Programming with Open GL Ed Angel Professor Emeritus
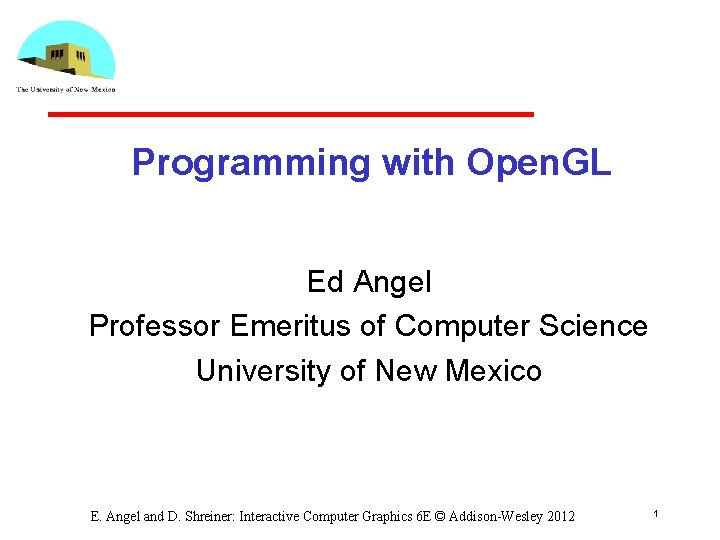
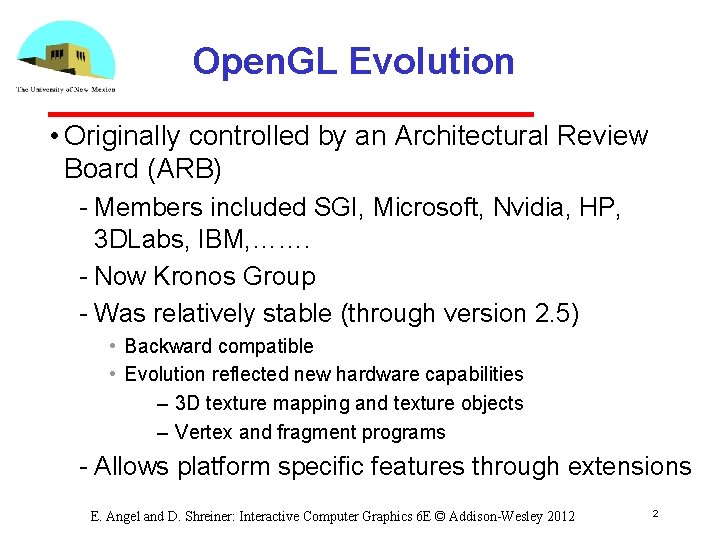
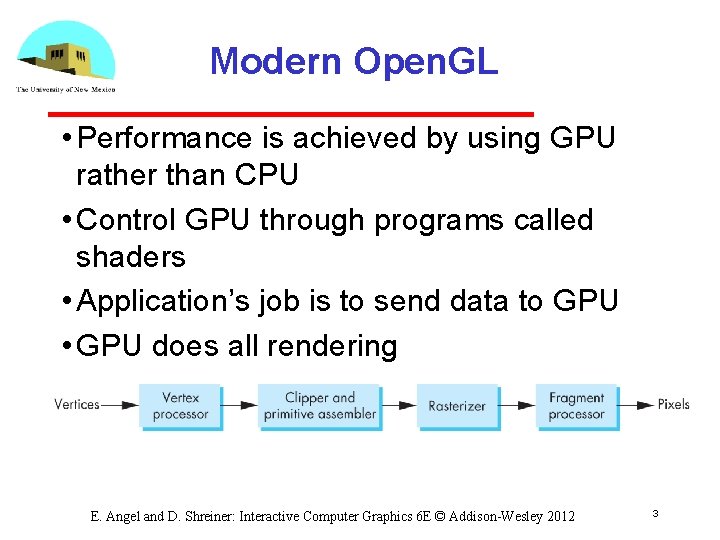
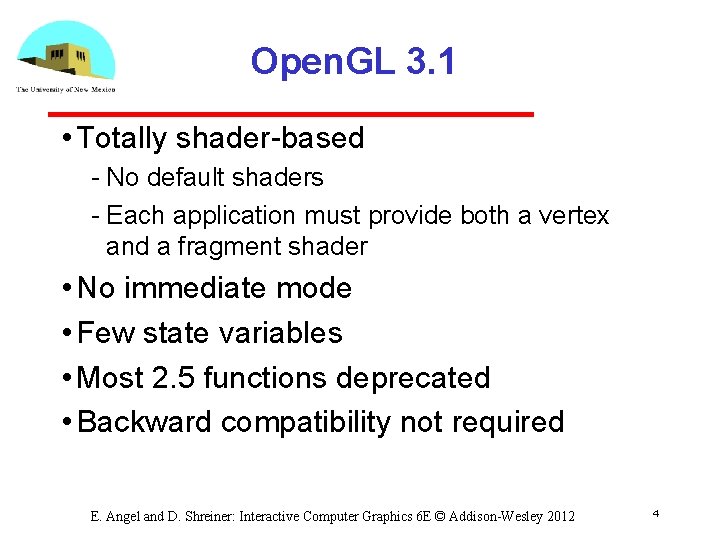
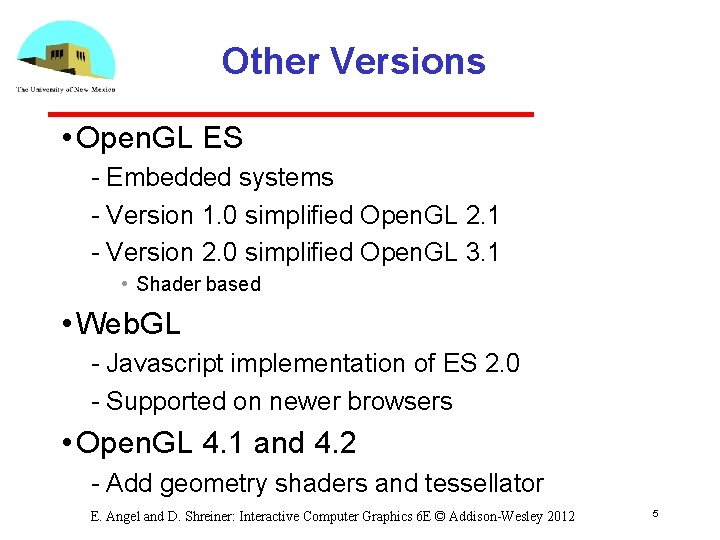
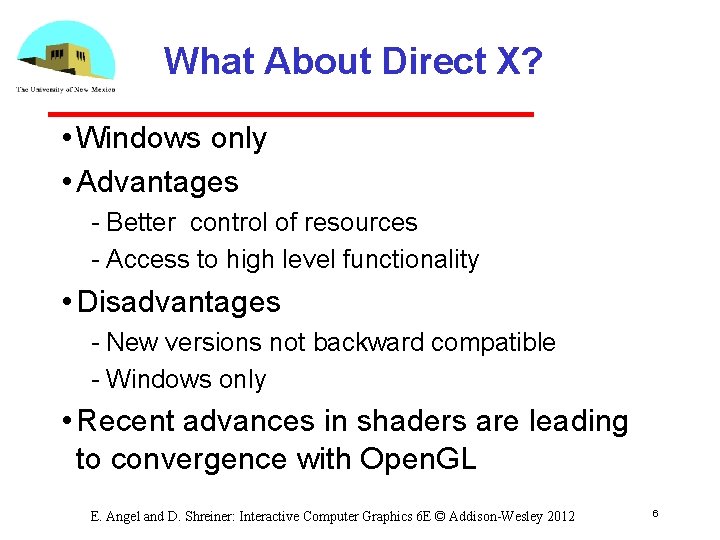
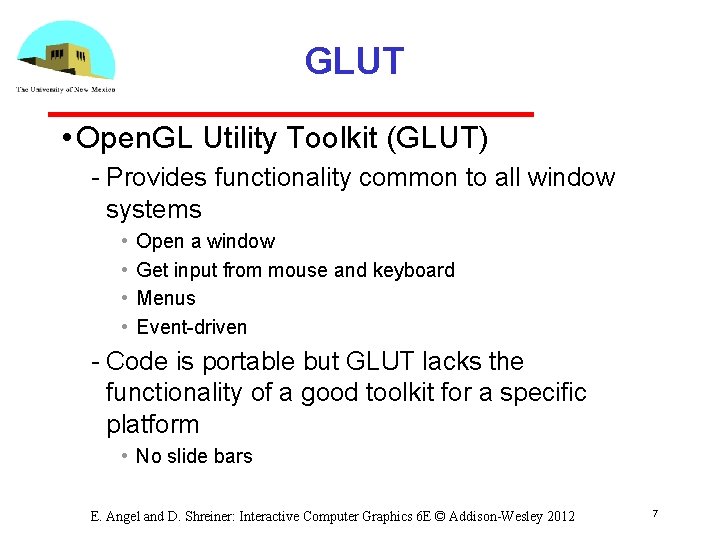
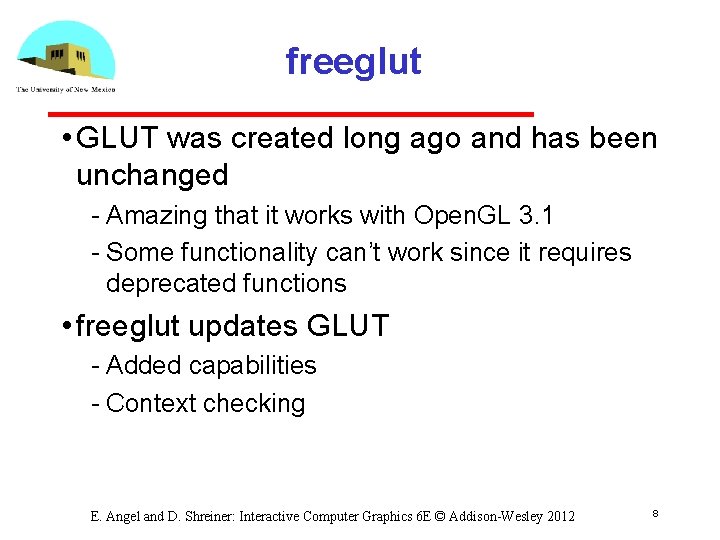
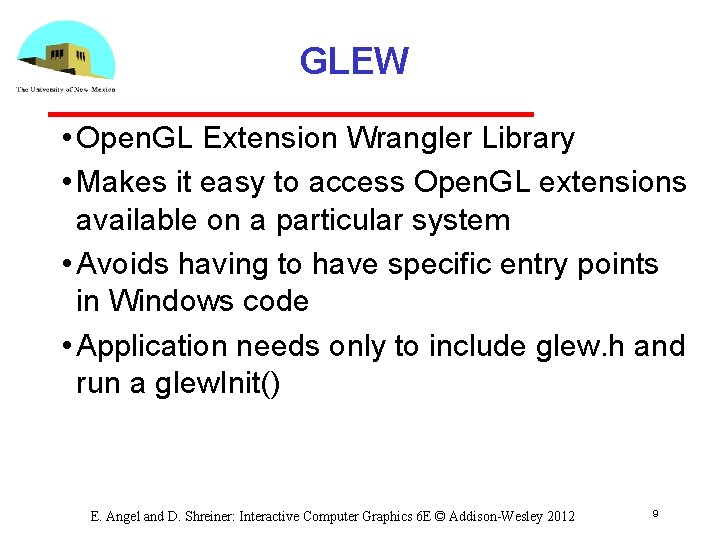
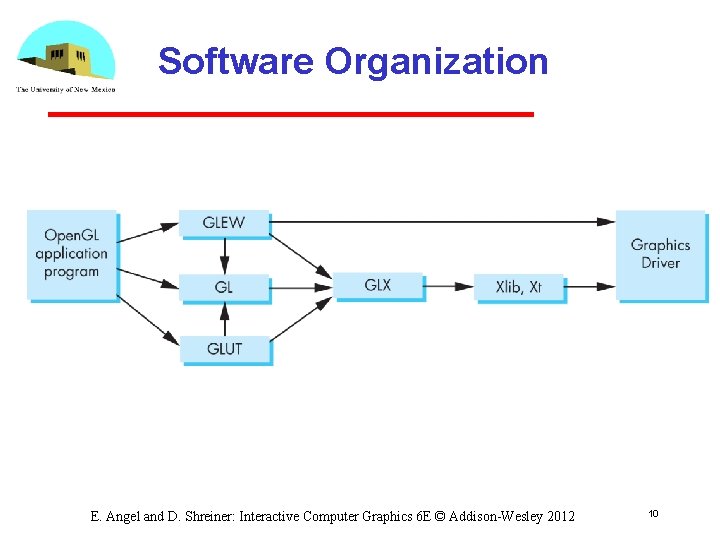
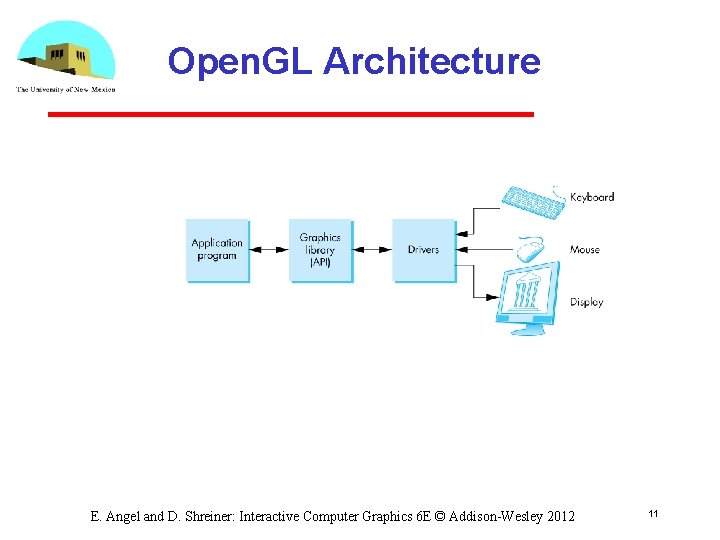
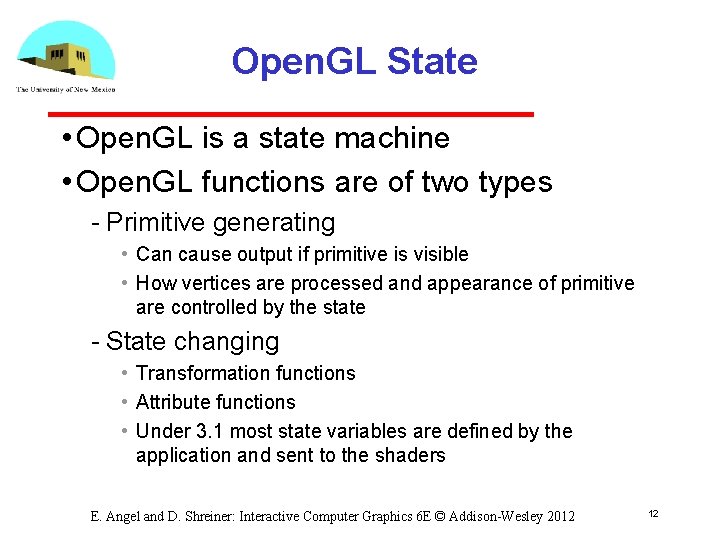
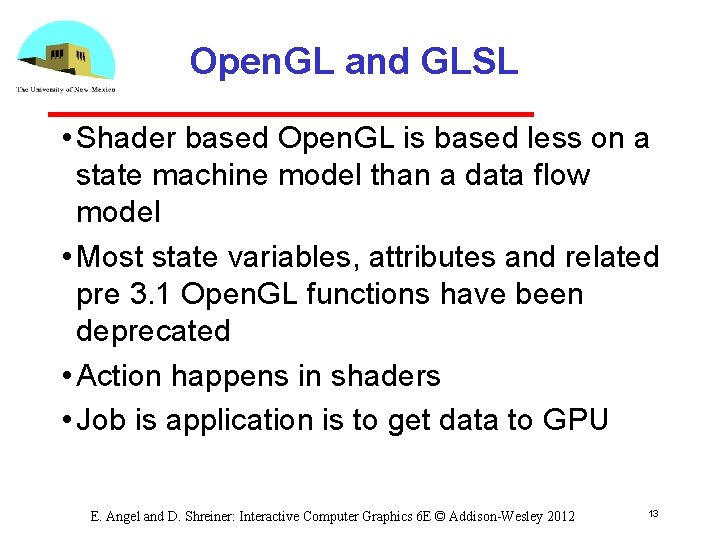
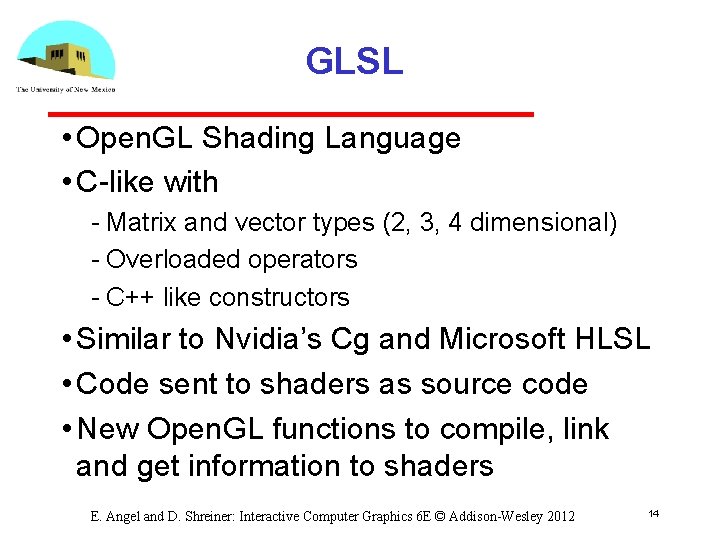
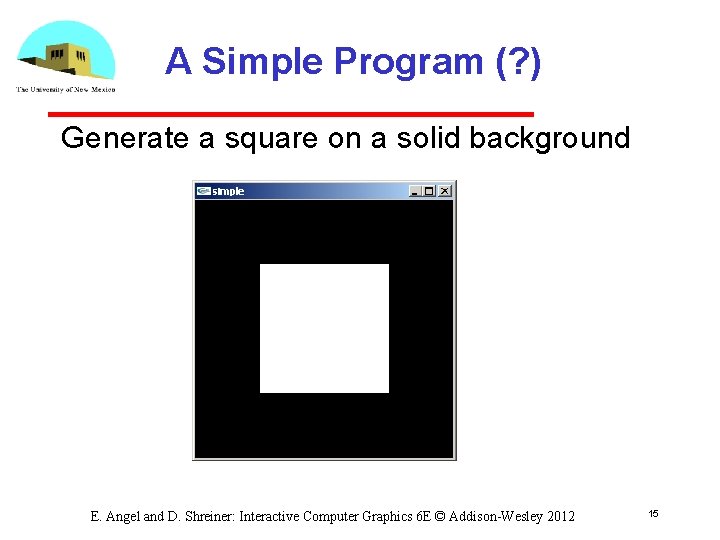
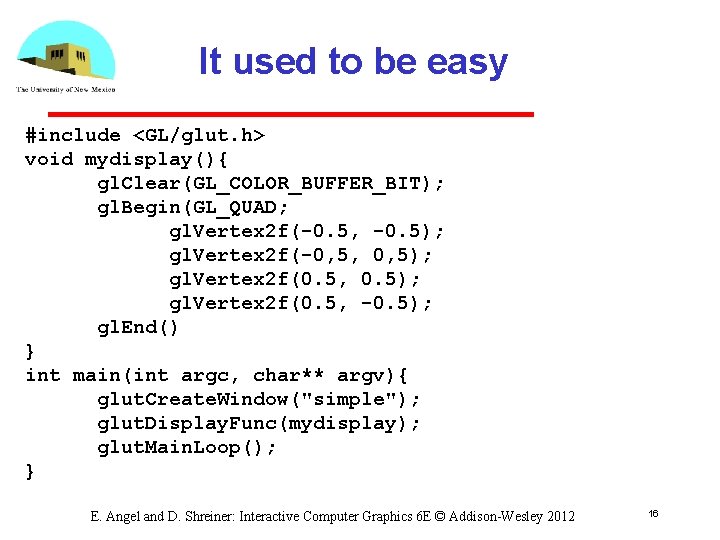
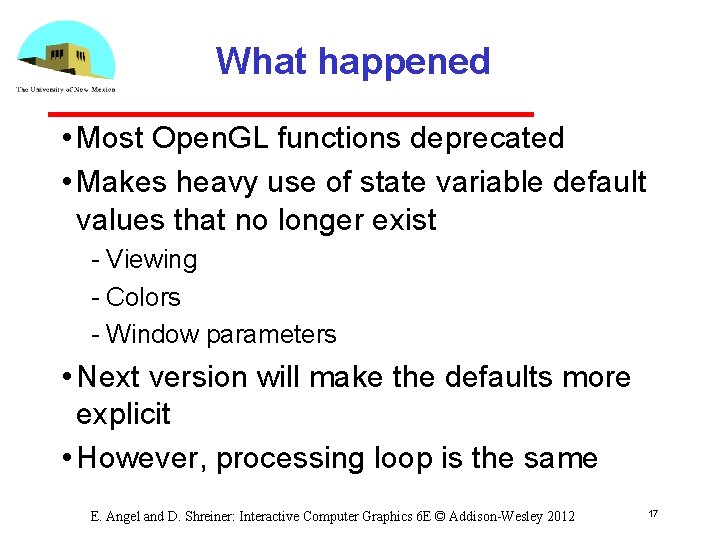
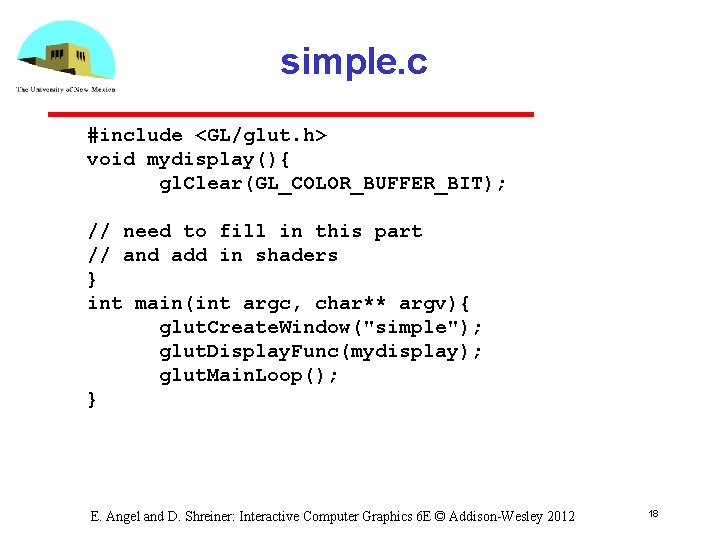
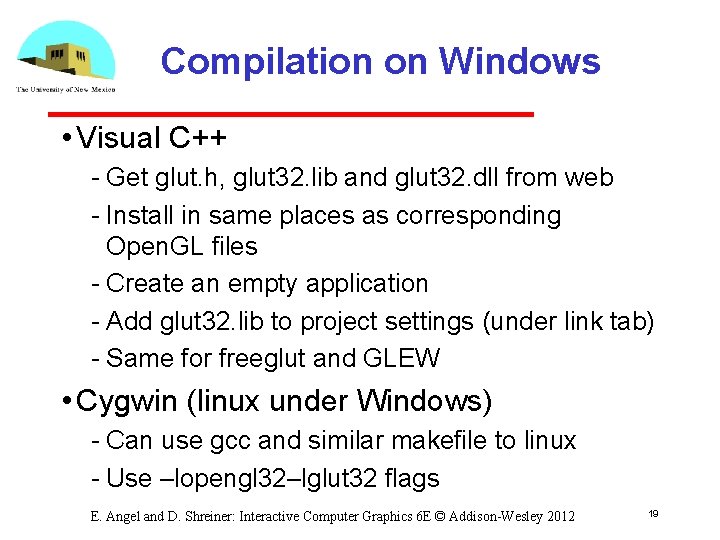
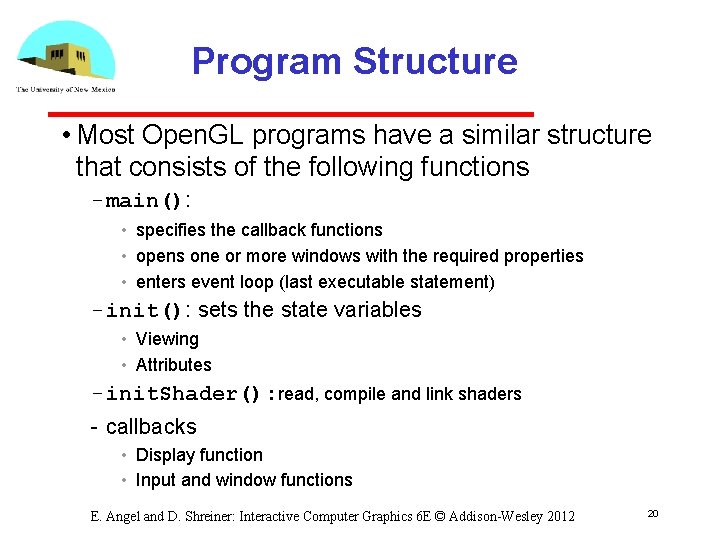
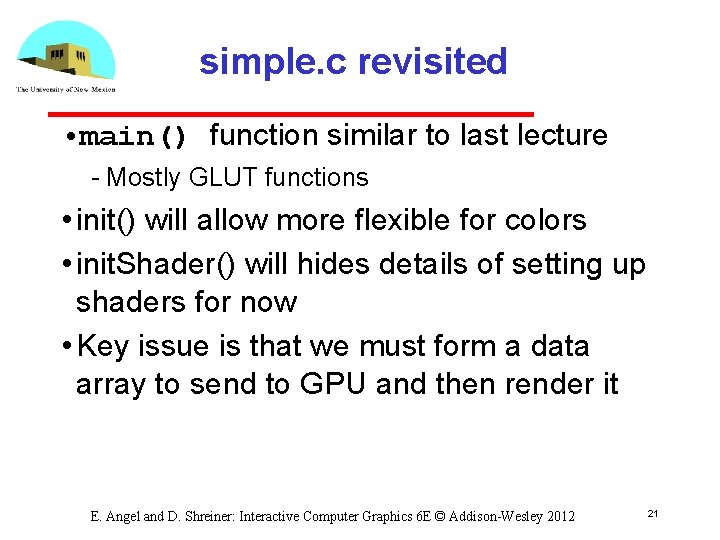
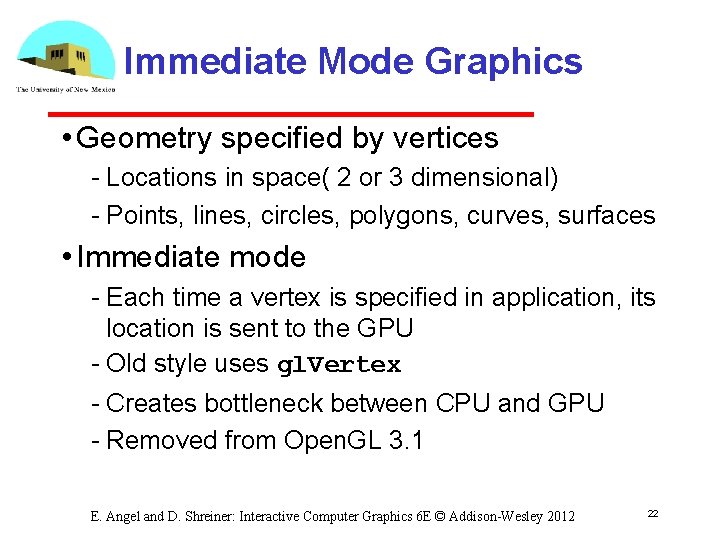
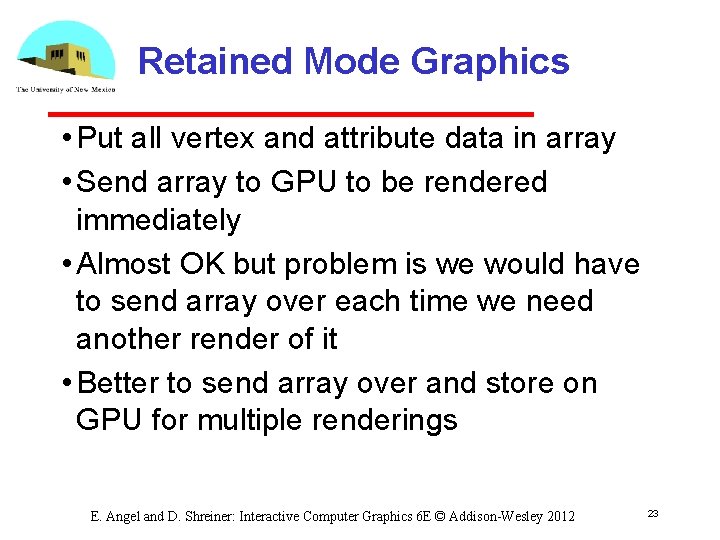
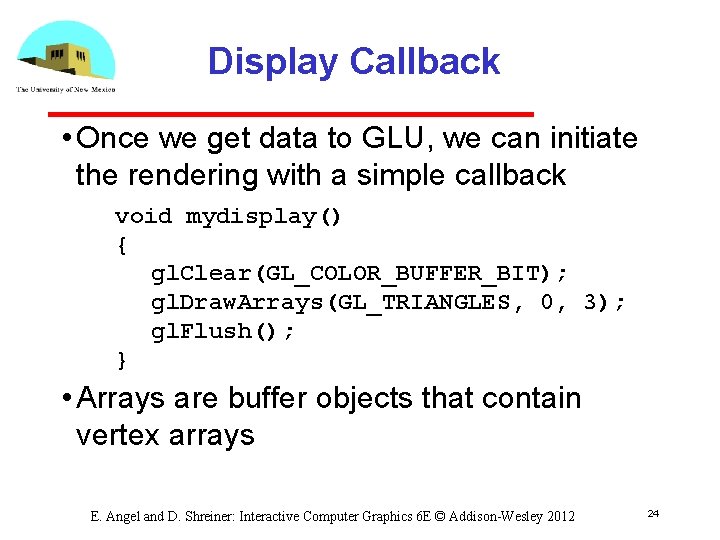
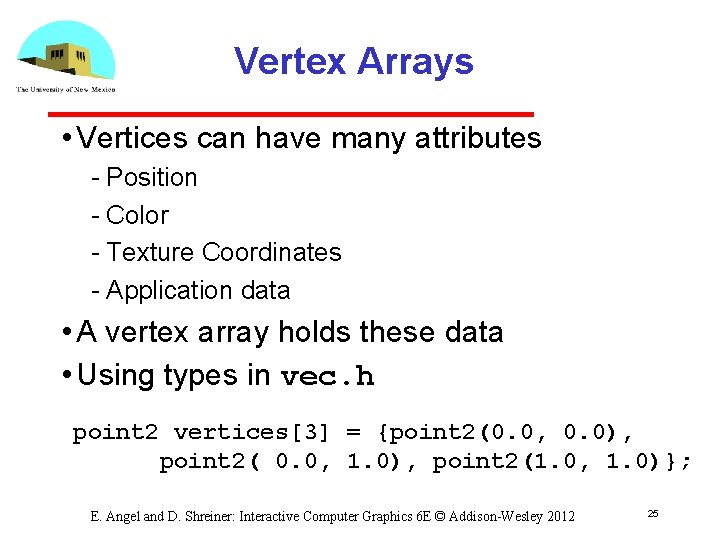
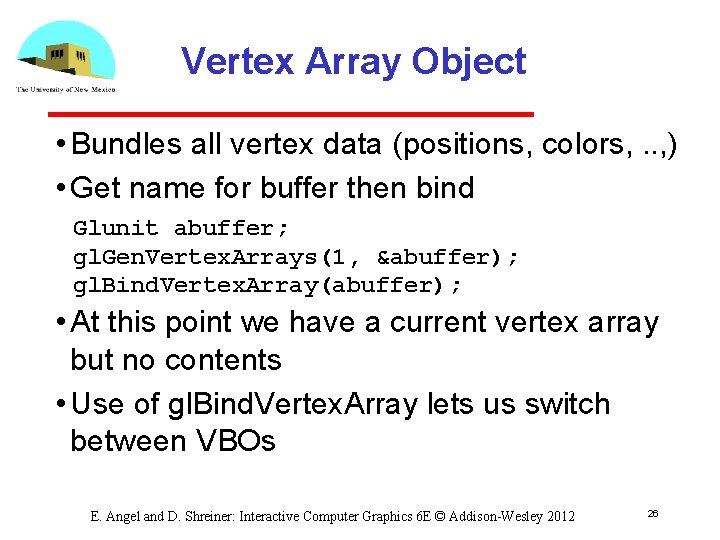
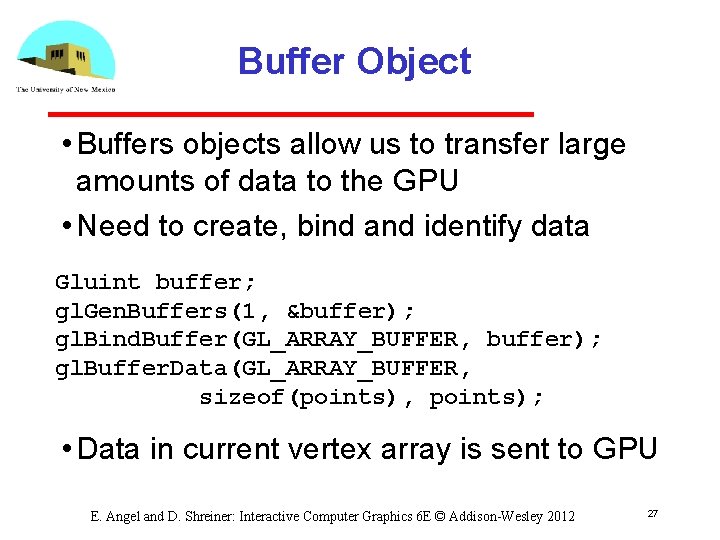
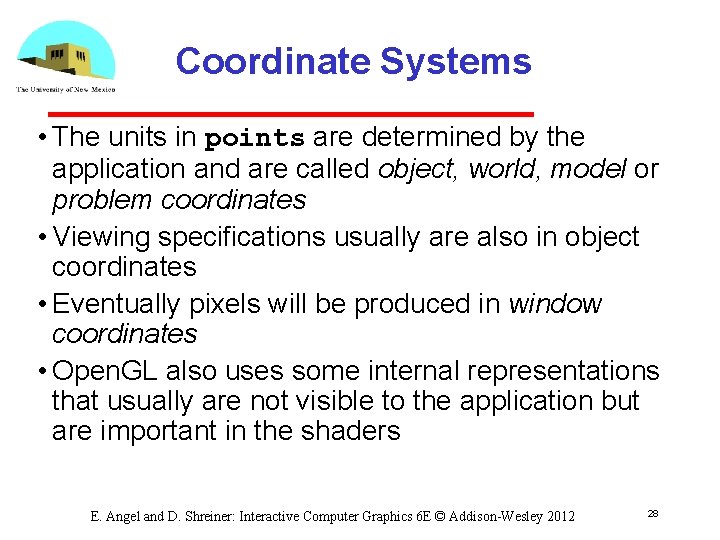
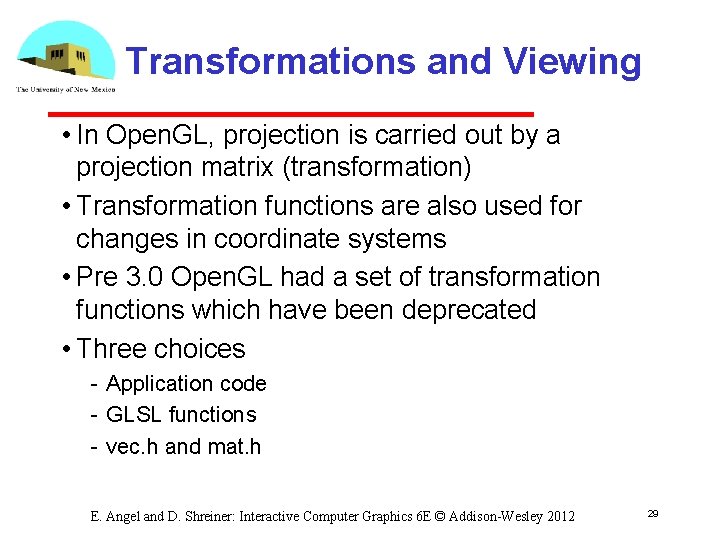
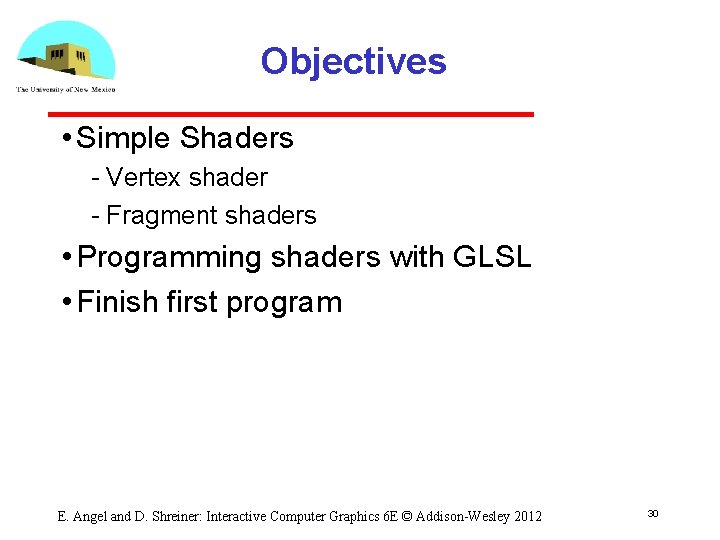
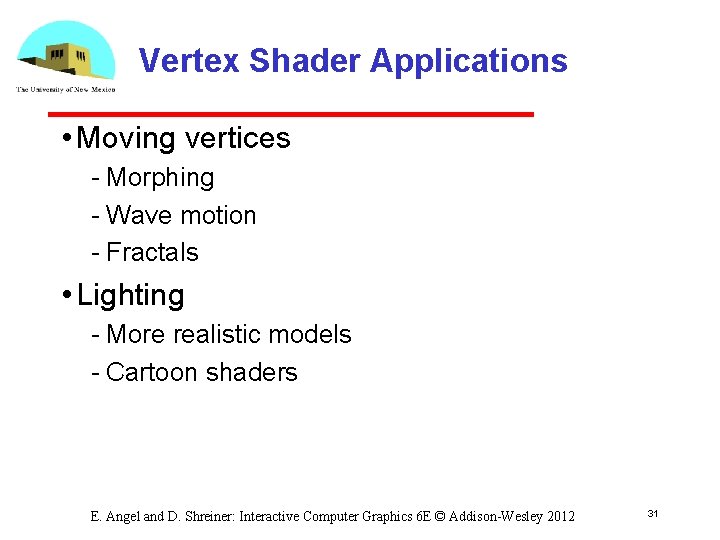
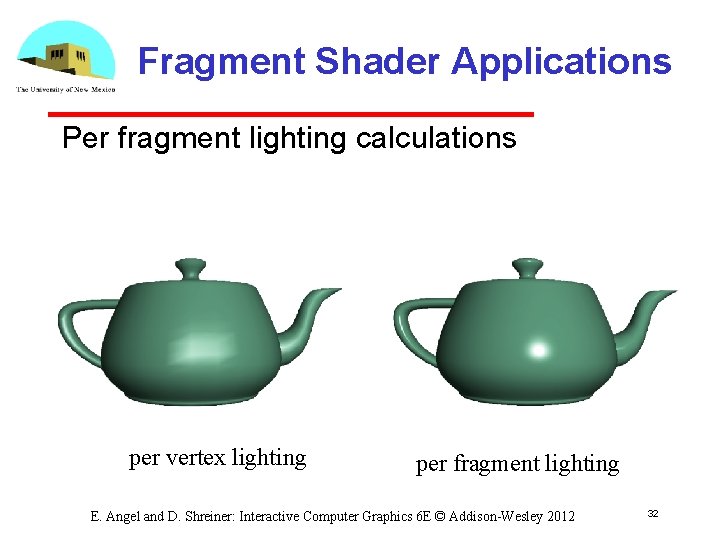
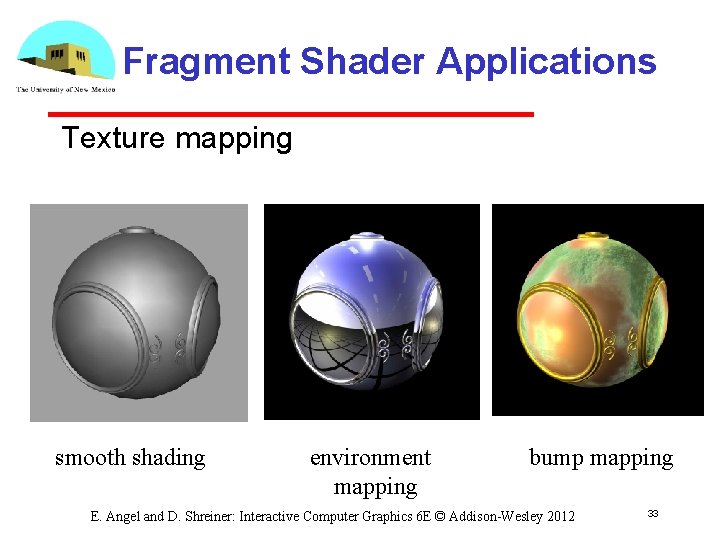
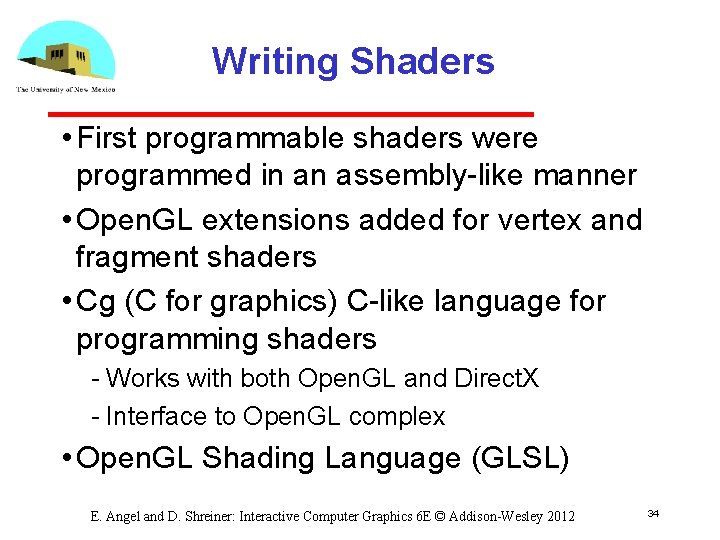
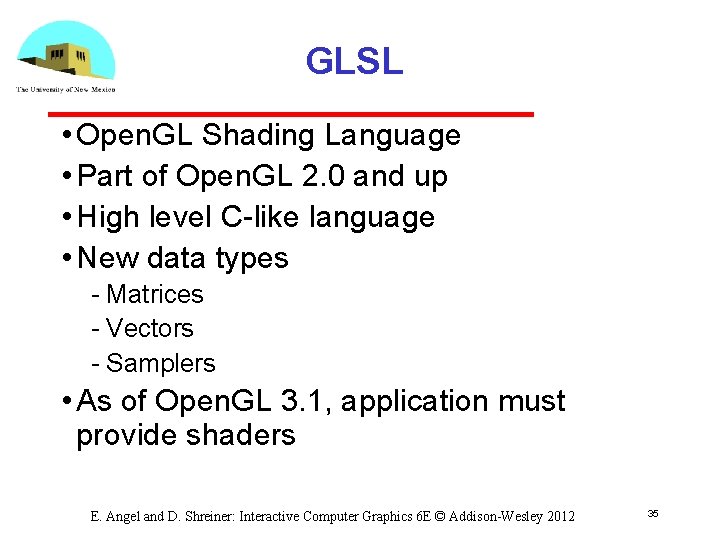
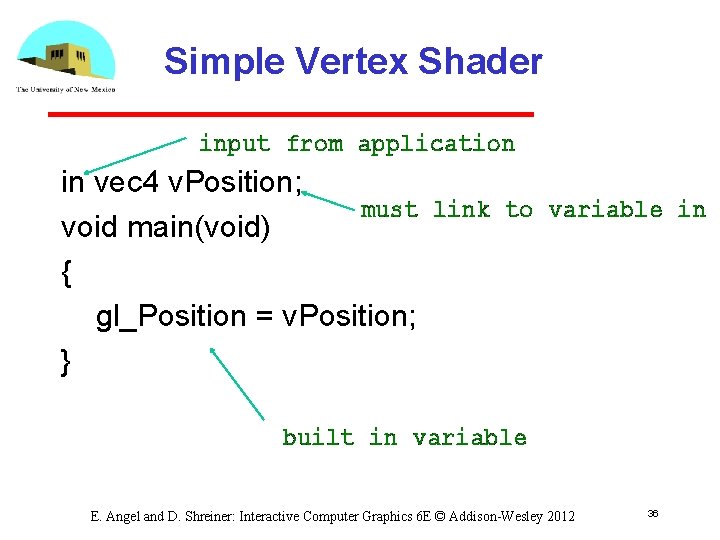
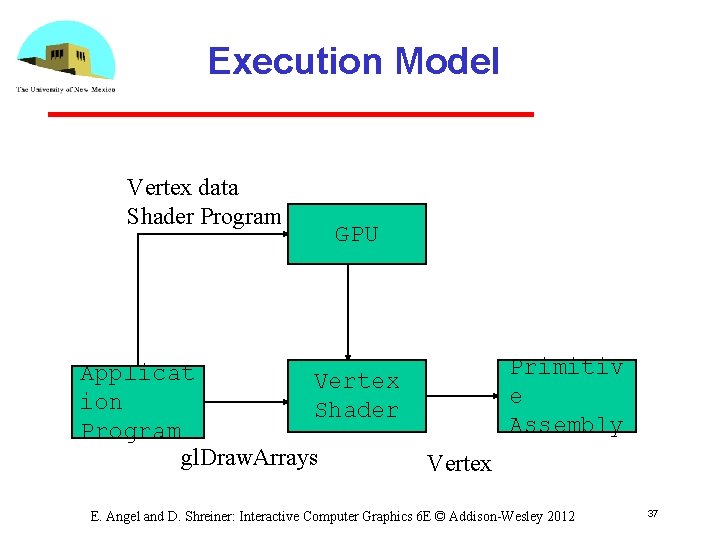
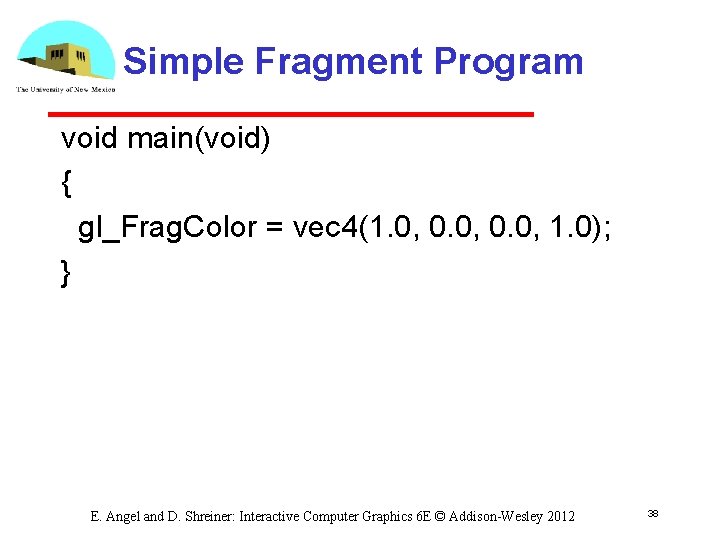
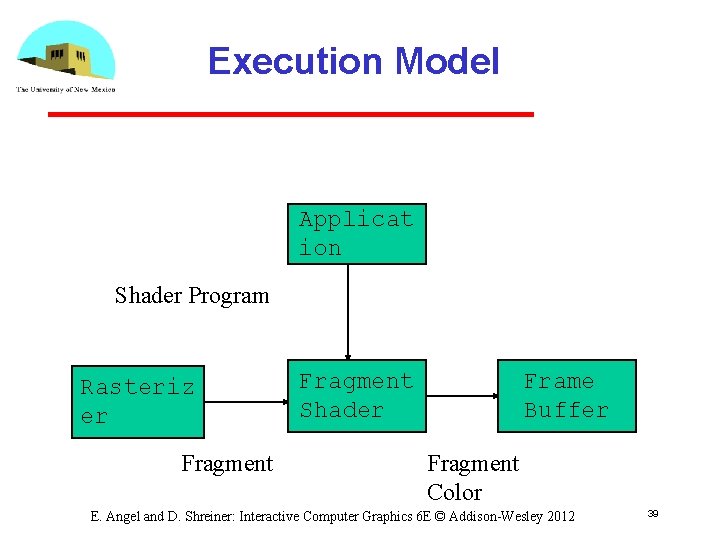
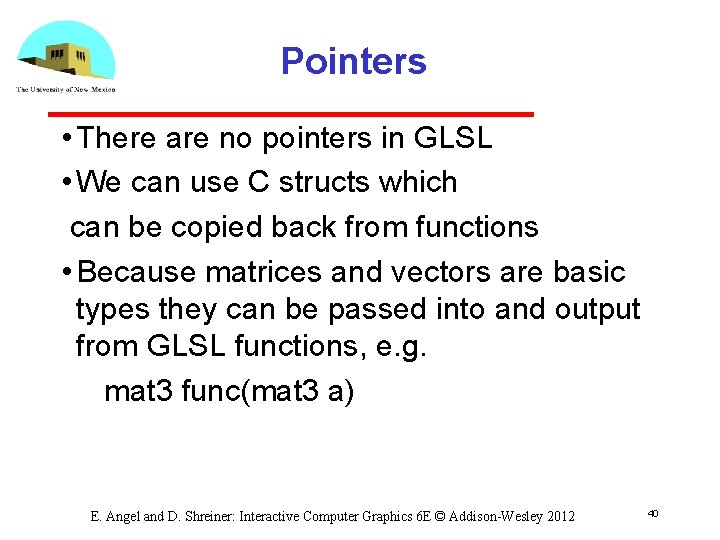
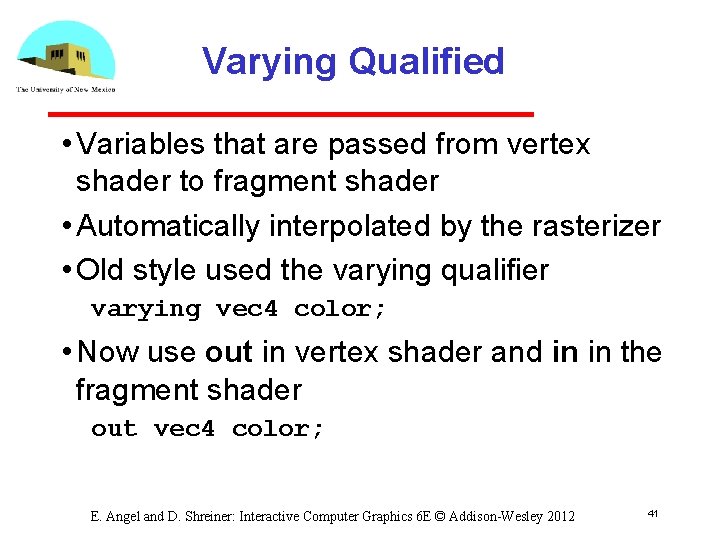
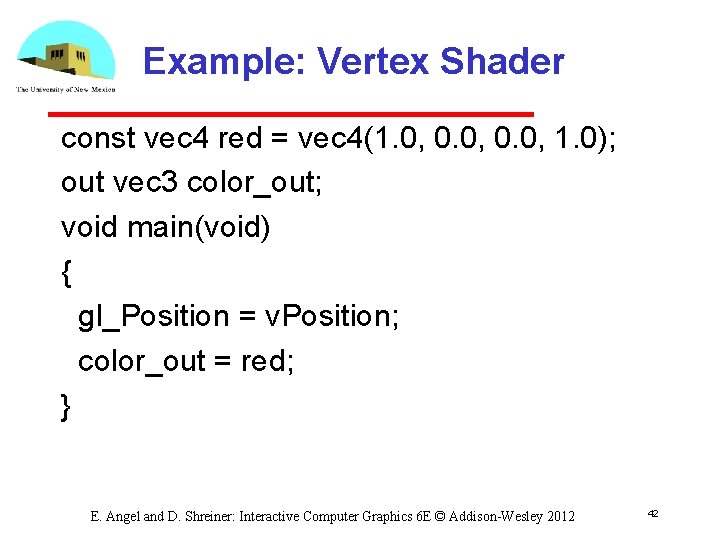
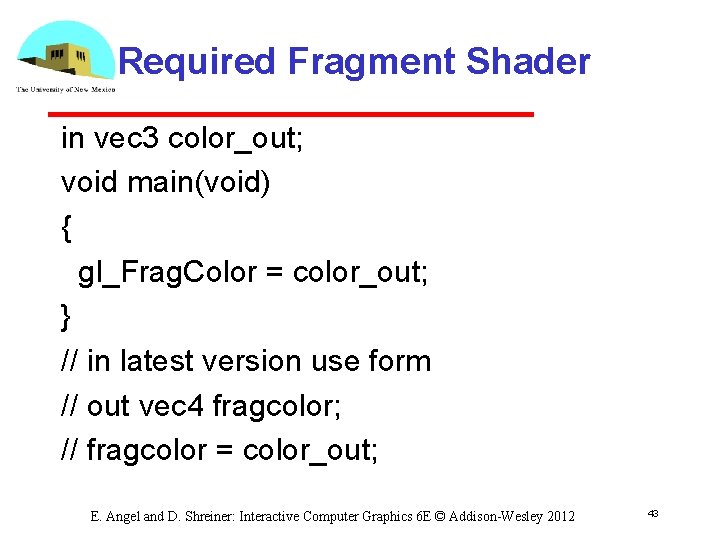
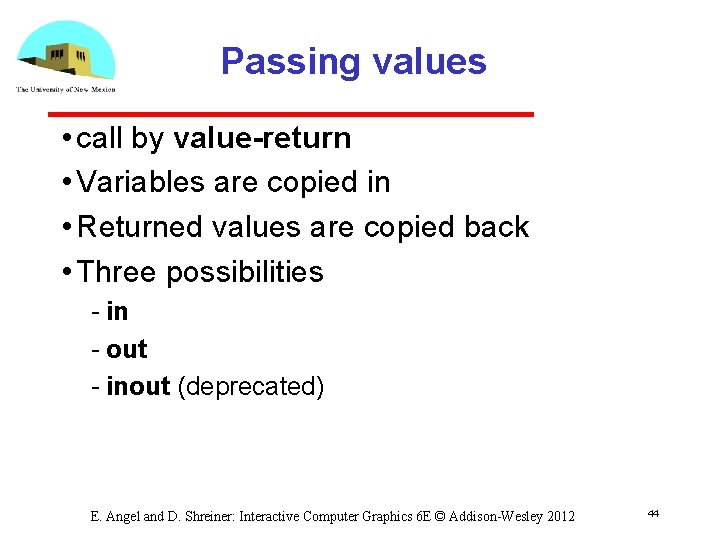
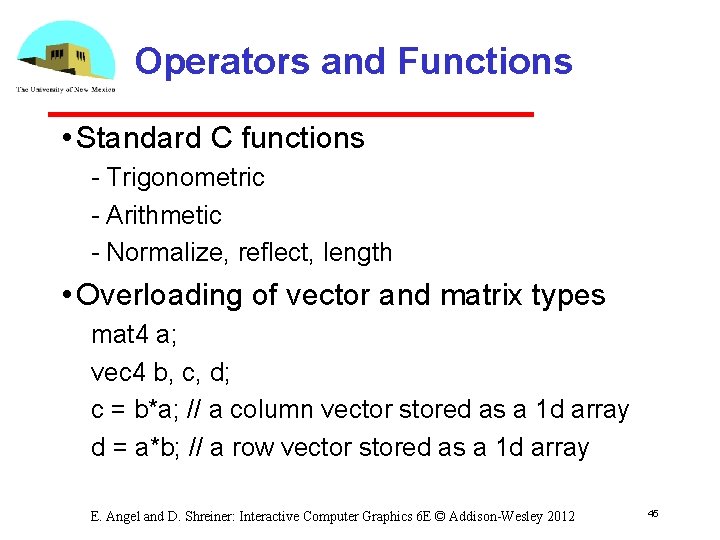
![Swizzling and Selection • Can refer to array elements by element using [] or Swizzling and Selection • Can refer to array elements by element using [] or](https://slidetodoc.com/presentation_image_h2/cbb2db02238232a56707f7243e368920/image-46.jpg)
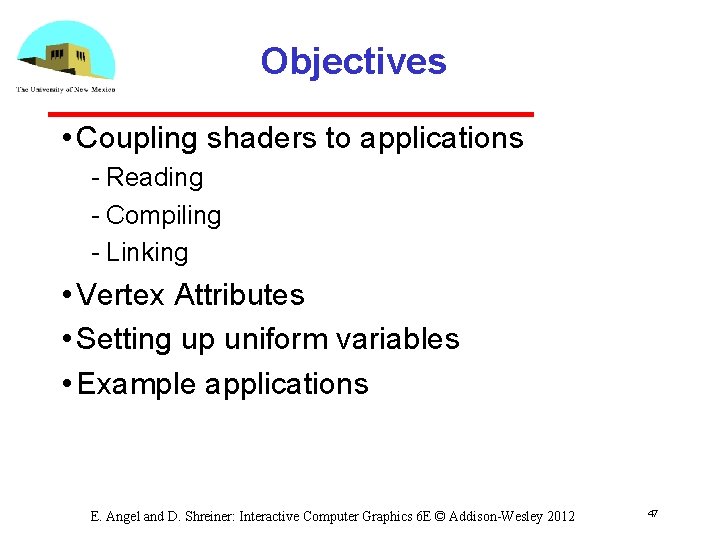
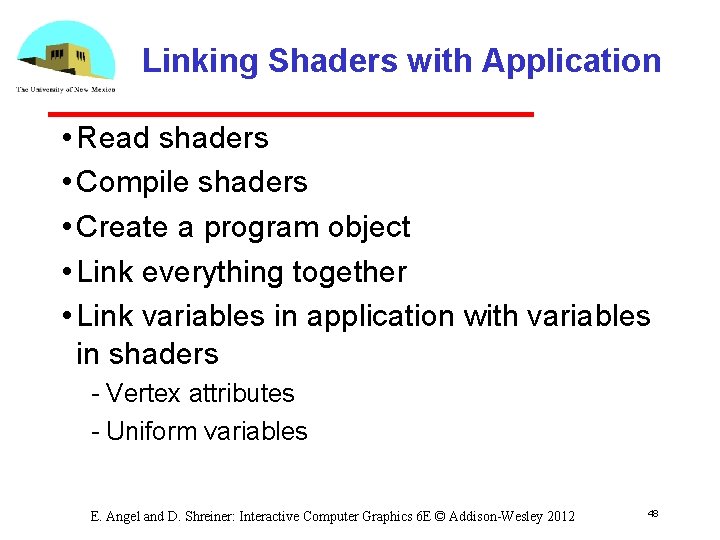
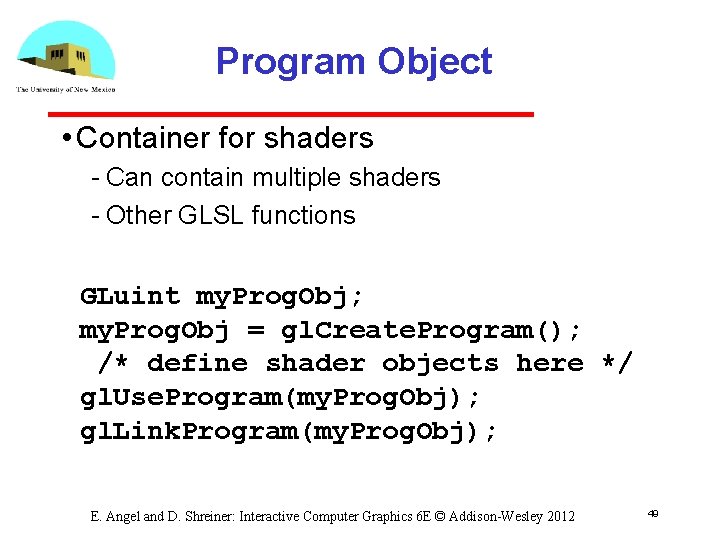
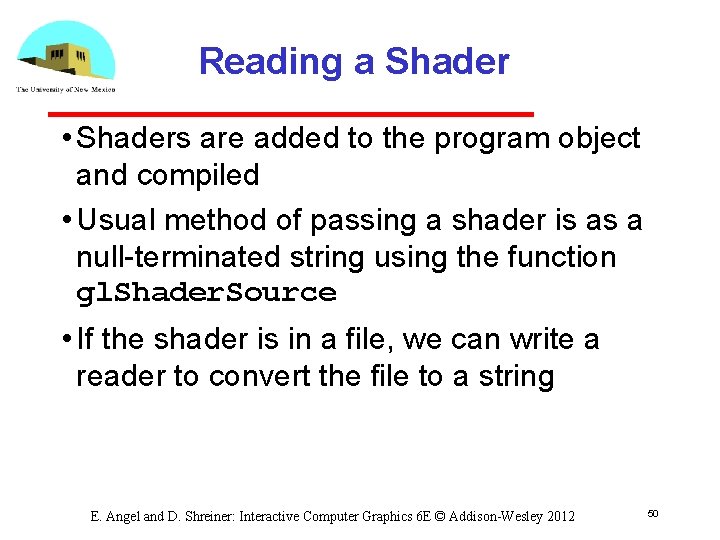
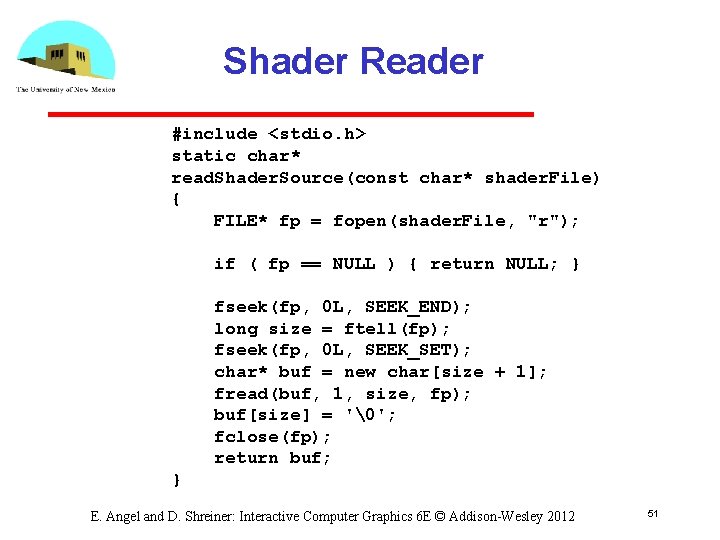
![Adding a Vertex Shader GLuint v. Shader; GLunit my. Vertex. Obj; GLchar v. Shaderfile[] Adding a Vertex Shader GLuint v. Shader; GLunit my. Vertex. Obj; GLchar v. Shaderfile[]](https://slidetodoc.com/presentation_image_h2/cbb2db02238232a56707f7243e368920/image-52.jpg)
