Programming with Open GL Part 3 Three Dimensions
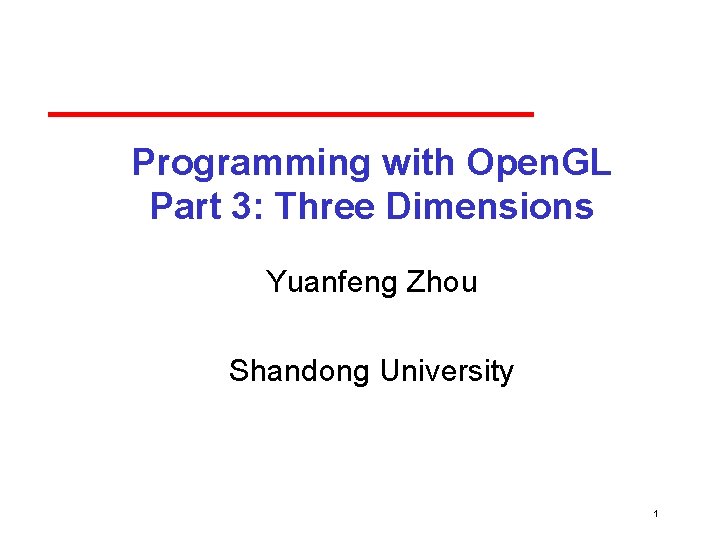
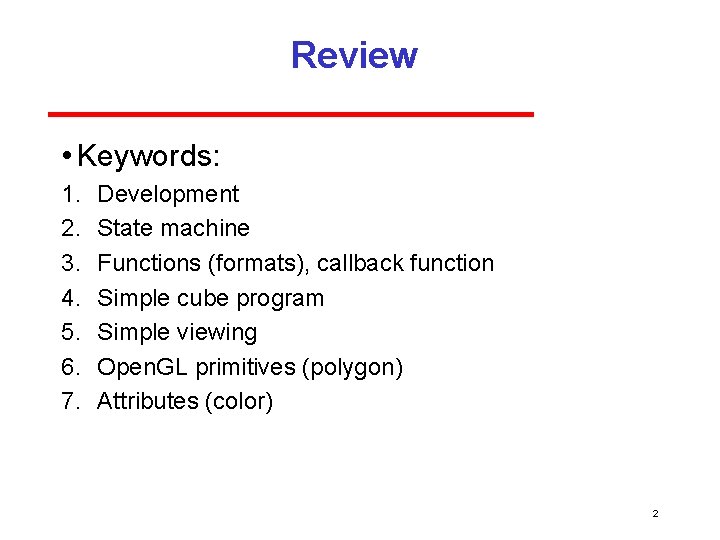
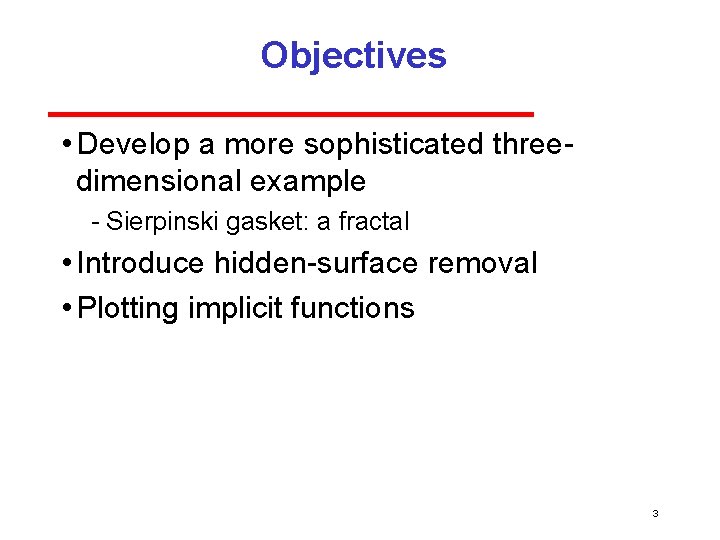
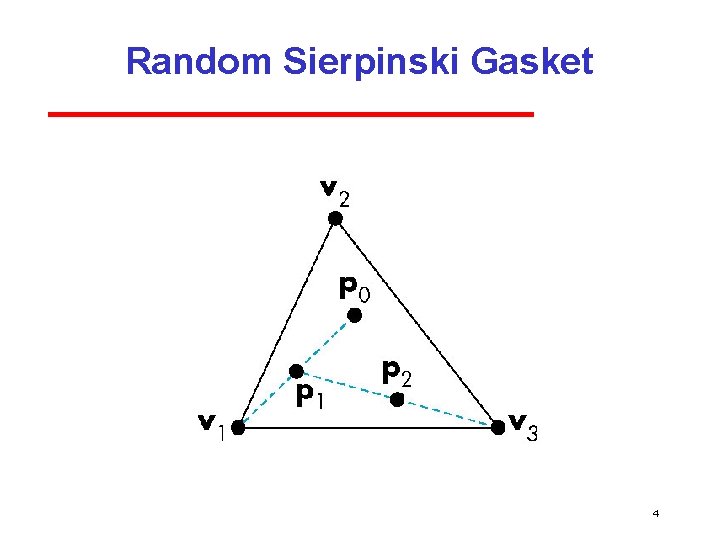
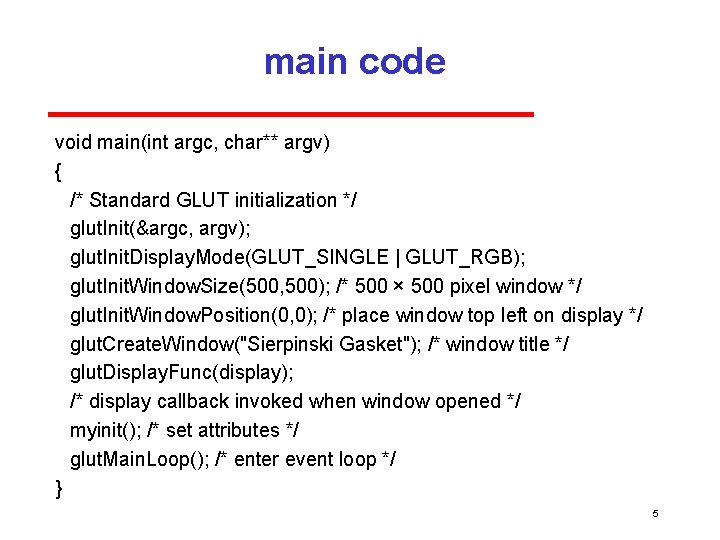
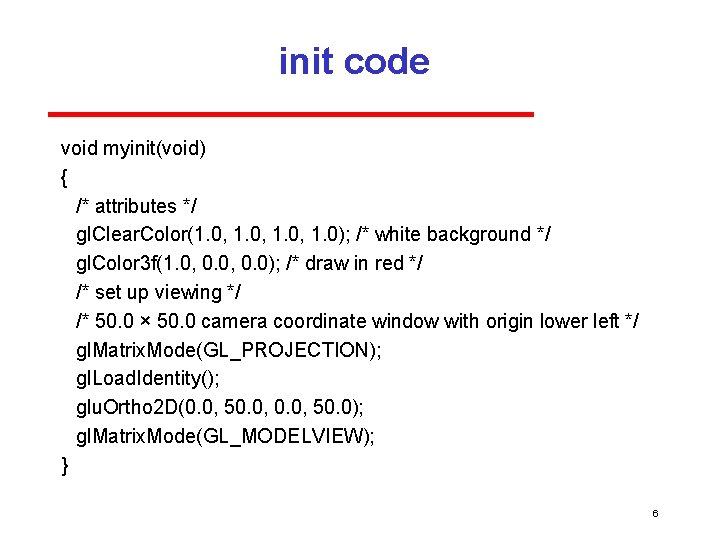
![display code void display(void) { /* A triangle */ GLfloat vertices[3][2]={{0. 0, 0. 0}, display code void display(void) { /* A triangle */ GLfloat vertices[3][2]={{0. 0, 0. 0},](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-7.jpg)
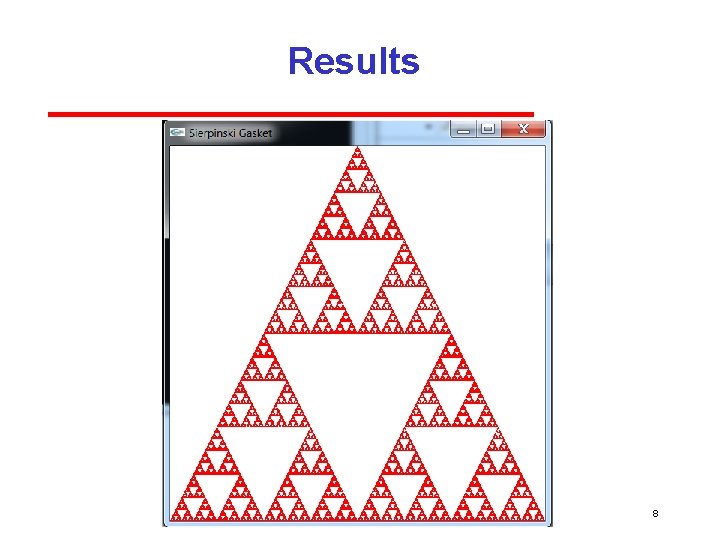
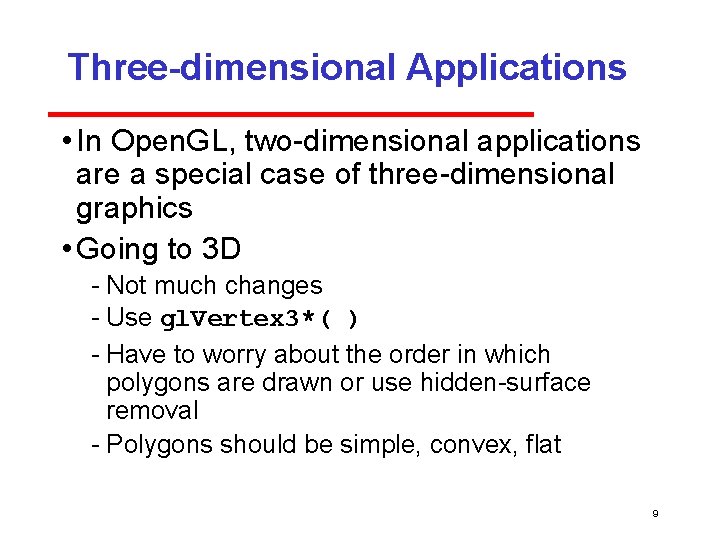
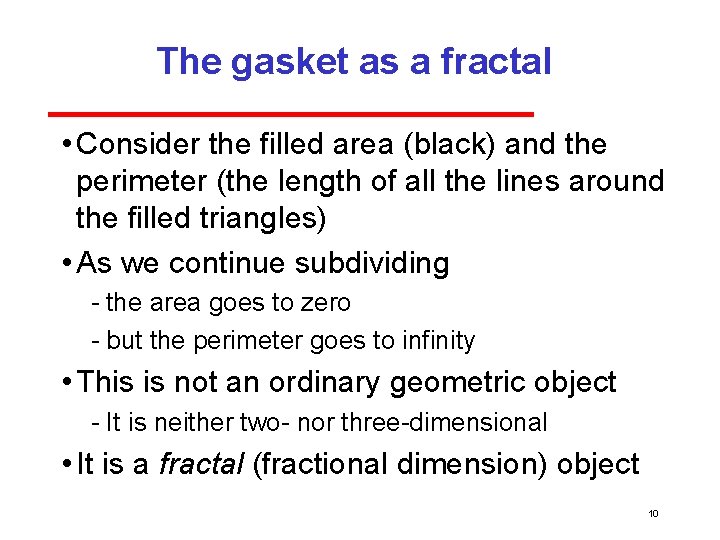
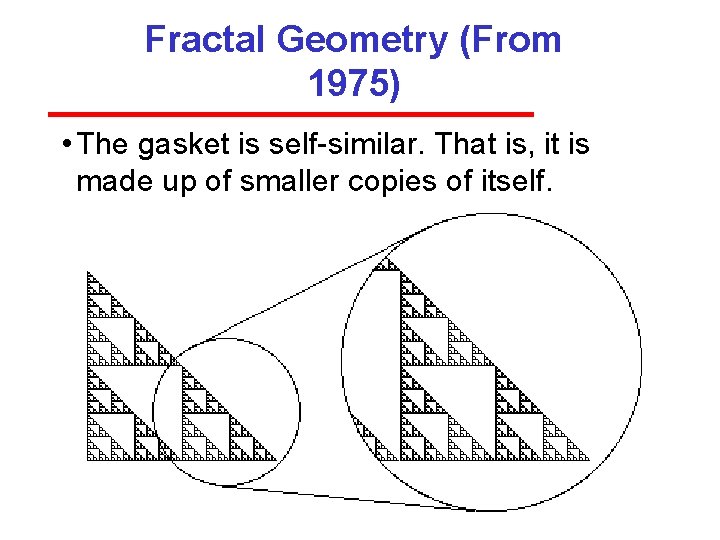
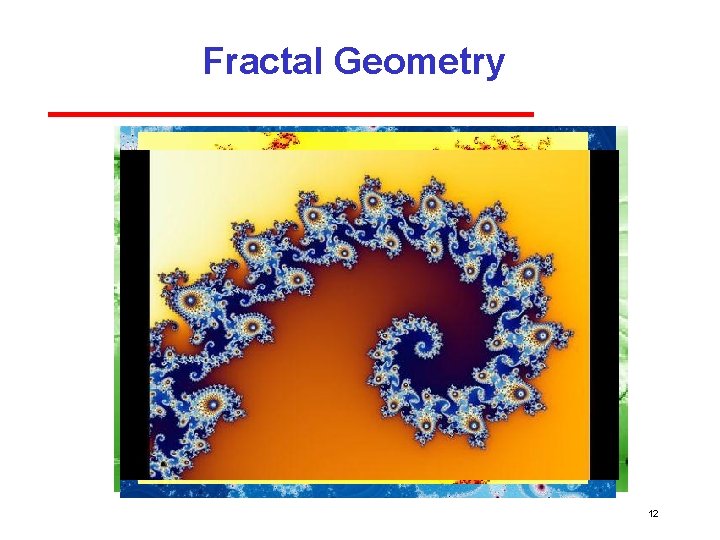
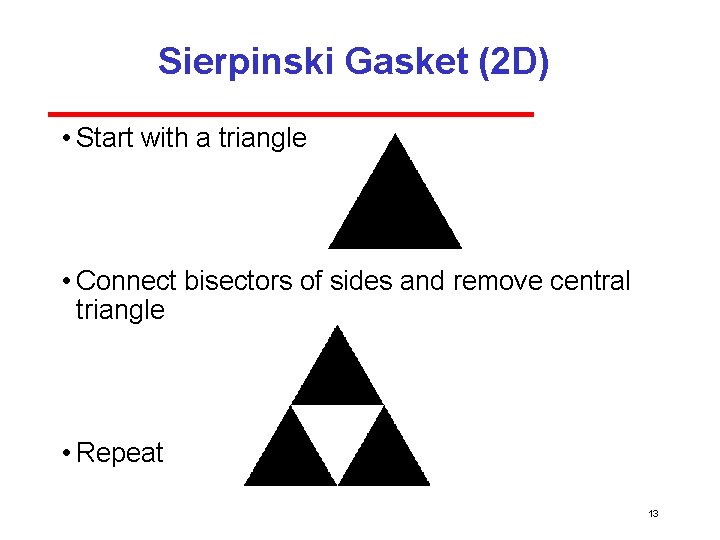
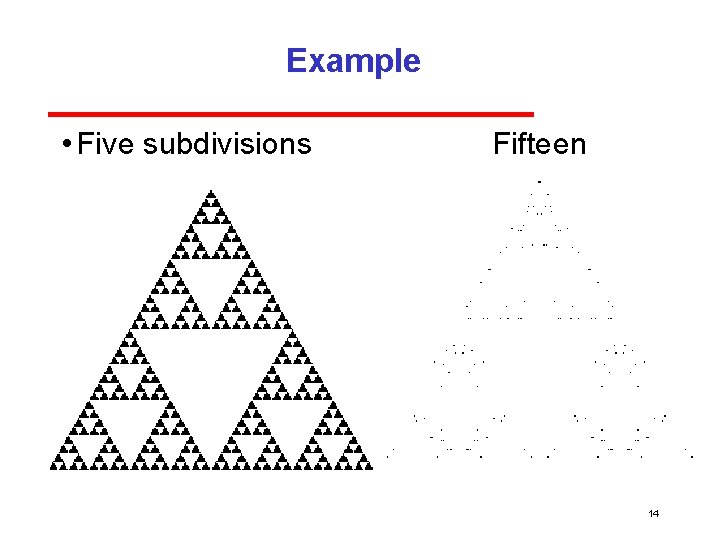
![Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58}, Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58},](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-15.jpg)
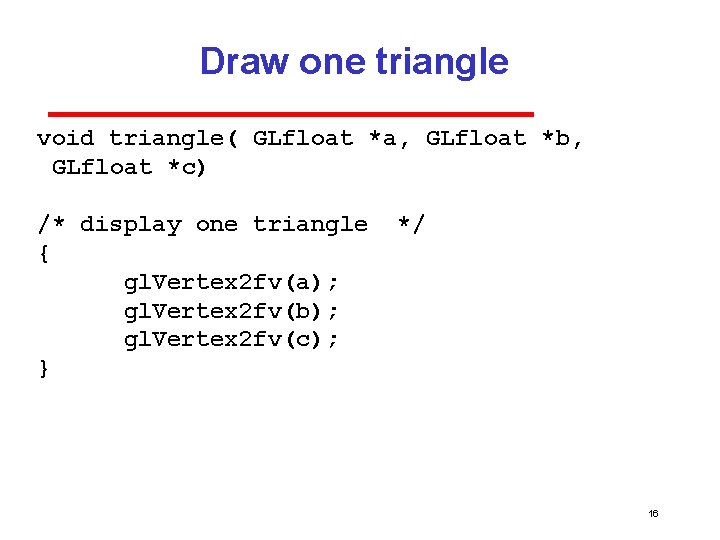
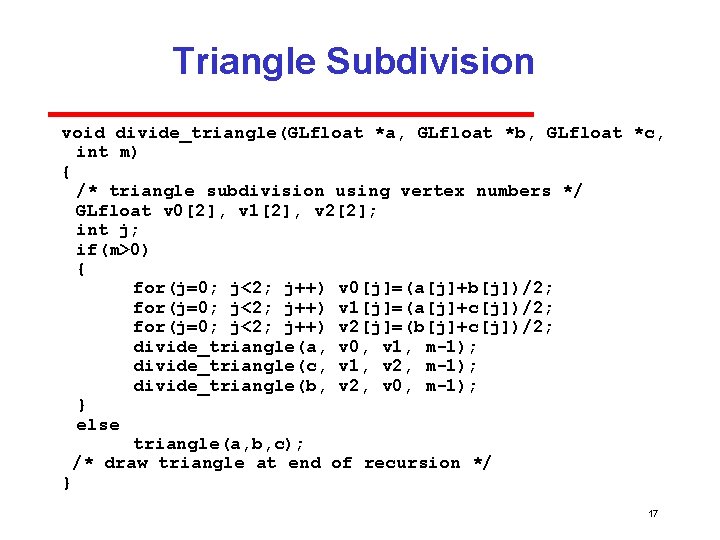
![display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2], display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2],](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-18.jpg)
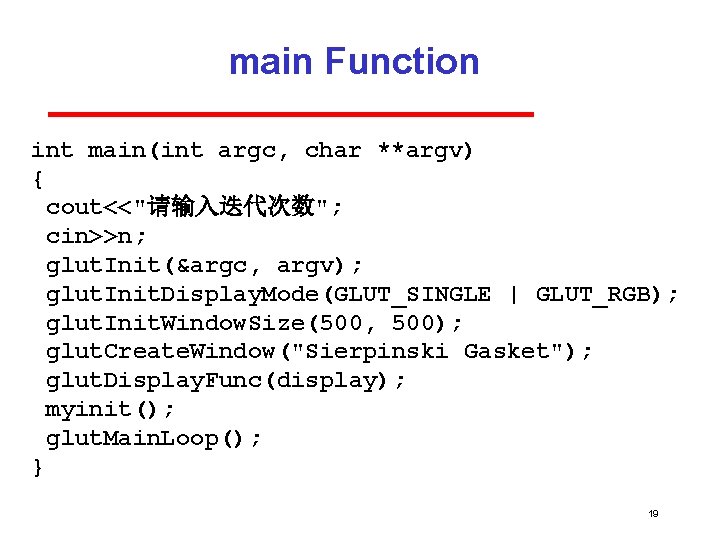
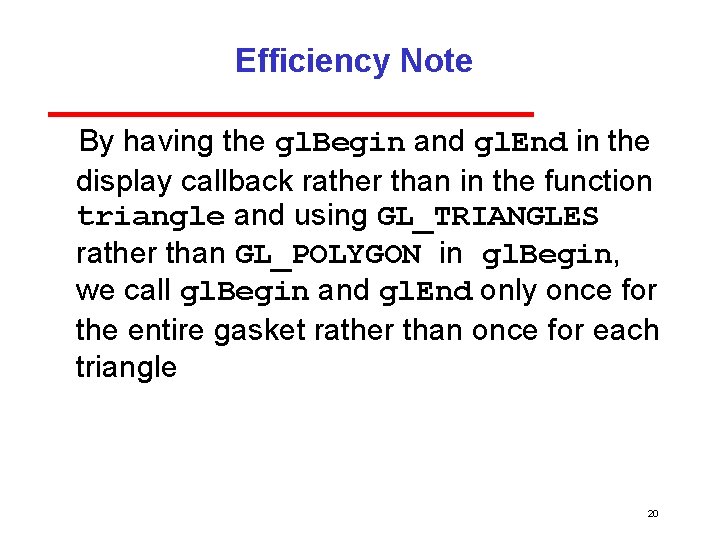
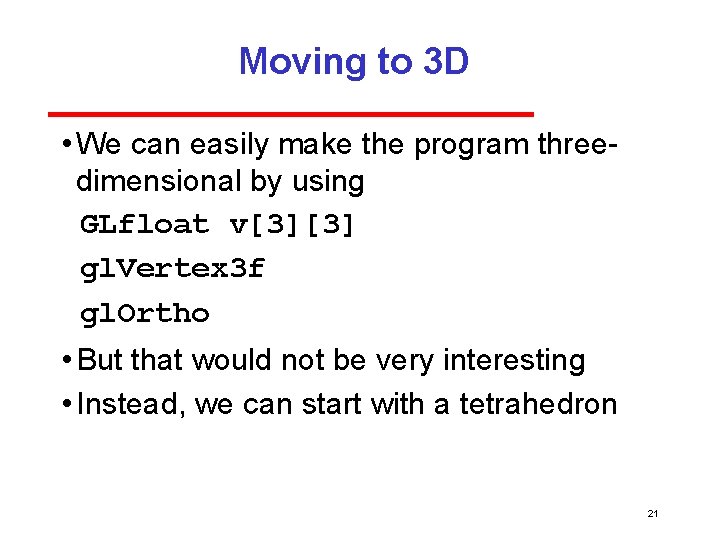
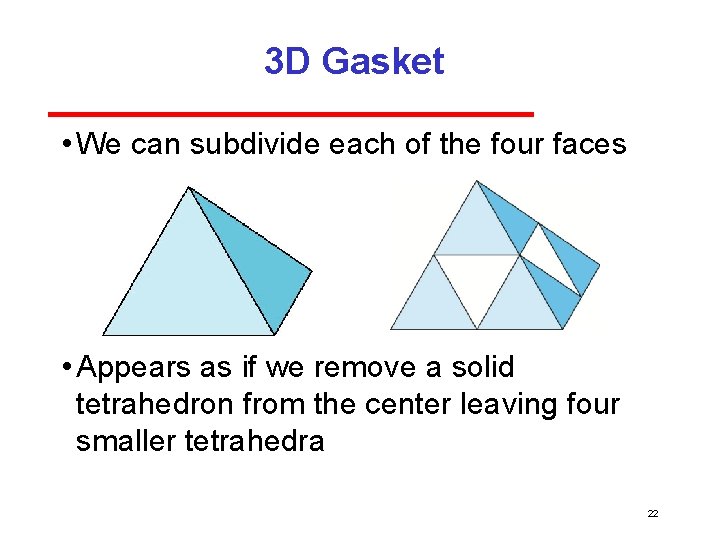
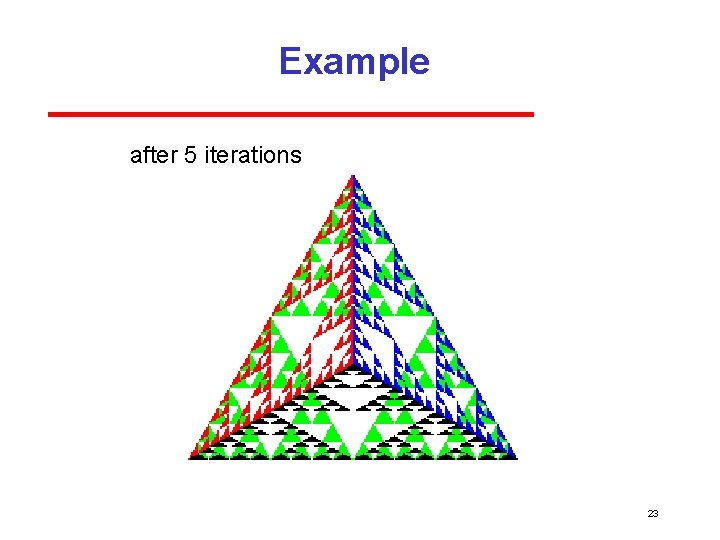
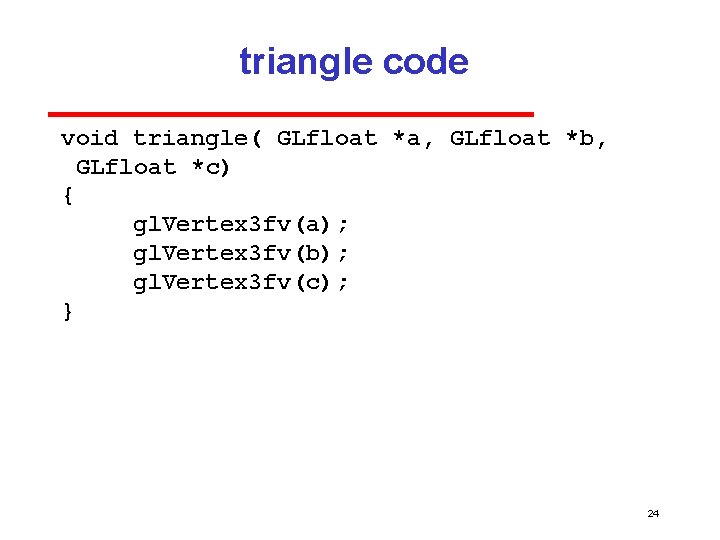
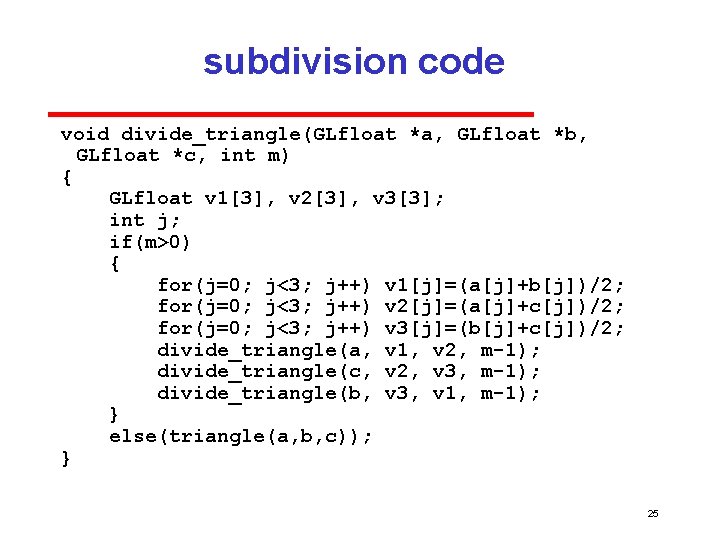
![tetrahedron code void tetrahedron(int m) { gl. Color 3 f(1. 0, 0. 0); divide_triangle(v[0], tetrahedron code void tetrahedron(int m) { gl. Color 3 f(1. 0, 0. 0); divide_triangle(v[0],](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-26.jpg)
