Queues ADT Queue head Elements are removed from
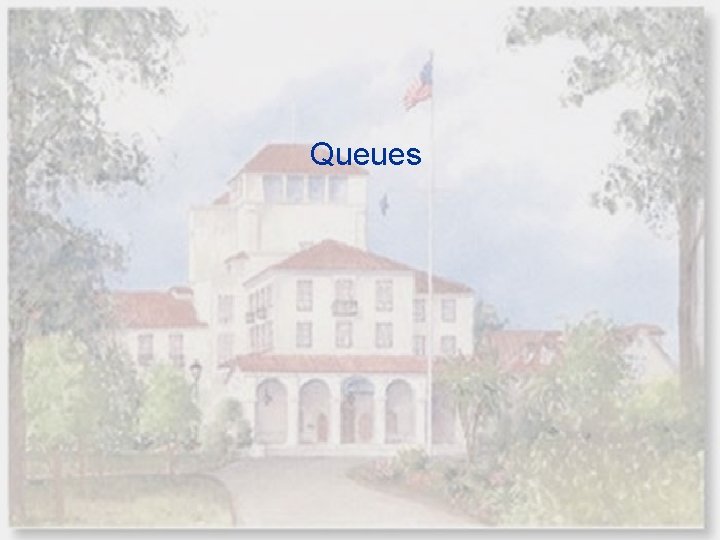
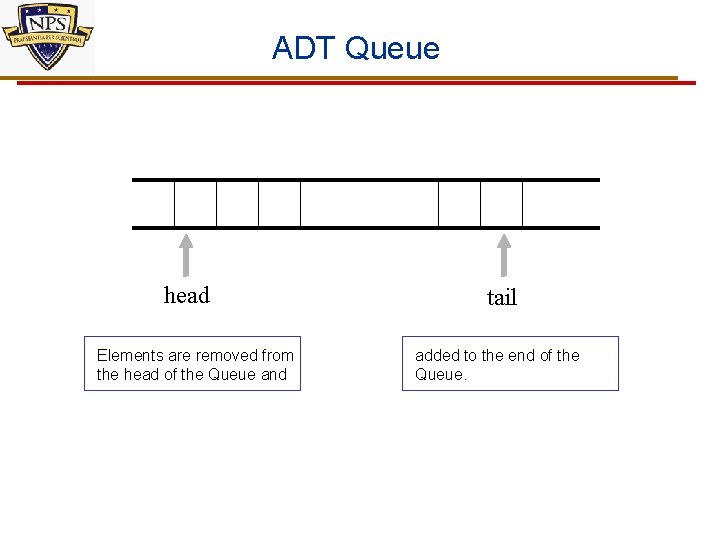
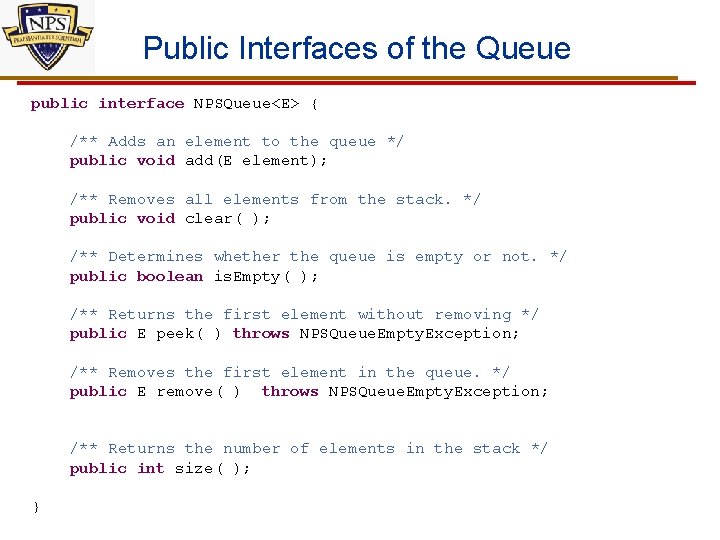
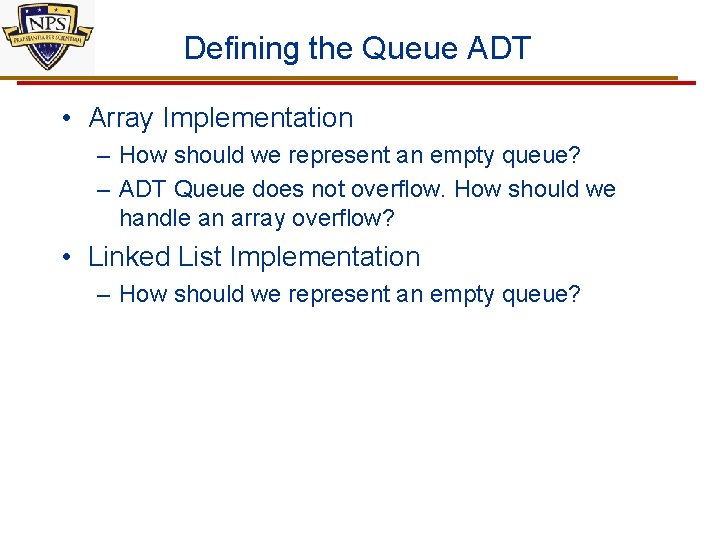
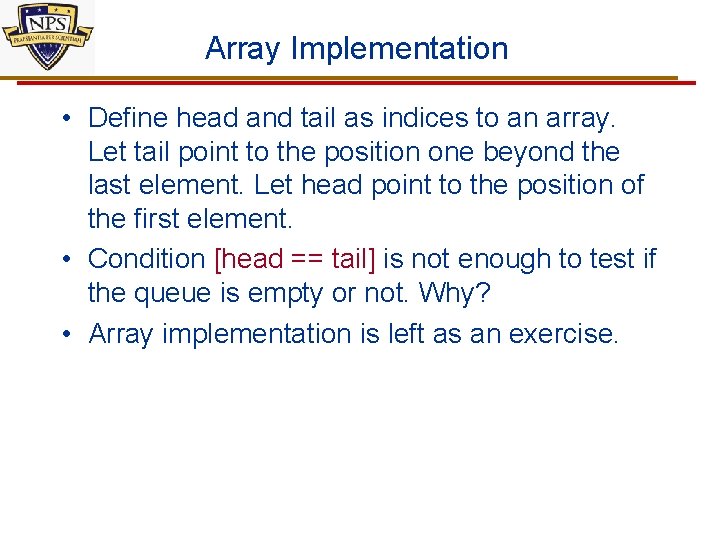
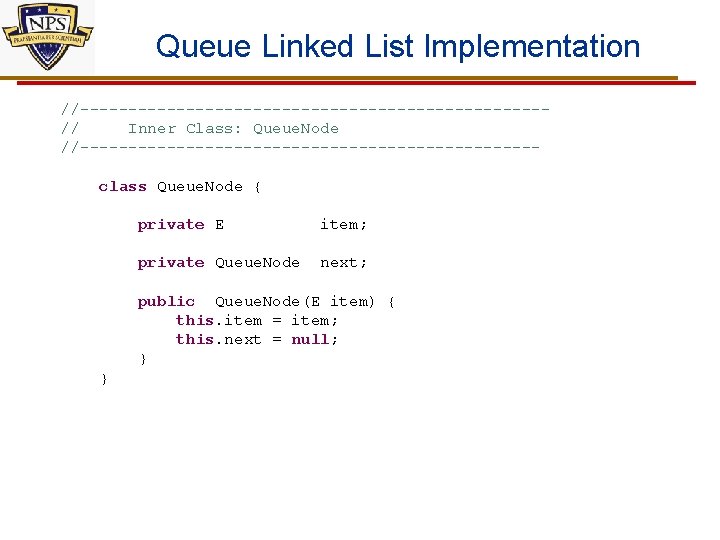
