Practical Session 3 The Stack The stack is
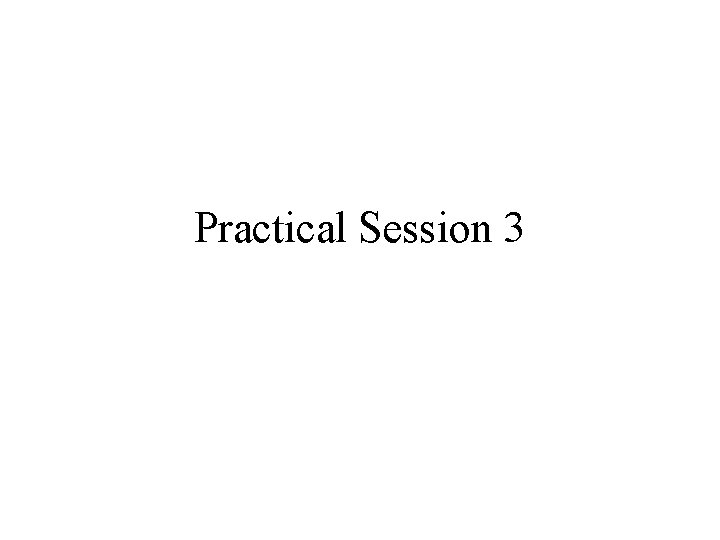
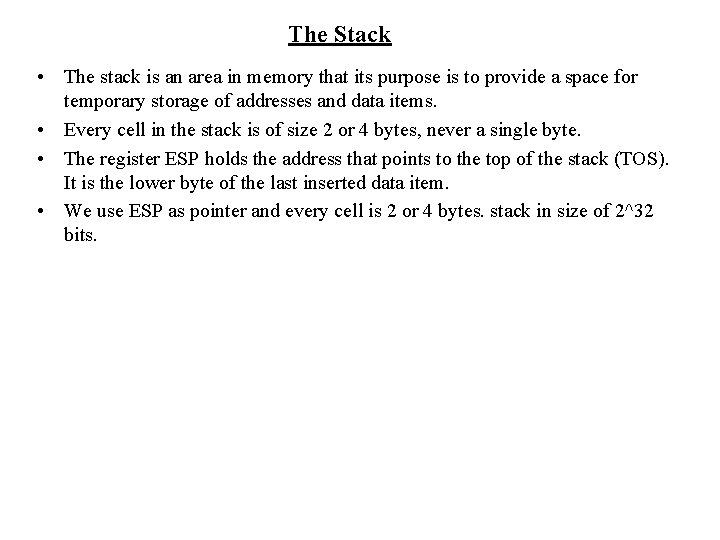
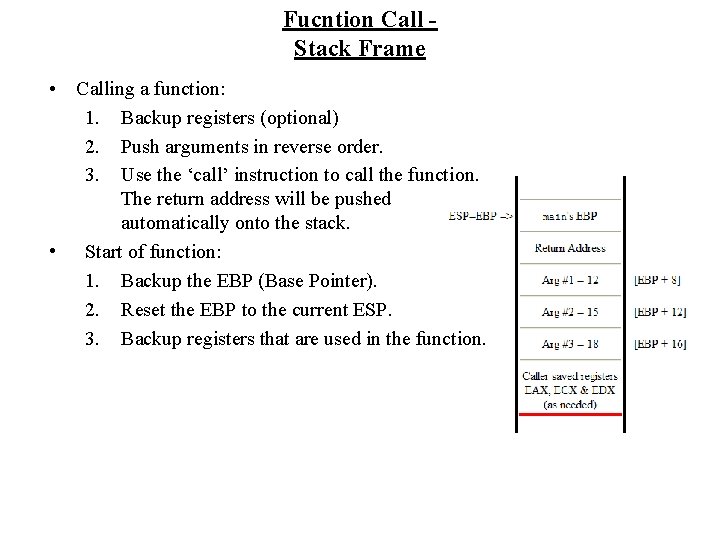
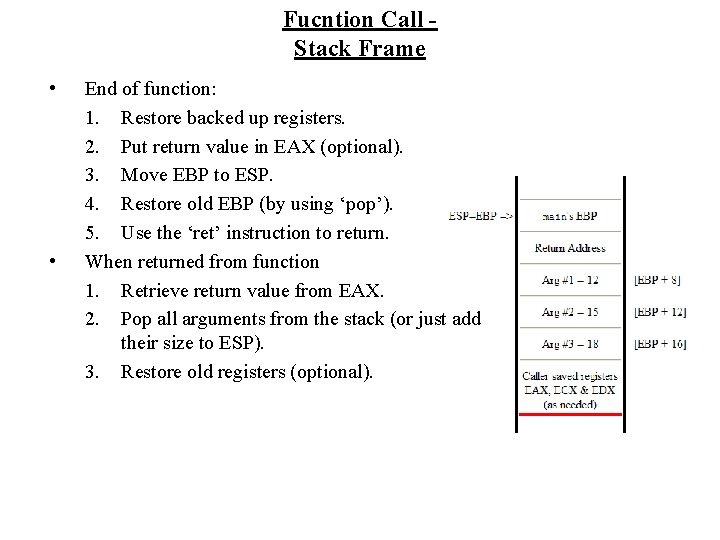
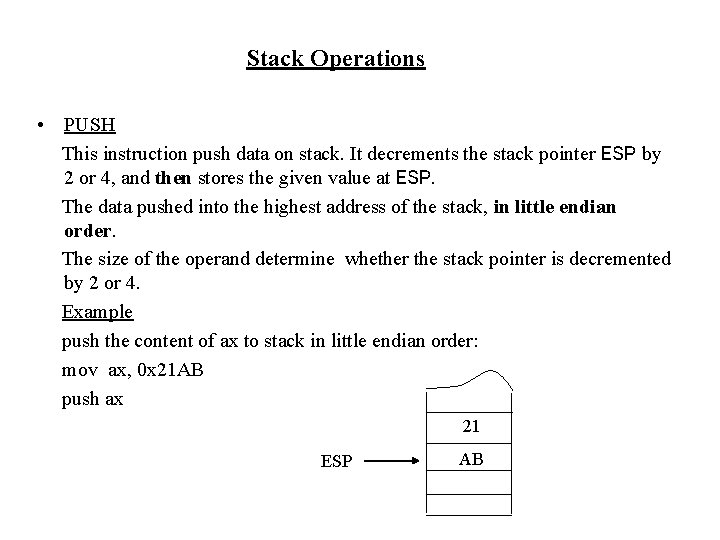
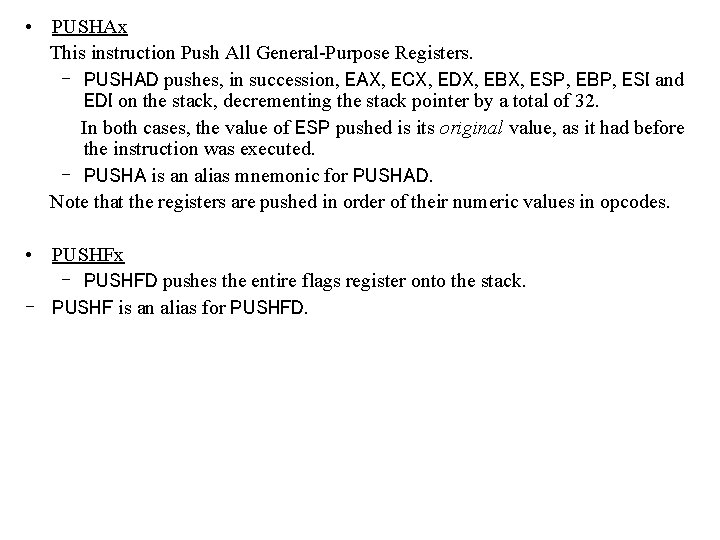
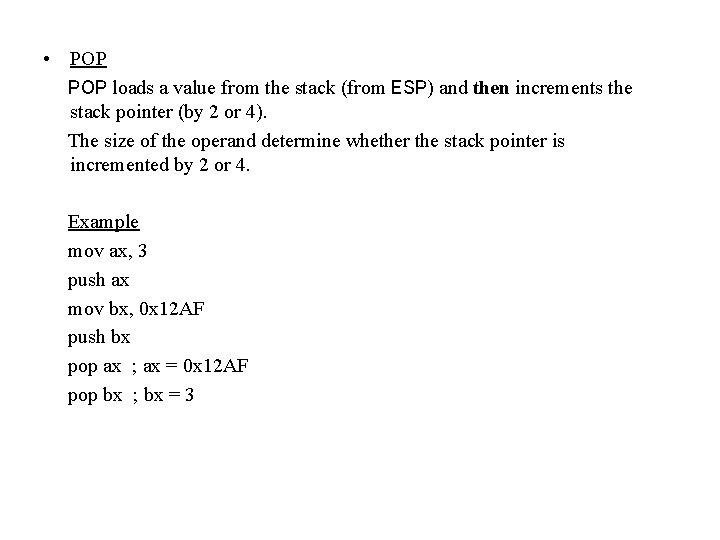
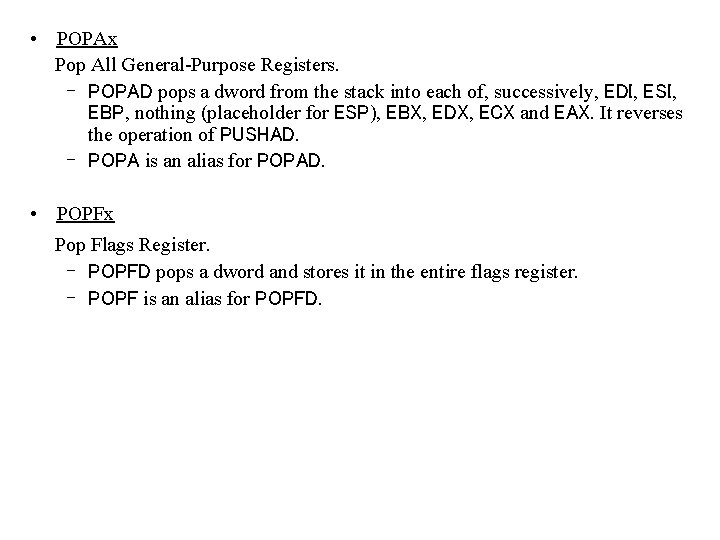
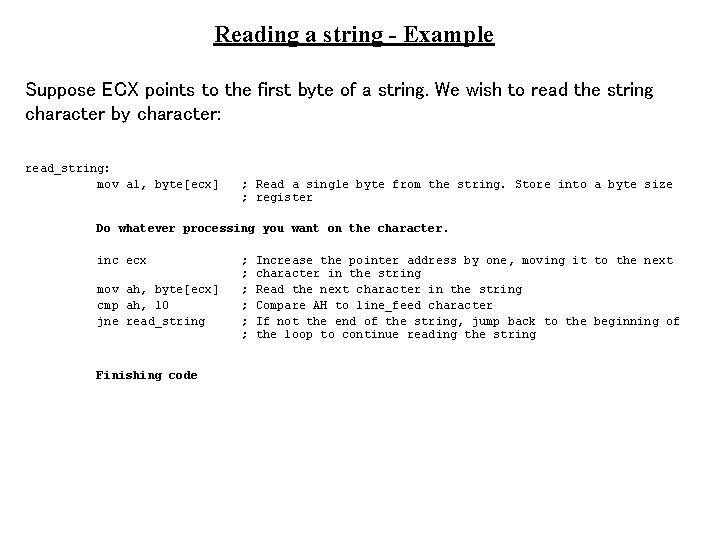
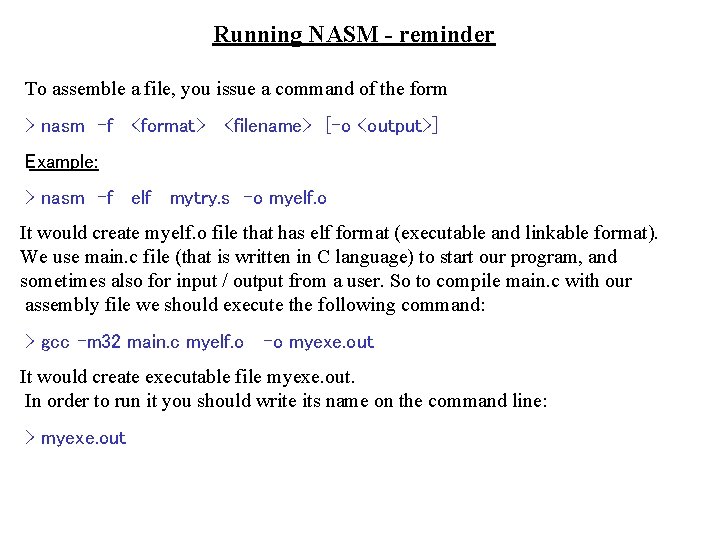
