Programming Structures 1 COMP 102 Prog Fundamentals Structures
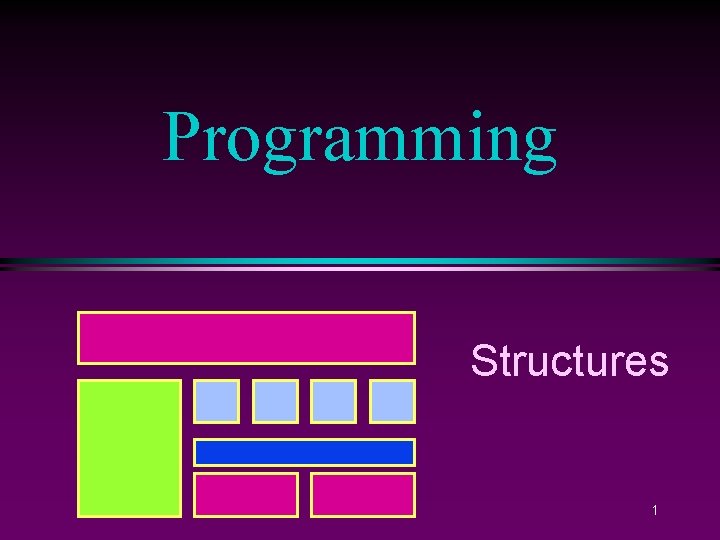
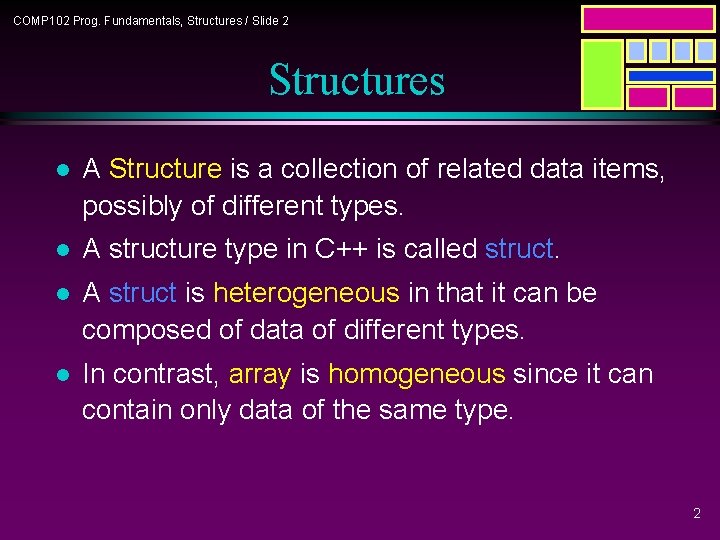
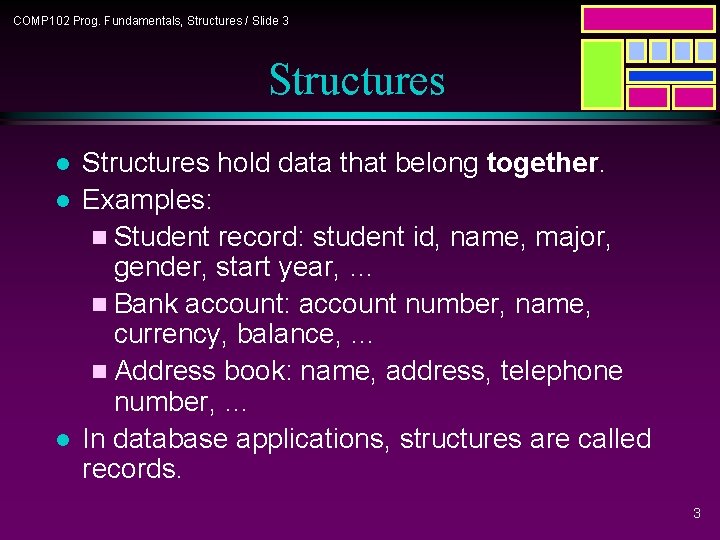
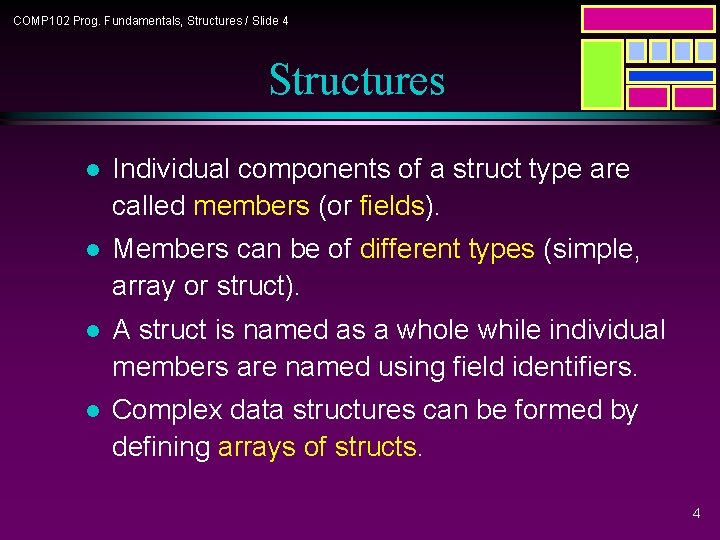
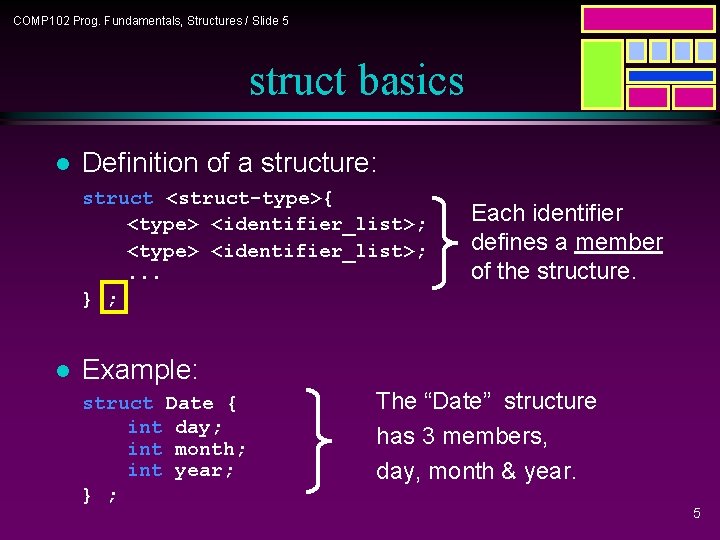
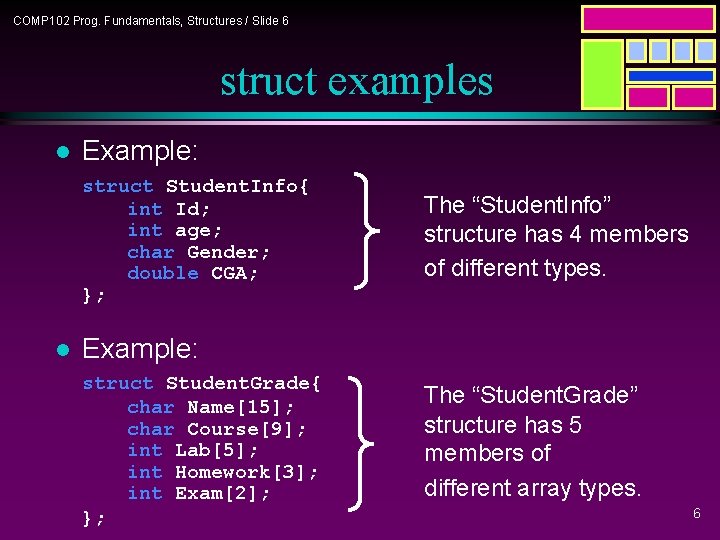
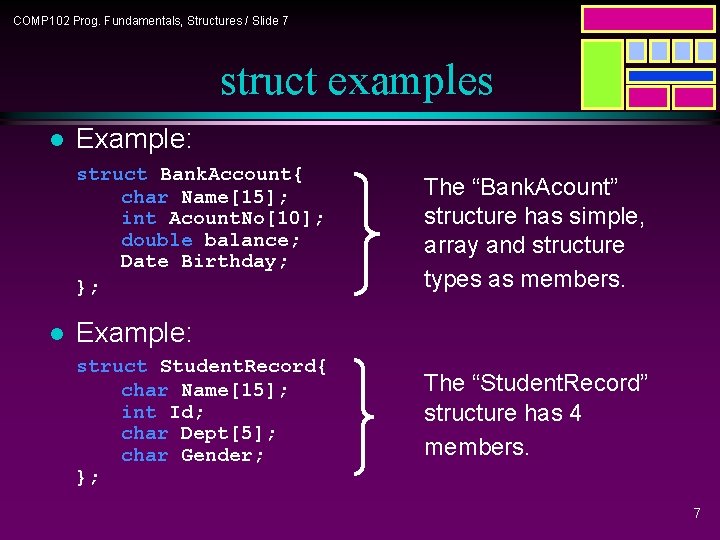
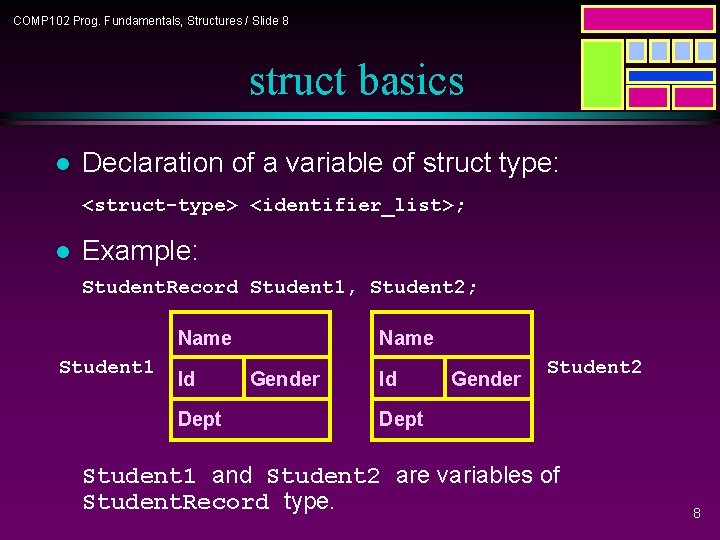
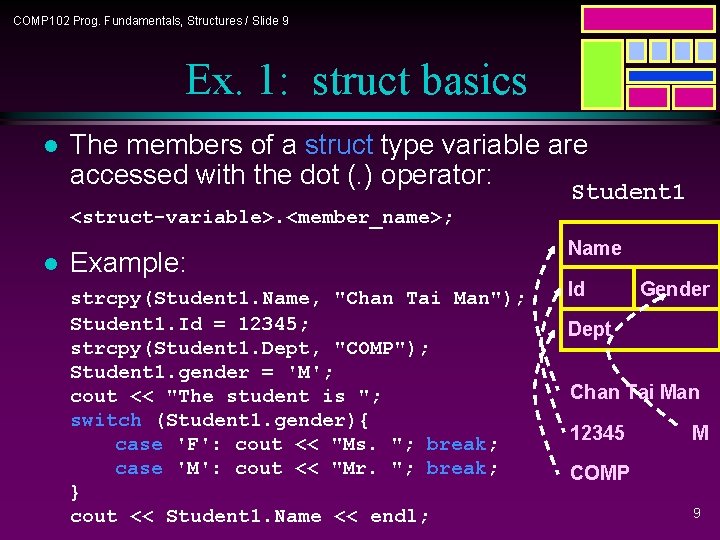
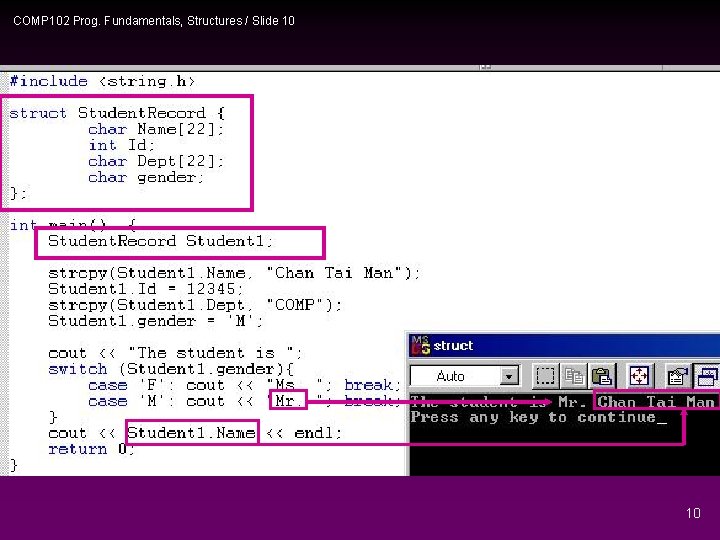
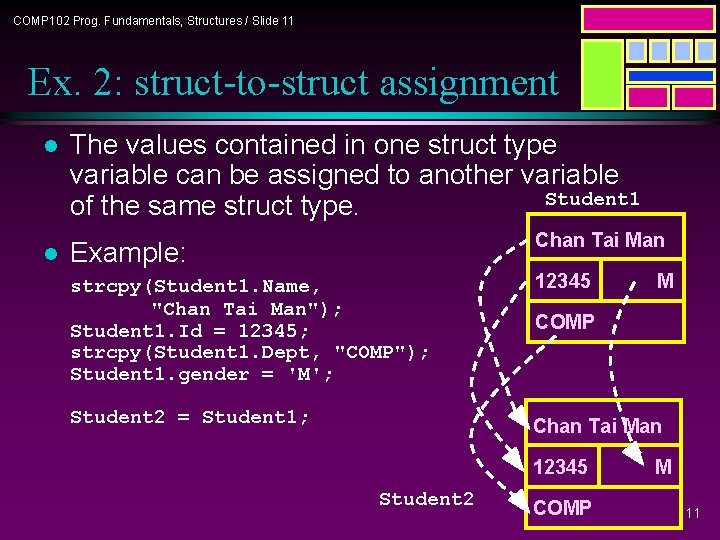
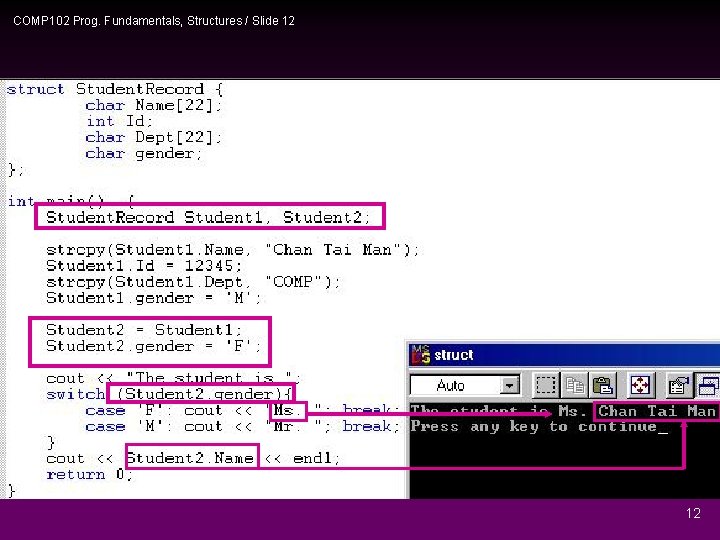
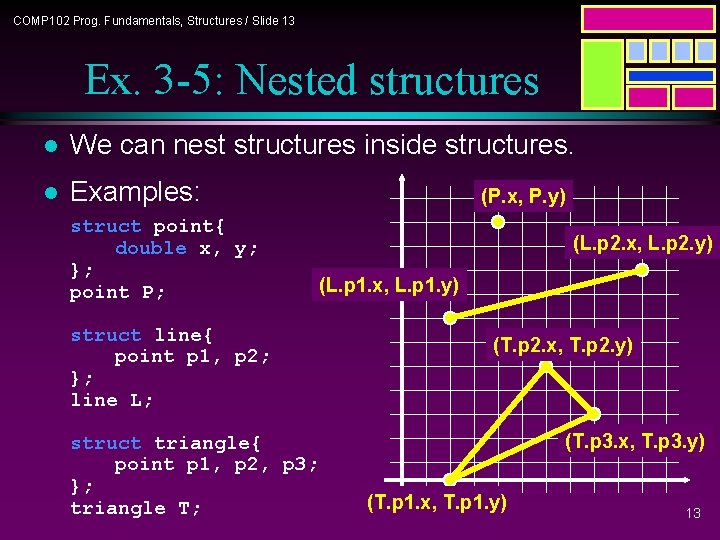
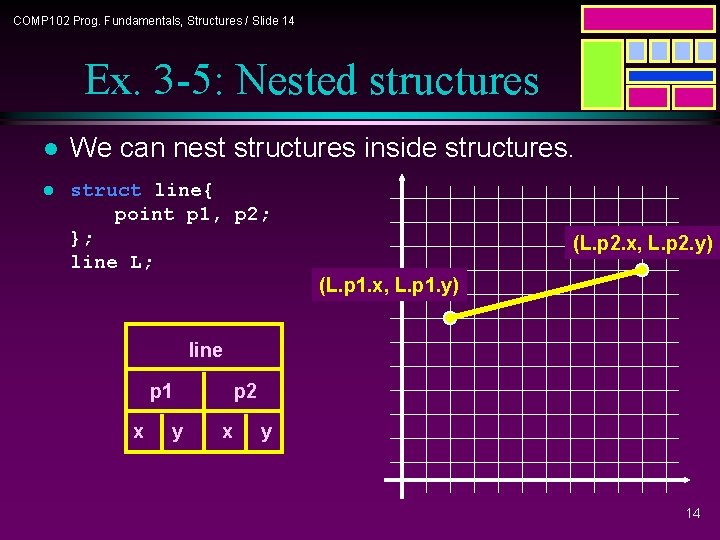
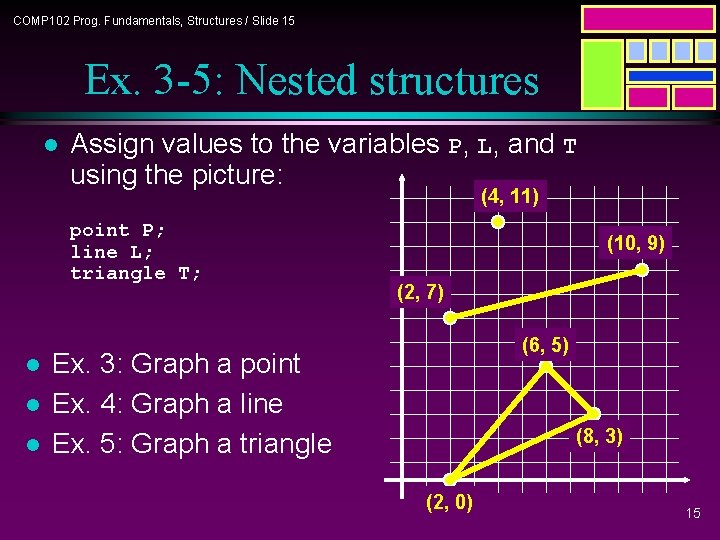
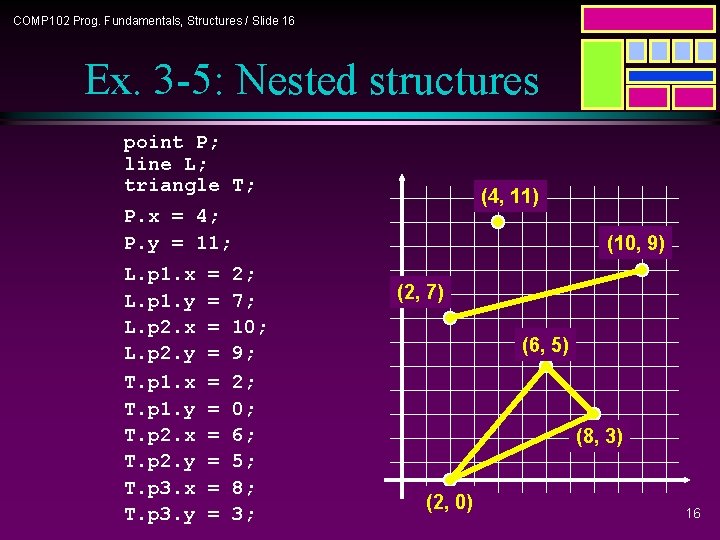
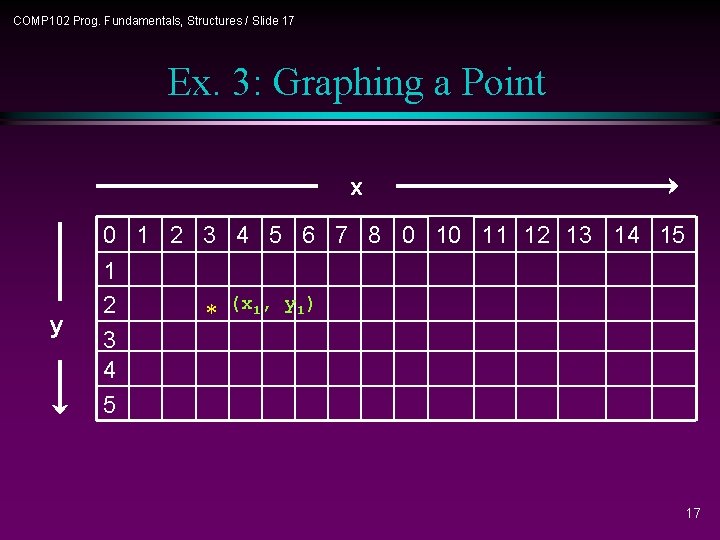
![struct point {int x, y; }; void user_input(point&); graph_point(char grid[NUMBER_ROWS][NUMBER_COLS], point); print_grid(char grid[NUMBER_ROWS][NUMBER_COLS]); set_background(char struct point {int x, y; }; void user_input(point&); graph_point(char grid[NUMBER_ROWS][NUMBER_COLS], point); print_grid(char grid[NUMBER_ROWS][NUMBER_COLS]); set_background(char](https://slidetodoc.com/presentation_image_h/9ebd185f975f142347aa562f394063ce/image-18.jpg)
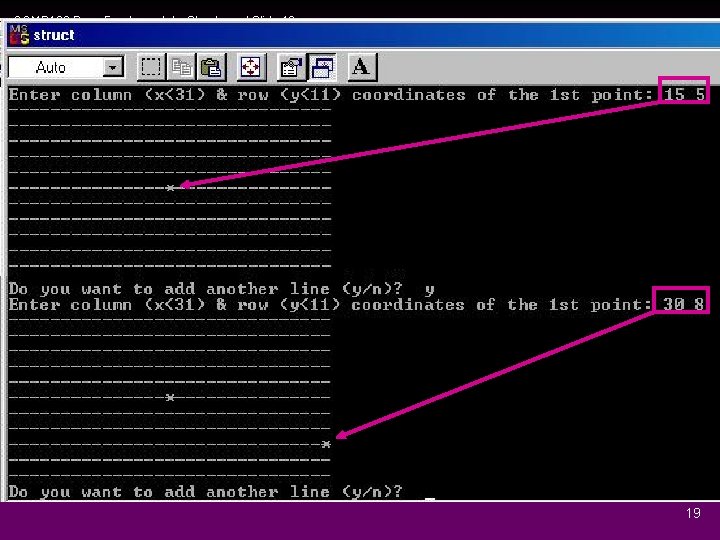
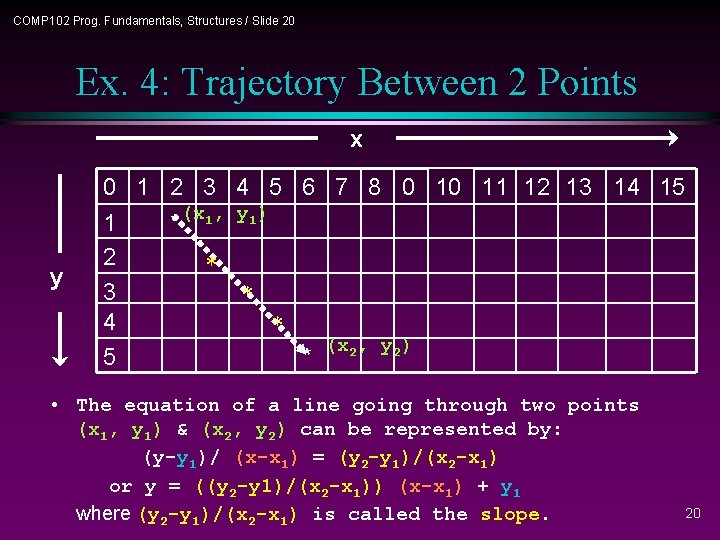
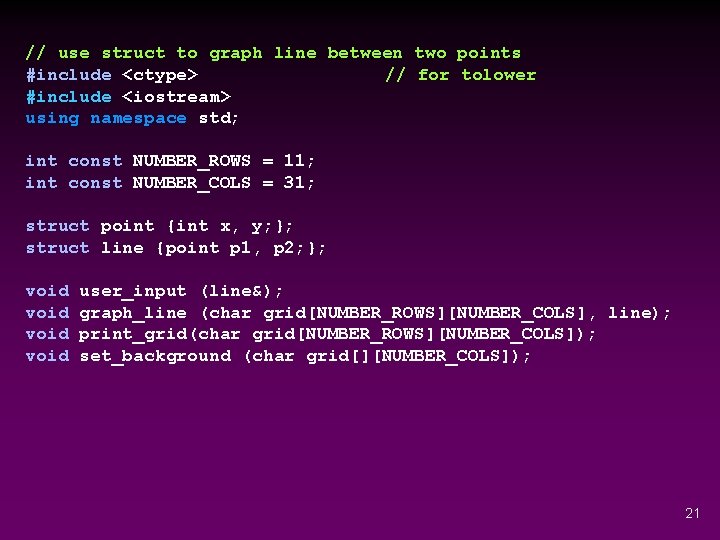
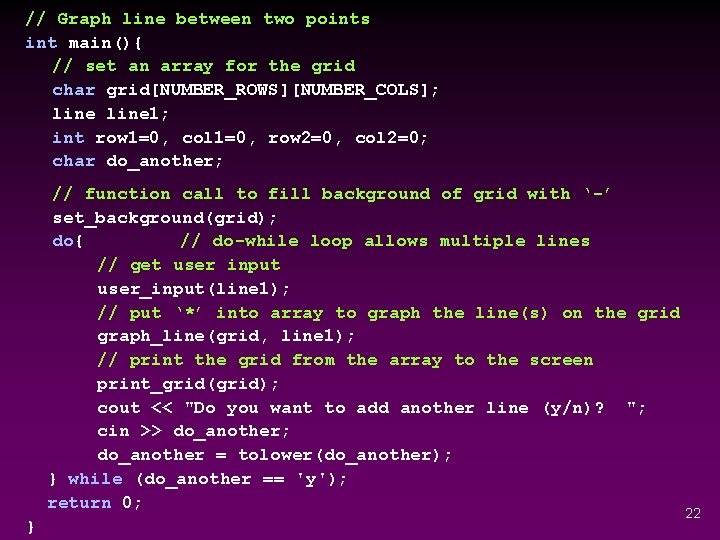
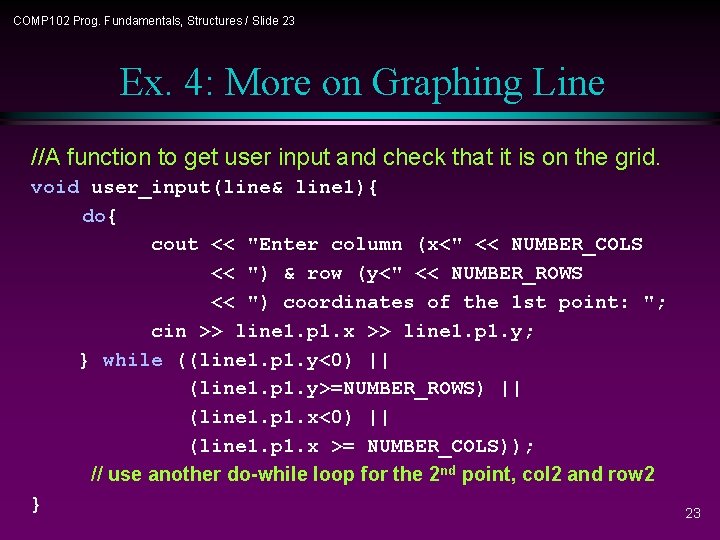
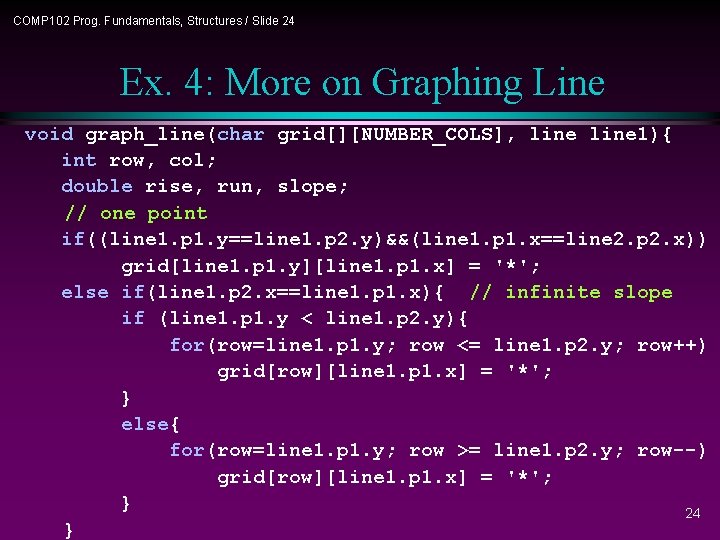
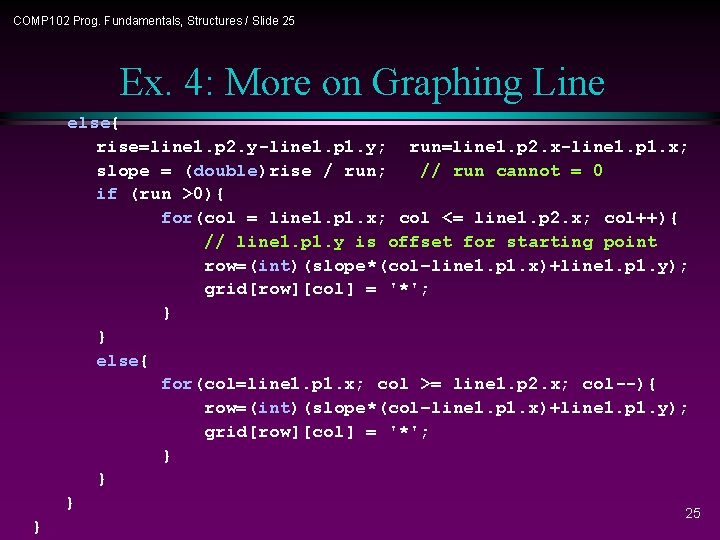
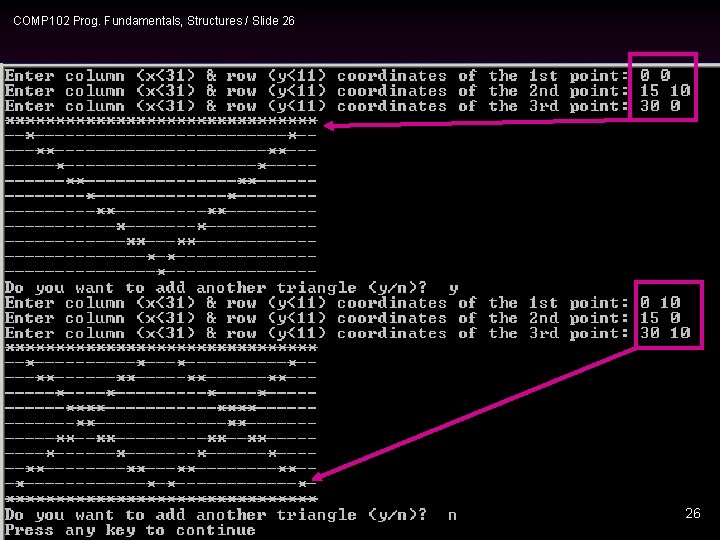
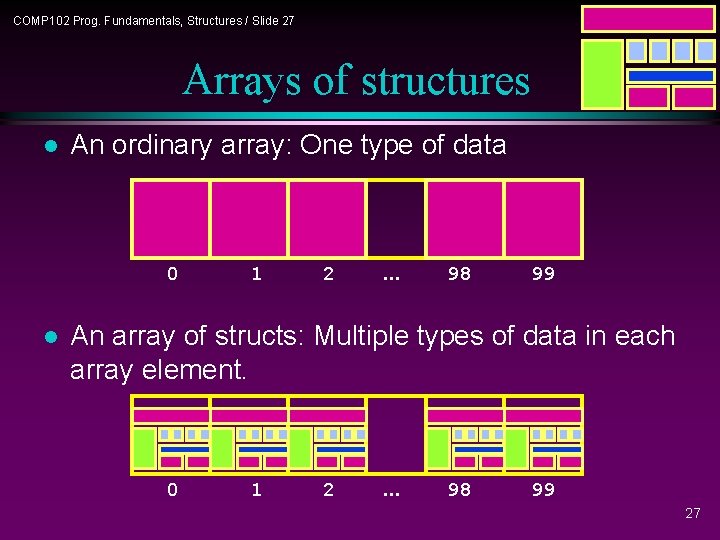
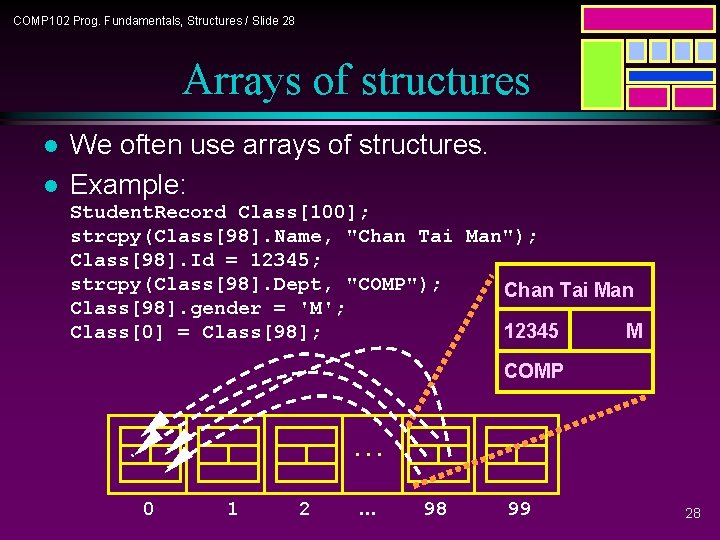
