Programming with Open GL Part 3 Three Dimensions
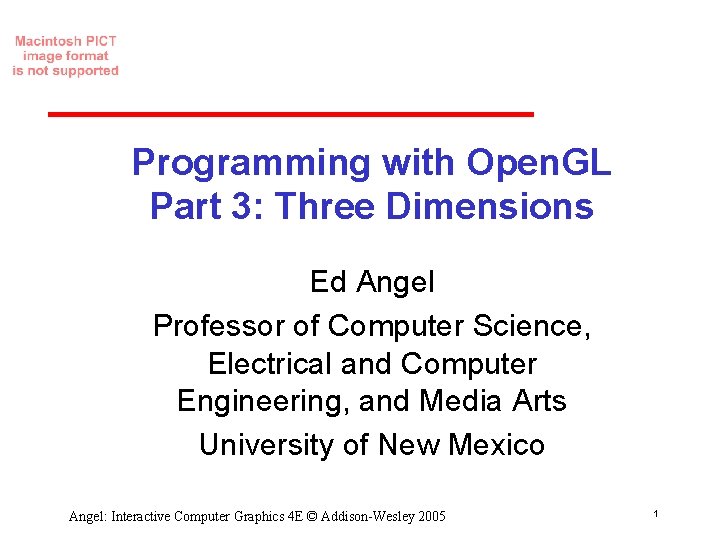
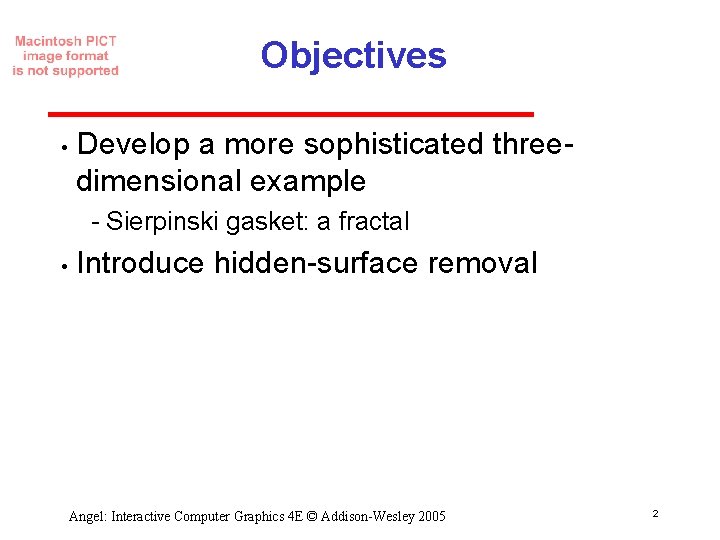
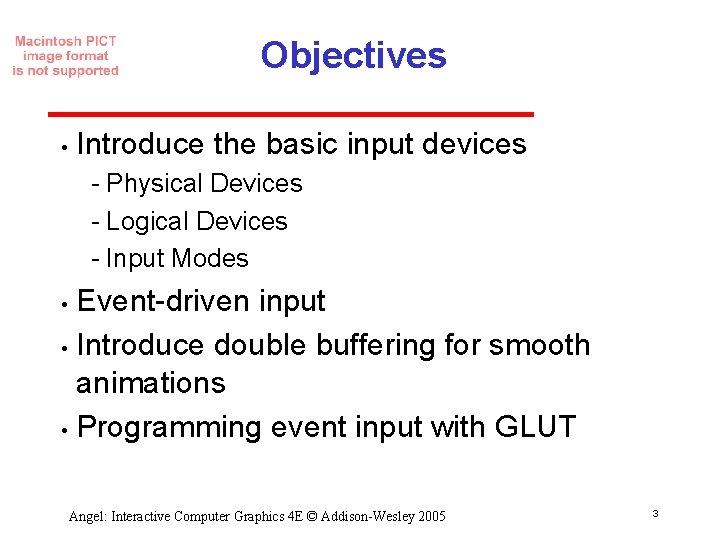
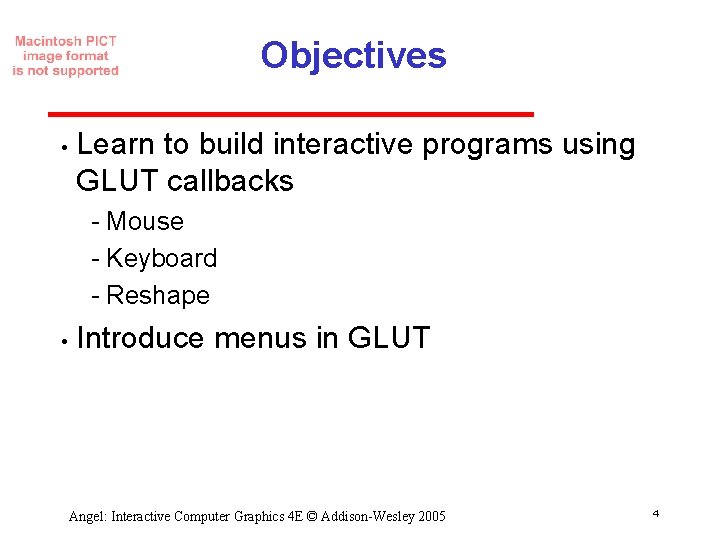
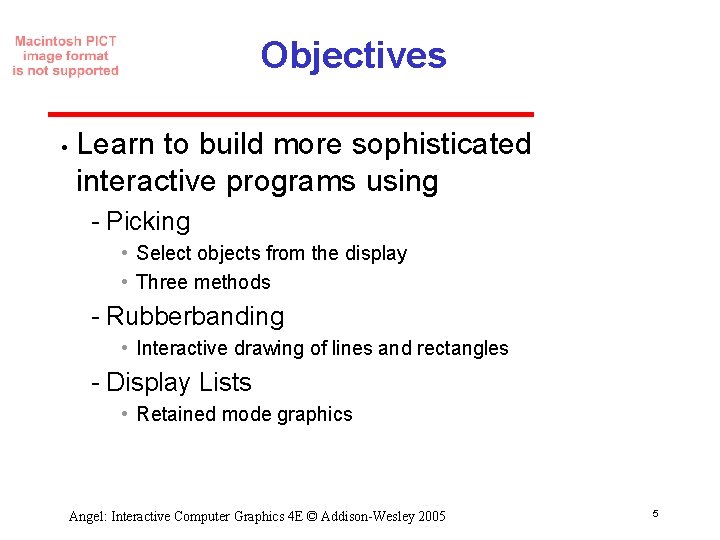
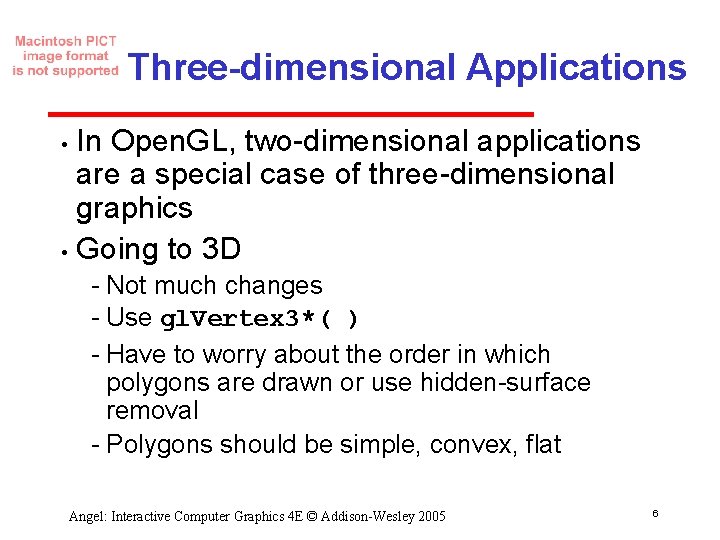
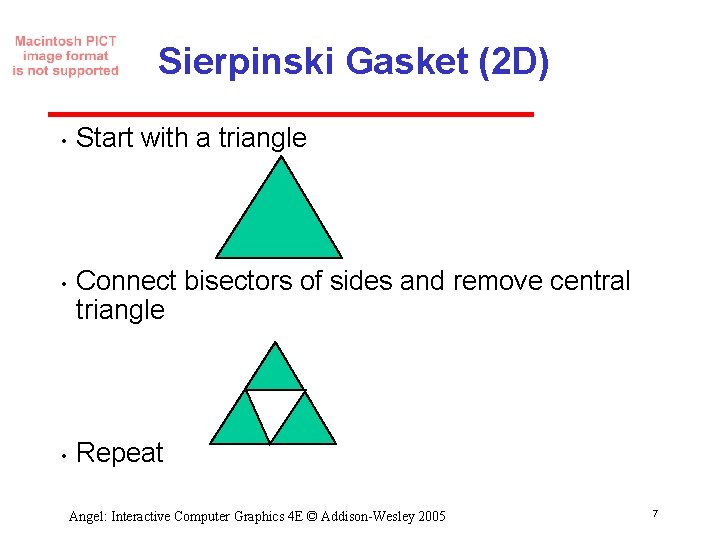
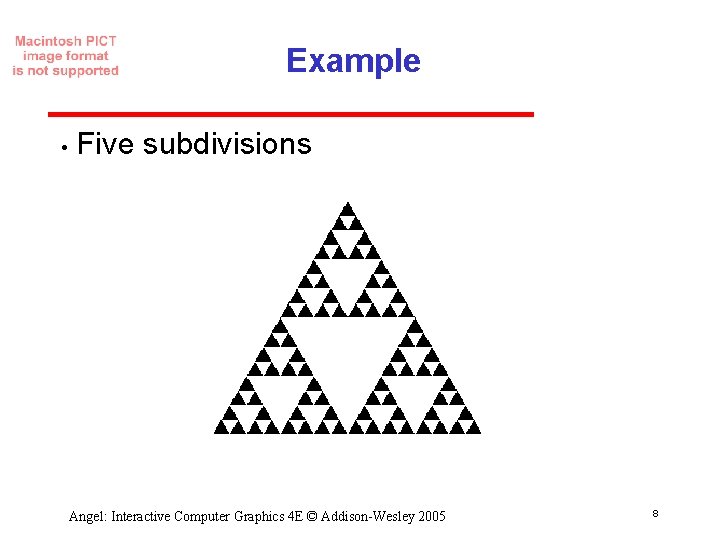
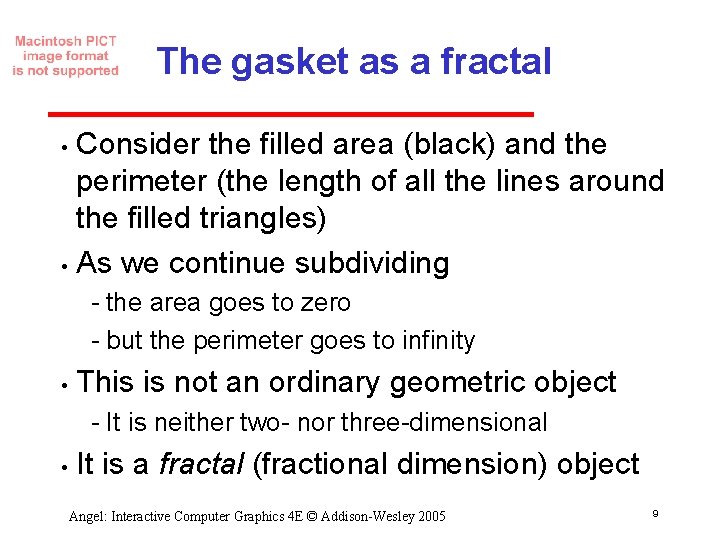
![Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58}, Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58},](https://slidetodoc.com/presentation_image/43dc5fc53e4c91a26f564ef593b7bbba/image-10.jpg)
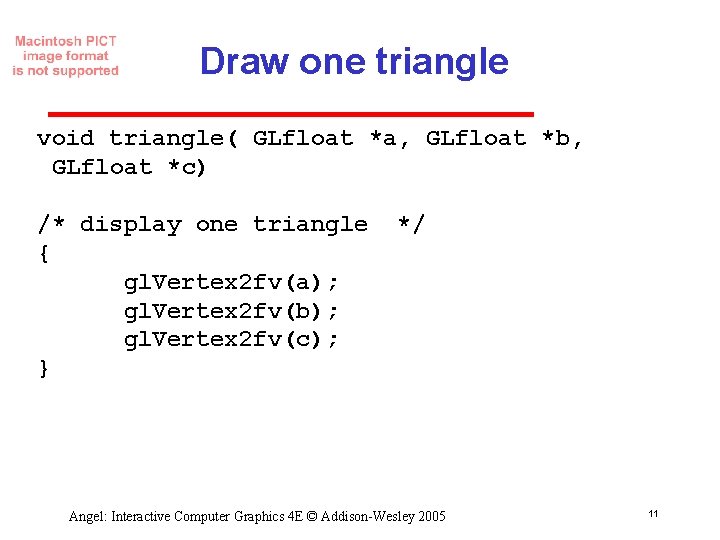
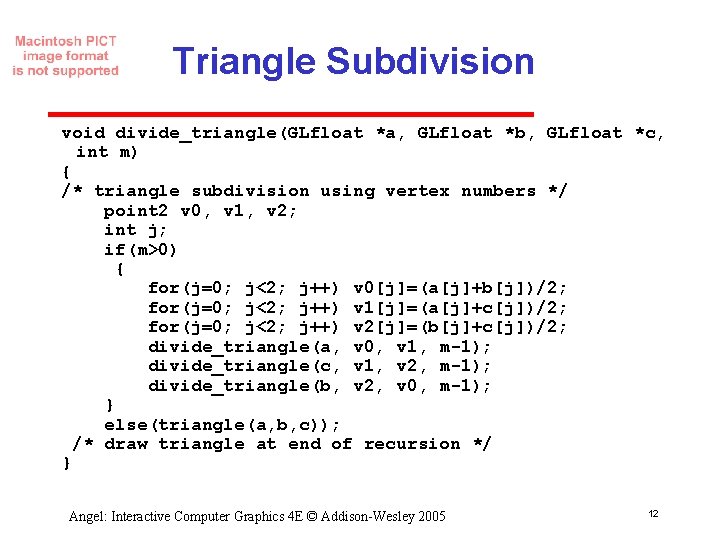
![display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2], display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2],](https://slidetodoc.com/presentation_image/43dc5fc53e4c91a26f564ef593b7bbba/image-13.jpg)
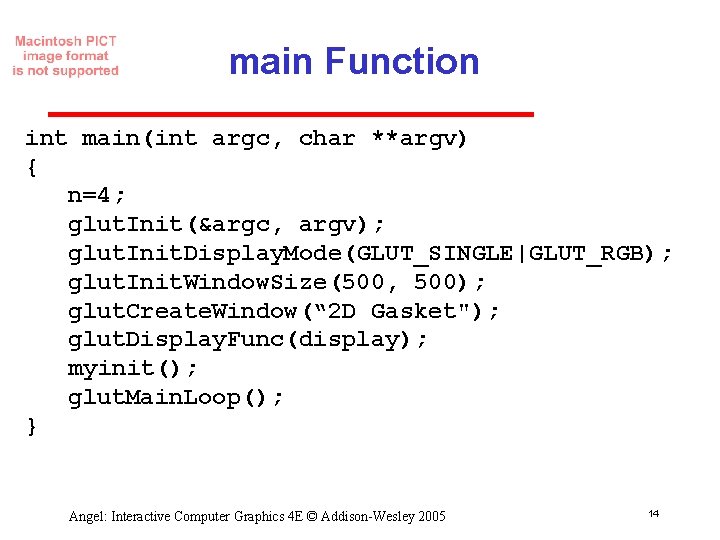
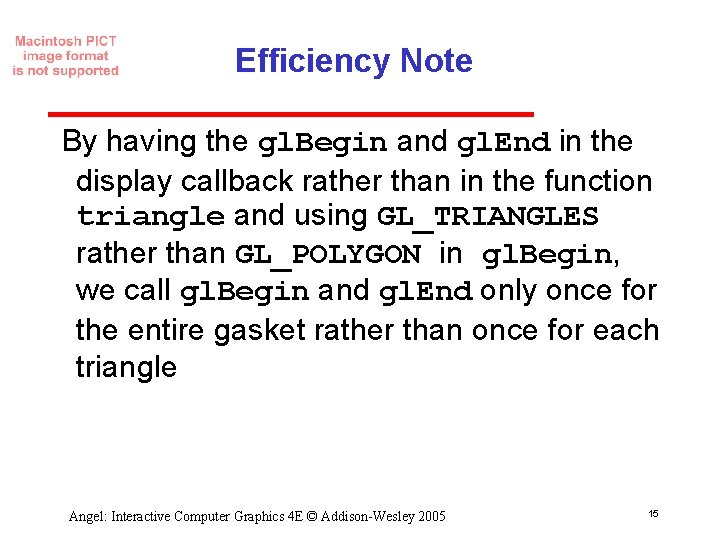
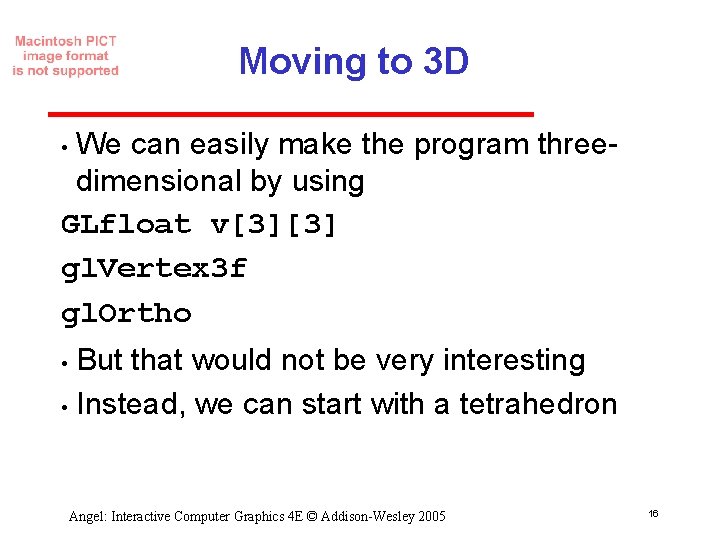
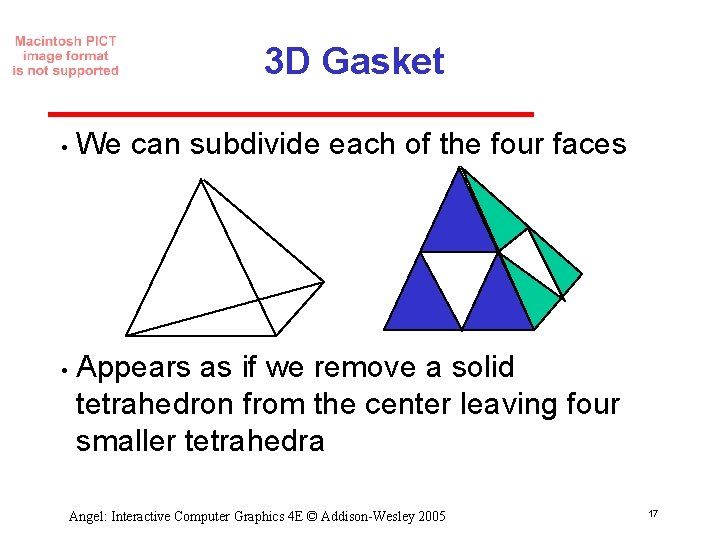
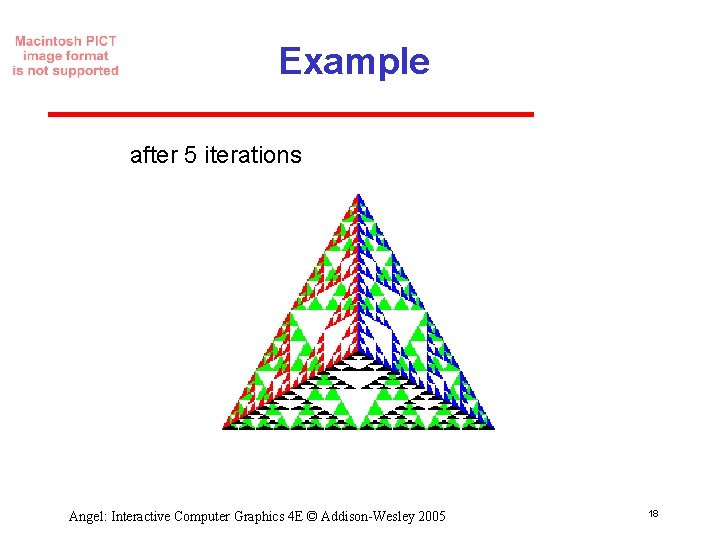
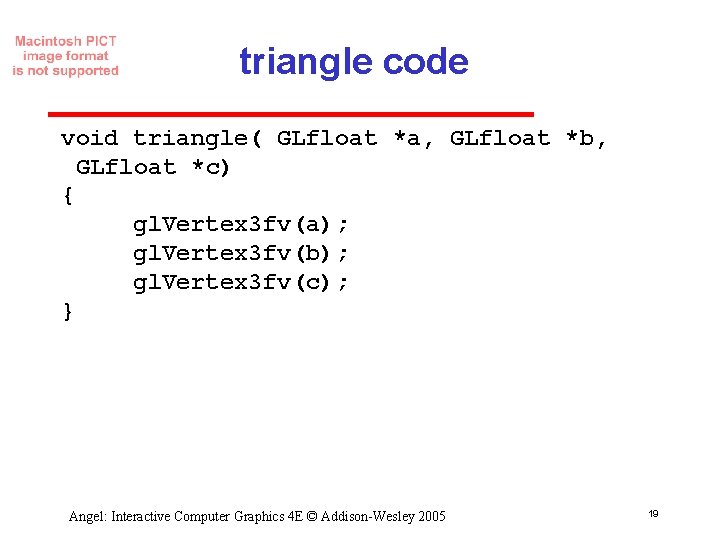
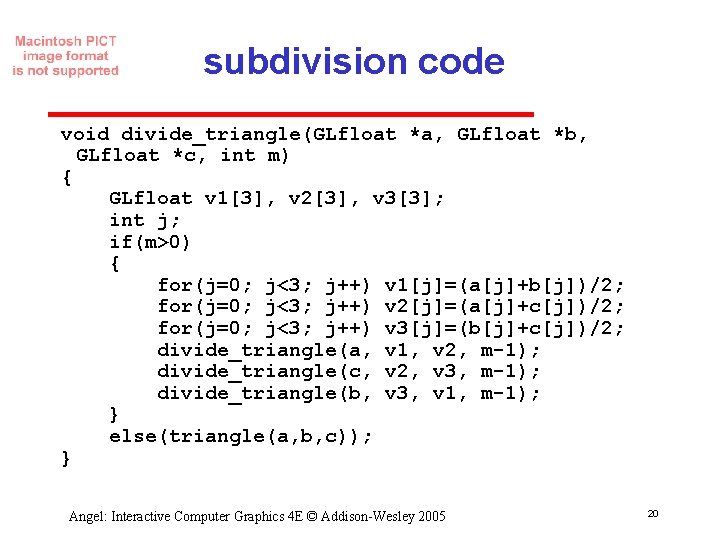
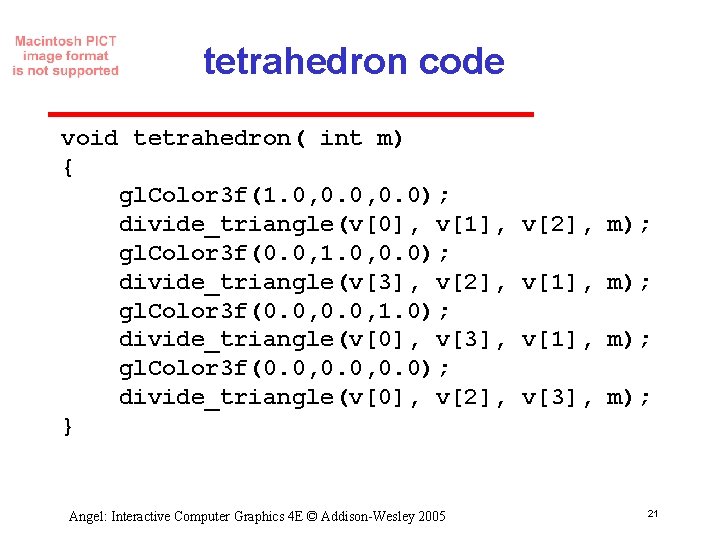
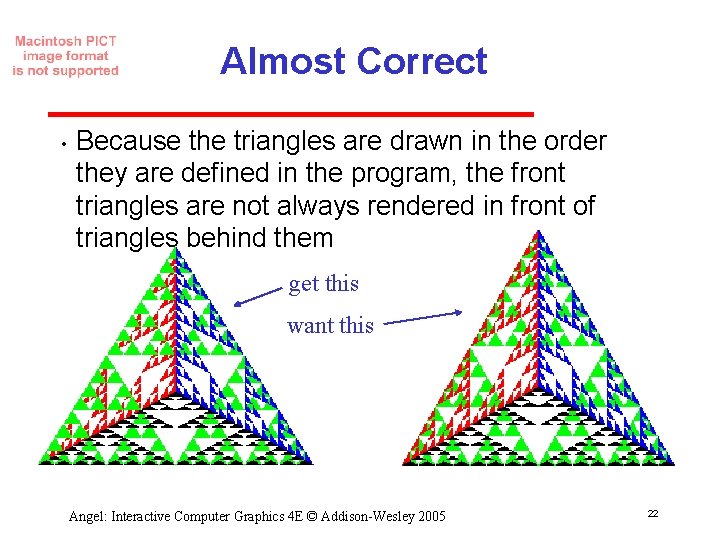
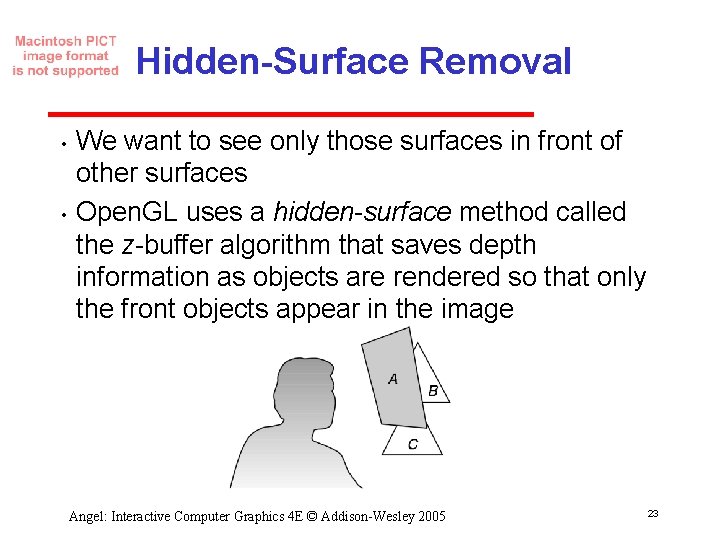
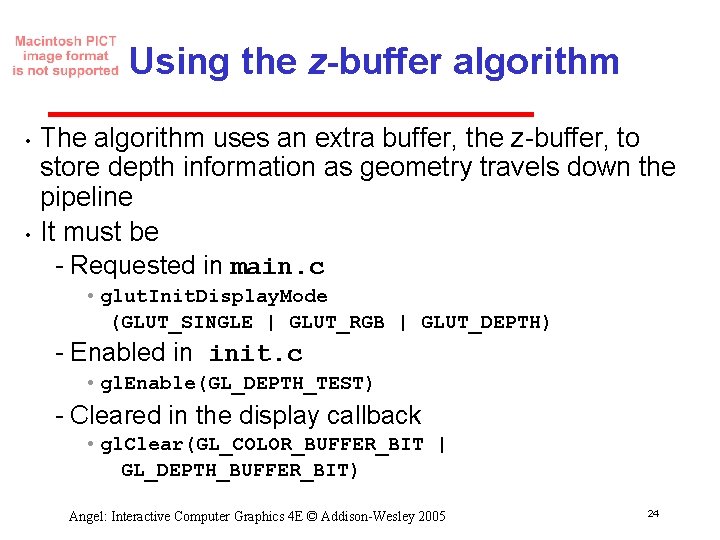
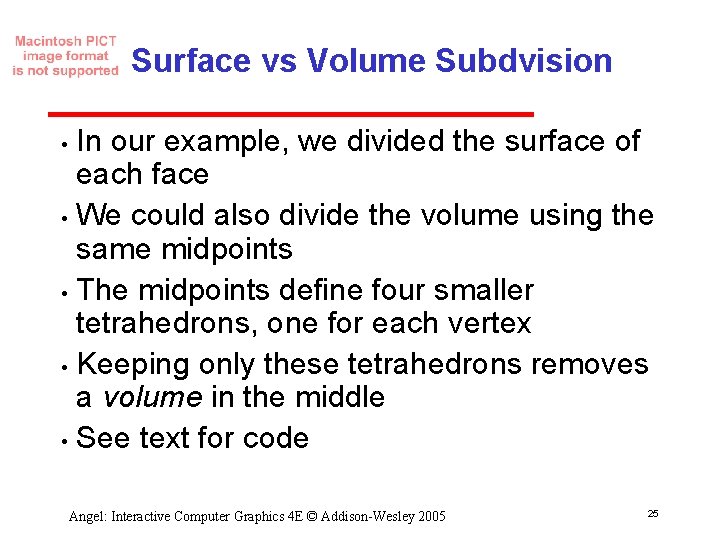
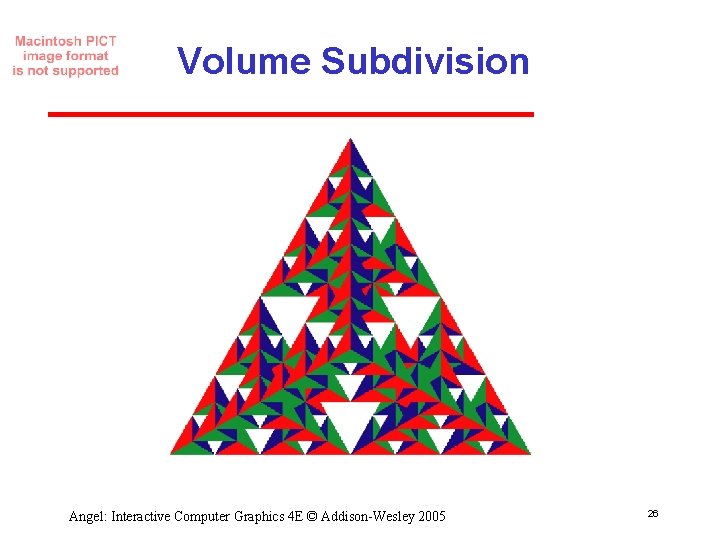
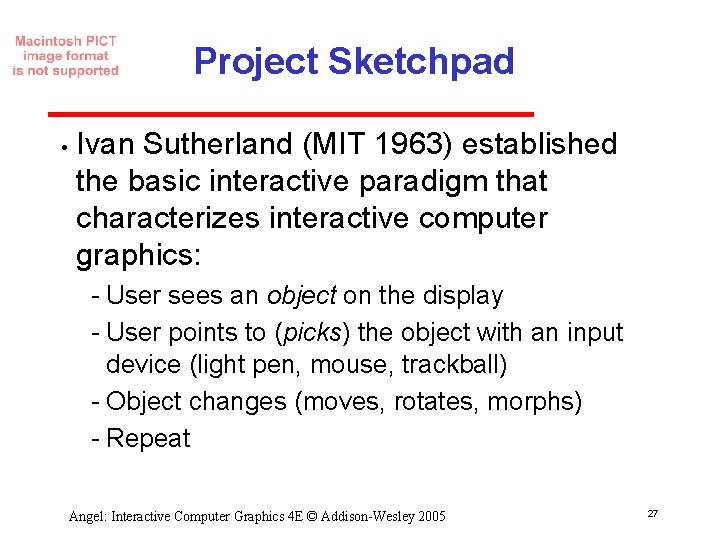
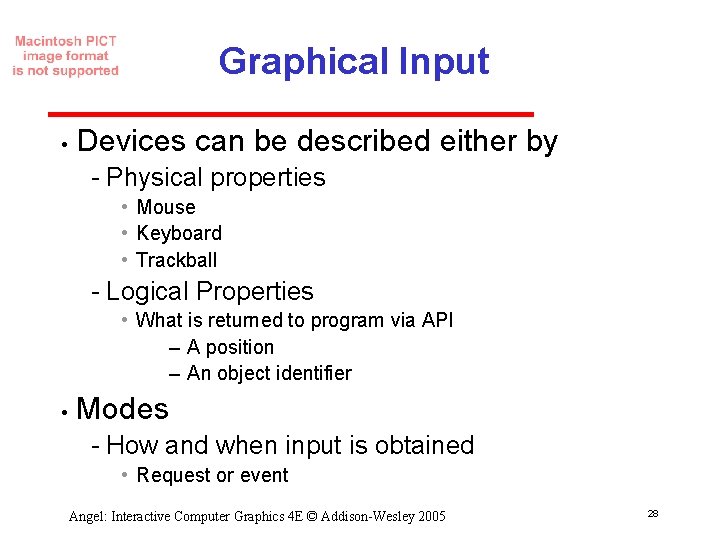
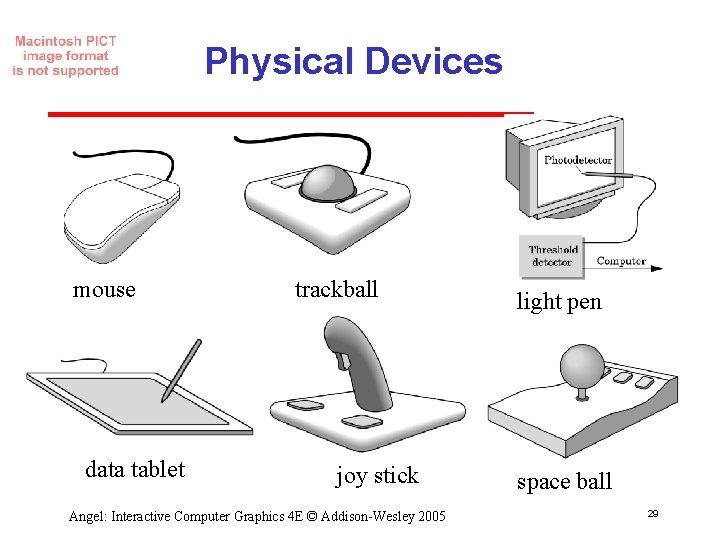
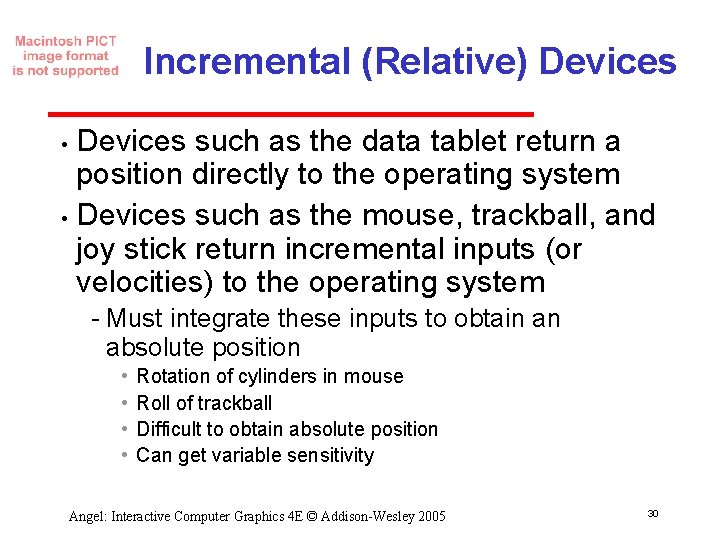
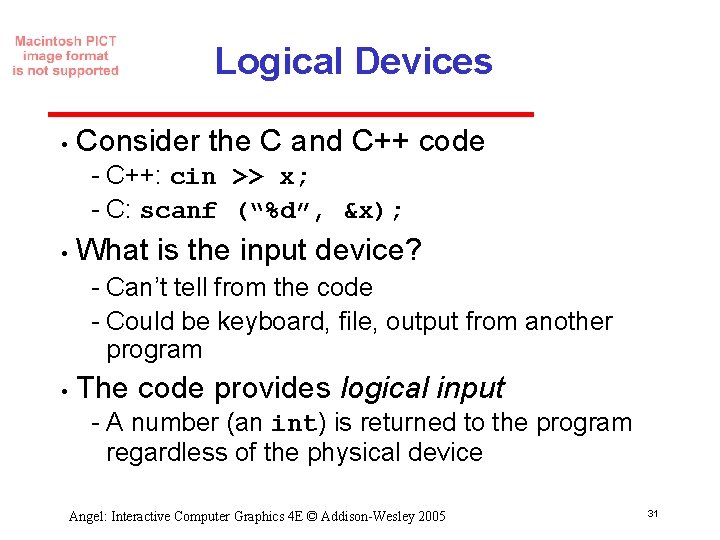
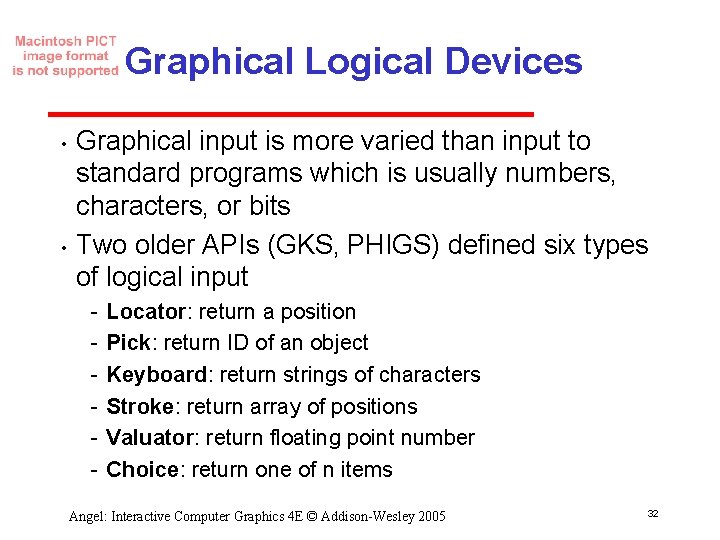
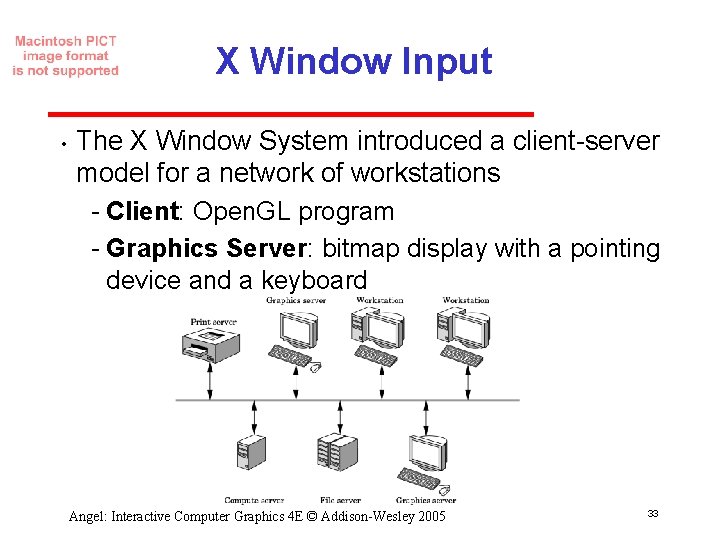
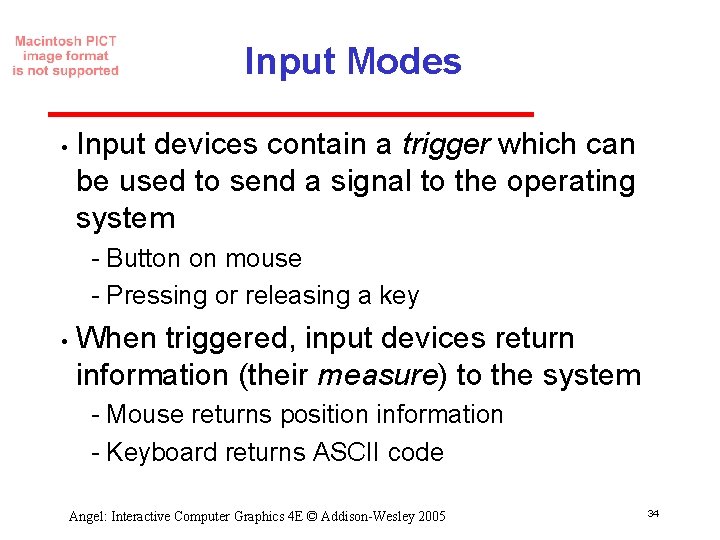
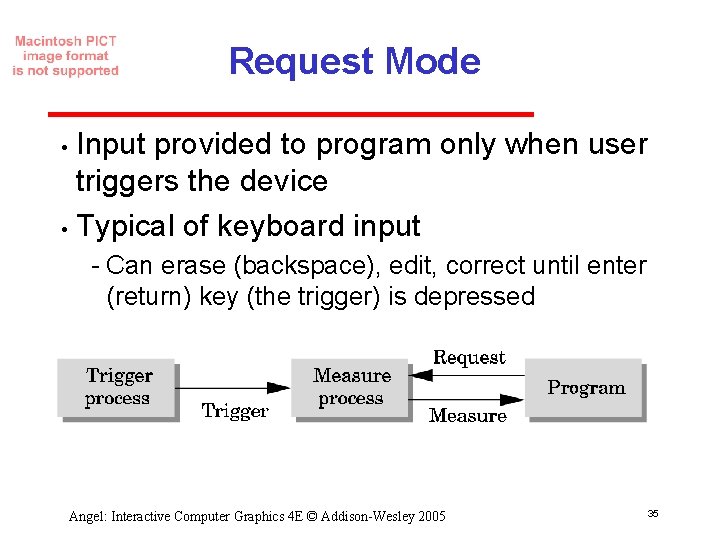
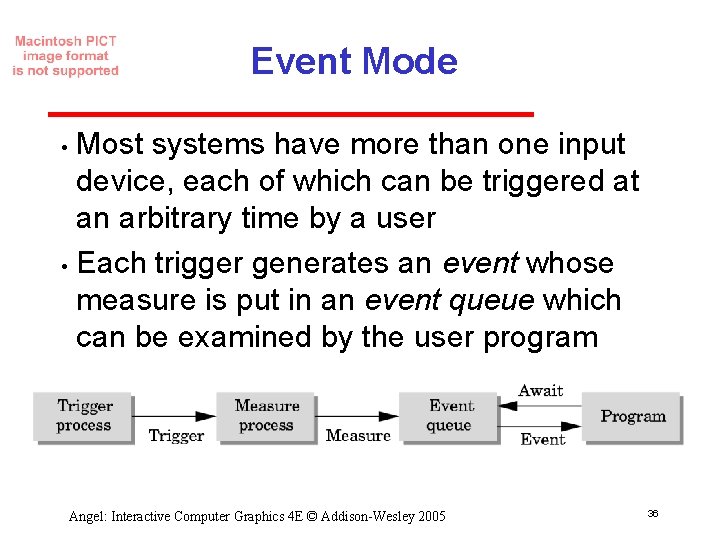
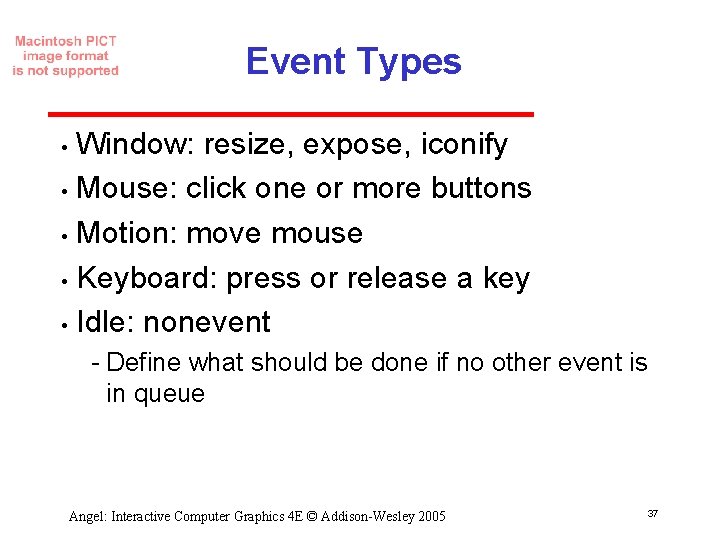
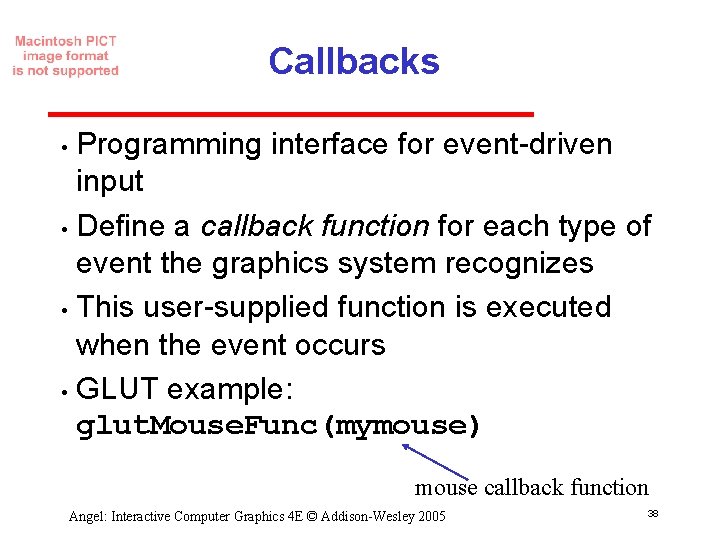
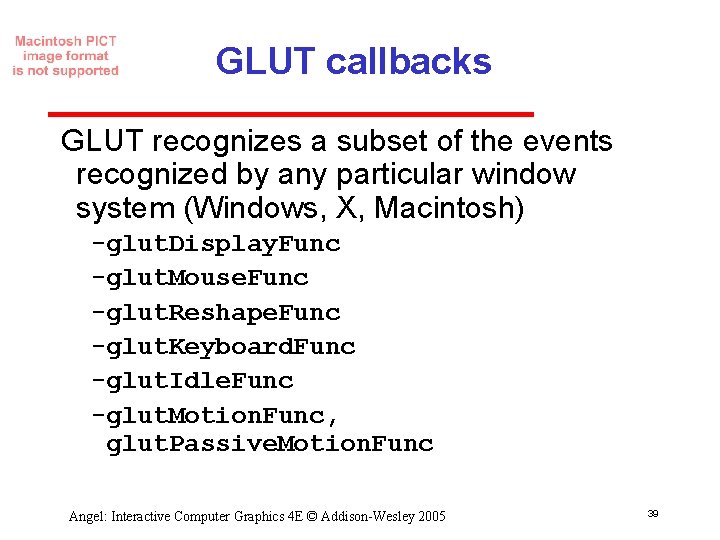
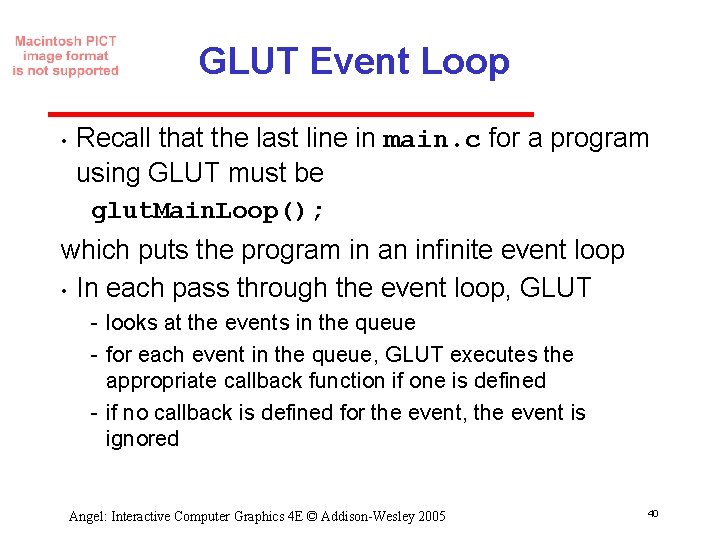
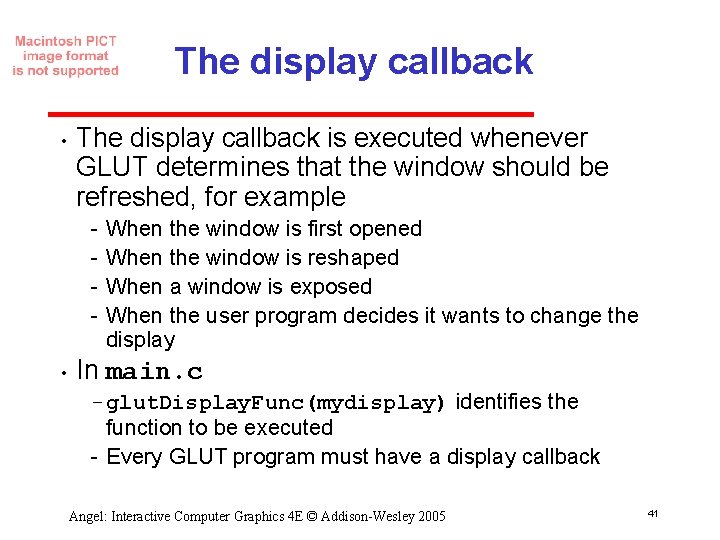
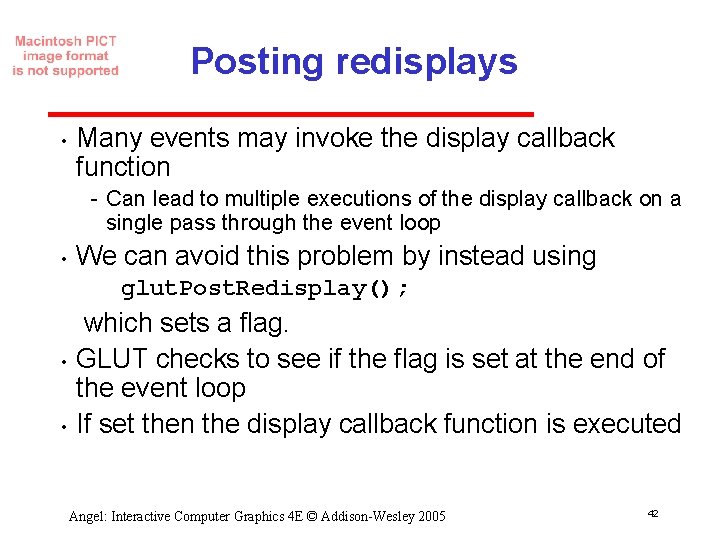
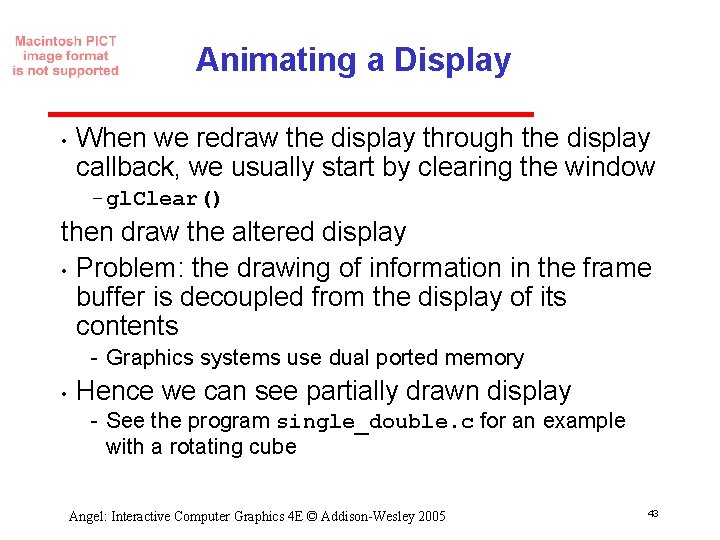
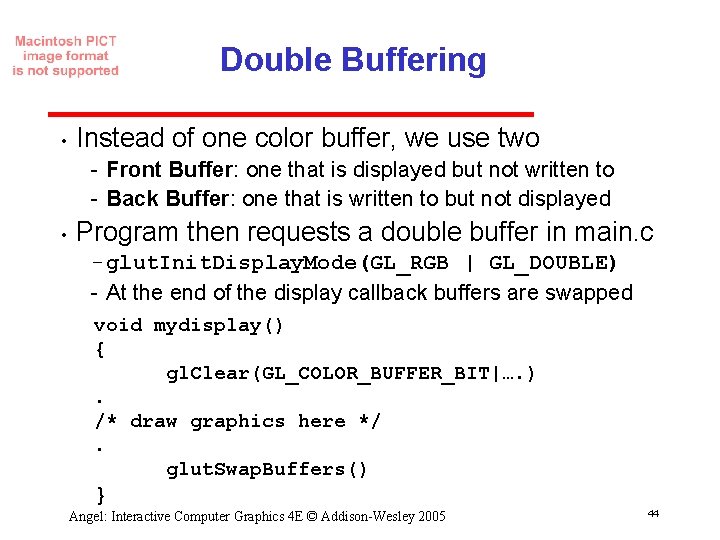
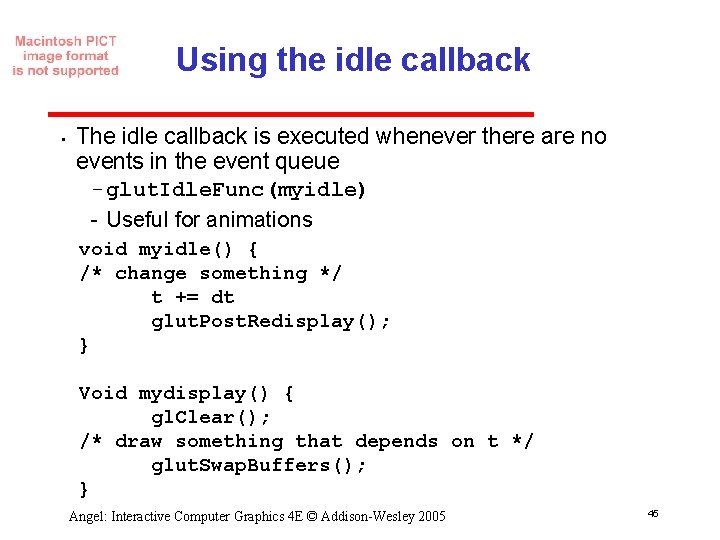
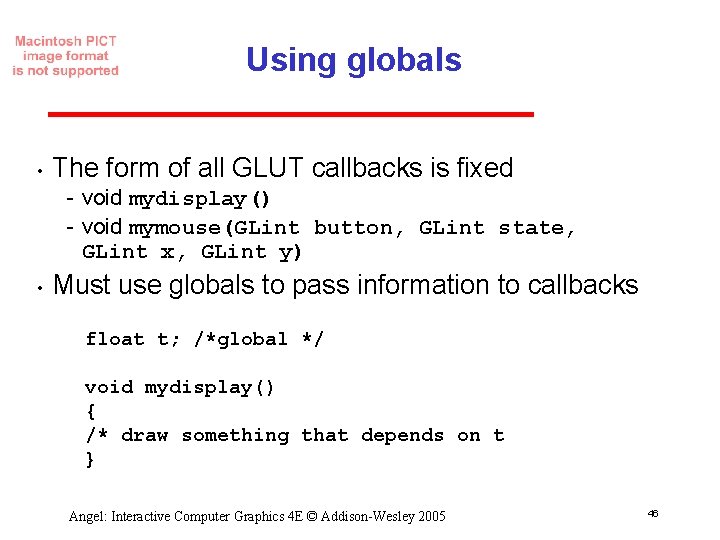
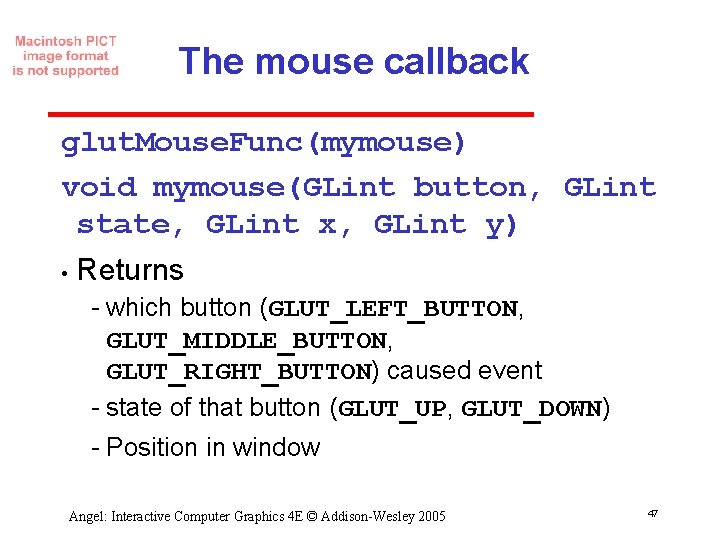
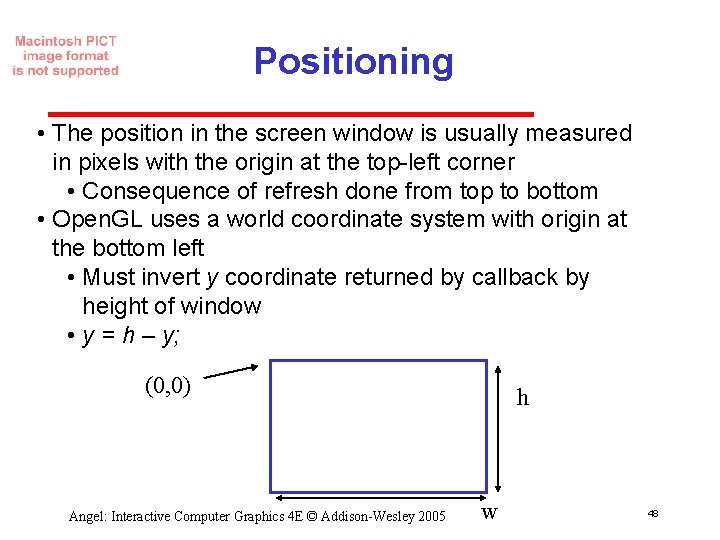
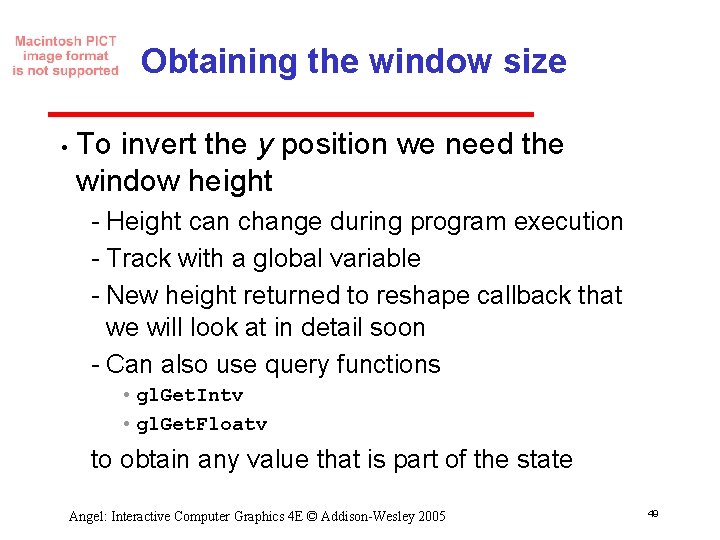
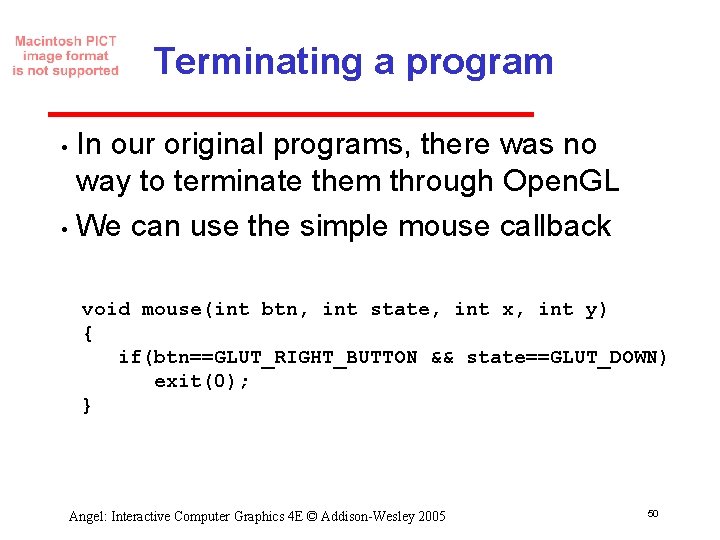
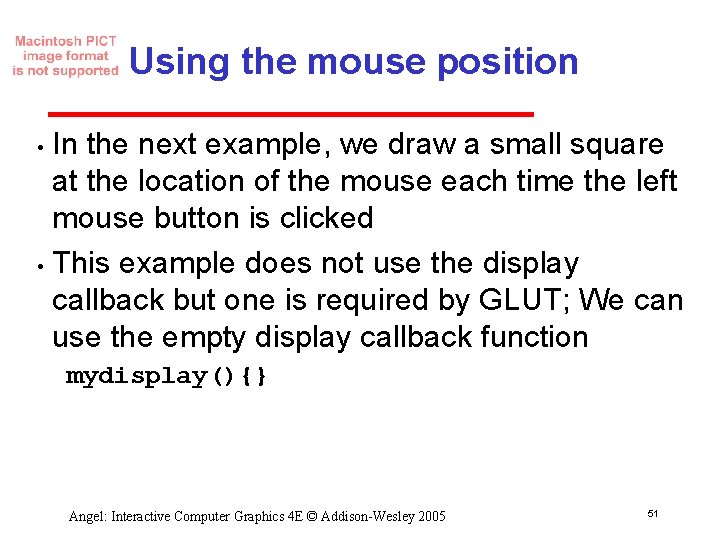
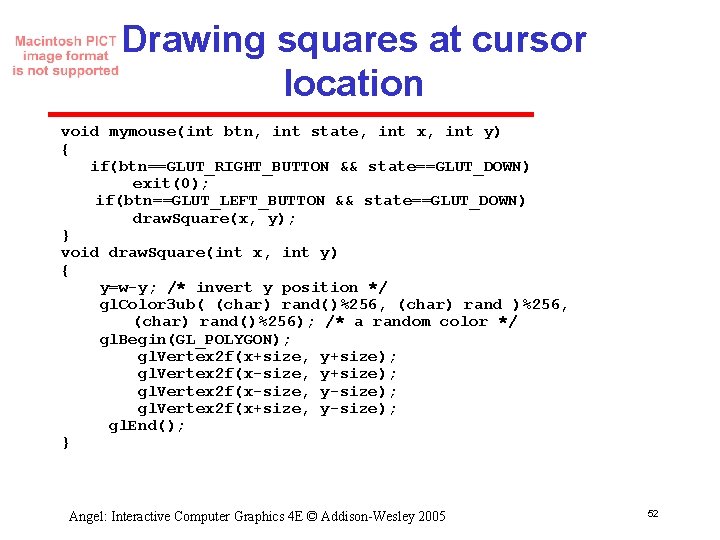
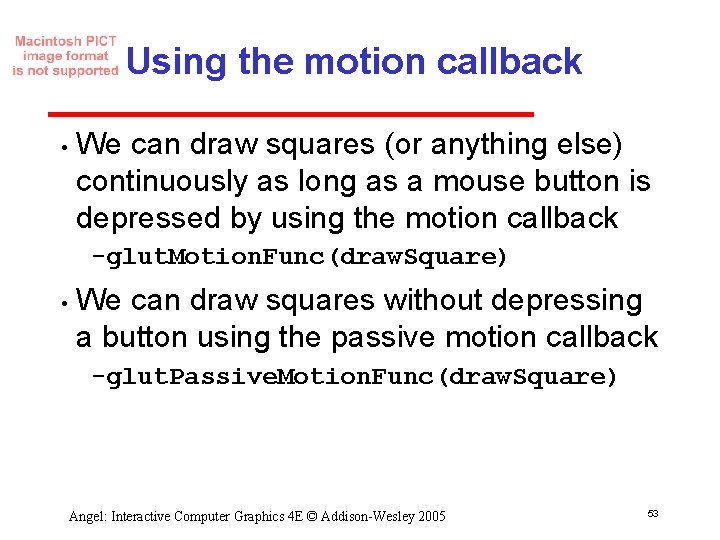
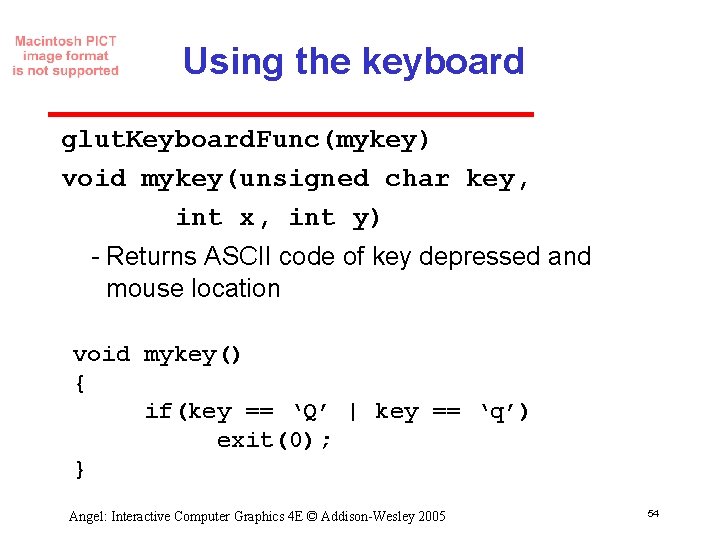
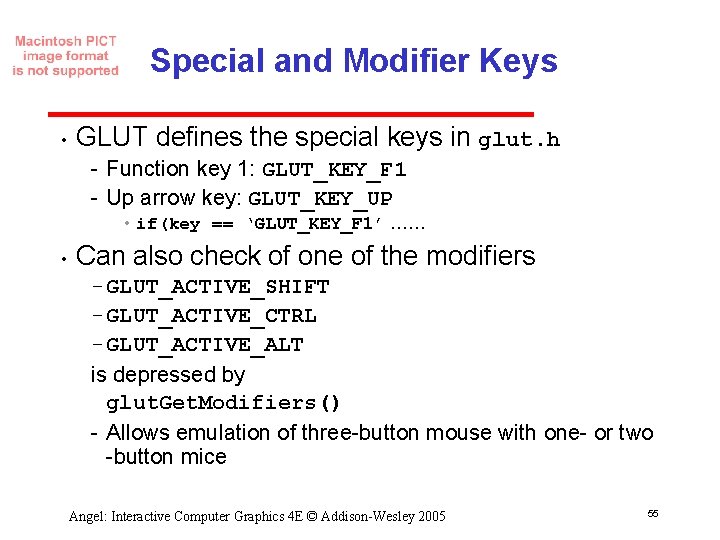
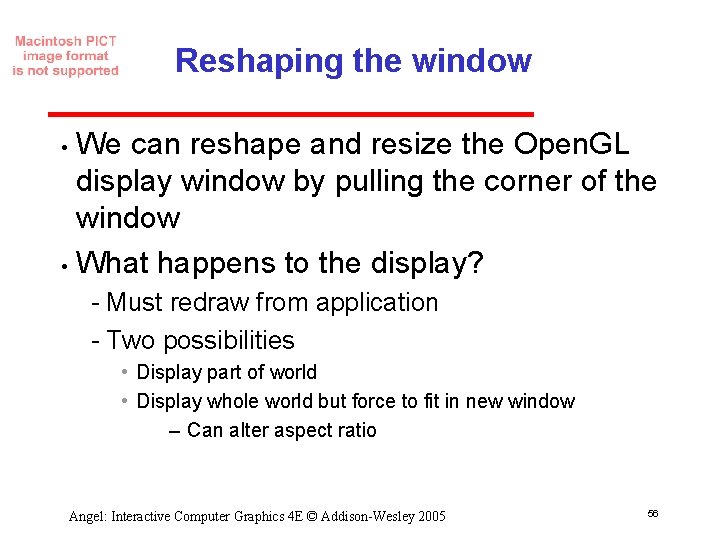
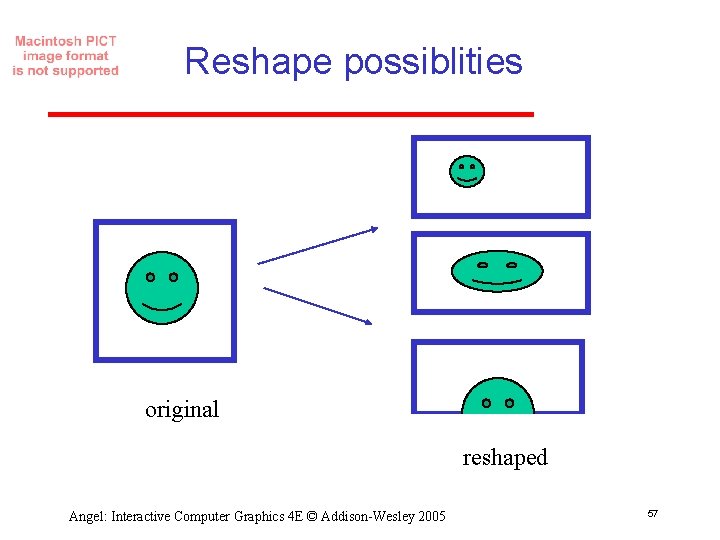
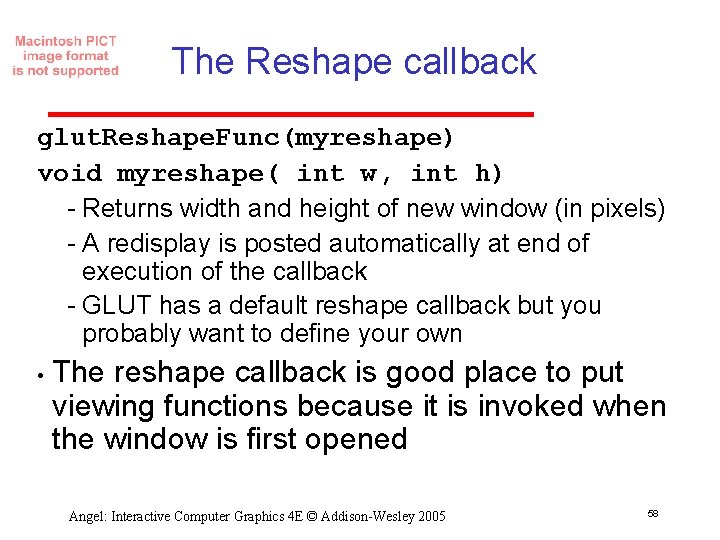
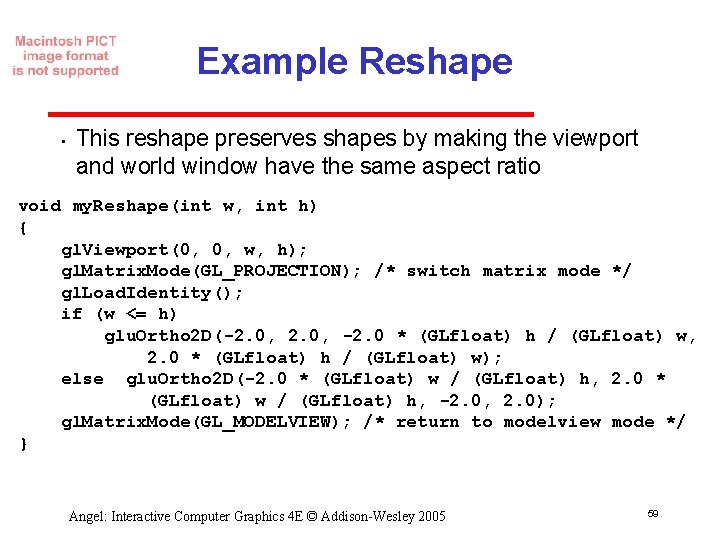
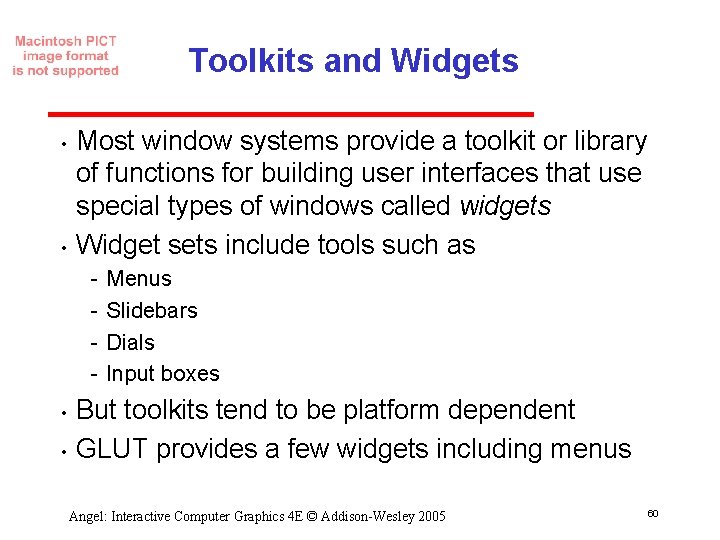
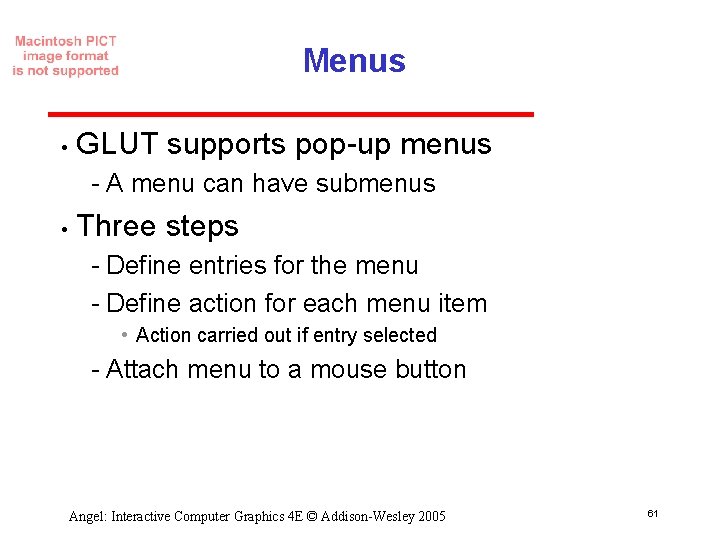
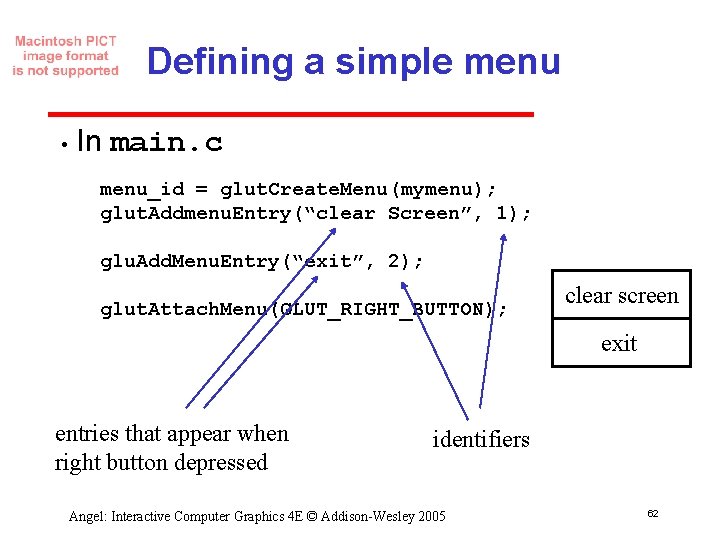
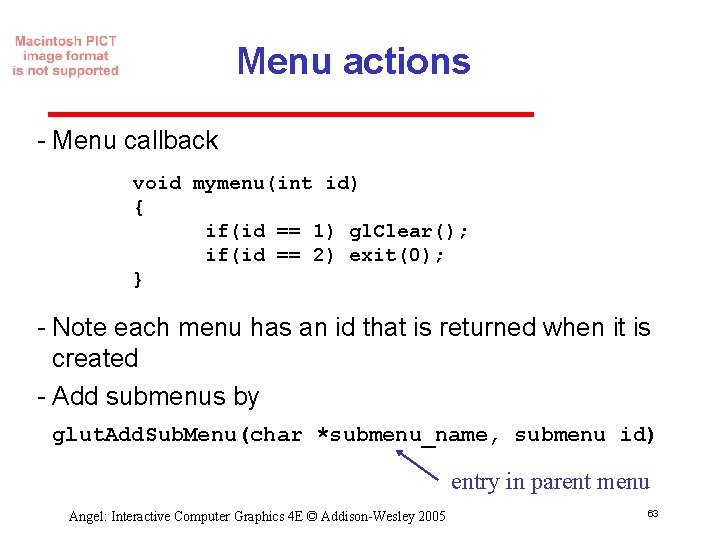
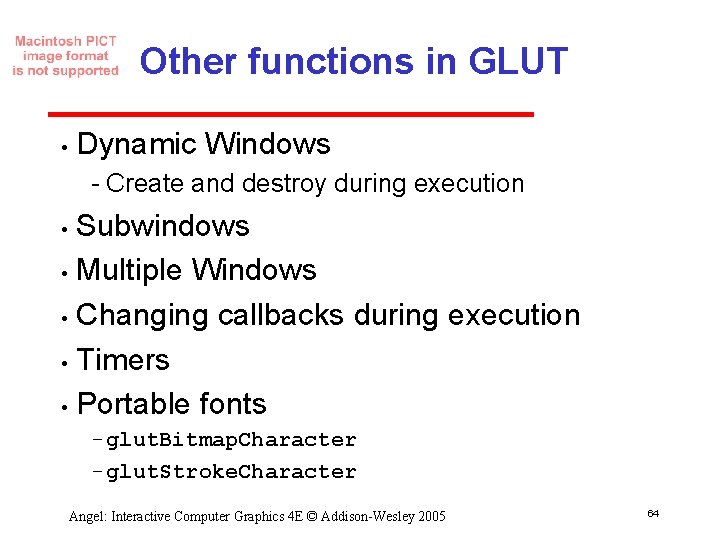
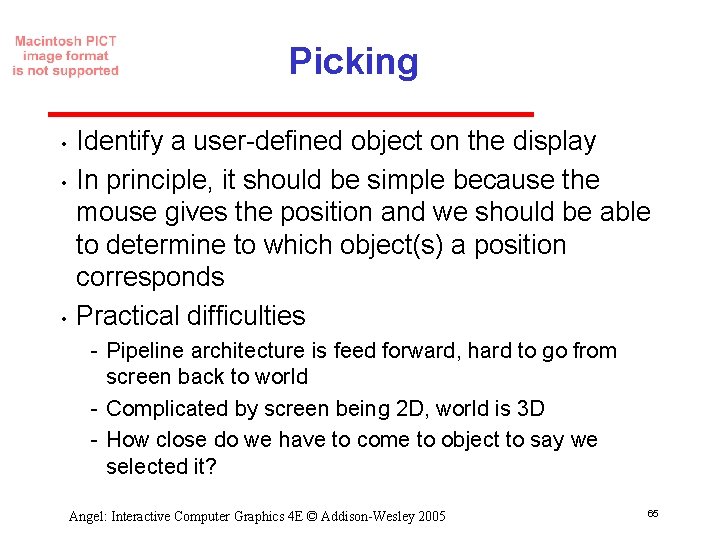
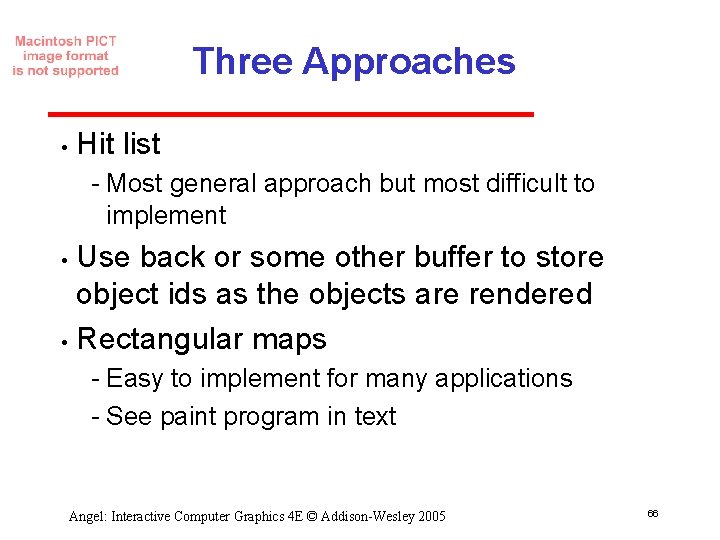
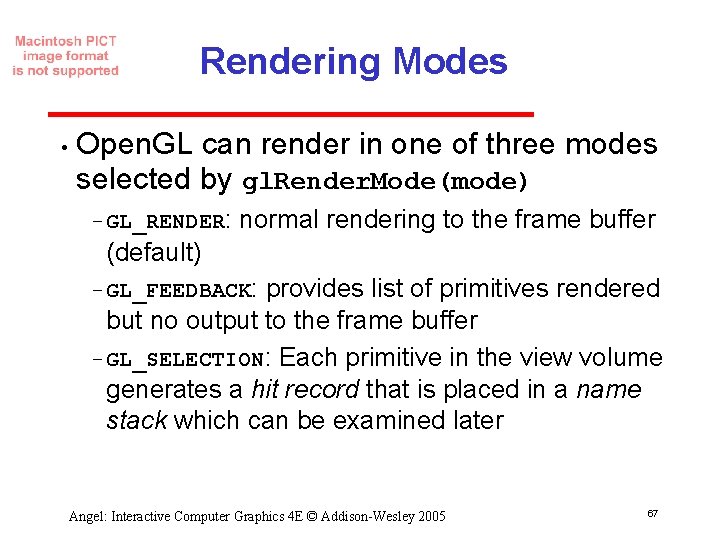
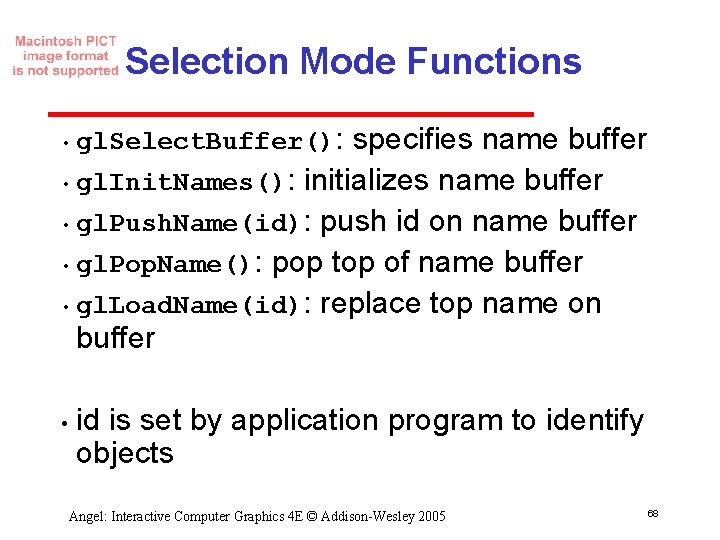
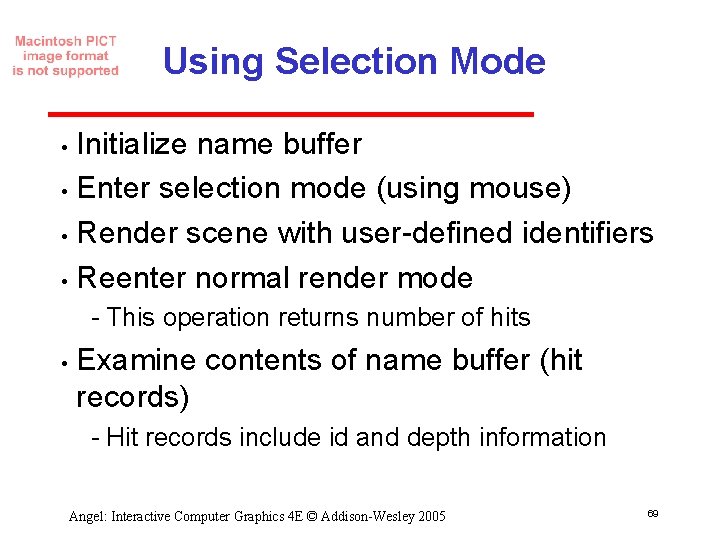
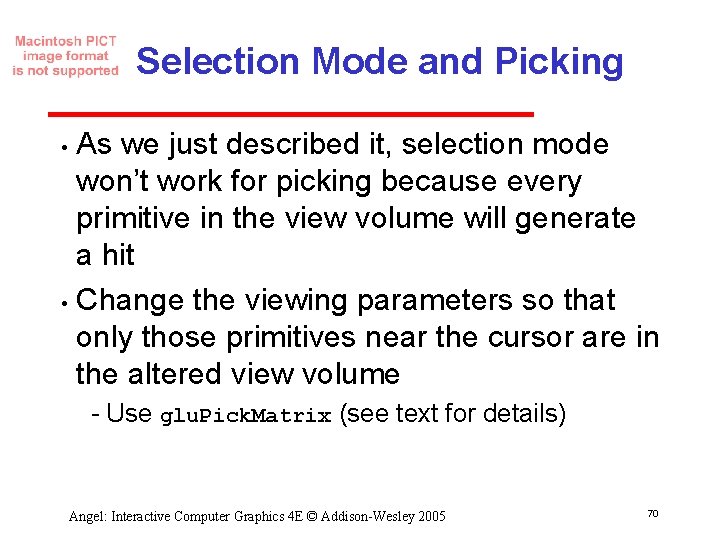
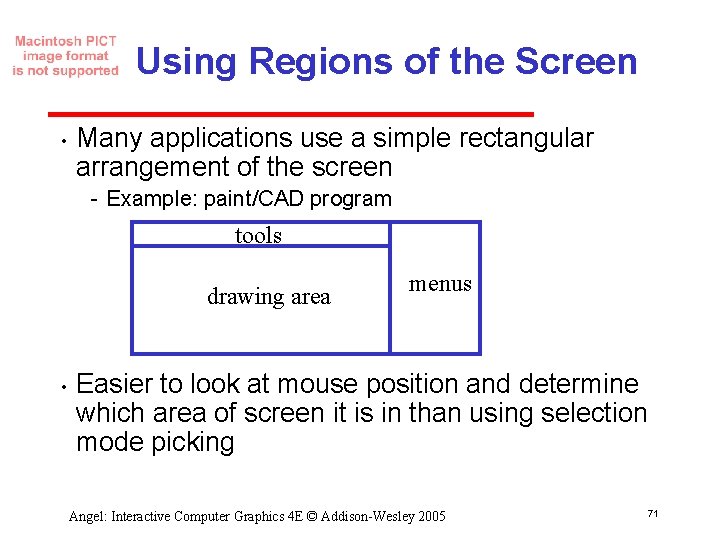
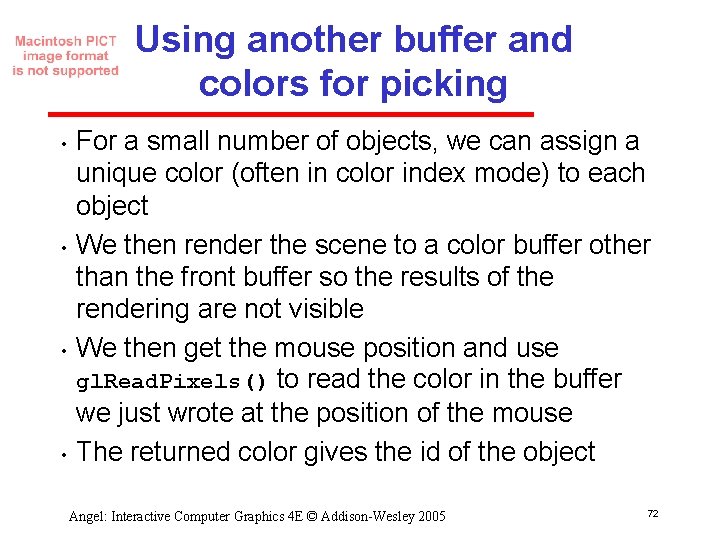
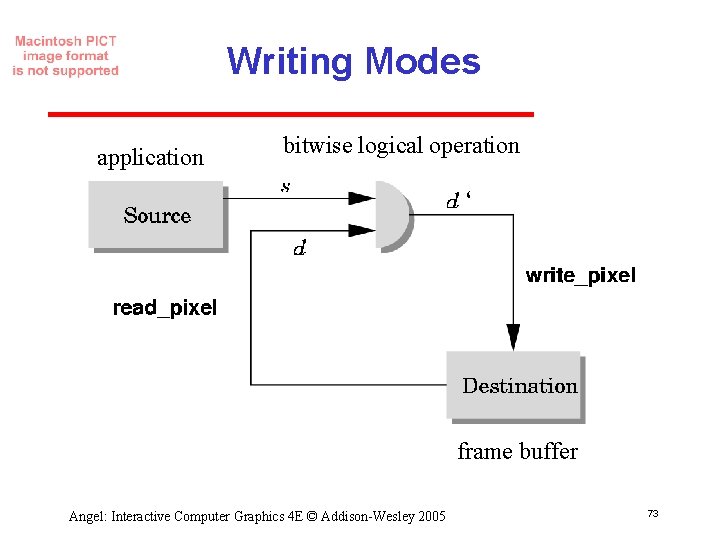
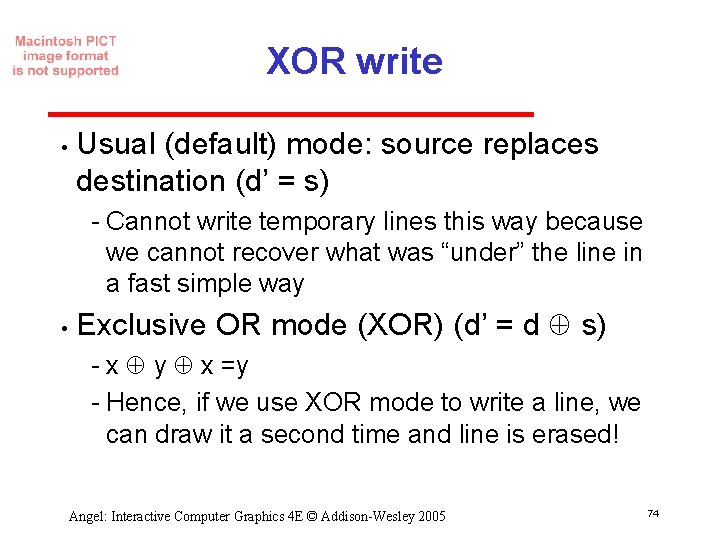
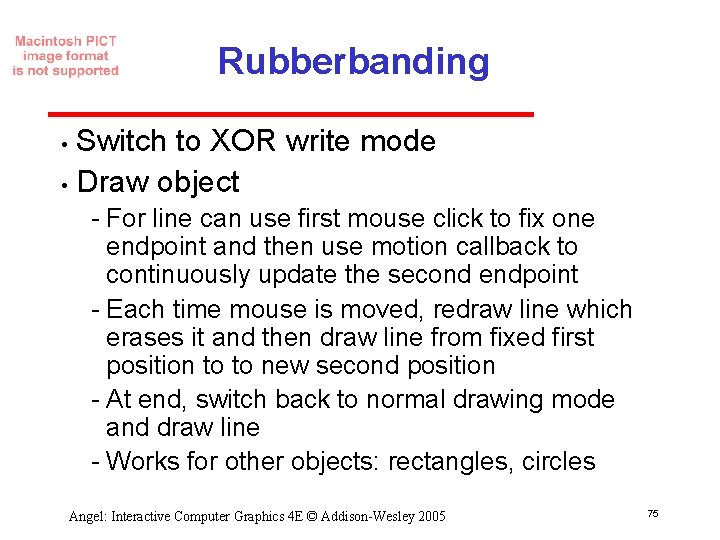
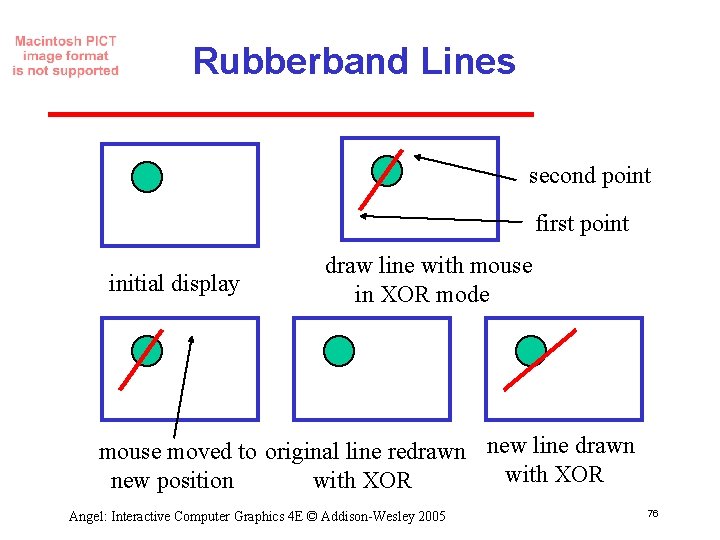
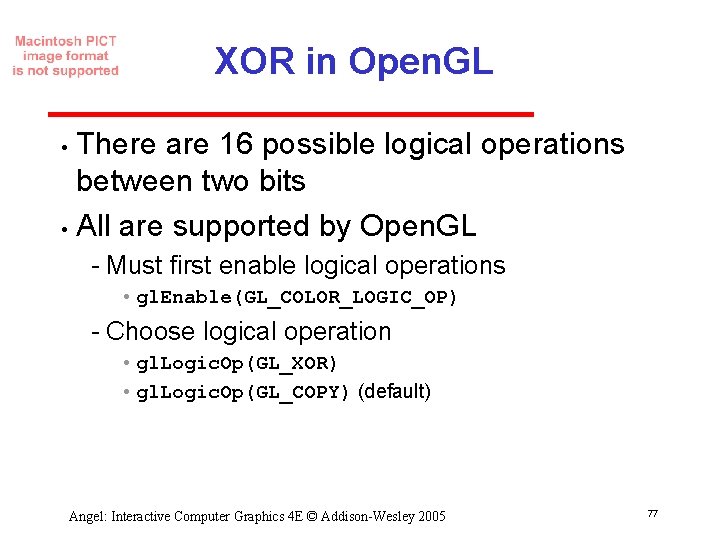
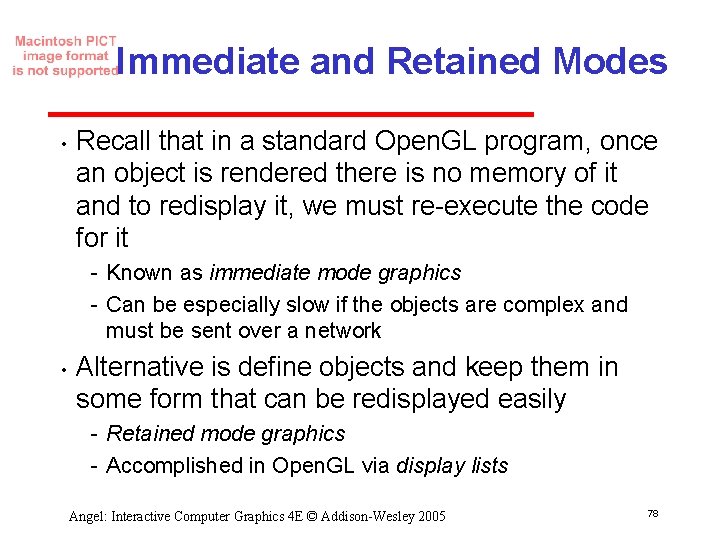
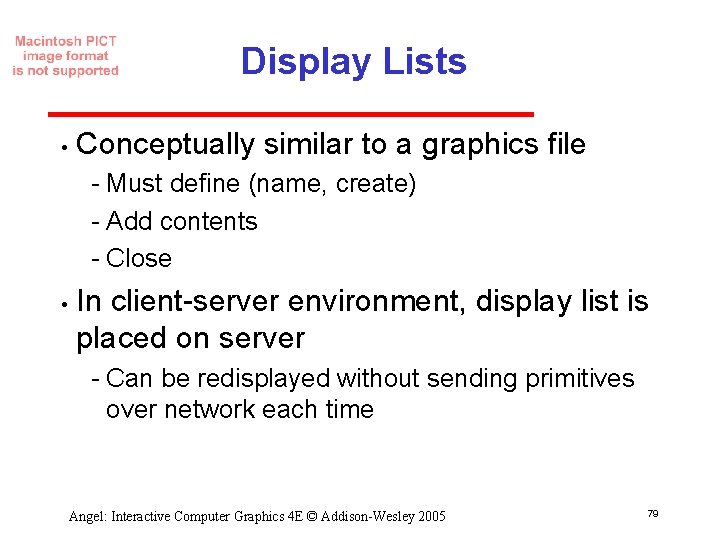
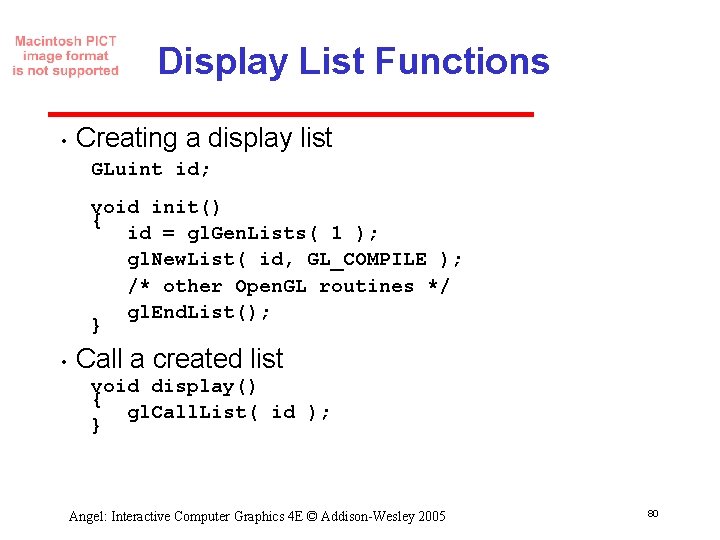
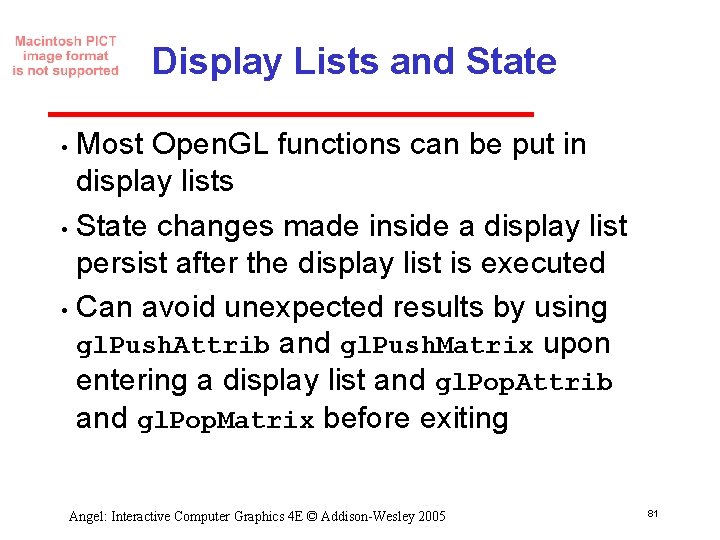
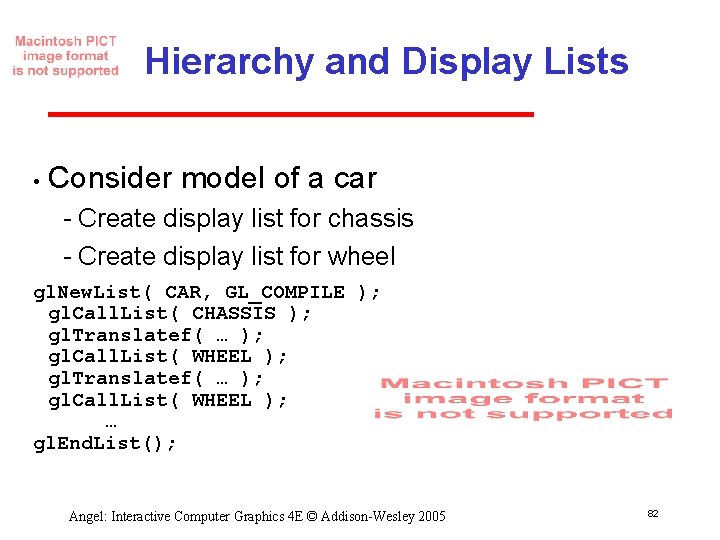
- Slides: 82
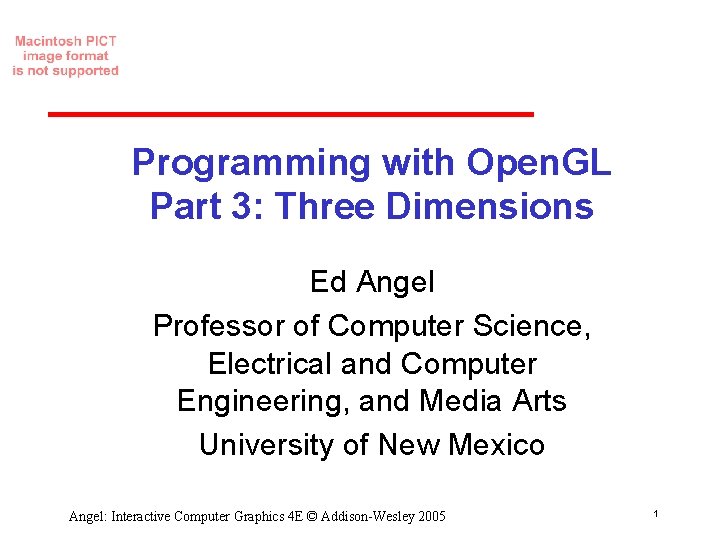
Programming with Open. GL Part 3: Three Dimensions Ed Angel Professor of Computer Science, Electrical and Computer Engineering, and Media Arts University of New Mexico Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 1
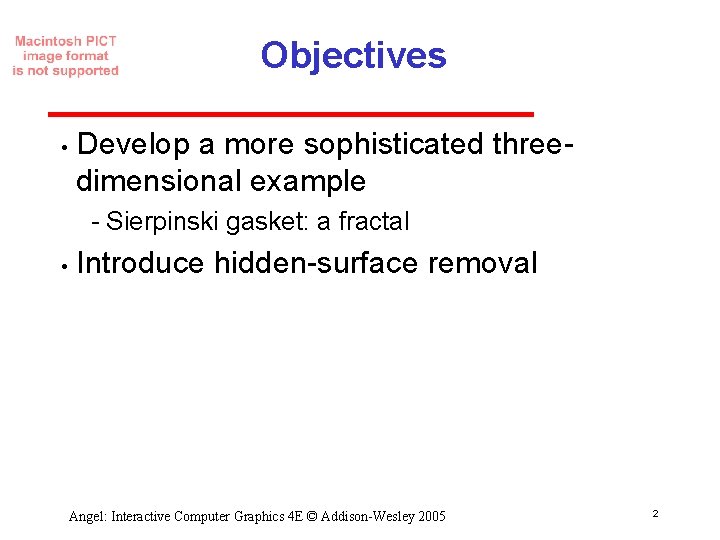
Objectives • Develop a more sophisticated three dimensional example Sierpinski gasket: a fractal • Introduce hidden surface removal Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 2
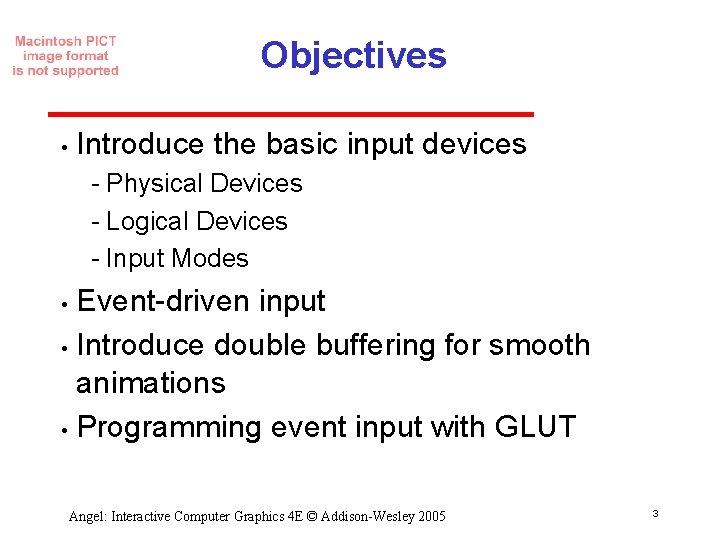
