Procedures 2 a MIPS code examples Making Use
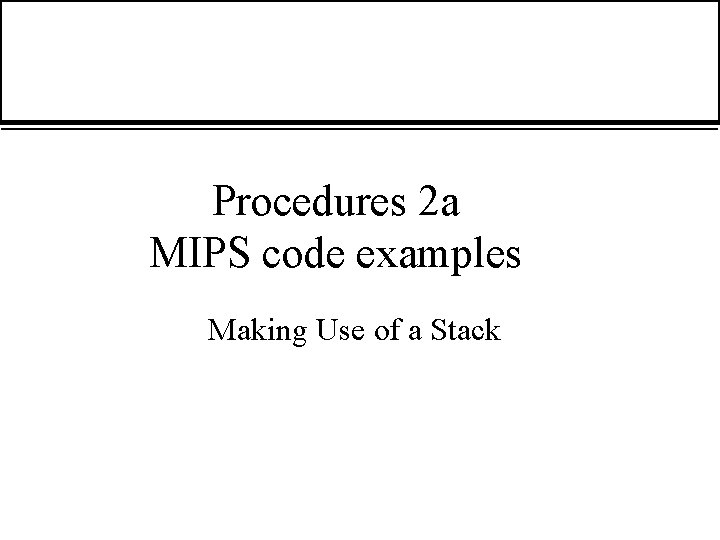
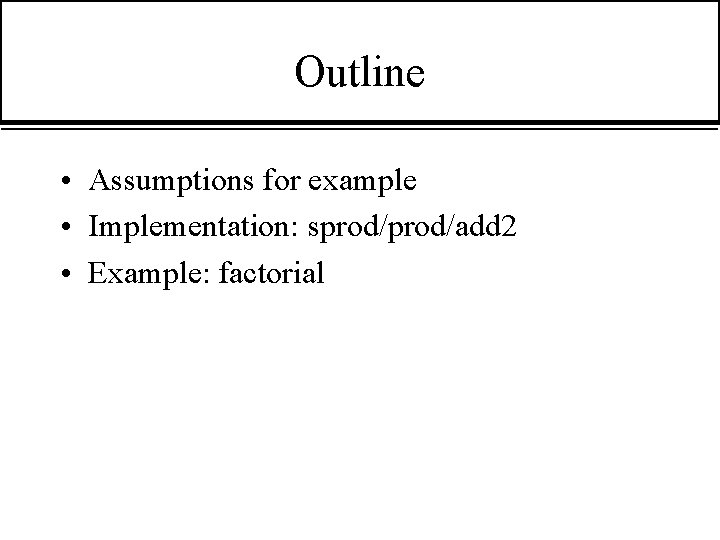
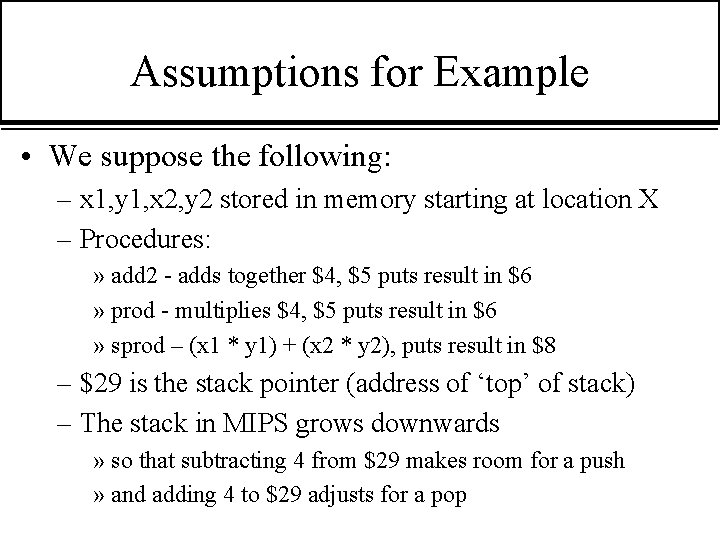
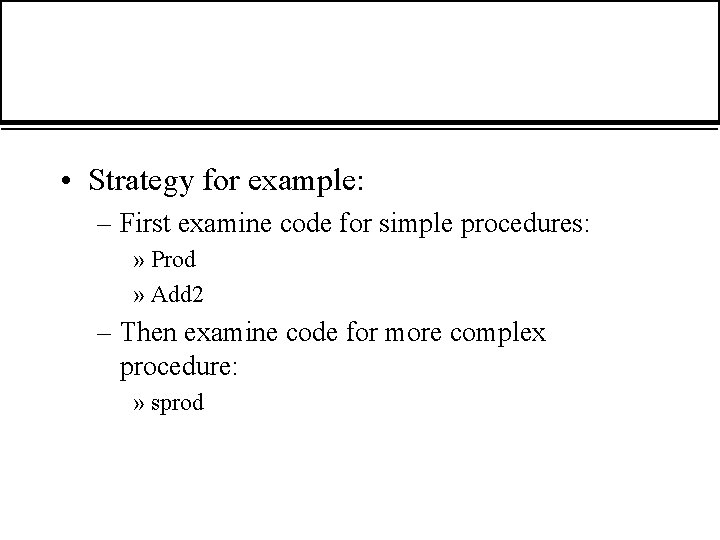
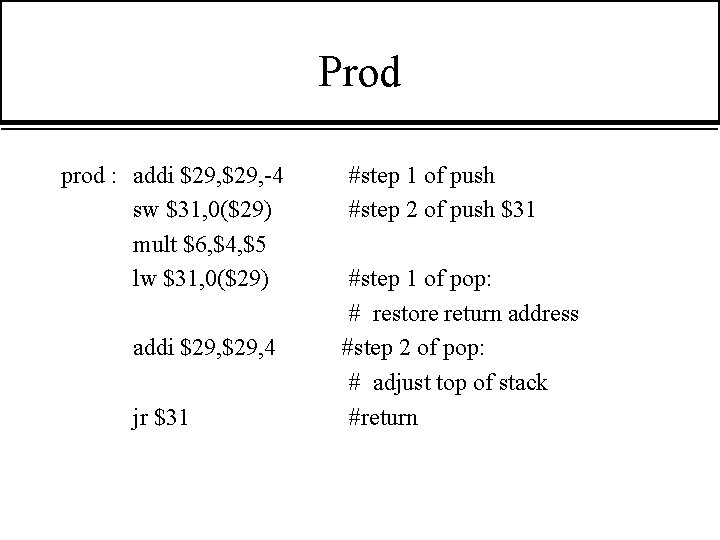
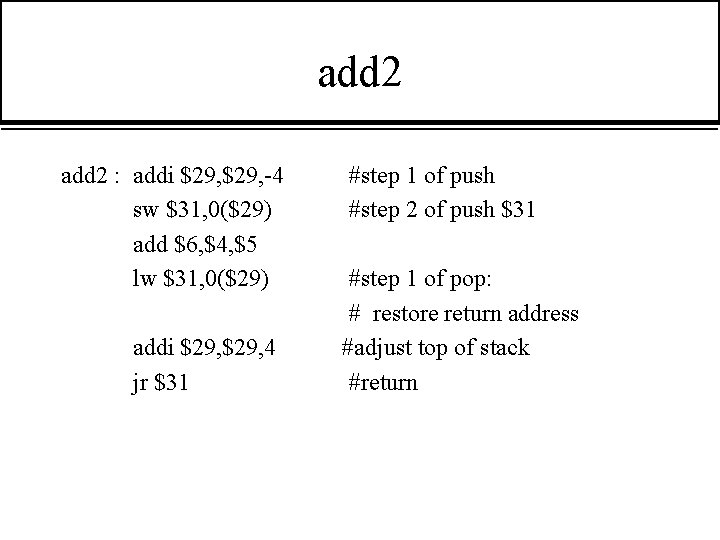
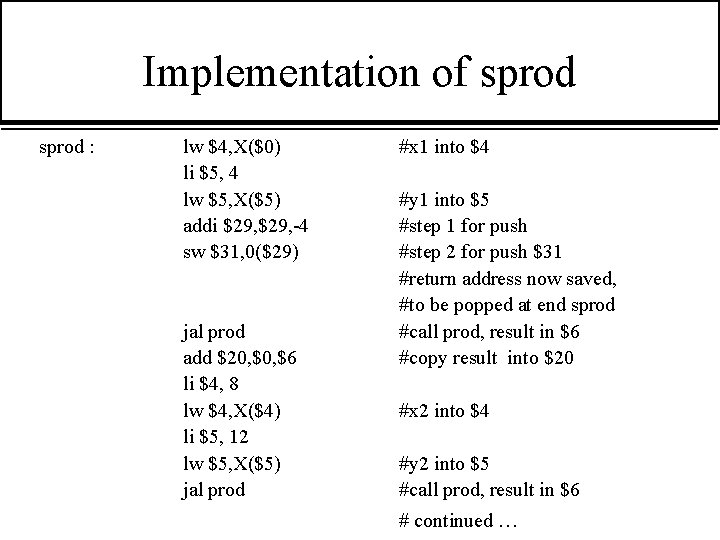
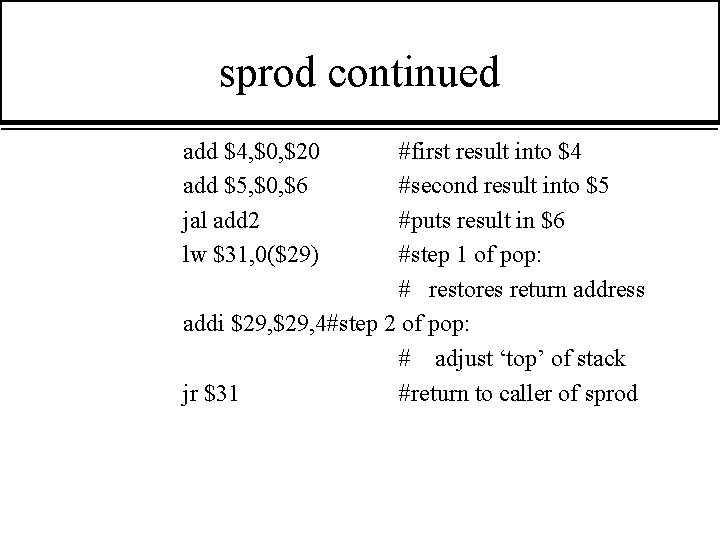
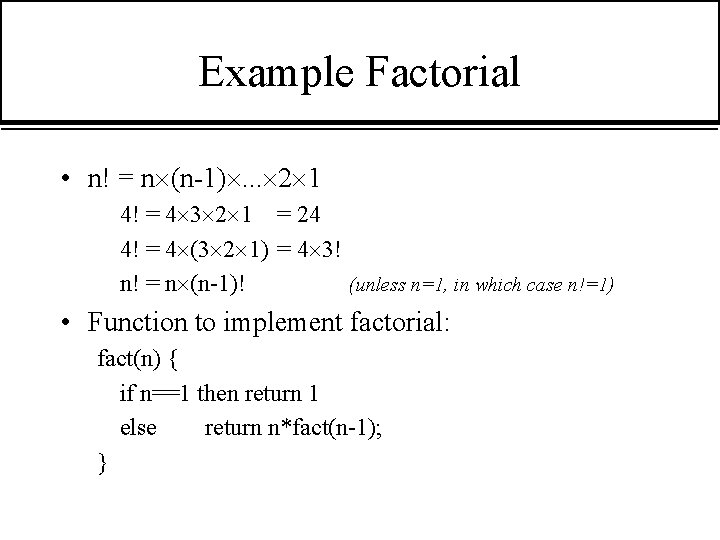
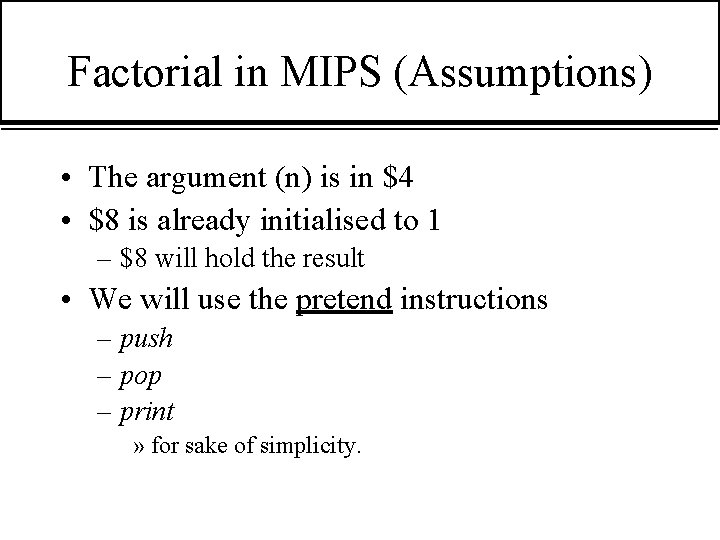
