Practical Session 10 Animation Animation kinds There are
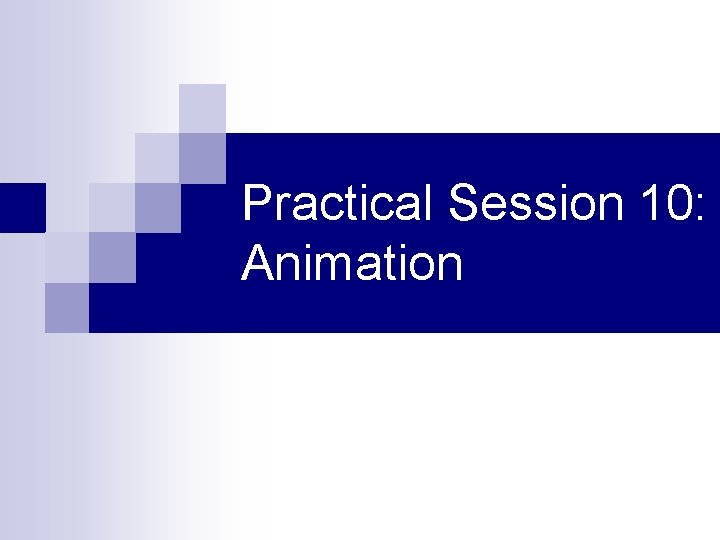
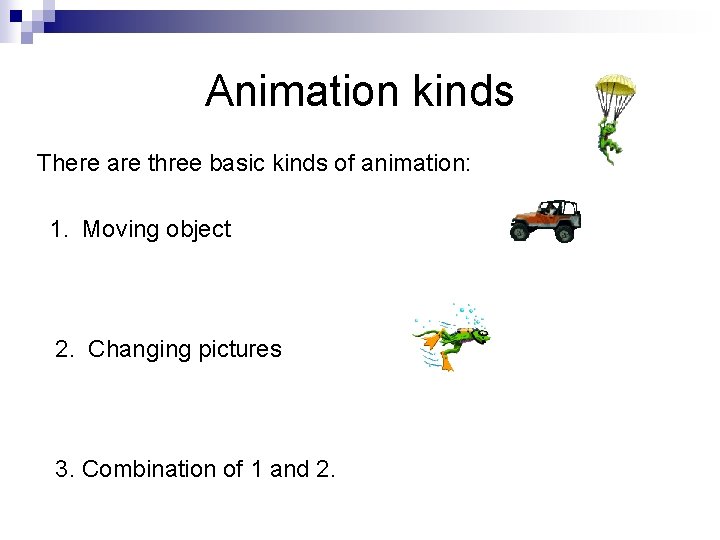
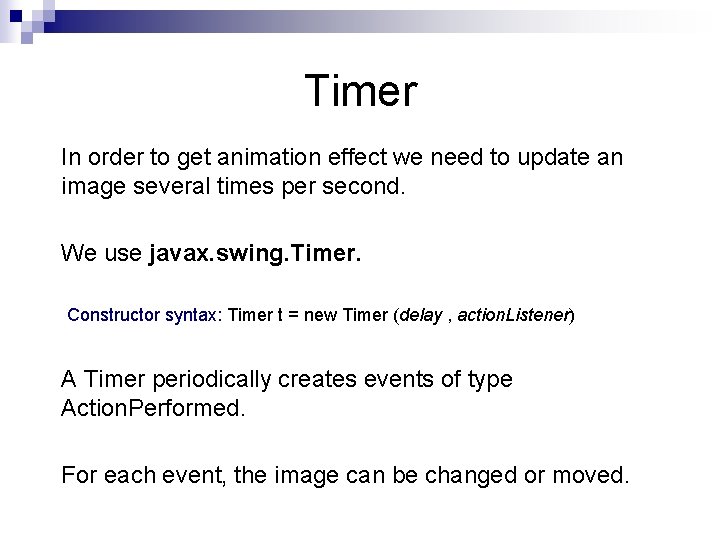
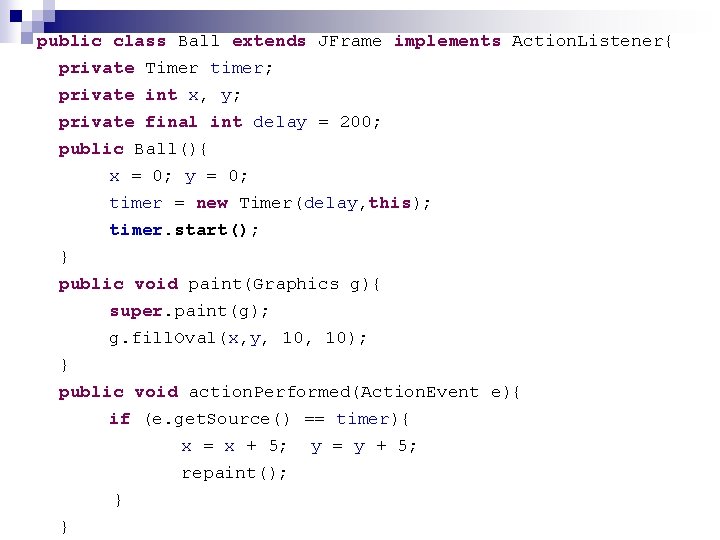
![Changing Pictures 1. Create an array of pictures. im = new Image. Icon[3]; im[0] Changing