Python Errors Exceptions Dongen Zhou dz 78drexel edu
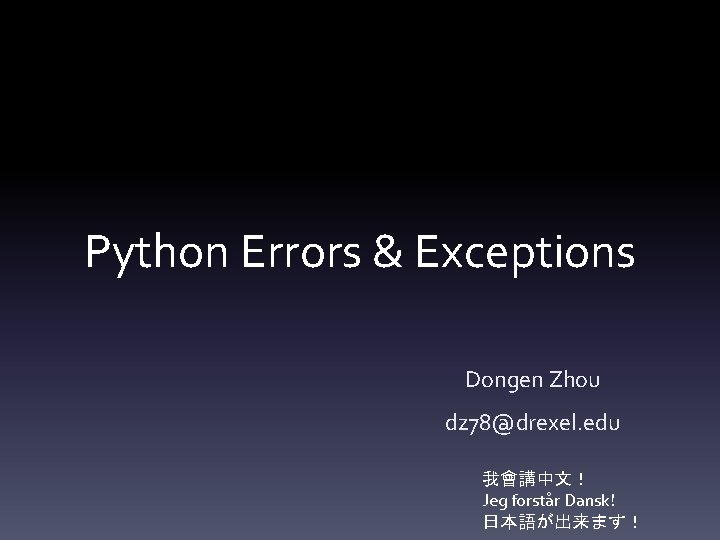
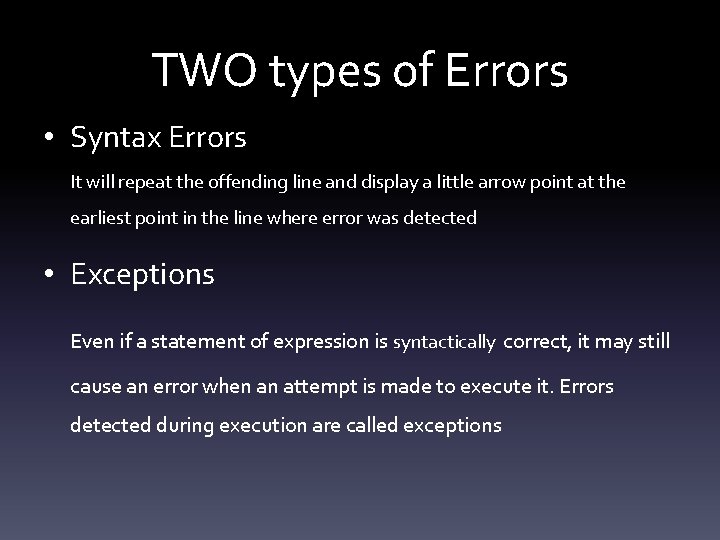
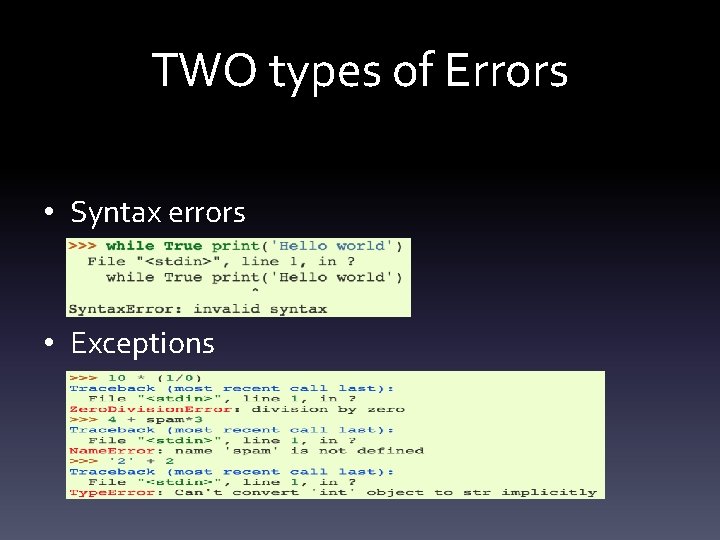
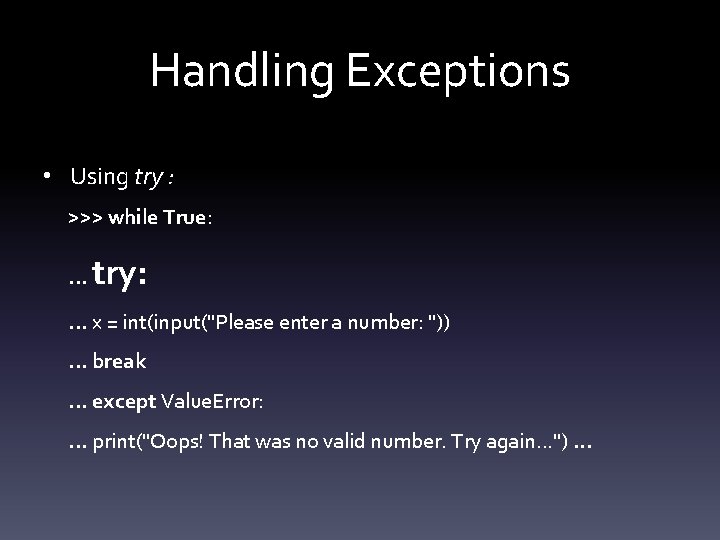
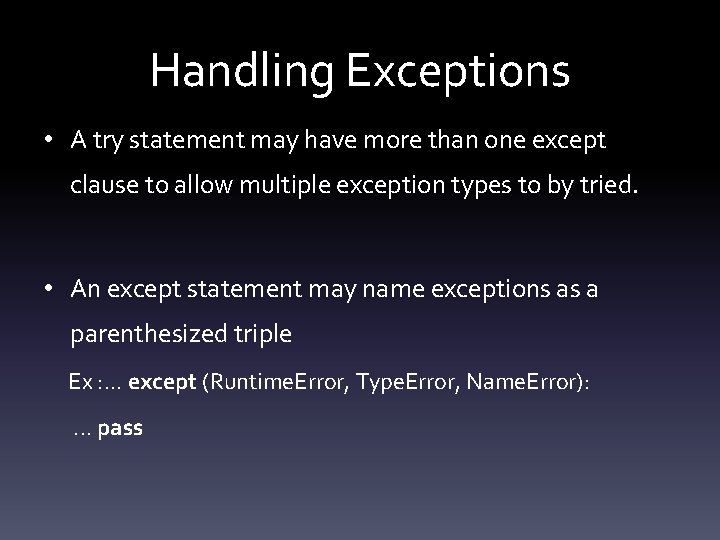
