Queues 1 Queues A queue is restricted list
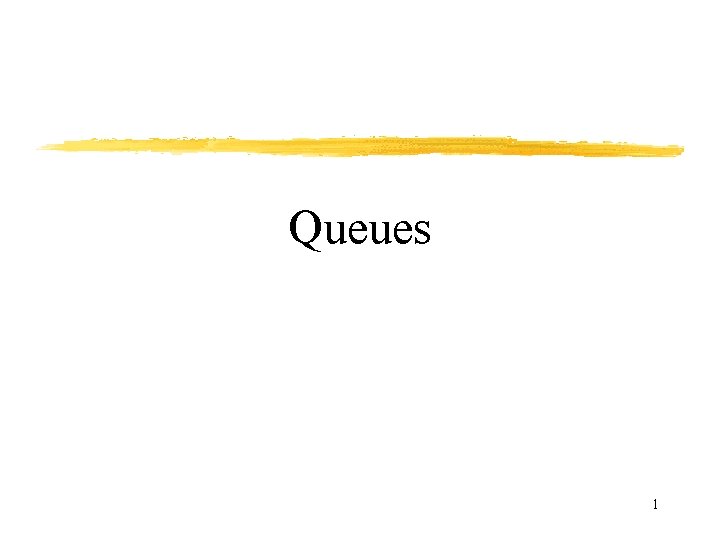
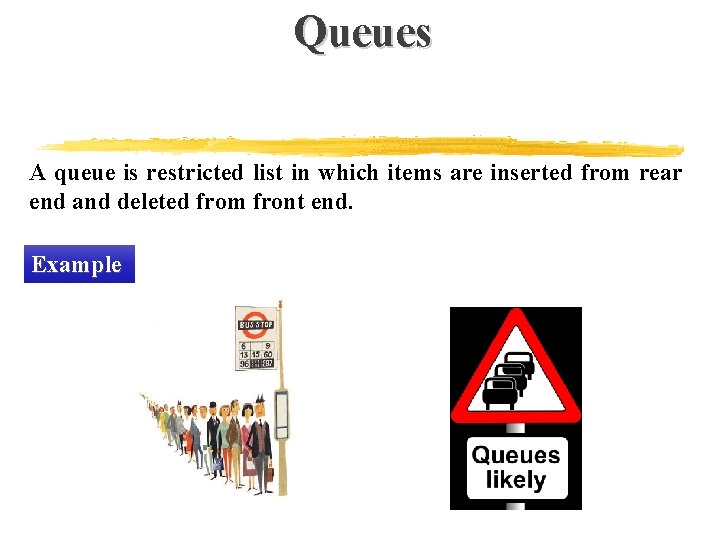
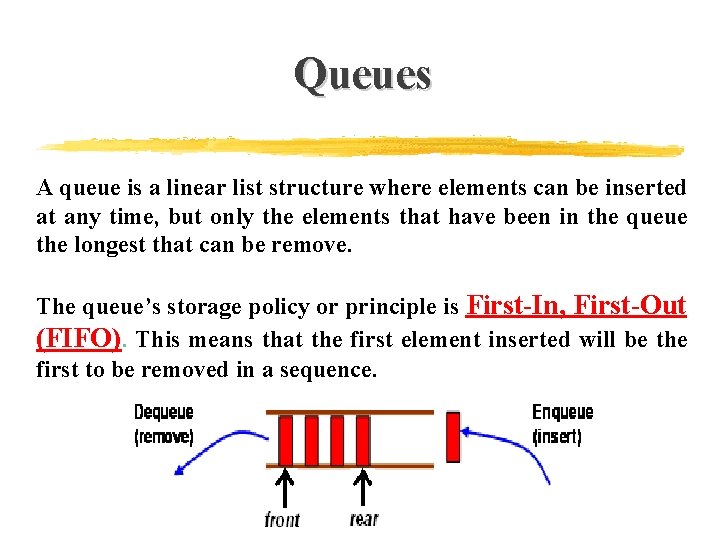
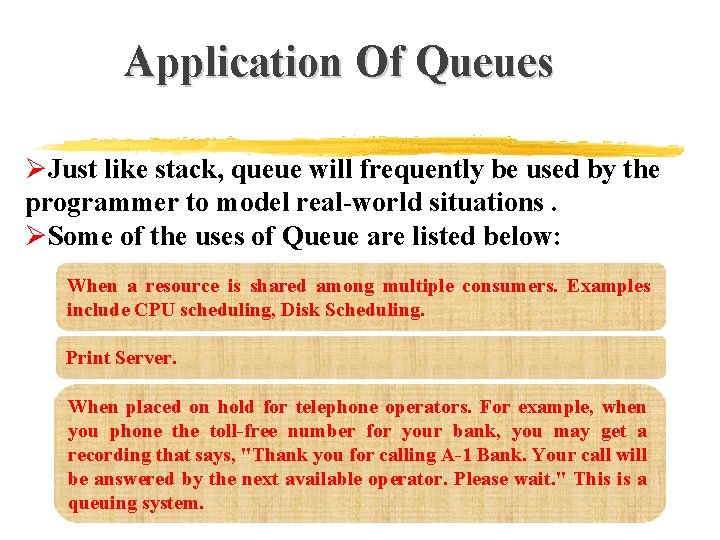
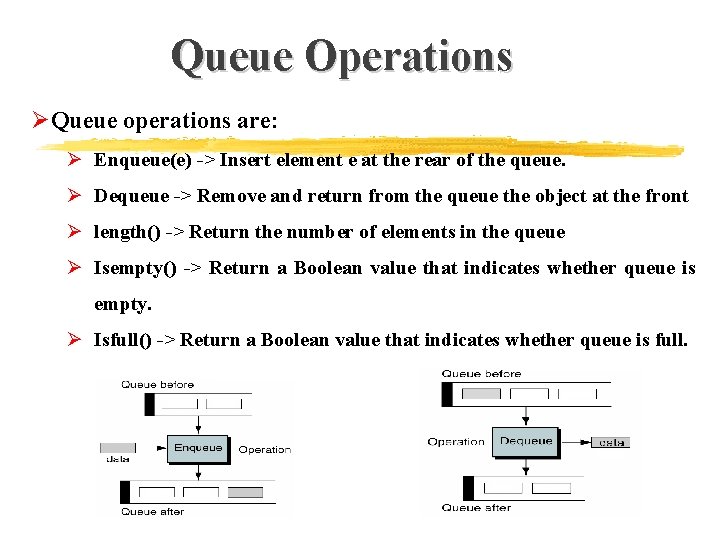
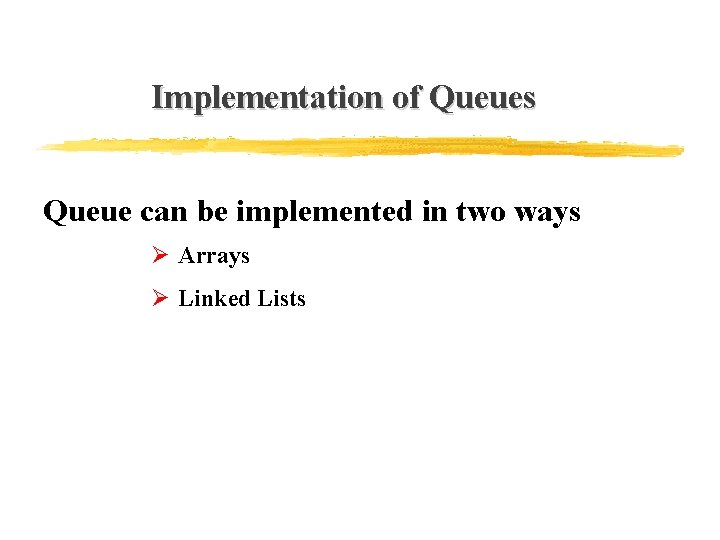
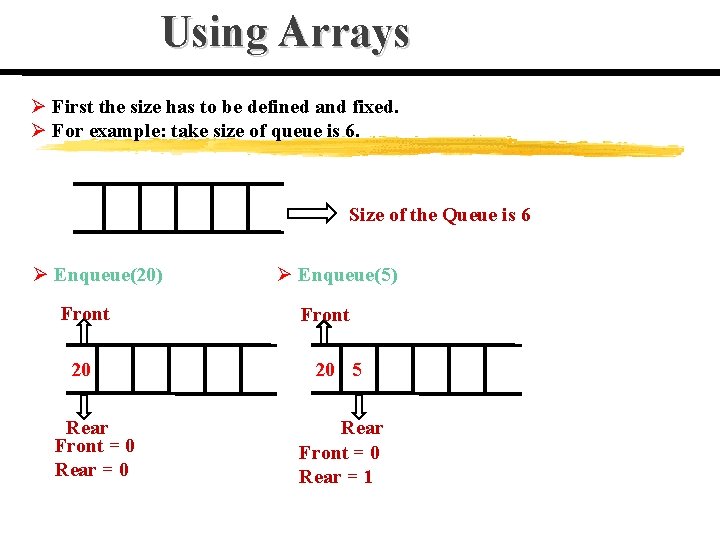
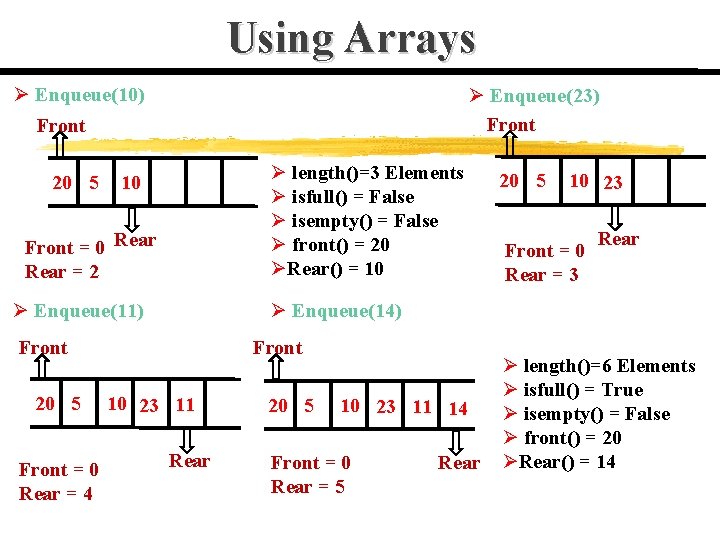
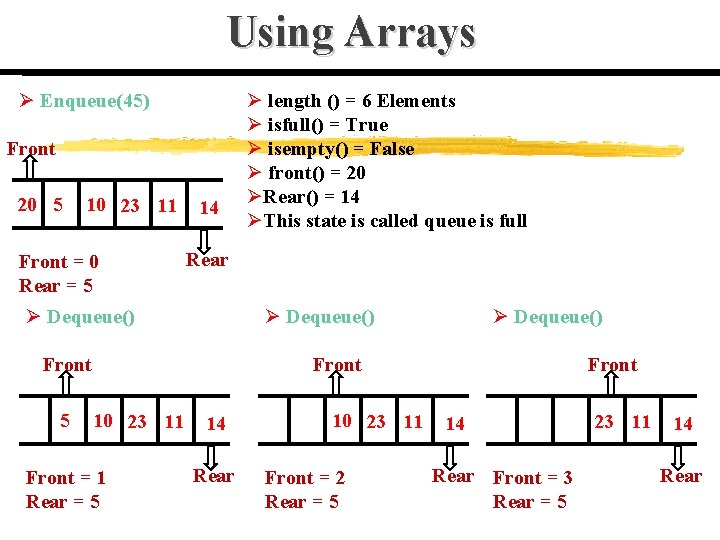
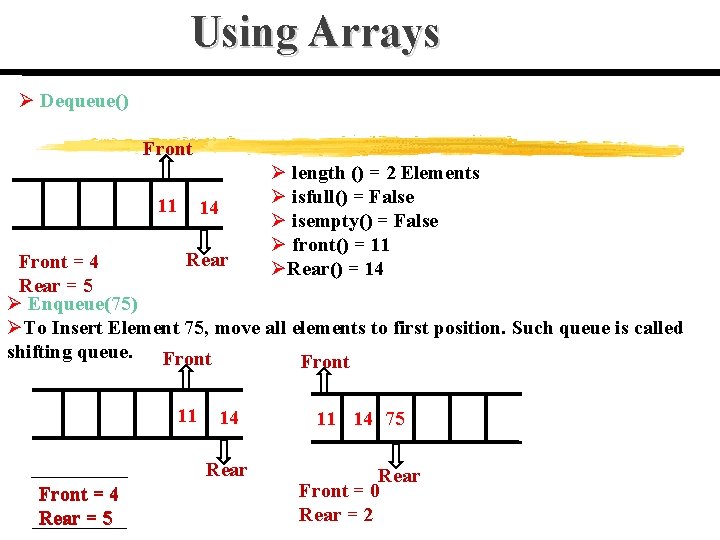
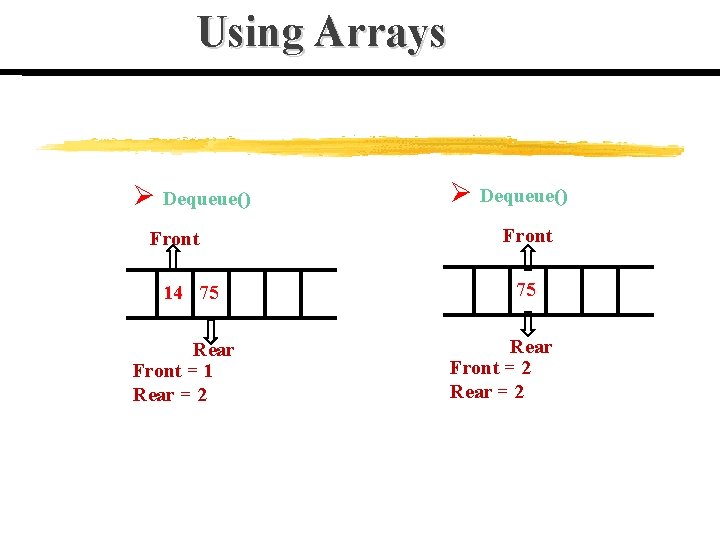