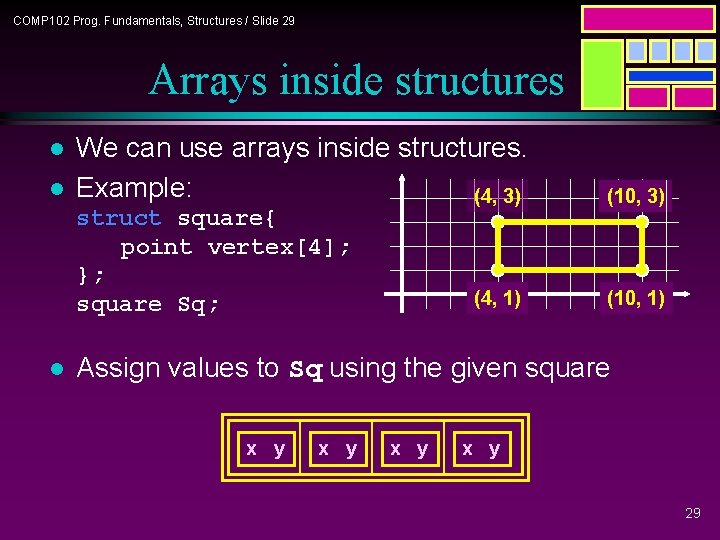
- Slides: 29
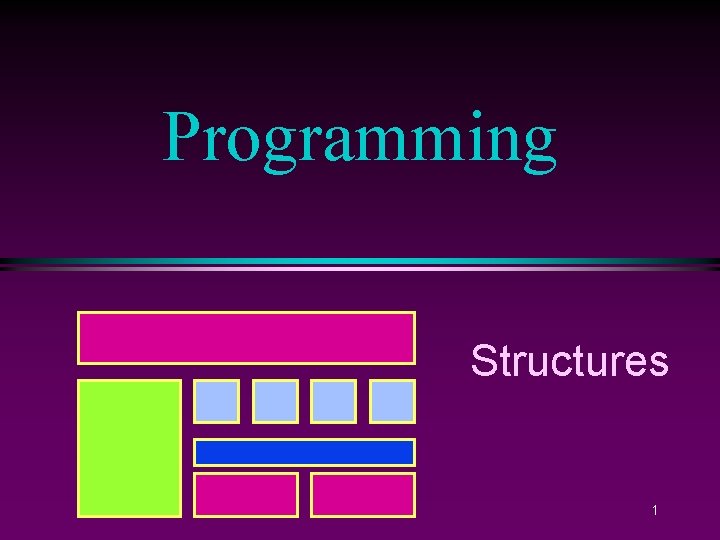
Programming Structures 1
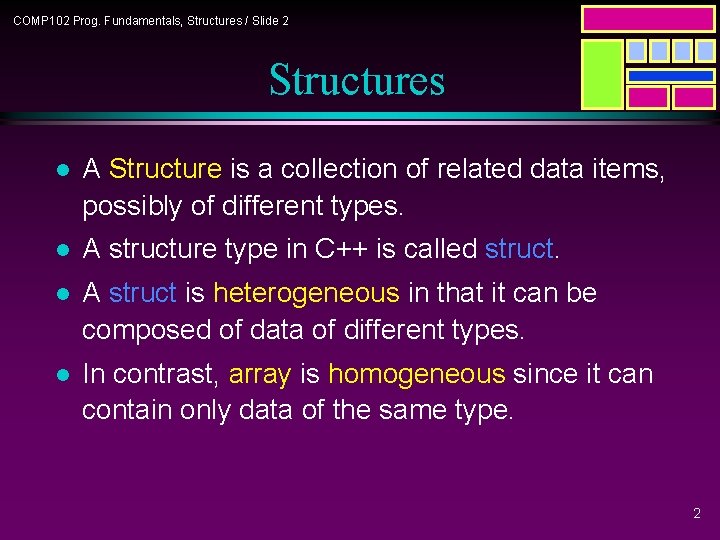
COMP 102 Prog. Fundamentals, Structures / Slide 2 Structures l A Structure is a collection of related data items, possibly of different types. l A structure type in C++ is called struct. l A struct is heterogeneous in that it can be composed of data of different types. l In contrast, array is homogeneous since it can contain only data of the same type. 2
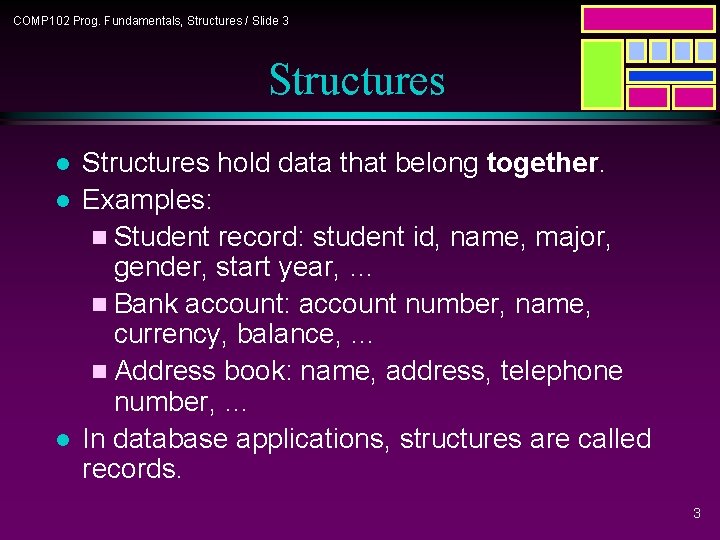
COMP 102 Prog. Fundamentals, Structures / Slide 3 Structures l l l Structures hold data that belong together. Examples: n Student record: student id, name, major, gender, start year, … n Bank account: account number, name, currency, balance, … n Address book: name, address, telephone number, … In database applications, structures are called records. 3
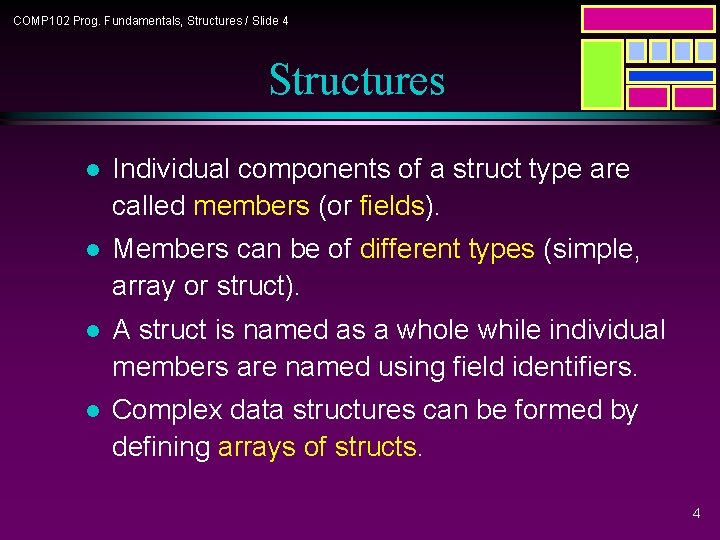
COMP 102 Prog. Fundamentals, Structures / Slide 4 Structures l Individual components of a struct type are called members (or fields). l Members can be of different types (simple, array or struct). l A struct is named as a whole while individual members are named using field identifiers. l Complex data structures can be formed by defining arrays of structs. 4
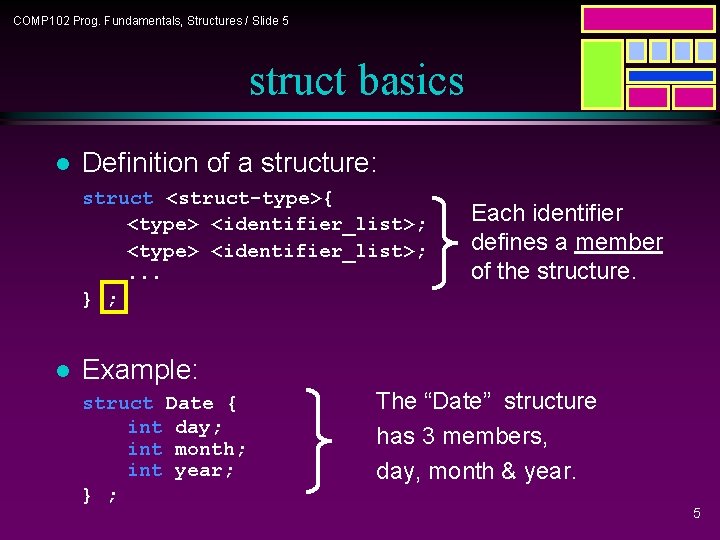
COMP 102 Prog. Fundamentals, Structures / Slide 5 struct basics l Definition of a structure: struct <struct-type>{ <type> <identifier_list>; . . . } ; l Each identifier defines a member of the structure. Example: struct Date { int day; int month; int year; } ; The “Date” structure has 3 members, day, month & year. 5