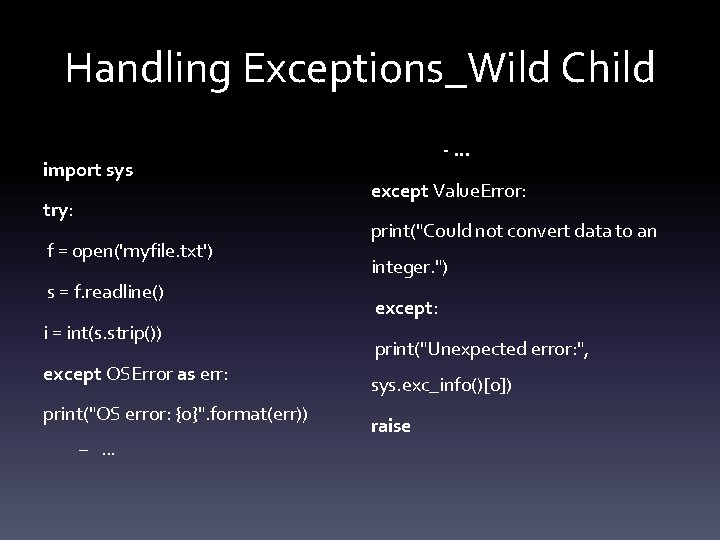
![Handling Exceptions_Else Clause for arg in sys. argv[1: ]: try: f = open(arg, 'r') Handling Exceptions_Else Clause for arg in sys. argv[1: ]: try: f = open(arg, 'r')](https://slidetodoc.com/presentation_image/0469b82fc4930b15ca1e9c3a349d4352/image-7.jpg)