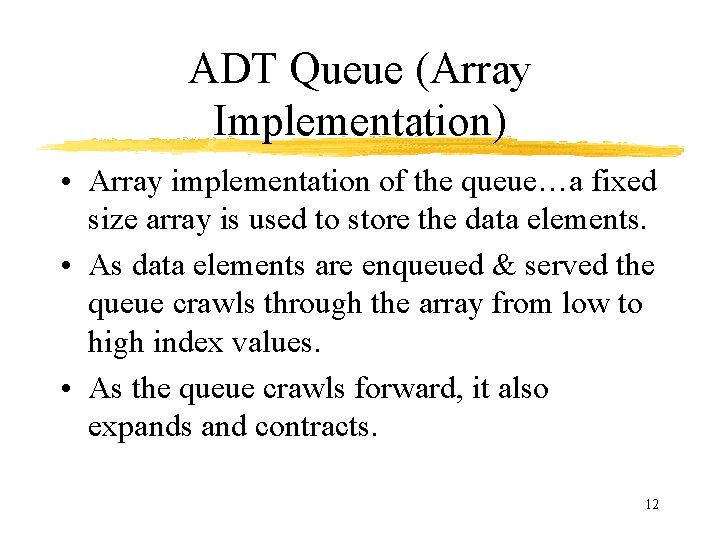
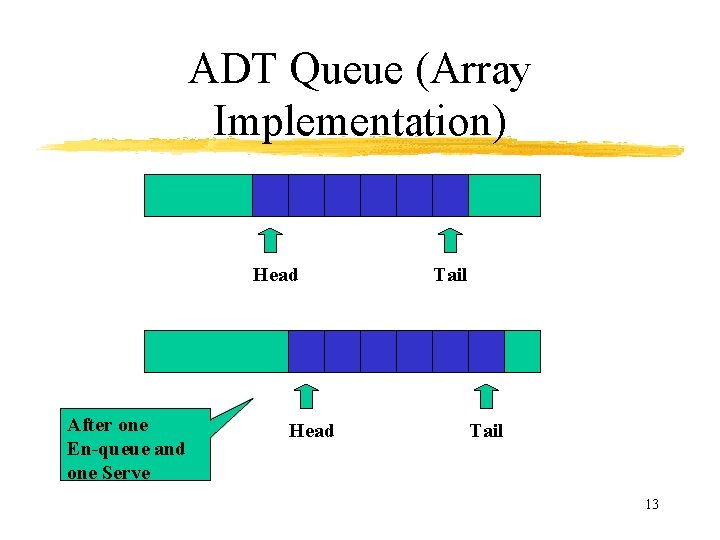
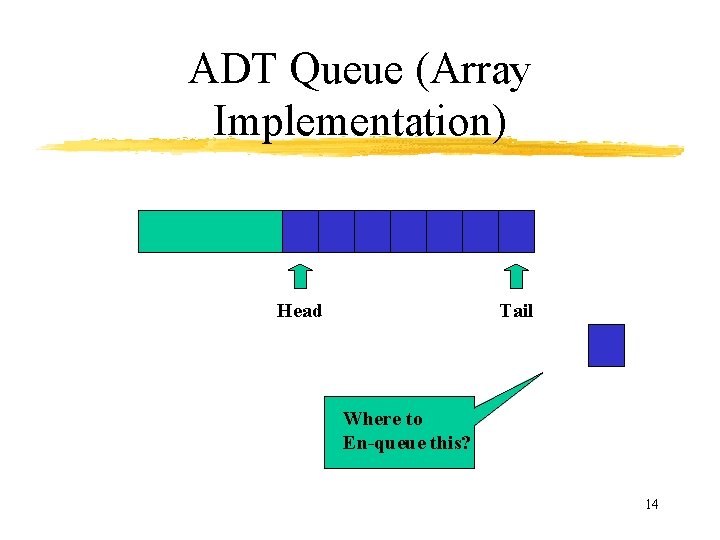
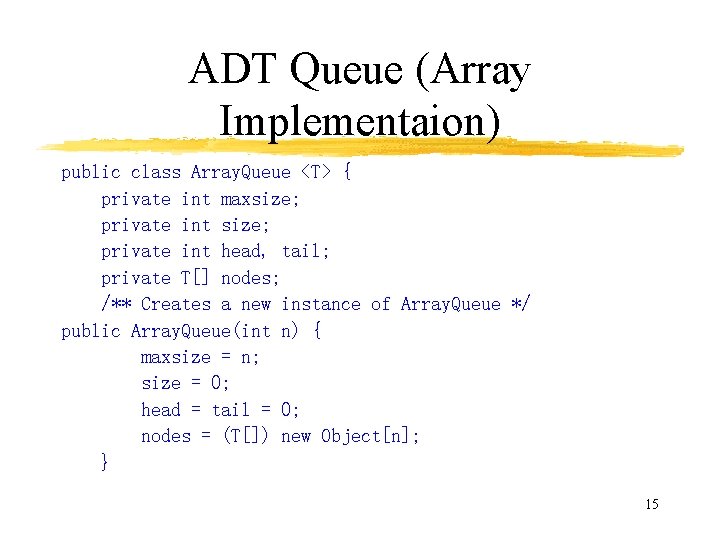
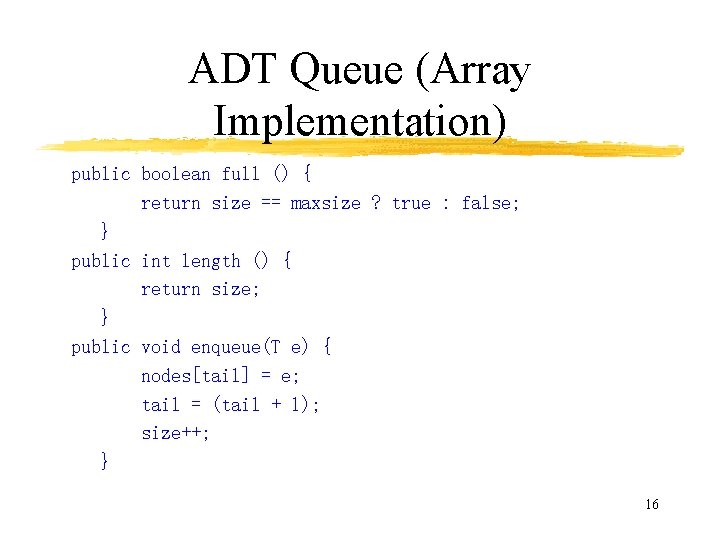
![ADT Queue (Array Implementation) public T serve () { T e = nodes[head]; head ADT Queue (Array Implementation) public T serve () { T e = nodes[head]; head](https://slidetodoc.com/presentation_image_h2/644e5cc27d85f3765f79ad4975b6125b/image-17.jpg)
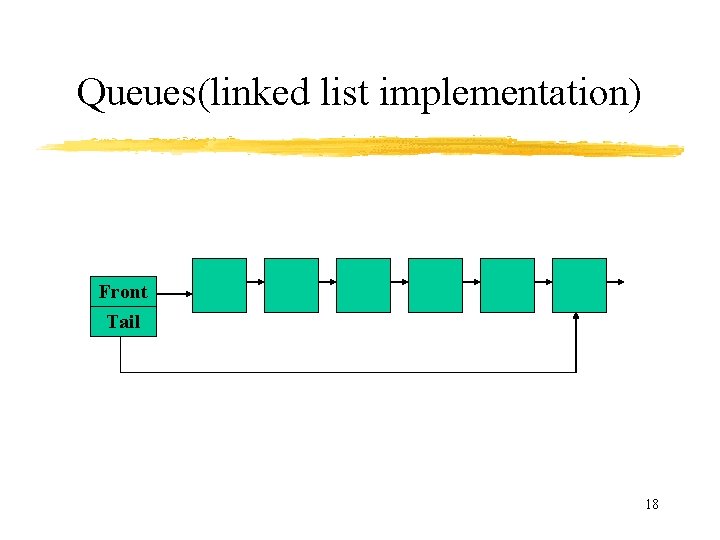
