Programming Techniques File Handling jamiedrfrostmaths com www drfrostmaths
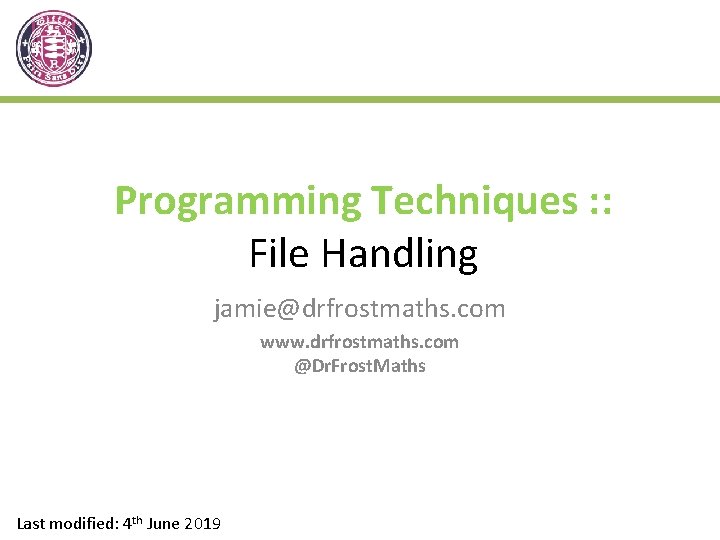
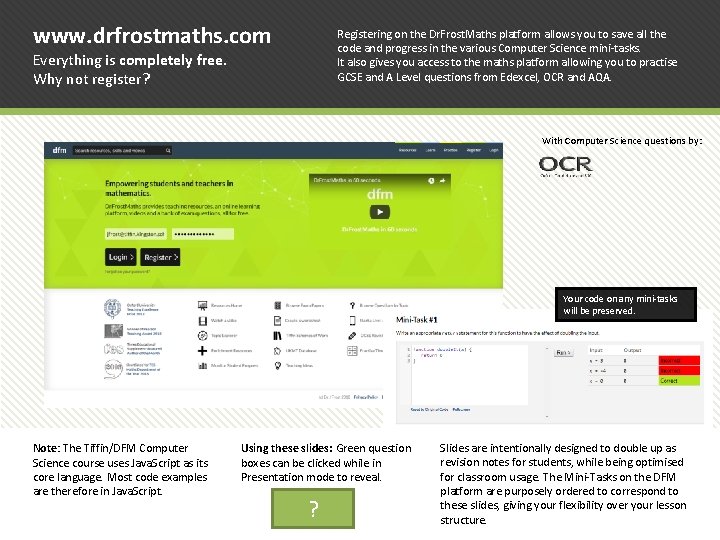
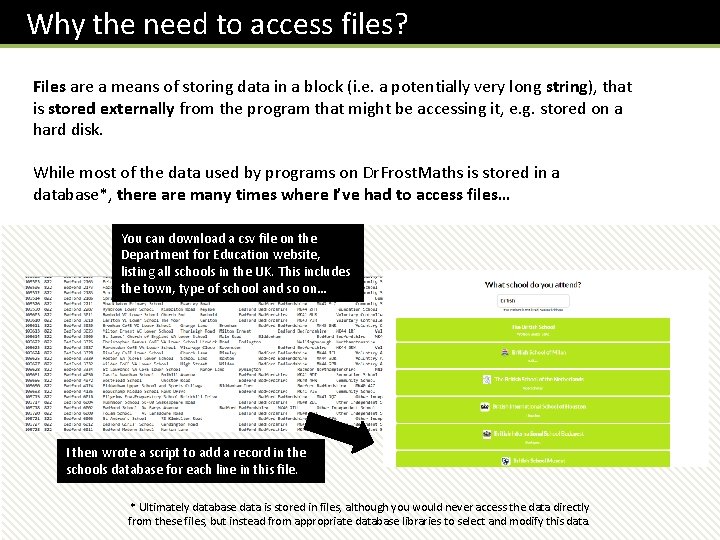
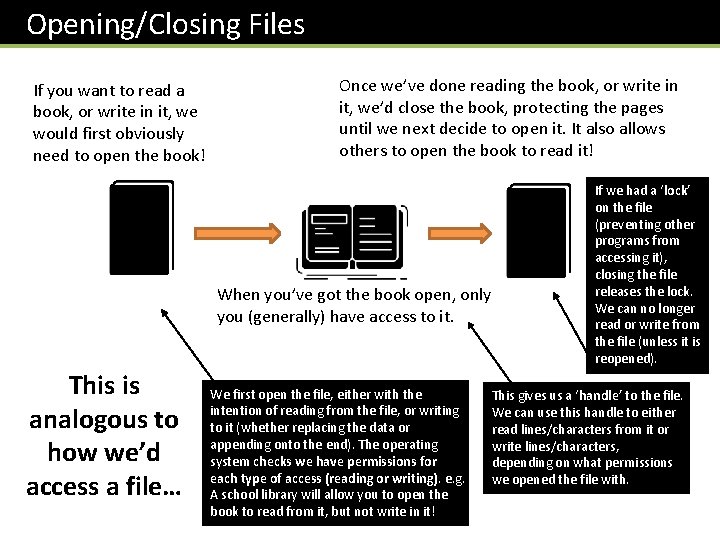
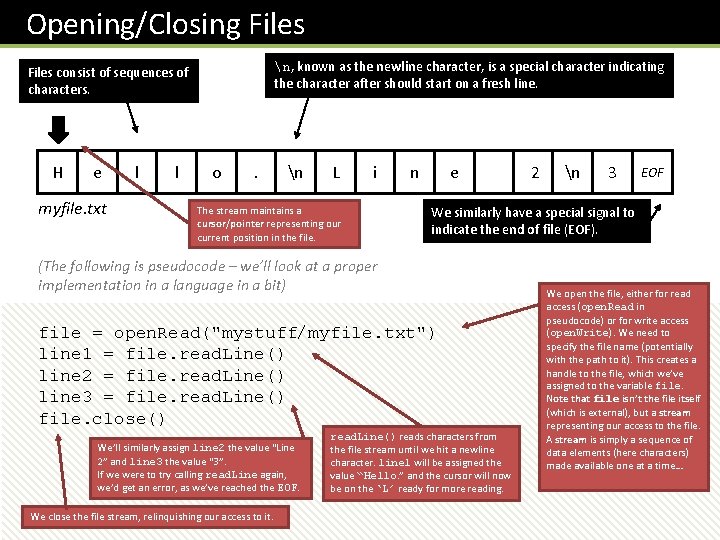
![Further Examples top 3 = ["Ashwin", "Alice", "Alex"] file = open. Write("rankings. txt") for Further Examples top 3 = ["Ashwin", "Alice", "Alex"] file = open. Write("rankings. txt") for](https://slidetodoc.com/presentation_image/5e481da7204e16430b3cf16c48ba605a/image-6.jpg)
![Further Examples countries. txt array countries[] file = open. Read("countries. txt") while NOT file. Further Examples countries. txt array countries[] file = open. Read("countries. txt") while