Push Counter java Push Counter java Authors LewisLoftus
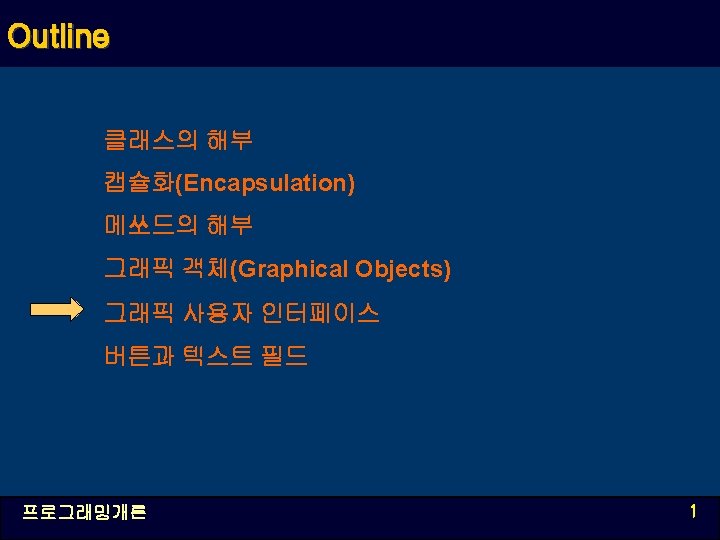
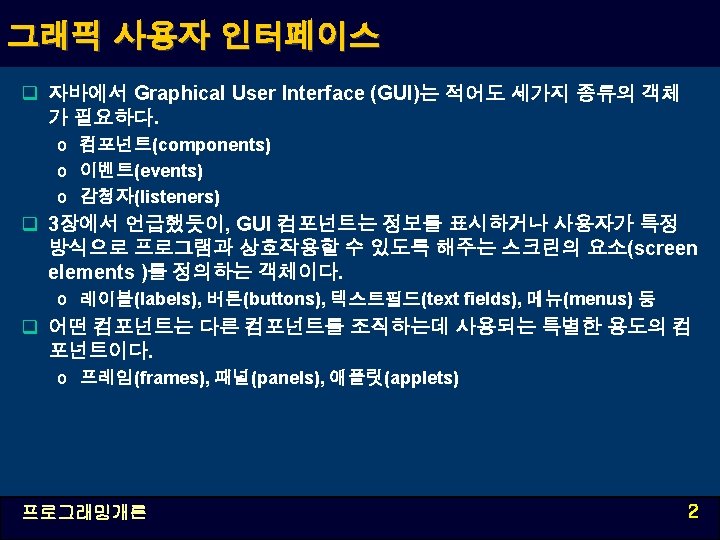
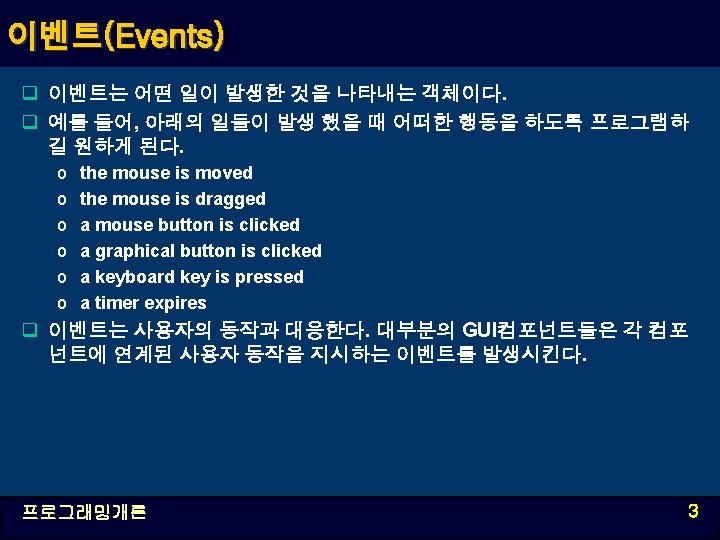
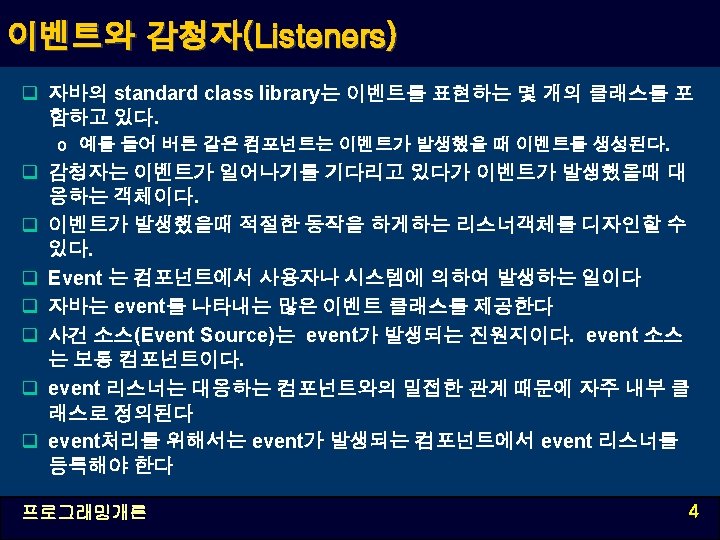
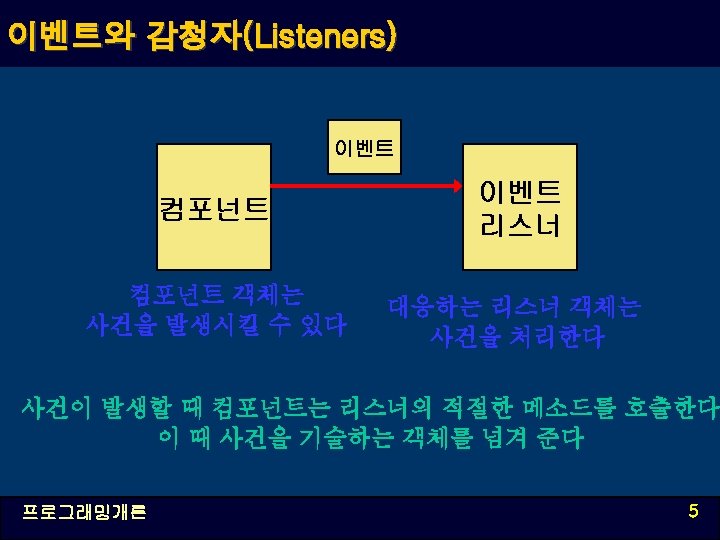
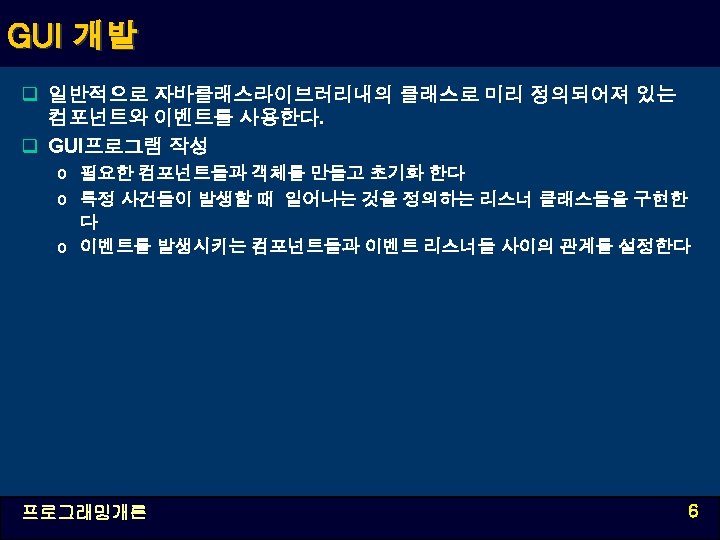
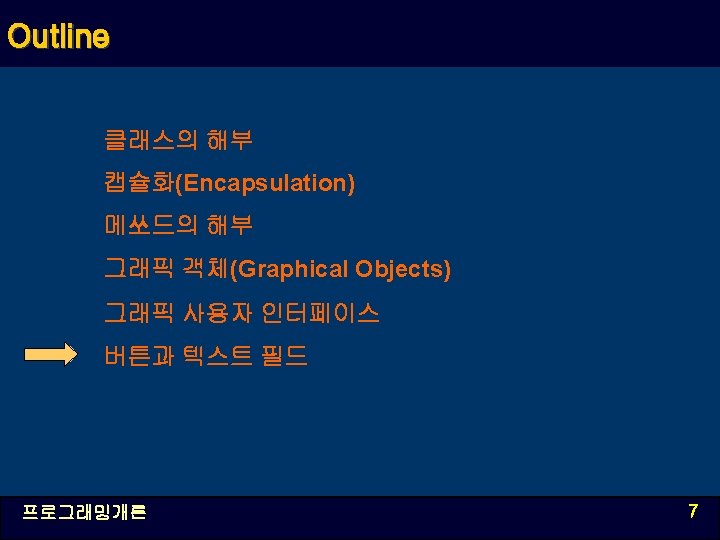
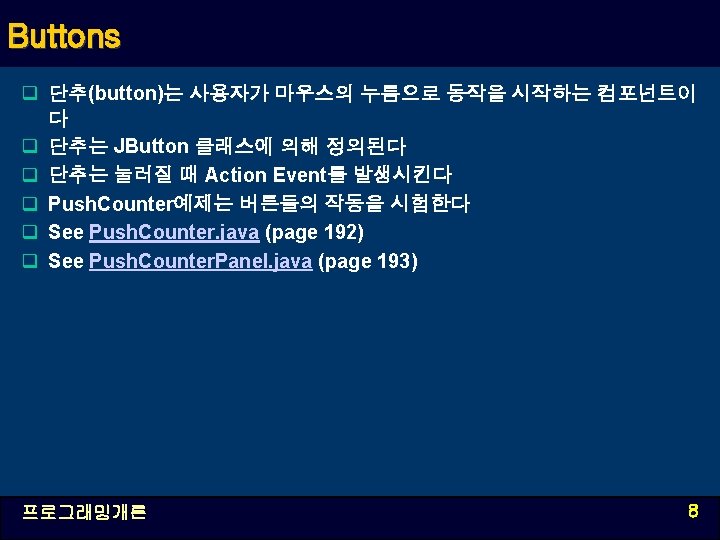
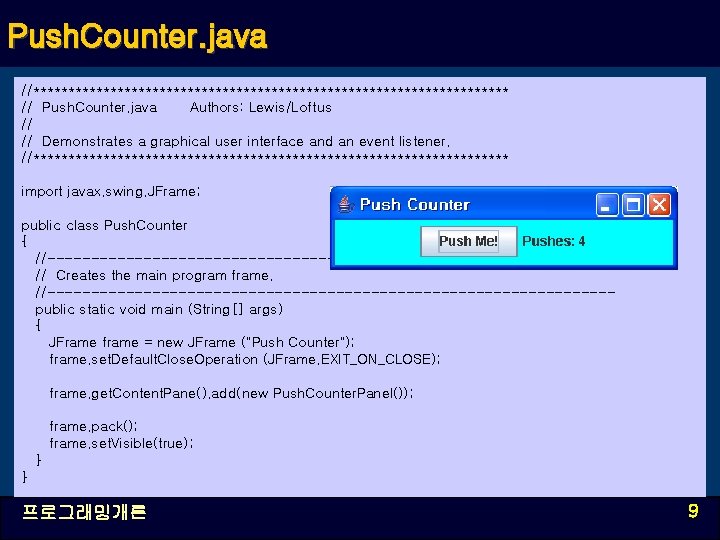
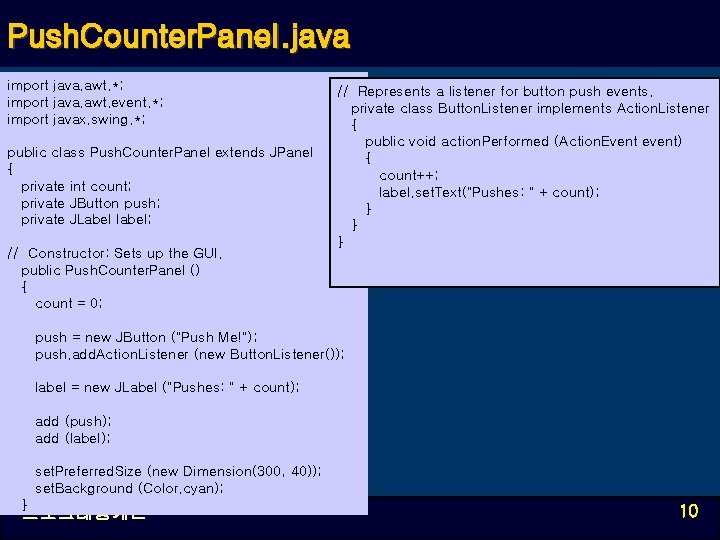
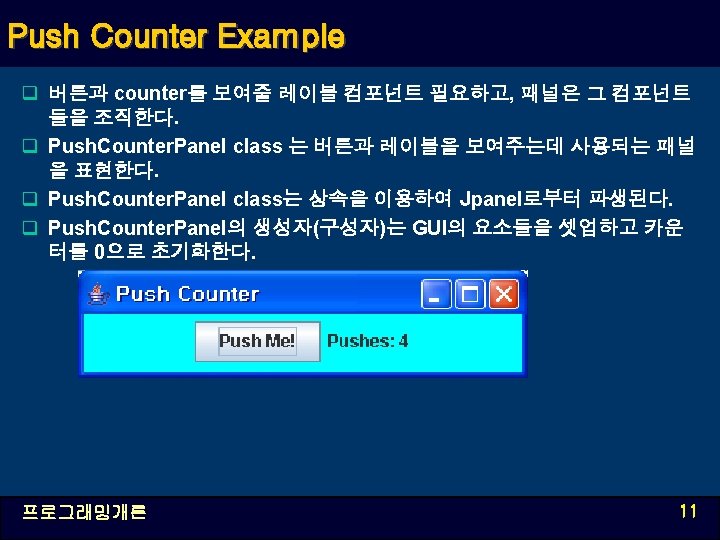
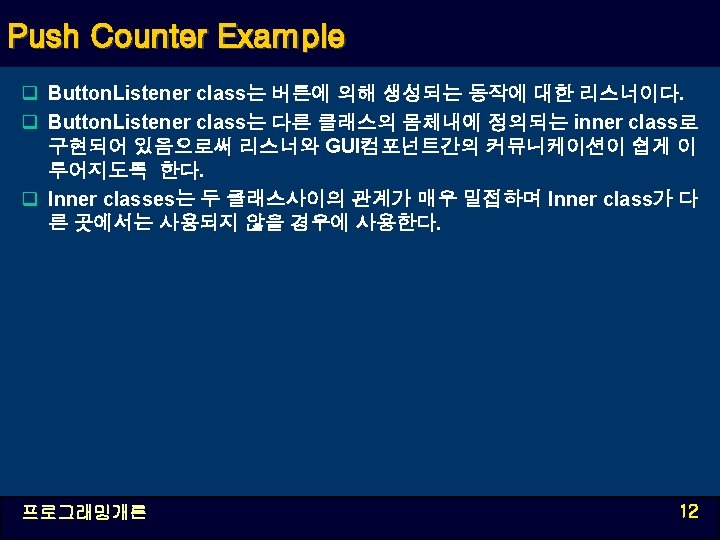
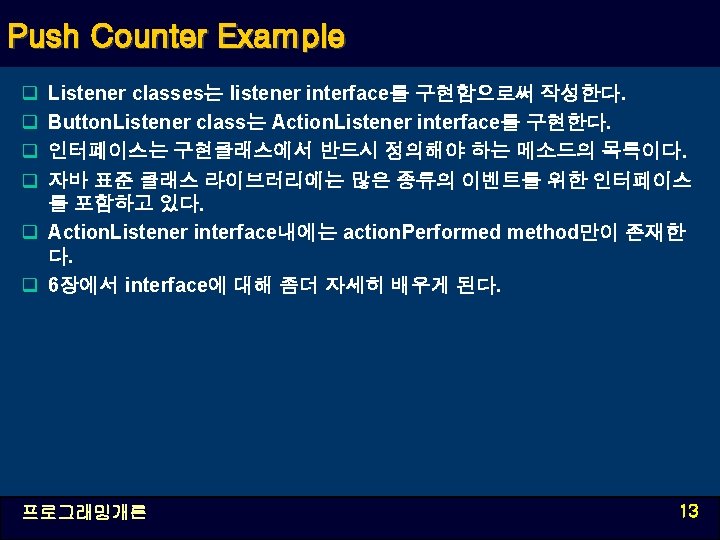
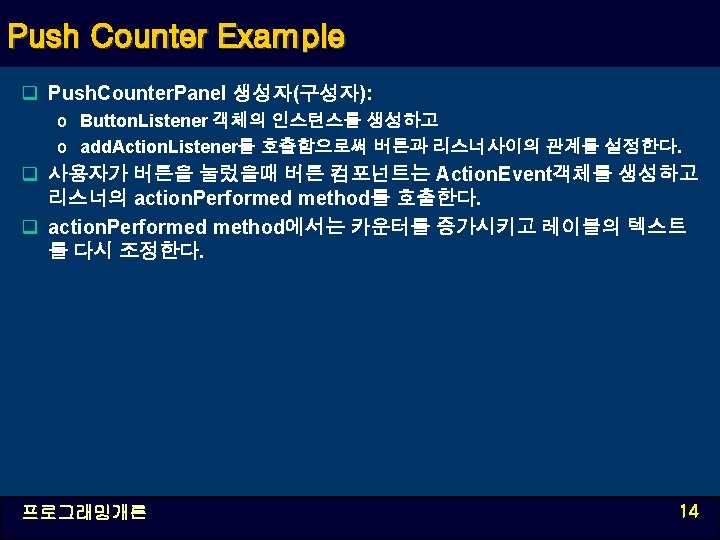
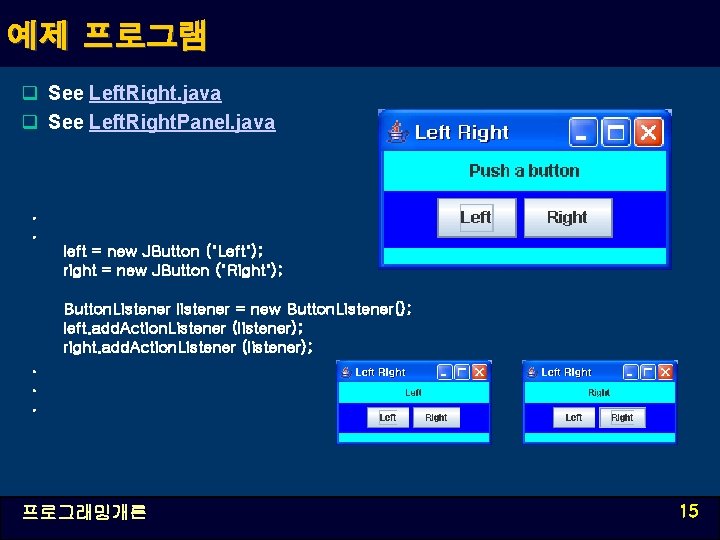
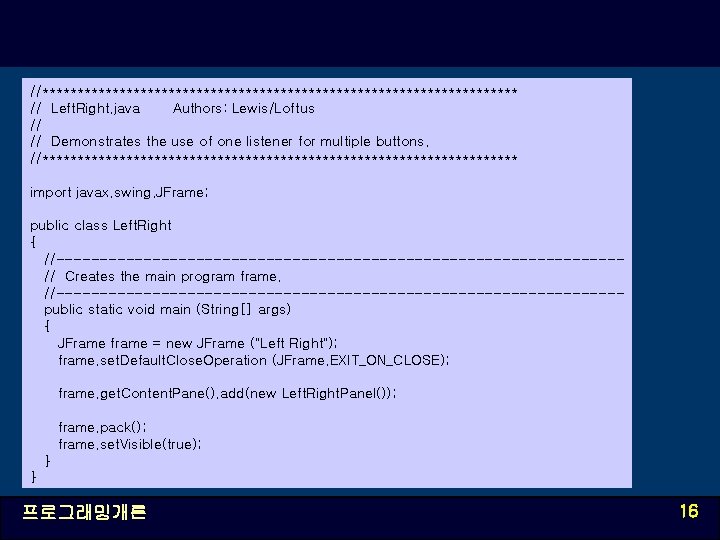
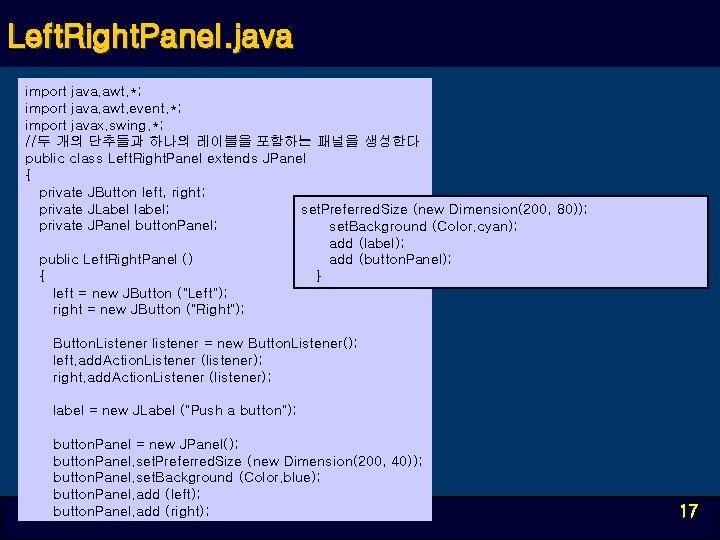
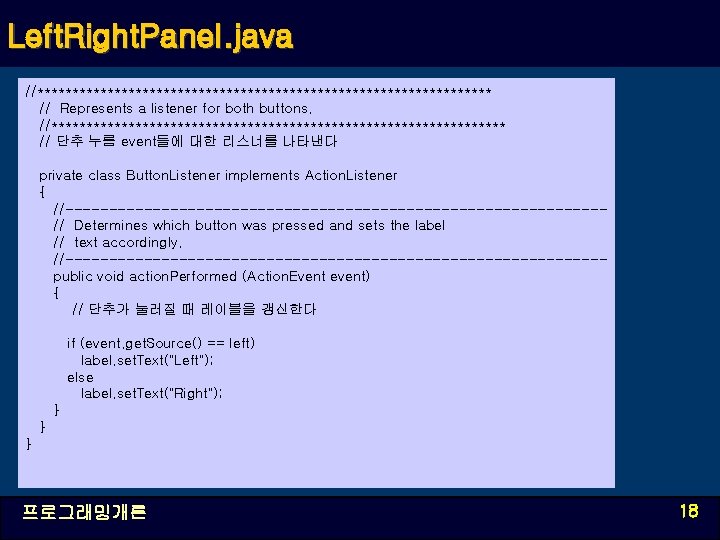
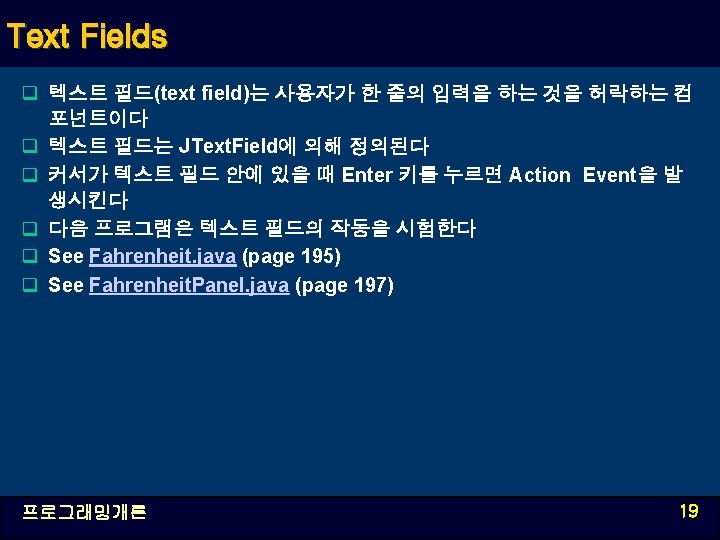
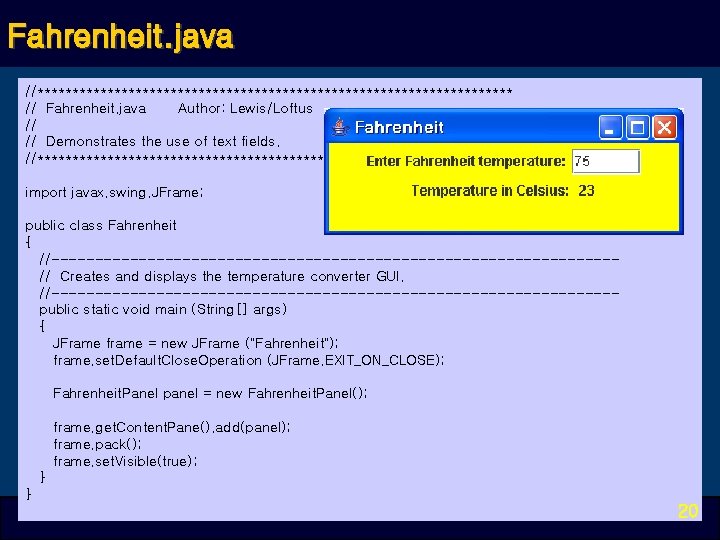
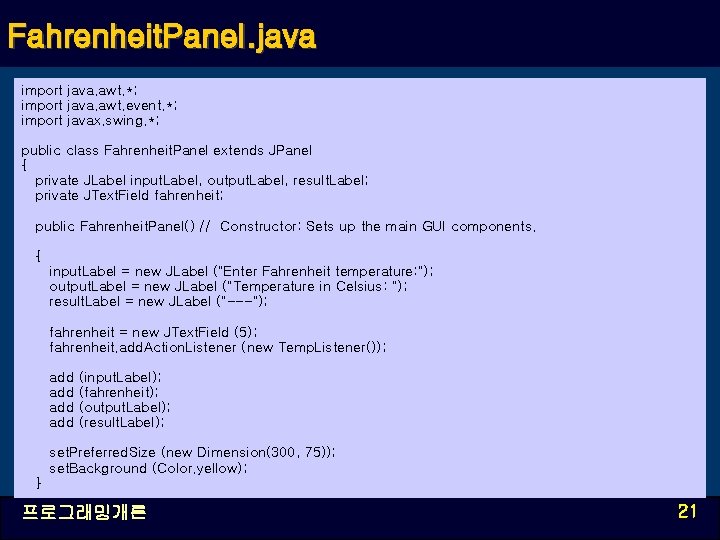
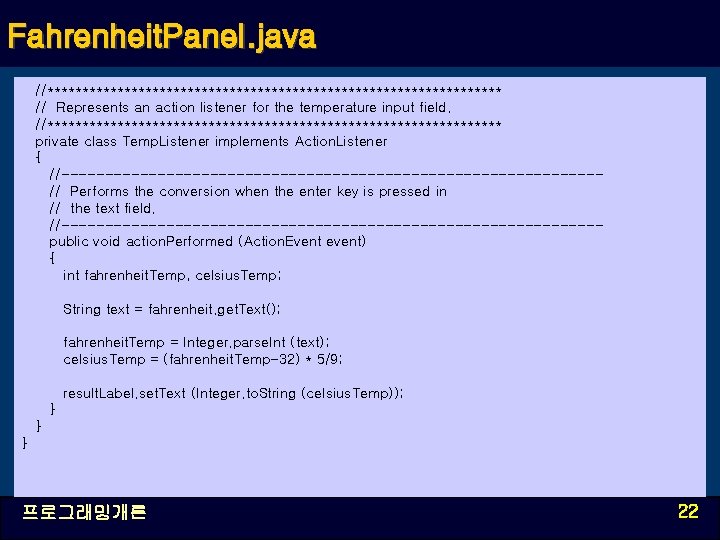
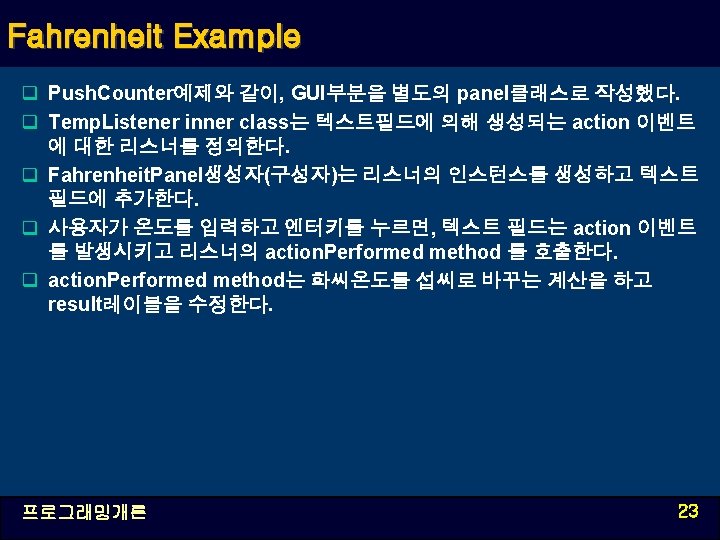
- Slides: 23
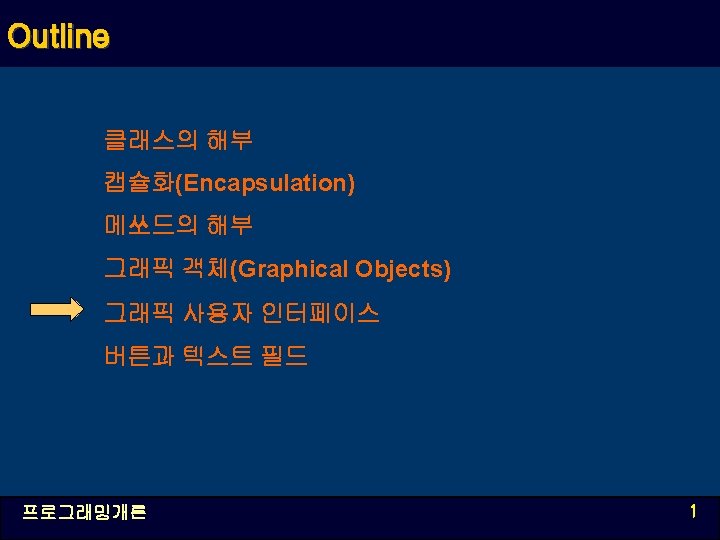