Objectives • Introduce the basic input devices Physical Devices Logical Devices Input Modes Event driven input • Introduce double buffering for smooth animations • Programming event input with GLUT • Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 3
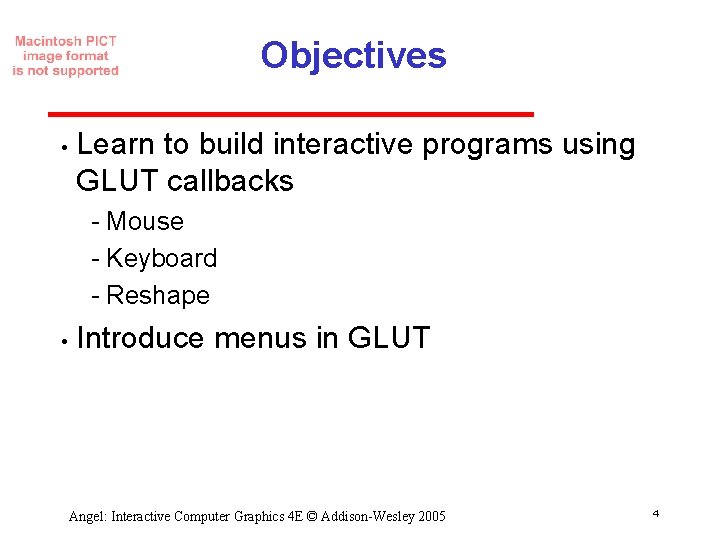
Objectives • Learn to build interactive programs using GLUT callbacks Mouse Keyboard Reshape • Introduce menus in GLUT Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 4
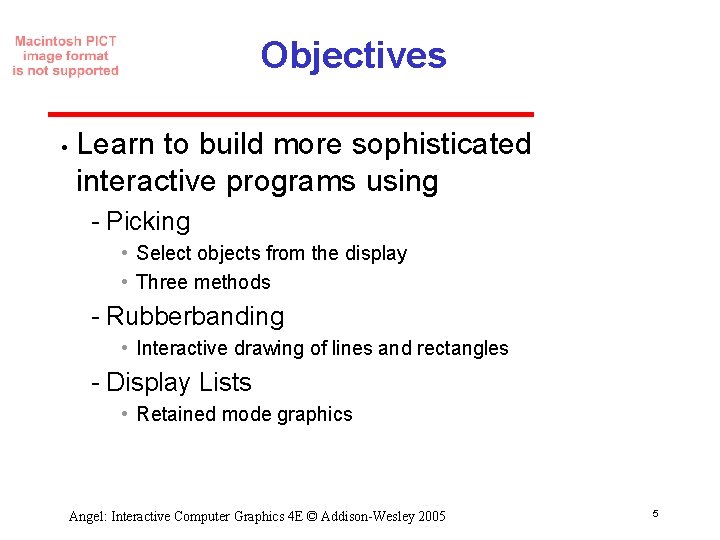
Objectives • Learn to build more sophisticated interactive programs using Picking • Select objects from the display • Three methods Rubberbanding • Interactive drawing of lines and rectangles Display Lists • Retained mode graphics Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 5
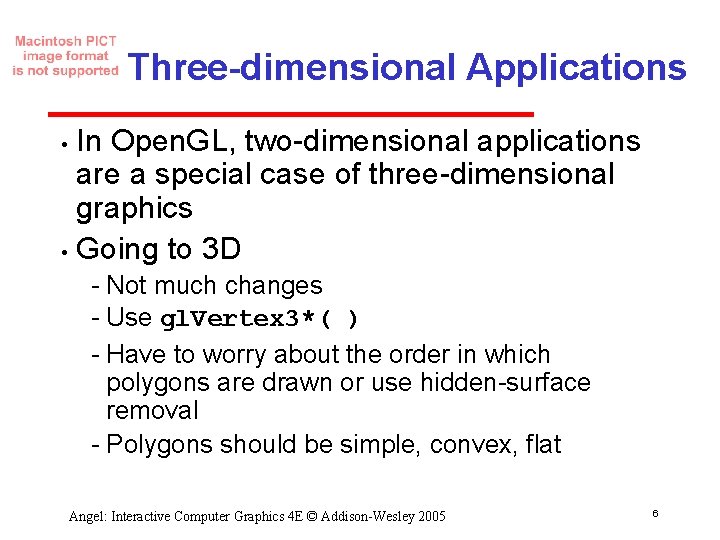
Three-dimensional Applications In Open. GL, two dimensional applications are a special case of three dimensional graphics • Going to 3 D • Not much changes Use gl. Vertex 3*( ) Have to worry about the order in which polygons are drawn or use hidden surface removal Polygons should be simple, convex, flat Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 6
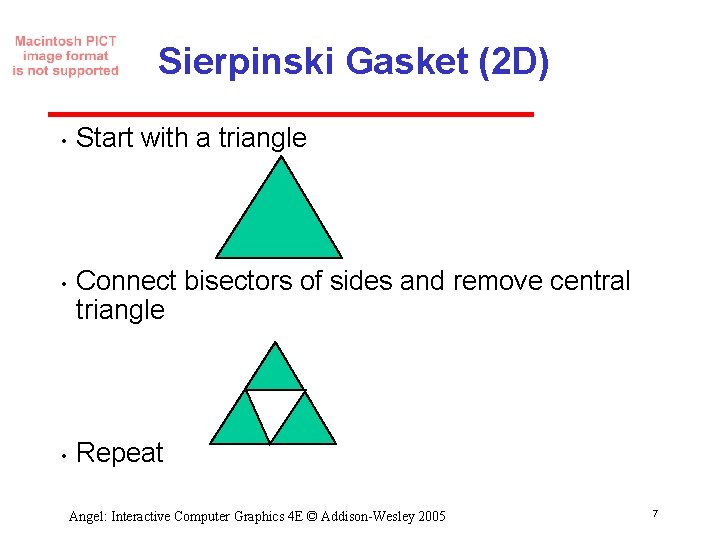
Sierpinski Gasket (2 D) • • • Start with a triangle Connect bisectors of sides and remove central triangle Repeat Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 7
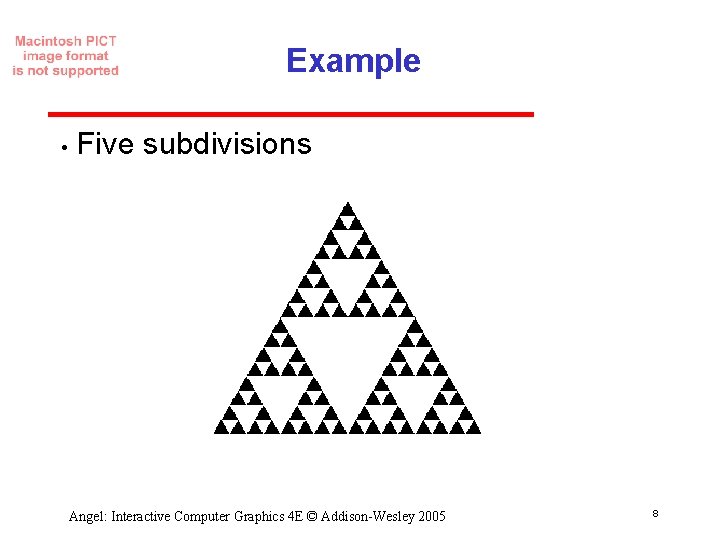
Example • Five subdivisions Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 8
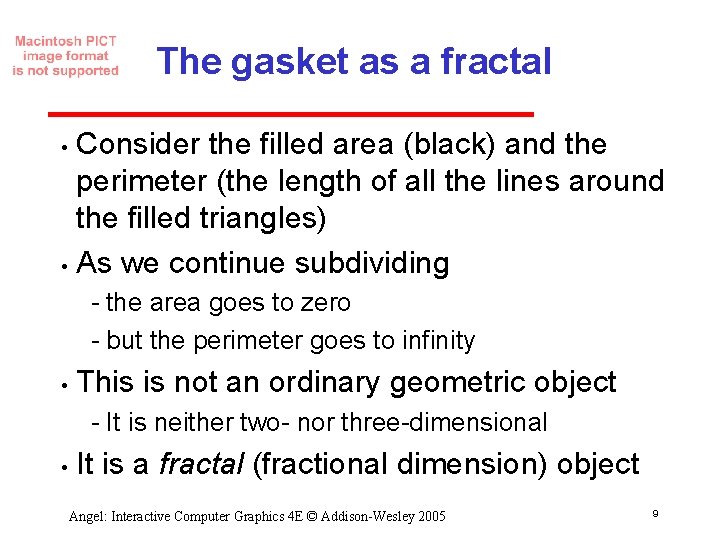
The gasket as a fractal Consider the filled area (black) and the perimeter (the length of all the lines around the filled triangles) • As we continue subdividing • the area goes to zero but the perimeter goes to infinity • This is not an ordinary geometric object It is neither two nor three dimensional • It is a fractal (fractional dimension) object Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 9
![Gasket Program include GLglut h initial triangle GLfloat v321 0 0 58 Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58},](https://slidetodoc.com/presentation_image/43dc5fc53e4c91a26f564ef593b7bbba/image-10.jpg)
Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58}, {0. 0, 1. 15}}; int n; /* number of recursive steps */ Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 10
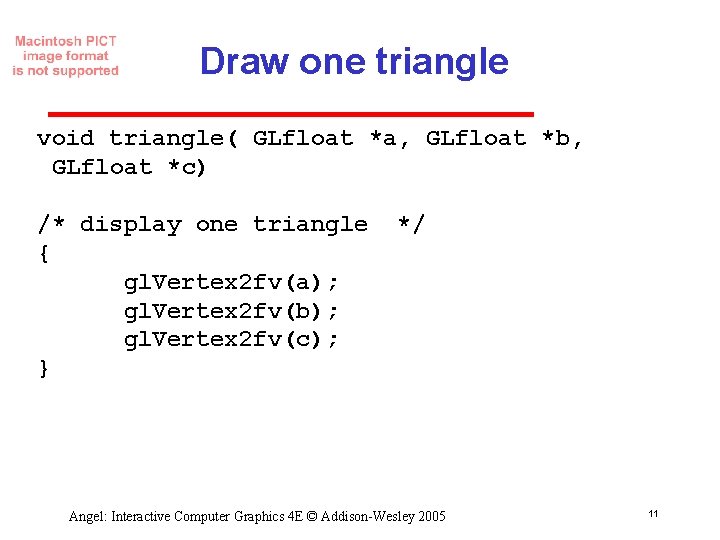
Draw one triangle void triangle( GLfloat *a, GLfloat *b, GLfloat *c) /* display one triangle { gl. Vertex 2 fv(a); gl. Vertex 2 fv(b); gl. Vertex 2 fv(c); } */ Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 11
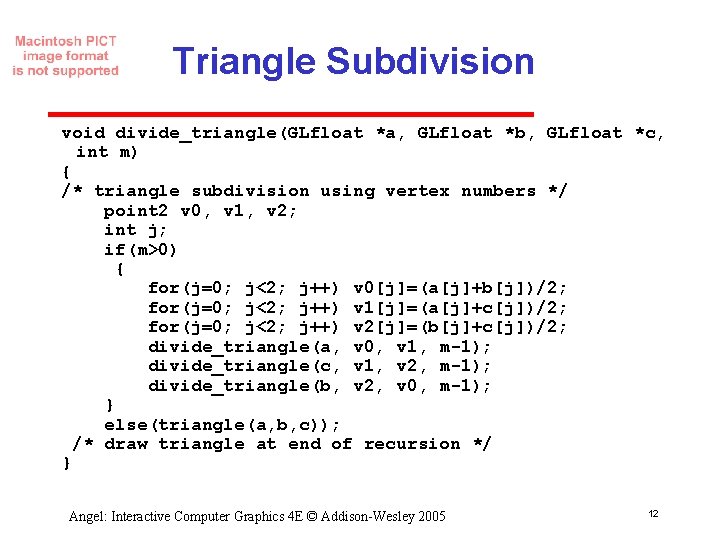
Triangle Subdivision void divide_triangle(GLfloat *a, GLfloat *b, GLfloat *c, int m) { /* triangle subdivision using vertex numbers */ point 2 v 0, v 1, v 2; int j; if(m>0) { for(j=0; j<2; j++) v 0[j]=(a[j]+b[j])/2; for(j=0; j<2; j++) v 1[j]=(a[j]+c[j])/2; for(j=0; j<2; j++) v 2[j]=(b[j]+c[j])/2; divide_triangle(a, v 0, v 1, m-1); divide_triangle(c, v 1, v 2, m-1); divide_triangle(b, v 2, v 0, m-1); } else(triangle(a, b, c)); /* draw triangle at end of recursion */ } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 12
![display and init Functions void display gl ClearGLCOLORBUFFERBIT gl BeginGLTRIANGLES dividetrianglev0 v1 v2 display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2],](https://slidetodoc.com/presentation_image/43dc5fc53e4c91a26f564ef593b7bbba/image-13.jpg)
display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2], n); gl. End(); gl. Flush(); } void myinit() { gl. Matrix. Mode(GL_PROJECTION); gl. Load. Identity(); glu. Ortho 2 D(-2. 0, 2. 0); gl. Matrix. Mode(GL_MODELVIEW); gl. Clear. Color (1. 0, 1. 0) gl. Color 3 f(0. 0, 0. 0); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 13
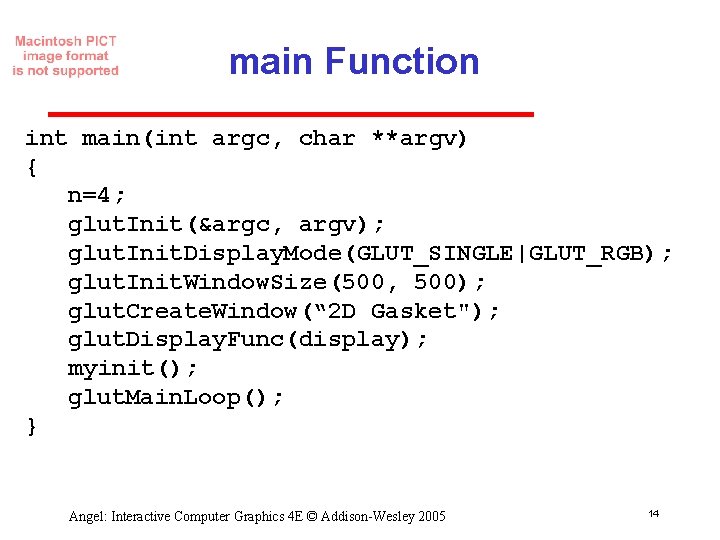
main Function int main(int argc, char **argv) { n=4; glut. Init(&argc, argv); glut. Init. Display. Mode(GLUT_SINGLE|GLUT_RGB); glut. Init. Window. Size(500, 500); glut. Create. Window(“ 2 D Gasket"); glut. Display. Func(display); myinit(); glut. Main. Loop(); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 14
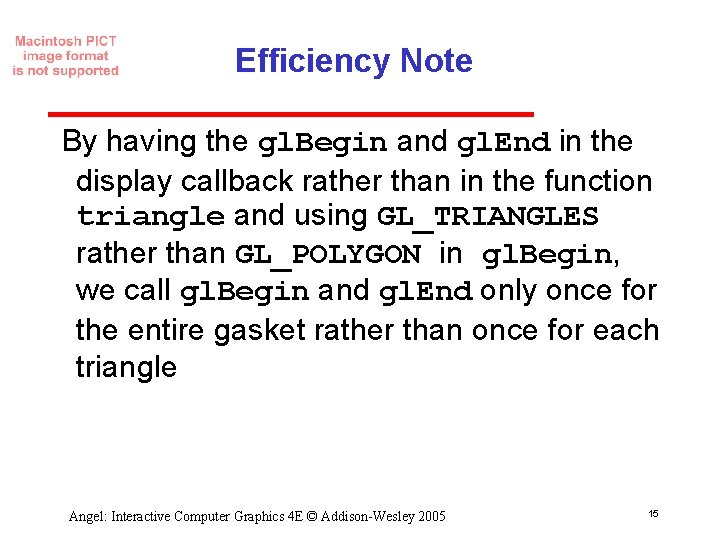