NOT file.](https://slidetodoc.com/presentation_image/5e481da7204e16430b3cf16c48ba605a/image-7.jpg)
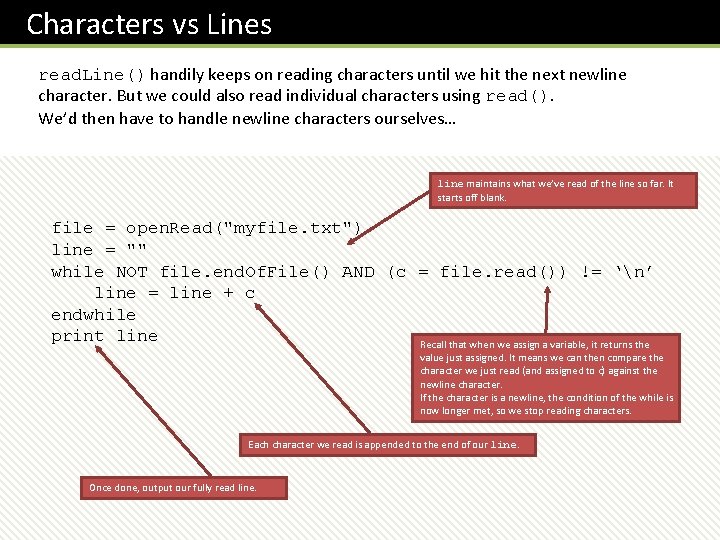
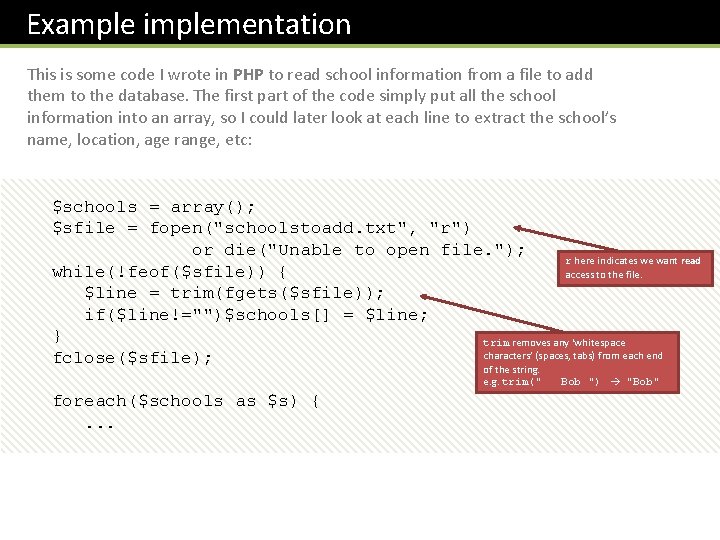
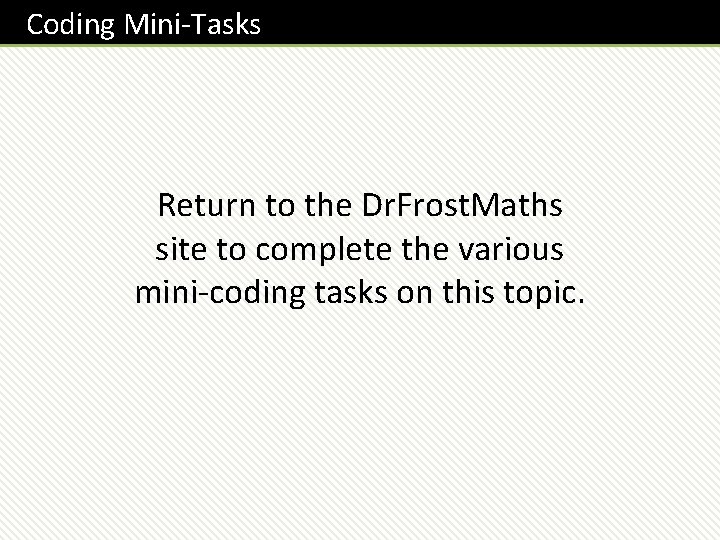
- Slides: 10
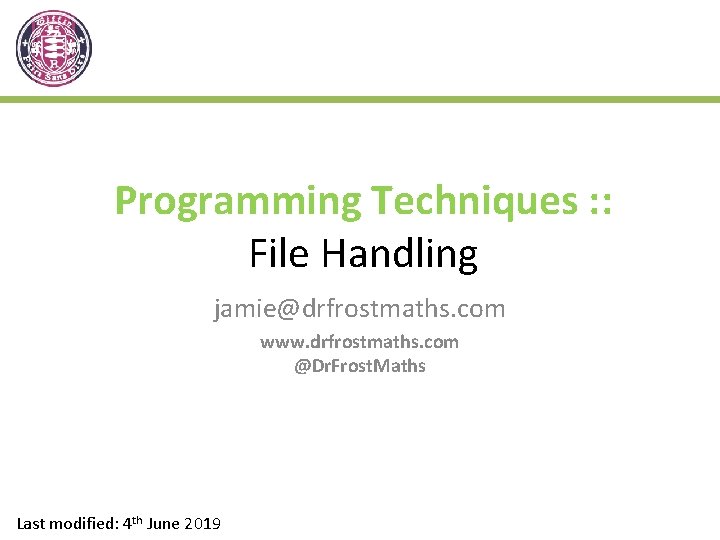
Programming Techniques : : File Handling jamie@drfrostmaths. com www. drfrostmaths. com @Dr. Frost. Maths Last modified: 4 th June 2019
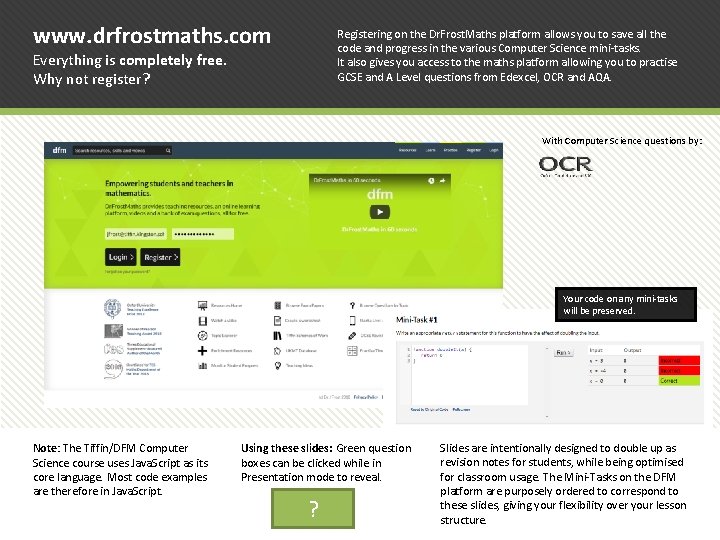
www. drfrostmaths. com Registering on the Dr. Frost. Maths platform allows you to save all the code and progress in the various Computer Science mini-tasks. It also gives you access to the maths platform allowing you to practise GCSE and A Level questions from