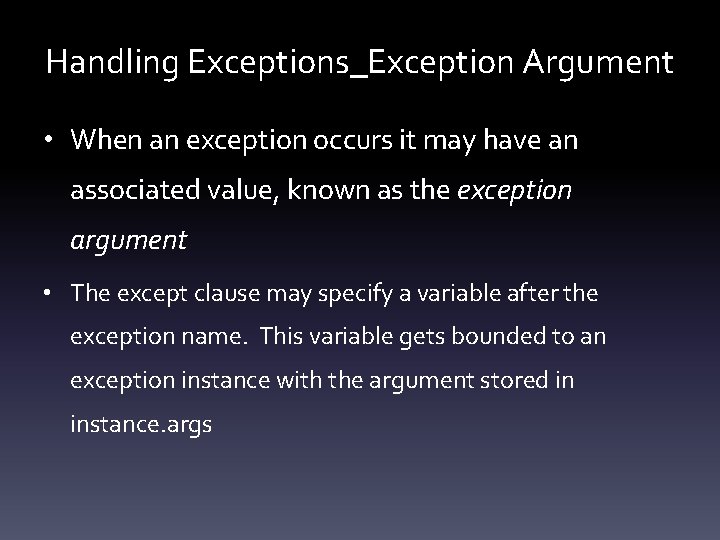
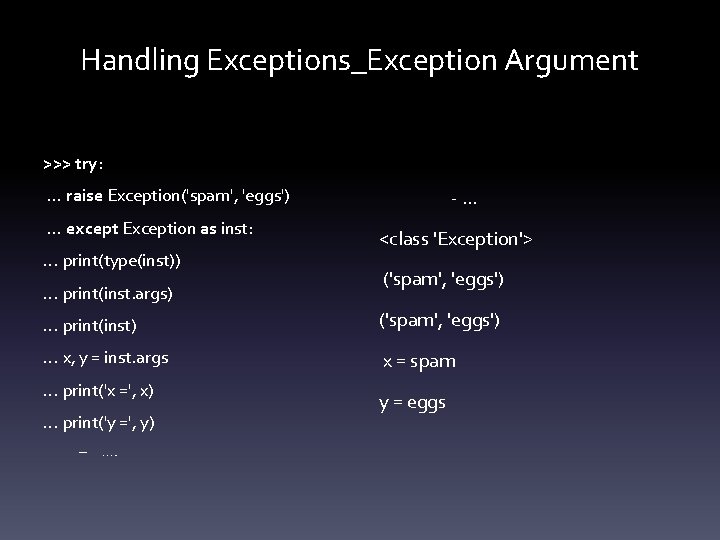
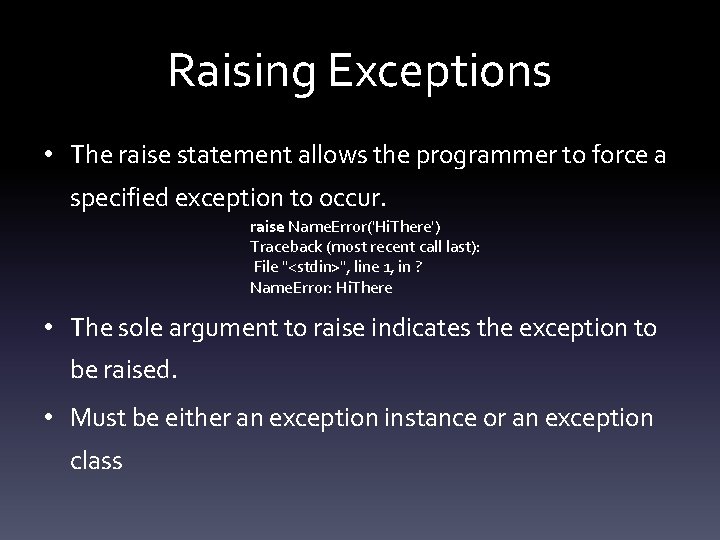
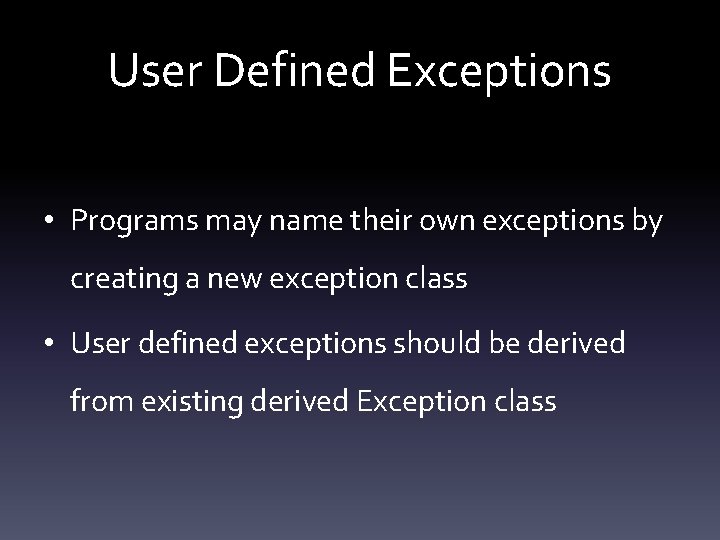
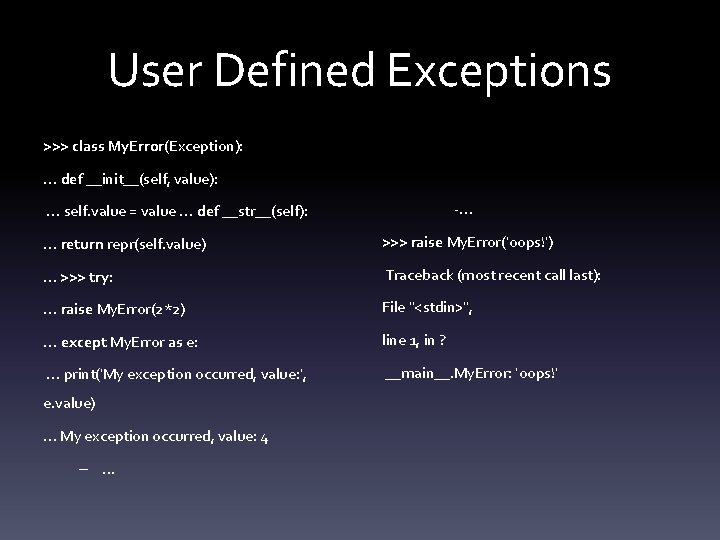
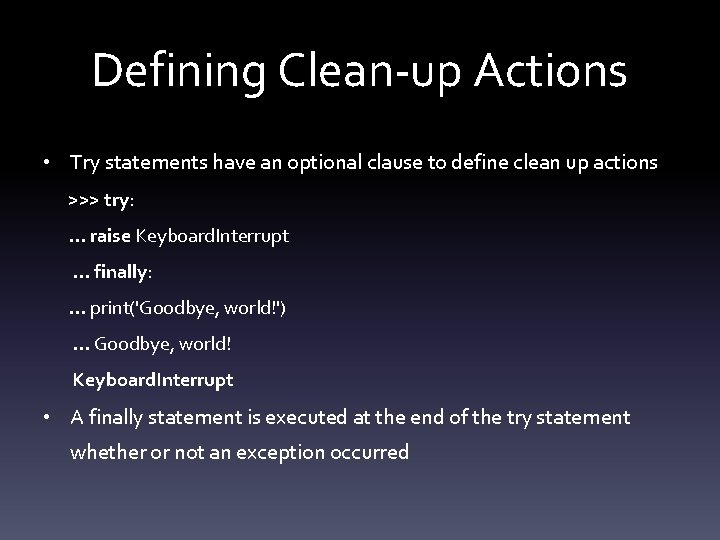