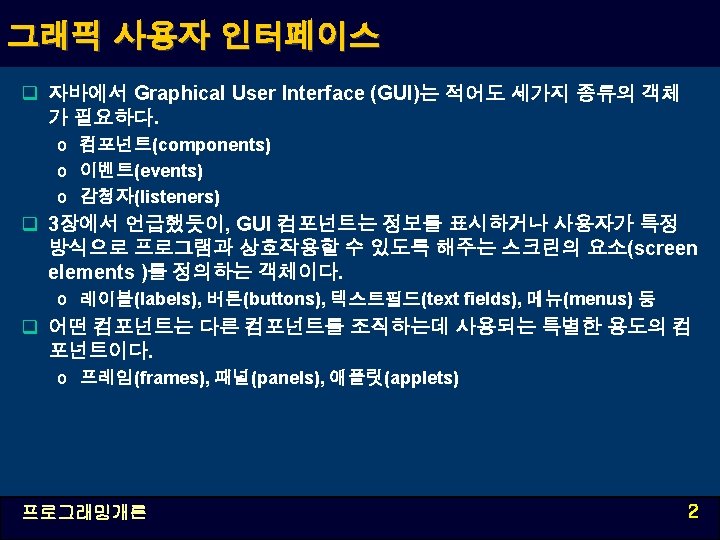
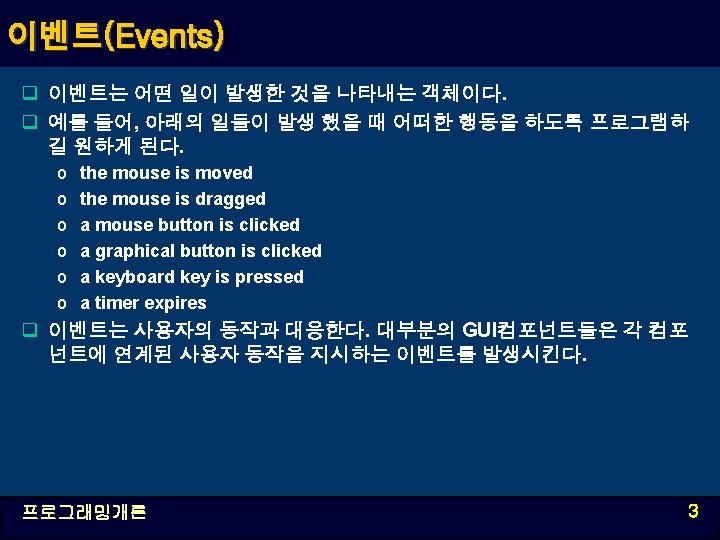
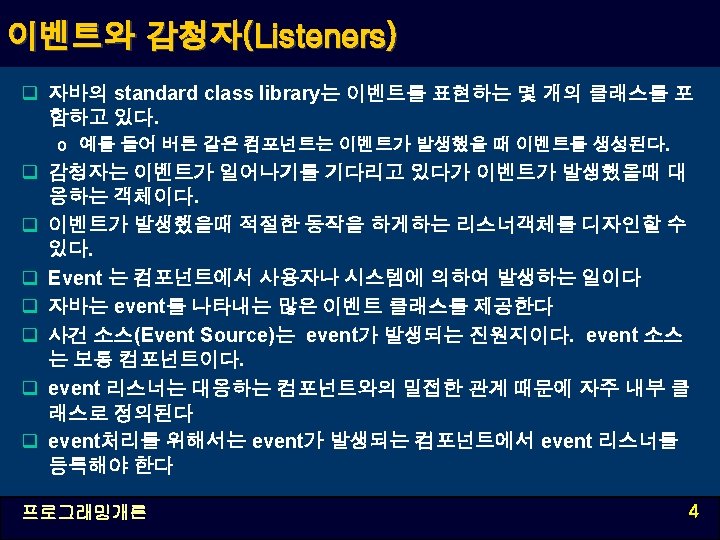
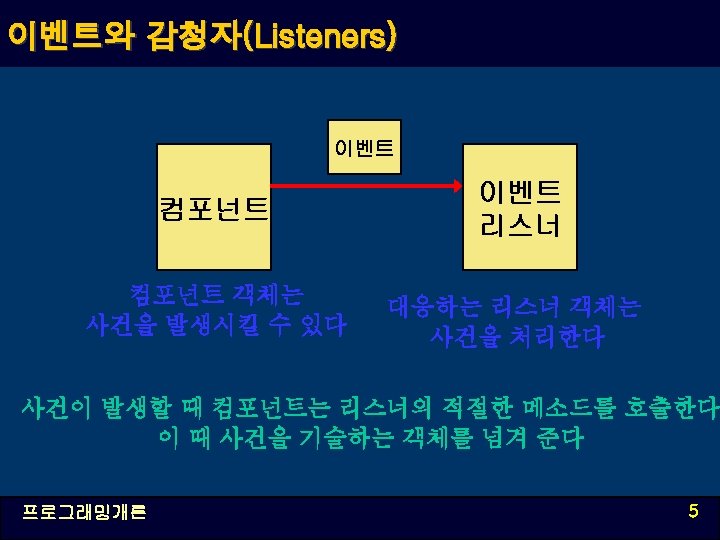
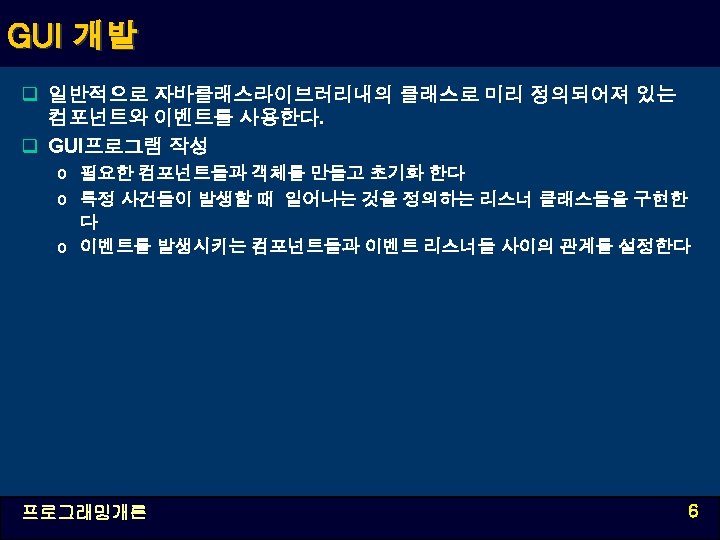
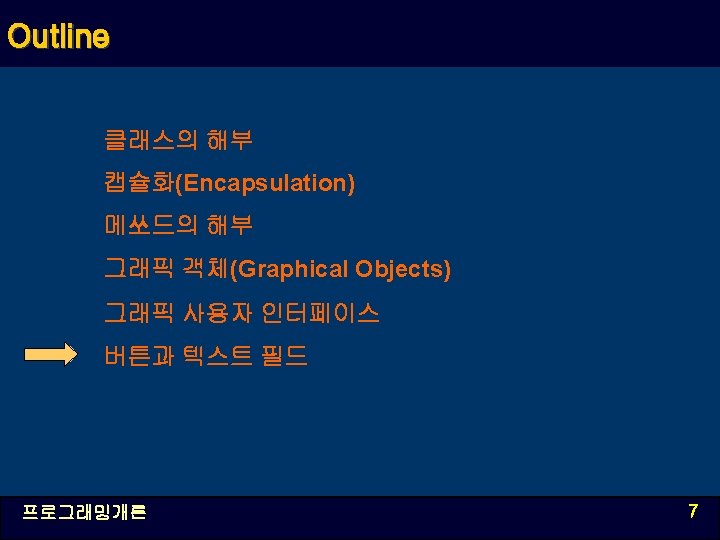
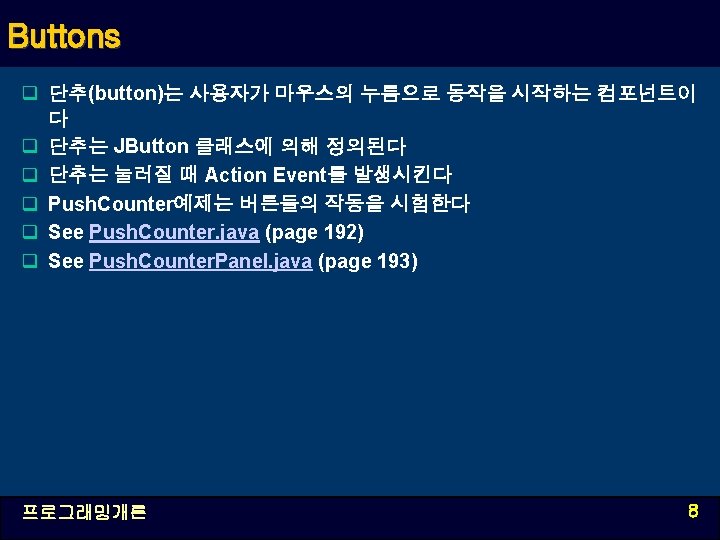
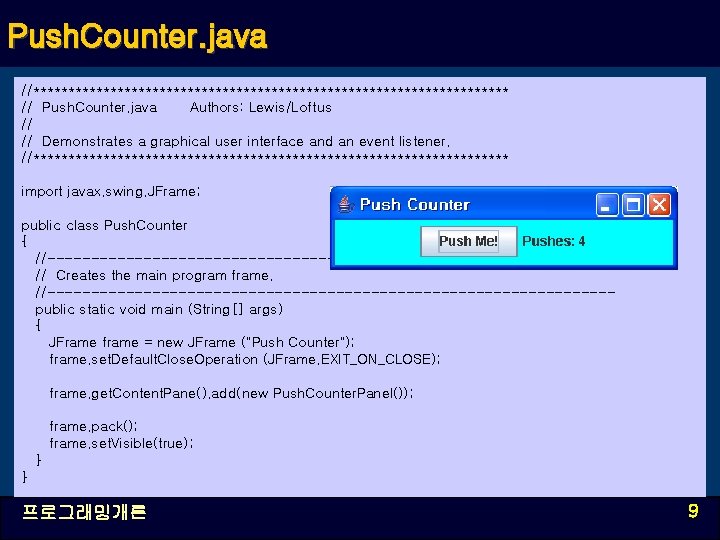
Push. Counter. java //********************************** // Push. Counter. java Authors: Lewis/Loftus // // Demonstrates a graphical user interface and an event listener. //********************************** import javax. swing. JFrame; public class Push. Counter { //--------------------------------// Creates the main program frame. //--------------------------------public static void main (String[] args) { JFrame frame = new JFrame ("Push Counter"); frame. set. Default. Close. Operation (JFrame. EXIT_ON_CLOSE); frame. get. Content. Pane(). add(new Push. Counter. Panel()); frame. pack(); frame. set. Visible(true); } } 프로그래밍개론 9
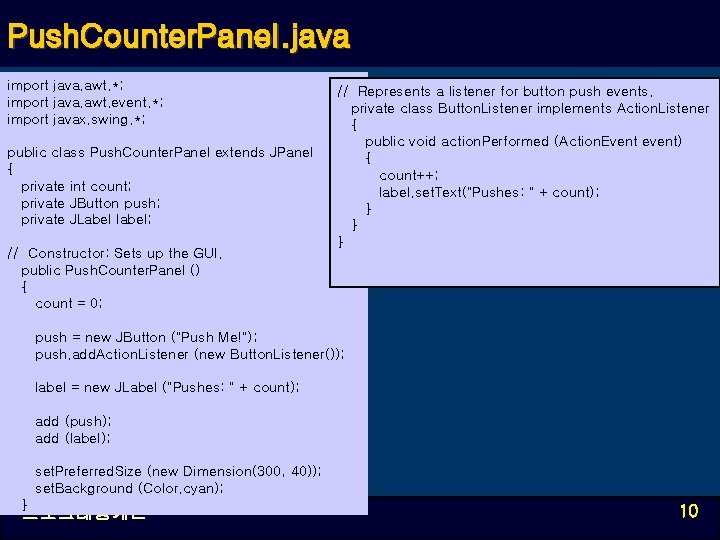