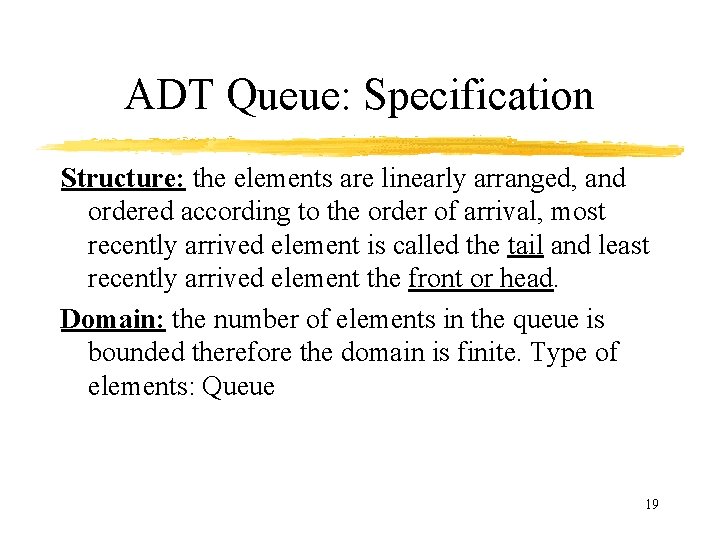
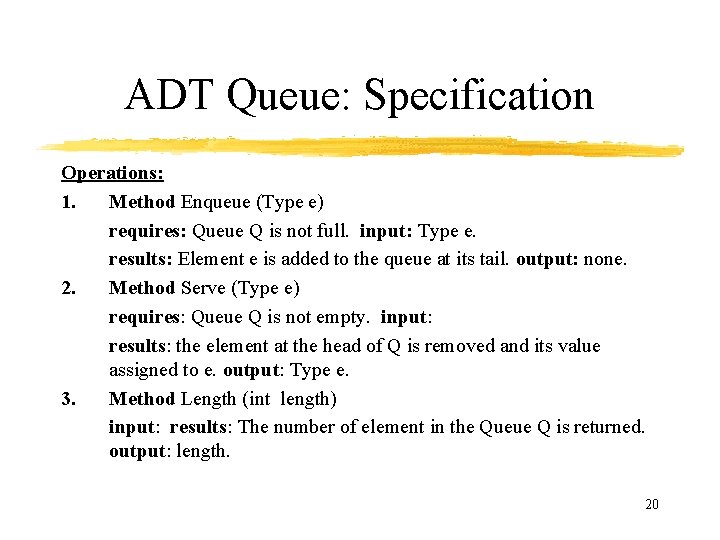
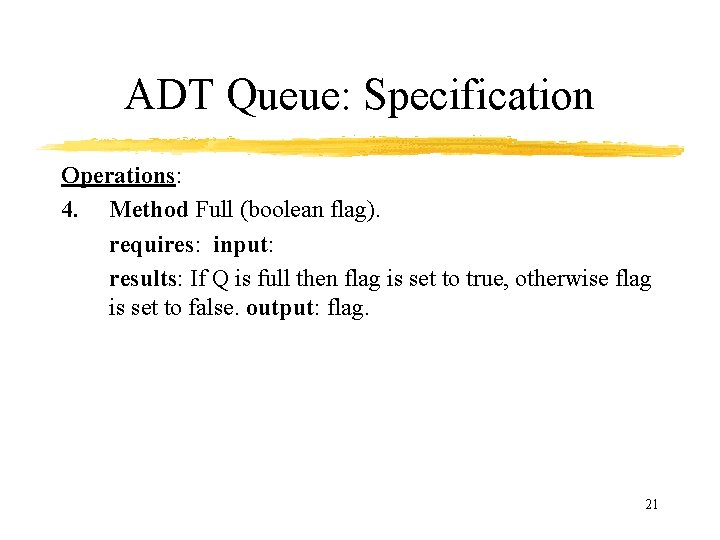
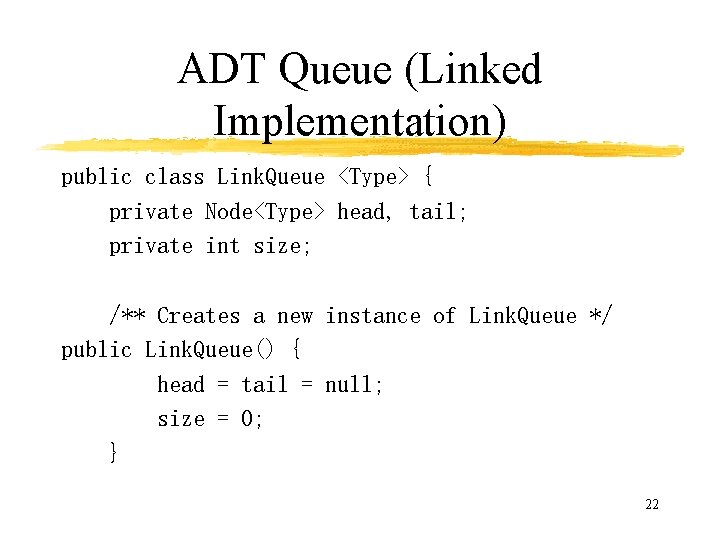
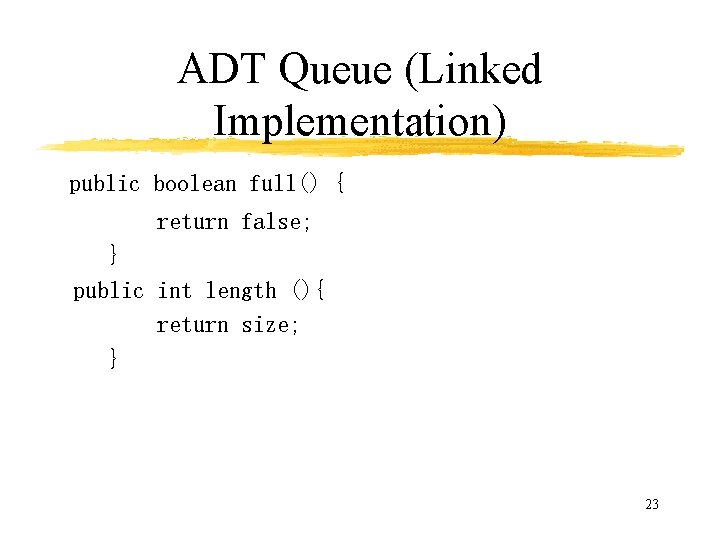
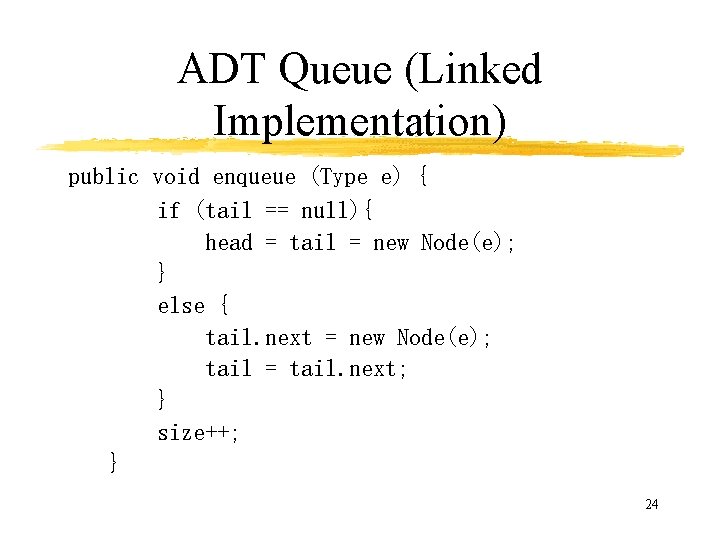
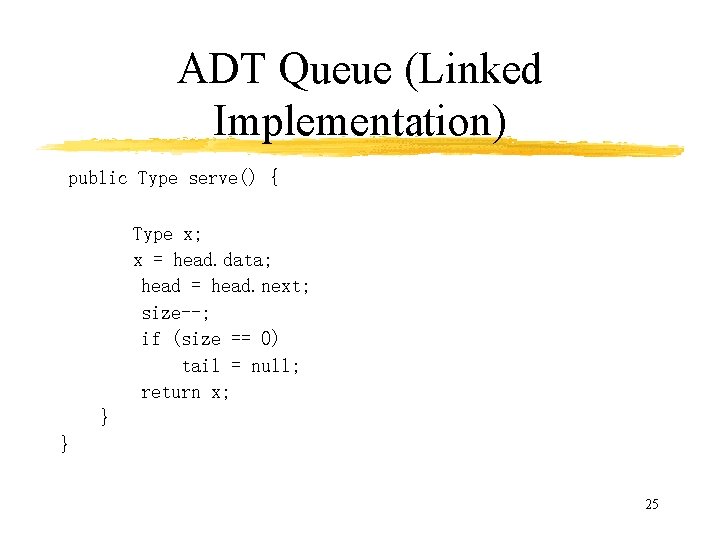
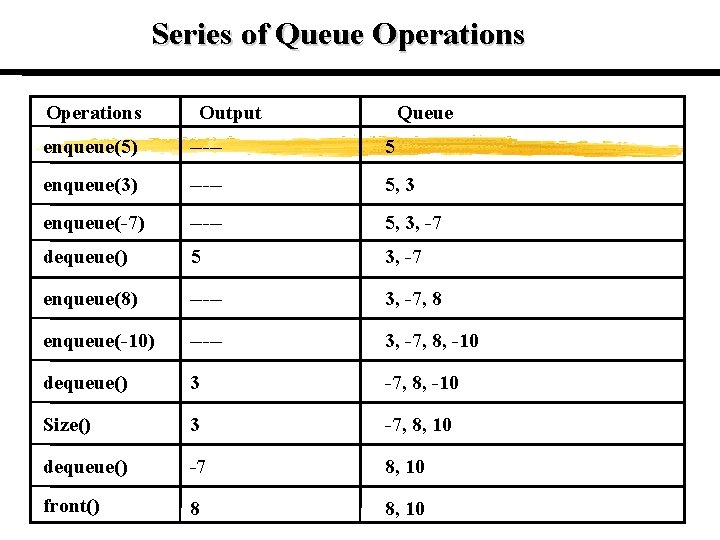
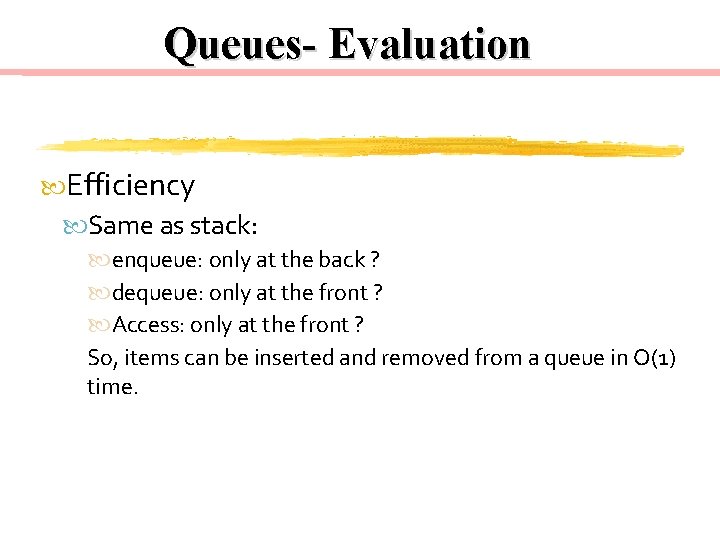
- Slides: 27
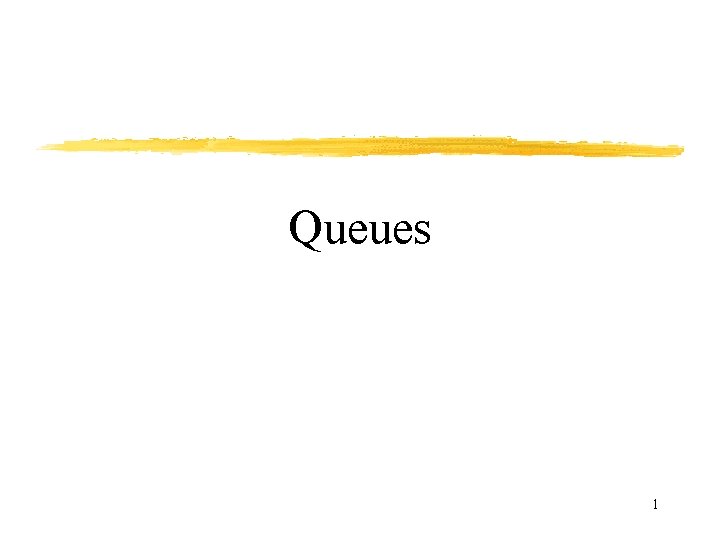
Queues 1
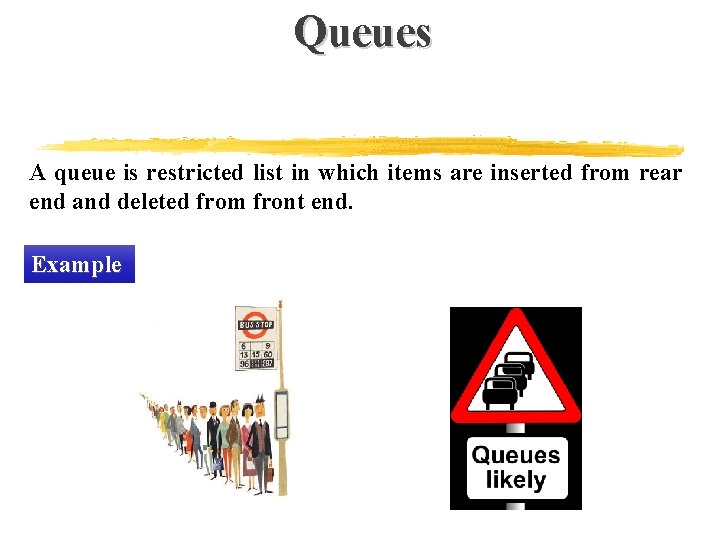