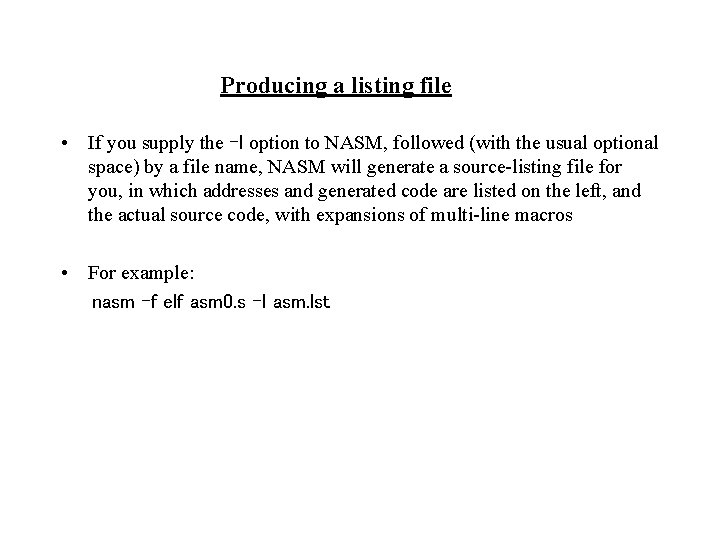
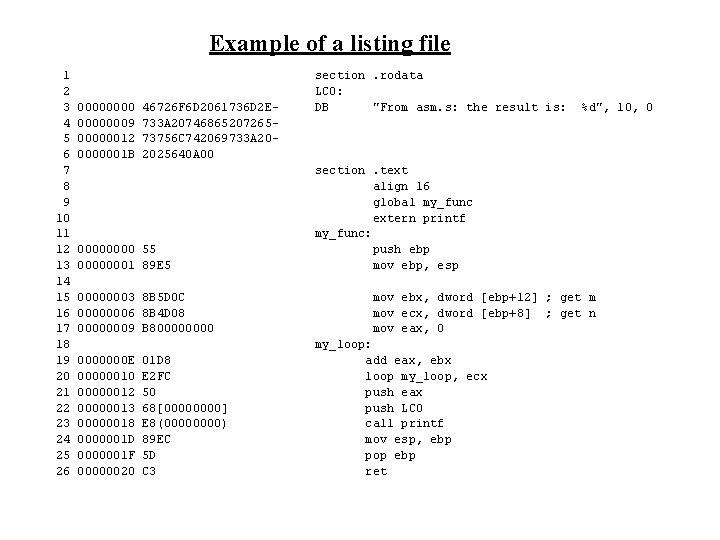
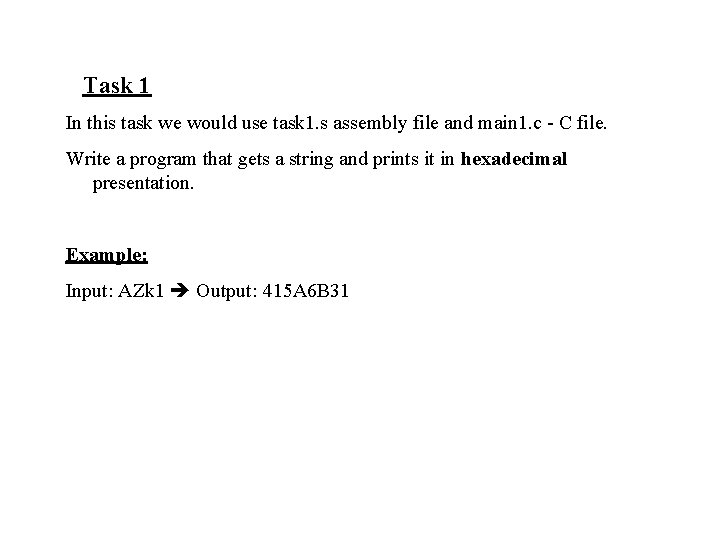
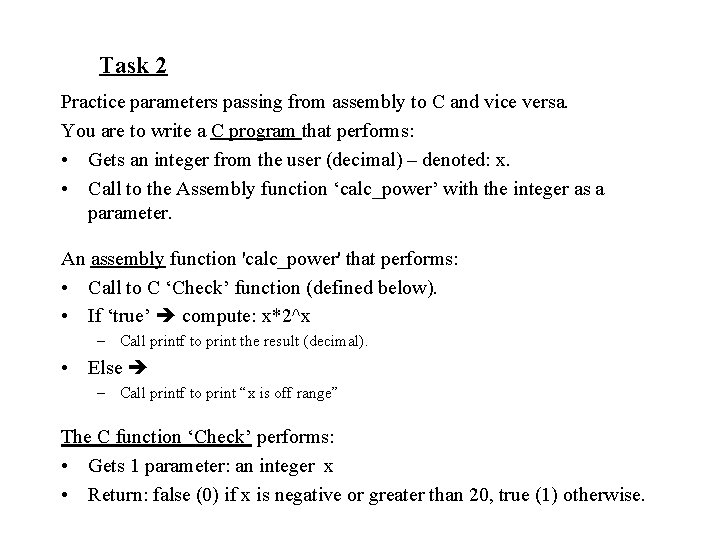
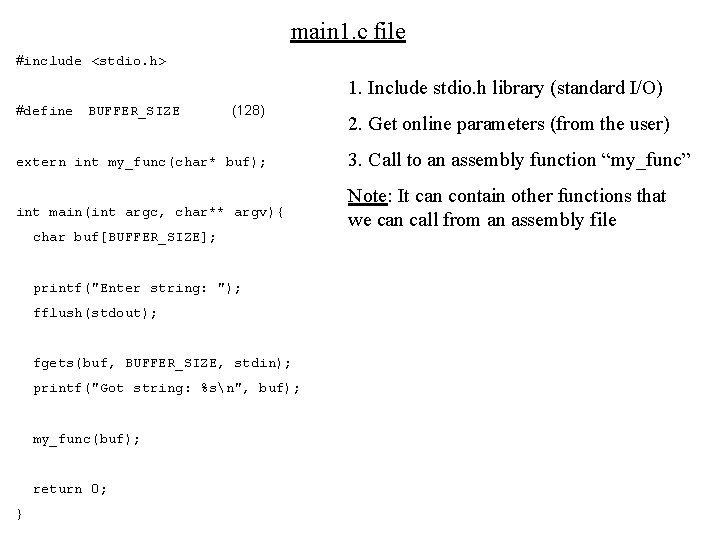
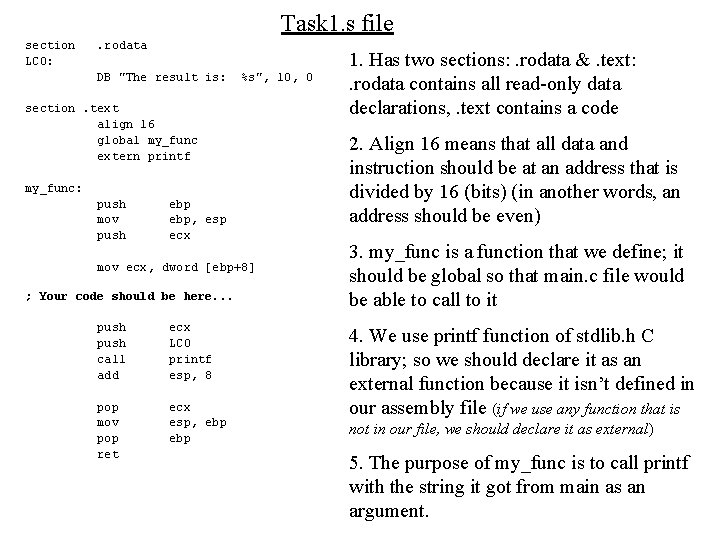
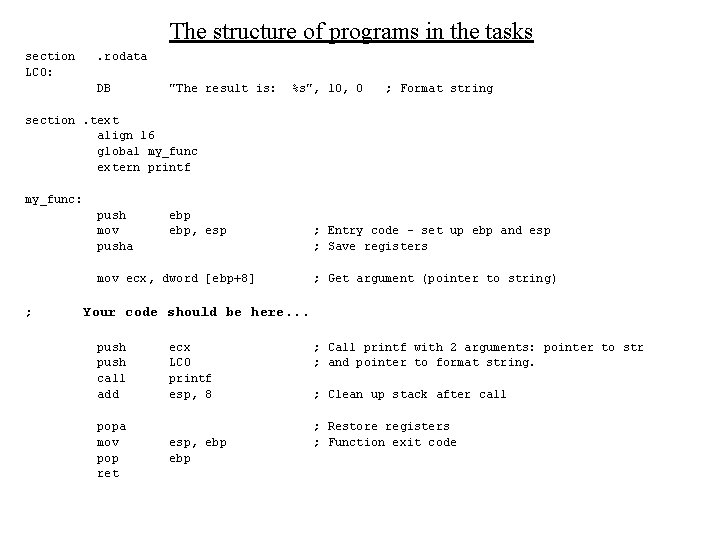
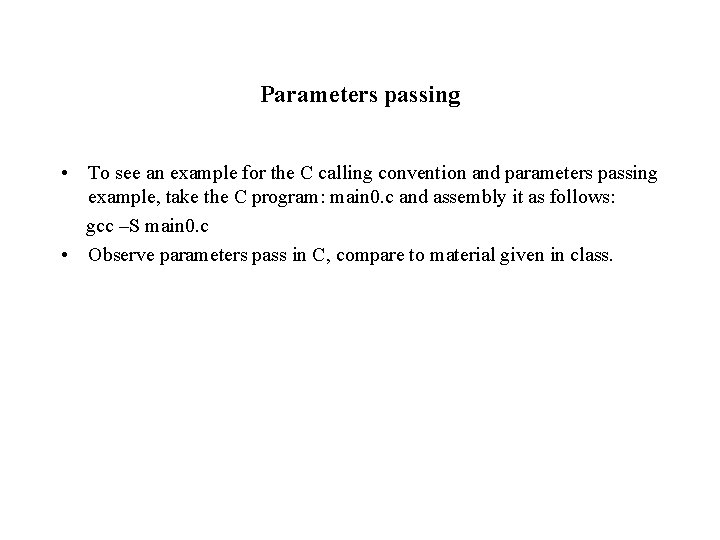
- Slides: 18
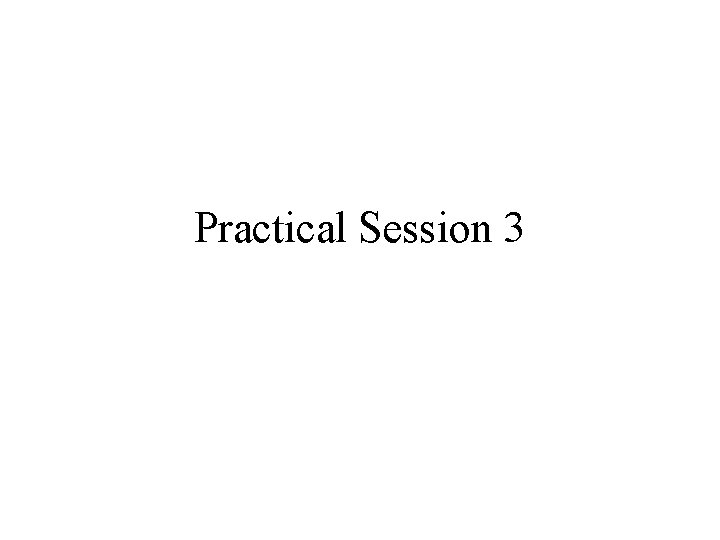
Practical Session 3