Push. Counter. Panel. java import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Push. Counter. Panel extends JPanel { private int count; private JButton push; private JLabel label; // Constructor: Sets up the GUI. public Push. Counter. Panel () { count = 0; // Represents a listener for button push events. private class Button. Listener implements Action. Listener { public void action. Performed (Action. Event event) { count++; label. set. Text("Pushes: " + count); } } } push = new JButton ("Push Me!"); push. add. Action. Listener (new Button. Listener()); label = new JLabel ("Pushes: " + count); add (push); add (label); set. Preferred. Size (new Dimension(300, 40)); set. Background (Color. cyan); } 프로그래밍개론 10
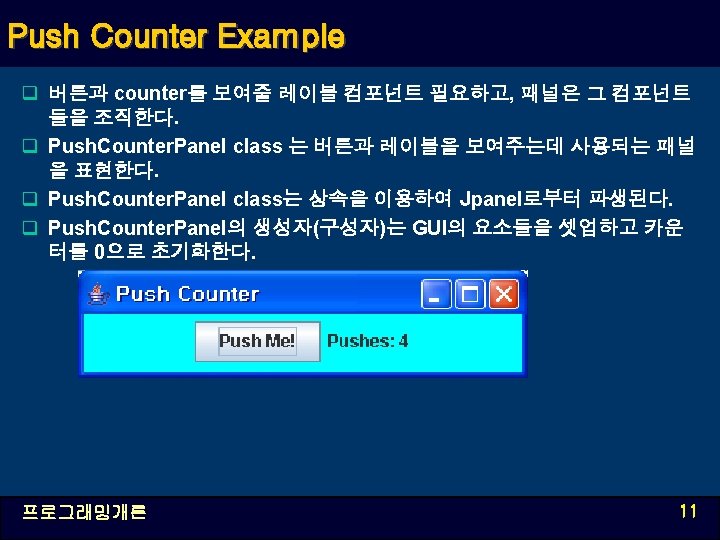
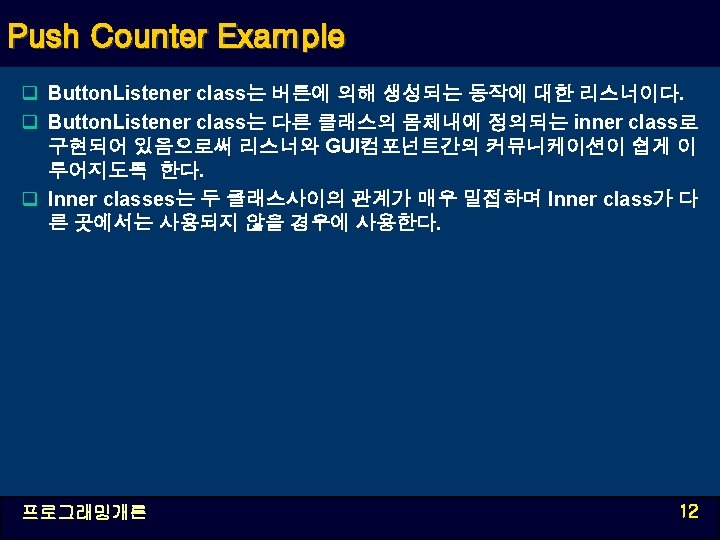
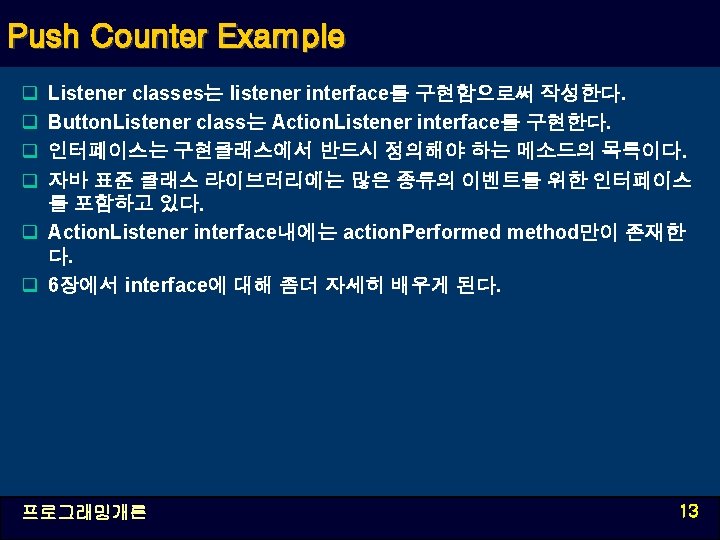
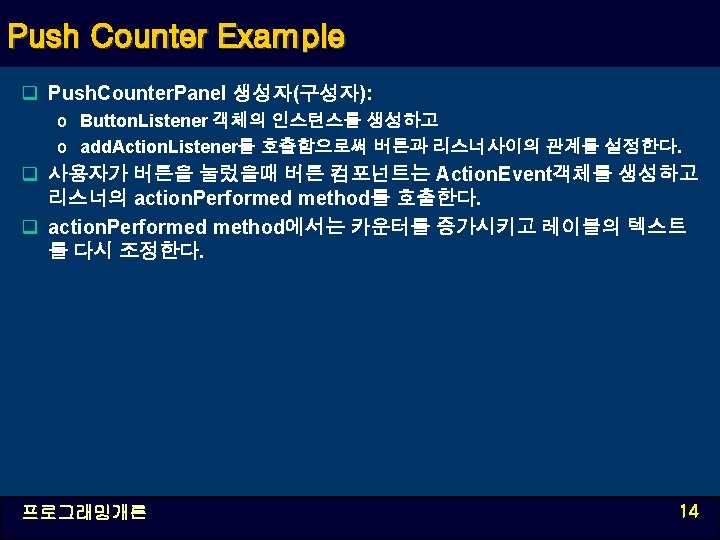
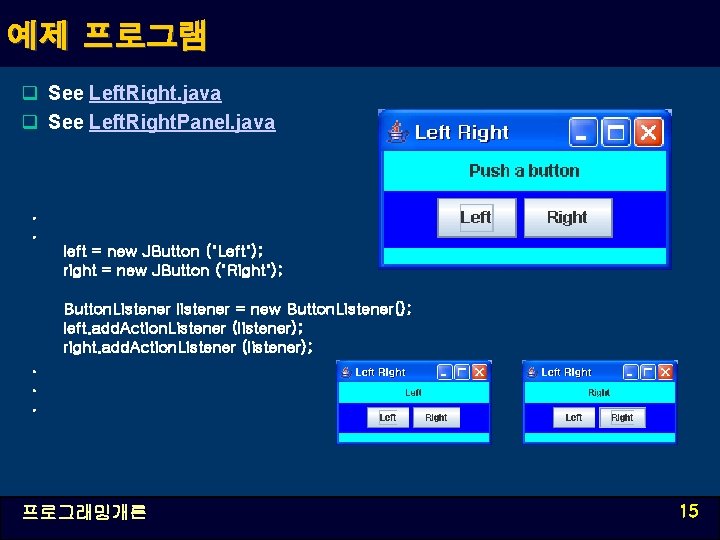
