Polymorphism Virtual function CSI 3125 Preliminaries page 1
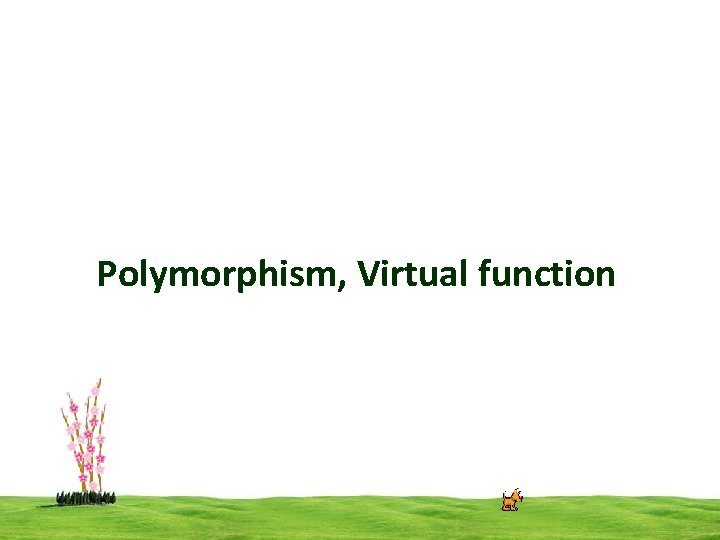
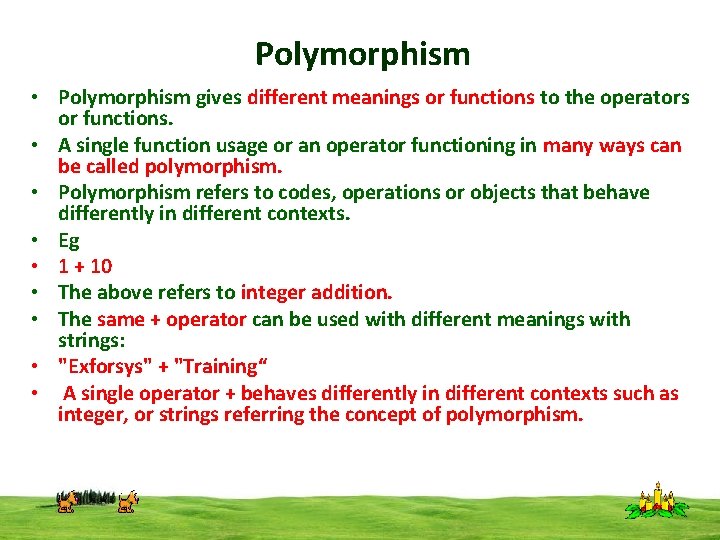
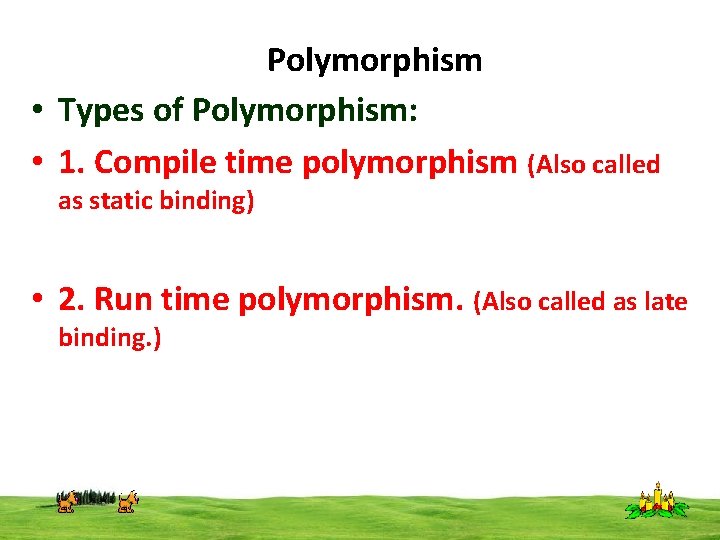
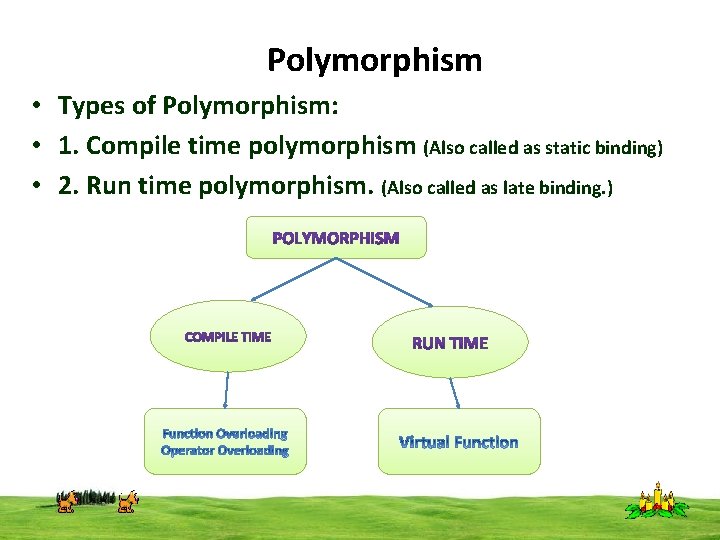
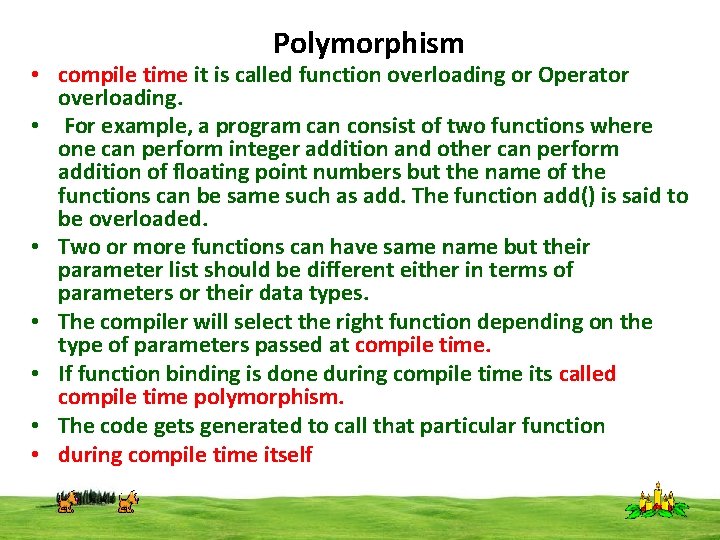
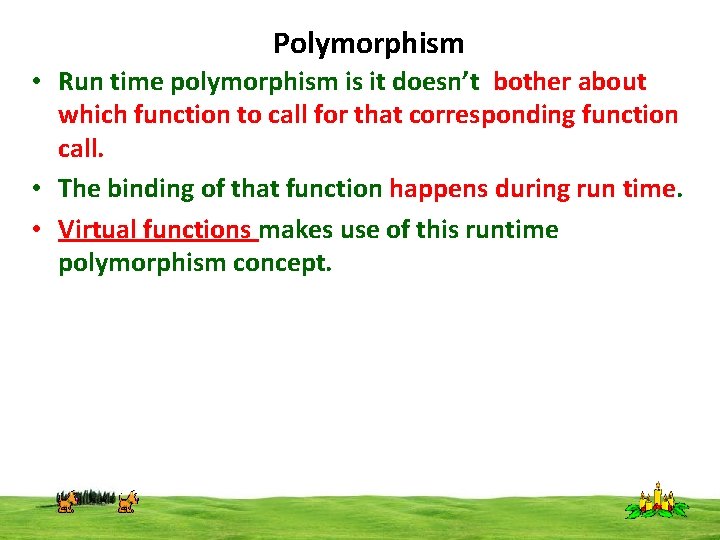
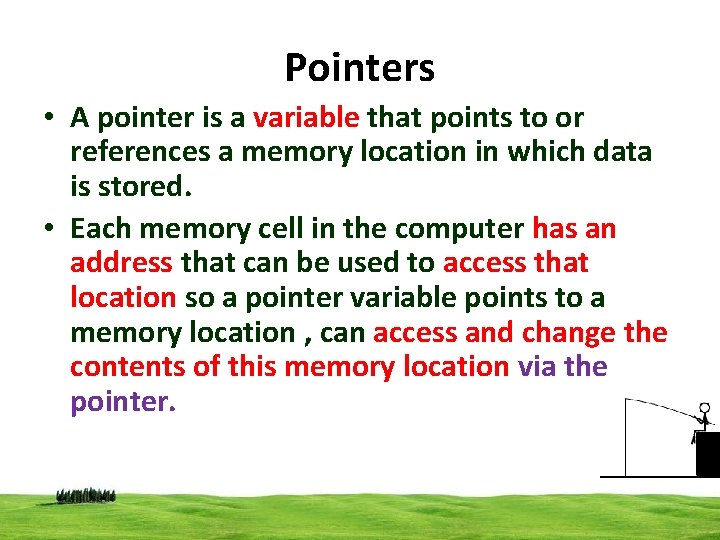
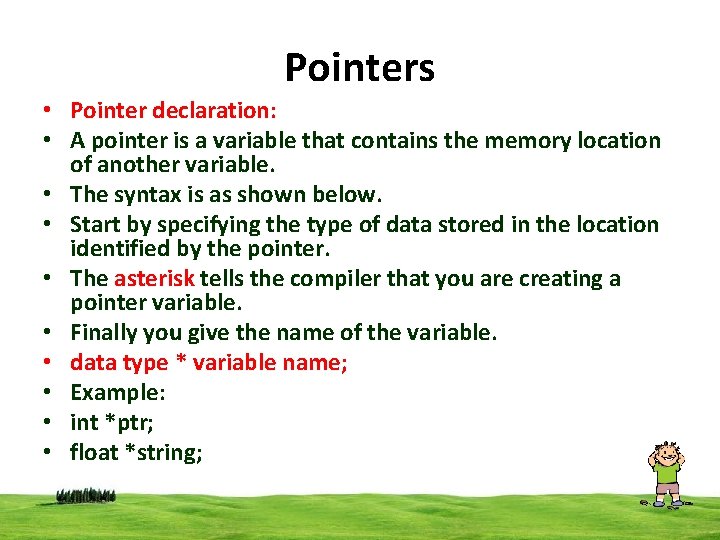
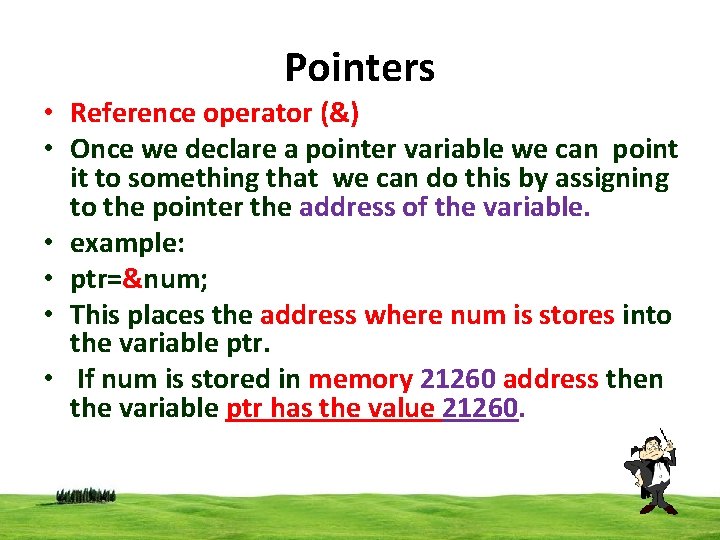
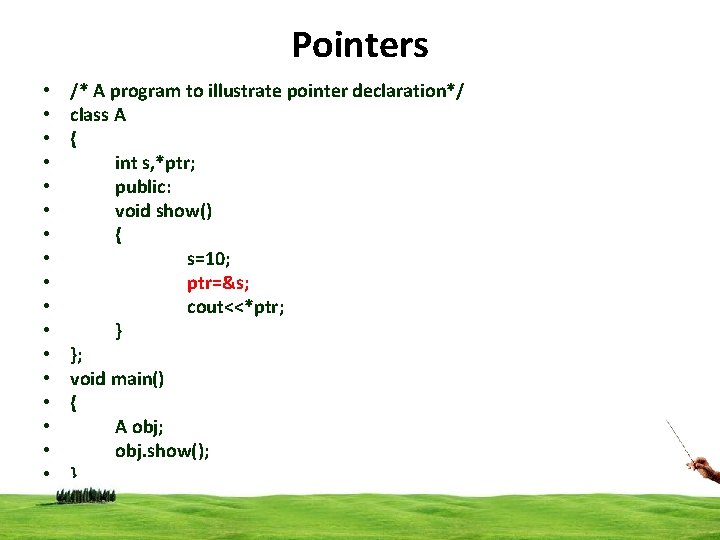
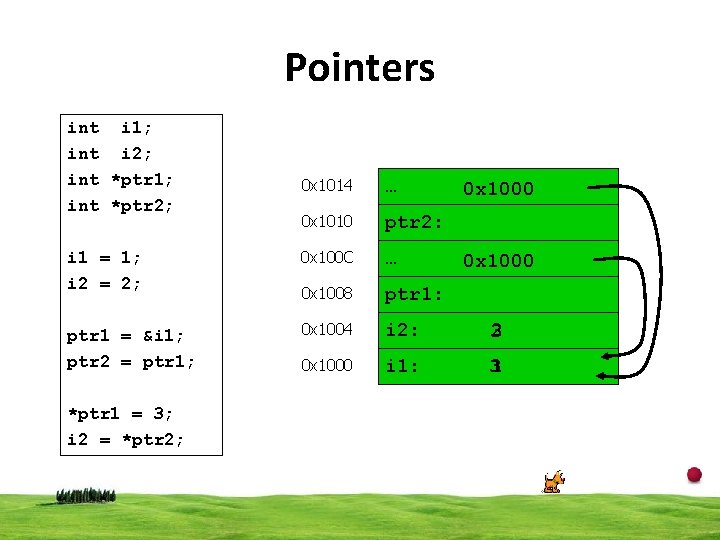
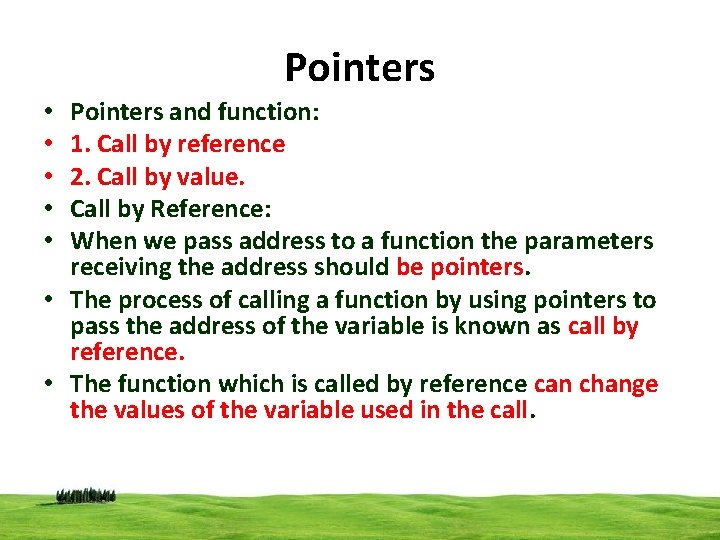
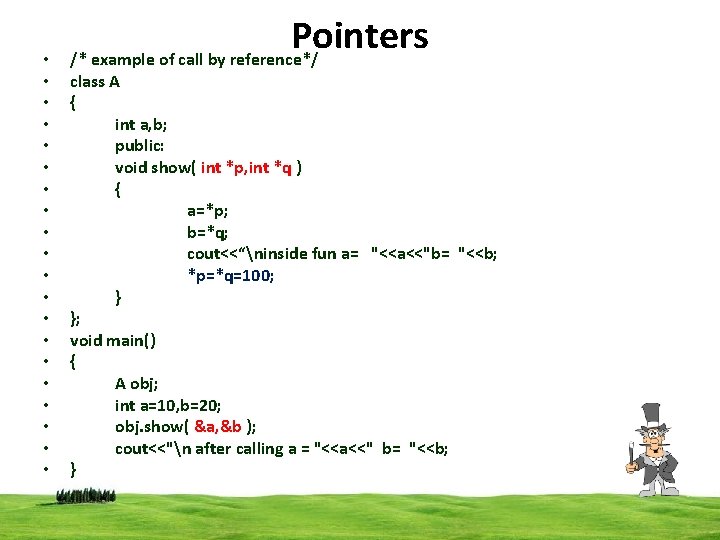
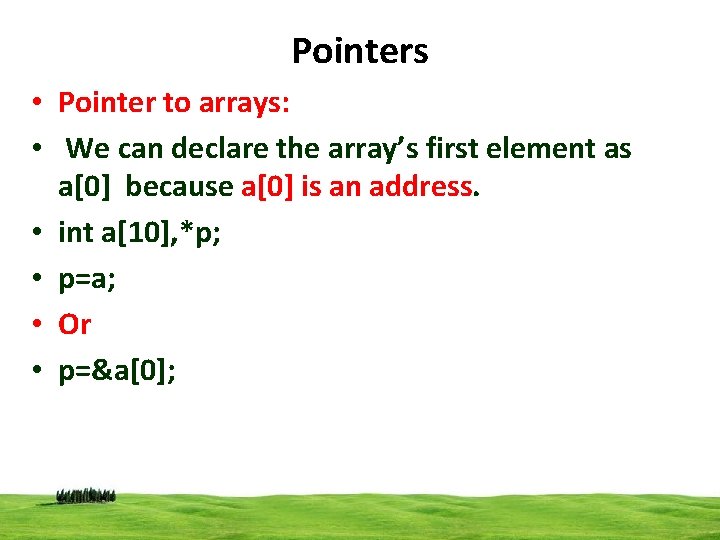
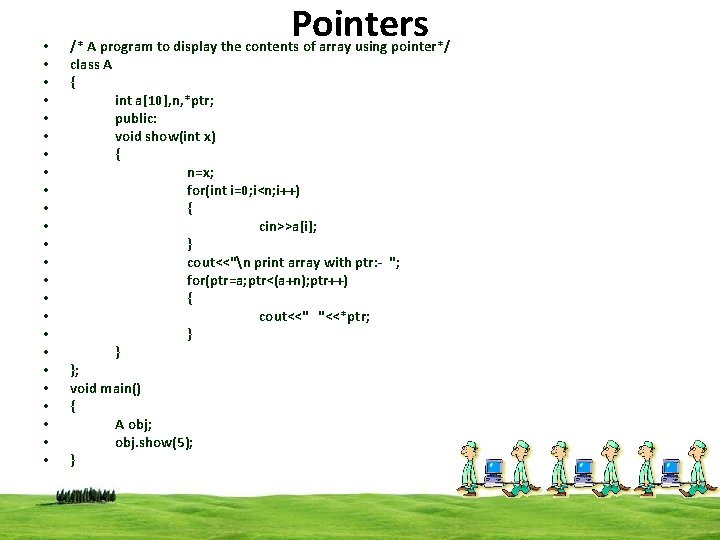
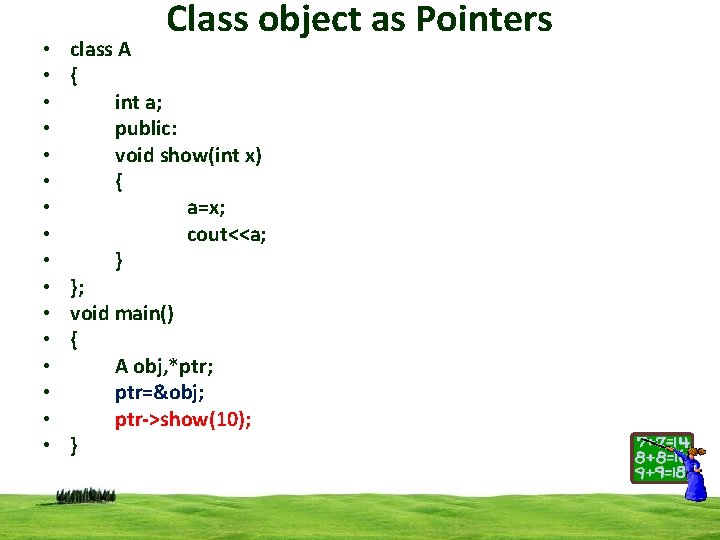
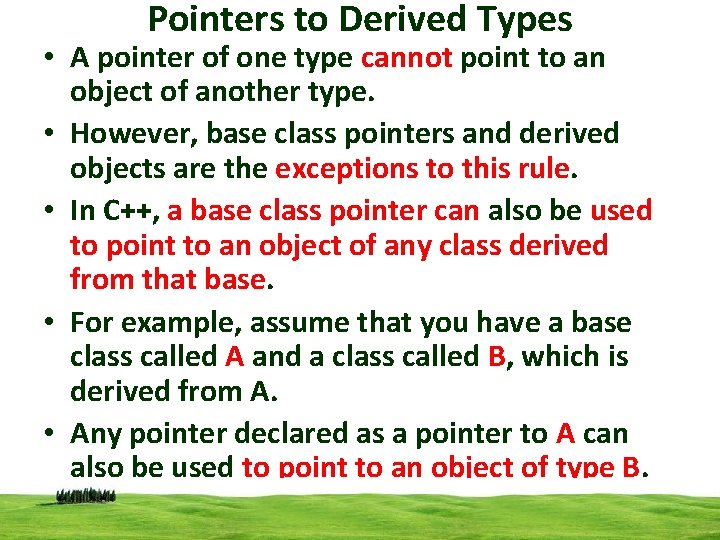
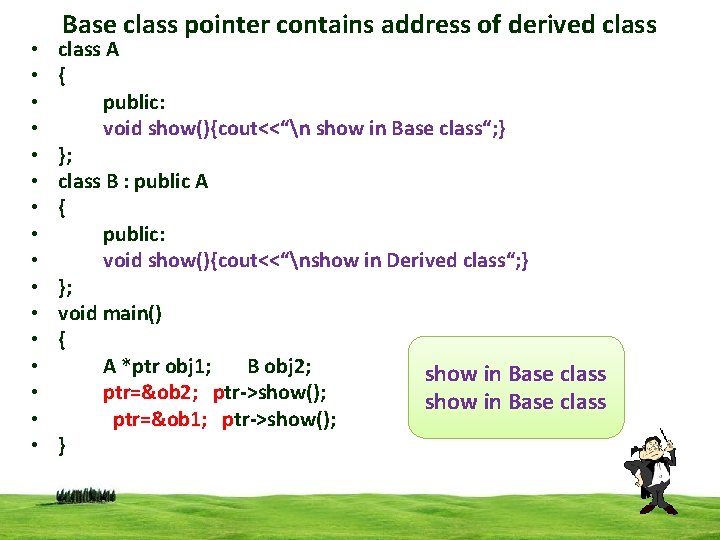
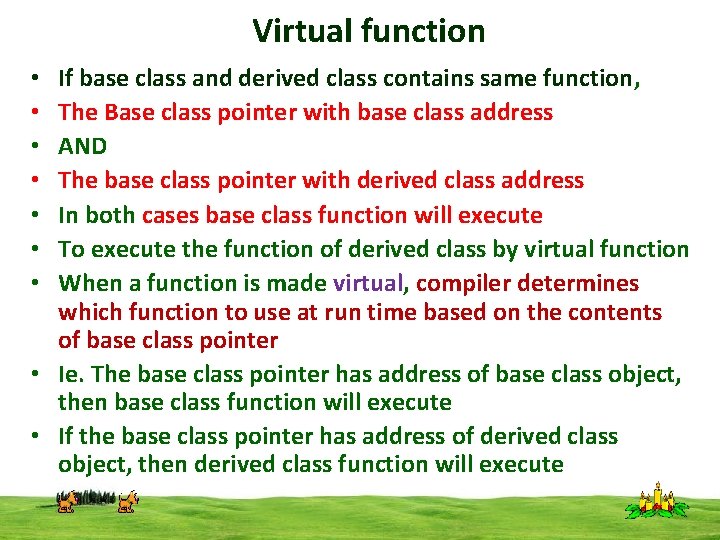
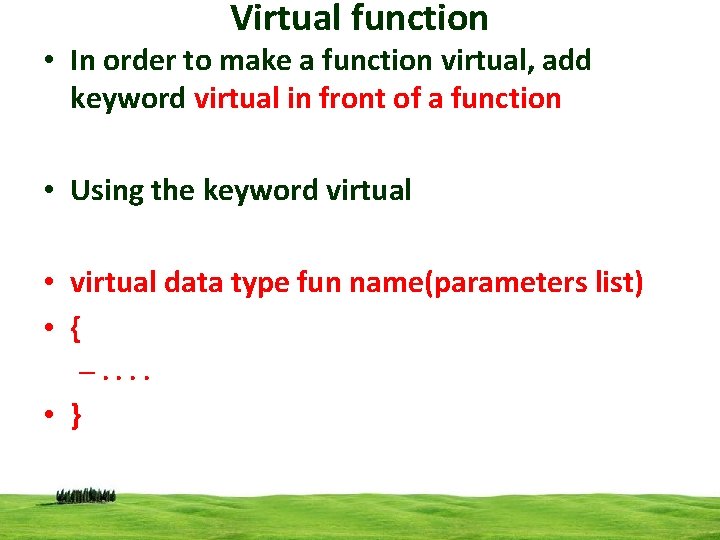
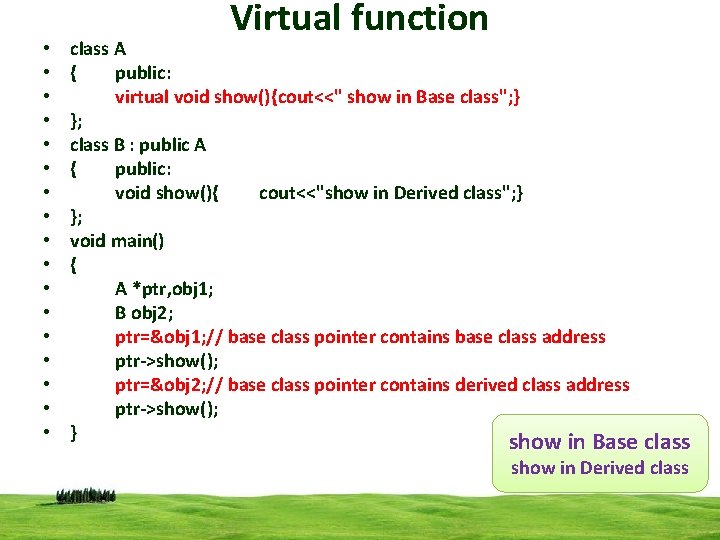
- Slides: 21
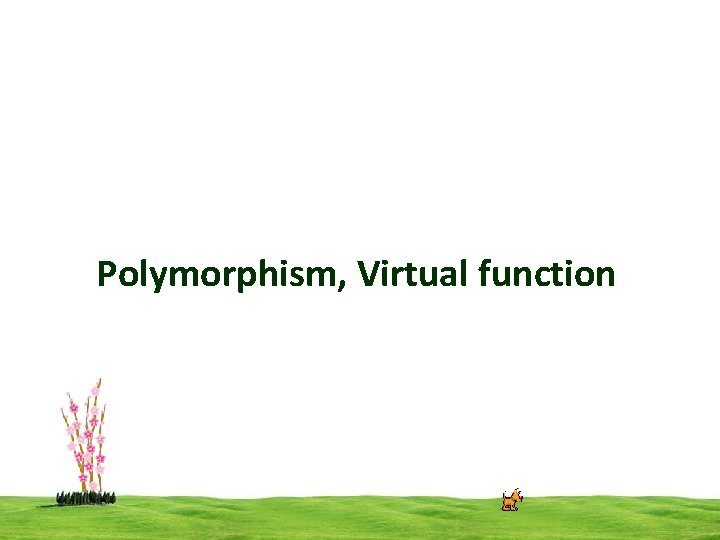
Polymorphism, Virtual function CSI 3125, Preliminaries, page 1
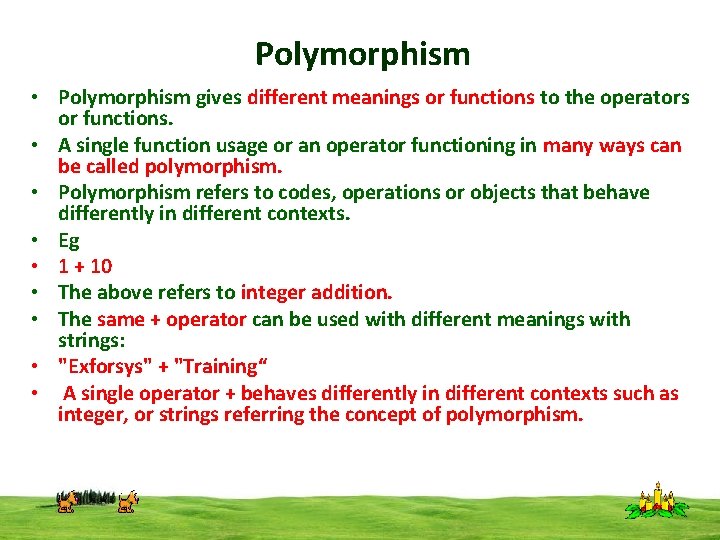