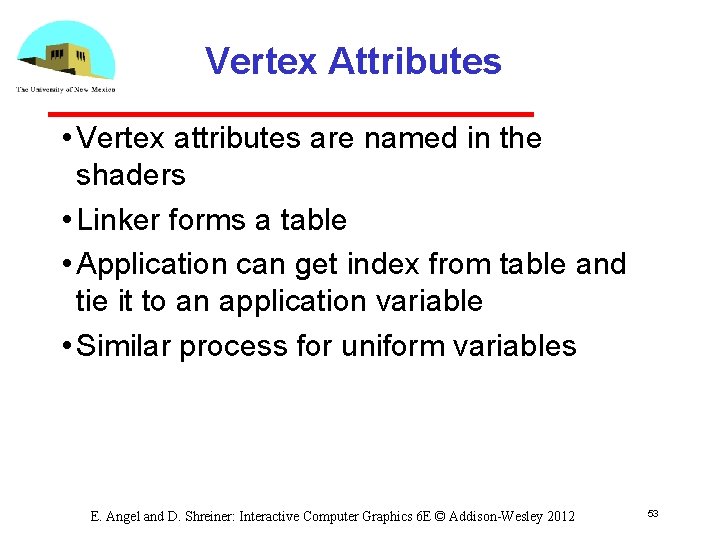
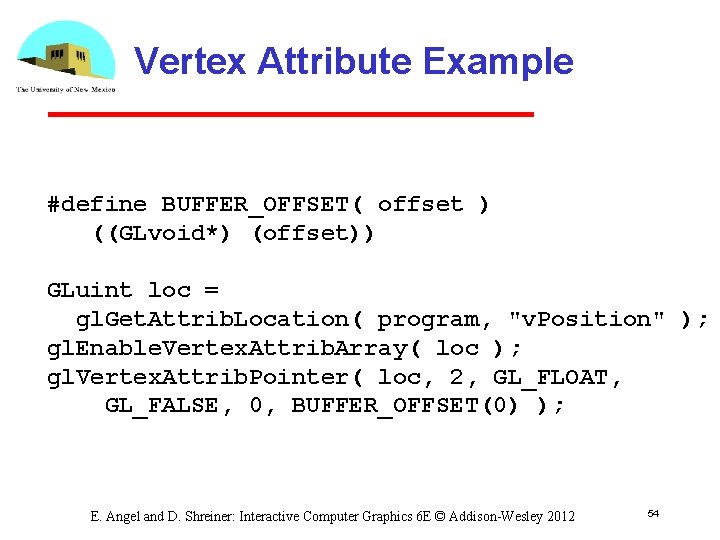
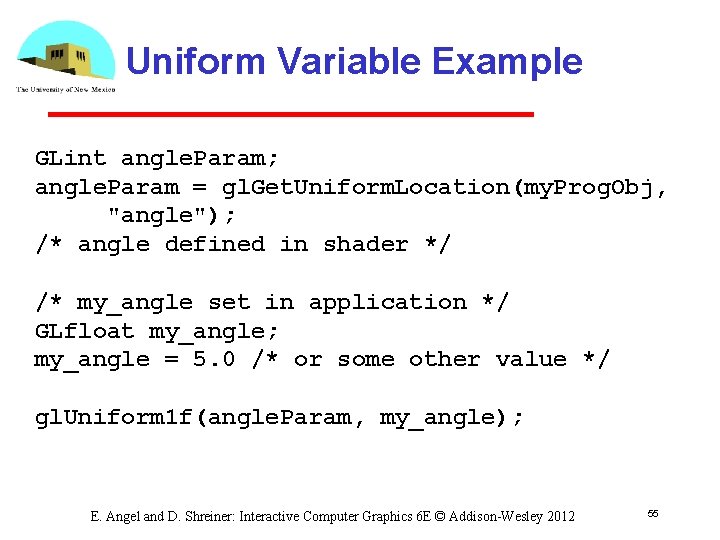
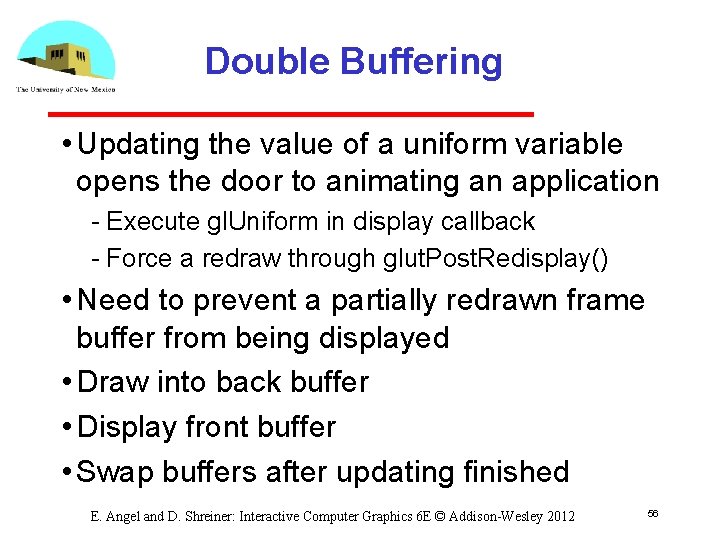
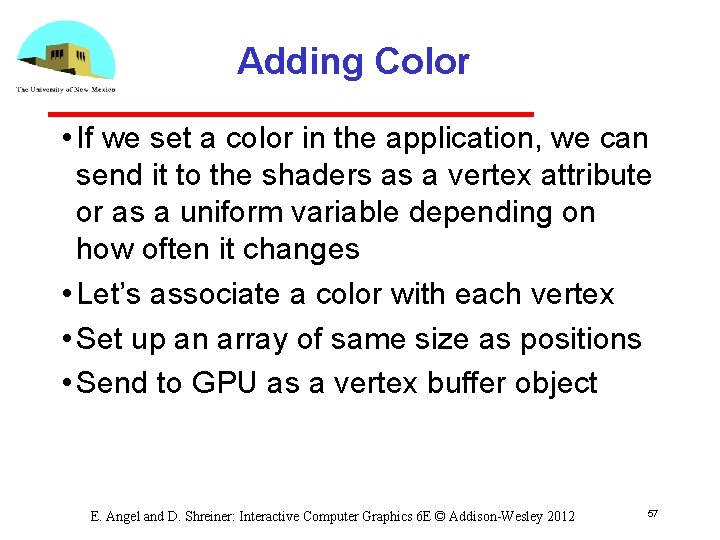
![Setting Colors typedef vec 3 color 3; color 3 base_colors[4] = {color 3(1. 0, Setting Colors typedef vec 3 color 3; color 3 base_colors[4] = {color 3(1. 0,](https://slidetodoc.com/presentation_image_h2/cbb2db02238232a56707f7243e368920/image-58.jpg)
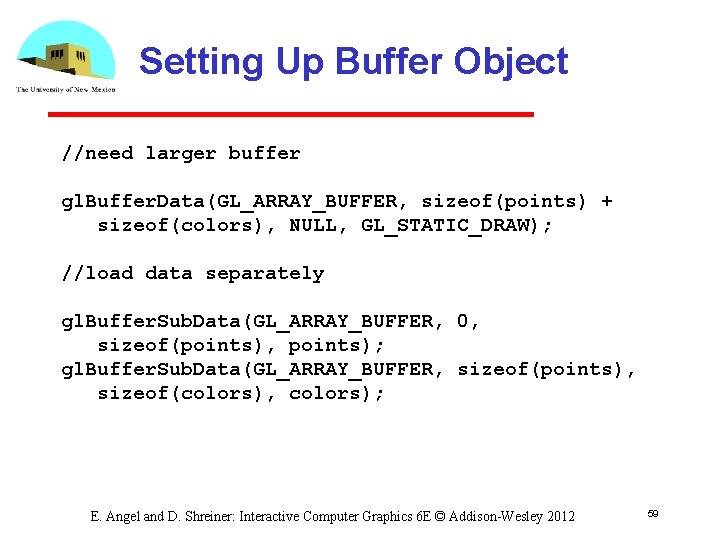
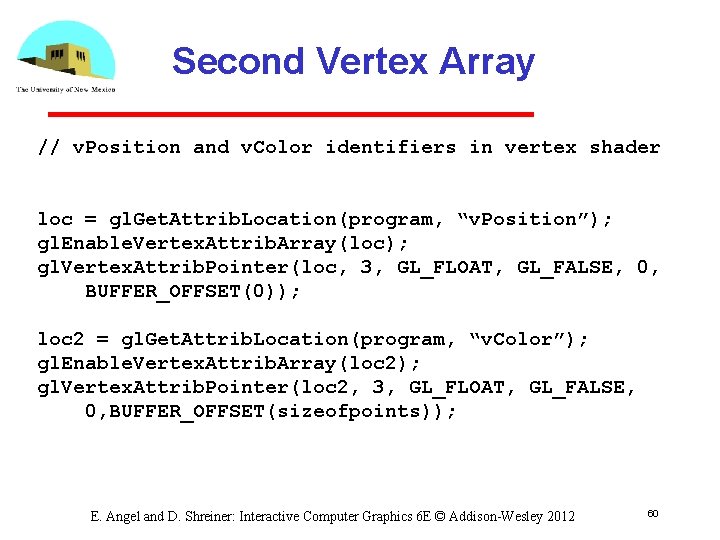
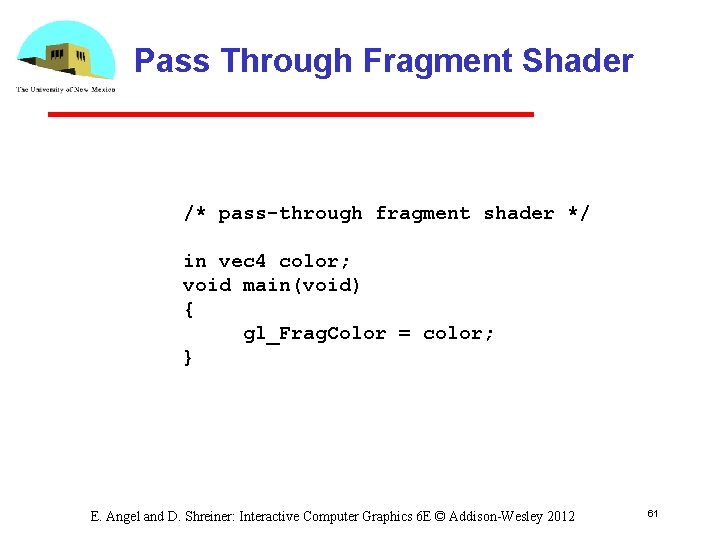
- Slides: 61
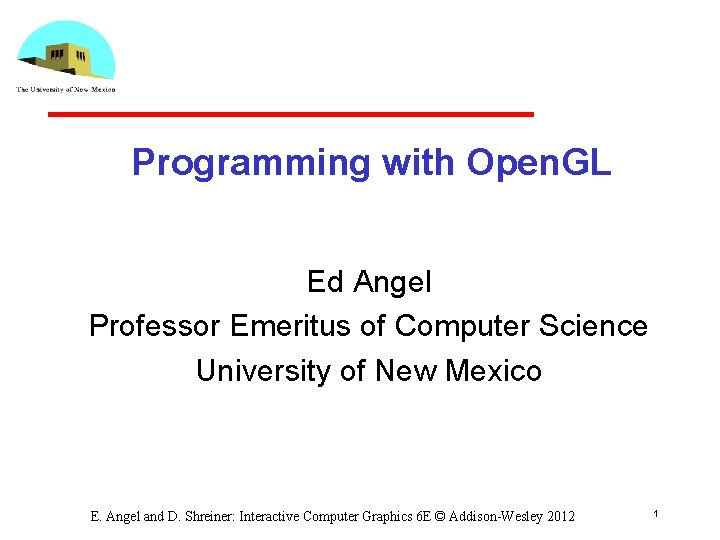
Programming with Open. GL Ed Angel Professor Emeritus of Computer Science University of New Mexico E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 1
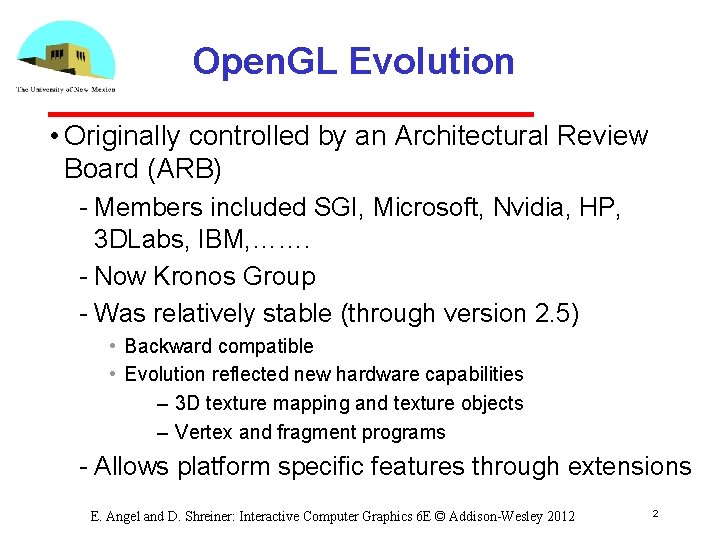
Open. GL Evolution • Originally controlled by an Architectural Review Board (ARB) Members included SGI, Microsoft, Nvidia, HP, 3 DLabs, IBM, ……. Now Kronos Group Was relatively stable (through version 2. 5) • Backward compatible • Evolution reflected new hardware capabilities – 3 D texture mapping and texture objects – Vertex and fragment programs Allows platform specific features through extensions E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 2
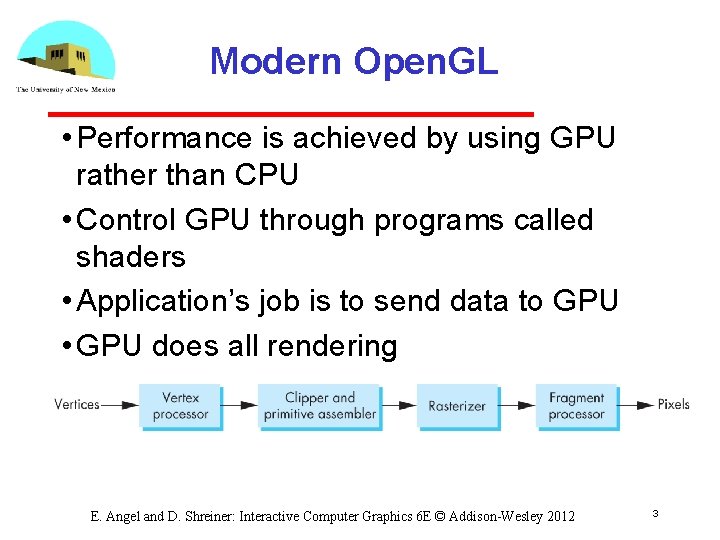
Modern Open. GL • Performance is achieved by using GPU rather than CPU • Control GPU through programs called shaders • Application’s job is to send data to GPU • GPU does all rendering E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 3
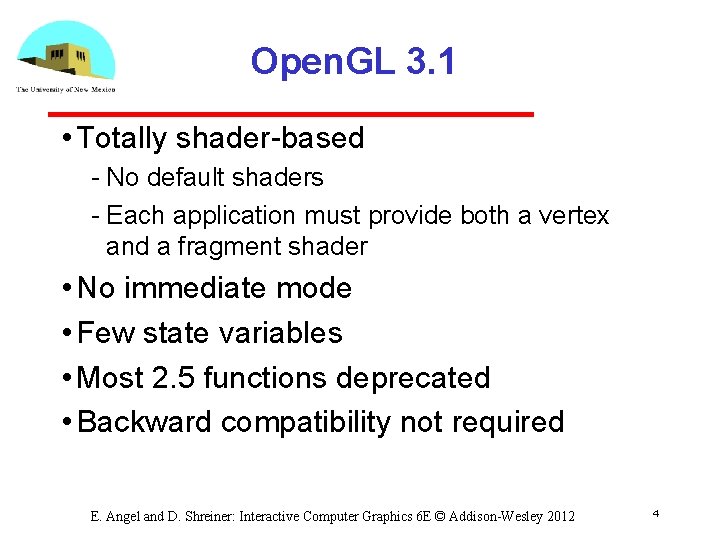
Open. GL 3. 1 • Totally shader based No default shaders Each application must provide both a vertex and a fragment shader • No immediate mode • Few state variables • Most 2. 5 functions deprecated • Backward compatibility not required E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 4
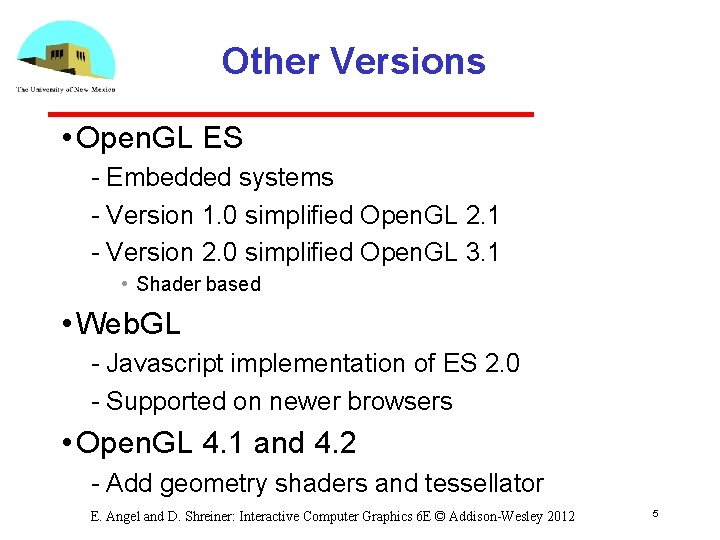
Other Versions • Open. GL ES Embedded systems Version 1. 0 simplified Open. GL 2. 1 Version 2. 0 simplified Open. GL 3. 1 • Shader based • Web. GL Javascript implementation of ES 2. 0 Supported on newer browsers • Open. GL 4. 1 and 4. 2 Add geometry shaders and tessellator E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 5
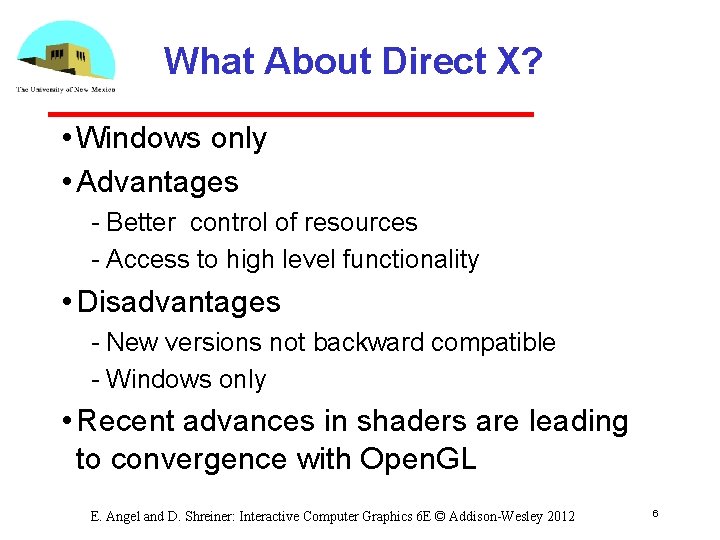
