Programming Languages and Compilers CS 421 William Mansky
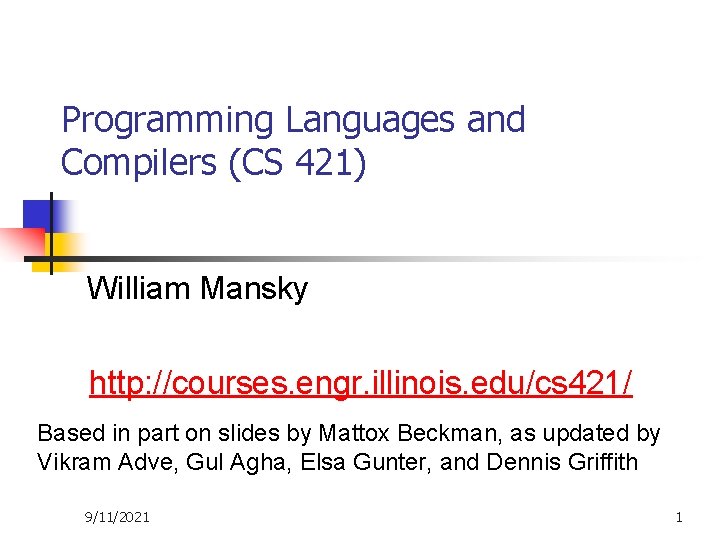
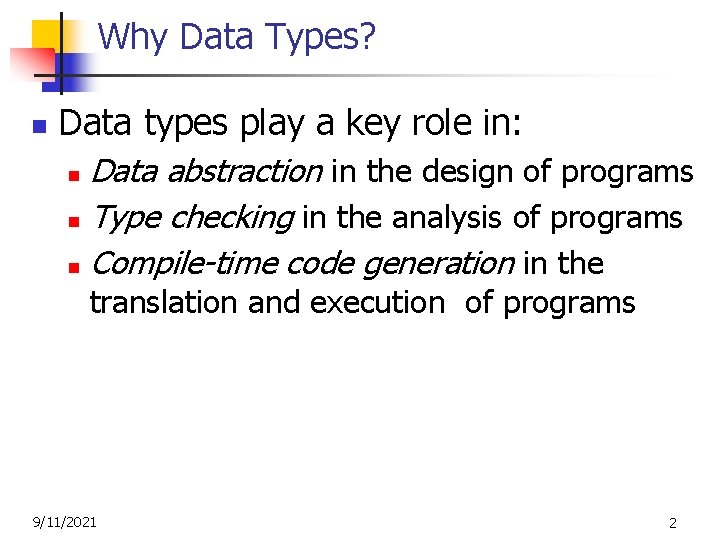
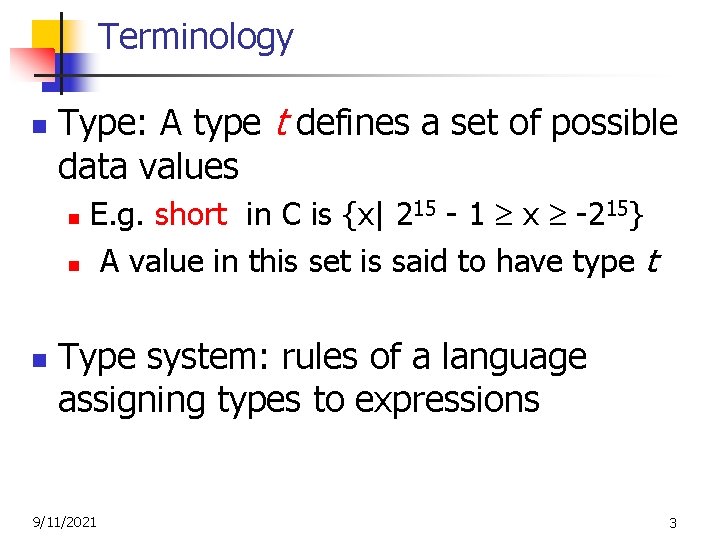
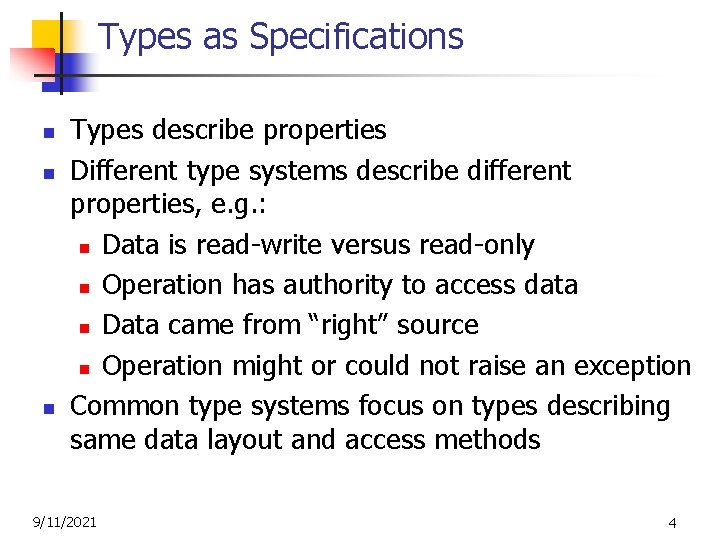
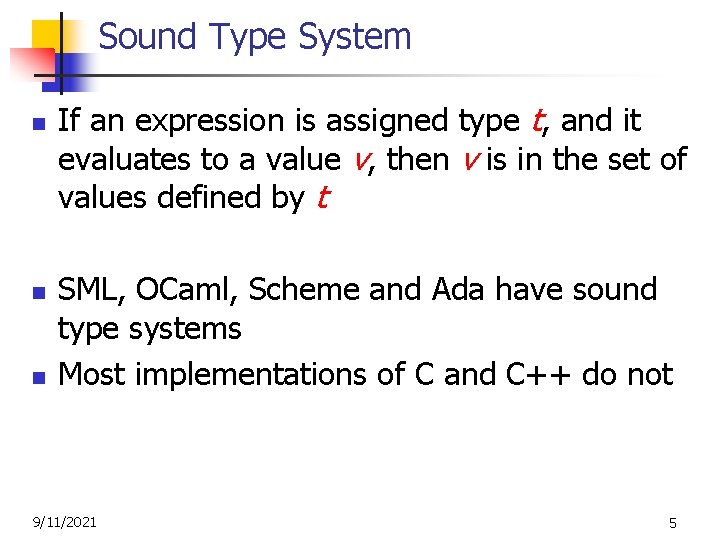
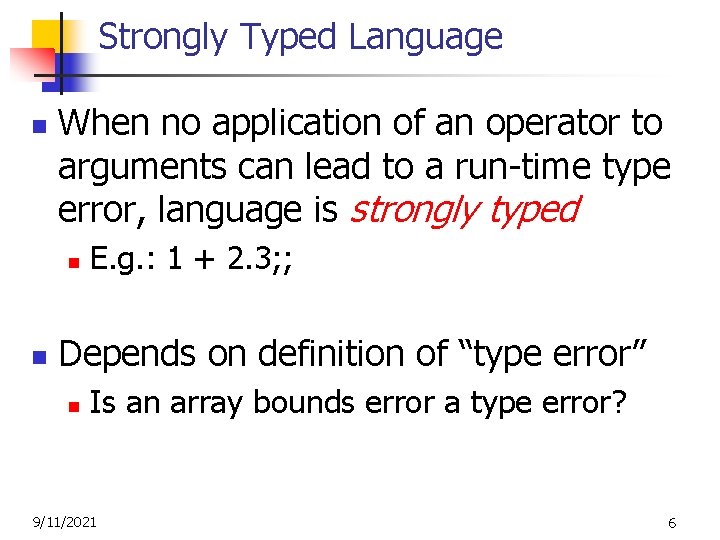
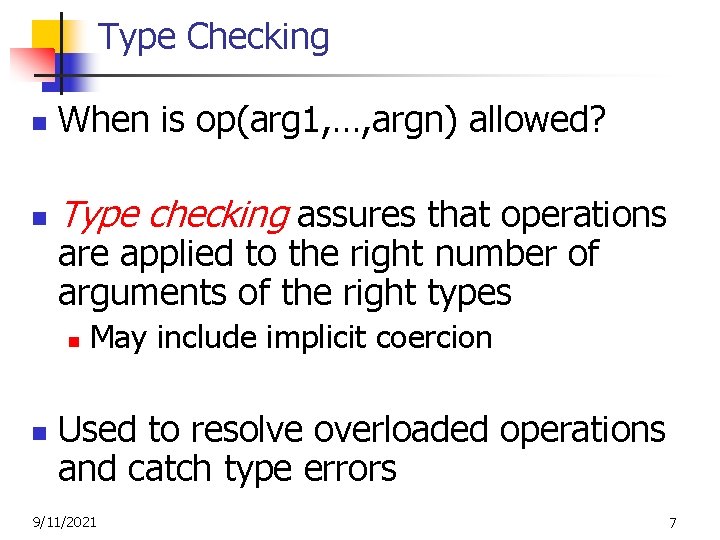
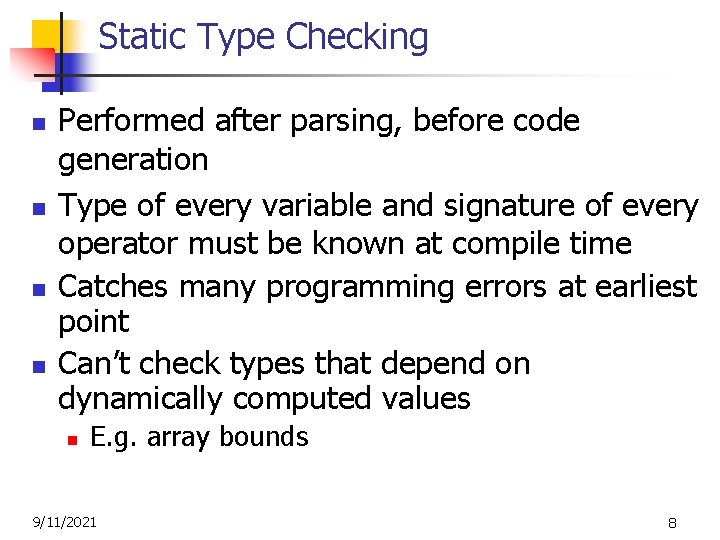
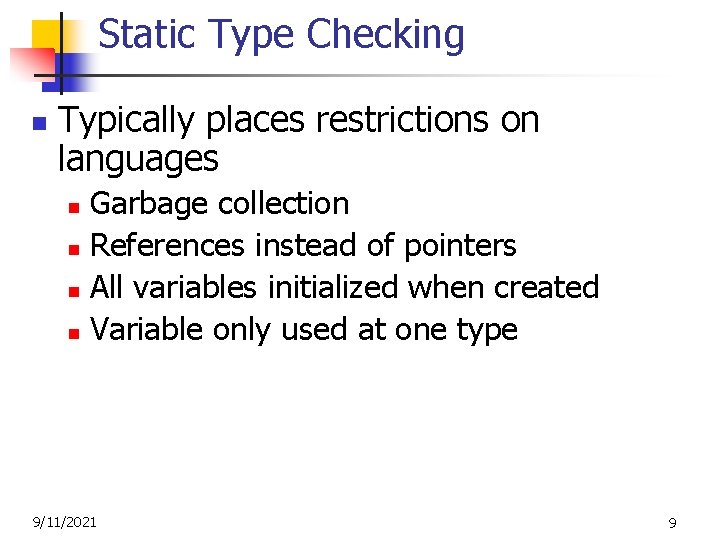
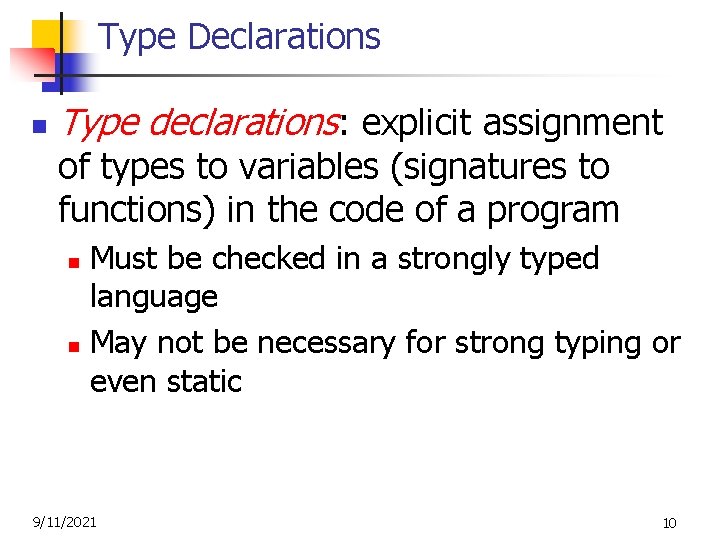
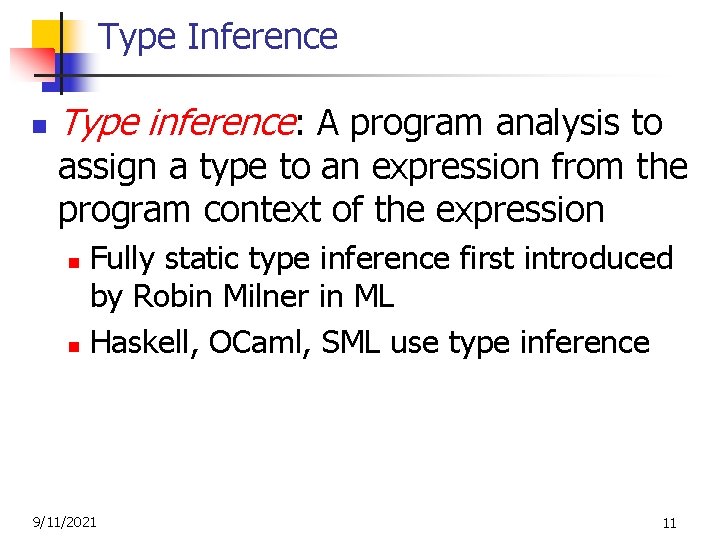
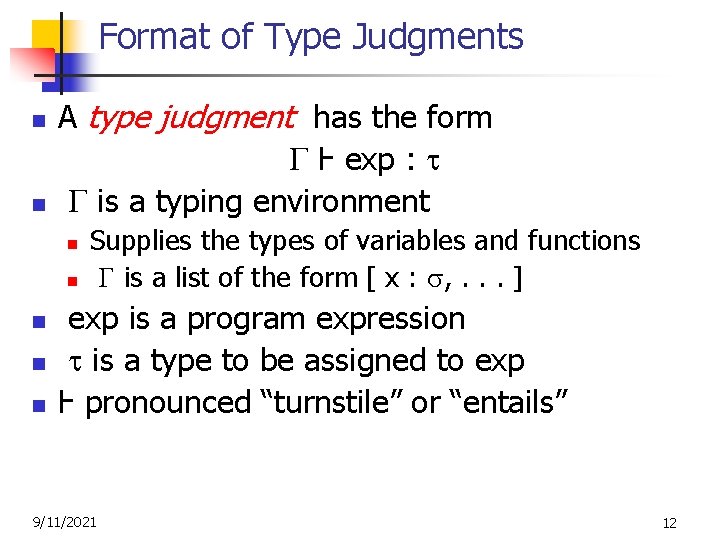
![Example Valid Type Judgments n n n [ ] Ⱶ true or false : Example Valid Type Judgments n n n [ ] Ⱶ true or false :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-13.jpg)
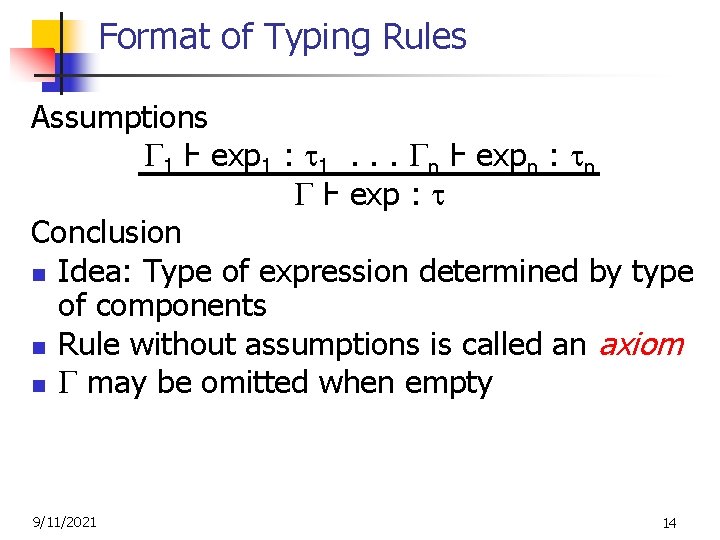
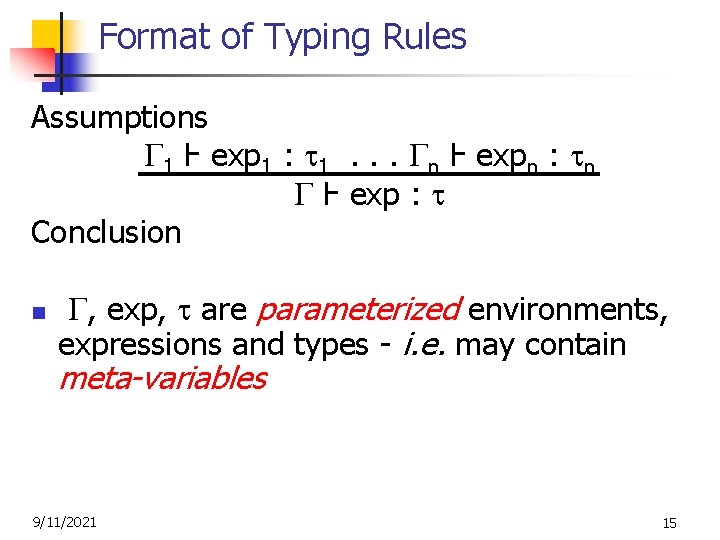
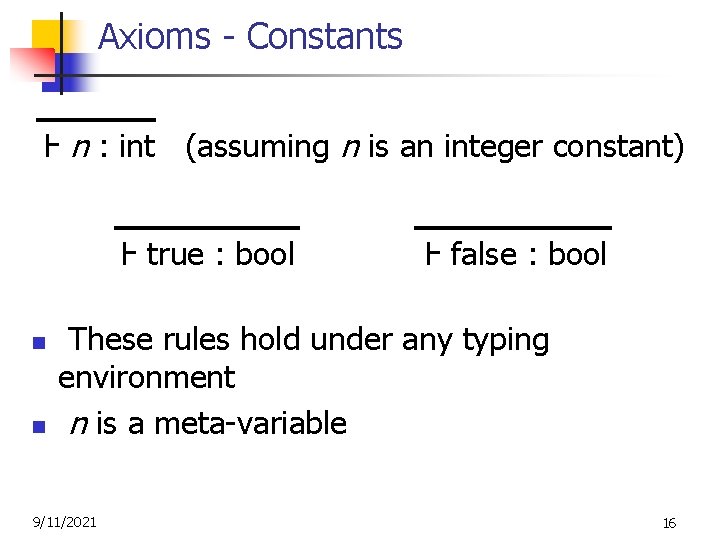
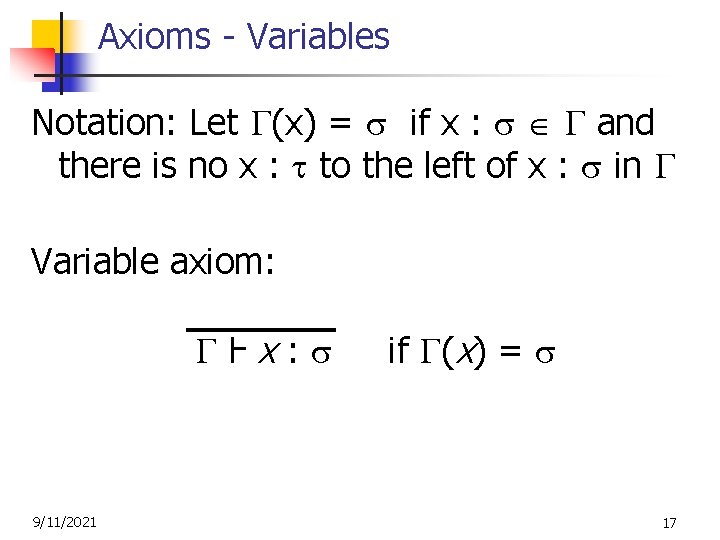
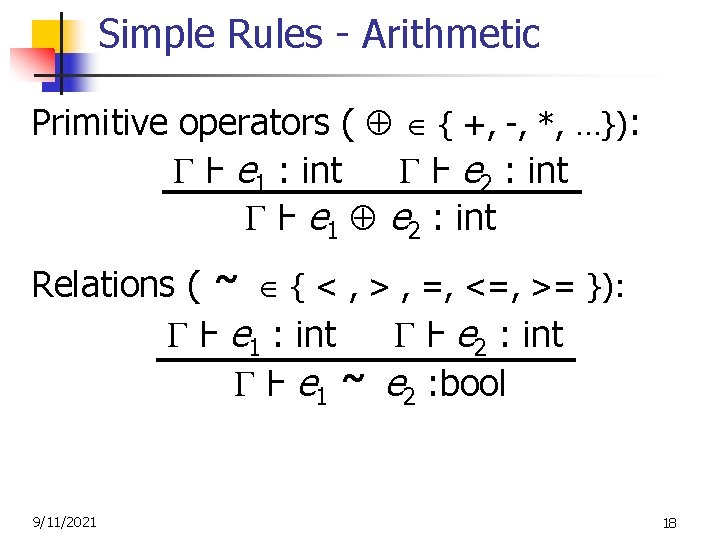
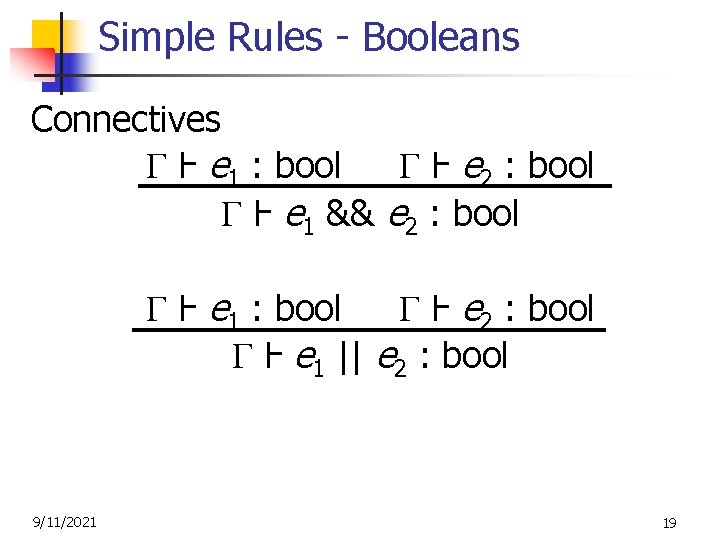
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-20.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-21.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-22.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-23.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-24.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-25.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-26.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-27.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-28.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-29.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-30.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-31.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-32.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-33.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-34.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-35.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-36.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-37.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-38.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-39.jpg)
![Simple Example n n n Let = [ x: int ; y: bool ] Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-40.jpg)
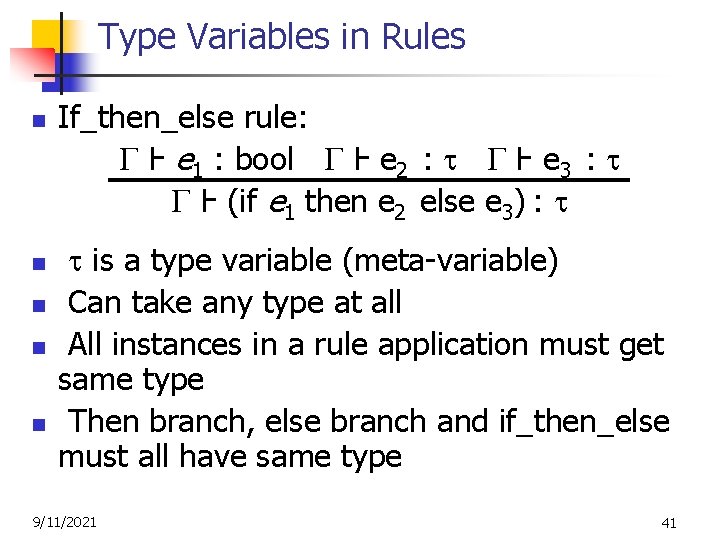
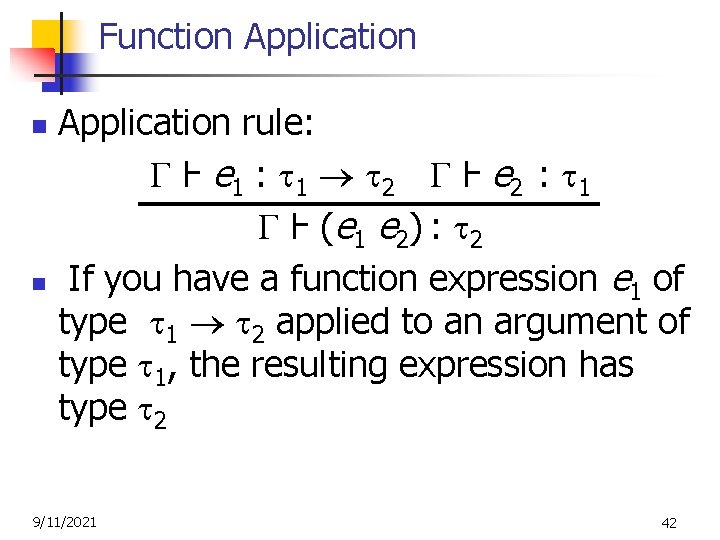
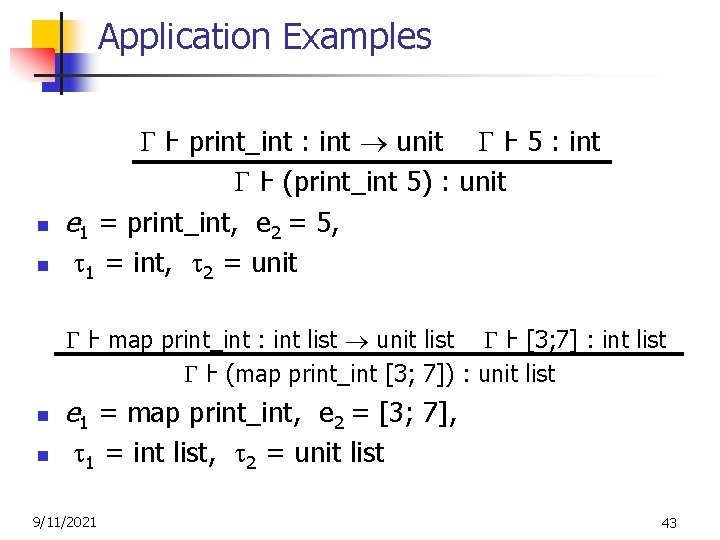
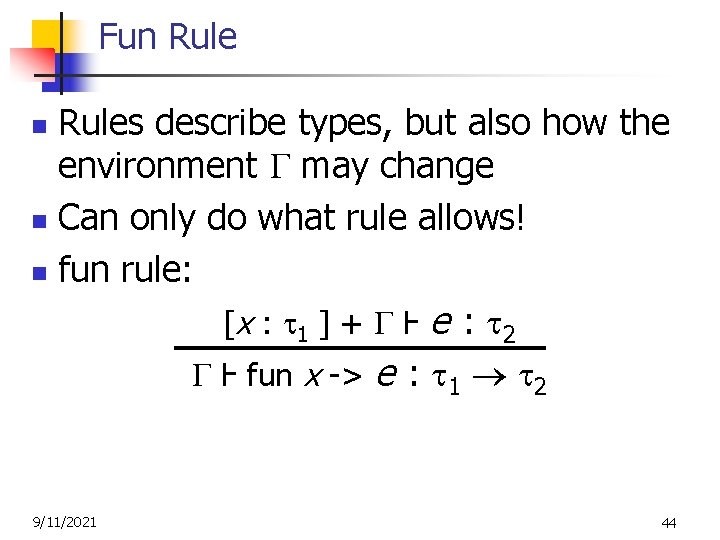
![Fun Examples [y : int ] + Ⱶ y + 3 : int Ⱶ Fun Examples [y : int ] + Ⱶ y + 3 : int Ⱶ](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-45.jpg)
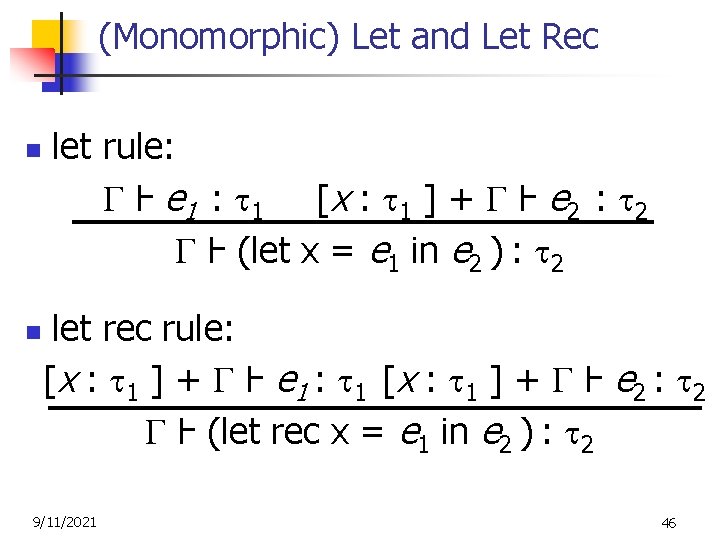
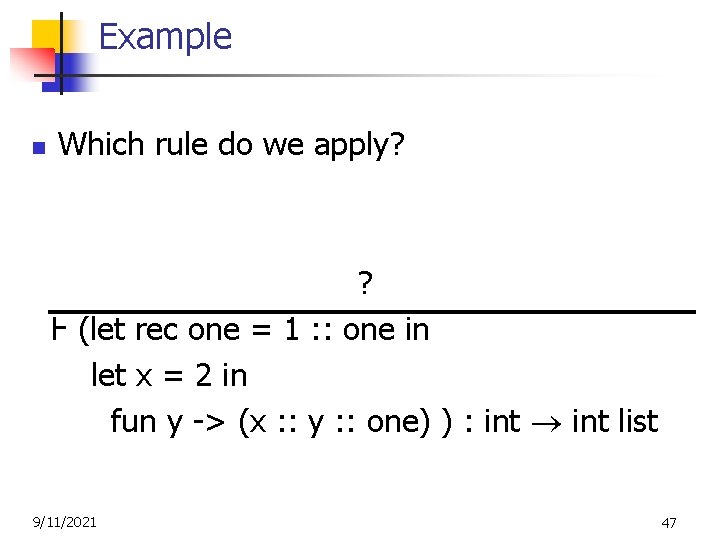