Polymorphism • Polymorphism gives different meanings or functions to the operators or functions. • A single function usage or an operator functioning in many ways can be called polymorphism. • Polymorphism refers to codes, operations or objects that behave differently in different contexts. • Eg • 1 + 10 • The above refers to integer addition. • The same + operator can be used with different meanings with strings: • "Exforsys" + "Training“ • A single operator + behaves differently in different contexts such as integer, or strings referring the concept of polymorphism. CSI 3125, Preliminaries, page 2
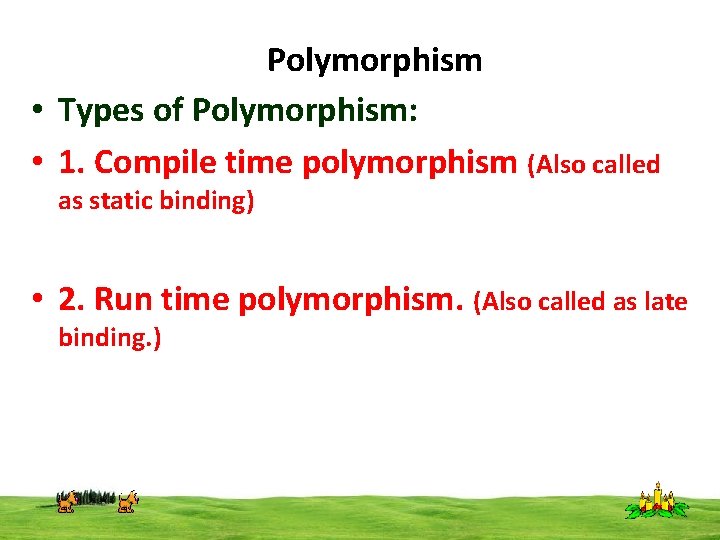
Polymorphism • Types of Polymorphism: • 1. Compile time polymorphism (Also called as static binding) • 2. Run time polymorphism. (Also called as late binding. ) CSI 3125, Preliminaries, page 3
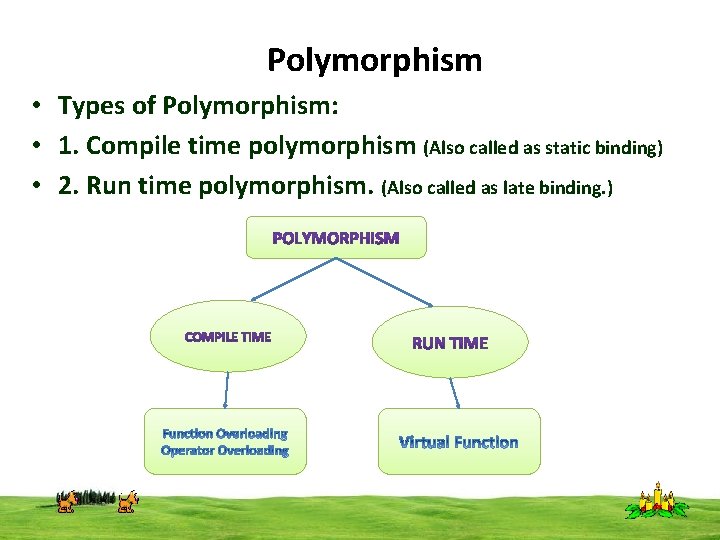
Polymorphism • Types of Polymorphism: • 1. Compile time polymorphism (Also called as static binding) • 2. Run time polymorphism. (Also called as late binding. ) CSI 3125, Preliminaries, page 4
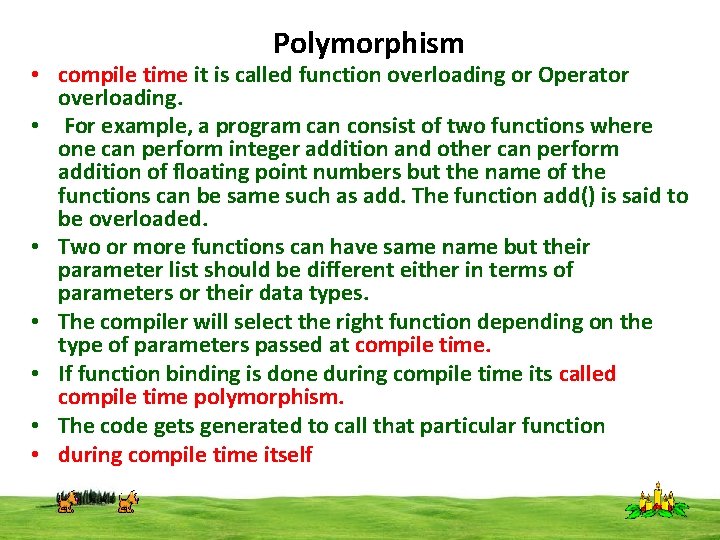