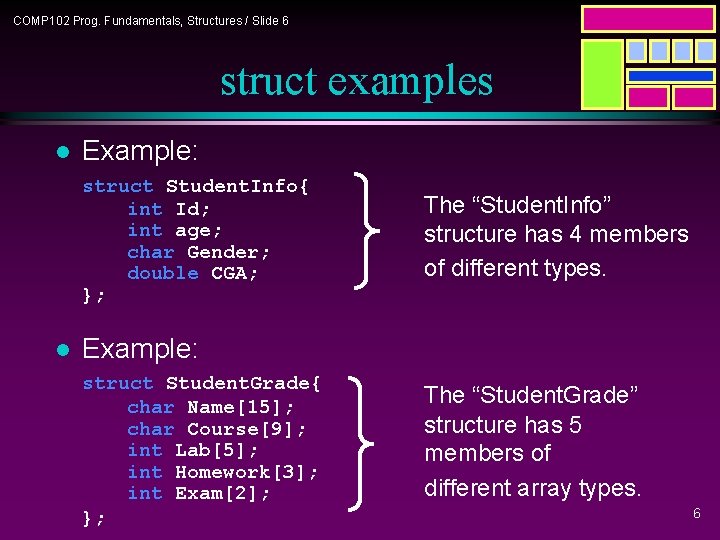
COMP 102 Prog. Fundamentals, Structures / Slide 6 struct examples l Example: struct Student. Info{ int Id; int age; char Gender; double CGA; }; l The “Student. Info” structure has 4 members of different types. Example: struct Student. Grade{ char Name[15]; char Course[9]; int Lab[5]; int Homework[3]; int Exam[2]; }; The “Student. Grade” structure has 5 members of different array types. 6
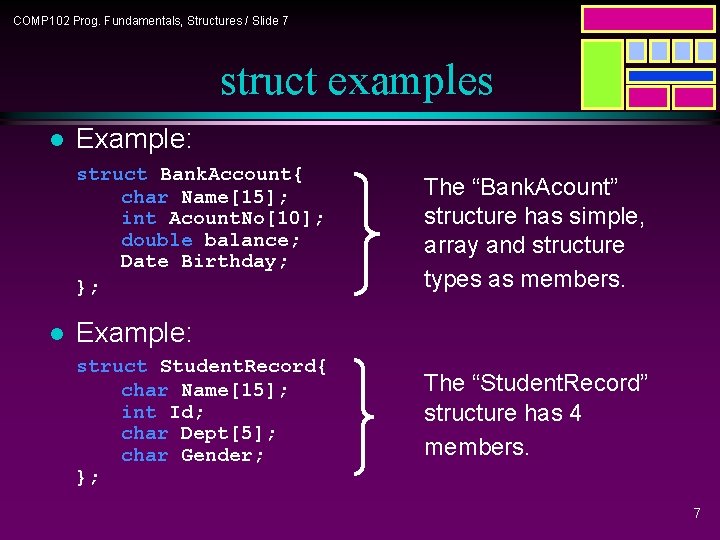
COMP 102 Prog. Fundamentals, Structures / Slide 7 struct examples l Example: struct Bank. Account{ char Name[15]; int Acount. No[10]; double balance; Date Birthday; }; l The “Bank. Acount” structure has simple, array and structure types as members. Example: struct Student. Record{ char Name[15]; int Id; char Dept[5]; char Gender; }; The “Student. Record” structure has 4 members. 7
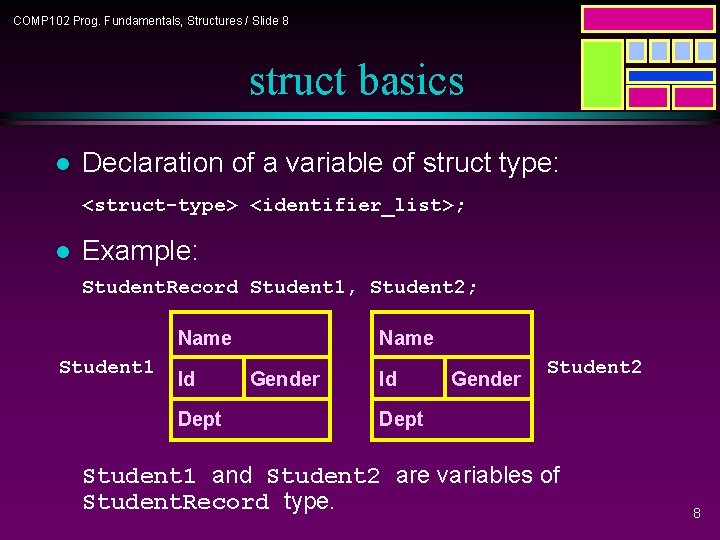
COMP 102 Prog. Fundamentals, Structures / Slide 8 struct basics l Declaration of a variable of struct type: <struct-type> <identifier_list>; l Example: Student. Record Student 1, Student 2; Name Student 1 Id Dept Name Gender Id Gender Student 2 Dept Student 1 and Student 2 are variables of Student. Record type. 8
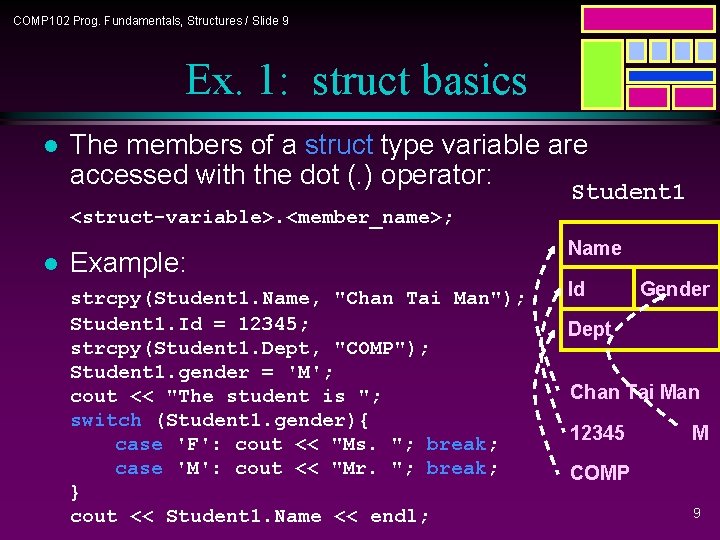
COMP 102 Prog. Fundamentals, Structures / Slide 9 Ex. 1: struct basics l The members of a struct type variable are accessed with the dot (. ) operator: Student 1 <struct-variable>. <member_name>; l Example: strcpy(Student 1. Name, "Chan Tai Man"); Student 1. Id = 12345; strcpy(Student 1. Dept, "COMP"); Student 1. gender = 'M'; cout << "The student is "; switch (Student 1. gender){ case 'F': cout << "Ms. "; break; case 'M': cout << "Mr. "; break; } cout << Student 1. Name << endl; Name Id Gender Dept Chan Tai Man 12345 M COMP 9
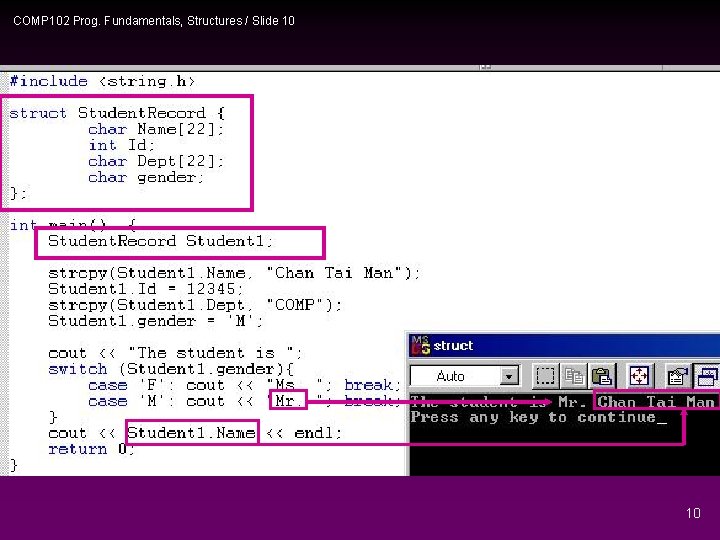
COMP 102 Prog. Fundamentals, Structures / Slide 10 10
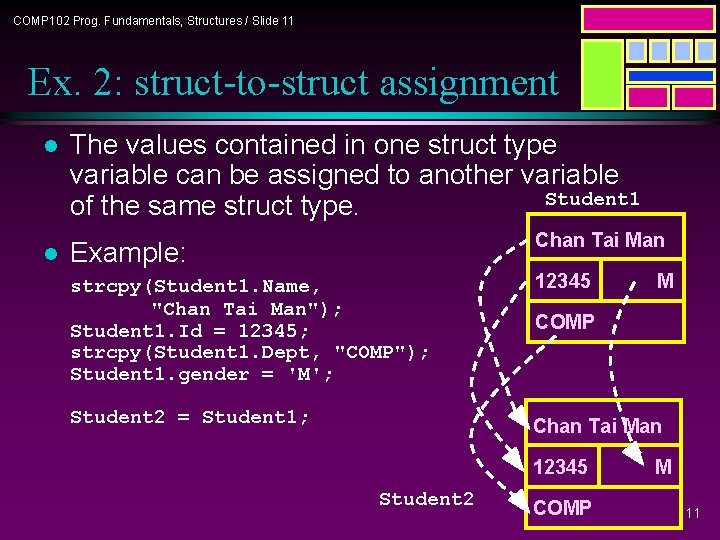
COMP 102 Prog. Fundamentals, Structures / Slide 11 Ex. 2: struct-to-struct assignment l l The values contained in one struct type variable can be assigned to another variable Student 1 of the same struct type. Example: Chan Tai Man strcpy(Student 1. Name, "Chan Tai Man"); Student 1. Id = 12345; strcpy(Student 1. Dept, "COMP"); Student 1. gender = 'M'; 12345 Student 2 = Student 1; Chan Tai Man COMP 12345 Student 2 M COMP M 11
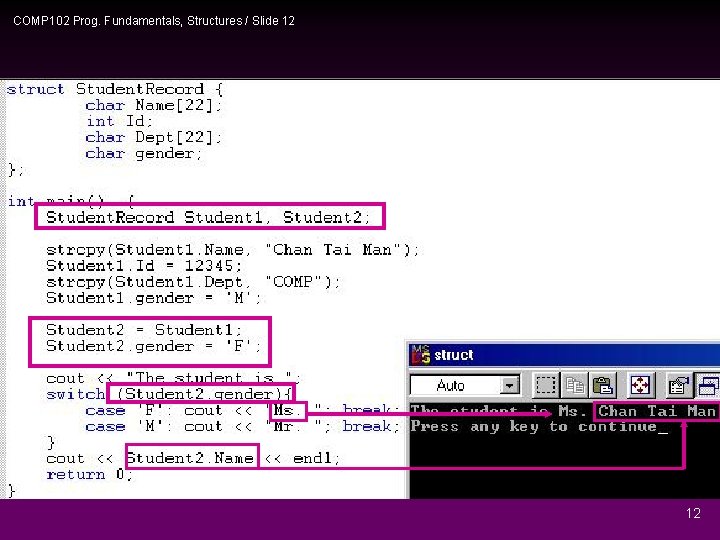
COMP 102 Prog. Fundamentals, Structures / Slide 12 12
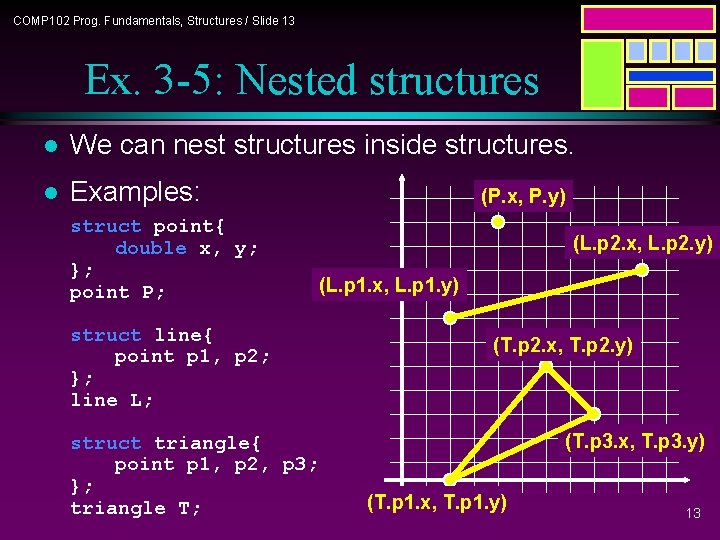
COMP 102 Prog. Fundamentals, Structures / Slide 13 Ex. 3 -5: Nested structures l We can nest structures inside structures. l Examples: struct point{ double x, y; }; point P; (P. x, P. y) (L. p 2. x, L. p 2. y) (L. p 1. x, L. p 1. y) struct line{ point p 1, p 2; }; line L; struct triangle{ point p 1, p 2, p 3; }; triangle T; (T. p 2. x, T. p 2. y) (T. p 3. x, T. p 3. y) (T. p 1. x, T. p 1. y) 13
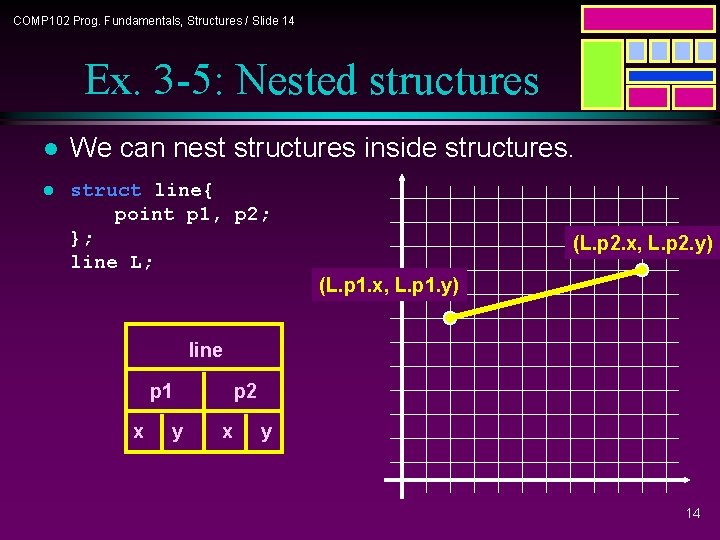
COMP 102 Prog. Fundamentals, Structures / Slide 14 Ex. 3 -5: Nested structures l We can nest structures inside structures. l struct line{ point p 1, p 2; }; line L; (L. p 2. x, L. p 2. y) (L. p 1. x, L. p 1. y) line p 1 x y p 2 x y 14