Efficiency Note By having the gl. Begin and gl. End in the display callback rather than in the function triangle and using GL_TRIANGLES rather than GL_POLYGON in gl. Begin, we call gl. Begin and gl. End only once for the entire gasket rather than once for each triangle Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 15
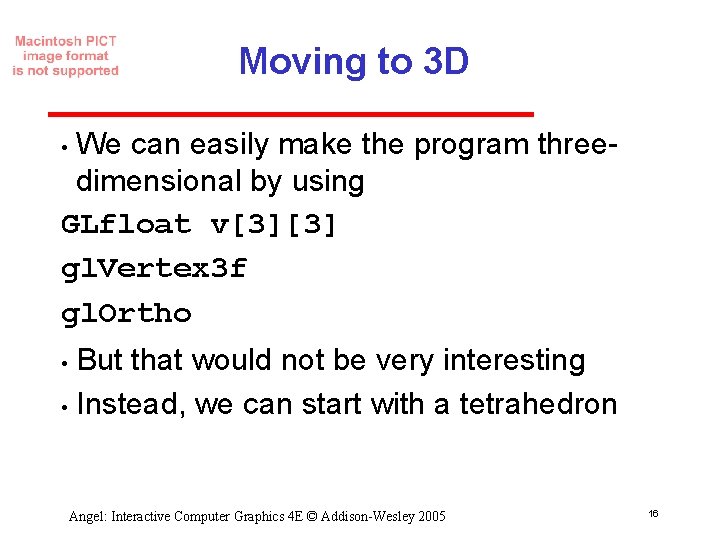
Moving to 3 D We can easily make the program three dimensional by using GLfloat v[3][3] gl. Vertex 3 f gl. Ortho • But that would not be very interesting • Instead, we can start with a tetrahedron • Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 16
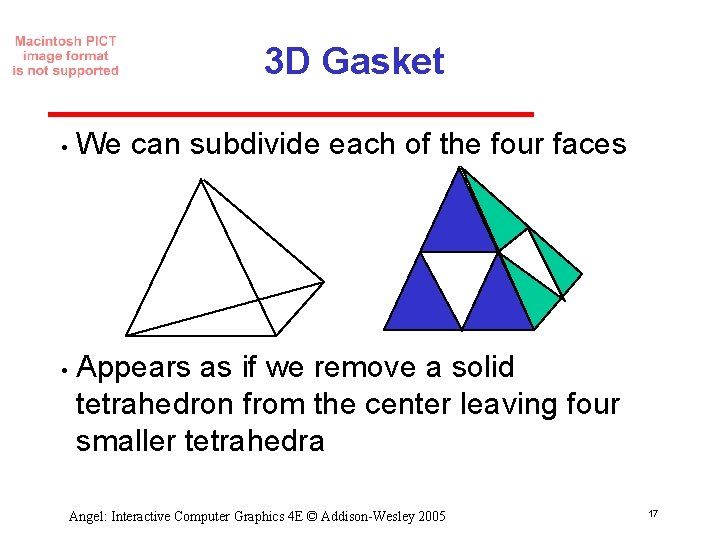
3 D Gasket • • We can subdivide each of the four faces Appears as if we remove a solid tetrahedron from the center leaving four smaller tetrahedra Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 17
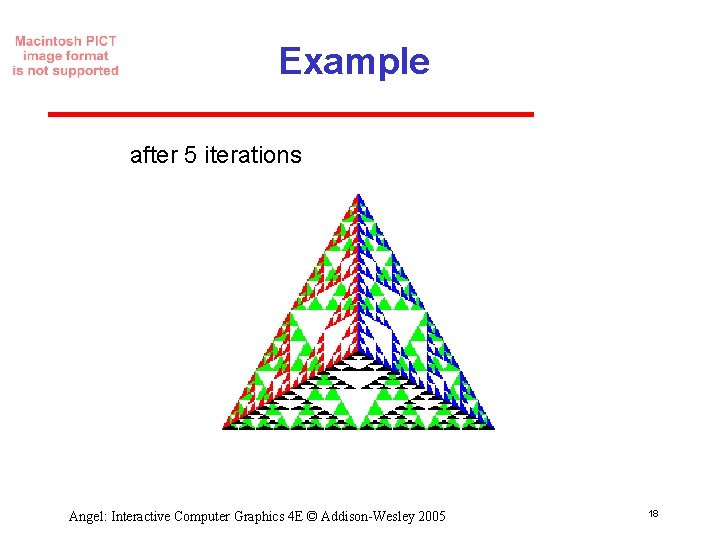
Example after 5 iterations Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 18
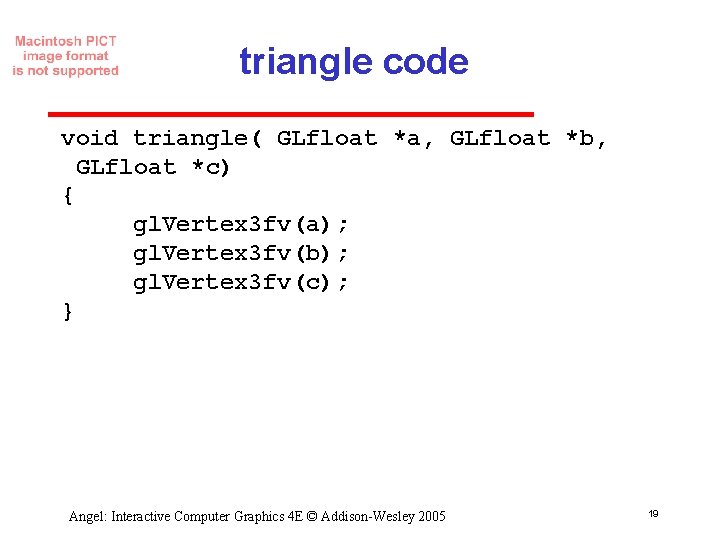
triangle code void triangle( GLfloat *a, GLfloat *b, GLfloat *c) { gl. Vertex 3 fv(a); gl. Vertex 3 fv(b); gl. Vertex 3 fv(c); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 19
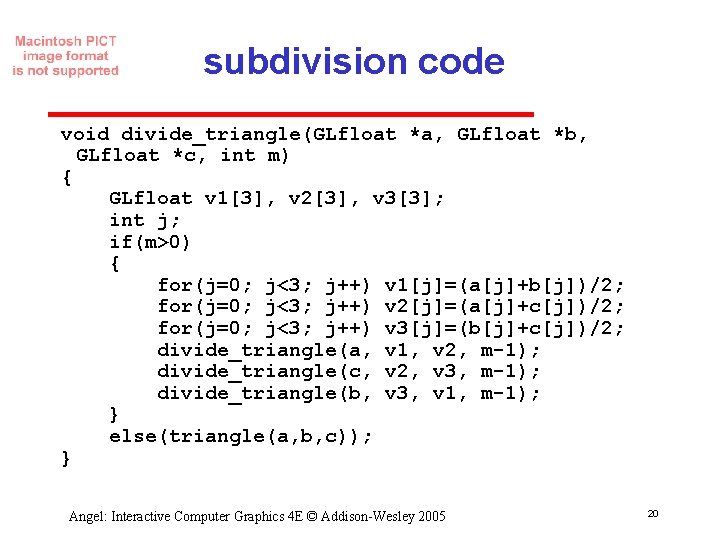
subdivision code void divide_triangle(GLfloat *a, GLfloat *b, GLfloat *c, int m) { GLfloat v 1[3], v 2[3], v 3[3]; int j; if(m>0) { for(j=0; j<3; j++) v 1[j]=(a[j]+b[j])/2; for(j=0; j<3; j++) v 2[j]=(a[j]+c[j])/2; for(j=0; j<3; j++) v 3[j]=(b[j]+c[j])/2; divide_triangle(a, v 1, v 2, m-1); divide_triangle(c, v 2, v 3, m-1); divide_triangle(b, v 3, v 1, m-1); } else(triangle(a, b, c)); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 20
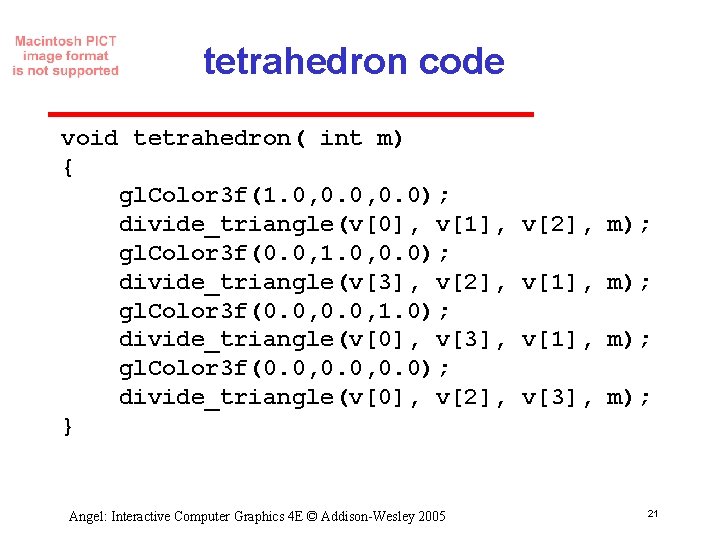
tetrahedron code void tetrahedron( int m) { gl. Color 3 f(1. 0, 0. 0); divide_triangle(v[0], v[1], gl. Color 3 f(0. 0, 1. 0, 0. 0); divide_triangle(v[3], v[2], gl. Color 3 f(0. 0, 1. 0); divide_triangle(v[0], v[3], gl. Color 3 f(0. 0, 0. 0); divide_triangle(v[0], v[2], } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 v[2], m); v[1], m); v[3], m); 21
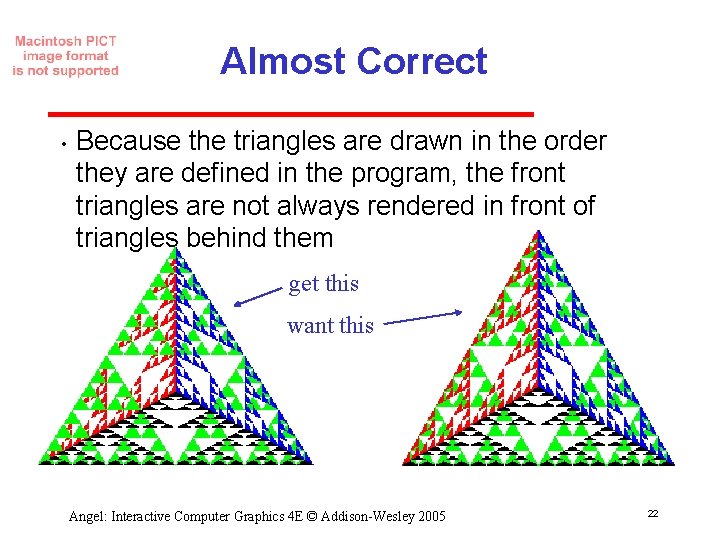
Almost Correct • Because the triangles are drawn in the order they are defined in the program, the front triangles are not always rendered in front of triangles behind them get this want this Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 22
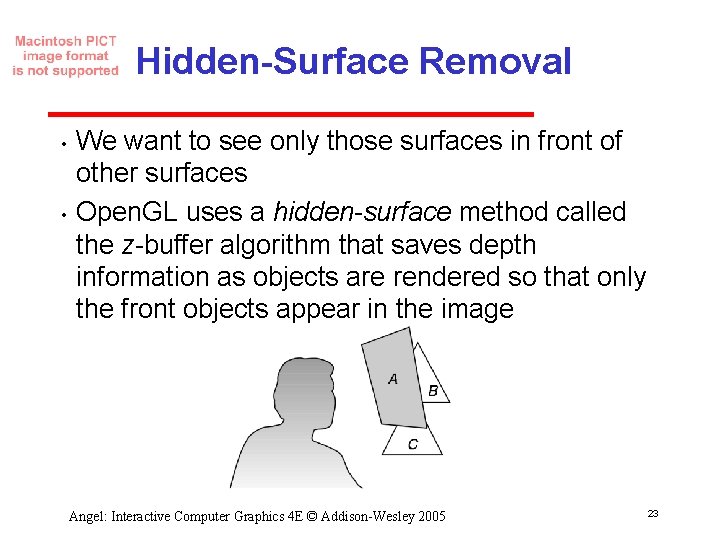
Hidden-Surface Removal • • We want to see only those surfaces in front of other surfaces Open. GL uses a hidden-surface method called the z buffer algorithm that saves depth information as objects are rendered so that only the front objects appear in the image Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 23
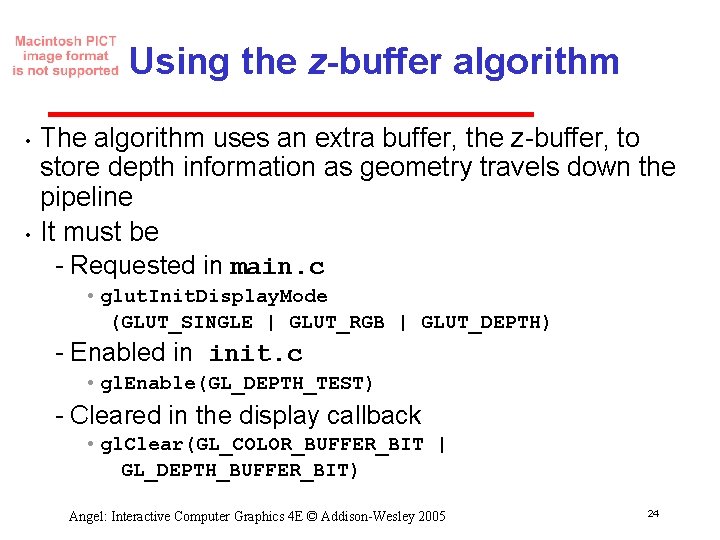
Using the z-buffer algorithm • • The algorithm uses an extra buffer, the z buffer, to store depth information as geometry travels down the pipeline It must be Requested in main. c • glut. Init. Display. Mode (GLUT_SINGLE | GLUT_RGB | GLUT_DEPTH) Enabled in init. c • gl. Enable(GL_DEPTH_TEST) Cleared in the display callback • gl. Clear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 24
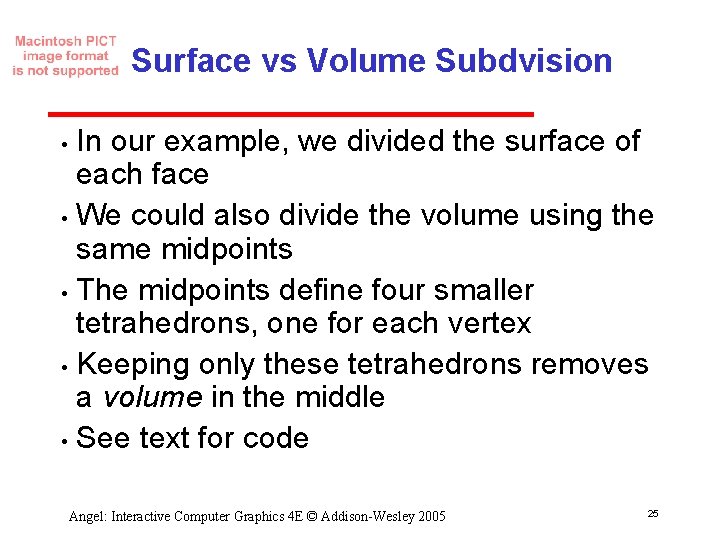
Surface vs Volume Subdvision In our example, we divided the surface of each face • We could also divide the volume using the same midpoints • The midpoints define four smaller tetrahedrons, one for each vertex • Keeping only these tetrahedrons removes a volume in the middle • See text for code • Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 25
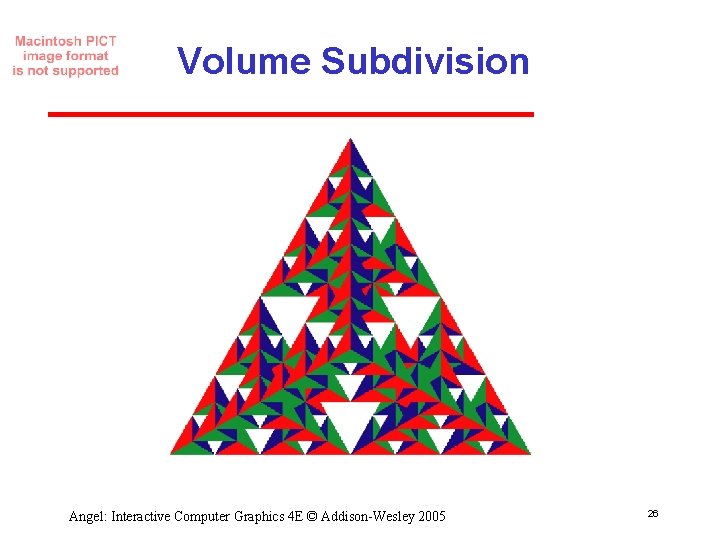
Volume Subdivision Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 26
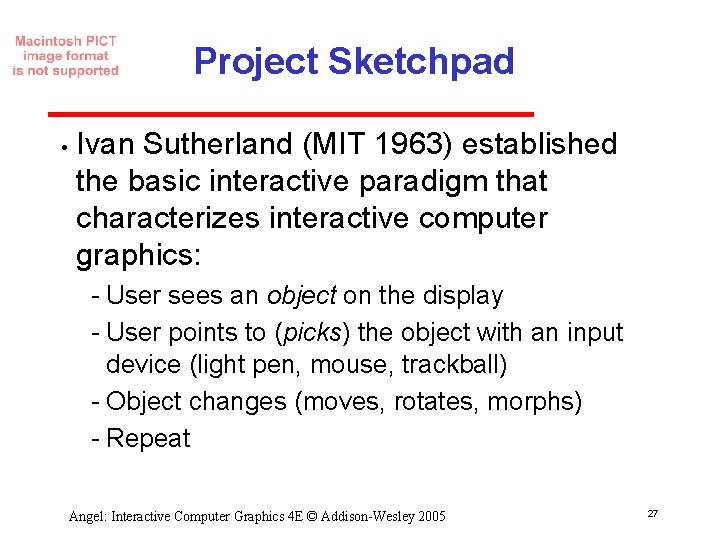
Project Sketchpad • Ivan Sutherland (MIT 1963) established the basic interactive paradigm that characterizes interactive computer graphics: User sees an object on the display User points to (picks) the object with an input device (light pen, mouse, trackball) Object changes (moves, rotates, morphs) Repeat Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 27
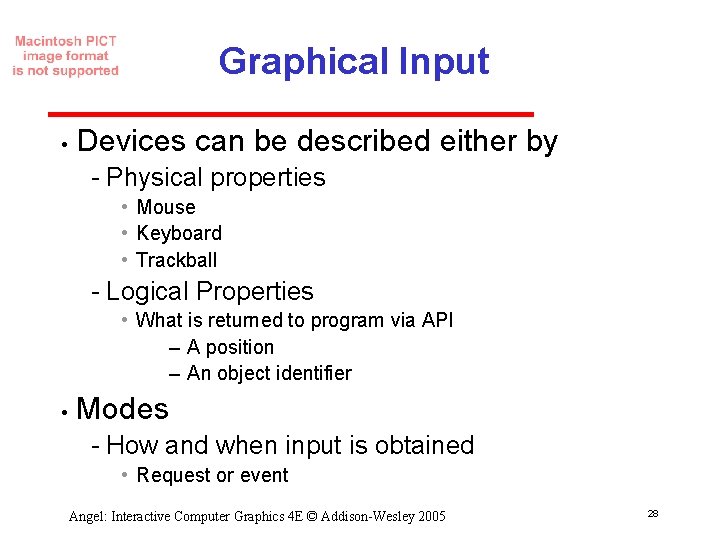
Graphical Input • Devices can be described either by Physical properties • Mouse • Keyboard • Trackball Logical Properties • What is returned to program via API – A position – An object identifier • Modes How and when input is obtained • Request or event Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 28