예제 프로그램 q See Left. Right. java q See Left. Right. Panel. java . . left = new JButton ("Left"); right = new JButton ("Right"); Button. Listener listener = new Button. Listener(); left. add. Action. Listener (listener); right. add. Action. Listener (listener); . . . 프로그래밍개론 15
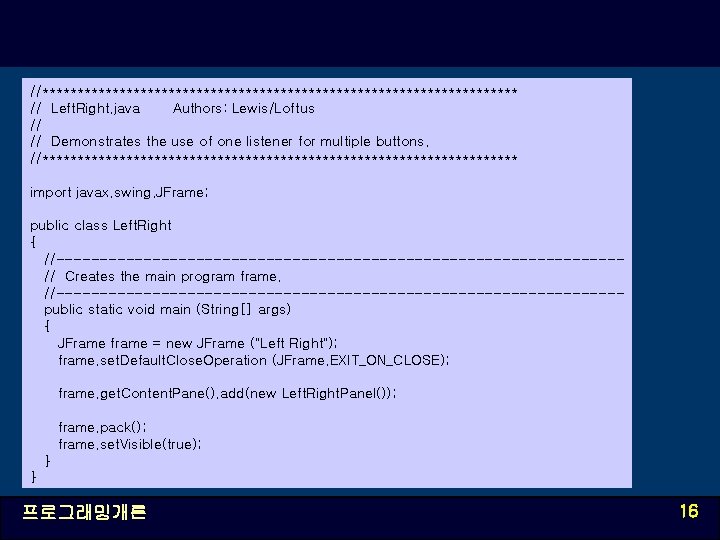
//********************************** // Left. Right. java Authors: Lewis/Loftus // // Demonstrates the use of one listener for multiple buttons. //********************************** import javax. swing. JFrame; public class Left. Right { //--------------------------------// Creates the main program frame. //--------------------------------public static void main (String[] args) { JFrame frame = new JFrame ("Left Right"); frame. set. Default. Close. Operation (JFrame. EXIT_ON_CLOSE); frame. get. Content. Pane(). add(new Left. Right. Panel()); frame. pack(); frame. set. Visible(true); } } 프로그래밍개론 16
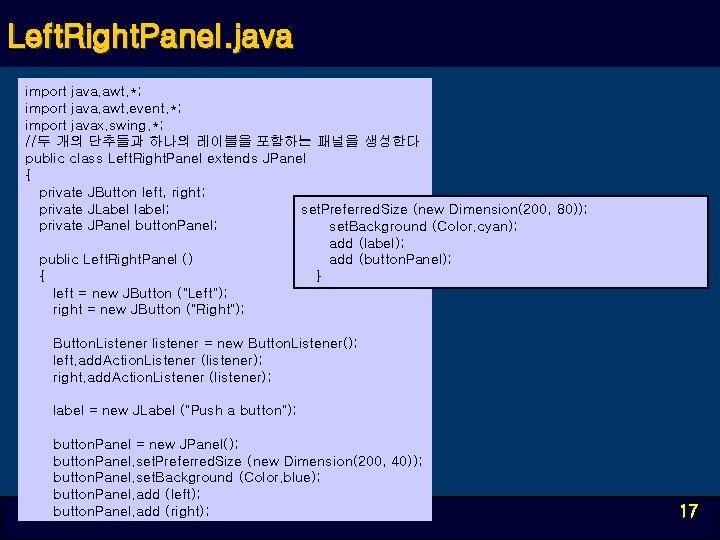
Left. Right. Panel. java import java. awt. *; import java. awt. event. *; import javax. swing. *; //두 개의 단추들과 하나의 레이블을 포함하는 패널을 생성한다 public class Left. Right. Panel extends JPanel { private JButton left, right; private