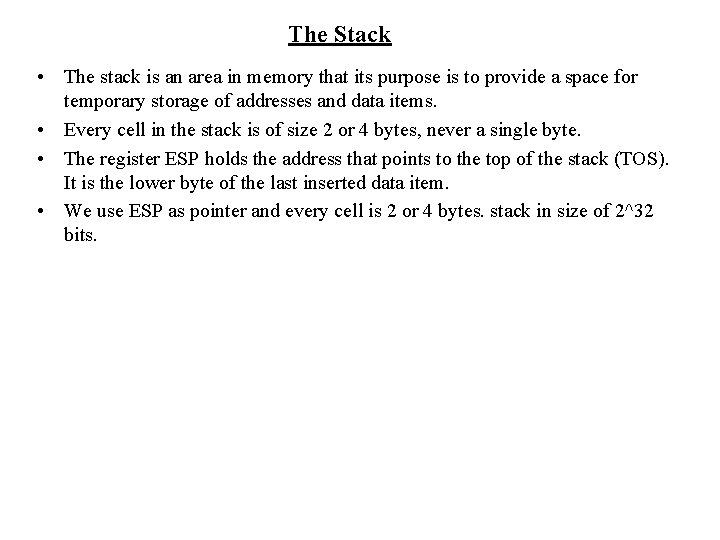
The Stack • The stack is an area in memory that its purpose is to provide a space for temporary storage of addresses and data items. • Every cell in the stack is of size 2 or 4 bytes, never a single byte. • The register ESP holds the address that points to the top of the stack (TOS). It is the lower byte of the last inserted data item. • We use ESP as pointer and every cell is 2 or 4 bytes. stack in size of 2^32 bits.