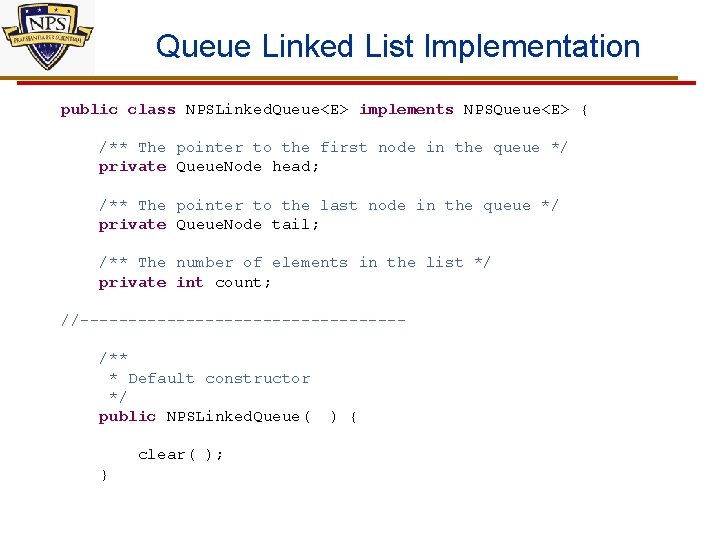
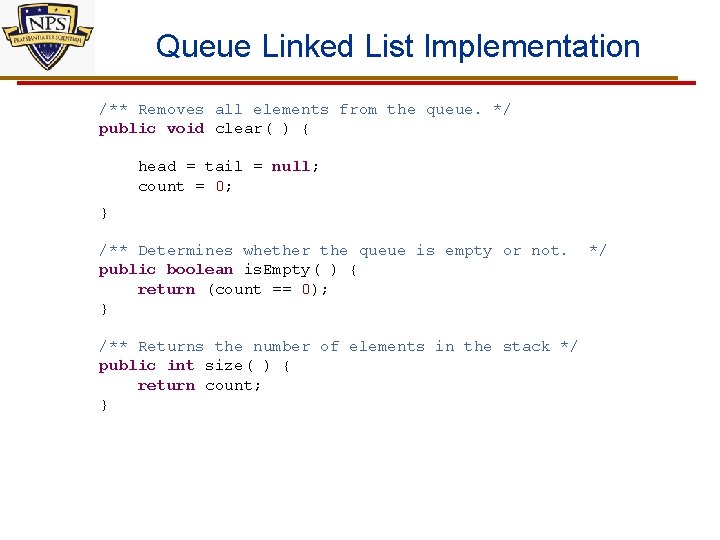
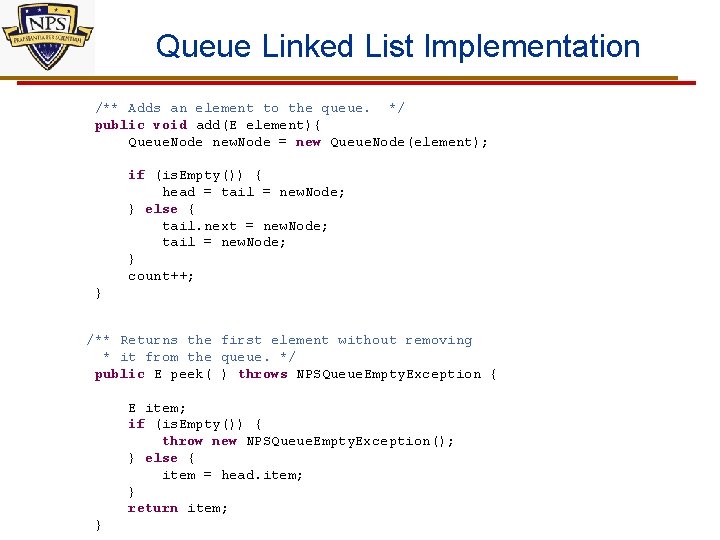
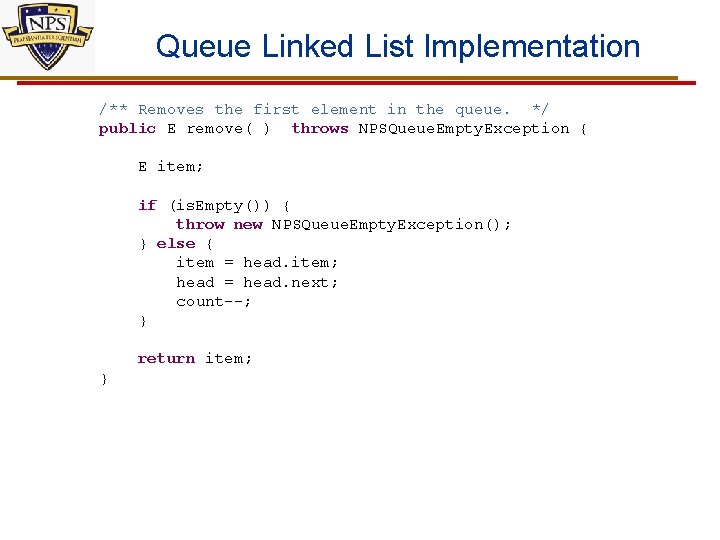
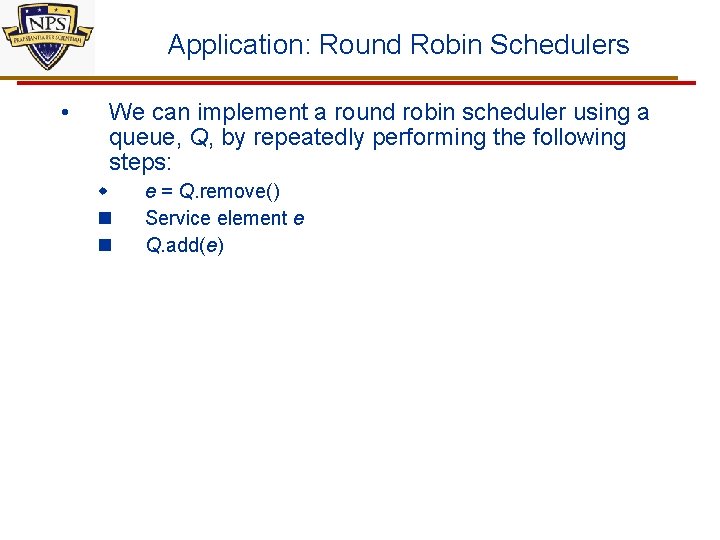
- Slides: 11
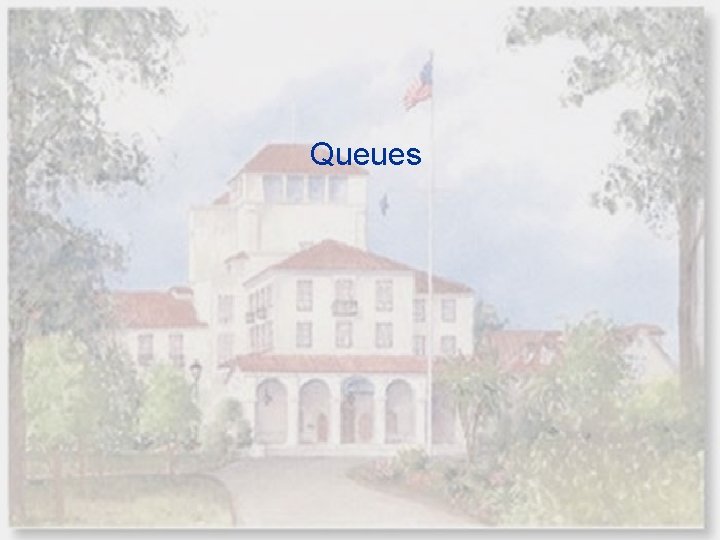
Queues
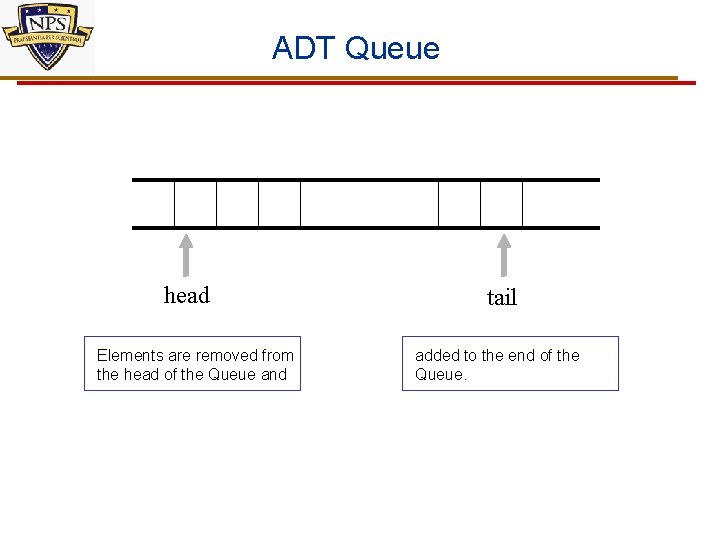