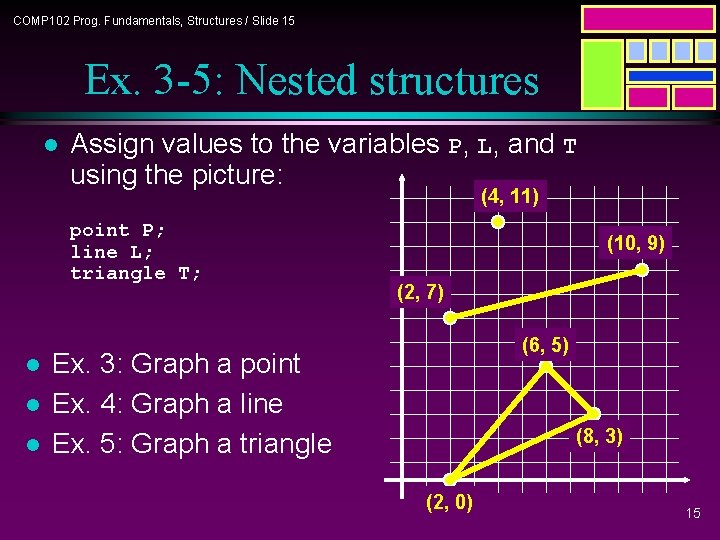
COMP 102 Prog. Fundamentals, Structures / Slide 15 Ex. 3 -5: Nested structures l Assign values to the variables P, L, and T using the picture: (4, 11) point P; line L; triangle T; l l l (10, 9) (2, 7) (6, 5) Ex. 3: Graph a point Ex. 4: Graph a line Ex. 5: Graph a triangle (8, 3) (2, 0) 15
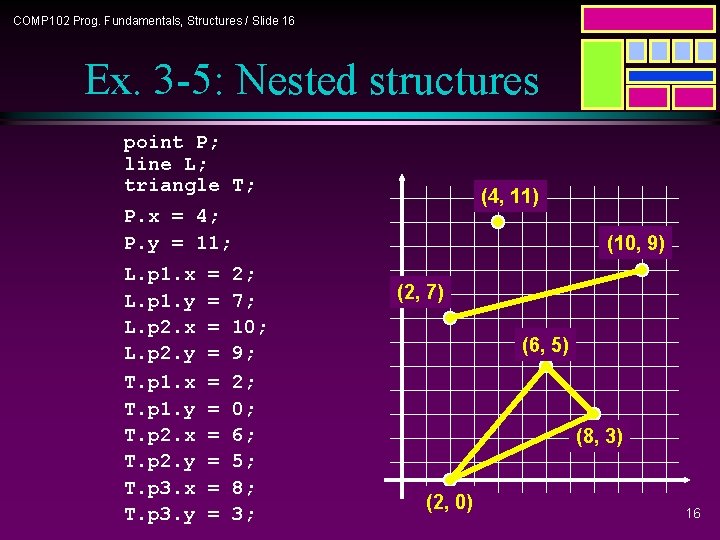
COMP 102 Prog. Fundamentals, Structures / Slide 16 Ex. 3 -5: Nested structures point P; line L; triangle T; (4, 11) P. x = 4; P. y = 11; L. p 1. x L. p 1. y L. p 2. x L. p 2. y T. p 1. x T. p 1. y T. p 2. x T. p 2. y T. p 3. x T. p 3. y = = = = = (10, 9) 2; 7; 10; 9; 2; 0; 6; 5; 8; 3; (2, 7) (6, 5) (8, 3) (2, 0) 16
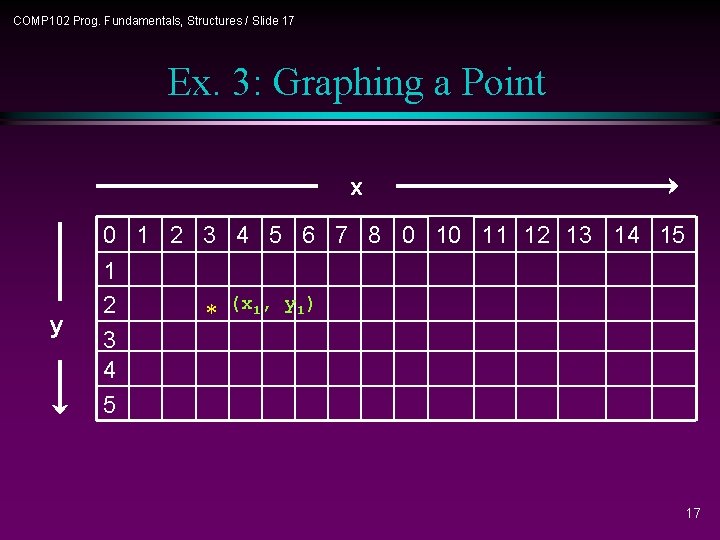
COMP 102 Prog. Fundamentals, Structures / Slide 17 Ex. 3: Graphing a Point x 0 1 2 3 4 5 6 7 8 0 10 11 12 13 14 15 y 1 2 3 4 5 * (x 1, y 1) 17
![struct point int x y void userinputpoint graphpointchar gridNUMBERROWSNUMBERCOLS point printgridchar gridNUMBERROWSNUMBERCOLS setbackgroundchar struct point {int x, y; }; void user_input(point&); graph_point(char grid[NUMBER_ROWS][NUMBER_COLS], point); print_grid(char grid[NUMBER_ROWS][NUMBER_COLS]); set_background(char](https://slidetodoc.com/presentation_image_h/9ebd185f975f142347aa562f394063ce/image-18.jpg)
struct point {int x, y; }; void user_input(point&); graph_point(char grid[NUMBER_ROWS][NUMBER_COLS], point); print_grid(char grid[NUMBER_ROWS][NUMBER_COLS]); set_background(char grid[][NUMBER_COLS]); void user_input(point& P){ // pass by reference // get user input and