Edexcel, OCR and AQA. Everything is completely free. Why not register? With Computer Science questions by: Your code on any mini-tasks will be preserved. Note: The Tiffin/DFM Computer Science course uses Java. Script as its core language. Most code examples are therefore in Java. Script. Using these slides: Green question boxes can be clicked while in Presentation mode to reveal. ? Slides are intentionally designed to double up as revision notes for students, while being optimised for classroom usage. The Mini-Tasks on the DFM platform are purposely ordered to correspond to these slides, giving your flexibility over your lesson structure.
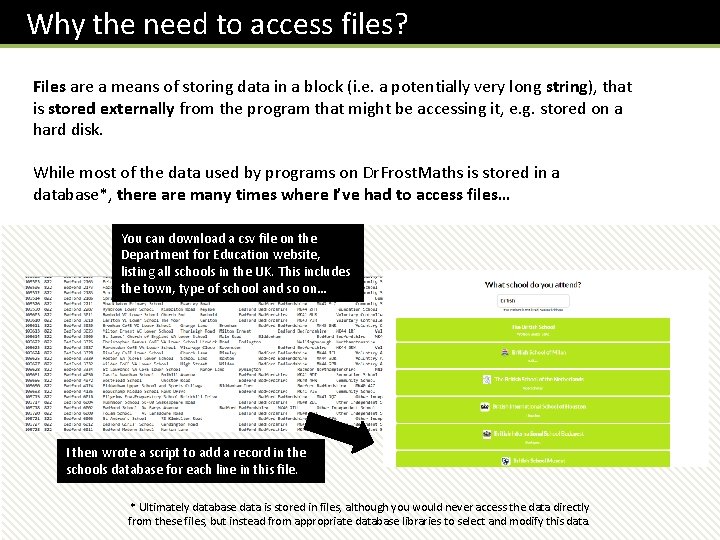
Why the need to access files? Files are a means of storing data in a block (i. e. a potentially very long string), that is stored externally from the program that might be accessing it, e. g. stored on a hard disk. While most of the data used by programs on Dr. Frost. Maths is stored in a database*, there are many times where I’ve had to access files… You can download a csv file on the Department for Education website, listing all schools in the UK. This includes the town, type of school and so on… I then wrote a script to add a record in the schools database for each line in this file. * Ultimately database data is stored in files, although you would never access the data directly from these files, but instead from appropriate database libraries to select and modify this data.