JLabel label; set. Preferred. Size (new Dimension(200, 80)); private JPanel button. Panel; set. Background (Color. cyan); add (label); public Left. Right. Panel () add (button. Panel); { } left = new JButton ("Left"); right = new JButton ("Right"); Button. Listener listener = new Button. Listener(); left. add. Action. Listener (listener); right. add. Action. Listener (listener); label = new JLabel ("Push a button"); button. Panel = new JPanel(); button. Panel. set. Preferred. Size (new Dimension(200, 40)); button. Panel. set. Background (Color. blue); button. Panel. add (left); button. Panel. add (right); 프로그래밍개론 17
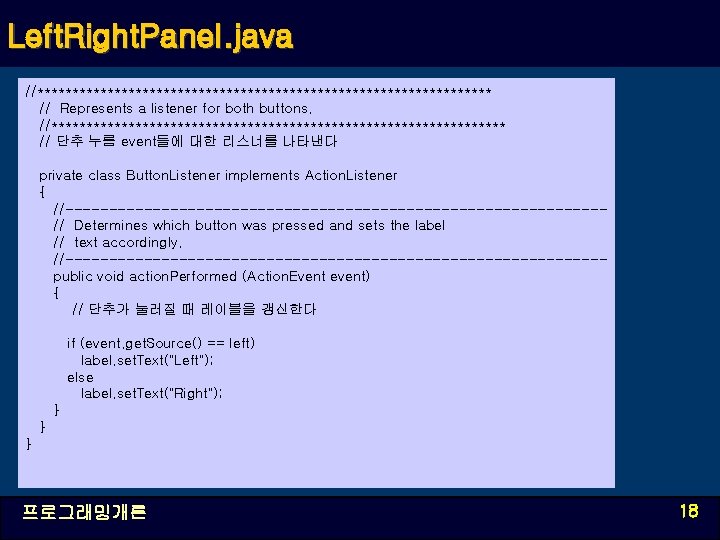
Left. Right. Panel. java //********************************* // Represents a listener for both buttons. //********************************* // 단추 누름 event들에 대한 리스너를 나타낸다 private class Button. Listener implements Action. Listener { //-------------------------------// Determines which button was pressed and sets the label // text accordingly. //-------------------------------public