ADT Queue head Elements are removed from the head of the Queue and tail added to the end of the Queue.
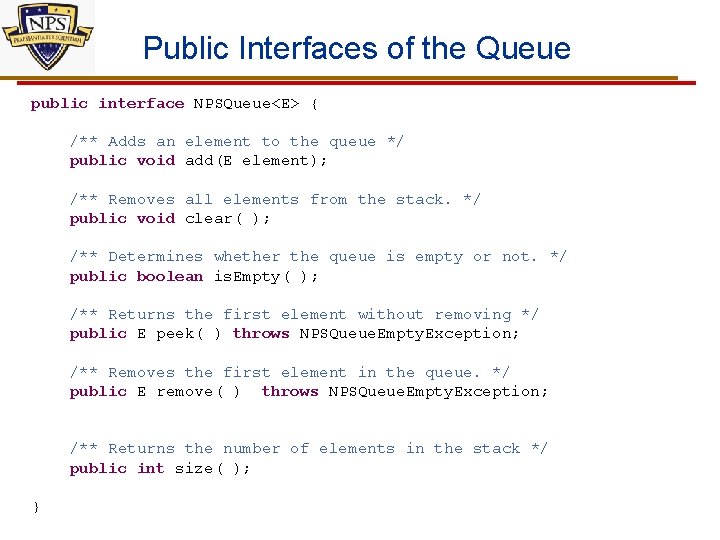
Public Interfaces of the Queue public interface NPSQueue<E> { /** Adds an element to the queue */ public void add(E element); /** Removes all elements from the stack. */ public void clear( ); /** Determines