![Example Let rec rule: 2 [one : int list] Ⱶ 1 (let x = Example Let rec rule: 2 [one : int list] Ⱶ 1 (let x =](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-48.jpg)
![Proof of 1 n Which rule? [one : int list] Ⱶ (1 : : Proof of 1 n Which rule? [one : int list] Ⱶ (1 : :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-49.jpg)
![Proof of 1 n 3 Application 4 [one : int list] Ⱶ ((: : Proof of 1 n 3 Application 4 [one : int list] Ⱶ ((: :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-50.jpg)
![Proof of 3 Constants Rule [one : int list] Ⱶ (: : ) : Proof of 3 Constants Rule [one : int list] Ⱶ (: : ) :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-51.jpg)
![Proof of 4 n Rule for variables [one : int list] Ⱶ one : Proof of 4 n Rule for variables [one : int list] Ⱶ one :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-52.jpg)
![Proof of 2 5 [x : int; one : int list] Ⱶ n Constant Proof of 2 5 [x : int; one : int list] Ⱶ n Constant](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-53.jpg)
![Proof of 5 ? [x : int; one : int list] Ⱶ fun y Proof of 5 ? [x : int; one : int list] Ⱶ fun y](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-54.jpg)
![Proof of 5 ? [y : int; x : int; one : int list] Proof of 5 ? [y : int; x : int; one : int list]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-55.jpg)
![Proof of 5 6 7 [y: int; x: int; one : int list] Ⱶ Proof of 5 6 7 [y: int; x: int; one : int list] Ⱶ](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-56.jpg)
![Proof of 6 Constant Variable […] Ⱶ (: : ) : int list […; Proof of 6 Constant Variable […] Ⱶ (: : ) : int list […;](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-57.jpg)
![Proof of 7 Pf of 6 [y/x] Variable [y : int; …] Ⱶ ((: Proof of 7 Pf of 6 [y/x] Variable [y : int; …] Ⱶ ((:](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-58.jpg)
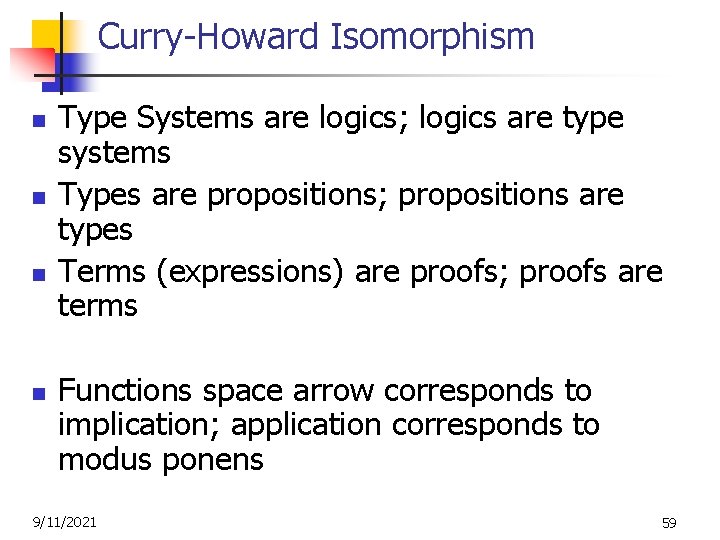
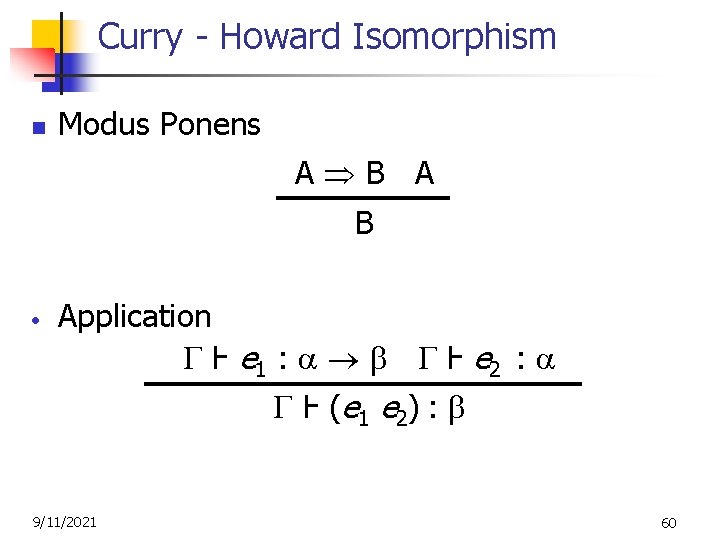
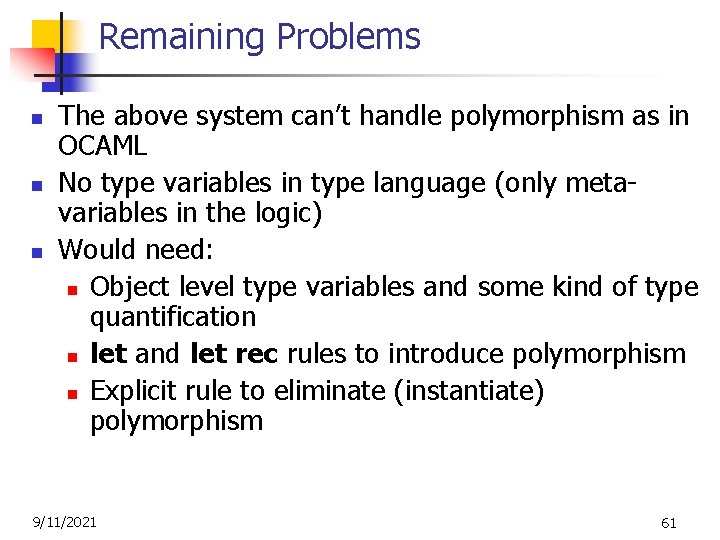
- Slides: 61
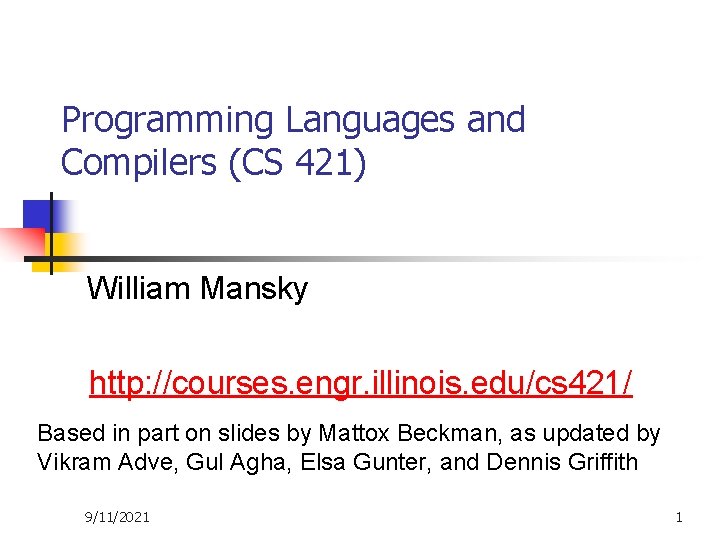
Programming Languages and Compilers (CS 421) William Mansky http: //courses. engr. illinois. edu/cs 421/ Based in part on slides by Mattox Beckman, as updated by Vikram Adve, Gul Agha, Elsa Gunter, and Dennis Griffith 9/11/2021 1
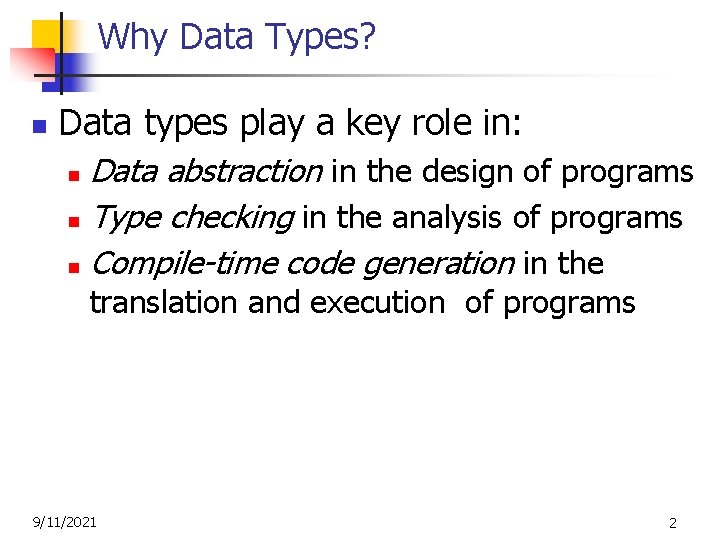
Why Data Types? n Data types play a key role in: Data abstraction in the design of programs n Type checking in the analysis of programs n Compile-time code generation in the n translation and execution of programs 9/11/2021 2
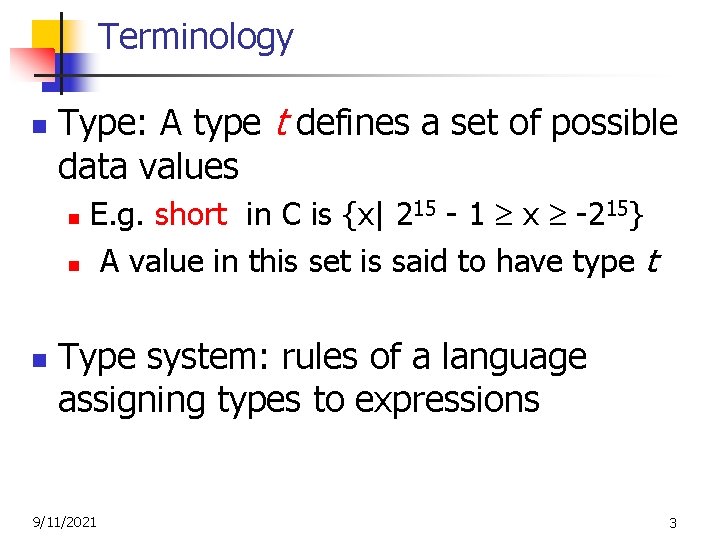
Terminology n Type: A type t defines a set of possible data values E. g. short in C is {x| 215 - 1 x -215} n A value in this set is said to have type t n n Type system: rules of a language assigning types to expressions 9/11/2021 3