Polymorphism • compile time it is called function overloading or Operator overloading. • For example, a program can consist of two functions where one can perform integer addition and other can perform addition of floating point numbers but the name of the functions can be same such as add. The function add() is said to be overloaded. • Two or more functions can have same name but their parameter list should be different either in terms of parameters or their data types. • The compiler will select the right function depending on the type of parameters passed at compile time. • If function binding is done during compile time its called compile time polymorphism. • The code gets generated to call that particular function • during compile time itself CSI 3125, Preliminaries, page 5
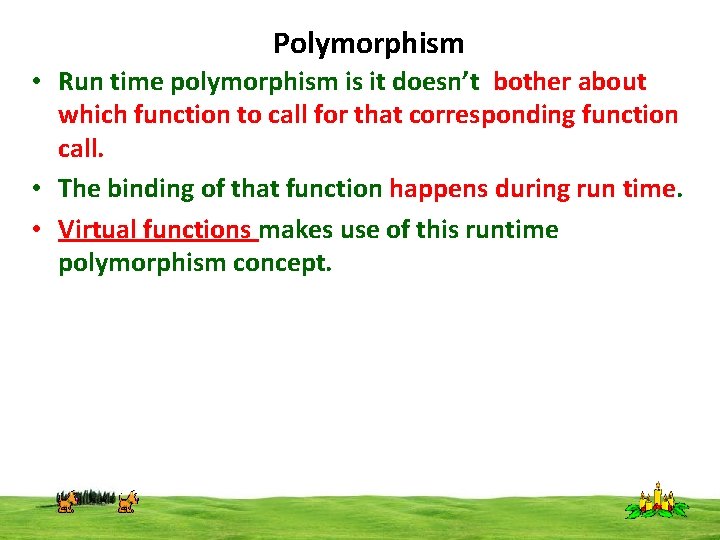
Polymorphism • Run time polymorphism is it doesn’t bother about which function to call for that corresponding function call. • The binding of that function happens during run time. • Virtual functions makes use of this runtime polymorphism concept. CSI 3125, Preliminaries, page 6
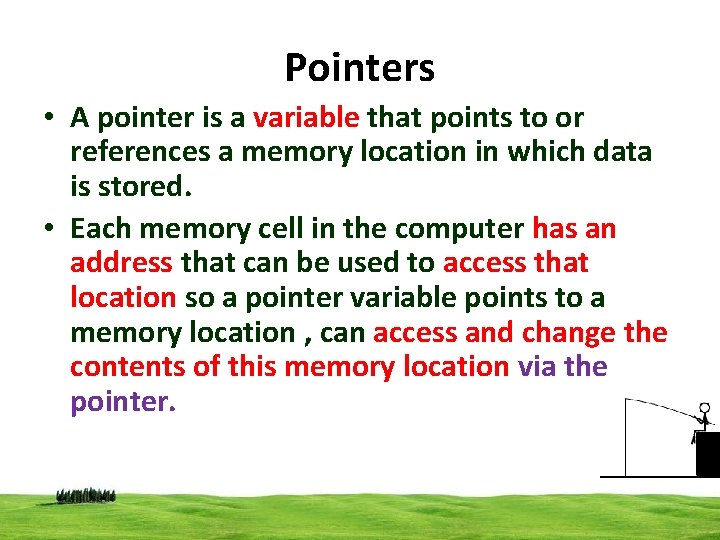