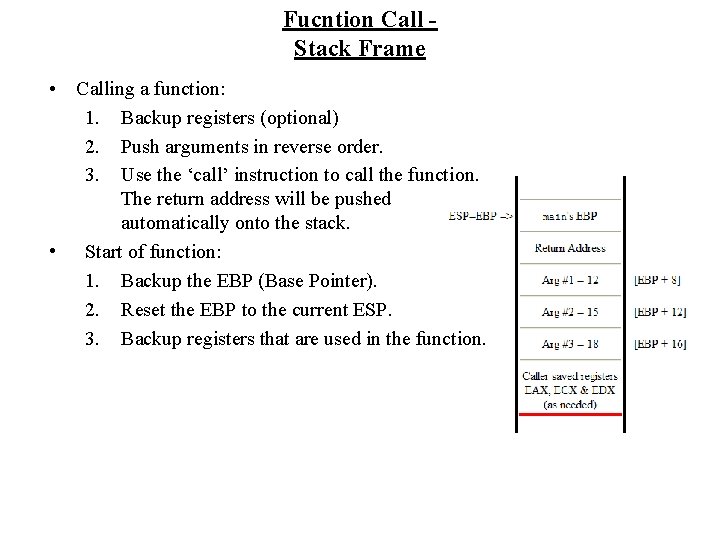
Fucntion Call Stack Frame • Calling a function: 1. Backup registers (optional) 2. Push arguments in reverse order. 3. Use the ‘call’ instruction to call the function. The return address will be pushed automatically onto the stack. • Start of function: 1. Backup the EBP (Base Pointer). 2. Reset the EBP to the current ESP. 3. Backup registers that are used in the function.