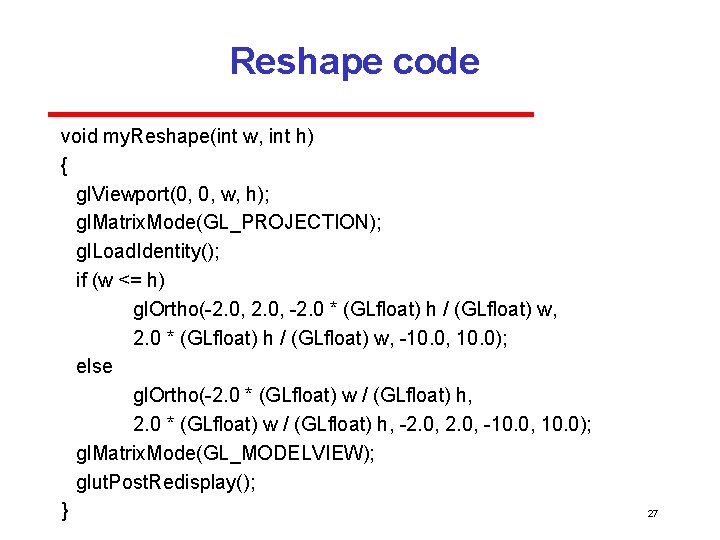
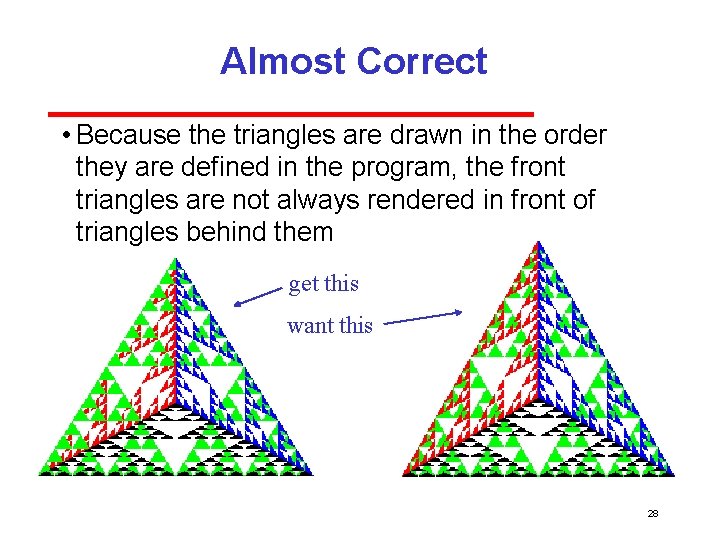
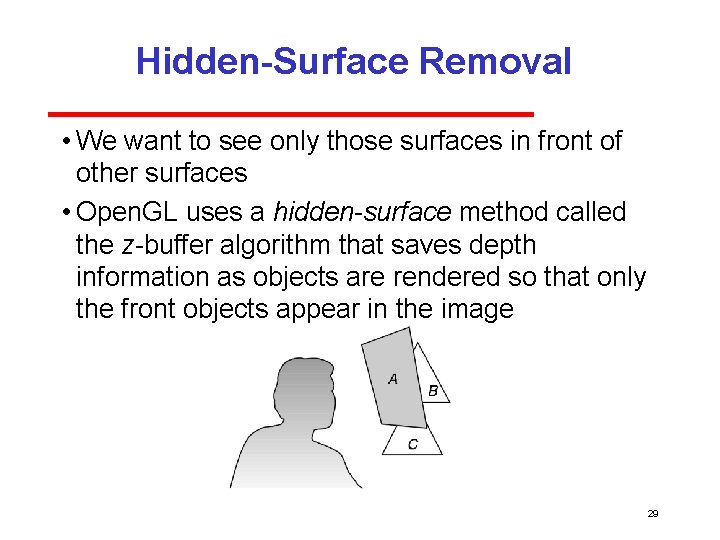
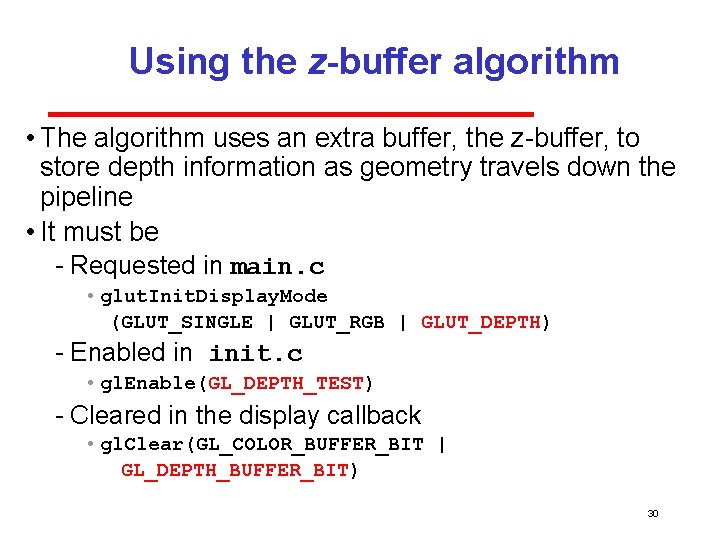
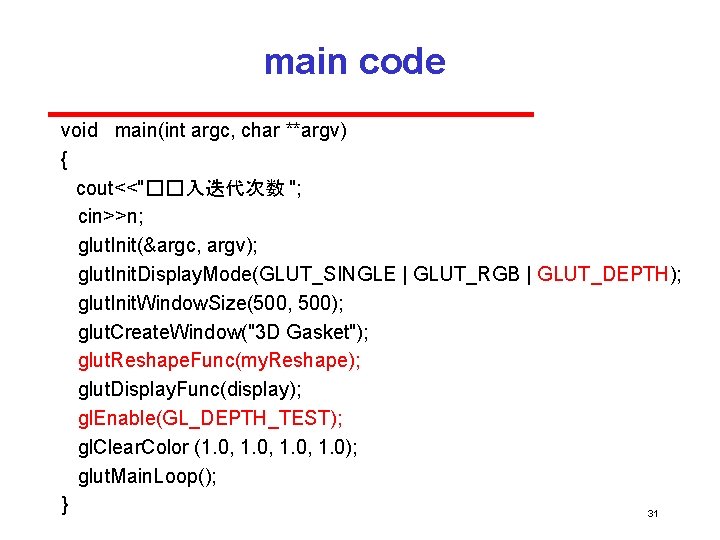
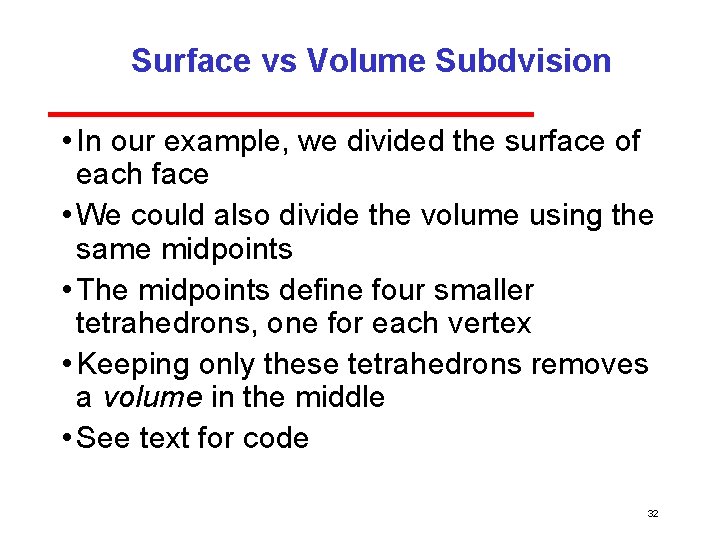
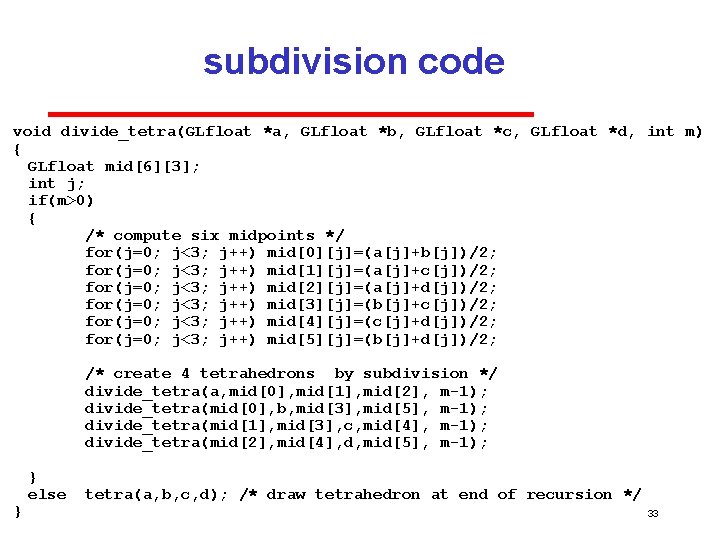
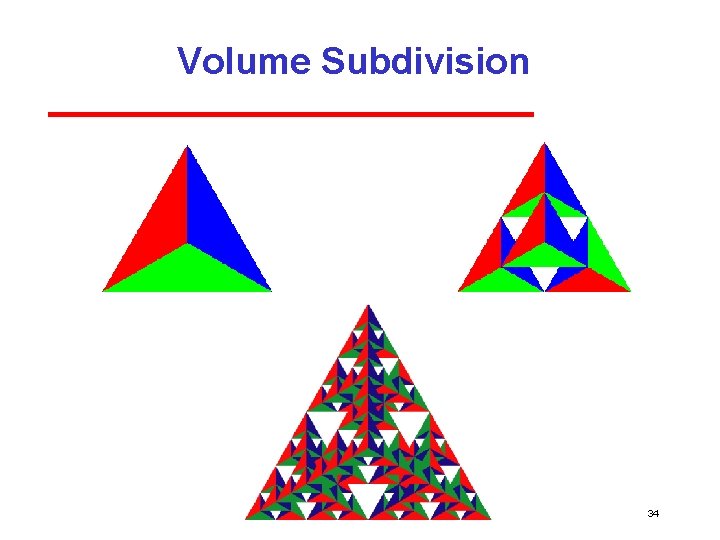
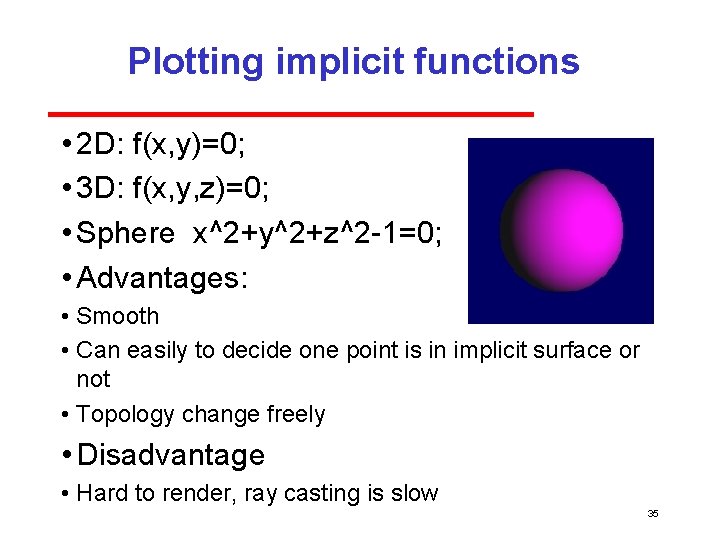
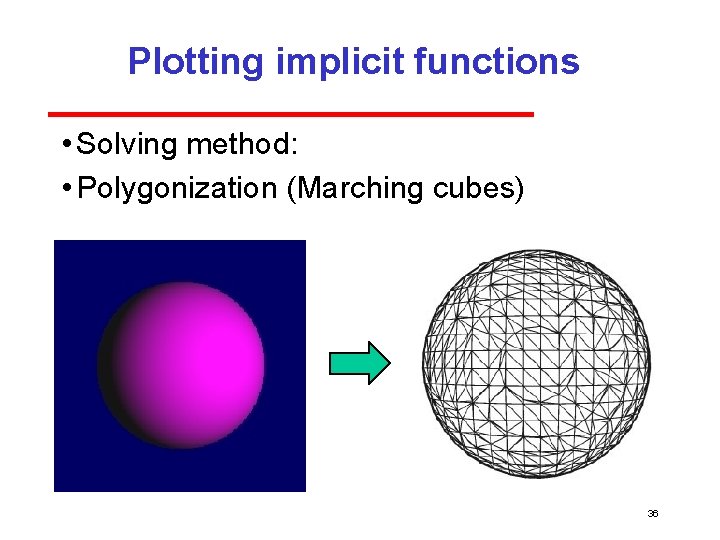
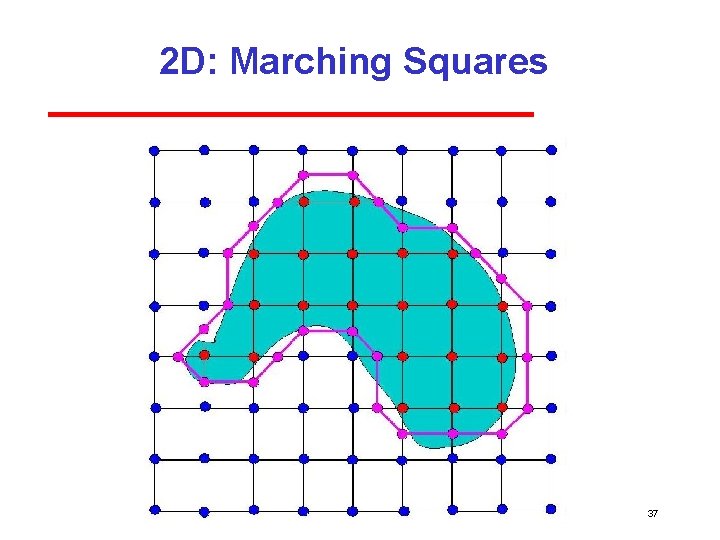
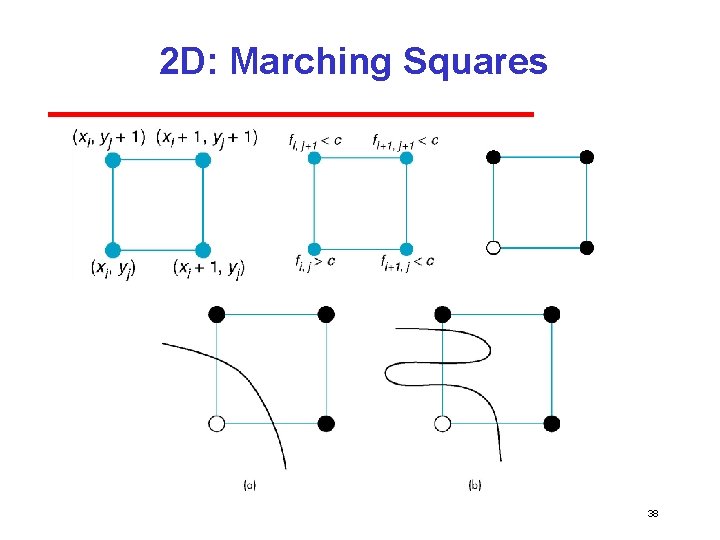
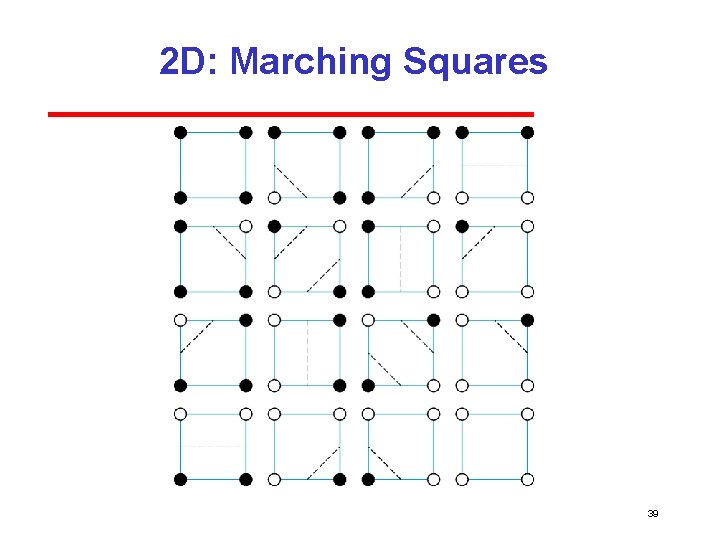
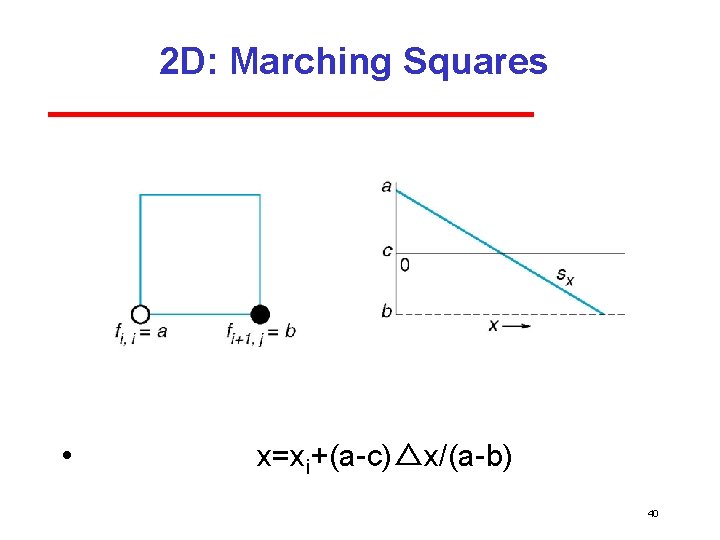
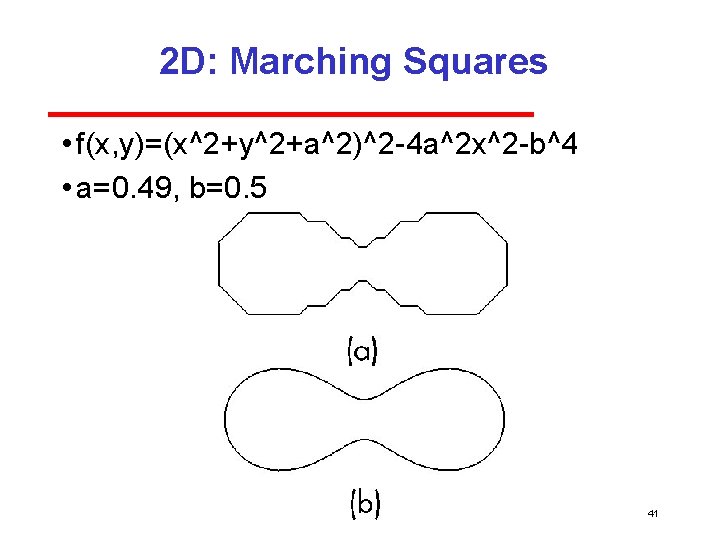
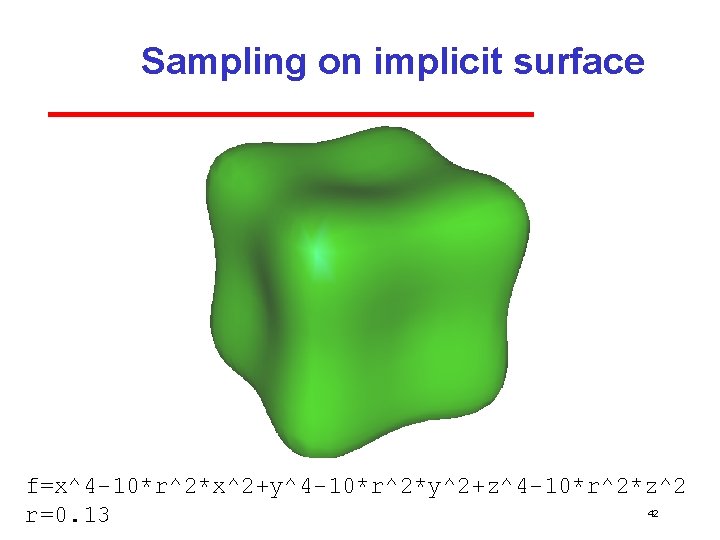
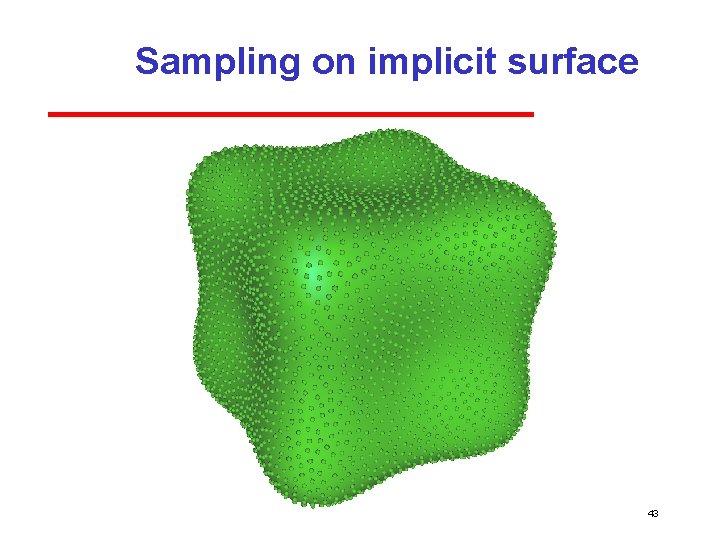
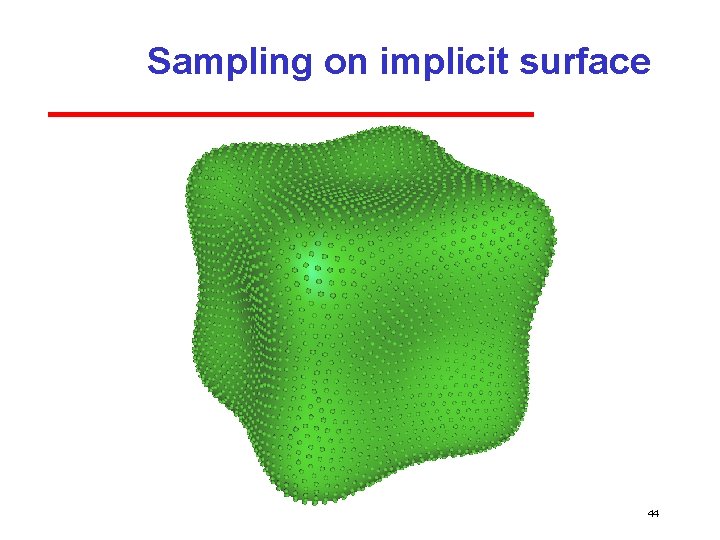
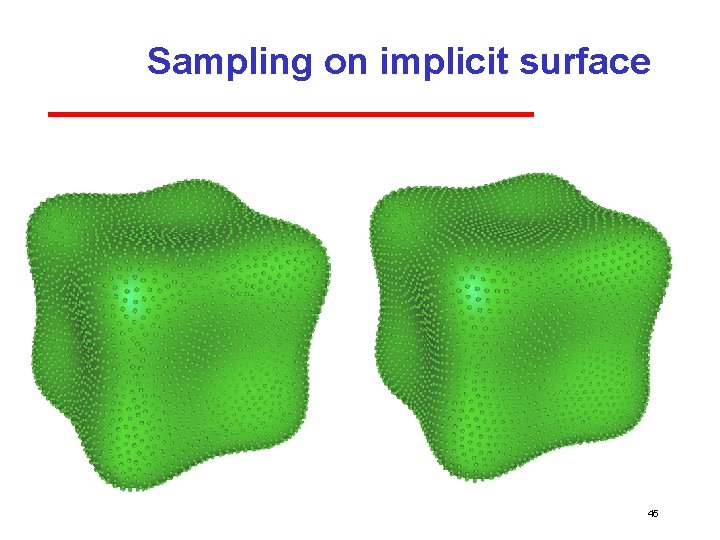
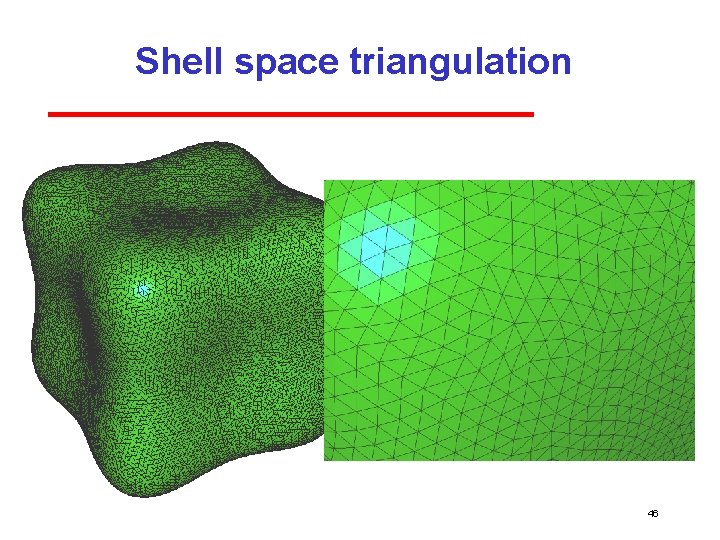
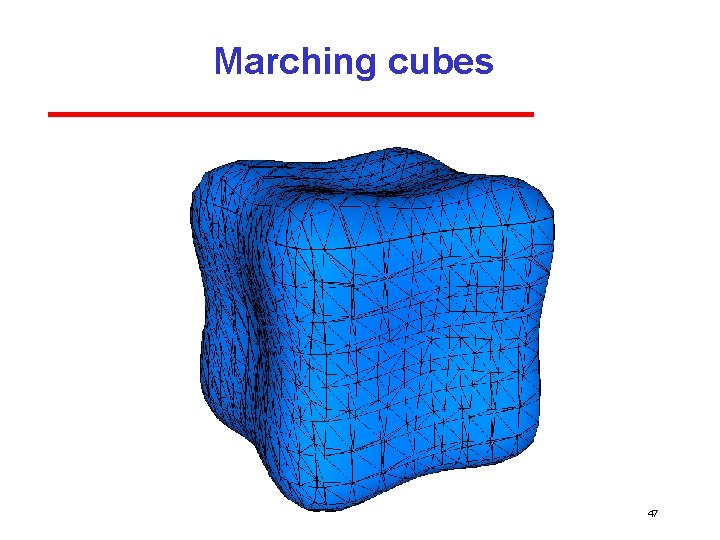
- Slides: 47
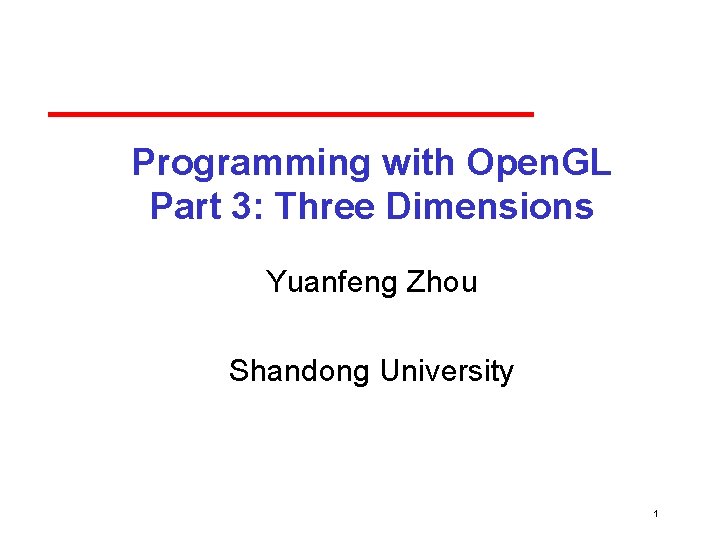
Programming with Open. GL Part 3: Three Dimensions Yuanfeng Zhou Shandong University 1
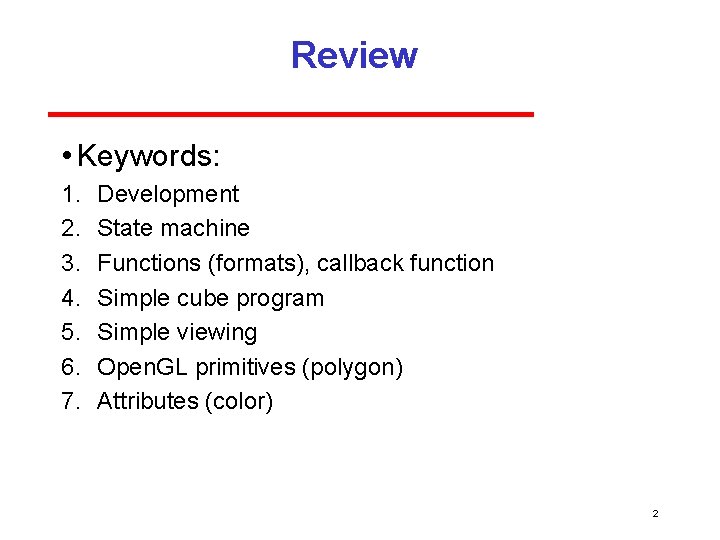
Review • Keywords: 1. 2. 3. 4. 5. 6. 7. Development State machine Functions (formats), callback function Simple cube program Simple viewing Open. GL primitives (polygon) Attributes (color) 2
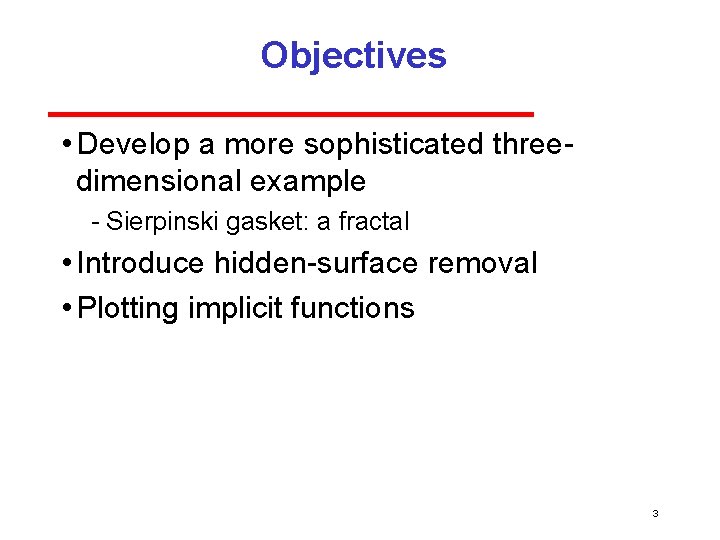
Objectives • Develop a more sophisticated three dimensional example Sierpinski gasket: a fractal • Introduce hidden surface removal • Plotting implicit functions 3
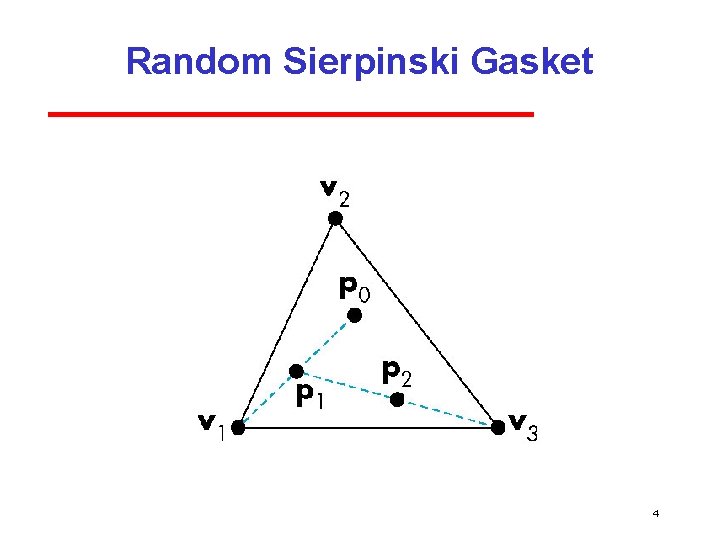
Random Sierpinski Gasket 4
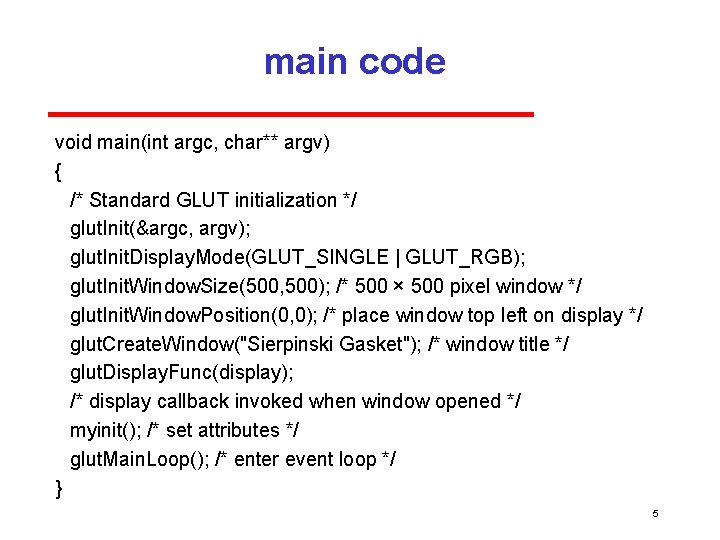
main code void main(int argc, char** argv) { /* Standard GLUT initialization */ glut. Init(&argc, argv); glut. Init. Display. Mode(GLUT_SINGLE | GLUT_RGB); glut. Init. Window. Size(500, 500); /* 500 × 500 pixel window */ glut. Init. Window. Position(0, 0); /* place window top left on display */ glut. Create. Window("Sierpinski Gasket"); /* window title */ glut. Display. Func(display); /* display callback invoked when window opened */ myinit(); /* set attributes */ glut. Main. Loop(); /* enter event loop */ } 5