Pointers • A pointer is a variable that points to or references a memory location in which data is stored. • Each memory cell in the computer has an address that can be used to access that location so a pointer variable points to a memory location , can access and change the contents of this memory location via the pointer. 7
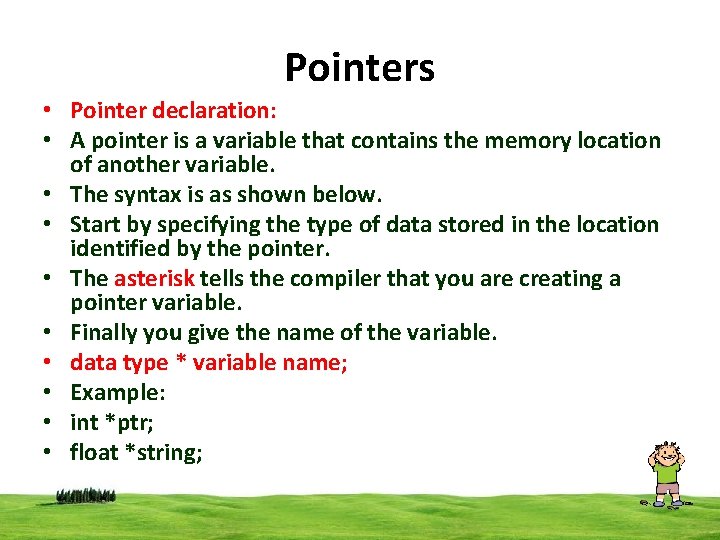
Pointers • Pointer declaration: • A pointer is a variable that contains the memory location of another variable. • The syntax is as shown below. • Start by specifying the type of data stored in the location identified by the pointer. • The asterisk tells the compiler that you are creating a pointer variable. • Finally you give the name of the variable. • data type * variable name; • Example: • int *ptr; • float *string; 8
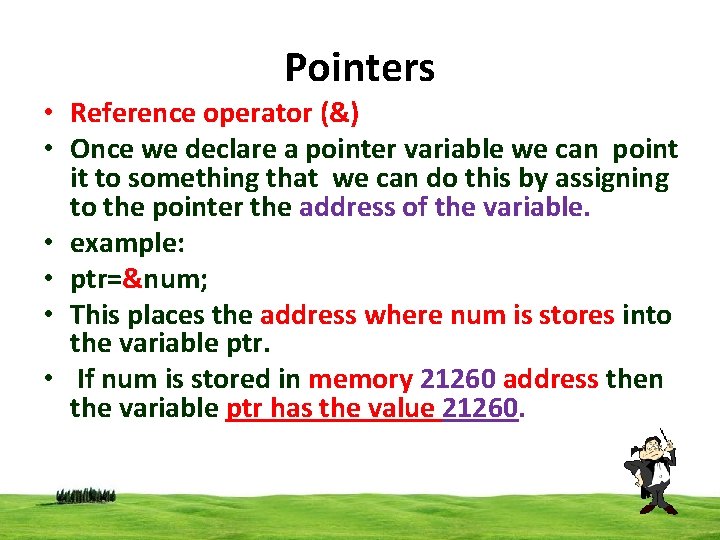
Pointers • Reference operator (&) • Once we declare a pointer variable we can point it to something that we can do this by assigning to the pointer the address of the variable. • example: • ptr=# • This places the address where num is stores into the variable ptr. • If num is stored in memory 21260 address then the variable ptr has the value 21260. 9
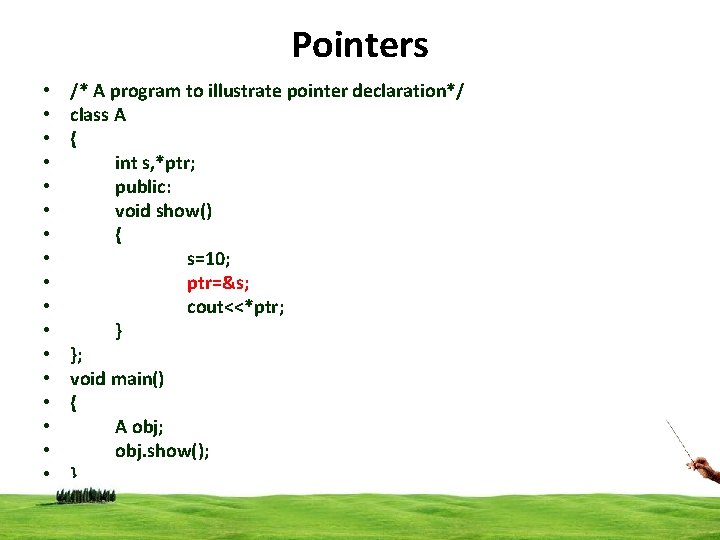
