Project 4 Function Pointers ENEE 150 0102 ANDREW
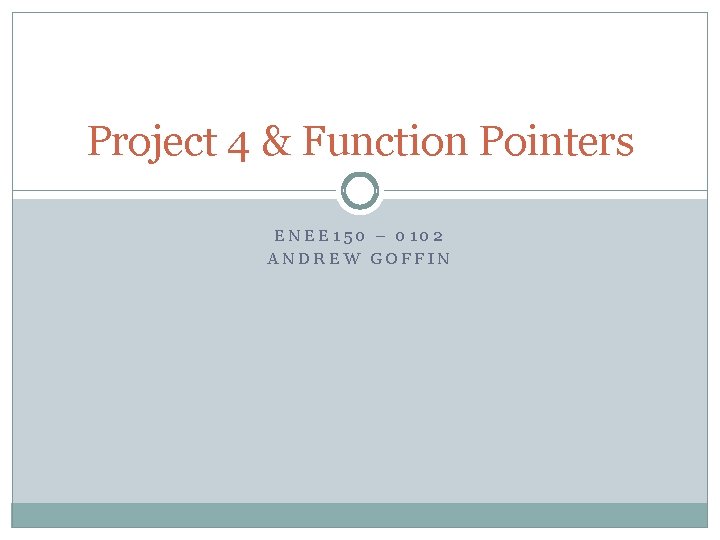
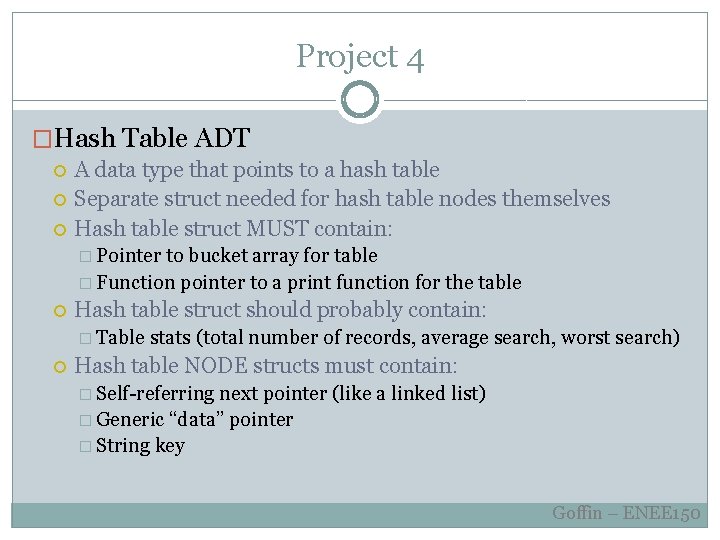
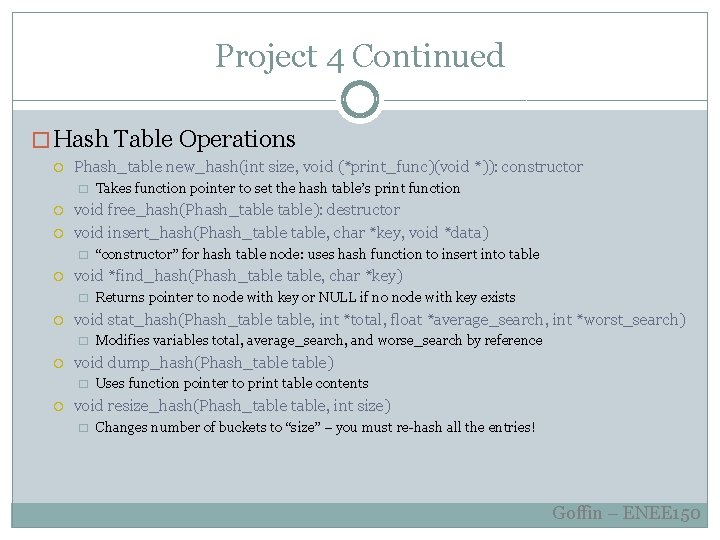
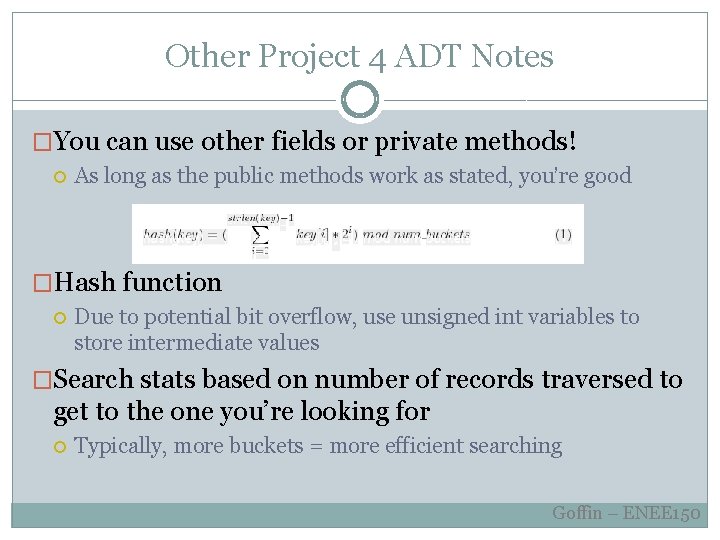
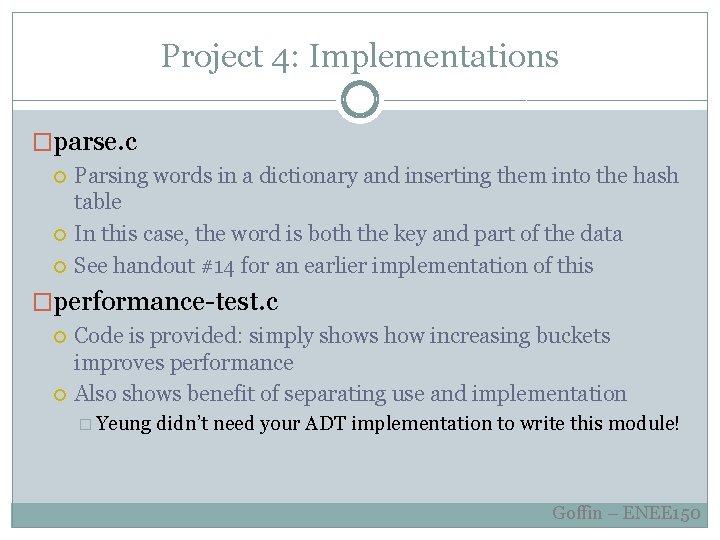
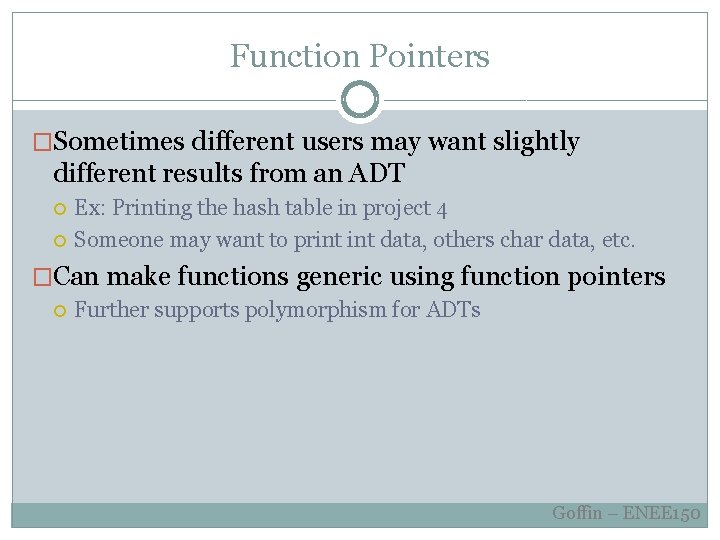
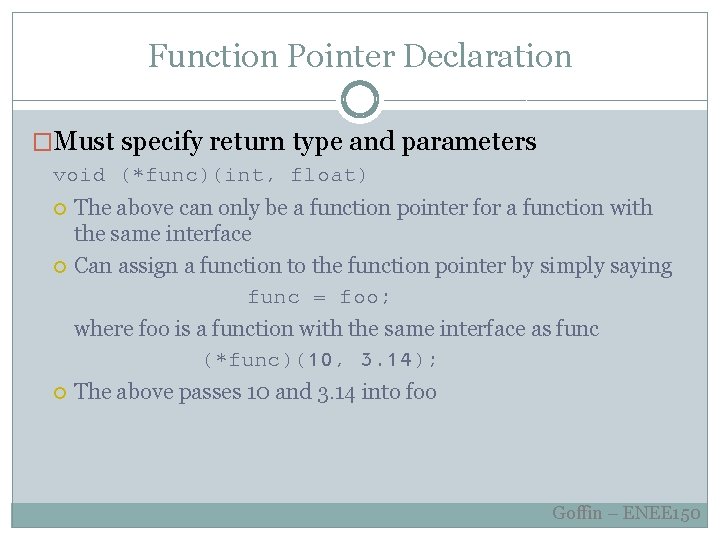
- Slides: 7
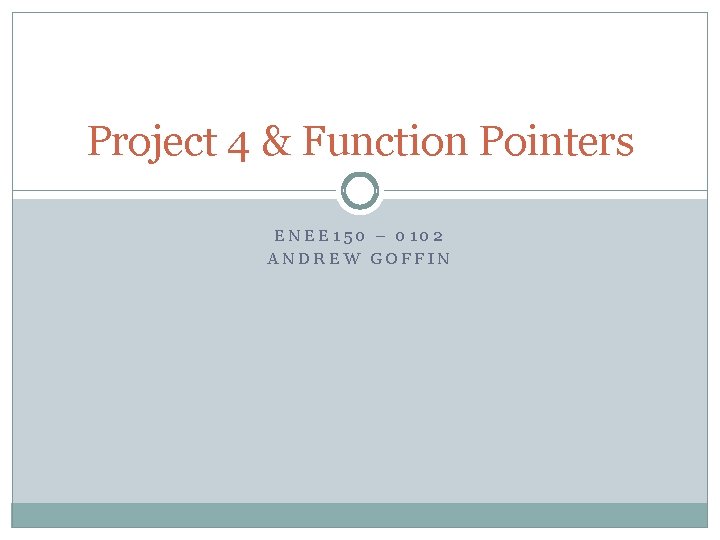
Project 4 & Function Pointers ENEE 150 – 0102 ANDREW GOFFIN
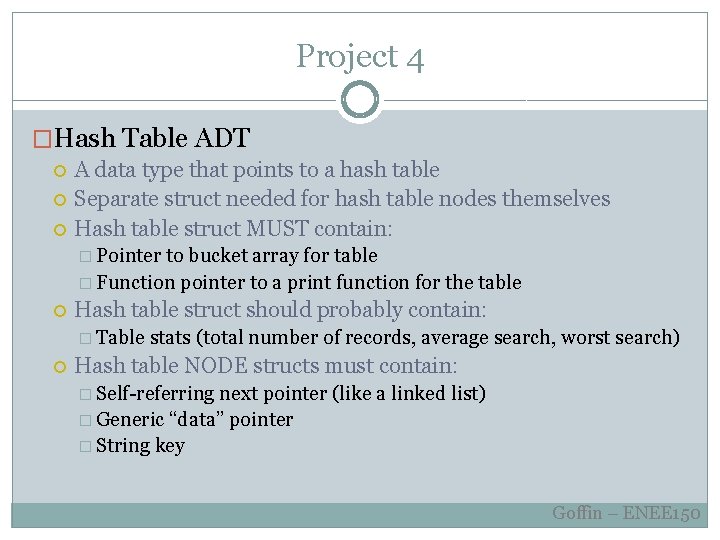
Project 4 �Hash Table ADT A data type that points to a hash table Separate struct needed for hash table nodes themselves Hash table struct MUST contain: � Pointer to bucket array for table � Function pointer to a print