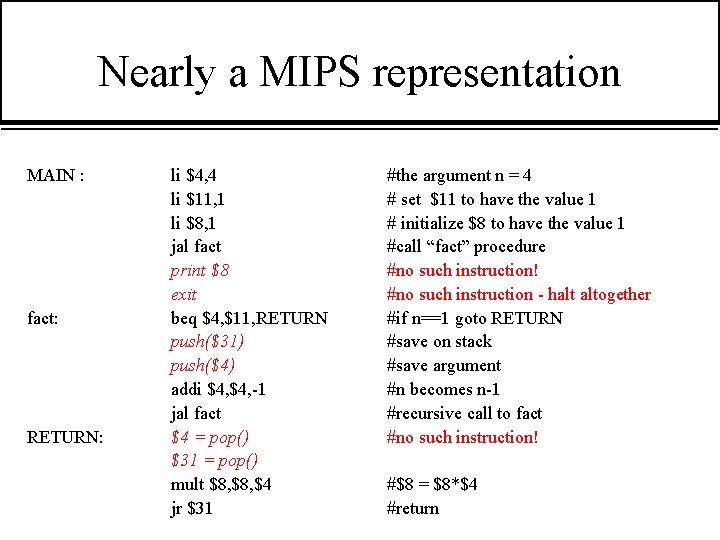
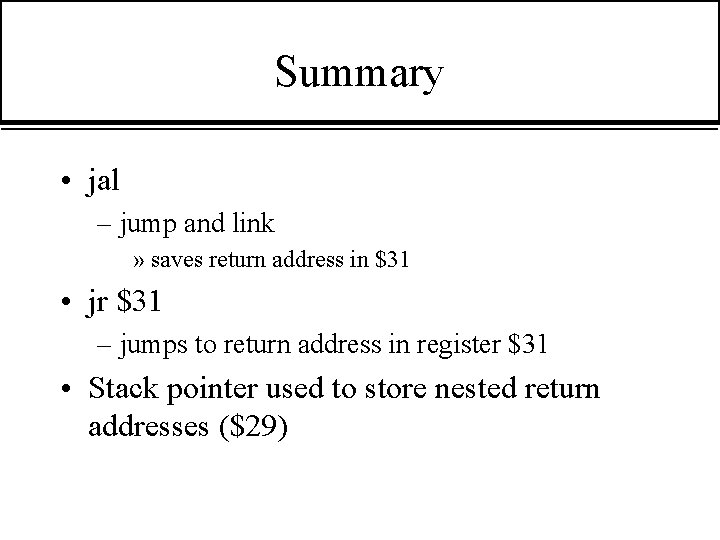
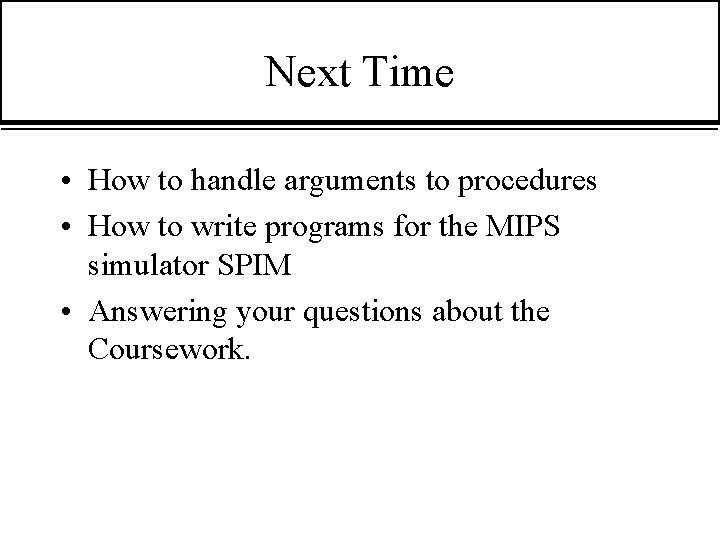
- Slides: 13
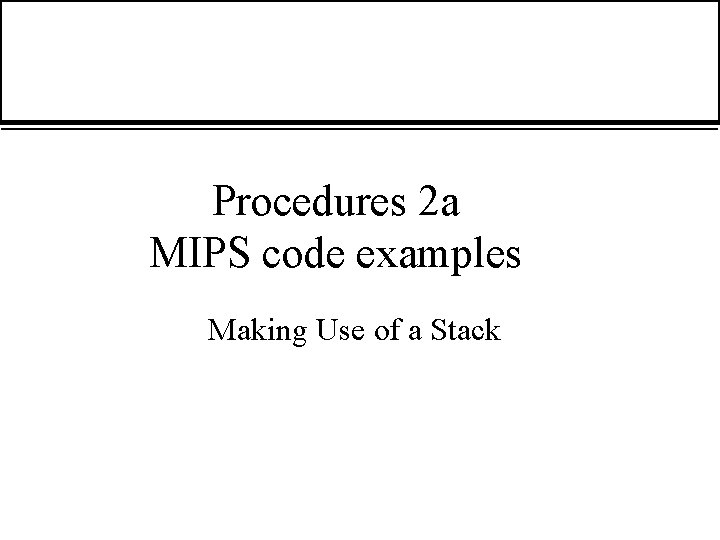
Procedures 2 a MIPS code examples Making Use of a Stack
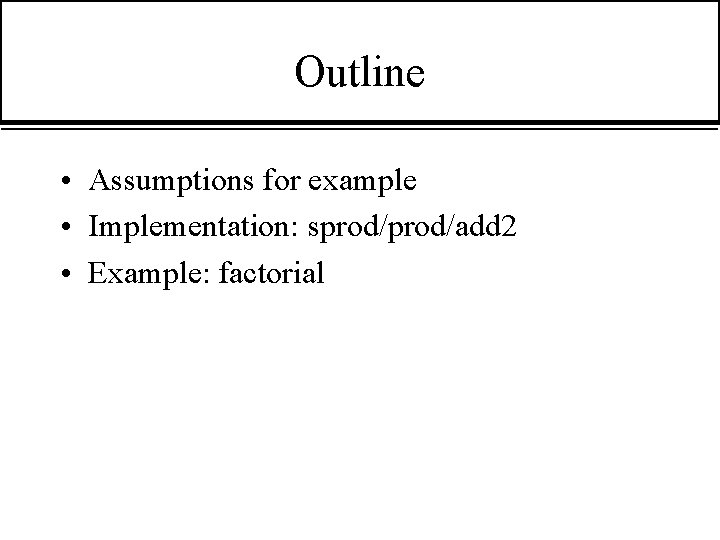
Outline • Assumptions for example • Implementation: sprod/add 2 • Example: factorial
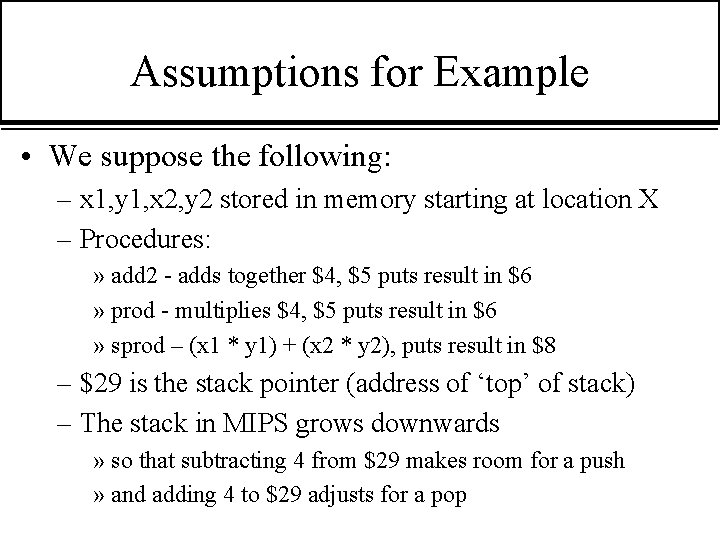