Pointers • • • • • /* A program to illustrate pointer declaration*/ class A { int s, *ptr; public: void show() { s=10; ptr=&s; cout<<*ptr; } }; void main() { A obj; obj. show(); } 10
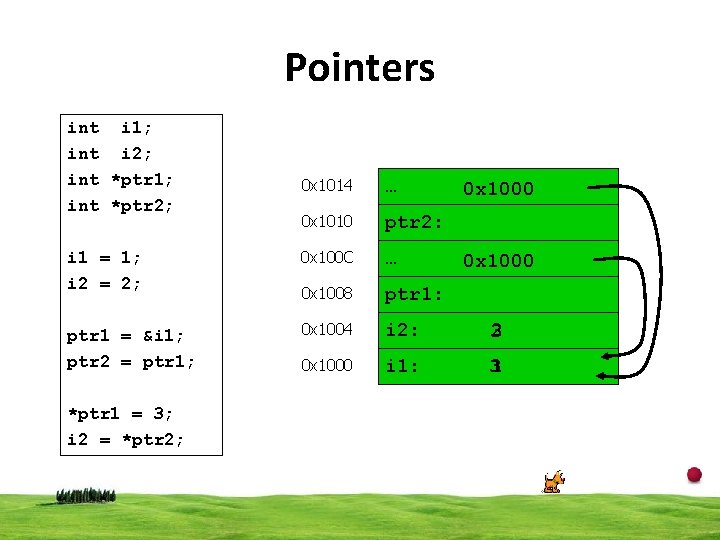
Pointers int i 1; int i 2; int *ptr 1; int *ptr 2; 0 x 1014 … 0 x 1010 ptr 2: i 1 = 1; i 2 = 2; 0 x 100 C … 0 x 1008 ptr 1: ptr 1 = &i 1; ptr 2 = ptr 1; 0 x 1004 i 2: 2 3 0 x 1000 i 1: 3 1 0 x 1000 *ptr 1 = 3; i 2 = *ptr 2; Cox & Ng Arrays and Pointers 11
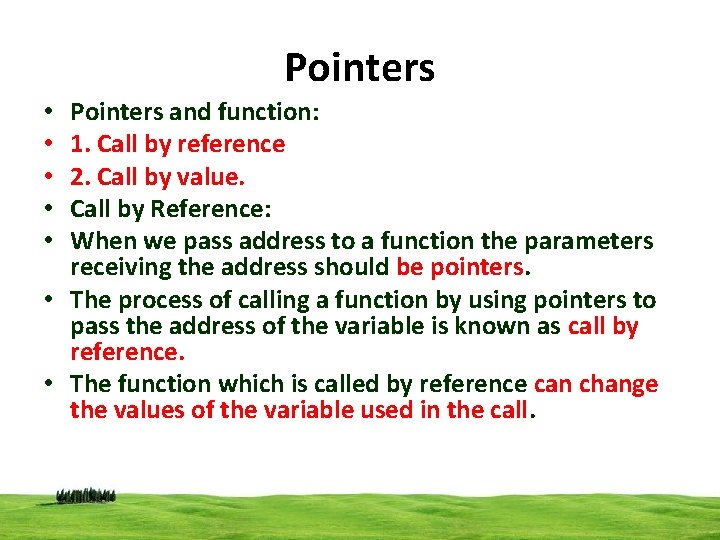
Pointers and function: 1. Call by reference 2. Call by value. Call by Reference: When we pass address to a function the parameters receiving the address should be pointers. • The process of calling a function by using pointers to pass the address of the variable is known as call by reference. • The function which is called by reference can change the values of the variable used in the call. • • • 12
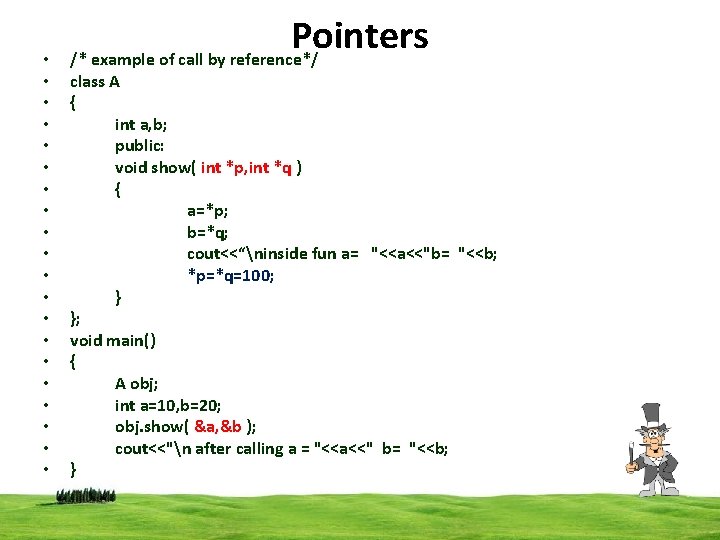
• • • • • Pointers /* example of call by reference*/ class A { int a, b; public: void show( int *p, int *q ) { a=*p; b=*q; cout<<“ninside fun a= "<<a<<"b= "<<b; *p=*q=100; } }; void main() { A obj; int a=10, b=20; obj. show( &a, &b ); cout<<"n after calling a = "<<a<<" b= "<<b; } 13