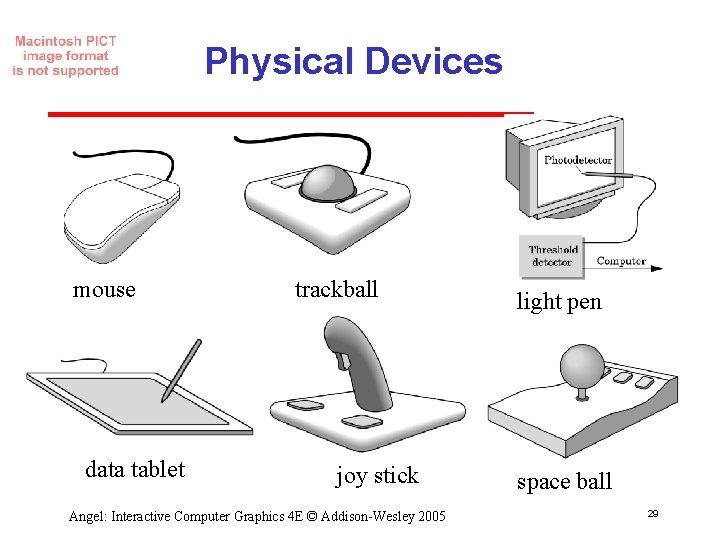
Physical Devices mouse data tablet trackball joy stick Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 light pen space ball 29
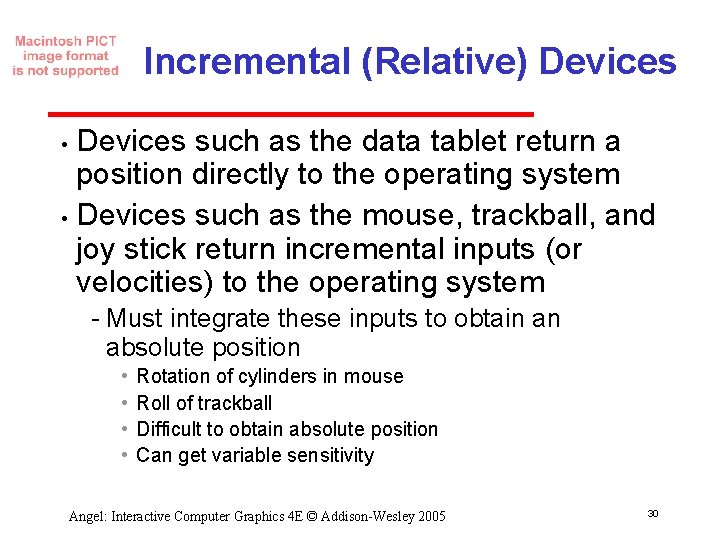
Incremental (Relative) Devices such as the data tablet return a position directly to the operating system • Devices such as the mouse, trackball, and joy stick return incremental inputs (or velocities) to the operating system • Must integrate these inputs to obtain an absolute position • • Rotation of cylinders in mouse Roll of trackball Difficult to obtain absolute position Can get variable sensitivity Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 30
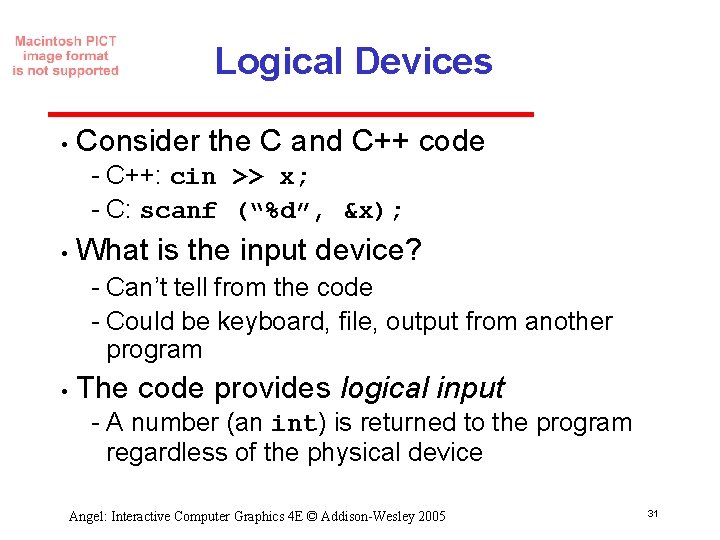
Logical Devices • Consider the C and C++ code C++: cin >> x; C: scanf (“%d”, &x); • What is the input device? Can’t tell from the code Could be keyboard, file, output from another program • The code provides logical input A number (an int) is returned to the program regardless of the physical device Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 31
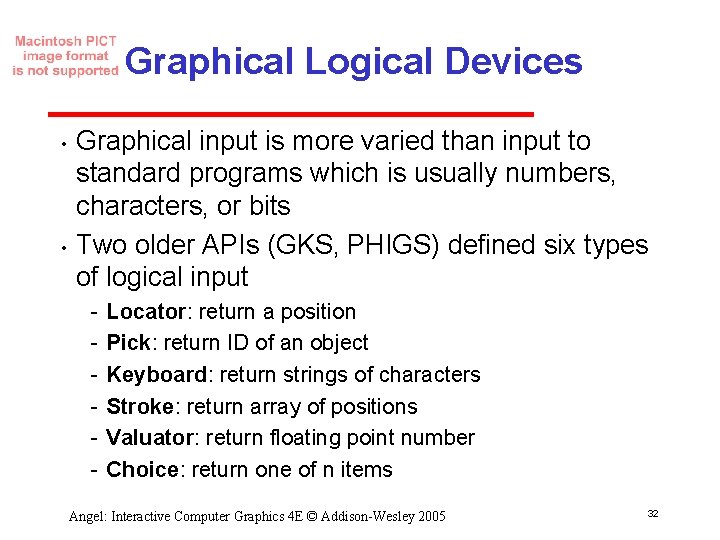
Graphical Logical Devices • • Graphical input is more varied than input to standard programs which is usually numbers, characters, or bits Two older APIs (GKS, PHIGS) defined six types of logical input Locator: return a position Pick: return ID of an object Keyboard: return strings of characters Stroke: return array of positions Valuator: return floating point number Choice: return one of n items Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 32
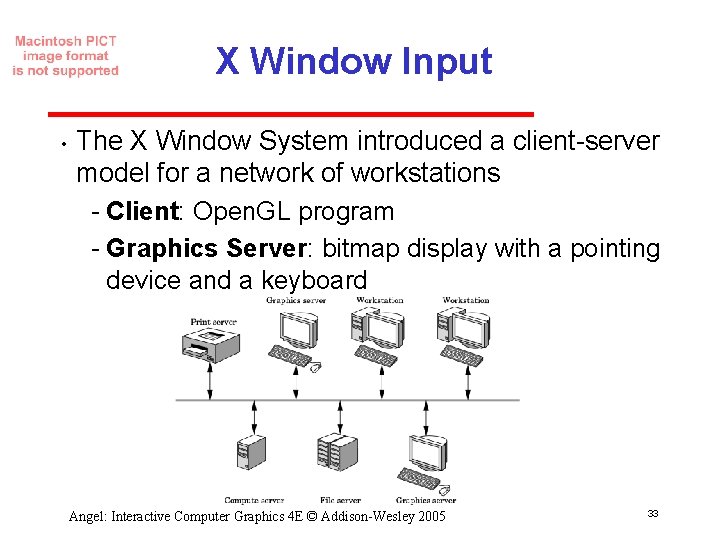
X Window Input • The X Window System introduced a client server model for a network of workstations Client: Open. GL program Graphics Server: bitmap display with a pointing device and a keyboard Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 33
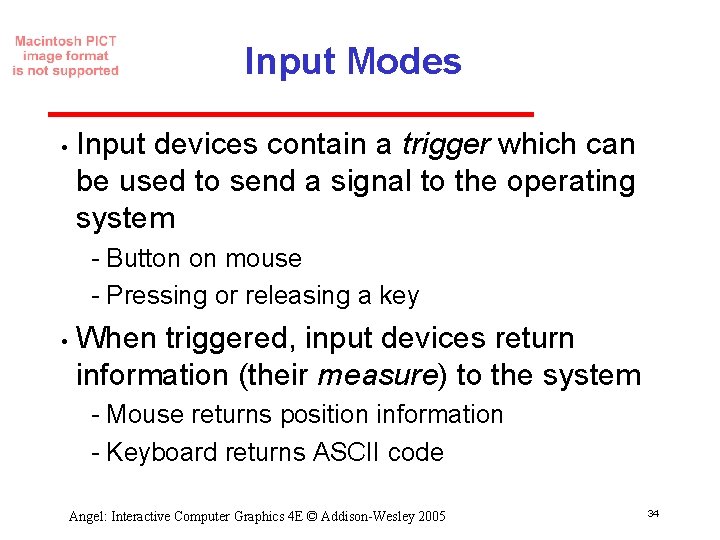
Input Modes • Input devices contain a trigger which can be used to send a signal to the operating system Button on mouse Pressing or releasing a key • When triggered, input devices return information (their measure) to the system Mouse returns position information Keyboard returns ASCII code Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 34
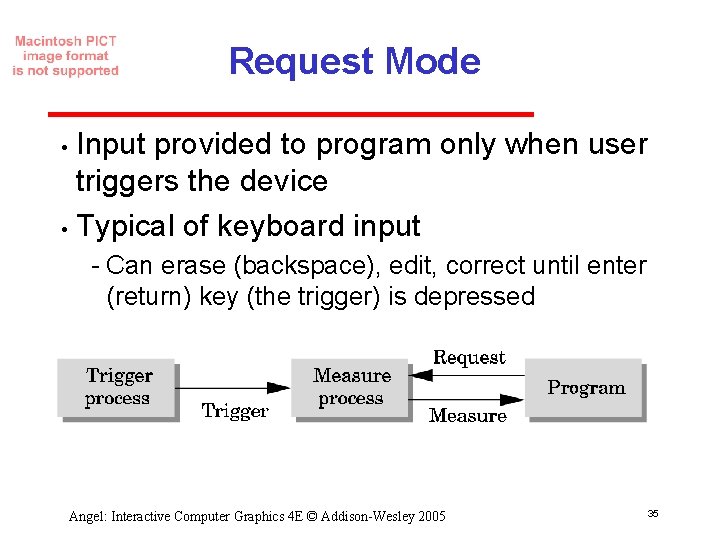
Request Mode Input provided to program only when user triggers the device • Typical of keyboard input • Can erase (backspace), edit, correct until enter (return) key (the trigger) is depressed Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 35
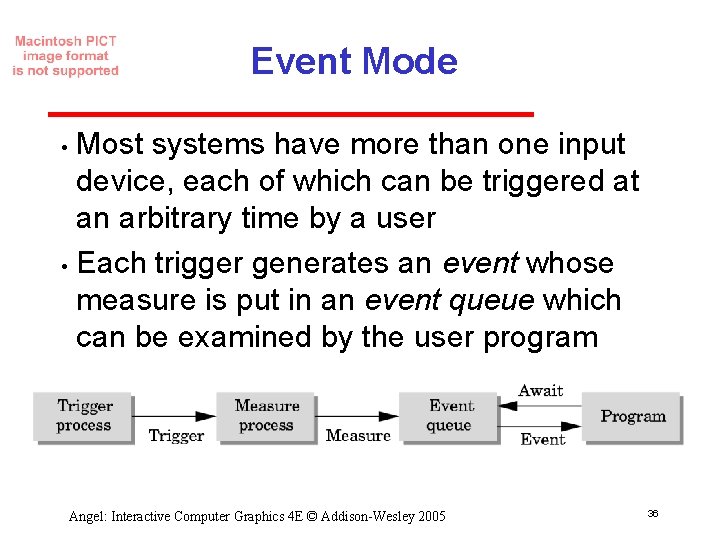
Event Mode Most systems have more than one input device, each of which can be triggered at an arbitrary time by a user • Each trigger generates an event whose measure is put in an event queue which can be examined by the user program • Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 36
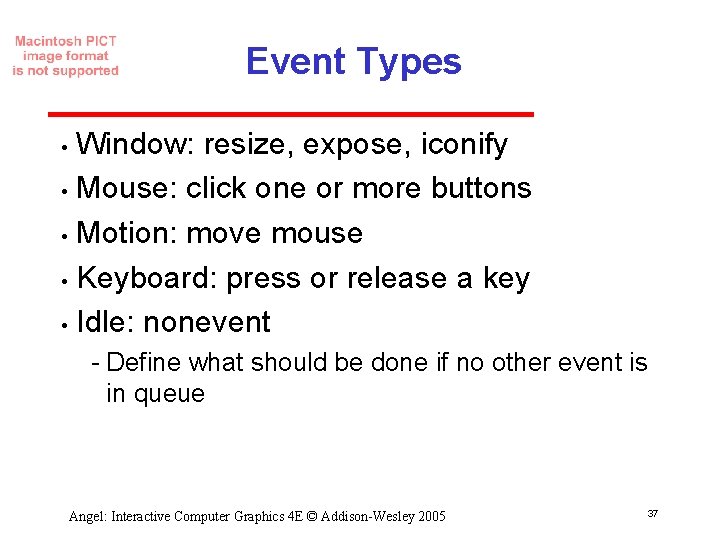
Event Types Window: resize, expose, iconify • Mouse: click one or more buttons • Motion: move mouse • Keyboard: press or release a key • Idle: nonevent • Define what should be done if no other event is in queue Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 37
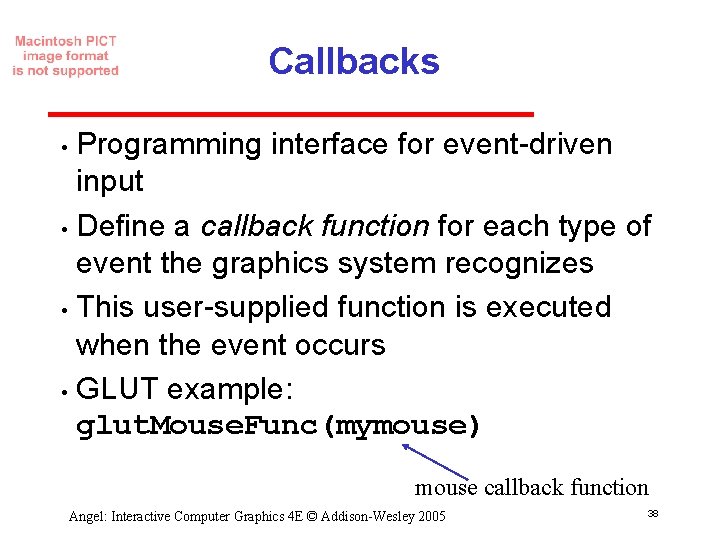
Callbacks Programming interface for event driven input • Define a callback function for each type of event the graphics system recognizes • This user supplied function is executed when the event occurs • GLUT example: glut. Mouse. Func(mymouse) • mouse callback function Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 38
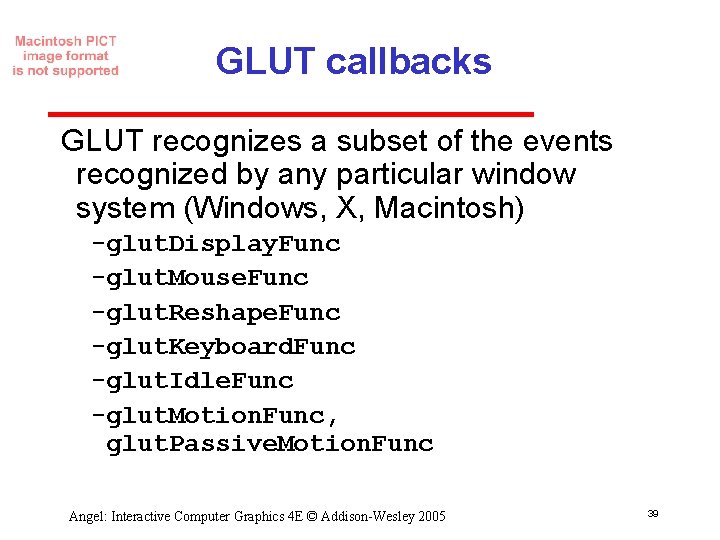
GLUT callbacks GLUT recognizes a subset of the events recognized by any particular window system (Windows, X, Macintosh) glut. Display. Func glut. Mouse. Func glut. Reshape. Func glut. Keyboard. Func glut. Idle. Func glut. Motion. Func, glut. Passive. Motion. Func Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 39
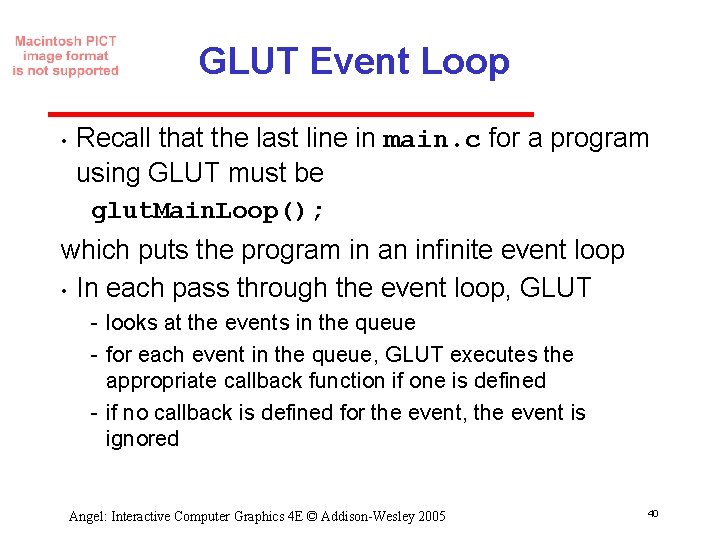
GLUT Event Loop • Recall that the last line in main. c for a program using GLUT must be glut. Main. Loop(); which puts the program in an infinite event loop • In each pass through the event loop, GLUT looks at the events in the queue for each event in the queue, GLUT executes the appropriate callback function if one is defined if no callback is defined for the event, the event is ignored Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 40