Pictures 1. Create an array of pictures. im = new Image. Icon[3]; im[0]](https://slidetodoc.com/presentation_image/36d49ad0ad1b5041b47b493207a449a5/image-5.jpg)
![public class Car extends JPanel implements Action. Listener{ private int frame; private Image. Icon[] public class Car extends JPanel implements Action. Listener{ private int frame; private Image. Icon[]](https://slidetodoc.com/presentation_image/36d49ad0ad1b5041b47b493207a449a5/image-6.jpg)
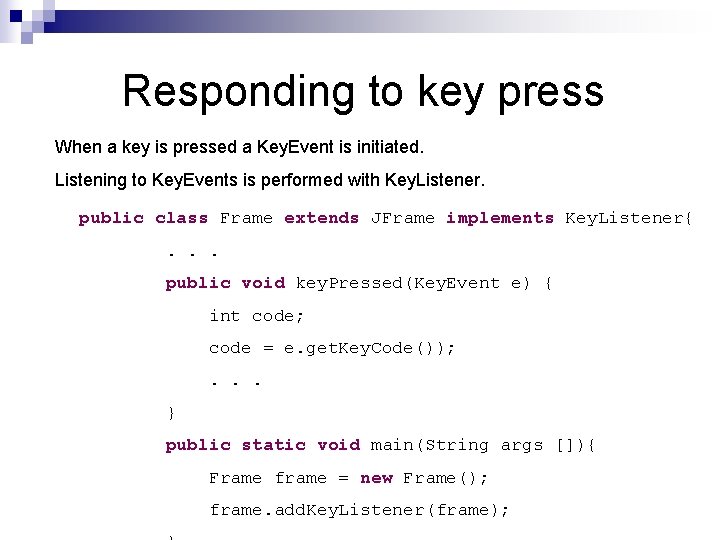
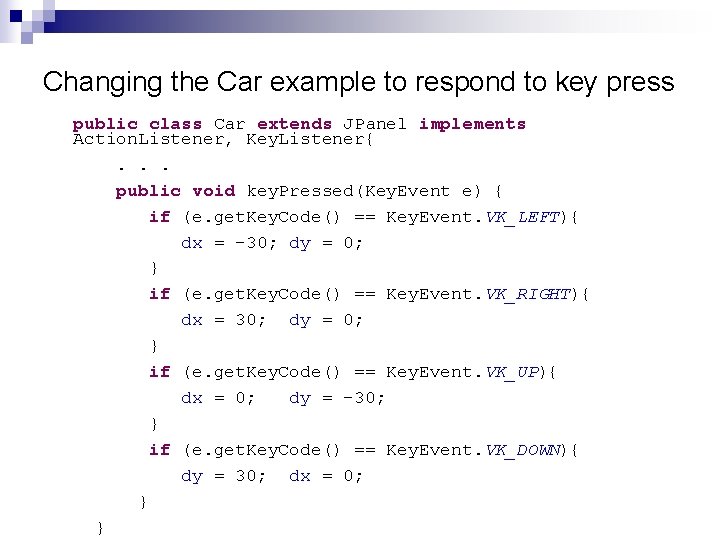
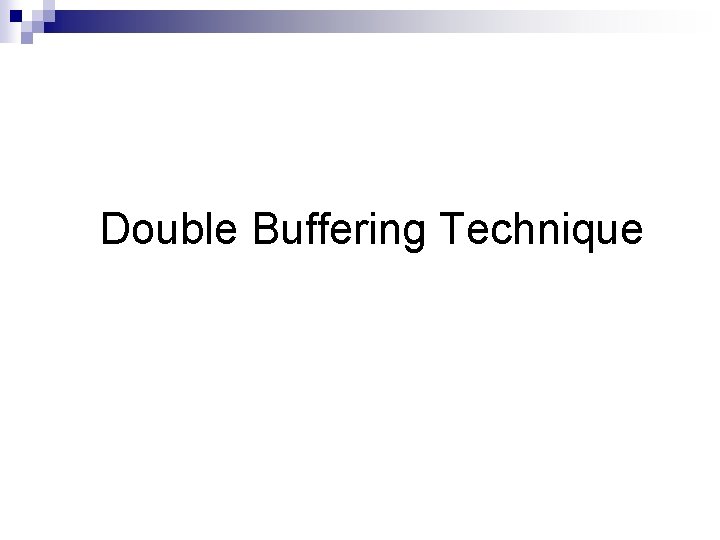
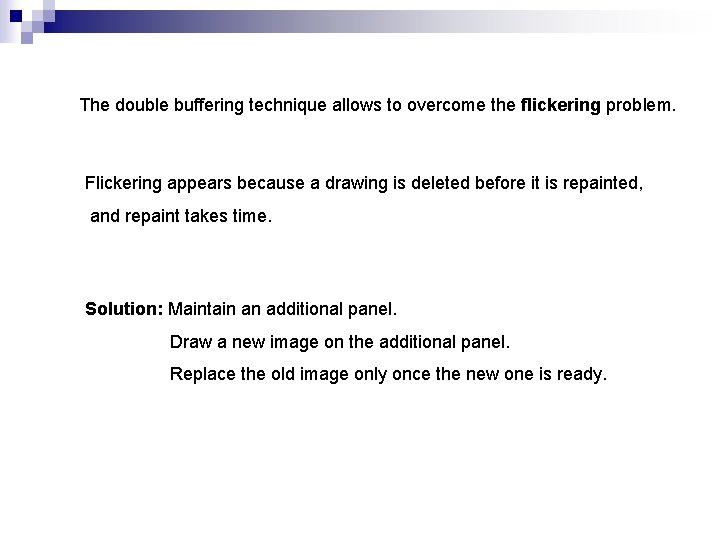