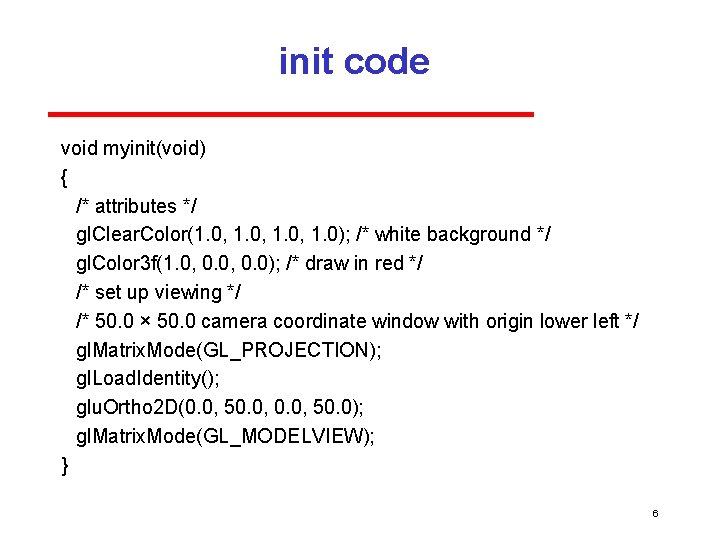
init code void myinit(void) { /* attributes */ gl. Clear. Color(1. 0, 1. 0); /* white background */ gl. Color 3 f(1. 0, 0. 0); /* draw in red */ /* set up viewing */ /* 50. 0 × 50. 0 camera coordinate window with origin lower left */ gl. Matrix. Mode(GL_PROJECTION); gl. Load. Identity(); glu. Ortho 2 D(0. 0, 50. 0); gl. Matrix. Mode(GL_MODELVIEW); } 6
![display code void displayvoid A triangle GLfloat vertices320 0 0 0 display code void display(void) { /* A triangle */ GLfloat vertices[3][2]={{0. 0, 0. 0},](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-7.jpg)
display code void display(void) { /* A triangle */ GLfloat vertices[3][2]={{0. 0, 0. 0}, {25. 0, 50. 0}, {50. 0, 0. 0}}; int i, j, k; GLfloat p[2] ={0, 0}; /* an arbitrary initial point inside traingle */ gl. Clear(GL_COLOR_BUFFER_BIT); /* clear the window */ gl. Begin(GL_POINTS); /* compute and plots 5000 new points */ for( k=0; k<10000; k++) { j=rand()%3; /* pick a vertex at random */ /* Compute point halfway between selected vertex and old point */ p[0] = (p[0]+vertices[j][0])/2. 0; p[1] = (p[1]+vertices[j][1])/2. 0; /* plot new point */ gl. Vertex 2 fv(p); } gl. End(); gl. Flush(); /* clear buffers */ } 7
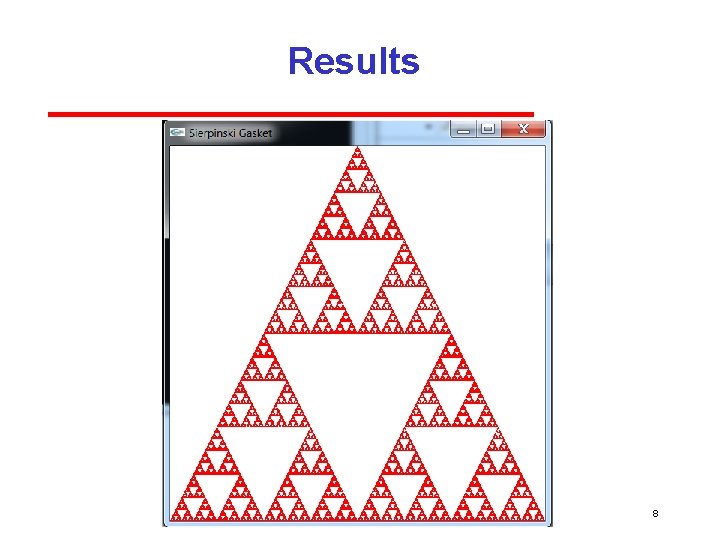
Results 8
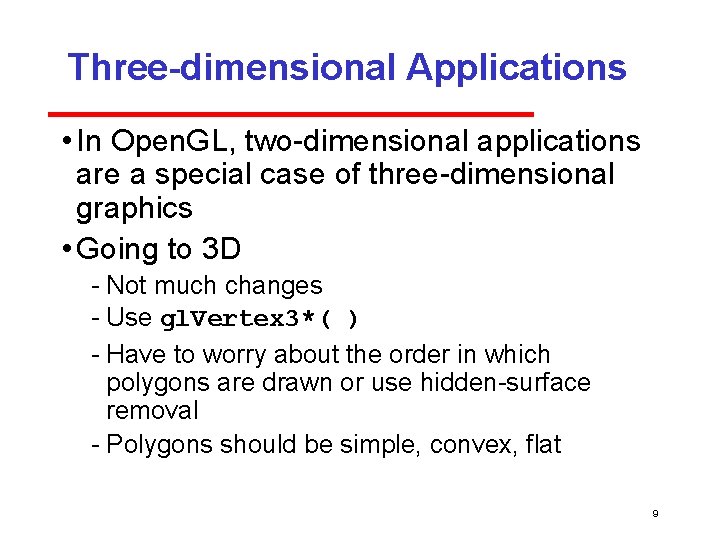
Three-dimensional Applications • In Open. GL, two dimensional applications are a special case of three dimensional graphics • Going to 3 D Not much changes Use gl. Vertex 3*( ) Have to worry about the order in which polygons are drawn or use hidden surface removal Polygons should be simple, convex, flat 9