function for the table Hash table struct should probably contain: � Table stats (total number of records, average search, worst search) Hash table NODE structs must contain: � Self-referring next pointer (like a linked list) � Generic “data” pointer � String key Goffin – ENEE 150
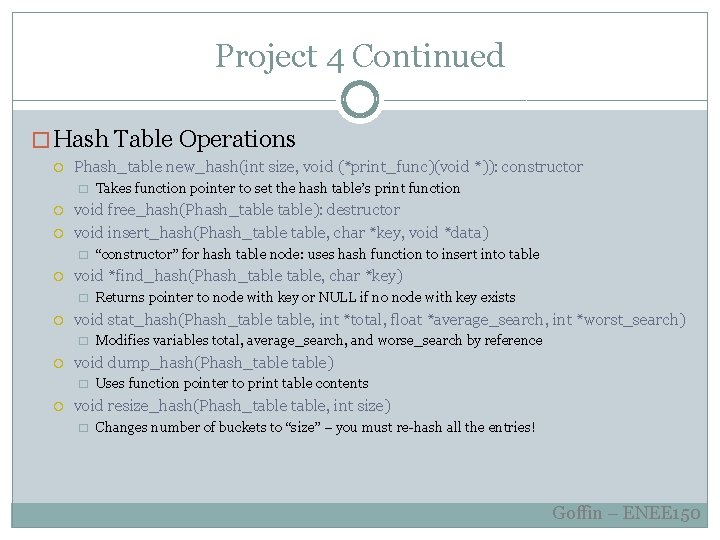
Project 4 Continued � Hash Table Operations Phash_table new_hash(int size, void (*print_func)(void *)): constructor � void free_hash(Phash_table): destructor void insert_hash(Phash_table, char *key, void *data) � Modifies variables total, average_search, and worse_search by reference void dump_hash(Phash_table) � Returns pointer to node with key or NULL if no node with key exists void