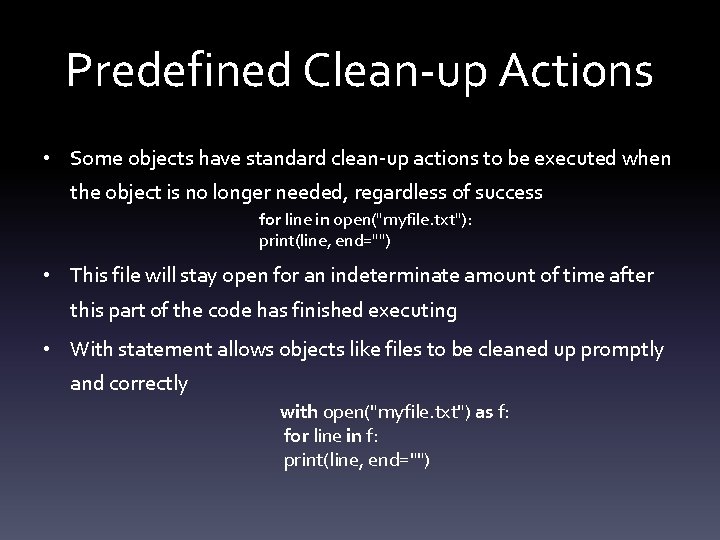
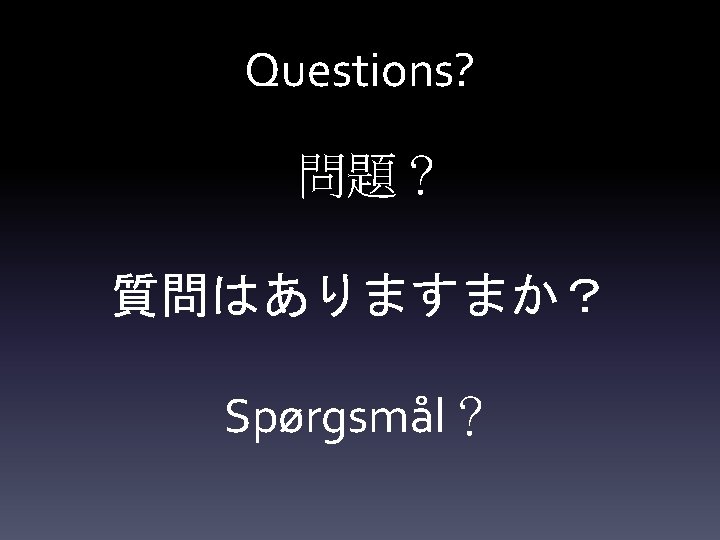
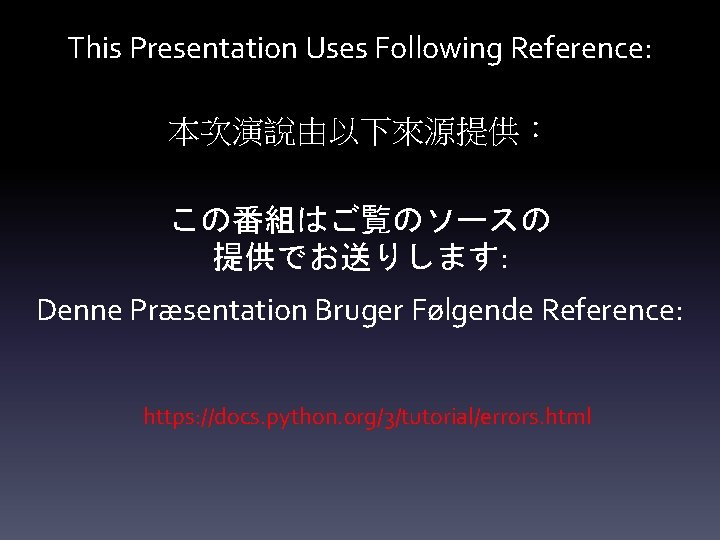
- Slides: 16
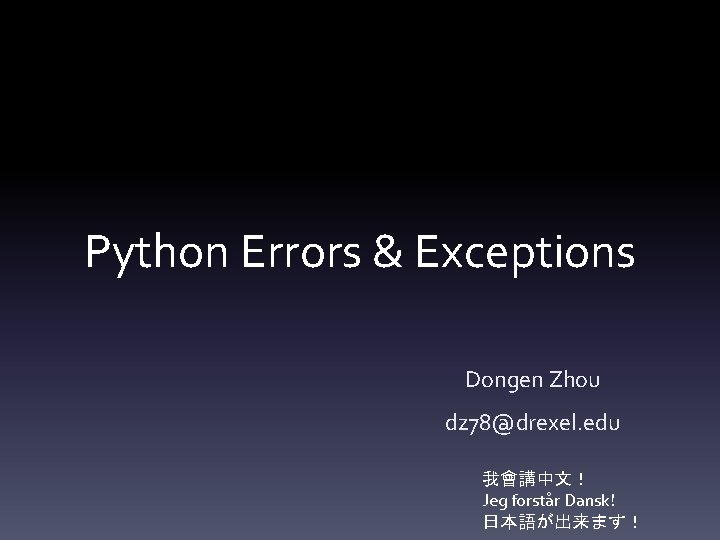
Python Errors & Exceptions Dongen Zhou dz 78@drexel. edu 我會講中文! Jeg forstår Dansk! 日本語が出来ます!