Queues A queue is restricted list in which items are inserted from rear end and deleted from front end. Example
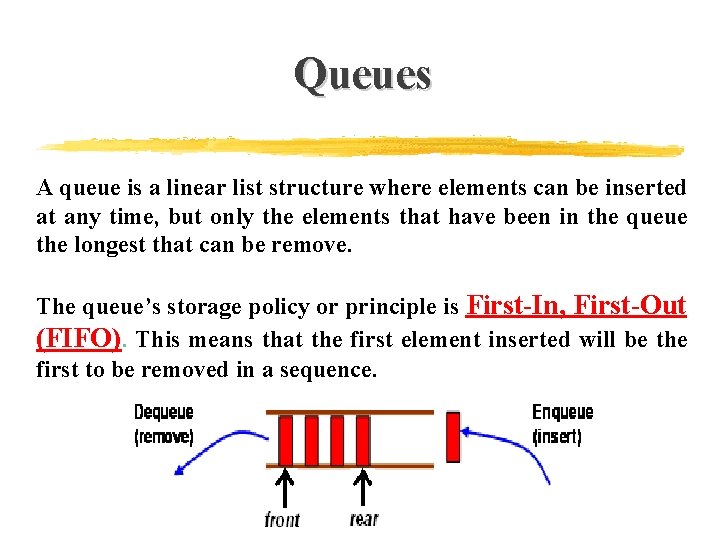
Queues A queue is a linear list structure where elements can be inserted at any time, but only the elements that have been in the queue the longest that can be remove. The queue’s storage policy or principle is First-In, First-Out (FIFO). This means that the first element inserted will be the first to be removed in a sequence.