stat_hash(Phash_table, int *total, float *average_search, int *worst_search) � “constructor” for hash table node: uses hash function to insert into table void *find_hash(Phash_table, char *key) � Takes function pointer to set the hash table’s print function Uses function pointer to print table contents void resize_hash(Phash_table, int size) � Changes number of buckets to “size” – you must re-hash all the entries! Goffin – ENEE 150
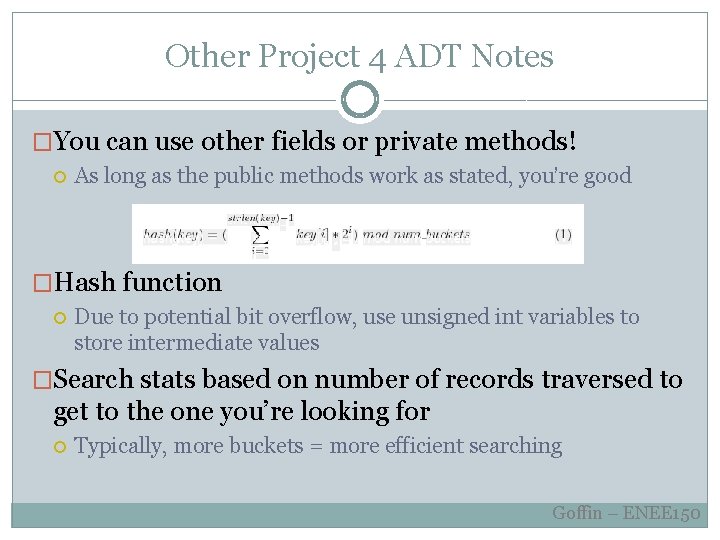
Other Project 4 ADT Notes �You can use other fields or private methods! As long as the public methods work as stated, you’re good �Hash function Due to potential bit overflow, use unsigned int variables to store intermediate values �Search stats based on number of records traversed to get to the one you’re looking for Typically, more buckets = more efficient searching Goffin – ENEE 150
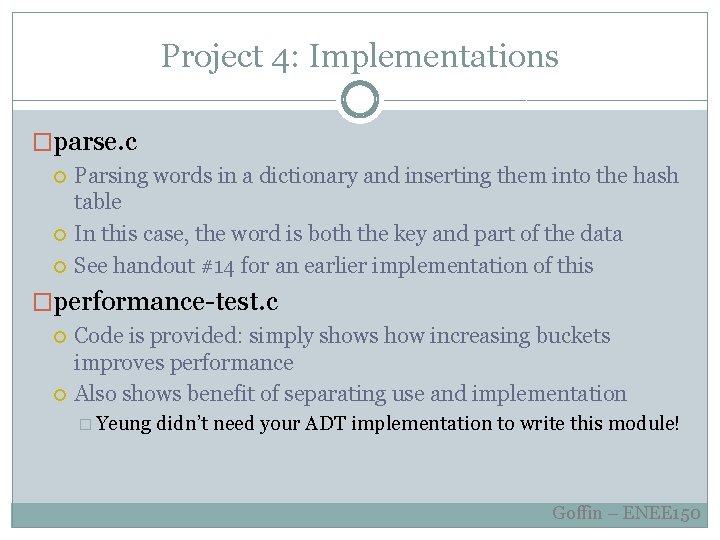
Project 4: Implementations �parse. c Parsing words in a dictionary and inserting them into the hash table In this case, the word is both the key and part of the data See handout #14 for an earlier implementation of this �performance-test. c Code is provided: simply shows how increasing buckets improves performance Also shows benefit of separating use and implementation � Yeung didn’t need your ADT implementation to write this module! Goffin – ENEE 150
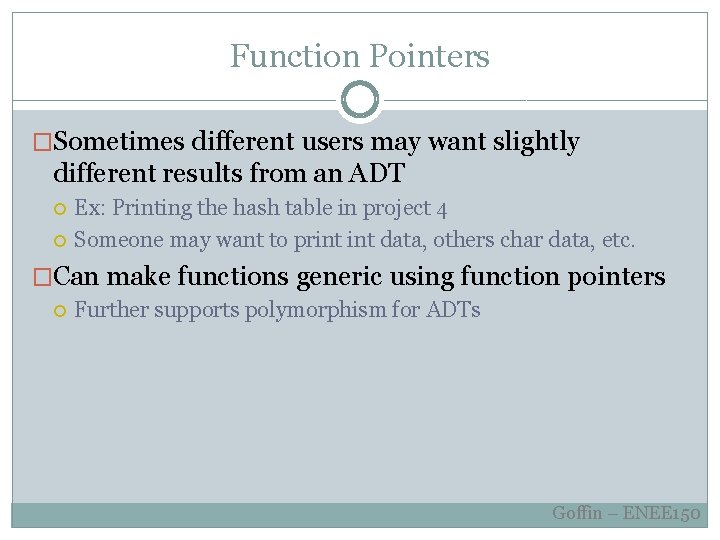