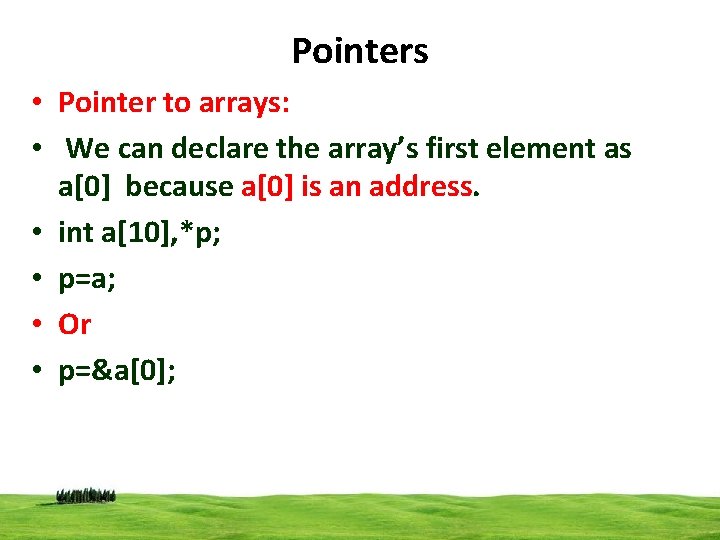
Pointers • Pointer to arrays: • We can declare the array’s first element as a[0] because a[0] is an address. • int a[10], *p; • p=a; • Or • p=&a[0]; 14
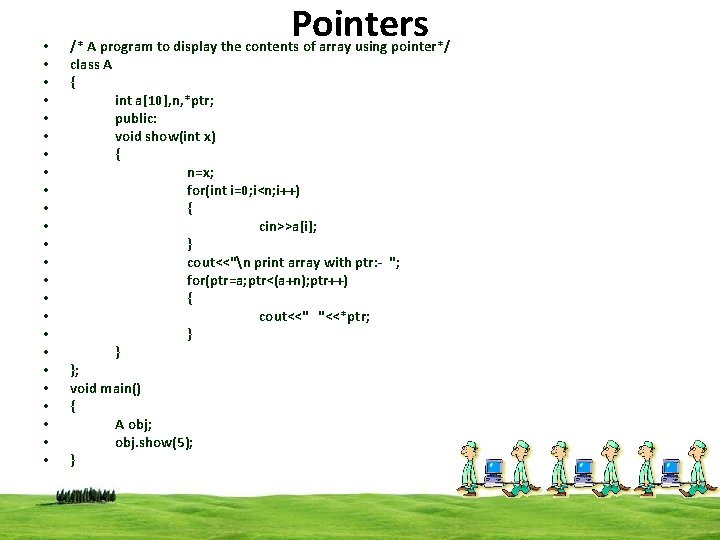
• • • • • • Pointers /* A program to display the contents of array using pointer*/ class A { int a[10], n, *ptr; public: void show(int x) { n=x; for(int i=0; i<n; i++) { cin>>a[i]; } cout<<"n print array with ptr: - "; for(ptr=a; ptr<(a+n); ptr++) { cout<<" "<<*ptr; } } }; void main() { A obj; obj. show(5); } 15
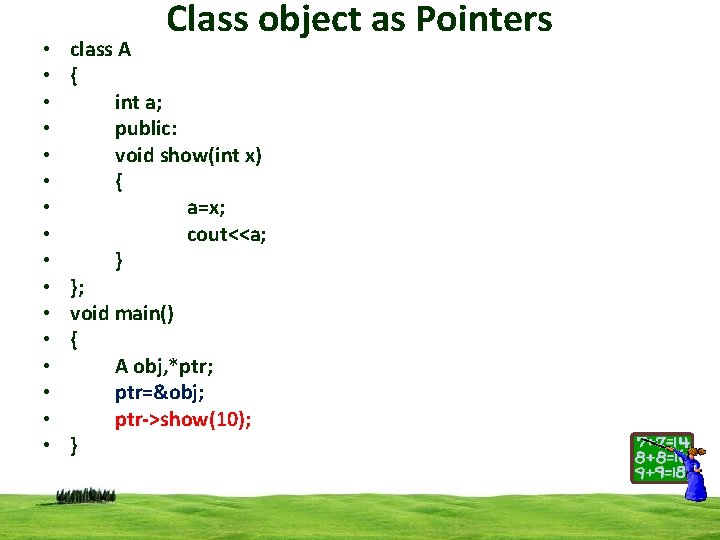
• • • • Class object as Pointers class A { int a; public: void show(int x) { a=x; cout<<a; } }; void main() { A obj, *ptr; ptr=&obj; ptr->show(10); } 16
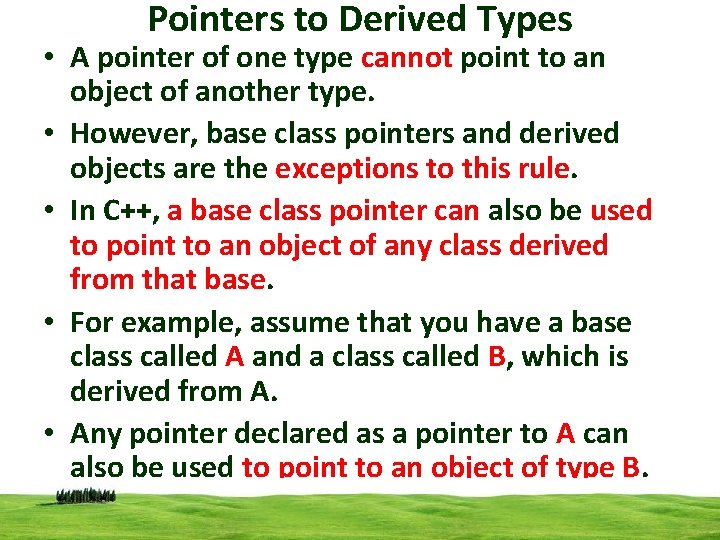
Pointers to Derived Types • A pointer of one type cannot point to an object of another type. • However, base class pointers and derived objects are the exceptions to this rule. • In C++, a base class pointer can also be used to point to an object of any class derived from that base. • For example, assume that you have a base class called A and a class called B, which is derived from A. • Any pointer declared as a pointer to A can also be used to point to an object of type B. 17