whether the queue is empty or not. */ public boolean is. Empty( ); /** Returns the first element without removing */ public E peek( ) throws NPSQueue. Empty. Exception; /** Removes the first element in the queue. */ public E remove( ) throws NPSQueue. Empty. Exception; /** Returns the number of elements in the stack */ public int size( ); }
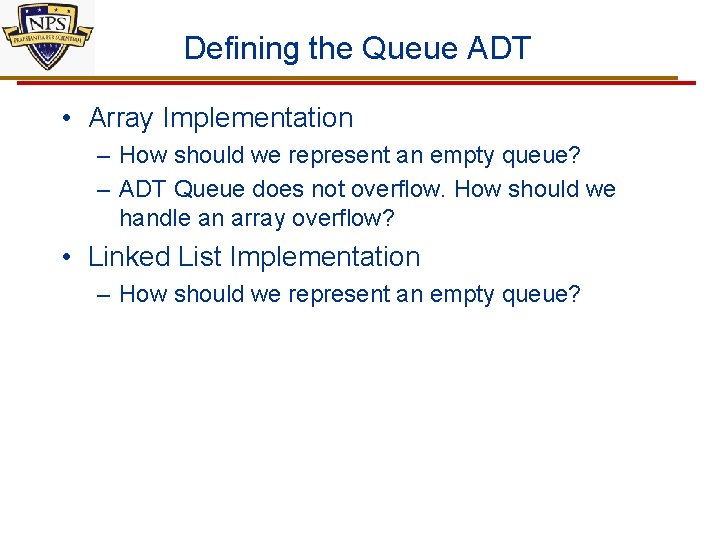
Defining the Queue ADT • Array Implementation – How should we represent an empty queue? – ADT Queue does not overflow. How should we handle an array overflow? • Linked List Implementation – How should we represent an empty queue?