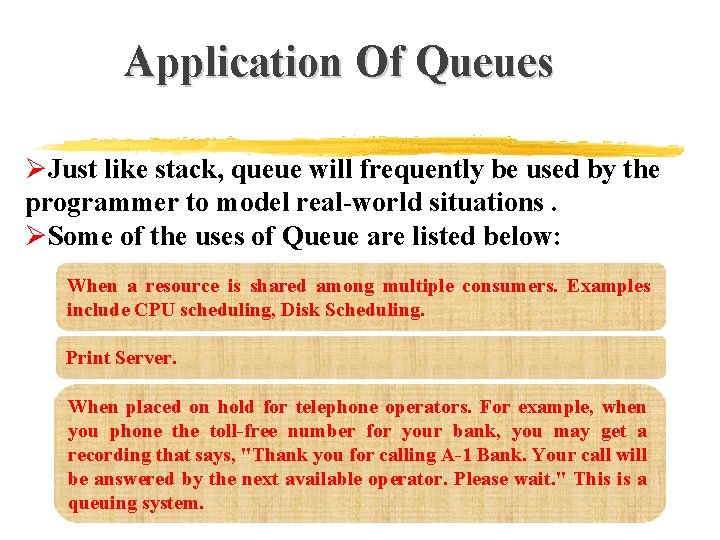
Application Of Queues ØJust like stack, queue will frequently be used by the programmer to model real-world situations. ØSome of the uses of Queue are listed below: When a resource is shared among multiple consumers. Examples include CPU scheduling, Disk Scheduling. Print Server. When placed on hold for telephone operators. For example, when you phone the toll-free number for your bank, you may get a recording that says, "Thank you for calling A-1 Bank. Your call will be answered by the next available operator. Please wait. " This is a queuing system. 6/10/2021
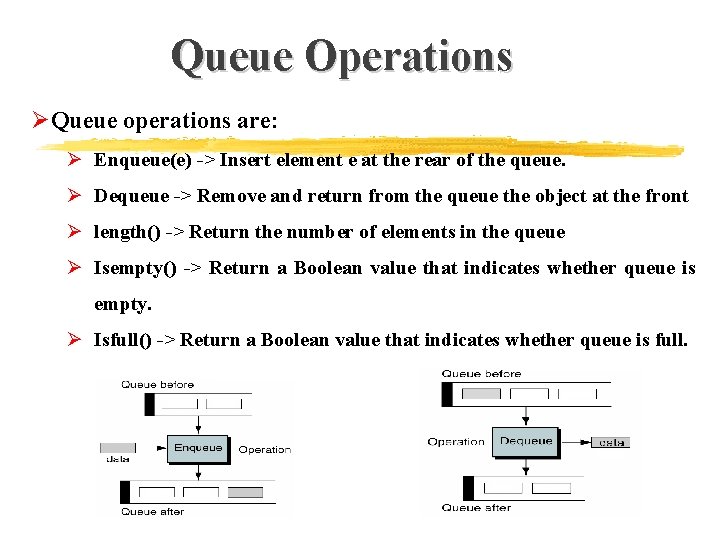
Queue Operations ØQueue operations are: Ø Enqueue(e) -> Insert element e at the rear of the queue. Ø Dequeue -> Remove and return from the queue the object at the front Ø length() -> Return the number of elements in the queue Ø Isempty() -> Return a Boolean value that indicates whether queue is empty. Ø Isfull() -> Return a Boolean value that indicates whether queue is full.
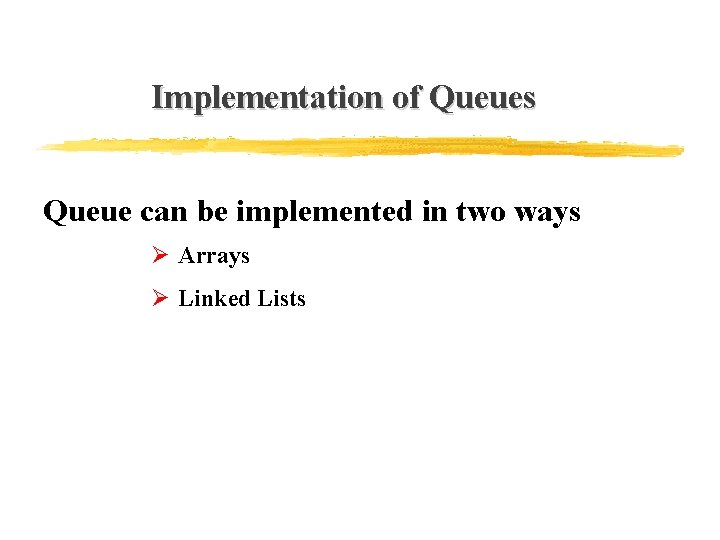
Implementation of Queues Queue can be implemented in two ways Ø Arrays Ø Linked Lists
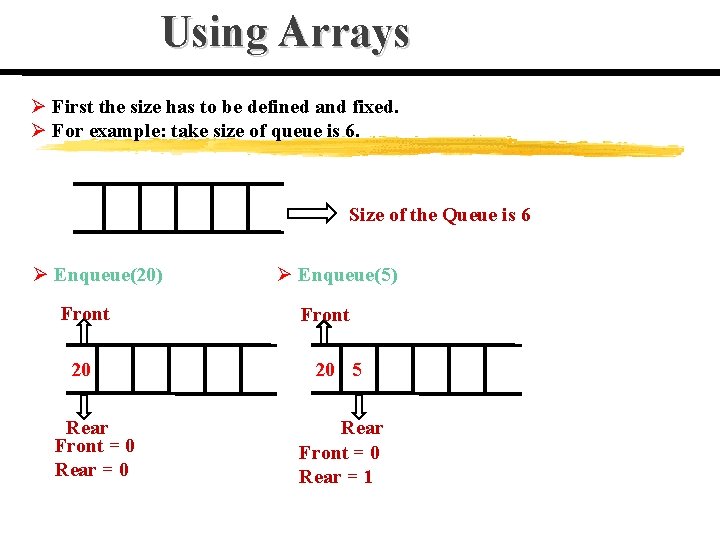
Using Arrays Ø First the size has to be defined and fixed. Ø For example: take size of queue is 6. Size of the Queue is 6 Ø Enqueue(20) Front 20 Rear Front = 0 Rear = 0 Ø Enqueue(5) Front 20 5 Rear Front = 0 Rear = 1
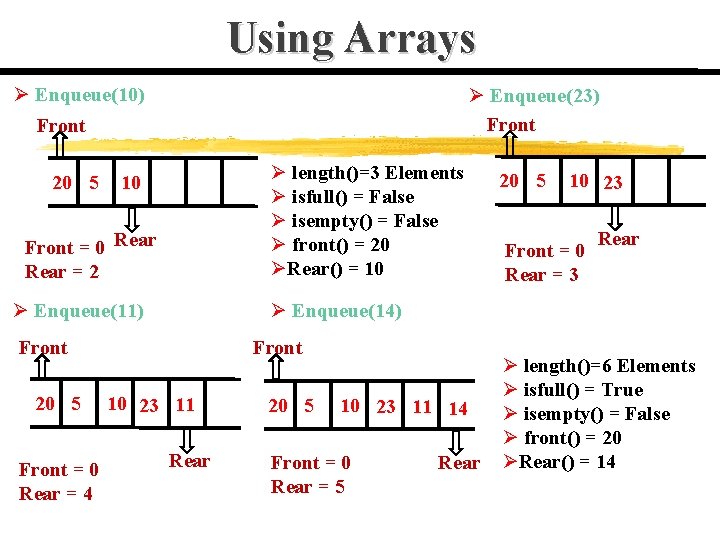
Using Arrays Ø Enqueue(10) Ø Enqueue(23) Front 20 5 Ø length()=3 Elements Ø isfull() = False Ø isempty() = False Ø front() = 20 ØRear() = 10 10 Front = 0 Rear = 2 Ø Enqueue(11) Front = 0 Rear = 4 10 23 Front = 0 Rear = 3 Rear Ø Enqueue(14) Front 20 5 Front 10 23 11 Rear 20 5 10 23 11 14 Front = 0 Rear = 5 Rear Ø length()=6 Elements Ø isfull() = True Ø isempty() = False Ø front() = 20 ØRear() = 14
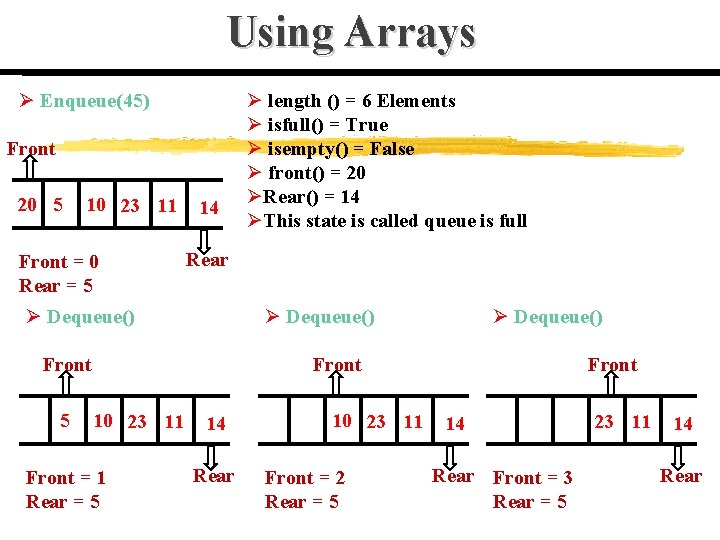