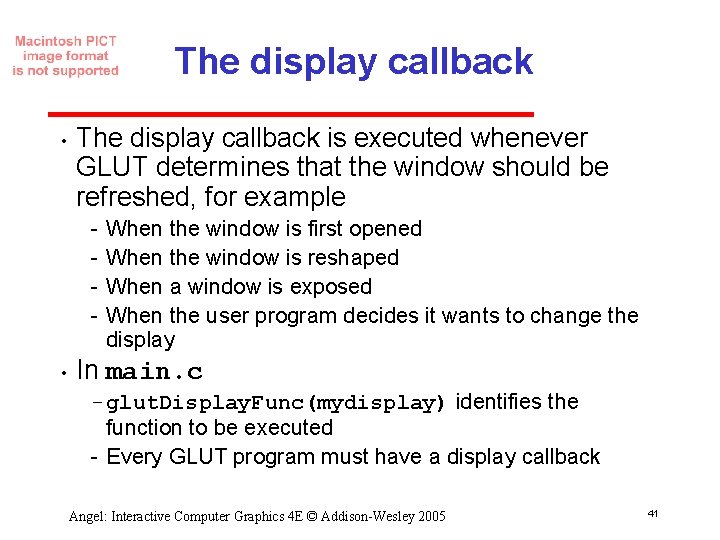
The display callback • The display callback is executed whenever GLUT determines that the window should be refreshed, for example • When the window is first opened When the window is reshaped When a window is exposed When the user program decides it wants to change the display In main. c glut. Display. Func(mydisplay) identifies the function to be executed Every GLUT program must have a display callback Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 41
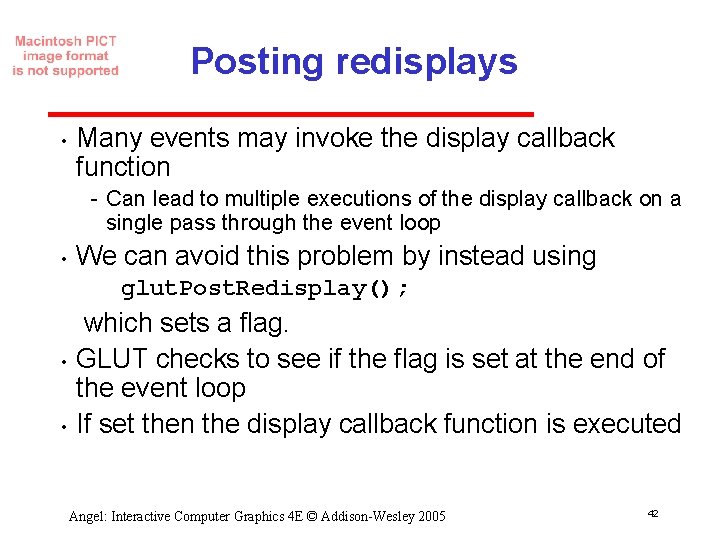
Posting redisplays • Many events may invoke the display callback function Can lead to multiple executions of the display callback on a single pass through the event loop • We can avoid this problem by instead using glut. Post. Redisplay(); • • which sets a flag. GLUT checks to see if the flag is set at the end of the event loop If set then the display callback function is executed Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 42
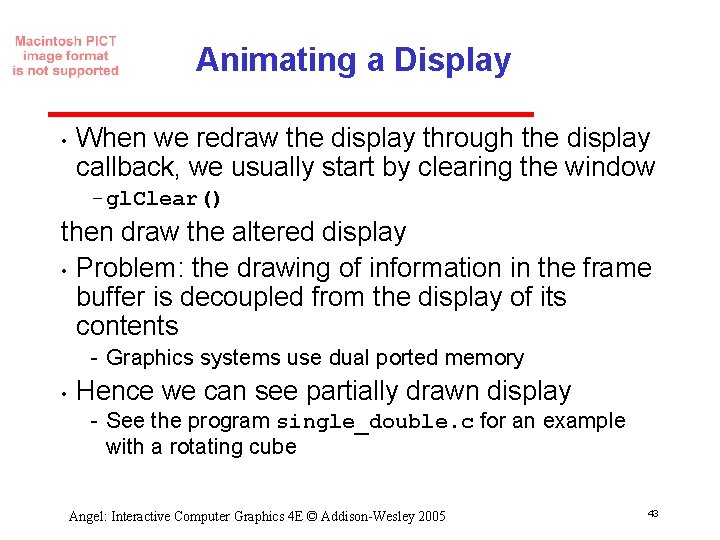
Animating a Display • When we redraw the display through the display callback, we usually start by clearing the window gl. Clear() then draw the altered display • Problem: the drawing of information in the frame buffer is decoupled from the display of its contents Graphics systems use dual ported memory • Hence we can see partially drawn display See the program single_double. c for an example with a rotating cube Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 43
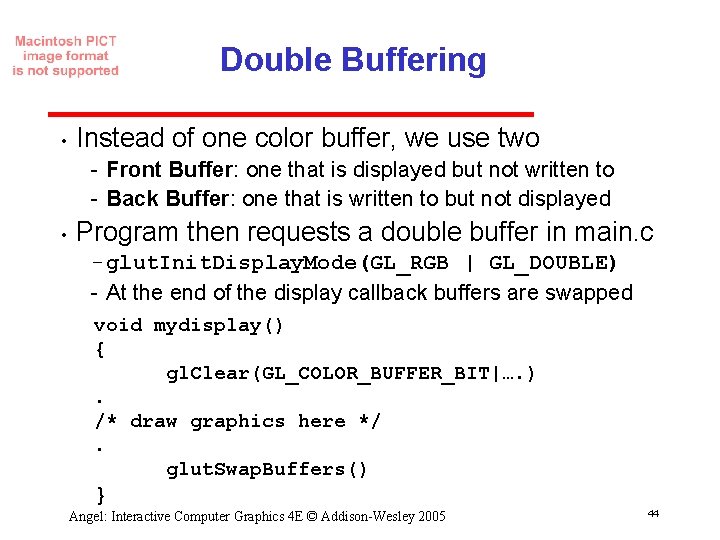
Double Buffering • Instead of one color buffer, we use two Front Buffer: one that is displayed but not written to Back Buffer: one that is written to but not displayed • Program then requests a double buffer in main. c glut. Init. Display. Mode(GL_RGB | GL_DOUBLE) At the end of the display callback buffers are swapped void mydisplay() { gl. Clear(GL_COLOR_BUFFER_BIT|…. ). /* draw graphics here */. glut. Swap. Buffers() } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 44
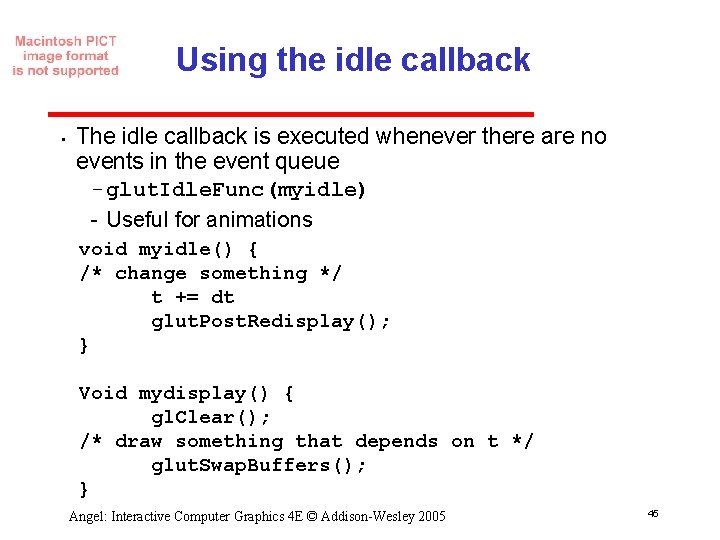
Using the idle callback • The idle callback is executed whenever there are no events in the event queue glut. Idle. Func(myidle) Useful for animations void myidle() { /* change something */ t += dt glut. Post. Redisplay(); } Void mydisplay() { gl. Clear(); /* draw something that depends on t */ glut. Swap. Buffers(); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 45
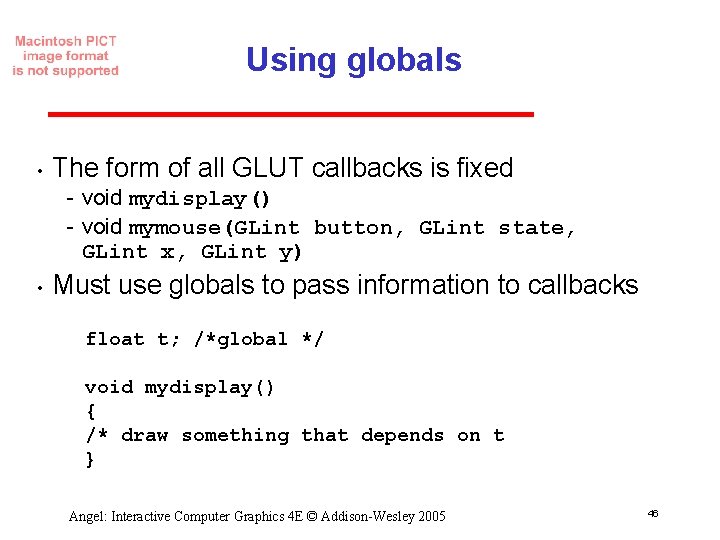
Using globals • The form of all GLUT callbacks is fixed void mydisplay() void mymouse(GLint button, GLint state, GLint x, GLint y) • Must use globals to pass information to callbacks float t; /*global */ void mydisplay() { /* draw something that depends on t } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 46
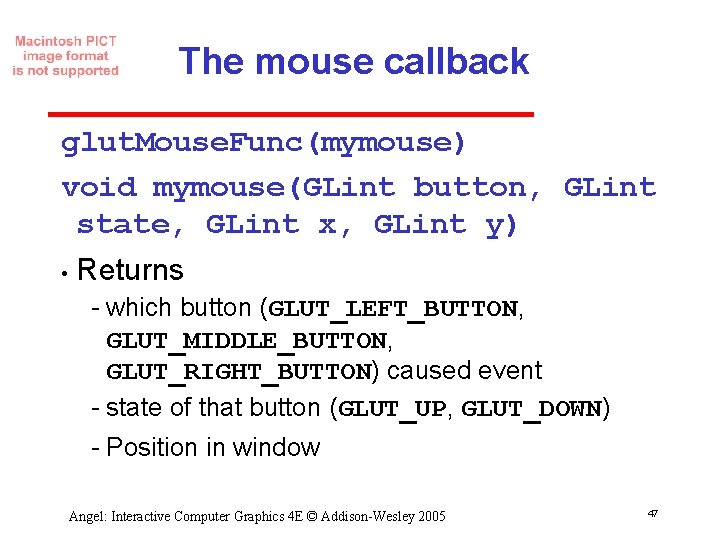
The mouse callback glut. Mouse. Func(mymouse) void mymouse(GLint button, GLint state, GLint x, GLint y) • Returns which button (GLUT_LEFT_BUTTON, GLUT_MIDDLE_BUTTON, GLUT_RIGHT_BUTTON) caused event state of that button (GLUT_UP, GLUT_DOWN) Position in window Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 47
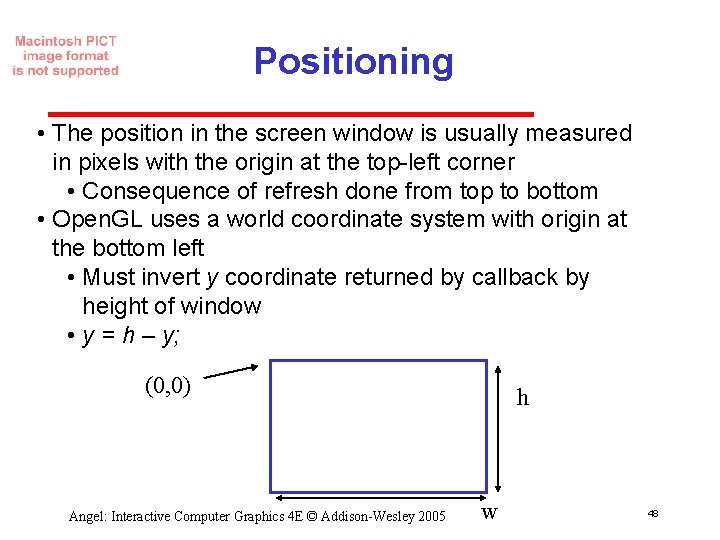
Positioning • The position in the screen window is usually measured in pixels with the origin at the top left corner • Consequence of refresh done from top to bottom • Open. GL uses a world coordinate system with origin at the bottom left • Must invert y coordinate returned by callback by height of window • y = h – y; (0, 0) Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 h w 48
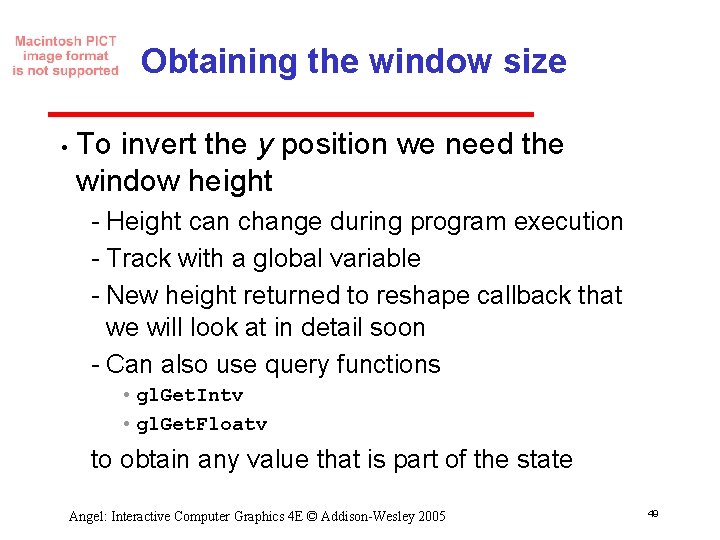
Obtaining the window size • To invert the y position we need the window height Height can change during program execution Track with a global variable New height returned to reshape callback that we will look at in detail soon Can also use query functions • gl. Get. Intv • gl. Get. Floatv to obtain any value that is part of the state Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 49
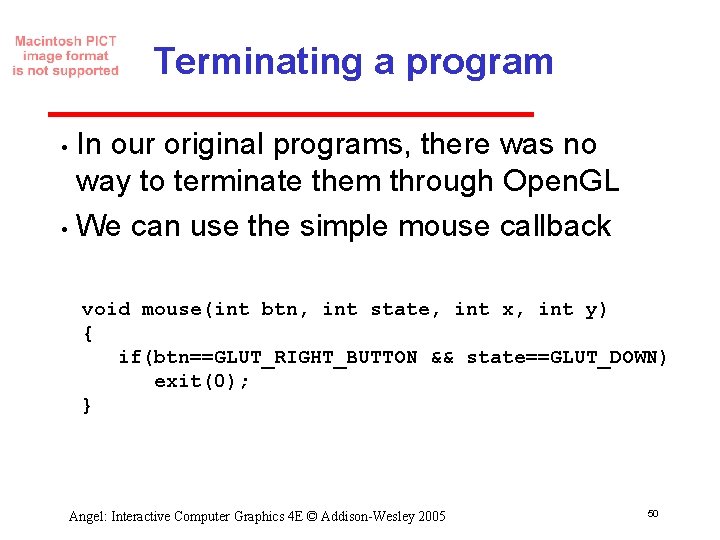