check that it is on the grid do{ cout << "Enter column (x<" << NUMBER_COLS << ") & row (y<" << NUMBER_ROWS <<") of the 1 st point: "; cin >> P. x >> P. y; } while ((P. y<0) || (P. y >= NUMBER_ROWS) || (P. x<0) || (P. x >= NUMBER_COLS)); } // Put a point on the grid void graph_point(char grid[][NUMBER_COLS], point P){ grid[P. y][P. x] = '*'; } 18
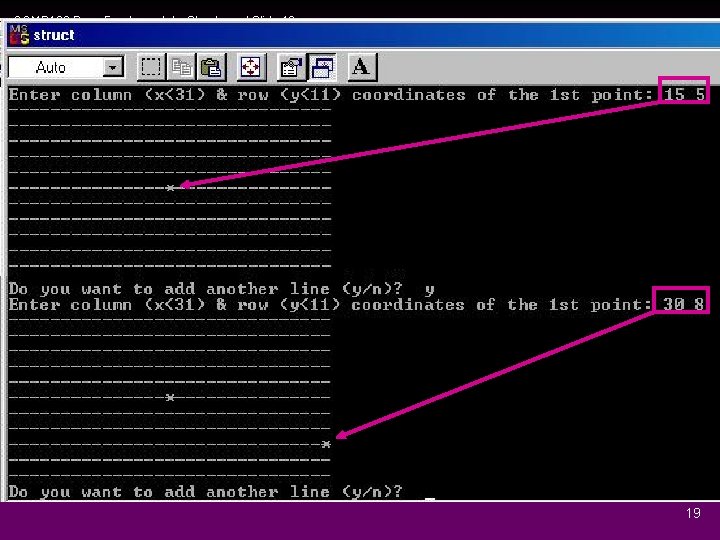
COMP 102 Prog. Fundamentals, Structures / Slide 19 19
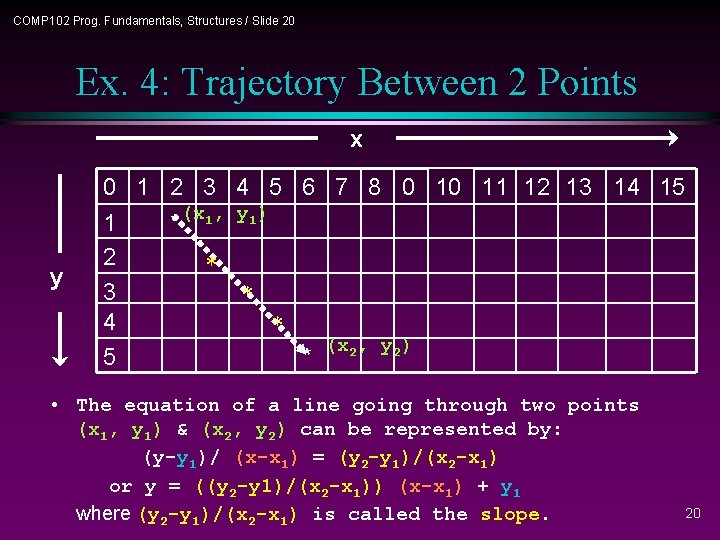
COMP 102 Prog. Fundamentals, Structures / Slide 20 Ex. 4: Trajectory Between 2 Points x 0 1 2 3 4 5 6 7 8 0 10 11 12 13 14 15 y 1 2 3 4 5 * (x 1, y 1) * * (x 2, y 2) • The equation of a line going through two points (x 1, y 1) & (x 2, y 2) can be represented by: (y-y 1)/ (x-x 1) = (y 2 -y 1)/(x 2 -x 1) or y = ((y 2 -y 1)/(x 2 -x 1)) (x-x 1) + y 1 where (y 2 -y 1)/(x 2 -x 1) is called the slope. 20
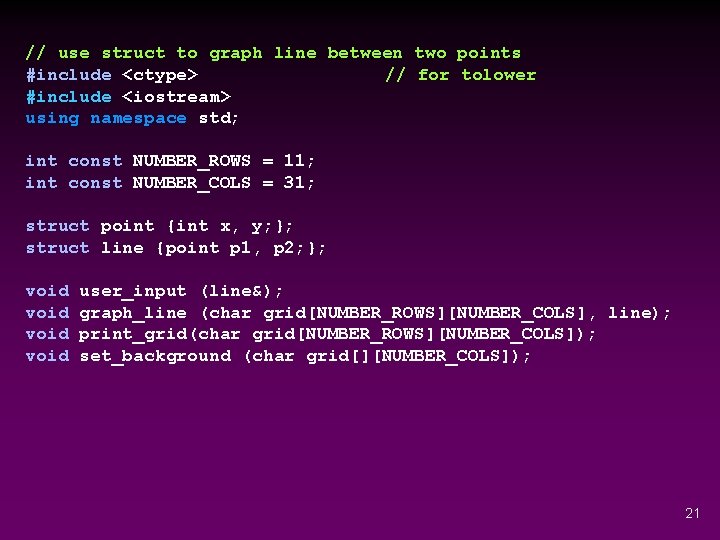
// use struct to graph line between two points #include <ctype> // for tolower #include <iostream> using namespace std; int const NUMBER_ROWS = 11; int const NUMBER_COLS = 31; struct point {int x, y; }; struct line {point p 1, p 2; }; void user_input (line&); graph_line (char grid[NUMBER_ROWS][NUMBER_COLS], line); print_grid(char grid[NUMBER_ROWS][NUMBER_COLS]); set_background (char grid[][NUMBER_COLS]); 21
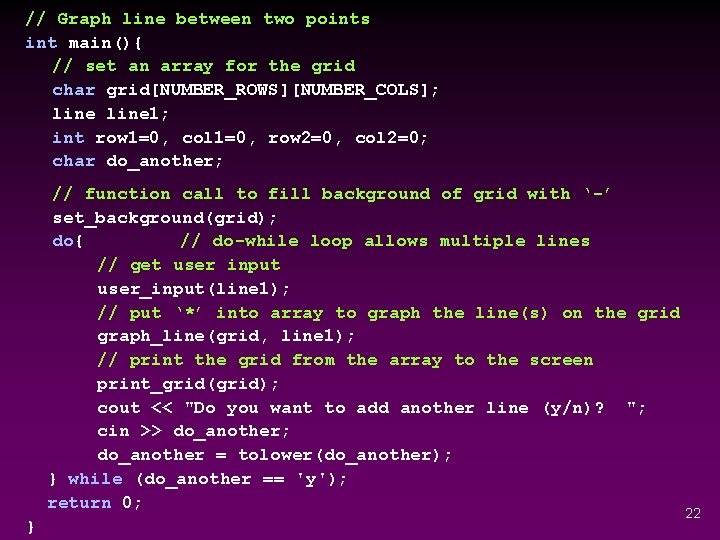