void action. Performed (Action. Event event) { // 단추가 눌러질 때 레이블을 갱신한다 if (event. get. Source() == left) label. set. Text("Left"); else label. set. Text("Right"); } } } 프로그래밍개론 18
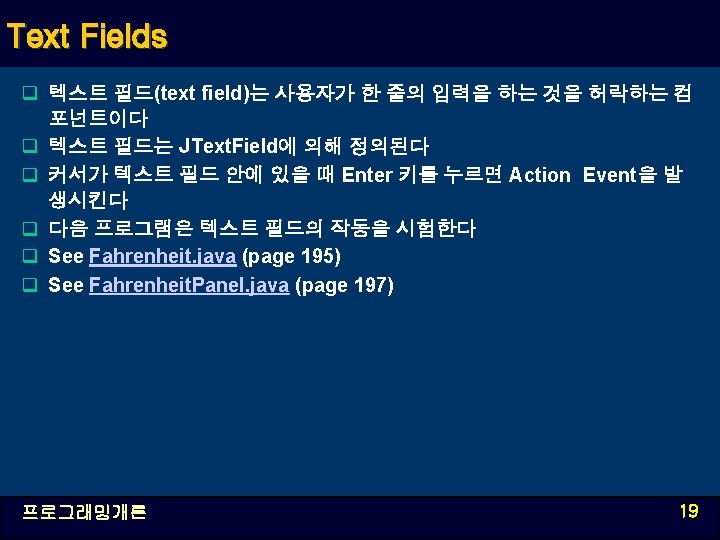
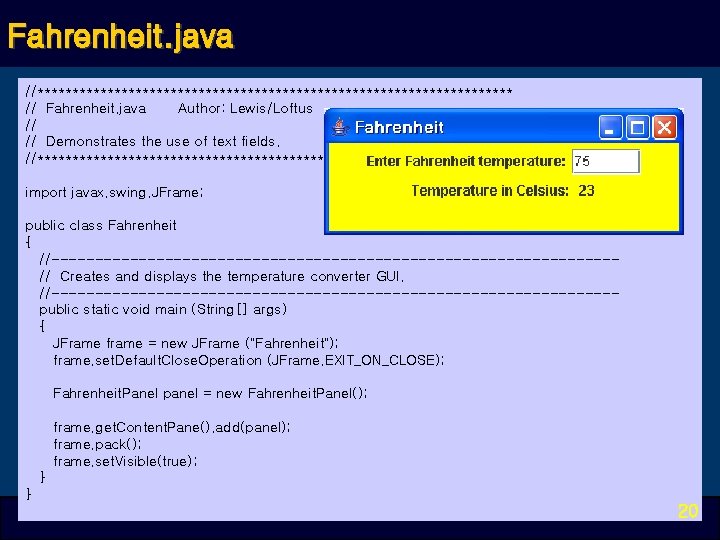
Fahrenheit. java //********************************** // Fahrenheit. java Author: Lewis/Loftus // // Demonstrates the use of text fields. //********************************** import javax. swing. JFrame; public class Fahrenheit { //--------------------------------// Creates and displays the temperature converter GUI. //--------------------------------public static void main (String[] args) { JFrame frame = new JFrame ("Fahrenheit"); frame. set. Default. Close. Operation (JFrame. EXIT_ON_CLOSE); Fahrenheit. Panel panel = new Fahrenheit. Panel(); frame. get. Content. Pane(). add(panel); frame. pack(); frame. set. Visible(true); } } 프로그래밍개론 20
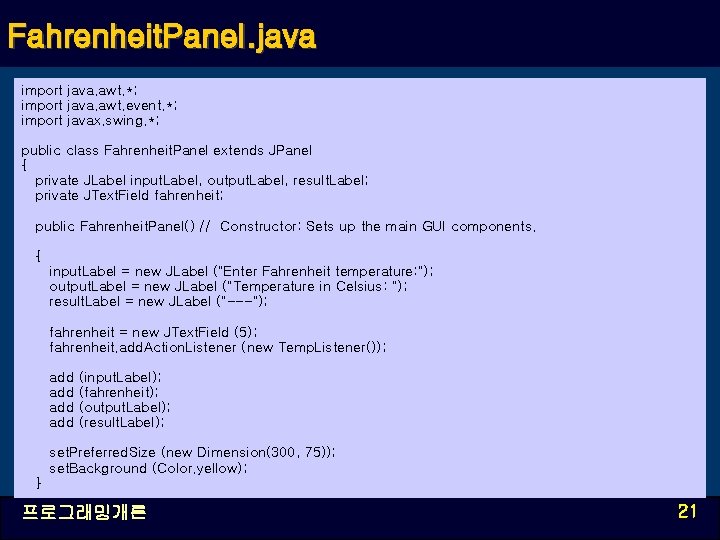
