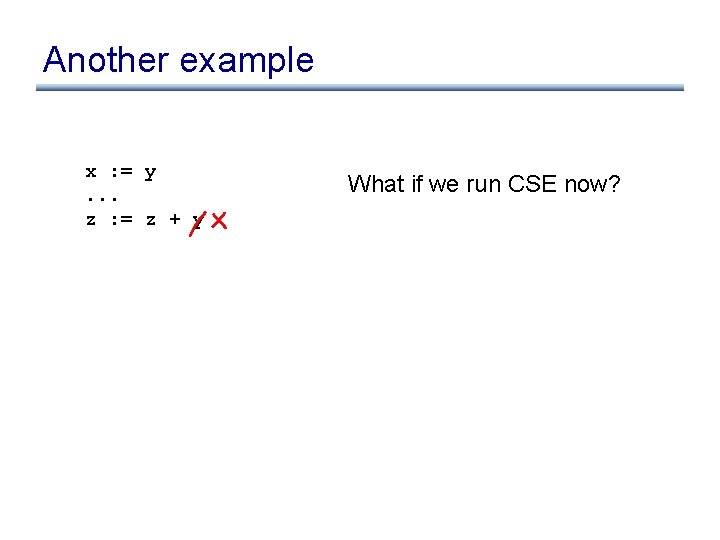
Another example x : = y. . . z : = z + y What if we run CSE now?
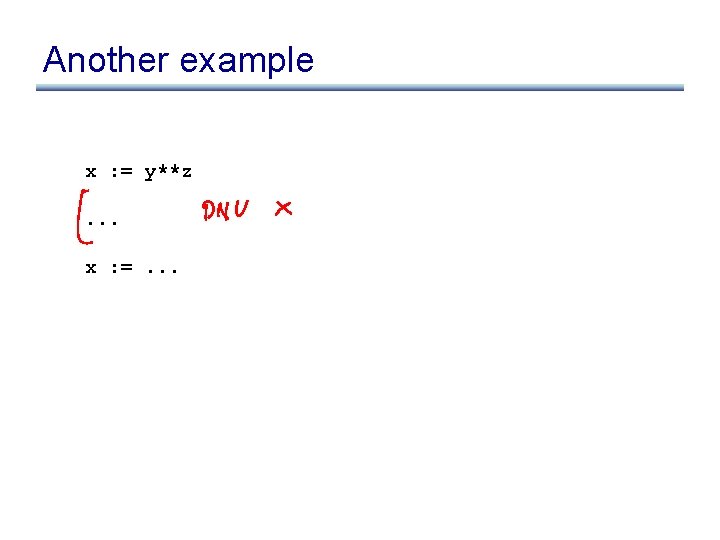
Another example x : = y**z. . . x : =. . .
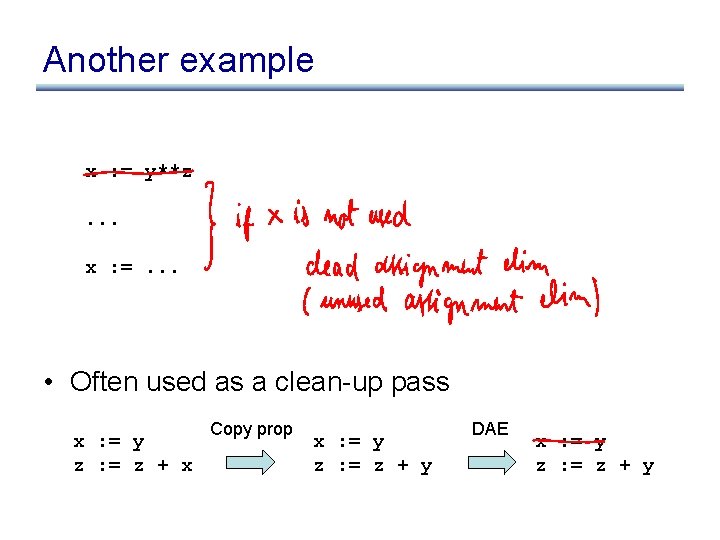
Another example x : = y**z. . . x : =. . . • Often used as a clean-up pass x : = y z : = z + x Copy prop x : = y z : = z + y DAE x : = y z : = z + y
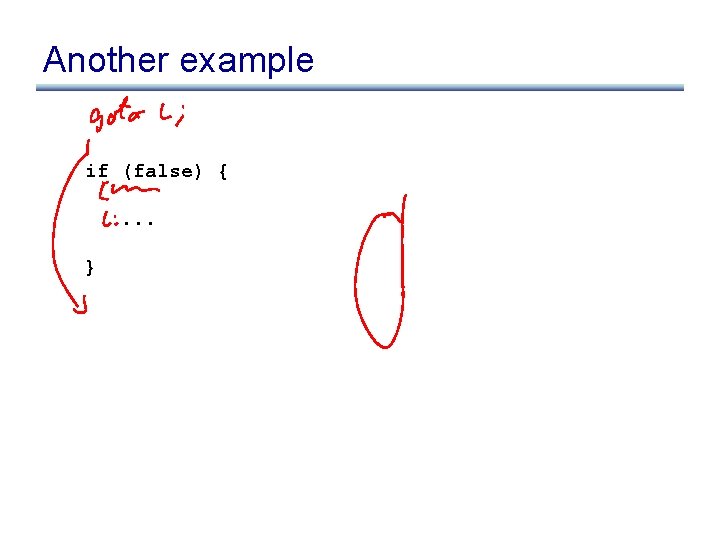
Another example if (false) {. . . }
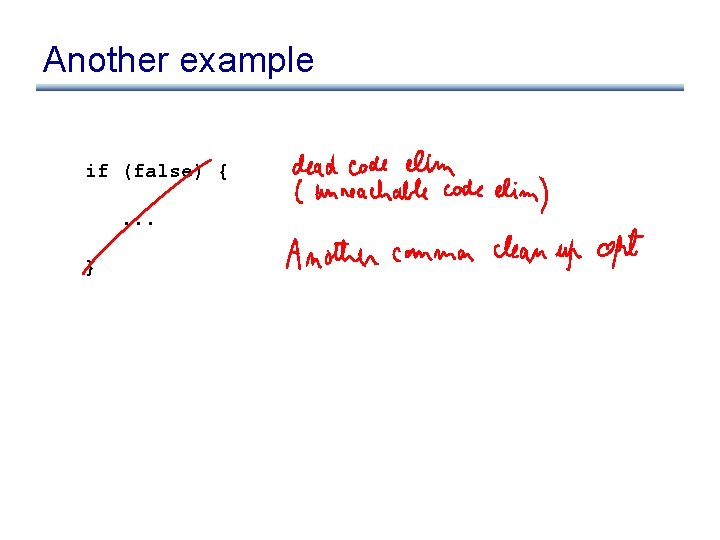
Another example if (false) {. . . }
![Another example In Java a new int 10 for index 0 Another example • In Java: a = new int [10]; for (index = 0;](https://slidetodoc.com/presentation_image_h2/8e4865a74e5da4a2f34c9aa2b853d8e2/image-31.jpg)
Another example • In Java: a = new int [10]; for (index = 0; index < 10; index ++) { a[index] = 100; }
![Another example In lowered Java a new int 10 for index Another example • In “lowered” Java: a = new int [10]; for (index =](https://slidetodoc.com/presentation_image_h2/8e4865a74e5da4a2f34c9aa2b853d8e2/image-32.jpg)
Another example • In “lowered” Java: a = new int [10]; for (index = 0; index < 10; index ++) { if (index < 0 || index >= a. length()) { throw Out. Of. Bounds. Exception; } a[index] = 0; }
![Another example In lowered Java a new int 10 for index Another example • In “lowered” Java: a = new int [10]; for (index =](https://slidetodoc.com/presentation_image_h2/8e4865a74e5da4a2f34c9aa2b853d8e2/image-33.jpg)
Another example • In “lowered” Java: a = new int [10]; for (index = 0; index < 10; index ++) { if (index < 0 || index >= a. length()) { throw Out. Of. Bounds. Exception; } a[index] = 0; }