Programming Techniques String Manipulation jamiedrfrostmaths com www drfrostmaths
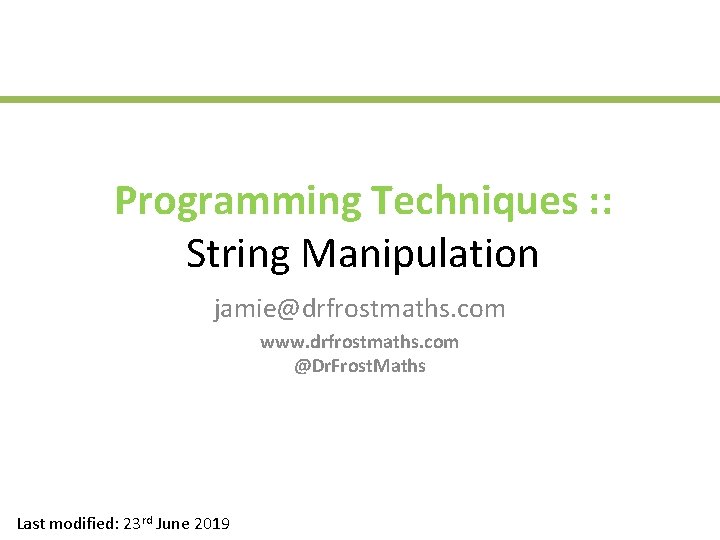
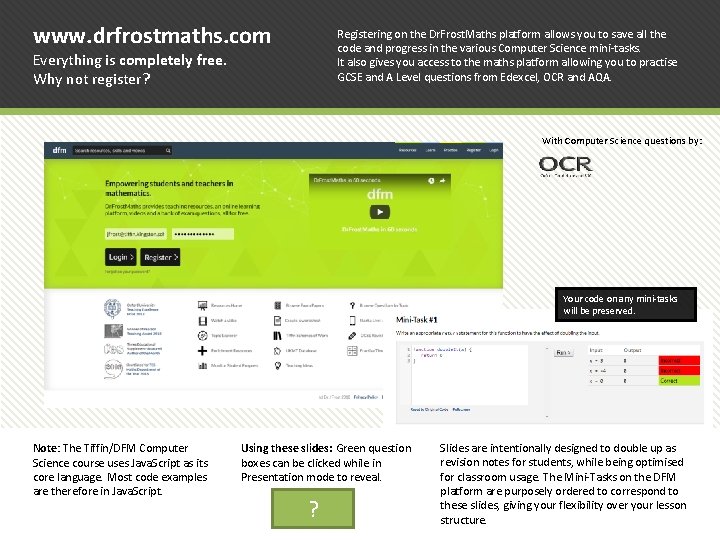
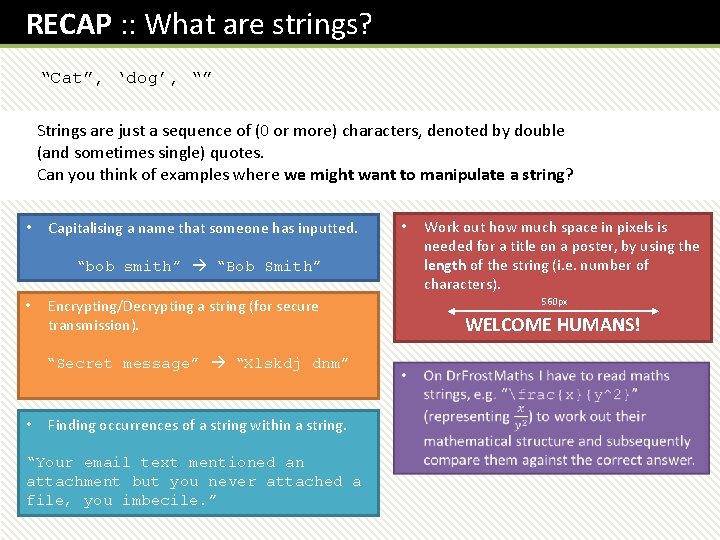
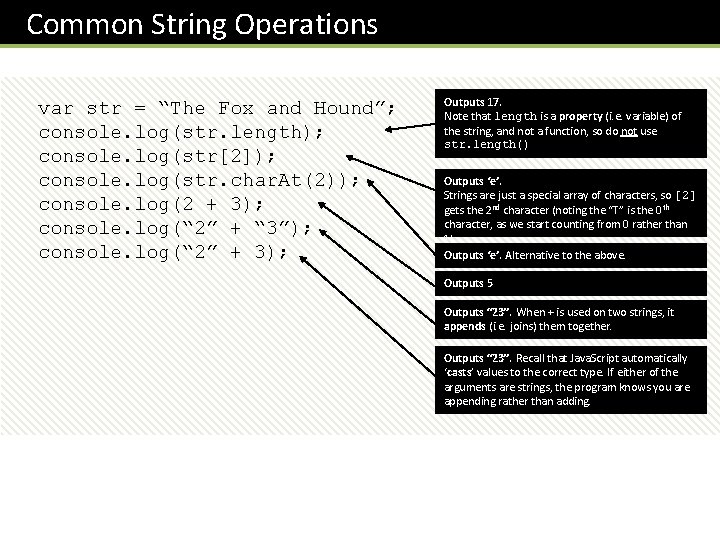
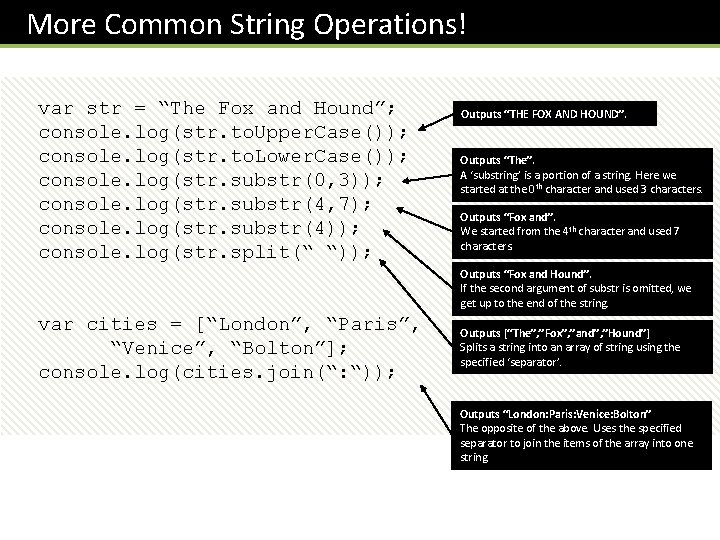
![Test Your Understanding “Dr Frost Maths”. substr(4, 3) “ros” ? “Bob” + “Robert”[1] “Bobo” Test Your Understanding “Dr Frost Maths”. substr(4, 