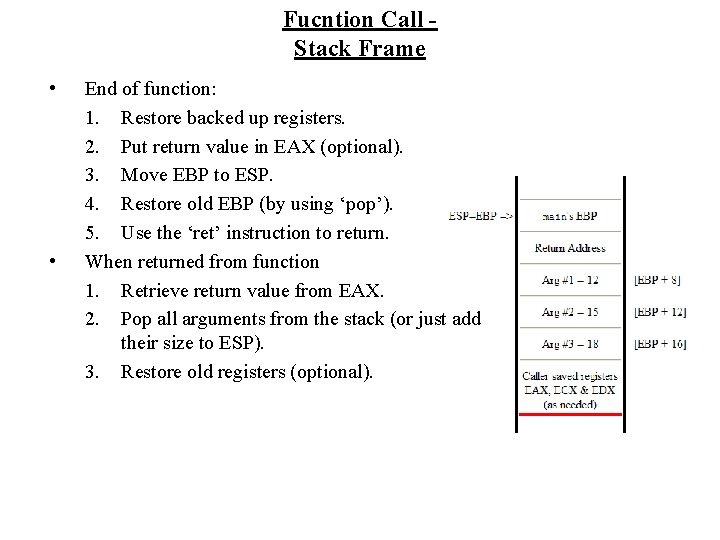
Fucntion Call Stack Frame • • End of function: 1. Restore backed up registers. 2. Put return value in EAX (optional). 3. Move EBP to ESP. 4. Restore old EBP (by using ‘pop’). 5. Use the ‘ret’ instruction to return. When returned from function 1. Retrieve return value from EAX. 2. Pop all arguments from the stack (or just add their size to ESP). 3. Restore old registers (optional).
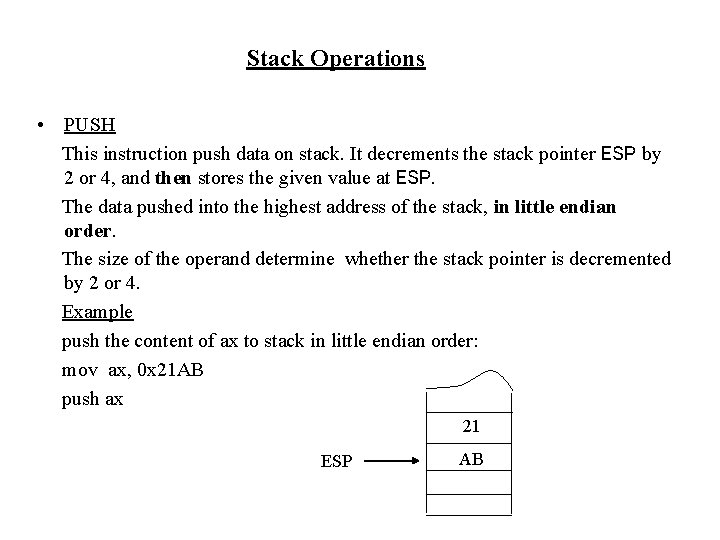
Stack Operations • PUSH This instruction push data on stack. It decrements the stack pointer ESP by 2 or 4, and then stores the given value at ESP. The data pushed into the highest address of the stack, in little endian order. The size of the operand determine whether the stack pointer is decremented by 2 or 4. Example push the content of ax to stack in little endian order: mov ax, 0 x 21 AB push ax 21 ESP AB
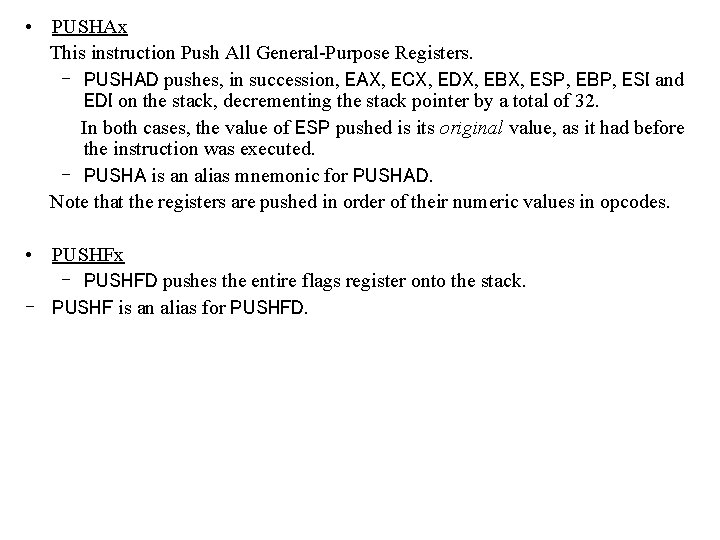
• PUSHAx This instruction Push All General-Purpose Registers. – PUSHAD pushes, in succession, EAX, ECX, EDX, EBX, ESP, EBP, ESI and EDI on the stack, decrementing the stack pointer by a total of 32. In both cases, the value of ESP pushed is its original value, as it had before the instruction was executed. – PUSHA is an alias mnemonic for PUSHAD. Note that the registers are pushed in order of their numeric values in opcodes. • PUSHFx – PUSHFD pushes the entire flags register onto the stack. – PUSHF is an alias for PUSHFD.