Assumptions for Example • We suppose the following: – x 1, y 1, x 2, y 2 stored in memory starting at location X – Procedures: » add 2 - adds together $4, $5 puts result in $6 » prod - multiplies $4, $5 puts result in $6 » sprod – (x 1 * y 1) + (x 2 * y 2), puts result in $8 – $29 is the stack pointer (address of ‘top’ of stack) – The stack in MIPS grows downwards » so that subtracting 4 from $29 makes room for a push » and adding 4 to $29 adjusts for a pop
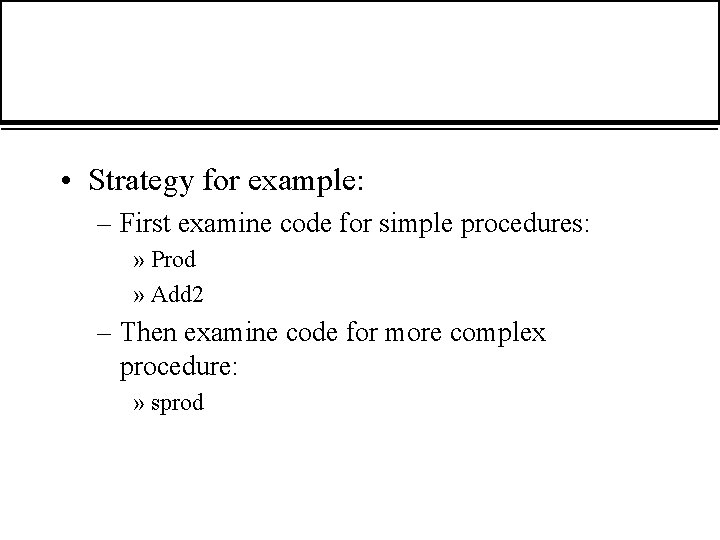