Using Arrays Ø Enqueue(45) Front 20 5 10 23 11 Front = 0 Rear = 5 14 Rear Ø Dequeue() Front 5 Ø length () = 6 Elements Ø isfull() = True Ø isempty() = False Ø front() = 20 ØRear() = 14 ØThis state is called queue is full Ø Dequeue() Front 10 23 11 Front = 1 Rear = 5 14 Rear 10 23 11 Front = 2 Rear = 5 Front 14 Rear Front = 3 Rear = 5 23 11 14 Rear
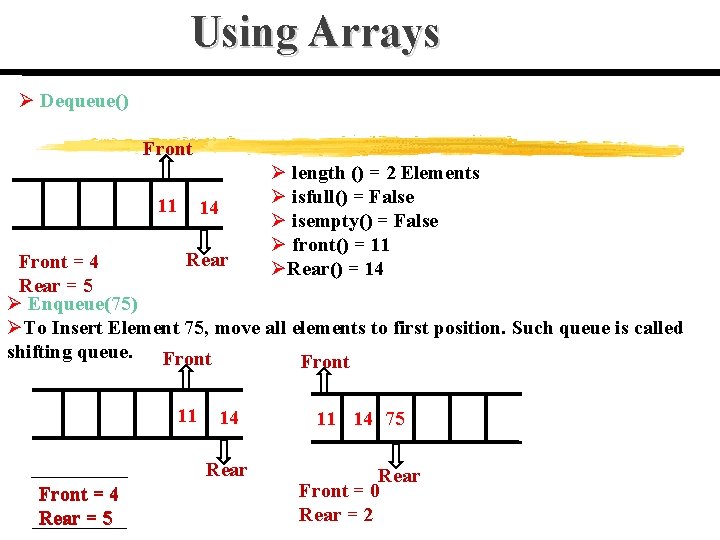
Using Arrays Ø Dequeue() Front 11 14 Ø length () = 2 Elements Ø isfull() = False Ø isempty() = False Ø front() = 11 ØRear() = 14 Rear Front = 4 Rear = 5 Ø Enqueue(75) ØTo Insert Element 75, move all elements to first position. Such queue is called shifting queue. Front 11 Front = 4 Rear = 5 14 11 14 75 Rear Front = 0 Rear = 2
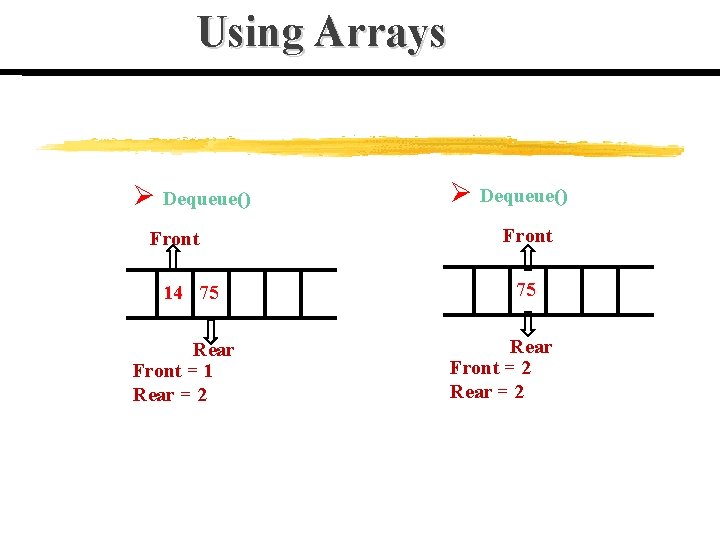
Using Arrays Ø Dequeue() Front 14 75 Rear Front = 1 Rear = 2 Ø Dequeue() Front 75 Rear Front = 2 Rear = 2
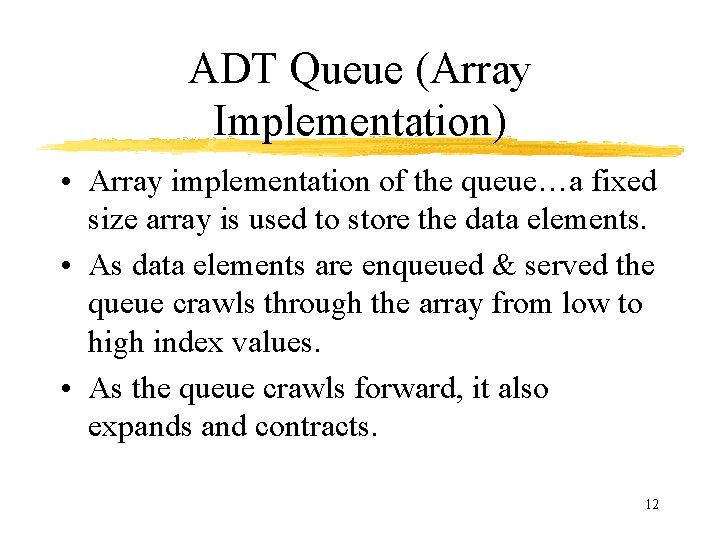
ADT Queue (Array Implementation) • Array implementation of the queue…a fixed size array is used to store the data elements. • As data elements are enqueued & served the queue crawls through the array from low to high index values. • As the queue crawls forward, it also expands and contracts. 12
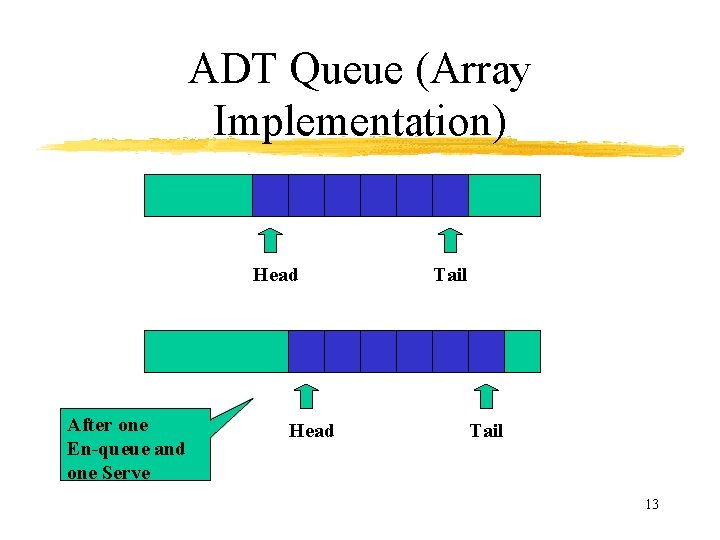
ADT Queue (Array Implementation) Head After one En-queue and one Serve Head Tail 13
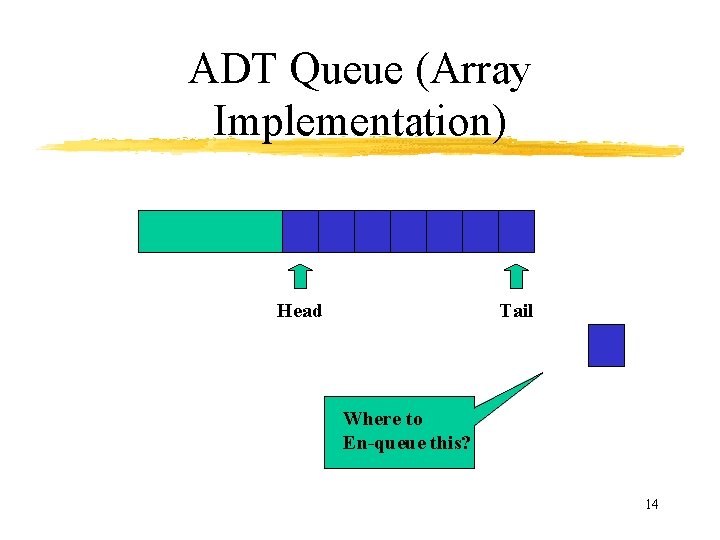
ADT Queue (Array Implementation) Head Tail Where to En-queue this? 14
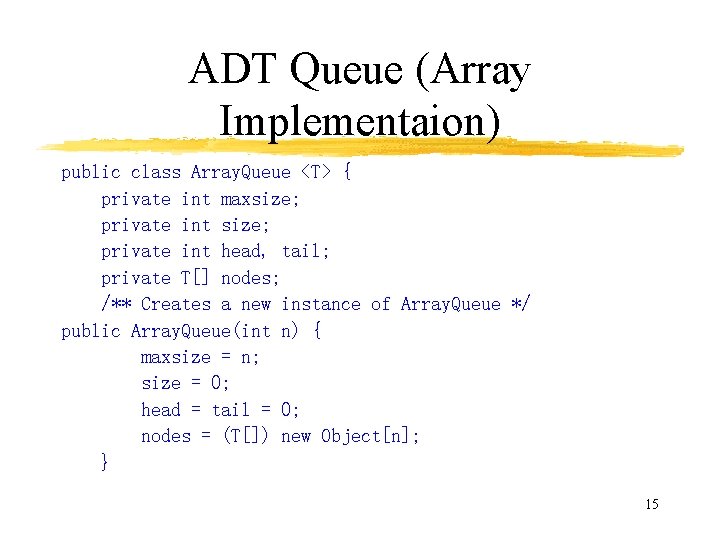
ADT Queue (Array Implementaion) public class Array. Queue <T> { private int maxsize; private int head, tail; private T[] nodes; /** Creates a new instance of Array. Queue */ public Array. Queue(int n) { maxsize = n; size = 0; head = tail = 0; nodes = (T[]) new Object[n]; } 15
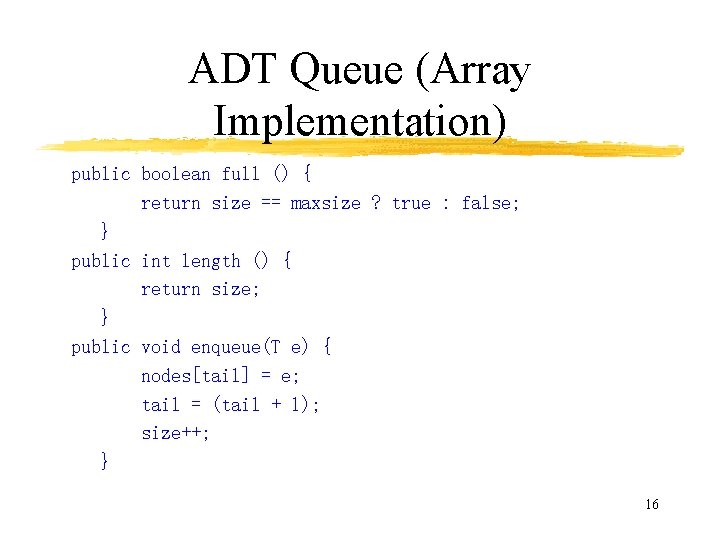