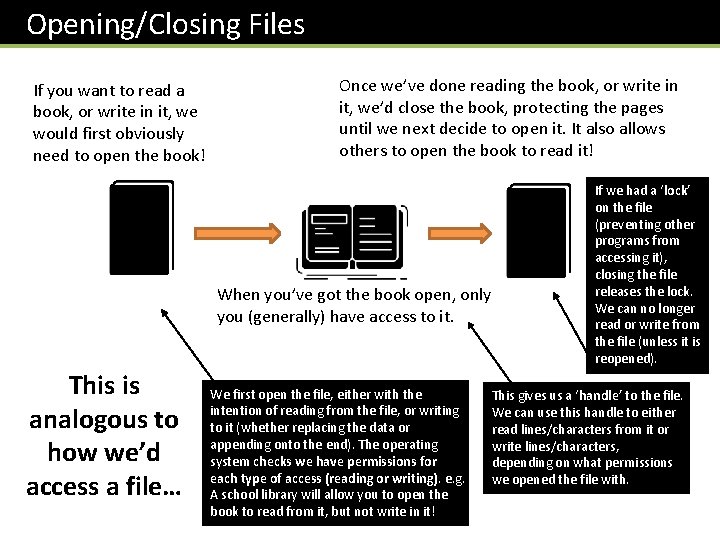
Opening/Closing Files If you want to read a book, or write in it, we would first obviously need to open the book! Once we’ve done reading the book, or write in it, we’d close the book, protecting the pages until we next decide to open it. It also allows others to open the book to read it! When you’ve got the book open, only you (generally) have access to it. This is analogous to how we’d access a file… We first open the file, either with the intention of reading from the file, or writing to it (whether replacing the data or appending onto the end). The operating system checks we have permissions for each type of access (reading or writing). e. g. A school library will allow you to open the book to read from it, but not write in it! If we had a ‘lock’ on the file (preventing other programs from accessing it), closing the file releases the lock. We can no longer read or write from the file (unless it is reopened). This gives us a ‘handle’ to the file. We can use this handle to either read lines/characters from it or write lines/characters, depending on what permissions we opened the file with.