• Strategy for example: – First examine code for simple procedures: » Prod » Add 2 – Then examine code for more complex procedure: » sprod
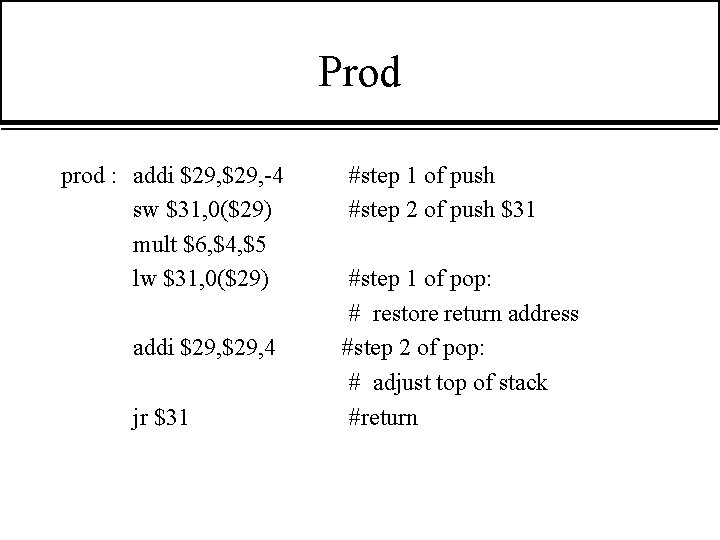
Prod prod : addi $29, -4 sw $31, 0($29) mult $6, $4, $5 lw $31, 0($29) addi $29, 4 jr $31 #step 1 of push #step 2 of push $31 #step 1 of pop: # restore return address #step 2 of pop: # adjust top of stack #return
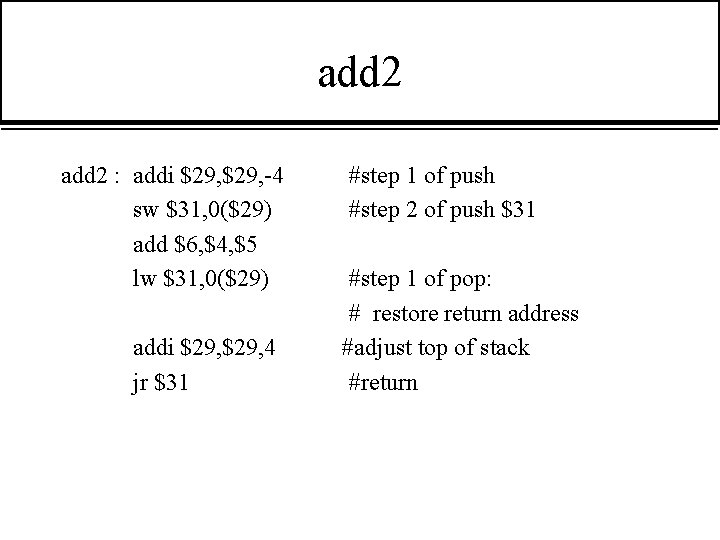