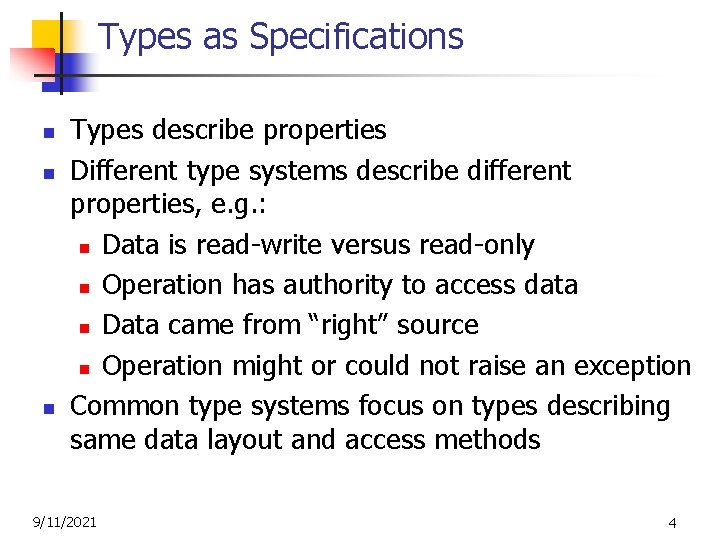
Types as Specifications n n n Types describe properties Different type systems describe different properties, e. g. : n Data is read-write versus read-only n Operation has authority to access data n Data came from “right” source n Operation might or could not raise an exception Common type systems focus on types describing same data layout and access methods 9/11/2021 4
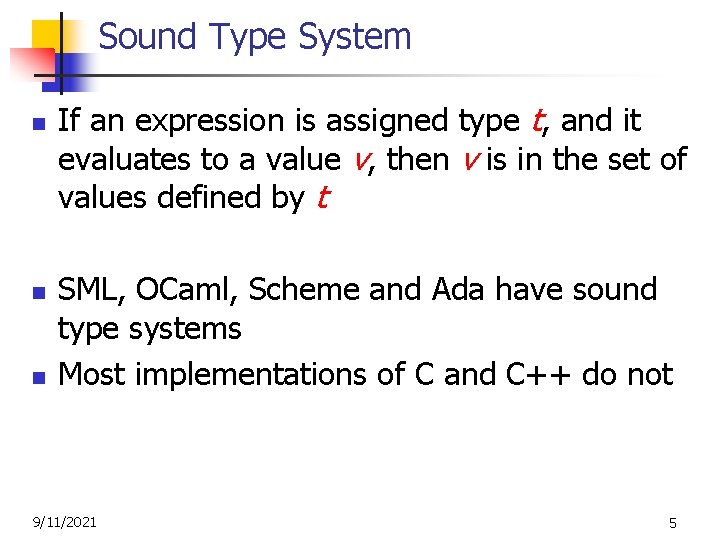
Sound Type System n n n If an expression is assigned type t, and it evaluates to a value v, then v is in the set of values defined by t SML, OCaml, Scheme and Ada have sound type systems Most implementations of C and C++ do not 9/11/2021 5
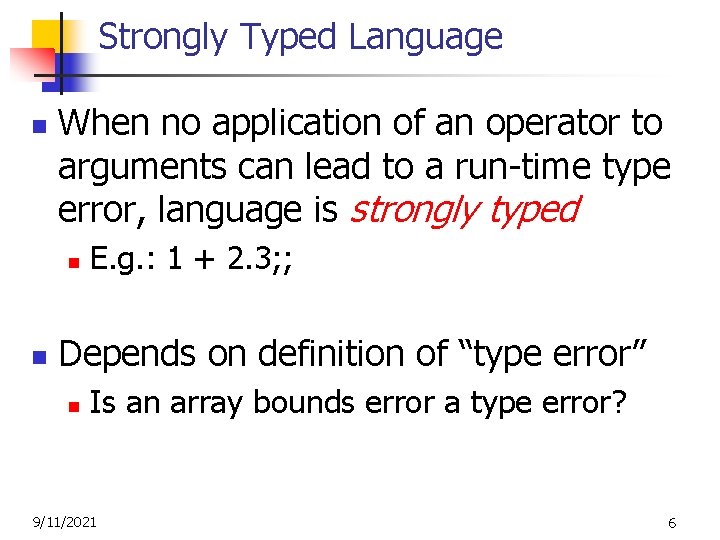
Strongly Typed Language n When no application of an operator to arguments can lead to a run-time type error, language is strongly typed n n E. g. : 1 + 2. 3; ; Depends on definition of “type error” n Is an array bounds error a type error? 9/11/2021 6
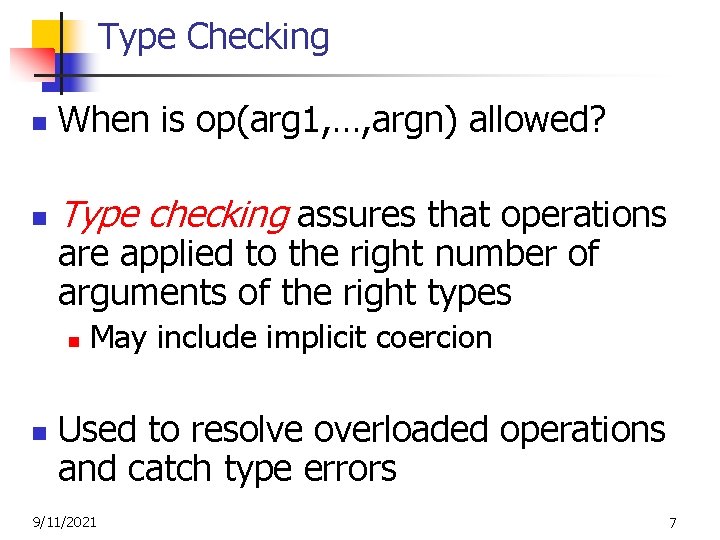
Type Checking n When is op(arg 1, …, argn) allowed? n Type checking assures that operations are applied to the right number of arguments of the right types n n May include implicit coercion Used to resolve overloaded operations and catch type errors 9/11/2021 7
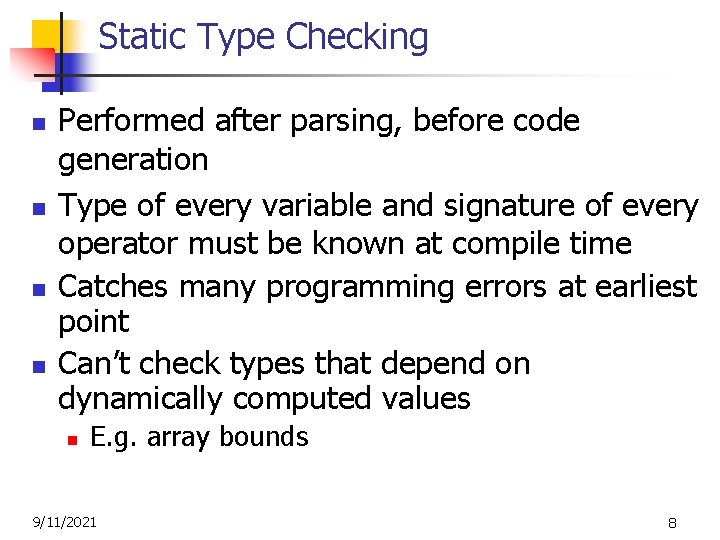
Static Type Checking n n Performed after parsing, before code generation Type of every variable and signature of every operator must be known at compile time Catches many programming errors at earliest point Can’t check types that depend on dynamically computed values n E. g. array bounds 9/11/2021 8
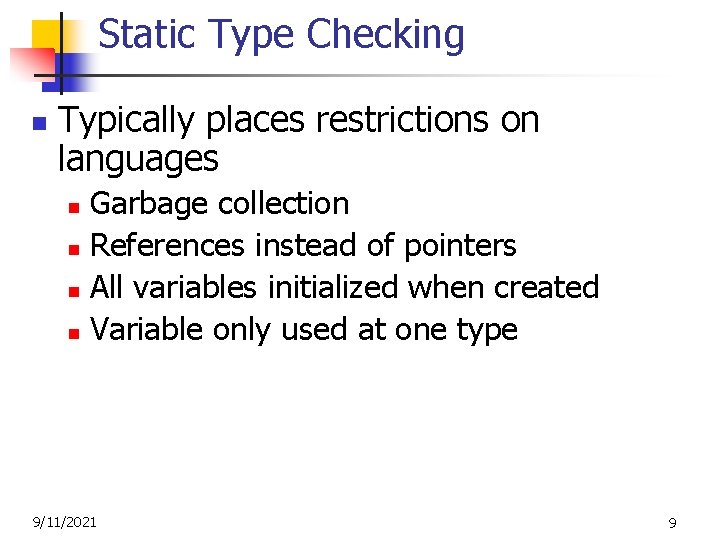
Static Type Checking n Typically places restrictions on languages Garbage collection n References instead of pointers n All variables initialized when created n Variable only used at one type n 9/11/2021 9
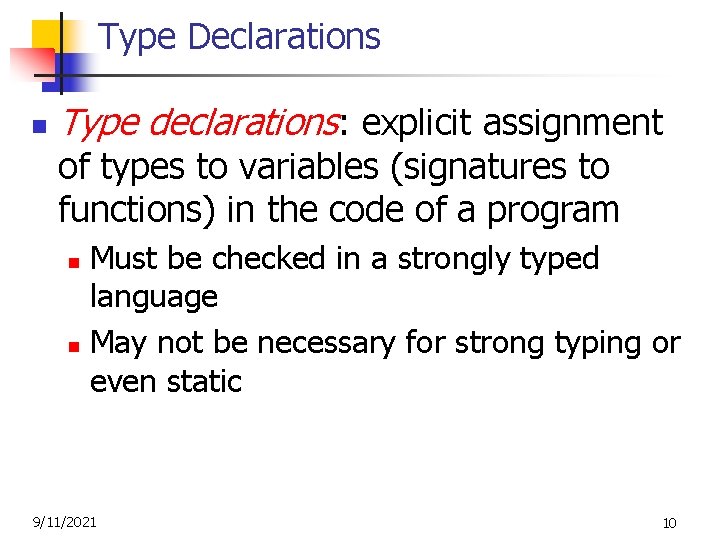
Type Declarations n Type declarations: explicit assignment of types to variables (signatures to functions) in the code of a program Must be checked in a strongly typed language n May not be necessary for strong typing or even static n 9/11/2021 10
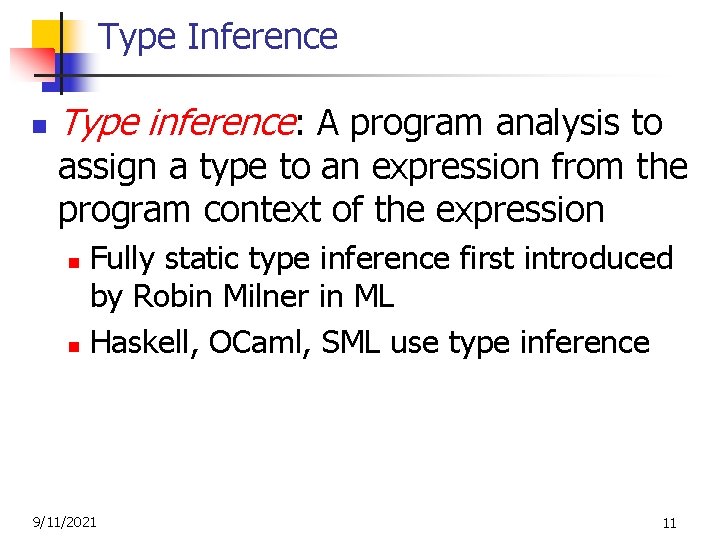
Type Inference n Type inference: A program analysis to assign a type to an expression from the program context of the expression Fully static type inference first introduced by Robin Milner in ML n Haskell, OCaml, SML use type inference n 9/11/2021 11
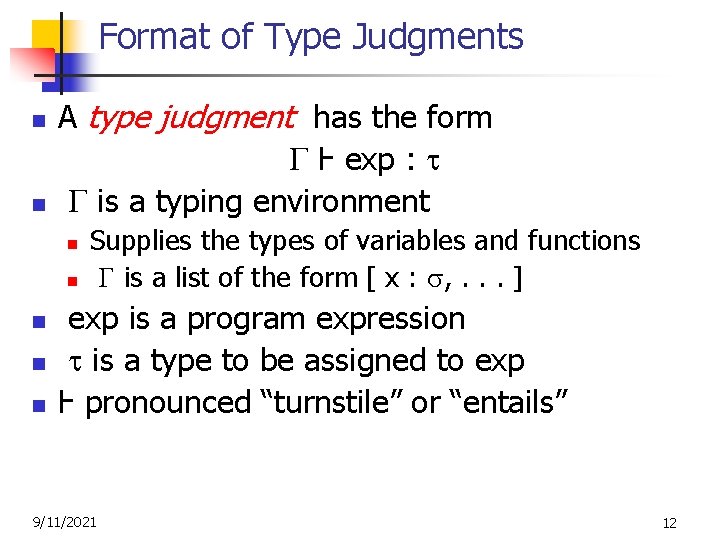