// Graph line between two points int main(){ // set an array for the grid char grid[NUMBER_ROWS][NUMBER_COLS]; line 1; int row 1=0, col 1=0, row 2=0, col 2=0; char do_another; // function call to fill background of grid with ‘-’ set_background(grid); do{ // do-while loop allows multiple lines // get user input user_input(line 1); // put ‘*’ into array to graph the line(s) on the grid graph_line(grid, line 1); // print the grid from the array to the screen print_grid(grid); cout << "Do you want to add another line (y/n)? "; cin >> do_another; do_another = tolower(do_another); } while (do_another == 'y'); return 0; } 22
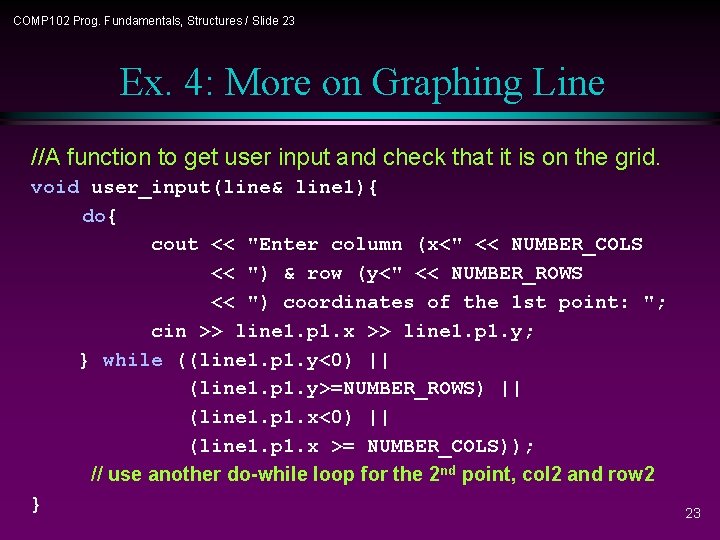
COMP 102 Prog. Fundamentals, Structures / Slide 23 Ex. 4: More on Graphing Line //A function to get user input and check that it is on the grid. void user_input(line& line 1){ do{ cout << "Enter column (x<" << NUMBER_COLS << ") & row (y<" << NUMBER_ROWS << ") coordinates of the 1 st point: "; cin >> line 1. p 1. x >> line 1. p 1. y; } while ((line 1. p 1. y<0) || (line 1. p 1. y>=NUMBER_ROWS) || (line 1. p 1. x<0) || (line 1. p 1. x >= NUMBER_COLS)); // use another do-while loop for the 2 nd point, col 2 and row 2 } 23
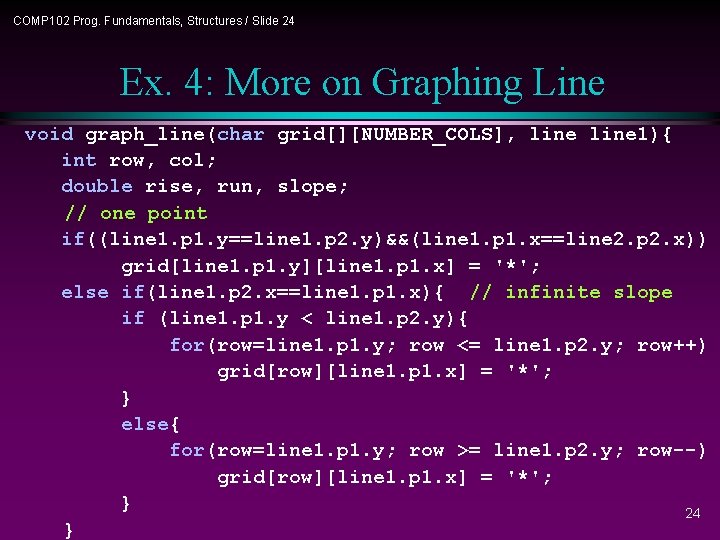
COMP 102 Prog. Fundamentals, Structures / Slide 24 Ex. 4: More on Graphing Line void graph_line(char grid[][NUMBER_COLS], line 1){ int row, col; double rise, run, slope; // one point if((line 1. p 1. y==line 1. p 2. y)&&(line 1. p 1. x==line 2. p 2. x)) grid[line 1. p 1. y][line 1. p 1. x] = '*'; else if(line 1. p 2. x==line 1. p 1. x){ // infinite slope if (line 1. p 1. y < line 1. p 2. y){ for(row=line 1. p 1. y; row <= line 1. p 2. y; row++) grid[row][line 1. p 1. x] = '*'; } else{ for(row=line 1. p 1. y; row >= line 1. p 2. y; row--) grid[row][line 1. p 1. x] = '*'; } 24 }