What About Direct X? • Windows only • Advantages Better control of resources Access to high level functionality • Disadvantages New versions not backward compatible Windows only • Recent advances in shaders are leading to convergence with Open. GL E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 6
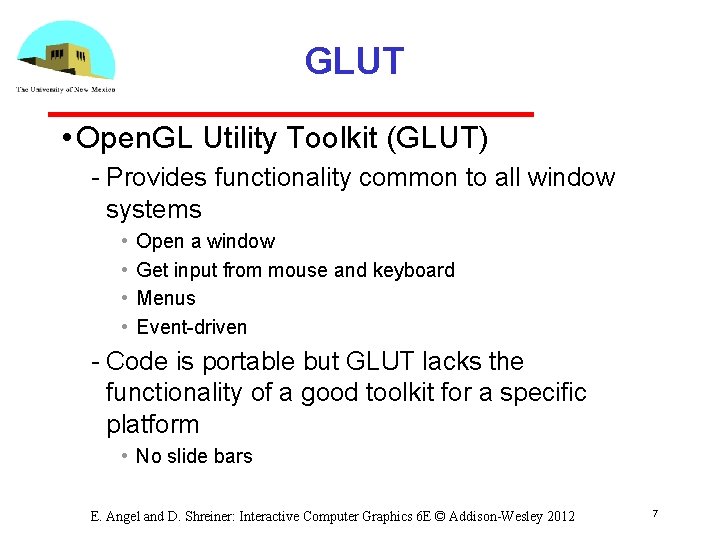
GLUT • Open. GL Utility Toolkit (GLUT) Provides functionality common to all window systems • • Open a window Get input from mouse and keyboard Menus Event driven Code is portable but GLUT lacks the functionality of a good toolkit for a specific platform • No slide bars E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 7
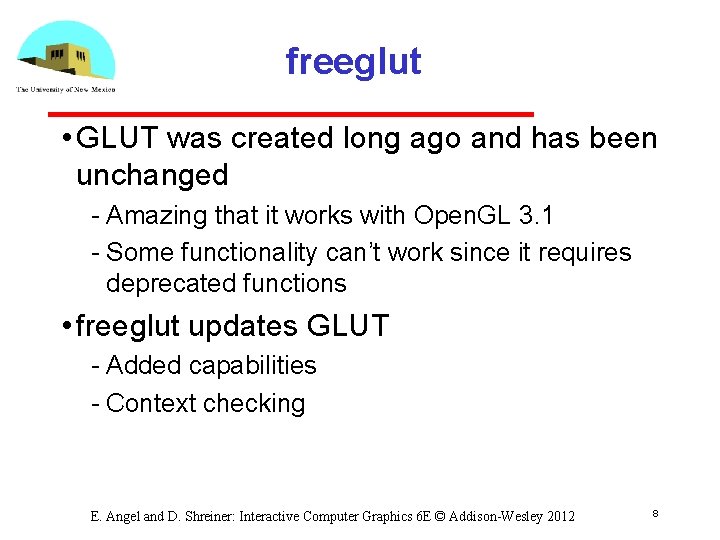
freeglut • GLUT was created long ago and has been unchanged Amazing that it works with Open. GL 3. 1 Some functionality can’t work since it requires deprecated functions • freeglut updates GLUT Added capabilities Context checking E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 8
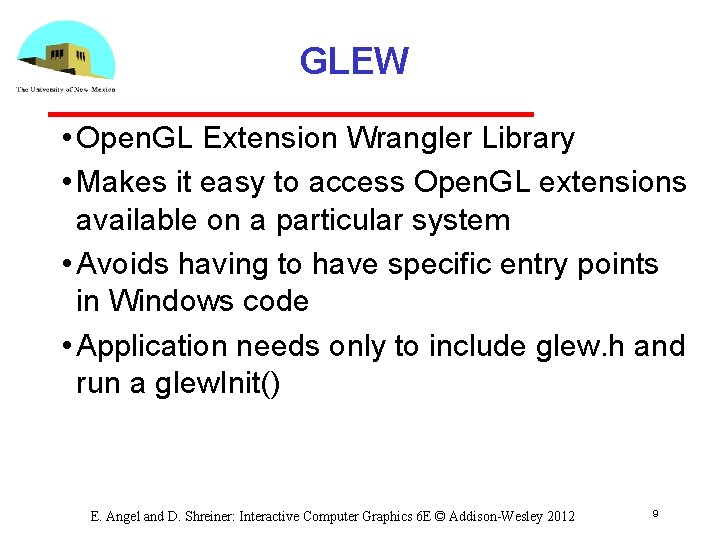
GLEW • Open. GL Extension Wrangler Library • Makes it easy to access Open. GL extensions available on a particular system • Avoids having to have specific entry points in Windows code • Application needs only to include glew. h and run a glew. Init() E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 9
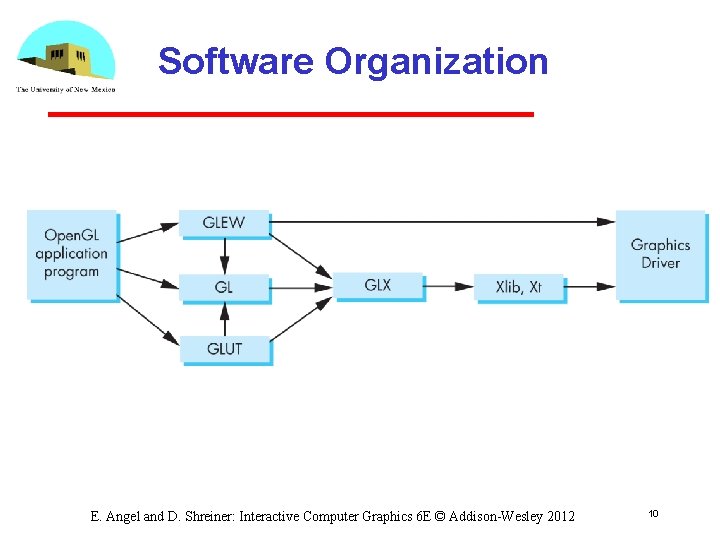
Software Organization E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 10
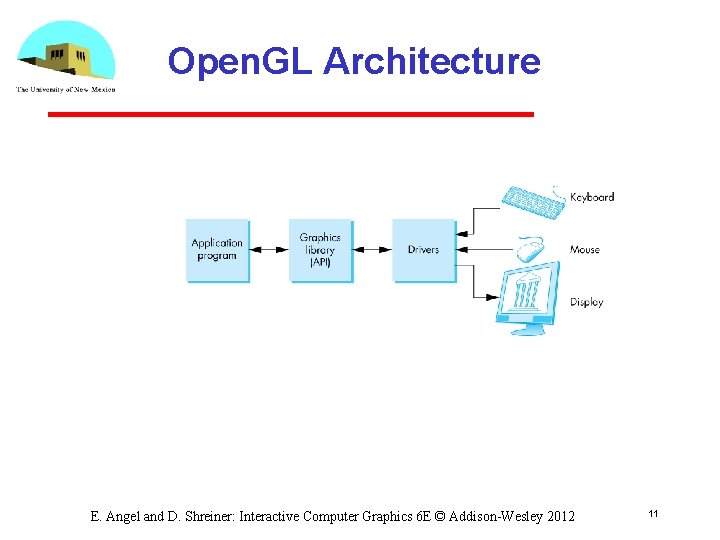
Open. GL Architecture E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 11
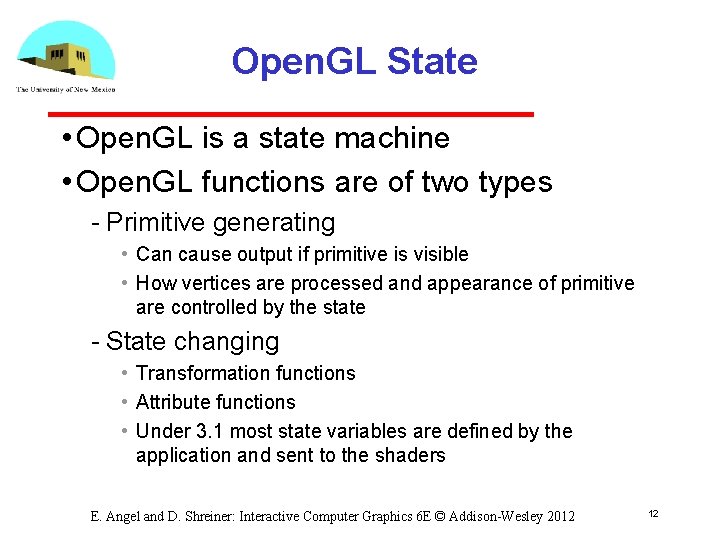
Open. GL State • Open. GL is a state machine • Open. GL functions are of two types Primitive generating • Can cause output if primitive is visible • How vertices are processed and appearance of primitive are controlled by the state State changing • Transformation functions • Attribute functions • Under 3. 1 most state variables are defined by the application and sent to the shaders E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 12
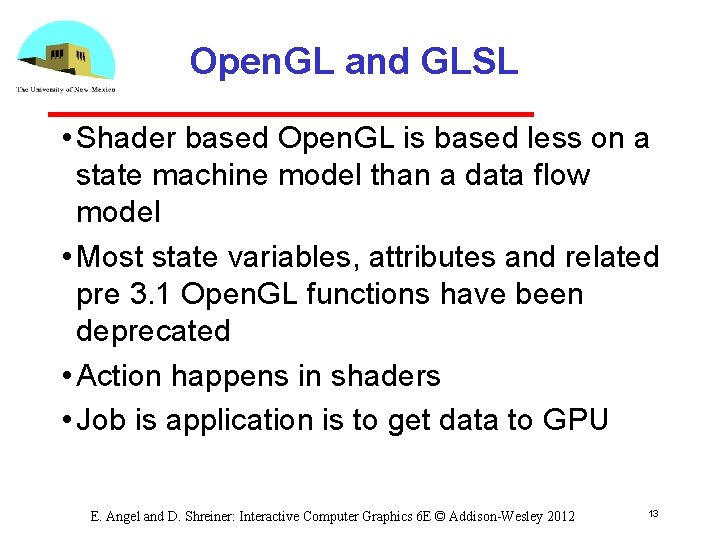
Open. GL and GLSL • Shader based Open. GL is based less on a state machine model than a data flow model • Most state variables, attributes and related pre 3. 1 Open. GL functions have been deprecated • Action happens in shaders • Job is application is to get data to GPU E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 13
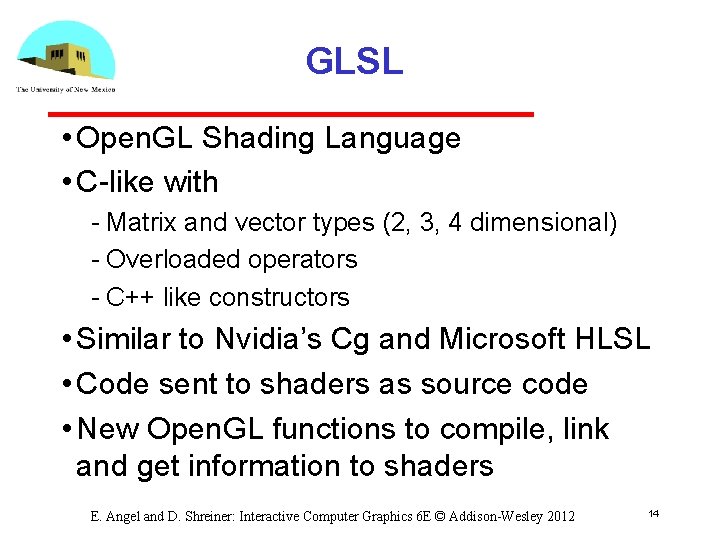