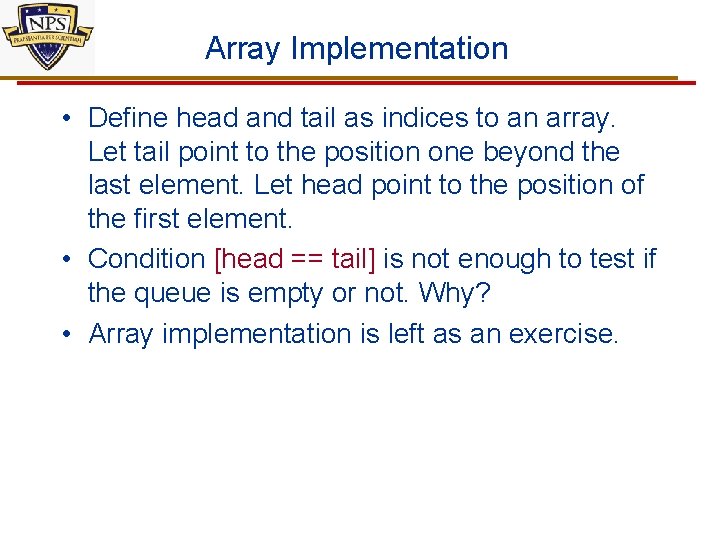
Array Implementation • Define head and tail as indices to an array. Let tail point to the position one beyond the last element. Let head point to the position of the first element. • Condition [head == tail] is not enough to test if the queue is empty or not. Why? • Array implementation is left as an exercise.
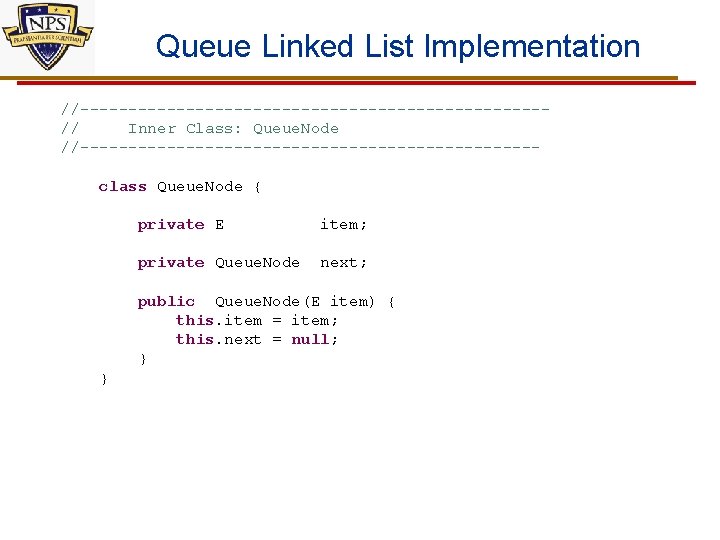
Queue Linked List Implementation //------------------------// Inner Class: Queue. Node //------------------------class Queue. Node { private E item; private Queue. Node next; public Queue. Node(E item) { this. item = item; this. next = null; } }
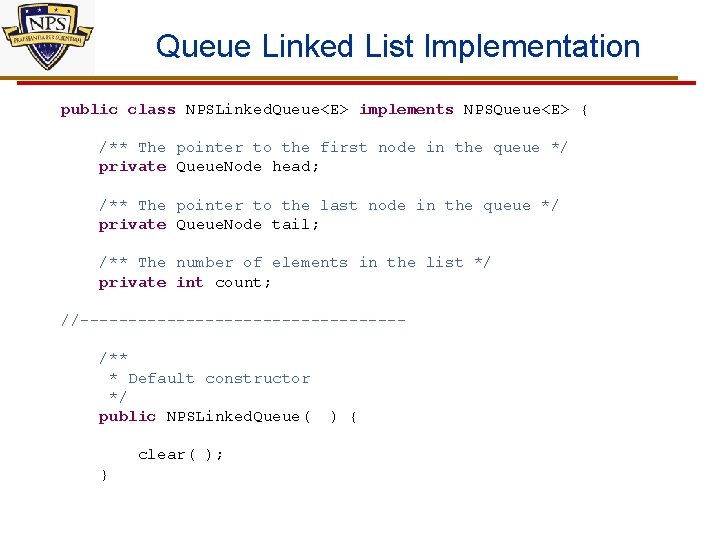
Queue Linked List Implementation public class NPSLinked. Queue<E> implements NPSQueue<E> { /** The pointer to the first node in the queue */ private Queue. Node