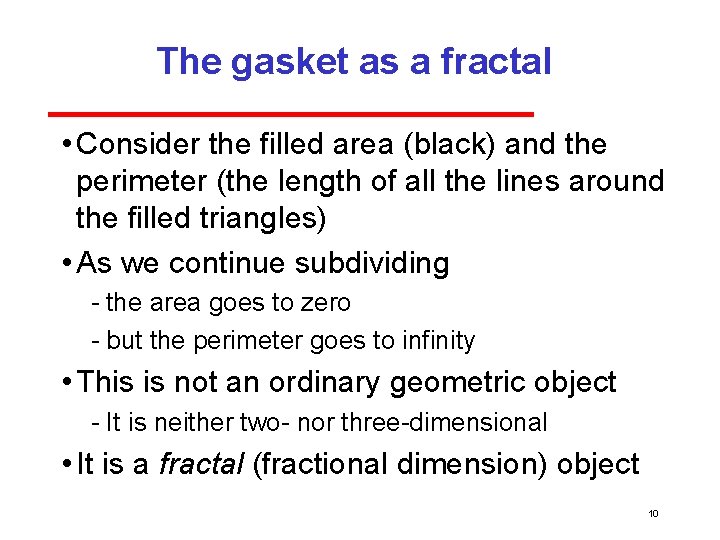
The gasket as a fractal • Consider the filled area (black) and the perimeter (the length of all the lines around the filled triangles) • As we continue subdividing the area goes to zero but the perimeter goes to infinity • This is not an ordinary geometric object It is neither two nor three dimensional • It is a fractal (fractional dimension) object 10
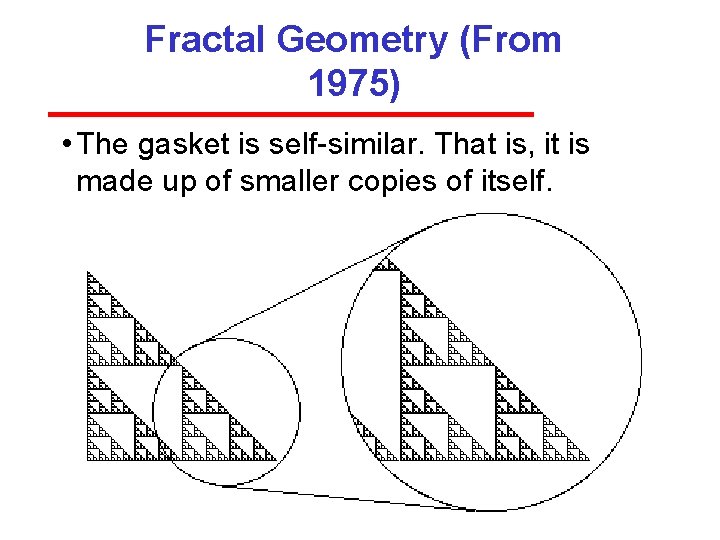
Fractal Geometry (From 1975) • The gasket is self similar. That is, it is made up of smaller copies of itself. 11
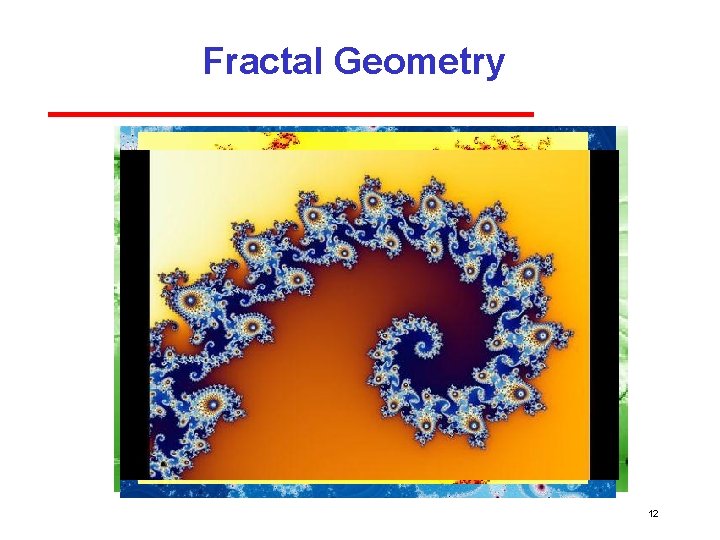
Fractal Geometry 12
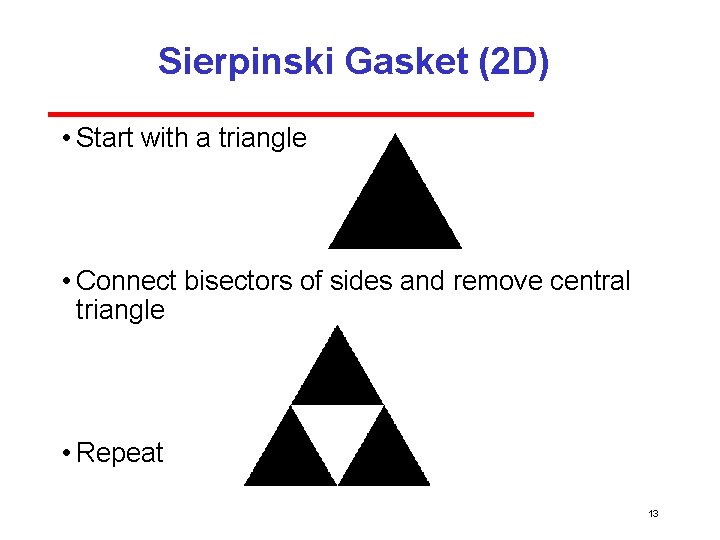
Sierpinski Gasket (2 D) • Start with a triangle • Connect bisectors of sides and remove central triangle • Repeat 13
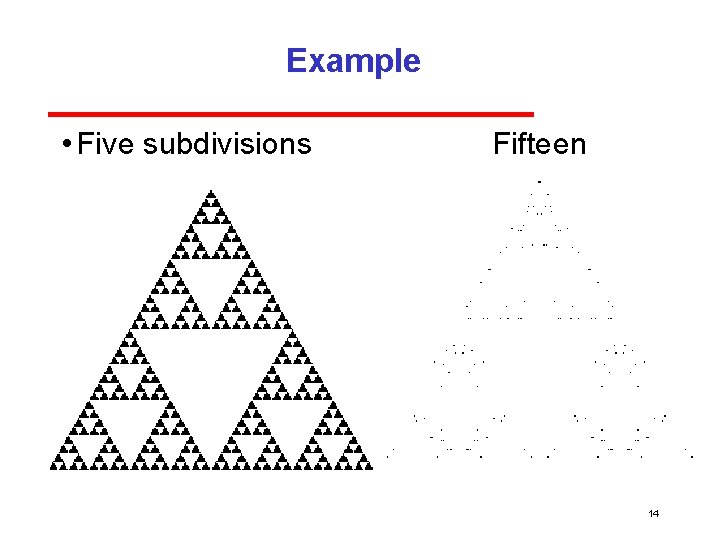
Example • Five subdivisions Fifteen 14
![Gasket Program include GLglut h initial triangle GLfloat v321 0 0 58 Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58},](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-15.jpg)
Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58}, {0. 0, 1. 15}}; int n; /* number of recursive steps */ 15
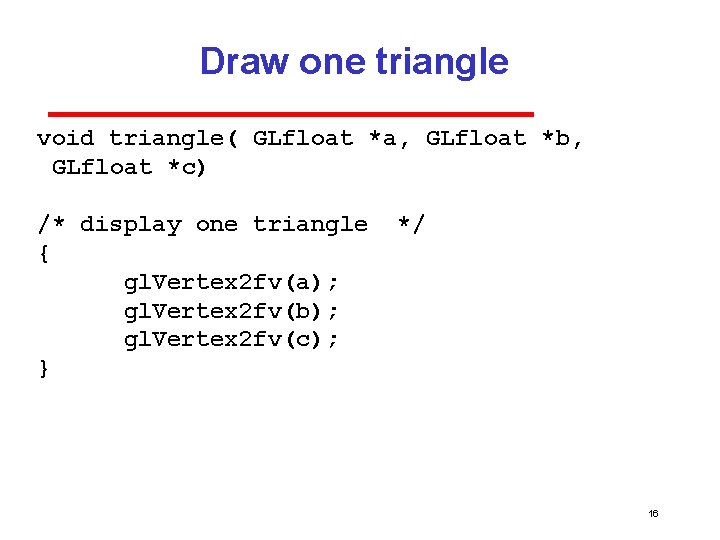
Draw one triangle void triangle( GLfloat *a, GLfloat *b, GLfloat *c) /* display one triangle { gl. Vertex 2 fv(a); gl. Vertex 2 fv(b); gl. Vertex 2 fv(c); } */ 16
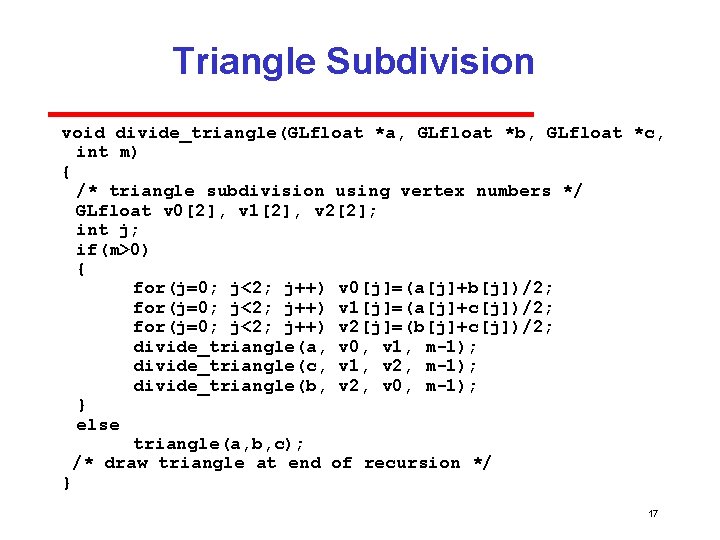
Triangle Subdivision void divide_triangle(GLfloat *a, GLfloat *b, GLfloat *c, int m) { /* triangle subdivision using vertex numbers */ GLfloat v 0[2], v 1[2], v 2[2]; int j; if(m>0) { for(j=0; j<2; j++) v 0[j]=(a[j]+b[j])/2; for(j=0; j<2; j++) v 1[j]=(a[j]+c[j])/2; for(j=0; j<2; j++) v 2[j]=(b[j]+c[j])/2; divide_triangle(a, v 0, v 1, m-1); divide_triangle(c, v 1, v 2, m-1); divide_triangle(b, v 2, v 0, m-1); } else triangle(a, b, c); /* draw triangle at end of recursion */ } 17