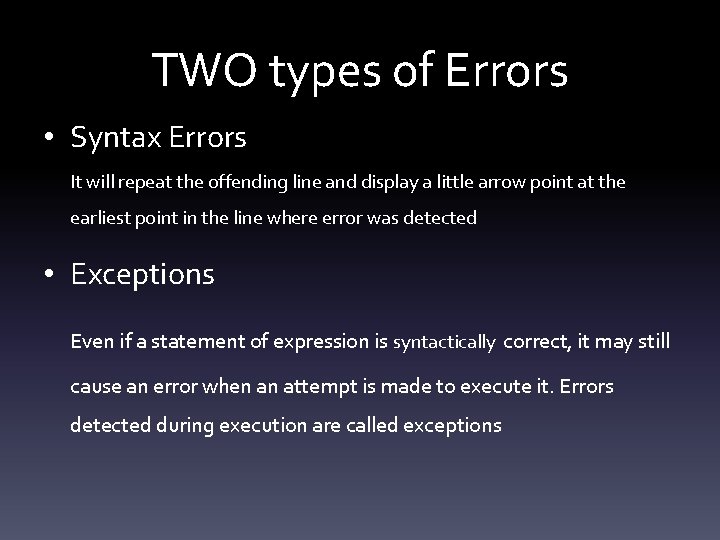
TWO types of Errors • Syntax Errors It will repeat the offending line and display a little arrow point at the earliest point in the line where error was detected • Exceptions Even if a statement of expression is syntactically correct, it may still cause an error when an attempt is made to execute it. Errors detected during execution are called exceptions
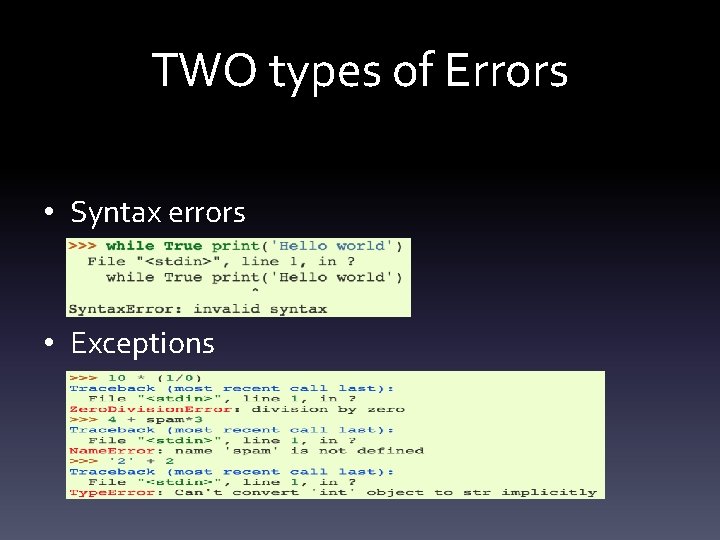
TWO types of Errors • Syntax errors • Exceptions
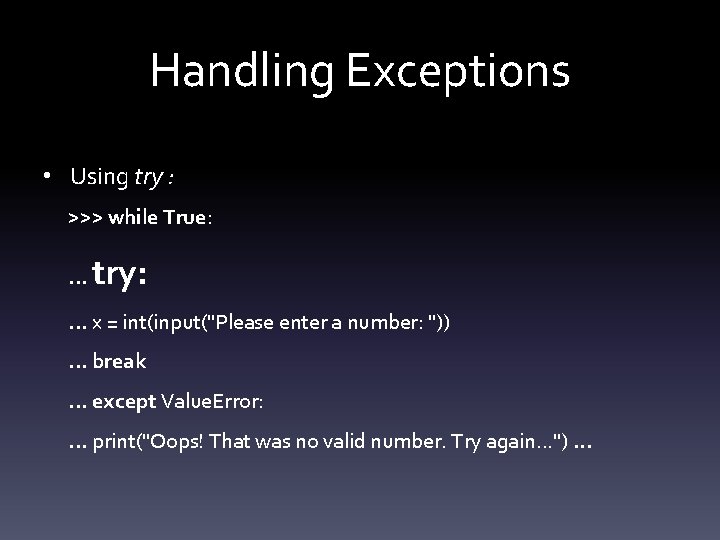
Handling Exceptions • Using try : >>> while True: . . . try: . . . x = int(input("Please enter a number: ")). . . break. . . except Value. Error: . . . print("Oops! That was no valid number. Try again. . . "). . .