GLSL • Open. GL Shading Language • C like with Matrix and vector types (2, 3, 4 dimensional) Overloaded operators C++ like constructors • Similar to Nvidia’s Cg and Microsoft HLSL • Code sent to shaders as source code • New Open. GL functions to compile, link and get information to shaders E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 14
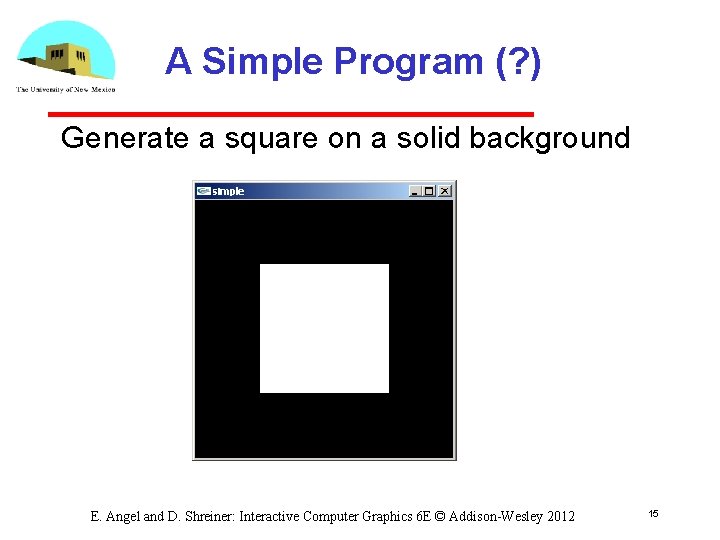
A Simple Program (? ) Generate a square on a solid background E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 15
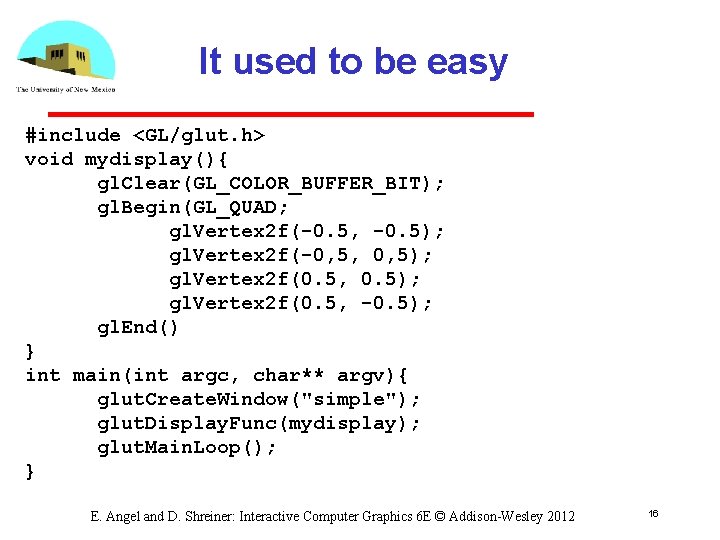
It used to be easy #include <GL/glut. h> void mydisplay(){ gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_QUAD; gl. Vertex 2 f(-0. 5, -0. 5); gl. Vertex 2 f(-0, 5, 0, 5); gl. Vertex 2 f(0. 5, 0. 5); gl. Vertex 2 f(0. 5, -0. 5); gl. End() } int main(int argc, char** argv){ glut. Create. Window("simple"); glut. Display. Func(mydisplay); glut. Main. Loop(); } E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 16
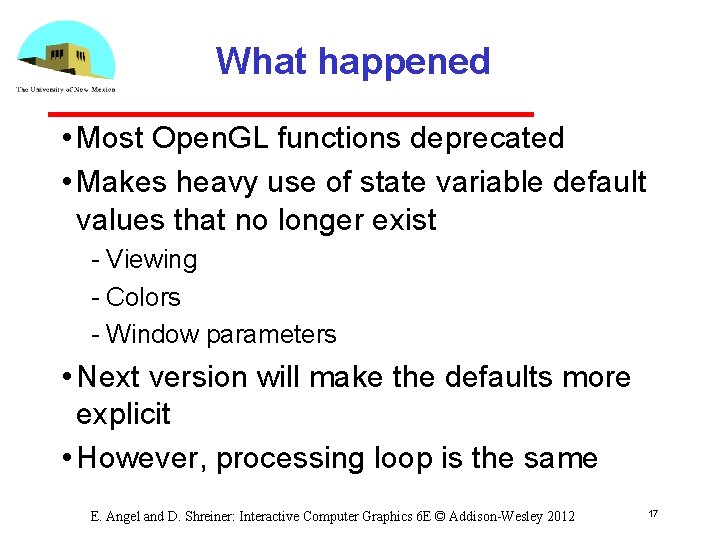
What happened • Most Open. GL functions deprecated • Makes heavy use of state variable default values that no longer exist Viewing Colors Window parameters • Next version will make the defaults more explicit • However, processing loop is the same E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 17
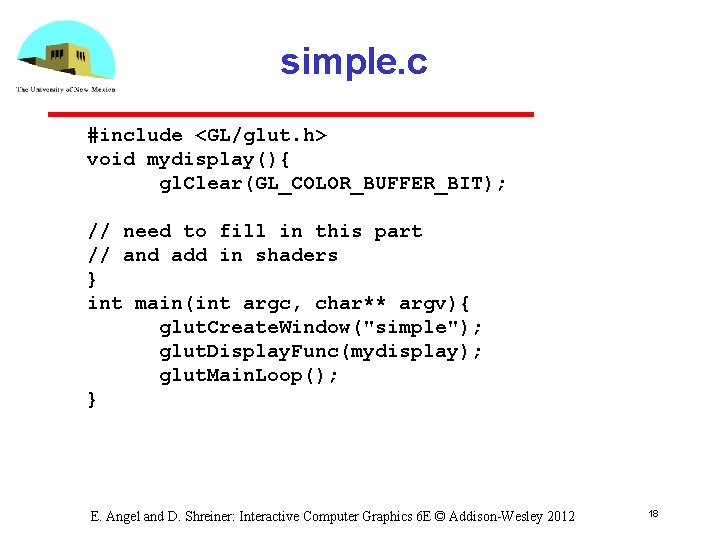
simple. c #include <GL/glut. h> void mydisplay(){ gl. Clear(GL_COLOR_BUFFER_BIT); // need to fill in this part // and add in shaders } int main(int argc, char** argv){ glut. Create. Window("simple"); glut. Display. Func(mydisplay); glut. Main. Loop(); } E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 18
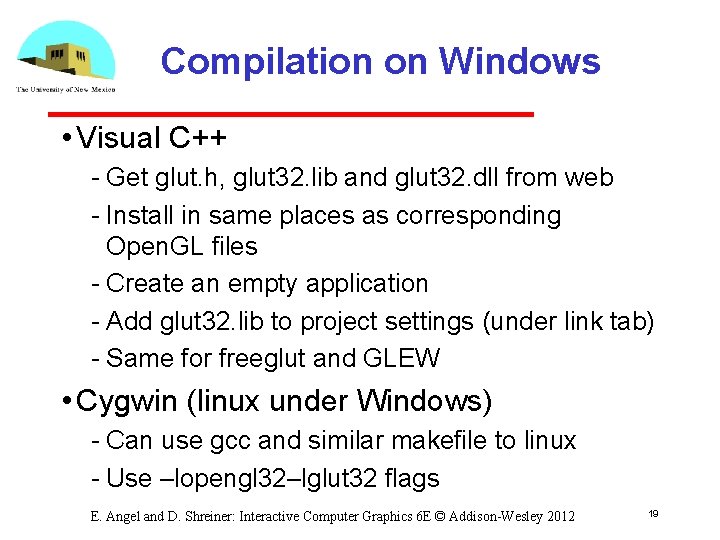
Compilation on Windows • Visual C++ Get glut. h, glut 32. lib and glut 32. dll from web Install in same places as corresponding Open. GL files Create an empty application Add glut 32. lib to project settings (under link tab) Same for freeglut and GLEW • Cygwin (linux under Windows) Can use gcc and similar makefile to linux Use –lopengl 32–lglut 32 flags E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 19
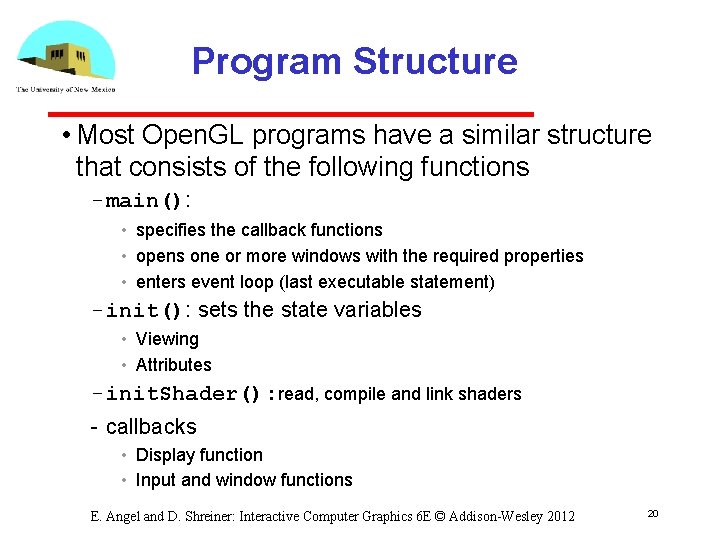
Program Structure • Most Open. GL programs have a similar structure that consists of the following functions main(): • specifies the callback functions • opens one or more windows with the required properties • enters event loop (last executable statement) init(): sets the state variables • Viewing • Attributes init. Shader(): read, compile and link shaders callbacks • Display function • Input and window functions E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 20