add 2 : addi $29, -4 sw $31, 0($29) add $6, $4, $5 lw $31, 0($29) addi $29, 4 jr $31 #step 1 of push #step 2 of push $31 #step 1 of pop: # restore return address #adjust top of stack #return
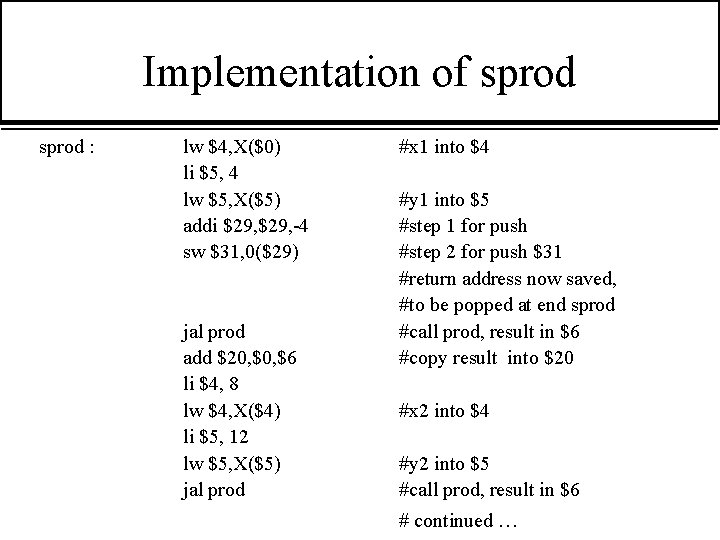
Implementation of sprod : lw $4, X($0) li $5, 4 lw $5, X($5) addi $29, -4 sw $31, 0($29) jal prod add $20, $6 li $4, 8 lw $4, X($4) li $5, 12 lw $5, X($5) jal prod #x 1 into $4 #y 1 into $5 #step 1 for push #step 2 for push $31 #return address now saved, #to be popped at end sprod #call prod, result in $6 #copy result into $20 #x 2 into $4 #y 2 into $5 #call prod, result in $6 # continued …
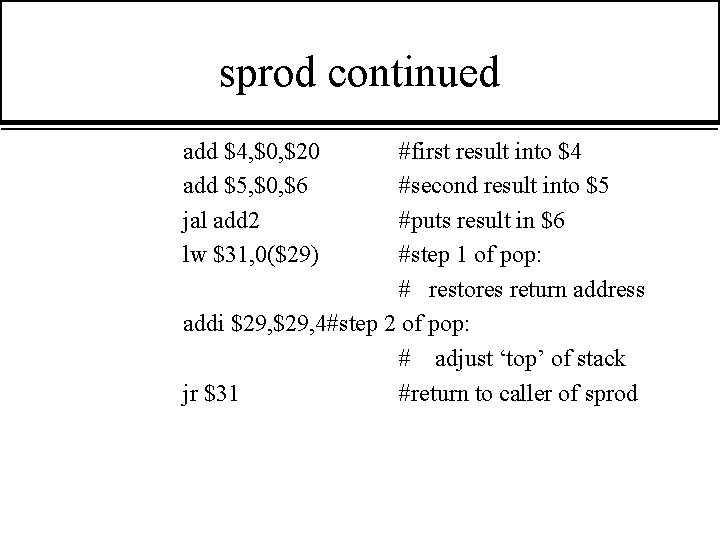
sprod continued add $4, $0, $20 add $5, $0, $6 jal add 2 lw $31, 0($29) #first result into $4 #second result into $5 #puts result in $6 #step 1 of pop: # restores return address addi $29, 4#step 2 of pop: # adjust ‘top’ of stack jr $31 #return to caller of sprod
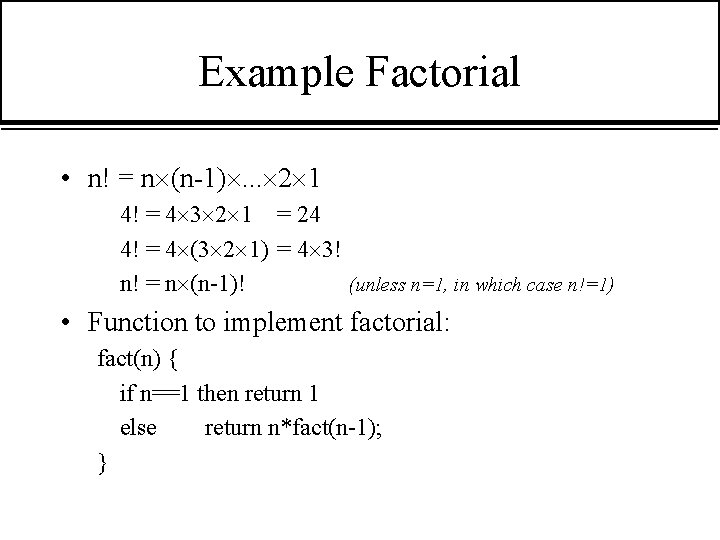