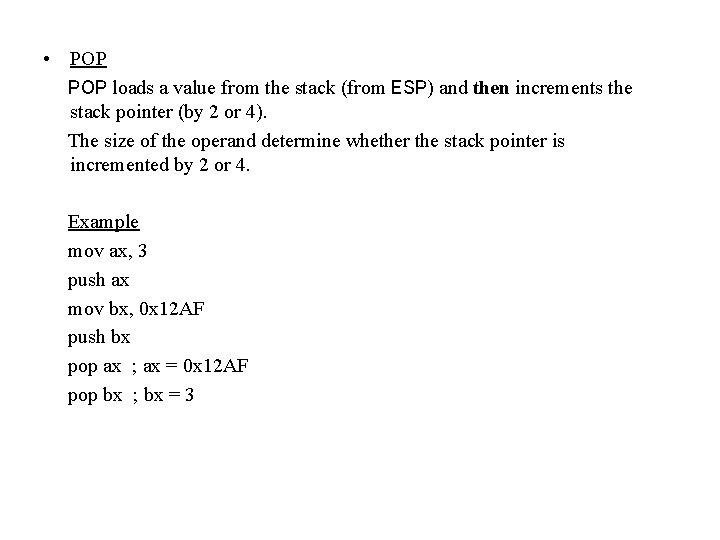
• POP loads a value from the stack (from ESP) and then increments the stack pointer (by 2 or 4). The size of the operand determine whether the stack pointer is incremented by 2 or 4. Example mov ax, 3 push ax mov bx, 0 x 12 AF push bx pop ax ; ax = 0 x 12 AF pop bx ; bx = 3
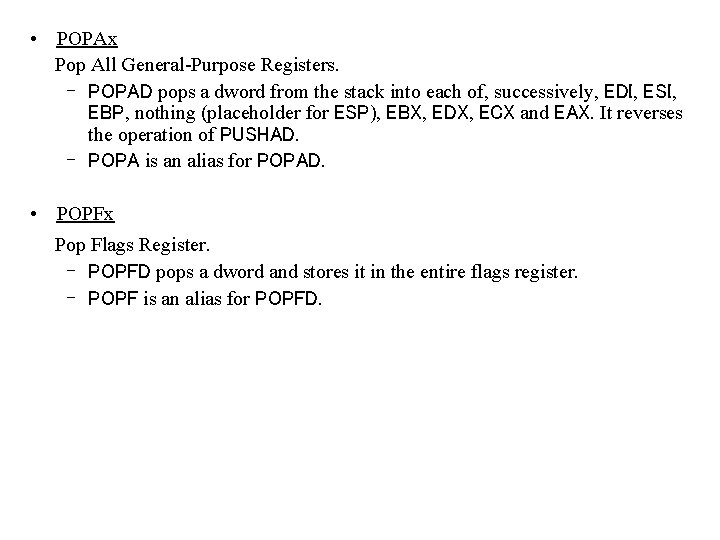
• POPAx Pop All General-Purpose Registers. – POPAD pops a dword from the stack into each of, successively, EDI, ESI, EBP, nothing (placeholder for ESP), EBX, EDX, ECX and EAX. It reverses the operation of PUSHAD. – POPA is an alias for POPAD. • POPFx Pop Flags Register. – POPFD pops a dword and stores it in the entire flags register. – POPF is an alias for POPFD.
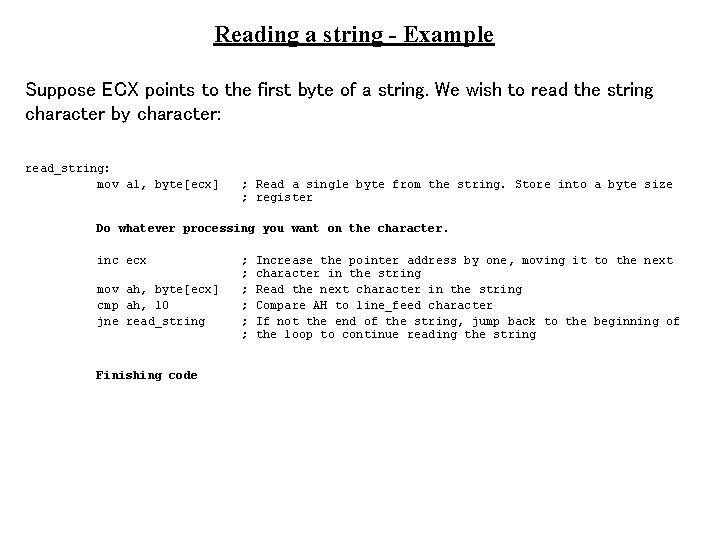
Reading a string - Example Suppose ECX points to the first byte of a string. We wish to read the string character by character: read_string: mov al, byte[ecx] ; Read a single byte from the string. Store into a byte size ; register Do whatever processing you want on the character. inc ecx mov ah, byte[ecx] cmp ah, 10 jne read_string Finishing code ; ; ; Increase the pointer address by one, moving it to the next character in the string Read the next character in the string Compare AH to line_feed character If not the end of the string, jump back to the beginning of the loop to continue reading the string
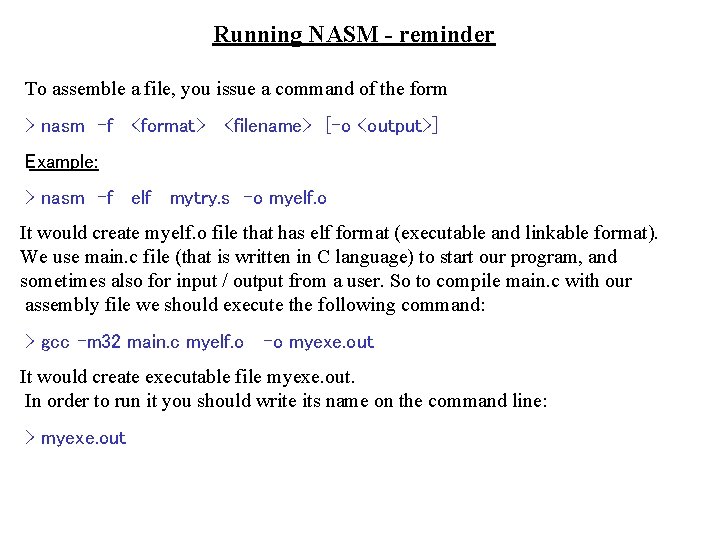
Running NASM - reminder To assemble a file, you issue a command of the form > nasm -f <format> <filename> [-o <output>] Example: > nasm -f elf mytry. s -o myelf. o It would create myelf. o file that has elf format (executable and linkable format). We use main. c file (that is written in C language) to start our program, and sometimes also for input / output from a user. So to compile main. c with our assembly file we should execute the following command: > gcc –m 32 main. c myelf. o -o myexe. out It would create executable file myexe. out. In order to run it you should write its name on the command line: > myexe. out