3) “ros” ? “Bob” + “Robert”[1] “Bobo”](https://slidetodoc.com/presentation_image/a2c2c3994db1b4d6b9b815a42b1a4dd9/image-6.jpg)
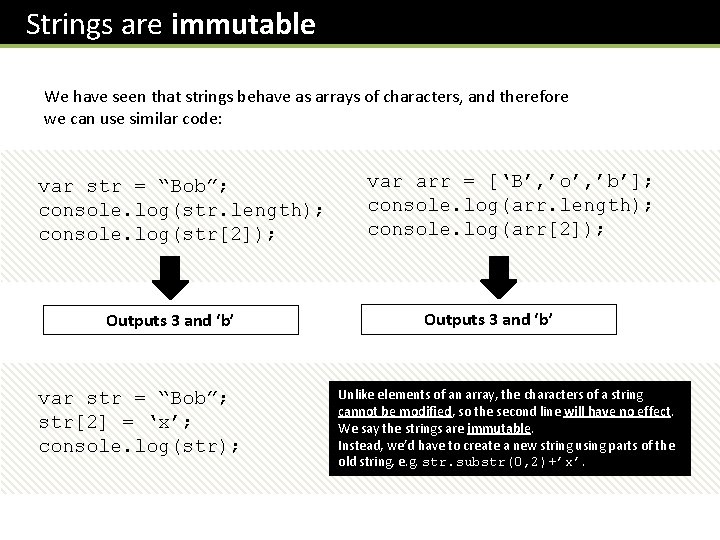
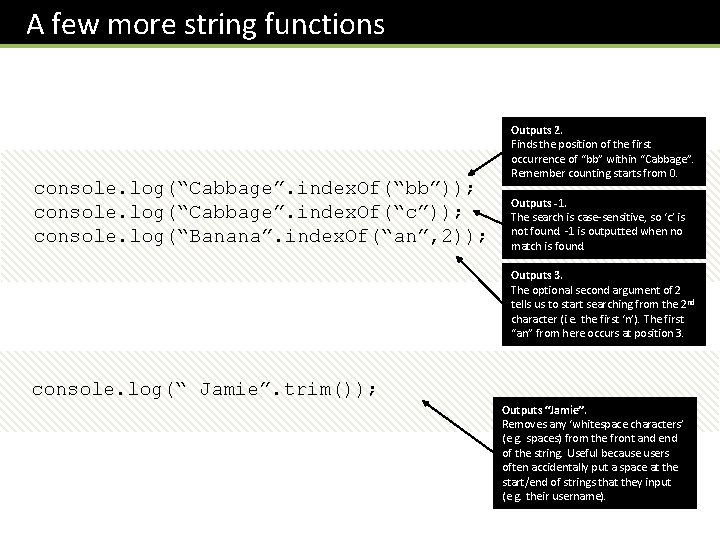
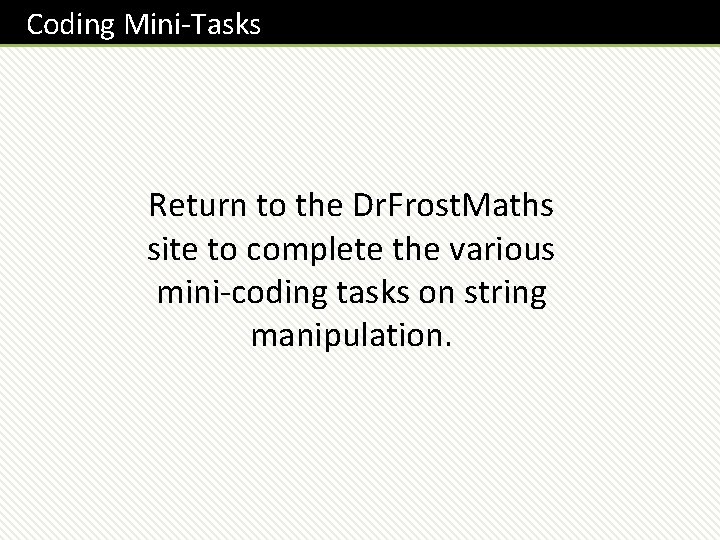
- Slides: 9
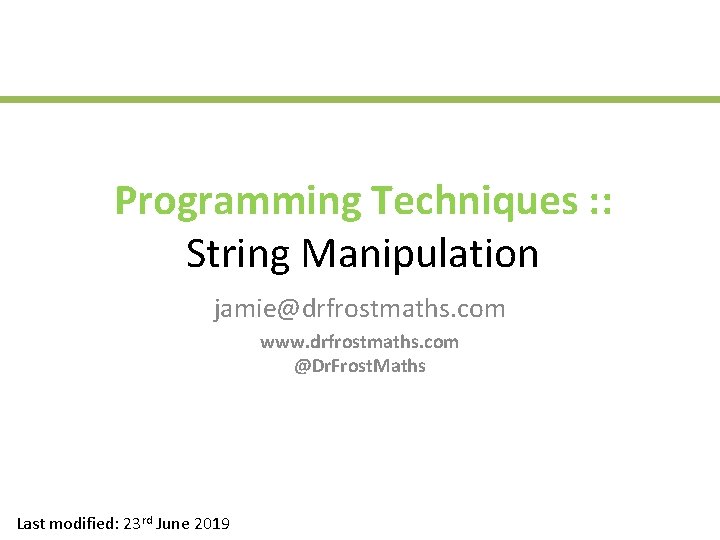
Programming Techniques : : String Manipulation jamie@drfrostmaths. com www. drfrostmaths. com @Dr. Frost. Maths Last modified: 23 rd June 2019
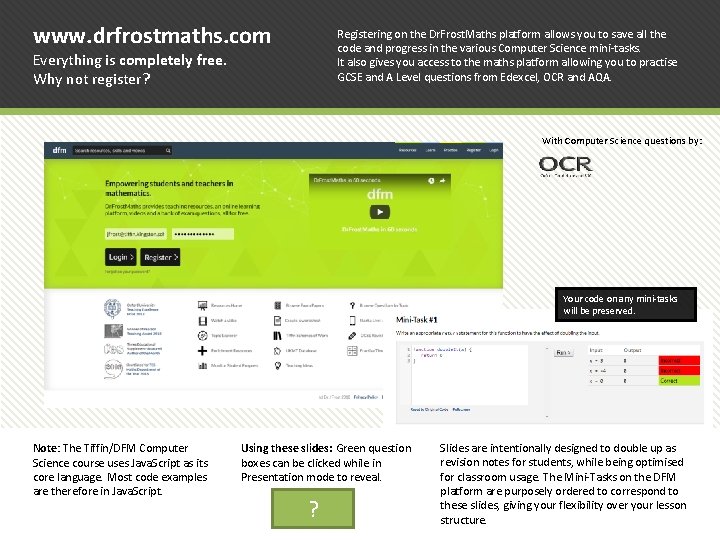
www. drfrostmaths. com Registering on the Dr. Frost. Maths platform allows you to save all the code and progress in the various Computer Science mini-tasks. It also gives you access to the maths platform allowing you to practise GCSE and A Level questions from Edexcel, OCR and AQA. Everything is completely free. Why not register? With Computer Science questions by: Your code on any mini-tasks will be preserved. Note: The Tiffin/DFM Computer Science course uses Java. Script as its core language. Most code examples are therefore in Java. Script. Using these slides: Green question boxes can be clicked while in Presentation mode to reveal. ? Slides are intentionally designed to double up as revision notes for students, while being optimised for classroom usage. The Mini-Tasks on the DFM platform are purposely ordered to correspond to these slides, giving your flexibility over your lesson structure.