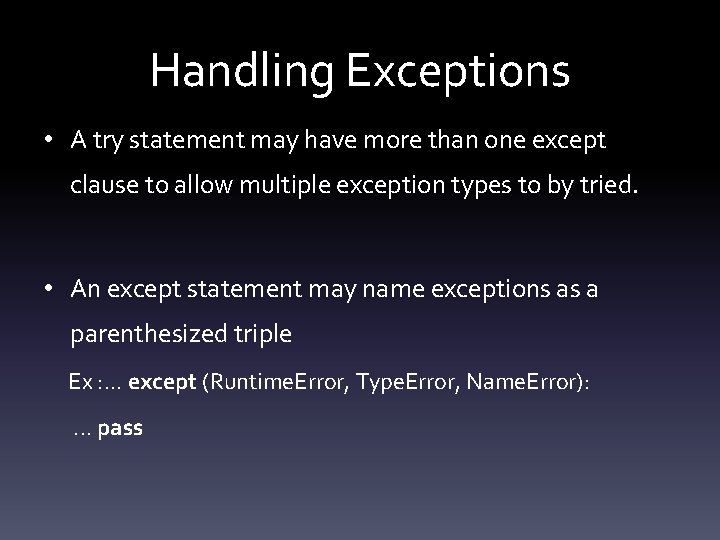
Handling Exceptions • A try statement may have more than one except clause to allow multiple exception types to by tried. • An except statement may name exceptions as a parenthesized triple Ex : . . . except (Runtime. Error, Type. Error, Name. Error): . . . pass
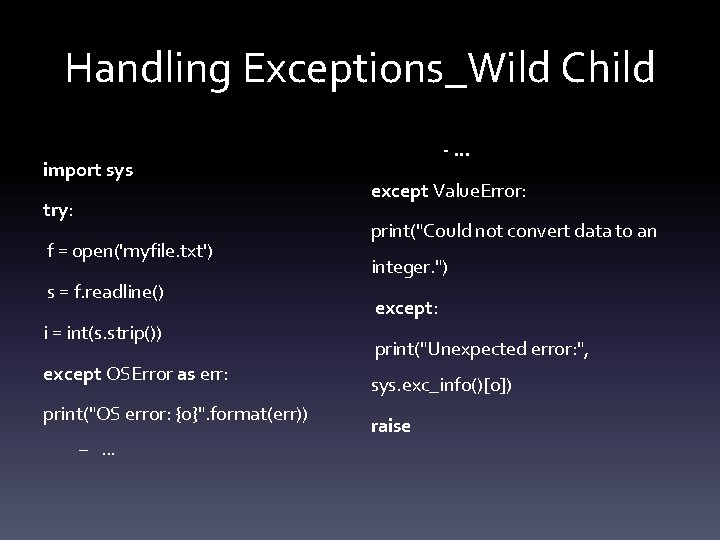
Handling Exceptions_Wild Child import sys try: f = open('myfile. txt') s = f. readline() i = int(s. strip()) except OSError as err: print("OS error: {0}". format(err)) – … -… except Value. Error: print("Could not convert data to an integer. ") except: print("Unexpected error: ", sys. exc_info()[0]) raise
![Handling ExceptionsElse Clause for arg in sys argv1 try f openarg r Handling Exceptions_Else Clause for arg in sys. argv[1: ]: try: f = open(arg, 'r')](https://slidetodoc.com/presentation_image/0469b82fc4930b15ca1e9c3a349d4352/image-7.jpg)
Handling Exceptions_Else Clause for arg in sys. argv[1: ]: try: f = open(arg, 'r') except IOError: print('cannot open', arg) else: print(arg, 'has', len(f. readlines()), 'lines') f. close()
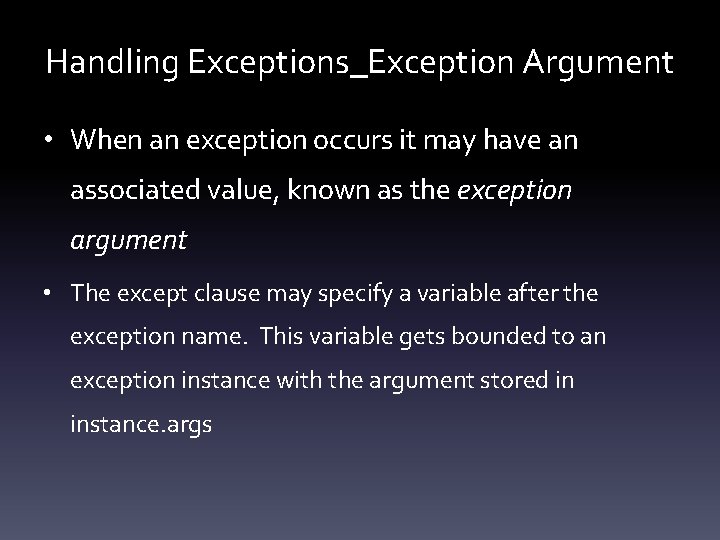
Handling Exceptions_Exception Argument • When an exception occurs it may have an associated value, known as the exception argument • The except clause may specify a variable after the exception name. This variable gets bounded to an exception instance with the argument stored in instance. args