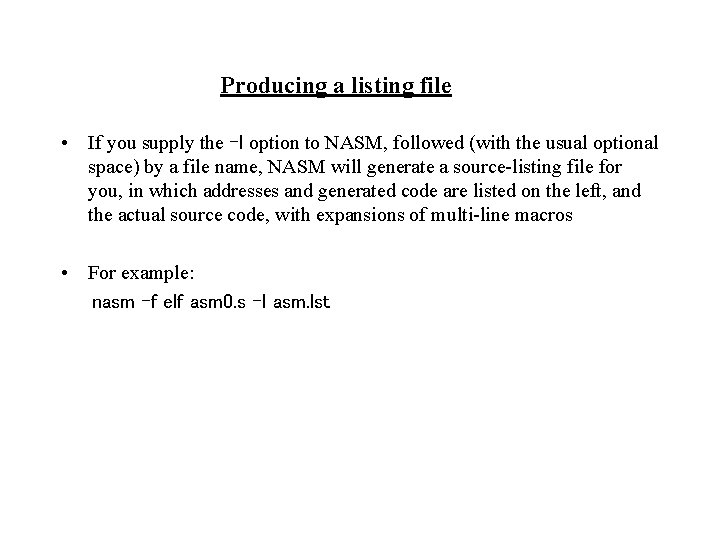
Producing a listing file • If you supply the -l option to NASM, followed (with the usual optional space) by a file name, NASM will generate a source-listing file for you, in which addresses and generated code are listed on the left, and the actual source code, with expansions of multi-line macros • For example: nasm -f elf asm 0. s -l asm. lst
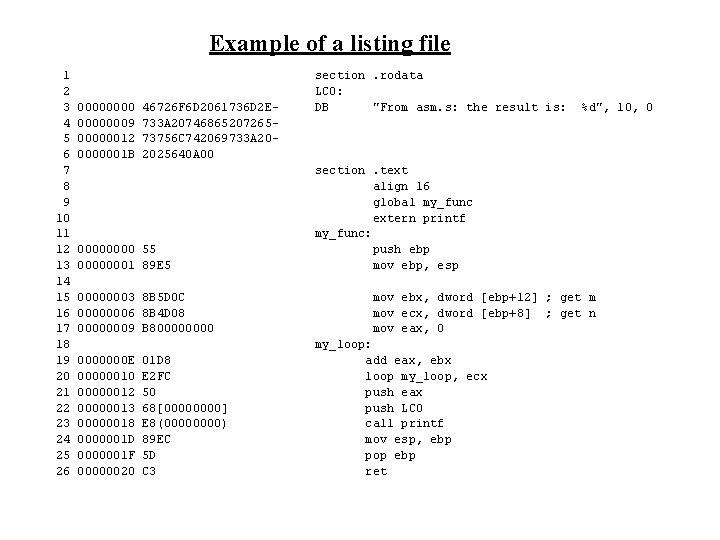
Example of a listing file 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 00000009 00000012 0000001 B 46726 F 6 D 2061736 D 2 E 733 A 2074686520726573756 C 742069733 A 202025640 A 00 0000 55 00000001 89 E 5 00000003 8 B 5 D 0 C 00000006 8 B 4 D 08 00000009 B 80000000 E 00000010 00000012 00000013 00000018 0000001 D 0000001 F 00000020 01 D 8 E 2 FC 50 68[0000] E 8(0000) 89 EC 5 D C 3 section. rodata LC 0: DB "From asm. s: the result is: %d", 10, 0 section. text align 16 global my_func extern printf my_func: push ebp mov ebp, esp mov ebx, dword [ebp+12] ; get m mov ecx, dword [ebp+8] ; get n mov eax, 0 my_loop: add eax, ebx loop my_loop, ecx push eax push LC 0 call printf mov esp, ebp pop ebp ret
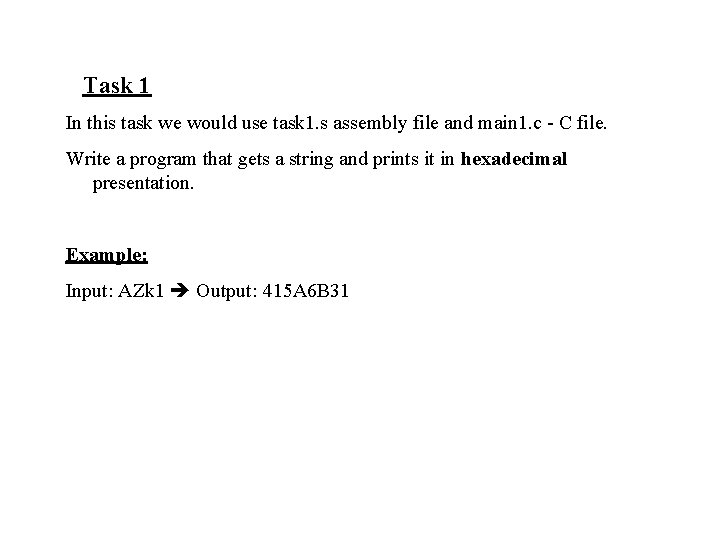
Task 1 In this task we would use task 1. s assembly file and main 1. c - C file. Write a program that gets a string and prints it in hexadecimal presentation. Example: Input: AZk 1 Output: 415 A 6 B 31
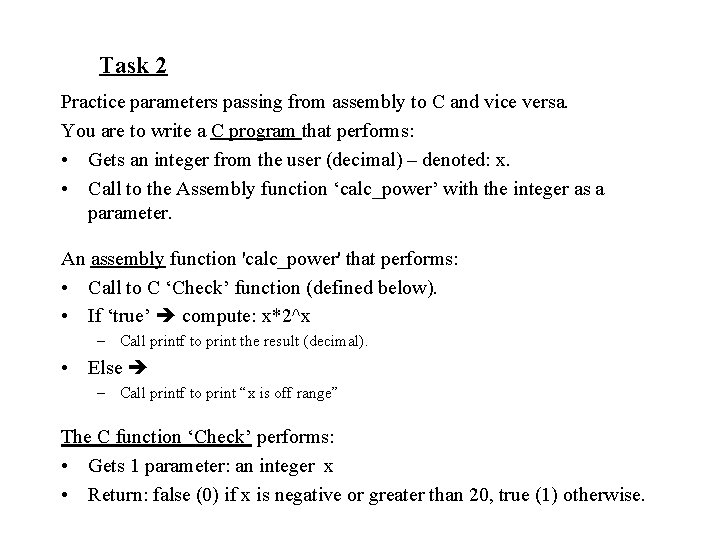
Task 2 Practice parameters passing from assembly to C and vice versa. You are to write a C program that performs: • Gets an integer from the user (decimal) – denoted: x. • Call to the Assembly function ‘calc_power’ with the integer as a parameter. An assembly function 'calc_power' that performs: • Call to C ‘Check’ function (defined below). • If ‘true’ compute: x*2^x – Call printf to print the result (decimal). • Else – Call printf to print “x is off range” The C function ‘Check’ performs: • Gets 1 parameter: an integer x • Return: false (0) if x is negative or greater than 20, true (1) otherwise.