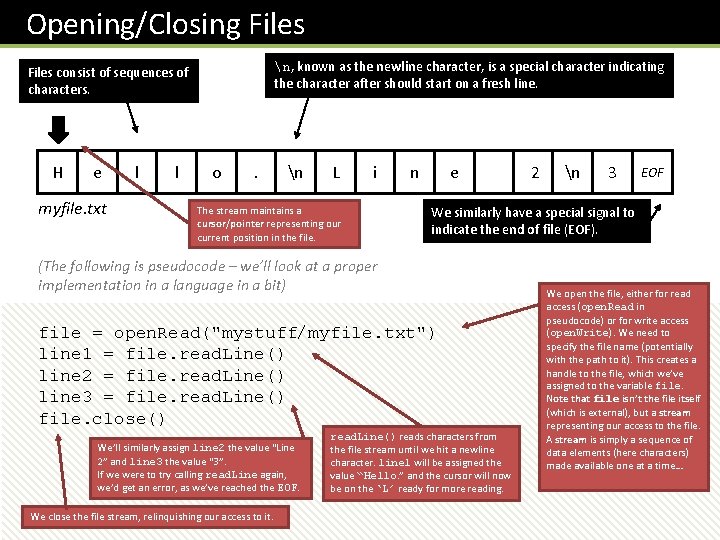
Opening/Closing Files n, known as the newline character, is a special character indicating the character after should start on a fresh line. Files consist of sequences of characters. H e myfile. txt l l o . n L i The stream maintains a cursor/pointer representing our current position in the file. n e file = open. Read("mystuff/myfile. txt") line 1 = file. read. Line() line 2 = file. read. Line() line 3 = file. read. Line() file. close() We close the file stream, relinquishing our access to it. n 3 EOF We similarly have a special signal to indicate the end of file (EOF). (The following is pseudocode – we’ll look at a proper implementation in a language in a bit) We’ll similarly assign line 2 the value “Line 2” and line 3 the value “ 3”. If we were to try calling read. Line again, we’d get an error, as we’ve reached the EOF. 2 read. Line() reads characters from the file stream until we hit a newline character. line 1 will be assigned the value “Hello. ” and the cursor will now be on the ‘L’ ready for more reading. We open the file, either for read access (open. Read in pseudocode) or for write access (open. Write). We need to specify the file name (potentially with the path to it). This creates a handle to the file, which we’ve assigned to the variable file. Note that file isn’t the file itself (which is external), but a stream representing our access to the file. A stream is simply a sequence of data elements (here characters) made available one at a time…
![Further Examples top 3 Ashwin Alice Alex file open Writerankings txt for Further Examples top 3 = ["Ashwin", "Alice", "Alex"] file = open. Write("rankings. txt") for](https://slidetodoc.com/presentation_image/5e481da7204e16430b3cf16c48ba605a/image-6.jpg)
Further Examples top 3 = ["Ashwin", "Alice", "Alex"] file = open. Write("rankings. txt") for i = 0 to 2 file. write. Line(str(i+1) + ": " + top 3[i]) next i file. close() 1: Ashwin 2: Alice ? New 3: Alexcontents of file This time we want to write to a file. We have the top 3 players in a game and want to output their names with their position.
![Further Examples countries txt array countries file open Readcountries txt while NOT file Further Examples countries. txt array countries[] file = open. Read("countries. txt") while NOT file.](https://slidetodoc.com/presentation_image/5e481da7204e16430b3cf16c48ba605a/image-7.jpg)
Further Examples countries. txt array countries[] file = open. Read("countries. txt") while NOT file. end. Of. File() countries[i] = file. read. Line() endwhile print(countries) Albania Armenia Australia Austria. . . end. Of. File() is a function which returns true if the cursor is at the end of the file, and false otherwise. By using it in a while loop, it ensures we keep reading lines until we can’t read any more. Append the line that’s just been read to the array countries.
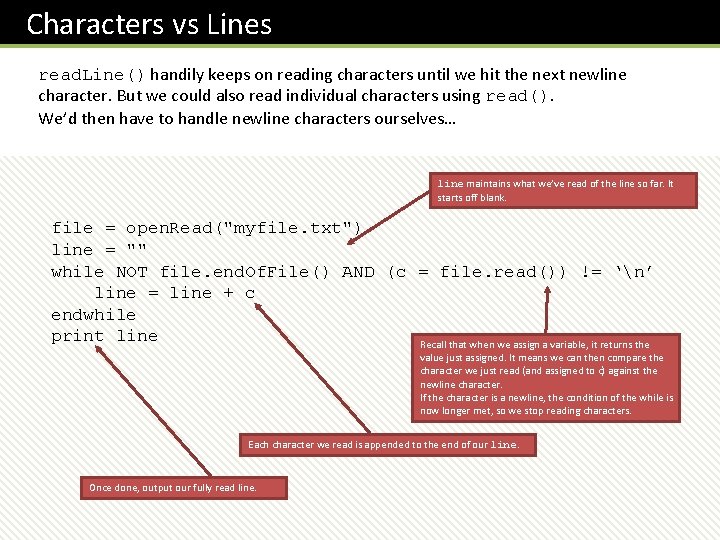
Characters vs Lines read. Line() handily keeps on reading characters until we hit the next newline character. But we could also read individual characters using read(). We’d then have to handle newline characters ourselves… line maintains what we’ve read of the line so far. It starts off blank. file = open. Read("myfile. txt") line = "" while NOT file. end. Of. File() AND (c = file. read()) != ‘n’ line = line + c endwhile print line Recall that when we assign a variable, it returns the value just assigned. It means we can then compare the character we just read (and assigned to c) against the newline character. If the character is a newline, the condition of the while is now longer met, so we stop reading characters. Each character we read is appended to the end of our line. Once done, output our fully read line.