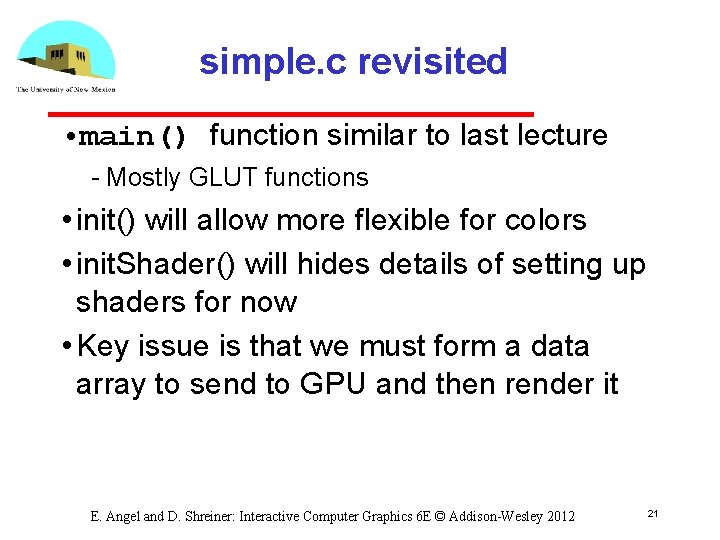
simple. c revisited • main() function similar to last lecture Mostly GLUT functions • init() will allow more flexible for colors • init. Shader() will hides details of setting up shaders for now • Key issue is that we must form a data array to send to GPU and then render it E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 21
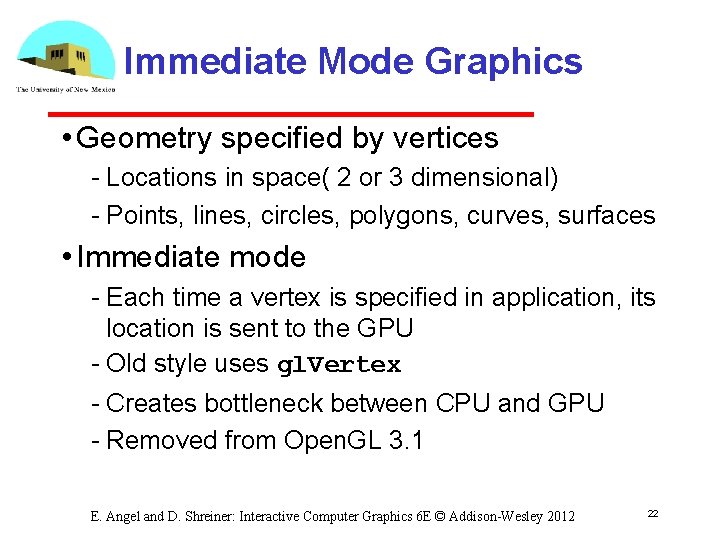
Immediate Mode Graphics • Geometry specified by vertices Locations in space( 2 or 3 dimensional) Points, lines, circles, polygons, curves, surfaces • Immediate mode Each time a vertex is specified in application, its location is sent to the GPU Old style uses gl. Vertex Creates bottleneck between CPU and GPU Removed from Open. GL 3. 1 E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 22
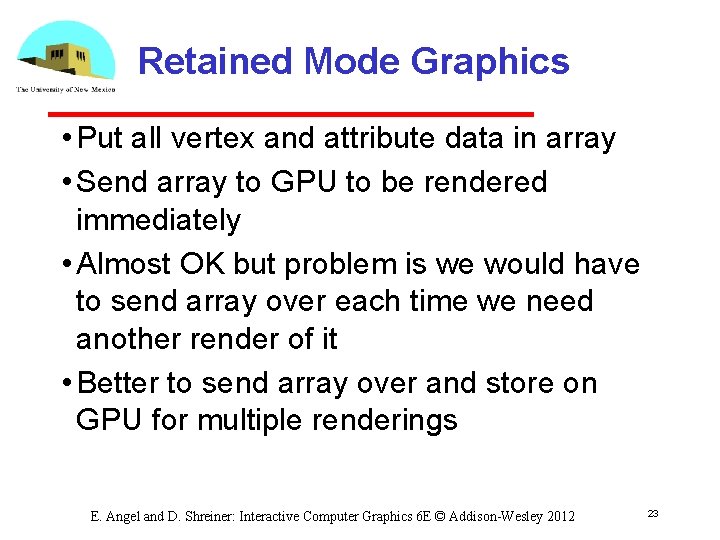
Retained Mode Graphics • Put all vertex and attribute data in array • Send array to GPU to be rendered immediately • Almost OK but problem is we would have to send array over each time we need another render of it • Better to send array over and store on GPU for multiple renderings E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 23
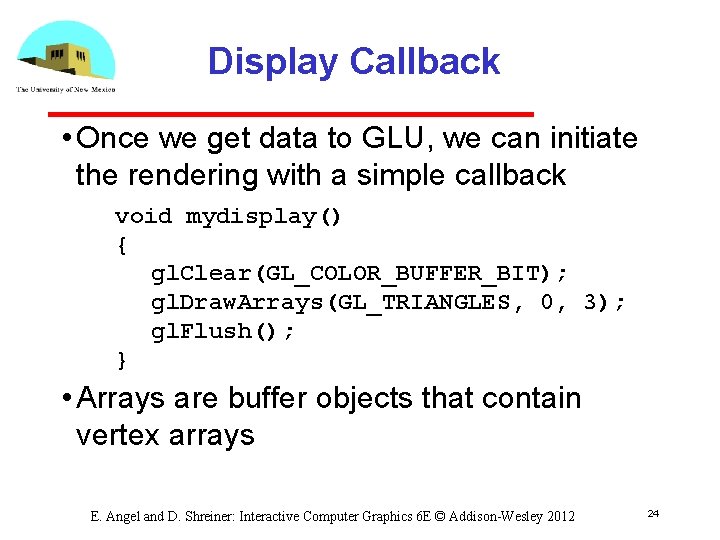
Display Callback • Once we get data to GLU, we can initiate the rendering with a simple callback void mydisplay() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Draw. Arrays(GL_TRIANGLES, 0, 3); gl. Flush(); } • Arrays are buffer objects that contain vertex arrays E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 24
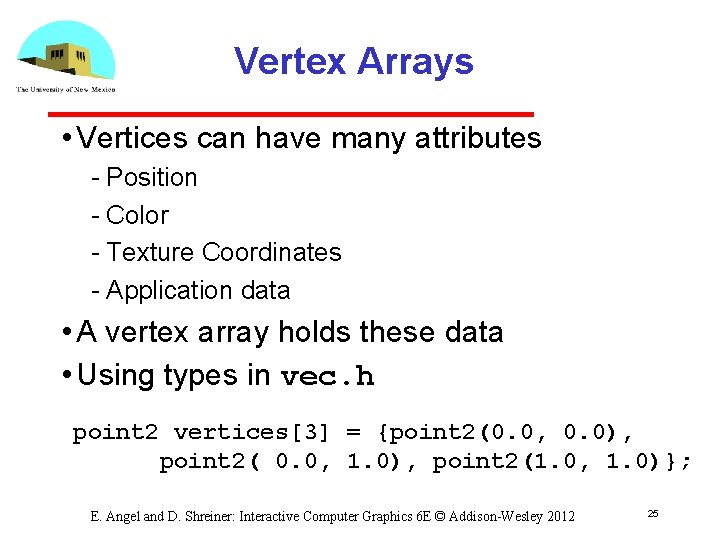
Vertex Arrays • Vertices can have many attributes Position Color Texture Coordinates Application data • A vertex array holds these data • Using types in vec. h point 2 vertices[3] = {point 2(0. 0, 0. 0), point 2( 0. 0, 1. 0), point 2(1. 0, 1. 0)}; E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 25
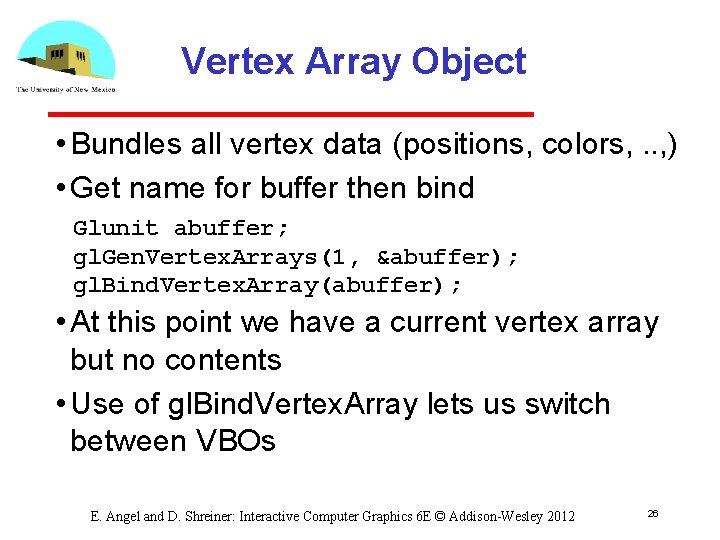
Vertex Array Object • Bundles all vertex data (positions, colors, . . , ) • Get name for buffer then bind Glunit abuffer; gl. Gen. Vertex. Arrays(1, &abuffer); gl. Bind. Vertex. Array(abuffer); • At this point we have a current vertex array but no contents • Use of gl. Bind. Vertex. Array lets us switch between VBOs E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 26
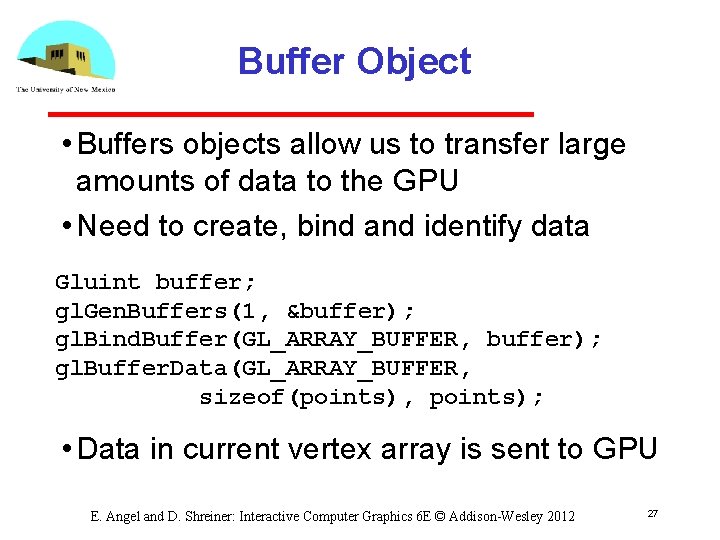