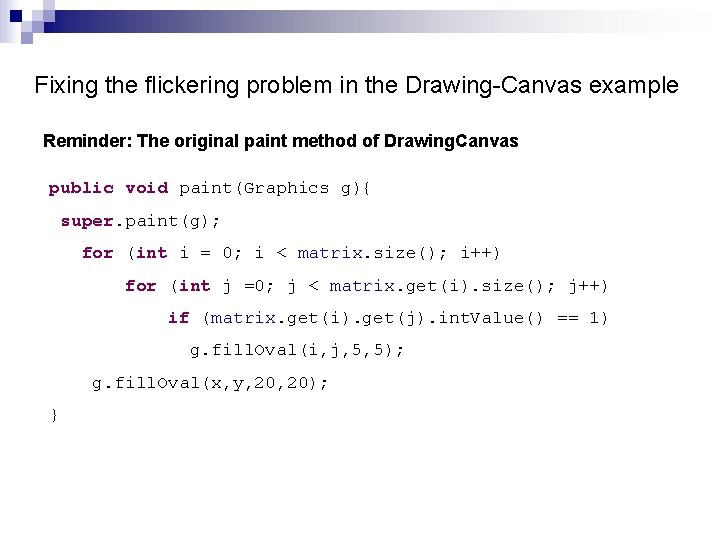
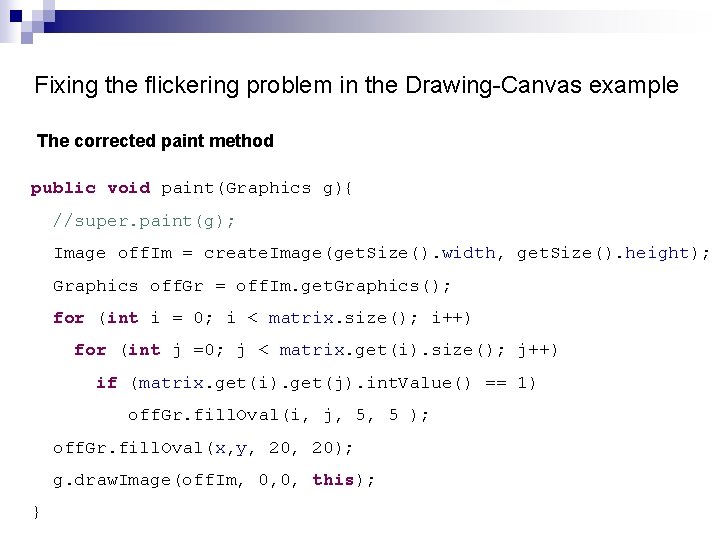
- Slides: 12
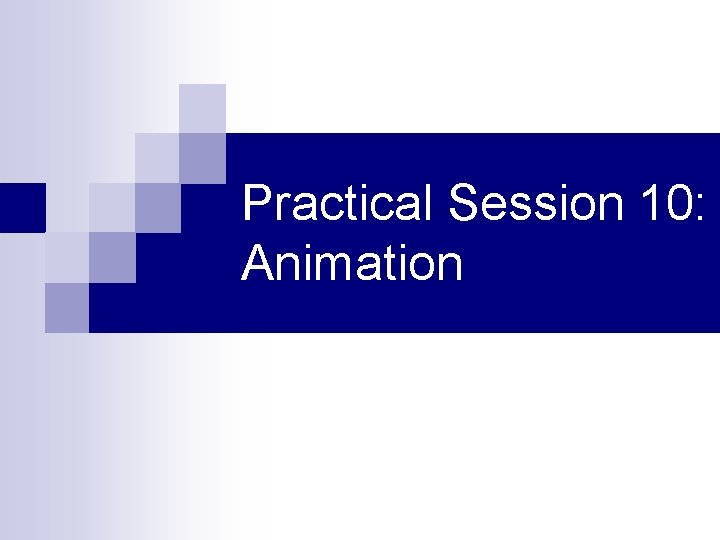
Practical Session 10: Animation
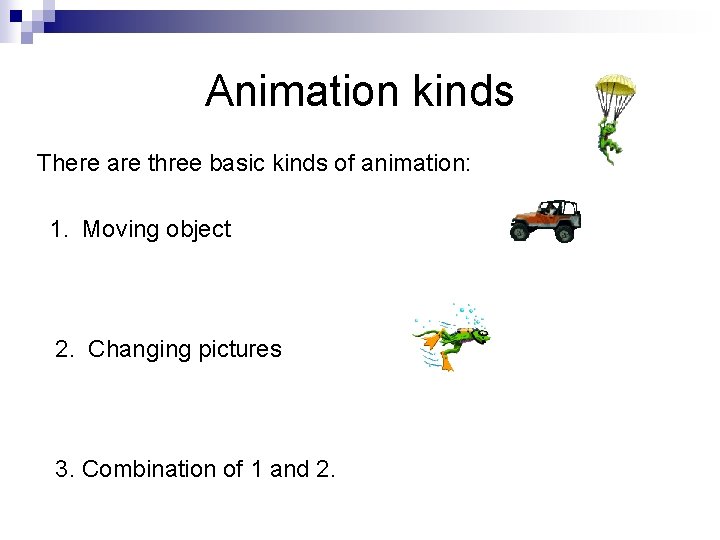
Animation kinds There are three basic kinds of animation: 1. Moving object 2. Changing pictures 3. Combination of 1 and 2.