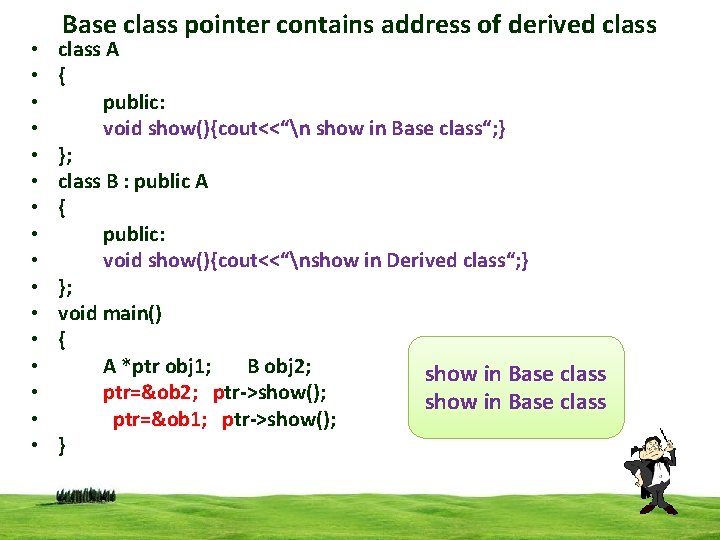
• • • • Base class pointer contains address of derived class A { public: void show(){cout<<“n show in Base class“; } }; class B : public A { public: void show(){cout<<“nshow in Derived class“; } }; void main() { A *ptr obj 1; B obj 2; show in Base class ptr=&ob 2; ptr->show(); show in Base class ptr=&ob 1; ptr->show(); } 18
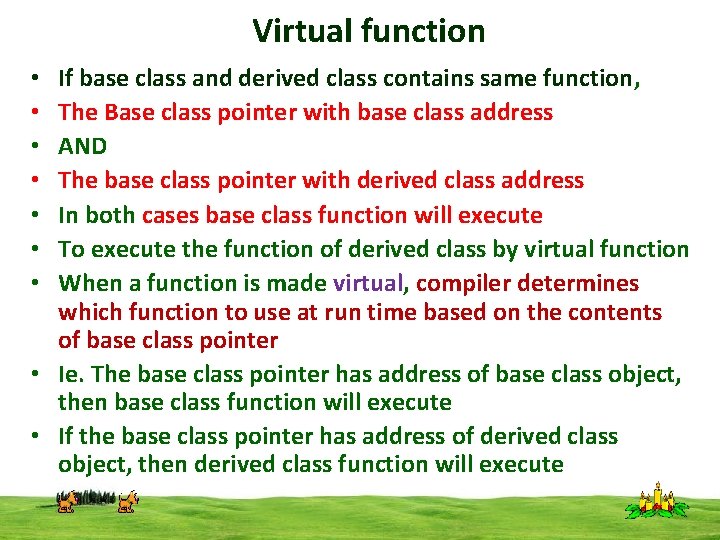
Virtual function If base class and derived class contains same function, The Base class pointer with base class address AND The base class pointer with derived class address In both cases base class function will execute To execute the function of derived class by virtual function When a function is made virtual, compiler determines which function to use at run time based on the contents of base class pointer • Ie. The base class pointer has address of base class object, then base class function will execute • If the base class pointer has address of derived class object, then derived class function will execute • • CSI 3125, Preliminaries, page 19