head; /** The pointer to the last node in the queue */ private Queue. Node tail; /** The number of elements in the list */ private int count; //-----------------/** * Default constructor */ public NPSLinked. Queue( clear( ); } ) {
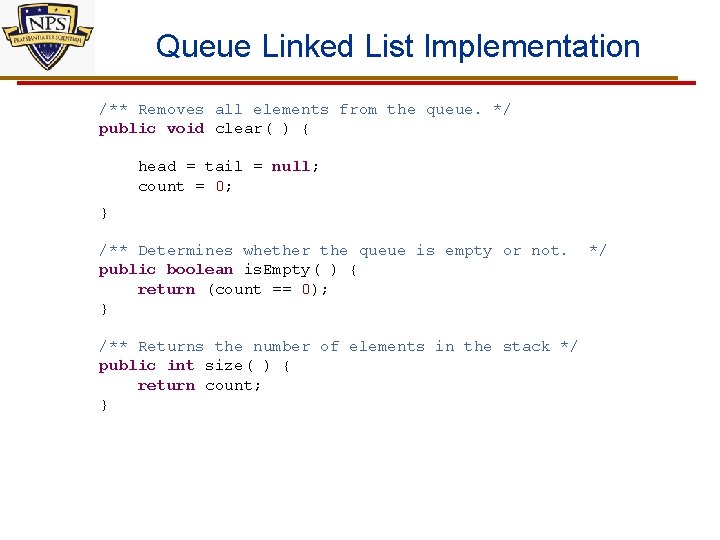
Queue Linked List Implementation /** Removes all elements from the queue. */ public void clear( ) { head = tail = null; count = 0; } /** Determines whether the queue is empty or not. public boolean is. Empty( ) { return (count == 0); } /** Returns the number of elements in the stack */ public int size( ) { return count; } */
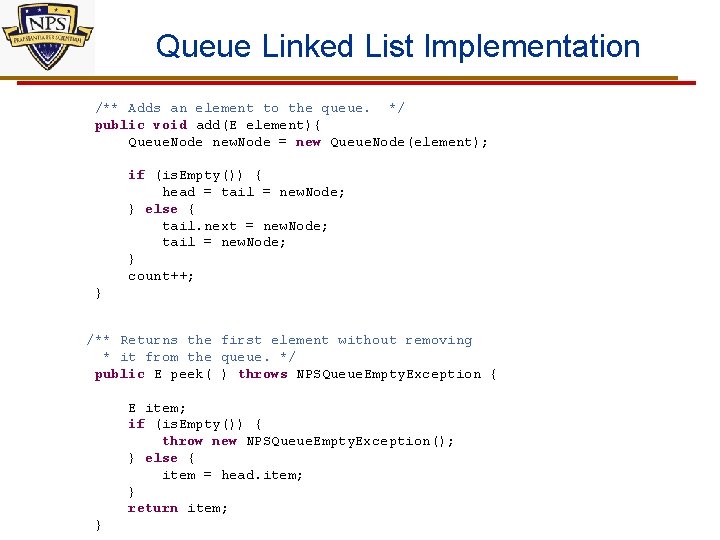
Queue Linked List Implementation /** Adds an element to the queue. */ public void add(E element){ Queue. Node new. Node = new Queue. Node(element); if (is. Empty()) { head = tail = new. Node; } else { tail. next = new. Node; tail = new. Node; } count++; } /** Returns the first element without removing * it from the queue. */ public E peek( ) throws NPSQueue. Empty. Exception { E item; if (is. Empty()) { throw new NPSQueue. Empty. Exception(); } else { item = head. item; } return item; }
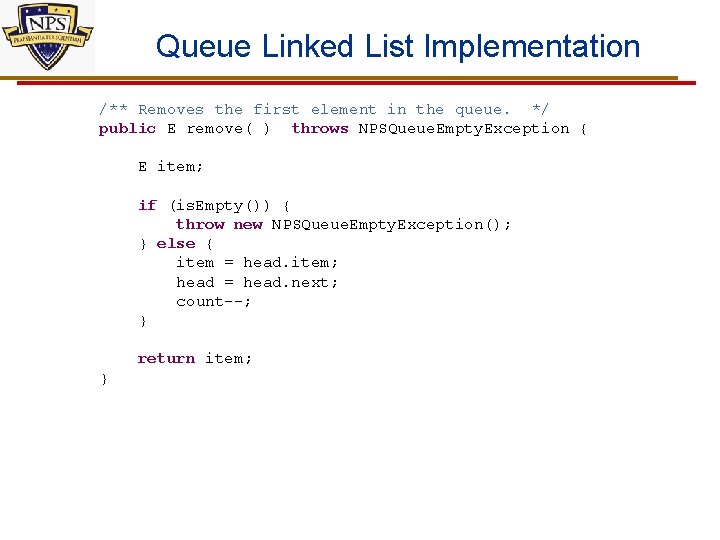
Queue Linked List Implementation /** Removes the first element in the queue. */ public E remove( ) throws NPSQueue. Empty. Exception { E item; if (is. Empty()) { throw new NPSQueue. Empty. Exception(); } else { item = head. item; head = head. next; count--; } return item; }
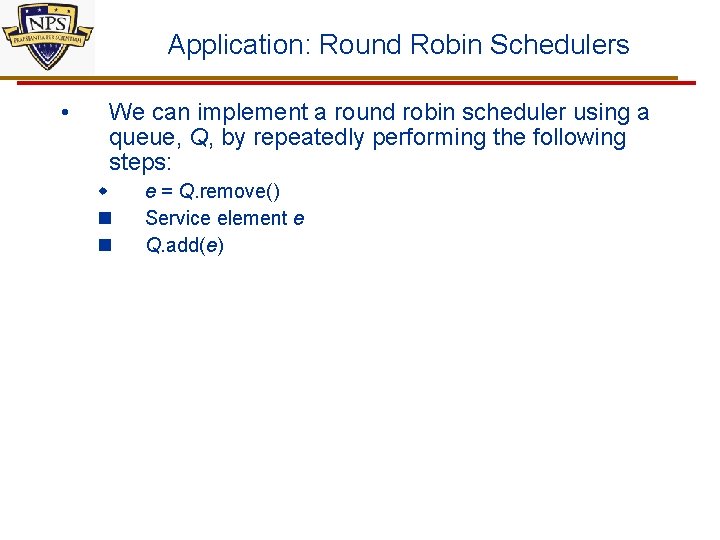
Application: Round Robin Schedulers • We can implement a round robin scheduler using a queue, Q, by repeatedly performing the following steps: e = Q. remove() Service element e Q. add(e)