Function Pointers �Sometimes different users may want slightly different results from an ADT Ex: Printing the hash table in project 4 Someone may want to print data, others char data, etc. �Can make functions generic using function pointers Further supports polymorphism for ADTs Goffin – ENEE 150
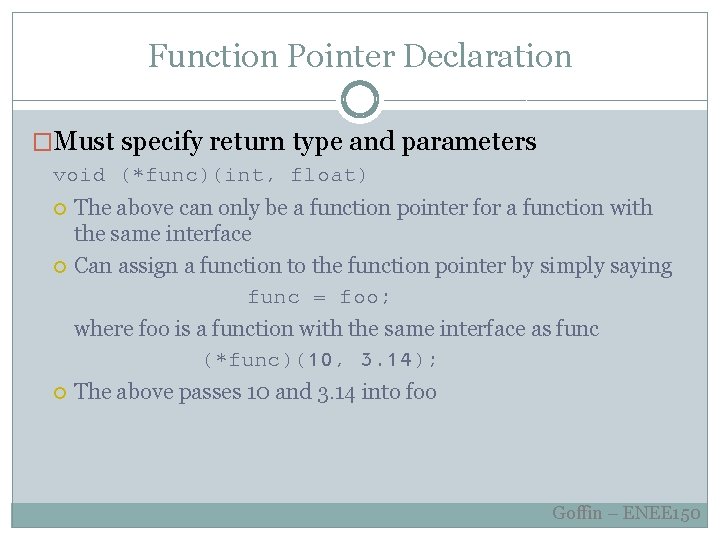
Function Pointer Declaration �Must specify return type and parameters void (*func)(int, float) The above can only be a function pointer for a function with the same interface Can assign a function to the function pointer by simply saying func = foo; where foo is a function with the same interface as func (*func)(10, 3. 14); The above passes 10 and 3. 14 into foo Goffin – ENEE 150