ADT Queue (Array Implementation) public boolean full () { return size == maxsize ? true : false; } public int length () { return size; } public void enqueue(T e) { nodes[tail] = e; tail = (tail + 1); size++; } 16
![ADT Queue Array Implementation public T serve T e nodeshead head ADT Queue (Array Implementation) public T serve () { T e = nodes[head]; head](https://slidetodoc.com/presentation_image_h2/644e5cc27d85f3765f79ad4975b6125b/image-17.jpg)
ADT Queue (Array Implementation) public T serve () { T e = nodes[head]; head = (head + 1); size--; return e; } } 17
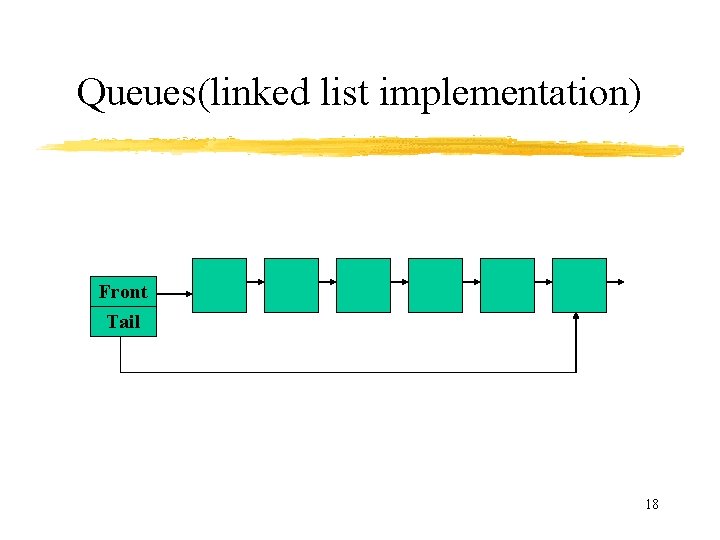
Queues(linked list implementation) Front Tail 18
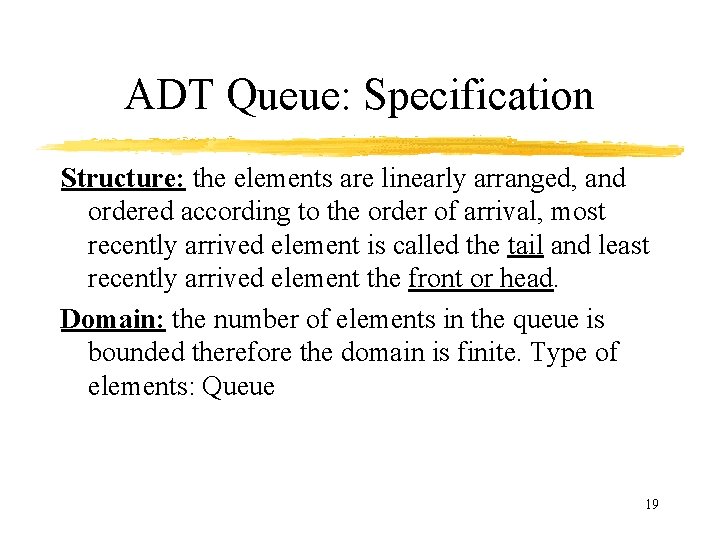
ADT Queue: Specification Structure: the elements are linearly arranged, and ordered according to the order of arrival, most recently arrived element is called the tail and least recently arrived element the front or head. Domain: the number of elements in the queue is bounded therefore the domain is finite. Type of elements: Queue 19
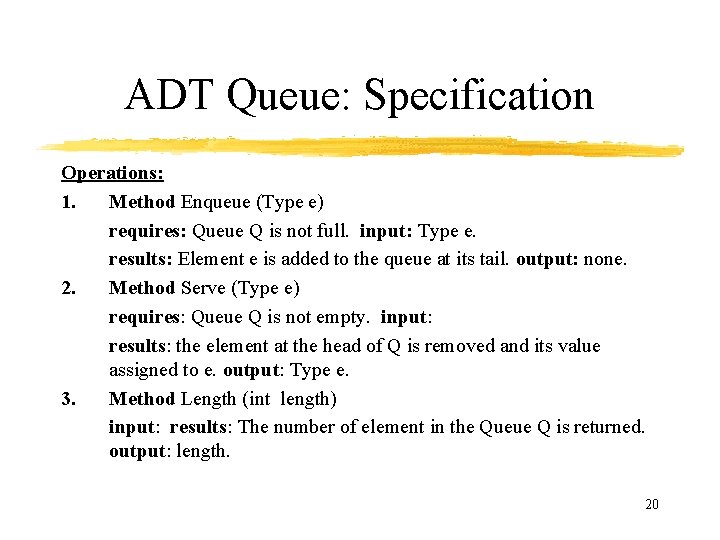
ADT Queue: Specification Operations: 1. Method Enqueue (Type e) requires: Queue Q is not full. input: Type e. results: Element e is added to the queue at its tail. output: none. 2. Method Serve (Type e) requires: Queue Q is not empty. input: results: the element at the head of Q is removed and its value assigned to e. output: Type e. 3. Method Length (int length) input: results: The number of element in the Queue Q is returned. output: length. 20
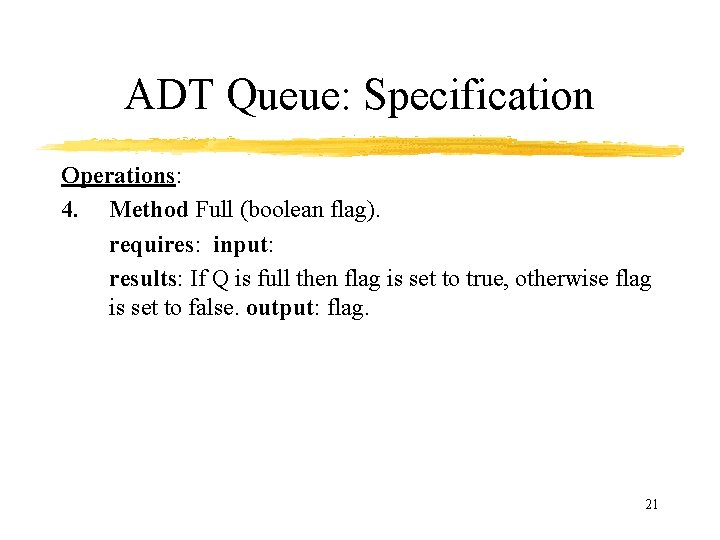
ADT Queue: Specification Operations: 4. Method Full (boolean flag). requires: input: results: If Q is full then flag is set to true, otherwise flag is set to false. output: flag. 21
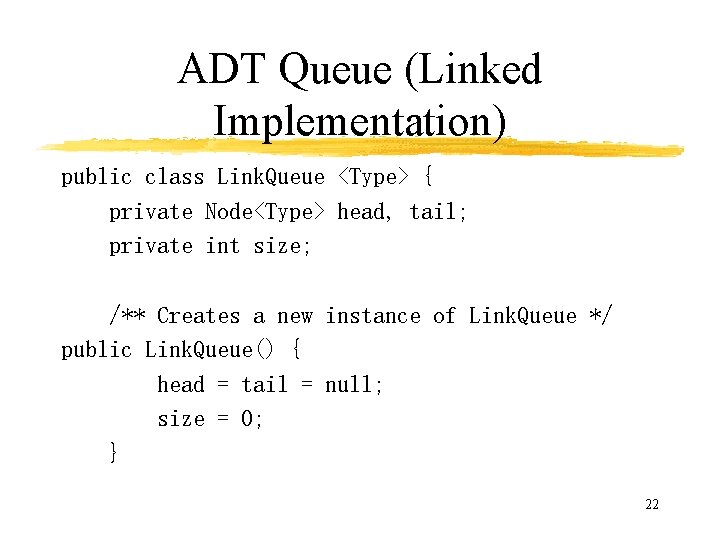
ADT Queue (Linked Implementation) public class Link. Queue <Type> { private Node<Type> head, tail; private int size; /** Creates a new instance of Link. Queue */ public Link. Queue() { head = tail = null; size = 0; } 22