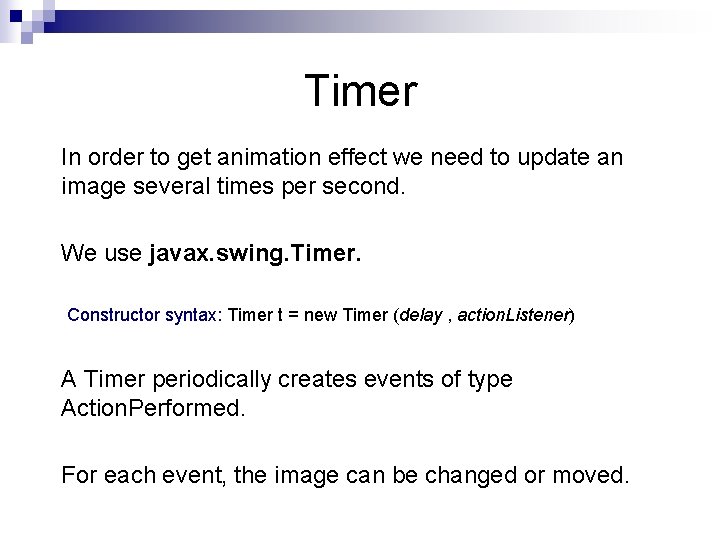
Timer In order to get animation effect we need to update an image several times per second. We use javax. swing. Timer. Constructor syntax: Timer t = new Timer (delay , action. Listener) A Timer periodically creates events of type Action. Performed. For each event, the image can be changed or moved.
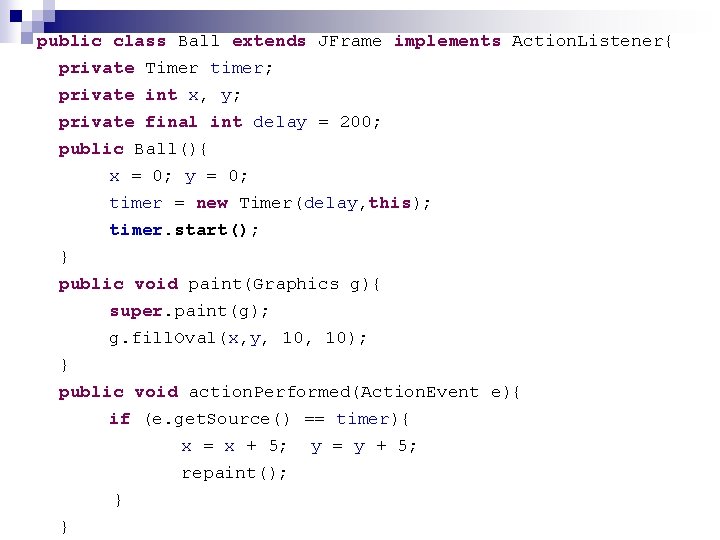
public class Ball extends JFrame implements Action. Listener{ private Timer timer; private int x, y; private final int delay = 200; public Ball(){ x = 0; y = 0; timer = new Timer(delay, this); timer. start(); } public void paint(Graphics g){ super. paint(g); g. fill. Oval(x, y, 10); } public void action. Performed(Action. Event e){ if (e. get. Source() == timer){ x = x + 5; y = y + 5; repaint(); } }
![Changing Pictures 1 Create an array of pictures im new Image Icon3 im0 Changing Pictures 1. Create an array of pictures. im = new Image. Icon[3]; im[0]](https://slidetodoc.com/presentation_image/36d49ad0ad1b5041b47b493207a449a5/image-5.jpg)
Changing Pictures 1. Create an array of pictures. im = new Image. Icon[3]; im[0] = new Image. Icon("car 1. gif"); im[1] = new Image. Icon("car 2. gif"); im[2] = new Image. Icon("car 3. gif"); 2. Define a field that