![display and init Functions void display gl ClearGLCOLORBUFFERBIT gl BeginGLTRIANGLES dividetrianglev0 v1 v2 display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2],](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-18.jpg)
display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2], n); gl. End(); gl. Flush(); } void myinit() { gl. Matrix. Mode(GL_PROJECTION); gl. Load. Identity(); glu. Ortho 2 D(-2. 0, 2. 0); gl. Matrix. Mode(GL_MODELVIEW); gl. Clear. Color (1. 0, 1. 0) gl. Color 3 f(0. 0, 0. 0); } 18
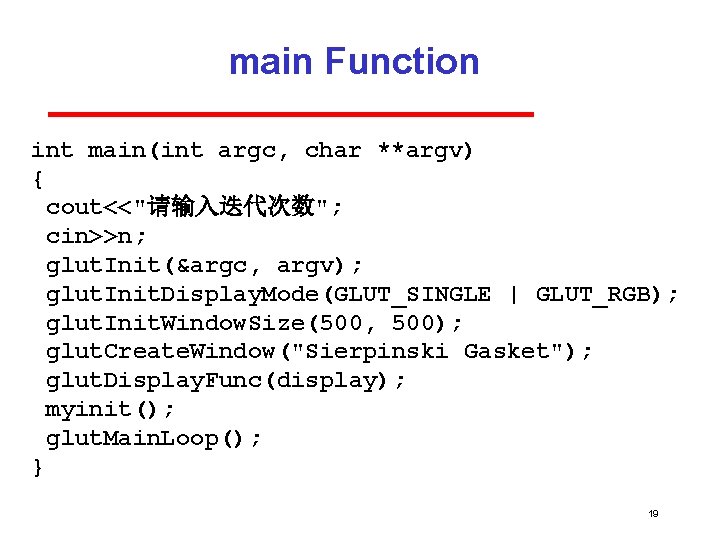
main Function int main(int argc, char **argv) { cout<<"请输入迭代次数"; cin>>n; glut. Init(&argc, argv); glut. Init. Display. Mode(GLUT_SINGLE | GLUT_RGB); glut. Init. Window. Size(500, 500); glut. Create. Window("Sierpinski Gasket"); glut. Display. Func(display); myinit(); glut. Main. Loop(); } 19
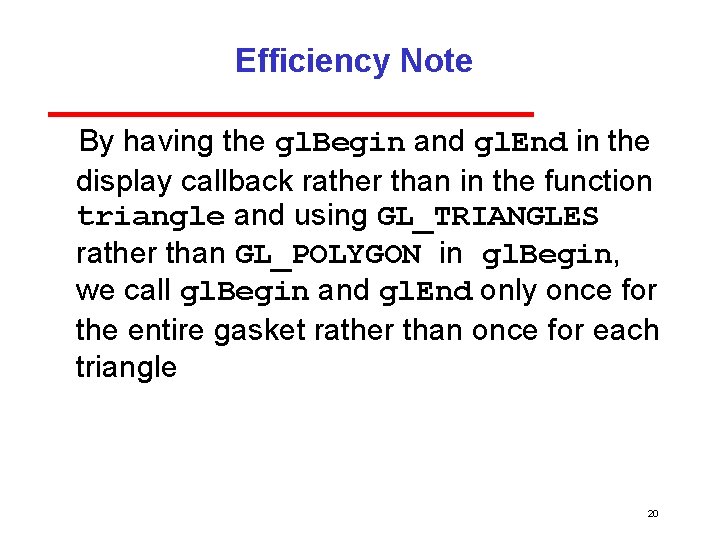
Efficiency Note By having the gl. Begin and gl. End in the display callback rather than in the function triangle and using GL_TRIANGLES rather than GL_POLYGON in gl. Begin, we call gl. Begin and gl. End only once for the entire gasket rather than once for each triangle 20
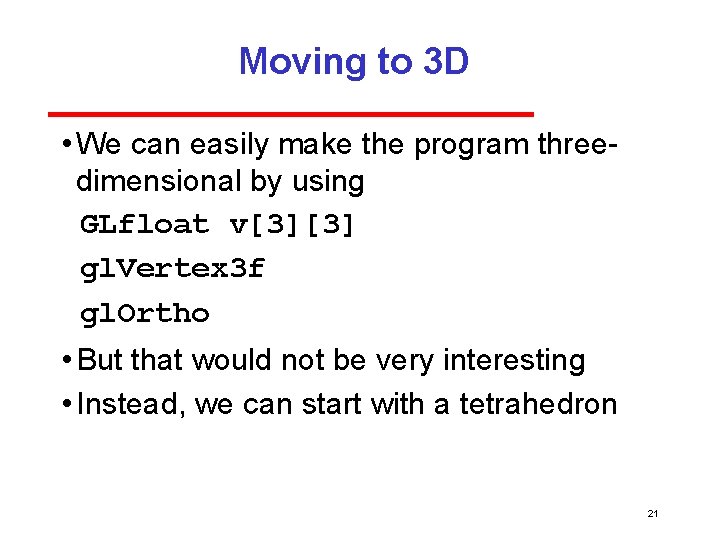
Moving to 3 D • We can easily make the program three dimensional by using GLfloat v[3][3] gl. Vertex 3 f gl. Ortho • But that would not be very interesting • Instead, we can start with a tetrahedron 21
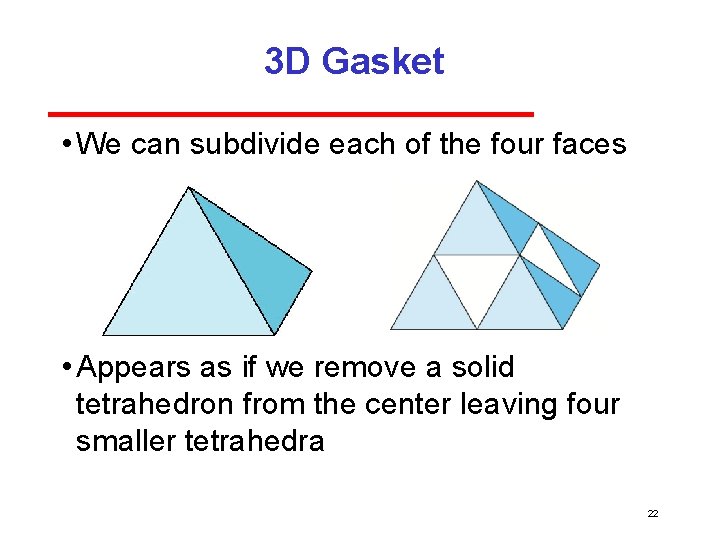
3 D Gasket • We can subdivide each of the four faces • Appears as if we remove a solid tetrahedron from the center leaving four smaller tetrahedra 22
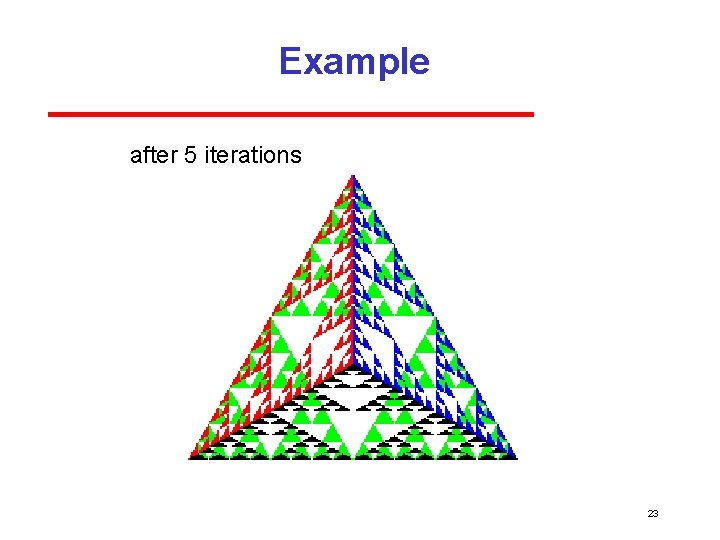
Example after 5 iterations 23