Buffer Object • Buffers objects allow us to transfer large amounts of data to the GPU • Need to create, bind and identify data Gluint buffer; gl. Gen. Buffers(1, &buffer); gl. Bind. Buffer(GL_ARRAY_BUFFER, buffer); gl. Buffer. Data(GL_ARRAY_BUFFER, sizeof(points), points); • Data in current vertex array is sent to GPU E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 27
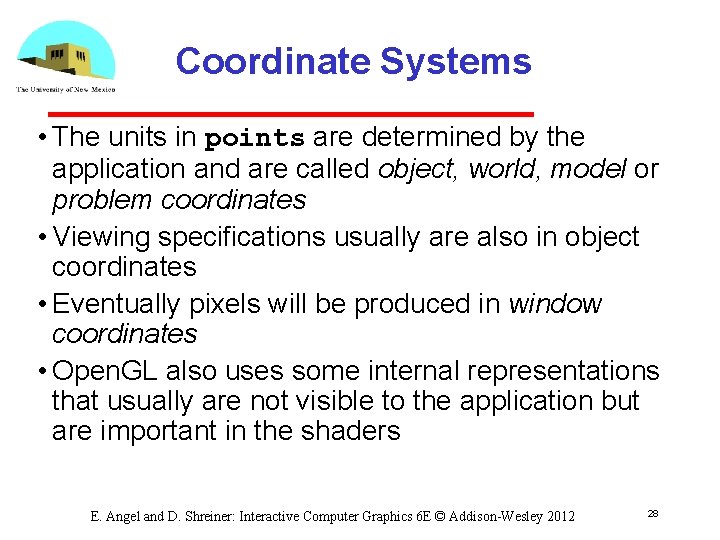
Coordinate Systems • The units in points are determined by the application and are called object, world, model or problem coordinates • Viewing specifications usually are also in object coordinates • Eventually pixels will be produced in window coordinates • Open. GL also uses some internal representations that usually are not visible to the application but are important in the shaders E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 28
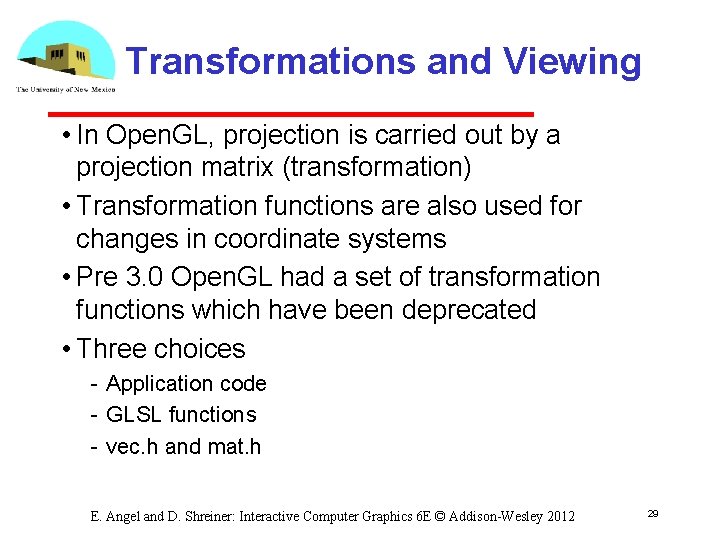
Transformations and Viewing • In Open. GL, projection is carried out by a projection matrix (transformation) • Transformation functions are also used for changes in coordinate systems • Pre 3. 0 Open. GL had a set of transformation functions which have been deprecated • Three choices Application code GLSL functions vec. h and mat. h E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 29
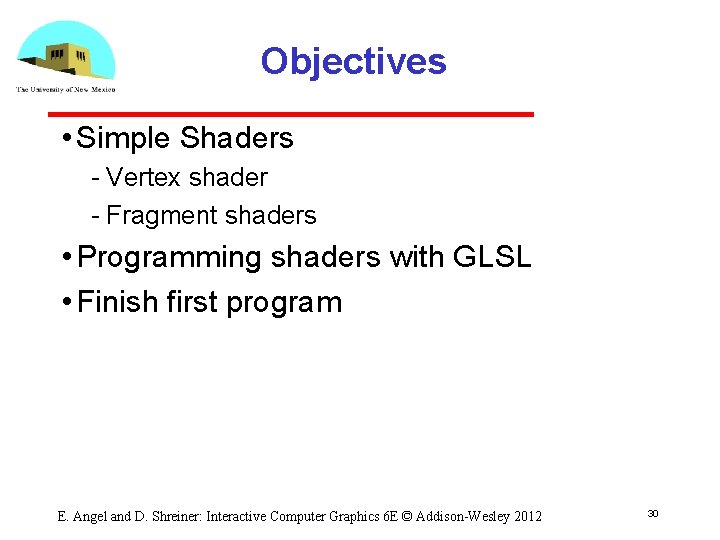
Objectives • Simple Shaders Vertex shader Fragment shaders • Programming shaders with GLSL • Finish first program E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 30
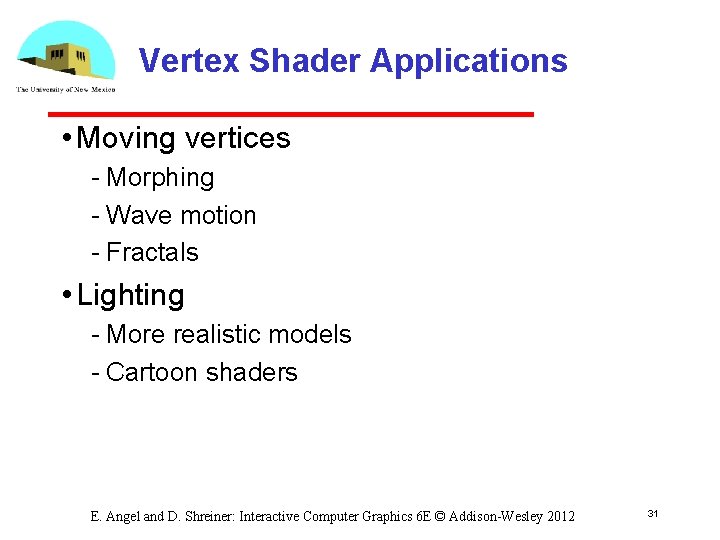
Vertex Shader Applications • Moving vertices Morphing Wave motion Fractals • Lighting More realistic models Cartoon shaders E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 31
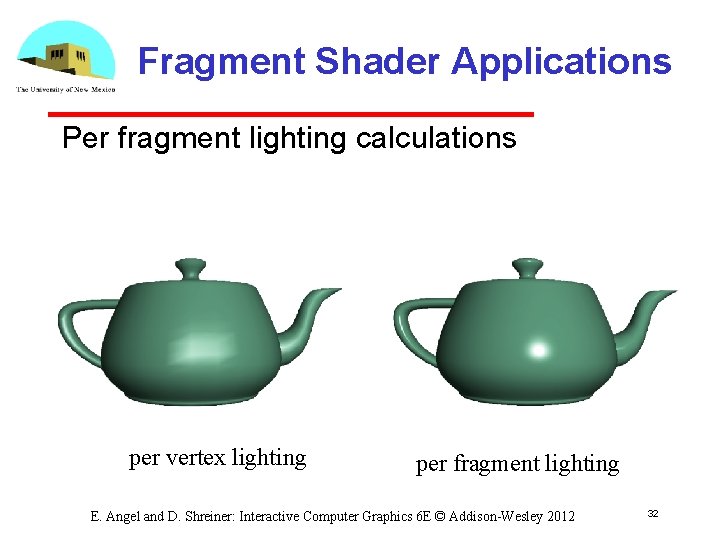
Fragment Shader Applications Per fragment lighting calculations per vertex lighting per fragment lighting E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 32
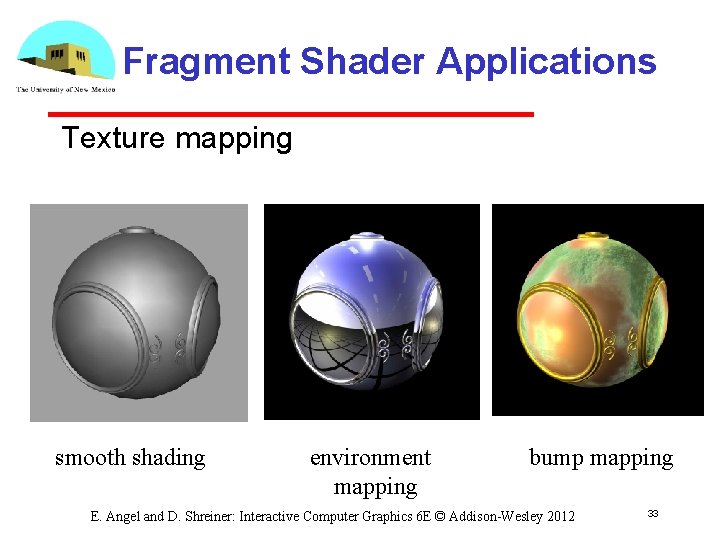
Fragment Shader Applications Texture mapping smooth shading environment mapping bump mapping E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 33
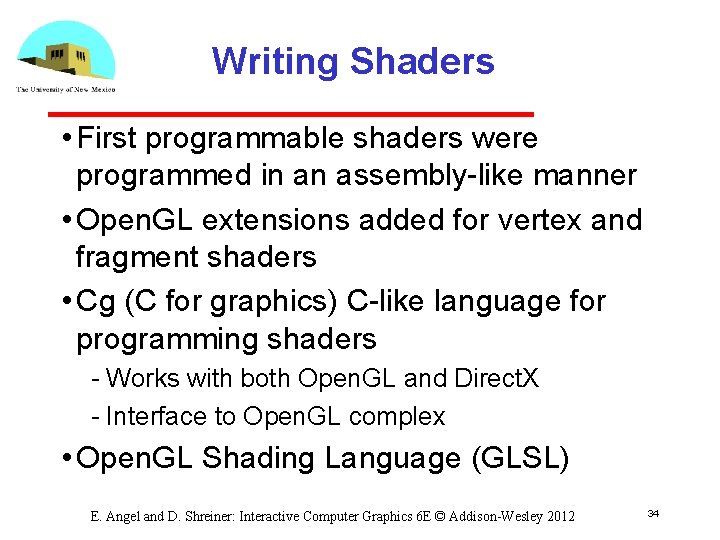
Writing Shaders • First programmable shaders were programmed in an assembly like manner • Open. GL extensions added for vertex and fragment shaders • Cg (C for graphics) C like language for programming shaders Works with both Open. GL and Direct. X Interface to Open. GL complex • Open. GL Shading Language (GLSL) E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 34
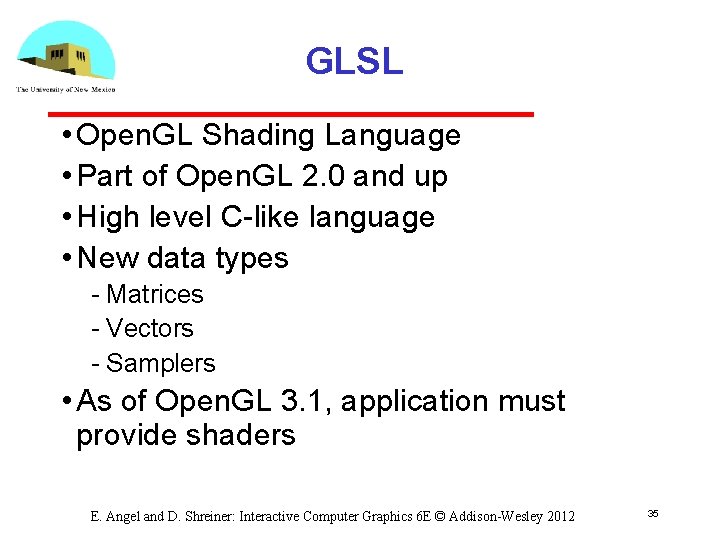