Format of Type Judgments n n A type judgment has the form Ⱶ exp : is a typing environment n n n Supplies the types of variables and functions is a list of the form [ x : , . . . ] exp is a program expression is a type to be assigned to exp Ⱶ pronounced “turnstile” or “entails” 9/11/2021 12
![Example Valid Type Judgments n n n Ⱶ true or false Example Valid Type Judgments n n n [ ] Ⱶ true or false :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-13.jpg)
Example Valid Type Judgments n n n [ ] Ⱶ true or false : bool [ x : int ] Ⱶ x + 3 : int [ p : int -> string ] Ⱶ p 5 : string 9/11/2021 13
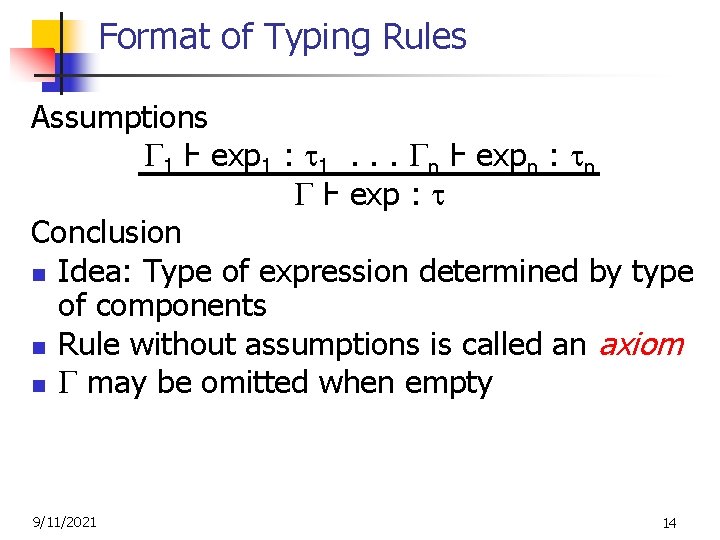
Format of Typing Rules Assumptions 1 Ⱶ exp 1 : 1. . . n Ⱶ expn : n Ⱶ exp : Conclusion n Idea: Type of expression determined by type of components n Rule without assumptions is called an axiom n may be omitted when empty 9/11/2021 14
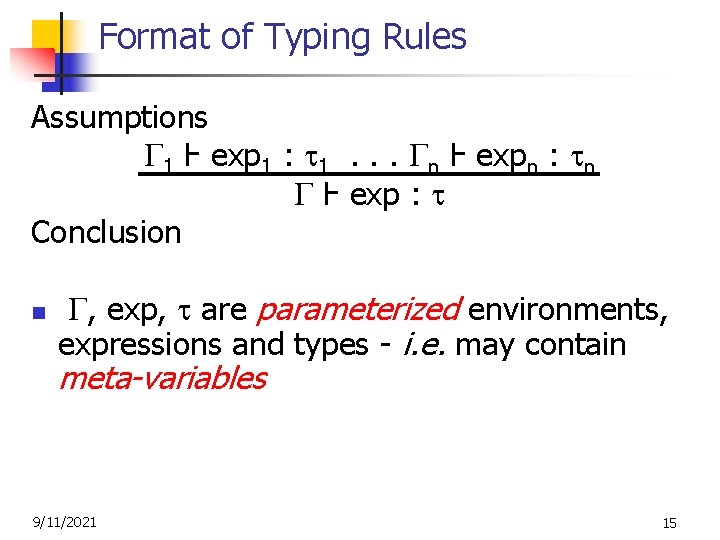
Format of Typing Rules Assumptions 1 Ⱶ exp 1 : 1. . . n Ⱶ expn : n Ⱶ exp : Conclusion n , exp, are parameterized environments, expressions and types - i. e. may contain meta-variables 9/11/2021 15
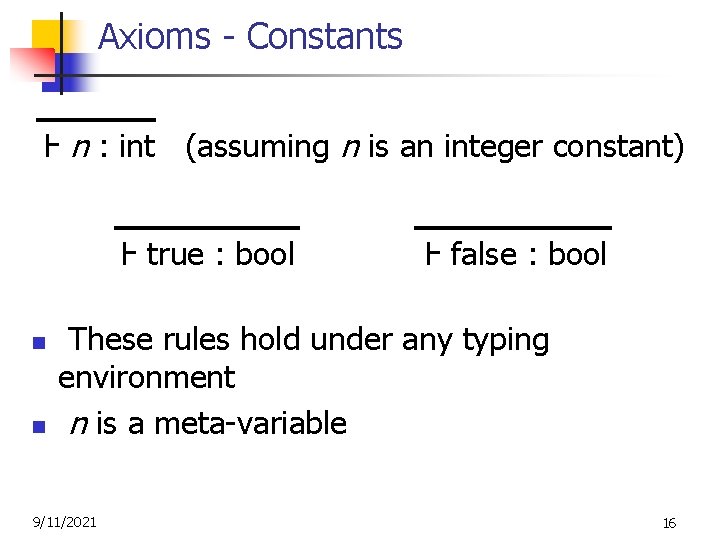
Axioms - Constants Ⱶ n : int (assuming n is an integer constant) Ⱶ true : bool n n Ⱶ false : bool These rules hold under any typing environment n is a meta-variable 9/11/2021 16
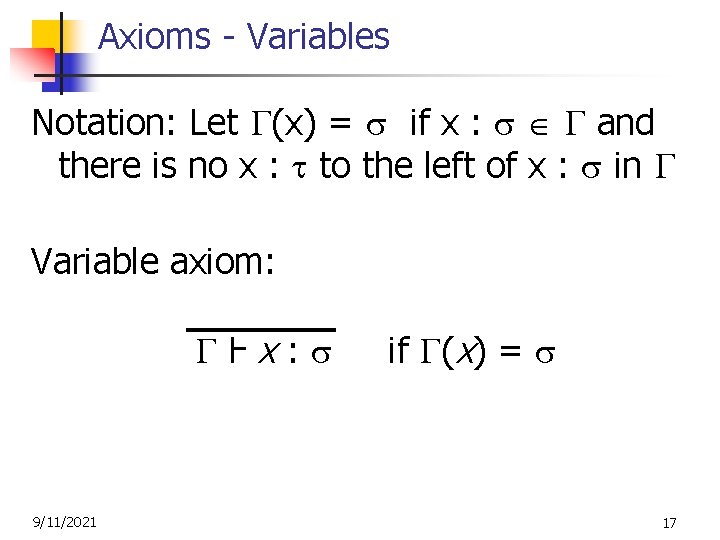
Axioms - Variables Notation: Let (x) = if x : and there is no x : to the left of x : in Variable axiom: Ⱶx: 9/11/2021 if (x) = 17
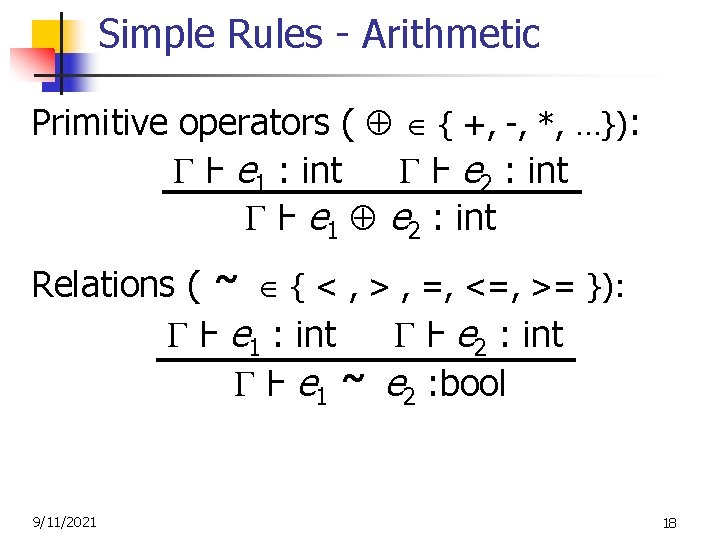
Simple Rules - Arithmetic Primitive operators ( { +, -, *, …}): Ⱶ e 1 : int Ⱶ e 2 : int Ⱶ e 1 e 2 : int Relations ( { < , > , =, <=, >= }): ˜ Ⱶ e 1 : int Ⱶ e 2 : int Ⱶ e 1 e 2 : bool ˜ 9/11/2021 18
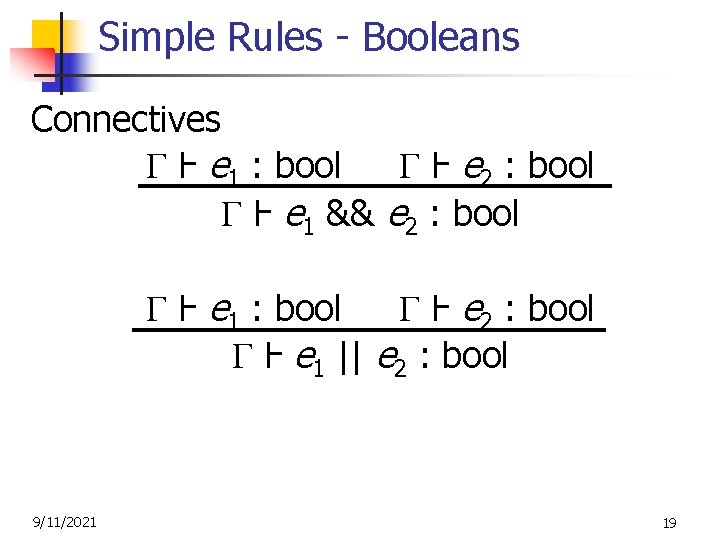
Simple Rules - Booleans Connectives Ⱶ e 1 : bool Ⱶ e 2 : bool Ⱶ e 1 && e 2 : bool Ⱶ e 1 : bool Ⱶ e 2 : bool Ⱶ e 1 || e 2 : bool 9/11/2021 19
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-20.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Start building the proof tree from the bottom up ? Ⱶ y || (x + 3 > 6) : bool 9/11/2021 20
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-21.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Which rule has this as a conclusion? ? Ⱶ y || (x + 3 > 6) : bool 9/11/2021 21
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-22.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Booleans: || Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 22
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-23.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 23
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-24.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Which rule has this as a conclusion? ? Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 24