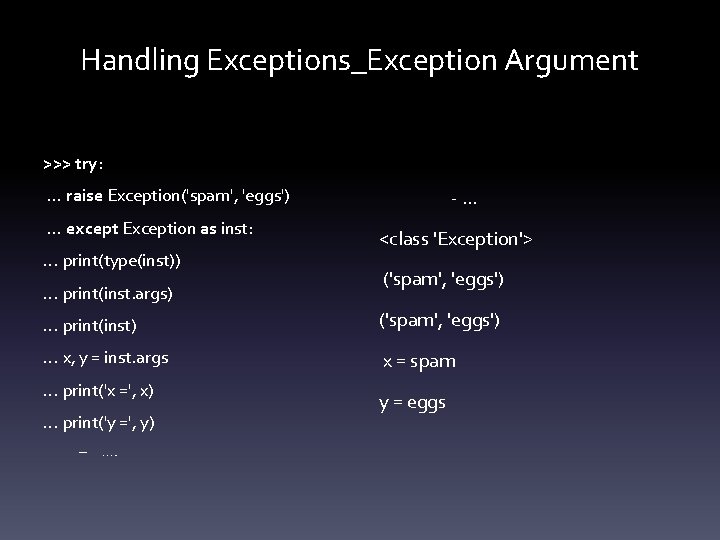
Handling Exceptions_Exception Argument >>> try: . . . raise Exception('spam', 'eggs'). . . except Exception as inst: . . . print(type(inst)). . . print(inst. args) -… <class 'Exception'> ('spam', 'eggs') . . . print(inst) ('spam', 'eggs') . . . x, y = inst. args x = spam . . . print('x =', x). . . print('y =', y) – …. y = eggs
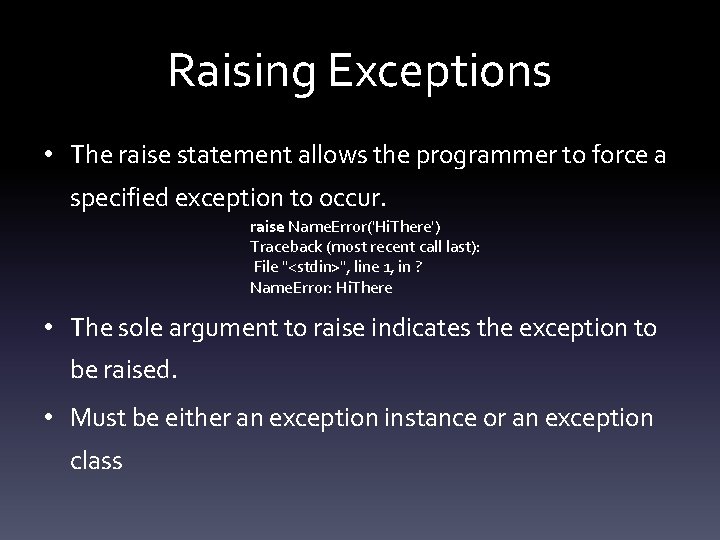
Raising Exceptions • The raise statement allows the programmer to force a specified exception to occur. raise Name. Error('Hi. There') Traceback (most recent call last): File "<stdin>", line 1, in ? Name. Error: Hi. There • The sole argument to raise indicates the exception to be raised. • Must be either an exception instance or an exception class
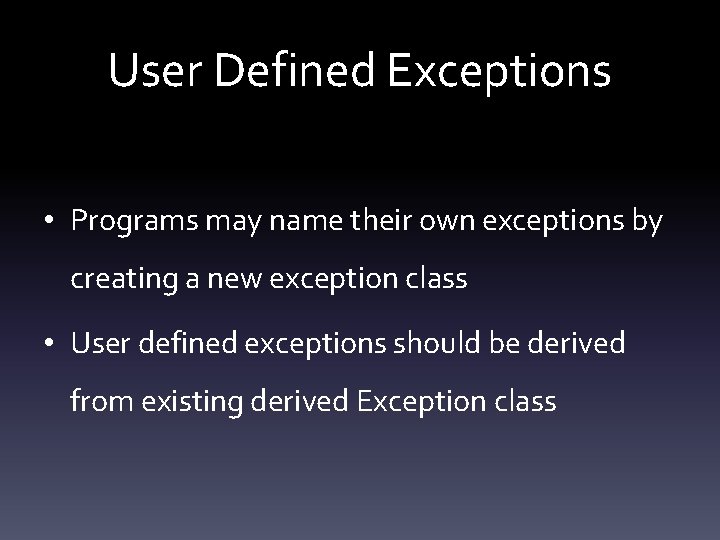
User Defined Exceptions • Programs may name their own exceptions by creating a new exception class • User defined exceptions should be derived from existing derived Exception class
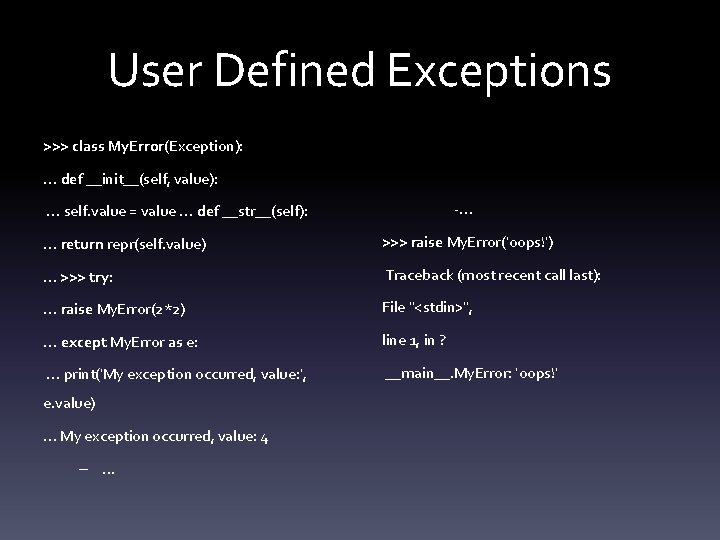
User Defined Exceptions >>> class My. Error(Exception): . . . def __init__(self, value): -… . . . self. value = value. . . def __str__(self): . . . return repr(self. value) >>> raise My. Error('oops!') . . . >>> try: Traceback (most recent call