GLSL • Open. GL Shading Language • Part of Open. GL 2. 0 and up • High level C like language • New data types Matrices Vectors Samplers • As of Open. GL 3. 1, application must provide shaders E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 35
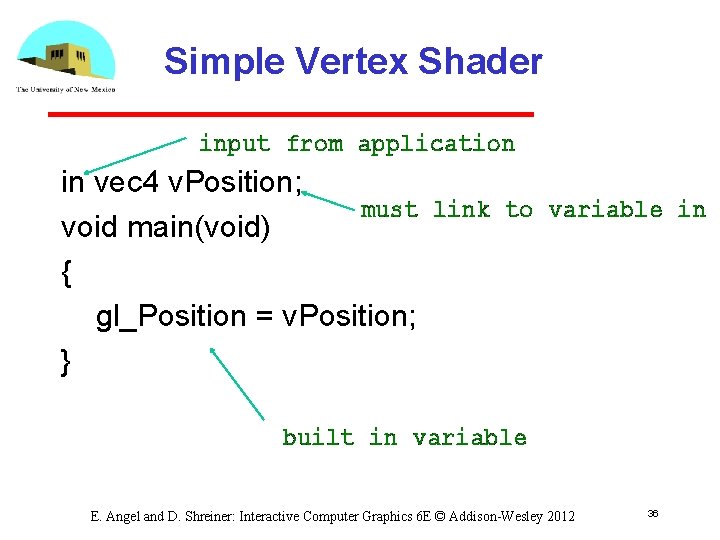
Simple Vertex Shader input from application in vec 4 v. Position; must void main(void) { gl_Position = v. Position; } link to variable in built in variable E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 36
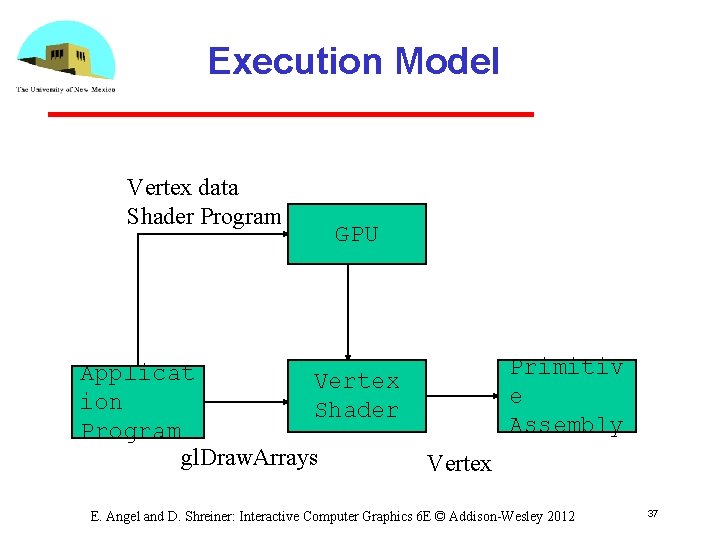
Execution Model Vertex data Shader Program GPU Applicat Vertex ion Shader Program gl. Draw. Arrays Primitiv e Assembly Vertex E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 37
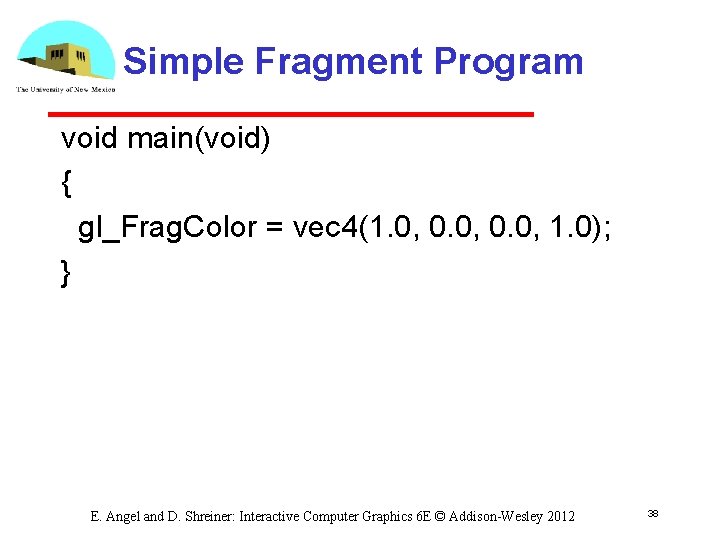
Simple Fragment Program void main(void) { gl_Frag. Color = vec 4(1. 0, 0. 0, 1. 0); } E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 38
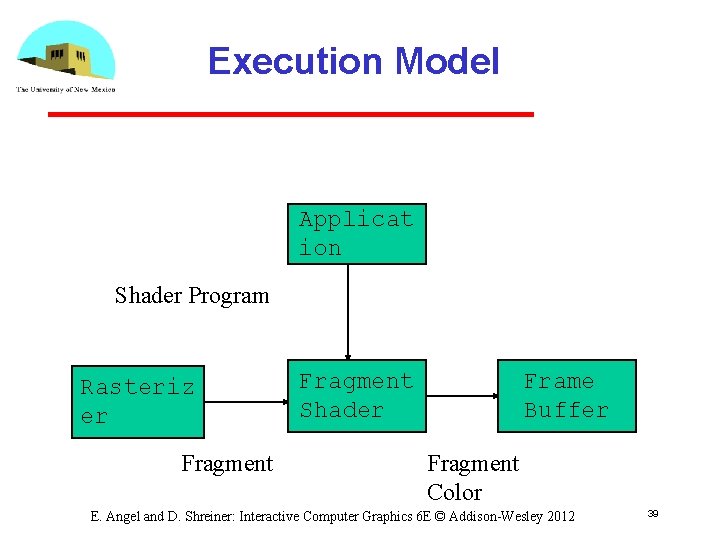
Execution Model Applicat ion Shader Program Rasteriz er Fragment Shader Frame Buffer Fragment Color E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 39
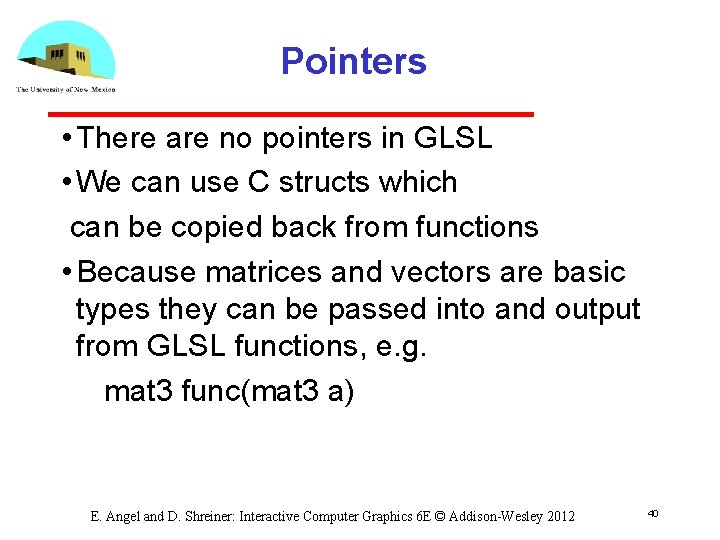
Pointers • There are no pointers in GLSL • We can use C structs which can be copied back from functions • Because matrices and vectors are basic types they can be passed into and output from GLSL functions, e. g. mat 3 func(mat 3 a) E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 40
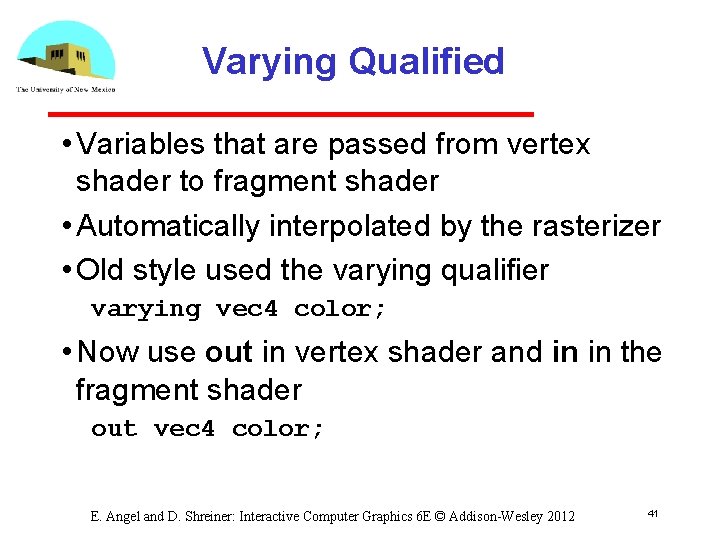
Varying Qualified • Variables that are passed from vertex shader to fragment shader • Automatically interpolated by the rasterizer • Old style used the varying qualifier varying vec 4 color; • Now use out in vertex shader and in in the fragment shader out vec 4 color; E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 41
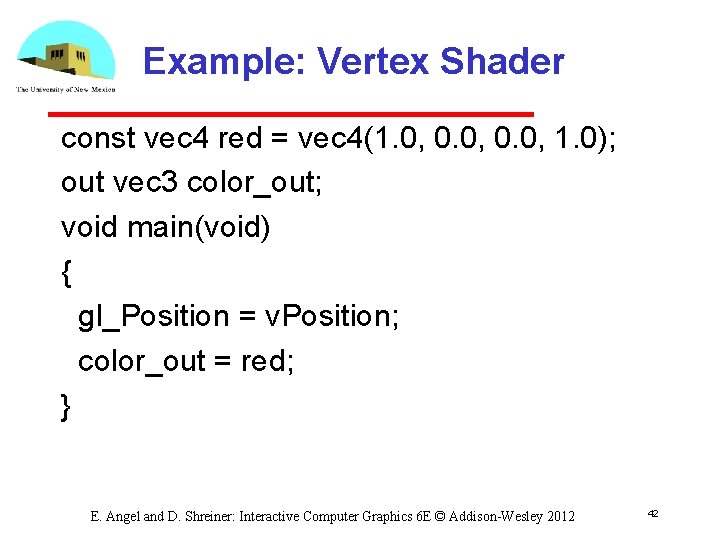
Example: Vertex Shader const vec 4 red = vec 4(1. 0, 0. 0, 1. 0); out vec 3 color_out; void main(void) { gl_Position = v. Position; color_out = red; } E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 42
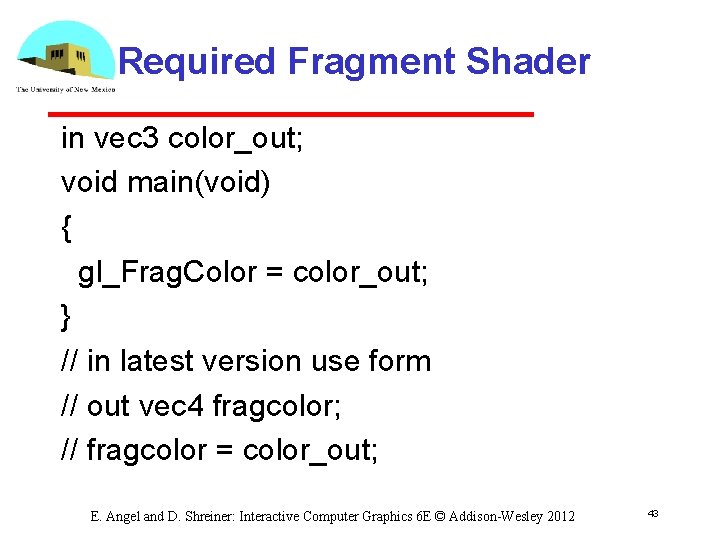
Required Fragment Shader in vec 3 color_out; void main(void) { gl_Frag. Color = color_out; } // in latest version use form // out vec 4 fragcolor; // fragcolor = color_out; E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 43
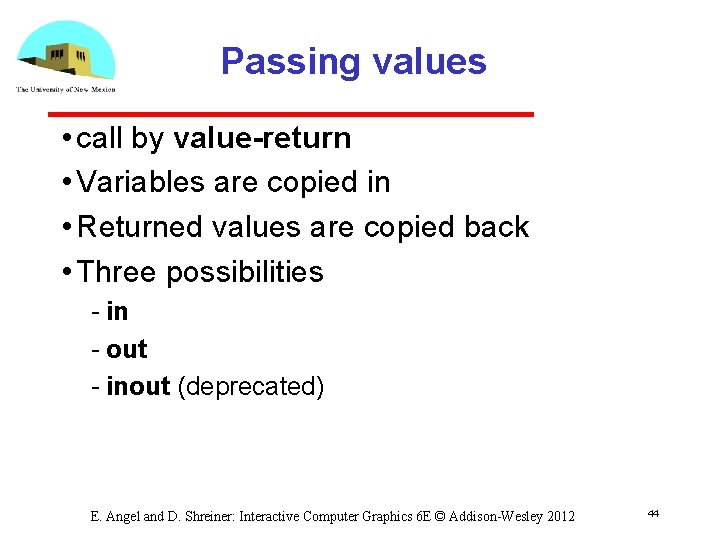
Passing values • call by value-return • Variables are copied in • Returned values are copied back • Three possibilities in out inout (deprecated) E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 44
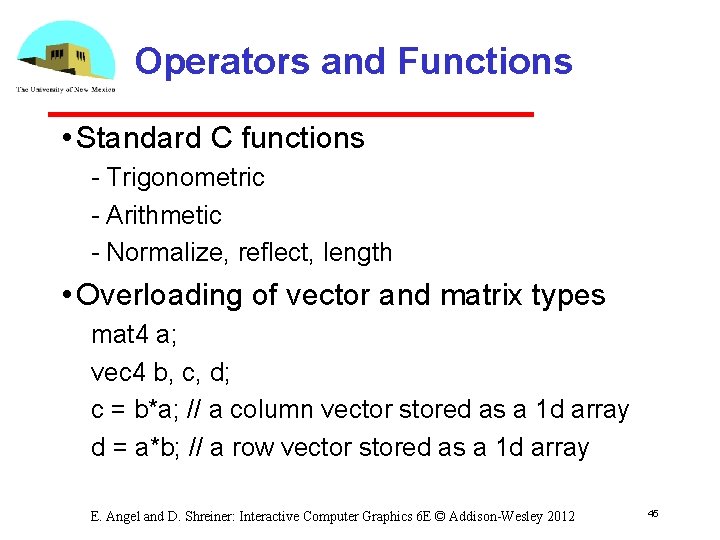