represents the current image number. private int frame = 0; 3. The paint method draws the appropriate image. im[frame]. paint. Icon(this, g, x, y); 4. Action. Performed, which is invoked by a timer, changes the current image. frame = (frame + 1) % 3;
![public class Car extends JPanel implements Action Listener private int frame private Image Icon public class Car extends JPanel implements Action. Listener{ private int frame; private Image. Icon[]](https://slidetodoc.com/presentation_image/36d49ad0ad1b5041b47b493207a449a5/image-6.jpg)
public class Car extends JPanel implements Action. Listener{ private int frame; private Image. Icon[] im; private Timer timer; private int x, y, dx, dy; public Car(){ frame = 0; x = 30; y = 30; dx = 15; dy = 15; im = new Image. Icon[3]; //. . . (create three images and assign to the array) timer = new Timer(200, this); timer. start(); } public void action. Performed(Action. Event e){ if (e. get. Source() == timer){ frame = (frame + 1) % 3; x = (x + dx) % get. Size(). width; y = (y + dy) % get. Size(). height; repaint(); } } public void paint(Graphics g){ super. paint(g); im[frame]. paint. Icon(this, g, x, y); }
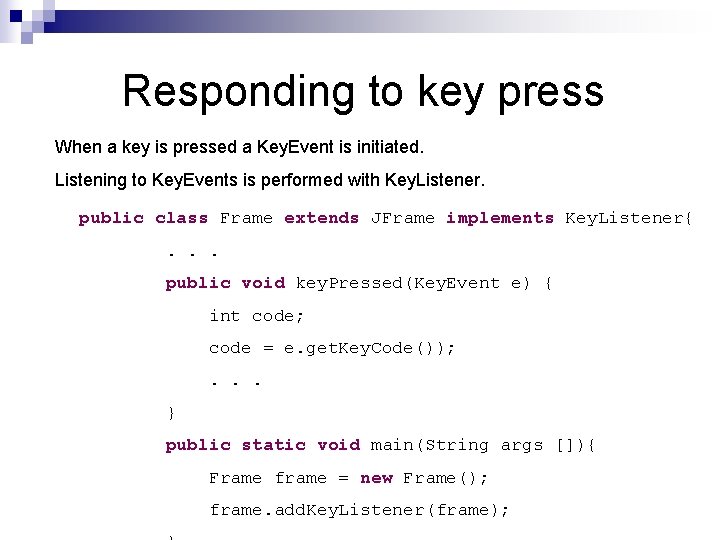