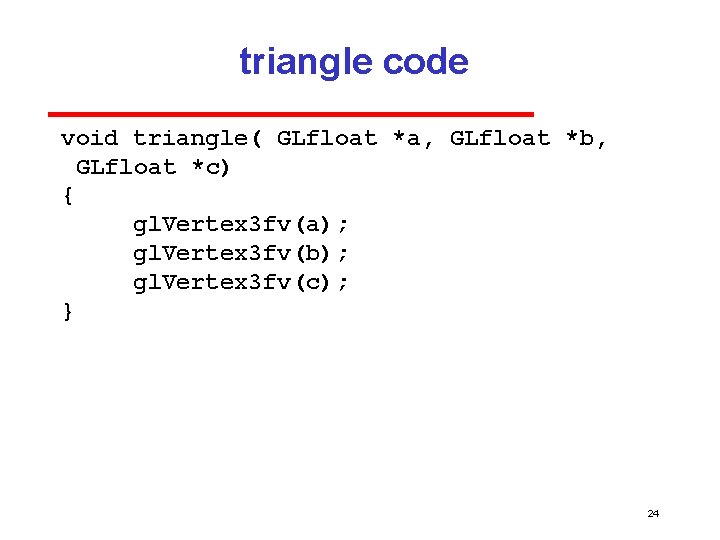
triangle code void triangle( GLfloat *a, GLfloat *b, GLfloat *c) { gl. Vertex 3 fv(a); gl. Vertex 3 fv(b); gl. Vertex 3 fv(c); } 24
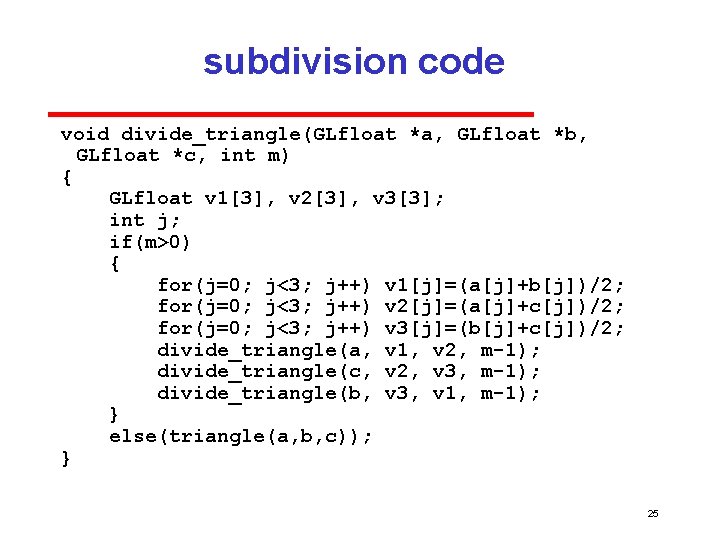
subdivision code void divide_triangle(GLfloat *a, GLfloat *b, GLfloat *c, int m) { GLfloat v 1[3], v 2[3], v 3[3]; int j; if(m>0) { for(j=0; j<3; j++) v 1[j]=(a[j]+b[j])/2; for(j=0; j<3; j++) v 2[j]=(a[j]+c[j])/2; for(j=0; j<3; j++) v 3[j]=(b[j]+c[j])/2; divide_triangle(a, v 1, v 2, m-1); divide_triangle(c, v 2, v 3, m-1); divide_triangle(b, v 3, v 1, m-1); } else(triangle(a, b, c)); } 25
![tetrahedron code void tetrahedronint m gl Color 3 f1 0 0 0 dividetrianglev0 tetrahedron code void tetrahedron(int m) { gl. Color 3 f(1. 0, 0. 0); divide_triangle(v[0],](https://slidetodoc.com/presentation_image_h/c1b0e622a7233767f4eda38827c63f79/image-26.jpg)
tetrahedron code void tetrahedron(int m) { gl. Color 3 f(1. 0, 0. 0); divide_triangle(v[0], v[1], gl. Color 3 f(0. 0, 1. 0, 0. 0); divide_triangle(v[3], v[2], gl. Color 3 f(0. 0, 1. 0); divide_triangle(v[0], v[3], gl. Color 3 f(0. 0, 0. 0); divide_triangle(v[0], v[2], } v[2], m); v[1], m); v[3], m); 26
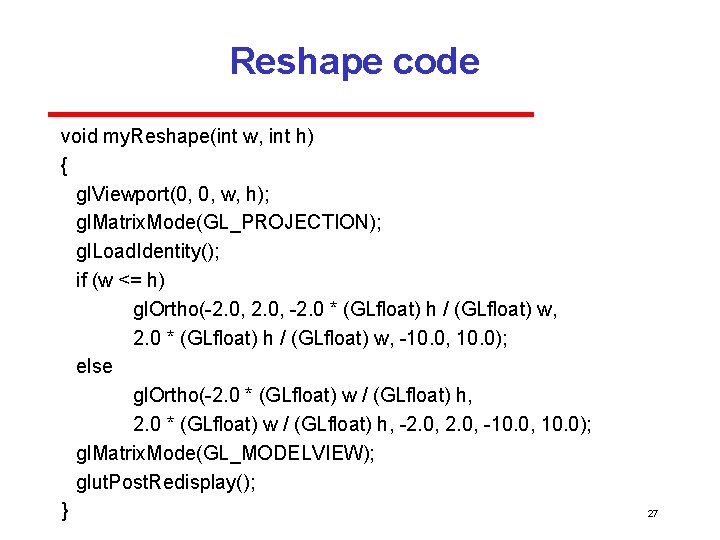
Reshape code void my. Reshape(int w, int h) { gl. Viewport(0, 0, w, h); gl. Matrix. Mode(GL_PROJECTION); gl. Load. Identity(); if (w <= h) gl. Ortho( 2. 0, 2. 0 * (GLfloat) h / (GLfloat) w, 10. 0, 10. 0); else gl. Ortho( 2. 0 * (GLfloat) w / (GLfloat) h, 2. 0, 10. 0, 10. 0); gl. Matrix. Mode(GL_MODELVIEW); glut. Post. Redisplay(); } 27
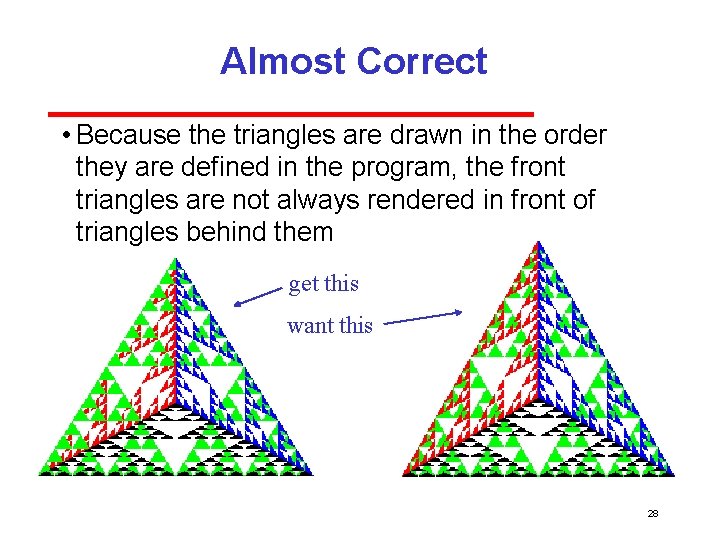
Almost Correct • Because the triangles are drawn in the order they are defined in the program, the front triangles are not always rendered in front of triangles behind them get this want this 28