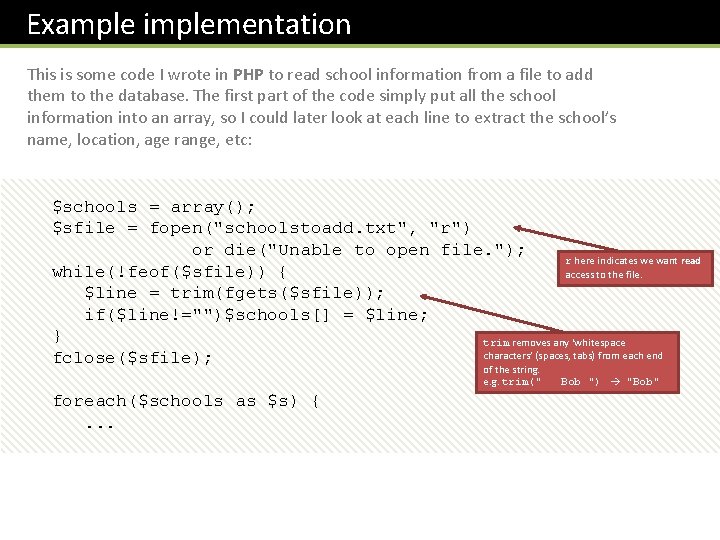
Example implementation This is some code I wrote in PHP to read school information from a file to add them to the database. The first part of the code simply put all the school information into an array, so I could later look at each line to extract the school’s name, location, age range, etc: $schools = array(); $sfile = fopen("schoolstoadd. txt", "r") or die("Unable to open file. "); r here indicates we want read while(!feof($sfile)) { access to the file. $line = trim(fgets($sfile)); if($line!="")$schools[] = $line; } trim removes any ‘whitespace characters’ (spaces, tabs) from each end fclose($sfile); of the string. e. g. trim(" foreach($schools as $s) {. . . Bob ") "Bob"
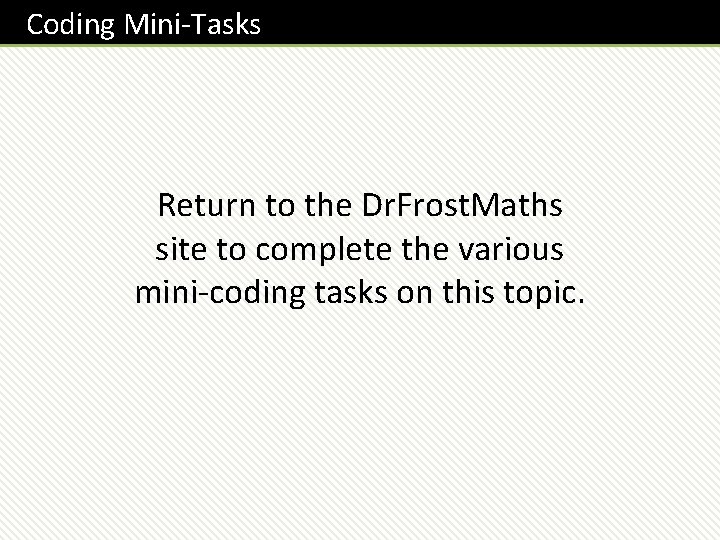
Coding Mini-Tasks Return to the Dr. Frost. Maths site to complete the various mini-coding tasks on this topic.