Responding to key press When a key is pressed a Key. Event is initiated. Listening to Key. Events is performed with Key. Listener. public class Frame extends JFrame implements Key. Listener{. . . public void key. Pressed(Key. Event e) { int code; code = e. get. Key. Code()); . . . } public static void main(String args []){ Frame frame = new Frame(); frame. add. Key. Listener(frame);
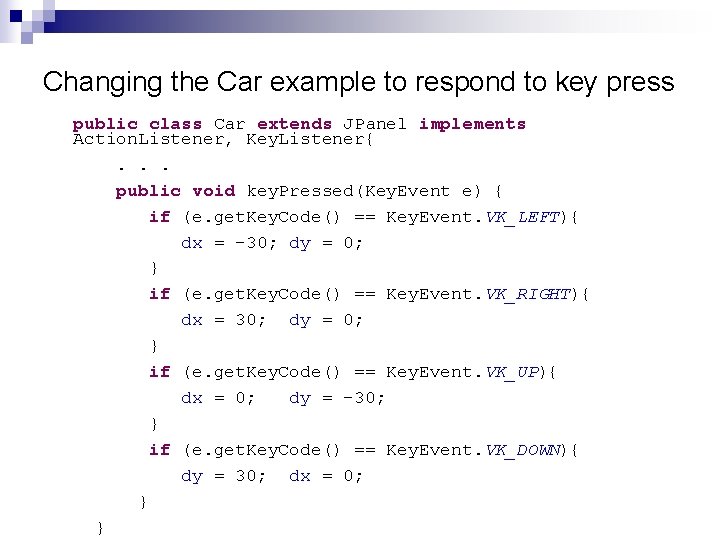
Changing the Car example to respond to key press public class Car extends JPanel implements Action. Listener, Key. Listener{. . . public void key. Pressed(Key. Event e) { if (e. get. Key. Code() == Key. Event. VK_LEFT){ dx = -30; dy = 0; } if (e. get. Key. Code() == Key. Event. VK_RIGHT){ dx = 30; dy = 0; } if (e. get. Key. Code() == Key. Event. VK_UP){ dx = 0; dy = -30; } if (e. get. Key. Code() == Key. Event. VK_DOWN){ dy = 30; dx = 0; } }
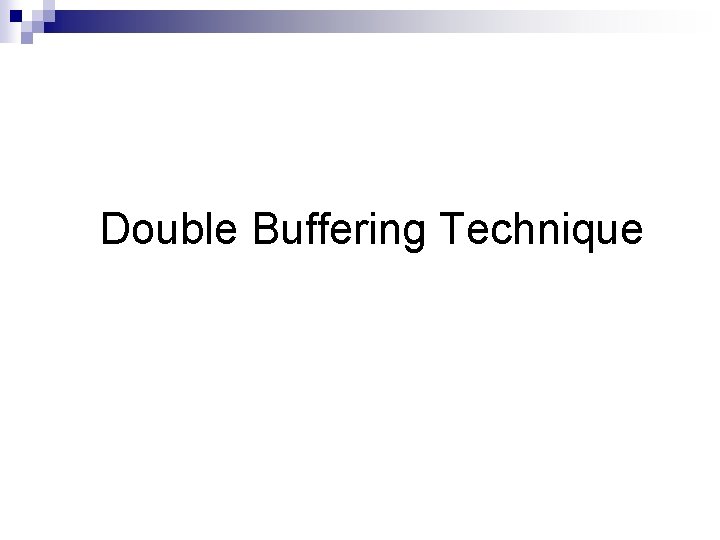
Double Buffering Technique
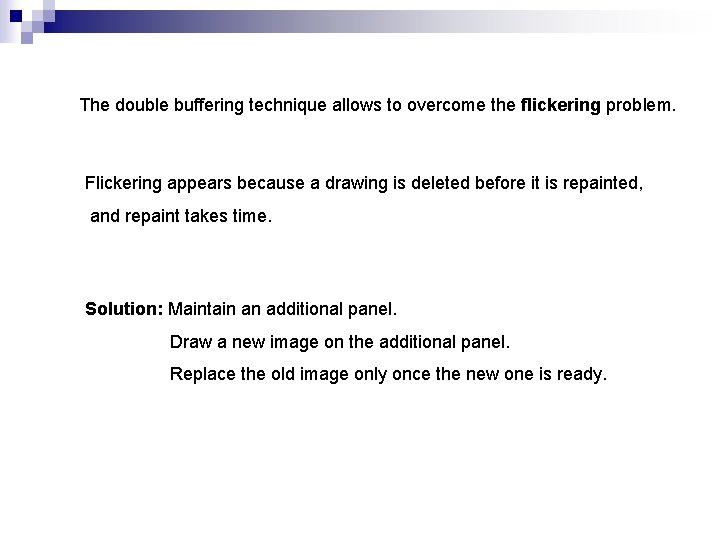
The double buffering technique allows to overcome the flickering problem. Flickering appears because a drawing is deleted before it is repainted, and repaint takes time. Solution: Maintain an additional panel. Draw a new image on the additional panel. Replace the old image only once the new one is ready.