Example Factorial • n! = n (n-1). . . 2 1 4! = 4 3 2 1 = 24 4! = 4 (3 2 1) = 4 3! n! = n (n-1)! (unless n=1, in which case n!=1) • Function to implement factorial: fact(n) { if n==1 then return 1 else return n*fact(n-1); }
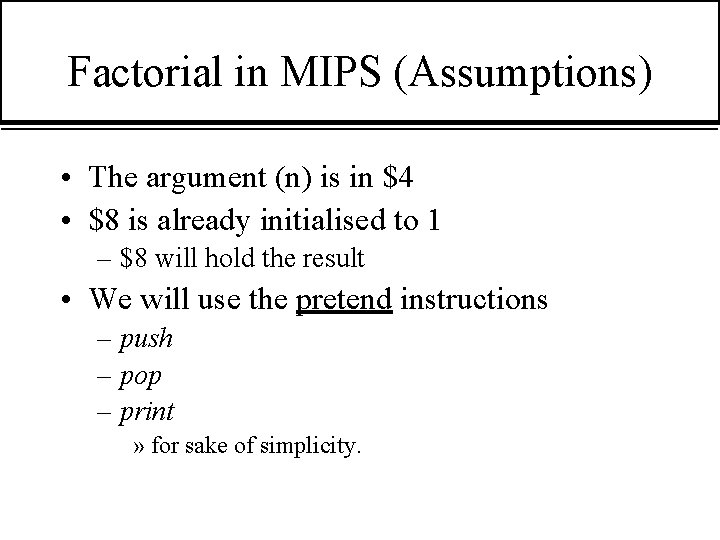
Factorial in MIPS (Assumptions) • The argument (n) is in $4 • $8 is already initialised to 1 – $8 will hold the result • We will use the pretend instructions – push – pop – print » for sake of simplicity.