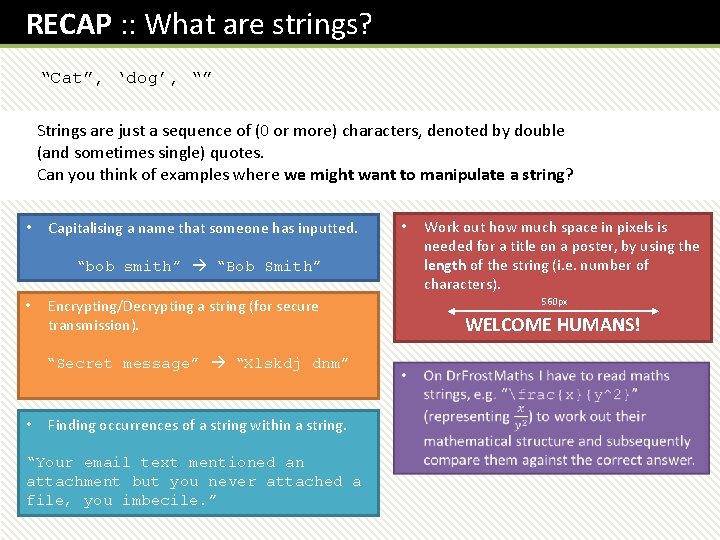
RECAP : : What are strings? “Cat”, ‘dog’, “” Strings are just a sequence of (0 or more) characters, denoted by double (and sometimes single) quotes. Can you think of examples where we might want to manipulate a string? • Capitalising a name that someone has inputted. • “bob smith” “Bob Smith” • • 560 px Encrypting/Decrypting a string (for secure transmission). “Secret message” “Xlskdj dnm” Finding occurrences of a string within a string. “Your email text mentioned an attachment but you never attached a file, you imbecile. ” Work out how much space in pixels is needed for a title on a poster, by using the length of the string (i. e. number of characters). WELCOME HUMANS!
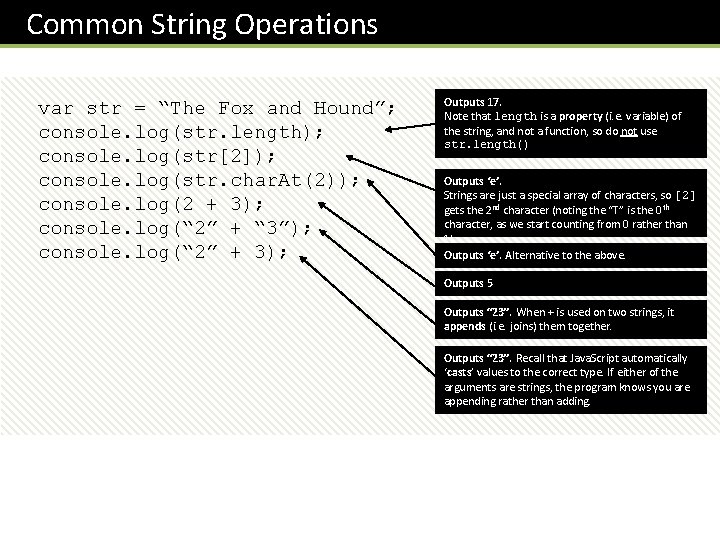
Common String Operations var str = “The Fox and Hound”; console. log(str. length); console. log(str[2]); console. log(str. char. At(2)); console. log(2 + 3); console. log(“ 2” + “ 3”); console. log(“ 2” + 3); Outputs 17. Note that length is a property (i. e. variable) of the string, and not a function, so do not use str. length() Outputs ‘e’. Strings are just a special array of characters, so [2] gets the 2 nd character (noting the “T” is the 0 th character, as we start counting from 0 rather than 1) Outputs ‘e’. Alternative to the above. Outputs 5 Outputs “ 23”. When + is used on two strings, it appends (i. e. joins) them together. Outputs “ 23”. Recall that Java. Script automatically ‘casts’ values to the correct type. If either of the arguments are strings, the program knows you are appending rather than adding.