Fahrenheit. Panel. java import java. awt. *; import java. awt. event. *; import javax. swing. *; public class Fahrenheit. Panel extends JPanel { private JLabel input. Label, output. Label, result. Label; private JText. Field fahrenheit; public Fahrenheit. Panel() // Constructor: Sets up the main GUI components. { input. Label = new JLabel ("Enter Fahrenheit temperature: "); output. Label = new JLabel ("Temperature in Celsius: "); result. Label = new JLabel ("---"); fahrenheit = new JText. Field (5); fahrenheit. add. Action. Listener (new Temp. Listener()); add add (input. Label); (fahrenheit); (output. Label); (result. Label); set. Preferred. Size (new Dimension(300, 75)); set. Background (Color. yellow); } 프로그래밍개론 21
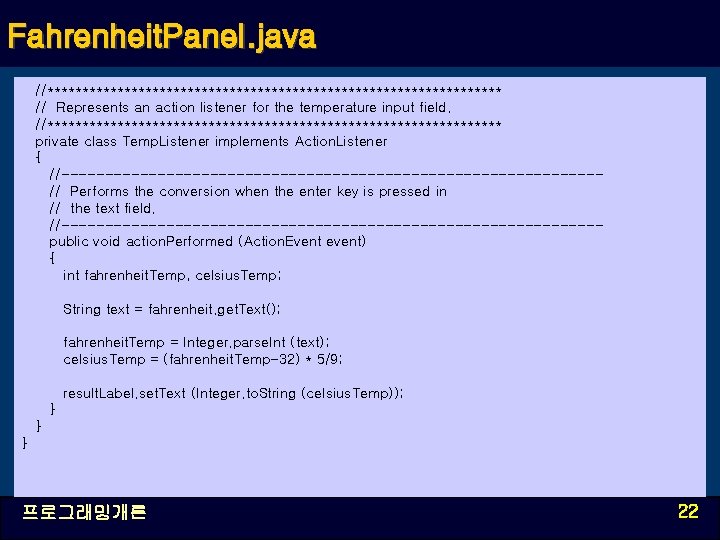
Fahrenheit. Panel. java //********************************* // Represents an action listener for the temperature input field. //********************************* private class Temp. Listener implements Action. Listener { //-------------------------------// Performs the conversion when the enter key is pressed in // the text field. //-------------------------------public