Operators and Functions • Standard C functions Trigonometric Arithmetic Normalize, reflect, length • Overloading of vector and matrix types mat 4 a; vec 4 b, c, d; c = b*a; // a column vector stored as a 1 d array d = a*b; // a row vector stored as a 1 d array E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 45
![Swizzling and Selection Can refer to array elements by element using or Swizzling and Selection • Can refer to array elements by element using [] or](https://slidetodoc.com/presentation_image_h2/cbb2db02238232a56707f7243e368920/image-46.jpg)
Swizzling and Selection • Can refer to array elements by element using [] or selection (. ) operator with x, y, z, w r, g, b, a s, t, p, q a[2], a. b, a. z, a. p are the same • Swizzling operator lets us manipulate components vec 4 a; a. yz = vec 2(1. 0, 2. 0); E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 46
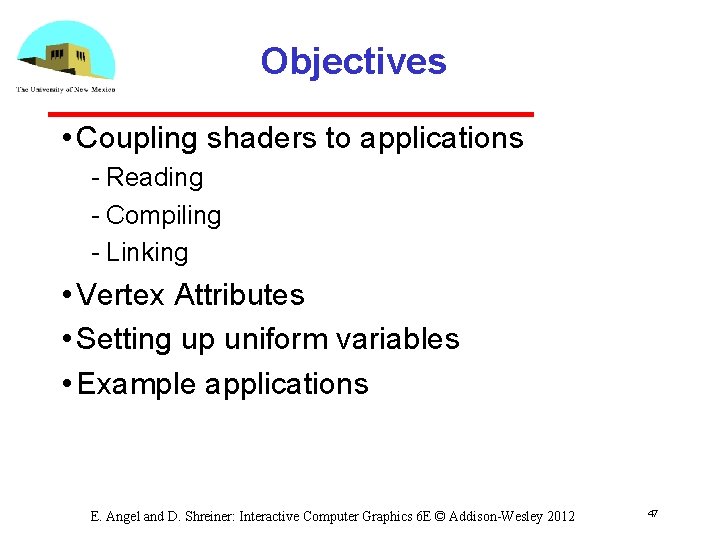
Objectives • Coupling shaders to applications Reading Compiling Linking • Vertex Attributes • Setting up uniform variables • Example applications E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 47
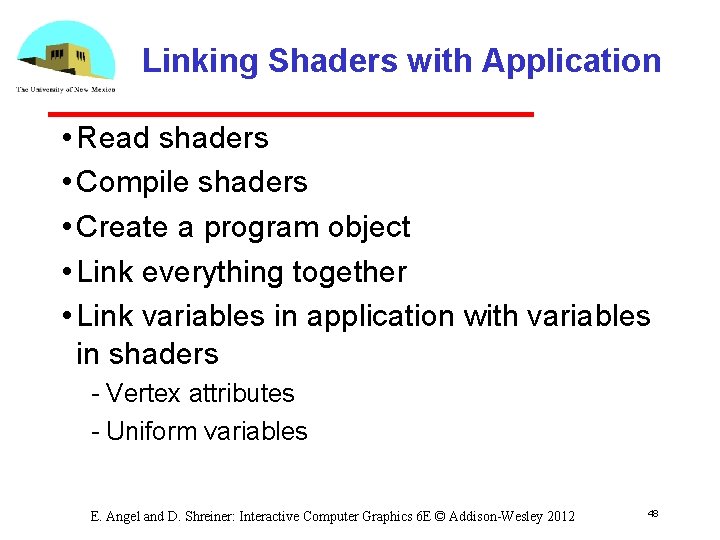
Linking Shaders with Application • Read shaders • Compile shaders • Create a program object • Link everything together • Link variables in application with variables in shaders Vertex attributes Uniform variables E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 48
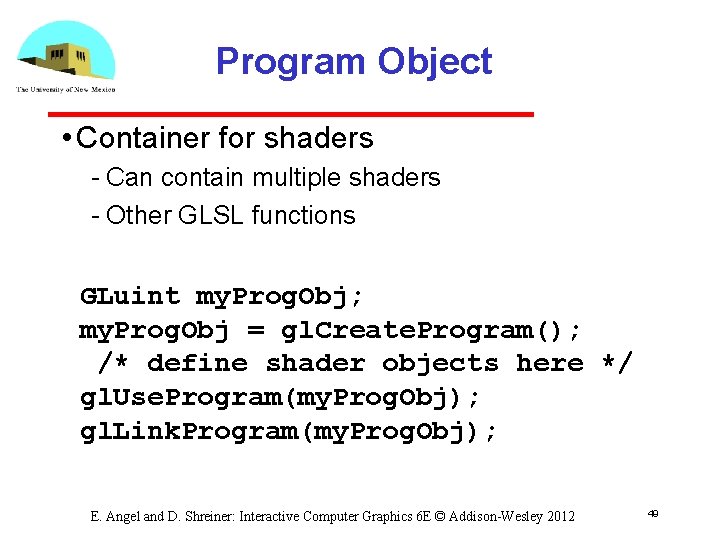
Program Object • Container for shaders Can contain multiple shaders Other GLSL functions GLuint my. Prog. Obj; my. Prog. Obj = gl. Create. Program(); /* define shader objects here */ gl. Use. Program(my. Prog. Obj); gl. Link. Program(my. Prog. Obj); E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 49
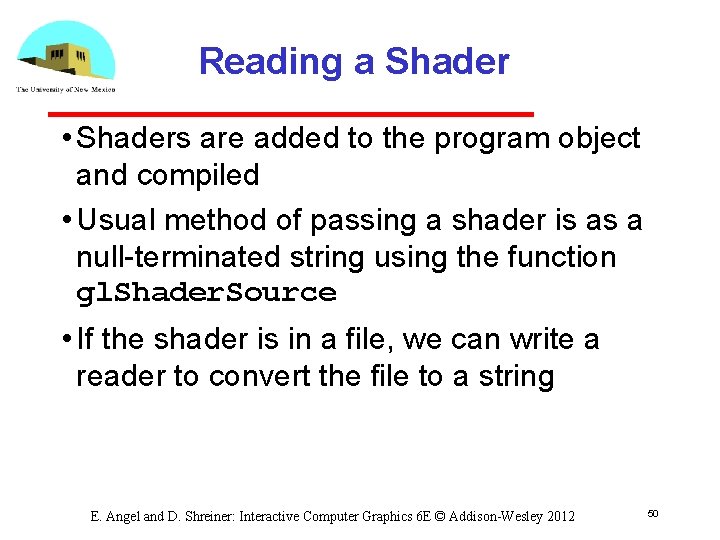
Reading a Shader • Shaders are added to the program object and compiled • Usual method of passing a shader is as a null terminated string using the function gl. Shader. Source • If the shader is in a file, we can write a reader to convert the file to a string E. Angel and D. Shreiner: Interactive Computer Graphics 6 E © Addison-Wesley 2012 50
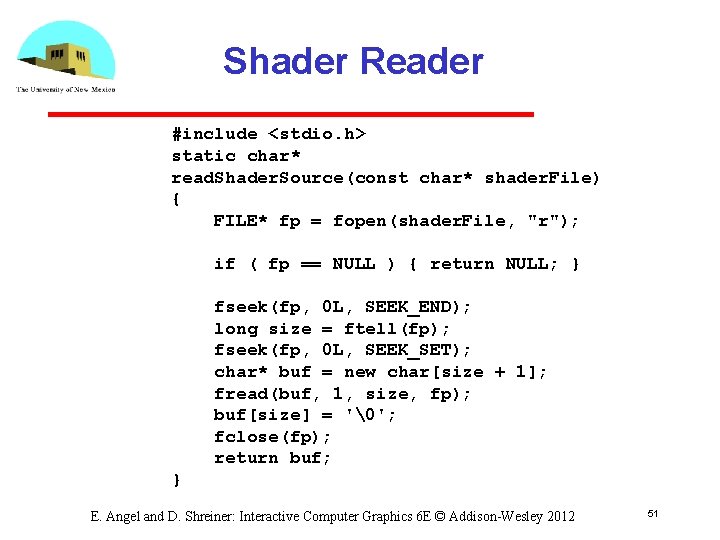
Shader Reader #include <stdio. h> static char* read. Shader. Source(const char* shader. File) { FILE* fp = fopen(shader. File, "r"); if ( fp == NULL ) { return NULL; } fseek(fp, 0 L, SEEK_END); long size = ftell(fp); fseek(fp, 0 L, SEEK_SET); char* buf = new char[size + 1]; fread(buf, 1, size, fp); buf[size] = '