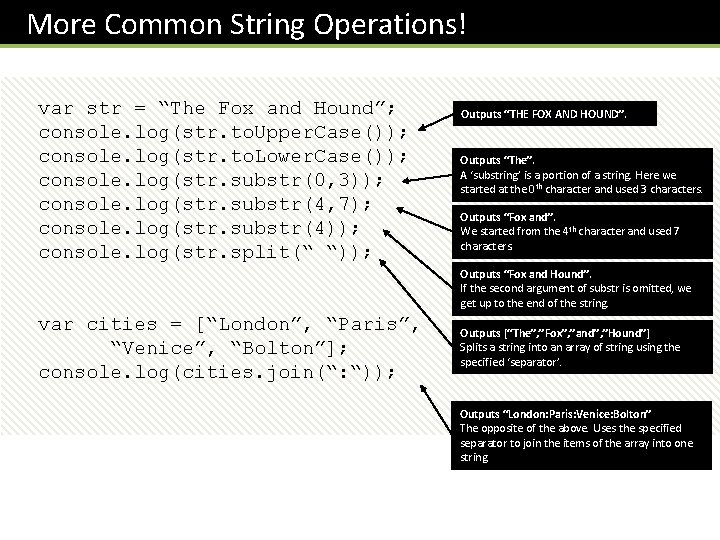
More Common String Operations! var str = “The Fox and Hound”; console. log(str. to. Upper. Case()); console. log(str. to. Lower. Case()); console. log(str. substr(0, 3)); console. log(str. substr(4, 7); console. log(str. substr(4)); console. log(str. split(“ “)); Outputs “THE FOX AND HOUND”. Outputs “The”. A ‘substring’ is a portion of a string. Here we started at the 0 th character and used 3 characters. Outputs “Fox and”. We started from the 4 th character and used 7 characters. Outputs “Fox and Hound”. If the second argument of substr is omitted, we get up to the end of the string. var cities = [“London”, “Paris”, “Venice”, “Bolton”]; console. log(cities. join(“: “)); Outputs [“The”, ”Fox”, ”and”, ”Hound”] Splits a string into an array of string using the specified ‘separator’. Outputs “London: Paris: Venice: Bolton” The opposite of the above. Uses the specified separator to join the items of the array into one string.
![Test Your Understanding Dr Frost Maths substr4 3 ros Bob Robert1 Bobo Test Your Understanding “Dr Frost Maths”. substr(4, 3) “ros” ? “Bob” + “Robert”[1] “Bobo”](https://slidetodoc.com/presentation_image/a2c2c3994db1b4d6b9b815a42b1a4dd9/image-6.jpg)
Test Your Understanding “Dr Frost Maths”. substr(4, 3) “ros” ? “Bob” + “Robert”[1] “Bobo” ? “Dr Frost Maths”. substr(0, 4). to. Upper. Case() “DR ? F” Can you write a program which inputs a string, and then capitalises the first letter, but with the rest of the string lower case, e. g. “a. Bc. De. F” “Abcdef” var str = prompt(“Write something”); console. log( str[0]. to. Upper. Case() ? + str. substr(1). to. Lower. Case());
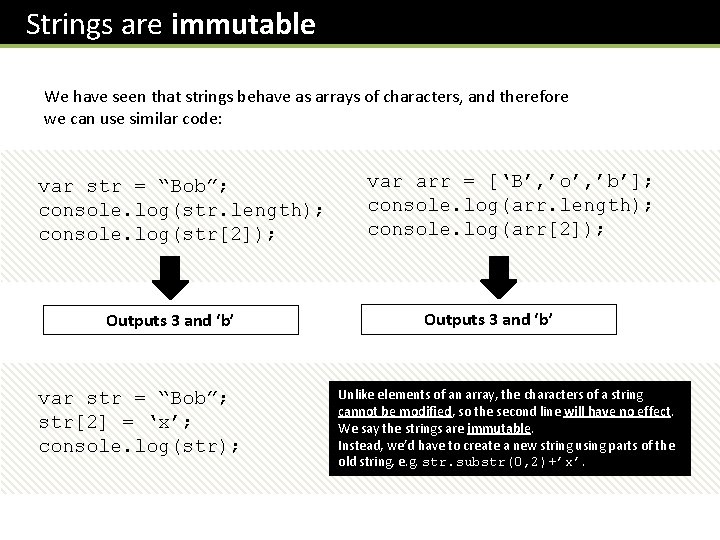
Strings are immutable We have seen that strings behave as arrays of characters, and therefore we can use similar code: var str = “Bob”; console. log(str. length); console. log(str[2]); Outputs 3 and ‘b’ var str = “Bob”; str[2] = ‘x’; console. log(str); var arr = [‘B’, ’o’, ’b’]; console. log(arr. length); console. log(arr[2]); Outputs 3 and ‘b’ Unlike elements of an array, the characters of a string cannot be modified, so the second line will have no effect. We say the strings are immutable. Instead, we’d have to create a new string using parts of the old string, e. g. str. substr(0, 2)+’x’.