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-25.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Axiom for variables Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 25
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-26.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 26
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-27.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Which rule has this as a conclusion? ? Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 27
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-28.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Arithmetic relations Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 28
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-29.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 29
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-30.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Which rule has this as a conclusion? ? Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 30
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-31.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Axiom for constants Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 31
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-32.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 32
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-33.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Which rule has this as a conclusion? ? Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 33
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-34.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Arithmetic operations Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 34
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-35.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 35
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-36.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 36
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-37.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Axiom for constants Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 37
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-38.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Pick an assumption to prove ? Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 38
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-39.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool Axiom for variables Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 39
![Simple Example n n n Let x int y bool Simple Example n n n Let = [ x: int ; y: bool ]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-40.jpg)
Simple Example n n n Let = [ x: int ; y: bool ] Show Ⱶ y || (x + 3 > 6) : bool No more assumptions! QED! Ⱶ x : int Ⱶ 3 : int Ⱶ x + 3 : int Ⱶ 6 : int Ⱶ y : bool Ⱶ x + 3 > 6 : bool Ⱶ y || (x + 3 > 6) : bool 9/11/2021 40
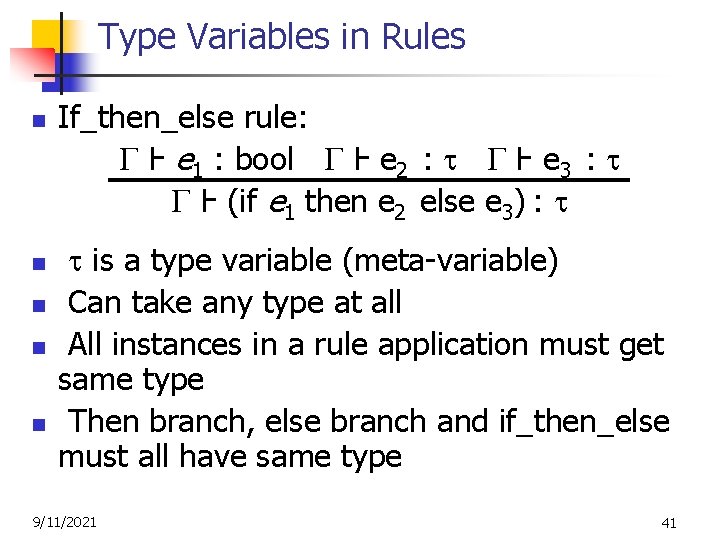
Type Variables in Rules n n n If_then_else rule: Ⱶ e 1 : bool Ⱶ e 2 : Ⱶ e 3 : Ⱶ (if e 1 then e 2 else e 3) : is a type variable (meta-variable) Can take any type at all All instances in a rule application must get same type Then branch, else branch and if_then_else must all have same type 9/11/2021 41
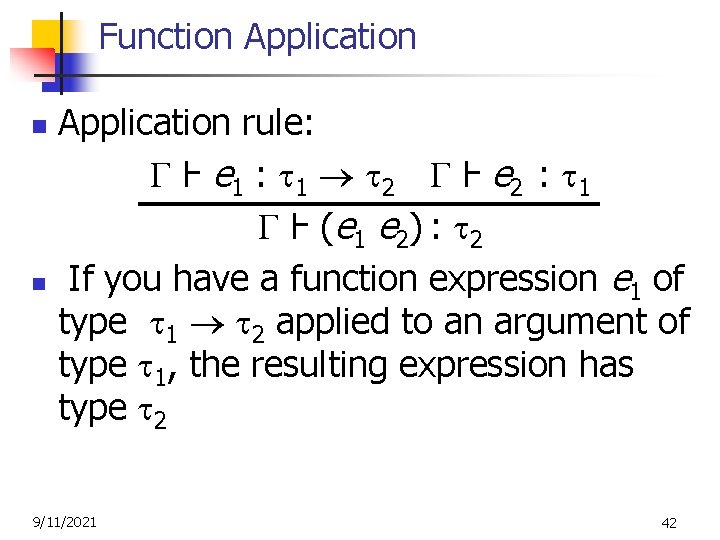
Function Application n n Application rule: Ⱶ e 1 : 1 2 Ⱶ e 2 : 1 Ⱶ ( e 1 e 2 ) : 2 If you have a function expression e 1 of type 1 2 applied to an argument of type 1, the resulting expression has type 2 9/11/2021 42
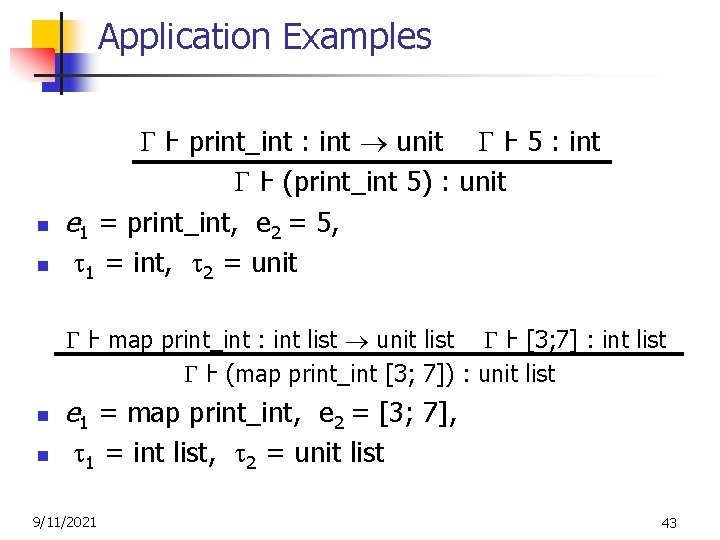
Application Examples n n Ⱶ print_int : int unit Ⱶ 5 : int Ⱶ (print_int 5) : unit e 1 = print_int, e 2 = 5, 1 = int, 2 = unit Ⱶ map print_int : int list unit list Ⱶ [3; 7] : int list Ⱶ (map print_int [3; 7]) : unit list n n e 1 = map print_int, e 2 = [3; 7], 1 = int list, 2 = unit list 9/11/2021 43
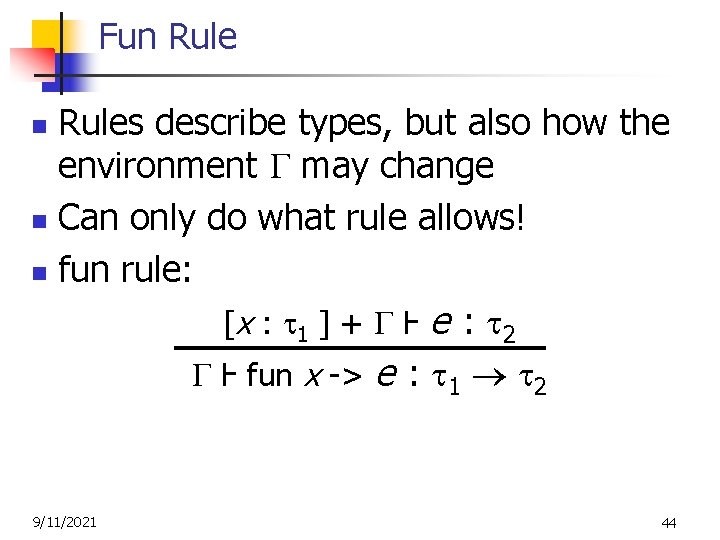
Fun Rules describe types, but also how the environment may change n Can only do what rule allows! n fun rule: [ x : 1 ] + Ⱶ e : 2 Ⱶ fun x -> e : 1 2 n 9/11/2021 44
![Fun Examples y int Ⱶ y 3 int Ⱶ Fun Examples [y : int ] + Ⱶ y + 3 : int Ⱶ](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-45.jpg)
Fun Examples [y : int ] + Ⱶ y + 3 : int Ⱶ fun y -> y + 3 : int [f : int bool ] + Ⱶ f 2 : : [true] : bool list Ⱶ (fun f -> f 2 : : [true]) : (int bool) bool list 9/11/2021 45
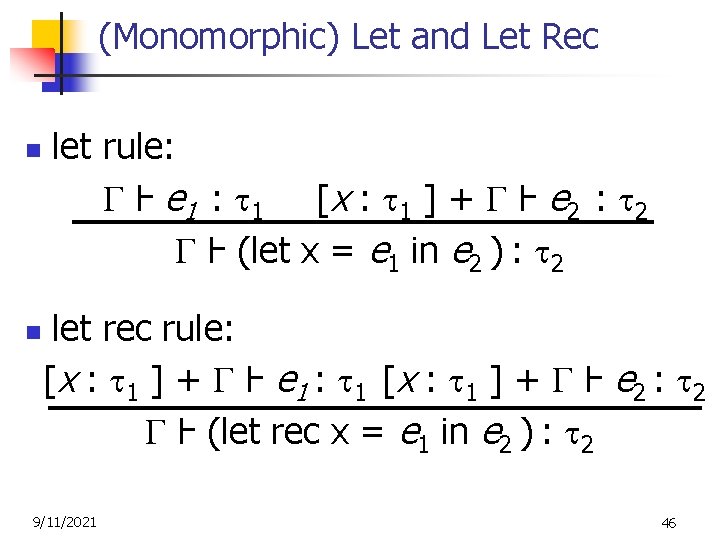
(Monomorphic) Let and Let Rec n n let rule: Ⱶ e 1 : 1 [ x : 1 ] + Ⱶ e 2 : 2 Ⱶ (let x = e 1 in e 2 ) : 2 let rec rule: [ x : 1 ] + Ⱶ e 1 : 1 [ x : 1 ] + Ⱶ e 2 : 2 Ⱶ (let rec x = e 1 in e 2 ) : 2 9/11/2021 46
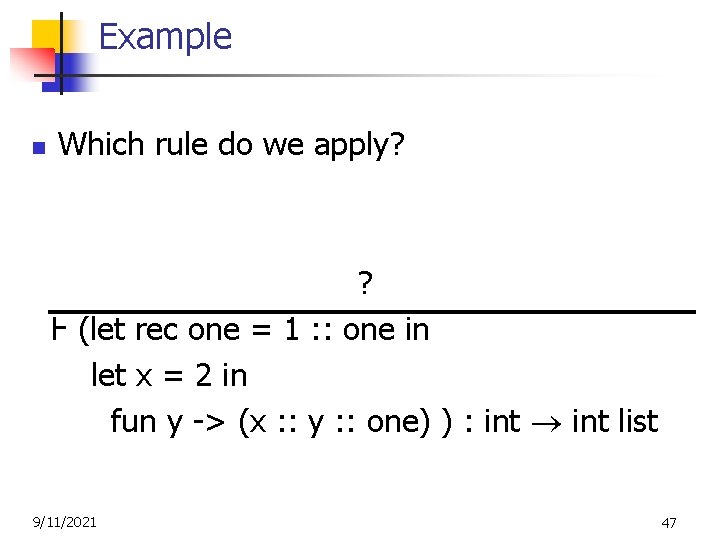
Example n Which rule do we apply? ? Ⱶ (let rec one = 1 : : one in let x = 2 in fun y -> (x : : y : : one) ) : int list 9/11/2021 47
![Example Let rec rule 2 one int list Ⱶ 1 let x Example Let rec rule: 2 [one : int list] Ⱶ 1 (let x =](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-48.jpg)
Example Let rec rule: 2 [one : int list] Ⱶ 1 (let x = 2 in [one : int list] Ⱶ fun y -> (x : : y : : one)) (1 : : one) : int list Ⱶ (let rec one = 1 : : one in let x = 2 in fun y -> (x : : y : : one)) : int list n 9/11/2021 48
![Proof of 1 n Which rule one int list Ⱶ 1 Proof of 1 n Which rule? [one : int list] Ⱶ (1 : :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-49.jpg)