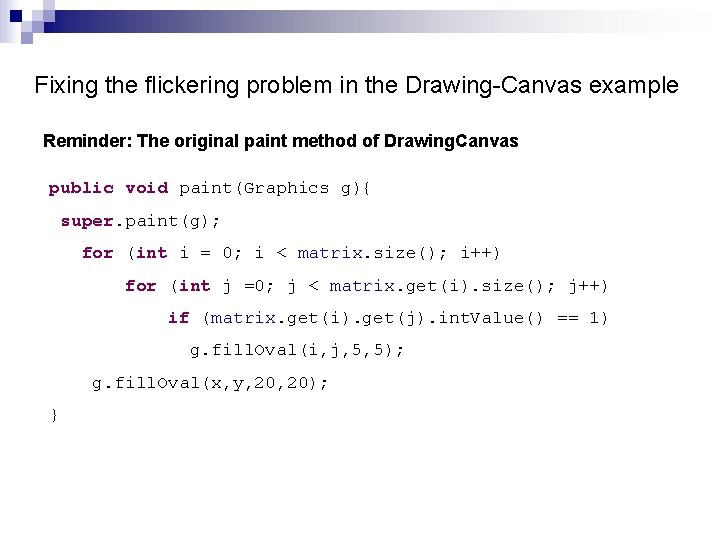
Fixing the flickering problem in the Drawing-Canvas example Reminder: The original paint method of Drawing. Canvas public void paint(Graphics g){ super. paint(g); for (int i = 0; i < matrix. size(); i++) for (int j =0; j < matrix. get(i). size(); j++) if (matrix. get(i). get(j). int. Value() == 1) g. fill. Oval(i, j, 5, 5); g. fill. Oval(x, y, 20); }
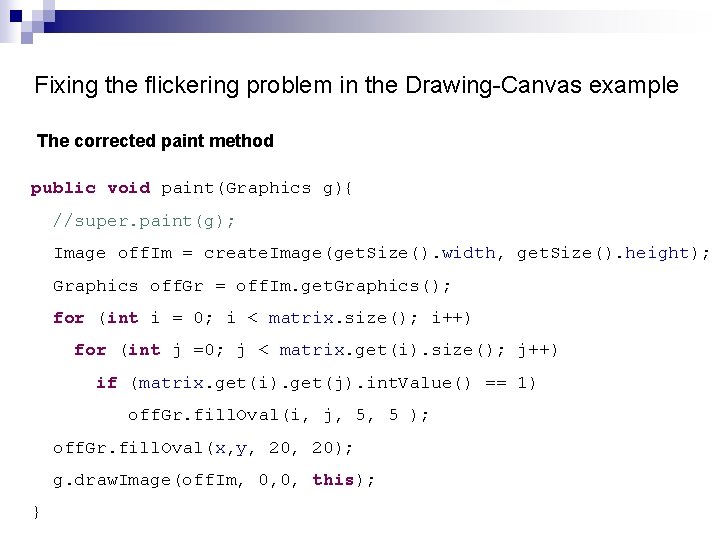
Fixing the flickering problem in the Drawing-Canvas example The corrected paint method public void paint(Graphics g){ //super. paint(g); Image off. Im = create. Image(get. Size(). width, get. Size(). height); Graphics off. Gr = off. Im. get. Graphics(); for (int i = 0; i < matrix. size(); i++) for (int j =0; j < matrix. get(i). size(); j++) if (matrix. get(i). get(j). int. Value() == 1) off. Gr. fill. Oval(i, j, 5, 5 ); off. Gr. fill. Oval(x, y, 20); g. draw. Image(off. Im, 0, 0, this); }
Antigentest åre
Practical session meaning
Multipicand
Practical session definition
Practical session meaning
Traditional vs computer animation
There are many different kinds of sports
There are four kinds of sentence
Stanzas in a poem
There are three kinds of lies
Kinds of letter
There are many different kinds of sports
How many kinds of energy are there