last): . . . raise My. Error(2*2) File "<stdin>", . . . except My. Error as e: line 1, in ? . . . print('My exception occurred, value: ', __main__. My. Error: 'oops!' e. value). . . My exception occurred, value: 4 – …
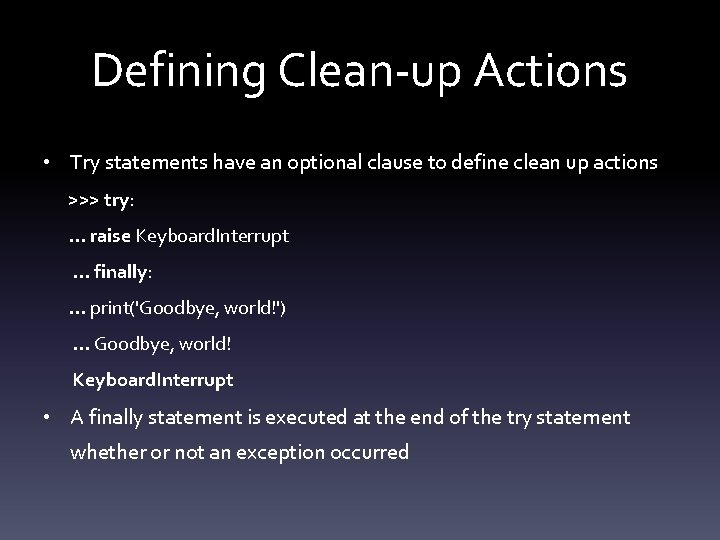
Defining Clean-up Actions • Try statements have an optional clause to define clean up actions >>> try: . . . raise Keyboard. Interrupt. . . finally: . . . print('Goodbye, world!'). . . Goodbye, world! Keyboard. Interrupt • A finally statement is executed at the end of the try statement whether or not an exception occurred
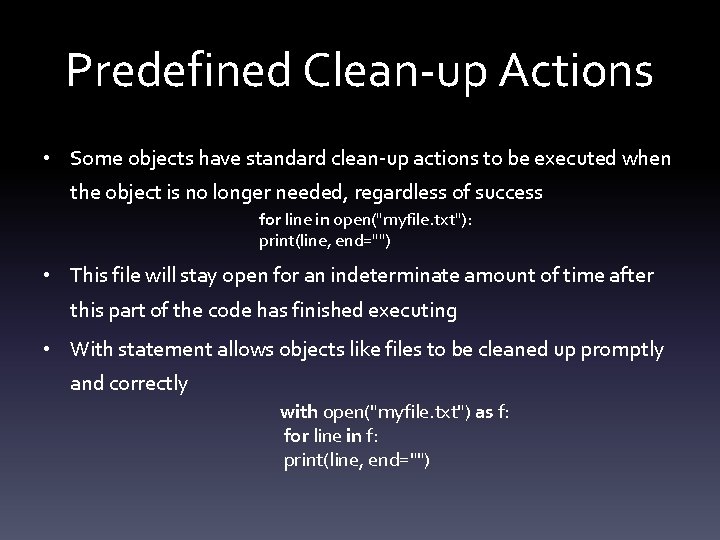
Predefined Clean-up Actions • Some objects have standard clean-up actions to be executed when the object is no longer needed, regardless of success for line in open("myfile. txt"): print(line, end="") • This file will stay open for an indeterminate amount of time after this part of the code has finished executing • With statement allows objects like files to be cleaned up promptly and correctly with open("myfile. txt") as f: for line in f: print(line, end="")
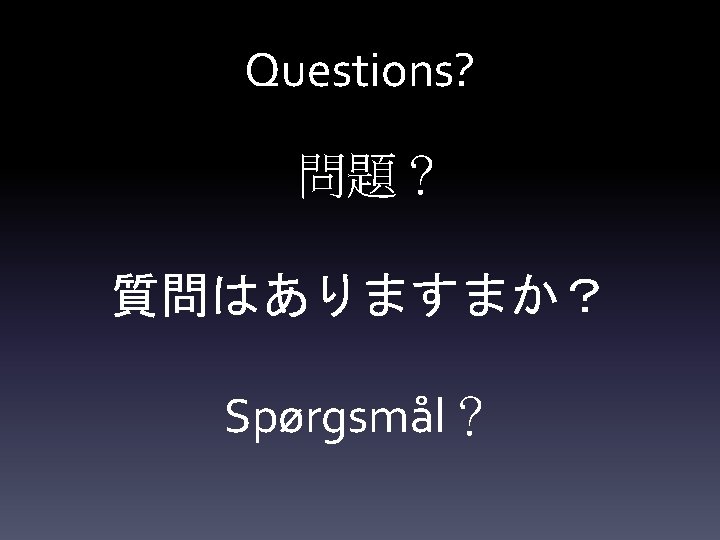
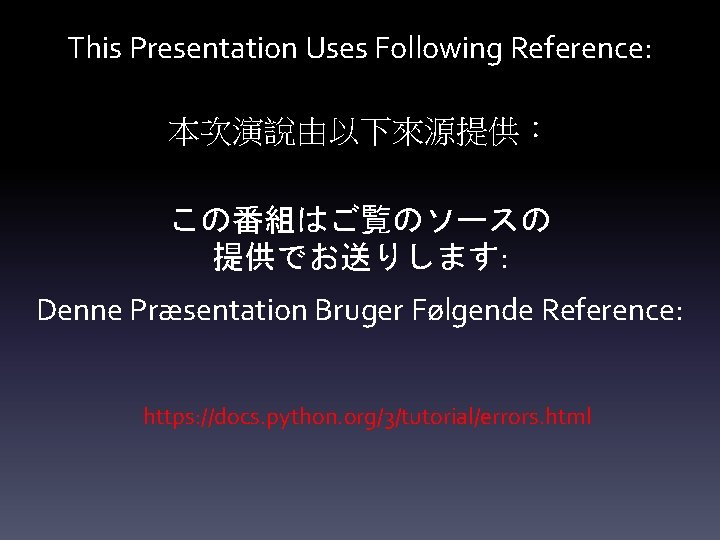
This Presentation Uses Following Reference: 本次演說由以下來源提供: この番組はご覧のソースの 提供でお送りします: Denne Præsentation Bruger Følgende Reference: https: //docs. python. org/3/tutorial/errors. html