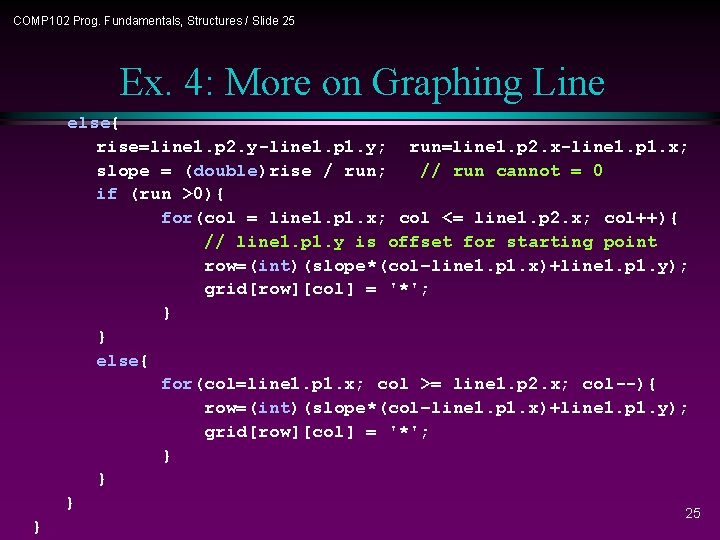
COMP 102 Prog. Fundamentals, Structures / Slide 25 Ex. 4: More on Graphing Line else{ rise=line 1. p 2. y-line 1. p 1. y; run=line 1. p 2. x-line 1. p 1. x; slope = (double)rise / run; // run cannot = 0 if (run >0){ for(col = line 1. p 1. x; col <= line 1. p 2. x; col++){ // line 1. p 1. y is offset for starting point row=(int)(slope*(col–line 1. p 1. x)+line 1. p 1. y); grid[row][col] = '*'; } } else{ for(col=line 1. p 1. x; col >= line 1. p 2. x; col--){ row=(int)(slope*(col–line 1. p 1. x)+line 1. p 1. y); grid[row][col] = '*'; } } 25
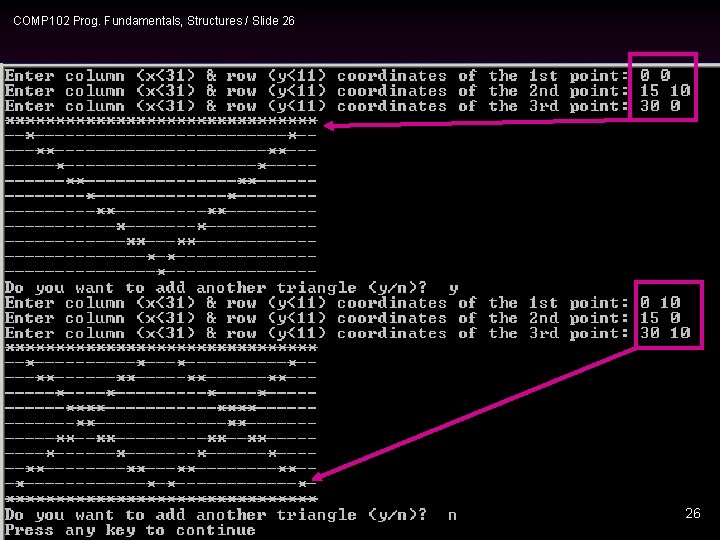
COMP 102 Prog. Fundamentals, Structures / Slide 26 26
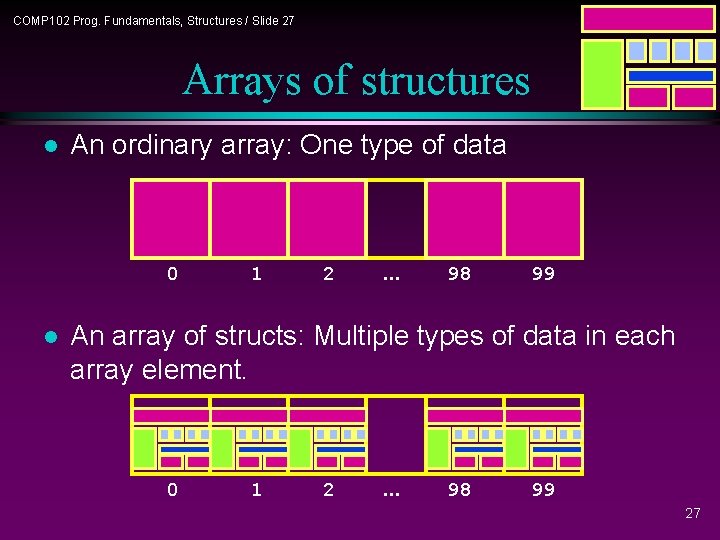
COMP 102 Prog. Fundamentals, Structures / Slide 27 Arrays of structures l An ordinary array: One type of data 0 l 1 2 … 98 99 An array of structs: Multiple types of data in each array element. 0 1 2 … 98 99 27
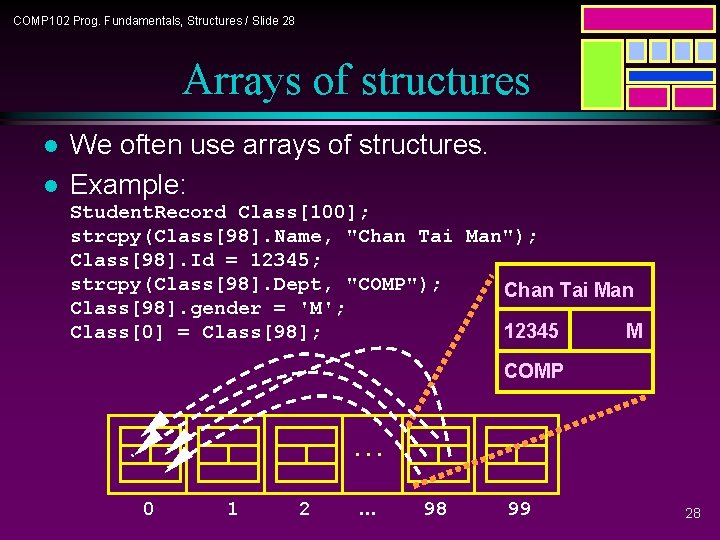
COMP 102 Prog. Fundamentals, Structures / Slide 28 Arrays of structures l l We often use arrays of structures. Example: Student. Record Class[100]; strcpy(Class[98]. Name, "Chan Tai Man"); Class[98]. Id = 12345; strcpy(Class[98]. Dept, "COMP"); Chan Tai Man Class[98]. gender = 'M'; 12345 M Class[0] = Class[98]; COMP . . . 0 1 2 … 98 99 28
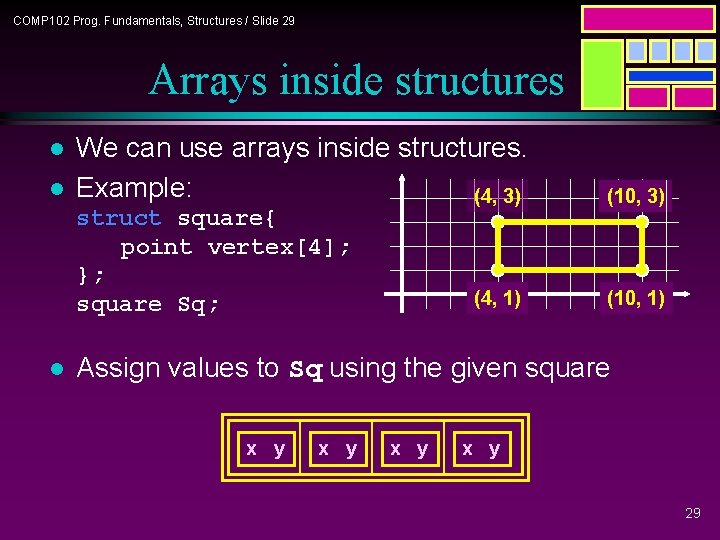
COMP 102 Prog. Fundamentals, Structures / Slide 29 Arrays inside structures l l We can use arrays inside structures. Example: (4, 3) struct square{ point vertex[4]; }; square Sq; l (4, 1) (10, 3) (10, 1) Assign values to Sq using the given square x y x y 29