Terminating a program In our original programs, there was no way to terminate them through Open. GL • We can use the simple mouse callback • void mouse(int btn, int state, int x, int y) { if(btn==GLUT_RIGHT_BUTTON && state==GLUT_DOWN) exit(0); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 50
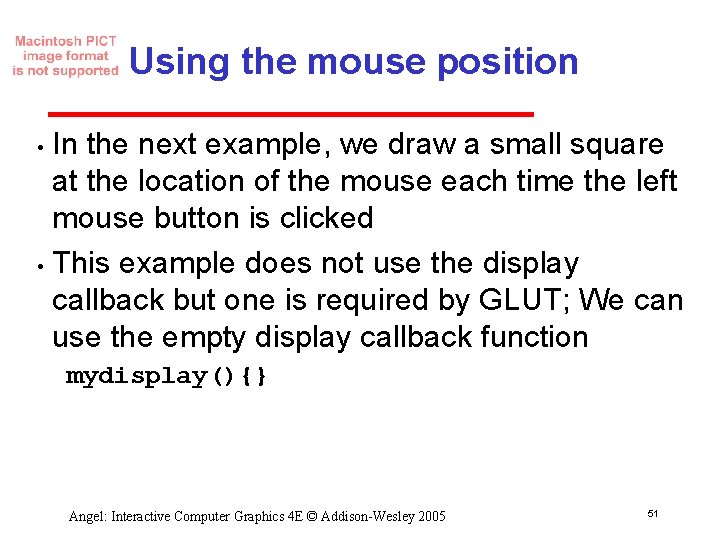
Using the mouse position In the next example, we draw a small square at the location of the mouse each time the left mouse button is clicked • This example does not use the display callback but one is required by GLUT; We can use the empty display callback function • mydisplay(){} Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 51
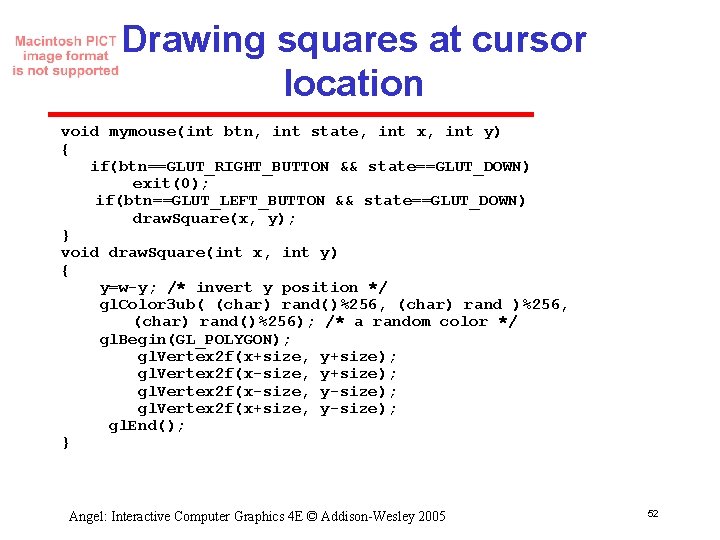
Drawing squares at cursor location void mymouse(int btn, int state, int x, int y) { if(btn==GLUT_RIGHT_BUTTON && state==GLUT_DOWN) exit(0); if(btn==GLUT_LEFT_BUTTON && state==GLUT_DOWN) draw. Square(x, y); } void draw. Square(int x, int y) { y=w-y; /* invert y position */ gl. Color 3 ub( (char) rand()%256, (char) rand()%256); /* a random color */ gl. Begin(GL_POLYGON); gl. Vertex 2 f(x+size, y+size); gl. Vertex 2 f(x-size, y-size); gl. Vertex 2 f(x+size, y-size); gl. End(); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 52
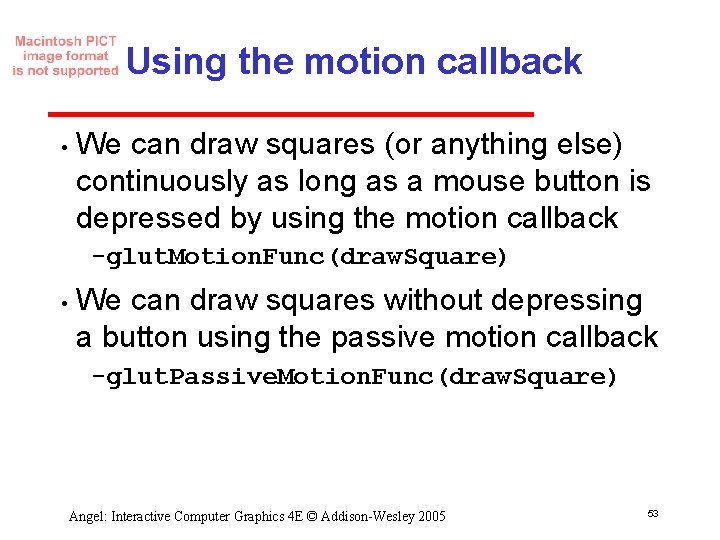
Using the motion callback • We can draw squares (or anything else) continuously as long as a mouse button is depressed by using the motion callback glut. Motion. Func(draw. Square) • We can draw squares without depressing a button using the passive motion callback glut. Passive. Motion. Func(draw. Square) Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 53
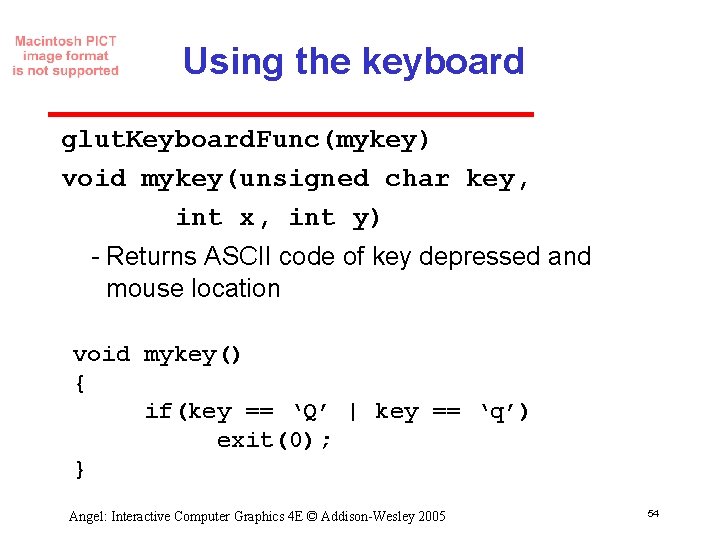
Using the keyboard glut. Keyboard. Func(mykey) void mykey(unsigned char key, int x, int y) Returns ASCII code of key depressed and mouse location void mykey() { if(key == ‘Q’ | key == ‘q’) exit(0); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 54
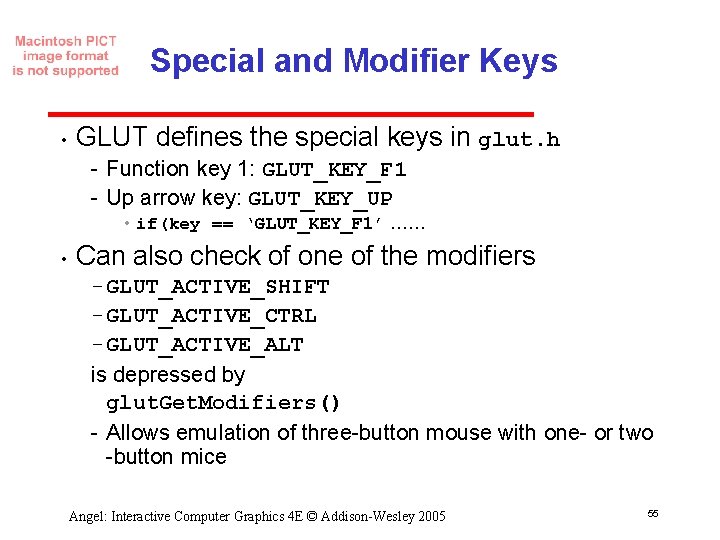
Special and Modifier Keys • GLUT defines the special keys in glut. h Function key 1: GLUT_KEY_F 1 Up arrow key: GLUT_KEY_UP • if(key == ‘GLUT_KEY_F 1’ …… • Can also check of one of the modifiers GLUT_ACTIVE_SHIFT GLUT_ACTIVE_CTRL GLUT_ACTIVE_ALT is depressed by glut. Get. Modifiers() Allows emulation of three button mouse with one or two button mice Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 55
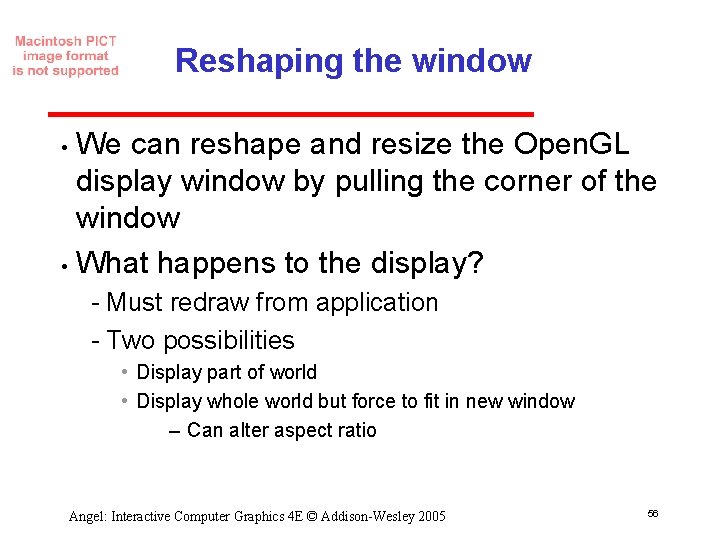
Reshaping the window We can reshape and resize the Open. GL display window by pulling the corner of the window • What happens to the display? • Must redraw from application Two possibilities • Display part of world • Display whole world but force to fit in new window – Can alter aspect ratio Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 56
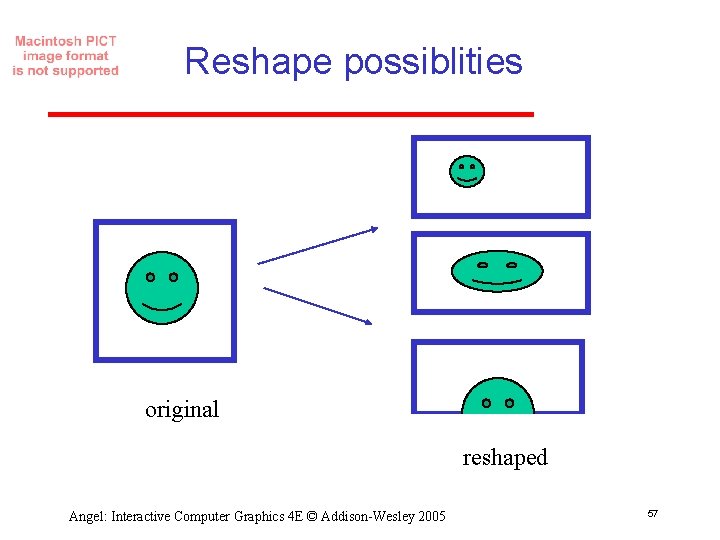
Reshape possiblities original reshaped Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 57
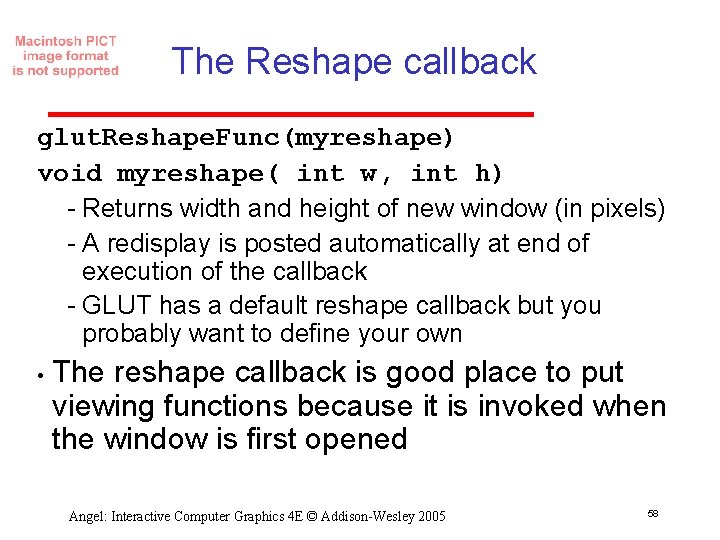
The Reshape callback glut. Reshape. Func(myreshape) void myreshape( int w, int h) Returns width and height of new window (in pixels) A redisplay is posted automatically at end of execution of the callback GLUT has a default reshape callback but you probably want to define your own • The reshape callback is good place to put viewing functions because it is invoked when the window is first opened Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 58
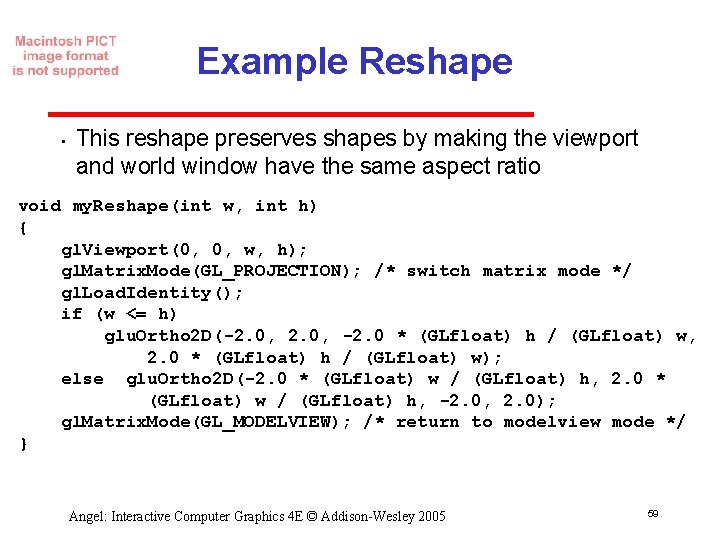
Example Reshape • This reshape preserves shapes by making the viewport and world window have the same aspect ratio void my. Reshape(int w, int h) { gl. Viewport(0, 0, w, h); gl. Matrix. Mode(GL_PROJECTION); /* switch matrix mode */ gl. Load. Identity(); if (w <= h) glu. Ortho 2 D(-2. 0, -2. 0 * (GLfloat) h / (GLfloat) w, 2. 0 * (GLfloat) h / (GLfloat) w); else glu. Ortho 2 D(-2. 0 * (GLfloat) w / (GLfloat) h, -2. 0, 2. 0); gl. Matrix. Mode(GL_MODELVIEW); /* return to modelview mode */ } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 59
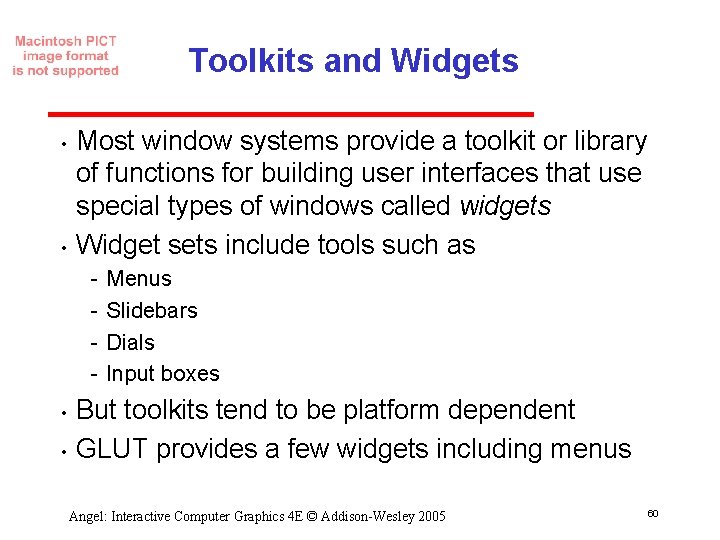
Toolkits and Widgets • • Most window systems provide a toolkit or library of functions for building user interfaces that use special types of windows called widgets Widget sets include tools such as • • Menus Slidebars Dials Input boxes But toolkits tend to be platform dependent GLUT provides a few widgets including menus Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 60