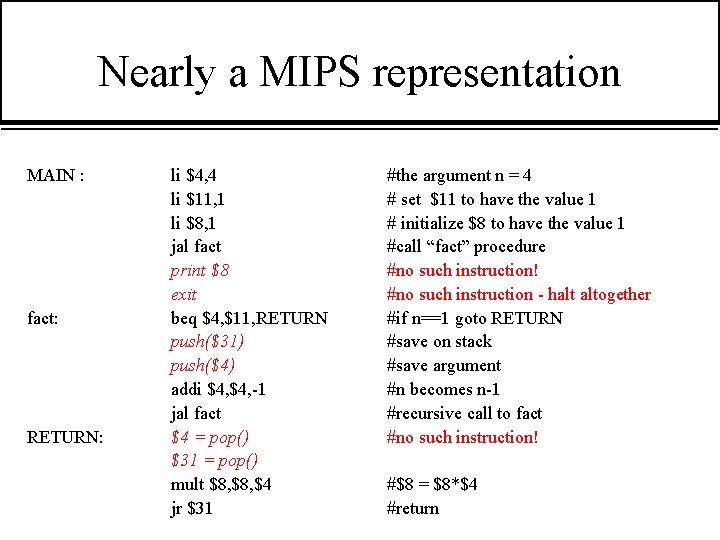
Nearly a MIPS representation MAIN : fact: RETURN: li $4, 4 li $11, 1 li $8, 1 jal fact print $8 exit beq $4, $11, RETURN push($31) push($4) addi $4, -1 jal fact $4 = pop() $31 = pop() mult $8, $4 jr $31 #the argument n = 4 # set $11 to have the value 1 # initialize $8 to have the value 1 #call “fact” procedure #no such instruction! #no such instruction - halt altogether #if n==1 goto RETURN #save on stack #save argument #n becomes n-1 #recursive call to fact #no such instruction! #$8 = $8*$4 #return
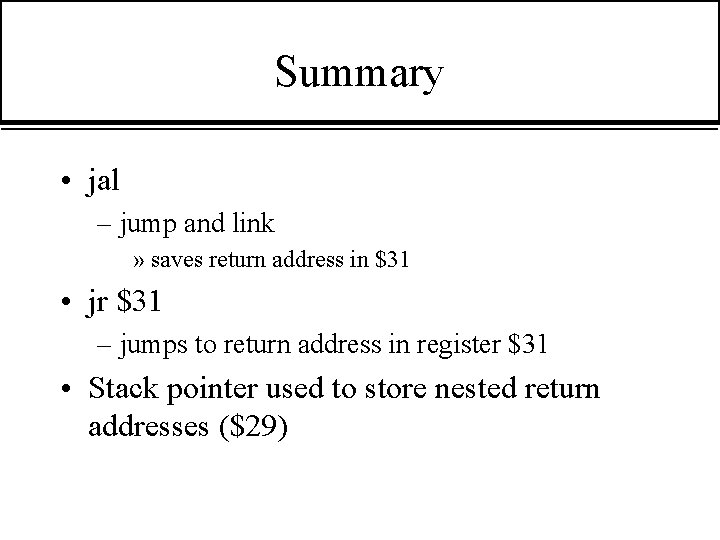
Summary • jal – jump and link » saves return address in $31 • jr $31 – jumps to return address in register $31 • Stack pointer used to store nested return addresses ($29)
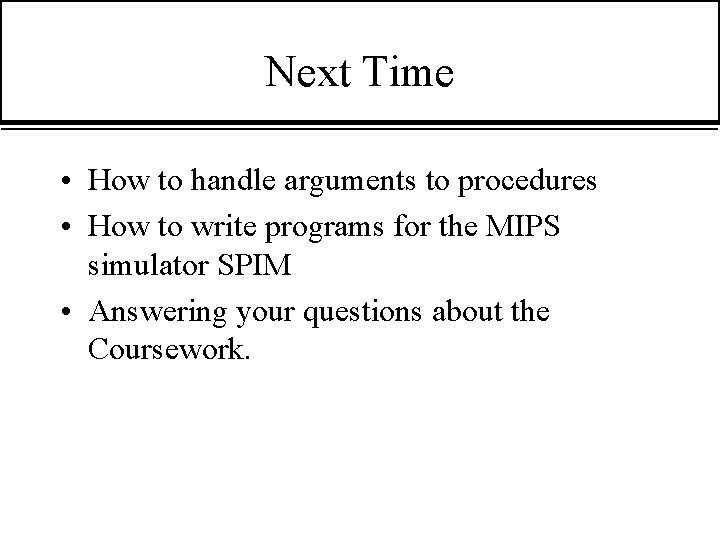
Next Time • How to handle arguments to procedures • How to write programs for the MIPS simulator SPIM • Answering your questions about the Coursework.