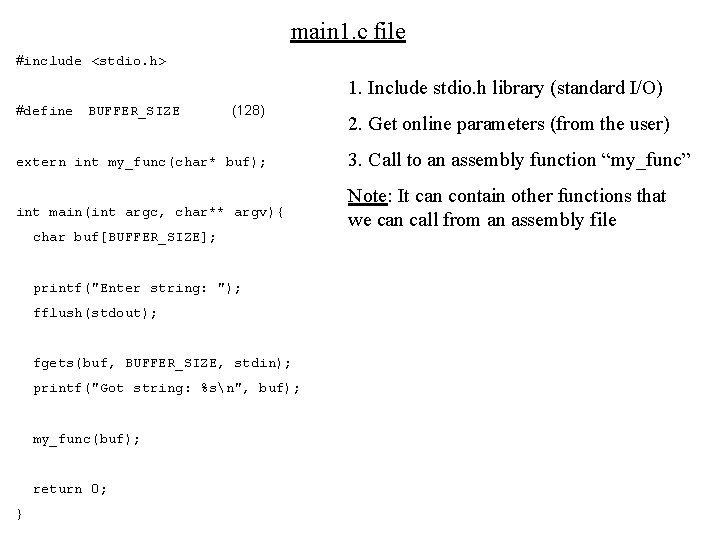
main 1. c file #include <stdio. h> 1. Include stdio. h library (standard I/O) #define BUFFER_SIZE (128) 2. Get online parameters (from the user) extern int my_func(char* buf); 3. Call to an assembly function “my_func” int main(int argc, char** argv){ Note: It can contain other functions that we can call from an assembly file char buf[BUFFER_SIZE]; printf("Enter string: "); fflush(stdout); fgets(buf, BUFFER_SIZE, stdin); printf("Got string: %sn", buf); my_func(buf); return 0; }
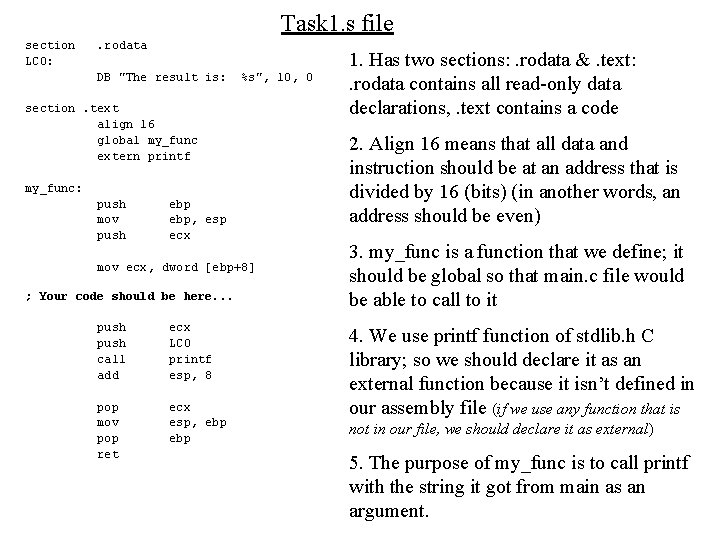
Task 1. s file section LC 0: . rodata DB "The result is: %s", 10, 0 section. text align 16 global my_func extern printf my_func: push mov push ebp, esp ecx mov ecx, dword [ebp+8] ; Your code should be here. . . push call add ecx LC 0 printf esp, 8 pop mov pop ret ecx esp, ebp 1. Has two sections: . rodata &. text: . rodata contains all read-only data declarations, . text contains a code 2. Align 16 means that all data and instruction should be at an address that is divided by 16 (bits) (in another words, an address should be even) 3. my_func is a function that we define; it should be global so that main. c file would be able to call to it 4. We use printf function of stdlib. h C library; so we should declare it as an external function because it isn’t defined in our assembly file (if we use any function that is not in our file, we should declare it as external) 5. The purpose of my_func is to call printf with the string it got from main as an argument.
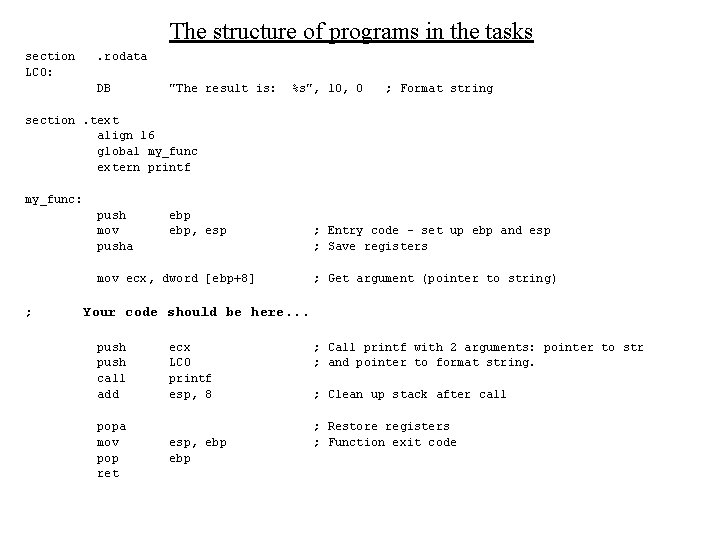
The structure of programs in the tasks section LC 0: . rodata DB "The result is: %s", 10, 0 ; Format string section. text align 16 global my_func extern printf my_func: push mov pusha ebp, esp mov ecx, dword [ebp+8] ; ; Entry code - set up ebp and esp ; Save registers ; Get argument (pointer to string) Your code should be here. . . push call add popa mov pop ret ecx LC 0 printf esp, 8 esp, ebp ; Call printf with 2 arguments: pointer to str ; and pointer to format string. ; Clean up stack after call ; Restore registers ; Function exit code
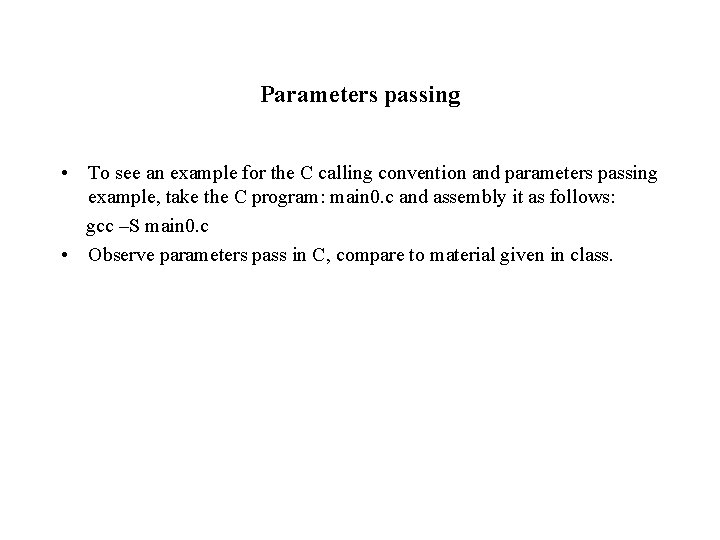
Parameters passing • To see an example for the C calling convention and parameters passing example, take the C program: main 0. c and assembly it as follows: gcc –S main 0. c • Observe parameters pass in C, compare to material given in class.