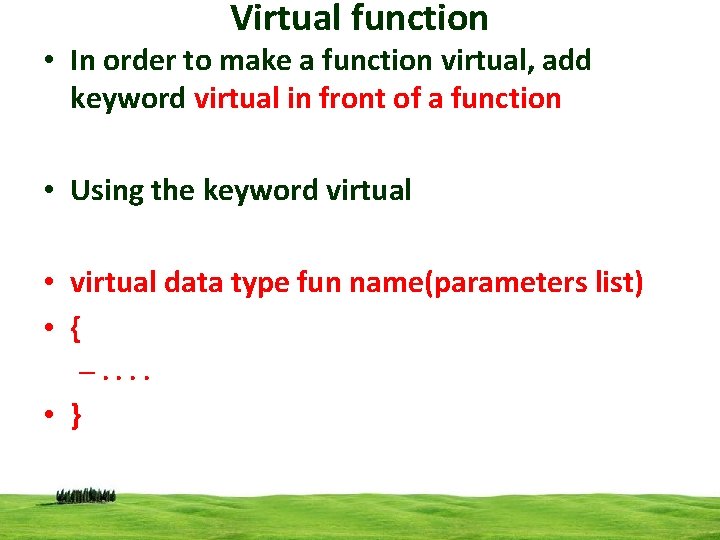
Virtual function • In order to make a function virtual, add keyword virtual in front of a function • Using the keyword virtual • virtual data type fun name(parameters list) • { –. . • } 20
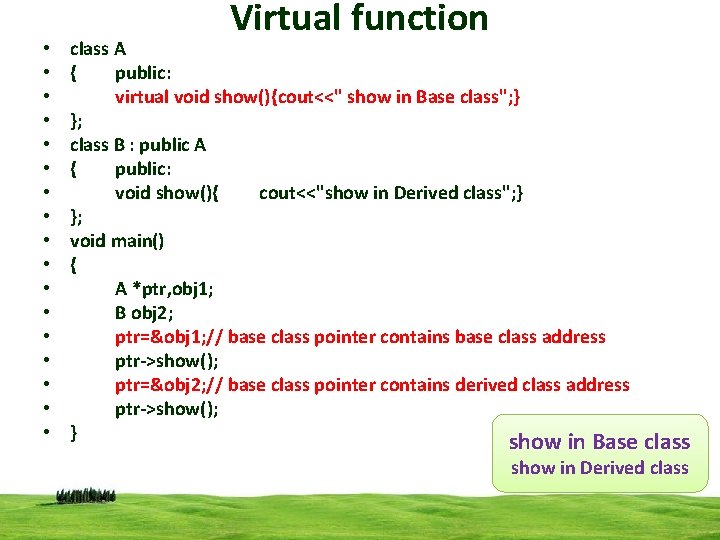
• • • • • Virtual function class A { public: virtual void show(){cout<<" show in Base class"; } }; class B : public A { public: void show(){ cout<<"show in Derived class"; } }; void main() { A *ptr, obj 1; B obj 2; ptr=&obj 1; // base class pointer contains base class address ptr->show(); ptr=&obj 2; // base class pointer contains derived class address ptr->show(); } show in Base class show in Derived class 21
Gs3125
Apa itu preliminaries
Preliminary material
Halaman preliminaries
Mathematical preliminaries in numerical computing
Chapter 1 mathematical preliminaries
Foundation synoynm
Running head apa format
Has virtual functions and accessible non-virtual destructor
Csi 3120
Csi3120
Csi 321
Csi in project management
Two people can have identical handwriting.
Csi varese
Csi framework
Csi-2 to usb
Unicode space 3140
Csi 3140
Csi 3140
Csi 3140
Csi 3131