Proof of 1 n Which rule? [one : int list] Ⱶ (1 : : one) : int list 9/11/2021 49
![Proof of 1 n 3 Application 4 one int list Ⱶ Proof of 1 n 3 Application 4 [one : int list] Ⱶ ((: :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-50.jpg)
Proof of 1 n 3 Application 4 [one : int list] Ⱶ ((: : ) 1) : int list one : int list [one : int list] Ⱶ (1 : : one) : int list 9/11/2021 50
![Proof of 3 Constants Rule one int list Ⱶ Proof of 3 Constants Rule [one : int list] Ⱶ (: : ) :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-51.jpg)
Proof of 3 Constants Rule [one : int list] Ⱶ (: : ) : int list 1 : int [one : int list] Ⱶ ((: : ) 1) : int list 9/11/2021 51
![Proof of 4 n Rule for variables one int list Ⱶ one Proof of 4 n Rule for variables [one : int list] Ⱶ one :](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-52.jpg)
Proof of 4 n Rule for variables [one : int list] Ⱶ one : int list 9/11/2021 52
![Proof of 2 5 x int one int list Ⱶ n Constant Proof of 2 5 [x : int; one : int list] Ⱶ n Constant](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-53.jpg)
Proof of 2 5 [x : int; one : int list] Ⱶ n Constant fun y -> (x : : y : : one)) [one : int list] Ⱶ 2: int list [one : int list] Ⱶ (let x = 2 in fun y -> (x : : y : : one)) : int list 9/11/2021 53
![Proof of 5 x int one int list Ⱶ fun y Proof of 5 ? [x : int; one : int list] Ⱶ fun y](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-54.jpg)
Proof of 5 ? [x : int; one : int list] Ⱶ fun y -> (x : : y : : one)) : int list 9/11/2021 54
![Proof of 5 y int x int one int list Proof of 5 ? [y : int; x : int; one : int list]](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-55.jpg)
Proof of 5 ? [y : int; x : int; one : int list] Ⱶ (x : : y : : one) : int list [x : int; one : int list] Ⱶ fun y -> (x : : y : : one)) : int list 9/11/2021 55
![Proof of 5 6 7 y int x int one int list Ⱶ Proof of 5 6 7 [y: int; x: int; one : int list] Ⱶ](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-56.jpg)
Proof of 5 6 7 [y: int; x: int; one : int list] Ⱶ ((: : ) x): int list (y : : one) : int list [y : int; x: int; one : int list] Ⱶ (x : : y : : one) : int list [x : int; one : int list] Ⱶ fun y -> (x : : y : : one)) : int list 9/11/2021 56
![Proof of 6 Constant Variable Ⱶ int list Proof of 6 Constant Variable […] Ⱶ (: : ) : int list […;](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-57.jpg)
Proof of 6 Constant Variable […] Ⱶ (: : ) : int list […; x : int; …] Ⱶ x: int [y : int; x : int; one : int list] Ⱶ ((: : ) x) : int list 9/11/2021 57
![Proof of 7 Pf of 6 yx Variable y int Ⱶ Proof of 7 Pf of 6 [y/x] Variable [y : int; …] Ⱶ ((:](https://slidetodoc.com/presentation_image_h2/a186556e7c5791d434f5e0bd118241b8/image-58.jpg)