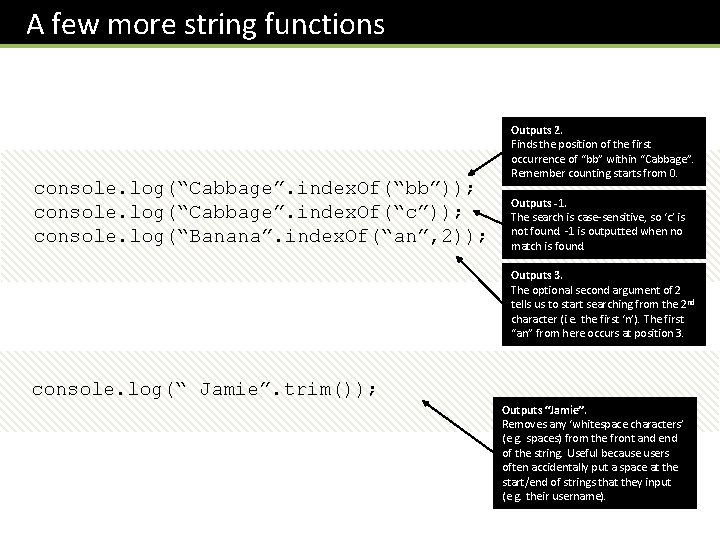
A few more string functions console. log(“Cabbage”. index. Of(“bb”)); console. log(“Cabbage”. index. Of(“c”)); console. log(“Banana”. index. Of(“an”, 2)); Outputs 2. Finds the position of the first occurrence of “bb” within “Cabbage”. Remember counting starts from 0. Outputs -1. The search is case-sensitive, so ‘c’ is not found. -1 is outputted when no match is found. Outputs 3. The optional second argument of 2 tells us to start searching from the 2 nd character (i. e. the first ‘n’). The first “an” from here occurs at position 3. console. log(“ Jamie”. trim()); Outputs “Jamie”. Removes any ‘whitespace characters’ (e. g. spaces) from the front and end of the string. Useful because users often accidentally put a space at the start/end of strings that they input (e. g. their username).
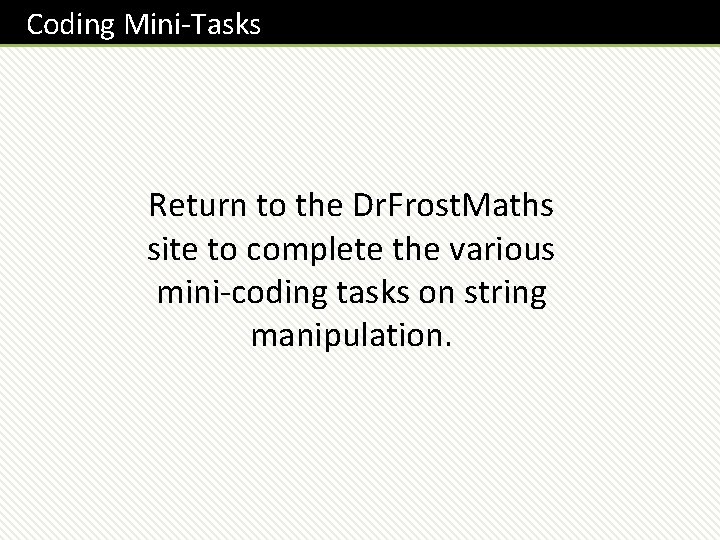
Coding Mini-Tasks Return to the Dr. Frost. Maths site to complete the various mini-coding tasks on string manipulation.