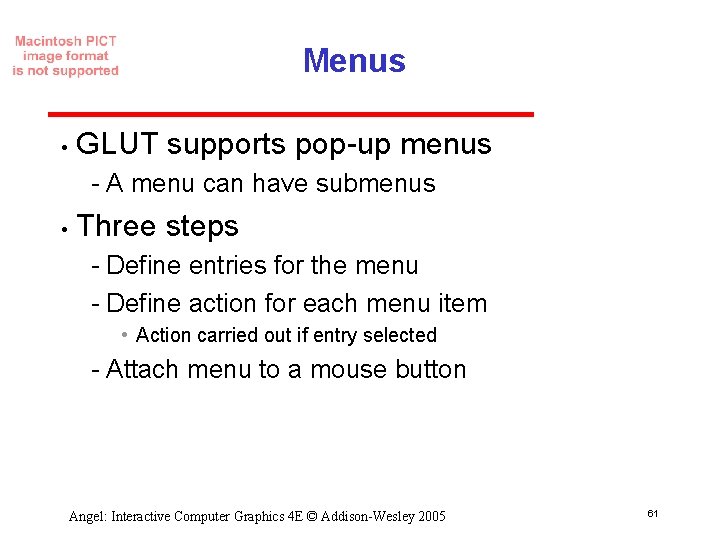
Menus • GLUT supports pop up menus A menu can have submenus • Three steps Define entries for the menu Define action for each menu item • Action carried out if entry selected Attach menu to a mouse button Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 61
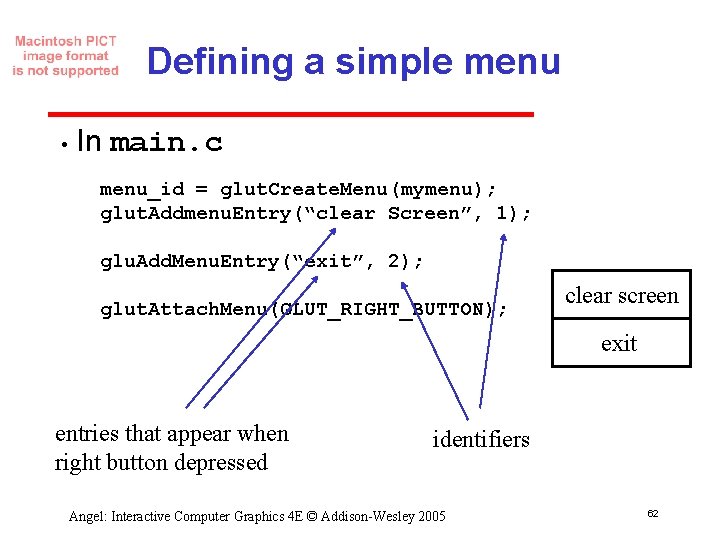
Defining a simple menu • In main. c menu_id = glut. Create. Menu(mymenu); glut. Addmenu. Entry(“clear Screen”, 1); glu. Add. Menu. Entry(“exit”, 2); glut. Attach. Menu(GLUT_RIGHT_BUTTON); clear screen exit entries that appear when right button depressed identifiers Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 62
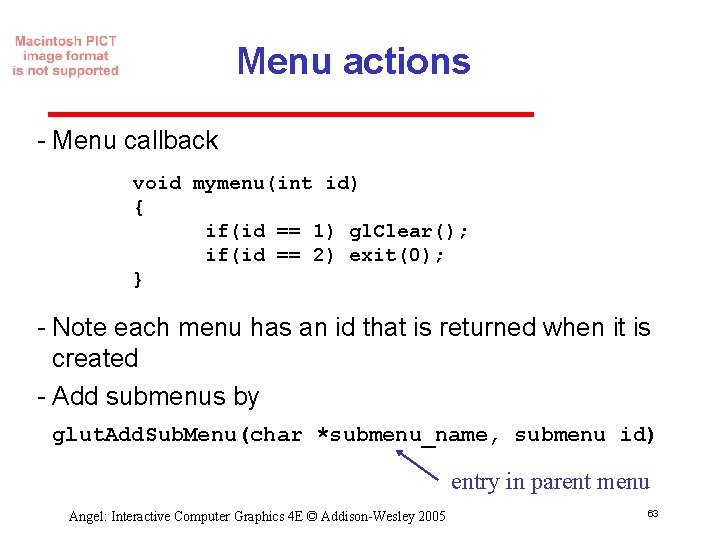
Menu actions Menu callback void mymenu(int id) { if(id == 1) gl. Clear(); if(id == 2) exit(0); } Note each menu has an id that is returned when it is created Add submenus by glut. Add. Sub. Menu(char *submenu_name, submenu id) entry in parent menu Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 63
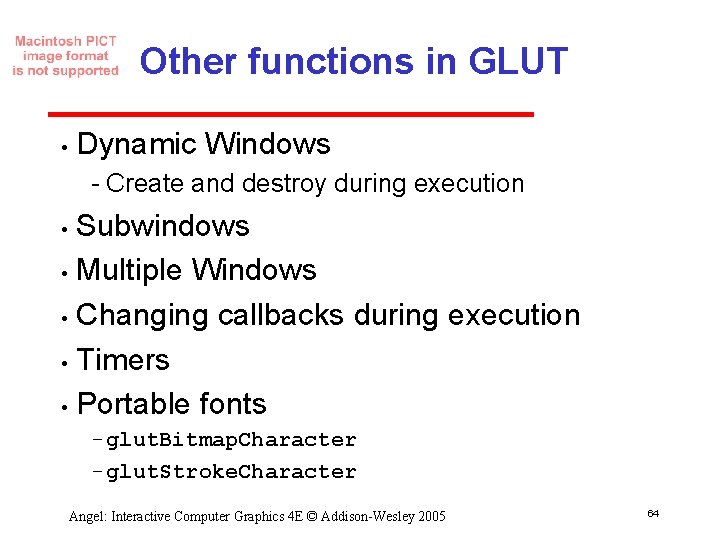
Other functions in GLUT • Dynamic Windows Create and destroy during execution Subwindows • Multiple Windows • Changing callbacks during execution • Timers • Portable fonts • glut. Bitmap. Character glut. Stroke. Character Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 64
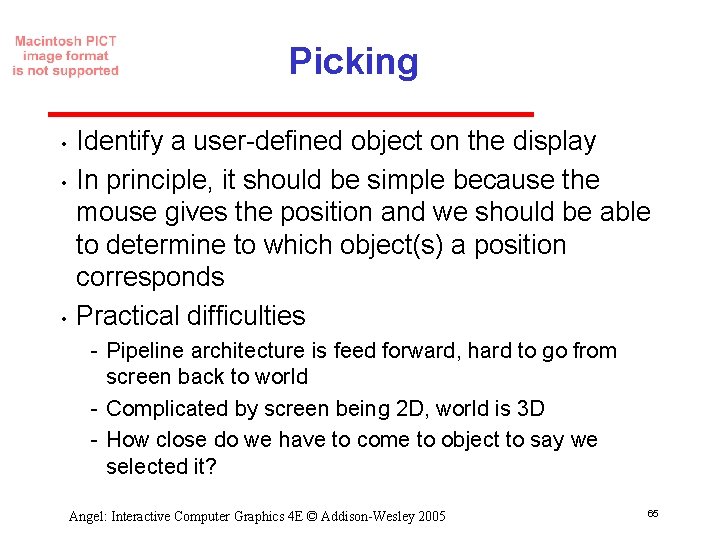
Picking • • • Identify a user defined object on the display In principle, it should be simple because the mouse gives the position and we should be able to determine to which object(s) a position corresponds Practical difficulties Pipeline architecture is feed forward, hard to go from screen back to world Complicated by screen being 2 D, world is 3 D How close do we have to come to object to say we selected it? Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 65
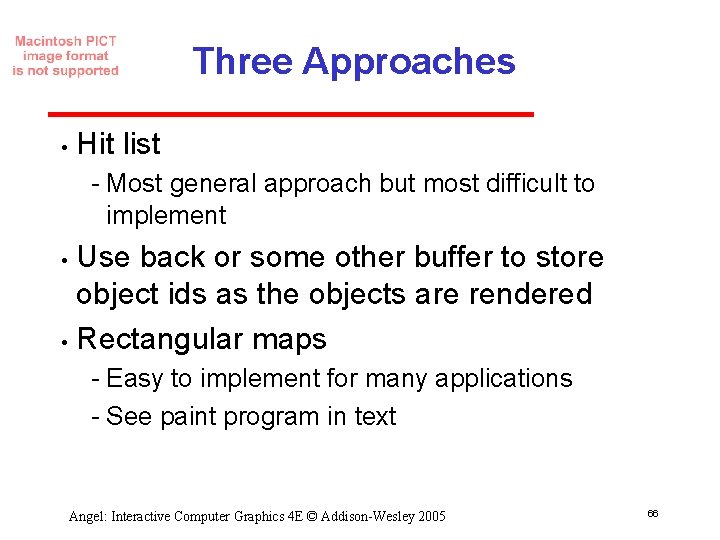
Three Approaches • Hit list Most general approach but most difficult to implement Use back or some other buffer to store object ids as the objects are rendered • Rectangular maps • Easy to implement for many applications See paint program in text Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 66
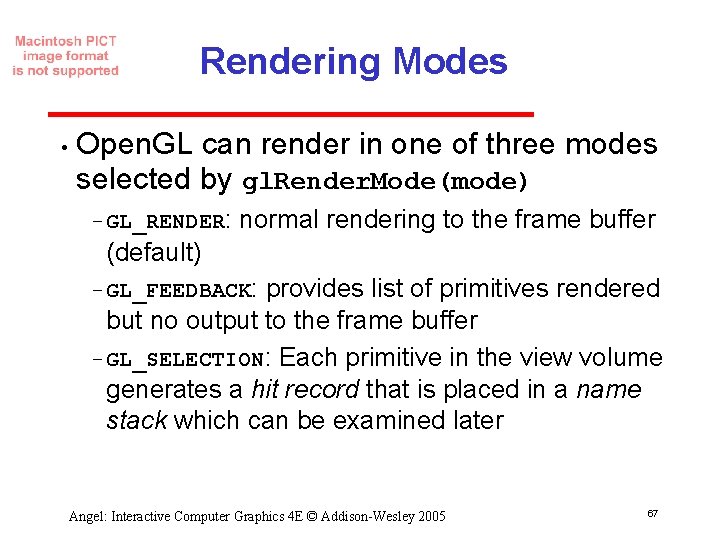
Rendering Modes • Open. GL can render in one of three modes selected by gl. Render. Mode(mode) GL_RENDER: normal rendering to the frame buffer (default) GL_FEEDBACK: provides list of primitives rendered but no output to the frame buffer GL_SELECTION: Each primitive in the view volume generates a hit record that is placed in a name stack which can be examined later Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 67
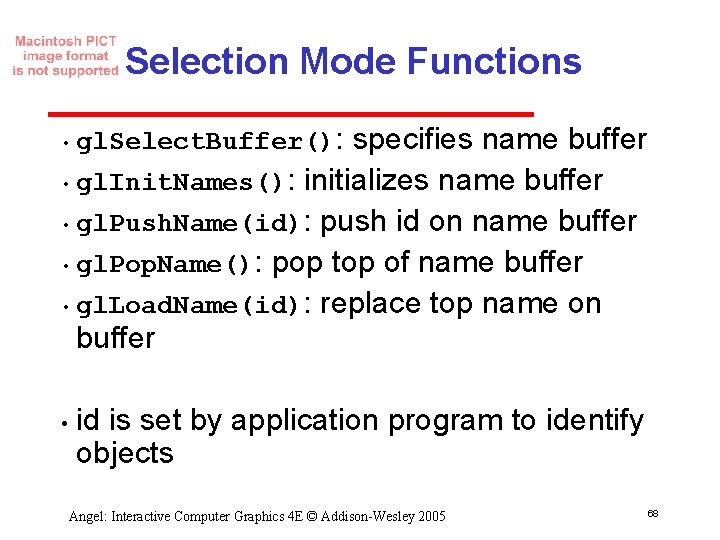
Selection Mode Functions • gl. Select. Buffer(): specifies name buffer • gl. Init. Names(): initializes name buffer • gl. Push. Name(id): push id on name buffer • gl. Pop. Name(): pop top of name buffer • gl. Load. Name(id): replace top name on buffer • id is set by application program to identify objects Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 68
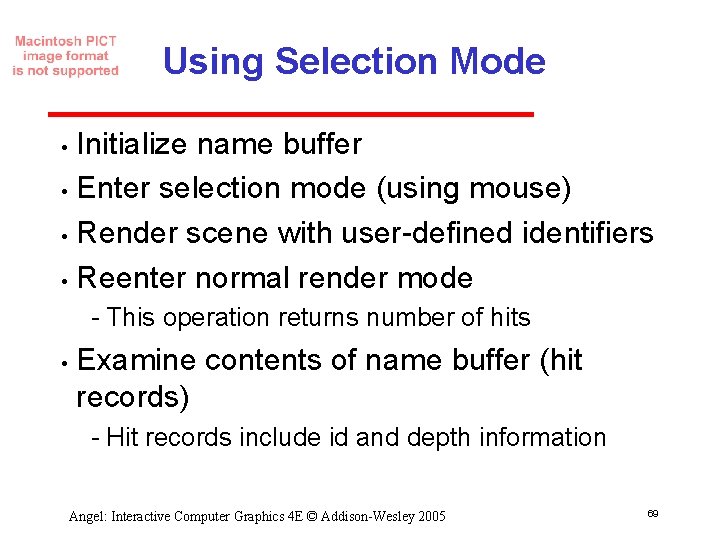
Using Selection Mode Initialize name buffer • Enter selection mode (using mouse) • Render scene with user defined identifiers • Reenter normal render mode • This operation returns number of hits • Examine contents of name buffer (hit records) Hit records include id and depth information Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 69
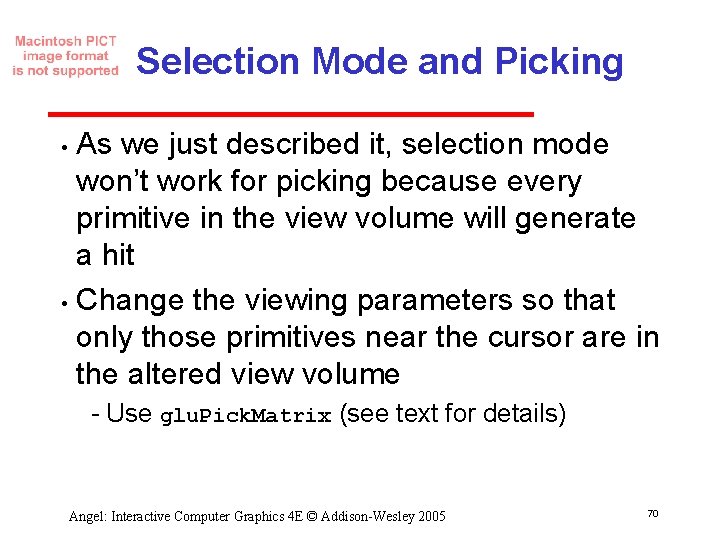
Selection Mode and Picking As we just described it, selection mode won’t work for picking because every primitive in the view volume will generate a hit • Change the viewing parameters so that only those primitives near the cursor are in the altered view volume • Use glu. Pick. Matrix (see text for details) Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 70
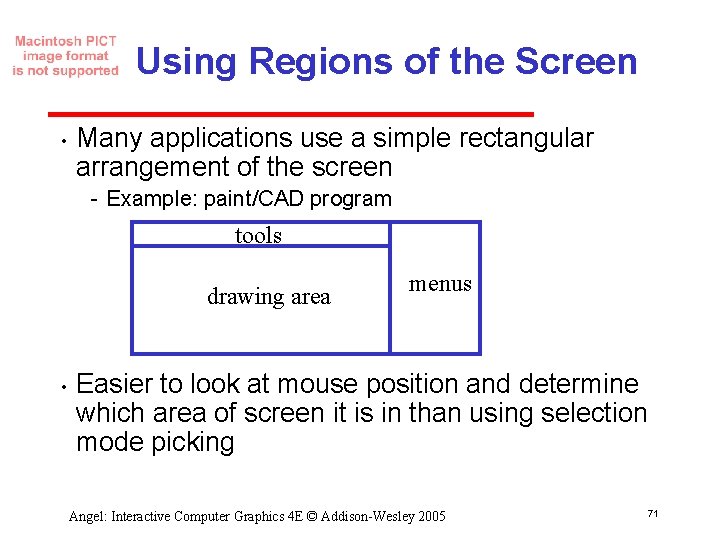
Using Regions of the Screen • Many applications use a simple rectangular arrangement of the screen Example: paint/CAD program tools drawing area • menus Easier to look at mouse position and determine which area of screen it is in than using selection mode picking Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 71