void action. Performed (Action. Event event) { int fahrenheit. Temp, celsius. Temp; String text = fahrenheit. get. Text(); fahrenheit. Temp = Integer. parse. Int (text); celsius. Temp = (fahrenheit. Temp-32) * 5/9; result. Label. set. Text (Integer. to. String (celsius. Temp)); } } } 프로그래밍개론 22
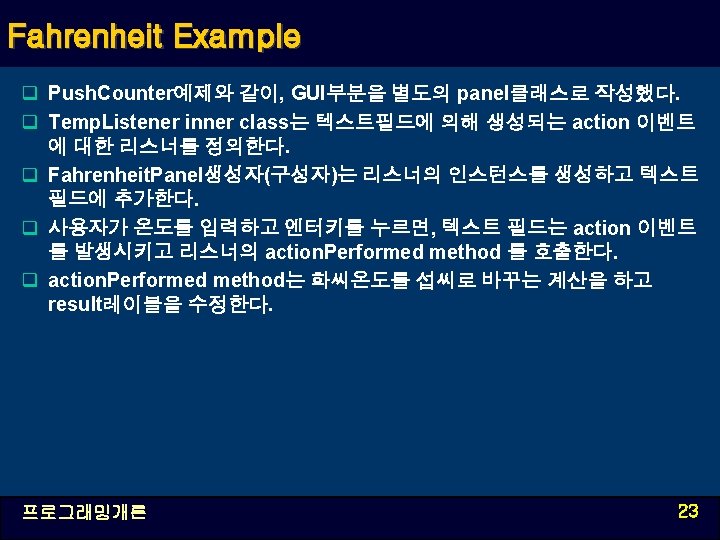
Push hard push fast fully recoil
Pea rhythm
Push hard push fast fully recoil
Push hard push fast fully recoil
Push hard push fast fully recoil
Asp medical
Counter control loop
American romanticism authors
Poster authors
A concrete object that represents something abstract
Apa et al in text
Inform persuade entertain examples
What is authors perspective
Authors purpose pie
Authors purpose practice
Identifying irony worksheet answer key
Author's bias worksheet
Whats the authors purpose
Working with context clues the raven
Postmodern authors
And authors viewpoint is the way in which he or she
Authors purpose and tone
Author's affiliation
Modern gothic literature vs traditional gothic