Proof of 7 Pf of 6 [y/x] Variable [y : int; …] Ⱶ ((: : ) y) […; one: int list] Ⱶ : int list one : int list [y : int; x : int; one : int list] Ⱶ (y : : one) : int list 9/11/2021 58
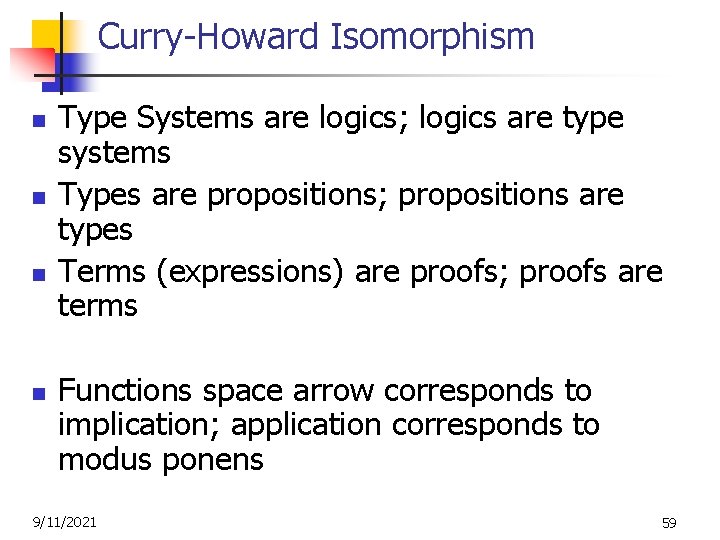
Curry-Howard Isomorphism n n Type Systems are logics; logics are type systems Types are propositions; propositions are types Terms (expressions) are proofs; proofs are terms Functions space arrow corresponds to implication; application corresponds to modus ponens 9/11/2021 59
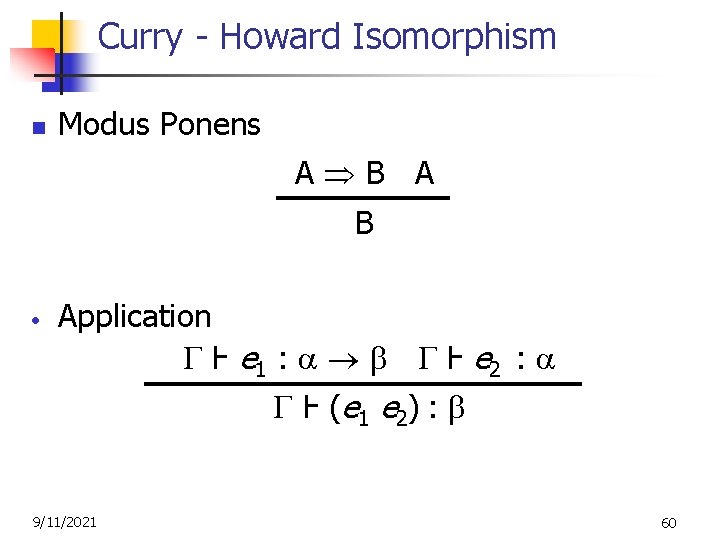
Curry - Howard Isomorphism n Modus Ponens A B • Application Ⱶ e 1 : Ⱶ e 2 : Ⱶ ( e 1 e 2 ) : 9/11/2021 60
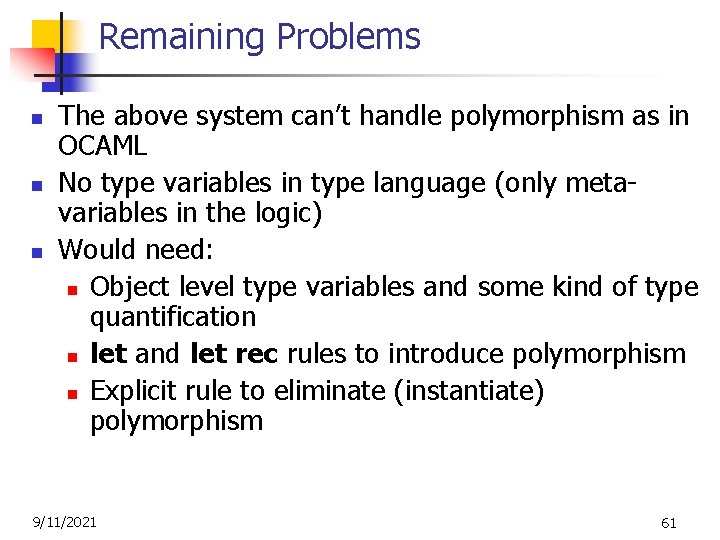
Remaining Problems n n n The above system can’t handle polymorphism as in OCAML No type variables in type language (only metavariables in the logic) Would need: n Object level type variables and some kind of type quantification n let and let rec rules to introduce polymorphism n Explicit rule to eliminate (instantiate) polymorphism 9/11/2021 61
Cs 421
Cs 421 uiuc
William mansky
William mansky
William mansky
Pros and cons of compilers and interpreters
Finding and understanding bugs in c compilers
Compilers: principles, techniques, and tools
Introduction to compiler construction
Real-time systems and programming languages
Advantages and disadvantages of programming languages
Real time programming language
C++ binarymove
Cross compilers
Crafting a compiler with c
Functions of compilers
Front end of a compiler
Real time example of multithreading in java
Programming languages levels
Introduction to programming languages
Plc programming languages
Joey paquet
Imperative programming languages
Alternative programming languages
Types of programming languages
Transmission programming languages
Adam doupe cse 340
Integral data types
Xenia programming languages
Mainstream programming languages
Cse 340 principles of programming languages
Programing languages
Programming languages
Programming languages
Programming languages
Tiny programming language
Brief history of programming languages
Lisp_q
Xkcd programming
If programming languages were cars
Reasons for studying concepts of programming languages
Cornell programming languages
Low level programming language
Middle level programming languages
The art of programming language
Multimedia programming languages
Storage management in programming languages
421 could not create socket
Hematocrit normal
Ist 421
Here is where your presentation begins
Rule4ml
421 rule
421 rule maintenance fluids
Uw cse 421
Fwm 421
Fwm 421
Business process integration definition
Cs 421 uiuc
Ist 421
Comp421
Cmsc 421