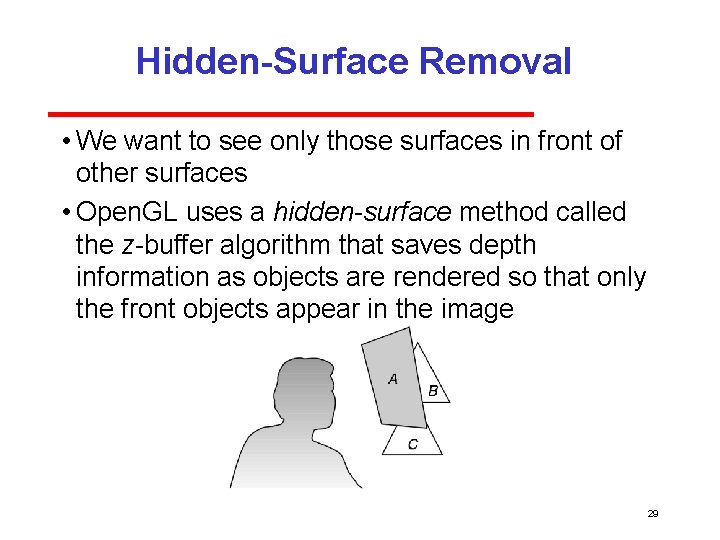
Hidden-Surface Removal • We want to see only those surfaces in front of other surfaces • Open. GL uses a hidden-surface method called the z buffer algorithm that saves depth information as objects are rendered so that only the front objects appear in the image 29
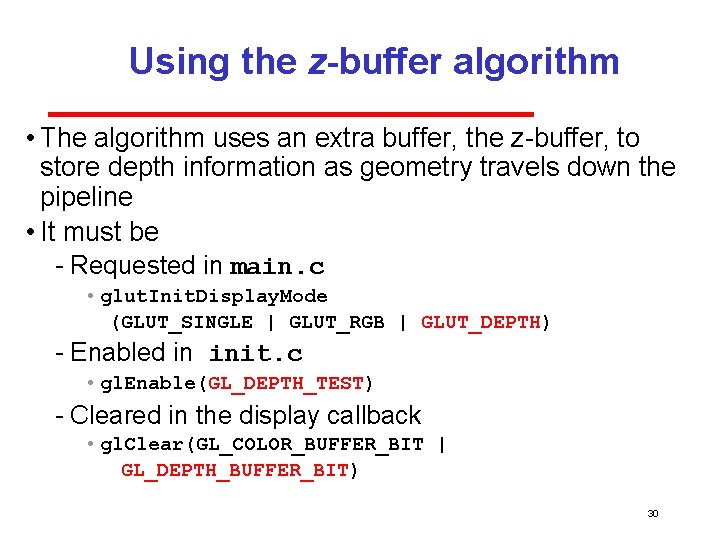
Using the z-buffer algorithm • The algorithm uses an extra buffer, the z buffer, to store depth information as geometry travels down the pipeline • It must be Requested in main. c • glut. Init. Display. Mode (GLUT_SINGLE | GLUT_RGB | GLUT_DEPTH) Enabled in init. c • gl. Enable(GL_DEPTH_TEST) Cleared in the display callback • gl. Clear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) 30
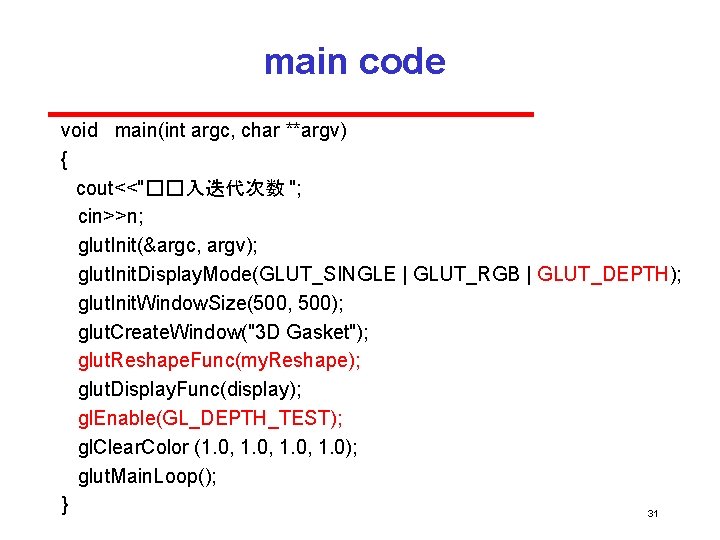
main code void main(int argc, char **argv) { cout<<"��入迭代次数 "; cin>>n; glut. Init(&argc, argv); glut. Init. Display. Mode(GLUT_SINGLE | GLUT_RGB | GLUT_DEPTH); glut. Init. Window. Size(500, 500); glut. Create. Window("3 D Gasket"); glut. Reshape. Func(my. Reshape); glut. Display. Func(display); gl. Enable(GL_DEPTH_TEST); gl. Clear. Color (1. 0, 1. 0); glut. Main. Loop(); } 31
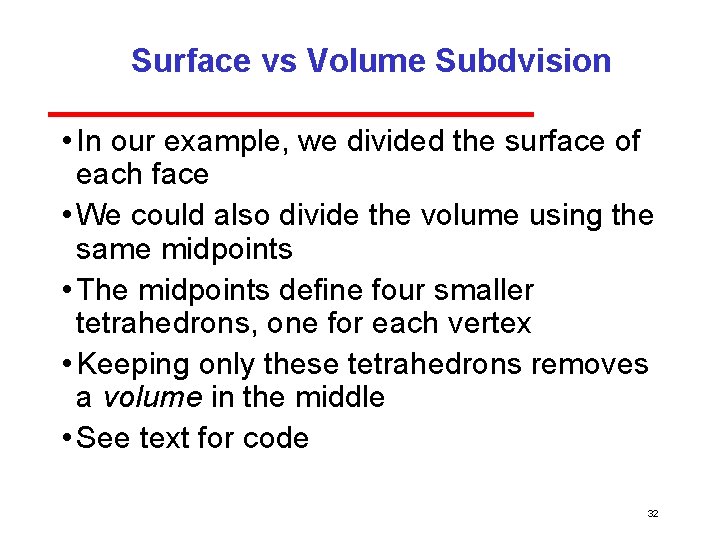
Surface vs Volume Subdvision • In our example, we divided the surface of each face • We could also divide the volume using the same midpoints • The midpoints define four smaller tetrahedrons, one for each vertex • Keeping only these tetrahedrons removes a volume in the middle • See text for code 32
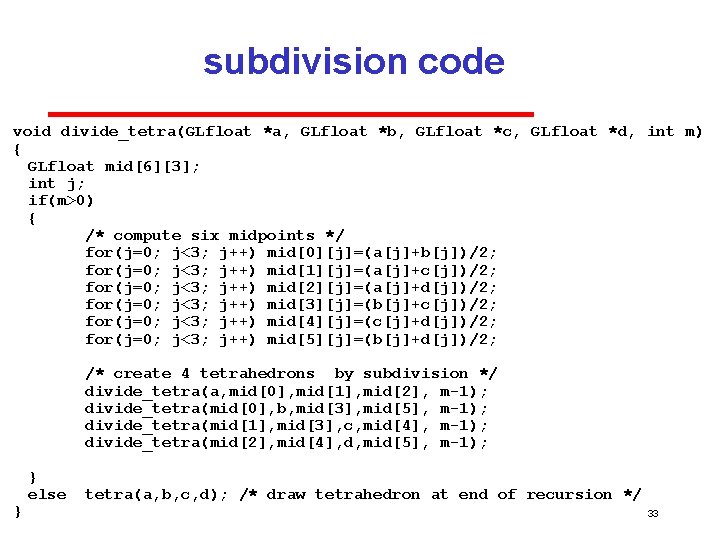
subdivision code void divide_tetra(GLfloat *a, GLfloat *b, GLfloat *c, GLfloat *d, int m) { GLfloat mid[6][3]; int j; if(m>0) { /* compute six midpoints */ for(j=0; j<3; j++) mid[0][j]=(a[j]+b[j])/2; for(j=0; j<3; j++) mid[1][j]=(a[j]+c[j])/2; for(j=0; j<3; j++) mid[2][j]=(a[j]+d[j])/2; for(j=0; j<3; j++) mid[3][j]=(b[j]+c[j])/2; for(j=0; j<3; j++) mid[4][j]=(c[j]+d[j])/2; for(j=0; j<3; j++) mid[5][j]=(b[j]+d[j])/2; /* create 4 tetrahedrons by subdivision */ divide_tetra(a, mid[0], mid[1], mid[2], m-1); divide_tetra(mid[0], b, mid[3], mid[5], m-1); divide_tetra(mid[1], mid[3], c, mid[4], m-1); divide_tetra(mid[2], mid[4], d, mid[5], m-1); } } else tetra(a, b, c, d); /* draw tetrahedron at end of recursion */ 33
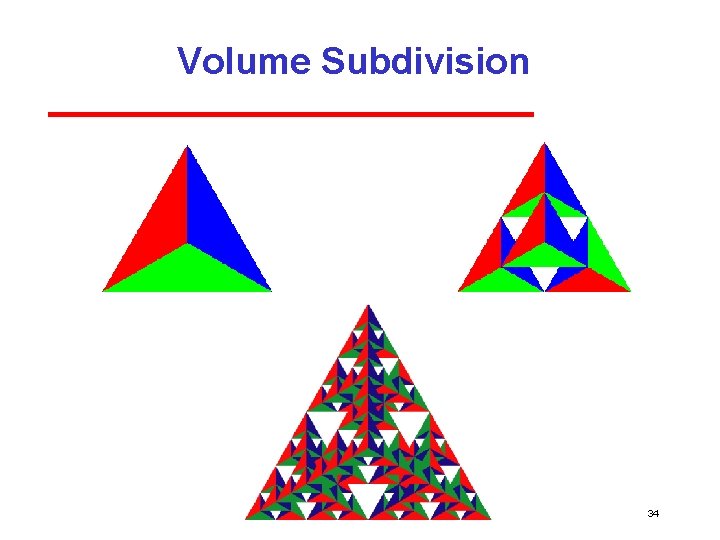
Volume Subdivision 34
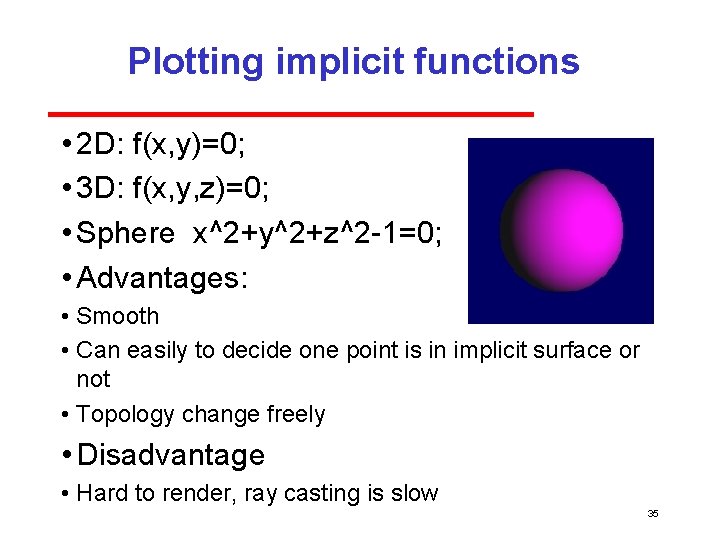
Plotting implicit functions • 2 D: f(x, y)=0; • 3 D: f(x, y, z)=0; • Sphere x^2+y^2+z^2 1=0; • Advantages: • Smooth • Can easily to decide one point is in implicit surface or not • Topology change freely • Disadvantage • Hard to render, ray casting is slow 35
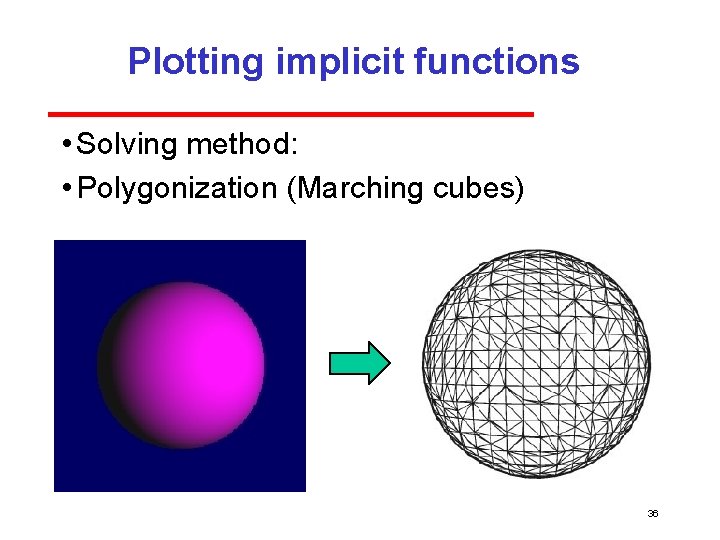
Plotting implicit functions • Solving method: • Polygonization (Marching cubes) 36
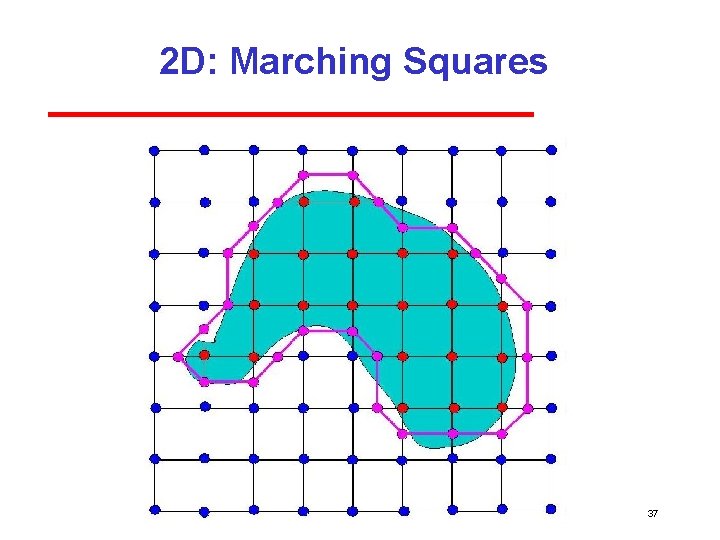
2 D: Marching Squares 37
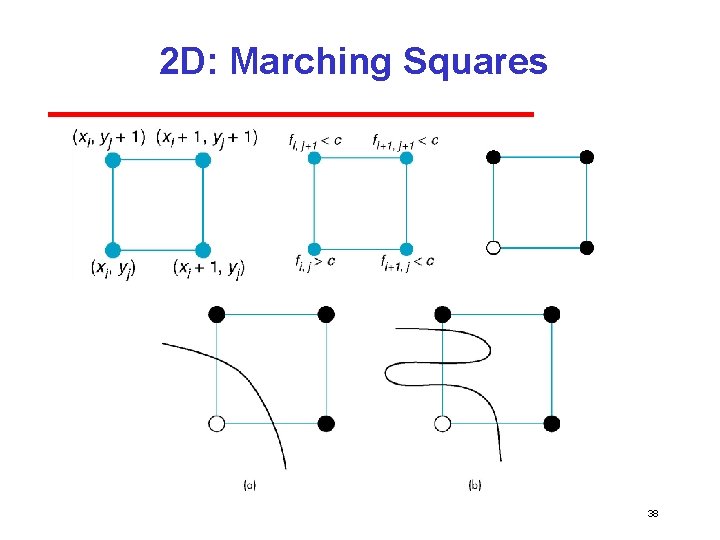
2 D: Marching Squares 38
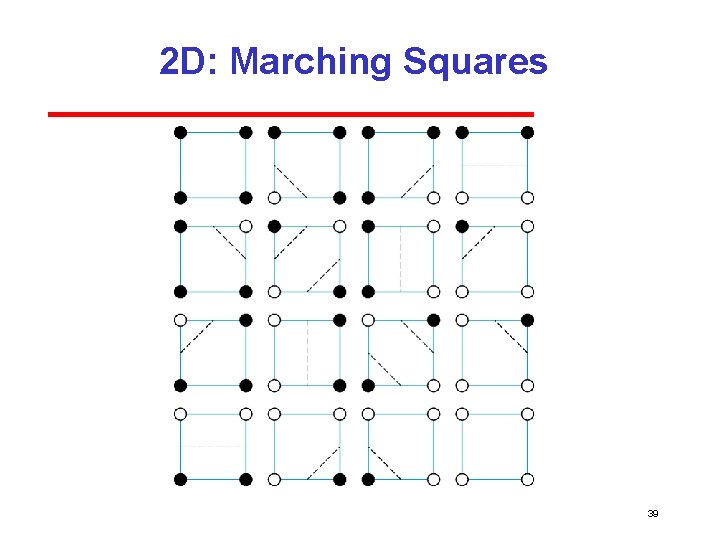
2 D: Marching Squares 39
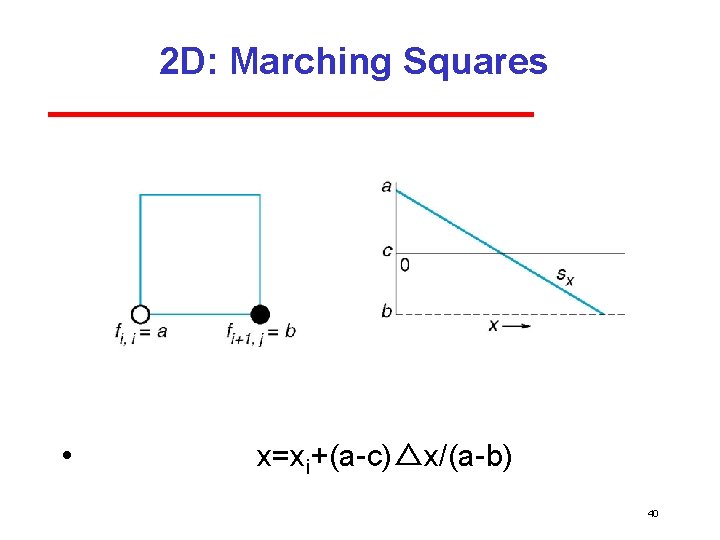
2 D: Marching Squares • x=xi+(a c)△x/(a b) 40
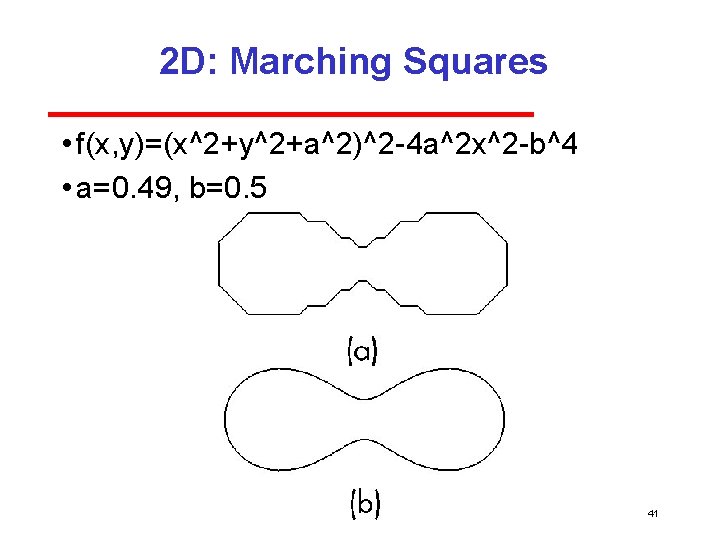
2 D: Marching Squares • f(x, y)=(x^2+y^2+a^2)^2 4 a^2 x^2 b^4 • a=0. 49, b=0. 5 41
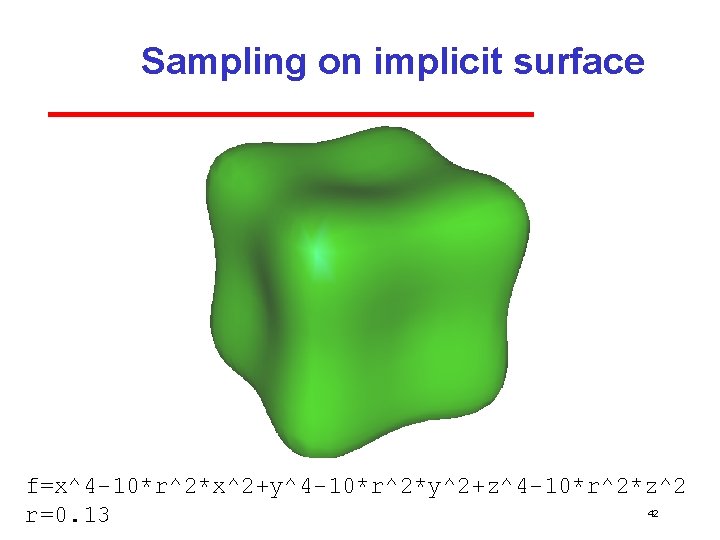
Sampling on implicit surface f=x^4 -10*r^2*x^2+y^4 -10*r^2*y^2+z^4 -10*r^2*z^2 42 r=0. 13
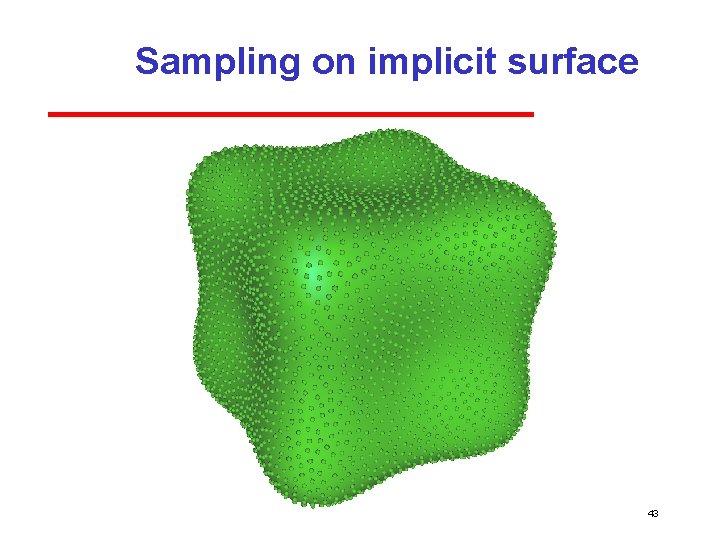
Sampling on implicit surface 43
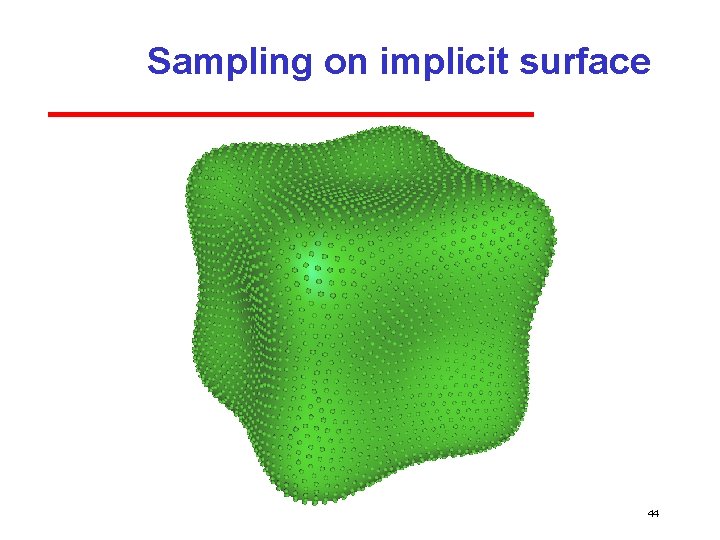
Sampling on implicit surface 44
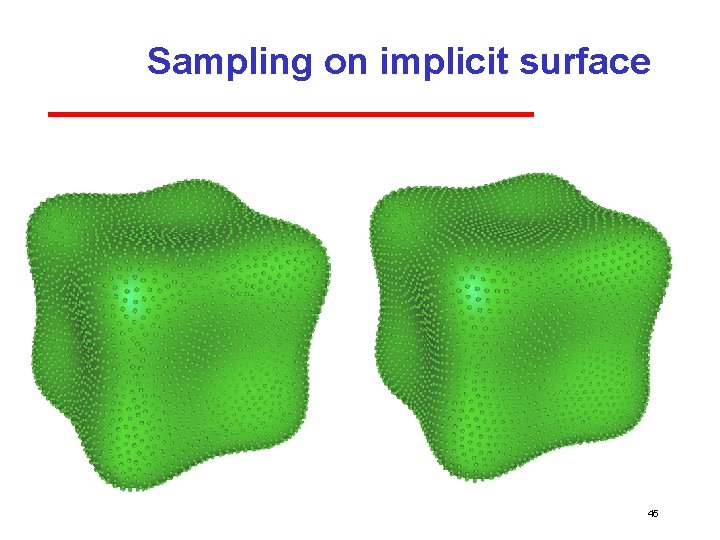
Sampling on implicit surface 45
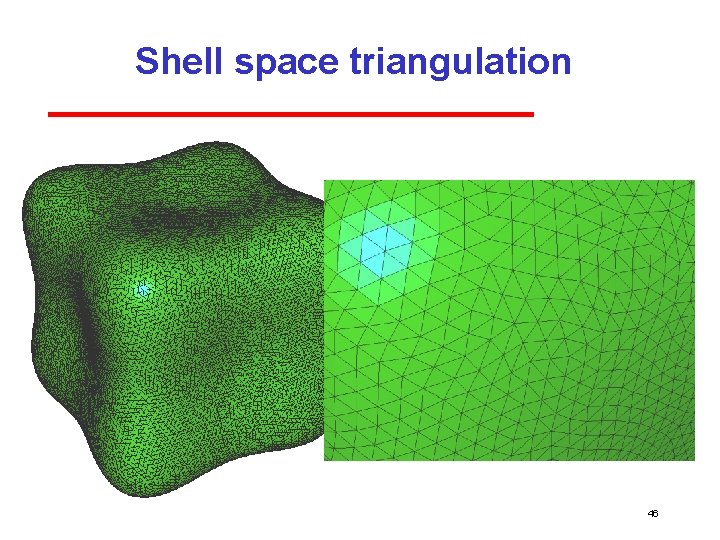
Shell space triangulation 46
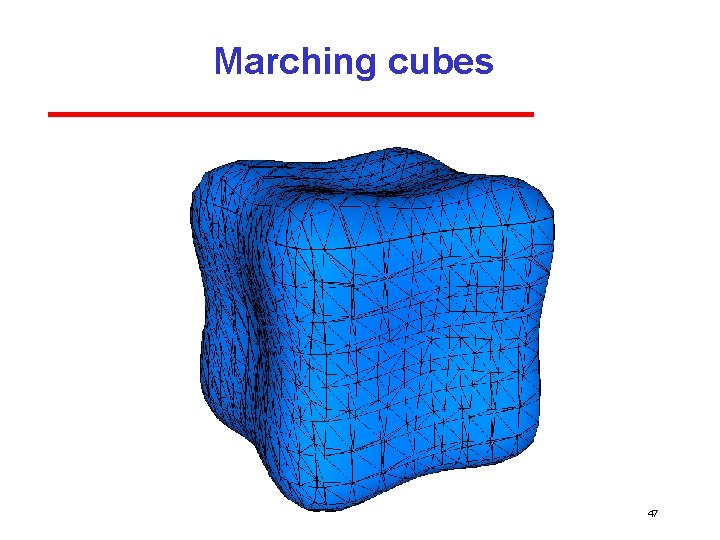
Marching cubes 47