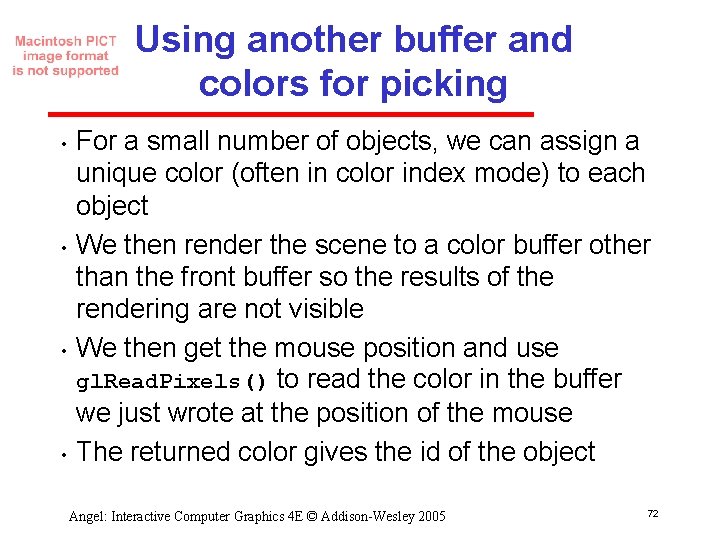
Using another buffer and colors for picking • • For a small number of objects, we can assign a unique color (often in color index mode) to each object We then render the scene to a color buffer other than the front buffer so the results of the rendering are not visible We then get the mouse position and use gl. Read. Pixels() to read the color in the buffer we just wrote at the position of the mouse The returned color gives the id of the object Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 72
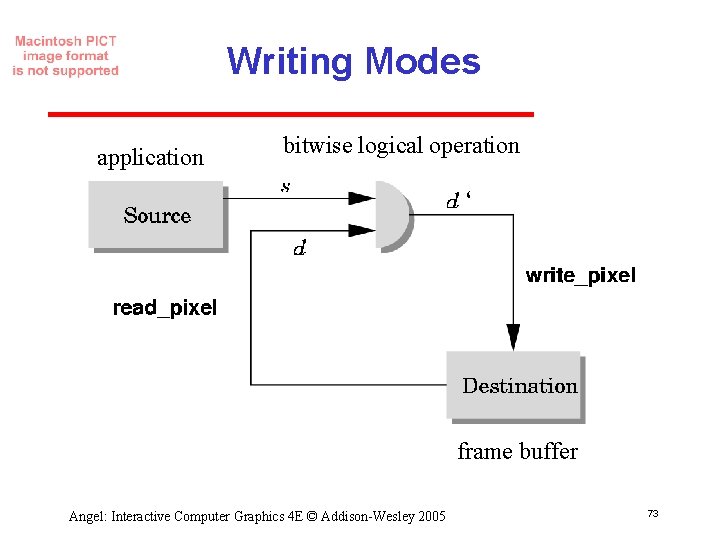
Writing Modes application bitwise logical operation ‘ frame buffer Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 73
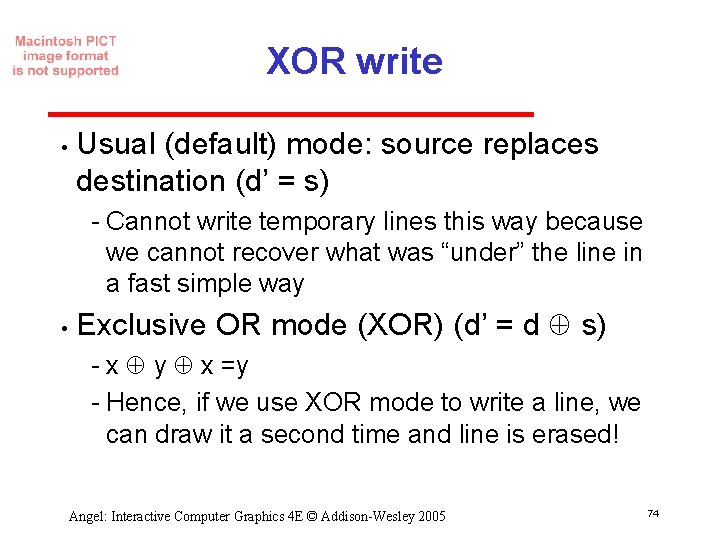
XOR write • Usual (default) mode: source replaces destination (d’ = s) Cannot write temporary lines this way because we cannot recover what was “under” the line in a fast simple way • Exclusive OR mode (XOR) (d’ = d s) x y x =y Hence, if we use XOR mode to write a line, we can draw it a second time and line is erased! Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 74
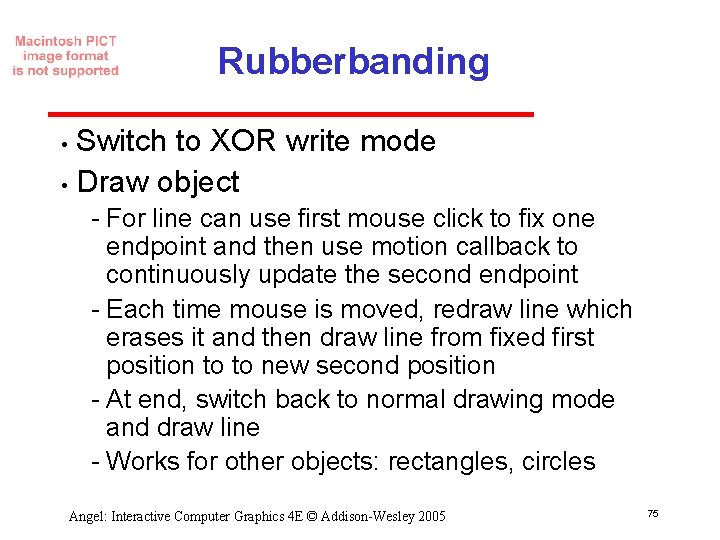
Rubberbanding Switch to XOR write mode • Draw object • For line can use first mouse click to fix one endpoint and then use motion callback to continuously update the second endpoint Each time mouse is moved, redraw line which erases it and then draw line from fixed first position to to new second position At end, switch back to normal drawing mode and draw line Works for other objects: rectangles, circles Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 75
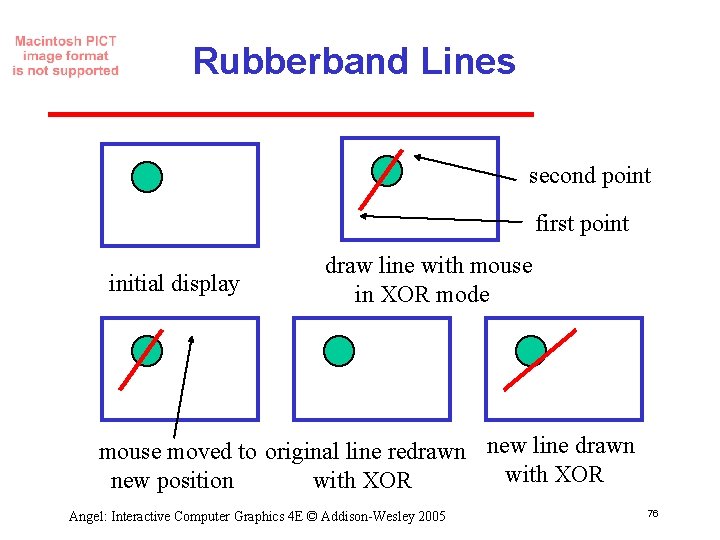
Rubberband Lines second point first point initial display draw line with mouse in XOR mode mouse moved to original line redrawn new line drawn with XOR new position with XOR Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 76
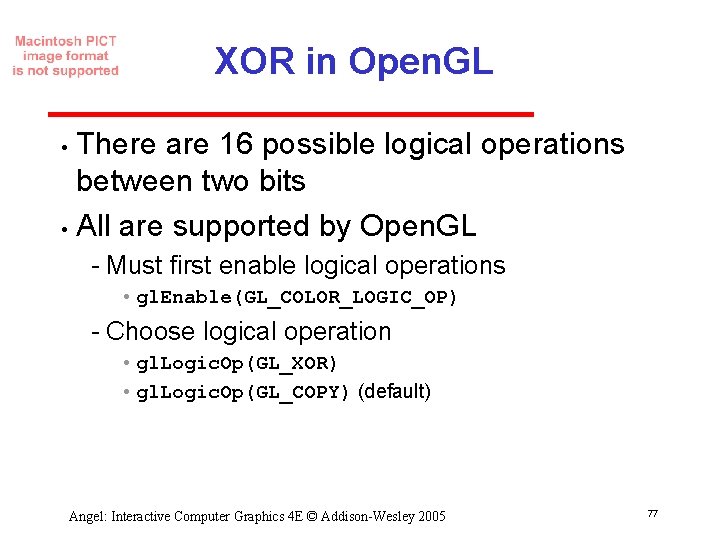
XOR in Open. GL There are 16 possible logical operations between two bits • All are supported by Open. GL • Must first enable logical operations • gl. Enable(GL_COLOR_LOGIC_OP) Choose logical operation • gl. Logic. Op(GL_XOR) • gl. Logic. Op(GL_COPY) (default) Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 77
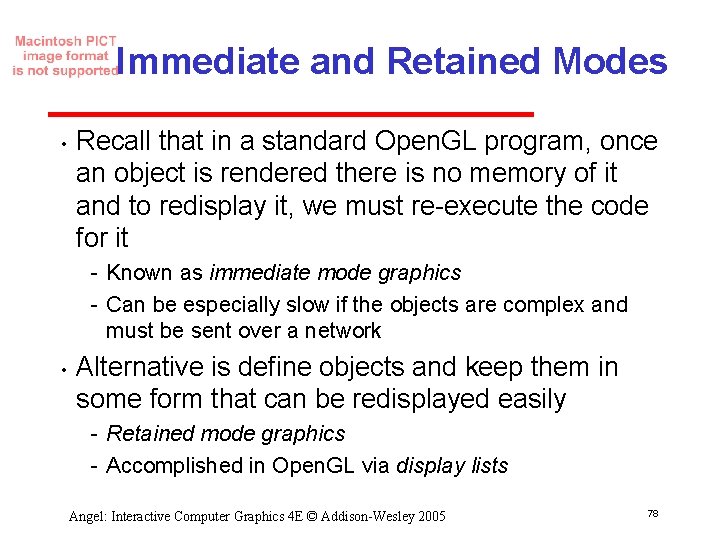
Immediate and Retained Modes • Recall that in a standard Open. GL program, once an object is rendered there is no memory of it and to redisplay it, we must re execute the code for it Known as immediate mode graphics Can be especially slow if the objects are complex and must be sent over a network • Alternative is define objects and keep them in some form that can be redisplayed easily Retained mode graphics Accomplished in Open. GL via display lists Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 78
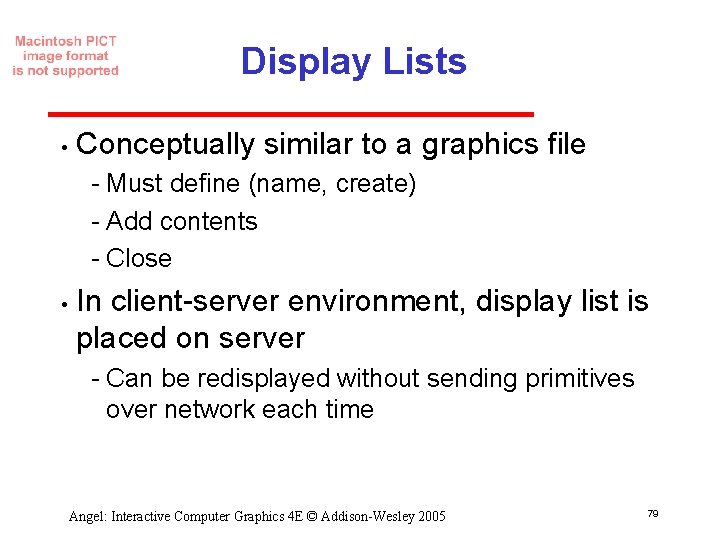
Display Lists • Conceptually similar to a graphics file Must define (name, create) Add contents Close • In client server environment, display list is placed on server Can be redisplayed without sending primitives over network each time Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 79
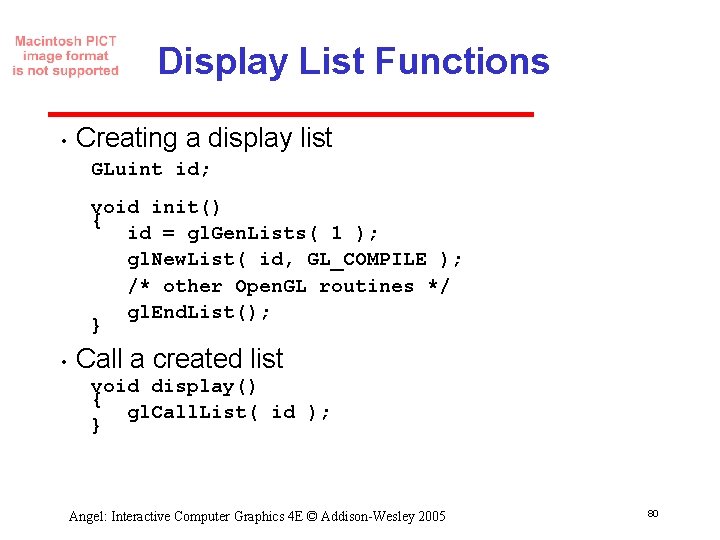
Display List Functions • Creating a display list GLuint id; void init() { id = gl. Gen. Lists( 1 ); gl. New. List( id, GL_COMPILE ); /* other Open. GL routines */ gl. End. List(); } • Call a created list void display() { gl. Call. List( id ); } Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 80
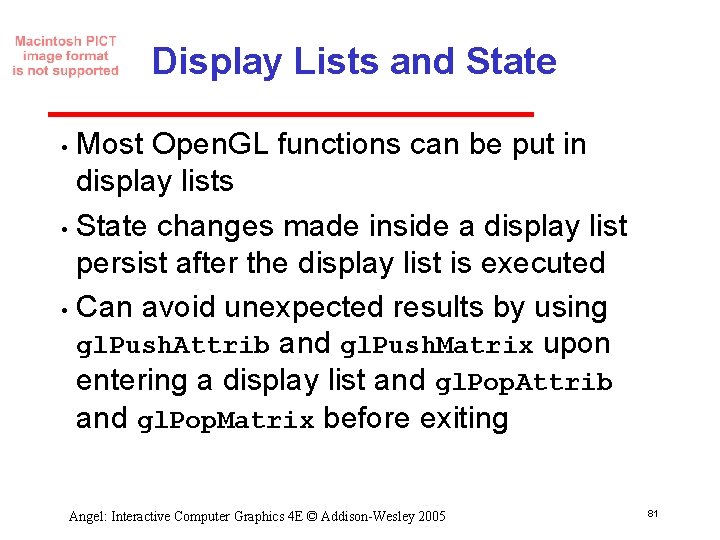
Display Lists and State Most Open. GL functions can be put in display lists • State changes made inside a display list persist after the display list is executed • Can avoid unexpected results by using gl. Push. Attrib and gl. Push. Matrix upon entering a display list and gl. Pop. Attrib and gl. Pop. Matrix before exiting • Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 81
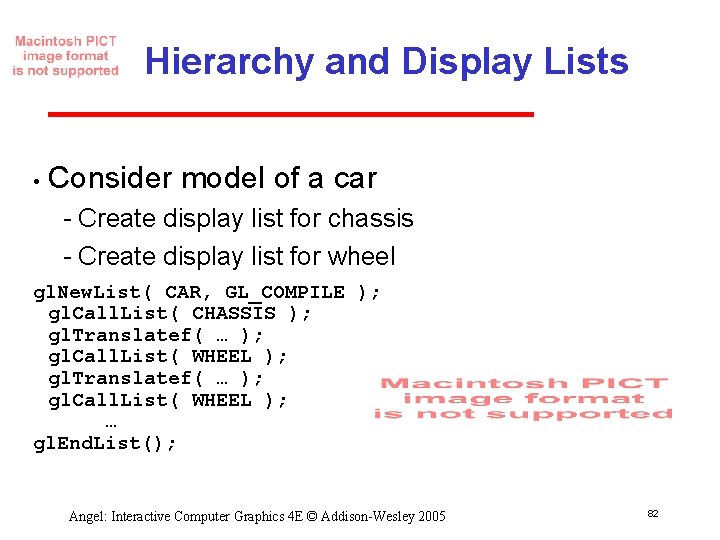
Hierarchy and Display Lists • Consider model of a car Create display list for chassis Create display list for wheel gl. New. List( CAR, GL_COMPILE ); gl. Call. List( CHASSIS ); gl. Translatef( … ); gl. Call. List( WHEEL ); … gl. End. List(); Angel: Interactive Computer Graphics 4 E © Addison-Wesley 2005 82