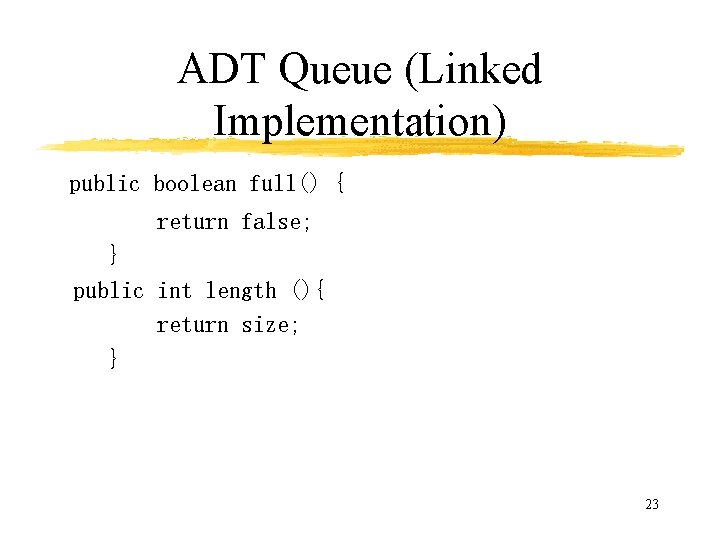
ADT Queue (Linked Implementation) public boolean full() { return false; } public int length (){ return size; } 23
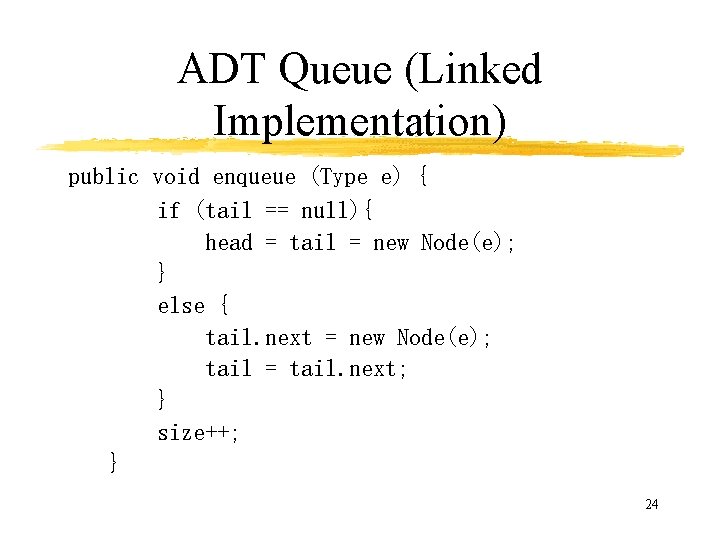
ADT Queue (Linked Implementation) public void enqueue (Type e) { if (tail == null){ head = tail = new Node(e); } else { tail. next = new Node(e); tail = tail. next; } size++; } 24
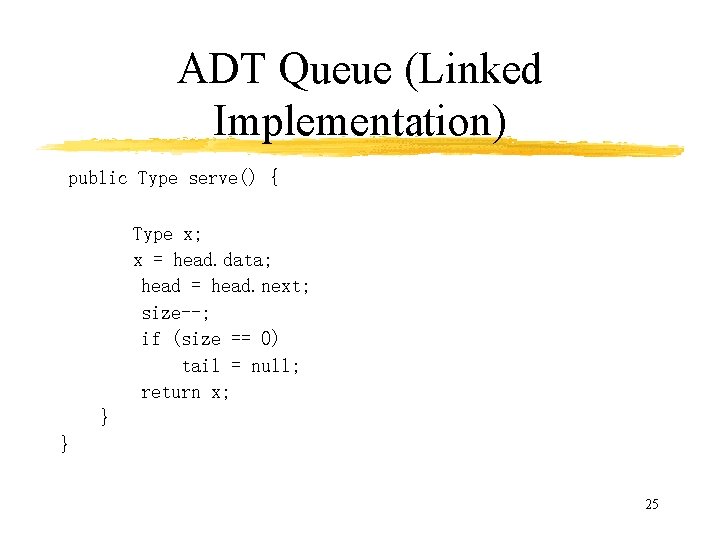
ADT Queue (Linked Implementation) public Type serve() { Type x; x = head. data; head = head. next; size--; if (size == 0) tail = null; return x; } } 25
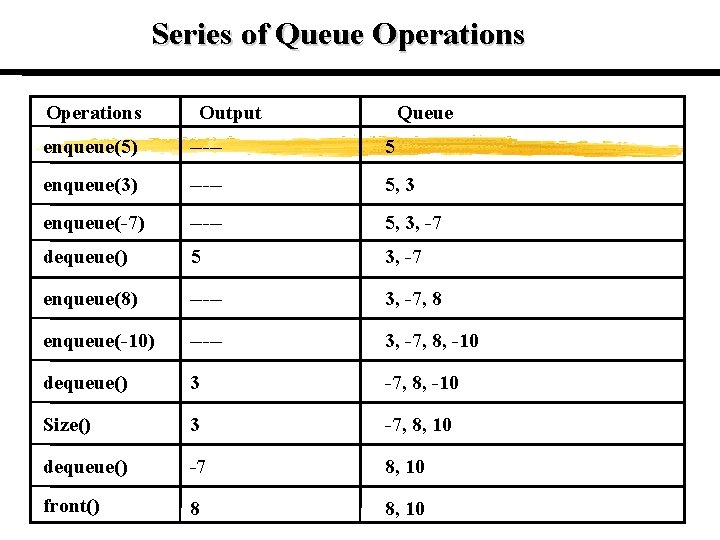
Series of Queue Operations Output Queue enqueue(5) ----- 5 enqueue(3) ----- 5, 3 enqueue(-7) ----- 5, 3, -7 dequeue() 5 3, -7 enqueue(8) ----- 3, -7, 8 enqueue(-10) ----- 3, -7, 8, -10 dequeue() 3 -7, 8, -10 Size() 3 -7, 8, 10 dequeue() -7 8, 10 front() 8 8, 10
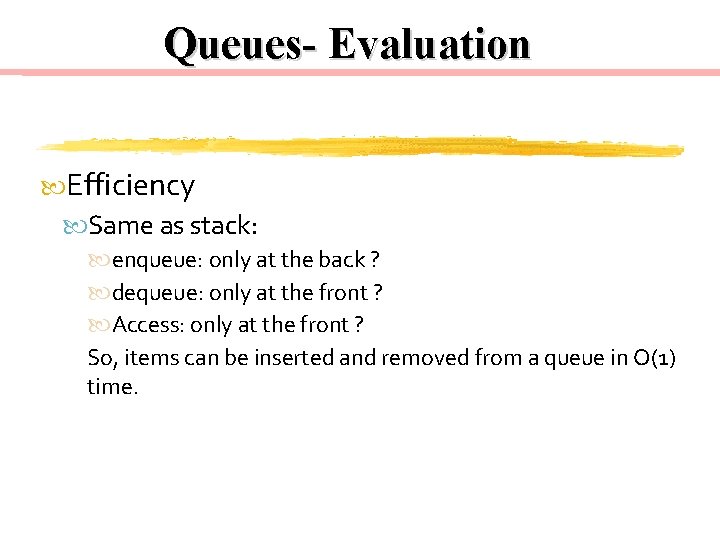
Queues- Evaluation Efficiency Same as stack: enqueue: only at the back ? dequeue: only at the front ? Access: only at the front ? So, items can be inserted and removed from a queue in O(1) time.
Ncic hosts restricted files and non-restricted files
Cjis meaning
Queue means
Queue/ antraian = ordered list
Difference between linked list and queue
Priority queue linked list python
Operasi pada queue
Jika noel(create(q)) adalah 0, maka front(create(q)) adalah
Rtos mailbox
Adaptable priority queues
Aganj
Representation of queues
Applications of priority queues
Python stack and queue
Java stacks and queues
Exercises on stacks and queues
Struktur data queue sering digunakan untuk
Priority queues: quiz
Message queues in unix
Define a queue
Java stacks and queues
What does restricted mode mean
Nfpp in motor insurance
Restricted copyright
What are narrations
Restricted executive bonus arrangement
Gdb restricted electives
Sound signals in restricted visibility